#DebuggingTechniques
Explore tagged Tumblr posts
Text
Debugging Tips and Tricks for C# Developers
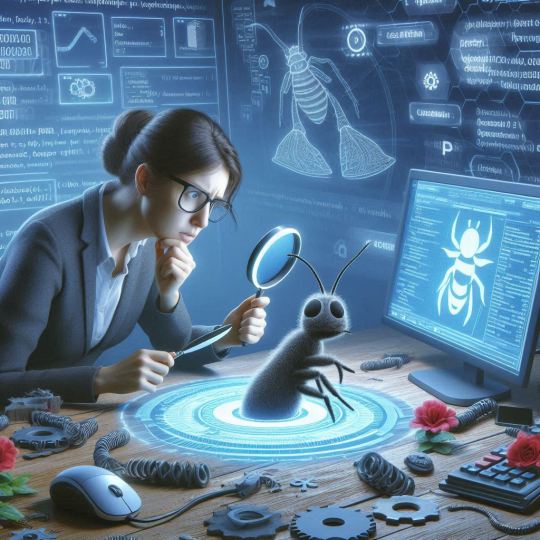
Debugging is an essential skill for any C# developer. Efficient debugging can save you countless hours of frustration and lead to quicker, more reliable code. Whether you're a beginner or an experienced developer, the following tips and tricks will enhance your debugging prowess in C#.
1. Utilize Breakpoints Effectively
Breakpoints are one of the most fundamental debugging tools. Here are some advanced ways to use them:
Conditional Breakpoints: Set breakpoints that trigger only when certain conditions are met. Right-click on a breakpoint and select "Conditions" to specify your criteria.
Hit Counts: Breakpoints can be set to activate only after being hit a specific number of times. This is useful for isolating issues in loops.
Actions: Add actions to breakpoints to log messages or run macros without interrupting execution.
2. Use the Immediate Window
The Immediate Window is a powerful tool for evaluating expressions, executing statements, and printing variable values during debugging sessions. To use it effectively:
Evaluate Expressions: Type any valid C# expression to see its value immediately.
Modify Variables: Change the value of variables on the fly to test different scenarios.
Call Methods: Invoke methods directly to test their behavior without altering your code.
3. Master Watch Windows
Watch windows allow you to monitor variables and expressions. There are several types of watch windows:
Watch Window: Add variables and expressions manually to keep an eye on their values.
Autos Window: Automatically displays variables used around the current statement.
Locals Window: Shows all local variables in the current scope.
4. Take Advantage of Data Tips
Hovering over variables provides a quick glimpse of their current value. For more detailed information:
Pin Data Tips: Pin data tips to keep them visible even when you move the cursor away.
Edit Data Tips: Modify the value of variables directly within the data tip.
5. Leverage Debugging Attributes
Certain attributes can be added to your code to facilitate debugging:
[DebuggerDisplay]: Customize how your objects appear in the debugger. For example:csharpCopy code[DebuggerDisplay("Name = {Name}, Age = {Age}")] public class Person { ... }
[DebuggerStepThrough]: Prevents the debugger from stepping into specific methods, keeping the focus on more relevant code.
6. Use Exception Settings
By default, the debugger breaks only on unhandled exceptions. You can configure it to break on all exceptions:
Exception Settings Window: Go to Debug > Windows > Exception Settings. Here, you can specify which exceptions should break execution.
7. Analyze Call Stacks
The Call Stack window is invaluable for understanding the sequence of method calls leading to a particular point:
Navigate Frames: Double-click on any frame to navigate to the corresponding code.
View Parameters and Locals: Right-click on a frame and select "View Parameters and Locals" to inspect the state at each level.
8. Trace with Debug and Trace Classes
Use the System.Diagnostics.Debug and System.Diagnostics.Trace classes to insert diagnostic messages into your code:
Debug.WriteLine: Writes information only when running in debug mode.
Trace.WriteLine: Writes information regardless of build configuration.
9. Enable Just-In-Time (JIT) Debugging
JIT debugging allows you to attach a debugger to your application if it crashes outside of the Visual Studio environment:
Configuration: Ensure that JIT debugging is enabled in your Visual Studio settings (Tools > Options > Debugging > Just-In-Time).
10. Remote Debugging
Debug applications running on remote machines by using Visual Studio's remote debugging tools:
Setup: Install the Remote Tools for Visual Studio on the target machine.
Attach to Process: From your development machine, use Debug > Attach to Process to connect to the remote process.
Conclusion
Mastering these debugging techniques will significantly improve your efficiency and effectiveness as a C# developer. By leveraging breakpoints, watch windows, data tips, and other tools, you can swiftly diagnose and resolve issues, leading to cleaner, more reliable code. Happy debugging!
#CSharp#Debugging#CodingTips#SoftwareDevelopment#Programming#DeveloperTips#VisualStudio#CSharpDeveloper#CodeDebugging#DotNet#TechTips#ProgrammingSkills#SoftwareEngineering#CodeQuality#DebuggingTechniques#ahextechnologies
0 notes