#commonjs
Explore tagged Tumblr posts
Text
importとrequireの対比表がわかりやすくて良いです。
2 notes
·
View notes
Text
You can learn NodeJS easily, Here's all you need:
1.Introduction to Node.js
• JavaScript Runtime for Server-Side Development
• Non-Blocking I/0
2.Setting Up Node.js
• Installing Node.js and NPM
• Package.json Configuration
• Node Version Manager (NVM)
3.Node.js Modules
• CommonJS Modules (require, module.exports)
• ES6 Modules (import, export)
• Built-in Modules (e.g., fs, http, events)
4.Core Concepts
• Event Loop
• Callbacks and Asynchronous Programming
• Streams and Buffers
5.Core Modules
• fs (File Svstem)
• http and https (HTTP Modules)
• events (Event Emitter)
• util (Utilities)
• os (Operating System)
• path (Path Module)
6.NPM (Node Package Manager)
• Installing Packages
• Creating and Managing package.json
• Semantic Versioning
• NPM Scripts
7.Asynchronous Programming in Node.js
• Callbacks
• Promises
• Async/Await
• Error-First Callbacks
8.Express.js Framework
• Routing
• Middleware
• Templating Engines (Pug, EJS)
• RESTful APIs
• Error Handling Middleware
9.Working with Databases
• Connecting to Databases (MongoDB, MySQL)
• Mongoose (for MongoDB)
• Sequelize (for MySQL)
• Database Migrations and Seeders
10.Authentication and Authorization
• JSON Web Tokens (JWT)
• Passport.js Middleware
• OAuth and OAuth2
11.Security
• Helmet.js (Security Middleware)
• Input Validation and Sanitization
• Secure Headers
• Cross-Origin Resource Sharing (CORS)
12.Testing and Debugging
• Unit Testing (Mocha, Chai)
• Debugging Tools (Node Inspector)
• Load Testing (Artillery, Apache Bench)
13.API Documentation
• Swagger
• API Blueprint
• Postman Documentation
14.Real-Time Applications
• WebSockets (Socket.io)
• Server-Sent Events (SSE)
• WebRTC for Video Calls
15.Performance Optimization
• Caching Strategies (in-memory, Redis)
• Load Balancing (Nginx, HAProxy)
• Profiling and Optimization Tools (Node Clinic, New Relic)
16.Deployment and Hosting
• Deploying Node.js Apps (PM2, Forever)
• Hosting Platforms (AWS, Heroku, DigitalOcean)
• Continuous Integration and Deployment-(Jenkins, Travis CI)
17.RESTful API Design
• Best Practices
• API Versioning
• HATEOAS (Hypermedia as the Engine-of Application State)
18.Middleware and Custom Modules
• Creating Custom Middleware
• Organizing Code into Modules
• Publish and Use Private NPM Packages
19.Logging
• Winston Logger
• Morgan Middleware
• Log Rotation Strategies
20.Streaming and Buffers
• Readable and Writable Streams
• Buffers
• Transform Streams
21.Error Handling and Monitoring
• Sentry and Error Tracking
• Health Checks and Monitoring Endpoints
22.Microservices Architecture
• Principles of Microservices
• Communication Patterns (REST, gRPC)
• Service Discovery and Load Balancing in Microservices
1 note
·
View note
Text
Why is it so damn difficult to set up a modern #TypeScript project that uses ESM instead of CommonJS and doesn't constantly throw errors about not being able to find things, or relative paths, or file extensions? 🤬🤬🤬
0 notes
Text
Difference between ES5 and ES6
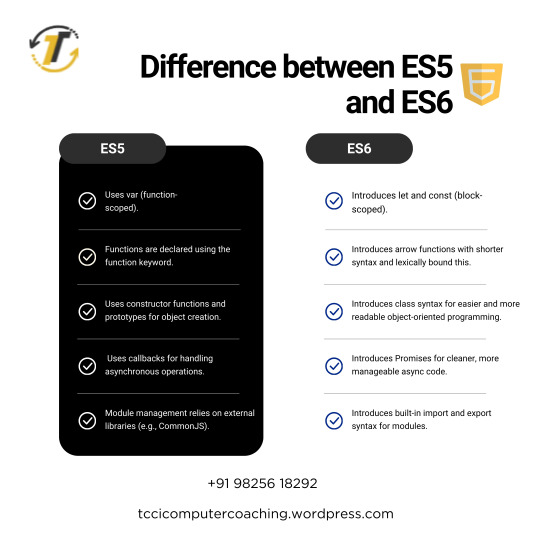
JavaScript is the must need programming language for both web and software engineering, and it keeps on changing with time. All developers should know the difference between ES5 and ES6 because major variations and advancements were made in those versions. ES5 started the foundation and had shown more advanced features in the version of ECMAScript 2015, also known as ES6. At TCCI Computer Coaching Institute, we ensure that each student understands these updates deeply so that they can be ahead in their programming journey.
What is ES5?
ES5, which stands for ECMAScript 5, was launched in 2009. This is the version of JavaScript which was seen to be stable, and it filled some of the most important holes that were there in the earlier versions. Key features of ES5 include:
Strict Mode: Strict mode allows developers to write secure and optimized code because it throws an error for all actions that are allowed in other versions.
JSON Support: ES5 provided support to work with JSON (JavaScript Object Notation), which is an essential feature for data interchange.
Array Methods: It introduced several new array methods, such as forEach(), map(), filter(), and reduce(), that simplify operations on arrays.
Getter/Setter Methods: ES5 allows the use of getter and setter methods, making it possible to control object properties.
What is ES6?
ES6, also commonly referred to as ECMAScript 2015, was a substantial update to the JavaScript language to include many new features as well as additions that make this language more powerful, concise, and much more modern in its expression. Some of its key features include:
Let and Const: There are let and const, added to declare a variable, with let offering better scoping than var, and const having no redefinition.
Arrow Functions: More concise syntax - also preserves this context.
Template Literals: Template literals ease string interpolation and multiline strings, and are much readable and less error-prone.
Classes: Classes, which are new in ES6, make the syntax for creating objects and handling inheritance much simpler than it used to be. It makes JavaScript resemble other more conventional object-oriented programming languages.
Promises: The promise is how asynchronous code works; promises handle success and failure states much easier.
Destructuring: This helps extract values from arrays and objects into separate variables in a simple syntax.
Modules: ES6 provided modules, where JavaScript code could be broken into reusable, maintainable pieces.
Key differences between ES5 and ES6
Variable declarations:
ES5 : Variables are declared using var.
ES6 : let and const keywords were introduced which provide block scoping and better predictability
Functions:
ES5: The function keyword is used to declare functions, and the this context can be a little tricky in some cases.
ES6: Arrow functions offer a more compact syntax and lexically bind the this keyword, avoiding common problems with this.
Object-Oriented Programming:
ES5: JavaScript uses prototype-based inheritance.
ES6: Introduces the class syntax, making object-oriented programming much more intuitive and easier to use.
Asynchronous Programming:
ES5: Callbacks are typically used for handling asynchronous code.
ES6: It introduced promises that made asynchronous operation cleaner.
Modules:
ES5 JavaScript does not natively support modules. Developers will use libraries such as CommonJS or RequireJS.
ES6 Native module support using the keywords import and export, which facilitates code organization and reusability
Template Literals:
ES5 String concatenation is done by using + operators, which can sometimes be error-prone and messy to read.
ES6 Template literals enable multi-line strings and interpolation of strings, enhancing readability.
Why Is It Important to Learn ES6?
At TCCI Computer Coaching Institute, we understand that staying updated with the latest advancements in JavaScript is crucial for your programming career. Learning ES6 is not just about getting familiar with new syntax, but also about adopting best practices that improve the quality and maintainability of your code.
As the JavaScript community shifts towards ES6 as the norm, most of the modern libraries and frameworks are built using the features of ES6. So, mastering ES6 will help you work with the latest technologies like React, Angular, and Node.js. The difference between ES5 and ES6 will also make you a more efficient developer in writing clean, efficient, and scalable code regardless of whether you are a beginner or a seasoned developer.
Learn ES6 with TCCI Computer Coaching Institute
We provide in-depth JavaScript training classes at TCCI Computer Coaching Institute that covers everything, starting from the basic knowledge of ES5 to the latest features added to ES6 and beyond. We ensure you have hands-on practice by giving practical exercise and real-world projects, along with individual guidance.
Learn at your pace with our online and offline classes.
Expert Trainers: Our trainers are industry experts with years of experience in teaching JavaScript and web development.
Real-World Applications: We focus on real-world programming challenges, ensuring that you are well-prepared for the job market.
It is one of the important steps in becoming a good JavaScript developer, as one should master the differences between ES5 and ES6. We provide the right environment and resources to learn and grow as a developer at TCCI Computer Coaching Institute. Join us today and start your journey toward mastering JavaScript!
Location: Ahmedabad, Gujarat
Call now on +91 9825618292
Get information from https://tccicomputercoaching.wordpress.com/
#Best Computer Training Institutes Bopal Ahmedabad#Best Javascript Course Training in Ahmedabad#Difference between ES5 and ES6#ES5 vs ES6#TCCI Computer Coaching Institute
0 notes
Text
2024年12月29日の記事一覧
(全 15 件)
トランプ次期大統領、TikTok禁止令施行延期を最高裁に要請
コーディングAI課金するならCodyが断トツ良い話
【2025最新版】M4 Mac miniをゲーミングPC運用するための情報まとめ【令和最新版】|みずき
NTT、25年6月に社名「日本電信電話」変更 国際化に弾み
続・ConsoleAppFrameworkとAWS CDKで爆速バッチ開発
『就職氷河期世代』/近藤絢子インタビュー
組織と個人|Dai Tamesue(為末大)
契約書にはなかった機能開発が“後から”必要に……契約外の作業に対する追加費用はベンダーに支払うべき?
import / exportの記法だけではない、CommonJS modulesとES modulesの違い
四国36万戸の大停電、操作ミスが起きた状況は北米で想定されていた
競争から「逃げてばかり」いる人は幸せになれない。大規模調査で判明した意外すぎる事実とは。
ElasticsearchでLIKE検索のような部分一致検索を高速に実現する方法
進化した正規表現:JavaScriptの正規表現の歴史と未来
計測と改善をひたすら繰り返したら、年間コストを1億円削減した──不確実性の高いプロジェクトに挑む
Gemini Code Assist
0 notes
Text
Customizable Accessible In-place Editing - edit-element
edit-element is a lightweight in-place editing JavaScript/TypeScript library that transforms any HTML element into an editable field directly within your webpage. It supports both CommonJS and ES Modules, includes TypeScript support, and is designed with accessibility in mind. You can set custom class names for elements being edited and define your preferred shortcut key for submitting…
0 notes
Text
How to Utilize Browserify to Streamline Frontend Development
Utilizing Browserify to Streamline Frontend Development Introduction In the world of frontend development, writing JavaScript code in a modular and scalable way can be challenging. The rise of Webpack has made bundling and minification easier, but it can still be overwhelming to manage multiple module systems like CommonJS and AMD. Browserify is a popular tool that aims to make frontend…
0 notes
Text
JavaScript Modules
Day 14 of our JavaScript & Node.js Series is live! We’re diving into JavaScript Modules—named & default exports, CommonJS vs. ES6, and bundling for better web performance. Test your knowledge with a quiz too! Check it out to boost your skills! 💻
Welcome to Day 14 of our 30-day JavaScript and Node.js learning series! In the last article, we discussed about the event loop in JavaScript . Today, we’ll dive deeper into one of the most crucial topics—JavaScript Modules. JavaScript modules have revolutionized the way we structure and organize our JavaScript code. By breaking down complex applications into smaller, reusable components, modules…
0 notes
Text
LEARN MERN STACK? HERE IS EVERYTHING YOU NEED TO KNOW : June 19, 2024
Stack of technology provides MERN stack. It is a set of technologies used to develop an application, including programming languages, frameworks, databases, front-end and back-end tools, and APIs. In the MERN Stack, the working components are MongoDB, ExpressJS, ReactJS, and NodeJS.
Learn MERN Stack
Here are the details of the roles each component fulfills when developing a web application with the MERN stack :
MongoDB:
MongoDB is a NoSQL database that stores data as a document, comprising key-value pairs similar to JSON (JavaScript Object Notation) objects. MongoDB is flexible, letting users make schemas, databases, tables, and more. In MongoDB, special credentials identified by a primary key form its basic unit. After installing MongoDB, users can utilize the Mongo shell, which provides a JavaScript interface for interacting with the database, such as querying, updating, and deleting records.
Express.JS
Express is a framework used for making the back-end of web applications with Node.js. It makes coding easier compared to using Node.js alone by providing shortcuts and tools. With this framework, developers can create better web applications and APIs. It also offers many add-ons, called middleware, to make coding quicker and simpler. Instead of writing all the server code from scratch with Node.js, developers use Express to speed up the process. It is known for being fast and organized, and it offers lots of extra features through plugins.
ReactJS
React is a JavaScript library that is used for building user interfaces. React is used for the development of single-page web applications, complex software applications, and mobile applications. React can switch rapidly changing data. React allows the client to code in JavaScript and create UI components. React is an open-source JavaScript library that can be used for creating views rendered in HTML. Unlike AngularJS, React is not a framework but a library.
Node.js
Node.js, in the beginning developed for Google Chrome and made open-source by Google in 2008, is a JavaScript runtime environment. It is designed for building scalable network applications and can run JavaScript code outside of a web browser. With Node.js, users can execute their code on servers without a browser. It comes with a node package manager, offering thousands of free packages (node modules) for download. Node.js works without an enclosing HTML page. Here, uses its module system, based on CommonJS, to combine multiple JavaScript files seamlessly.
Advantages of a MERN Stack Developer
One of the main advantages of the MERN stack is that developers use only JavaScript throughout the entire development process. JavaScript is a versatile programming language used for both client-side and server-side tasks. In contrast, when working with another full-stack setup that involves multiple programming languages, developers often face challenges in integrating them seamlessly. However, stack developers only need to be proficient in JavaScript and JSON.
The MERN Stack ecosystem is always a growing platform that receives regular updates with new tools and technologies, which are focused at improving the development processes. So, the demand never goes down. If you are interested in learning more about MERN Stack, be sure to check out the best MERN Stack training in India. Currently, the Srishti campus is the best training center in Kerala. There are a lot of online MERN Stack training centers available in Kerala.
0 notes
Text
5 Ways JavaScript Is Improving Modules for Developers - The New Stack
0 notes
Text
Angular: whats new in 10
The version v10 has been published and announced by this blog post. Although it may not appear as impactful as v9 (with Ivy and all), this release displays Angular team’s commitment to keep Angular up-to-date and relevant. I found this very exciting.
Today, in this blog we will learn what are the new features that are introduced in Angular 10. So, I jumped into the details of what changed and how to migrate. Here is what we found.
Pre-requisite: patience
MAJOR CHANGES:
New Date Range Picker
Optional Stricter Settings
Warnings about CommonJS imports
Deprecations and Removals
New Default Browser Configuration
As you go through the blog, their will be lot of theory in this blog,.. soo i request you all to be patient enough to go through the updates.
NEW DATE RANGE PICKER
https://byteridge.com/blog/images/0_ruU5G-8_hqEp3UBY-1.gif
To use the new date range picker, you can use the mat-date-range-input and mat-date-range-picker components.
See this example on StackBlitz. Learn more about date range selection.
2. OPTIONAL STRICT MODE We all know how much time will be saved by using strict mode,.. All the extra spaces a line and syntactic errors are displayed right away so that we need not wait until the code has been complied . You can opt for strict mode just by entering 3 magical words ❤ ng new --strict USES OF ENABLING STRICT MODE
Enables strict mode in TypeScript
Turns template type checking to Strict
Default bundle budgets have been reduced by ~75%
Configures linting rules to prevent declarations of type any
Configures your app as side-effect free to enable more advanced tree-shaking
https://byteridge.com/blog/images/commonjs.png
Keeping Up to Date with the Ecosystem a Typescript 3.9 : Angular 9 was released with TypeScript 3.7 support. Soon TypeScript 3.8 was released and Angular v9.1 supported it. Not long after, another TypeScript version, 3.9, is released and Angular responds with v10, keeping up. b TSLib 2.9 : TSlib is updated to version 2.0. The runtime library for TypeScript contains helper functions that have now been updated to TSlib 2.0. c TSLint v6 : The extensible static tool that checks TypeScript code for readability and functionality errors if any. Modern editors support it and build unique systems that can be customized with their own lint configurations, rules, and formatters. Starting with version 10 you will see a new tsconfig.base.json. This additional tsconfig.json file better supports the way that IDEs and build tooling resolve type and package configurations.
0 notes
Text
Using Top Level Await In Typescript: Simplifying Asynchronous Javascript Code
TypeScript code where you needed to use the “await” keyword outside of an asynchronous function? TypeScript 3.8 introduced a new feature called “Top Level Await” which allows you to use the “await” keyword at the top level of your code. Isn’t that exciting?
In this article, we will explore what exactly Top Level Await is, how it works, and the benefits and limitations of using it in TypeScript.
What is Top Level Await in TypeScript?
Have you ever encountered situations where you wanted to use the ‘await’ keyword at the top level of your TypeScript code? Well, you’re in luck because TypeScript now supports top level await!
In traditional JavaScript and TypeScript, the ‘await’ keyword can only be used within an ‘async’ function. This means that you need to wrap your code in an ‘async’ function and call it to use the ‘await’ keyword. However, this can sometimes lead to messy and unoptimized code. With the introduction of top level await TypeScript, you can now use the ‘await’ keyword directly at the top level of your code without the need for an ‘async’ function. This makes your code cleaner, more readable, and easier to maintain.
Top level await allows you to write asynchronous code in a synchronous manner. It provides a convenient way to handle Promises and async/await syntax without the need for additional boilerplate code. When using top level await, you can directly await a Promise or any other asynchronous operation in the global scope. This means that you don’t need to wrap your code in an ‘async’ function or use an immediately invoked async function expression.This feature is especially useful when you want to perform initialization tasks or fetch data from an API before your application starts running. Instead of chaining multiple ‘await’ calls or nesting them within ‘async’ functions, you can now simply use top level await to await the promises and continue with the execution of your code.
However, it’s important to note that top level await is currently an experimental feature in TypeScript and may not be supported by all JavaScript runtime environments or transpilers. It is recommended to check the compatibility of the runtime environment or transpiler you are using before using this feature in production code.
How Does Top Level Await Work?
Top level await changes this by allowing you to use await at the top level of your module, meaning you can use it outside of any function or block scope. This way, you can directly await a promise without having to wrap your code in an async function.
When you use top level await in TypeScript, the compiler automatically wraps your code in an immediately invoked async function. This function encapsulates your top level await expression and executes it synchronously, blocking the execution of any following statements until the promise is resolved or rejected.
For example, let’s say you have a module that needs to fetch some data from an API. With top level await, you can write code like this:import { fetchData } from './api';const data = await fetchData();console.log(data);
In this example, the fetchData function returns a promise that resolves to some data. Instead of using a traditional then callback, we can directly await the promise at the top level of our module and assign the result to the data variable. The console log statement will only execute once the promise is successfully resolved.
Top level await also allows you to use try-catch blocks to handle promise rejections. If the awaited promise is rejected, the catch block will be executed just like in regular async/await code.
It’s important to note that top level await is only available in modules that are marked as ES modules (i.e., have the module flag set to “ESNext” or “ES2020”). If you’re using CommonJS modules, you won’t be able to directly use top level await.
Furthermore, you need to ensure that your runtime environment supports top level await.
Benefits of Using Top Level Await in TypeScript
Top level await offers several benefits for asynchronous programming in TypeScript:
Simplified Syntax:
With top level await, you can directly use “await” at the outermost level of your code, eliminating the need for async function wrappers. This results in cleaner and more readable code.
Improved Error Handling:
Using top level await allows you to handle errors in a centralized manner. Instead of scattering error handling throughout multiple async functions, you can use a try/catch block at the top level to catch and handle errors from the awaited operations.
Easier Debugging:
Top level await simplifies the debugging process by providing a straightforward way to pause the execution and inspect the state of the code. This can be especially useful when working with complex asynchronous operations.
Faster Development:
By eliminating the need for explicit async function wrappers, top level await reduces boilerplate code, resulting in faster development time. It allows you to directly use “await” wherever needed, reducing the cognitive load of managing async functions.
Limitations of Top Level Await in TypeScript
While using top level await in TypeScript can be beneficial, it is important to note that there are also some limitations to be aware of. These limitations may impact your decision to use top level await in certain scenarios.
1. Compatibility
One major limitation of top level await in TypeScript is that it is only supported in environments that have native support for ECMAScript modules and top level await. This means that if you are working with older versions of JavaScript or in environments that do not support these features, you will not be able to use top level await.
Additionally, not all browsers and JavaScript engines have implemented top level await yet, which can restrict where you can use this feature. It is important to check the compatibility of your target environments before deciding to use top level await in your TypeScript code.
2. Module Systems
Another limitation of top level await is its interaction with module systems. When using top level await, your code must be within an ECMAScript module, which may require additional configuration and setup, especially if you are working with existing codebases that use different module systems.
For example, if you are using CommonJS modules in Node.js, you would need to convert your code to ECMAScript modules in order to use top level await. This can be a time-consuming process, and may not be feasible in all projects.
3. Debugging and Tooling
Debugging and tooling support for top level await in TypeScript can also be limited. Some IDEs and development tools may not fully understand and handle top level await, which can lead to issues with code completion, type checking, and other features.
Similarly, when debugging code that uses top level await, the debugger may not be able to step through the asynchronous code in the expected manner. This can make debugging more challenging and time-consuming.
4. Code Complexity
Using top level await can also introduce additional complexity to your code. Especially if you are not familiar with asynchronous programming concepts. It may require a deeper understanding of promises, async/await syntax, and potential pitfalls such as handling errors and cancellations.
This added complexity can make your code harder to read, understand, and maintain, especially for developers. It is important to consider the skill level and experience of the developers who will be working with the codebase.
5. Performance Implications
Lastly, it is worth noting that using top level await can have performance implications, especially in certain scenarios. Top level await blocks the execution of the module until the awaited promise is resolved. It can potentially delay the loading and execution of other modules or scripts that depend on it.
This can lead to slower application startup times and decreased performance, especially in large codebases with complex dependencies. It is important to carefully consider the performance impact of using top level await in your specific use cases.
Conclusion
While top level await can be a powerful feature in TypeScript, it is important to be aware of its limitations. By understanding these limitations, you can make an informed decision and ensure that your codebase remains maintainable and performant.
0 notes
Text
Node.js / Migrando de CommonJS a ES6
Hoy tomaremos nuestra app Notas y la migraremos de CommonJJS a ES6 para poder empezar de ahora en mas con esta caracteristica, espero les sea de utilidad!
Bienvenidos sean a este post, hoy continuaremos con la aplicacion Notas. Como mencionamos al momento de hablar de Express, este al ser creado antes de ES6 solo implementa a CommonJS pero eso no quita que se pueda implementar, para ello necesitaremos el proyecto que iniciamos en el post anterior, en caso de no tenerlo simplemente sigan los pocos pasos mencionados y listo, en nuestra aplicacion…
View On WordPress
0 notes
Text
You can learn JavaScript easily, Here's all you need to get started:
1.Variables
• var
• let
• const
2.Data Types
• number
• string
• boolean
• nUll
• undefined
• symbol
3.Declaring variables
• var
• let
• const
4.Expressions
Primary expressions
• this
• Literals
• []
• {}
• function
• class
• function*
• async function
• async function*
• /ab+c/i
• 'string'
• ()
Left-hand-side expressions
• Property accessors
• ?.
• new
• new .target
• import.meta
• super
• import()
5.operators
• Arithmetic Operators: +, -, *, /, %
• Comparison Operators: ==, ===,!=,!==, <, >, <=, >=
• Logical Operators: &&, ||, !
6.Control Structures
• if
• else if
• else
• switch
• case
• default
7.Iterations/Loop
• do…..while
• for
• for...in
• for...of
• for await...of
• while
8.Functions
• Arrow Functions
• Default parameters
• Rest parameters
• arguments
• Method definitions
• getter
• setter
9.Objects and Arrays
• Object Literal: { key: value }
• Array Literal: [element1, element2, ...]
• Object Methods and Properties
• Array Methods: push(), pop(), shift(), unshift(), splice(), slice(), forEach(), map(), filter()
10.Classes and Prototypes
• Class Declaration
• Constructor Functions
• Prototypal Inheritance
• extends keyword
• super keyword
• Private class features
• Public class fields
• static
• Static initialization blocks
11.Error Handling
• try,
• catch,
• finally (exception handling)
12.Closures
• Lexical Scope
• Function Scope
• Closure Use Cases
13.Asynchronous JavaScript
• Callback Functions
• Promises
• async/await Syntax
• Fetch API
• XMLHttpRequest
14.Modules
• import and export Statements (ES6 Modules)
• CommonJS Modules (require, module.exports)
15.Event Handling
• Event Listeners
• Event Obiect
• Bubbling and Capturing
16.DOM Manipulation
• Selecting DOM Elements
• Modifying Element Properties
• Creating and Appending Elements
17.Regular Expressions
• Pattern Matching
• RegExp Methods: test(), exec(), match(), replace()
18.Browser APIs
• localStorage and sessionStorage
• navigator Obiect
• Geolocation API
• Canvas API
19.Web APIs
• setTimeout(), setlnterval()
• XMLHttpRequest
• Fetch API
• WebSockets
20. Functional Programming
• Higher-Order Functions
• map(), reduce(), filter()
• Pure Functions and Immutability
21.Promises and Asynchronous Patterns
• Promise Chaining
• Error Handling with Promises
• Async/Await
22.ES6+ Features
• Template Literals
• Destructuring Assignment
• Rest and Spread Operators
• Arrow Functions
• Classes and Inheritance
• Default Parameters
• let, const Block Scoping
23.Browser Object Model (BOM)
• window Object
• history Object
• location Obiect
• navigator Object
24.Node.js Specific Concepts
• require()
• Node.js Modules (module.exports)
• File System Module (fs)
• pm (Node Package Manager)
25.Testing Frameworks
• Jasmine
• Mocha
• Jest
0 notes
Text
My thoughts on TypeScript
When I was a child, I used to play construction with my Dad’s music cassette collection. Sometimes, I would mix up the cases and the cassettes inside for fun. When my Dad wanted to listen to the music he liked, he would be disturbed when a completely different song play. And he would be frustrated when he couldn’t find the real one.
I feel the same frustration whenever I try to access a property in a JavaScript object that is supposed to be available and It doesn’t exist.
JavaScript gives me “God” like powers where I can create objects in one form and change it into something different at my whim. Like turning a fox into a horse or turning blood to wine. But this power gave me problems just like how I gave my dad problems.
If I had the magic lamp, I would ask Genie Smith to find me a way to designate types to data & objects when I write code and not when I execute it. And he would’ve said “Dude you can use TypeScript. It has what you need”.
If you don’t know what TypeScript is, it is an open source programming language designed to provide type-safety to JS projects with its strict type system.
After learning typescript for a week, here are my thoughts on TypeScript.
1. A Super set of JavaScript
Typescript uses the same syntax as JavaScript with nifty additional features. And I love it.
Typescript is just like JS but has a strict syntactic structure with stringent datatype rules. I would say it as a meta-data to JavaScript as it gives additional information about types and object structures. It kind of reminds me of C++.
2. Type safety
The type system in TypeScript, the set of rules on how to assign data types or types for short to variables, objects and other elements of my code is very stringent. This ensures that I do not assign a Person object to an Animal object or add a string with a number. This is called type safety in computer programming. Though JavaScript has type safety, it’s more lenient in my opinion.
3. A bouncer
I feel that typescript is like a bouncer in a bar who push away people when they do not follow the party etiquettes. It’s because TS pushes me back whenever there is an inadvertent type-related error until I fix it. It might seem tedious, It’s helpful nonetheless. The TS compiler helped me prevent spending a lot of time to debug the error which is the case in JS.
4. Code Hinting
My favourite part of typescript is its ability to present hints while I code. When combined with powerful code editors like VS Code or Atom, the contextual code suggestions helped me reduce errors and increased my typing speed. TS can do this because it’s a statically typed language. It means information about types are available to the compiler before the compilation starts. This availability of information helps the editors compile my code on the go and provide contextual suggestions.
5. Red squiggly lines
Available separately the linter when enabled in the editor, can detect errors of syntactic, type and even contextual nature. It presents the errors by underlining the error part with red squiggly lines as I type. This makes error correction easier and faster
6. Planning Ahead
New nifty features in typescript like the call signatures, object structure definition and interfaces allow me to plan ahead on how I’ll be applying my design to the code. For example, the call signatures are similar to function declaration in C allow me to sketch out the number of parameters needed and the return type. And the Object structure definition allows me to design a skeleton for an object before I define it.
7. Versatility
What makes typescript versatile is its wide variety of configuration options. I can enable and disable different options to cater to my project’s needs.
One of the settings I used a lot is the target option. It flipped between commonJS module system and the es5 module system while learning.
8. Just too many options
TS has just too many configuration options for a beginner. The ignorance of the purpose of some of these options led me to trouble. I did not know I had to include a separate library to use the DOM functions. I was like “What do you mean getElementById is not defined?”
9. Type definition
What makes typescript great is, it allows programmers to define new types for their need. Making use of this feature the definitely.org community has created high-quality type definitions for popular JS frameworks like JQuery, node.js and Angular which allows the use of these frameworks in Typescript.
But, could not find enough information on how to use a JS plugin or framework if it is not supported by the definitely typed community.
10. Partial to node.js
Typescript has so many features that I found useful. But in terms of documentation, it is partial to node.js. I found lots of learning aids about TS for node.js. But I couldn’t find equivalent amounts of learning aids for front end programming.
Conclusion
As a beginner, all these strict rules felt time-consuming since it took less time to write the same in JS. Over time, I realized the usefulness of TS and started using its features as I learnt them. I’ve decided to use typescript in my next side project instead of JavaScript.
References
“Programming TypeScript, Making your Javascript applications to scale” by Borris Cherney. ISBN - 9781492037651
“Type system”, Wikipedia
“Data type”, Wikipedia
0 notes
Text
tsconfig compilerOptions: A Comprehensive Guide to Optimizing TypeScript Compilation
Introduction
In the world of web development, TypeScript has gained immense popularity for its ability to bring static typing to JavaScript. To harness the full power of TypeScript, it is essential to configure the tsconfig.json file, particularly the compilerOptions. This article will serve as a comprehensive guide to optimizing TypeScript compilation by exploring various compiler options and their impact on the development process.
Understanding the tsconfig.json File
Before diving into the various compiler options, it's essential to understand the purpose of the tsconfig.json file. This file serves as the configuration file for TypeScript projects and allows developers to specify the compiler options, files to include or exclude, and other project-specific settings.
Setting the Target ECMAScript Version
One of the crucial decisions in the compilerOptions is choosing the target ECMAScript version. By setting the target option, developers can indicate the desired output ECMAScript version. For instance, if compatibility with older browsers is a concern, targeting ECMAScript 5 might be preferable.
Selecting Module Code Generation
The module option in the compilerOptions determines how TypeScript generates module code. Developers can choose from options like CommonJS, AMD, ES6, and more, depending on the project's requirements and the targeted environment.
Enabling Strict Type-Checking
TypeScript offers strict type-checking options that help catch potential errors at compile-time. Enabling options like strict, noImplicitAny, and strictNullChecks can enhance code quality and prevent runtime errors.
Customizing Output Paths
The outDir option allows developers to specify a custom output directory for compiled JavaScript files. This can help keep the project organized and separate the compiled files from the source code.
Bundling with Webpack
When working on large projects, bundling TypeScript files is essential for performance optimization. Developers can utilize Webpack and configure ts-loader to bundle TypeScript files efficiently.
Using Source Maps
Source maps are invaluable for debugging TypeScript code in the browser. By enabling the sourceMap option, developers can easily map the compiled JavaScript code back to the original TypeScript code, making debugging a breeze.
Using Declaration Files
TypeScript provides declaration files (.d.ts) to describe the shape of external JavaScript libraries. Developers can leverage the declaration option to generate these declaration files during compilation.
Targeting Different Environments
To target specific environments, developers can use the lib option to include different sets of TypeScript library files. This ensures that the compiled code is compatible with the chosen environment.
Handling Module Resolution
The moduleResolution option controls how TypeScript resolves module dependencies. Developers can choose between node and classic based on the project's module resolution strategy.
Optimizing for Speed
By setting the incremental option to true, TypeScript will cache the results of the compilation to boost subsequent compilation speeds. This is particularly helpful in large projects with multiple dependencies.
Including and Excluding Files
The include and exclude options allow developers to specify which files should be included or excluded from the compilation process. This can help fine-tune the scope of the TypeScript compiler.
Strictly Checking Function Parameters
The strictFunctionTypes option enforces strict checks on function types, ensuring greater type safety and avoiding potential bugs related to function compatibility.
Using Experimental Features
TypeScript continually evolves, introducing experimental features that are not part of the stable release yet. Developers can explore these features by enabling the experimentalDecorators and emitDecoratorMetadata options.
Conclusion
Configuring the compilerOptions in the tsconfig.json file is a critical step in optimizing TypeScript compilation for any project. By carefully selecting the right options, developers can enhance code quality, improve performance, and ensure better compatibility with different environments. Typescript Documentation https://www.typescriptlang.org/tsconfig
FAQs
- Q: What happens if I don't specify a target option in compilerOptions?A: If the target option is not specified, TypeScript will default to the ES3 target. - Q: Can I use multiple declaration files in my TypeScript project?A: Yes, you can generate multiple declaration files, each describing different parts of your project. - Q: Does enabling strict mode make TypeScript less flexible?A: Enabling strict mode enhances type-checking but doesn't reduce TypeScript's flexibility. It ensures better code quality and robustness. - Q: Is it possible to use TypeScript without a bundler?A: Yes, TypeScript can be used without a bundler, but bundling is recommended for better performance and organization in larger projects. - Q: How can I learn about the latest experimental features in TypeScript?A: The official TypeScript documentation and GitHub repository often highlight the latest experimental features and their usage. Read the full article
0 notes