I know stuff. I know some stuff so well, that people fly me around the globe to talk about that stuff. Some stuff I know well enough to give day long tutorials. Most stuff can;t be properly explained in depth during a one hour presentation. That stuff I put here. Read the stuff I write here and you might just learn some stuff. If you know this stuff too and want to have a polite conversation, find me on Twitter or Slack and we'll talk about stuff.
Don't wanna be here? Send us removal request.
Text
Time in the Palm of Your Hand
Tests involving time tend to be difficult. I’m sure we’ve all had problems with tests that failed sporadically because the action crossed over to a new second before the assertion. It happens so often that I look for time factors on any test that has inconsistent results. Fortunately, in Python, you can mock the functions that most of us use to get time, now() and utcnow(). I do it so infrequently myself that I keep ending up searching my code for how I did it last time. As a resource to you and a reminder for me, the trick is patching the datetime.datetime object with unitest.patch.object: https://docs.python.org/3/library/unittest.mock.html#patch-object. It has to be done at the datetime.datetime object level like so: @patch.object(datetime, 'datetime', Mock(wraps=datetime.datetime)). Also, you have to make sure you code only imports datetime.datetime like so: from datetime import datetime. Now you are ready to set return values like this: datetime.datetime.utcnow.return_value = datetime.datetime(2016, 1, 1, 0, 0, 0, 0, UTC). This will set a consistent response for utcnow()...AWESOME!
Here an example of a test for Python2.7:
from unittest import TestCase import datetime from mock import patch, Mock from pytz import UTC def get_now(): from datetime import datetime return datetime.utcnow() class TestPingHandler(TestCase): @patch.object(datetime, 'datetime', Mock(wraps=datetime.datetime)) def test_get_returns_expected_api_time(self): expected = datetime.datetime(2016, 1, 1, 0, 0, 0, 0, UTC) datetime.datetime.utcnow.return_value = expected actual = get_now() self.assertEqual(expected, actual)
0 notes
Text
PHP Machinist 3.0 Released
PHP Machinist 3.0 was released yesterday with very little fanfare. The latest major release had minimal impact on functionality but broke backwards compatibility with the 2.1.0 release in order to become PSR-1 compliant. Placing the code base under scrutiny by the new SensioLabs Insight product was the push needed to get the codebase compliant,
0 notes
Text
Functional Testing The Right Way - Part 1 - The Right Tools
Preface
This is part one of a multi-part series on doing functional testing the right way. Good functional testing is not as easy as it sounds. It's taken me years to to get to the point where I can get the business, operations, QA, and development teams to agree that the tests we make are valuable, useful, and easy to implement. I hope that sharing what I have learned can reduce the learning curve for others that have the desire to implement functional testing.
Part 1 - The Right Tools
There are a multitude of tools available for functional testing. I have used a number of them and most fall short of what should be the overall goal of functional testing, to provide useful insight into functional flaws of an application with minimal upkeep. Many tools can provide the ability to know when functionality is broken. The hard part is providing useful insight. Even harder is providing this insight with minimal upkeep.
In the beginning of my foray into functional testing, we used Selenium testing. Not directly integrating with the API but performing browser tests and converting them in to unit tests. It was easy to create tests but they provided little insight. This was especially true in a continuous integration (CI) environment. The results, a failure message of "Failed to assert false was true" and a picture of a web page, still haunt my memories. Obviously, there were some very important pieces missing from this process. Why did the test fail and how do I recreate the specific error reliably to fix the issue? We had hundreds of these tests and a team of dedicated test writers to write tests. We had invested in infrastructure to display broken builds on overhead monitors and send email and text alerts. All of these things ended up just being noise and were summarily ignored as the development team couldn't diagnose the bugs and the operations team didn't see an increase in system quality. In a word, useless.
Our next attempt at functional testing was HTML Unit. This was an improvement over our Selenium implementations as we had more control over tests and could give better failure messages. We had gained some insight but at what cost? Writing HTML Unit tests is a grind at best. It would take at least as much time to write tests as it would to write the code. This required a specific skill set with dedicated resources. Not having as many test writers meant that testing was always behind coding and quality in delivery only improved slightly. It was better but not what we were really trying to accomplish.
In our third attempt, we were forced to find a real solution. I was part of new team that was taking over some extremely important and highly visible legacy software. This was the worst possible software for a test minded developer. It was written in such a fashion that the code itself was not unit testable. This led to consistently releasing bugs in core components. It was a real quality issue that had the operations group, specifically the finance team, unwilling to trust any of the data produced by the system. Making the situation more painful was that nearly every penny of revenue for the company was reliant on this application to work reliably and accurately. It's hard to imagine a worse scenario for a software developer who takes pride in their work. Our standard procedure of using unit testing to cover for the lack of functional testing was not possible. This all led to one of the team members performing a comparative analysis on four testing candidates: Selenium, HTML Unit, RSpec, and Cucumber. Each product respectively had a significant reduction in the amount of work required to write useable tests. In the end we chose Cucumber for these major factors:
It is based on creating reusable code blocks in "steps" that you build upon.
The tests can be verified and validated by the business as the steps use natural language for their definition.
Recreation of the test failure is simple as the steps provide step by step instructions on recreating the error.
Tests can be executed in a headless environment for speed as well as utilizing the Selenium API for real browser testing.
The tests are living and accurate documentation of the application functionality.
We have since migrated from Cucumber to Behat as we are primarily a PHP shop and writing steps in your primary language is certainly preferable.
It's been over three since we decided to use BDD tools for providing functional testing and I have never regretted the decision. I have also leaned that there is a right way and a wrong way to use BDD tools. Most of those lessons learned from doing it the wrong way. That will be left for the next installment of the series.
1 note
·
View note
Text
PHP Machinist 2.0 Released
The long anticipated release of PHP Machinist 2.0 is finally here. For 2.0, Behat support was split out into it's own package and is now a Behat Extension. Here are the significant changes:
PHP Machinist
More thorough documentation with better examples
Doctrine ORM support for blueprints
PHP Machinist Behat Extension
Useful documentation with working examples
behat.yml configuration of connections and blueprints
Data verification steps as well as data fixture steps
Injection of the Machinist instance via a Machinist aware interface for Behat contexts
Check both of them out on Github:
PHP Machinist
PHP Machinist Behat Extension
0 notes
Text
How Composer Changed the World of PHP
Let's go back...way back...to the before time...the long long ago. The time before Composer. A time when finding a useful PHP library was difficult and sharing a useful library was harder. A time when libraries were global shared code. A time when publishing libraries required a web server and a complicated system for managing pre-release and release versions. A time when most shared libraries had few contributors and little visibility. A time when your hosting provider made decisions about which libraries were available for your application. A time when it was just easier to write your own code instead of using someone else's library.
The lack of an easy to use dependency manager was greatly amplified in Symfony 2.0 development. Symfony's modular design is fantastic. The ability to create distinct reusable modules is absolutely the correct was to develop large enterprise applications. However, modular design leads to a difficult if not impossible scenario for managing dependencies for unit testing. At the Selling Source, we had very smart people trying to solve this problem. It was a large problem. Much larger than our small team could resolve with our limited resources. We tried multiple solutions. Our solutions made the situation tolerable but required a certain level of sacrifice in either testability, functionality, or modularity.
When I discovered Composer, it solved so many of the issues that are associated with creating highly testable modular code. It did it so well that my team and I began migrating to it immediately. At that time, Composer was raw. I mean really raw. There were times when you used the self update feature and the current release was completely broken. We are talking PHP fatal error broken. We had to change our bootstrap for autoloading and formatting of the configuration file a few times as well. Also, as there weren't many libraries registered with packagist.org, the central repository for Composer, we had to manually create external package definitions in each project's configuration files. None of these issues deterred us from the product as the alternative was so much worse.
Now, let's forward to today. Composer is the first tool in my PHP arsenal. It is literally the most important tool for my development in PHP. It may arguably be the single most important tool in all PHP development. There are more than 9,300 open source PHP packages on packagist.org that have been installed more than a mind blowing 19 million times over the last year. If you need a piece of functionality for an application, it is extremely likely that there is a well designed and developed open source library available. You will likely never have to write an API client again. And if you do, you can easily turn it into an open source project to get community support for the library. All that's needed is a public VCS repository and a registration with packagist.org. Even if you need a local package repository for private code, you have options in Satis, a fairly simple application for making shared Composer package definitions, or your own Packagist server if you need the added complexity it provides.
I'm sure that as you read this, or even before you read this, you made your own opinion about the usefulness of Composer. My opinion is that Composer makes PHP one of the easiest platforms to develop applications of any size, regardless of you framework or lack of a framework. The ability to reuse your code and use the code of others has greatly reduced the time to stand up any application. You may not believe me but the proof is in the pudding. For Start Up Weekend in Las Vegas, I built a stripped down community site in 52 hours. At a Las Vegas hack-a-thon, I was a member of a team of PHP developers that wrote an application in six hours. The total amount of PHP code the team had two write was 80 lines. Yes, you read that correctly, 80 lines. 80 lines of code that had a useable home page, integration with the U.S. Homeland Security terror alert feed, inbound and outbound SMS integration, and inbound and outbound email integration. 80 lines of code! I'll say it again, 80 lines of code! The rest of the code was written by other developers and wired up using Composer. It's also worth mentioning that there were many teams at this hack-a-thon trying to implement similar functionality. There were NodeJS, Ruby, and Java teams. Our team of 3 PHP developers was the only team to completely finish their application. A year or two ago, that would have been unthinkable. Composer has changed the world of PHP.
0 notes
Text
Vegas Startups, It's Almost Too Easy
It's been a while since I made a post. I've been knee deep in a Las Vegas startup. My involvement may be serendipity. It may be part of some master plan by Devin Egan, CTO of LaunchKey. It's likely a bit of both. Either way, I was able to stumble into help launch an Internet start up in Las Vegas that may actually succeed. If it fails, it will only be our fault. Everything required to succeed had been placed in front of us. It's almost too easy.
Fresh off a trip to ZendCon 2012 with some co-workers at the Selling Source, I was excited about what seems to be a brave new order in the PHP community. I wanted to indulge my inner geek and immerse myself in everything tech. A few weeks later I received a free ticket for Startup Weekend with his claim that it would be an excellent networking opportunity. Ticket in hand, I headed down on a Friday night to see what it was all about.
The first night at Startup Weekend involves a little meet and greet, a little warm up exercise, and pitches. We all voted on the pitches and the pitch presenters began team building. A double dating site pitch from an energetic and engaging Adam Kramer intrigued me. I conducted a quick two minute interview and joined the team. The rest of the weekend was a whirlwind of activity which involved taking an Adam's idea and evolving it into a viable business proposition with a working demo in less than two days.
During those two days, we had coaches helping us with revenue stream modeling, legal implications, design considerations, and crafting our final pitch. I may have slept for three or four hours the whole weekend. Some of the sleeplessness was due being truly excited about the events of the weekend. The rest was due to the monumental task of creating a working demo of a community site in two days. Most of the other teams had more developers and a seemingly more reasonable scope for their application. But we weren't deterred. Hard work and unwavering determination resulted in a final product that took third place overall, second place for design, and gave us all the belief that we really had something that could succeed. A product people would use that would help strengthen their relationships. Coupla was born.
It's only been just over a month now and it seems like a year. In that time we've received an immense amount of support from the Las Vegas community. We attended "office hours" with the likes of Aaron Battalion, Co-Founder and CTO of Living Social who helped us hone our zero-budget customer acquisition strategy. We met with Jason Mendenhall of Switch who helped us find an affordable cloud strategy that would house or systems in Las Vegas. These invaluable resource gave us the ability to bootstrap the business and not have to deal with the pressures of attaining capital to get our startup off the ground.
We've also been drawing on the global community to build our stable, secure, and scalable infrastructure for mere pennies. We've been able to get our corporate email, calendaring, shared storage, and office suite from Google for $5 dollars per user per month. We have attained world class software lifecycle, ticketing and agile project management tool in Jira/Greenhopper as well as unlimited private repositories in BitBucket hosted by Atlassian for $30 dollars per month. We set up a cloud with three instances and unlimited throughput for our application at a whopping $65 per month through Joyent. That's right at $110 per month for world class software and services that provide everything our three person team could need. It is truly mind blowing when you think about it.
That leaves the rest to us. The three brave souls who met just over a month ago and started down the trail of their very own internet startup. If we don't make it, it certainly won't be because there were insurmountable challenges of infrastructure , hosting, and scalability placed before us.
1 note
·
View note
Text
It's a Trap or Too Small to Fail
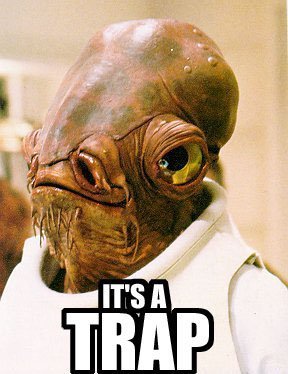
In the world of software development, the most dangerous projects seem to be the quick, small, or "one and done" projects. They lead us down the path of bypassing our standard process. Most of us know better but we just continue to do it. In the end, we come to same result, failure. It boggles the mind to think that we don't see the trap after all these years. Admiral Ackbar, where are you when we need you?
We've all been enticed with "knocking it out." It's so small, it seems overkill to put it under test. It's just a one off that won't live longer than a week we tell ourselves. The timeline is too tight for proper testing. Who has the time to go back and pretty up our code? Who's going to see it anyway? Some of us have even been told not to "waste to much time testing" by some project manager or business owner. However we got there, we found ourselves cutting corners to simply knock it out.
You'd think we would know better. Even though we've proven to ourselves time and time again that doing it the right way always is the fastest path. We know that even though we think a piece of code will only last for a short period of time, it ends up living forever and developers curse our names as they try and wade through the mire we left behind. You'd think we'd be tired of cleaning the egg off of our face from explaining to the business owner how this bug and that bug could creep into this tiny little project and why we have to rewrite the whole thing to make it work as expected. But, for some reason, we think this time it will be different. Why do we get a glimmer of a cup half-full mentality only when we're about to do something truly foolish.
And then it happens, the inevitable failure. We curse our own stupidity. We ask ourselves, "how did I let this happen again?" We wonder how we fell for it one more time. We try and fix that bug or add that forgotten feature that wasn't in the original spec. We do it and spend twice as long as it would have taken to do it right the first time. We tell ourselves it will never happen again.
The reality is, we made the same mistake everyone makes. I still haven't met the expert that hasn't fallen into this trap from time to time. We all strive for the for perfection; but, alas, we are human. Silly fallible humans that will fall into the occasional trap from time to time. Al we can do is shake our heads. Proclaim what ridiculous morons we were and move on to the next pursuit of perfection.
0 notes
Text
Doing it Right or Doing it Fast
I was listening to a presenter the other day and I heard the phrase that dives me absolutely nuts. What he said was that you have to make a decision. You can do it fast or you can do it right. Just the idea that a revered software professional doesn't understand that doing it right is doing it fast. Code the right way is tested, readable, and meets the business requirement. When you leave out any out any one of these facets, you're going to run into trouble. In a world of incomplete specifications and agile methodologies, you have to refactor your code before you even deliver a finished product. Producing untested and/or unreadable code makes every following iteration longer. You also introduce the likelihood that your code doesn't even work. This will require extra QA and coding time. So, for Pete's sake, let's just remember that doing it right is the fastest way to do it in the first place.
0 notes
Text
How Symfony 2 Saved Me From Python
Now, before all you Python lovers get in an uproar, I’m not saying there is anything wrong with Python at all. So, relax!
In 2011, my company was desperately searching for a real, honest to goodness, heavyweight framework to use for our core application development. That meant a wide range of uses including XML based APIs, customer portals, giant admin sites, and a website framework that would be used as a single code base for hundreds of sites. We had tried all of the PHP frameworks in different projects and still have some Zend Framework based sites, even though we all learned to hate Zend Framework. We had also recently decided to migrate to and then away from Java as it was a poor fit for a lot of our uses. Trying to turn PHP developers into Java developers didn’t work out so fantastically either. We finally came to the fork in the road. Should we move Ruby on Rails or Python and Django? As Python seemed to be a much easier language to migrate towards from PHP, we were all ready to become Python developers. Not too mention that Django is fairly cool as well.
So, what’s wrong with Python and Django? Absolutely nothing. But the pain of transforming a large installed base and ramping up 20 developers on a whole new language is no small feat. We were certainly prepared to bite the bullet because every framework in PHP was simply terrible and Django seemed like our only option. But then, we caught wind of symfony 2. We had probably dismissed it before because none of us really saw Symfony 1 as a step up from Zend Framework. The fact that it utilized Doctrine 2 was a bonus as we were already using Doctrine 2 in projects. Behat integration was a welcome site as well. We were already migrating from Cucumber to Behat for automated integration testing. It was also using dependency injection that looked strikingly similar to Spring so it made that learning curve much smoother.
We did our first live application while Symfony 2 was in RC2 but it was stable enough for our immediate needs. There weren’t many serious hurdles we had to face with non-stable software and were willing to take the risks. The project ended up going nowhere and turned out to be a fantastic throw away learning project. I’m sure the business folks that paid three developers for a year to build it didn’t feel that way but it seemed to work out well for us developers.
Symfony 2 survived and we’re now in the middle of a project to migrate all of our revenue generating websites, about 160 of them, over to a common framework based in Symfony2. We have learned a ton about the pitfalls of both over bundling and under bundling. We also learned that bundles don’t remove the need for a multi-tier architecture. All of this “learning” created a bit of technical debt but the project has been a success overall. We’ll see what the future holds as the code base calls us on that technical debt.
0 notes