#codeigniter development outsourcing
Explore tagged Tumblr posts
Text
Robust Laravel Application Development - Lessons Learned
Introduction
Building a robust Laravel application that can scale, handle high traffic, and remain maintainable over time is not without its challenges. Over the years, developers and teams have encountered several common pitfalls and learned invaluable lessons while building and maintaining large-scale Laravel applications.

Developing robust applications with Laravel requires careful planning, attention to detail, and adherence to best practices. It is also essential to have an in-depth understanding of the intricacies of secure and scalable architecture. This would ideally mean trusting a software development outsourcing company like Acquaint Softtech.
This article provides more information on the lessons learned from building robust Laravel applications. It focuses on best practices, common mistakes, and solutions to improve application scalability, security, maintainability, and performance.
Laravel Application Development

Laravel has rapidly become one of the most popular PHP frameworks due to its expressive syntax, rich ecosystem, and developer-friendly features. With Laravel, developers can build modern web applications with ease, thanks to the abundance of built-in tools, comprehensive documentation, and support for modern PHP standards.
It is a powerful PHP framework that offers developers a structured and efficient way to build web applications. There are many reasons why Laravel is the ideal web framework. It has an elegant syntax, follows the MVC architecture, and has a special Blade templating engine.
Besides this, Laravel also eliminates the need to build an authentication system from scratch since it is in-built. The special object-relational mapping, Eloquent CRM helps simplify the database operations.
But that's not all. Not only does Laravel have good features to build a robust solution, but it also has the necessary tools and features to help you test it. Testing, debugging, and validation are highly simplified with Lararel. It allows the use of special test tools like PHPUnit and PEST.
Here are a few interesting statistics:
2706 of the Laravel powered websites are from the Web Development sector. (6sense)
Over 303,718 Americans use Laravel, while this figure is 54,648 in the United Kingdom and 31,053 in Russia. (BuiltWith)
As data collected in 2024, 7.9% of developers use the Laravel framework worldwide while 4.7% use Ruby on Rails, 3.2% use Symfony framework and 1.7% use Codeigniter.
Node.js is one of the most popular with 40.8% using it; React comes close second at 39.5%
Lessons Learned

Understand the MVC Architecture Thoroughly : Lesson 1
Laravel adheres to the Model-View-Controller (MVC) architecture, which separates the application logic from the user interface. This separation is critical for building scalable and maintainable applications. One of the key lessons learned from working with Laravel is that a deep understanding of the MVC architecture is essential for building a robust application.
Model: Represents the data layer and handles business logic.
View: The user interface that the end-user interacts with.
Controller: Mediates between the Model and the View, processing user requests and sending the appropriate response.
A common mistake is allowing business logic to seep into the controller or view. This breaks the MVC pattern, making the application harder to maintain and test. Adhering to strict separation of concerns ensures that each application part can be developed, tested, and maintained independently.
Key Takeaway: Always ensure that business logic resides in models or services, controllers handle user requests, and views strictly present data without containing any application logic.
Use Eloquent ORM Effectively : Lesson 2
Laravel's built-in Object Relational Mapping (ORM) tool (Eloquent) provides a simple and elegant way to interact with the database. However, improper use of Eloquent can lead to performance issues, especially in large applications with complex database queries.
Common Mistakes: N+1 Query Problem: The N+1 problem occurs when a query retrieves a list of items but then executes additional queries to fetch related items for each entry. This can lead to hundreds of unnecessary database queries. To avoid this, Laravel offers the with() method for eager loading relationships.
php code
// Bad
$posts = Post::all();
foreach ($posts as $post) {
echo $post->author->name;
}
// Good (Using eager loading)
$posts = Post::with('author')->get();
foreach ($posts as $post) {
echo $post->author->name;
}
Mass Assignment Vulnerabilities: Laravel's MassAssignment allows you to bulk insert or update data in the database. However, safeguarding against mass assignment can expose your application to vulnerabilities. Developers should always use $fillable or $guarded properties in their models to protect against mass assignment.
php code
// Protecting against mass assignment
protected $fillable = ['title', 'content', 'author_id'];
Solutions: Always use eager loading to avoid the N+1 problem.
Define fillable attributes to protect against mass assignment.
Avoid complex queries in models. Instead, use Query Builder for more advanced database interactions that Eloquent struggles with.
Key Takeaway: Use Eloquent for simple CRUD operations, but be mindful of performance concerns and security risks. When necessary, fall back on Laravel’s Query Builder for complex queries.
Service Layer for Business Logic : Lesson 3
As Laravel applications grow, keeping controllers slim and models focused on data becomes increasingly important. This is where the service layer comes in. Service classes allow developers to isolate business logic from controllers and models, making the application more modular and easier to test.
Example:
Instead of handling user registration logic in the controller, a service class can be created to handle it.
PHP code
// In the UserController
public function store(UserRequest $request)
{
$this->userService->registerUser($request->all());
}
// In UserService
public function registerUser(array $data)
{
$user = User::create($data);
// Other business logic related to registration
}
This pattern makes it easier to maintain, test, and scale the application as business logic becomes more complex.
Key Takeaway: Introduce a service layer early on for business logic to improve code organization and maintainability.
Prioritize Security at Every Step : Lesson 4
Security is a fundamental aspect of building a robust Laravel application. One of the lessons learned from years of Laravel development is that security should not be an afterthought. Laravel provides many built-in security features, but it’s up to developers to use them correctly.
Key Security Practices:
Sanitize Input: Always sanitize user inputs to prevent XSS (Cross-Site Scripting) and SQL injection attacks. Laravel automatically escapes output in Blade templates, which helps protect against XSS. For SQL injection protection, always use Eloquent or Query Builder.
Use CSRF Protection: Laravel automatically protects against Cross-Site Request Forgery (CSRF) by including a CSRF token in forms. Developers must ensure that CSRF protection is not disabled unintentionally.
Authentication and Authorization: Laravel offers built-in authentication and authorization mechanisms through policies, gates, and middleware. Use them to control access to resources and ensure users have the necessary permissions.
Password Hashing: Always store user passwords using Laravel’s Hash facade, which uses bcrypt by default. Avoid storing plain-text passwords in the database.
PHP
$user->password = Hash::make($request->password);
Rate Limiting: To prevent brute force attacks, implement rate limiting using Laravel’s RateLimiter facade.
Secure API Development: When building APIs, use OAuth2 or Laravel Sanctum for token-based authentication. Also, rate limiting for API endpoints should be implemented to prevent abuse.
Key Takeaway: Leverage Laravel's built-in security features and be proactive in identifying potential vulnerabilities.
Lesson 5: Cache Strategically for Performance
Caching is critical for improving the performance of Laravel applications, especially as they scale. Laravel supports various caching mechanisms, including file, database, Redis, and Memcached.
Best Practices for Caching:
Cache Expensive Queries: If your application frequently runs heavy queries, consider caching the results to reduce the load on the database.
PHP code
$users = Cache::remember('users', 60, function () {
return User::all();
});
Route Caching: Laravel provides a route:cache Artisan command to cache all routes in your application. This can significantly improve the performance of larger applications.
Bash CODE
php artisan route:cache
View Caching: Similarly, views can be cached to avoid unnecessary recompilation.
Bash CODE
php artisan view:cache
Optimize Configuration Loading: Use config:cache to combine all configuration files into a single file, reducing the time it takes to load configurations during requests.
bash CODE
php artisan config:cache
Key Takeaway: Use caching strategically to optimize performance and reduce server load, especially for database-heavy applications.
Optimize Database Interactions : Lesson 6
Efficient database interaction is critical for building scalable Laravel applications. Poorly optimized queries or over-reliance on ORM abstractions can lead to performance bottlenecks.
Best Practices for Database Optimization:
Database Indexing: Ensure that frequently queried columns are indexed. Laravel’s migration system supports creating indexes with ease.
php code
Schema::table('users', function (Blueprint $table) {
$table->index('email');
});
Avoid SELECT * Queries: Always specify the columns you need in a query. Fetching all columns unnecessarily increases the load on the database and memory usage.
php code
$users = User::select('id', 'name', 'email')->get();
Use Pagination: For large datasets, always paginate results rather than fetching everything at once.
php code
$users = User::paginate(15);
Database Query Profiling: Use Laravel’s built-in query log to identify slow queries. You can enable query logging with the following code:
php code
DB::enableQueryLog();
Key Takeaway: Optimize database queries by using indexes, avoiding unnecessary data fetching, and employing efficient pagination techniques.
Test Your Application : Lesson 7
Testing is a crucial aspect of robust Laravel application development. Laravel comes with PHPUnit integration, making it easy to write unit tests, feature tests, and browser tests. However, many developers tend to skip or neglect testing due to time constraints, which often leads to bugs and regressions.
Testing Best Practices:
Unit Tests: Unit tests focus on testing individual components or methods. They are fast and reliable but don’t cover the whole application flow.
php code
public function testUserCreation()
{
$user = User::factory()->create();
$this->assertDatabaseHas('users', ['email' => $user->email]);
}
Feature Tests: Feature tests allow you to test the entire application flow, from requests to responses. They ensure that the application behaves as expected in real-world scenarios.
php code
public function testUserRegistration()
{
$response = $this->post('/register', [
'name' => 'John Doe',
'email' => '[email protected]',
'password' => 'secret',
'password_confirmation' => 'secret',
]);
$response->assertStatus(302);
$this->assertDatabaseHas('users', ['email' => '[email protected]']);
}
Continuous Integration: Integrate automated tests with a continuous integration (CI) tool such as GitHub Actions or Travis CI. This ensures that your tests are automatically run whenever new code is pushed, preventing broken code from being deployed.
Key Takeaway: Always write tests for critical application components and workflows to ensure stability and reliability.
Prioritize Code Maintainability and Readability : Lesson 8
Laravel is known for its elegant syntax and developer-friendly structure, but as the application grows, maintaining clean and readable code becomes challenging.
Best Practices for Maintainability:
Follow PSR Standards: Laravel encourages following the PHP-FIG’s PSR standards, such as PSR-2 for coding style and PSR-4 for autoloading. Following these standards ensures that your codebase remains consistent and easy to navigate.
Use Named Routes: Using named routes instead of hard-coded URLs makes it easier to manage changes in the URL structure.
php code
Route::get('/user/profile', 'UserProfileController@show')->name('profile');
Refactor Regularly: As the application evolves, refactor code regularly to avoid technical debt. Use Laravel’s php artisan make:command to create custom Artisan commands that automate repetitive tasks.
Comment and Document: Clear comments and documentation help other developers (or even yourself in the future) understand the purpose of the code. Use Laravel’s DocBlocks to document classes, methods, and variables.
Key Takeaway: Invest in code quality by following coding standards, refactoring regularly, and documenting thoroughly.
Leverage Laravel’s Ecosystem : Lesson 9
Laravel has an extensive ecosystem that can simplify development and add powerful features to your application. Leveraging this ecosystem can save Laravel application development time and reduce complexity.
Key Laravel Tools and Packages:
Laravel Horizon: Provides a dashboard and tools to monitor queues and jobs in real-time.
Laravel Echo: Integrates WebSockets and real-time broadcasting capabilities into Laravel applications.
Laravel Telescope: A debugging assistant that allows you to monitor requests, queries, exceptions, logs, and more.
Laravel Sanctum: API authentication using tokens is especially useful for single-page applications (SPAs).
Laravel Vapor: A serverless deployment platform for Laravel that enables you to deploy Laravel applications on AWS Lambda without managing infrastructure.
Key Takeaway: Use Laravel’s ecosystem of tools and packages to extend your application’s capabilities and streamline development.
Prepare for Scalability : Lesson 10
As your Laravel application grows, you need to ensure that it can scale to handle increased traffic and data.
Key Scalability Considerations:
Horizontally Scale Your Database: As your user base grows, consider using database replication to scale horizontally. Laravel supports multiple database connections and read-write splitting.
Use Job Queues: Offload time-consuming tasks (such as sending emails or processing files) to queues. Laravel’s queue system supports drivers like Redis, Beanstalkd, and Amazon SQS.
Optimize Load Balancing: For high-traffic applications, consider using a load balancer to distribute incoming traffic across multiple application servers.
Containerization: Use Docker to containerize your Laravel application. Containerization ensures that your application can run consistently across various environments and can be scaled easily using orchestration tools like Kubernetes.
Key Takeaway: Plan for scalability from the start by using job queues, load balancing, and database replication.
Use Configuration and Environment Management Properly : Lesson 11
Lesson: Mismanaged environment configurations can lead to security and stability issues.
Practice: Store sensitive configurations like database credentials, API keys, and service configurations in .env files. Utilize Laravel’s configuration caching (config:cache) for production environments.
Tip: Regularly audit .env files and restrict sensitive data from being logged or committed to version control.
Some of the other lessons learned from developing a robust Laravel solution include that it has Artisan. This allows one to use a command line tool, Artisan, that can automate repetitive tasks. This serves the purpose of accelerating the development process. It is possible to simplify the process of managing the packages with the help of 'Composer'. API development is a breeze with Laravel since it has in-built support for RESTful APIs.
Benefits Of A Robust Laravel Solution
A robust Laravel solution offers a multitude of benefits for businesses and developers, ranging from enhanced security and scalability to faster development and easier maintenance.
Hire remote developers from Acquaint Softtech to build a top-notch solution. We have over 10 years of experience developing cutting-edge solutions.
Here's a detailed look at the key benefits of using a robust Laravel solution:
Scalability and Performance Optimization
Enhanced Security Features
Faster Time to Market
Modular and Maintainable Codebase
Seamless API Integration
Efficient Database Management
Simplified User Authentication and Authorization
Robust Testing Capabilities
Efficient Task Scheduling and Background Processing
Vibrant Community and Ecosystem
Advanced Caching Mechanisms
Support for Multilingual and Multi-tenant Applications
Built-in Tools for SEO and Content Management
Conclusion
Building a robust Laravel application requires a deep understanding of best practices, design patterns, and the ability to anticipate challenges. By learning from the lessons shared above, developers can avoid common pitfalls and ensure that their applications are secure, performant, maintainable, and scalable. Following these guidelines not only enhances the quality of the code but also helps deliver applications that stand the test of time
Analyzing case studies of successful Laravel applications can provide a roadmap for best practices and strategies. At the same time, these lessons learned from developing a robust, scalable, and secure Laravel application are also of great value.
#Robust Laravel Application Development#Laravel Application Development#Laravel Application#Hire Laravel Developers
0 notes
Text
PHP Web Development India: A Growing Hub for Global Businesses

In recent years, PHP web development in India has become a significant driver for businesses across the globe, as it offers cost-effective solutions and exceptional services. PHP, a powerful and flexible scripting language, powers a vast majority of websites and web applications due to its ease of use and compatibility with various databases. In India, the demand for PHP web development services has skyrocketed, making it a key destination for global businesses looking to create dynamic websites and scalable web applications. Whether it is an e-commerce platform, a content management system (CMS), or a custom web solution, Indian developers are providing top-notch PHP development services at a fraction of the cost compared to other countries. This blog will explore the current trends in PHP web development in India, why it has become so popular, and how businesses can leverage this trend to their advantage.
Why is PHP Web Development So Popular in India?
PHP is a versatile language that has been around since 1995. It has evolved over time and remains a cornerstone of web development for a variety of reasons:
Open-Source Nature: PHP is open-source, meaning developers can use and modify it freely. This significantly reduces the overall development costs for businesses. Indian developers, well-versed in working with open-source platforms, are known for delivering high-quality PHP solutions at an affordable price point.
Huge Talent Pool: India is home to a large pool of skilled developers who are highly proficient in PHP. Many of them have experience working with global clients across different industries, including finance, healthcare, education, and e-commerce. The country’s thriving IT industry, supported by robust educational institutions, ensures a steady supply of top-tier PHP developers.
Cost Efficiency: Compared to Western countries, the cost of PHP web development in India is significantly lower. This makes India an attractive outsourcing destination for businesses looking to save on development costs without compromising on quality. Companies in the US, UK, and other Western nations are increasingly outsourcing PHP development work to India to take advantage of these cost savings.
Wide Range of Frameworks: PHP developers in India are adept at working with popular frameworks like Laravel, CodeIgniter, Symfony, and Zend, which allow for faster and more efficient web application development. These frameworks provide a structured way of developing applications, which makes the code more maintainable and scalable.
Key PHP Web Development Trends in India
The PHP web development landscape in India is constantly evolving, with new trends emerging regularly. Some of the key trends that are currently shaping the industry include:
1. Integration with Modern Technologies
As businesses demand more from their websites and web applications, Indian PHP developers are integrating modern technologies like artificial intelligence (AI), machine learning, and blockchain with PHP solutions. This enables companies to offer enhanced user experiences, smarter features, and better security for their customers.
2. Mobile App Integration
With the rise of mobile-first users, integrating web applications with mobile apps has become essential. PHP developers are now focusing on creating seamless connections between websites and mobile applications. This trend is particularly beneficial for businesses looking to provide a consistent user experience across web and mobile platforms.
3. Custom Solutions for Businesses
Every business has unique requirements, and custom PHP solutions are increasingly becoming the norm. Instead of relying on pre-built templates, businesses are opting for custom PHP development to ensure their website or web application meets their specific needs. This shift towards tailored solutions is helping businesses stay ahead of the competition.
4. Security and Scalability
With cyber threats becoming more sophisticated, security is a top priority for businesses. PHP developers in India are focusing on building secure applications by using encryption, secure payment gateways, and other security measures. Additionally, they are ensuring that websites and applications built with PHP are scalable, meaning businesses can grow their online presence without worrying about performance issues.
Mobile App Cost Calculator: Helping You Plan Your Budget
If you are considering creating a mobile application alongside your website, it’s important to understand the cost implications. Developing a mobile app requires a detailed understanding of both the functional and non-functional requirements. Many businesses turn to a mobile app cost calculator to estimate how much they will need to spend on their mobile app development project. This tool can provide a rough estimate based on various factors such as platform (Android, iOS), design complexity, app features, and development time.
If you're interested in exploring the benefits of PHP development services for your business, we encourage you to book an appointment with our team of experts.
Book an Appointment
Choosing the Right PHP Web Development Partner in India
As you consider PHP development for your business, it is important to select the right partner. Many Indian companies specialize in PHP development, but finding the right custom PHP website development company in India is key to ensuring the success of your project. Here are a few things to consider when choosing a development partner:
Portfolio and Experience: Look for companies that have experience working on projects similar to yours. A diverse portfolio can help you assess the capabilities of a company.
Client Reviews and Testimonials: Reading client reviews and testimonials can provide insights into the company's reputation and work quality.
Expertise in PHP Frameworks: Ensure that the company has expertise in the latest PHP frameworks and technologies to deliver a modern and scalable solution.
Support and Maintenance: Post-launch support and maintenance are crucial for long-term success. Choose a company that offers reliable support and can handle ongoing updates and issues.
In conclusion, India’s thriving PHP web development industry continues to attract global businesses due to its cost-effectiveness, skilled talent pool, and high-quality solutions. Whether you are building a simple website, an advanced web application, or a mobile app, PHP development in India offers unparalleled advantages. By partnering with the right custom PHP website development company in India, businesses can ensure they stay ahead in the competitive digital landscape.
0 notes
Text
best php Development Company in India | Taction Software
In today’s digital age, having a robust web presence is crucial for businesses to thrive. As one of the most popular server-side scripting languages, PHP is a preferred choice for developing dynamic websites and applications. When it comes to PHP development in India, Taction Softwarestands out as a leading provider, offering expert solutions that align with the latest industry standards and client expectations.
Why Choose Taction Software?
Expertise in PHP Development
Taction Software boasts a team of skilled PHP developers who are well-versed in utilizing the latest PHP frameworks and technologies, including Laravel, CodeIgniter, and Symfony. This expertise allows them to build scalable, high-performing applications tailored to meet diverse business needs. Whether it's a simple website or a complex web application, Taction Software can deliver solutions that enhance user experience and functionality.
Custom Solutions
Every business has unique requirements, and Taction Software understands this well. They specialize in creating custom PHP applications, ensuring that each project is tailored according to the client's specific needs. From e-commerce platforms to content management systems, Taction Software is committed to delivering personalized solutions that drive results.

Agile Development Process
Taction Software follows an agile development methodology, which promotes flexibility and collaboration throughout the development process. This approach enables the team to adapt to changing requirements swiftly, ensuring that projects stay on track and within budget. Regular feedback and iterations help refine the end product, resulting in higher quality software that meets client expectations
Quality Assurance
Quality is paramount in software development, and Taction Software takes it seriously. The company employs a rigorous testing process to identify and resolve any bugs or issues before the final launch. By ensuring that the application functions seamlessly across different platforms and devices, Taction Software guarantees a smooth user experience and minimizes post-launch challenges.
Timely Delivery
In the fast-paced digital landscape, time is of the essence. Taction Software prides itself on its ability to deliver projects on time without compromising on quality. Their commitment to meeting deadlines helps clients get their products to market swiftly, allowing them to leverage opportunities ahead of their competitors.
Post-Launch Support
The relationship with clients doesn't end at project delivery. Taction Software offers comprehensive post-launch support and maintenance services to ensure that applications continue to function optimally. Clients can rely on Taction Software for updates, troubleshooting, and any modifications required as their business evolves.
Client-Centric Approach
Understanding clients’ needs is at the heart of Taction Software’s philosophy. The company invests time in understanding the client’s vision, goals, and challenges, which allows them to provide solutions that truly resonate. Their transparent communication and collaborative approach foster trust and long-lasting partnerships.
Services Offered by Taction Software
Taction Software offers a wide range of PHP development services, including:
Custom PHP Application Development: Tailor-made solutions that align with your business objectives.
E-commerce Development: Building robust online stores with seamless payment gateways and user-friendly interfaces.
CMS Development: Creating dynamic content management systems that empower clients to manage their content effortlessly.
API Development and Integration: Developing and integrating APIs for enhanced functionality and interoperability with other systems.
Web Application Development: Crafting sophisticated web applications that engage users and drive conversions.
Why Outsource PHP Development to India?
India has emerged as a global hub for IT services and software development. Outsourcing PHP development to India, particularly to a trusted company like Taction Software, offers several advantages:
Cost-Effectiveness: Indian IT companies provide high-quality services at competitive rates, ensuring clients get value for their investment.
Access to Skilled Talent: India has a large pool of highly skilled software developers proficient in PHP and other programming languages.
Time Zone Advantage: The time zone differences can be leveraged for round-the-clock development, enabling faster project completion.
Conclusion
When looking for the Best PHP Development Company in India, Taction Software stands out for its commitment to quality, customized solutions, and client-centric approach. With a team of experienced developers and a track record of successful projects, Taction Software is well-equipped to turn your web development ideas into reality. Whether you’re a startup or an established enterprise, partnering with Taction Software can help you harness the power of PHP for your business growth.
Taction Software
Contact Us
INDIA
visit : https://tactionsoftware.com/php-development/
Mobile :- +91-7827150289
Email :- [email protected]
Address :- A-83, Sector-63,Noida, India
(UP) -201301
#php development company#php development services#php web development company#php web development services#php website development services#php development service
0 notes
Text
Who is providing PHP web development services?
PHP (Hypertext Preprocessor) has long been a favored language for web development due to its flexibility, speed, and ease of integration with various database systems. As businesses and developers continue to rely on PHP for creating dynamic websites and applications, the demand for proficient PHP web development services has surged. This article explores who provides these services and highlights why SmartXaaS stands out as one of the premier platforms for PHP web development.
The Landscape of PHP Web Development Services
1. Freelance Developers
Freelancers are a significant part of the PHP web development landscape. Many skilled developers offer their services on platforms like Upwork, Freelancer, and Fiverr. They can work on projects ranging from small websites to large applications. Freelancers often provide personalized service and flexible pricing models, making them an attractive option for businesses with varying budgets.
2. Web Development Agencies
Web development agencies are another key player in the PHP ecosystem. These agencies typically have teams of developers, designers, and project managers, allowing them to handle larger and more complex projects. They often have a portfolio showcasing various PHP applications and websites, providing potential clients with a clear understanding of their capabilities.
3. In-House Development Teams
Many businesses, especially larger organizations, opt to maintain in-house development teams for their PHP projects. This approach allows for greater control over the development process and ensures that the team's expertise aligns with the company's specific needs. However, this option can be more costly, as it involves hiring full-time staff.
4. Outsourcing Companies
Outsourcing companies, particularly in regions like India and Eastern Europe, offer competitive pricing for PHP development services. These firms typically employ a large pool of skilled developers and can deliver projects more cost-effectively than local agencies. However, communication and project management can sometimes be challenging due to time zone differences and cultural factors.
5. Online Platforms and Marketplaces
Various online platforms specialize in connecting businesses with PHP developers. These platforms offer a range of services, from finding individual freelancers to hiring entire teams. They streamline the hiring process, making it easier for businesses to find suitable developers based on their project requirements.
Why Choose SmartXaaS for PHP Web Development?
While there are many providers of PHP web development services, SmartXaaS distinguishes itself through a combination of expertise, innovative solutions, and customer-centric approach. Here are several reasons why SmartXaaS is considered one of the best platforms for PHP web development services.
1. Expertise and Experience
SmartXaaS boasts a team of highly skilled developers with extensive experience in PHP web development. They have successfully completed numerous projects across various industries, from e-commerce to finance. Their expertise enables them to handle complex PHP applications, ensuring high-quality and scalable solutions.
2. Customized Solutions
One of the standout features of SmartXaaS is its commitment to delivering tailored solutions to meet individual client needs. Rather than offering one-size-fits-all services, SmartXaaS takes the time to understand each client's unique requirements and business goals. This personalized approach results in applications that align perfectly with the client's vision and objectives.
3. Adoption of Modern Technologies
SmartXaaS keeps pace with the rapidly evolving tech landscape by adopting modern technologies and methodologies. This includes using frameworks like Laravel, Symfony, and CodeIgniter, which enhance the efficiency and scalability of PHP applications. By leveraging these advanced tools, SmartXaaS ensures that its solutions are not only current but also future-proof.
4. Focus on Quality and Performance
Quality is paramount at SmartXaaS. The platform follows rigorous testing and quality assurance processes to ensure that every application meets high standards of performance and security. This dedication to quality results in robust applications that provide an excellent user experience, reducing the likelihood of issues post-launch.
5. Agile Development Methodology
SmartXaaS employs an agile development methodology, which emphasizes collaboration, flexibility, and customer feedback throughout the development process. This approach allows for iterative improvements and adjustments based on client input, ensuring that the final product aligns closely with their expectations.
6. Comprehensive Support and Maintenance
The relationship between SmartXaaS and its clients doesn't end with project delivery. The platform offers ongoing support and maintenance services, ensuring that applications remain updated, secure, and fully functional. This level of commitment is crucial for businesses looking to maintain their digital presence and adapt to changing market needs.
7. Transparent Pricing and Processes
SmartXaaS believes in transparency when it comes to pricing and project management. Clients receive detailed estimates and regular updates throughout the development process, allowing them to stay informed and in control. This transparency fosters trust and confidence, essential components of successful business relationships.
8. Positive Client Feedback
SmartXaaS has built a solid reputation through positive client feedback and testimonials. Many clients commend the platform for its professionalism, technical expertise, and ability to deliver projects on time and within budget. This track record of satisfaction speaks volumes about the quality of services provided by SmartXaaS.
9. Strong Portfolio
SmartXaaS has a diverse portfolio showcasing its ability to handle a variety of projects, from simple websites to complex web applications. This portfolio serves as a testament to the platform’s capabilities and offers potential clients insight into the kind of results they can expect.
10. Commitment to Innovation
In an industry that constantly evolves, SmartXaaS emphasizes the importance of innovation. The platform continuously explores new technologies, tools, and trends in PHP development to enhance its offerings. This commitment to innovation ensures that clients benefit from the latest advancements in web development.
Conclusion
The demand for PHP web development services continues to grow, driven by the need for dynamic and interactive web applications. As businesses seek skilled providers, the landscape offers various options, including freelancers, agencies, in-house teams, and outsourcing companies. Among these, SmartXaaS stands out as a premier platform for PHP web development services, offering a unique blend of expertise, customized solutions, and a commitment to quality and innovation. By choosing SmartXaaS, businesses can ensure they receive top-notch PHP development services that align with their specific needs and goals, setting the stage for success in the digital marketplace.
#php web development agency#Php web development company#Php web development#website development#website marketing
0 notes
Text
How to Hire Skilled PHP Developers in India: A Complete Guide
Hiring the right PHP developers is critical for businesses looking to build dynamic and scalable web applications, websites or mobile applications. India has become a global hub for PHP development due to its pool of talented and cost-effective PHP developers. Whether you are looking for a full-stack PHP developer India or want to outsource PHP developer India, this guide will help you understand the steps to hire the best.
1. Understanding Your Project Requirements
Before hiring a PHP web developer for hire India, it’s important to define your project’s scope and technical needs. Are you building a custom web application or an e-commerce platform? Do you need ongoing PHP development services India or a short-term collaboration? Understanding your requirements will help you target experienced PHP developers India who specialize in the relevant technologies.
For instance, if you're developing an advanced application with a complex backend, you may require a full-stack PHP developer India who can handle both the frontend and backend. Alternatively, if you're focused on a specific project, you might want to hire dedicated PHP developer India for more concentrated efforts.
2. Benefits of Hiring PHP Developers in India
India is home to some of the best PHP developers in India, offering a wide range of skills and expertise at affordable rates. Indian developers are renowned for their technical proficiency, problem-solving skills, and ability to deliver high-quality code.
When you choose to outsource PHP developer India, you gain access to an India-based PHP development team with a strong understanding of global best practices in web development. The PHP development company India can provide a diverse team of developers proficient in various PHP frameworks like Laravel, Symfony, and CodeIgniter, which can be crucial for PHP application development India.
3. Where to Find Skilled PHP Developers
You can find PHP web development India services through multiple channels:
Freelance Platforms: Websites like Upwork and Freelancer host profiles of remote PHP developer India candidates, where you can evaluate portfolios and reviews.
PHP Development Agencies: Working with a PHP development agency India ensures you get a team of experienced PHP developers India who work under a structured process.
Job Portals: Sites like Google and LinkedIn are ideal platforms for those looking to hire Indian PHP programmers for full-time positions.
4. Qualities to Look for in a PHP Developer
Hiring offshore PHP developers India requires careful evaluation to ensure the candidates align with your project needs. Look for the following traits when selecting a developer:
Technical Expertise: The developer should be proficient in PHP, MySQL, and various frameworks that meet your requirements for custom PHP development services India.
Experience: Choose experienced PHP developers India who have a portfolio of projects similar to your needs. Their experience with PHP web development India will ensure they can deliver a scalable, secure, and efficient application.
Communication Skills: Good communication is essential, especially when hiring a remote PHP developer India. Clear communication will ensure smooth progress and fewer misunderstandings.
Problem-solving Abilities: Your developer should be capable of tackling unforeseen issues during development.
5. Choosing Between Freelancers vs Agencies
When hiring, you can opt for either a freelance PHP web developer for hire India or an established agency. Here's how to decide:
Freelancers: If you're on a tight budget, you can find affordable PHP developers India through freelance platforms. However, freelancers may lack the stability and long-term commitment needed for larger projects.
Agencies: A PHP development agency India provides the advantage of having a team of developers, designers, and testers working together to deliver a holistic solution. This is ideal for larger, ongoing projects where you need comprehensive support from a PHP development company India.
6. Cost Considerations
One of the main reasons companies choose to hire from India is the affordability. While the quality of work is comparable to global standards, cost-effective PHP developers India charge significantly less than their Western counterparts. The cost to hire PHP developer India can vary depending on factors like expertise, project complexity, and engagement model.
For businesses looking to lower development costs without sacrificing quality, PHP developer outsourcing India is a highly effective option.
7. Collaboration Models
When you decide to hire a PHP developer outsourcing India, you can choose from various engagement models:
Full-time Hire: Ideal for long-term projects where you need a dedicated resource to work exclusively on your tasks.
Part-time Hire: Best for projects with a more flexible timeline or limited scope.
Project-based: For companies looking for specific PHP application development India services, this model provides a one-time collaboration where the development team works on a set project with a defined deadline.
8. Ensuring Post-Development Support
After your website or application goes live, ongoing maintenance is crucial to ensure smooth functioning. When you hire a dedicated PHP developer India or work with a PHP development company India, clarify if they offer post-launch support and maintenance services. This will ensure that any bugs or performance issues can be addressed promptly.
Conclusion
India offers a vast talent pool of PHP web developers for hire India who can deliver high-quality work at affordable rates. Whether you're looking to hire PHP developer India for short-term or long-term projects, this guide provides the essential steps to find and evaluate the right candidates. By outsourcing to a reliable PHP development services India provider, you can ensure your project is in skilled hands while saving costs and ensuring timely delivery.
#Hire PHP developer India#PHP development services India#Outsource PHP developer India#Best PHP developers in India#PHP web developer for hire India#Affordable PHP developers India#Experienced PHP developers India#PHP development company India
0 notes
Text
Hire Expert CodeIgniter Developers in Ahmedabad | i-Quall - Your Reliable Development Partner
In today’s competitive digital landscape, businesses need robust, scalable, and efficient web applications to stay ahead. CodeIgniter, a powerful PHP framework, has emerged as a preferred choice for developers due to its simplicity and flexibility. This blog provides a comprehensive guide to hiring dedicated CodeIgniter developers in Ahmedabad, helping you understand why partnering with a professional development team like i-Quall is essential for your business's success.
Ahmedabad, a growing IT hub, has become a hotspot for talented developers, especially in PHP frameworks like CodeIgniter. Businesses across the globe are recognizing the potential of this city and looking to hire dedicated CodeIgniter developers in Ahmedabad for their web development projects. CodeIgniter offers a lightweight yet powerful toolkit for developers, allowing them to create dynamic, feature-rich web applications.
Hiring the right team of CodeIgniter developers can make all the difference when it comes to project execution. With a dedicated development team, you get the advantage of expertise, timely delivery, and an agile approach. i-Quall is a well-known name in Ahmedabad’s tech community, offering top-notch CodeIgniter development services. This blog will take you through a step-by-step process of how you can hire the best CodeIgniter developers in Ahmedabad, ensuring your project’s success.
Step 1: Understand Your Project Requirements Before you start your search for CodeIgniter developers in Ahmedabad, it's crucial to have a clear understanding of your project needs. Are you looking for a simple website, an eCommerce platform, or a custom web application? The clearer your requirements, the better you'll be able to communicate with potential developers. Define your goals, timelines, and the level of expertise needed from the developers. Targeted Keyword: Hire CodeIgniter developers Ahmedabad
Step 2: Look for Experienced CodeIgniter Developers in Ahmedabad Once you have a detailed project outline, it’s time to begin the search. Ahmedabad is home to a vast pool of talented developers specializing in CodeIgniter development. Start by shortlisting companies or individuals with proven expertise in the framework. Check their portfolios and past projects to assess their competency. i-Quall stands out as a reliable development partner with a history of delivering successful CodeIgniter-based projects. Targeted Keyword: CodeIgniter developers Ahmedabad
Step 3: Evaluate Technical Skills Technical expertise is paramount when hiring developers. Make sure your potential CodeIgniter developers in Ahmedabad have a thorough understanding of PHP, MySQL, and MVC architecture, along with proficiency in CodeIgniter. Additionally, familiarity with front-end technologies like HTML5, CSS3, and JavaScript is beneficial. Request for code samples or technical interviews to evaluate their coding standards and problem-solving skills. Targeted Keyword: Hire CodeIgniter developers
Step 4: Consider Communication and Collaboration Effective communication is key to any successful project. Whether you’re located in Ahmedabad or outsourcing from another city, make sure your CodeIgniter developers are able to communicate clearly and frequently. Collaboration tools like Slack, Trello, or Jira can help ensure smooth communication throughout the development process. Targeted Keyword: CodeIgniter developers in Ahmedabad
Step 5: Check for Customization and Scalability Options A significant advantage of working with expert CodeIgniter developers is their ability to create highly customizable and scalable solutions. Ensure the developers can handle future enhancements, updates, and scaling your web application as your business grows. i-Quall, with its extensive experience in CodeIgniter web development, ensures that your platform is flexible and can adapt to changing business needs. Targeted Keyword: i-Quall CodeIgniter developers Ahmedabad
CodeIgniter is often chosen over other PHP frameworks for its speed, flexibility, and easy integration with third-party libraries. For businesses in Ahmedabad looking to develop fast, secure, and dynamic web applications, hiring CodeIgniter developers is the ideal solution. A skilled developer can use CodeIgniter’s built-in libraries and packages to handle tasks like database management, form validation, session management, and more. Additionally, CodeIgniter developers in Ahmedabad who are experienced with APIs can also help integrate your web applications with external services, providing your users with seamless functionality.
Advantages of CodeIgniter Development:
Lightweight framework, ensuring fast loading times
Built-in security features, reducing vulnerabilities
SEO-friendly URL structure
Flexibility to develop custom applications tailored to your business needs
Extensive community support and continuous updates
With i-Quall’s team of expert CodeIgniter developers, you can leverage these benefits to build a robust online presence that meets your specific requirements.
Let’s consider a scenario where you need to develop an eCommerce platform with a custom inventory management system. i-Quall's CodeIgniter developers in Ahmedabad can create a highly customized solution for you by integrating user-friendly interfaces, secure payment gateways, and seamless third-party API integrations like shipping and accounting systems. By utilizing CodeIgniter’s flexibility, the development team can ensure a smooth user experience while maintaining the scalability of the platform to accommodate future growth.
The i-Quall team’s ability to understand complex project requirements and deliver tailored solutions is what sets them apart in the Ahmedabad tech community.
When looking to hire CodeIgniter developers in Ahmedabad, i-Quall stands out as a top choice. With years of experience in PHP development and an impressive portfolio of successful projects, i-Quall provides end-to-end solutions for businesses looking to build secure and scalable web applications. Their dedicated CodeIgniter developers have the expertise to turn your vision into a reality, providing personalized service and ensuring that your project is completed on time and within budget.
Conclusion
Hiring the right CodeIgniter developers in Ahmedabad is essential for the success of your web development project. From understanding your project needs to finding the right team, this guide has walked you through the steps to ensure you make an informed decision. Partnering with i-Quall gives you access to a team of highly skilled and experienced developers who will provide customized solutions that meet your business goals.
With their deep expertise in CodeIgniter development, i-Quall is ready to take on your next project and deliver outstanding results. Whether you're building an eCommerce site, a custom application, or upgrading an existing platform, i-Quall Infoweb is the ideal partner for your web development journey in Ahmedabad.
URL : https://www.i-quall.com/ahmedabad/codeigniter-developers-ahmedabad-for-hire/
0 notes
Text
Why should you choose AppsRhino For PHP Based Projects?
A PHP web developer creates the server side of the website by employing a large number of libraries and frameworks PHP offers. It is user-friendly, easy to integrate, and is supported by almost all hosting servers.
When you have a project that's crucial to your organization and cannot risk making a mistake, you need a partner who has got your back.
While hiring a PHP web developer, you must remember that when you sit together and discuss, you will speak to a person, not their skills.
Outsourcing is probably the best when you want to hire a PHP coder. You get dedicated, seasoned developers. Our expert team shortlists the best candidates per your needs and budget. If you are considering hiring freelancers, note that they are comparatively less safe and less dedicated. They might be working on multiple projects simultaneously, which may lead to data breaches. When you outsource from us, you get a dedicated PHP web developer.
A good PHP developer does more than just write web page code, test it, and maintain it. PHP web developers may also work on projects of various complexity. This requires a high level of analytical and problem-solving skills.
A proficient PHP developer collaborates with other trained specialists to produce different programs and debug when necessary. A good developer is also conversant with most PHP frameworks, such as Laravel, Yii, Codeigniter, Zend, Symfony, Kohana, CakePHP, and Joomla.
Hiring capable and enthusiastic PHP developers involve thoroughly understanding the company's requirements. When dealing with the top PHP developers, keep in mind that they must adhere to a strict method.
Understanding the benefits of hiring a PHP web developer is critical to identify the best fit for your company. Knowing PHP and its related frameworks through and out is vital when selecting the right software developers who will operate securely and efficiently for a project.
0 notes
Text
Hire Full Stack Developers to manage your ongoing projects
Are you looking to hire full stack developers at onsite or remote? Connect and find expert full stack programmers to develop a prototype or an enterprise-grade web application with rapid deployment at a 40% lower cost. Our full stack coders for hire, are available on hourly or full time on TnM - time and material model. If you are looking to hire full stack developers or to set up a team Fullstack experts including PM, senior developers and fullstack developers - LETS TALK!!!
>>Explore Hiring Options - https://www.provab.com/hire-fullstack-developers.html
Provab Technosoft offers a dedicated team of full stack developers with expertise in all major frameworks viz Zend, Laravel, CodeIgniter, CakePHP, Symfony among others. These developers also possess strong proficiency in multiple industry verticals and can respond to functional know-how of these industries. Our dedicated fullstack programmers work as an integral part of customer’s product development team and work as full-time staff or ad-hoc basis as per the need.
Provab has a team of 300+ professionals and its dedicated full stack application services team follows the agile methodology to bring the customer idea into reality by deploying web portals, enterprise web applications, intranet systems and custom applications.
Developers in Bench -
https://www.provab.com/hire-bench-developers.html
PROVAB’s Full Stack Web Development Services
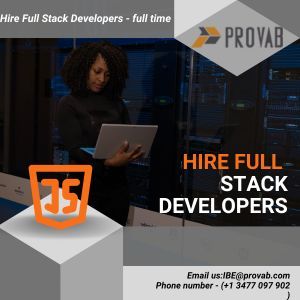
Full stack developers at PROVAB hold extensive experience in everything from back-end to front-end database and thus deliver best in class solutions to the clients. Our professional full-stack programmers are well-versed in Fullstack to MEAN Stack, Node.js, Angular.JS, Ruby on Rails, ReactJS, Laravel, and others. Our experts have unrivaled experience in the full-stack software development field.
1. Full Stack Application Development
Custom full stack application development gives you greater control over code and implementation. Hire Full Stack developers at PROVAB to set your team.
2. Full Stack ECommerce Development
Trust our experienced full-stack programmers with Fullstack based e-commerce development to build and deploy high-performance online storefronts for any business.
3. Product Development In Full-stack
Outsource your product development from ideation to delivery. Hire Full Stack developers offshore and get your product done under work for hire agreement.
4. Booking Engines In Fullstack
Outsource your booking engine development from ideation to delivery. Hire Full Stack developers offshore and get your booking portal done under work for hire agreement.
Advantage of hiring remote web developers
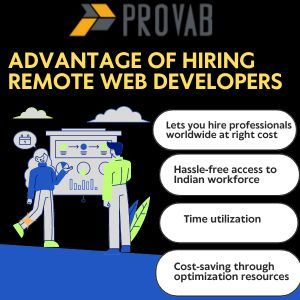
Lets you hire professionals worldwide at right cost
Hassle-free access to Indian workforce
Time utilization
Cost-saving through optimization resources
Higher retention rates with short, mid-term and long term assignments
There are certain clear advantages of hiring top web & mobile app developers in India, which is now a hub of all leading IT / software companies.
Flexibility
Nowadays most of the company functions as a R.O.W.E (Result Only Work Environment), but some still have a stronghold on the old fashioned only working apart from any other activities. But if you are looking to hire top talent, try to lose the traditional workplace as much as you can. The great way to pull the attention of new talent to your company is by listing your remote company's flexible work policy in its job description.
Where to look
To source remote app developers who can be right for your company, look for specialized sites that cater to your platform-specific app developers like PHP, JavaScript, .NET. Node.Js, AngularJS. I suggest Linked.in to be the best site where you can find key roles and responsibilities of app and web app developer explained properly. You can also find certification done, as well as its day to day industry update activities to judge its skills.
Setup A Web & App Team in India -
Seek out their soft skills
Education, experience and technical knowledge is a common thing found in every candidate. Instead, I'll suggest HRs' to look for soft skills i.e., time management, tech-savvy and strong communicator that is necessary to work remotely.
How tools plan a major role to define superior productivity while working remotely
Using online tools such as project management software, collaborative creation tools and more help you to feel comfortable and saves time while communicating with the expert teammates. The alternative saves you time and allows you to majorly concentrate on app development. The software schedules meetings regularly, phone calls and also a creation of work reports. It notifies regular updates on call and progress reports. The software also assigns tasks, and a deadline of it so that it will be meaningful, creative and important to you.
How to optimize the performance of remote app development team in India?
Take Interview: A remote developer is a judge based on how efficiently and timely communication and tasks are handled.
Sign Legal Agreement: Agreement such as NDA (Non-Disclosure Agreement) and other needful as per the client requirements.
Rapid Communication Tools: Remote developers should know how to handle communication software and also project management tools to help clients to see their progress with screen sharing, add tickets, keep track of work, etc.
Define Task for Development Team: Assign a project manager to take a regular look at the project, provide reports and updated regularly.
Work Tracking Software: The software would provide a complete detailed report of the working hours, coding, and time is taken. This will both parties to have an idea on the on-going development.
Manage HR Policy: Usually, HR policy doesn't include timing, leaves, break time, etc.
Conclusion:
Remote full stack developers have a very good working relationship with various companies across India and also deal with multiple clients at any one time. Years full of professional learning, travel, personal life, and new experience provide them with involved exposure, which gives a clear way to new ideas. It has been seen that remote app programmers are more inspired, passionate and motivated about their work as flexibility plays a vital role in the working environment. Hiring a remote app developer can be a little difficult, but it is also a major step to reduce onboard expenses cost your business.
#full stack developer#web developer#hire full stack developer#marketing#tech developer#technology developers
1 note
·
View note
Text
Hire CodeIgniter Developer: Unleashing the Power of Versatile Web Development.
In today's fast-paced digital environment, selecting the appropriate web development framework is vital. One framework that has gained a lot of recognition is CodeIgniter. As more companies realize the advantages of CodeIgniter, the need for highly skilled developers within the framework has increased. We'll discuss the various aspects of hiring CodeIgniter developers and the reasons why it's a smart move for companies of all sizes.
Why Hire a CodeIgniter Developer?
The advantages of using CodeIgniter in web development
CodeIgniter is a popular choice due to its ease of use and lightweight. It is a great platform for creating robust web-based applications. Its MVC (Model-View-Controller) architecture streamlines the development process, ensuring efficiency and maintainability.
The requirement for expertise in the field
Although CodeIgniter is user-friendly, unlocking its full potential requires a professional. Employing a committed CodeIgniter developer will ensure that your project gets optimized code, a fast cycle of development, and a flexible architecture.
Qualities to Look for in a CodeIgniter Developer
Expertise and technical skills in CodeIgniter
A competent developer must show proficiency in PHP, the language that is the foundation of CodeIgniter. Experience with MVC architecture, as well as database management and third-party integrations, are vital.
Skills for problem-solving
Effective developers aren't just coding; They solve issues. It would help if you looked for candidates who can overcome obstacles, optimize code, and improve the overall effectiveness in the process of development.
Teamwork and communication
In the world of collaboration in web development, efficient collaboration and communication are essential. The developer you choose should seamlessly join your team to ensure a smooth workflow and positive project results.
Where to Find CodeIgniter Developers
Online platforms for hiring developers
Numerous online platforms, like Upwork and Toptal, offer an array of skilled CodeIgniter developers. Check these platforms out to find developers who possess the expertise the project needs.
Outsourcing vs. in-house hiring
Choose whether to hire an outside contractor or internally based on the requirements of your project and budgetary constraints. Outsourcing can result in savings, while hiring in-house allows you to control your development processes.
Hiring Process and Best Practices
Making a compelling job description
It is essential to clearly define the abilities and experience you're looking to acquire in the job description. This helps potential applicants be aware of the requirements and only apply if they meet the requirements.
Conducting successful interviews
Create interview questions that test both technical abilities as well as problem-solving capabilities. Practiced coding exercises and scenario-based questions can offer valuable insights into a candidate's abilities.
Examining the CodeIgniter's abilities and experiences
Ask for examples of past CodeIgniter projects and evaluate the quality of the code, the adherence to the highest standards, and the overall performance of the project.
CodeIgniter Developer Rates
Understanding the cost-related factors
Developer rates for CodeIgniter vary according to the developer's experience, the developer's location, and the complexity of the project. Take these considerations into account when creating the budget that best suits your goals for the project.
The balance between quality and budgetary factors
Although cost is an important aspect, you should consider the quality of your. A proficient CodeIgniter developer can charge more, but they can produce the most efficient and effective project, which ultimately saves money in the long term.
Benefits of Hiring a Dedicated CodeIgniter Developer
Flexibility and customization
The dedicated developers can provide a great degree of personalization, tailoring their approach to meet your specific project's requirements. This allows you to ensure that your software meets your specific requirements for business.
Faster development cycles
A dedicated developer who is who is focused on the project, development times are accelerated. This means a faster time-to-market, which is essential in today's highly fast-paced business environment.
Real-world Success Stories
Examples of projects that have been successful using CodeIgniter
Present real-world projects that have had the success they have achieved using CodeIgniter. Highlight the positive impact on business as well as the satisfaction of customers who have gained from the capabilities of the framework.
Impact on the business and client satisfaction
Show how CodeIgniter has significantly influenced the business outcome, with a focus on customer approval and helping to achieve goals for projects.
Challenges in Hiring CodeIgniter Developers
Common hiring obstacles
Recognize the common issues in finding CodeIgniter developers, for example, competition on the job market and the requirement for current abilities. Create strategies to get over these obstacles.
Risk mitigation during your hiring procedure
Provide a list of steps to reduce risks during recruitment, like thorough screening references, reference checks, and a trial period to determine the compatibility of your team.
Continuous Learning and Adaptation
The changing web development landscape
Recognize the dynamism of web development and stress the importance of continual learning. CodeIgniter developers must stay up-to-date on the most recent developments and technology to stay efficient in their work.
It is important to stay up-to-date in CodeIgniter.
Encourage developers to participate in ongoing learning, go to conferences, and join and participate in the CodeIgniter community to stay informed of the latest developments and improvements to the framework.
Future Trends in CodeIgniter Development
New technologies with their implications
Discuss the upcoming trends and technologies that could impact CodeIgniter development. Be sure to highlight how staying at the forefront of these trends will make sure that projects are future-proof and will ensure long-term success.
How CodeIgniter's relevance remains
Discover the versatility of CodeIgniter and discover how it will continue to improve to meet the requirements of the modern web. It is a trusted option for companies looking to the future.
Conclusion
In the end, employing a CodeIgniter developer is a smart option for companies seeking effective, customizable, scalable, and flexible web-based development solutions. By evaluating the strengths and abilities of potential developers, creating a streamlined hiring procedure, and understanding the advantages of a dedicated expert, companies can tap into their fullest potential with CodeIgniter.
FAQs
Q1. What exactly is CodeIgniter, and what makes it so popular?
A. CodeIgniter is a PHP framework that is known for its speed, simplicity, and lightweight. Its popularity is due to its simplicity of use, its efficient development process, and the widespread support of its community.
Q2. What can I do to assess the capabilities of a CodeIgniter Developer?
A. Assess a developer's abilities by reviewing the previous CodeIgniter projects, conducting technical interviews, and assessing their problem-solving skills by using practical exercises.
Q3. Are there specific industries that could benefit from the CodeIgniter platform?
A. CodeIgniter is versatile and applies to a range of sectors, such as e-commerce, finance, healthcare, and much more. Its versatility makes it ideal for a variety of tasks.
Q4. Can I employ CodeIgniter specialists on a per-project base?
A. Yes, many developers are willing to provide service on a contract basis. Platforms such as Upwork or Toptal are great resources for finding skilled CodeIgniter developers for short-term projects.
Q5. What is it that makes CodeIgniter the most popular option in web-based development?
A. CodeIgniter's simplicity, speed, and flexibility make it a top option. Its MVC architecture makes development easier and provides a scalable platform for developing custom web-based applications.
0 notes
Text
A Comprehensive Guide To Hire Dedicated PHP Developers!
The demand for skilled PHP developers is steadily increasing in today's digital landscape. With PHP being a popular programming language for web development, businesses must find dedicated PHP developers who can bring their projects to life. Hiring dedicated PHP developers offers numerous advantages, including specialized expertise, cost-effectiveness, and efficient project delivery. In this comprehensive guide, we will walk you through the essential steps to successfully hire dedicated PHP developers and ensure the success of your web development projects.
Assessing Your Project Requirements
Before diving into the hiring process, assessing your project requirements is essential. Define your project goals, scope, and timeline to understand your aim clearly. Determine the specific skill sets and expertise required for your PHP development needs, such as experience with popular PHP frameworks like Laravel or CodeIgniter. Additionally, consider the size of your development team and the level of experience desired, as this will help you identify suitable candidates who can seamlessly integrate into your project.
Finding Reliable PHP Developers
To find reliable PHP developers, explore online job portals and freelancing platforms catering to the IT industry. These platforms often have a vast pool of PHP developers showcasing their skills and experience. Another valuable approach is seeking recommendations from industry peers, colleagues, or professional networks. They can provide insights into developers they have previously worked with or recommend reputable PHP development companies or IT outsourcing firms. Partnering with a trusted PHP development company can save you time and effort in recruitment and ensure access to a reliable talent pool.
Evaluating PHP Developer Candidates
When evaluating PHP developer candidates, review their resumes, portfolios, and past projects carefully. Please pay attention to their experience with PHP development, the complexity of the projects they have worked on, and the outcomes achieved. Conduct technical interviews and coding assessments tailored to your project requirements to assess their PHP skills. Evaluating the PHP developer candidates will help you gauge their problem-solving abilities, communication skills, and teamwork capabilities, which are vital for successful collaboration.
Assessing Cultural Fit and Communication Skills
Assertive communication is crucial for effective collaboration with PHP developers, especially when working remotely or across different time zones. Evaluate the candidates' proficiency in English, as it plays a significant role in seamless communication. Consider cultural compatibility as well, as it ensures smooth interactions and an understanding of business practices. Assess the candidates' ability to adapt to different work environments and work harmoniously with your existing team.
Considering Experience and Expertise
When evaluating PHP developer candidates, their experience and expertise are key factors. Look for candidates with a solid background in PHP development, including experience with relevant frameworks and technologies. Assess their knowledge of database management systems, such as MySQL or MongoDB, and familiarity with frontend technologies like HTML, CSS, and JavaScript. The more diverse and comprehensive their skill set, the better equipped they will be to handle your project requirements.
Understanding Dedicated Hiring Models
Explore different hiring models to determine which one best suits your project needs. Full-time engagement ensures dedicated focus and availability, ideal for long-term projects. Part-time or freelance engagements can be suitable for smaller projects or specific tasks. Project-based engagement allows you to hire PHP developers per project, providing flexibility and cost-effectiveness. Assess the advantages and limitations of each hiring model based on your project requirements, budget, and preferred level of control and engagement.
Ensuring Quality and Professionalism
To ensure the quality and professionalism of the PHP developers you hire, check references and reviews from previous clients. Quality assessment will give you insights into their reputation and work ethics. Assess their ability to adhere to project deadlines, deliver high-quality code, and provide ongoing support. A dedicated PHP developer should demonstrate a solid commitment to delivering exceptional results and ensuring client satisfaction.
Negotiating and Finalizing the Contract
Once you have identified the right PHP developer(s), it's time to discuss the terms, conditions, and project milestones. Engage in open and transparent negotiations to establish a mutually beneficial agreement. Discuss the pricing, payment structure, and any additional clauses, such as confidentiality agreements, to protect your project's sensitive information. Finalize the contract and ensure that both parties are in alignment before moving forward.
Onboarding and Collaboration
Successful collaboration with dedicated PHP developers starts with a smooth onboarding process. Establish clear communication channels and project management tools to facilitate effective collaboration. Provide the necessary project documentation, guidelines, and access to required resources to ensure the developers have everything they need to get started. Foster a supportive and inclusive work environment to ensure the successful integration of the dedicated PHP developer(s) into your existing team.
Managing the Development Process
Implement project management methodologies and agile practices to streamline the development process. Conduct regular progress meetings to track progress and provide feedback to ensure alignment with project goals. Foster open communication channels to promptly address any challenges or modifications required during the development phase. Regularly assess the project's progress to ensure it stays on track and meets the desired outcomes.
Conclusion
In conclusion, hiring dedicated PHP developers is crucial to achieving successful web development projects. By following this comprehensive guide, you can confidently navigate the hiring process, assess candidates effectively, and select the right PHP developers for your specific project requirements. Emphasize the importance of selecting candidates with the right skills, experience, cultural fit, and communication abilities to ensure a fruitful collaboration. By investing time and effort into hiring dedicated PHP developers, you are paving the way for successful PHP development outcomes and driving the growth of your business.
0 notes
Text
Web Development Companies in London and the UK: Choosing the Right Partner for Your Business
In today's digital age, businesses need a strong online presence to compete in the market. This requires a well-designed website or web application that is optimized for user experience, performance, and security. However, developing and maintaining a website or web application requires specialized skills and expertise, which is why many businesses turn to web development companies for help.
web development agency in uk
London and the UK are home to some of the best web development companies in the world. These companies specialize in web design, software development, and ecommerce website development, among other services. Whether you are a small startup or a large enterprise, finding the right web development partner can make all the difference in achieving your business goals.
When searching for a web development company, there are several factors to consider. Firstly, you need to ensure that the company has a good track record of delivering high-quality projects on time and within budget. You can check their portfolio and read client reviews to get a sense of their capabilities and approach.
progressive web applications london
It's also important to consider the company's expertise and experience in the specific areas of web development that you require. For example, if you need an ecommerce website, you should look for a company that specializes in ecommerce website development. Similarly, if you require custom software development, you should look for a company with expertise in that area.
wordpress maintenance services
Outsourcing software development is another option that many businesses consider, especially those that do not have in-house development teams. Outsourcing can provide cost savings and access to a larger talent pool, but it also requires careful planning and management to ensure that the project is delivered successfully. When outsourcing, you should look for a company that has experience in working with remote teams and can provide clear communication and project management processes.
One of the most popular services offered by web development companies is ecommerce website development. With the growth of online shopping, businesses need to have a strong ecommerce presence to reach customers and drive sales. Ecommerce website development companies specialize in developing websites that are optimized for online transactions, with features such as shopping carts, payment gateways, and order management systems.
ecommerce website development company
PHP development is another specialized service offered by web development companies in the UK. PHP is a popular programming language used for developing dynamic websites and web applications. PHP development companies specialize in developing websites and applications using PHP frameworks such as Laravel, CodeIgniter, and Symfony.
custom software development
In conclusion, choosing the right web development partner is crucial for businesses looking to establish a strong online presence. Whether you are looking for a web development company in London or anywhere else in the UK, you need to consider factors such as expertise, experience, and track record when making your decision. With the help of a professional web development partner, you can develop a website or web application that meets your business goals and helps you stay ahead of the competition.
0 notes
Link
We will evaluate here why the PHP is better than other PHP frameworks here by considering our own web application development knowledge and what the other developers say why to use Codeigniter?
Codeigniter is open source development framework which follows the MVC framework pattern. Its goal is to give you the easy and proper way to develop the project much faster than you could do.
Reasons why CodeIgniter is better than other PHP Frameworks:
1. Execution Time
2. File Organization
3. Configuration
4. Security
5. Less Code and faster development
6. Community Support
7. Easy Error Handling
8. Step by Step Testing With Development Phase
9. Easy Template Solution
10. Codeigniter Cache Class
Stellen Infotech is a CodeIgniter Development Outsourcing Company in India, provides a Custom CodeIgniter Framework, PHP Programming, PHP outsourcing services to get better result in business. If you are thinking to get more result for your business, then development is only the way, through which you can get more chances to express your business globally.
#codeigniter php web application development services#custom codeigniter development company#codeigniter development company#codeigniter development#codeigniter development outsourcing#offshore codeigniter development company india#codeigniter framework#outsource codeigniter development
1 note
·
View note
Link
Node js in 2021 looks like a massive trend that is going to evolve even more. It offers some undeniable advantages that make it the right choice for software developers. Get new tech ideas and insights, read the latest news in the tech industry: https://lnkd.in/dxsR59t See our exhaustive analysis to make the best choice for your next upcoming project. Our Node.js specialists not only conduct lots of successful Node.js software projects. They also conduct training, present at IT conferences, organize online webinars and write insightful articles about Node.js.
#nodejs#software defined storage#software#developers & startups#business#hire codeigniter developer#hire python developers#hirededicateddevelopers#california#outsourcing software developers
1 note
·
View note
Link

What is CodeIgniter Framework?
CodeIgniter is based on the popular MVC (model–view–controller) development pattern. As controller classes are a necessary part of development under CodeIgniter, models and views are optional. CodeIgniter is most often noted for its speed when compared to other PHP frameworks.
Why Choose CodeIgniter Development Services for Web Development?
Security: CodeIgniter is very secure with built-in protection against CSRF and XSS attacks.
Easy Customization: Developers can easily customize existing libraries, write new libraries, and use shortcodes in CodeIgniter
Community Support: With over 57000 registered members on the CodeIgniter forum Less Coding, Faster Development: Requiring shorter and fewer codes in this PHP framework
Performance: CodeIgniter is lightweight and performs greatly.
What you can do with CodeIgniter Service from Capital Numbers?
1) Maintenance and Support 2) Cloud Solutions 3) CodeIgniter Application Development 4) CodeIgniter Web Development 5) Automated Testing 6) Custom Database Development 7) Migrate from other application to CodeIgniter without losing data
So, Why Choose Capital Numbers for CodeIgniter Development?
With Capital Numbers, you can:
Increase your profitability by over 50%.
Hire qualified developers and get guaranteed results.
Chat with your developer instantly on a day-to-day basis.
Start in 24 hrs, no minimum commitments.
Work with a team at a modern, secure, Google® verified office.
Visit our website today!
#Hire-CodeIgniter-Developers#CodeIgniter-Application-Development#Remote-CodeIgniter-Development-Service#Outsource-CodeIgniter-Development#Expert CodeIgniter Team#Capital Numbers CodeIgniter#CodeIgniter development service#CodeIgniter Web Development
0 notes
Text
Outsource CodeIgniter Developer
SiliconValley is a leading Codeigniter web development company in India providing CodeIgniter framework development solutions for PHP web development and apps. CodeIgniter development team creates dynamic web sites with the popular model–view–controller (MVC) development.
Our Service in Codeigniter:-
We are specialized in PHP based projects & having experienced team of PHP programmers.
PHP developers can easily perform the migration from one server to another as well as easy installation.
Website: https://www.siliconinfo.com/php-web-programming-development-design/usa-php-developer-programmer.html
E-mail:[email protected]
#OutsourceCodeIgniterProgrammer#OutsourceCodeIgniterDeveloper#OutsourceCodeIgniterDevelopment#CodeIgniterProgramming#CodeIgniterProgrammer#CodeIgniterDevelopment#CodeIgniterDeveloper#SiliconValley
1 note
·
View note
Text
Custom Development - The Best Way To Take Your Business To The Next Level!
Businesses, today, need to overcome a plethora of challenges in order to beat their competition. With the advent of technological era, it has become easier for business owners to create a strategic advantage for themselves. And, Custom Web Application Development certainly plays a pivotal role in achieving this objective. Enterprises need to rely on powerful custom web applications to meet their specific business requirements & target objectives. These applications are built using a variety of dynamic open source frameworks such as PHP, CakePHP, Symfony, CodeIgniter, Zend etc.
With the help of services such as PHP Development, businesses can get dynamic and 100% tailored web applications that help cut down operational expenses and streamline various activities. One can also get dynamic database driven websites by leveraging the benefits offered by PHP & MySQL technologies. When you go for a custom web app for your organization, it not only helps improve day to day efficiency but also enhances the actual return on investment too. You just need to outsource your custom web application project to a reliable company and that's it! Your things will be taken care in a meticulous manner.
Custom web applications such as a CRM tool or online content management system can do wonders for your business enterprise. Take for instance, SugarCRM based web applications. These help owners streamline complex customer information at one place. Managers can easily access data with just a few clicks and that's it! These advanced web applications also help you to monitor various sales, marketing and promotional activities.
Talking about custom cms applications, these allow you to have full control over online content. There is various open source software available for this such as WordPress, Drupal and Joomla. Customizing these software applications as per your business needs can bring in huge benefits. For instance, WordPress helps you run a custom blogging application to promote products and services. You can even use it to start your own affiliate network. Same is the case with Drupal or Joomla!
Besides custom web applications, these days, mobile applications have also become indispensable for businesses. These help reach a wider target audience and improves the overall brand awareness too. With Android Application Development solutions, you can get powerful business apps for mobile devices that help market products & services in a cost-effective yet professional manner. The demand for custom mobile apps, in recent years, has witnesses a lot of surge. Thanks to the introduction of smart phones such as iPhone, Blackberry, Windows, Symbian, Android and lot more.
With custom web solutions including Magento Development, internet merchants can set up elegant ecommerce shops exactly as per their marketing requirements. These online stores allow customers to shop in a seamless manner without worrying about the security aspects. Moreover, these days, developers include additional custom functionalities such as the ones based on AJAX to offer customers a powerful online experience. They even integrate social media platforms with your online stores so that customers can share, recommend, like and discuss about your products on the virtual platform. All this would not have been possible without custom development services.
Using Custom Web Application Development services from a professional organization, you can not only save your cost but also time. These companies used well-defined methodologies and advanced project management techniques to deliver your projects on time and within budget. All you need to do is some secondary research in identifying & selecting the right organization which listens & understands to your online branding goals. Their team of web application developers will do the needful in a very short span of time.
Read more here about David Stoccardo Custom Development .
1 note
·
View note