#cpp pointers
Explore tagged Tumblr posts
Text
Pointers in C++ are simple to learn but very useful for building programs. Learn these to manipulate memory in C++:
Pointer Arithmetic
Array of Pointers
Passing Pointers to Functions
Return Pointer from Functions
Source: Tutorialspoint
0 notes
Text
CodeBeauty
POINTERS introduction
#include <iostream> using namespace std; int main(){ /* int n = 5; cout << n << endl; output: 5 */
// is for a comment line and /**/ is for multiple line comments //I make it like this so you can paste it immediately into a compiler //variable - &address - *pointers. //START //just like variable, a pointer is a container as well, storing an address (a memory location) //n is a variable meaning that it is a container storing certain value //because it's a container, it has it's address inside the memory, a physical location //in order to check the location / which address :
/* int n = 5; cout << &n << endl; output: 006FFD64 */
//because it's not always fun to remember long address numbers, so we give them names - variables. we create a pointer to name an address and easily access it.
//POINTERS //how to create a pointer holding address of our n variable:
int n = 5; cout << &n << endl;int* ptr = &n; cout << ptr <<endl; //in order to indicate that you're creating a pointer, use * star (int*) //then give it a random name (ptr) //assign it (to ptr) the address of our n variable which is = &n (= assign)(& address)(n variable) cout << *ptr << endl; // a star * before the pointer's name will only reference the pointer and then find the VALUE of the ADDRESS //which is our original variable n //basically we're asking for the value 5, not the address 006FFD64 //CHANGE THE VALUE (5) stored in the pointer ptr address: *ptr = 10 ; //a star * means access the memory location and (=)assign a NEW (10) value //let's check if it's now 10 instead of 5 cout << *ptr <<endl; cout << n ; //both *ptr and n now show 10
//NOTES //pointer has to be of the SAME TYPE as the variable it is pointing to //int pointer to int variable, not int to float //char pointer to char variable, bool pointer to a bool variable, etc //you can't assign a value to a pointer, it has to point to an address //first create a (example) variable to store that variable's & address to the pointer //pointers are problem solvers and not the casual way it's used in these examples system("pause>0"); return 0; }
#I make it like this so you can copy it into a compiler#codebeauty#learn c++#studyblr#studyinspo#studyspo#coding#pointers#cpp#c plus plus#c++#c++ language#c++ programming#computer science#cppprogramming#learn coding#learn cpp#learn to code#study coding#programming#learn#study
3 notes
·
View notes
Text
0 notes
Text
C language MCQ . . . . write your answer in the comment section https://bit.ly/48J8x0O You can check the answer at the above link at Q.no. 1
#c#cpp#programming#arrays#programminglanguage#pointers#java#python#computerscience#computerengineering#javatpoint
0 notes
Text
discovering that changing a file's extension from .cpp to .c makes visual studio interpret it as strict C instead of C++, which fills my head with possibilities for doing pointer crimes.
2 notes
·
View notes
Text
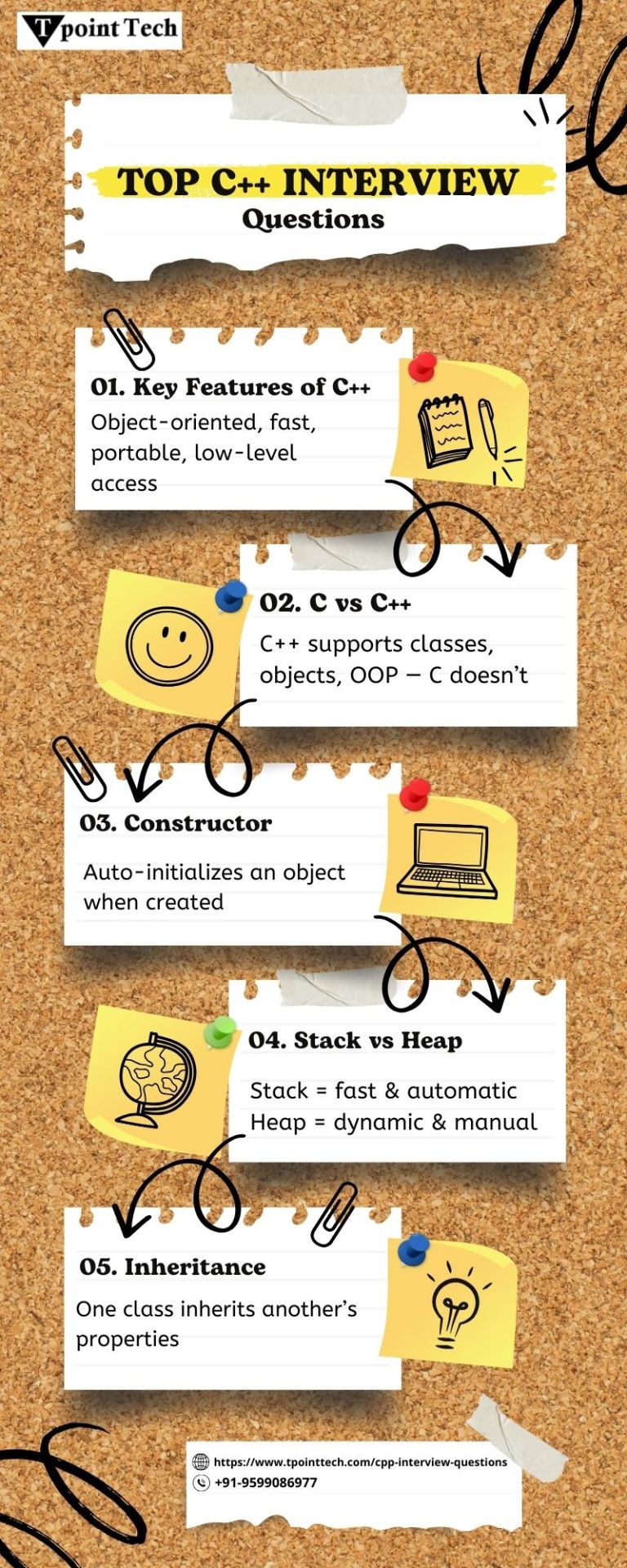
Top C++ Interview Questions
Preparing for a C++ interview? Knowing the top C++ interview questions can help you stand out. These questions cover essential topics like object-oriented programming, memory management, inheritance, constructors, pointers, virtual functions, and more. Interviewers often focus on both theoretical concepts and practical understanding of how C++ works. By reviewing key questions, you can strengthen your grasp of the language and confidently explain core features. Mastering these topics is crucial for landing roles in software development, game programming, or systems engineering.
For more information and interview questions, you can also visit Tpointtech, where you can find many related topics. Contact Information:
Address : G-13, 2nd Floor, Sec-3, Noida, UP, 201301, India
Mobile: +91-9599086977
Email: [email protected]
Website: https://www.tpointtech.com/how-to-split-strings-in-cpp
0 notes
Text
youtube
ESP8266
Visual Studio, CPP
Modul szeparáció, pointer, refernce (*, &)
0 notes
Text
From Zero to C++ Hero: The Ultimate Beginner’s Guide to Mastering C++ If you're reading this, chances are you're ready to dive into the world of programming and have chosen C++ as your starting point. Congratulations! C++ is a powerful, versatile language used in everything from game development to high-performance computing. But where should you begin? Learning C++ can seem daunting, especially if you're a beginner. Don't worry—this guide will walk you through the best ways to learn C++, step by step, in a way that’s engaging and effective. Why Learn C++? Before we dive into the how, let’s talk about the why. C++ is one of the foundational programming languages. It’s known for its: Performance: C++ is a compiled language, making it faster than many others. Versatility: It’s used in various domains like game development, operating systems, and even financial modeling. Career Opportunities: Mastering C++ can open doors to lucrative job roles. Understanding why C++ matters will keep you motivated as you tackle its complexities. Step 1: Get Familiar with the Basics 1.1 Understand What C++ Is C++ is an object-oriented programming language. It builds on C and introduces features like classes and inheritance, which make it powerful yet complex. 1.2 Install a Compiler and IDE Before writing any code, you need the right tools: Compiler: Popular choices include GCC and Clang. IDE: Visual Studio Code, Code::Blocks, or CLion are beginner-friendly. 1.3 Learn Syntax Basics Start with: Variables and data types Loops (for, while) Conditionals (if, else if, else) Functions There are plenty of beginner tutorials and videos to guide you through these. Step 2: Use Structured Learning Resources 2.1 Books "C++ Primer" by Stanley Lippman: Great for beginners. "Accelerated C++" by Andrew Koenig: Ideal for fast learners. 2.2 Online Courses Platforms like Udemy, Coursera, and Codecademy offer interactive courses: Look for beginner-friendly courses with hands-on projects. 2.3 Tutorials and Documentation The official C++ documentation and sites like GeeksforGeeks and Tutorialspoint are excellent references. Step 3: Practice, Practice, Practice 3.1 Start with Small Projects Begin with simple projects like: A calculator A number guessing game 3.2 Participate in Coding Challenges Platforms like HackerRank, LeetCode, and Codeforces offer challenges tailored for beginners. They’re a fun way to improve problem-solving skills. 3.3 Contribute to Open Source Once you're comfortable, contributing to open-source projects is a fantastic way to gain real-world experience. Step 4: Learn Advanced Topics As you gain confidence, dive into: Object-oriented programming (OOP) Data structures and algorithms File handling Memory management and pointers Step 5: Build Real-World Projects 5.1 Why Projects Matter Building projects will solidify your skills and make your portfolio stand out. 5.2 Project Ideas Simple Games: Like Tic-Tac-Toe or Snake. Basic Software: A to-do list or budgeting app. Algorithms Visualization: Create a visual representation of sorting algorithms. Step 6: Join Communities and Stay Updated 6.1 Online Forums Participate in forums like Stack Overflow or Reddit’s r/cpp to ask questions and share knowledge. 6.2 Meetups and Conferences If possible, attend local programming meetups or C++ conferences to network with professionals. 6.3 Follow Experts Stay updated by following C++ experts and blogs for tips and best practices. Tools and Resources Round-Up Here are some of the best tools to boost your learning: Compilers and IDEs GCC Visual Studio Code Online Platforms Codecademy HackerRank Books "C++ Primer" "Accelerated C++" Final Thoughts Learning C++ is a journey, not a sprint. Start with the basics, practice consistently, and don’t hesitate to seek help when stuck.
With the right resources and determination, you’ll be writing efficient, high-performance code in no time. Happy coding!
0 notes
Text
Understanding C++ cstring and the strcat() Function
In C++, working with strings is a common task that requires knowledge of string manipulation functions. One of the fundamental functions used in string manipulation is strcat(), which is part of the C Standard Library and is available in C++ cstring strcat(). The strcat() function is used to concatenate two null-terminated C strings (arrays of characters).
What is strcat()? The strcat() function is defined in the library, and it stands for "string concatenate." It appends one string to the end of another. The function modifies the first string (destination) by appending the second string (source) to it.
Function Syntax cpp Copy code char* strcat(char* destination, const char* source); destination: A pointer to the destination string where the source string will be appended. source: A pointer to the source string that is to be appended to the destination string. The function returns a pointer to the destination string after the concatenation.
Key Characteristics of strcat() Null-terminated strings: Both strings must be null-terminated, meaning they must end with a '\0' character. Without the null terminator, the function may lead to undefined behavior. Modifies the destination string: The strcat() function does not create a new string; instead, it modifies the content of the destination string. Overwrites memory: Ensure that the destination string has enough space allocated to accommodate both the original content and the new content being appended. Example Code Here is an example demonstrating how to use strcat() in C++:
cpp Copy code
include
include
int main() { // Declaring two strings char destination[50] = "Hello, "; const char* source = "World!";// Using strcat() to concatenate strings strcat(destination, source); // Display the concatenated result std::cout << "Result after concatenation: " << destination << std::endl; return 0;
} Output:
rust Copy code Result after concatenation: Hello, World! How It Works The destination string is initialized with the text "Hello, ", and it has enough space to hold the final result. The source string "World!" is appended to the destination string using the strcat() function. The result is printed, showing the concatenated string "Hello, World!". Important Considerations Buffer Overflow: Always ensure that the destination string has enough allocated space to store both the original and the appended string. Buffer overflows can lead to undefined behavior and security vulnerabilities. Security Concerns: In modern C++, it is recommended to use safer alternatives such as std::string for handling strings, as it automatically manages memory and helps avoid issues like buffer overflow. Conclusion The strcat() function from the library is a simple and useful tool for concatenating C-style strings. However, developers should be cautious about memory management and consider safer alternatives such as std::string in more complex C++ applications to avoid common pitfalls associated with manual memory handling.
0 notes
Text
Learn C++ Language at TCCI

C++ (or “C-plus-plus”) is a common programming language for creating software. It is an object-oriented language. In other words, it emphasizes using data fields with unique attributes (aka objects) rather than logic or functions. A common example of an object is a user account on a website.
This complete C++ course is designed to take you from complete beginner to advanced C++ programmer. This CPP course covers everything you need to become a master of C++.
This C++ course starts with the fundamentals of C++, including basic syntax, variables, data types, and operators. As you progress, in this advanced c++ course you will master essential programming concepts such as control structures, functions and pointers. The course also delves into more advanced topics of C++ such as object-oriented programming (OOP), where you'll learn about classes, objects, inheritance, polymorphism, encapsulation, and abstraction.
So whether you're preparing for a career in software engineering, want to enhance your programming skills, or aim to succeed in competitive coding, this comprehensive C++ course provides the knowledge and tools you need to excel.
C++ which is one of the most powerful and widely used programming languages. At TCCI-TRIRID Computer Coaching Institute, we provide comprehensive and industry-relevant C++ training designed for beginners to advanced learners. Our expert instructors guide you through fundamentals, object-oriented programming concepts, and real-world application development.
C++ contains following topics at TCCI:
Introduction to C++, Basic Syntax, Object Oriented Concept, Data Types and Variables, Constants, Literals, Modifiers, Operators, Loop Controls, Decision Making, Class Structure with Object, Function, Arrays, String, Inheritance, Constructor-Destructor, Exception Handling, Files etc.
For More Information:
Call us @ +91 98256 18292
Visit us @ https://tccicomputercoaching.wordpress.com/
#TCCI computer coaching institute#Best computer institute near me#C++ language classes in Ahmedabad#Computer Training Bopal Ahmedabad#Best computer classes in Iskon Crossroad Ahmedabad
1 note
·
View note
Text
C++ Selection Operators: Mastering Class Member Access and Header Guards
Dive into C++ Selection Operators! Master #ifndef, #define, member functions, dot operator, pointers, and arrow operator. Boost your C++ skills and write cleaner, more efficient code. #CPP #ProgrammingTips #CodingSkills
C++ selection operators play a crucial role in object-oriented programming, allowing developers to access class members efficiently. In this comprehensive guide, we’ll explore essential C++ concepts including #ifndef, #define, member functions, the dot operator, pointers, and the arrow selection operator. By understanding these fundamental elements, you’ll enhance your ability to write clean,…
0 notes
Text
CPP Programming Classes at Sunbeam Institute Pune
Enhance your programming skills with CPP Programming Classes at Sunbeam Institute Pune. Gain expertise in object-oriented programming, data structures, algorithms, and advanced C++ concepts. With industry-expert instructors, real-world projects, and 100% placement assistance, Sunbeam Institute ensures you are well-prepared for a successful career in software development.
Why Choose Sunbeam Institute for CPP Programming?
Expert trainers with hands-on industry experience.
Comprehensive syllabus covering OOP concepts, data structures, and memory management.
Live coding sessions to sharpen problem-solving skills.
Key Course Modules:
Core C++ Concepts: OOPs, classes, inheritance, and polymorphism.
Advanced Topics: Pointers, dynamic memory allocation, and templates.
Data Structures & Algorithms: Master linked lists, trees, and sorting algorithms.
Who Should Enroll?
Students and professionals looking to strengthen their programming foundation.
Individuals seeking careers in software development, game development, or embedded systems.
Course Benefits:
In-depth understanding of C++ programming.
Hands-on projects to solidify your coding skills.
Placement assistance to help kickstart your career in software development.
0 notes
Text
C language MCQ . . . . write your answer in the comment section https://bit.ly/3UA5nJb You can check the answer at the above link at Q.no. 50
0 notes
Text
look you say that as a meme but...
templates are turning complete (lol)
templates are actually fill-in-the blank code. so, you cannot just have a typical .hpp and .cpp structure. because you know, the .hpp would only define the interface (control panel) but not the implementation (guts). you have to manually instantiate the implementation for the template otherwise linking errors. can you tell that I got bit by this and I'm bitter???
sfinae (if the first template didn't work try a different one. this is like having half-, one-, two-, and three-gallon containers. you have three gallons to store. you literally try filling each of them until you get the last container. lol!)
anonymous namespace
inline namespace?!
const-ing the this parameter of a method is just weird syntax to me.
forwarding references? wtf is this shit?
coroutines, what does this mean in cpp and why does cpp have this?
concepts (I just don't know, I think they're type classes but funky)
the most vexing parse (skill issue)
vector>> (X_X)
you can use macros to change true to false. or.. any keyword. lol.
operator overloading to make things that shouldn't be. ostream pipe for example...
static
varargs are silly imo
pointer-to-member operators. JUST LEARNED THIS!
rule of five (so silly)
PIMPL (okay buddy)
basic datatype are relatively sized and only specify minimal sizes.
at least we have cstdint (and some others?)
rvalues/lvalues, which aren't really right/left values.
move semantics, I think it makes sense, just not immediately intuitive.
and im so sure, this is just start. cpp have so many features that work together to make insane things possible!
that cat has every right to be like that. this meme is real.
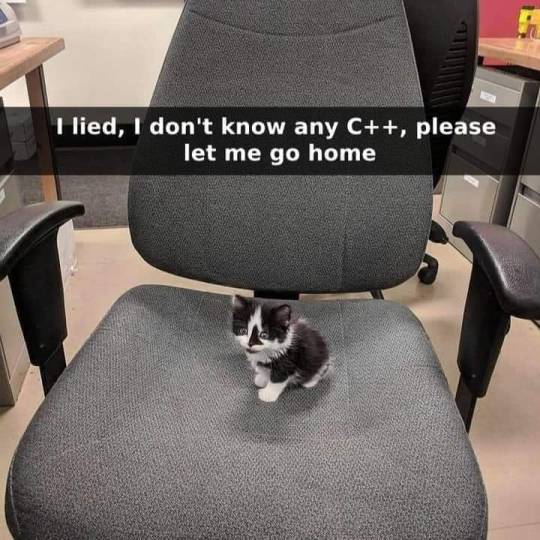
792 notes
·
View notes
Text
1 note
·
View note
Text
This Week in Rust 468
Hello and welcome to another issue of This Week in Rust! Rust is a programming language empowering everyone to build reliable and efficient software. This is a weekly summary of its progress and community. Want something mentioned? Tweet us at @ThisWeekInRust or send us a pull request. Want to get involved? We love contributions.
This Week in Rust is openly developed on GitHub. If you find any errors in this week's issue, please submit a PR.
Updates from Rust Community
Official
Announcing Rust 1.65.0
Project/Tooling Updates
rust-analyzer changelog #154
IntelliJ Rust Changelog #182
This Month in Rust OSDev: October 2022 | Rust OSDev
This Week in Fyrox
Cap'n Proto - Generic Associated Types
Gitoxide - Cloning the Linux kernel in under a minute
This week in Fluvio #48: The programmable streaming platform
Observations/Thoughts
Scoped threads in Rust, and why its async counterpart would be unsound
34 Must Know Terms for Embedded Rust Newbies
what if pointer properties were in the rust type system
mmap(1Tb): A Rust arena allocator (ab)using Linux overcommit
Contributing to Rust: Bootstrapping the Rust Compiler (rustc)
The ‘Viral’ Secure Programming Language That’s Taking Over Tech
[FR] Rust par le Métal : move, clone, copy (series)
[FR] Rust par le Métal (Annexe) : Les bases de nombres (series)
Rust Walkthroughs
Tauri vs. Electron for Tray Apps
Running Rust in AWS Lambda with SAM — Part 1
Tower, Episode 2: calling a Tower Service
Writing an eBPF/XDP Load-Balancer in Rust
Floating Point Arcade
[video] Cost-Efficient Rust in Practice, Rust Tokyo
Research
Can Rust-wrapped C++ offer performance and stability benefits?
Miscellaneous
Lynx Joins AdaCore and Ferrous Systems to Bring Rust to Embedded Developers
[video] RUST 1.65 - let-else statements - new language feature!!!
[video] The Rust Programming Language for Game Tooling
[video] Bencher—Catch Performance Regressions in CI @ Rust DC, September 20, 2022 w/ Everett Pompeii
[video] Impractical Rust: The HATETRIS World Record @ Rust DC, October 18, 2022 w/Dave Freiberg+Felipe Suero
[DE] Programmiersprache Rust 1.65 führt Generic Associated Types ein
[DE] Ferris Talk #12: Web-APIs mit Rust erstellen
Crate of the Week
This week's crate is enum_delegate, a crate to replace dynamic dispatch with enum dispatch.
Thanks to Devin Brite for the suggestion!
Please submit your suggestions and votes for next week!
Call for Participation
Always wanted to contribute to open-source projects but didn't know where to start? Every week we highlight some tasks from the Rust community for you to pick and get started!
Some of these tasks may also have mentors available, visit the task page for more information.
FOSDEM 2023 Rust devroom CFP
If you are a Rust project owner and are looking for contributors, please submit tasks here.
Updates from the Rust Project
396 pull requests were merged in the last week
(almost) always use ObligationCtxt when dealing with canonical queries
fix(generic_const_exprs):fix predicate inheritance for children of opaque types
make cpp-like debuginfo type names for slices and str consistent
track where diagnostics were created
rustc_metadata: encode even less doc comments
add multivalue target feature to WASM target
allow use of -Clto=thin with -Ccodegen-units=1 in general
lint against usages of struct_span_lint_hir
stable Lower lint level for read_zero_byte_vec
reduce span of let else irrefutable_let_patterns warning
suggest use .. to fill in the rest of the struct fields
better error for rustc_strict_coherence misuse
correctly resolve Inherent Associated Types
don't silently eat label before block in block-like expr
interpret: fix align_of_val on packed types
make proc_macro_derive_resolution_fallback a hard error
make underscore_literal_suffix a hard error
normalize types when deducing closure signature from supertraits
miri: implement condvars for Windows
miri: initOnce: synchronize with completion when already complete
rewrite implementation of #[alloc_error_handler]
remove bounds check when array is indexed by enum
stabilize the instruction_set feature
implement std::marker::Tuple, use it in extern "rust-call" and Fn-family traits
futures: do not require Clone for Shared::peek
libtest: run all tests in their own thread, if supported by the host
rustdoc: fix merge of attributes for reexports of local items
rustdoc: make Item::visibility computed on-demand
bindgen: add support for the "C-unwind" ABI
bindgen: add the --override-abi option
bindgen: allow callback composition
bindgen: wrap unsafe function's bodies in unsafe blocks
clippy: add allow-print-in-tests config
clippy: add new lint let_underscore_future
clippy: extend needless_collect
clippy: fix ICE in redundant_allocation
clippy: fix unnecessary_join turbofish in suggest message
clippy: improve needless_lifetimes
clippy: move needless_collect to nursery
clippy: shrink missing_{safety, errors, panics}_doc spans
rust-analyzer: add "Convert match to let-else" assist
rust-analyzer: add config for inserting must_use in generate_enum_as_method
rust-analyzer: extracted method from trait impl is placed in existing impl
rust-analyzer: generalize reborrow hints as adjustment hints
rust-analyzer: show signature help when calling generic types implementing FnOnce
rust-analyzer: fix the length displayed for byte string literals with escaped newlines
rust-analyzer: async trait method for unnecessary_async
rust-analyzer: fix reference searching only accounting substrings instead of whole identifiers
rust-analyzer: make custom expr prefix completions to understand refs
rust-analyzer: fixed local shadowing the caller's argument issue
rust-analyzer: lower unsafety of fn pointer and fn item types
rust-analyzer: migrate assists to format args captures, part 3
rust-analyzer: scip: generate symbols for local crates
Rust Compiler Performance Triage
A relatively noisy week (most of those have been dropped below, and comments left on GitHub), but otherwise a quiet one in terms of performance changes, with essentially no significant changes occuring.
Triage done by @simulacrum. Revision range: 822f8c2..57d3c58
2 Regressions, 2 Improvements, 3 Mixed; 3 of them in rollups 39 artifact comparisons made in total
See the full report for more details.
Call for Testing
An important step for RFC implementation is for people to experiment with the implementation and give feedback, especially before stabilization. The following RFCs would benefit from user testing before moving forward:
No RFCs issued a call for testing this week.
If you are a feature implementer and would like your RFC to appear on the above list, add the new call-for-testing label to your RFC along with a comment providing testing instructions and/or guidance on which aspect(s) of the feature need testing.
Approved RFCs
Changes to Rust follow the Rust RFC (request for comments) process. These are the RFCs that were approved for implementation this week:
crates.io token scopes
Final Comment Period
Every week, the team announces the 'final comment period' for RFCs and key PRs which are reaching a decision. Express your opinions now.
RFCs
[disposition: close] RFC 3283: Backward compatible default features
[disposition: close] RFC: Rust SemVer 2
[disposition: postpone] Add named path bases to cargo
Tracking Issues & PRs
No Tracking Issues or PRs entered Final Comment Period this week.
New and Updated RFCs
[new] Create an Operational Semantics Team
[new] Add a --compile-time-deps build flag to cargo build
[new] RFC: Anonymous Associated Types
Upcoming Events
Rusty Events between 2022-11-09 - 2022-12-07 🦀
Virtual
2022-11-09 | Virtual (Cardiff, UK) | Rust and C++ Cardiff
Introduction to Rust Atomics
2022-11-09 | Virtual (Darmstadt, DE) | betterCode
betterCode Rust
2022-11-09 | Virtual (Malaysia, MY) | Rust Malaysia
Rust Meetup November 2022 - a couple of lightning talks
2022-11-10 | Virtual (Budapest, HU) | HWSW free!
RUST! RUST! RUST! meetup (online formában!)
2022-11-10 | Virtual (Nürnberg, DE) | Rust Nuremberg
Rust Nürnberg online #19
2022-11-12 | Virtual | Rust GameDev
Rust GameDev Monthly Meetup
2022-11-15 | Virtual (Nairobi, KE / New York, NY, US)| Data Umbrella Africa
Online: Introduction to Rust Programming | New York Mirror
2022-11-15 | Virtual (Washington, DC, US) | Rust DC
Mid-month Rustful
2022-11-16 | Virtual (Vancouver, BC, CA) | Vancouver Rust
Rust Study/Hack/Hang-out
2022-11-17 | Virtual (Amsterdam, NL) | ITGilde Tech-Talks
Introduction “Rust” an ITGilde Tech Talk delivered by Pascal van Dam
2022-11-17 | Virtual (Stuttgart, DE) | Rust Community Stuttgart
Rust-Meetup
2022-11-21 | Virtual (Paris, FR) | Meetup Paris - École Supérieure de Génie Informatique (ESGI)
Découverte de WebAssembly
2022-11-22 | Virtual (Berlin, DE) | Berlin.rs
Rust Hack and Learn
2022-11-24 | Virtual (Linz, AT) | Rust Linz
Rust Meetup Linz - 27th Edition
2022-11-29 | Virtual (Dallas, TX, US) | Dallas Rust
Last Tuesday
2022-11-30 | Virtual (Munich, DE) | Rust Munich
Rust Munich 2022 / 3 - hybrid
2022-12-01 | Virtual (Charlottesville, VA, US) | Charlottesville Rust Meetup
Exploring USB with Rust
2022-12-06 | Virtual (Buffalo, NY, US) | Buffalo Rust Meetup
Buffalo Rust User Group, First Tuesdays
2022-12-07 | Virtual (Indianapolis, IN, US) | Indy Rust
Indy.rs - with Social Distancing
Europe
2022-11-15 | Tampere, FI | Rust Finland
Rust Meetup in Tampere
2022-11-16 | Paris, FR | Stockly
Rust Meetup in Paris - hosted by Stockly
2022-11-23 | Bratislava, SK | Bratislava Rust Meetup Group
Initial Meet and Greet Rust meetup
2022-11-24 | København, DK | Copenhagen Rust Group
Hack Night #31
2022-11-30 | Amsterdam, NL | Rust Nederland
Rust in Critical Infrastructure
2022-11-30 | Lille, FR | Rust Lille
Rust Lille #1
2022-11-30 | Munich, DE + Virtual | Rust Munich
Rust Munich 2022 / 3 - hybrid
North America
2022-11-10 | Columbus, OH, US | Columbus Rust Society
Monthly Meeting
2022-11-15 | San Francisco, CA, US | San Francisco Rust Study Group
Rust Hacking in Person
2022-11-29 | Austin, TX, US | ATX Rustaceans
Atx Rustaceans Meetup
2022-12-01 | Lehi, UT, US | Utah Rust
Utah Rust meetup
Oceania
2022-11-09 | Sydney, NSW, AU | Rust Sydney
RustAU Sydney - Last physical for 2022 !
2022-11-22 | Canberra, ACT, AU | Canberra Rust User Group
November Meetup
If you are running a Rust event please add it to the calendar to get it mentioned here. Please remember to add a link to the event too. Email the Rust Community Team for access.
Jobs
Please see the latest Who's Hiring thread on r/rust
Quote of the Week
Meanwhile the Rust shop has covers on everything and tag-out to even change settings of the multi-axis laser cutter, but you get trusted with said laser cutter on your first day, and if someone gets hurt people wonder how to make the shop safer.
– masklinn on r/rust
Thanks to Anton Fetisov for the suggestion!
Please submit quotes and vote for next week!
This Week in Rust is edited by: nellshamrell, llogiq, cdmistman, ericseppanen, extrawurst, andrewpollack, U007D, kolharsam, joelmarcey, mariannegoldin, bennyvasquez.
Email list hosting is sponsored by The Rust Foundation
Discuss on r/rust
1 note
·
View note