#how Javascript Static Initialization Blocks
Explore tagged Tumblr posts
Text
Backend Web Development Using Node.js
Node.js has revolutionized web development by enabling developers to write server-side code using JavaScript. If you're already comfortable with JavaScript on the frontend, transitioning to backend development with Node.js is a logical next step. In this post, we'll introduce the fundamentals of backend development using Node.js and how to build scalable, efficient web applications.
What is Node.js?
Node.js is a JavaScript runtime built on Chrome’s V8 engine. It allows developers to use JavaScript to write backend code, run scripts outside the browser, and build powerful network applications. Node.js is known for its non-blocking, event-driven architecture, making it highly efficient for I/O-heavy applications.
Why Use Node.js for Backend Development?
JavaScript Everywhere: Use a single language for both frontend and backend.
Asynchronous and Non-blocking: Great for handling many connections at once.
Vast Ecosystem: Thousands of modules available via npm (Node Package Manager).
Scalability: Ideal for microservices and real-time applications like chats or games.
Setting Up a Node.js Project
Install Node.js from nodejs.org
Create a new project folder:
Initialize the project:
Create your main file:
Basic Server Example
const http = require('http'); const server = http.createServer((req, res) => { res.writeHead(200, {'Content-Type': 'text/plain'}); res.end('Hello, Node.js Backend!'); }); server.listen(3000, () => { console.log('Server running on http://localhost:3000'); });
Using Express.js for Easier Development
Express.js is a popular web framework for Node.js that simplifies routing and middleware management.npm install express const express = require('express'); const app = express(); app.get('/', (req, res) => { res.send('Welcome to the Node.js backend!'); }); app.listen(3000, () => { console.log('Express server running on http://localhost:3000'); });
Common Backend Tasks with Node.js
Handle routing and API endpoints
Connect to databases (MongoDB, PostgreSQL, etc.)
Manage user authentication and sessions
Process form data and JSON
Serve static files
Popular Libraries and Tools
Express.js: Web framework
Mongoose: MongoDB object modeling
dotenv: Environment variable management
JWT: JSON Web Tokens for authentication
Nodemon: Auto-restart server on code changes
Best Practices
Use environment variables for sensitive data
Structure your project using MVC or service-based architecture
Use middleware for error handling and logging
Validate and sanitize user input
Secure your APIs with authentication and rate-limiting
Conclusion
Node.js is a powerful and flexible choice for backend development. Its ability to use JavaScript on the server-side, combined with a rich ecosystem of libraries, makes it ideal for building modern web applications. Start small, experiment with Express, and gradually add more features to build robust backend services.
0 notes
Text
Getting Started with TypeScript: From JavaScript to Typed Code
Embark on a journey to elevate your JavaScript skills with our comprehensive TypeScript tutorial for beginners. This guide, "Getting Started with TypeScript: From JavaScript to Typed Code," is designed to demystify TypeScript and empower you to write more robust, maintainable, and scalable applications.
JavaScript, while incredibly versatile, can sometimes become challenging to manage in large-scale projects due to its dynamic nature. TypeScript, a superset of JavaScript, addresses these challenges by introducing static typing. This typescript tutorial will guide you through the transition, demonstrating how to leverage the benefits of type checking without sacrificing the flexibility you love about JavaScript.
We begin by understanding the fundamental differences between JavaScript and TypeScript. You'll learn how TypeScript's static typing catches errors during development, preventing runtime bugs and enhancing code predictability. We’ll delve into the process of setting up your development environment, including installing TypeScript and configuring your compiler. This initial setup is crucial for a smooth learning experience, ensuring you're ready to dive into the core concepts.
Our typescript tutorial for beginners then explores the essential building blocks of TypeScript: types. We'll cover primitive types like number, string, and boolean, as well as more complex types such as arrays, tuples, and enums. You'll discover how to annotate variables and functions with these types, providing clarity and structure to your code.
Functions play a vital role in any programming language, and TypeScript enhances their functionality with type annotations for parameters and return values. This allows for better function signatures and helps ensure that functions are used correctly. We'll demonstrate how to define function types, handle optional parameters, and work with rest parameters, equipping you with the tools to write more reliable and expressive functions.
Interfaces and classes are cornerstones of object-oriented programming, and TypeScript provides powerful features to work with them. You'll learn how to define interfaces to enforce contracts between objects and how to create classes with properties and methods. We'll explore inheritance, polymorphism, and access modifiers, giving you a solid foundation in object-oriented principles.
Generics are another powerful feature of TypeScript, allowing you to write reusable components that work with a variety of types. This typescript tutorial will guide you through the process of creating generic functions and classes, enabling you to build flexible and adaptable code.
We'll also cover modules and namespaces, which are essential for organizing large codebases. You'll learn how to break your code into manageable modules and how to use namespaces to prevent naming conflicts. This modular approach promotes code reusability and maintainability.
Finally, we'll demonstrate how to integrate TypeScript with popular JavaScript frameworks and libraries, such as React, Angular, and Node.js. This practical application of TypeScript will solidify your understanding and show you how to apply your newfound knowledge in real-world scenarios.
0 notes
Text
How to Build a Fast, Secure, and Scalable Website
In today’s digital age, having a website that is fast, secure, and scalable is essential for businesses and developers alike. Whether you are building a personal blog, an e-commerce platform, or an enterprise-level application, ensuring optimal performance, security, and scalability should be a priority. A well-optimized website enhances user experience, improves search engine rankings, and ensures seamless operation even during traffic surges. In this blog, we’ll explore best practices to help you build a website that meets these critical criteria and supports business growth effectively.
1. Optimize for Speed
Website speed plays a crucial role in user experience and search engine rankings. Here are some key strategies to enhance website performance:
Use a Fast Hosting Provider
Choosing the right hosting provider can significantly impact your website's speed and reliability. Opt for a service that offers high-speed performance, such as cloud hosting or dedicated servers. Popular hosting providers like AWS, Google Cloud, and DigitalOcean offer scalable and high-performance solutions tailored to different business needs.
Leverage Content Delivery Networks (CDNs)
CDNs store copies of your website’s static assets across multiple global servers, reducing latency and load times for users in different locations. Using a CDN ensures your website loads quickly, even for visitors accessing it from distant geographic locations.
Optimize Images and Media Files
Large image files can slow down your website significantly. Use tools like TinyPNG or WebP format to compress images without sacrificing quality. Additionally, consider using responsive image formats that adjust dynamically based on device resolution and screen size.
Minify CSS, JavaScript, and HTML
Reducing the size of your code by minifying CSS, JS, and HTML files can significantly improve loading times. Tools like UglifyJS, CSSNano, and HTMLMinifier can help streamline your code while maintaining its functionality.
Implement Lazy Loading
Lazy loading ensures that images, videos, and other heavy resources load only when needed, improving initial page load speed. This technique reduces the amount of data that needs to be loaded immediately when a user lands on your page.
Use Browser Caching
By enabling caching, browsers can store copies of static resources, reducing the need to reload them each time a user visits the website. This helps in significantly improving return visits and reducing server load.
2. Enhance Security Measures
With cyber threats on the rise, securing your website is non-negotiable. Here’s how to protect your site effectively:
Use HTTPS and SSL Certificates
Securing your website with HTTPS by installing an SSL certificate encrypts data between users and your site, preventing cyber-attacks like man-in-the-middle attacks and data breaches.
Regularly Update Software and Plugins
Keep your CMS, themes, and plugins updated to prevent vulnerabilities that hackers may exploit. Outdated software is one of the primary security risks leading to data theft and unauthorized access.
Implement Web Application Firewall (WAF)
A WAF helps filter and monitor HTTP traffic between a web application and the Internet, blocking malicious attacks. It acts as a shield against threats such as SQL injection and cross-site scripting (XSS).
Enable Two-Factor Authentication (2FA)
Adding an extra layer of security, such as 2FA, ensures that only authorized users gain access to sensitive areas of your website. This significantly reduces the risk of unauthorized access due to password breaches.
Perform Regular Security Audits
Use tools like Sucuri or Qualys to conduct regular security scans and identify potential vulnerabilities before hackers do. Conducting periodic penetration testing also helps in strengthening website security.
Implement Secure Authentication and Data Encryption
Use strong passwords, encrypt stored data, and implement security headers to protect against threats like cross-site scripting (XSS) and SQL injection attacks. Keeping security policies updated helps in mitigating emerging cybersecurity risks.
3. Ensure Scalability
Scalability is essential to handle traffic spikes without compromising performance. Here’s how to design a scalable website:
Adopt a Microservices Architecture
Instead of a monolithic structure, use a microservices-based approach where different parts of the application can scale independently. This allows for easier updates and better fault isolation.
Use Load Balancing
Distribute traffic efficiently across multiple servers to prevent overload and downtime. Load balancers like Nginx or AWS Elastic Load Balancing can help maintain high availability even during peak traffic periods.
Leverage Cloud Infrastructure
Cloud platforms like AWS, Google Cloud, and Azure offer auto-scaling features that adjust resources based on traffic demands. This ensures that your website remains responsive even during unexpected traffic surges.
Optimize Database Performance
Use indexing, query optimization, and caching techniques (e.g., Redis or Memcached) to improve database efficiency and handle high loads. A well-optimized database enhances website performance and response time.
Use Asynchronous Processing
For tasks that do not require immediate execution, implement asynchronous processing using message queues (e.g., RabbitMQ, Apache Kafka) to enhance performance. This allows the website to handle multiple processes efficiently without performance lags.
Monitor and Scale Automatically
Set up monitoring tools like New Relic, Google Analytics, or AWS CloudWatch to track performance metrics and automate scaling decisions. Proactive monitoring helps in identifying performance bottlenecks and making necessary optimizations.
Conclusion
Building a fast, secure, and scalable website requires a strategic approach that balances performance optimization, security best practices, and scalable architecture. By implementing these techniques, you can create a website that provides a seamless experience for users while ensuring security and reliability as your business grows.
Need help with website development? At Chirpin, we specialize in building high-performance, secure, and scalable websites. As a digital marketing agency in Delhi, we also provide digital marketing services in Delhi NCR to help businesses enhance their online presence. Whether you are looking for the best digital marketing company in Delhi or need professional digital marketing services in Delhi NCR, Chirpin has you covered. Our expert team works with businesses of all sizes, helping them establish a strong online presence and drive more leads. If you are looking for the best digital marketing company in Delhi NCR that offers comprehensive web development and marketing solutions, contact us today to bring your digital vision to life!
exhibition stand builders in dubai exhibition stand contractors in dubai exhibition contractors in dubai exhibition stand builders dubai dubai exhibition stand contractor exhibition stand designers in dubai best exhibition stand contractor in dubai best exhibition stand builders dubai exhibition stand design company in dubai exhibition stand design dubai dubai exhibition stands exhibition company in dubai exhibition companies in dubai exhibition stand builder abu dhabi exhibition stand contractor in abu dhabi exhibition stand contractors abu dhabi exhibition stand companies in abu dhabi exhibition stand design company abu dhabi exhibition stand in abu dhabi
0 notes
Text
How to Speed Up Your Webflow Site in 5 Easy Steps
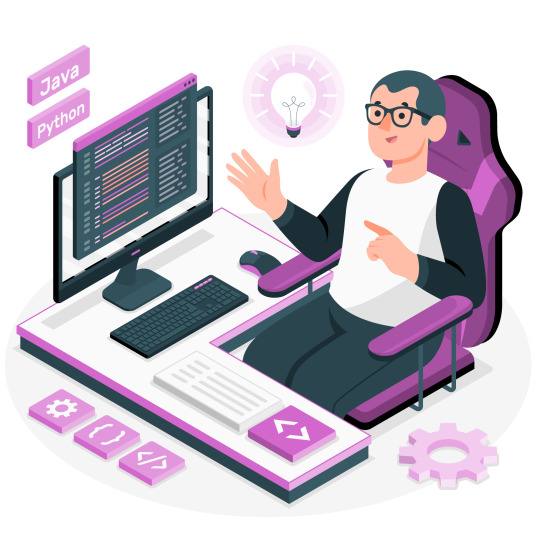
In the digital age, website speed is paramount. A fast-loading site not only improves user experience but also boosts your search engine ranking. Webflow is a powerful tool for web design, but to fully harness its potential, you must ensure your site is optimized for speed. Here, we outline five essential steps to enhance your Webflow site's performance.
1. Optimize Your Images
Because they are frequently the largest files on a webpage, images have a big impact on load speeds. Here’s how to ensure your images are optimized:
a. Choose the Right Format
Using the appropriate image format can make a big difference. JPEG is ideal for photographs due to its compression capabilities, while PNG is better for graphics with fewer colors. WebP, a newer format, offers superior compression and quality, making it a good choice for most images.
b. Compress Images
Use tools like TinyPNG or ImageOptim to compress your images without compromising quality. Compressed images load faster and reduce the overall weight of your site.
c. Use Responsive Images
Leverage Webflow’s responsive image feature. It automatically generates various image sizes for different devices, ensuring that users only download the smallest necessary version.
d. Lazy Load Images
To ensure that images load only when they enter the viewport, enable lazy loading. This speeds up perceived performance and cuts down on the initial load time.
2. Minimize and Combine Files
Minimizing and combining your site’s CSS, JavaScript, and HTML files reduces the number of HTTP requests, which in turn speeds up your site.
a. Minify CSS, JavaScript, and HTML
Minification removes unnecessary characters (like white spaces, commas, and comments) from your code without affecting its functionality. Tools like UglifyJS for JavaScript and CSSNano for CSS are great for this purpose.
b. Combine Files
Whenever possible, merge several JavaScript and CSS files into one.This reduces the number of requests the browser needs to make, speeding up page load times.
c. Load JavaScript Asynchronously
By loading JavaScript files asynchronously, you ensure they don’t block the rendering of the page. This means the browser can continue loading other elements while it fetches the JavaScript.
3. Use a Content Delivery Network (CDN)
A CDN distributes your content across various servers worldwide, allowing users to load your site from a server closer to their location, which significantly reduces load times.
a. Choose the Right CDN
Popular CDNs like Cloudflare, Fastly, and Amazon CloudFront offer reliable services that can greatly enhance your site's speed and performance.
b. Enable Caching
CDNs cache your content, so subsequent visits to your site are faster. Ensure your CDN is configured to cache static assets like images, CSS, and JavaScript files.
c. Optimize Delivery
Leverage your CDN’s features to optimize content delivery. For instance, enabling Brotli or GZIP compression can further reduce file sizes and accelerate load times.
4. Reduce Webfont Usage
While webfonts can enhance your site’s aesthetic, they can also slow it down. Here’s how to optimize webfonts for better performance:
a. Limit the Number of Fonts
Employ a small selection of font weights and families.Each additional font adds to the load time, so stick to a maximum of two to three families.
b. Use Modern Formats
WOFF2 is the latest webfont format, offering better compression and faster loading times than older formats like TTF or EOT.
c. Optimize Loading
Consider loading webfonts asynchronously using the font-display: swap CSS property. This allows text to be displayed immediately using a fallback font until the webfont is fully loaded.
5. Enable Browser Caching
Browser caching stores certain files on the user’s device, so they don’t have to be re-downloaded on subsequent visits. This drastically reduces load times for returning visitors.
a. Set Expiration Dates
Set expiration dates on your server according to the different kinds of files.Static assets like images and CSS files can have a longer expiration period, while dynamic content should have shorter periods.
b. Leverage Cache-Control Headers
Use cache-control headers to define how and for how long browsers should cache your resources. The max-age directive specifies the maximum amount of time a resource is considered fresh.
c. Validate Cached Resources
Use the ETag and Last-Modified headers to validate cached resources. This ensures users receive the latest version of a resource if it has changed, while still benefiting from caching when possible.
Conclusion
By following these five steps—optimizing images, minimizing and combining files, using a CDN, reducing webfont usage, and enabling browser caching—you can significantly improve your Webflow site’s speed and performance. In addition to improving user experience, a quicker website raises your search engine rating and increases website traffic.
Website:- How to Speed Up Your Webflow Site in 5 Easy Steps
#Webflow#WebsiteSpeed#PerformanceOptimization#ImageOptimization#WebDesign#UserExperience#SEO#CDN#MinifyCSS#JavaScript#BrowserCaching#Webfonts#ResponsiveImages#LazyLoading#FileCompression#AsyncJavaScript
0 notes
Text
How to Optimize Your Website for Speed and Performance
In today's fast-paced digital world, website speed and performance are crucial for maintaining user engagement and achieving higher search engine rankings. Here's a comprehensive guide to help you optimize your website for speed and performance effectively.
Minimize HTTP Requests
Reducing the number of elements on your page and combining CSS, JavaScript, and HTML files can significantly decrease load times.
Use Asynchronous Loading for CSS and JavaScript
Loading scripts asynchronously prevents them from blocking the rendering of the page, ensuring faster load times.
Optimize Images
Compress and resize images without losing quality. Using modern image formats like WebP can also improve load times.
Enable Browser Caching
Set an expiry date or a maximum age in the HTTP headers for static resources to enhance load speed for returning visitors.
Minimize and Optimize Code
Minify your CSS, JavaScript, and HTML to remove unnecessary characters. Combining multiple files can also reduce load times.
Use a Content Delivery Network (CDN)
Distributing your content across multiple locations globally can reduce latency and improve load times for users worldwide.
Enable Compression
Using Gzip or Brotli to compress your website's files can significantly reduce the size of the data your server sends to browsers.
Reduce Server Response Time
Optimize server settings and consider using faster web hosting solutions to decrease response times.
Implement AMP (Accelerated Mobile Pages)
Creating lighter, faster versions of your web pages for mobile devices can drastically improve load times on mobile.
Optimize Web Fonts
Limit the number of web fonts and use modern formats like WOFF2 to ensure faster loading of text content.
Eliminate Render-Blocking Resources
Loading critical CSS and JavaScript inline and deferring non-essential scripts can speed up page rendering.
Use Lazy Loading
Load images and videos only when they are about to enter the viewport to improve initial load times.
By following these tips, you can significantly enhance your website's speed and performance, providing a better experience for your users and improving your SEO rankings.
Plassey Technologies is one of the Top 10 Website Development Agency in Gurgaon, known for delivering high-performance websites tailored to meet business needs.
0 notes
Text
BrainyDX: Your Partner in Core Web Vitals Optimization & Website Success
In today’s digital world, where everyone expects things instantly, having a fast website that’s easy to use is no longer optional – it’s critical. Here’s where Core Web Vitals (CWV) can make a big difference for your website’s visitors. Understanding and optimizing these metrics is crucial for any website owner or SEO professional in 2024.
Core Web Vitals Explained
Core Web Vitals, created by Google, are 3 important measurements that track how users experience your website in the real world. They focus on how fast your site loads(Loading Speed), how quickly it responds to clicks(Responsiveness), and how stable the layout is(visual stability). By making sure your website performs well in these areas, you can create a frustration-free experience for your web visitors, which can ultimately lead to a higher ranking in search results. Also, it can provide you with more conversions.
The Three CWV Metrics
There are currently three core web vitals that Google prioritizes:
Largest Contentful Paint: This metric measures how long it takes for the major content on your webpage (usually text content or images) to become fully visible to the user. According to Google, for a positive user experience, your website’s LCP should load in under 2.5 seconds.
Interaction to Next Paint: Introduced in March 2024, It replaces First Input Delay (FID) and measures how responsive your website feels to user interactions like clicking buttons or typing in forms. An INP of under 100 milliseconds is considered ideal.
Cumulative Layout Shift: This metric measures how often unexpected layout shifts occur on your webpage, which will be really frustrating for users. A low CLS score (ideally below 0.1) indicates a stable visual experience.
Why Core Web Vitals Matter in 2024
There are several compelling reasons why CWV are more important in 2024:
SEO Ranking Factor: Google has explicitly stated that Core Web Vitals are a ranking factor in their search algorithms. Strong CWVs can boost your website’s ranking in search results, bringing in more visitors naturally.
Improved User Experience: A fast, responsive, and visually stable website provides a significantly better user experience. This translates into higher engagement, lower bounce rates, and ultimately, more conversions.
Mobile-First Indexing: When Google ranks websites in search results, they mainly look at the mobile version of your site for indexing. Because most mobile users expect websites to load quickly and work smoothly, optimizing CWV is essential for ranking well in mobile search results.
Enhanced Brand Reputation: A website that performs well technically reflects positively on your brand image. Visitors perceive a fast and user-friendly website as trustworthy and reliable.
Website Optimisation for CWV
You can use the following important strategies to strengthen your Core Website Vitals:
Image Optimization: The loading speed of your website might be considerably slowed down by large image files. Optimise image dimensions for the web and use image compression technologies.
Reduce Render-Blocking Resources: Files such as CSS and JavaScript can prevent the content on your website from rendering. Think about applying strategies like code splitting, postponing non-essential resources, and minifying.g.
Make Use of Browser Caching: Caching enables browsers to save webpage components locally, saving them from having to be downloaded again on different visits. Implement effective browser caching strategies for static content.
Prioritize Critical Rendering Path: Determine the important resources to render the web page’s initial content and make sure they load first.
Minimize HTTP Requests: To optimize website loading speed, external resource requests should be minimized. Additionally, whenever feasible, file consolidation techniques can be employed to further reduce overall HTTP requests.
Utilize Content Delivery Network: A CDN essentially creates a global network of servers for your website’s content. This brings your content closer to users around the world, reducing delays (latency) and ensuring faster loading times regardless of their location.
Measuring and Monitoring CWV
We have several free tools available to help you assess and track Core Web Vitals. These tools are most popular in 2024:
Google Search Console: This is a tool by Google that provides insights into your website’s health. It includes a dedicated Core Web Vitals report that shows you how your website is performing over time. This report highlights any areas where your website might need improvement and helps you prioritize what to focus on first.
PageSpeed Insights: Another free tool from Google, PageSpeed Insights analyzes your website’s overall performance and provides specific recommendations for improvement. We’ll use advanced industry technology to assess your website and thoroughly identify opportunities for improvement.
Lighthouse: This is a free, open-source auditing tool developed by Google. It conducts a comprehensive assessment of your website’s functionality, including a detailed analysis of CWV. Lighthouse provides you with scores for each metric and suggests specific ways to improve them.
By using these tools regularly, you can get insights of your website’s performance and can find areas where Core Web Vitals optimization can make a positive impact.
Conclusion
In today’s competitive online landscape, website optimization for CWV is no longer optional. By prioritizing these key metrics, you can ensure a smooth, frustration-free experience for your visitors, which ultimately leads to better SEO performance, higher conversions, and a stronger overall online presence.
How We Can Help You Optimize Core Web Vitals
Here at BrainyDX Technologies, we are experts in website optimization and SEO. Our team can help you:
Analyze Core Web Vitals: We’ll use advanced industry tools to analyze your website performance and find ways to make it even better.
Develop a CWV optimization strategy: We will create a customized plan to address your specific website’s needs and ensure your CWVs meet Google’s recommendations.
Implement optimization techniques: We can make the technical adjustments needed to improve overall speed of your website, make it react faster to clicks, and ensure everything stays in place when it loads.
Monitor and track progress: We’ll regularly check website performance and keep you updated with reports to help it keep thriving.
We can improve your website’s speed, user experience, and search ranking? We offer a free consultation to discuss Core Web Vitals and how it can help you achieve your SEO goals.
#corewebvitals#cwv#digitalmarketing#websiteoptimization#seo#webdev#webperformance#googleranking#userexperience
1 note
·
View note
Text
Optimizing Front-End Performance: Strategies for Faster Websites
Optimising front-end speed is essential in the digital age because people expect websites to load extremely quickly. In addition to improving user experience, a quicker website boosts conversion rates and ranks higher in search results. These are some essential tactics to make sure your website is loading as quickly as possible.
Cut Down on HTTP Requests Every element on a webpage—stylesheets, scripts, and images—needs an HTTP request. Page load times can be greatly accelerated by lowering the quantity of these queries.
Techniques:
Combine Files: To cut down on requests, combine CSS and JavaScript files. Small CSS and JavaScript Inlines: You should inline small code segments straight into your HTML. Use CSS Sprites: To display the necessary portion of a picture, combine numerous images into a single file and use CSS. 2. Image Optimisation A webpage's loading speed is frequently mostly attributed to its images. Enhancing them can result in significant increases in performance.
Methods:
Choose the Right Format: JPEGs are best for photos; PNGs are best for transparent pictures; SVGs are best for simple graphics and icons. Compress photos: Utilise tools like ImageOptim or TinyPNG to resize photos without sacrificing quality. Images with lazy loading load only after they appear in the viewer. 3. Use a content delivery network, or CDN. A content delivery network stores the static files for your website across several different sites (CDN). This speeds up load times by cutting down on the user's path to the server.
Benefits
By transmitting files from the nearest server, latency is decreased. Enhanced Reliability: Distributed servers reduce the possibility of downtime.
4. CSS, and Utilise Caching in Browsers Browsers can save static files using caching to avoid having to reload them on each visit.
Techniques:
Set expiration dates to specify how long files should be stored in browsers. For static materials like CSS, JavaScript, and pictures, use extended expiration dates. Control Headers for Caches: To define caching policies, use these. 5. Condense and Minify the Code Your code files may download and parse more quickly if they are smaller.
Techniques:
Minify HTML,avaScript: Get rid of extraneous characters, spaces, and comments. Gzip Compression: To compress files before sending them to the browser, enable Gzip on your server.
6. Set priorities Above-the-Fold Content By loading important content above the fold first, visitors to your website will have more time to interact with it.
Techniques:
Important CSS: For material that appears above the fold, extract and inline CSS are needed. JavaScript files that are not necessary should be loaded asynchronously or delayed until the primary content has loaded. 7. Improve Web Fonts Web fonts can speed up a website while also improving its looks.
Techniques:
Subset Fonts: Just add the characters that are required. Preload Fonts: To load fonts ahead of time, use the link rel="preload" element. Employ Contemporary Formats: For optimal compression and performance, use WOFF2.
8. Put Server Side Rendering (SSR) into Practice Server-side rendering can significantly enhance initial load times and SEO for websites with a lot of JavaScript.
Advantages:
Faster First Load: Users see a completely displayed page since HTML is created on the server. Improved SEO: The pre-rendered HTML is easily crawled by search engines. 9. Lessen Scripts from Third Parties Ads, social media widgets, and analytics tools are examples of third-party scripts that can significantly increase load times and cause performance problems.
Techniques:
Third-Party Script Audit: Continually examine and eliminate superfluous scripts. Load Asynchronously: To avoid blocking, make sure third-party scripts are loaded asynchronously.
10. Consistently check and assess performance Performance bottlenecks can be found and fixed with the aid of ongoing testing and monitoring.
Instruments:
Google Lighthouse: Offers recommendations and performance evaluations. WebPageTest: Provides in-depth performance analysis for websites. GTmetrix: Provides thorough insights by combining data from WebPageTest and Google Lighthouse.
In summary Front-end performance optimisation calls for a variety of strategies and constant work. By putting these tactics into practice, you may drastically improve the speed of your website, giving visitors a better experience and improving search engine rankings. Remember that in today's fast-paced digital world, having a quicker website gives you a competitive advantage. Enhance your expertise and stay ahead in the digital game by enrolling in our comprehensive front end developer course at LearNowx. Learn the newest methods and industry best practices to build lightning-fast websites.
0 notes
Text
How to Optimize Visualforce Pages for Better Performance
Optimizing Visualforce pages for better performance involves several key practices to enhance user experience and minimize load times. Here are some tips:
Reduce View State Size: Keep the view state size as small as possible to minimize bandwidth consumption and improve page load times. Avoid using unnecessary components and minimize the use of apex:form and apex:pageBlock components.
Limit Data Binding: Only bind data that is necessary for rendering the page. Avoid binding large data sets or unnecessary fields.
Minimize Server-Side Processing: Reduce the number of server-side calls by consolidating logic and data retrieval operations. Use Apex controllers efficiently to fetch only the required data.
Optimize SOQL Queries: Make sure your SOQL queries are selective and efficient. Use WHERE clauses to filter records and limit the number of rows returned.
Implement Lazy Loading: Load data dynamically as needed, especially for large data sets. Use techniques such as pagination and lazy loading to minimize initial page load times.
Cache Data: Cache frequently accessed data in static variables or in Salesforce caching mechanisms like platform cache or custom caching solutions. This reduces the need for repeated data retrieval from the database.
Use Client-Side Caching: Leverage browser caching by setting appropriate cache-control headers and utilizing techniques like JavaScript caching where applicable.
Optimize JavaScript and CSS: Minimize the size of JavaScript and CSS files by removing unnecessary code and whitespace. Combine multiple files into a single file where possible to reduce HTTP requests.
Enable Gzip Compression: Enable Gzip compression on your server to reduce the size of transmitted data and improve page load times.
Optimize Images: Compress images to reduce file size without sacrificing quality. Use image formats like JPEG or PNG, and consider lazy loading images that are below the fold.
Use Asynchronous Processing: Execute long-running operations asynchronously to prevent blocking the UI and improve responsiveness.
Implement Caching Mechanisms: Utilize browser caching and caching mechanisms provided by Visualforce, such as apex:cacheable, to cache rendered output or expensive computations.
Optimize Visualforce Markup: Minimize the use of complex Visualforce components and keep markup simple and clean. Avoid excessive nesting of components and use standard HTML elements where possible.
Monitor and Analyze Performance: Regularly monitor page load times and analyze performance using tools like Salesforce Inspector or browser developer tools. Identify bottlenecks and areas for improvement.
By following these best practices, you can optimize Visualforce pages for better performance, resulting in improved user experience and increased efficiency.
visit our website: bytesfarms.com
0 notes
Text
WordPress: Eliminate Render-Blocking Resources for a Faster Website
In today's fast-paced digital world, a slow website can be a major turn-off for visitors. It's not just user experience that's at stake – search engines like Google also consider website speed as a ranking factor. One common issue that can slow down your WordPress website is render-blocking resources. In this article, we'll delve into what render-blocking resources are, why they matter, and most importantly, how to eliminate them to ensure your WordPress website performs at its best.
Originhttps://worldgoit.com/archives/posts/software-development/wordpress-eliminate-render-blocking-resources-for-a-faster-website/
Table of Contents
- Introduction - Understanding Render-Blocking Resources - Impact on Website Performance - Identifying Render-Blocking Resources - Best Practices for Elimination - 1. Asynchronous Loading - 2. Deferred JavaScript - 3. Browser Caching - 4. Content Delivery Networks (CDNs) - 5. Minification and Compression - 6. Prioritize Above-the-Fold Content - 7. Modern Web Development Tools - Implementing Solutions Step-by-Step - Conclusion - FAQs
Introduction
When a user visits your WordPress website, their browser needs to load various resources like HTML, CSS, and JavaScript. Render-blocking resources are JavaScript and CSS files that prevent the page from loading until they are fully processed. This can significantly slow down the rendering of your web page, leading to a poor user experience.
Understanding Render-Blocking Resources
Render-blocking resources act as roadblocks for your website's rendering process. Browsers pause rendering to fetch and process these resources, delaying the display of the page content. JavaScript files, especially those placed in the header, are major culprits. CSS files can also impact rendering if not handled properly.
Impact on Website Performance
Website speed matters more than ever in a world where attention spans are shrinking. Studies show that visitors tend to abandon sites that take more than a couple of seconds to load. Additionally, search engines consider page speed as a ranking factor, meaning slower websites might rank lower in search results.
Identifying Render-Blocking Resources
To tackle this issue, you must first identify which resources are causing the delay. There are various online tools and plugins available that can analyze your website and provide a list of render-blocking resources. This step is crucial in understanding what needs to be optimized.
Best Practices for Elimination
1. Asynchronous Loading By loading resources asynchronously, you allow the browser to continue rendering the page while fetching the resources in the background. This can greatly improve the perceived loading speed. 2. Deferred JavaScript Deferring JavaScript means postponing its execution until after the initial rendering. This prevents JavaScript from blocking other resources and speeds up the page load. Recommendation Plugin and Youtube Async JavaScript Autoptimize https://youtu.be/ElpcjGBgTGk?si=ue1rvzQPs0YI971R 3. Browser Caching Leverage browser caching to store static resources locally. Returning visitors will then have these resources cached, resulting in faster load times. 4. Content Delivery Networks (CDNs) CDNs distribute your website's resources across multiple servers worldwide. This reduces the physical distance between the user and the server, leading to quicker resource retrieval. 5. Minification and Compression Minify your CSS and JavaScript files by removing unnecessary characters. Additionally, compressing these files reduces their size, making them quicker to load. 6. Prioritize Above-the-Fold Content Load essential resources first, especially those needed for above-the-fold content. This way, users can see and interact with the main content sooner. 7. Modern Web Development Tools Consider using modern build tools like Webpack or Rollup. These tools can bundle and optimize your resources, reducing the number of requests made by the browser
Implementing Solutions Step-by-Step
- Start by analyzing your website's current performance using online tools. - Identify the specific resources causing the delay. - Update your WordPress theme and plugins to their latest versions. - Utilize asynchronous loading for non-essential resources. - Defer JavaScript where possible and optimize CSS delivery. - Leverage browser caching and consider a reliable CDN. - Minify and compress CSS and JavaScript files. - Prioritize above-the-fold content for faster initial rendering. - Explore modern web development tools for advanced optimization.
Conclusion
A fast-loading website is a key factor in retaining visitors and achieving higher search engine rankings. By understanding and addressing render-blocking resources, you can significantly improve your WordPress site's performance. Implementing the strategies mentioned in this article will help you create a smoother, more enjoyable user experience while boosting your website's SEO efforts.
before after
FAQs
Q1: What are render-blocking resources? Render-blocking resources are JavaScript and CSS files that prevent a webpage from rendering until they are fully loaded and processed. Q2: How do render-blocking resources affect my website? Render-blocking resources can slow down your website's loading speed, leading to a poor user experience and potentially lower search engine rankings. Q3: How can I identify render-blocking resources on my WordPress site? There are various online tools and plugins available that can analyze your website and provide a list of render-blocking resources. Q4: What is asynchronous loading? Asynchronous loading allows the browser to continue rendering a webpage while fetching resources in the background, improving perceived loading speed. Q5: Can using a Content Delivery Network (CDN) help with render-blocking resources? Yes, a CDN can distribute your website's resources across multiple servers, reducing the distance between the user and the server and speeding up resource retrieval. Read the full article
1 note
·
View note
Text
How to design faster website?
How to design faster website?
Designing a faster website is crucial for improving user experience, SEO rankings, and overall performance. Here are several tips and best practices to help you create a faster website:
Optimize Images and Media:
Use image compression tools to reduce the file size of images while maintaining quality.
Use responsive images and the element to serve different image sizes based on the user's device and screen size.
Lazy loading: Load images only when they come into the user's viewport.
Minimize HTTP Requests:
Combine CSS and JavaScript files to reduce the number of HTTP requests.
Minify and concatenate CSS and JavaScript files to reduce their size.
Utilize browser caching for static assets to reduce the need for repeated downloads.
Content Delivery Network (CDN):
Use a CDN to distribute your website's content across multiple servers geographically closer to your users. This reduces latency and speeds up loading times.
Optimize Code:
Ensure your code is clean and well-structured.
Remove unused CSS and JavaScript code.
Reduce the use of external scripts and plugins that add unnecessary overhead.
Use Browser Caching:
Set appropriate caching headers for static assets (images, CSS, JavaScript) to instruct the browser to store these resources locally, reducing server requests on subsequent visits.
Reduce Server Response Time:
Choose a reliable and fast web hosting provider.
Use server-side caching mechanisms (e.g., opcode caching, object caching, and page caching).
Optimize your server-side code and database queries.
Optimize Fonts:
Minimize the number of fonts and font variations used on your site.
Consider using system fonts or icon fonts for faster loading.
Asynchronous Loading:
Load non-essential scripts asynchronously to prevent blocking page rendering.
GZIP Compression:
Enable GZIP compression on your web server to reduce the size of transmitted data.
Responsive Design:
Ensure your website is responsive and mobile-friendly to optimize performance on all devices.
Reduce Redirects:
Minimize the use of unnecessary redirects as they add latency to page loading.
Optimize Third-party Resources:
Limit the use of third-party widgets and scripts. If necessary, load them asynchronously.
Image Formats:
Choose the appropriate image format (JPEG, PNG, WebP) for your images. WebP typically offers better compression.
Content Delivery Optimization:
Use a Content Delivery Network (CDN) to serve static content from servers closer to the user's location.
Prioritize Above-the-Fold Content:
Load critical resources (CSS, JavaScript) for above-the-fold content first to ensure a faster initial render.
Monitor and Test:
Regularly monitor your website's performance using tools like Google PageSpeed Insights, GTmetrix, or Pingdom.
Conduct performance testing and optimize accordingly.
Use a Content Management System (CMS) Wisely:
If using a CMS like WordPress, choose lightweight themes and plugins. Remove any unnecessary plugins.
Implement HTTP/2 or HTTP/3:
If your server supports it, consider upgrading to HTTP/2 or HTTP/3 for faster loading of assets.
Optimize for Mobile:
Mobile users often have slower connections. Ensure your site is optimized for mobile performance.
Regularly Update and Maintain:
Keep your website's software, plugins, and themes up to date to benefit from performance improvements and security updates.
Looking for a responsive and faster website? We're a leading website designing company in Chennai, Porur, Adyar specializing in creating high-performance websites for your business needs. Get in touch for a blazing fast, mobile-friendly web presence.
#website designing company in porur#website designing company in chennai#website designing company#website designing#website
0 notes
Text
Top 10 Angular-Built Websites And Applications
Angular is a popular front-end web application framework that is extensively used for designing single-page web applications. This framework was developed by Google. It has been a leader among the most popular frameworks on the web since the moment it came into being in 2009 and continues to be of great significance. Its first version was AngularJS, also known as Angular 1. For many years, this version was widely used, and several well-known apps were created using AngularJS. However, once Angular v.2 was released, it lost the "JS" ending and the framework is now just known as Angular.
Angular is used to create a lot of websites and applications because of its outstanding functionality, simplicity, and potential. Websites built with Angular are user-friendly, feature-rich, and responsive. Angular apps and websites have established a strong reputation and have been used by tech giants like Microsoft, Gmail, and Samsung. With time, Angular has continued to reach new heights, grabbing big fish in the industry like Gmail, PayPal, and Upwork. So, what exactly is Angular? Let’s take a look!
What is Angular?
Unless you are already familiar with it, Angular is an open-source framework for web application development. Misko Hevery and Adam Abrons found Angular in 2009. It is based on TypeScript, a statically-typed superset of JavaScript, and provides a set of powerful features to design web applications. Web solutions built in Angular are scalable, dynamic, and responsive. Angular is a crucial component of the popular frontend development combo the ‘JavaScript Big 3’ – a combination of the frameworks React, Angular, and Vue.
It has features like MVC architecture, an extensible template language, bidirectional data flow, and HTML templates that make it easier and more convenient for web developers to create top-notch AngularJS websites. Angular also includes a powerful set of tools for managing application state, routing, form validation, etc. Moreover, it provides seamless integration with other popular libraries and frameworks, such as RxJS, Bootstrap, and Material Design.
Angular comes with a component-based architecture. An Angular application has a tree of components. Here, each component is a fundamental building block of the user interface. Components can be reused across different parts of an application and can communicate with each other using services.
Some of the key benefits of partnering with an AngularJS development company for web development include faster development time, better code organization and maintainability, improved performance, and an improved end-user experience. Angular is widely used by developers and companies around the world and has a large and active community of contributors and users.
Best Examples of Angular Applications and Websites
1. Gmail
One of the top Angular application examples is Gmail. What’s a better way to prove Angular’s potential than by handling the world’s No. 1 email service? If you take a good look at the Gmail service, you will find out how powerful Angular is. Gmail has been using Angular since 2009. Gmail has more than 1.5 billion daily users. And, Angular helps it to effortlessly handle the heavy traffic generated by such a huge user base. As such, this single-page app runs speedily, flawlessly, and without crashes.
Take a look at the key features of Gmail! It offers functionalities like a simple and intuitive interface, data rendering on the front end, and offline access to cached data. Angular manages every action within a single HTML page, whether you're reading an email, writing a new message, or switching tabs. When new emails and messages come in, it provides data on the front end at once. Instantaneous initial loading takes no more than a few seconds, after which you can start working with emails and tabs right away. Even if you go offline, you can still get access to some of the features.
Gmail's innovative feature ‘live Hangouts chats’ is simple and yet, powerful. You can add Hangouts to your Angular apps. The other notable features include mail drafting with in-built syntax and grammar, predictive messaging, and spell-check.
2. PayPal
I am sure that most individuals, even the ones without technical backgrounds, know about this multinational payment company. This angular application acts as an electronic replacement for conventional paper methods like cheques and money orders and supports rapid online money transfers. The company operates as an online payment processor and more than 305 million people have active accounts on PayPal's platform. The app performs flawlessly and can handle substantial traffic.
Because PayPal is used for financial transactions, it requires an instant, real-time data transfer with high-tech security features. Thanks to Angular, the app caters to these requirements successfully. Using the Angular tool checkout.js, PayPal users enjoy a seamless checkout experience. Customers can make purchases and complete the entire transaction without ever leaving the website.
3. Forbes
Forbes is a popular American business magazine that publishes articles on business, industry, making investments, and topics associated with marketing. Related topics like technology, communications, science, politics, and law are covered by Forbes as well. The Forbes website was developed using Angular 9+ and various cutting-edge technologies, including Core-JS, BackboneJS, LightJS, and others. It is one of the most visited websites in the world with more than 74 million monthly users in the US.
Many Forbes readers belong to the business world or are celebrities. Hence, the website is expected to work smoothly and take minimal time to load accurate information from dependable sources. Thanks to Angular, Forbes offers an uninterrupted and enriching UX. Angular’s code reusability feature, makes the Forbes website performant on any operating system, browser, or device. Besides, the website boasts of features such as self-updating pages, an attractive design to attract attention, and simplicity in use.
4. Upwork
Upwork is one of the most popular Angular apps available. This service makes it possible for companies to locate freelancers, agencies, and independent professionals for any kind of project from any corner of the world. When the COVID-19 pandemic broke out three years ago, there was a recession that damaged the infrastructure of many industries. During such moments of crisis, Upwork helped many companies and freelancers by connecting them.
Using Upwork, businesses can find a freelancer who satisfies their specific requirements. At the same time, the platform helps freelancers to find out employers and projects that suit their requirements. Freelancers can search for jobs by applying filters like project type, project length, etc. So this "world’s work marketplace" has millions of users on its website daily, which is operated smoothly by Angular. Upwork uses Angular as part of its technology stack to offer a responsive single-page interface. Thanks to Angular, Upwork has features like mobile app calling, payment getaways, intuitive architecture, and private security and verification mechanisms. Like many other Angular-written websites, Upwork exhibits excellent webpage performance.
5. Deutsche Bank
Another great example of an AngularJS website is Deutsche Bank, a German investment and financial services company that uses AngularJS. Angular was used to create the developer portal's front page. Deutsche Bank’s Application Programming Interface can be accessed through the developer’s portal. This API serves as a point of access for the numerous international organizations looking to integrate the transaction systems of Deutsche Bank into their web software solutions.
6. Weather.com
This website, as its name implies, provides weather forecasts. It was bought by IBM in 2015. When examining Angular websites, weather.com is one to keep an eye out for. There is no better way to find out how powerful Angular is than to analyze how weather.com works.
In addition to providing accurate hourly and daily weather forecasts, the website also provides daily headlines, live broadcasts, factoids, air quality tracking, and entertainment material. The best example of how Angular functions is Weather.com, which displays real-time broadcasting on websites or apps with numerous geographical locations. Angular makes it simpler to process data on websites with a large number of users and frequent changes. Not only does it show high-definition aerial shots of places, but also provides alerts on severe weather conditions and weather-related disasters.
7. Delta Airlines
Among many AngularJS web application development models, one such website is Delta. One of the most competitive American Airlines, Delta Air Lines provides flight tickets to 300+ locations in 60 different countries. They, therefore, have a large number of users on their website at once, thanks to this level of service.
Angular 9+ was used by Delta to create a robust and quick website. Delta has deployed the framework on its homepage, and that decision has been a game changer for them.
8. Guardian
The Guardian is a UK-based newspaper that was started in 1821. It’s not surprising that The Guardian has developed into a dependable source of news and information throughout the world, given that it first provided news offline and later had a sizable online presence.
It is available online and has been developed with AngularJS. It provides reliable news from all over the world and reaches several thousand people every day, be it about finance, football, etc. So due to the endless number of people spending time on their websites and generating traffic, they used AngularJS to create a highly readable and accessible web app.
9. Wikiwand
Wikiwand founder Lior Grossman once said: "It did not make rational sense to us that what is now the fifth most widely visited website in the globe, which is used by nearly one-half of the world's population, has an interface that has not been up-to-date in more than a decade." We realized that Wikipedia’s UI was cluttered, tricky to read (large segments of tiny text), impossible to navigate, and not user-friendly. So, their team leveraged Angular app development to create Wikiwand, a software wrapper meant for the articles of Wikipedia.
From children to adults, we all use Wikipedia as our source of information for so many things. Even though we all love Wikipedia, we will have to admit its interface is outdated, difficult to read and navigate, full of distracting stuff, etc. This is where Wikiwand uses its magic; it provides a modern touch to Wikipedia pages with the use of Angular technology. It has enhanced the readability as well as navigation. Now, you get advanced navigation features on both the web and mobile apps which ultimately results in a better user experience.
10. Google
Google uses a lot of technologies to improve its services. Given that Google created Angular in the first place, it makes sense that Google would use it. That’s why it is one of the best examples of an Angular application. Because Angular is so effective for single web pages, Google uses it in many of its products.
Google Play Store
Google Voice application
Google Open Source
Google Play Book
The Google.org website
Google Arts and Culture
Wrap Up:
This concludes our list of the top 10 Angular websites and Angular applications, and well-known companies that best demonstrate Angular's potential. Anything that well-known and large companies use must be of the highest caliber. The Angular webpages and web applications that are listed in this article are all widely recognized globally. If there is one thing you should take away from this, it should be that Angular is a flexible framework that can improve the usability and interactivity of your web applications.
Now that you've gotten some helpful recommendations on the kinds of projects for which this common framework is suitable, you know when it's most important to focus on building Angular webpages and which Angular tools to use to get the best results. Therefore, it is important to seek technical assistance from a specialized Angular development agency before beginning any project.
0 notes
Text
What are the criteria to hire a node js developer?
Introduction to Node.js
It is a cross-platform environment for executing JavaScript code outside an open-source browser. It's important to note that NodeJS is neither a framework nor a programming language. Most folks are perplexed and believe it is a framework or programming language. We frequently utilize Node.js to create backend services such as APIs, Web Apps, and Mobile Apps. Large corporations such as Paypal, Netflix, Walmart, and others use it in their manufacturing.
This programming language was created to enhance the capabilities of JavaScript in web browsers. Because Node.js features a non-blocking event-driven I/O mechanism, it's an excellent choice for building dependable and scalable apps. This language is being used by the
Hire Node.js developers
A Nodejs developer's primary role is writing, developing, and deploying web-based server-side programming to support business needs. A Nodejs developer is expected to have a good foundation and knowledge of many forms of JavaScript server-side programming, such as ExpressJS, StrongLoop, and others. Node js Backend or Backend development comprises the integration of third-party web services, and Node js developers assist frontend developers in completing the entire application development process.
Developers that work with Node.js have a particular set of duties and expertise. It's more challenging to gain the skills required because it's a more sophisticated job that requires knowledge of multiple technologies, but it pays higher.
The most crucial Node.js developer requirements are mentioned below. If you want to get recruited, you'll need to know Node.js (and, by extension, JavaScript), but there's a little more to it. If you want to work as a Node.js developer, you need to look into the following skills. After reading all the responsibilities handled by the developer, you can hire nodejs developers at a cost-effective price and attain the benefits.
Node JS junior developer
JS knowledge is required.
experience in commercial development or a full-fledged pet project
a thorough understanding of the platform's features;\
Ability to type both statically and dynamically;
Working with the Framework skills;
Understanding software design principles, as well as a unit and integration testing.
Senior Node JS programmer
Knowledge of cloud infrastructure
a track record of working on a variety of initiatives;
The ability to solve abstractly phrased problems quickly and come up with novel solutions;
Work experience in a high-volume environment;
The ability to address difficulties with performance;
Ability to assist developers at a lower level;
Knowledge of software development methodologies, algorithms, data structures, and architectural approaches is required.
Understanding how microservices interact and more.
What Does It Cost To Hire A Node JS Developer?
The cost of a developer's services is one of the essential considerations when choosing one. This consumes a significant portion of the budget. The price may involve the efforts of various team members and will be determined by the amount of time spent on the project. In addition, the cost of nodejs development company of developer services can vary greatly depending on the specialist's location and experience.
The starting salary of a Node js developer is between $70,492 and $72,000. The typical Node js developer pay for senior developers ranges between $110,000 and $130,000 per year.
It is critical to consider the developer's experience, review their portfolio of projects, and determine if they specialize in the areas required. You can sign up for consultation with the company of your choice if you want to estimate the cost of your project.
Factors influencing salary
The major drivers of IT talent pricing will always be market demand, experience level, education, and location. However, other characteristics can make one Node.js Developer more valuable than another on the open market, such as:
Their familiarity with development technologies such as Node Package Manager (npm) and Grunt.
Ability to deal with more challenging system difficulties, such as juggling user authentication and authorization across multiple systems and servers.
Working knowledge of agile and lean approaches.
Expertise in project management
Conclusion
To summarize, if you want to hire a Node.js developer or the backend with nodejs to get the best results and have your project successful, you should hunt for the finest organizations that outsource Node.js development services.Also, figure out how much money you're willing to spend on your project's development and hire a suitable Node.js engineer with that in mind. Depending on the length of project, you can choose from a variety of engagement options, such as freelancers or professional developers. Overall, finding a nodejs as backend developer with all the necessary skills is a manageable process that necessitates careful thought and examination of various aspects.
0 notes
Text
How to Optimize Website Speed for SEO & Better UX
Introduction
Website speed is a crucial factor in determining search engine rankings and user experience. A slow website leads to poor engagement, high bounce rates, and lower conversions. Google has officially recognized page speed as a ranking factor, making it essential for businesses to optimize their website’s performance. In this article, we will explore effective strategies to improve your website speed for better SEO and user experience.
1. Why Website Speed Matters for SEO & UX
SEO Impact
Google’s search algorithms prioritize websites that load quickly, providing a better user experience. If your website is slow, search engines may rank your pages lower, reducing your organic traffic. Key SEO benefits of faster websites include:
Higher rankings on search engine results pages (SERPs)
Improved crawlability for search engines
Increased mobile-friendliness, crucial after Google’s mobile-first indexing update
User Experience (UX) Impact
Website visitors expect pages to load quickly. If a site takes more than three seconds to load, over 50% of users will abandon it. Faster websites offer:
Higher user engagement and session duration
Lower bounce rates
Improved conversions and revenue generation
2. How to Measure Website Speed
Before making improvements, analyze your website’s speed using tools such as:
Google PageSpeed Insights (https://pagespeed.web.dev/)
GTmetrix (https://gtmetrix.com/)
Lighthouse (Chrome DevTools) (https://developers.google.com/web/tools/lighthouse)
These tools assess key performance indicators such as Largest Contentful Paint (LCP), First Input Delay (FID), and Cumulative Layout Shift (CLS).
3. Best Practices to Improve Website Speed
A. Optimize Images
Compress images using tools like TinyPNG or ImageOptim.
Use next-gen image formats like WebP instead of JPEG/PNG.
Implement lazy loading to defer image loading until they are needed.
B. Enable Browser Caching
Store frequently accessed resources in a user’s browser cache to speed up repeat visits.
Set expiration dates for static files (CSS, JavaScript, images) using .htaccess settings.
C. Minimize HTTP Requests
Reduce the number of elements on a page (scripts, images, CSS files).
Combine multiple CSS and JavaScript files into a single file.
D. Use a Content Delivery Network (CDN)
A CDN distributes your website’s content across multiple global servers, reducing latency and speeding up load times for users in different locations. Popular CDN providers include:
Cloudflare
Amazon CloudFront
Akamai
E. Optimize Server Response Time
Choose a reliable and high-performance web hosting provider.
Use caching plugins for CMS platforms like WordPress (e.g., WP Rocket, W3 Total Cache).
Optimize your database by removing unnecessary data and post revisions.
F. Reduce JavaScript & CSS Blocking
Minify JavaScript and CSS files using tools like UglifyJS and CSSNano.
Implement asynchronous loading (async or defer attributes) to prevent render-blocking.
G. Implement Lazy Loading
Lazy loading ensures that images and videos load only when they appear on the user’s screen, significantly improving initial page load speed. This can be implemented with:<img src="image.jpg" loading="lazy" alt="Example image">
4. Mobile Speed Optimization
With Google’s mobile-first indexing, optimizing for mobile users is crucial. Key steps include:
Using Accelerated Mobile Pages (AMP) for faster rendering.
Optimizing for responsive design and reducing unnecessary elements on mobile.
Testing mobile performance using Google’s Mobile-Friendly Test (https://search.google.com/test/mobile-friendly).
5. Final Thoughts
Website speed optimization is no longer optional—it is a necessity for better SEO rankings and improved user experience. By implementing these strategies, businesses can enhance performance, increase traffic, and drive higher conversions. Regularly monitor and improve your website’s speed to stay ahead of the competition.
Contact Us for SEO Assistance
Need help optimizing your website speed? Contact our expert team today!
📞 Mobile: +91 9655877577 🌐 Website: https://intellimindz.com/seo-training-in-tirupur/
0 notes
Link
In this tutorial, we’re going to use Angular with HERE data, and display and interact with that geolocation data using Leaflet.
When it comes to development, I’m all about choosing the right tool for the job. While HERE offers a great interactive map component as part of its JavaScript SDK, there might be reasons to explore other interactive map rendering options. Take Leaflet for example. With Leaflet, you can provide your own tile layer while working with a very popular and easy to use open source library.
In a past tutorial, you’ll remember that I had demonstrated how to use Angular with HERE, but this time around we’re going to shake things up a bit. We’re going to use Angular with HERE data, but we’re going to display and interact with it using Leaflet.
To get a better idea of what we plan to accomplish, take a look at the following animated image:
We’re going to display an interactive map, geocode some addresses, and display those addresses on the map as markers using Leaflet, Angular, and the HERE RESTful API.
Starting With a New Angular Project and the Leaflet Dependencies
Before going forward, the assumption is that you have the Angular CLI installed and that you have a free HERE developer account. With these requirements met, go ahead and execute the following command:
ng new leaflet-project
The above command will create our new Angular project, but that project won’t be ready to use Leaflet. We have to first include the necessary JavaScript and CSS files.
Open the project’s src/index.html file and make it look like the following:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>LeafletProject</title>
<base href="/">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" type="image/x-icon" href="favicon.ico">
<link rel="stylesheet" href="https://unpkg.com/[email protected]/dist/leaflet.css">
</head>
<body>
<app-root></app-root>
<script src="https://unpkg.com/[email protected]/dist/leaflet.js"></script>
</body>
</html>
Notice that we’ve included the CSS library as well as the JavaScript library for Leaflet. Technically, this is all that is required to start using Leaflet with HERE in an Angular application. However, we’re going to do some more preparation work to set us up for some awesome features.
Open the project’s src/app/app.module.ts file and include the following:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { HttpClientModule } from '@angular/common/http';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Because we are no longer using the HERE JavaScript SDK, we are now relying on HTTP requests to the RESTful API. By default, HTTP in Angular is disabled, so by including the HttpClientModule and adding it to the imports array of the @NgModule block, we are enabling it.
While not absolutely necessary, we could also clean up the CSS a bit to allow us a full-screen map. Open the project’s src/styles.css file and include the following:
body {
margin: 0;
}
Now everything will take up the full width and height without the default margins.
It may seem like we’ve done a lot as of now, but it has been strictly configuration. Now we’re going to get into the fun stuff, which is development with Leaflet and Angular.
Create an Angular Component to Represent an Interactive HERE Map
While there are many ways to start using maps in your project, the cleanest approach is to create a dedicated Angular component so the maps can be reused throughout the application.
From the Angular CLI, execute the following:
ng g component here-map
The above command will generate several files for us that will represent our component. We’re going to start by opening the project’s src/app/here-map/here-map.component.html file and including the following markup:
<div #map [style.height]="height" ></div>
In the above markup, you’ll notice the #map attribute. We’re going to use this as a reference so we can access this DOM element from our TypeScript code. You’ll also notice that we have a dynamic height and a static width. It is easy to full screen the width of a component, but you have to be a CSS wizard to full screen the height. To cheat, we’re going to use JavaScript to determine the height and set it as a variable after everything initializes.
With the HTML in place, open the project’s src/app/here-map/here-map.component.ts file and include the following:
import { Component, OnInit, ViewChild, ElementRef, Input } from '@angular/core';
import { HttpClient } from '@angular/common/http';
declare var L: any;
@Component({
selector: 'here-map',
templateUrl: './here-map.component.html',
styleUrls: ['./here-map.component.css']
})
export class HereMapComponent implements OnInit {
@ViewChild("map")
public mapElement: ElementRef;
@Input("appId")
public appId: string;
@Input("appCode")
public appCode: string;
private map: any;
public srcTiles: string;
public height: string;
public constructor(private http: HttpClient) {
this.height = window.innerHeight + "px";
}
public ngOnInit() {
this.srcTiles = "https://2.base.maps.api.here.com/maptile/2.1/maptile/newest/reduced.day/{z}/{x}/{y}/512/png8?app_id=" + this.appId + "&app_code=" + this.appCode + "&ppi=320";
}
public ngAfterViewInit() {
this.map = L.map(this.mapElement.nativeElement, {
center: [37.7397, -121.4252],
zoom: 10,
layers: [L.tileLayer(this.srcTiles)],
zoomControl: true
});
}
public dropMarker(address: string) {
this.http.get("https://geocoder.api.here.com/6.2/geocode.json", {
params: {
app_id: this.appId,
app_code: this.appCode,
searchtext: address
}
}).subscribe(result => {
let location = result.Response.View[0].Result[0].Location.DisplayPosition;
let marker = new L.Marker([location.Latitude, location.Longitude]);
marker.addTo(this.map);
});
}
}
This file and the above code is the bulk of our project. To make things easier to understand, we’re going to look at the above code piece by piece.
Within the HereMapComponent class, we have the following:
@ViewChild("map")
public mapElement: ElementRef;
@Input("appId")
public appId: string;
@Input("appCode")
public appCode: string;
The ViewChild is referencing our #map attribute from the HTML. This is how we’re getting a reference to the HTML component for further usage. Each of the @Input annotations represents a possible attribute that the user can provide. In our example, the user will be providing the app id and app code found in their developer dashboard.
Remember that dynamic height I was talking about. We’re setting it here:
public constructor(private http: HttpClient) {
this.height = window.innerHeight + "px";
}
We are calculating the inner browser height and setting it using JavaScript. This way we can avoid all the tricky vertical height stuff that comes with standard CSS.
Because we’re using Leaflet, we’re relying heavily on the various HERE RESTful APIs and this includes the Map Tile API.
public ngOnInit() {
this.srcTiles = "https://2.base.maps.api.here.com/maptile/2.1/maptile/newest/reduced.day/{z}/{x}/{y}/512/png8?app_id=" + this.appId + "&app_code=" + this.appCode + "&ppi=320";
}
Using the provided app id and app code we can construct our URL for getting tiles from the API. This is set in the ngAfterViewInit when we initialize Leaflet.
public ngAfterViewInit() {
this.map = L.map(this.mapElement.nativeElement, {
center: [37.7397, -121.4252],
zoom: 10,
layers: [L.tileLayer(this.srcTiles)],
zoomControl: true
});
}
When initializing Leaflet, we are using the HTML component that we referenced, are centering the map on certain coordinates, and are using our HERE Tile API for the layer.
While we won’t be working with markers and geocoding by default, we’re going to lay the foundation within our component:
public dropMarker(address: string) {
this.http.get("https://geocoder.api.here.com/6.2/geocode.json", {
params: {
app_id: this.appId,
app_code: this.appCode,
searchtext: address
}
}).subscribe(result => {
let location = result.Response.View[0].Result[0].Location.DisplayPosition;
let marker = new L.Marker([location.Latitude, location.Longitude]);
marker.addTo(this.map);
});
}
When the dropMarker method is executed, we make a request to the HERE Geocoder API with a search query. In our scenario, the search query is an address or location. The results are then used to create a marker which is added to the map.
Before we can start using our new component, we need to wire it up. Open the project’s src/app/app.module.ts file and include the following:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { HttpClientModule } from '@angular/common/http';
import { AppComponent } from './app.component';
import { HereMapComponent } from './here-map/here-map.component';
@NgModule({
declarations: [
AppComponent,
HereMapComponent
],
imports: [
BrowserModule,
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Notice that this time around we have imported our component and added it to the declarations array of the @NgModule block.
The tough stuff is over and we can now work towards displaying our map.
Displaying and Interacting With the Angular Map Component
Since this is a simple project, we don’t have a router. Instead, everything will be rendered inside the project’s src/app/app.component.html file. Open this file and include the following:
<here-map #map appId="APP-ID-HERE" appCode="APP-CODE-HERE"></here-map>
There are a few things to take note of in the above. First, it is only coincidence that we’ve added a #mapattribute. We don’t truly need to add one and if we did, it doesn’t need to have the same name as the previous component. Second, notice the appId and appCode attributes. You’ll want to swap the placeholder values with your own tokens.
Now open the project’s src/app/app.component.ts file and include the following:
import { Component, OnInit, ElementRef, ViewChild } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
@ViewChild("map")
public mapElement: ElementRef;
public ngOnInit() { }
public ngAfterViewInit() {
this.mapElement.dropMarker("tracy, ca");
this.mapElement.dropMarker("lathrop, ca");
}
}
We want to be able to make use of the dropMarker function that sits in our map component. To do this we need to gain a reference to our HTML component with the #map attribute. Once we have a reference, then we can start calling the public functions that reside in it.
In our example, we are adding two markers for two cities in California.
Conclusion
You just saw how to use Leaflet in your Angular project to work with HERE data. HERE is flexible so you’re able to use whatever renderer you want to display your interactive maps. In my previous tutorialwe used the default renderer, but this time we used Leaflet which is just one of many options.
1 note
·
View note
Text
How to Get Into Google News - Whiteboard Friday
Posted by Polemic
Today we're tackling a question that many of us have asked over the years: how do you increase your chances of getting your content into Google News? We're delighted to welcome renowned SEO specialist Barry Adams to share the framework you need to have in place in order to have a chance of appearing in that much-coveted Google News carousel.

Click on the whiteboard image above to open a high-resolution version in a new tab!
Video Transcription
Hi, everyone. I'm Barry Adams. I'm a technical SEO consultant at Polemic Digital and a specialist in news SEO. Today we're going to be talking about how to get into Google News. I get a lot of questions from a lot of people about Google News and specifically how you get a website into Google News, because it's a really great source of traffic for websites. Once you're in the Google News Index, you can appear in the top stories carousel in Google search results, and that can send a lot of traffic your way.
How do you get into Google News' manually curated index?
So how do you get into Google News? How do you go about getting your website to be a part of Google News' manual index so that you can get that top stories traffic for yourself? Well, it's not always as easy as it makes it appear. You have to jump through quite a few hoops before you get into Google News.
1. Have a dedicated news website
First of all, you have to have a dedicated news website. You have to keep in mind when you apply to be included in Google News, there's a team of Googlers who will manually review your website to decide whether or not you're worthy of being in the News index. That is a manual process, and your website has to be a dedicated news website.
I get a lot of questions from people asking if they have a news section or a blog on their site and if that could be included in Google News. The answer tends to be no. Google doesn't want news websites in there that aren't entirely about news, that are commercial websites that have a news section. They don't really want that. They want dedicated news websites, websites whose sole purpose is to provide news and content on specific topics and specific niches.
So that's the first hurdle and probably the most important one. If you can't clear that hurdle, you shouldn't even try getting into Google News.
2. Meet technical requirements
There are also a lot of other aspects that go into Google News. You have to jump through, like I said, quite a few hoops. Some technical requirements are very important to know as well.
Have static, unique URLs.
Google wants your articles and your section pages to have static, unique URLs so that an article or a section is always on the same URL and Google can crawl it and recrawl it on that URL without having to work with any redirects or other things. If you have content with dynamically generated URLs, that does not tend to work with Google News very well. So you have to keep that in mind and make sure that your content, both your articles and your static section pages are on fixed URLs that tend not to change over time.
Have your content in plain HTML.
It also helps to have all your content in plain HTML. Google News, when it indexes your content, it's all about speed. It tries to index articles as fast as possible. So any content that requires like client-side JavaScript or other sort of scripting languages tends not to work for Google News. Google has a two-stage indexing process, where the first stage is based on the HTML source code and the second stage is based on a complete render of the page, including executing JavaScript.

For Google News, that doesn't work. If your content relies on JavaScript execution, it will never be seen by Google News. Google News only uses the first stage of indexing, based purely on the HTML source code. So keep your JavaScript to a minimum and make sure that the content of your articles is present in the HTML source code and does not require any JavaScript to be seen to be present.
Have clean code.
It also helps to have clean code. By clean code, I mean that the article content in the HTML source code should be one continuous block of code from the headline all the way to the end. That tends to result in the best and most efficient indexing in Google News, because I've seen many examples where websites put things in the middle of the article code, like related articles or video carousels, photo galleries, and that can really mess up how Google News indexes the content. So having clean code and make sure the article code is in one continuous block of easily understood HTML code tends to work the best for Google News.
3. Optional (but more or less mandatory) technical considerations
There's also quite a few other things that are technically optional, but I see them as pretty much mandatory because it really helps with getting your content picked up in Google News very fast and also makes sure you get that top stories carousel position as fast as possible, which is where you will get most of your news traffic from.
Have a news-specific XML sitemap.
Primarily the news XML sitemap, Google says this is optional but recommended, and I agree with them on that. Having a news-specific XML sitemap that lists articles that you've published in the last 48 hours, up to a maximum of 1,000 articles, is absolutely necessary. For me, I think this is Google News' primary discovery mechanism when they crawl your website and try to find new articles.
So that news-specific XML sitemap is absolutely crucial, and you want to make sure you have that in place before you submit your site to Google News.
Mark up articles with NewsArticle structured data.
I also think it's very important to mark up your articles with news article structured data. It can be just article structured data or even more specific structured data segments that Google is introducing, like news article analysis and news article opinion for specific types of articles.
But article or news article markup on your article pages is pretty much mandatory. I see your likelihood of getting into the top stories carousel much improved if you have that markup implemented on your article pages.
Helpful-to-have extras:
Also, like I said, this is a manually curated index. So there are a few extra hoops that you want to jump through to make sure that when a Googler looks at your website and reviews it, it ticks all the boxes and it appears like a trustworthy, genuine news website.
A. Multiple authors
Having multiple authors contribute to your website is hugely valuable, hugely important, and it does tend to elevate you above all the other blogs and small sites that are out there and makes it a bit more likely that the Googler reviewing your site will press that Approve button.
B. Daily updates
Having daily updates definitely is necessary. You don't want just one news post every couple of days. Ideally, multiple new articles every single day that also should be unique. You can have some sort of syndicated content on there, like from feeds, from AP or Reuters or whatever, but the majority of your content needs to be your own unique content. You don't want to rely too much on syndicated articles to fill your website with news content.
C. Mostly unique content
Try to write as much unique content as you possibly can. There isn't really a clear ratio for that. Generally speaking, I recommend my clients to have at least 70% of the content as unique stuff that they write themselves and publish themselves and only 30% maximum syndicated content from external sources.
D. Specialized niche/topic
It really helps to have a specialized niche or a specialized topic that you focus on as a news website. There are plenty of news sites out there that are general news and try to do everything, and Google News doesn't really need many more of those. What Google is interested in is niche websites on specific topics, specific areas that can provide in-depth reporting on those specific industries or topics. So if you have a very niche topic or a niche industry that you cover with your news, it does tend to improve your chances of getting into that News Index and getting that top stories carousel traffic.
So that, in a nutshell, is how you get into Google News. It might appear to be quite simple, but, like I said, quite a few hoops for you to jump through, a few technical things you have to implement on your website as well. But if you tick all those boxes, you can get so much traffic from the top stories carousel, and the rest is profit. Thank you very much.
This has been my Whiteboard Friday.
Further resources:
Google News Help
Publisher Center Help
Google News Initiative
Optimizing for Google News: A SlideShare presentation from Barry's talk at Digitalzone 2018 discussing how to optimize websites for visibility in Google News.
Video transcription by Speechpad.com
Sign up for The Moz Top 10, a semimonthly mailer updating you on the top ten hottest pieces of SEO news, tips, and rad links uncovered by the Moz team. Think of it as your exclusive digest of stuff you don't have time to hunt down but want to read!
2 notes
·
View notes
Text
How to Get Into Google News - Whiteboard Friday
Posted by Polemic
Today we're tackling a question that many of us have asked over the years: how do you increase your chances of getting your content into Google News? We're delighted to welcome renowned SEO specialist Barry Adams to share the framework you need to have in place in order to have a chance of appearing in that much-coveted Google News carousel.

Click on the whiteboard image above to open a high-resolution version in a new tab!
Video Transcription
Hi, everyone. I'm Barry Adams. I'm a technical SEO consultant at Polemic Digital and a specialist in news SEO. Today we're going to be talking about how to get into Google News. I get a lot of questions from a lot of people about Google News and specifically how you get a website into Google News, because it's a really great source of traffic for websites. Once you're in the Google News Index, you can appear in the top stories carousel in Google search results, and that can send a lot of traffic your way.
How do you get into Google News' manually curated index?
So how do you get into Google News? How do you go about getting your website to be a part of Google News' manual index so that you can get that top stories traffic for yourself? Well, it's not always as easy as it makes it appear. You have to jump through quite a few hoops before you get into Google News.
1. Have a dedicated news website
First of all, you have to have a dedicated news website. You have to keep in mind when you apply to be included in Google News, there's a team of Googlers who will manually review your website to decide whether or not you're worthy of being in the News index. That is a manual process, and your website has to be a dedicated news website.
I get a lot of questions from people asking if they have a news section or a blog on their site and if that could be included in Google News. The answer tends to be no. Google doesn't want news websites in there that aren't entirely about news, that are commercial websites that have a news section. They don't really want that. They want dedicated news websites, websites whose sole purpose is to provide news and content on specific topics and specific niches.
So that's the first hurdle and probably the most important one. If you can't clear that hurdle, you shouldn't even try getting into Google News.
2. Meet technical requirements
There are also a lot of other aspects that go into Google News. You have to jump through, like I said, quite a few hoops. Some technical requirements are very important to know as well.
Have static, unique URLs.
Google wants your articles and your section pages to have static, unique URLs so that an article or a section is always on the same URL and Google can crawl it and recrawl it on that URL without having to work with any redirects or other things. If you have content with dynamically generated URLs, that does not tend to work with Google News very well. So you have to keep that in mind and make sure that your content, both your articles and your static section pages are on fixed URLs that tend not to change over time.
Have your content in plain HTML.
It also helps to have all your content in plain HTML. Google News, when it indexes your content, it's all about speed. It tries to index articles as fast as possible. So any content that requires like client-side JavaScript or other sort of scripting languages tends not to work for Google News. Google has a two-stage indexing process, where the first stage is based on the HTML source code and the second stage is based on a complete render of the page, including executing JavaScript.

For Google News, that doesn't work. If your content relies on JavaScript execution, it will never be seen by Google News. Google News only uses the first stage of indexing, based purely on the HTML source code. So keep your JavaScript to a minimum and make sure that the content of your articles is present in the HTML source code and does not require any JavaScript to be seen to be present.
Have clean code.
It also helps to have clean code. By clean code, I mean that the article content in the HTML source code should be one continuous block of code from the headline all the way to the end. That tends to result in the best and most efficient indexing in Google News, because I've seen many examples where websites put things in the middle of the article code, like related articles or video carousels, photo galleries, and that can really mess up how Google News indexes the content. So having clean code and make sure the article code is in one continuous block of easily understood HTML code tends to work the best for Google News.
3. Optional (but more or less mandatory) technical considerations
There's also quite a few other things that are technically optional, but I see them as pretty much mandatory because it really helps with getting your content picked up in Google News very fast and also makes sure you get that top stories carousel position as fast as possible, which is where you will get most of your news traffic from.
Have a news-specific XML sitemap.
Primarily the news XML sitemap, Google says this is optional but recommended, and I agree with them on that. Having a news-specific XML sitemap that lists articles that you've published in the last 48 hours, up to a maximum of 1,000 articles, is absolutely necessary. For me, I think this is Google News' primary discovery mechanism when they crawl your website and try to find new articles.
So that news-specific XML sitemap is absolutely crucial, and you want to make sure you have that in place before you submit your site to Google News.
Mark up articles with NewsArticle structured data.
I also think it's very important to mark up your articles with news article structured data. It can be just article structured data or even more specific structured data segments that Google is introducing, like news article analysis and news article opinion for specific types of articles.
But article or news article markup on your article pages is pretty much mandatory. I see your likelihood of getting into the top stories carousel much improved if you have that markup implemented on your article pages.
Helpful-to-have extras:
Also, like I said, this is a manually curated index. So there are a few extra hoops that you want to jump through to make sure that when a Googler looks at your website and reviews it, it ticks all the boxes and it appears like a trustworthy, genuine news website.
A. Multiple authors
Having multiple authors contribute to your website is hugely valuable, hugely important, and it does tend to elevate you above all the other blogs and small sites that are out there and makes it a bit more likely that the Googler reviewing your site will press that Approve button.
B. Daily updates
Having daily updates definitely is necessary. You don't want just one news post every couple of days. Ideally, multiple new articles every single day that also should be unique. You can have some sort of syndicated content on there, like from feeds, from AP or Reuters or whatever, but the majority of your content needs to be your own unique content. You don't want to rely too much on syndicated articles to fill your website with news content.
C. Mostly unique content
Try to write as much unique content as you possibly can. There isn't really a clear ratio for that. Generally speaking, I recommend my clients to have at least 70% of the content as unique stuff that they write themselves and publish themselves and only 30% maximum syndicated content from external sources.
D. Specialized niche/topic
It really helps to have a specialized niche or a specialized topic that you focus on as a news website. There are plenty of news sites out there that are general news and try to do everything, and Google News doesn't really need many more of those. What Google is interested in is niche websites on specific topics, specific areas that can provide in-depth reporting on those specific industries or topics. So if you have a very niche topic or a niche industry that you cover with your news, it does tend to improve your chances of getting into that News Index and getting that top stories carousel traffic.
So that, in a nutshell, is how you get into Google News. It might appear to be quite simple, but, like I said, quite a few hoops for you to jump through, a few technical things you have to implement on your website as well. But if you tick all those boxes, you can get so much traffic from the top stories carousel, and the rest is profit. Thank you very much.
This has been my Whiteboard Friday.
Further resources:
Google News Help
Publisher Center Help
Google News Initiative
Optimizing for Google News: A SlideShare presentation from Barry's talk at Digitalzone 2018 discussing how to optimize websites for visibility in Google News.
Video transcription by Speechpad.com
Sign up for The Moz Top 10, a semimonthly mailer updating you on the top ten hottest pieces of SEO news, tips, and rad links uncovered by the Moz team. Think of it as your exclusive digest of stuff you don't have time to hunt down but want to read!
2 notes
·
View notes