#laravel session
Explore tagged Tumblr posts
Text
Prevent Session Replay Attacks in Laravel: Best Practices
Introduction
Session replay attacks are a major security risk in web applications, especially in frameworks like Laravel. These attacks can lead to unauthorized access or compromise sensitive user data. In this blog post, we will explore what session replay attacks are, how they occur in Laravel applications, and most importantly, how to prevent them using best practices. We’ll also share a practical coding example to help you implement secure session handling in your Laravel app.

What is a Session Replay Attack?
A Session Replay Attack occurs when an attacker intercepts or steals a valid session ID and reuses it to impersonate the legitimate user. This type of attack exploits the session handling mechanism of web applications and can allow attackers to gain unauthorized access to sensitive information or perform actions on behalf of the user.
In Laravel, session management is a critical aspect of maintaining security, as Laravel uses cookies and sessions to store user authentication and other sensitive data. If the session management is not properly secured, attackers can easily exploit it.
How Session Replay Attacks Work in Laravel
Session replay attacks typically work by capturing a valid session cookie, either through methods like Cross-Site Scripting (XSS) or Man-in-the-Middle (MITM) attacks, and replaying it in their own browser. In Laravel, the session data is stored in cookies by default, so if the attacker gains access to a session cookie, they can replay the session request and hijack the user’s session.
To demonstrate this risk, let’s take a look at how a session ID might be captured and replayed:
// Example of a Laravel session where sensitive information might be stored session(['user_id' => 1, 'role' => 'admin']);
If an attacker intercepts the session cookie (usually via XSS or another method), they could replay the request and access sensitive data or perform admin-level actions.
How to Prevent Session Replay Attacks in Laravel
1. Use HTTPS Everywhere
Ensure that your Laravel application enforces HTTPS to protect session cookies from being intercepted in transit. HTTP traffic is unencrypted, so it's easy for attackers to sniff session cookies. By forcing HTTPS, all communications between the client and server are encrypted.
To enforce HTTPS in Laravel, add this to your AppServiceProvider:
public function boot() { if (env('APP_ENV') !== 'local') { \URL::forceScheme('https'); } }
This will ensure that Laravel always generates URLs using HTTPS.
2. Regenerate Session IDs After Login
One effective way to prevent session hijacking and replay attacks is to regenerate the session ID after the user logs in. This ensures that attackers cannot reuse a session ID that was valid before the login.
In Laravel, you can regenerate the session ID using the following code:
public function authenticated(Request $request, $user) { $request->session()->regenerate(); }
This should be added in your LoginController to regenerate the session after a successful login.
3. Set Secure and HttpOnly Flags on Cookies
Ensure that your session cookies are marked as Secure and HttpOnly. The Secure flag ensures that the cookie is only sent over HTTPS, and the HttpOnly flag prevents JavaScript from accessing the cookie.
In Laravel, you can configure this in the config/session.php file:
'secure' => env('SESSION_SECURE_COOKIE', true), 'http_only' => true,
These settings help protect your session cookies from being stolen via JavaScript or man-in-the-middle attacks.
4. Use SameSite Cookies
The SameSite cookie attribute can help mitigate Cross-Site Request Forgery (CSRF) attacks and prevent the session from being sent in cross-site requests. You can set it in the session configuration:
'samesite' => 'Strict',
This ensures that the session is only sent in requests originating from the same domain, thus reducing the risk of session replay attacks.
5. Enable Session Expiry
You can also mitigate session replay attacks by setting an expiration time for your sessions. Laravel allows you to define the lifetime of your session in the config/session.php file:
'lifetime' => 120, // in minutes 'expire_on_close' => true,
Setting an expiration time ensures that even if a session ID is captured, it will only be valid for a limited period.
Coding Example for Secure Session Handling
Here’s a full example demonstrating how to implement some of these best practices to prevent session replay attacks in Laravel:
// Middleware to regenerate session on each request public function handle($request, Closure $next) { // Regenerate session ID session()->regenerate(); // Set secure cookies config(['session.secure' => true]); config(['session.http_only' => true]); return $next($request); }
By including this middleware in your Laravel app, you can regenerate session IDs on every request and ensure secure cookie handling.
Using the Free Website Security Checker Tool
If you’re unsure whether your Laravel application is susceptible to session replay attacks or other security issues, you can use the Website Vulnerability Scanner tool. This tool analyzes your website for vulnerabilities, including insecure session management, and provides actionable insights to improve your app’s security.

Screenshot of the free tools webpage where you can access security assessment tools.
The free tool provides a comprehensive security analysis that helps you identify and mitigate potential security risks.
Conclusion
Session replay attacks are a serious security threat, but by implementing the best practices discussed above, you can effectively protect your Laravel application. Make sure to use HTTPS, regenerate session IDs after login, and properly configure session cookies to minimize the risk of session hijacking.
To check if your Laravel app is vulnerable to session replay attacks or other security flaws, try out our free Website Security Scanner tool.
For more security tips and blog updates, visit our blog at PentestTesting Blog.

An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities.
By following these security best practices and using the tools available at PentestTesting.com, you can enhance the security of your Laravel application and protect it from session replay attacks.
1 note
·
View note
Text
Top 10 Laravel Development Companies in the USA in 2024
Laravel is a widely-used open-source PHP web framework designed for creating web applications using the model-view-controller (MVC) architectural pattern. It offers developers a structured and expressive syntax, as well as a variety of built-in features and tools to enhance the efficiency and enjoyment of the development process.
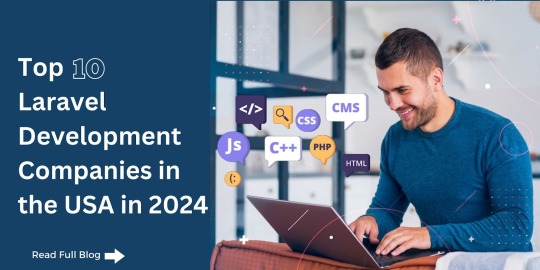
Key components of Laravel include:
1. Eloquent ORM (Object-Relational Mapping): Laravel simplifies database interactions by enabling developers to work with database records as objects through a powerful ORM.
2. Routing: Laravel provides a straightforward and expressive method for defining application routes, simplifying the handling of incoming HTTP requests.
3. Middleware: This feature allows for the filtering of HTTP requests entering the application, making it useful for tasks like authentication, logging, and CSRF protection.
4. Artisan CLI (Command Line Interface): Laravel comes with Artisan, a robust command-line tool that offers commands for tasks such as database migrations, seeding, and generating boilerplate code.
5. Database Migrations and Seeding: Laravel's migration system enables version control of the database schema and easy sharing of changes across the team. Seeding allows for populating the database with test data.
6. Queue Management: Laravel's queue system permits deferred or background processing of tasks, which can enhance application performance and responsiveness.
7. Task Scheduling: Laravel provides a convenient way to define scheduled tasks within the application.
What are the reasons to opt for Laravel Web Development?
Laravel makes web development easier, developers more productive, and web applications more secure and scalable, making it one of the most important frameworks in web development.
There are multiple compelling reasons to choose Laravel for web development:
1. Clean and Organized Code: Laravel provides a sleek and expressive syntax, making writing and maintaining code simple. Its well-structured architecture follows the MVC pattern, enhancing code readability and maintainability.
2. Extensive Feature Set: Laravel comes with a wide range of built-in features and tools, including authentication, routing, caching, and session management.
3. Rapid Development: With built-in templates, ORM (Object-Relational Mapping), and powerful CLI (Command Line Interface) tools, Laravel empowers developers to build web applications quickly and efficiently.
4. Robust Security Measures: Laravel incorporates various security features such as encryption, CSRF (Cross-Site Request Forgery) protection, authentication, and authorization mechanisms.
5. Thriving Community and Ecosystem: Laravel boasts a large and active community of developers who provide extensive documentation, tutorials, and forums for support.
6. Database Management: Laravel's migration system allows developers to manage database schemas effortlessly, enabling version control and easy sharing of database changes across teams. Seeders facilitate the seeding of databases with test data, streamlining the testing and development process.
7. Comprehensive Testing Support: Laravel offers robust testing support, including integration with PHPUnit for writing unit and feature tests. It ensures that applications are thoroughly tested and reliable, reducing the risk of bugs and issues in production.
8. Scalability and Performance: Laravel provides scalability options such as database sharding, queue management, and caching mechanisms. These features enable applications to handle increased traffic and scale effectively.
Top 10 Laravel Development Companies in the USA in 2024
The Laravel framework is widely utilised by top Laravel development companies. It stands out among other web application development frameworks due to its advanced features and development tools that expedite web development. Therefore, this article aims to provide a list of the top 10 Laravel Development Companies in 2024, assisting you in selecting a suitable Laravel development company in the USA for your project.
IBR Infotech
IBR Infotech excels in providing high-quality Laravel web development services through its team of skilled Laravel developers. Enhance your online visibility with their committed Laravel development team, which is prepared to turn your ideas into reality accurately and effectively. Count on their top-notch services to receive the best as they customise solutions to your business requirements. Being a well-known Laravel Web Development Company IBR infotech is offering the We provide bespoke Laravel solutions to our worldwide customer base in the United States, United Kingdom, Europe, and Australia, ensuring prompt delivery and competitive pricing.
Additional Information-
GoodFirms : 5.0
Avg. hourly rate: $25 — $49 / hr
No. Employee: 10–49
Founded Year : 2014
Verve Systems
Elevate your enterprise with Verve Systems' Laravel development expertise. They craft scalable, user-centric web applications using the powerful Laravel framework. Their solutions enhance consumer experience through intuitive interfaces and ensure security and performance for your business.
Additional Information-
GoodFirms : 5.0
Avg. hourly rate: $25
No. Employee: 50–249
Founded Year : 2009
KrishaWeb
KrishaWeb is a world-class Laravel Development company that offers tailor-made web solutions to our clients. Whether you are stuck up with a website concept or want an AI-integrated application or a fully-fledged enterprise Laravel application, they can help you.
Additional Information-
GoodFirms : 5.0
Avg. hourly rate: $50 - $99/hr
No. Employee: 50 - 249
Founded Year : 2008
Bacancy
Bacancy is a top-rated Laravel Development Company in India, USA, Canada, and Australia. They follow Agile SDLC methodology to build enterprise-grade solutions using the Laravel framework. They use Ajax-enabled widgets, model view controller patterns, and built-in tools to create robust, reliable, and scalable web solutions
Additional Information-
GoodFirms : 4.8
Avg. hourly rate: $25 - $49/hr
No. Employee: 250 - 999
Founded Year : 2011
Elsner
Elsner Technologies is a Laravel development company that has gained a high level of expertise in Laravel, one of the most popular PHP-based frameworks available in the market today. With the help of their Laravel Web Development services, you can expect both professional and highly imaginative web and mobile applications.
Additional Information-
GoodFirms : 5
Avg. hourly rate: < $25/hr
No. Employee: 250 - 999
Founded Year : 2006
Logicspice
Logicspice stands as an expert and professional Laravel web development service provider, catering to enterprises of diverse scales and industries. Leveraging the prowess of Laravel, an open-source PHP framework renowned for its ability to expedite the creation of secure, scalable, and feature-rich web applications.
Additional Information-
GoodFirms : 5
Avg. hourly rate: < $25/hr
No. Employee: 50 - 249
Founded Year : 2006
Sapphire Software Solutions
Sapphire Software Solutions, a leading Laravel development company in the USA, specialises in customised Laravel development, enterprise solutions,.With a reputation for excellence, they deliver top-notch services tailored to meet your unique business needs.
Additional Information-
GoodFirms : 5
Avg. hourly rate: NA
No. Employee: 50 - 249
Founded Year : 2002
iGex Solutions
iGex Solutions offers the World’s Best Laravel Development Services with 14+ years of Industry Experience. They have 10+ Laravel Developer Experts. 100+ Elite Happy Clients from there Services. 100% Client Satisfaction Services with Affordable Laravel Development Cost.
Additional Information-
GoodFirms : 4.7
Avg. hourly rate: < $25/hr
No. Employee: 10 - 49
Founded Year : 2009
Hidden Brains
Hidden Brains is a leading Laravel web development company, building high-performance Laravel applications using the advantage of Laravel's framework features. As a reputed Laravel application development company, they believe your web application should accomplish the goals and can stay ahead of the rest.
Additional Information-
GoodFirms : 4.9
Avg. hourly rate: < $25/hr
No. Employee: 250 - 999
Founded Year : 2003
Matellio
At Matellio, They offer a wide range of custom Laravel web development services to meet the unique needs of their global clientele. There expert Laravel developers have extensive experience creating robust, reliable, and feature-rich applications
Additional Information-
GoodFirms : 4.8
Avg. hourly rate: $50 - $99/hr
No. Employee: 50 - 249
Founded Year : 2014
What advantages does Laravel offer for your web application development?
Laravel, a popular PHP framework, offers several advantages for web application development:
Elegant Syntax
Modular Packaging
MVC Architecture Support
Database Migration System
Blade Templating Engine
Authentication and Authorization
Artisan Console
Testing Support
Community and Documentation
Conclusion:
I hope you found the information provided in the article to be enlightening and that it offered valuable insights into the top Laravel development companies.
These reputable Laravel development companies have a proven track record of creating customised solutions for various sectors, meeting client requirements with precision.
Over time, these highlighted Laravel developers for hire have completed numerous projects with success and are well-equipped to help advance your business.
Before finalising your choice of a Laravel web development partner, it is essential to request a detailed cost estimate and carefully examine their portfolio of past work.
#Laravel Development Companies#Laravel Development Companies in USA#Laravel Development Company#Laravel Web Development Companies#Laravel Web Development Services
2 notes
·
View notes
Text
Where Can You Find the Best Web Development Services?
In today’s digital-first world, having a professionally built website is no longer optional—it’s essential. Whether you're a startup or an established enterprise, your website serves as the face of your brand, a sales engine, and a hub for customer engagement. But with thousands of agencies claiming to offer top-tier solutions, where can you truly find the best web development services?
If you’re located in California or looking to work with U.S.-based professionals, web development companies in Sacramento have become a go-to option for businesses of all sizes. Let’s explore what makes Sacramento a hotspot for web development and how to choose the right agency for your needs.
Why Sacramento?
You might think of cities like San Francisco or Los Angeles when considering tech talent, but Sacramento is fast becoming a rising star in the digital services space. Here's why:
Lower overhead costs compared to Silicon Valley
Access to a growing pool of tech talent
Proximity to major business hubs
Thriving community of startups and innovation centers
As a result, web development companies Sacramento based are known for delivering high-quality results with competitive pricing.
What to Look for in a Web Development Company
Finding the “best” web development service means evaluating agencies based on your specific goals. Here’s a checklist to guide you:
1. Experience & Portfolio
Review the agency’s past projects. Do they align with your vision? Have they worked with businesses in your industry?
2. Technology Stack
The best companies use modern tools and frameworks—think React, Vue.js, Laravel, Node.js, and headless CMS solutions.
3. Responsive Design
Your website must look and function perfectly across all devices. Check if the agency prioritizes mobile-first, responsive design.
4. SEO & Performance
Fast load times and search-engine readiness are critical. Many top Sacramento agencies integrate on-page SEO and site optimization as part of the build.
5. Custom Solutions
Avoid one-size-fits-all templates. The best developers create tailored solutions that reflect your brand and meet your goals.
6. Support & Maintenance
A reliable agency will provide post-launch support, updates, and performance monitoring to ensure long-term success.
Top Web Development Services Offered in Sacramento
Here are common services you can expect from leading web development companies Sacramento is home to:
Custom website design & development
E-commerce development (Shopify, WooCommerce, Magento)
Web application development
CMS development (WordPress, Drupal, Webflow)
UI/UX design and prototyping
API integrations and back-end development
SEO and performance optimization
Some agencies also offer branding, content strategy, and digital marketing to give you a comprehensive digital presence.
Benefits of Working with Local Sacramento Web Developers
While remote teams are great, there are clear advantages to partnering with a local Sacramento-based company:
Face-to-face collaboration and strategy sessions
Faster turnaround and responsive communication
Better understanding of the regional market and customer behavior
Supporting local businesses and talent
Final Thoughts
When looking for the best web development services, it’s important to balance technical capabilities, communication, cost, and cultural fit. If you're searching within the United States, web development companies Sacramento consistently stand out for their innovation, reliability, and client-focused approach.
consistently stand out for their innovation, reliability, and client-focused approach.
Whether you're building a new website or revamping an old one, Sacramento has the talent and expertise to bring your digital vision to life.
Ready to Launch Your Next Web Project?
Partner with a top-rated Sacramento web development team and create a website that drives results.
0 notes
Text
Infix LMS Nulled Script 7.2.0

Unlock Premium eLearning with Infix LMS Nulled Script Revolutionize your online education platform with the Infix LMS Nulled Script—a powerful and feature-rich Learning Management System designed to empower educators, institutions, and eLearning startups. Whether you're launching a virtual academy, selling digital courses, or managing students remotely, Infix LMS offers the perfect toolkit to build and scale a professional learning ecosystem. What Is Infix LMS Nulled Script? Infix LMS Nulled Script is the nulled version of the premium Infix Learning Management System. This nulled script removes licensing restrictions, giving users free access to all its premium features. Ideal for startups or developers on a tight budget, this version allows you to test, deploy, and expand your eLearning project without the usual financial burden. Technical Specifications Technology Stack: Laravel 8+, PHP 7.4+, MySQL, Bootstrap 5 Responsive Design: 100% mobile-friendly and optimized for all devices Multi-Language Support: Fully translatable interface with RTL compatibility Role Management: Admin, Instructor, Student & Support Staff roles Payment Gateways: PayPal, Stripe, Razorpay, and more Features and Benefits The Infix LMS Nulled Script comes loaded with cutting-edge features that deliver seamless learning experiences for both instructors and students: Interactive Course Builder: Easily create, manage, and organize courses with multimedia content, quizzes, and certificates. Student Management: Comprehensive dashboard to track progress, attendance, and performance. Live Classes: Integrate Zoom and BigBlueButton for real-time learning sessions. Subscriptions & Monetization: Offer paid courses, subscriptions, or one-time purchases with integrated payment systems. Custom Branding: Fully customizable UI to match your brand identity. Perfect Use Cases Whether you're an independent tutor or managing a full-scale online school, Infix LMS Nulled Script adapts to various eLearning scenarios: Educational Institutions: Schools, colleges, and universities can digitize their curriculum and manage remote learning effortlessly. Corporate Training: Businesses can build internal training portals to upskill employees. Coaches & Freelancers: Share knowledge through paid courses and build an audience-driven business. Easy Installation Guide Installing the Infix LMS Nulled Script is straightforward. Follow these steps: Download the nulled package from our secure server. Unzip and upload the files to your hosting environment. Create a database and import the provided SQL file. Configure the .env file with your database credentials. Run the setup wizard via your browser and follow the prompts. Within minutes, you’ll be ready to launch your own learning platform—completely free and fully functional. Why Choose the Nulled Version? Opting for the Infix LMS Nulled Script gives you access to a premium-grade product without the licensing limitations. This is ideal for testing, development, or running a live project if you're working with budget constraints. You also get the freedom to customize the platform to your specific needs without worrying about vendor lock-in. Frequently Asked Questions (FAQs) Is it safe to use the Infix LMS Nulled Script? Yes, the version available on our site is clean, secure, and free from malicious code. However, always use trusted sources like ours to avoid vulnerabilities. Can I use this nulled script on multiple domains? Absolutely. With the nulled version, there are no licensing restrictions. Deploy it on as many domains as you like. Will I get updates and support? Support is not officially available, but updates may be released on our site periodically. Stay tuned for the latest releases. Get Started Today Unlock the full potential of online education with the Infix LMS.Whether you're launching a solo project or managing thousands of learners, this platform has the features you need to succeed—completely free of charge.
Looking for more powerful tools? Check out Slider Revolution NULLED for stunning visual content or explore WPML pro NULLED for advanced multilingual site functionality.
0 notes
Text
Turn Your Idea Into a Powerful App with Bangalore’s Leading Mobile Developers
In today’s mobile-first world, having a high-performing app isn’t just a competitive advantage—it’s essential. Businesses are rapidly embracing mobile technology to connect with their audience, streamline operations, and boost revenue. But to truly make an impact, you need more than just code—you need the right mobile app development company in Bangalore with a proven track record of delivering results.
Our Experience: Built on Real-World Results
With over a decade of experience in mobile app development, our Bangalore-based team has delivered 250+ successful projects across industries such as fintech, healthcare, e-commerce, education, logistics, and more. Our hands-on experience ensures that every app we design and develop is rooted in business logic, user behavior, and market demand. We don't believe in one-size-fits-all solutions. Whether you're a funded startup or a large enterprise, we build custom mobile apps that align with your specific business goals.
Our Expertise: From Concept to Code and Beyond
What makes us a top mobile app development company in Bangalore is our multidisciplinary team of experts. Our developers are proficient in native platforms (iOS, Android) and cross-platform frameworks like Flutter, React Native, and Kotlin Multiplatform. But we go beyond just writing code.
UI/UX Design: Seamless, intuitive, and user-centric interfaces.
Backend Architecture: Scalable and secure APIs built with Node.js, Django, or Laravel.
Agile Methodology: Fast iterations, continuous feedback, and transparent processes.
App Store Deployment: Smooth launch on Apple App Store and Google Play Store.
Every project includes quality assurance, performance testing, and post-launch support—because we’re invested in your long-term success.
Our Authoritativeness
Over the years, we've built more than apps—we've built trust. Our portfolio includes collaborations with high-growth startups and Fortune 500 companies, many of whom have seen measurable results after launch.
We've been featured in top B2B review platforms like Clutch, GoodFirms, and DesignRush as one of the top mobile app development companies in Bangalore. Client testimonials highlight our commitment to deadlines, product quality, and innovation-driven mindset.
Our Trustworthiness
Trust is the cornerstone of every successful tech partnership. That’s why we maintain complete transparency in communication, pricing, and development timelines. Every project starts with a discovery session where we understand your goals, followed by clear milestones, timelines, and deliverables.
We also sign NDAs, follow GDPR compliance, and offer IP protection—so your app idea stays secure from start to finish.
Let’s Build Something That Delivers Real Results
Your business deserves more than an average app. It deserves a digital product that drives growth, delights users, and makes an impact. If you’re looking for a mobile app development company in Bangalore that combines experience, technical expertise, and genuine partnership—we’d love to work with you.
0 notes
Text
Exploring the Tools and Technologies Behind Full Stack Web Development
In today’s digital age, having a strong online presence is no longer optional—it’s essential. Whether it’s an e-commerce store, a mobile app, or a blog, the foundation behind any successful web product is solid web development. Among the most in-demand areas in this field is full stack web development. If you're curious about how websites are built from the ground up, or you’re planning to step into the world of programming, this guide is for you.
Here, we’ll be exploring the tools and technologies behind full stack web development, and also take a closer look at how training platforms like full stack by TechnoBridge are shaping the developers of tomorrow.
What is Full Stack Web Development?
Full stack web development refers to the development of both front-end (what users see) and back-end (how things work behind the scenes) parts of a web application. A full stack developer is someone who has the skills and knowledge to work on both ends of the system.
This includes:
Creating user interfaces with HTML, CSS, and JavaScript
Managing server-side logic using frameworks like Node.js or Django
Storing and retrieving data from databases such as MongoDB or MySQL
Version control using Git and GitHub
Deploying apps using platforms like Heroku or AWS
It’s an exciting field where you get to build a complete product and not just a part of it. The sense of ownership and creativity in this space is unmatched.
Key Technologies Used in Full Stack Development
Let’s break down the stack into its major components and explore the popular tools and technologies developers use today:
1. Front-End Technologies (Client Side)
These tools create everything the user interacts with:
HTML5: Structure of the web pages
CSS3: Styling and layout
JavaScript: Interactive elements
Frameworks: React.js, Angular, Vue.js – for building dynamic user interfaces
2. Back-End Technologies (Server Side)
These tools handle the logic and database interactions:
Languages: Node.js (JavaScript), Python, Ruby, PHP, Java
Frameworks: Express.js, Django, Laravel, Spring Boot
APIs: RESTful and GraphQL for connecting front-end with back-end
3. Database Management
Store and retrieve data:
SQL Databases: MySQL, PostgreSQL
NoSQL Databases: MongoDB, CouchDB
4. Version Control and Collaboration
Keep track of changes and work in teams:
Git: Local version control
GitHub/GitLab: Cloud repositories and collaboration
5. Deployment & DevOps Tools
Make your app live and maintain it:
Cloud Platforms: AWS, Azure, Google Cloud
Containerization: Docker
CI/CD Tools: Jenkins, GitHub Actions
Why Full Stack Development is in Demand
With companies increasingly leaning on digital platforms, the demand for professionals who can understand both the client and server sides of development is soaring. Being a full stack web developer means you can:
Build complete web applications independently
Troubleshoot issues across the entire stack
Communicate effectively with both design and backend teams
Offer more value to startups and small businesses
And that’s where training programs like full stack by TechnoBridge come into play.
Learning Full Stack Development with TechnoBridge
If you're planning to start a career in web development or upskill your current profile, full stack by TechnoBridge is a solid place to begin. The program is carefully crafted to guide beginners and intermediates through each layer of full stack web development.
Here’s what you can expect from full stack by TechnoBridge:
Hands-on projects that simulate real-world scenarios
Mentorship from industry experts
Training in both front-end and back-end frameworks
Exposure to DevOps and deployment techniques
Job-oriented sessions with placement support
Such training ensures that you're not just learning to code, but you're learning to build and deliver products that users can interact with seamlessly.
Final Thoughts
As we continue to rely more on digital solutions, full stack developers will remain at the heart of innovation. Whether you’re dreaming of building your own product, working in a startup, or joining a tech giant, understanding the full stack web landscape is your key to staying relevant and competitive.
If you’re looking to dive into this journey, consider programs like full stack by TechnoBridge to gain practical skills, confidence, and a strong professional foundation.
0 notes
Text
PHP Training in Chandigarh – A Complete Guide for Aspiring Web Developers
In the rapidly evolving landscape of web development, PHP remains a foundational technology powering millions of websites globally. From WordPress to Facebook (in its early years), PHP has proved to be a robust and versatile scripting language. Chandigarh, being a prominent educational and IT hub in Northern India, has become a go-to destination for students and professionals seeking high-quality PHP training. This article delves into everything you need to know about PHP training in Chandigarh, from its significance to career prospects and the best training institutes.
Why Learn PHP?
PHP (Hypertext Preprocessor) is a server-side scripting language primarily used for web development. It's open-source, easy to learn, and has extensive support from the developer community. Here are a few reasons why learning PHP is a smart choice:
Widely Used: Over 75% of websites that use server-side scripting languages still rely on PHP.
Open Source: No licensing fees make it cost-effective for individuals and startups.
Integration Friendly: PHP works seamlessly with databases like MySQL, PostgreSQL, and Oracle.
Flexible and Scalable: From simple landing pages to complex enterprise web applications, PHP scales well.
High Demand: Despite the emergence of new languages, PHP developers remain in high demand globally.
The Growing IT Scene in Chandigarh
Chandigarh has steadily emerged as a major center for IT education and development. The presence of IT parks, MNCs, and local startups has fueled demand for skilled developers. With a rising number of digital marketing agencies, software houses, and web development companies in Mohali, Panchkula, and Chandigarh, PHP training institutes have become a critical part of the local educational ecosystem.
Who Should Take PHP Training?
PHP training is suitable for:
Students pursuing B.Tech, BCA, MCA, or M.Sc. (IT)
Fresh graduates aiming to build a career in web development
Working professionals who want to upskill or shift to backend development
Entrepreneurs and freelancers looking to create and manage their own websites
No prior programming experience is required for beginners' courses, making PHP an accessible entry point into the tech industry.
PHP Training Curriculum – What You Will Learn
A comprehensive PHP training course typically includes both core and advanced topics. Here's a breakdown of a standard PHP training curriculum in Chandigarh:
1. Introduction to Web Development
Basics of HTML, CSS, JavaScript
Understanding client-server architecture
2. Core PHP
Syntax, variables, and data types
Control structures: loops, if/else, switch
Functions and arrays
Form handling
Sessions and cookies
3. Database Integration
Introduction to MySQL
CRUD operations using PHP and MySQL
Database connectivity and configuration
4. Advanced PHP
Object-Oriented Programming (OOP) in PHP
Error and exception handling
File handling and data encryption
PHP security best practices
5. Frameworks and CMS (Optional but Valuable)
Introduction to Laravel or CodeIgniter
Basics of WordPress development
MVC architecture
6. Live Projects and Internships
Real-time project development
Deployment on live servers
Version control (Git basics)
Key Features of PHP Training Institutes in Chandigarh
When choosing a training institute in Chandigarh for PHP, consider the following features:
Experienced Trainers: Trainers with industry experience can bridge the gap between theoretical knowledge and practical application.
Hands-on Training: Good institutes emphasize coding, not just theory.
Live Projects: Implementing real-world projects enhances understanding and employability.
Placement Assistance: Many institutes offer job support through resume building, mock interviews, and tie-ups with local companies.
Flexible Timings: Options for weekend or evening batches are a boon for working professionals and students.
Top Institutes Offering PHP Training in Chandigarh
Here are some of the reputed institutes offering PHP training in Chandigarh:
1. Webtech Learning
Located in Sector 34, Webtech Learning offers a well-rounded PHP training program with live projects and job assistance. They are known for their experienced faculty and industry connections.
2. Chandigarh Institute of Internet Marketing (CIIM)
CIIM offers specialized PHP and web development training with certification and job placement support. They focus heavily on project-based learning.
3. ThinkNEXT Technologies
Located in Mohali, ThinkNEXT is an ISO-certified training institute offering comprehensive PHP training with internships and certifications.
4. Morph Academy
Morph Academy offers PHP training with a focus on web design, development, and integration with other technologies like WordPress and Laravel.
5. Netmax Technologies
Another well-known institute offering hands-on PHP training with flexible course durations and career counseling services.
Duration and Fees
The duration of PHP training courses in Chandigarh typically ranges from 1 to 6 months, depending on the course depth and inclusion of frameworks or internships. Short-term crash courses may also be available for those looking to learn quickly.
Basic Course (1–2 months): ₹8,000 – ₹12,000
Advanced Course (3–6 months): ₹15,000 – ₹25,000
Some institutes offer EMI options and combo packages with other web technologies like JavaScript, React, or Node.js.
Career Opportunities After PHP Training
PHP opens up several career paths in web development. Here are some roles you can apply for after completing your training:
PHP Developer
Web Developer
Backend Developer
Full Stack Developer (with knowledge of frontend tools)
WordPress Developer
Software Engineer (Web Applications)
Popular companies in Chandigarh, Mohali, and Panchkula that frequently hire PHP developers include Net Solutions, IDS Infotech, SmartData Enterprises, and Webdew.
Freelancing and Entrepreneurship
PHP is not just for job seekers. Many developers work as freelancers on platforms like Upwork, Freelancer, and Fiverr. If you have an entrepreneurial mindset, you can build your own websites, e-commerce stores, or even SaaS platforms using PHP and open-source tools.
Certification and Resume Building
Upon completion of PHP training, most institutes provide a certificate that adds credibility to your resume. However, what matters most to employers is your portfolio – the projects you’ve built and the skills you demonstrate in interviews.
Make sure your resume includes:
Technical skills (PHP, MySQL, HTML, CSS, JavaScript, etc.)
Live project links (GitHub or hosted sites)
Internship experiences (if any)
Certifications
Conclusion
PHP training in Chandigarh is an excellent investment for anyone looking to enter the web development field. With the city's growing IT ecosystem and the availability of high-quality training institutes, you can gain both the knowledge and practical experience required to start a successful career. Whether you're a student, job seeker, or freelancer, learning PHP can open the doors to numerous opportunities in the digital world.
0 notes
Text
Laravel Training Institute in Indore – Build Scalable Web Applications with Expert Guidance
Introduction: Step Into the Future of Web Development with Laravel
Laravel is among the most powerful PHP frameworks used for building dynamic, secure, and scalable web applications. Whether you're a budding developer or a working professional aiming to upgrade your skills, joining a structured Laravel training in Indore can significantly boost your career. Infograins TCS helps you build a strong foundation in Laravel with real-time projects, hands-on sessions, and industry exposure.

Overview: What Our Laravel Training Program Covers
At Infograins TCS, we offer a comprehensive Laravel training program designed to help learners understand both fundamental and advanced concepts. From routing, middleware, and templating to Eloquent ORM and RESTful APIs, every essential topic is covered under expert supervision. Our course structure is practical and job-oriented, making us a preferred Laravel training institute in Indore for aspiring developers.
Pros: Why Laravel Training Is a Game-Changer
Industry-Relevant Curriculum – Our syllabus is aligned with current market demands, covering real-time scenarios.
Hands-On Projects – Practical sessions and live assignments ensure skill development and confidence.
Career Support – Resume building, mock interviews, and job placement support are part of the program.
Expert Faculty – Learn from industry professionals with real project experience in Laravel and PHP.
This Laravel training in Indore is perfect for those seeking hands-on experience with a framework that powers high-performing web apps.
Why Choose Us: Your Learning Partner at Every Step
Infograins TCS is known for its commitment to quality education and student satisfaction. With a focus on personalized learning and practical training, we ensure that every student is ready to face real-world development challenges. As a top Laravel training institute in Indore, we emphasize industry-ready skills that make our learners job-ready from day one.
Our More Courses: Diversify Your IT Skills
Apart from Laravel, we offer a range of career-boosting IT training programs including:
PHP Core & Advanced Training
Full Stack Development
Node.js & Express Training
Python & Django Training
Front-End Technologies (React, Angular, Vue.js) These programs are designed to make you a versatile developer with in-demand technical skills.
Why We Are a Helping Partner in Your Career Growth
At Infograins TCS, we’re more than a training institute—we’re your career partner. From counseling to course completion, we guide you every step of the way. Our strong industry network and placement support system ensure you don't just learn Laravel but also land a job that values your skills and dedication.
FAQS : Frequently Asked Questions
1. What is the duration of the Laravel training course? Our Laravel training course typically spans 6 to 8 weeks, with both weekday and weekend batches available for your convenience.
2. Do I need prior knowledge of PHP before joining? Yes, a basic understanding of PHP is recommended since Laravel is a PHP framework. We also offer a PHP fundamentals course for beginners.
3. Will I get to work on live projects during the course? Absolutely! Our course includes multiple live projects and assignments to provide practical exposure and build real-world coding confidence.
4. Is there placement assistance after course completion? Yes, we provide job placement support including resume preparation, mock interviews, and connections with hiring partners.
5. Can I opt for online Laravel training sessions? Yes, we offer both classroom and online training modes to cater to local and remote learners.
Ready to Master Laravel with Experts?
If you're looking to accelerate your web development career, now’s the time to enroll in Laravel training that delivers results. Infograins TCS offers the perfect platform to gain practical knowledge and industry skills. Visit Infograins TCS to register and take the first step toward becoming a professional Laravel developer.
0 notes
Text
Sky Appz Academy: Best Full Stack Development Training in Coimbatore
Revolutionize Your Career with Top-Class Full Stack Training
With today's digital-first economy, Full Stack developers have emerged as the pillars of the technology sector. Sky Appz Academy in Coimbatore is at the cutting edge of technology training with a full-scale Full Stack Development course that makes beginners job-ready professionals. Our 1000+ hour program is a synergy of theoretical training and hands-on practice, providing students with employers' sought skills upon graduation.
Why Full Stack Development Should be Your Career?
The technological world is transforming at a hitherto unknown speed, and Full Stack developers are the most skilled and desired experts in the job market today. As per recent NASSCOM reports:
High Demand: There is a 35% year-over-year rise in Full Stack developer employment opportunities
Lucrative Salaries: Salary ranges for junior jobs begin from ₹5-8 LPA, while mature developers get ₹15-25 LPA
Career Flexibility: Roles across startups, businesses, and freelance initiatives
Future-Proof Skills: Full Stack skills stay up-to-date through technology changes
At Sky Appz Academy, we've structured our course work to not only provide coding instructions, but also to develop problem-solving skills and engineering thinking necessary for long-term professional success.
In-Depth Full Stack Course
Our carefully structured program encompasses all areas of contemporary web development:
Frontend Development (300+ hours)
•Core Foundations: HTML5, CSS3, JavaScript (ES6+)
•Advanced Frameworks: React.js with Redux, Angular
•Responsive Design: Bootstrap 5, Material UI, Flexbox/Grid
•State Management: Context API, Redux Toolkit
•Progressive Web Apps: Service workers, offline capabilities
Backend Development (350+ hours)
•Node.js Ecosystem: Express.js, NestJS
•Python Stack: Django REST framework, Flask
•PHP Development: Laravel, CodeIgniter
•API Development: RESTful services, GraphQL
•Authentication: JWT, OAuth, Session management
Database Systems (150+ hours)
•SQL Databases: MySQL, PostgreSQL
•NoSQL Solutions: MongoDB, Firebase
•ORM Tools: Mongoose, Sequelize
•Database Design: Normalization, Indexing
•Performance Optimization: Query tuning, caching
DevOps & Deployment (100+ hours)
•Cloud Platforms: AWS, Azure fundamentals
•Containerization: Docker, Kubernetes basics
•CI/CD Pipelines: GitHub Actions, Jenkins
• Performance Monitoring: New Relic, Sentry
• Security Best Practices: OWASP top 10
What Sets Sky Appz Academy Apart?
1)Industry-Experienced Instructors
• Our faculty includes senior developers with 8+ years of experience
• Regular guest lectures from CTOs and tech leads
• 1:1 mentorship sessions for personalized guidance
Project-Based Learning Approach
• 15+ mini-projects throughout the course
• 3 major capstone projects
• Real-world client projects for select students
• Hackathons and coding competitions
State-of-the-Art Infrastructure
• Dedicated coding labs with high-end systems
• 24/7 access to learning resources
• Virtual machines for cloud practice
•\tNew software and tools
Comprehensive Career Support
•Resume and LinkedIn profile workshops
•Practice technical interviews (100+ held every month)
•Portfolio development support
•Private placement drives with 150+ recruiters
•Access to alumni network
Detailed Course Structure
•Month 1-2: Building Foundations
•Web development basics
•JavaScript programming logic
•Version control using Git/GitHub
•Algorithms and data structures basics
Month 3-4: Core Development Skills
•Frontend frameworks in-depth
•Backend architecture patterns
•Database design and implementation
•API development and integration
Month 5-6: Advanced Concepts & Projects
•Microservices architecture
•Performance optimization
•Security implementation
•Deployment strategies
•Capstone project development
Career Outcomes and Placement Support
•Our graduates have been placed successfully in positions such as:
•Full Stack Developer
•Frontend Engineer
•Backend Specialist
•Web Application Developer
•UI/UX Engineer
•Software Developer
Placement Statistics (2024 Batch):
•94% placement rate within 3 months
•Average starting salary: ₹6.8 LPA
•Highest package: ₹14.5 LPA
•150+ hiring partners including startups and MNCs
Our placement cell, dedicated to serving our students, offers:
•Regular recruitment drives
•Profile matching with company needs
•Salary negotiation support
•Continuous upskilling opportunities
Flexible Learning Options
•Understanding the varied needs of our students, we provide:
•Weekday Batch: Monday-Friday (4 hours/day)
• Weekend Batch: Sat-Sun (8 hours/day)
• Hybrid Model: Blend online and offline learning
• Self-Paced Option: For working professionals
Who Should Enroll?
Our course is perfect for:
• Fresh graduates interested in tech careers
• Working professionals who wish to upskillCareer changers joining IT field
• Entrepreneurs to create their own products
• Freelancers who wish to increase service offerings
Admission Process
Application: Fill online application
Counseling: Career counseling session
Assessment: Simple aptitude test
Enrollment: Payment of fees and onboarding
EMI options available
Scholarships for deserving students
Group discounts applicable
Why Coimbatore for Tech Education?
•Coimbatore has become South India's budding tech hub with:
•300+ IT organizations and startups
•Lower cost of living than metros
•Vibrant developer community
•Very good quality of life
Take the First Step Toward Your Dream Career
Sky Appz Academy's Full Stack Development course is not just a course - it is a career change experience. With our industry-relevant course material, experienced mentors, and robust placement assistance, we bring you all it takes to shine in the modern-day competitive tech industry.
Limited Seats Left! Come over to our campus at Gandhipuram or speak with one of our counselors today to plan a demo class and see how we can guide you to become successful in technology.
Contact Information:
Sky Appz Academy
123 Tech Park Road, Gandhipuram
Coimbatore - 641012
Website: www.skyappzacademy.com
Frequently Asked Questions
Q: Do we need programming background?
A: No, but basic computer know-how is required.
Q: What is the class size?
A: We maintain 15:1 student-teacher ratio for personalized attention.
Q: Do you provide certification?
A: Yes, course completion certificate with project portfolio.
Q: Are there installment options?
A: Yes, we offer convenient EMI plans.
Q: What if I miss classes?
A: Recorded sessions and catch-up classes are available.
Enroll Now!
By
Skyappzacademy
0 notes
Text
Prevent Session Fixation in Laravel: A Guide with Code Example
Introduction to Session Fixation in Laravel
Session fixation is a security vulnerability that allows an attacker to fix the session ID of a user before they log in. This exploit can lead to session hijacking, where an attacker takes control of a user's session and potentially gains access to sensitive data.

In this blog post, we will walk you through how to prevent session fixation in Laravel, a popular PHP framework, with easy-to-understand coding examples.
What is Session Fixation?
In session fixation attacks, the attacker provides a user with a session ID (usually through a URL or cookie) that the user unknowingly uses. Once the user logs in, the attacker can hijack their session and perform malicious actions, thinking they are the legitimate user.
Why is Session Fixation Dangerous?
If attackers successfully execute a session fixation attack, they can access personal data, modify account settings, or even steal sensitive information like login credentials or payment details. This could lead to severe data breaches and compromise the integrity of your application.
Laravel's Built-in Protection Against Session Fixation
Laravel comes with built-in security features, but there are specific steps you can take to prevent session fixation more effectively.
Step 1: Regenerate Session ID After Login
Laravel allows you to regenerate the session ID after the user logs in, which is an effective way to prevent session fixation. This can be done using the Regenerate method.
Here’s a simple example of how to regenerate the session ID when a user logs in:
use Illuminate\Support\Facades\Auth; use Illuminate\Support\Facades\Session; public function login(Request $request) { $credentials = $request->only('email', 'password'); if (Auth::attempt($credentials)) { // Regenerate session ID to prevent session fixation Session::regenerate(); // Redirect the user to their intended page return redirect()->intended('/home'); } return redirect()->back()- >withErrors(['Invalid credentials']); }
In the example above, after the user successfully logs in, the Session::regenerate() method regenerates the session ID, thus preventing the attacker from using a pre-set session ID.
Step 2: Use Secure Cookies
Another way to secure the session in Laravel is to make sure that cookies are only sent over secure HTTPS connections. This can be easily enabled by modifying your config/session.php file:
// config/session.php 'secure' => env('SESSION_SECURE_COOKIE', true),
This ensures that session cookies are only transmitted over HTTPS, preventing attackers from capturing session cookies over unsecured HTTP connections.
Step 3: Set the HTTPOnly Flag
Make sure that the session cookie is flagged as HttpOnly, which prevents JavaScript from accessing the session cookie.
Add this to your config/session.php file:
// config/session.php 'http_only' => true,
This ensures that your session cookie is not vulnerable to XSS (Cross-Site Scripting) attacks, further improving security.
Test Your Website for Session Fixation Vulnerabilities
You can easily check if your website is vulnerable to session fixation using our free Website Security Scanner tool. Simply input your website URL and let our tool scan for common vulnerabilities, including session fixation.

Screenshot of the free tools webpage where you can access security assessment tools.
Once the scan is complete, you’ll receive a comprehensive report with detailed information on any vulnerabilities found.
Example Vulnerability Report
Here’s a sample vulnerability assessment report generated by our tool. It highlights various vulnerabilities and provides guidance on how to resolve them.

An example of a vulnerability assessment report generated with our free tool provides insights into possible vulnerabilities.
By using the tool, you can ensure that your Laravel application is protected against session fixation and other common security issues.
Conclusion
Preventing session fixation is a crucial part of securing your Laravel application. By regenerating session IDs after login, using secure cookies, and setting the HttpOnly flag, you can significantly reduce the risk of session hijacking.
For more advanced security checks, use our tool to test website security free to scan your site for potential vulnerabilities.
Stay secure and keep your users’ data safe by implementing these practices in your Laravel application.
If you find this guide helpful, feel free to share it with your friends and colleagues. For more tips on web security, follow us on Twitter or LinkedIn.
#cyber security#cybersecurity#data security#pentesting#security#the security breach show#laravel#session
1 note
·
View note
Text
Behind the Scenes of Food Delivery App Development and Its Backend Technical Breakdown

Ever wondered what fuels your food orders behind the scenes? This Food Delivery App Development Guide uncovers the backend magic, key models, and cost factors inspiring your next tech move.
What really happens behind the curtain of food delivery app development?
It’s more than just “order and deliver,” it’s a symphony of code, cloud, and consumer behavior.
You tap a screen, and voilà! A hot pizza lands at your door in 30 minutes. Seems magical, right? But beneath that clean, user-friendly interface is an orchestra of backend brilliance; databases humming, APIs talking, GPS tracking ticking like clockwork.
Welcome to the unseen world of food delivery app development where every second counts, and every click is backed by thousands of lines of code.
In this Food Delivery App Development Guide, we take you behind the kitchen doors of app engineering, revealing how a top food delivery app development company builds, launches, and scales powerful delivery platforms.
“A successful food delivery app isn’t just about UX/UI; it’s about syncing real-world logistics with digital precision in real time.”
Why is backend architecture the unsung hero?
Think of the backend like the heart of a high-performance kitchen. While customers interact with the shiny menu (frontend), the backend makes the magic happen: managing users, processing payments, routing orders, and updating delivery status in milliseconds.
This is where frameworks like Node.js, Django, or Laravel come in, paired with cloud infrastructures like AWS, Google Cloud, or Azure for scalability. Real-time communication, geolocation, and predictive analytics? That’s all handled in the backend.
And don’t even get us started on load balancing during peak meal hours, when everyone’s ordering dinner at once!
Here’s what a typical backend system must handle:
User authentication & session management
Menu sync and order logic
Payment processing with PCI compliance
Real-time GPS tracking for delivery agents
Push notifications and SMS updates
Feedback and review integration
Admin panel with analytics and business controls
All of this needs to run fast, secure, and scalable. And that’s just the beginning.
What are the different types of food delivery app models, and how do they affect backend development?
Not all food delivery apps are built the same, and that changes everything.
Just like there’s a difference between fine dining and fast food, there’s a huge difference between how different types of food delivery app models operate. Your backend architecture, cost, and scalability all hinge on which model you go with.
Let’s break them down.
1. Order-Only Model (Aggregator)
Think: Zomato, Yelp
In this model, your app serves as a directory of restaurants where users browse, choose, and place an order but the restaurants handle the delivery themselves. Backend here focuses on user flow, restaurant listings, reviews, and menu management.
Less complex logistics.
Heavy focus on review and discovery algorithms.
2. Order + Delivery Model (Logistics Focused)
Think: Uber Eats, DoorDash
Here, your app is responsible for both ordering and delivery, making backend complexity shoot up.
Need real-time driver assignment algorithms
Integration with delivery tracking
Complex backend for managing delivery radius, ETA, and driver incentives
“This model requires a robust dispatch system that mimics the precision of ride-hailing apps but faster.”
3. Full-Stack Model (Cloud Kitchens)
Think: Rebel Foods, Faasos
The business owns the entire food chain, kitchen to doorstep. Here, the backend needs to integrate kitchen inventory systems, chef dashboards, and production analytics.
Full control, full responsibility.
Complex backend logic meets physical kitchen workflows.
How does backend complexity influence food delivery app development cost?
The more brains in the backend, the higher the budget
We get asked this all the time: “What’s the real food delivery app development cost?”
Well, the answer is, it depends. On features, model, integrations, scale, and most importantly, the backend.
A rough breakdown of food delivery app development cost:
Basic Aggregator App: $10,000 — $25,000
Order + Delivery Model: $30,000 — $70,000
Full-Stack Cloud Kitchen Platform: $60,000 — $120,000+
Keep in mind, this doesn’t include ongoing server costs, maintenance, or updates. You’re not just building an app, you’re building a living ecosystem.
Where does most of the cost go?
Backend engineering & API integrations
Server architecture for scalability
Security protocols and payment gateway compliance
Real-time systems: Chat, notifications, tracking
“A $30,000 backend today can save you $300,000 in scaling headaches tomorrow.”
What tools, tech stacks, and APIs power a modern food delivery app backend?
Your backend stack is your secret sauce.
Just like a kitchen needs the right knives, your backend needs the right tech. Choosing the wrong tools can burn your budget and your user experience.
Popular backend stacks for food delivery apps development guide:
Node.js + Express.js: real-time, scalable
Django + Python: fast development, security-first
Laravel + PHP: great for MVPs and modular builds
Pair them with:
PostgreSQL or MongoDB for data storage
Redis for caching and lightning-fast speed
Firebase or Twilio for chat & notifications
Stripe, Razorpay for secure payments
Must-have 3rd-party API integrations:
Google Maps API: For geolocation and route mapping
SendGrid / Twilio: For SMS and email notifications
Stripe / PayPal / Razorpay: For payments
ElasticSearch: For lightning-fast search results
AWS S3 / Cloudinary: For media storage
Backend DevOps you can’t ignore:
CI/CD pipelines for smooth updates
Docker/Kubernetes for container orchestration
Load balancing to handle traffic surges
Monitoring tools like New Relic or Datadog
These aren’t just buzzwords, they’re the digital equivalent of hiring a Michelin-starred chef for your app’s kitchen.
How do you optimize performance, scalability, and reliability in food delivery apps?
Achieving flawless performance is no accident; it’s an art.
The difference between a viral app and one that crashes on Friday night dinner rush? Architecture.
When it comes to food delivery apps development guide, performance isn’t just about speed; it’s about predictability and efficiency at scale. To stay competitive, especially in a saturated market, your app needs to perform well under varying loads and unpredictable surges, like during lunch hours or special offers.
If your app is sluggish, unresponsive, or crashes under heavy load, it’s more than a bad user experience, it’s a lost customer. And that loss of trust can be costly.
Performance Optimization Strategies:
1: Database Query Optimization:
Food delivery apps rely heavily on database queries for everything; from pulling restaurant menus to tracking orders. Slow queries can bring down performance. Optimizing these queries- indexing tables, reducing join complexity, and using caching mechanisms like Redis ensures quick response times even with large datasets.
2: Data Caching:
Instead of fetching the same data from the database every time, caching frequently accessed data can drastically speed up the app. For example, caching restaurant menus, popular dishes, and user profiles reduces the load on the server, while improving app speed. Tools like Redis or Memcached are excellent for caching.
3: Load Balancing:
To avoid a server crash when user demand spikes, use load balancing to distribute traffic across multiple servers. Auto-scaling ensures your app can handle traffic surges (e.g., during lunch rush or major promotions). Cloud providers like AWS, Azure, and Google Cloud offer auto-scaling features that dynamically adjust based on real-time traffic.
4: Minimizing API Latency:
APIs are at the heart of food delivery apps development guide interactions for payments, geolocation, and order management. Optimizing API calls and minimizing latency is crucial for real-time operations. Reduce the number of unnecessary API calls and compress data to optimize speed. GraphQL is also a good alternative to REST APIs, as it allows you to fetch only the data you need.
Strategies for rock-solid backend performance:
Scalability is about ensuring your app doesn’t break under increasing demands. Whether you’re growing your user base, expanding into new cities, or dealing with new features like real-time tracking and live chat, scalability is key to future-proofing your app. But scaling isn’t just about adding more resources; it’s about architecting your app in a way that allows it to grow effortlessly.
Microservices architecture: Divide backend functions into small, manageable services (auth, orders, tracking, etc.)
Cloud-based auto-scaling: Scale servers dynamically as traffic increases
CDNs: Use Content Delivery Networks to reduce latency
Caching: Cache frequently used data like menu items, restaurant listings, etc.
Scalability Optimization Strategies:
1: Microservices Architecture:
Scaling traditional monolithic apps can be cumbersome, especially when you add more users or features. By breaking down your backend into microservices (individual, decoupled services for payment, tracking, notifications, etc.), you can scale each service independently based on demand. This allows faster deployment, better fault isolation, and smoother scaling of individual components.
2: Cloud Infrastructure:
Leveraging cloud-based infrastructure for auto-scaling ensures that your app can handle increased load without impacting user experience. Cloud services like AWS, Azure, and Google Cloud allow you to use elastic load balancing, auto-scaling groups, and serverless computing to handle spikes in traffic efficiently.
3: Database Sharding and Partitioning:
As your app scales, your database will become more strained. Database sharding (splitting large databases into smaller, more manageable pieces) ensures data is distributed across multiple servers, making it more efficient and faster to access. It reduces bottlenecks and ensures data scalability in case of heavy traffic.
4: CDNs (Content Delivery Networks):
Use CDNs (such as Cloudflare or AWS CloudFront) to cache static content like images, menus, and other media files closer to the user’s location. This dramatically reduces latency and improves page load times. It’s crucial for scaling without overloading your original server.
Reliability: Keeping your app up and running smoothly
Reliability is all about uptime, availability, and redundancy. In food delivery, even a few minutes of downtime can result in lost orders, frustrated customers, and a damaged reputation. You need to ensure your app remains operational even in the event of a failure.
Disaster Recovery and Backup Systems:
A critical part of reliability is having a disaster recovery plan in place. Automated backups of databases and server snapshots ensure that in the event of a crash, you can restore data and bring the app back up within minutes. Regular testing of disaster recovery plans is also essential.
Fault Tolerance via Redundancy:
A reliable app needs to be fault tolerant. This means setting up redundant systems so if one part of the system fails, there’s another part to take over. Using multiple server instances in different geographic regions ensures that, even if one server fails, others continue serving your users without disruption.
Monitoring Tools:
Real-time monitoring tools like Datadog, New Relic, or Prometheus can track your app’s performance and alert you to issues before they affect users. These tools help you identify and resolve performance bottlenecks, security vulnerabilities, and other issues quickly, ensuring high availability at all times.
Continuous Deployment and Testing:
CI/CD pipelines (Continuous Integration/Continuous Deployment) allow you to release updates without interrupting service. Automated testing ensures that new code doesn’t introduce bugs, and the app remains reliable even after updates.
Real-World Example: Scaling and Optimizing Food Delivery App Performance
We worked with a fast-growing food delivery startup that was struggling with performance issues during peak hours. They were using a monolithic architecture, which caused slowdowns when thousands of users were simultaneously placing orders.
Solution:
Migrated them to a microservices architecture.
Optimized their database queries by indexing and caching.
Integrated AWS auto-scaling to handle traffic surges.
Result:
App response time decreased by 70% during high traffic periods.
Uptime improved to 99.99%, with zero service disruptions during scaling.
Real-world case study:
We helped a mid-tier food delivery app go from 300 to 10,000 orders/day by optimizing:
Their order assignment algorithm
Real-time location tracking via Redis streams
Server load balancing with AWS Elastic Load Balancer
Results? 80% faster performance, zero downtime, and increased retention.
Want a deeper dive into features, costs, and models?
Take a bite out of our in-depth blog right here Food Delivery App Development Guide, the ultimate blueprint for entrepreneurs ready to launch or scale their food tech vision.
Conclusion: What’s cooking in the backend defines your food app’s success
The future of food delivery isn’t just in the flavor, it’s in the functionality. In a world where customer patience is thinner than a pizza crust, your backend needs to be fast, reliable, and scalable.
Whether you’re eyeing an MVP or going full-stack cloud kitchen mode, your backend architecture isn’t just a technical detail, it’s your business backbone.
So, the next time someone says, “It’s just a food app,” hand them this guide. Because now you know what it really takes.
0 notes
Text
The Best PHP Course in Coimbatore to Boost Your Programming Skills
Introduction
PHP is a powerful and widely adopted server-side scripting language designed for building dynamic and interactive web applications. Many popular websites, including Facebook, WordPress, and Wikipedia, are built using PHP for their functionality and scalability. If you are passionate about web development, mastering PHP is a great step toward a successful programming career.
In today’s digital world, businesses require robust and scalable web applications. PHP allows developers to create feature-rich websites that interact with databases and provide seamless user experiences. Its open-source nature and large developer community make it a preferred choice for beginners and experienced programmers.
For those looking to gain expertise in PHP, Codei5 Academy offers the best PHP course in Coimbatore. The course is designed to provide students with in-depth knowledge of PHP programming, database management, and frameworks like Laravel. With hands-on training, real-world projects, and expert guidance, students can build strong programming skills and become job-ready.
Why Choose PHP for Web Development?
PHP has been a dominant force in web development for years. It is a preferred choice for developers due to its simplicity, versatility, and wide range of applications. Below are some of the major advantages of learning PHP:
Beginner-Friendly Language – PHP has a simple and intuitive syntax, making it easy for beginners to learn and use.
High Demand for PHP Developers – Many companies require skilled PHP developers to build and maintain web applications.
Cross-Platform Compatibility – PHP works on different operating systems like Windows, Linux, and macOS.
Seamless Database Integration – It integrates easily with databases like MySQL, PostgreSQL, and MongoDB.
Scalability – PHP is suitable for building both small websites and large enterprise-level applications.
Vibrant Developer Community – PHP benefits from a large and active community that continuously contributes to updates and enhancements.
What You Will Learn in the Best PHP Course in Coimbatore
At Codei5 Academy, students will receive structured training covering both fundamentals and advanced aspects of PHP development. The curriculum is designed to provide practical exposure, ensuring that learners can apply their knowledge in real-world scenarios.
Core Topics Covered in the PHP Course
PHP Fundamentals
Understanding PHP syntax and structure
Variables, data types, and operators
Loops, arrays, and function
Database Management with MySQL
Introduction to MySQL and database concepts
CRUD (Create, Read, Update, Delete) operations
Writing optimized SQL queries
Object-Oriented Programming (OOP) in PHP
Understanding classes and objects
Inheritance, polymorphism, and encapsulation
Writing reusable and efficient code
Building Dynamic Web Applications
Creating interactive web pages using PHP
Form handling and validation
Working with session management and cookies
PHP Frameworks
Introduction to Laravel and CodeIgniter
MVC architecture and its benefits
Building applications using PHP frameworks
Security Best Practices in PHP
Protecting applications from SQL injection and XSS attacks
Secure user authentication and data encryption
Implementing best practices for coding and security
API Development in PHP
Understanding RESTful APIs
Creating and consuming APIs using PHP
Integrating third-party APIs in applications
Features of the Best PHP Course at Codei5 Academy
Choosing the right training institute is crucial for gaining practical knowledge and hands-on experience. Codei5 Academy offers a well structured PHP course designed to prepare students for real-world web development challenges.
Key Features of the PHP Course
Industry-Focused Curriculum – Covers all essential topics required in the job market.
Hands-On Training – Real-time projects to enhance coding and problem-solving skills.
Expert-Led Classes – Learn from experienced PHP developers with practical knowledge.
Convenient Learning Modes – Offers both classroom and online training to suit different learning preferences.
Placement Assistance – Career guidance and job placement support for students.
Practical Project Implementation – Gain experience by working on real-world projects, helping you build a strong portfolio.
Why Codei5 Academy is the Best Choice for PHP Training?
When choosing a PHP training institute, it is essential to consider factors like curriculum, teaching methodology, and placement support. Codei5 Academy stands out as the best PHP course in Coimbatore due to its commitment to quality education and practical learning.
Reasons to Choose Codei5 Academy for PHP Training
Recognized as the best PHP course in Coimbatore for hands-on learning.
Industry-relevant training with real-world projects to improve coding skills.
100% job assistance and career guidance for students.
Access to the latest PHP tools and technologies for modern web development.
Expert mentors with years of experience in PHP development.
A supportive learning environment that encourages problem-solving and creativity.
Conclusion
PHP remains one of the most valuable skills for aspiring web developers. Learning PHP opens up numerous career opportunities, allowing developers to build powerful, secure, and scalable applications.
By enrolling in the best PHP course in Coimbatore at Codei5 Academy, students gain in-depth knowledge, hands-on experience, and job-ready skills. Whether you are a beginner or an experienced programmer, this course provides the right foundation to advance your career in web development.
Take the next step in your programming journey and build a successful career in PHP development today!
#course#training#internship#education#php course#php development#php programming#php#php development services#developers
0 notes
Text
10 Best PHP Frameworks for Web Development in 2025
PHP has long been one of the most popular languages for web development, powering millions of websites across the globe. With constant improvements and an ever-growing developer community, PHP frameworks have become essential tools for creating robust, scalable, and secure web applications. As we move into 2025, several PHP frameworks continue to dominate the web development space. In this article, we will explore the top 10 PHP frameworks that are expected to lead the charge in web development this year.
1. Laravel
Laravel remains the most popular PHP framework in 2025, and for good reason. Known for its elegant syntax and developer-friendly tools, Laravel simplifies common web development tasks such as routing, authentication, sessions, and caching. It’s perfect for building everything from simple websites to complex web applications. For businesses looking to develop a responsive website or a complex e-commerce website, Laravel services from India can help deliver affordable and high-quality solutions tailored to your needs.
2. Symfony
For those seeking flexibility and long-term support, Symfony is an excellent option. Symfony is known for its stability and can be used to create both large-scale applications and smaller projects. Its reusable components and tools allow for easy integration with other technologies, including WordPress development. If you're aiming to create enterprise-grade applications, Symfony development services from India offer scalable solutions that can meet the growing demands of your business.
3. CodeIgniter
CodeIgniter is a lightweight PHP framework known for its simplicity and speed. With minimal configuration required, it’s great for developers who want to quickly build a static website or a dynamic website. The ease of use and scalability make it an ideal option for small businesses. If you're looking for rapid deployment, you can opt for CodeIgniter development services from India, where experienced professionals will ensure a swift, cost-effective project launch.
4. Zend Framework
The Zend Framework is another powerful tool for web developers, particularly those focusing on building secure, enterprise-grade applications. It’s highly customizable and follows the principles of object-oriented programming (OOP). Whether you want to build a high-performance website, large-scale Zend Framework services, or e-commerce platform services, India provides expert developers at competitive prices to help you scale and optimize your web application.
5. CakePHP
CakePHP is known for its rapid development capabilities and ease of use. It offers a solid set of tools that make it easier to build applications quickly while maintaining flexibility and scalability. For businesses focusing on PHP development, CakePHP services from India can help you build a dynamic website or a responsive website that is both user-friendly and performance-oriented, ensuring a smooth user experience.
6. Phalcon
Phalcon is a high-performance PHP framework built as a C-extension, which results in faster performance compared to most other PHP frameworks. If you’re working on a website where performance is crucial, consider Phalcon development services from India, where skilled developers can optimize your site for speed, scalability, and security, ensuring top-tier performance across all devices.
7. Yii
For those who want to build robust, fast, and secure web applications, Yii (Yes, It’s Easy) is a framework that stands out in 2025. Its rich set of features includes caching support, database access, and security components. Whether you're building a dynamic website or an e-commerce website, Yii development services from India will help you achieve high performance and scalability without compromising quality.
8. FuelPHP
FuelPHP is a flexible and scalable framework that supports both static websites and dynamic websites. It’s a full-stack framework that allows developers to use multiple design patterns and includes features like an ORM (Object-Relational Mapping) and support for WordPress theme setup. When you take FuelPHP development services from India, you're ensuring a tailored solution that meets both your immediate needs and future scalability.
9. Slim
Slim is a micro-framework that focuses on simplicity and performance. It’s perfect for developers building RESTful APIs or small-scale applications. Due to its minimalistic nature, Slim allows for quicker development times. If you're looking for a dynamic website that integrates seamlessly with external services, consider Slim development services from India, where expert developers can quickly deliver high-quality solutions for your project. Additionally, when transitioning from WordPress or integrating it into your project, it’s essential to avoid common WordPress mistakes, which can negatively affect site performance and security. By working with skilled developers from India, you can ensure a smooth integration while maintaining optimal functionality and enhancing user experience.
10. Lumen
Lumen, a micro-framework developed by the creators of Laravel, is optimized for building lightweight applications and APIs. It’s perfect for businesses that require high-performance web applications but don’t need the full power of Laravel. For e-commerce websites or WordPress websites requiring a fast and secure backend, Lumen development services from India provide cost-effective, reliable solutions to help you scale your project efficiently.
Conclusion
Choosing the right PHP framework is crucial for the success of your web development projects. Whether you’re building a WordPress website, a dynamic website, or a complex e-commerce website, there is a PHP framework that will suit your needs. In 2025, these frameworks—Laravel, Symfony, CodeIgniter, Zend, CakePHP, Phalcon, Yii, FuelPHP, Slim, and Lumen—offer the flexibility, scalability, and performance that modern web developers require.
For your next project, consider taking PHP development services from India to leverage the expertise and cost-effective solutions provided by skilled developers.
For the latest trends in web development and technology, keep an eye on social media platforms for valuable insights and updates from industry experts.
1 note
·
View note
Text
Local Expertise Meets Innovation: Top-Rated Website Development Companies in Udaipur.
Choosing a website development company in Udaipur isn't just about finding someone to build a website; it’s about partnering with local experts who understand the business environment and know how to create a strong online presence. This perfect blend of local expertise and cutting-edge innovation is what sets Udaipur-based web development firms apart.
From aesthetic design to seamless functionality, these companies are helping Udaipur businesses make their mark online — not just locally, but globally.
Why Choose a Local Web Development Company in Udaipur?
Working with a web development company in Udaipur comes with several benefits:
Better Communication: Being in the same city makes meetings, updates, and feedback sessions easy and efficient.
Understanding Local Market Needs: Udaipur web development experts are familiar with the local economy, culture, and customer behavior, allowing them to craft customized solutions.
Trust and Relationship Building: A local IT company in Udaipur can offer a personal touch that’s often missing in remote collaborations.
This local connection ensures a smoother development process and better alignment with your business goals.
What to Look for in a Top Web Development Company
When searching for the best web development company in Udaipur, keep these points in mind:
Strong Portfolio: Look at the company's past work. A diverse portfolio shows versatility and experience.
Skilled Team: A team of professional web developers in Udaipur with expertise in various technologies like HTML5, CSS3, React, WordPress, and more.
Client Testimonials: Honest feedback from previous clients reflects credibility.
Custom Development Solutions: Whether you need a static or dynamic website, eCommerce store, or custom CMS, the company should offer tailored solutions.
Transparent Pricing and Support: Look for clarity in pricing and ongoing support post-deployment.
Top-Rated Website Development Companies in Udaipur (2025 List)
Here are some of the leading top web development companies in Udaipur known for their local expertise and quality services:
WebSenor Technologies
Services: Website design, web development, app development, digital marketing
Highlights: 10+ years of experience, international client base, WordPress and custom development
Website: https://websenor.com
Advaiya Solutions
Services: Enterprise web apps, cloud solutions, business intelligence
Highlights: Strong focus on data-driven design, known for corporate projects
Obbserv
Services: Digital marketing, web design, UI/UX, eCommerce
Highlights: Specializes in SEO-friendly website development in Udaipur
W3villa Technologies
Services: Web development, blockchain, software consulting
Highlights: Innovation-focused, especially in fintech and SaaS platforms
Techtic Solutions
Services: Mobile and web apps, eCommerce development, React/Node-based projects
Highlights: Modern tech stack and excellent client communication
Services Offered by These Companies
Most website designing companies in Udaipur offer a comprehensive set of services, including:
Website Design & UI/UX: Visually appealing, user-friendly designs
Custom CMS Development: WordPress, Joomla, Shopify, or custom-built systems
eCommerce Website Development Udaipur: Solutions for both B2B and B2C platforms
SEO-Friendly & Responsive Websites: Optimized for all devices and search engines
Website Maintenance & Security: Ongoing support, updates, and backups
These companies ensure a mix of aesthetics, performance, and functionality to help businesses grow online.
Local Innovation Driving Global Results
Udaipur's web development firms aren’t just keeping up with the times; they’re driving digital change. Here’s how:
Latest Web Technologies: Use of frameworks like Angular, React, Vue.js, and Laravel
AI & Automation: Chatbots, recommendation engines, and automation tools
SEO Integration: From the ground up, sites are built to perform well on search engines
Mobile Optimization: Websites that load fast and work great on all devices
This innovation, combined with custom website development, allows local businesses to compete on a global scale.
Client Success Stories
1. Local Boutique Boosts Online Sales by 3X
A Udaipur-based boutique partnered with a local web design company in Udaipur to launch a responsive eCommerce site. Within six months, they saw a 3X increase in online orders.
2. Hotel Website Drives Direct Bookings
An established hotel brand in Udaipur revamped their website with SEO-friendly content and a custom booking engine. The result? 40% more direct bookings and reduced reliance on travel portals.
3. Education Platform Scales with WordPress
A coaching institute used a WordPress website development Udaipur service to launch their e-learning portal. Traffic doubled in 4 months thanks to mobile optimization and smart UX design.
Conclusion
Choosing the right website development company in Udaipur can make a big difference for your business. From understanding your local audience to building modern, user-focused websites, these companies bring together experience and innovation.
Whether you're a startup or an established business, working with a Udaipur-based web design firm means you’ll get personalized service, expert solutions, and trustworthy support.
Ready to take your online presence to the next level? Partner with a top-rated Udaipur web development company today!
0 notes
Text
Best Laravel Full Stack Online Course | Learn from Top Teachers at Gritty Tech
Introduction to Gritty Tech’s Mission in Education and Tutoring
Gritty Tech is dedicated to providing high-quality education to students worldwide. Our Laravel Full Stack Online Course is designed to help learners master the essential skills required to build dynamic and scalable web applications. With expert instructors, a well-structured curriculum, and hands-on experience, Gritty Tech ensures that students gain a competitive edge in the web development industry For More...
About Gritty Tech
Gritty Tech is an industry-leading edtech platform that provides high-quality educational resources. Our Laravel Full Stack Online Course is crafted to meet the needs of students and professionals who aspire to become proficient full-stack developers. With a focus on practical learning and real-world applications, we aim to bridge the gap between theoretical knowledge and industry requirements.
Our Mission, Vision, and Goals
At Gritty Tech, our mission is to empower learners by providing them with the best possible educational experience. Our Laravel Full Stack Online Course is structured to cater to both beginners and advanced learners. Our vision is to create an accessible and flexible learning environment that enables students to master full-stack development at their own pace. Our goal is to produce industry-ready professionals who can excel in Laravel development.
Professional Teachers in All Languages
Our team of professional educators at Gritty Tech is composed of industry experts with years of experience in Laravel and full-stack development. Our Laravel Full Stack Online Course is designed and taught by professionals who bring real-world experience into the classroom. The course is available in multiple languages, ensuring that students from different backgrounds can grasp the concepts effectively.
Unique Teaching Approach
Gritty Tech follows a unique teaching approach that combines theoretical lessons with practical applications. Our Laravel Full Stack Online Course includes interactive sessions, live coding exercises, and project-based learning to ensure that students gain hands-on experience. The course content is regularly updated to align with the latest industry trends and technologies.
Unique Course Content
Our Laravel Full Stack Online Course covers:
Introduction to Laravel and Full Stack Development
Setting up the development environment
Working with Laravel MVC architecture
Database management and migrations
Frontend and backend integration
API development with Laravel
Authentication and security measures
Deployment strategies
Real-world project implementation
With structured modules, students can easily navigate through the course and gain expertise in Laravel full-stack development.
Flexible Learning Options for Students Worldwide
At Gritty Tech, we understand that every student has different learning preferences. Our Laravel Full Stack Online Course is available in multiple formats, including live sessions, recorded lectures, and self-paced learning. With flexible scheduling, students can learn at their convenience without compromising their daily commitments.
Encouragement for Parents and Students to Book a Session Today
If you or your child is interested in mastering full-stack development, Gritty Tech offers the perfect solution. Our Laravel Full Stack Online Course provides structured learning, industry-relevant projects, and expert guidance. Don’t miss out on the opportunity to build a successful career in web development. Enroll today and take the first step towards mastering Laravel!
Easy Ways to Get in Touch and Book Your First Lesson
Enrolling in our Laravel Full Stack Online Course is simple. You can:
Visit our website and sign up for a free trial session.
Contact our support team via email or phone for enrollment assistance.
Join our live demo session to explore the course structure and teaching methodology.
Follow us on social media for updates and special offers.
Frequently Asked Questions (FAQs)
1. What is the Laravel Full Stack Online Course at Gritty Tech?
The Laravel Full Stack Online Course at Gritty Tech is a comprehensive training program designed to teach students full-stack web development using Laravel.
2. Who can enroll in the Laravel Full Stack Online Course?
Anyone interested in web development, from beginners to experienced developers, can enroll in our Laravel Full Stack Online Course.
3. What topics are covered in the Laravel Full Stack Online Course?
The Laravel Full Stack Online Course covers frontend and backend development, database management, API development, authentication, and more.
4. Is the Laravel Full Stack Online Course available in different languages?
Yes, our Laravel Full Stack Online Course is available in multiple languages to cater to students from diverse backgrounds.
5. How is the Laravel Full Stack Online Course structured?
The Laravel Full Stack Online Course is structured into modules with video lectures, hands-on projects, and interactive exercises.
6. What are the career opportunities after completing the Laravel Full Stack Online Course?
After completing the Laravel Full Stack Online Course, students can work as full-stack developers, backend developers, or Laravel specialists.
7. How long does it take to complete the Laravel Full Stack Online Course?
The duration of the Laravel Full Stack Online Course depends on the learning pace of the student. Self-paced and live batch options are available.
8. Can I get a certification after completing the Laravel Full Stack Online Course?
Yes, students receive a certification upon successfully completing the Laravel Full Stack Online Course, which enhances their career prospects.
9. What makes the Laravel Full Stack Online Course at Gritty Tech unique?
Our Laravel Full Stack Online Course stands out due to expert-led training, real-world projects, and flexible learning options.
10. How can I enroll in the Laravel Full Stack Online Course?
Students can enroll in the Laravel Full Stack Online Course by visiting our website, contacting our support team, or joining a demo session.
Conclusion
Gritty Tech’s Laravel Full Stack Online Course is the ultimate learning experience for aspiring developers. With expert instructors, structured content, and real-world applications, our course provides students with the skills needed to excel in Laravel full-stack development. Enroll today and take the first step towards a successful career in web development!
0 notes
Text
PHP Training in Indore – Master Web Development with Infograins TCS
In the dynamic world of web development, PHP remains a powerful, flexible, and widely-used server-side scripting language. Whether you're just starting or looking to enhance your backend development skills, enrolling in a professional PHP Training in Indore can be a game-changer. At Infograins TCS, we offer specialized courses tailored for aspiring developers, students, and working professionals who want to build strong foundations in PHP.

Overview
PHP is the backbone of many well-known websites and platforms, from Facebook to WordPress. It’s an open-source, easy-to-learn scripting language that integrates well with databases like MySQL. Our training program covers everything from PHP fundamentals to advanced programming, providing in-depth knowledge and hands-on experience. As a trusted PHP training in Indore, Infograins TCS ensures a balanced approach between theory and practical implementation.
Pros of Learning PHP
Wide Industry Demand: PHP developers are highly sought after in startups and established companies alike.
Ease of Learning: Its straightforward syntax makes it an excellent choice for beginners.
Extensive Community Support: PHP has a massive global developer community for guidance and support.
Framework Integration: Learn to work with frameworks like Laravel and CodeIgniter to enhance productivity.
Open Source Advantage: No licensing fees mean low development costs and great flexibility.
Why Choose Us
Infograins TCS stands out as a leading PHP institute in Indore because of its experienced trainers, structured curriculum, and real-world training approach. We focus on delivering industry-aligned knowledge through live projects, mock interviews, and resume-building sessions. Our mentors are industry professionals with years of development and training experience, ensuring every student gains practical, applicable knowledge.
Our More Services
Alongside PHP, we offer training in a wide range of IT domains including Java, Python, AngularJS, Node.js, React, UI/UX design, and more. Whether you're interested in front-end development, backend technologies, or full-stack development, Infograins TCS provides versatile training options tailored to current market needs. From coding bootcamps to internship opportunities, we’re your one-stop solution for IT skill development.
Why We as a Helping Partner
At Infograins TCS, we don’t just teach — we mentor, guide, and support every learner’s journey toward success. We believe in building careers, not just providing certifications. As your learning partner, we help you unlock real opportunities in the IT industry. Through our strong industry connections, personalized attention, and commitment to excellence, we have become a preferred destination for those seeking a quality PHP Course in Indore and beyond.
Looking to build a strong foundation in web development? Infograins TCS is your trusted place for professional PHP Training Institute in Indore. Get trained by industry experts, work on real-time projects, and kickstart a rewarding IT career with us!
In the dynamic world of web development, PHP remains a powerful, flexible, and widely-used server-side scripting language. Whether you're just starting or looking to enhance your backend development skills, enrolling in a professional PHP Training in Indore can be a game-changer. At Infograins TCS, we offer specialized courses tailored for aspiring developers, students, and working professionals who want to build strong foundations in PHP.
0 notes