#pentesting
Explore tagged Tumblr posts
Text
Symfony Clickjacking Prevention Guide
Clickjacking is a deceptive technique where attackers trick users into clicking on hidden elements, potentially leading to unauthorized actions. As a Symfony developer, it's crucial to implement measures to prevent such vulnerabilities.

🔍 Understanding Clickjacking
Clickjacking involves embedding a transparent iframe over a legitimate webpage, deceiving users into interacting with hidden content. This can lead to unauthorized actions, such as changing account settings or initiating transactions.
🛠️ Implementing X-Frame-Options in Symfony
The X-Frame-Options HTTP header is a primary defense against clickjacking. It controls whether a browser should be allowed to render a page in a <frame>, <iframe>, <embed>, or <object> tag.
Method 1: Using an Event Subscriber
Create an event subscriber to add the X-Frame-Options header to all responses:
// src/EventSubscriber/ClickjackingProtectionSubscriber.php namespace App\EventSubscriber; use Symfony\Component\EventDispatcher\EventSubscriberInterface; use Symfony\Component\HttpKernel\Event\ResponseEvent; use Symfony\Component\HttpKernel\KernelEvents; class ClickjackingProtectionSubscriber implements EventSubscriberInterface { public static function getSubscribedEvents() { return [ KernelEvents::RESPONSE => 'onKernelResponse', ]; } public function onKernelResponse(ResponseEvent $event) { $response = $event->getResponse(); $response->headers->set('X-Frame-Options', 'DENY'); } }
This approach ensures that all responses include the X-Frame-Options header, preventing the page from being embedded in frames or iframes.
Method 2: Using NelmioSecurityBundle
The NelmioSecurityBundle provides additional security features for Symfony applications, including clickjacking protection.
Install the bundle:
composer require nelmio/security-bundle
Configure the bundle in config/packages/nelmio_security.yaml:
nelmio_security: clickjacking: paths: '^/.*': DENY
This configuration adds the X-Frame-Options: DENY header to all responses, preventing the site from being embedded in frames or iframes.
🧪 Testing Your Application
To ensure your application is protected against clickjacking, use our Website Vulnerability Scanner. This tool scans your website for common vulnerabilities, including missing or misconfigured X-Frame-Options headers.

Screenshot of the free tools webpage where you can access security assessment tools.
After scanning for a Website Security check, you'll receive a detailed report highlighting any security issues:

An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities.
🔒 Enhancing Security with Content Security Policy (CSP)
While X-Frame-Options is effective, modern browsers support the more flexible Content-Security-Policy (CSP) header, which provides granular control over framing.
Add the following header to your responses:
$response->headers->set('Content-Security-Policy', "frame-ancestors 'none';");
This directive prevents any domain from embedding your content, offering robust protection against clickjacking.
🧰 Additional Security Measures
CSRF Protection: Ensure that all forms include CSRF tokens to prevent cross-site request forgery attacks.
Regular Updates: Keep Symfony and all dependencies up to date to patch known vulnerabilities.
Security Audits: Conduct regular security audits to identify and address potential vulnerabilities.
📢 Explore More on Our Blog
For more insights into securing your Symfony applications, visit our Pentest Testing Blog. We cover a range of topics, including:
Preventing clickjacking in Laravel
Securing API endpoints
Mitigating SQL injection attacks
🛡️ Our Web Application Penetration Testing Services
Looking for a comprehensive security assessment? Our Web Application Penetration Testing Services offer:
Manual Testing: In-depth analysis by security experts.
Affordable Pricing: Services starting at $25/hr.
Detailed Reports: Actionable insights with remediation steps.
Contact us today for a free consultation and enhance your application's security posture.
3 notes
·
View notes
Text

Zoey. One cat three trials.
#artists on tumblr#lineart#stippling#eyes#linedrawing#pet portrait#cat#drawing#pen and ink#pentesting
4 notes
·
View notes
Text
Building Your Own Cyberdeck:
What do you do when you have extra time between a job and your next? How about building your own Cyberdeck? Check this article out for tips on building your own!
The Ultimate Hacker Project For aspiring cybersecurity professionals, cyberpunk enthusiasts, hardware hackers, and circuit benders, one of the best hands-on projects you can take on is building your own cyberdeck. Despite overwhelming schedules full of training programs, full time work weeks, sometimes limited funds, and the endless possibilities of hardware combinations, many fans of the…
View On WordPress
#Cyber#Cyber Security#cyberdeck#cyberpunk#Cybersecurity Specialist#Ethical Hacking#hack#hacker#infosec#IT#IT professional#mobile#mobile computer#Pentesting#programming#project
30 notes
·
View notes
Text
Greetings fellow freaks and geeks!
Allow me to introduce myself:
My name is Haru
Im (at the time of posting this) 18!
Im a Therian and a furry :3
Im pansexual and genderfluid (so if possible please ask for my pronouns)
I enjoy Cybersecurity, general technological devices, music (punk, rock, indie and a whole lot more)
Im diagnosed autistic, ADHD, dislexic, disbraxic, disgraphic and more ^^;
Im currently self teaching for red team cybersecurity, art and guitar as well as fursuit making and other crafty things
Currently this is my main blog where ill post just whatever and i plan on making 2 other blogs but ill announce when that is ;3
For now enjoy the insane ramblings about anything and everything all of the time.
#intro post#introduction#blog intro#main blog#furry#therian#punk rock#dedsec#hack the planet#cybersecurity#red team#pentesting
2 notes
·
View notes
Text
youtube
3 notes
·
View notes
Photo
Hello guys! How are you doing? I am trying to keep up with Angular studies, plus I am doing a short Pentest classes in order to understand it a little bit more, as I requested some Pentests at work for a special team inside the company. It is very interesting, to understand the vulnerabilities that can occurs, and how to think about them while we are creating the architecture of applications, databases, etc. I will start new Spring boot courses as soon as possible too. Yesterday I started for real in the architecture team at work, and I am terrified as I already have some deadlines I must look forward to things I am not an expert yet (✖﹏✖) Well, I will do my best, and ask for help whenever I need (≖͞_≖̥) Keep studying, keep pursuing your dreams!
#study#studyblr#studyblr community#programming#programming community#programming struggles#coding#coding community#angular#springboot#must learn java#java#pentest#pentesting#software development#study daily#javascript#keep dreaming#keep fighting#keep studying#somebody help#study struggles
11 notes
·
View notes
Text

🌐📚 Elevate your cloud skills prowess with our training courses! 🎓💻
💡 Gear up for success with our in-depth training designed to help you nail the Cloud certification exam. 💯🥇
📅 Enroll today and take the first step towards unlocking endless opportunities! Don't miss out on this incredible offer. ⏳🔓
For Additional Info🔔 🟢Whatsapp: https://wa.me/9677781155 , https://wa.me/7558184348 , https://wa.me/9677724437 📨Drop: https://m.me/elysiumacademy.org 🌐Our website: https://elysiumacademy.org/networking-course-certification/ 📌Live Visit: https://maps.app.goo.gl/YegrK4aKEWbEY2nc8 🔖Appointment: https://elysiumacademy.org/appointment-booking/
#elysiumacademy#no1academy#jobassurance#tesbo#no1trainingacademy#elysiumacademy_madurai#cybersecurity#ethicalhacking#hackers#programming#hack#technology#security#coding#tech#ethicalhacker#pentesting#cyber#malware#programmer#informationsecurity#AWS#cloudskillawareness#coder
2 notes
·
View notes
Text
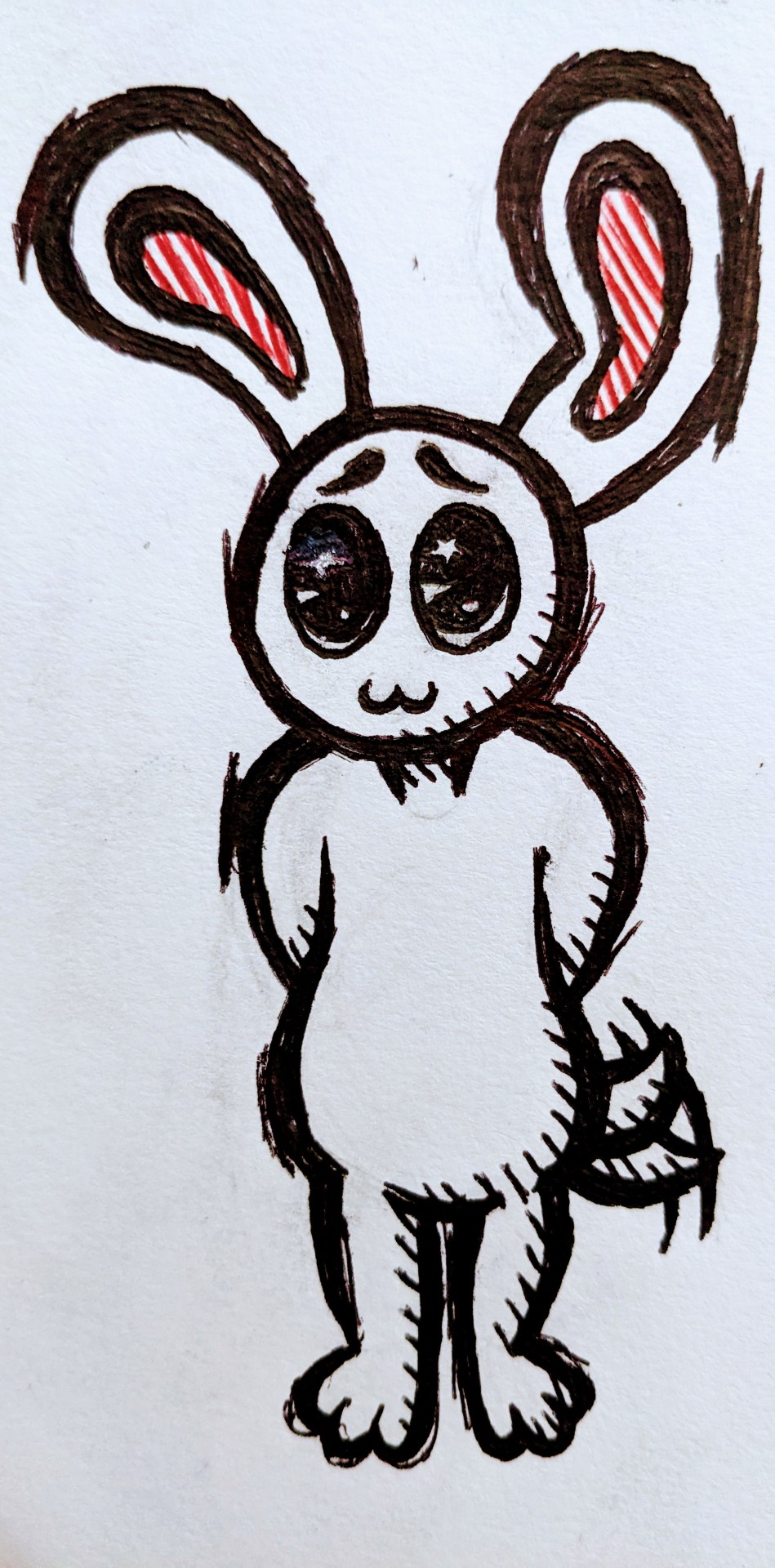
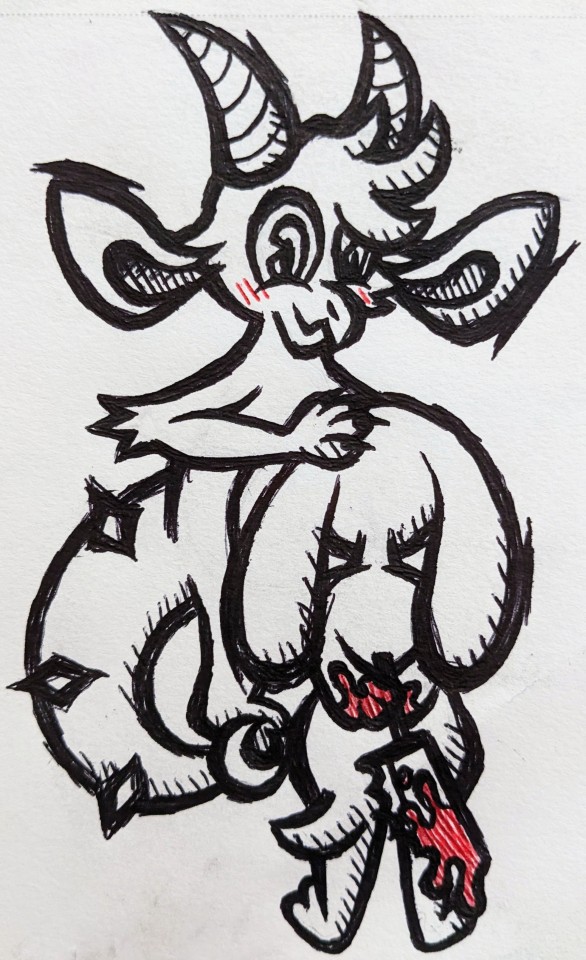
Guess what I'm now obsessed with :)
#chikin nuggit#fwench fwy#iscream#I don't know what their ship name is#traditional art#traditional doodle#doodle#sketch#sketchbook#pen and paper#pentesting#pen and ink
9 notes
·
View notes
Text
Signal boosting
Thank you
#progblr#lainposting#196#free wifi#public service announcement#psa#network hacking#hacking#wifi#wifi hacking#ip address#pentesting
135K notes
·
View notes
Text
Are You Prepared for 2025's Top Cyber Threats?
Cybercriminals are getting smarter—and no business is too small to be targeted. From phishing emails and ransomware attacks to insider threats and credential stuffing, understanding today’s most dangerous cyber risks is the first step to securing your systems and data.
0 notes
Text
Prevent HTTP Parameter Pollution in Laravel with Secure Coding
Understanding HTTP Parameter Pollution in Laravel
HTTP Parameter Pollution (HPP) is a web security vulnerability that occurs when an attacker manipulates multiple HTTP parameters with the same name to bypass security controls, exploit application logic, or perform malicious actions. Laravel, like many PHP frameworks, processes input parameters in a way that can be exploited if not handled correctly.

In this blog, we’ll explore how HPP works, how it affects Laravel applications, and how to secure your web application with practical examples.
How HTTP Parameter Pollution Works
HPP occurs when an application receives multiple parameters with the same name in an HTTP request. Depending on how the backend processes them, unexpected behavior can occur.
Example of HTTP Request with HPP:
GET /search?category=electronics&category=books HTTP/1.1 Host: example.com
Different frameworks handle duplicate parameters differently:
PHP (Laravel): Takes the last occurrence (category=books) unless explicitly handled as an array.
Express.js (Node.js): Stores multiple values as an array.
ASP.NET: Might take the first occurrence (category=electronics).
If the application isn’t designed to handle duplicate parameters, attackers can manipulate input data, bypass security checks, or exploit business logic flaws.
Impact of HTTP Parameter Pollution on Laravel Apps
HPP vulnerabilities can lead to:
✅ Security Bypasses: Attackers can override security parameters, such as authentication tokens or access controls. ✅ Business Logic Manipulation: Altering shopping cart data, search filters, or API inputs. ✅ WAF Evasion: Some Web Application Firewalls (WAFs) may fail to detect malicious input when parameters are duplicated.
How Laravel Handles HTTP Parameters
Laravel processes query string parameters using the request() helper or Input facade. Consider this example:
use Illuminate\Http\Request; Route::get('/search', function (Request $request) { return $request->input('category'); });
If accessed via:
GET /search?category=electronics&category=books
Laravel would return only the last parameter, category=books, unless explicitly handled as an array.
Exploiting HPP in Laravel (Vulnerable Example)
Imagine a Laravel-based authentication system that verifies user roles via query parameters:
Route::get('/dashboard', function (Request $request) { if ($request->input('role') === 'admin') { return "Welcome, Admin!"; } else { return "Access Denied!"; } });
An attacker could manipulate the request like this:
GET /dashboard?role=user&role=admin
If Laravel processes only the last parameter, the attacker gains admin access.
Mitigating HTTP Parameter Pollution in Laravel
1. Validate Incoming Requests Properly
Laravel provides request validation that can enforce strict input handling:
use Illuminate\Http\Request; use Illuminate\Support\Facades\Validator; Route::get('/dashboard', function (Request $request) { $validator = Validator::make($request->all(), [ 'role' => 'required|string|in:user,admin' ]); if ($validator->fails()) { return "Invalid Role!"; } return $request->input('role') === 'admin' ? "Welcome, Admin!" : "Access Denied!"; });
2. Use Laravel’s Input Array Handling
Explicitly retrieve parameters as an array using:
$categories = request()->input('category', []);
Then process them safely:
Route::get('/search', function (Request $request) { $categories = $request->input('category', []); if (is_array($categories)) { return "Selected categories: " . implode(', ', $categories); } return "Invalid input!"; });
3. Encode Query Parameters Properly
Use Laravel’s built-in security functions such as:
e($request->input('category'));
or
htmlspecialchars($request->input('category'), ENT_QUOTES, 'UTF-8');
4. Use Middleware to Filter Requests
Create middleware to sanitize HTTP parameters:
namespace App\Http\Middleware; use Closure; use Illuminate\Http\Request; class SanitizeInputMiddleware { public function handle(Request $request, Closure $next) { $input = $request->all(); foreach ($input as $key => $value) { if (is_array($value)) { $input[$key] = array_unique($value); } } $request->replace($input); return $next($request); } }
Then, register it in Kernel.php:
protected $middleware = [ \App\Http\Middleware\SanitizeInputMiddleware::class, ];
Testing Your Laravel Application for HPP Vulnerabilities
To ensure your Laravel app is protected, scan your website using our free Website Security Scanner.

Screenshot of the free tools webpage where you can access security assessment tools.
You can also check the website vulnerability assessment report generated by our tool to check Website Vulnerability:

An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities.
Conclusion
HTTP Parameter Pollution can be a critical vulnerability if left unchecked in Laravel applications. By implementing proper validation, input handling, middleware sanitation, and secure encoding, you can safeguard your web applications from potential exploits.
🔍 Protect your website now! Use our free tool for a quick website security test and ensure your site is safe from security threats.
For more cybersecurity updates, stay tuned to Pentest Testing Corp. Blog! 🚀
3 notes
·
View notes
Text
Coding and Scripting for Beginner Hackers
Learning to code and write scripts is a crucial skill for getting into ethical hacking and cybersecurity. Scripting allows you to automate repetitive tasks, develop your own custom tools, analyze data, and program everything from small hacking tools to machine learning models. Understanding and knowing how to code in different languages can be extremely useful when doing deep dives into malware…
View On WordPress
#Cyber Security#cybersecurity careers#Ethical Hacking#hacer certifications#hacking for beginners#hacking guide#hacking homelab#infosec#learn to code#learn to hack#Penetration Testing#Pentesting
4 notes
·
View notes
Text
Stop Guessing, Start Testing
Traditional pen tests give you a report. Siemba’s PTaaS gives you real-time insights, prioritized by risk.
✔️ Test continuously—not once a year ✔️ Get business-context aware results ✔️ Collaborate easily with security + dev teams
Fix what matters faster.
0 notes
Text
An Overview of Burp Suite: Acquisition, Features, Utilisation, Community Engagement, and Alternatives.
Introduction:
Burp Suite is one of the strongest web application security testing software tools used by cybersecurity experts, as well as ethical hackers. PortSwigger created Burp Suite, which provides potent scanning, crawling, and exploiting tools for web application vulnerabilities.
What is Burp Suite?
Burp Suite is one of the tools to conduct security testing of web applications. It assists security testers in detecting vulnerabilities and weaknesses like SQL injections, XSS, CSRF, etc.
Steps in Obtaining Burp Suite
Burp Suite is available for download on the PortSwigger official website. It is available in three versions:
Community Edition (Free)
Professional Edition (Subscription-Based)
Enterprise Edition (For Organisations)
Important Tools in Burp Suite
Proxy – Captures browser traffic
Spider – Crawls web application content
Scanner – Scans automatically for vulnerabilities (Pro only)
Intruder – Performs automated attack activities.
Repeater – Manually send requests.
Decoder – Translates encoded data.
Comparer – Compares HTTP requests/responses
Extender – Allows extensions through the BApp Store
How to Use Burp Suite
Set your browser to use Burp Proxy.
Capture and manipulate HTTP/S requests.
Utilise tools such as Repeater and Intruder for testing.
Scan server responses for risks.
Export reports for audit purposes.
Burp Suite Community
Burp Suite has a highly engaged worldwide user base of security experts. PortSwigger Forum and GitHub repositories have discussions, plugins, and tutorials. Many experts are contributing through YouTube, blogs, and courses.
Alternatives to Burp Suite
If you're searching for alternatives, then look at:
OWASP ZAP (Open Source)
Acunetix
Netsparker
Nikto
Wfuzz
Conclusion:
Burp Suite is widely used for web application security testing. Mastery of Burp Suite is one step towards web application security for both novice and professional ethical hackers.

#BurpSuite#CyberSecurity#EthicalHacking#PenTesting#BugBounty#InfoSec#WebSecurity#SecurityTools#AppSec#OWASP#HackingTools#TechTools#WhiteHatHacker#CyberTools#BurpSuiteCommunity#NetworkSecurity#PortSwigger#WebAppTesting#SecurityScanner#CyberAwareness
0 notes
Text

0 notes