Text
CodeBattle: The Ultimate 1v1 Real-Time Coding Battle Platform
Introduction
Hello coder! How are you , In the world of competitive programming and coding challenges, real-time battles are becoming increasingly popular. CodeBattle is a cutting-edge 1v1 real-time coding battle platform designed to test programmersâ skills in a fast-paced MCQ-based format. Whether youâre a beginner or an experienced coder, CodeBattle offers an exciting and challenging way to improve your coding knowledge.
In this article, we will dive deep into the development of CodeBattle, covering project structure, technology stack, real-time matchmaking, styling tips, and live demo setup. Additionally, we will provide code snippets to help you understand the implementation better.
Features of CodeBattle
Real-time 1v1 Coding Battles
MCQ-based Questions with a 20-second timer
Live Scoreboard
Leaderboard System (Daily, Weekly, and All-time Rankings)
Secure Authentication (Google/Firebase Login)
Admin Panel to manage questions & users
Fully Responsive UI
Tech Stack: React (Next.js), Node.js, Express.js, MongoDB, and Socket.io
Project Structure
CodeBattel/ âââ frontend/ # React (Next.js) UI â âââ components/ # Reusable Components â âââ pages/ # Next.js Pages (Home, Play, Leaderboard, etc.) â âââ styles/ # CSS Modules / Tailwind CSS â âââ utils/ # Helper Functions â âââ backend/ # Node.js Backend â âââ models/ # MongoDB Models â âââ routes/ # Express Routes (API Endpoints) â âââ controllers/ # Business Logic â âââ config/ # Configuration Files â âââ socket/ # Real-time Matchmaking with Socket.io â âââ index.js # Main Server Entry Point â âââ README.md # Project Documentation
Building the Frontend with React (Next.js)
1. Installing Dependencies
npx create-next-app@latest codebattel cd codebattel npm install socket.io-client axios tailwindcss npm install --save firebase
2. Setting up Tailwind CSS
npx tailwindcss init -p
Edit tailwind.config.js:module.exports = { content: ["./pages/**/*.{js,ts,jsx,tsx}", "./components/**/*.{js,ts,jsx,tsx}"], theme: { extend: {}, }, plugins: [], };
Developing the 1v1 Battle System
1. Setting Up Real-Time Matchmaking
import { io } from "socket.io-client"; import { useEffect, useState } from "react";const socket = io("http://localhost:5000");export default function BattleRoom() { const [question, setQuestion] = useState(null); const [timer, setTimer] = useState(20); useEffect(() => { socket.emit("joinBattle"); socket.on("newQuestion", (data) => { setQuestion(data); setTimer(20); }); }, []); return ( <div> <h1>CodeBattel</h1> {question && ( <div> <h2>{question.text}</h2> <ul> {question.options.map((opt, index) => ( <li key={index}>{opt}</li> ))} </ul> <p>Time Left: {timer} sec</p> </div> )} </div> ); }
Building the Backend with Node.js & Socket.io
1. Installing Dependencies
npm init -y npm install express socket.io mongoose cors dotenv
2. Creating the Server
const express = require("express"); const http = require("http"); const { Server } = require("socket.io"); const app = express(); const server = http.createServer(app); const io = new Server(server, { cors: { origin: "*" } });let rooms = []; io.on("connection", (socket) => { socket.on("joinBattle", () => { if (rooms.length > 0) { let room = rooms.pop(); socket.join(room); io.to(room).emit("newQuestion", { text: "What is React?", options: ["Library", "Framework", "Language", "None"] }); } else { let newRoom = "room-" + socket.id; rooms.push(newRoom); socket.join(newRoom); } }); });server.listen(5000, () => console.log("Server running on port 5000"));
Live Demo Setup
Clone the repo:
git clone https://github.com/ashutoshmishra52/codebattel.git cd codebattel
Install dependencies:
npm install && cd backend && npm install
Run the project:
npm run dev
Open http://localhost:3000 in your browser.
FAQ
Q1: What is CodeBattle?
CodeBattle is a 1v1 real-time coding battle platform where players answer multiple-choice questions under a 20-second timer.
Q2: How does matchmaking work?
Players are randomly paired in real time using Socket.io.
Q3: Can I add my own questions?
Yes! The Admin Panel allows you to add/edit/delete MCQs.
Q4: How do I contribute?
Check out the GitHub repository and submit a pull request.
About the Author
Ashutosh Mishra is a full-stack developer, AI researcher, and content writer with 4+ years of experience. He is the founder of CodeWithAshutosh, a platform dedicated to teaching web development, AI, and competitive coding.
For more coding tutorials and projects, follow Ashutosh Mishra.
Conclusion
CodeBattle is an innovative way to enhance your coding skills in a competitive environment. With real-time battles, an engaging UI, and a powerful backend, it stands out as a top-tier coding battle platform. Start coding, challenge your friends, and rise up the leaderboard!
0 notes
Text
How To Make Multiple Choice Quiz In Html Code
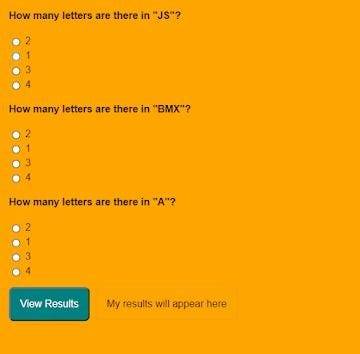
first, you play Multiple Choice Questions (MCQ) Quiz and then click on View results The results page will then be shown. This Multiple Choice Questions (MCQ) Quiz will be finished using JavaScript Code, a powerful language that allows for anything.
100+ JavaScript Projects For Beginners With Source Code
Live Preview Of Multiple Choice Questions Source Code and Preview:-
As you are looking in the project preview how the thing is organized.
Following is the feature of our project:-
We have arranged the Questions and options in the list format using the tag.
Then we set the option using span and defining the radio button and giving the appropriate value.
Multiple Choice Quiz Html Code:-
Now Iâll be telling you to define the structure using HTML. Not from scratch, just the code which is under the body tag.
We have the following part in the HTML section:
Portfolio Website Using HTML CSS And JavaScript ( Source Code)
First, we call the ul class which we have defined the class as a quiz.
Then using the tag we have set our question in the tag.
Then we used a label tag and called the radio button given the value and using span we have given the answer.
Similarly, we have done this for all the options and for all the questions.
Go through the code below and run it in our IDLE before CSS Styling.
<ul class="quiz"> <li> <h4>How many letters are there in "JS"?</h4> <ul class="choices"> <li> <label ><input type="radio" name="question0" value="A" /><span >2</span ></label > </li> <li> <label ><input type="radio" name="question0" value="B" /><span >1</span ></label > </li> <li> <label ><input type="radio" name="question0" value="C" /><span >3</span ></label > </li> <li> <label ><input type="radio" name="question0" value="D" /><span >4</span ></label > </li> </ul> </li> <li> <h4>How many letters are there in "BMX"?</h4> <ul class="choices"> <li> <label ><input type="radio" name="question1" value="A" /><span >2</span ></label > </li> <li> <label ><input type="radio" name="question1" value="B" /><span >1</span ></label > </li> <li> <label ><input type="radio" name="question1" value="C" /><span >3</span ></label > </li> <li> <label ><input type="radio" name="question1" value="D" /><span >4</span ></label > </li> </ul> </li> <li> <h4>How many letters are there in "A"?</h4> <ul class="choices"> <li> <label ><input type="radio" name="question2" value="A" /><span >2</span ></label > </li> <li> <label ><input type="radio" name="question2" value="B" /><span >1</span ></label > </li> <li> <label ><input type="radio" name="question2" value="C" /><span >3</span ></label > </li> <li> <label ><input type="radio" name="question2" value="D" /><span >4</span ></label > </li> </ul> </li> </ul> <button class="view-results" onclick="returnScore()">View Results</button> <span id="myresults" class="my-results">My results will appear here</span>
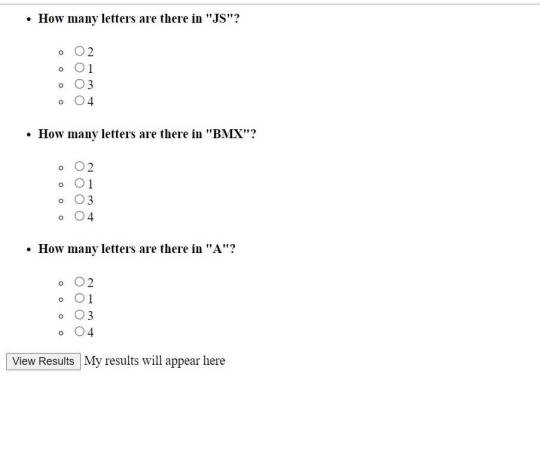
CSS Code For Styling Multiple Choice Quiz:-
By CSS design we will design our whole page here it is just a quiz so weâll just add a background color, color to the button, and font family for the whole body.
And set the padding of the questions and options so that it doesnât get messy and looks in a systematic order.
10+ Javascript Project Ideas For Beginners( Project Source Code)
The analysis will be aided by the CSS code below. After adding this file to your link rel-tag, wait for the results. We will add some of the basic styling to our quiz app using the default selector, and we will add styling to various quiz app elements using the class selector.
this is simple css code. we do not add any heavy css code because our main aim is to create Multiple Choice Questions (MCQ) functionality. if you need more Better Ui you can add more css code in this css section.
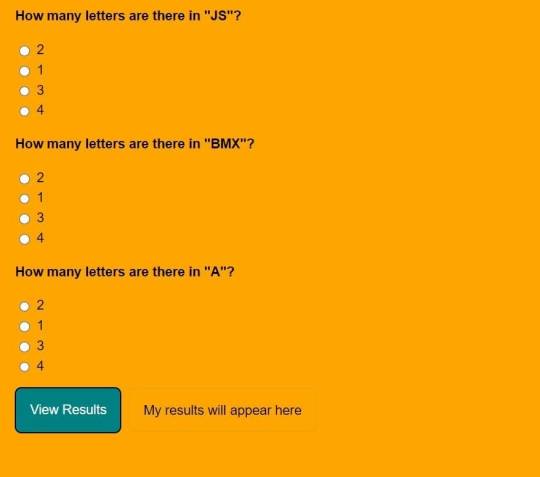
JavaScript Multiple Choice Quiz Code:-
In the JavaScript Code of Multiple Choice Quiz section, we will add logic for initializing our page. The logic must know what is correct and incorrect, So weâll define there the correct option and then weâll set that when the user clicks on the button the logic will generate and tell the user about his/her score.
Restaurant Website Using HTML and CSS
Through this blog, we have learned how to design Multiple Choice Quizzes using HTML, CSS & JavaScript.
Final Output Of Multiple Choice Quiz in HTML and JS Code:
Now Iâm looking for some positive reviews from your side.
So, How was the blog Learners,
If you want a more interesting blog like this then please check our Blog sites. Stay tuned because every day you will learn something new here.
I hope that Iâm able to make you understand this topic and that you have learned something new from this blog. If you faced any difficulty feel free to reach out to us with the help of the comment box and if you liked it, please show your love in the comment section. This fills bloggersâ hearts with enthusiasm for writing more new blogs.
Ecommerce Website Using Html Css And Javascript Source Code
Happy Coding
click and get full article and get complete source code
Thatâs it, folks. In this article, we shared 10+ Portfolio Website templates with cool and different designs.
Hope you liked this article. Share this with your fellow developers. Comment down below your thoughts and suggestions, we would love to hear from you.
See our other articles on codewithrandom and gain knowledge in Front-End Development.
Thank you and keep learning!!
follow us on Instagram:Â Ashutosh Mishra
2 notes
·
View notes
Text
10+ HTML CSS Portfolio Websites (Demo + Source Code)
Portfolio Websites Using HTML and CSS (Source Code)
Are you looking for the best portfolio websites with source code?
Another blog, another step towards our learning journey, Hey my enthusiastic developers, welcome to Codewithrandom with a new blog. Today we will see different portfolio websites for web developers with source code. Whether you are a beginner or a professional web developer these best portfolio websites with source code will always help you.
We have the best-handpicked portfolio websites with source code. Learn Custom-made free portfolio source code with code and demo for you.
Related Project:-
Create Portfolio Website Using HTML and CSS (Source Code)
Personal Portfolio Website Using HTML & CSS With Source Code
25+ Web Developer Portfolios Including Jack Jeznach
Letâs see some cool 10+ Portfolio Website Templates.
1. Personal Webpage
Bradley Engelhardt HTML, CSS, and JS A single page scrolling portfolio site I was working on using fullpage.js, wow.js, and animate.css.
2. Portfolio
Emerald Teve HTML, CSS, and JS A personal portfolio that is mostly made for web browsers, but can be accessed on phones as well. Looks better in portrait mode on phones, though.
3. Portfolio Page
Yago EstĂ©vez HTML(Pug), CSS, and JS(Babel) After 7 months of programming everyday and more than 30 projects built, this is last project I made for the FreeCodeCamp curriculum. Itâs been a great journey and I learnt A LOT of new stuff!
4. Portfolio page with animations
Islam Ibakaev HTML, CSS(SCSS), and JS Super awesome portfolio with off-canvas menu and a lot of animations.
5. Portfolio
click and get full article and get complete source code
Thatâs it, folks. In this article, we shared 10+ Portfolio Website templates with cool and different designs.
Hope you liked this article. Share this with your fellow developers. Comment down below your thoughts and suggestions, we would love to hear from you.
See our other articles on codewithrandom and gain knowledge in Front-End Development.
Thank you and keep learning!!
follow us on Instagram: Ashutosh Mishra
1 note
·
View note
Text
Spin Wheel Game using HTML and JavaScript (Source Code)
Hello Coder, Welcome to todayâs tutorial on Codewithrandom. Weâll learn how to make a Spin Wheel Game Using Html, Css, and JavaScript Code. This Spin Wheel Game feature is mostly used in mobile games where the player has to rotate it for getting more coins.
In the Spin wheel we have a spin wheel have circle and circle divided into 6,7 or 8 part and every part give a coin, any discount percentage, or anything related to website content we click on spin and the wheel start spinning and stop at an arrow so we get what our luck is, that it spins the wheel.
25 FREE HTML Games (Source Code)
The HTML(Hypertext Markup Language) part will design the structure of the spin wheel game with some appropriate labels in it.
Then the CSS(Cascading Stylesheet) will help to style the app with some colors and weâll provide an alignment and a position to it.
Then lastly the JS(JavaScript) code will help the app to make responsive as per the need of the user.
A spin wheel is a circle created with the help of CSS, along with different block-level elements for adding the color and number scheme inside the wheel, and then using the javascript function to move the circle for a particular time period, the wheel stops at a random number, and then using the javascript function to check the details and reward according to the number.
Before we start with our project, letâs understand some of the basic concepts related to it that will help us better understand the project.
How do we make a spin wheel?
To create a spin wheel, we will be using a block-level element like a div, adding a class to the element, and then using the class inside the CSS file. Using the border property, we will set the border to 50% to give a circle-like look to the element.
Can we customize the spin wheel?
Yes, you can customize the spin wheel as per the requirements; you can add sound movement as the user spins the wheel; and you can also add a 3D look to the spin and wheel by adding new designs.
50+ HTML, CSS & JavaScript Projects With Source Code
I hope you have got an idea about the project.
This HTML Code has fully defined the structure for our spin wheel app. In this code, basically user get confuse in understanding meta tags but donât worry.
Gym Website Using HTML and CSS With Source Code
Tip: You call simply use (!) an exclamation mark to define it if you are using VS Code.
Now lets get back to the code. Here we have defined a container in which we have made a canvas which contains our wheel. We have defined a button to spin and then we have linked a image of wheel. Now lets us style and align the structure using CSS.
In this CSS code we have style the background with three using hex value from lighter to brighter diagonally. Then we have styled our container, canvas, button. Padded and aligned the image of the wheel. The text that will display the value after the rotation it has been aligned and provided a suitable font color. Let us code the JavaScript part to make it user responsive.
Weather App Using Html,Css And JavaScript
In this JavaScript we have we have defined the buttons, wheels & values in the starting. Then under var section we have given each pie a color using the hex code. Then we have defined the logical part to make the wheel rotate and give the value to the user. Let us see the final output of the project Spin Wheel App using HTML, CSS & JS.
Restaurant Website Using HTML And CSS With Source Code
Final Output Of Spin Wheel Game using HTML and JavaScript
If you find out this Blog helpful, then make sure to search Codewithrandom on Google for Front End Projects with Source codes and make sure to Follow the @ashutoshmishra.52 Instagram page.
click and read full article and get fully complete source code
Ashutosh Mishra
0 notes
Text
Online Book Store Project in HTML and CSS (Source Code)
Online Book Store Website Project in HTML and CSS Code
Hello Coder! Welcome to Codewithrandom Blog. Today we are going to create Online Book Store Project Using Html and Css. We Added a Book Slider, Author Category, and Book category, popular books by category in the Online Book Store Website.
The Online Book Store Website Project is a front-end development project based on the concept of an E-commerce store. But How? It is because it enables the option of ordering, packaging, and delivery of the book available in this Book store UIÂ and hence we can buy according to our interests.
Preview Of Online Bookstore Project:-
Also in this project, we can search for books we want and make it to add to cart option for later buying. and also there is a rating option for every book in which we can confirm the quality of books based on the star rating. then we can order the books and make payment and enjoy reading.
Before we start with our project, we need to understand some of the basic concepts about Book store website using HTML
What is an online book store?
An online book store is a web app where the digital version or pdf version of every book is present in one place. The online book store is the same as an offline bookstore, where people need to find their desired book from a bundle of books, but in the online book store, you just need to search for the book name, and then you can proceed with the book payment.
What are the benefits of using an online book store?
1. All books are present on one website.
2. The purchase and sale of books are easy.
3. No Chances of book lost.
4. Easily accessible anywhere.
5. You can also share with anyone.
50+ HTML, CSS & JavaScript Projects With Source Code
The Project here is developed with the help of HTML and CSS, and the respective code is given below.
read full article and get complete source code
1 note
·
View note
Text
100+ Navigation Bar HTML and CSS (Free Demo +Source Code)
Navigation Bar Using HTML and CSS
Hello Developers! Welcome to Codewithrandom with another informative blog. Today weâll see how to make a Navigation Bar with Source Code. Here is the Latest Collection of free Navigation Bar codes in HTML and CSS. This is the Updated Collection of April 2023 with 36 New Navbar Source codes added.
What is a navigation bar?
A Navigation bar or a side menu is an integral part of any website, used for quick navigation links, a search bar, login/signup links, company logos, etc. Without a Navbar, any website looks incomplete.
Here weâll show you how to create a Simple Navigation Bar In HTML and CSS with 100+ examples.
Related article â 100+ HTML, CSS, and JavaScript Projects With Source Code ( Beginners to Advanced)
Restaurant Website Using HTML And CSS With Source Code
Letâs see some cool Navigation bars in HTML and CSS.
1. Responsive Side Navigation Bar
Letâs start our list with a simple, light-themed left-sided navigation bar. Only navigation bar icons are visible on load but on clicking the hamburger icon side bar expands.
2. Bootstrap Navigation Bar
Simple and responsive navigation bar. This one is on top with several different categories and also a search bar. Additionally, it also has a login and signup button.
How To Build Interest Calculator Using JavaScript
3. Transparent Navigation
This is a very well made Navigation bar by Manas Yadav, when you click a navigation bar button it auto scrolls to its location on the page. Can be used for homepages.
4. Sticky Slider Navigation (Responsive)
Another navigation bar auto-scrolls but this one is even better with more satisfying animations and design.
5. Navigation bar design
A navigation bar with a gradient in its background with a cool gradient and blinking effect on hover.
Thatâs it, folks. In this article, we shared the Navigation Bar In HTML And CSS Source Code with cool and different designs. We covered everything from simple and minimal Navigation bars to bars with auto scrolls, cool transitions, and even 3D icons. Hope you liked this article. Share this with your fellow developers. Comment down below with your thoughts and suggestions
See our other articles on Codewithrandom and gain knowledge in Front-End Development.
Thank you
read full article and get complete source code
1 note
·
View note
Text
Create News Website Using HTML and CSS (Source Code)
Hello Coder! Welcome to the Codewithrandom blog. In this article, we create News Website Using Html And Css With Source Code. We Create Fox News Template Design Using Html And Css With Code.
Fox News Template is one of the news websites built using HTML and CSS. Fox is a brand used for design templates and illustrators and moreover in popular design software like adobe XD, Photoshop, and more.
50+ HTML, CSS and JavaScript Projects With Source Code
This Template is based on a News article with a news heading, a detailed summary of the incident, the time of duration, and who posted⊠basically, this project helps to determine what the news website on the internet is like.
So make sure to work on it by your standards. the source code for the project is given below.
News Website Html Code:-
this is Html Code for News Website, you can see we did not create a boilerplate of html code because it baisc a thing. you just create a boilerplate in html code then copy and paste this code. also, important thing donât forget to link css file to html.
Only Html Code Output of News Website:-
Live Preview Of News Website Html and Css:-
Hope I think you got an idea of what a news template is like. and we create a total output for his project after HTML and CSS.
Restaurant Website Using HTML and CSS
Now you can use it for your own purpose but make sure to do adjustments on your own in order to learn and understand the project.
Good luck and best of lucks for your future. Also if you need a complete code to access your project then you can make use of the link given below.
get full source code and complete article click here
follow us Ashutosh Mishra
1 note
·
View note
Text
Create Feedback Form In HTML Code
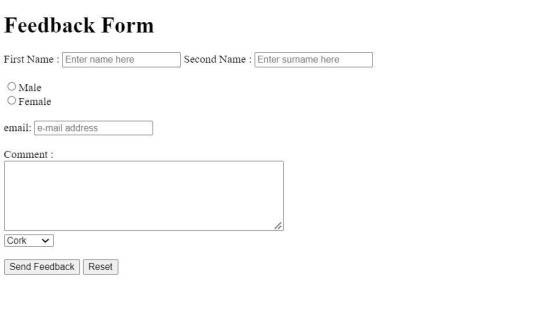
Hello coders, welcome to the codewithrandom blog. In todayâs blog, we are going to see how to create a simple feedback form using HTML code only that contains the first name, second name, checkbox, email address comment, and more HTML form input that is required for the feedback form.
A feedback form is a form where the user can enter their personal details, write about their point as feedback, and submit it. The feedback may be in any form, like events, trail classes, products, etc. So likewise, we are going to create this project using HTML code only.
100+ JavaScript Projects For Beginners With Source Code
Before we dive into the projectâs step-by-step method, letâs understand some general concepts about feedback forms using HTML.
What is a feedback form?
A feedback form is a type of survey form that most websites or tele-customer support employ to get user feedback. This survey form is used to collect different feedback from different users, which helps them analyze the most common problems of multiple users and provide them with
What is a form element?
A form element is an HTML predefined code that helps in creating different types of forms to collect different types of data. The basic format of a form element is:<form> /* form Elements*/ </form>
What are the different types of forms?
The different types of Forms are:
1. login Form
2. Signup Form
3. Newsletter form.
4. Feedback form.
5. Common Entrance Form.
So, Letâs Begin Our Feedback Form Project By Adding The Source Codes. For That, First, We Are Using The Html Code.
Html Feedback Form Code:-
Here, we have successfully added HTML code. In this code, first we create a title with an h1 tag and then create a form class with a method. Now, inside the form class, we started adding every item. Like first name, second name, gender, email, comment (feedback), and lastly, submit button.
Restaurant Website Using HTML and CSS
Each one is added with the input tags with separate names and placeholders, and the comment box, which is the feedback area, is done with a text area tag with a name and columns. Additionally, we have created a section for choosing countries by drop-down list, and that is done with an option tag that specifies the country name for selection. Lastly, we created two separate buttons, âSend Feedbackâ and âReset,â with the help of input tags that consist of type and value.
So thatâs all. We have created a feedback form using simple HTML. Now we can take a preview of our project in the mentioned output section.
Ecommerce Website Using HTML, CSS, & JavaScript (Source Code)
Final Output Feedback Form in HTML Code:-
click and get more and complete source code
0 notes
Text
15+ HTML CSS Projects With Source Code( instagram clone)
15+ HTML CSS Projects With Source Code
Hello Coder! In this article, we give you 10+ HTML and CSS projects with source code. We also add mini projects in HTML and CSS with source code so you can learn and practice more.
We will provide you best HTML and CSS beginner projects to advance Project in this article. Whoever practices these projects will be able to understand all of his concepts. They will acquire appropriate skills that are in demand and aid in landing jobs as a result of this. This directly benefits developers in enhancing their skills.
Also, Checkout These 50+ Project Using HTML, CSS, and JavaScript Project With Source Codeđ
50+ HTML, CSS and JavaScript Projects With Source Code
1. Survey form Using HTML and CSS
Input HTML is essentially the only thing the survey form requires. You get knowledge of HTML input such as text-area fields, checkboxes, radio buttons, file uploads, date pick-up input, input validation without JavaScript, and many others with this project..
Every website needs a form to connect its user to the web owner so everyone must know that to create a form. this is the best HTML CSS project for new learners.
Source Code link đ
Survey form source code
Simple Portfolio Website Using Html And Css With Source Code
2. Parallax website
Using the web design method of parallax scrolling, the website background scrolls more slowly than the foreground. As users scroll down the website, a 3D effect appears, adding depth and making the browsing experience more engaging.
In this project, you learn many things including background images property and scroll property. this is Html Css Project that makes the website 3d scroll.
Source Code link đ
Parallax website source code
3. User profile card
When we visit any website we see a card on every website but it may be an invitation card, or information card inbox with some short detail.
So in this project, youâll learn about how to create a simple type of card and also make it better with your CSS skills. this is HTML CSS project that you can use in your portfolio.
But Wait! Before moving on to the source code link, I would like to tell you that we have launched an E-Book âMaster Frontend Development: Zero to Heroâ Just for You. This E-Book includes hand-crafted lessons on HTML, CSS, Javascript, and on Bootstrap. Not only that, but this E-Book also has hundreds of projects and interview questions. Hurry! limited-time offer, Here is the link to the E-Book â Master Frontend Development: Zero to Hero
Source Code linkđ
User profile card
Gym Website Using HTML ,CSS and JavaScript (Source Code)
4. Dynamic Js Quiz
Itâs a JavaScript project in essence, but the design may be made with only HTML and CSS without any functionality. The project rating will be eliminated if you want to add a quiz option. This project teaches you about radio buttons and how to use JavaScript to add content.
Quiz project source code
5. Landing page
A landing page is a page that a user arrives at after clicking a particularly targeted hyperlink. Unlike the homepage or any other page on the website, a landing page is a separate page.
It solely fulfills the precise function for which it was designed, which could range from recommending a service to your visitor to selling or promoting a product. For your convenience, weâve provided a link to a landing page from Codepen below.
Source Code link đ
Landing page HTML CSS
Ecommerce Website Using HTML, CSS, and JavaScript (Source Code)
6. Instagram home clone
The Instagram home page clone is clearly visible in this project. Itâs not responsive, but itâs still one of the greatest projects for someone who knows HTML and CSS.
For HTML/CSS developers, this is an advanced-level project since you learn a lot from it, including how to use Flexbox, grids, positioning, hover effects, 3D effects, and section dividing among many other things. A simple front-end project can be expanded upon by adding backend support to become a full-stack project. For your portfolio, you absolutely need a full-stack clone website.
Source Code link đ
Instagram home clone
click me and get full article and get complete source code
0 notes
Text
100+ JavaScript Projects For Beginners With Source Code
Hello developers! In this article, we will provide you with 100+ JavaScript Projects For Beginners With Source Code. This article would enhance your JavaScript skill set and help you become an expert developer.
Weâll share more than 100 JavaScript Projects For Beginners In 2023 to aid developers in gaining practical project experience.
50+HTML, CSS and JavaScript Projects With Source Code
We will provide you best beginner-level JavaScript projects to advance the Project in this article. Whoever practices these projects will be able to understand all of his concepts. They will acquire appropriate skills that are in demand and aid in landing jobs as a result of this.
Furthermore, if youâre looking to deepen your understanding of JavaScript, here is a free JavaScript course that you can consider.
JavaScript Project with source code projects is available on this page. This is a thorough compilation of beginner to advanced-level projects for users of various skill levels.
So that you can get the source code for any JavaScript project, we share the project name, description, and Source Code link with you.
Advanced JavaScript Projects With Source Code
Project details-
Browser support: Chrome, Edge, Firefox, Opera, Safari
Responsive: Yes
language: Html, CSS and JavaScript
Project code download:Â Click Here

Project details-
Browser support: Chrome, Edge, Firefox, Opera, Safari
Responsive: Yes
language: Html, CSS and JavaScript
Project code download:Â Click Here

click me to see complete article and get full source code freely available
follow us Ashutosh Mishra
instagram :Â @geekcoder.52
0 notes
Text
15+ Shopping Cart Template HTML and CSS(Free Demo+Code)
Shopping Cart Template HTML and CSS
Hello Coder! Welcome to Codewithrandom with a new blog. Today We will share the Top 15 Shopping Cart Templates using HTML and CSS.
What is a shopping cart HTML template?
A shopping cart is an essential part of any E-commerce or retailerâs website or online platform store, which provides all its services under one roof right from browsing products to placing orders to the payment gateway.
Shopping Carts are used by clients/customer to shortlist their favorite products and lead them or proceed with them.
Related Article â 50+ HTML, CSS, and JavaScript Projects With Source Code
                  15+ Awesome Bootstrap Sidebar Menu Templates
Here today we will learn to design our own Bootstrap Shopping Cart UI comprising of a payment button along with items shortlisted displayed efficiently.Â
Training for web developers and IT Trainees to create a dynamic shopping cart interface.
Happy exploring and learning!
Below are the Shopping Cart Template HTML CSS example collections with Free Source code.
1. Bootstrap 4 shopping bag checkout with the order summary
Here You Can See How The Above Bootstrap Shopping Cart Project Depicts The Bootstrap 4 shopping bag checkout with the order summary Implemented Using HTML And CSS.
In The Above-Displayed Bootstrap Shopping Cart Project, We Have For you a Bootstrap 4 e-commerce shopping cart with item summary Using HTML, And CSS.
3. Bootstrap 4 e-commerce shopping cart with plus minus icons
Here You Can See How The Above Bootstrap Shopping Cart Project Depicts a Bootstrap 4 e-commerce shopping cart with plus-minus icons Implemented Using HTML And CSS.
Read also:Â 100+ JavaScript Projects With Source Code ( Beginners to Advanced)
read complete article and get full source code freely
0 notes
Text
Personal Portfolio Website Using HTML and CSS
Personal Portfolio Website Using HTML and CSS With Source Code
Hello Coder, today weâre going to learn how to Create a Personal Portfolio Website Using HTML and CSS With Source Code. A portfolio can be compared to a digital resume that displays a userâs skills for a client. A developerâs portfolio is a website they use to advertise their abilities so that employers can recruit them based on their skill set.
By following these instructions, you can simply make this Personal Portfolio Responsive website in HTML CSS Code. Simply by adhering to the procedures mentioned below, you will be able to develop this amazing personal portfolio website.
On this portfolio website, there is a button on the right, some navigation links in the middle, and a navigation bar with a logo on the left. The next step is to click the âDownload CVâ button that is located next to the text that describes the authorâs name and occupation on the left side of this website, as shown in the image.
Before we dive into the projectâs step-by-step solution, we need to understand some general concepts about personal portfolio websites using HTML and CSS.
Create Stylish Login Page In HTML And CSS Code
What is a Personal Portfolio Website?
A persona portfolio website is a digital type of resume that is present on the web. Instead of using a hard copy of the resume and roaming different companies for jobs, this is the best method to share your online resume with different recruiters along with your project work, experience, and achievements all in one place. This helps recruiters find the most suitable candidate for the jobs, and using a personal portfolio website for job interviews gives an edge to different candidates without a personal portfolio.
What are the benefits of a Personal Portfolio Website?
The benefits of using a personal portfolio website are:
increase in selection chance.
provide maximum reach.
allows you to showcase your skills.
50+HTML, CSS, and JavaScript Projects With Source Code
100+ HTML,CSS and JavaScript Projects With Source Code ( Beginners to Advanced)
Live Preview Of Personal Portfolio Website Source Code:-
If you like this portfolio and would want the source code, I have included all the codes for this program below the link to this program, from which you can quickly obtain the source files of this program. By adding your own unique touches, you can use this portfolio to advance it.
Gym Website Using HTML and CSS With Source Code
Personal Portfolio Website Html Code:-
Read more and get complete source code freely available
You have completed learning Personal Portfolio Website Using HTML And CSS With Source Code. I hope I have explained to you in this tutorial how I created this Personal Portfolio Website.
 Source code Click HereÂ
If you enjoyed reading this post and have found it useful for you, then please give share it with your friends, and follow me to get updates on my upcoming posts. You can connect with me on Instagram.
if you have any confusion Comment below or you can contact us by filling out our Contact Us form from the home section. đ€đ
follow on codewithrandom
 Source code Click HereÂ
0 notes
Text
E-commerce Website Using HTML, CSS, and JavaScript (Source Code)
E-commerce Website Using HTML, CSS, and JavaScript
Hello Coder, In this article, we are going to create an e-commerce website using HTML, CSS, and JavaScript with source code. This is a simple e-commerce website that has a product slider with images, a product section with a product card, and all the necessary features that e-commerce websites have.
Dear Coders: Letâs explore how to create an E-commerce website source code using HTML, CSS, and JavaScript. We use HTML code to build the foundation of an e-commerce website, and CSS code to style it. Every code is discussed in depth. Next, we leverage certain functionality from an e-commerce website, and to do this, we write JavaScript code.
What is an E-commerce Website?
An e-commerce website is an online or digital shopping complex from which users can order different types of items from the comfort of their homes. These online shopping websites let you select clothing 24/7, from electronics to food items; all things can be delivered to their homes.
What are the benefits of an E-commerce Website?
The benefits of an E-commerce website are:
24Ă7 customer support
Different payment methods.
Fully return policy.
Delivery all over the world.
Itâs time to move to your e-commerce website if youâre becoming tired of buying on Amazon, Flipkart, or any other e-commerce website and want to try something different.
100+ HTML,CSS and JavaScript Projects With Source Code ( Beginners to Advanced)
Hey, wait one second, you do not have your own website? Donât feel bad; I am here to help you learn. How to build an e-commerce website, so stay connected to the blog till the end. Once you have your e-commerce website, do shopping as much as you want.
Video Tutorial Of E-commerce Website Source Code:-
youtube
Letâs have a look at the websiteâs appearance before going into the process used to create it. You can browse my elegant online store.
Live Preview Online E-commerce Website Source Code:-Â Â Here you can check it.
After viewing my responsive e-commerce website plan, you likely already have an idea for your own website.
Ecommerce Website Code
The following skills are necessary for creating this webpage. They are the essential components you should possess for creating an e-commerce website:
1. HTML. 2. CSS. 3. Javascript(Basics).
HTML Code For E-commerce Website:-
The Skelton system (the frame/structure of bones) of the human body gives a structure/layout to the human body. Similarly, HTML code does the same it gives the layout of the websites.
Donât worry you donât need any biological knowledge for this website.
Restaurant Website Using HTML and CSS
Every webpage has three main sections:- 1.Header(Navigation bar/menu/logo,etc.) 2.Main section(main content). 3. Footer.
1. Header:-
Below there is a picture of the navigation bar which contains the brand name/logo, search bar, and menu items, this all comes under the header.
1. In the header section, firstly make three divs. One for the logo, the second for the search bar, and the third for menu lists.
In the second class, I used the search icon I have imported through Ionicon, for any type of icon used in website development you can use it.
Gym Website Using HTML and CSS With Source Code
Input is a tag used for many purposes like checkboxes, radio, email input, date, time, and others. But here we are using for search bar so type is text. For more ideas, you can refer to our other blogs.
As our website should be Responsive(A Responsive website is automatically adjusted for different screen sizes and viewports.)
For Example:-Blogger website is also responsive you can easily use comfortably it on a phone(small screens) and PC(big-sized screens).
So when we are using the website there is a problem that it is not able to show all the menu lists on the navbar. Finally, we decided to make a button-operated menu list. So when you click on the menu icon It will open into a menu list which you can see in the next image.
10+ HTML CSS Projects For Beginners (Source Code)
ou have seen how will solve the problem but now itâs time to code. Add a menu class in which a close icon you can see in the above image and also add a menu icon in the heading1 class.
2. Section:-
Section portion which mainly contains the content of a website is divided section mainly into two portions:-
section1 which contains an image-slider contains images. Section 2 which contains the container class contains different items.
Use the image tag to add various images to the image slider. Add a container named âclassâ inside Section 2 so that it inserts things named âclassesâ on your website as many times as you like. Add the item name, item price, item image, and item data classes to every item. You can also add one more category if youâd like.
read more and get complete source code
full credit follow us @codewithrandom
follow me @geekscoder.52
0 notes
Text
Travel/Tourism Website Project Using HTML and CSS Code
Create A Travel/Tourism Website Project Using HTML and CSS Code
Hello Coder, This post will teach you how to create a Travel/Tourism Website Project Using HTML and CSS Code. The webpage Iâm going to share with you in this article is about traveling. Itâs quite simple, and youâll have your own Travel Tourism Website by the end of this article.
What is a Travel website?
A travel website is a sort of business that provides customers with travel-related services. When it comes to the future of this industry, numerous aspects will influence how consumers plan their vacations. The rise of mobile usage, greater competition, and a shift in customer expectations are among these factors. The ideal tool for tourists is the travel website creator.
50+ HTML, CSS and JavaScript Projects With Source Code
People are increasingly using their mobile gadgets when traveling, for example. As a result, businesses should ensure that their websites are mobile-friendly. To enhance their profits, companies employ an easy website builder for small enterprises. They should also be aware that competition among online booking sites is increasing.
I hope now you have a general idea of what the project entails. In our article, we will go over this project step by step.
Step1: Setting Up the Structure (HTML)
Let us begin by establishing a Travel/Tourism project. Create a new index.html file in a new subdirectory to house the website layout. copy and paste the HTML code given below into your HTML file.
read more...
0 notes
Text
50+HTML, CSS and JavaScript Projects With Source Code
The 50+ HTML, CSS, and Javascript projects list is a boon for beginner developers who wish to make a career in the field of web development. But for that, developers have to go through a lot of learning and project building, and while on the path of skill enhancement, they take a bit of stress because they donât easily find the topics on which they create their projects. So in the world of web development, we have curated a list of 50+ beginner-friendly HTML, CSS, and JavaScript projects.
Almost 50 of the most significant projects will be covered in this post; these Web Development Projects With Source Code will help you build a strong foundation. You will gain practical experience with the project and be able to develop new, large projects that involve numerous websites as a result of working on this front-end project.
You Get 50+ Web Development Projects with Source Code for total beginners using HTML, CSS, and JavaScript.
We include projects using HTML CSS and Javascript with source code from beginner to intermediate levels covered in this article.
you get all project source code with code explanation. project is very helpful for practicing coding skills and logic building so you definitely need to create some projects that help you to get a dream job and you can add projects to your CV/Resume.
If you want more frontend projects then donât forget to visit my 100+ HTML, CSS, and JavaScript Projects with the source code, So Must Visit the articleđ.
100+ JavaScript Projects With Source Code ( Beginners to Advanced)
1. Social media share icon animationÂ
Project details â Social media icons are used for the identification of specific social media platforms. Each social media platform has different icons. Social media platforms are used to connect people from faraway places and provide a feeling of closeness. Adding animations to icons provides great user interaction.
Browser support:Â Chrome, Edge, Firefox, Opera, Safari
Responsive:Â Yes
language:Â Html, CSS
Project Code Download:Â Click Here
Project Demo :
3. Card With Hover Effect
Project Details â Cards are small pages that are used to display product information. Cards with a hover effect provide a great user experience, and when the user hovers over the cards, information about the product is displayed to the customer. These cards are generally used on e-commerce websites.
Browser Support:Â Chrome, Edge, Firefox, Opera, Safari
Responsive:Â Yes
language:Â Html, CSS
Project Code Download:Â Click Here
Project Demo:
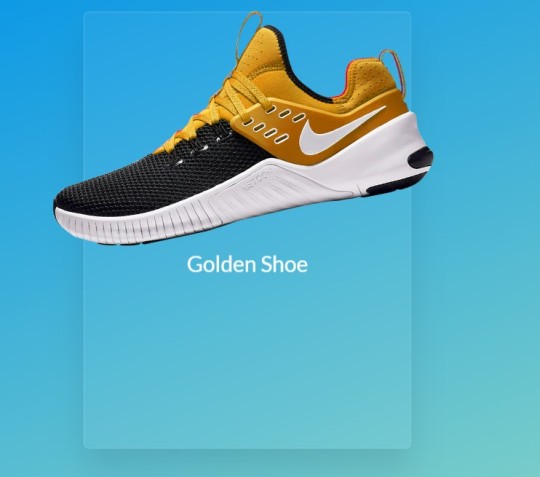
read full artical and more intresting projects with source code
3 notes
·
View notes
Text
25 HTML Games(Free code + Demo)
FREE HTML Games With Source Code
So our developers, these are the Simple HTML game codes that you can Copy And Paste to Your VS Code Editor, and Your Game will be ready to Run in the Browser.
Related Article:-
20+ JavaScript Game For Beginners (Demo + Free Code)
Build the Flappy Bird Game using HTML and JavaScript Code
Build a Maze Game Using JavaScript (Source Code)
2. Beer Filling Game
3. Shoot EasterBunny
4 . An Exciting HTML Game
5. Reaction Wall Game
7. JUST HTML GAME â no css, no js
8. The spy: an html game
9. Backbone Tetris
10. Piano Tiles [ JavaScript ]
read more artical click me
follow on instagram
@codewithrandom
@geekscoder.52
0 notes
Text
Restaurant Website Using HTML And CSS With Source Code
Restaurant Website Using HTML And CSS With Source Code
Hello coder, Welcome to the Codewithrandom blog. In this article, we create a Restaurant Website using HTML and CSS with Source Code. This is a Simple Restaurant Website with a main home page, types of food available, a food menu, customer reviews, and a contact form section on the restaurant website.
The HTML code for the Restaurant Website is the first thing we develop, and after that, the CSS for styling and JavaScript code. we add JavaScript for smooth scrolling on our website otherwise javascript is optional for this project.
Letâs Start a Responsive and Amazing Restaurant Website Using HTML and CSS. Letâs code a Simple website, We use 1,000+ lines of code to make our Restaurant Website Fully Responsive.
100+ HTML,CSS and JavaScript Projects With Source Code ( Beginners to Advanced)
Basic Knowledge About Project
Itâs a front-end intermediate-level project that uses some advanced frameworks like CSS and JavaScript to make the project more elegant and responsive. We have provided you with the project with step-by-step explanations, which will help you better understand the project.
Before we dive into the step-by-step solution to the project, letâs understand some of the basic concepts about restaurant websites using HTML, CSS, and Javascript.
What is an online Restaurant Website?
An online restaurant website is a digital version of any restaurant that allows users to order food directly from the restaurant while sitting at home. These websites were created for customers who wish to eat restaurant food at home. The consumer can select items from the food menu and make payments for faster deliveries.
What are the benefits of Online Restaurant Websites?
reaches the maximum number of customers.
Easy to order
Hassle-free payments
increase in customer reach.
increase in profit.
Live Preview Of Restaurant Website Source Code:-
Restaurant Website Html Code:-
In this HTML code, we create a complete navbar for the footer structure of the restaurant website. first, we link our CSS and JavaScript files in html code. now we add a font awesome icon CDN link in the code because we create the What Our Customers Say section on the website and we show that review with a star so we use a font awesome icon.
Gym Website Using HTML ,CSS and JavaScript (Source Code)
then we create the structure of the navbar and itâs very important in our website because we create a responsive navbar in the project. then we create some simple sections of the restaurant website like what food is available and a food menu list.
and lastly, we create a contact form and a footer copyright line at end of the website.
This is almost 300 lines of HTML code for the restaurant website, and you can see the output below with only the HTML code output of the restaurant menu. Then we add CSS code for styling our restaurant website.
Output Of Only HTML Code For Restaurant Website:-
This is all image output that we create using only HTML code. Now the time is to style our restaurant website using cssđ.
Restaurant Website HTML CSSÂ Code:-
In CSS code we include Poppins font from Google font. then we style all sections of the website step by step. we write comments in CSS code so if you want to customize any of the restaurant website parts you can change that section code and itâs all done.
Responsive Resume/CV Website Using HTML & CSS
This is our whole CSS code with 600+ lines. We style our Restaurant Website step by step. We style the utility class and then style our navbar. After the navbar, we styled every html sectionđ„.
At the end of the code, we use media queries to make our Restaurant Website completely responsive and mobile-friendly. So you can see the output with CSS code. Then we add a little bit of javascript for the scroll effect otherwise our website is completely ready with code.
Ecommerce Website Using HTML, CSS, and JavaScript (Source Code)
Output Of HTML CSS Code Restaurant Website:-
You can see this awesomeđ output with HTML + CSS Code for Restaurant Website. Now we add JavaScript for smooth scrolling.
this is completely optional to add JavaScrpt to this project.
Thatâs it for our Whole restaurant website code. We write every useful code like making the website mobile-friendly and adding media queries for this. Add JQuery code for smooth scrolling on the restaurant website.
50+ HTML, CSS & JavaScript Projects With Source Code
[su_button id=âdownloadâ url=âhttps://drive.google.com/drive/folders/1Wx9W99uIH3WiO1nkz6aJYCuXVv_ibGmA?usp=sharingâ target=âblankâ style=â3dâ size=â11âł wide=âyesâ center=âyesâ icon=âicon: downloadâ]DOWNLOAD NOW[/su_button]
Final Output For Restaurant Website Using HTML and CSS Code
You can see this video output to see the complete output. You can see how smooth the website scrolls and this design.
Hope you like this Restaurant Website Project, we create your own and use this project in any project as a part project like the reviews section, and a contact form. If you need any more project-related frontend. Visit our homepage and you get 100+ projectsđ.
Age Calculator Using Html Css Javascript ( Source Code )
if you have any confusion Comment below or you can contact us by filling out our Contact Us form from the home section. đ€đ
Code By â Sanket Bodake
was written by â Codewithrandom
FAQ For Restaurant Website
Which code editor do you use for this Restaurant Website project coding?
I personally recommend using VS Code Studio, itâs very simple and easy to use.
is this project responsive or not?
Yes! This project is responsive.
What is an online Restaurant Website?
An online restaurant website is a digital version of any restaurant that allows users to order food directly from the restaurant while sitting at home. These websites were created for customers who wish to eat restaurant food at home. The consumer can select items from the food menu and make payments for faster deliveries.
What are the benefits of Online Restaurant Websites?
1. Reach the maximum number of customers. 2. Easy to order 3. Hassle-free payments 4. Restaurant Like food at Home. 5. Increase in profit.
2 notes
·
View notes