#Spring Boot vs. Spring MVC
Explore tagged Tumblr posts
Text
Why Java Is Still the King in 2025—and How Cyberinfomines Makes You Job-Ready with It

1. Java in 2025: Still Relevant, Still Dominating Despite the rise of new languages like Python, Go, and Rust, Java is far from dead—it’s actually thriving.
In 2025, Java powers:
40%+ of enterprise backend systems
90% of Android apps
Global banking & fintech infrastructures
E-commerce giants like Amazon, Flipkart & Alibaba
Microservices and cloud-native platforms using Spring Boot
Java is reliable, scalable, and highly in demand. But just learning syntax won’t get you hired. You need hands-on experience, framework expertise, and the ability to solve real-world problems.
That’s exactly what Cyberinfomines delivers.
2. The Problem: Why Most Java Learners Don’t Get Jobs Many students learn Java but still fail to land jobs. Why?
❌ They focus only on theory ❌ They memorize code, don’t build projects ❌ No real understanding of frameworks like Spring Boot ❌ Can’t explain their code in interviews ❌ Lack of problem-solving or debugging skills
That’s where Cyberinfomines’ Training changes the game—we teach Java like it’s used in real companies.
3. How Cyberinfomines Bridges the Gap At Cyberinfomines, we:
✅ Teach Core + Advanced Java with daily coding tasks ✅ Use real-world problem statements (not academic ones) ✅ Give exposure to tools like IntelliJ, Git, Maven ✅ Build full-stack projects using Spring Boot + MySQL ✅ Run mock interviews and HR prep ✅ Help you create a Java portfolio for recruiters
And yes—placement support is part of the package.
4. Java Course Curriculum: Built for the Real World Core Java
Data types, loops, arrays, OOP principles
Exception handling, packages, constructors
File handling & multithreading
Classes vs Interfaces
String manipulation & memory management
Advanced Java
JDBC (Java Database Connectivity)
Servlet Lifecycle
JSP (Java Server Pages)
HTTP Requests & Responses
MVC Design Pattern
Spring Framework + Spring Boot
Dependency Injection & Beans
Spring Data JPA
RESTful API Creation
Security & authentication
Connecting with front-end apps (React/Angular)
Tools Covered
IntelliJ IDEA
Eclipse
Postman
Git & GitHub
MySQL & Hibernate
Live Projects
Library Management System
Employee Leave Tracker
E-Commerce REST API
Blog App with full CRUD
Interview Preparation
DSA using Java
Java-based coding problems
100+ mock interview questions
HR round preparation
Resume writing workshops
5. Who Should Learn Java in 2025? You should choose Java if you are:
A fresher who wants a strong foundation
A non-tech graduate looking to switch to IT
A teacher/trainer who wants to upskill
A professional aiming for backend roles
Someone interested in Android development
A student looking to crack placement drives or government IT jobs
6. Real Success Stories from Our Java Learners
Amit (BSc Graduate) – Now working as a Java backend developer at an IT firm in Pune. Built his confidence with live projects and mock tests.
Pooja (Mechanical Engineer) – Switched from core to IT after completing Cyberinfomines’ Java program. Cracked TCS with flying colors.
Rahul (Dropout) – Didn’t finish college but now works remotely as a freelance Spring Boot developer for a US-based startup.
Every story started with zero coding experience. They ended with real jobs.
7. Top Java Careers in 2025 & Salary Trends In-demand roles include:
Java Backend Developer
Full Stack Developer (Java + React)
Android Developer (Java)
Spring Boot Microservices Architect
QA Automation with Java + Selenium
API Developer (Spring + REST)
Starting salary: ₹4.5 – ₹8 LPA (for freshers with strong skills) Mid-level: ₹10 – ₹20 LPA Freelancers: ₹1,000 – ₹2,500/hour
Java is stable, scalable, and pays well.
8. Certifications, Tools & Practical Add-Ons After training, you’ll earn:
Cyberinfomines Java Developer Certificate
Portfolio with at least 3 GitHub-hosted projects
Proficiency in IntelliJ, Maven, Git, MySQL
Resume aligned with Java job descriptions
Interview recordings and performance feedback
9. What Makes Cyberinfomines Java Training Different
✔ Human mentorship, not just videos ✔ Doubt sessions + code reviews ✔ Classes in Hindi & English ✔ Live assignments + evaluation ✔ Placement-oriented approach ✔ No-nonsense teaching. Only what’s needed for jobs.
We focus on you becoming employable, not just completing a course.
10. Final Words: Code Your Future with Confidence Java in 2025 isn’t just relevant—it’s crucial.
And with Cyberinfomines, you don’t just learn Java.
You learn how to:
Solve real problems
Write clean, scalable code
Work like a developer
Get hired faster
Whether you’re starting fresh or switching paths, our Java course gives you the skills and confidence you need to build a future-proof career.
📞 Have questions? Want to get started?
Contact us today: 📧 [email protected] 📞 +91-8587000904-905, 9643424141 🌐 Visit: www.cyberinfomines.com
0 notes
Text
Essential Full Stack Development Interview Questions to Prepare For Your Next Job Opportunity
The demand for skilled full stack developers continues to grow as more companies seek professionals who can handle both the front-end and back-end development of applications. Preparing for a full stack development interview involves understanding a wide range of concepts that cover various technologies, frameworks, and programming practices.
To set yourself apart and confidently face interviews, consider exploring these essential full stack development interview questions. And for an insightful video overview of full stack interview preparation, check out this Full Stack Developer Interview Preparation Guide.
1. What is Full Stack Development?
Full stack development refers to the practice of working on both the front-end (client-side) and back-end (server-side) of a web application. A full stack developer is proficient in multiple technologies that enable them to build fully functional web applications from start to finish.
Key Points to Discuss:
Differences between front-end, back-end, and full stack development.
Advantages of hiring a full stack developer for a project.
2. What Are the Most Commonly Used Front-End Technologies?
Front-end development involves creating the user interface and ensuring a seamless user experience. The most popular front-end technologies include:
HTML: The standard markup language for creating web pages.
CSS: Used to style and layout web pages.
JavaScript: Essential for interactive features.
Frameworks/Libraries: React, Angular, and Vue.js.
Follow-Up Questions:
How do these technologies differ in terms of use cases?
Can you explain the benefits of using a front-end framework like React over vanilla JavaScript?
3. Explain the Role of Back-End Technologies in Full Stack Development.
The back-end of an application handles the server, database, and business logic. Key technologies include:
Node.js: A JavaScript runtime for server-side programming.
Express.js: A web application framework for Node.js.
Databases: SQL (e.g., MySQL, PostgreSQL) and NoSQL (e.g., MongoDB).
Other Languages: Python (Django, Flask), Ruby (Rails), and Java (Spring Boot).
Important Discussion Points:
RESTful services and APIs.
Authentication and authorization mechanisms (e.g., JWT, OAuth).
4. How Do You Ensure Code Quality and Maintainability?
Interviewers often ask this question to understand your approach to writing clean, maintainable code. Emphasize:
Version Control: Using Git and platforms like GitHub for collaborative coding.
Linting Tools: ESLint for JavaScript and other language-specific tools.
Code Reviews: The importance of peer reviews for improving code quality.
Best Practices: Following design patterns and SOLID principles.
5. Can You Discuss the MVC Architecture?
The Model-View-Controller (MVC) architecture is a common design pattern used in full stack development. Each part of the pattern has a distinct role:
Model: Manages data and business logic.
View: The user interface.
Controller: Connects the Model and View, handling input and output.
Why It’s Important:
Helps organize code, making it more scalable and easier to maintain.
Many frameworks, such as Django and Ruby on Rails, are built on MVC principles.
6. What Is REST and How Is It Used in Full Stack Development?
Representational State Transfer (REST) is an architectural style used to design networked applications:
Key Features: Stateless, cacheable, and uses standard HTTP methods (GET, POST, PUT, DELETE).
Implementation: Building RESTful APIs to enable communication between client and server.
Common Follow-Ups:
How do RESTful APIs differ from GraphQL?
Can you provide an example of designing a RESTful API?
7. Explain the Role of Databases and When to Use SQL vs. NoSQL.
Choosing between SQL and NoSQL depends on the application's needs:
SQL Databases: Structured, table-based databases like MySQL and PostgreSQL. Best for applications requiring complex queries and data integrity.
NoSQL Databases: Flexible, schema-less options like MongoDB and Cassandra. Ideal for handling large volumes of unstructured data.
Typical Questions:
What are the ACID properties in SQL databases?
When would you prefer MongoDB over a relational database?
8. How Do You Implement User Authentication?
User authentication is crucial for any secure application. Discuss:
Methods: Sessions, cookies, JSON Web Tokens (JWT).
Frameworks: Passport.js for Node.js, Auth0 for advanced solutions.
Best Practices: Storing passwords securely using hashing algorithms like bcrypt.
9. What Are Webpack and Babel Used For?
These tools are essential for modern JavaScript development:
Webpack: A module bundler for bundling JavaScript files and assets.
Babel: A JavaScript compiler that allows you to use next-gen JavaScript features by transpiling code to be compatible with older browsers.
Related Questions:
How do you optimize your build for production using Webpack?
What is tree shaking, and how does it improve performance?
10. How Do You Handle Error Handling in JavaScript?
Error handling is vital for ensuring that applications are resilient:
Try-Catch Blocks: For handling synchronous errors.
Promises and .catch(): For managing asynchronous operations.
Error Handling Middleware: Used in Express.js for centralized error management.
Important Concepts:
Logging errors and using tools like Sentry for real-time monitoring.
Creating user-friendly error messages.
Preparing thoroughly for full stack development interviews by understanding these questions will set you on the path to success. For a comprehensive walkthrough and additional insights, make sure to check out this YouTube guide, where these topics are discussed in detail to boost your interview readiness.
0 notes
Text
Top Full Stack Developer Interview Questions (2024)
In the dynamic landscape of technology, Full Stack Development has emerged as a crucial field, demanding professionals with a diverse skill set and a deep understanding of both frontend and backend technologies. As companies continue to embrace digital transformation, the demand for skilled Full Stack Developers remains high. This article delves into the top Full Stack Developer interview questions for 2024, focusing on the Java Full Stack roadmap and Python Full Stack syllabus.
Introduction to Full Stack Development
Full stack development refers to the practice of working on both the front end and back end of a web application or software. A full stack developer is someone who is proficient in working with both the client-side and server-side technologies, allowing them to handle all aspects of the development process.
In a typical full stack development scenario, the front end involves creating the user interface and user experience (UI/UX) of the application. This includes designing and developing components such as web pages, forms, buttons, navigation menus, and interactive elements using technologies like HTML, CSS, JavaScript, and front-end frameworks like React, Angular, or Vue.js.
On the other hand, the back end involves working with the server-side logic, databases, and server management. This includes tasks such as handling user authentication, processing data, managing server requests, and interacting with databases to store and retrieve information. Technologies commonly used in back-end development include programming languages like Node.js, Python, Java, or PHP, along with frameworks like Express.js, Django, Spring Boot, or Laravel.
Full stack developers are required to have a diverse skill set that encompasses both front-end and back-end technologies. They need to understand how to integrate these technologies seamlessly to build fully functional and responsive web applications. Additionally, they should be familiar with version control systems like Git, deployment processes, and have a good grasp of software development principles and best practices.
Overall, full stack development offers a holistic approach to building web applications, allowing developers to work on all layers of the software stack and deliver end-to-end solutions that meet user requirements effectively.
Top Interview Questions for Full Stack Developers
Technical Questions
Sure, here are some top interview questions for full-stack developers:
Frontend Development:
What are the key differences between HTML, CSS, and JavaScript?
Explain the box model in CSS and how it affects layout.
What is responsive web design, and how do you ensure your web applications are responsive?
How do you optimize website performance, both in terms of loading speed and user experience?
What are CSS preprocessors like Sass or Less, and why would you use them?
Backend Development:
What is the difference between server-side scripting and client-side scripting?
Explain the role of databases in web development and discuss different types of databases you are familiar with.
What is RESTful API, and how do you design and consume RESTful APIs?
How do you handle authentication and authorization in a web application?
Discuss the importance of caching in backend development and some popular caching strategies.
Full-Stack Development:
What is the MEAN (MongoDB, Express.js, AngularJS, Node.js) stack, and how does it differ from the MERN (MongoDB, Express.js, React, Node.js) stack?
Explain the concept of MVC (Model-View-Controller) architecture and how it's implemented in web development.
How do you handle state management in a single-page application (SPA)?
Discuss the advantages and disadvantages of monolithic vs microservices architecture for web applications.
What tools and technologies do you use for version control, continuous integration, and deployment?
Problem-Solving:
Given a scenario, how would you approach debugging a frontend/backend issue in a web application?
Describe a challenging project you worked on and how you overcame technical obstacles during its development.
Implement a simple algorithm (e.g., reverse a string, find the largest number in an array) using a programming language of your choice.
How do you ensure code quality and maintainability in a collaborative development environment?
Discuss your experience with testing methodologies such as unit testing, integration testing, and end-to-end testing.
Soft Skills and Communication:
How do you prioritize tasks and manage your time effectively when working on multiple projects or tasks simultaneously?
Describe a situation where you had to work in a team and resolve conflicts or disagreements effectively.
How do you stay updated with the latest trends and technologies in web development?
Explain a complex technical concept to a non-technical stakeholder or client.
Discuss a project where you had to quickly learn a new technology or framework and how you approached the learning process.
These questions cover a range of topics and skills that are important for full-stack developers, including technical knowledge, problem-solving abilities, communication skills, and project management experience. Adjust the complexity of the questions based on the seniority level of the position you are hiring for.
Behavioral Questions
Certainly, here are some behavioral questions tailored for full-stack developers:
Adaptability and Learning:
Describe a time when you had to quickly learn a new technology or programming language for a project. How did you approach the learning process, and what was the outcome?
Can you give an example of a challenging technical problem you faced and how you overcame it through self-directed learning or seeking help from others?
Problem-Solving and Decision Making:
Walk me through a complex issue you encountered during a project. How did you analyze the problem, identify possible solutions, and make a decision on the best course of action?
Describe a situation where you had to prioritize tasks or features in a project with tight deadlines. How did you decide what to focus on first, and what was the result?
Collaboration and Communication:
Discuss a project where you worked closely with a team of developers, designers, or other stakeholders. How did you ensure effective communication and collaboration among team members?
Can you share an example of a time when you had to present technical information or solutions to non-technical stakeholders? How did you ensure they understood the key points?
Handling Challenges and Failures:
Describe a project or task that didn't go as planned. What challenges did you face, and how did you handle the situation? What did you learn from the experience?
Have you ever made a mistake in your code that caused a significant issue? How did you identify and rectify the error, and what steps did you take to prevent similar mistakes in the future?
Leadership and Initiative:
Have you ever taken the lead on a project or initiative? What was your role, and how did you ensure the project's success?
Describe a time when you proposed an innovative solution or improvement to an existing process or technology. How was your idea received, and what was the outcome?
These behavioral questions focus on the candidate's ability to adapt, solve problems, collaborate effectively, learn from challenges, and take initiative. They provide insights into the candidate's past experiences and behaviors, which can help assess their fit for the role and the team dynamics.
Java Full Stack Developer Interview Questions
Sure, here are some Java Full Stack Developer interview questions:
What is the difference between JDK, JRE, and JVM?
Explain the concept of object-oriented programming and its key principles.
What is a servlet? How does it differ from an applet?
What is JDBC? How do you connect Java applications to databases using JDBC?
Can you explain the Spring framework and its core features?
What is RESTful web services? How do you implement RESTful APIs in Java?
Explain the MVC (Model-View-Controller) architecture and its advantages in web development.
What are some commonly used design patterns in Java? Provide examples.
How do you handle transactions in a Java application? Discuss the transaction management options.
What tools and technologies do you use for front-end development in a Java Full Stack environment?
Can you explain the concept of microservices architecture? How does it differ from monolithic architecture?
How do you ensure security in a Java web application? Discuss some best practices.
What is Docker, and how do you use it in Java application deployment?
Discuss the differences between SOAP and RESTful web services.
Can you explain the concept of dependency injection and how it is implemented in Spring?
These questions cover a range of topics typically encountered in Java Full Stack Developer interviews. Candidates should be able to demonstrate their understanding of Java programming, web development concepts, frameworks like Spring, and related technologies.
Python Full Stack Developer Interview Questions
Certainly! Here are some Python Full Stack Developer interview questions:
What is the difference between Python 2 and Python 3? Why should we use Python 3 for new projects?
Explain the concept of virtual environments in Python and why they are useful.
How does Flask differ from Django? When would you choose one over the other for a web development project?
What is ORM (Object-Relational Mapping)? Provide an example of an ORM library used in Python.
Describe the process of deploying a Flask or Django application to a production server.
What are decorators in Python? How can decorators be used in web development?
Explain the role of WSGI (Web Server Gateway Interface) in Python web applications.
What is RESTful API? How would you design and implement a RESTful API using Python?
Discuss the importance of testing in software development. What are some popular testing frameworks used in Python?
How do you handle database migrations in Django or Flask applications?
Describe the difference between synchronous and asynchronous programming. When would you choose asynchronous programming in Python?
What are some strategies for optimizing the performance of a Python web application?
Discuss the security considerations you would take into account when developing a web application in Python.
Have you worked with any cloud platforms for deploying Python applications? If so, which ones and what was your experience?
Can you explain the concept of caching in web development? How would you implement caching in a Python-based web application?
These questions cover a range of topics relevant to python full stack syllabus , including web frameworks, databases, testing, optimization, security, and deployment. Adjust the complexity of the questions based on the candidate's level of experience.
Tips for Acing Full Stack Developer Interviews
Review and practice coding exercises related to data structures, algorithms, and design patterns.
Showcase your portfolio projects and highlight your contributions and problem-solving skills.
Prepare for behavioral questions by reflecting on past experiences and achievements.
Stay updated with the latest trends and technologies in Full Stack Development.
Conclusion
In conclusion, Full Stack Developer interviews in 2024 require a comprehensive understanding of various technologies, from core programming languages to advanced frameworks and tools. By preparing diligently and showcasing your skills and experiences effectively, you can increase your chances of landing a rewarding Full Stack Developer role.
FAQs
What is the role of a Full Stack Developer? A Full Stack Developer is responsible for designing, developing, and maintaining web applications, handling both frontend and backend aspects of the software.Which programming languages are essential for Full Stack Development? Key languages include JavaScript, Python, Java, and frameworks such as React, Angular, Spring Boot, and Django.How can I improve my skills as a Full Stack Developer? Practice coding regularly, work on real-world projects, stay updated with industry trends, and participate in online communities and forums.What are some common challenges faced by Full Stack Developers? Challenges may include managing diverse technologies, ensuring scalability and performance, and staying updated with rapid technological advancements.What opportunities does Full Stack Development offer in the job market? Full Stack Developers are in high demand across industries, offering lucrative career prospects and opportunities for growth and innovation.
and innovation.
0 notes
Text
Head-to-Head: PHP vs. Java - Which Language Reigns Supreme?
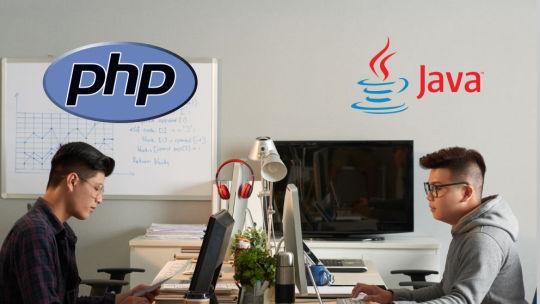
Head-to-Head: PHP vs. Java - Which Language Reigns Supreme? The debate between PHP and Java has long been a topic of discussion among developers, with proponents of each language advocating for its superiority in various aspects of web development, enterprise applications, and system architecture. In this head-to-head comparison, we'll delve into the strengths, weaknesses, and use cases of PHP and Java to determine which language reigns supreme in the world of software development.
Overview of PHP:
PHP, initially created as a server-side scripting language for web development, has gained widespread popularity for its simplicity, flexibility, and ease of use. Here are some key considerations regarding PHP:
Simplicity and Ease of Use:
PHP is renowned for its straightforward syntax and easy learning curve, making it accessible to beginners and experienced developers alike.
Its scripting nature allows developers to embed PHP code directly into HTML, enabling dynamic content generation and server-side processing.
Web Development Focus:
PHP is primarily designed for web development, with built-in features for processing form data, interacting with databases, and generating dynamic web pages.
It integrates seamlessly with popular web servers like Apache and Nginx and databases like MySQL, PostgreSQL, and SQLite.
Vibrant Ecosystem:
PHP boasts a vibrant ecosystem of frameworks, libraries, and tools that streamline web development tasks and accelerate project delivery.
Frameworks like Laravel, Symfony, and CodeIgniter provide robust MVC architecture, routing, ORM, and other features for building scalable and maintainable web applications.
Overview of Java:
Java, renowned for its platform independence, scalability, and robustness, is widely used for building enterprise-grade applications, backend systems, and large-scale distributed systems. Here are some key considerations regarding Java:
Write Once, Run Anywhere (WORA):
Java's WORA principle enables developers to write code once and run it on any platform that supports Java, including Windows, macOS, Linux, and various mobile devices.
This platform independence is achieved through the Java Virtual Machine (JVM), which provides a consistent runtime environment for Java applications.
Scalability and Performance:
Java offers scalability and performance advantages, making it suitable for building large-scale enterprise applications that can handle high volumes of concurrent users and transactions.
Its robust type system, memory management features, and multithreading support contribute to improved application performance and responsiveness.
Enterprise Integration:
Java's extensive ecosystem and enterprise-grade features make it well-suited for integrating with existing systems, middleware, and enterprise solutions.
Frameworks like Spring Boot, Jakarta EE (formerly Java EE), and Apache Camel provide comprehensive support for building enterprise applications, RESTful APIs, and microservices.
Head-to-Head Comparison:
Performance:
Java generally offers better performance and scalability compared to PHP, especially for large-scale enterprise applications and systems with high concurrency requirements.
PHP's performance has improved over the years, but it may still lag behind Java in terms of raw processing power and efficiency.
Developer Productivity:
PHP's simplicity and ease of use contribute to faster development cycles and rapid prototyping, making it suitable for small to medium-sized web projects.
Java's verbose syntax and boilerplate code may require more time and effort upfront but can lead to more maintainable and scalable codebases over the long term.
Ecosystem and Tooling:
PHP has a robust ecosystem of frameworks, libraries, and tools tailored for web development, with a focus on simplicity, flexibility, and ease of use.
Java's ecosystem is broader and more diverse, catering to a wide range of use cases, including web development, enterprise integration, mobile development, and big data processing.
Use Cases and Project Requirements:
The choice between PHP and Java ultimately depends on the specific requirements, scalability needs, and performance considerations of the project at hand.
PHP may be a better fit for small to medium-sized web projects, startups, and rapid prototyping, while Java shines in large-scale enterprise applications, middleware, and mission-critical systems.
Conclusion:
In conclusion, both PHP and Java have their strengths and weaknesses, making them suitable for different types of projects and development scenarios. While PHP excels in simplicity, ease of use, and rapid development, Java boasts scalability, performance, and enterprise-grade features. The choice between PHP and Java should be based on the specific requirements, project goals, and scalability needs of the application, ensuring that developers choose the language that best aligns with their project's objectives and long-term vision. Ultimately, the language that reigns supreme depends on the context of the project and the priorities of the development team.
#software engineering#Php Vs Java#application development#app development#mobile app development#programming
0 notes
Text
CHEAT SHEET TO
FULL STACK SOFTWARE DEVELOPMENT (IN 21 WEEKS)
Below is a more extended schedule to cover all the topics we've listed for approximately 2-3 months. This schedule assumes spending a few days on each major topic and sub-topic for a comprehensive understanding. Adjust the pace based on your comfort and learning progress:
Week 1-2: Introduction to Programming – Python
- Programming Structure and Basic Principles
- Programming Constructs - Loops, Functions, Arrays, etc.
Week 3: Git and Version Control
- Git Basics
- Collaborative Git Workflow
Week 4: HTML and CSS
- HTML Basics
- CSS Styling and Layout
Week 5: Object-Oriented Programming - Python
- Object-Oriented Paradigms
- Exception Handling, Collections
Week 6: Data Structures - Linear Data Structures
- Arrays, Strings, Stacks, Queues
- Linked Lists
Week 7: Data Structures - Binary Trees and Tree Traversals
- Binary Trees and Binary Search Trees
- Tree Traversal Algorithms
Week 8: Algorithms - Basic Algorithms and Analysis
- Recursion
- Searching and Sorting Algorithms
- Algorithm Analysis
Week 9: Algorithms - Advanced Algorithms and Evaluation
- Greedy Algorithms
- Graph Algorithms
- Dynamic Programming
- Hashing
Week 10: Database Design & Systems
- Data Models
- SQL Queries
- Database Normalization
- JDBC
Week 11-12: Server-Side Development & Frameworks
- Spring MVC Architecture
- Backend Development with Spring Boot
- ORM & Hibernate
- REST APIs
Week 13: Front End Development - HTML & CSS (Review)
- HTML & CSS Interaction
- Advanced CSS Techniques
Week 14-15: Front-End Development - JavaScript
- JavaScript Fundamentals
- DOM Manipulation
- JSON, AJAX, Event Handling
Week 16: JavaScript Frameworks - React
- Introduction to React
- React Router
- Building Components and SPAs
Week 17: Linux Essentials
- Introduction to Linux OS
- File Structure
- Basic Shell Scripting
Week 18: Cloud Foundations & Containers
- Cloud Service Models and Deployment Models
- Virtual Machines vs. Containers
- Introduction to Containers (Docker)
Week 19-20: AWS Core and Advanced Services
- AWS Organization & IAM
- Compute, Storage, Network
- Database Services (RDS, DynamoDB)
- PaaS - Elastic BeanStalk, CaaS - Elastic Container Service
- Monitoring & Logging - AWS CloudWatch, CloudTrail
- Notifications - SNS, SES, Billing & Account Management
Week 21: DevOps on AWS
- Continuous Integration and Continuous Deployment
- Deployment Pipeline (e.g., AWS CodePipeline, CodeCommit, CodeBuild, CodeDeploy)
- Infrastructure as Code (Terraform, CloudFormation)
Please adjust the schedule based on your individual learning pace and availability. Additionally, feel free to spend more time on topics that particularly interest you or align with your career goals. Practical projects and hands-on exercises will greatly enhance your understanding of these topics.
0 notes
Photo

Spring Boot vs. Spring MVC vs. Spring: How Do They Compare? ☞ http://on.geeklearn.net/3a6c6f1288 #SpringBoot #SpringMVC #Spring
#Spring Boot vs. Spring MVC vs. Spring#Spring Boot#Spring MVC#Spring#Spring Boot vs. Spring MVC#Spring Boot vs. Spring#Spring MVC vs. Spring
2 notes
·
View notes
Photo

Spring Boot vs. Spring MVC vs. Spring: How Do They Compare? ☞ http://on.geeklearn.net/3a6c6f1288 #SpringBoot #SpringMVC #Spring
#Spring Boot vs. Spring MVC vs. Spring#Spring Boot#Spring MVC#Spring#Spring Boot vs. Spring MVC#Spring Boot vs. Spring#Spring MVC vs. Spring
2 notes
·
View notes
Photo

Spring Boot vs. Spring MVC vs. Spring: How Do They Compare? ☞ http://on.geeklearn.net/3a6c6f1288 #SpringBoot #SpringMVC #Spring
#Spring Boot vs. Spring MVC vs. Spring#Spring Boot#Spring MVC#Spring#Spring Boot vs. Spring MVC#Spring Boot vs. Spring#Spring MVC vs. Spring
0 notes
Photo

Spring Boot vs. Spring MVC vs. Spring: How Do They Compare? ☞ http://on.geeklearn.net/3a6c6f1288 #SpringBoot #SpringMVC #Spring
#Spring Boot vs. Spring MVC vs. Spring#Spring Boot#Spring MVC#Spring#Spring Boot vs. Spring MVC#Spring Boot vs. Spring#Spring MVC vs. Spring
0 notes
Photo

Spring Boot vs. Spring MVC vs. Spring: How Do They Compare? ☞ http://on.geeklearn.net/3a6c6f1288 #SpringBoot #SpringMVC #Spring
#Spring Boot vs. Spring MVC vs. Spring#Spring Boot#Spring MVC#Spring#Spring Boot vs. Spring MVC#Spring Boot vs. Spring#Spring MVC vs. Spring
0 notes
Photo

Spring Boot vs. Spring MVC vs. Spring: How Do They Compare? ☞ http://on.geeklearn.net/3a6c6f1288 #SpringBoot #SpringMVC #Spring
#Spring Boot vs. Spring MVC vs. Spring#Spring Boot#Spring MVC#Spring#Spring Boot vs. Spring MVC#Spring Boot vs. Spring#Spring MVC vs. Spring
0 notes
Photo

Spring Boot vs. Spring MVC vs. Spring: How Do They Compare? ☞ http://on.geeklearn.net/3a6c6f1288 #SpringBoot #SpringMVC #Spring
#Spring Boot vs. Spring MVC vs. Spring#Spring Boot#Spring MVC#Spring#Spring Boot vs. Spring MVC#Spring Boot vs. Spring#Spring MVC vs. Spring
0 notes
Photo

Spring Boot vs. Spring MVC vs. Spring: How Do They Compare? ☞ http://on.geeklearn.net/3a6c6f1288 #SpringBoot #SpringMVC #Spring
#Spring Boot vs. Spring MVC vs. Spring#Spring Boot#Spring MVC#Spring#Spring Boot vs. Spring MVC#Spring Boot vs. Spring#Spring MVC vs. Spring
0 notes
Photo

Spring Boot vs. Spring MVC vs. Spring: How Do They Compare? ☞ http://on.geeklearn.net/3a6c6f1288 #SpringBoot #SpringMVC #Spring
#Spring Boot vs. Spring MVC vs. Spring#Spring Boot#Spring MVC#Spring#Spring Boot vs. Spring MVC#Spring Boot vs. Spring#Spring MVC vs. Spring
0 notes
Photo

Spring Boot vs. Spring MVC vs. Spring: How Do They Compare? ☞ http://on.geeklearn.net/3a6c6f1288 #SpringBoot #SpringMVC #Spring
#Spring Boot vs. Spring MVC vs. Spring#Spring Boot#Spring MVC#Spring#Spring Boot vs. Spring MVC#Spring Boot vs. Spring#Spring MVC vs. Spring
0 notes