#Symfony
Explore tagged Tumblr posts
Text
Symfony Clickjacking Prevention Guide
Clickjacking is a deceptive technique where attackers trick users into clicking on hidden elements, potentially leading to unauthorized actions. As a Symfony developer, it's crucial to implement measures to prevent such vulnerabilities.

🔍 Understanding Clickjacking
Clickjacking involves embedding a transparent iframe over a legitimate webpage, deceiving users into interacting with hidden content. This can lead to unauthorized actions, such as changing account settings or initiating transactions.
🛠️ Implementing X-Frame-Options in Symfony
The X-Frame-Options HTTP header is a primary defense against clickjacking. It controls whether a browser should be allowed to render a page in a <frame>, <iframe>, <embed>, or <object> tag.
Method 1: Using an Event Subscriber
Create an event subscriber to add the X-Frame-Options header to all responses:
// src/EventSubscriber/ClickjackingProtectionSubscriber.php namespace App\EventSubscriber; use Symfony\Component\EventDispatcher\EventSubscriberInterface; use Symfony\Component\HttpKernel\Event\ResponseEvent; use Symfony\Component\HttpKernel\KernelEvents; class ClickjackingProtectionSubscriber implements EventSubscriberInterface { public static function getSubscribedEvents() { return [ KernelEvents::RESPONSE => 'onKernelResponse', ]; } public function onKernelResponse(ResponseEvent $event) { $response = $event->getResponse(); $response->headers->set('X-Frame-Options', 'DENY'); } }
This approach ensures that all responses include the X-Frame-Options header, preventing the page from being embedded in frames or iframes.
Method 2: Using NelmioSecurityBundle
The NelmioSecurityBundle provides additional security features for Symfony applications, including clickjacking protection.
Install the bundle:
composer require nelmio/security-bundle
Configure the bundle in config/packages/nelmio_security.yaml:
nelmio_security: clickjacking: paths: '^/.*': DENY
This configuration adds the X-Frame-Options: DENY header to all responses, preventing the site from being embedded in frames or iframes.
🧪 Testing Your Application
To ensure your application is protected against clickjacking, use our Website Vulnerability Scanner. This tool scans your website for common vulnerabilities, including missing or misconfigured X-Frame-Options headers.

Screenshot of the free tools webpage where you can access security assessment tools.
After scanning for a Website Security check, you'll receive a detailed report highlighting any security issues:

An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities.
🔒 Enhancing Security with Content Security Policy (CSP)
While X-Frame-Options is effective, modern browsers support the more flexible Content-Security-Policy (CSP) header, which provides granular control over framing.
Add the following header to your responses:
$response->headers->set('Content-Security-Policy', "frame-ancestors 'none';");
This directive prevents any domain from embedding your content, offering robust protection against clickjacking.
🧰 Additional Security Measures
CSRF Protection: Ensure that all forms include CSRF tokens to prevent cross-site request forgery attacks.
Regular Updates: Keep Symfony and all dependencies up to date to patch known vulnerabilities.
Security Audits: Conduct regular security audits to identify and address potential vulnerabilities.
📢 Explore More on Our Blog
For more insights into securing your Symfony applications, visit our Pentest Testing Blog. We cover a range of topics, including:
Preventing clickjacking in Laravel
Securing API endpoints
Mitigating SQL injection attacks
🛡️ Our Web Application Penetration Testing Services
Looking for a comprehensive security assessment? Our Web Application Penetration Testing Services offer:
Manual Testing: In-depth analysis by security experts.
Affordable Pricing: Services starting at $25/hr.
Detailed Reports: Actionable insights with remediation steps.
Contact us today for a free consultation and enhance your application's security posture.
3 notes
·
View notes
Text
In this blog, we will explore the top 5 Symfony providers that can help you with everything from system migration to keeping your application secure and running at its best.
2 notes
·
View notes
Text
When deciding between Laravel and Symfony, it's essential to consider your project needs. Laravel shines with its user-friendly syntax and extensive out-of-the-box functionalities, making it ideal for rapid development and handling common web application tasks with ease. Its vibrant community and ecosystem offer a wide range of packages, which can be a huge time-saver for developers looking to implement complex features quickly.
On the other hand, Symfony is known for its robustness, flexibility, and scalability, making it a preferred choice for large, enterprise-level applications. With a component-based architecture, Symfony allows developers to pick and choose components, making it highly customizable. If you value performance and long-term support, Symfony might be the better choice, while Laravel is perfect for projects needing fast deployment and intuitive development.
2 notes
·
View notes
Text
How To Become A Full Stack Php Developer
A career in Full-Stack Development is one of the most versatile and in-demand paths in tech. Full-stack developers can build both the frontend (user interface) and backend (server-side) of web applications, making them valuable assets to any development team.
✅ Why Choose Full-Stack Development?
High Demand – Companies seek developers who can handle both ends of a project.
Versatility – Work on a wide range of projects and technologies.
Good Pay – Full-stack roles often come with higher salary potential.
Freelance & Startup Friendly – Ideal for solo projects or joining small teams.
A PHP Developer builds and maintains the backend of websites and web applications using PHP, one of the most widely used server-side scripting languages. PHP is behind popular platforms like WordPress, Laravel, and many custom web systems.
📞 Phone Number: +91 9511803947 📧 Email Address: [email protected]
#PHPDeveloper#PHPCoding#WebDevelopment#BackendDeveloper#Laravel#Symfony#CodeIgniter#Programming#TechCareer#DeveloperLife#FullStackDeveloper#WebDev#SoftwareEngineer#LearnPHP
0 notes
Text
EldenFreight shipping rate API in Symfony
youtube
0 notes
Text
1 note
·
View note
Text
🌟 Exploring the Top PHP Frameworks in 2024
From Laravel’s robust ecosystem to Symfony’s enterprise-level capabilities, PHP frameworks are shaping the digital world. Dive into the strengths, challenges, and future trends these tools must embrace by 2025.
Read the full post here: https://gegosoft.com/top-php-frameworks-in-2024/
0 notes
Text
Symfony Development
Symfony, a comprehensive web application framework, is a valuable asset in PHP development, streamlining the creation and maintenance of web applications while minimizing repetitive coding tasks. Symfony furnishes developers with a framework, tools, and components that accelerate the development of web applications. Opting for this framework enables you to launch your applications earlier and without hassle.

0 notes
Text
Weak SSL/TLS Configuration in Symfony — Risks & Fixes
Introduction
Secure communication is critical in today’s web applications, especially when handling sensitive user data. Symfony, one of the most popular PHP frameworks, relies heavily on SSL/TLS protocols to secure HTTP connections. However, weak SSL/TLS configurations can expose your application to man-in-the-middle (MITM) attacks, data breaches, and regulatory penalties.
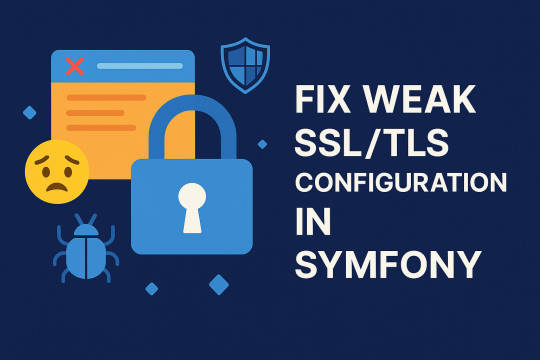
In this blog, we’ll explore what weak SSL/TLS configuration means in Symfony applications, why it’s dangerous, and how you can fix it with practical coding examples. Plus, you’ll get a peek at how our Website Vulnerability Scanner online free, helps identify such vulnerabilities automatically.
What is Weak SSL/TLS Configuration?
SSL (Secure Sockets Layer) and TLS (Transport Layer Security) are cryptographic protocols designed to secure communication over the internet. A weak SSL/TLS configuration involves:
Using deprecated protocols like SSL 2.0, SSL 3.0, or early TLS versions (TLS 1.0/1.1).
Employing weak cipher suites (e.g., RC4, DES).
Missing forward secrecy (PFS).
Poor certificate validation or expired certificates.
These weaknesses can be exploited to intercept or alter data during transmission.
Common SSL/TLS Weaknesses in Symfony
Symfony itself relies on the underlying web server (Apache, Nginx) or PHP cURL/OpenSSL for TLS. Misconfigurations can happen at various layers:
Web server allows weak protocols/ciphers.
PHP cURL requests do not enforce strict SSL verification.
Symfony HTTP clients or bundles not configured for secure TLS options.
Detecting Weak SSL/TLS Configurations with Our Free Tool
You can scan your website for SSL/TLS issues quickly at https://free.pentesttesting.com/.
Screenshot of the Website Vulnerability Scanner tool webpage

Screenshot of the free tools webpage where you can access security assessment tools.
Our tool will generate a detailed vulnerability report, highlighting SSL/TLS weaknesses among other issues.
Assessment report screenshot to check Website Vulnerability

An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities.
How to Fix Weak SSL/TLS Configuration in Symfony
1. Configure Your Web Server Correctly
Ensure your Apache or Nginx server uses strong protocols and ciphers. For example, in Nginx:
ssl_protocols TLSv1.2 TLSv1.3; ssl_ciphers 'ECDHE-ECDSA-AES256-GCM-SHA384:ECDHE-RSA-AES256-GCM-SHA384'; ssl_prefer_server_ciphers on; ssl_session_cache shared:SSL:10m; ssl_session_tickets off;
This disables weak protocols and uses strong cipher suites with Perfect Forward Secrecy.
2. Enforce SSL Verification in PHP cURL Requests
When Symfony makes external HTTP calls via PHP cURL, enforce strict SSL checks.
$ch = curl_init('https://api.example.com/secure-endpoint'); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, true); // Verify SSL certificate curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 2); // Verify host name matches cert $response = curl_exec($ch); if(curl_errno($ch)) { throw new \Exception('SSL Error: ' . curl_error($ch)); } curl_close($ch);
3. Use Symfony HTTP Client with Secure Defaults
Since Symfony 4.3+, the HTTP Client component uses secure defaults, but always ensure SSL verification is enabled:
use Symfony\Component\HttpClient\HttpClient; $client = HttpClient::create([ 'verify_peer' => true, 'verify_host' => true, ]); $response = $client->request('GET', 'https://secure-api.example.com/data'); $statusCode = $response->getStatusCode(); $content = $response->getContent();
4. Regularly Update Your Certificates and Libraries
Expired or self-signed certificates can break trust chains. Use trusted CAs and update OpenSSL and PHP regularly.
Bonus: Automate SSL/TLS Testing in Your Symfony CI/CD Pipeline
You can add an automated SSL check using tools like testssl.sh or integrate vulnerability scanning APIs such as our free tool’s API (check details at https://free.pentesttesting.com/).
About Pentest Testing Corp.
For comprehensive security audits, including advanced web app penetration testing, check out our service at Web App Penetration Testing Services.
Also, don’t miss our cybersecurity insights and updates on our blog.
Subscribe to our newsletter for the latest tips: Subscribe on LinkedIn.
Conclusion
Weak SSL/TLS configurations put your Symfony apps and users at significant risk. By following secure web server settings, enforcing SSL verification in PHP/Symfony, and leveraging automated scanning tools for a Website Security test, you can greatly improve your application’s security posture.
Stay safe, keep scanning, and secure your Symfony apps today!
If you found this blog helpful, please share and follow our blog at Pentest Testing Corp.
1 note
·
View note
Text
In this blog, you'll learn about PHP's benefits for website development, including how it enhances performance, functionality, and user engagement. It covers dynamic website creation, responsive design with PHP, and the advantages of using PHP for fast development, cross-platform compatibility, and strong community support.
1 note
·
View note
Text
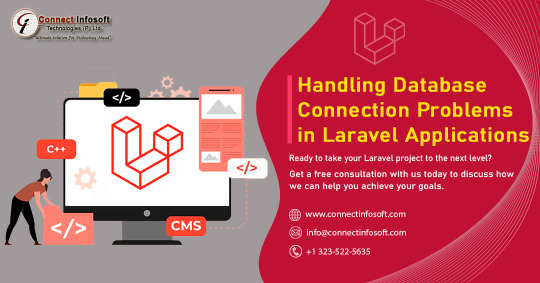
Handling Database Connection Problems in Laravel Applications
Ready to take your Laravel project to the next level? Get in touch with us today to discuss how we can help you achieve your goals.
#connectinfosoft#laraveldevelopment#laraveldevelopmentservice#laraveldeveloper#laraveldevelopmentcompany#laraveldevelopmentteam#laravelexperts#phpframework#phpdevelopment#customlaravel#webdevelopment#laravelprogramming#codeigniter#symfony#laravelapps#phpdevelopmentservices#laravelcommunity#laravelcode#fullstackdevelopment#backenddevelopment#laravelprojects#appdevelopment#softwaredevelopment#webdevelopmentcompany#webdesigningcompany#usa#india#canada#unitedstates#bulgaria
1 note
·
View note
Text
full stack developer in PHP Coding Bit IT Solution
A full stack developer in PHP is someone who is skilled in both the frontend and backend aspects of web development, with PHP serving as the core backend language. On the frontend, they use HTML, CSS, and JavaScript to create responsive, interactive user interfaces. Familiarity with tools like Bootstrap for design and JavaScript libraries such as jQuery or frameworks like Vue.js or React can enhance the user experience and development speed. On the backend, a PHP full stack developer must understand the core PHP language, including its syntax, functions, and object-oriented programming principles. To build more structured and scalable applications, they often use PHP frameworks like Laravel, Symphony, or CodeIgniter.
Frontend (Client-Side)
These are the technologies that control what users see and interact with:
HTML – Structure of web pages.
CSS – Styling and layout.
JavaScript – Interactivity and dynamic behavior.
Frameworks/Libraries: jQuery, Vue.js, React (optional but valuable)
Bootstrap – Popular CSS framework for responsive design. 📞 Phone Number: +91 9511803947 📧 Email Address: [email protected]
#FullStackDeveloper#WebDevelopment#CodingLife#DevLife#Programmer#TechStack#PHP#Laravel#Symfony#CodeIgniter#BackendDevelopment#PHPLaravel#PHPCoding
0 notes