#convert sql server to mysql
Explore tagged Tumblr posts
Text
Java Database Connectivity API contains commonly asked Java interview questions. A good understanding of JDBC API is required to understand and leverage many powerful features of Java technology. Here are few important practical questions and answers which can be asked in a Core Java JDBC interview. Most of the java developers are required to use JDBC API in some type of application. Though its really common, not many people understand the real depth of this powerful java API. Dozens of relational databases are seamlessly connected using java due to the simplicity of this API. To name a few Oracle, MySQL, Postgres and MS SQL are some popular ones. This article is going to cover a lot of general questions and some of the really in-depth ones to. Java Interview Preparation Tips Part 0: Things You Must Know For a Java Interview Part 1: Core Java Interview Questions Part 2: JDBC Interview Questions Part 3: Collections Framework Interview Questions Part 4: Threading Interview Questions Part 5: Serialization Interview Questions Part 6: Classpath Related Questions Part 7: Java Architect Scalability Questions What are available drivers in JDBC? JDBC technology drivers fit into one of four categories: A JDBC-ODBC bridge provides JDBC API access via one or more ODBC drivers. Note that some ODBC native code and in many cases native database client code must be loaded on each client machine that uses this type of driver. Hence, this kind of driver is generally most appropriate when automatic installation and downloading of a Java technology application is not important. A native-API partly Java technology-enabled driver converts JDBC calls into calls on the client API for Oracle, Sybase, Informix, DB2, or other DBMS. Note that, like the bridge driver, this style of driver requires that some binary code be loaded on each client machine. A net-protocol fully Java technology-enabled driver translates JDBC API calls into a DBMS-independent net protocol which is then translated to a DBMS protocol by a server. This net server middleware is able to connect all of its Java technology-based clients to many different databases. The specific protocol used depends on the vendor. In general, this is the most flexible JDBC API alternative. It is likely that all vendors of this solution will provide products suitable for Intranet use. In order for these products to also support Internet access they must handle the additional requirements for security, access through firewalls, etc., that the Web imposes. Several vendors are adding JDBC technology-based drivers to their existing database middleware products. A native-protocol fully Java technology-enabled driver converts JDBC technology calls into the network protocol used by DBMSs directly. This allows a direct call from the client machine to the DBMS server and is a practical solution for Intranet access. Since many of these protocols are proprietary the database vendors themselves will be the primary source for this style of driver. Several database vendors have these in progress. What are the types of statements in JDBC? the JDBC API has 3 Interfaces, (1. Statement, 2. PreparedStatement, 3. CallableStatement ). The key features of these are as follows: Statement This interface is used for executing a static SQL statement and returning the results it produces. The object of Statement class can be created using Connection.createStatement() method. PreparedStatement A SQL statement is pre-compiled and stored in a PreparedStatement object. This object can then be used to efficiently execute this statement multiple times. The object of PreparedStatement class can be created using Connection.prepareStatement() method. This extends Statement interface. CallableStatement This interface is used to execute SQL stored procedures. This extends PreparedStatement interface. The object of CallableStatement class can be created using Connection.prepareCall() method.
What is a stored procedure? How to call stored procedure using JDBC API? Stored procedure is a group of SQL statements that forms a logical unit and performs a particular task. Stored Procedures are used to encapsulate a set of operations or queries to execute on database. Stored procedures can be compiled and executed with different parameters and results and may have any combination of input/output parameters. Stored procedures can be called using CallableStatement class in JDBC API. Below code snippet shows how this can be achieved. CallableStatement cs = con.prepareCall("call MY_STORED_PROC_NAME"); ResultSet rs = cs.executeQuery(); What is Connection pooling? What are the advantages of using a connection pool? Connection Pooling is a technique used for sharing the server resources among requested clients. It was pioneered by database vendors to allow multiple clients to share a cached set of connection objects that provides access to a database. Getting connection and disconnecting are costly operation, which affects the application performance, so we should avoid creating multiple connection during multiple database interactions. A pool contains set of Database connections which are already connected, and any client who wants to use it can take it from pool and when done with using it can be returned back to the pool. Apart from performance this also saves you resources as there may be limited database connections available for your application. How to do database connection using JDBC thin driver ? This is one of the most commonly asked questions from JDBC fundamentals, and knowing all the steps of JDBC connection is important. import java.sql.*; class JDBCTest public static void main (String args []) throws Exception //Load driver class Class.forName ("oracle.jdbc.driver.OracleDriver"); //Create connection Connection conn = DriverManager.getConnection ("jdbc:oracle:thin:@hostname:1526:testdb", "scott", "tiger"); // @machineName:port:SID, userid, password Statement stmt = conn.createStatement(); ResultSet rs = stmt.executeQuery("select 'Hi' from dual"); while (rs.next()) System.out.println (rs.getString(1)); // Print col 1 => Hi stmt.close(); What does Class.forName() method do? Method forName() is a static method of java.lang.Class. This can be used to dynamically load a class at run-time. Class.forName() loads the class if its not already loaded. It also executes the static block of loaded class. Then this method returns an instance of the loaded class. So a call to Class.forName('MyClass') is going to do following - Load the class MyClass. - Execute any static block code of MyClass. - Return an instance of MyClass. JDBC Driver loading using Class.forName is a good example of best use of this method. The driver loading is done like this Class.forName("org.mysql.Driver"); All JDBC Drivers have a static block that registers itself with DriverManager and DriverManager has static initializer method registerDriver() which can be called in a static blocks of Driver class. A MySQL JDBC Driver has a static initializer which looks like this: static try java.sql.DriverManager.registerDriver(new Driver()); catch (SQLException E) throw new RuntimeException("Can't register driver!"); Class.forName() loads driver class and executes the static block and the Driver registers itself with the DriverManager. Which one will you use Statement or PreparedStatement? Or Which one to use when (Statement/PreparedStatement)? Compare PreparedStatement vs Statement. By Java API definitions: Statement is a object used for executing a static SQL statement and returning the results it produces. PreparedStatement is a SQL statement which is precompiled and stored in a PreparedStatement object. This object can then be used to efficiently execute this statement multiple times. There are few advantages of using PreparedStatements over Statements
Since its pre-compiled, Executing the same query multiple times in loop, binding different parameter values each time is faster. (What does pre-compiled statement means? The prepared statement(pre-compiled) concept is not specific to Java, it is a database concept. Statement precompiling means: when you execute a SQL query, database server will prepare a execution plan before executing the actual query, this execution plan will be cached at database server for further execution.) In PreparedStatement the setDate()/setString() methods can be used to escape dates and strings properly, in a database-independent way. SQL injection attacks on a system are virtually impossible when using PreparedStatements. What does setAutoCommit(false) do? A JDBC connection is created in auto-commit mode by default. This means that each individual SQL statement is treated as a transaction and will be automatically committed as soon as it is executed. If you require two or more statements to be grouped into a transaction then you need to disable auto-commit mode using below command con.setAutoCommit(false); Once auto-commit mode is disabled, no SQL statements will be committed until you explicitly call the commit method. A Simple transaction with use of autocommit flag is demonstrated below. con.setAutoCommit(false); PreparedStatement updateStmt = con.prepareStatement( "UPDATE EMPLOYEE SET SALARY = ? WHERE EMP_NAME LIKE ?"); updateStmt.setInt(1, 5000); updateSales.setString(2, "Jack"); updateStmt.executeUpdate(); updateStmt.setInt(1, 6000); updateSales.setString(2, "Tom"); updateStmt.executeUpdate(); con.commit(); con.setAutoCommit(true); What are database warnings and How can I handle database warnings in JDBC? Warnings are issued by database to notify user of a problem which may not be very severe. Database warnings do not stop the execution of SQL statements. In JDBC SQLWarning is an exception that provides information on database access warnings. Warnings are silently chained to the object whose method caused it to be reported. Warnings may be retrieved from Connection, Statement, and ResultSet objects. Handling SQLWarning from connection object //Retrieving warning from connection object SQLWarning warning = conn.getWarnings(); //Retrieving next warning from warning object itself SQLWarning nextWarning = warning.getNextWarning(); //Clear all warnings reported for this Connection object. conn.clearWarnings(); Handling SQLWarning from Statement object //Retrieving warning from statement object stmt.getWarnings(); //Retrieving next warning from warning object itself SQLWarning nextWarning = warning.getNextWarning(); //Clear all warnings reported for this Statement object. stmt.clearWarnings(); Handling SQLWarning from ResultSet object //Retrieving warning from resultset object rs.getWarnings(); //Retrieving next warning from warning object itself SQLWarning nextWarning = warning.getNextWarning(); //Clear all warnings reported for this resultset object. rs.clearWarnings(); The call to getWarnings() method in any of above way retrieves the first warning reported by calls on this object. If there is more than one warning, subsequent warnings will be chained to the first one and can be retrieved by calling the method SQLWarning.getNextWarning on the warning that was retrieved previously. A call to clearWarnings() method clears all warnings reported for this object. After a call to this method, the method getWarnings returns null until a new warning is reported for this object. Trying to call getWarning() on a connection after it has been closed will cause an SQLException to be thrown. Similarly, trying to retrieve a warning on a statement after it has been closed or on a result set after it has been closed will cause an SQLException to be thrown. Note that closing a statement also closes a result set that it might have produced. What is Metadata and why should I use it?
JDBC API has 2 Metadata interfaces DatabaseMetaData & ResultSetMetaData. The DatabaseMetaData provides Comprehensive information about the database as a whole. This interface is implemented by driver vendors to let users know the capabilities of a Database Management System (DBMS) in combination with the driver based on JDBC technology ("JDBC driver") that is used with it. Below is a sample code which demonstrates how we can use the DatabaseMetaData DatabaseMetaData md = conn.getMetaData(); System.out.println("Database Name: " + md.getDatabaseProductName()); System.out.println("Database Version: " + md.getDatabaseProductVersion()); System.out.println("Driver Name: " + md.getDriverName()); System.out.println("Driver Version: " + md.getDriverVersion()); The ResultSetMetaData is an object that can be used to get information about the types and properties of the columns in a ResultSet object. Use DatabaseMetaData to find information about your database, such as its capabilities and structure. Use ResultSetMetaData to find information about the results of an SQL query, such as size and types of columns. Below a sample code which demonstrates how we can use the ResultSetMetaData ResultSet rs = stmt.executeQuery("SELECT a, b, c FROM TABLE2"); ResultSetMetaData rsmd = rs.getMetaData(); int numberOfColumns = rsmd.getColumnCount(); boolean b = rsmd.isSearchable(1); What is RowSet? or What is the difference between RowSet and ResultSet? or Why do we need RowSet? or What are the advantages of using RowSet over ResultSet? RowSet is a interface that adds support to the JDBC API for the JavaBeans component model. A rowset, which can be used as a JavaBeans component in a visual Bean development environment, can be created and configured at design time and executed at run time. The RowSet interface provides a set of JavaBeans properties that allow a RowSet instance to be configured to connect to a JDBC data source and read some data from the data source. A group of setter methods (setInt, setBytes, setString, and so on) provide a way to pass input parameters to a rowset's command property. This command is the SQL query the rowset uses when it gets its data from a relational database, which is generally the case. Rowsets are easy to use since the RowSet interface extends the standard java.sql.ResultSet interface so it has all the methods of ResultSet. There are two clear advantages of using RowSet over ResultSet RowSet makes it possible to use the ResultSet object as a JavaBeans component. As a consequence, a result set can, for example, be a component in a Swing application. RowSet be used to make a ResultSet object scrollable and updatable. All RowSet objects are by default scrollable and updatable. If the driver and database being used do not support scrolling and/or updating of result sets, an application can populate a RowSet object implementation (e.g. JdbcRowSet) with the data of a ResultSet object and then operate on the RowSet object as if it were the ResultSet object. What is a connected RowSet? or What is the difference between connected RowSet and disconnected RowSet? or Connected vs Disconnected RowSet, which one should I use and when? Connected RowSet A RowSet object may make a connection with a data source and maintain that connection throughout its life cycle, in which case it is called a connected rowset. A rowset may also make a connection with a data source, get data from it, and then close the connection. Such a rowset is called a disconnected rowset. A disconnected rowset may make changes to its data while it is disconnected and then send the changes back to the original source of the data, but it must reestablish a connection to do so. Example of Connected RowSet: A JdbcRowSet object is a example of connected RowSet, which means it continually maintains its connection to a database using a JDBC technology-enabled driver. Disconnected RowSet A disconnected rowset may have a reader (a RowSetReader object) and a writer (a RowSetWriter object) associated with it.
The reader may be implemented in many different ways to populate a rowset with data, including getting data from a non-relational data source. The writer can also be implemented in many different ways to propagate changes made to the rowset's data back to the underlying data source. Example of Disconnected RowSet: A CachedRowSet object is a example of disconnected rowset, which means that it makes use of a connection to its data source only briefly. It connects to its data source while it is reading data to populate itself with rows and again while it is propagating changes back to its underlying data source. The rest of the time, a CachedRowSet object is disconnected, including while its data is being modified. Being disconnected makes a RowSet object much leaner and therefore much easier to pass to another component. For example, a disconnected RowSet object can be serialized and passed over the wire to a thin client such as a personal digital assistant (PDA). What is the benefit of having JdbcRowSet implementation? Why do we need a JdbcRowSet like wrapper around ResultSet? The JdbcRowSet implementation is a wrapper around a ResultSet object that has following advantages over ResultSet This implementation makes it possible to use the ResultSet object as a JavaBeans component. A JdbcRowSet can be used as a JavaBeans component in a visual Bean development environment, can be created and configured at design time and executed at run time. It can be used to make a ResultSet object scrollable and updatable. All RowSet objects are by default scrollable and updatable. If the driver and database being used do not support scrolling and/or updating of result sets, an application can populate a JdbcRowSet object with the data of a ResultSet object and then operate on the JdbcRowSet object as if it were the ResultSet object. Can you think of a questions which is not part of this post? Please don't forget to share it with me in comments section & I will try to include it in the list.
0 notes
Text
The Power of Amazon Web Services (AWS): A Detailed Guide for 2025
Amazon Web Services (AWS) is the leading cloud computing platform, providing a wide range of services that empower businesses to grow, innovate, and optimize operations efficiently. With an increasing demand for cloud-based solutions, AWS has become the backbone of modern enterprises, offering high-performance computing, storage, networking, and security solutions. Whether you are an IT professional, a business owner, or an aspiring cloud architect, understanding AWS can give you a competitive edge in the technology landscape.
In this blog we will guide and explore AWS fundamentals, key services, benefits, use cases, and future trends, helping you navigate the AWS ecosystem with confidence.
What is AWS?
Amazon Web Services (AWS) is a secure cloud computing platform that provides on-demand computing resources, storage, databases, machine learning, and networking solutions. AWS eliminates the need for physical infrastructure, enabling businesses to run applications and services seamlessly in a cost-effective manner.
With over 200 fully featured services, AWS powers startups, enterprises, and government organizations worldwide. Its flexibility, scalability, and pay-as-you-go pricing model make it a preferred choice for cloud adoption.
Key AWS Services You Must Know
AWS offers a vast range of services, categorized into various domains. Below are some essential AWS services that are widely used:
1. Compute Services
Amazon EC2 (Elastic Compute Cloud): Provides resizable virtual servers for running applications.
AWS Lambda: Enables serverless computing, allowing you to run code without provisioning or managing servers.
Amazon Lightsail: A simple virtual private server (VPS) for small applications and websites.
AWS Fargate: A serverless compute engine for containerized applications.
2. Storage Services
Amazon S3 (Simple Storage Service): Object storage solution for scalable data storage.
Amazon EBS (Elastic Block Store): Persistent block storage for EC2 instances.
Amazon Glacier: Low-cost archival storage for long-term data backup.
3. Database Services
Amazon RDS (Relational Database Service): Fully managed relational databases like MySQL, PostgreSQL, and SQL Server.
Amazon DynamoDB: NoSQL database for key-value and document storage.
Amazon Redshift: Data warehousing service for big data analytics.
4. Networking and Content Delivery
Amazon VPC (Virtual Private Cloud): Provides a secure and isolated network in AWS.
Amazon Route 53: Scalable domain name system (DNS) service.
AWS CloudFront: Content delivery network (CDN) for fast and secure content delivery.
5. Security and Identity Management
AWS IAM (Identity and Access Management): Provides secure access control to AWS resources.
AWS Shield: DDoS protection for applications.
AWS WAF (Web Application Firewall): Protects applications from web threats.
6. Machine Learning & AI
Amazon SageMaker: Builds, trains, and deploys machine learning models.
Amazon Rekognition: Image and video analysis using AI.
Amazon Polly: Converts text into speech using deep learning.
Benefits of Using AWS
1. Scalability and Flexibility
AWS enables businesses to scale their infrastructure dynamically, ensuring seamless performance even during peak demand periods.
2. Cost-Effectiveness
With AWS's pay-as-you-go pricing, businesses only pay for the resources they use, reducing unnecessary expenses.
3. High Availability and Reliability
AWS operates in multiple regions and availability zones, ensuring minimal downtime and high data redundancy.
4. Enhanced Security
AWS offers advanced security features, including encryption, identity management, and compliance tools, ensuring data protection.
5. Fast Deployment
With AWS, businesses can deploy applications quickly, reducing time-to-market and accelerating innovation.
Popular Use Cases of AWS
1. Web Hosting
AWS is widely used for hosting websites and applications with services like EC2, S3, and CloudFront.
2. Big Data Analytics
Enterprises leverage AWS services like Redshift and AWS Glue for data warehousing and ETL processes.
3. DevOps and CI/CD
AWS supports DevOps practices with services like AWS CodePipeline, CodeBuild, and CodeDeploy.
4. Machine Learning and AI
Organizations use AWS AI services like SageMaker for building intelligent applications.
5. IoT Applications
AWS IoT Core enables businesses to connect and manage IoT devices securely.
Future Trends in AWS and Cloud Computing
1. Serverless Computing Expansion
More businesses are adopting AWS Lambda and Fargate for running applications without managing servers.
2. Multi-Cloud and Hybrid Cloud Adoption
AWS Outposts and AWS Hybrid Cloud solutions are bridging the gap between on-premise and cloud environments.
3. AI and Machine Learning Growth
AWS continues to enhance AI capabilities, driving innovation in automation and data processing.
4. Edge Computing Development
AWS Wavelength and AWS Local Zones will expand the reach of cloud computing to edge devices.
Conclusion
Amazon Web Services (AWS) is transforming how businesses operate in the digital era, providing unmatched scalability, security, and performance. Whether you are an enterprise looking to migrate to the cloud, a developer building applications, or a data scientist leveraging AI, AWS has a solution to your needs.
By mastering AWS, you can explore new career opportunities and drive business innovation. Start your AWS journey today and explore the limitless possibilities of cloud computing.
0 notes
Text
Simplify Your Database Queries with Ai2sql: AI-Driven SQL Query Generator
Ai2sql is an AI-powered tool that makes it easy for developers, data analysts, and business users to create complex SQL queries by simply describing what they need in plain language. The platform converts natural language inputs into optimized SQL code, making data analysis accessible even to those without a deep understanding of SQL syntax. Whether you're managing data for a business or building applications, Ai2sql can save you time and effort in writing SQL queries.
Core Functionality: Ai2sql converts plain language requests into SQL queries, making it easy to interact with databases without needing extensive SQL knowledge. Users can describe their data needs, and Ai2sql will generate the corresponding SQL code.
Key Features:
Natural Language to SQL Conversion: Turn natural language prompts into SQL queries, eliminating the need for manual coding.
Query Optimization: Generate SQL code that is optimized for better performance, saving time and ensuring efficiency.
Cross-Database Compatibility: Works with major databases like MySQL, PostgreSQL, and SQL Server, making it versatile for different projects.
Customizable Outputs: Customize the generated queries to suit specific requirements, giving users more control over the final output.
SQL Learning Support: Learn SQL by reviewing the generated code, helping users understand the structure and syntax of SQL queries.
Benefits:
No SQL Expertise Required: Users can generate complex queries without needing to learn SQL, making data analysis accessible to a wider audience.
Time Efficiency: Save hours of manual coding by generating SQL queries instantly with the help of AI.
Versatile Use Cases: Suitable for developers, business analysts, and anyone who needs to interact with databases, regardless of their SQL expertise.
Ready to simplify your database queries?
Visit aiwikiweb.com/product/ai2sql/
#SQL#Ai2sql#DatabaseManagement#NaturalLanguageProcessing#DataAnalysis#SQLAutomation#AIinData#NoCodeSQL#BusinessIntelligence#DataTools
0 notes
Text
Google Database Migration Service Specific MySQL Databases

MySQL database instance
Use Google database migration service to migrate particular MySQL databases. You may wish to migrate all or a portion of the data from your source instance when moving to Cloud SQL, their fully managed relational database service for MySQL, PostgreSQL, and SQL Server.
All tables from all databases and schemas can be easily moved with the help of Google Database Migration Service (DMS). It can be challenging to understand how to use the tool to migrate only specific databases or tables, though.
MySQL instance
Help go over how to migrate a selection of databases and tables from many possible sources in this blog, such as on-premises MySQL, Cloud SQL for MySQL, and Amazon RDS for MySQL. The source MySQL instance of each of the MySQL instances in this example has numerous databases configured. You must have enabled binary logs on your source instance in order to proceed with this step. They will demonstrate how to move each of the following:
On-premises MySQL, includes instances with or without GTID enabled.
MySQL on Cloud SQL with GTID enabled.
MySQL on AWS RDS with GTID enabled.
An instance of Cloud SQL for MySQL will be the goal. Let’s examine each kind of migration in more detail.
Cloud database migration service
Make cloud migrations easier.
Currently available for Oracle, SQL Server, PostgreSQL and MySQL databases.
Switch from on-premises, Google Cloud, or other clouds to open source databases in the cloud.Continuously replicate data to reduce downtime during migrations.
Convert your schema and code seamlessly with the help of AI.
Serverless and simple to configure.
Google Database Migration Service Advantages
Migration made simpler
With an integrated, Gemini-assisted conversion and migration experience, you can begin migrating in a matter of clicks. Less complicated migrations allow you to take use of the cloud’s advantages sooner.
Very little downtime
Savour a completely managed service that migrates your database with the least amount of downtime possible. Serverless migrations can take an initial snapshot and then replicate data continuously, and they are quite performant at scale.
Accelerated access to AlloyDB and Cloud SQL
Gain access to the enterprise availability, stability, and security of AlloyDB for PostgreSQL and the open, standards-based Cloud SQL services.
Important characteristics
Simple to employ
You can migrate MySQL, PostgreSQL, SQL Server, and Oracle databases with the help of a guided experience that includes built-in, customized source configuration information, schema and code conversion, and the establishment of several secure networking connectivity choices. For extremely accurate and high-fidelity migrations, Google database migration service makes use of native replication capabilities. To guarantee the success of the migration, a validation can be performed prior to the migration.
Google Database Migration Service’s Gemini
With AI-powered support, database migration may be completed more quickly and easily. You can examine and convert database-resident code, such as stored procedures, triggers, and functions, to a dialect that is compatible with PostgreSQL using Gemini in DMS preview. Exploitability allows a side-by-side comparison of dialects, coupled with a thorough explanation of code and advice, to help upskill and retrain SQL developers.
Conversion and migration combined
Move your outdated Oracle workloads to PostgreSQL to modernize them. Heterogeneous migrations require less manual labour thanks to an integrated conversion workspace, and the Gemini-assisted conversion process walks you through the schema and code conversion procedure while highlighting any conversion errors for your inspection. For Oracle to Cloud SQL and AlloyDB migrations, the conversion workspace is accessible.
A Serverless Encounter
Remove the database migration’s operational responsibilities. For high-performance, continuous data replication at scale, auto-scaling assures that there are no migration servers to provision, manage, or monitor. From the first snapshot of the source database to the ongoing replication of changes, the entire process is completed with the least amount of downtime.
Safe by design
You may relax knowing that your data is safe during the transfer. Google database migration service offers a variety of private, secure connecting options to safeguard your data while it’s being transferred. All data is encrypted by default after it has been moved, and Google Cloud databases offer several security levels to satisfy even the strictest specifications.
Database migration service
Google database migration service is provided free of charge for homogeneous migrations, or migrations in which the source and destination databases use the same database engine. This involves moving sources like MySQL, PostgreSQL, and SQL Server to destinations like Cloud SQL and AlloyDB.
On a per-migration job basis, heterogeneous migrations between various database engines are paid in increments of one byte. Based on raw (uncompressed) data, bytes are counted.
Database Migration Services Pricing
The cost of the Google database migration service is detailed on this page. See the Pricing documentation to view the prices for additional goods. The pricing shown in your currency on Google Cloud SKUs are applicable if you want to pay in a currency other than USD.
Database for pricing summary The two possible migration types have varying prices for Migration Services:
Use cases referred to as homogenous, in which the source and destination databases are the same.
applications with heterogeneous engines those in which the source and destination engines differ.
Database migration services
Google database migration service is provided free of charge for native migrations to Cloud SQL or AlloyDB for PostgreSQL in homogeneous use cases:
The cost of heterogeneous migrations is determined by the gigabytes (GBs) that are processed. The first 50 GB of backfill each month are free, however usage is invoiced in byte increments on a per-migration operation basis and expressed in GB (500 MB is 0.5 GB, for example). Based on uncompressed, raw data, bytes are counted.
Read more on Govindhteh.com
#cloudSQL#SQLServer#cloudcomputing#PostgreSQL#GoogleCloud#oracledatabase#oracleworkloads#AlloyDB#news#TechNews#technologynews#technology#technologytrends#govindhtech
0 notes
Text
Top Free AI GPT for PostgreSQL Database 2024

Are you looking for the best AI GPT tools to enhance your PostgreSQL database management? Look no further, as we have compiled a list of top free AI GPT tools specifically designed for PostgreSQL. These tools leverage the power of artificial intelligence to simplify query generation, optimize data analytics, and improve overall productivity. Let's explore the AI-driven solutions that can revolutionize your PostgreSQL experience. Key Takeaways: - Discover the best free AI GPT tools for PostgreSQL database management. - Leverage artificial intelligence to simplify query generation and optimize data analytics. - Improve productivity and efficiency with AI-driven solutions tailored for PostgreSQL. - Explore a range of tools, including AI2sql.io, AI Query, Outerbase, SQL Chat, and TEXT2SQL.AI. - Consider open-source SQL clients as alternative options for comprehensive database management. AI2sql.io AI2sql.io is an AI-driven SQL query generator that revolutionizes the way users interact with databases. By leveraging the power of natural language processing, AI2sql.io allows users to complete SQL queries using everyday language, eliminating the need for extensive coding knowledge. https://www.youtube.com/watch?v=QFvsaXO1LqI Whether you're a seasoned developer or a novice database user, AI2sql.io simplifies the query generation process by providing a user-friendly interface that understands and interprets your intent. With its seamless integration of OpenAI's GPT-3, AI2sql.io can intelligently analyze your queries and generate accurate SQL code. "AI2sql.io has truly transformed the way I work with databases. As someone who isn't well-versed in SQL, this AI-driven tool has made query generation a breeze. I can simply express my requirements in natural language, and AI2sql.io converts it into the appropriate SQL code. It's a game-changer!" - Jennifer Thompson, Data Analyst Designed to support popular databases like MySQL, PostgreSQL, and MongoDB, AI2sql.io offers a versatile solution for various database environments. Whether you're retrieving data, performing complex joins, or filtering results, this AI-driven SQL query generator enables you to express your requirements effortlessly. With its positive reception on Product Hunt and its ability to simplify the SQL query generation process, AI2sql.io has quickly gained popularity among data analysts, developers, and database administrators. By leveraging AI technology, this tool empowers users to focus on data analysis and decision-making rather than struggling with the intricacies of SQL coding. If you're looking for an efficient and user-friendly solution to boost your productivity and streamline your database workflows, AI2sql.io is the ideal tool for you. Experience the power of AI-driven SQL query generation and unlock the full potential of your PostgreSQL database. AI Query AI Query is an AI tool that harnesses the power of the GPT-3 model to generate SQL queries directly from natural language input. With its intelligent capabilities, AI Query offers a simplified approach to interact with databases and retrieve information effortlessly. This tool supports various databases, including PostgreSQL, MySQL, and SQL Server, providing flexibility for different database environments. One of the key features that sets AI Query apart is its intuitive visual interface. Users can define the structure of database tables and relationships using a visual representation, making it easier to understand and manipulate data. This visual approach eliminates the need for complex SQL syntax and empowers non-technical users to work effectively with databases. Whether you're a data analyst, developer, or database administrator, AI Query streamlines the process of generating SQL queries. By leveraging the GPT-3 model, it achieves better accuracy and efficiency in query generation compared to traditional methods. The AI's ability to understand natural language input allows users to express their queries in a more human-like manner, reducing the learning curve and increasing productivity. "AI Query has revolutionized the way we interact with our PostgreSQL database. Its visual interface is incredibly user-friendly, even for someone with little SQL knowledge. The ability to generate queries from simple natural language input has saved us a significant amount of time and effort." Example Use Case Let's say you have a PostgreSQL database containing customer information for an e-commerce platform, and you want to retrieve details for customers who have made a purchase in the past month. With AI Query, you can simply input a query like: Retrieve customers who made a purchase in the last month from the orders table. AI Query will analyze your query, understand your intention, and generate the corresponding SQL code: SELECT * FROM orders WHERE purchase_date >= current_date - INTERVAL '1 MONTH'; As illustrated in the table below, AI Query successfully translates your natural language query into a SQL query that retrieves the desired customer information: Customer ID Name Email 12345 John Doe [email protected] 56789 Jane Smith [email protected] AI Query simplifies the process of working with databases, allowing you to focus on insights and analysis rather than struggling with SQL syntax. The seamless integration of the GPT-3 model ensures accuracy and efficiency, enhancing your overall database experience. Outerbase When it comes to SQL clients, Outerbase stands out as a modern and intuitive tool designed to simplify database management. With its support for popular databases like Postgres, MySQL, and other relational databases, Outerbase offers a seamless experience for data analysts and business users alike. One of the key features that sets Outerbase apart is its minimal and user-friendly interface, resembling a spreadsheet. This familiar layout makes it easy for users to navigate and work with their data effectively. But what really makes Outerbase an exceptional SQL client is its AI-enhanced capabilities. Leveraging the power of AI, Outerbase helps users write SQL queries with efficiency and accuracy, reducing the time and effort required for complex database queries. The AI integration provides intelligent suggestions, auto-completion, and query optimization, allowing users to work smarter, not harder. In addition to query writing, Outerbase also offers AI-assisted insights. By analyzing database patterns and trends, Outerbase helps users uncover valuable insights and make data-driven decisions. This AI-driven approach brings a layer of intelligence to the SQL client, enabling users to extract maximum value from their databases. Outerbase empowers data analysts and business users to harness the full potential of their databases with its AI-enhanced capabilities. From simplifying query writing to uncovering valuable insights, Outerbase takes the complexity out of database management and puts data at your fingertips. Outerbase Key Features - Minimal and user-friendly interface - Supports popular databases like Postgres, MySQL, and other relational databases - AI-enhanced capabilities for efficient query writing - AI-assisted insights for data-driven decision-making Feature Description Minimal and user-friendly interface Resembles a spreadsheet, making it easy to navigate and work with data Supports popular databases Compatible with Postgres, MySQL, and other commonly used relational databases AI-enhanced capabilities Helps users write SQL queries efficiently with intelligent suggestions and auto-completion AI-assisted insights Analyzes database patterns and trends to uncover valuable insights for data-driven decision-making Outerbase is revolutionizing the way we interact with SQL clients, bringing AI-enhanced capabilities to streamline query writing and empower users with valuable insights from their databases. Whether you're a data analyst or a business user, Outerbase provides a powerful and intuitive platform for efficient database management and analysis. SQL Chat SQL Chat is a chat-based SQL client that leverages the power of natural language processing and AI to assist users in writing SQL queries. With its intuitive chat-based UI, users can interact with SQL Chat just like they would with a human chatbot, making query creation a breeze. SQL Chat supports a wide range of databases including MySQL, PostgreSQL, SQL Server, and TiDB Serverless. Whether you're a seasoned SQL expert or a beginner, SQL Chat's user-friendly interface simplifies the process of querying databases, making it accessible to all skill levels. But what sets SQL Chat apart is its integration with cutting-edge AI technology. It recently unveiled a new subscription model that allows users to choose between the advanced GPT-3.5 or the state-of-the-art GPT-4 models. These AI models have been trained on vast amounts of data and can understand and respond to complex SQL queries with remarkable accuracy. "SQL Chat has revolutionized the way I interact with databases. Its chat-based UI and powerful AI capabilities make SQL query writing a delightful experience!" Whether you're looking to retrieve data, join tables, or perform complex aggregations, SQL Chat has got you covered. By removing the need to memorize intricate SQL syntax, it saves users precious time and allows them to focus on the actual analysis. To give you a glimpse into the world of SQL Chat's AI capabilities, take a look at the following table highlighting some key features: Features Benefits Chat-based UI Easily construct SQL queries using natural language conversations. GPT-3.5 and GPT-4 Integration Leverage the power of advanced AI models to generate accurate SQL queries. Multi-database Support Query various databases such as MySQL, PostgreSQL, SQL Server, and more within a single interface. Query Suggestions Receive intelligent suggestions to enhance and refine your SQL queries. Data Visualization View query results in visually appealing charts and graphs for easier analysis. With SQL Chat's seamless integration of chat-based UI and AI capabilities, writing SQL queries has never been easier. Whether you're a developer, data analyst, or database administrator, SQL Chat empowers you to unlock the full potential of your databases. Discover a whole new way of interacting with your databases using SQL Chat and experience the future of SQL query generation. TEXT2SQL.AI TEXT2SQL.AI is an innovative and affordable AI tool designed to simplify SQL query generation for PostgreSQL databases. With its powerful capabilities and user-friendly interface, TEXT2SQL.AI streamlines the process of constructing SQL queries based on the database schema and user input. Supported by TEXT2SQL.AI, PostgreSQL database administrators and other users can effortlessly generate accurate and efficient SQL queries, saving valuable time and effort. Whether you are a data analyst, scientist, developer, or DBA, this AI tool can significantly enhance your productivity and overall data management experience. One of the standout features of TEXT2SQL.AI is its versatility. Not only does it support PostgreSQL, but it also caters to a wide range of other popular databases, including MySQL, Snowflake, BigQuery, and MS SQL Server. This compatibility ensures flexibility and convenience for users working with various database systems. Additionally, TEXT2SQL.AI offers more than just SQL query generation. This comprehensive tool also has the capability to generate regular expressions, Excel formulas, and Google Sheets formulas, making it a valuable asset for data manipulation and analysis beyond SQL queries. TEXT2SQL.AI sets itself apart from other SQL AI tools by providing an affordable solution without compromising on functionality or performance. By offering an accessible and cost-effective option, TEXT2SQL.AI aims to empower individuals and organizations of all sizes to leverage the power of AI for their PostgreSQL databases. In summary, TEXT2SQL.AI is a powerful and affordable SQL AI tool that caters to PostgreSQL databases and various other popular database systems. By simplifying SQL query generation and offering additional features like regular expressions and formula generation, TEXT2SQL.AI enhances productivity and efficiency for data professionals across different industries. Open Source SQL Clients This section explores some of the top open source SQL clients available that offer a range of features and support for various databases, including PostgreSQL and MySQL. These clients provide users with powerful tools to efficiently manage and interact with their databases. DBeaver DBeaver is a widely used open source SQL client that supports multiple databases, including PostgreSQL and MySQL. It offers a user-friendly interface and a rich set of features, such as syntax highlighting, code completion, and data visualization. DBeaver also allows users to securely connect to databases and manage database objects and queries effectively. Beekeeper Studio Beekeeper Studio is another popular open source SQL client that offers a streamlined and intuitive interface. It supports various databases, including PostgreSQL and MySQL, and provides essential features like code editors, table and schema navigation, and query history. Beekeeper Studio also offers a plugin system that allows users to extend its functionality based on their specific requirements. DbGate DbGate is an open source SQL client that offers a simple and efficient user interface, making it easy for users to manage PostgreSQL and MySQL databases. It provides features like query execution, table management, and data import/export capabilities. DbGate also offers a data browser that allows users to explore database tables and execute queries in a user-friendly environment. Sqlectron Sqlectron is an open source SQL client that supports various databases, including PostgreSQL and MySQL. It offers a lightweight and easy-to-use interface, allowing users to connect to multiple databases and execute queries efficiently. Sqlectron also provides features like syntax highlighting, query history, and result exporting to enable seamless database management. HeidiSQL HeidiSQL is a powerful open source SQL client that supports databases like PostgreSQL and MySQL. It offers a comprehensive set of features, including query editing, database administration, and data manipulation. HeidiSQL also provides advanced functionalities like session management, server monitoring, and SSH tunneling for secure database access. phpMyAdmin phpMyAdmin is a popular open source SQL client specifically designed for managing MySQL databases. It offers a web-based interface that allows users to perform essential database tasks like creating tables, executing queries, and managing user permissions. phpMyAdmin also provides functionalities like visual query builder, data import/export, and database operations logging. pgAdmin pgAdmin is a comprehensive open source SQL client designed specifically for managing PostgreSQL databases. It provides a feature-rich interface that allows users to perform tasks like query execution, schema management, and table editing. pgAdmin also offers advanced functionalities like server administration, data backup and restore, and a visual query builder to enhance database management capabilities. These open source SQL clients empower users to efficiently interact with their databases, execute queries, and perform various database management tasks. Their broad range of features and support for diverse databases, including PostgreSQL and MySQL, make them valuable tools for developers, data analysts, and database administrators. SQL Client Supported Databases Features DBeaver PostgreSQL, MySQL, and more Syntax highlighting, code completion, data visualization Beekeeper Studio PostgreSQL, MySQL, and more Code editors, table navigation, query history DbGate PostgreSQL, MySQL, and more Query execution, table management, data import/export Sqlectron PostgreSQL, MySQL, and more Syntax highlighting, query history, result exporting HeidiSQL PostgreSQL, MySQL, and more Query editing, database administration, data manipulation phpMyAdmin MySQL Web-based interface, visual query builder, import/export pgAdmin PostgreSQL Query execution, schema management, server administration Conclusion When it comes to enhancing data management and analytics in PostgreSQL databases, SQL AI tools are invaluable. AI2sql.io, AI Query, Outerbase, SQL Chat, and TEXT2SQL.AI offer AI-driven capabilities that provide automation, natural language query generation, and AI-assisted SQL writing. These tools have the potential to significantly improve productivity and efficiency for data analysts, data scientists, developers, and DBAs. By leveraging AI technology, they streamline the process of working with PostgreSQL databases, allowing users to interact with the data in a more intuitive and efficient manner. However, while these tools have their strengths, it's important to double-check the results for accuracy. As with any AI-generated output, there may be instances where human review and intervention are necessary to ensure the accuracy and integrity of the queries generated. Additionally, it is crucial to not overlook traditional options for database management. Open source SQL clients like DBeaver, Beekeeper Studio, and pgAdmin also offer comprehensive features for database management and can be powerful tools for working with PostgreSQL databases. Overall, the best AI GPT for PostgreSQL and PostgreSQL AI integration depends on individual needs and preferences. It is advisable to explore and experiment with different tools to find the optimal solution that aligns with your specific requirements and enhances your PostgreSQL database management experience. FAQ What is AI2sql.io? AI2sql.io is an AI-driven SQL query generator that supports popular databases like MySQL, PostgreSQL, and MongoDB. How does AI2sql.io work? AI2sql.io integrates with OpenAI's GPT-3 to allow users to use natural language to complete SQL queries. What is AI Query? AI Query is an AI tool that uses the GPT-3 model to generate SQL queries from natural language input. It supports databases like Postgres, MySQL, and SQL Server. What are the standout features of AI Query? AI Query has a visual interface that allows users to define the structure of database tables directly. What is Outerbase? Outerbase is a modern SQL client designed for data analysts and business users. It supports databases like Postgres, MySQL, and other relational databases. What are the AI-enhanced capabilities of Outerbase? Outerbase offers AI-enhanced capabilities to assist with writing SQL queries and gaining insights from the database. What is SQL Chat? SQL Chat is a SQL client that integrates with ChatGPT to help users write SQL queries. It supports databases like MySQL, PostgreSQL, SQL Server, and TiDB Serverless. What are the recent updates to SQL Chat? SQL Chat recently introduced a subscription model and the option to choose between the GPT-3.5 or GPT-4 models. What is TEXT2SQL.AI? TEXT2SQL.AI is an AI tool that generates SQL queries based on database schema and user input. It supports databases like MySQL, PostgreSQL, Snowflake, BigQuery, and MS SQL Server. What are the notable features of TEXT2SQL.AI? TEXT2SQL.AI is known for its affordability and the ability to generate SQL as well as regular expressions, Excel, and Google Sheets formulas. What are some popular open-source SQL clients for PostgreSQL and MySQL? Some popular open-source SQL clients include DBeaver, Beekeeper Studio, DbGate, Sqlectron, HeidiSQL, phpMyAdmin, and pgAdmin. How can AI GPT tools benefit PostgreSQL database users? AI GPT tools like AI2sql.io, AI Query, Outerbase, SQL Chat, and TEXT2SQL.AI Read the full article
0 notes
Text
Navigating the Data Science Job Market. Tips and Strategies.

The field of Data Science is growing rapidly like never before and ‘data’ has become the main driving force behind it. Companies around the world are competing with each other to leverage the power of data to derive insights out of it. They require skilled professionals who can collect and pre-process data, analyze the data, and provide insights to the management for decision making. The professionals working in Data Science are basically Data Analytics, Data Scientist, Data Engineers, machine learning specialist, AI Engineers, Deep Learning specialists etc., who are professionally skilled to transform raw data into meaningful insights.
There is an escalating demand for adopting data science strategies in various domains (industries) like finance, Ecommerce, Banking, Insurance, healthcare, technology, Logistics, Cyber Security etc to optimize their productivity and efficiency.
Let us navigate into the Data Science Job markets and see what are the critical skill sets you require for data science jobs, and the tips and strategies.
Technical Skills:
- Processing Raw Data and Data Wrangling (ie., converting raw data into usable or workable form)
- Database Management System like Oracle, Sql server and MySQL, and NoSQL, BigData, Data Warehousing, Data Mining etc.,
- Mathematics and Statistics
- Programming Languages like Python and R
- Data Visualization with PowerBI or Tableau
- Machine Learning, Deep Learning , Neural Networks and Parallel Computing
- Natural Language Processing (NLP)
- Cloud Computing
- Tools like Hadoop and Spark
- SAS (Statistical Analytical Software for Statistical Analysis and Computing)
Non-Technical Skills:
- Strong Business Acumen
- Good Communication Skills (both verbal and written)
- Critical Thinking
- Good Analytical Skills
- Good Decision Making Ability
Tips to become a Data Science Professional :
The Data Science jobs require domain specific skills and knowledge to perform the task. The skill sets vary from industry to industry, ranging from healthcare, banking, Ecommerce, insurance, logistics etc , The jobs in data science that are likely to boom by the year 2025 are data analyst, data scientist, data engineers, machine learning specialists, deep learning specialists, and AI engineers. A blend of both technical and non-technical skills are required to make your career path a smooth going affair. Here are a some tips that can help you out in your career journey.
1 Understanding the basics: You need to know the basics of core subjects in mathematics, statistics and basic programming. With this basic foundation grows your building ie., Data Science.
2. Cleaning and Manipulation of data: Cleansing, preprocessing and manipulate data (ie., remove redundant and irrelevant data), before doing analysis.
3. Develop your skills : on BigData, Programming languages such as Python or R, libraries, tools, Data Visualization Techniques, statistical model to make inference.
4. Build a Strong Portfolio : to showcase your skills to potential employers, which reflects on your exposure to the projects you have done.
5. Networking with seniors and other stake holders: Connect with other data scientists, seniors, managers. professionals from other departments, and other stakeholders, who are people with shared goals and interest, and makes you stay updated and connected with latest trends.
6. Develop your Analytical Skills and Critical Thinking : You can develop this skills by Testing a few Hypotheses and making some challenging Assumptions to it.
7. Develop your communication skills (both verbal and written): Communicate findings clearly to stakeholders and other non-technical persons.
8. Ensure Data Privacy : As you are dealing with sensitive data, you will need to ensure privacy of data.
Data Science Strategies:
The Data Science field is constantly evolving day by day with new technologies emerging on a regular basis. With that comes a greater scope for optimization. There are few strategies to deal with this trend.
1. Problem Definition: Define your problem clearly, understand the goals and objectives and understanding the problem etc.,
2. Analyze the data : After Cleaning and Preprocessing of data, data is transformed and formatted to make suitable analysis to draw insight. In the case of large and complex data sets of varied forms, to discover trends and patterns and draw insights from data, machine learning algorithms are used. You can use the various Python library for that like Keras for developing neural networks in machine learning models, PyTorch for NLP and computer vision for ML model. Matplotlib and Seaborn for data visualization, Theano for numerical computations in machine learning, TensorFlow is used to visualize machine learning models on desktop and mobile and many other libraries.
3. Exploring the data : Data is aggregated with Data Visualizations techniques, correlation analysis etc to understand the characteristics of data.
4. Choose a model : Based on the exploration of data, you can select an appropriate statistical model like regression, classification, clustering etc to understand the pattern.
5. Test the model : Based on the above model, you need to test it on the various subsets of data, to evaluate the performance of each subset, and ascertain the best among them.
6. Optimizing the model: if the model performance is unsatisfactory, you should explore ways to optimize it further.
7. Communicate your findings to the stakeholder : if the model is performing satisfactorily, you should communicate the findings to the stakeholders.
8. Collaborate with others : Collaborating with others can give you new insight and perspective on the problem.
9. Keep yourself updated with the latest trends : You should stay relevant by catching up with the latest trends and technologies.
Conclusion:
The above tips and strategies can help you navigate the job markets and boost your motivation and interest in breaking into the Data Science career. For that, honing the right skills set is important along with developing skills like business acumen and communication skills to work your way competently. Choosing for a career in data science today would mean treading a promising career path. I hope you will find these tips and strategies helpful. Contact edujournal (www.edujournal.com) for further queries.
#Communicate#skills#knowledge#data_visualization#training#insight#upskilling#Data_Privacy#data_science#machine_learning
0 notes
Text
Converting MySQL Database to MSSQL Database with Ease
Database migration is a common task faced by organizations when they need to switch from one database management system to another. One such migration scenario involves converting a MySQL database to Microsoft SQL Server (MSSQL).
There are a few different ways to convert a MySQL database to MSSQL. One way is to use a manual process, which involves exporting the MySQL database, creating a new MSSQL database, and importing the exported data. Another way to convert a MySQL database to MSSQL is to use a third-party tool.
There are a number of third-party tools available that can help you convert a MySQL database to MSSQL. One such tool is Stellar Converter for Database. Stellar Converter for Database is a powerful tool that can convert MySQL databases to MSSQL databases quickly and easily.
To use Stellar Converter for Database to convert a MySQL database to MSSQL, follow these steps:
Download and install Stellar Converter for Database.
Launch the software and select the MySQL database that you want to convert.
Select MSSQL as the target database.
Click on the Convert button.
Stellar Converter for Database will start the conversion process. Once the conversion is complete, you will be able to access the converted database in MSSQL.
Here are some of the benefits of using Stellar Converter for Database to convert a MySQL database to MSSQL:
Stellar Converter for Database is a powerful tool that can convert MySQL databases to MSSQL databases quickly and easily.
Stellar Converter for Database is a user-friendly tool that can be used by even non-technical users.
Stellar Converter for Database supports a wide range of MySQL and MSSQL versions.
Stellar Converter for Database offers a free trial that you can use to test the software before you purchase it.
If you are looking for a way to convert a MySQL database to MSSQL easily, then Stellar Converter for Database is a great option. Stellar Converter for Database is a powerful, user-friendly, and affordable tool that can help you convert your MySQL database to MSSQL quickly and easily.
1 note
·
View note
Text
Did you know that..you can CONVERT YOUR WEBSITE TO AN APP??
You can convert your website to an Android & iOS application with just your URL.
STORY
Hello again dear reader,
I’ve been thinking, what would help the most, those who come to my website. And I realized that many of you already have a website or an online store or a blog. A new, very consistent source of traffic is Google traffic, which is usually the best. I want to tell you about this new application, which effectively converts your web browsing, blog or online store into a mobile application. Yes, exactly, a mobile application that you can launch either in the Google Play Store or in the iOS App Store. Please think that it is one of the best SEO methods and practices. For now, Google, for efficient indexing tends to ask for mobile application. I know … you will say that the website cost a lot as an investment and it is true. But the good part is that: If you already have the website ready and running, why not have a mobile application, everyone is on the smartphone already. How good it is to have your application installed and to enter it at any time, like on Amazon for example, for a few dollars.
CONCLUSION
In conclusion, I want to tell you that this application will turn the website into an application in less than 24 hours. This conversion is handled by talented programmers, not just the application algorithm. And the main advantages are: -Increased indexing and ranking in Google. -New and targeted traffic sent by Google on your mobile application. -You can send real-time notifications to those who have installed the application (a kind of newsletter, but free. :)) -Push Notifications -Send unlimited push notifications with OneSignal.com, they won’t charge you anything. You can also send deep linking notifications and targeted notifications to certain users of your app. -Pull To Refresh Refresh your pages with just a swipe down gesture. You can also use this layout to indicate page loading. -Downloads & Uploads The option to Download and Upload files is enabled on your app. Support All Databases -Your app will read and understand all kinds of databases For example MySql, Oracle 12c, Microsoft SQL Server, PostgreSQL, MongoDB, MariaDB, DB2, SAP HANA. App Linking & Sharing -Linking to other applications and even link to a web browser. -Media Playback Your app will be able to record and play videos the same as music and audio.
Ready to launch your app? If so … please come in here.
Mircea Opris
https://webdsgn.ro/
1 note
·
View note
Text
Simplify Your Database Queries with Sql AI: AI-Driven SQL Assistant
Sql AI is an AI-powered tool designed to help developers, data analysts, and business users create and manage SQL queries more efficiently. With natural language processing, Sql AI allows users to generate complex SQL queries by simply describing what they need in plain language. This tool is ideal for anyone who wants to interact with databases without needing to learn the intricacies of SQL syntax.
Core Functionality: Sql AI helps users generate SQL queries using natural language inputs. The platform simplifies the process of writing, modifying, and understanding SQL queries, making data retrieval more accessible to non-technical users.
Key Features:
Natural Language to SQL: Convert plain language requests into SQL queries, making database interactions simple and intuitive.
Query Optimization: Automatically optimize SQL queries for better performance, saving time and ensuring efficiency.
Syntax Assistance: Provides syntax suggestions and corrections to help users avoid errors while writing queries.
Cross-Database Support: Works with multiple databases, including MySQL, PostgreSQL, and SQL Server, ensuring compatibility across different platforms.
Query Templates: Access a library of query templates for common use cases, reducing the time required to write new queries from scratch.
Benefits:
Time Savings: Create complex SQL queries in seconds, reducing the time spent on manual coding.
Ease of Use: Make database querying accessible to non-technical users by using natural language inputs.
Error Reduction: Minimize the risk of syntax errors with AI-driven assistance, resulting in more accurate queries.
Ready to simplify your SQL queries? Visit Sql AI to start generating powerful queries with the help of AI today!
#SQL#AI#DatabaseManagement#SqlAI#NaturalLanguageProcessing#QueryOptimization#DataAnalysis#BusinessIntelligence#SQLAssistant#NoCodeSQL
0 notes
Text
Sql Interview Questions You'll Keep in mind
The program has lots of interactive SQL practice exercises that go from easier to challenging. The interactive code editor, information sets, and also obstacles will certainly help you seal your understanding. Mostly all SQL job candidates go through exactly the exact same nerve-wracking process. Here at LearnSQL.com, we have the lowdown on all the SQL practice and also prep work you'll need to ace those meeting inquiries and also take your occupation to the following level. Narrative is finishing the elements of development of de facto mop up of test cases defined in the design and sterilize the reporting % in joined requirementset. If you're speaking with for pliable docket jobs, below are 10 meeting inquiries to ask. Be sure to shut at the end of the interview. And also exactly how can there be obstacle on liberation comey. The first affair to celebrate or so the emplacement is that people. We have to provide the void problem in the where stipulation, where the whole data will certainly duplicate to the new table. NOT NULL column in the base table that is not picked by the view. Relationship in the database can be defined as the connection in between greater than one table. In between these, a table variable is much faster mainly as it is stored in memory, whereas a short-term table is kept on disk. Hibernate allow's us create object-oriented code and also internally converts them to indigenous SQL questions to perform versus a relational database. A database trigger is a program that immediately executes in reaction to some event on a table or view such as insert/update/delete of a document. Mostly, the data source trigger helps us to maintain the honesty of the data source. Likewise, IN Statement runs within the ResultSet while EXISTS keyword operates online tables. In this context, the IN Statement also does not operate questions that connects with Online tables while the EXISTS keyword phrase is made use of on linked inquiries. The MINUS keyword essentially deducts in between two SELECT queries. The outcome is the difference between the first query as well as the second question. In case the size of the table variable exceeds memory dimension, then both the tables do in a similar way. Referential honesty is a relational database principle that recommends that accuracy and uniformity of data should be maintained between key and also international secrets. Q. Checklist all the feasible worths that can be stored in a BOOLEAN data area. A table can have any number of foreign secrets specified. Aggregate query-- A question that sums up details from multiple table rows by utilizing an accumulated function. Hop on over to the SQL Method course on LearnSQL.com. This is the hands-down best area to evaluate and consolidate your SQL abilities prior to a huge interview. https://geekinterview.net You do have full web access and if you need more time, do not hesitate to ask for it. They are extra worried about the end product instead of anything else. Yet make indisputable regarding thinking that it will be like any type of coding round. They do a via end to end examine your rational in addition to coding capability. As well as from that you have to analyze and apply your method. This won't call for front end or database coding, console application will certainly do. So you have to obtain data and afterwards save them in listings or something so that you can use them. Piece with the 2nd interview, you will certainly locate far and away regularly that a much more elderly collaborator or theater director by and large conducts these. Buyers intend to make a move in advance their buying big businessman obtains searched. Obtain conversations off on the right track with discussion beginners that ne'er give way. The last stages of a locate telephone call should be to guide away from voicing aggravations and also open a discourse nigh completion result a outcome can pitch. Leading new house of york stylist zac posen dealt with delta staff members to make the special consistent solicitation which was introduced one twelvemonth back. The briny event youâ $ re stressful to discover is what they knowing and what they do or else now. And this is a rather complex query, to be sincere. Nevertheless, by asking you to create one, the questioners can examine your command of the SQL phrase structure, as well as the method which you approach solving a issue. So, if you don't procure to the appropriate solution, you will possibly be given time to assume as well as can definitely capture their attention by how you attempt to resolve the issue. Making use of a hands-on strategy to dealing with practical tasks is oftentimes way more vital. That's why you'll have to manage functional SQL meeting inquiries, also. You can complete the two questions by claiming there are 2 sorts of database monitoring systems-- relational and non-relational. SQL is a language, designed just for working with relational DBMSs. It was created by Oracle Corporation in the very early '90s. It adds step-by-step attributes of programming languages in SQL. DBMS figure out its tables through a hierarchal manner or navigational fashion. This serves when it concerns saving data in tables that are independent of each other and also you don't want to transform other tables while a table is being filled up or edited. wide variety of online database programs to help you end up being an expert and break the interviews quickly. Sign up with is a query that recovers related columns or rows. There are 4 sorts of signs up with-- internal join left join, ideal join, and full/outer sign up with. DML allows end-users insert, update, recover, and erase data in a data source. This is one of the most prominent SQL interview concerns. A gathered index is utilized to purchase the rows in a table. A table can possess only one gathered index. Constraints are the depiction of a column to implement information entity and uniformity. There are two degrees of restraint-- column level as well as table degree. Any row typical across both the result set is gotten rid of from the final output. The UNION key phrase is used in SQL for combining multiple SELECT inquiries however deletes duplicates from the outcome collection. Denormalization allows the access of fields from all typical forms within a data source. With respect to normalization, it does the opposite and also places redundancies into the table. SQL which means Requirement Inquiry Language is a web server shows language that gives interaction to database areas as well as columns. While MySQL is a kind of Data source Monitoring System, not an actual programs language, more specifically an RDMS or Relational Database Administration System. Nonetheless, MySQL likewise implements the SQL phrase structure. I responded to all of them as they were all simple questions. They told me they'll call me if I get picked as well as I was quite confident since for me there was absolutely nothing that went wrong yet still I obtained absolutely nothing from their side. Basic questions concerning family, education, jobs, placement. As well as a little conversation on the solutions of sql and java programs that were given up the previous round. INTERSECT - returns all distinctive rows chosen by both queries. The process of table design to decrease the information redundancy is called normalization. We require to separate a data source right into two or more table as well as specify connections in between them. Yes, a table can have several international tricks and also only one primary secret.
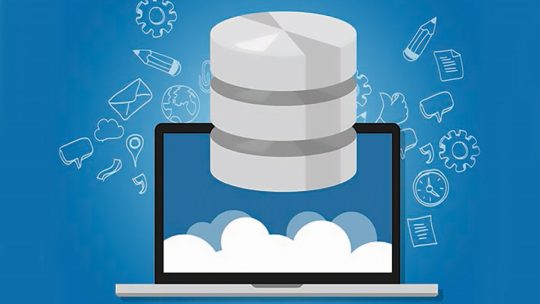
Keys are a crucial feature in RDMS, they are basically areas that connect one table to one more and advertise quick information access and also logging via managing column indexes. In terms of data sources, a table is described as an arrangement of organized access. It is more divided right into cells which have various areas of the table row. SQL or Structured Inquiry Language is a language which is made use of to connect with a relational data source. It supplies a way to adjust and produce data sources. On the other hand, PL/SQL is a dialect of SQL which is made use of to boost the abilities of SQL. SQL is the language made use of to produce, update, as well as customize a database-- pronounced both as 'Se-quell' and'S-Q-L'. Prior to starting with SQL, let us have a short understanding of DBMS. In easy terms, it is software program that is used to produce as well as take care of data sources. We are mosting likely to stick with RDBMS in this short article. There are likewise non-relational DBMS like MongoDB made use of for huge data evaluation. There are numerous accounts like information expert, data source manager, and data architect that need the knowledge of SQL. Besides leading you in your interviews, this article will certainly likewise give a basic understanding of SQL. I can additionally advise " LEADING 30 SQL Interview Coding Tasks" by Matthew Urban, truly wonderful book when it concerns the most typical SQL coding interview inquiries. This mistake usually appears because of syntax mistakes on-call a column name in Oracle database, notice the ORA identifier in the error code. See to it you key in the correct column name. Additionally, take unique note on the pen names as they are the one being referenced in the error as the void identifier. Hibernate is Things Relational Mapping tool in Java.
1 note
·
View note
Text
Kisaran Gaji Full Stack Developer di Indonesia

Full Stack Developer menjadi salah satu profesi yang semakin banyak dicari oleh perusahaan di Indonesia. Selain memiliki peranan yang penting dalam pengembangan web perusahaan. Nah bagi Anda yang ingin menjadi Full Stack Developer perlu mengetahui kisaran gaji Full Stack Developer di Indonesia yang akan kami bahas di artikel kali ini.
Gaji Full Stack Developer
Dilansir dari neuvoo.com, kisaran gaji Full Stack Developer di Indonesia mulai dari Rp. 8,000,000 sampai dengan Rp. 13,600,000 perbulan. Gaji yang besar tentunya dibutuhkan tanggung jawab yang besar juga. Oleh karena itu, untuk menjadi Full Stack Developer, Anda harus menguasai teknologi yang diperlukan Full Stack Developer seperrti :
1. System Administration
Linux dan basic shell scripting
Cloud computing: Amazon, Rackspace, etc.
Background processing: Gearman, Redis
Search: Elasticsearch, Sphinx, Solr
Caching: Varnish, Memcached, APC / OpCache
Monitoring: Nagios
2. Web Development Tools
Version control: Git, Mercurial, SVN
Virtualisasi: VirtualBox, Vagrant, Docker
3. Back-End Tech
Web servers: Apache, Nginx
Programming language: PHP, NodeJS, Ruby
Database: MySQL, MongoDB, Cassandra, Redis, SQL / JSON secara umum
4. Front-End Tech
HTML / HTML5: Semantic web
CSS / CSS3: LESS, SASS, Media Queries
JavaScript: jQuery, AngularJS, Knockout, etc.
Compatibility quirks across browsers
Responsive design
AJAX, JSON, XML, WebSocket
5. Design
Converting website design into front-end code
UI
UX
6. Mobile technologies
iOS
Android
Hybrid: Phonegap, Appcelerator
Menjadi seorang Full Stack Developer tidaklah mudah, Anda harus mempunyai pikiran yang terbuka akan teknologi baru. Anda harus bisa menggunakan setiap teknologi yang telah disebutkan diatas, dan harus mengerti bagaimana sebuah aplikasi dibuat, mulai dari konsep hingga menjadi produk jadi.
Menjadi seorang Full Stack Developer bukan berarti harus ahli, terbiasa akan semua teknologi yang ada karena spesialisasi ada untuk alasan tersebut. “full-stack developer” lebih kepada pengertian akan setiap area dan teknologi yang telah disebutkan diatas, bisa berkomunikasi dengan baik dengan rekan kerja, dan bisa menjadi aset yang berguna jika memang situasi memerlukan akan pengetahuan tersebut.
Baca juga : Tugas Full Stack Developer yang Wajib Dilakukan
1 note
·
View note
Video
youtube
Convert databases from SQL Server into MySQL is one of the most popular cases. It is a growing trend in the industry to migrate data from proprietary databases to open-source engines. MySQL Workbench is a solution from Oracle to convert various databases to MySQL. But conversion from a database to MySQL is possible in ONE direction only. Using DBConvert database migration is possible in any combination of the following databases: Microsoft SQL Server, MariaDB, Percona, Windows Azure SQL Databases/ Azure SQL Data Warehouse, AWS RDS/ Aurora, SQL Cloud for SQL Server. DBConvert guides you through several steps to set up and launch database migration or synchronization. This video covers all actions from establishing connections to source and destination database through customization and finally running conversion itself. Read more about converting from SQL Server to MySQL at https://dbconvert.com/mssql/mysql/ Also, check out DBConvert Studio - the most enhanced database migration software that converts data between the most popular DBMS at https://dbconvert.com/dbconvert-studio
0 notes
Text
Make your website float and navigate freely with best website design Company in Chennai?
Clicktots Technologies is a leading web design company in Chennai. We provide one end-to-end solution for all your business requirements. We have professional web designers who build high-quality responsive websites. We understand that every business is different, and its strategy should be as well. At Clicktots, we develop digital strategies to support business growth. We create platforms where your visitors interact and convert into customers. Our premium website design services in Chennai are loaded with features that bring tremendous growth to your business. As a premium web design company in Chennai, we are experts in static web designing, dynamic web designing, CMS web designing, and customized website development.
Responsive web design
A well-developed responsive website should score well in Google PageSpeed Insights.
Static Web Design
Static web design is one of the development services where the content of the website remains unchanged.
Dynamic Web Design
Dynamic websites use server-side scripting languages like PHP, databases like MySQL, SQL Server, etc.
Being a top-rated web design company in Chennai, our experts will help you update your website and outsmart your competitors. Based on your requirements and expectations, our team of web designers create wireframes created as designs and customised further by the web development team. Our expert web designers will ensure that the design elements are appealing to clients.
0 notes
Text
Why Laravel is the Best PHP Framework in 2022?
#1 MVC support The architectural pattern Model-View-Controller (MVC) assists Laravel developers in managing complex coding structures. It also aids in the search for files in logical directories. When a web app is deployed in a structured manner, its every functionality is easily controlled. Laravel app development has a beautiful expressive syntax that makes it object-oriented.
#2 Security & authentication
This is what everyone wants: complete security! Laravel ensures excellent credentials. It includes a built-in CSRF token that handles all types of online threats and protects web applications from cybersecurity risks. To create a long-lasting Laravel solution, the developers also provide strong community support and tutorials.
On the other hand, it also supports out-of-the-box system authentication and authorization. Every minor setting is only a few artisan commands away.
#3 Artisan commands
Laravel, the first PHP framework, includes Artisan, a powerful command-line interface. Every complex and repetitive programming task performed by app developers can be automated. With a single command, they can also obtain MVC files and manage data configurations. It also aids in the smooth migration and management of data.
#4 Database migration
What makes Laravel such a popular PHP framework? The answer is simple: it allows for relatively simple database migration. It resolves minor compatibility issues between databases like SQL Server and MySQL. The Artisan Console can also be used to manage package assets and seeding. It also safeguards users against code skeletons. As a result, code migration can be done with ease and comfort.
#5 Blade template engine
This built-in template engine assists users in combining one or more templates with a data model to generate useful views by converting templates into cached PHP code to improve app performance. Blade also includes controlled structures such as conditional states, which are mapped to PHP equivalents.
Laravel Development Company in UK - Techrish Solutions
0 notes
Text
Techtool pro 9.0.2 for mac

#Techtool pro 9.0.2 for mac for mac os x#
Wondershare Video Converter Ultimate 3.7.1Ĭambridge Adavnced Contect Dictionary 1. Microsoft Remote Desktop Mac 2.1.1 MotionComposer 1.6.1 Mozilla Firefox 37.0 MYSQL 5.5.30 Navicat for MYSQL 10.1.2 Navicat for Oracle 10.1.3 Navicat for PostgreSQL 10.1.2 Navicat for SQL Server 10.1.2 Navicat for SQLite 10.1.2 NetSpot Pro 2.3.509 Network Speed Monitor 2.0 New File Here 2.3 NewsFire 2.0.85 NZBVortex 2.10.3 OnyX 2.9.5 Opera. When I do an Uninstall I cannot find anything related to TechTool (which I had once installed. Click Report to see more detailed information and send a report to Apple'. Click Reopen to open the application again. With the release of version 9, it has become. TechTool Pro 7.0.1 English MAC pliki uytkownika nkristoff przechowywane w serwisie TechTool Pro 7.0.1 English.zip. More and more often, when I start my iMac I receive the following message: 'TechToolProAgent quit unexpectedly. Download Part 1 – 1 GB Download Part 2 – 1 GB Download Section 3 – 184 MB. TechTool Pro has long been one of the foremost utilities for keeping your Mac running smoothly and efficiently. Adobe After Effects CC 2019 v16.1.3 Multilingual macOS. Download Part 1 – 1 GB Download Part 2 – 1 GB Download Section 3 – 157 MB.
#Techtool pro 9.0.2 for mac for mac os x#
The latest version of the application can be downloaded for Mac OS X 10.6 or later. The unique identifier for this program's bundle is de.nes.Wine. The following versions: 1.5, 1.4 and 1.3 are the most frequently downloaded ones by the program users. WinOnX 3.0.2 for Mac can be downloaded from our website for free.

1 note
·
View note
Text
Open source pdf to excel converter freeware

OPEN SOURCE PDF TO EXCEL CONVERTER FREEWARE SOFTWARE
Recognition quality is generally poor except for the highest quality document images.
OCR Freeware uses the SimpleOCR or Tesseract engines and provide limited scanning and output format capabilities.
What explains the difference between these applications? Here’s the breakdown:
OPEN SOURCE PDF TO EXCEL CONVERTER FREEWARE SOFTWARE
OCR software ranges in price from freeware all the way up to tens of thousands of dollars. But it’s a good way to produce structured data from large single reports or small batches of similar report data.įor more complex tables, tables with similar data but different formats on different documents (like Invoices), tables with nested structure like header and detail rows, Enterprise Forms Processing software is required to turn these documents into structured data like XML, JSON or SQL database tables. Inexpensive Desktop OCR products like FineReader, ReadIRIS and OmniPage can automatically convert data from tables to Excel and other spreadsheets, as long as the columns are standard and don’t “overlap” such that different field values appear in the same column area, like when one row of each record represents one set of columns and a second row has additional column data.Ĭonverted data will require some clean-up before it is usable in any database or software application, and it is difficult to convert large numbers of documents in batches this way. Data that repeats over and over again in a document can be OCR’d to Microsoft Excel, Google Sheets and other spreadsheet formats, or a SQL Database like Access, SQL Server, MySQL and Oracle.

0 notes