#cpp programming homework help
Explore tagged Tumblr posts
Text
C++ Made Easy: Common Mistakes Students Make in Programming Assignments
C++ is a powerful and versatile programming language widely used in various applications, from system software to game development. However, students often encounter significant challenges when tackling C++ assignments.
In this guide, we will explore the frequent pitfalls students face in C++ programming and how to avoid them, also how to complete your assignments on time with AssignmentDude and its C++ Homework help services.
The Importance of C++
C++ is not just another programming language; it is a foundational skill for many aspiring software developers, engineers, and computer scientists.
Its ability to handle low-level memory manipulation while providing high-level abstractions makes it unique.
C++ is used in various domains, including game development (e.g., Unreal Engine), system programming (e.g., operating systems), and even high-performance applications (e.g., financial systems).
However, with great power comes great responsibility — and complexity.
Why Students Struggle
Many students find themselves overwhelmed by the intricacies of C++. From understanding syntax to grasping object-oriented programming concepts, the learning curve can be steep.
This is where AssignmentDude comes into play. Offering expert C++ homework help, AssignmentDude provides personalized assistance tailored to your unique learning needs.
Whether you’re grappling with basic concepts or advanced topics, our team is here to guide you through your assignments and enhance your understanding of C++.
The Role of AssignmentDude
At AssignmentDude, we understand that programming assignments can be daunting. Our dedicated tutors are not only proficient in C++, but they also excel in teaching complex concepts in an engaging manner.
By seeking help from AssignmentDude, you can ensure that you receive high-quality solutions that not only meet your assignment requirements but also deepen your understanding of the material.
Common Mistakes Students Make in C++ Programming Assignments
Understanding the common mistakes students make can significantly improve your coding skills and enhance your performance on assignments. Below are some frequent pitfalls:
1. Syntax Errors
C++ has a strict syntax that must be followed precisely. Even minor errors can lead to compilation failures.
Common Syntax Errors
Missing Semicolons: Every statement must end with a semicolon. Forgetting this can halt compilation.
Example:
cpp
int main() {
int x = 10 // Missing semicolon here
return 0;
}
Mismatched Brackets: Ensure that every opening bracket has a corresponding closing bracket.
cpp
int main() {
if (x > 0) {
cout << “Positive number” << endl;
// Missing closing brace for if statement
}
Incorrect Case Sensitivity: Variable names are case-sensitive; using inconsistent naming conventions can lead to errors.
Example:
cpp
int Value = 5;
cout << value; // Error: ‘value’ was not declared
Tips for Avoiding Syntax Errors
Use an IDE: Integrated Development Environments (IDEs) like Visual Studio or Code::Blocks highlight syntax errors as you code.
Regularly Compile: Frequent compilation helps catch errors early in the development process.
2. Misunderstanding Data Types
C++ offers various data types, each serving different purposes. Misusing these types can lead to unexpected behavior.
Key Data Types
Integer vs. Float: Using an integer when a float is required can cause loss of precision.
Example:
cpp
float pi = 3; // This will lose precision; should be float pi = 3.14;
Character vs. String: Mixing up single characters and strings can lead to compilation errors.
Example:
cpp
char letter = “A”; // Error: cannot initialize a char with a string literal
Best Practices for Data Types
Know Your Types: Familiarize yourself with the different data types available in C++. Use sizeof() to check sizes if you’re unsure.
cpp
cout << “Size of int: “ << sizeof(int) << endl;
cout << “Size of float: “ << sizeof(float) << endl;
cout << “Size of double: “ << sizeof(double) << endl;
Use Type Casting: When necessary, use explicit type casting to avoid implicit conversions that might lead to bugs.
Example:
cpp
double num = 10.5;
int wholeNum = (int)num; // Explicitly casting double to int
3. Improper Use of Pointers
Pointers are one of C++’s most powerful features but also one of its most confusing aspects for beginners.
Common Pointer Mistakes
Dereferencing Null Pointers: Attempting to access memory through a null pointer leads to runtime errors.
Example:
cpp
int* ptr = nullptr;
cout << *ptr; // Runtime error: dereferencing a null pointer
Memory Leaks: Failing to deallocate memory allocated with new results in memory leaks.
Example:
cpp
int* arr = new int[10];
// Missing delete[] arr; leads to memory leak
Strategies for Managing Pointers
Initialize Pointers: Always initialize pointers before use.
cpp
int* ptr = nullptr; // Safe initialization
Use Smart Pointers: Consider using smart pointers (like std::unique_ptr or std::shared_ptr) from the Standard Template Library (STL) to manage memory automatically.
cpp
std::unique_ptr<int> ptr(new int(10)); // Automatically deallocates when out of scope
Example of Smart Pointer Usage
cpp
#include <iostream>
#include <memory>
void example() {
std::unique_ptr<int> ptr(new int(5));
std::cout << *ptr << std::endl; // Outputs: 5
} // Memory automatically freed here when ptr goes out of scope
int main() {
example();
}
4. Not Following Object-Oriented Principles
C++ supports object-oriented programming (OOP), which can be challenging for beginners who are unfamiliar with its principles.
Common OOP Mistakes
Improper Encapsulation: Failing to use access specifiers (public, private) correctly can expose sensitive data.
cpp
class BankAccount {
public:
double balance; // Should be private
public:
void deposit(double amount) { balance += amount; }
double getBalance() { return balance; }
};
Ignoring Inheritance Rules: Misunderstanding how inheritance works can lead to design flaws in class hierarchies.
cpp
class Base {
public:
void show() { cout << “Base class” << endl; }
};
class Derived : Base { // Should use ‘public’ inheritance
public:
void display() { cout << “Derived class” << endl; }
};
Derived obj;
obj.show(); // Error due to private inheritance by default
Tips for Effective OOP Design
Understand OOP Concepts: Take time to learn about encapsulation, inheritance, polymorphism, and abstraction.
Design Before Coding: Spend time designing your classes and their interactions before jumping into coding.
Example of OOP Design Principles
cpp
class Shape {
public:
virtual double area() = 0; // Pure virtual function makes this an abstract class
};
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double area() override { return M_PI * radius * radius; } // Override area method
};
class Rectangle : public Shape {
private:
double width, height;
public:
Rectangle(double w, double h) : width(w), height(h) {}
double area() override { return width * height; } // Override area method
};
5. Lack of Comments and Documentation
Students often neglect commenting their code or providing documentation, making it difficult for others (and themselves) to understand their logic later on.
Importance of Comments
Clarity: Comments help clarify complex logic and decisions made during coding.
cpp
// Function calculates factorial recursively
int factorial(int n) {
if (n <= 1) return 1; // Base case for recursion
return n * factorial(n — 1); // Recursive case calls itself with decremented value
}
Maintenance: Well-documented code is easier to maintain and update later on.
Best Practices for Commenting
Comment What’s Necessary: Avoid over-commenting; focus on explaining complex sections or non-obvious logic.
Use Consistent Style: Maintain a consistent commenting style throughout your codebase.
Example of Good Commenting Practice:
cpp
// Calculate area of circle given radius r
double area(double r) {
return M_PI * r * r; // M_PI is defined in <cmath>
}
6. Inadequate Testing
Testing is crucial in programming; however, many students skip this step or do it inadequately.
Common Testing Mistakes
Not Testing Edge Cases: Ensure that your code handles unusual inputs gracefully.
cpp
void divide(int a, int b) {
if (b == 0) {
throw std::invalid_argument(“Division by zero”); // Handle division by zero error gracefully
}
}
Relying Solely on Manual Testing: Automated tests can catch errors more efficiently than manual testing alone.
Effective Testing Strategies
Write Unit Tests: Create unit tests for individual functions or classes to ensure they work as intended.
cpp
#include <cassert>
void testDivide() {
assert(divide(10, 2) == 5);
try {
divide(10, 0);
} catch (const std::invalid_argument& e) {
assert(std::string(e.what()) == “Division by zero”);
}
}
Use Test Frameworks: Utilize testing frameworks like Google Test or Catch2 to streamline the testing process.
Example Using Google Test Framework:
cpp
#include <gtest/gtest.h>
TEST(FactorialTest, PositiveNumbers) {
EXPECT_EQ(factorial(5), 120);
}
TEST(DivideTest, DivisionByZero) {
EXPECT_THROW(divide(10, 0), std::invalid_argument);
}
7. Ignoring Compiler Warnings
Compilers provide warnings for potential issues in the code that may not prevent compilation but could lead to runtime errors or undefined behavior.
Why Compiler Warnings Matter
Ignoring compiler warnings can result in subtle bugs that are hard to trace later on.
How to Address Warnings
Pay Attention: Always read compiler warnings carefully and address them promptly.
bash
# Example warning message from g++
warning: comparison between signed and unsigned integer expressions [-Wsign-conversion]
Understand Warning Types: Familiarize yourself with different types of warnings and their implications on your code’s behavior.
Strategies for Success in C++ Programming Assignments
To avoid these common mistakes and enhance your programming skills, consider implementing the following strategies:
1. Practice Regularly
Consistent practice is key to mastering C++. Work on small projects or coding exercises regularly to reinforce your understanding of concepts.
Suggested Practice Projects:
Implement basic data structures like linked lists or stacks.
Create small games such as Tic-Tac-Toe or Snake using console input/output.
Build a simple banking system that allows deposits and withdrawals while ensuring proper encapsulation.
Example Project Idea:
Creating a simple text-based banking application could involve designing classes such as Account, Customer, and methods for depositing and withdrawing funds while ensuring proper encapsulation and error handling.
Sample Code Snippet:
cpp
class Account {
private:
double balance;
public:
Account() : balance(0) {}
void deposit(double amount) {
if (amount > 0)
balance += amount;
else
throw std::invalid_argument(“Deposit amount must be positive.”);
}
void withdraw(double amount) {
if (amount > balance)
throw std::invalid_argument(“Insufficient funds.”);
balance -= amount;
}
double getBalance() const { return balance; }
};
2. Utilize Resources Wisely
Take advantage of online resources such as tutorials, forums, and documentation. Websites like AssignmentDude offer specialized help that clarifies complex topics and provides detailed explanations tailored to your needs.
Recommended Resources:
cplusplus.com: A comprehensive reference site for C++ standard libraries.
GeeksforGeeks: Offers tutorials and articles on various C++ topics.
Stack Overflow: A community where you can ask questions about specific problems you’re facing.
Online Courses:
Consider enrolling in online courses focused on C++, such as those offered by Coursera or Udemy. These platforms often provide structured learning paths along with hands-on projects that reinforce concepts learned through lectures.
3. Collaborate with Peers
Working with classmates can provide new perspectives and solutions to problems you might be facing alone. Study groups can foster collaboration and deeper understanding through discussion and shared problem-solving approaches.
Group Study Techniques:
Pair programming sessions where two students work together at one computer.
text
Student A writes code while Student B reviews it live,
providing suggestions based on best practices learned through their studies.
Group discussions focusing on specific problems encountered during assignments.
Benefits of Collaboration:
Collaborating with peers allows you to share knowledge about different approaches to solving problems while also providing motivation through accountability.
4. Seek Help When Needed
Don’t hesitate to ask for help when you’re stuck. Services like AssignmentDude are designed specifically for this purpose, offering expert guidance tailored to your needs.
Our team is ready to assist you at any stage of your learning journey — whether it’s clarifying concepts or debugging code.
How AssignmentDude Can Help:
Personalized tutoring sessions focused on areas where you struggle most.
Detailed explanations alongside solutions so you understand the reasoning behind them.
Testimonials from Students:
“I was struggling with pointers and memory management until I reached out to AssignmentDude! Their tutors explained everything clearly.”
“Thanks to AssignmentDude’s help with my last assignment, I finally understood object-oriented principles!”
5. Review and Refactor Code
After completing an assignment, take the time to review your code critically. Look for areas where you can improve efficiency or readability by refactoring your code based on best practices learned during your studies.
Refactoring Tips:
Break down large functions into smaller ones for better readability.
cpp
// Original function doing too much work at once
void processOrder(Order order) {
validateOrder(order);
calculateTotal(order);
saveToDatabase(order);
}
// Refactored version separating concerns into smaller functions
void processOrder(Order order) {
if (!validateOrder(order)) return;
double total = calculateTotal(order);
saveToDatabase(order);
}
Additional Tips for Mastering C++
As you continue your journey into C++, here are some additional tips that may help you succeed:
Embrace Debugging Tools
Learning how to effectively use debugging tools such as GDB (GNU Debugger) can greatly enhance your ability to troubleshoot issues within your code.
Debuggers allow you to step through your code line by line, inspect variables at runtime, and understand exactly where things may be going wrong.
Using GDB Example:
To start debugging with GDB:
Compile your program with debugging information:
bash
g++ -g my_program.cpp -o my_program
Start GDB:
bash
gdb ./my_program
Set breakpoints at lines where you suspect issues:
text
break main.cpp:10
run
Step through the program line by line using next or step commands while inspecting variable values using print.
This hands-on approach will help solidify your understanding as you see how changes affect program execution directly.
Explore Standard Template Library (STL)
The Standard Template Library offers a wealth of pre-built data structures and algorithms that can save you time and effort when developing applications in C++.
Familiarize yourself with containers like vectors, lists, maps, sets, etc., as well as algorithms such as sort(), find(), etc., which will make your coding more efficient.
Example Using STL Vectors:
cpp
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 2, 8, 1};
std::sort(numbers.begin(), numbers.end()); // Sorts vector
std::cout << “Sorted numbers:”;
for(int num : numbers)
std::cout << “ “ << num;
return 0;
}
Using STL effectively allows you not only to write cleaner code but also enhances performance since these implementations are optimized by experts.
Keep Learning Beyond Assignments
Consider exploring additional resources such as online courses (Coursera, Udemy), textbooks focused on advanced C++ topics (like “Effective C++” by Scott Meyers), or participating in coding competitions (like Codeforces or LeetCode). These activities will deepen your understanding beyond just completing assignments.
Recommended Books:
Effective Modern C++ by Scott Meyers — Focuses on best practices when using modern features introduced in C++11/C++14.
The C++ Programming Language by Bjarne Stroustrup — Written by the creator of C++, this book covers both foundational concepts as well as advanced topics extensively.
Conclusion
Navigating the complexities of C++ programming assignments doesn’t have to be an isolating experience filled with frustration and confusion.
With resources like AssignmentDude at your disposal, you have the opportunity to enhance your understanding and skills while avoiding common pitfalls that many students encounter along the way.
By recognizing these common mistakes and employing effective strategies for success — such as utilizing debugging tools effectively exploring STL — you’ll not only improve your grades but also develop a deeper knowledge.
#do my programming homework#programming assignment help#urgent assignment help#assignment help service#final year project help#php assignment help#python programming
0 notes
Text
Solved CS211 Homework 4 The aim of this homework assignment is to practice using a class
The aim of this homework assignment is to practice using a class written by someone else and use dynamic allocation. This homework assignment will also help build towards project 1, which will be to write a program implementing the Roster Management Program. For this assignment, write a program that uses the class Date. As an added challenge, I will not provide you with the .cpp file for this…
0 notes
Link
#c++#cpp#cplusplusprogramming#cplusplus#c++ language#c++ programming#c++ homework help#c++ tutorial#anakmales#belajarpemrograman#indonesia#development#programming#programmer
0 notes
Text
C++ Project Help
Why Is https://www.bestassignmentsupport.com/programming-assignment-help/cpp-assignment-help.php the Best Site to Get the Best Help for C++ Programming Assignment Help
It is quite obvious that when you are seeking the best Help with C++ programming assignment help with experts there are a lot of sites to avail C++ programming help with experts from and you get confused which site will be the best to get do my C++ homework. For this, you will be happy to know that https://www.bestassignmentsupport.com/programming-assignment-help/cpp-assignment-help.php serves the best C++ Help service in the field and this not just what we are saying but our customers are happy with our help with C++ programming assignment help service. We have a plethora of features with online C++help to make our online C++ programming assignment help services flexible for you like;
· 24 hours active customer support system
· Affordable rates and prices
· Loads of discounts available
· Top quality materials
· 100% plagiarism free
· No grammatical errors
· No spelling errors
· Multiple subject help
· Payment security
Who Can You Bring Your C++ Programming Assignment Help under Our Notice
You can bring the assignment under our notice by reaching us through our site service Do my C++ homework. For C++ programming help, you just need to follow a few short and very simple steps to explain your content request to us. These steps have been mentioned below; So for online C++ help services by experts do not hesitate and wait for deciding rather hurry and get your C plus plus assignment help deal settled with us and win the best grade reward!
#C++assignmenthelp#C++homeworkhelp#C++projecthelp#C++onlineexperts#C++#bestassignmentsupport.com#onlineC++helpers
1 note
·
View note
Link
Codingzap is the leading online solution provider for C++ programming assignments and homework. Get the best C++ homework help from our C++ experts. For more information visit us today!
0 notes
Text
Computer Science homework help
Computer Science homework help
Your program must provide the following functionality and adhere to the following constraints:
Your int main() should be in its own .c/.cpp file
All .c/.cpp files, except your main.c/main.cpp, must have associated header files. You may not #include a .c/.cpp
file, only header files
Allow the user to input the file containing the simulation configuration
o Do NOT hardcode the filename into your…
View On WordPress
0 notes
Text
How Much Should You Pay for Programming Homework Help Services?
ifferent platforms that offer programming homework help provide their services at different price ranges. Programming being a technical field, most agencies tend to charge expensively, some even going over the margin.
To ensure you get services worth your pay, you have to do intense web research to find the best firm to submit your “Do my programming homework” request to. Also, you can ask for recommendations from friends and colleagues. However, you should be having a rough estimate of what you expect to pay to avoid cases of being overcharged.
Most firms use certain criteria, commonly referred to as “Price Calculator,” to determine what to charge for a specific assignment help request. Most Programming assignment expert help Aussies will consider the following for pricing;
- The Urgency Of The Assignment Solutions
Generally, the more urgent your assignment is, the more you will be charged. Most scholars once assigned their task by their professor, tend to keep it aside until the last minute. It is at this point they tend to have a look at their homework. They may spend a day, still trying to crack the solutions, and the chances are that their efforts could end up futile. They may be left with one option, which is finding “programming assignment expert Aussies” agency. Since they aired their help request within a very short deadline, they end up paying higher than students who submitted their help request with enough time. We always advise scholars to have a look at their assignment as a first thing after being issued. Can you handle it? Then prepare your schedule and work on it early enough. If you find itchallenging, then you can submit your help request soon enough, saving you a couple of dollars.
- The Complexity Of The Project
Not all assignments and projects carry the same weight in terms of complexity. Also, some fields of programming are way more demanding than others. This also acts as a major determinant in fixing the quote to be issued to you. Have in mind whatever is expected of you in the assignment even before submitting your programming homework help request.
- Length Of The Assignment
Usually, the word count and the number of pages are used in determining how much to pay for help services. CPP, which stands for Cost Per Page, is a term used for this. This means that the more the number of pages your assignment solutions has, the higher the cost, and vice-versa. You can go ahead and enquire from your help provider at what rate are they charging you per page.
These are the major determinants used to calculate the assignment charges in almost every homework help firm. This includes our site, Programming Homework Helper.
We are a goal-oriented firm. Thus we offer our services, at the most reasonable prices in the market. Also, upon hiring our services, you stand a chance to enjoy great discounts at our site, now and then.
Why should you pay excessively, while you can get unmatched answers to your “Do my programming homework” request, at a friendly price? Talk to us at any time for help in programming assignment, project, and tutor services.
0 notes
Text
5 Things to Keep in Mind When Enrolling in an Online Degree Programs
The trend of online learning has taken over the world. In our busy hectic life, online degree works as a life saver for students who are not only passionate about their career but also want some time to earn for living.
Therefore, before going for an online degree, here are some basic points you should keep in mind.
1. Don’t go for a Worthless Online Degree
Your money is worth everything. Remember, you are going for an online degree to save your time not to waste your money on any low quality or say fake university. Hence, before choosing your university, make sure that it must be accredited. Accreditation from an organization is the first and foremost thing you should keep in your mind. Trust me, this is one of the aspects your future boss will not be settled with.
To earn an accreditation, a university or school must meet some certain guidelines or standards set by a particular accrediting organization. By a certified accreditation, you can easily know whether your university is officially authorized or not. This is the only way of knowing that your online degree has some value.
This is for your benefits, your education, your money and above all this is for your career we are talking about. So if you have any doubt, don’t just sit. Go for the research and assure yourself.
2. Your friends are not Ahead of You!
My friend is going for a regular degree and I am getting mine just by sitting at home. Will he get a better education and much more knowledge than me?
This will be the very first question that would strike my mind before planning to take an online degree. According to a research, there is no significant proof or valid evidence to show that online education is qualitatively or quantitatively any less valuable than traditional classroom education. In fact, a study shows that students who prefer online learning tend to give more time to self-study and that directly lead to better results.
However, there is no doubt that helping hand and guidance of a classroom professor can change your life upside down. This is the only benefit you will not be able to take from online learning, but not having a bad company of college seniors or peer pressure is the advantage you are going to be blessed with.
3. You are not alone!
Believe me, you are not the only one to give preference to online learning. You don’t need to feel inferior or caught between two stools for choosing to take an online degree. Though online education is not well known in India, but according to a survey, approximately one-third of the teenagers in the USA choose online degree over a regular one.
Moreover, 80% of accredited institutions in India and the world are now offering an online platform to connect with the students. All the elite institutions ranging from Harvard University, MIT, Caltech, LSE, Oxford, Cambridge, NUS, Australian National University, Monash University, ETH Zurich, Stanford university, University of Tokyo, Universiti Malaya, Qatar University, Zayed University Dubai and Abu Dhabi, all of them and hundreds of others are giving online classroom access. The percentage of students taking online degree is increasing rapidly due to the facilities and money saving schemes universities and schools provide.
So keep running! You are in still in the race…
4. You can save for a World Tour
Yes! It’s true. You can seriously save money even for a world tour. I’m not talking about just tuition fees; you can save your money from many other things also. Okay, let’s calculate.
Do you have your own transport for going to college? No? Write your daily transportation cost on the list.
Are you planning to go abroad for further studies? Yes? Write your hostel rent in your list.
Can you cook? No? Add some more bucks in the list.
College parties, college functions, get together and the list goes on and on. So think of it. How much you can save just by your single right decision. On the top of it, you can save time too!
But wait! There are some online universities which charge twice or thrice as high as those of regular public institutions. Don’t go crazy over fancy universities that pops-up on your screen. Make sure to choose a college wisely to avoid incurring unnecessary debt and yes of course to enjoy your trip.
5. Future! Future! Future!
You are doing everything for your bright future and a strong and successful career. So your major concern should be your future and your future depends on the reputation of the university to choose. The reputation of your university is really important from the perspective of your future employment. In India, online education is still a new program and you should not trust a university which is still struggling in reaching the standards of a certified and prominent education hub. Look for a university with at least 5-6 years of experience in online education, a well-known brand name, best online teaching methods, good learning environment, program diversity, on demand class availability, top-class online resources and a reputation that is worth enough investing your valuable money.
Make your search goal oriented. Don’t just go with the flow; think about your future before choosing a university.
Have you enrolled in an Online University yet ?
p.s. If you are looking for best online tutors to help you study for online degree and certification programs from the comfort of your home, then do check out www.assignmenthelp.net
Assignmenthelp.net offers best and affordable online homework help and assignment help service for students from all universities and colleges around the world. They have the best academic tutors and custom essay writers for all subjects, including, Accounting, Finance, Economics, Management studies, business majors, statistics, Perdisco, MYOB, STATA, SPSS, Eviews, computer science, programming languages like JAVA, Python, R, CPP, NLP, machine learning and science courses like Physics, Chemistry, Biology, Maths and engineering subjects
#online degree#online degree programs#online certifications#study abroad#study online#Ivy league#MOOCs#open courses#university#college#student life#student problems#online homework help#online assignment help#assignment help tutors#UK tutors#USA tutors#UAE tutors#Australia tutors online#assignmenthelp.net reviews
2 notes
·
View notes
Text
Three behavioural barriers to solid retirement planning, and how you can overcome them
I read with great interest a recently released Ontario Securities Commission (OSC) report entitled Encouraging Retirement Planning through Behavioural Insights. It provides strategies for governments, employers, financial advisers and those planning for retirement to implement to make it easier to become financially independent.
Behavioural finance has risen to prominence recently, in part because of the work of “nudge” economist Richard Thaler, who won the Nobel Prize last year for his pioneering work in the field of behavioural economics. There can be psychological impediments to making financial decisions that are in our own best interest, but there are simple things we can do to encourage behaviour today that leads to better outcomes tomorrow.
The OSC and the behavioural insights team that prepared the report identified several barriers to retirement planning and provided potential remedies. I have narrowed down and elaborated upon my favourites.
How much money will you need after you retire? Likely less than you think
How you draw down your retirement savings could save you thousands — this program proves it
What today’s savers can learn from today’s seniors about retirement planning
Barrier 1: People will avoid making a retirement plan because of the perceived length and complexity of the process.
I have experienced this first-hand. We give our clients homework to do up-front before we begin to work with them. Sometimes it takes months and sometimes they do not complete it at all.
The report suggests that governments, financial advisers and employers provide a template to people to create a retirement plan with some data already completed. I can think of a few ideas.
Service Canada could include a templated retirement planning tool with Canada Pension Plan (CPP) Statement of Contribution mailouts or Old Age Security (OAS) applications. This could include data from other government agencies like Statistics Canada or Canada Revenue Agency on retiree spending and income. While information about “average” retirees is imperfect given everyone is different, having general data on low, middle and high-income retirees could provide insight for near-retirees to apply to their personal situation.
Investment advisers or financial institutions could provide a retirement planning questionnaire pre-populated with existing information about the client, their family, their accounts, etc. This way, the retirement planning process would already be underway.
Employers have information about their employees, their salaries, their net payroll deposits, their pensions and their benefits. It is similarly feasible for human resources to pre-populate a retirement plan with this existing data.
Individuals engaging in retirement planning — on their own or with a professional — should aim to break the process down into manageable steps rather than trying to do it all at once. Start by developing a budget or spending summary; then identify retirement income sources; and finally estimate retirement funding using online resources or by engaging a retirement planner.
Barrier 2: People tend to go with the default option, and the default is not to plan for retirement.
One suggestion from the OSC report is for employers to make retirement planning part of their new hire on-boarding process. Financial institutions and advisers could do the same with new clients. By providing a standard template, they could expedite the data collection process and strike while the iron is hot with a new employee or client. Employers or financial advisers would then pre-book an appointment with a retirement planner to develop the plan.
This would be a great way for employees to know their employers are committed to their financial and overall well-being. For financial institutions and advisers, it is a clear way to ensure the relationship is about more than just a product sale.
A further intervention for employers would be to schedule an employee retirement planning meeting during the workday. This way, an employee would have to choose to opt out rather than needing to take the initiative to book an appointment on their own. Since many employees have spouses or partners, their exclusion from a workday appointment may not be ideal, but there are options like joining by phone or web conference if they could not participate in person.
Governments incentivize certain behaviours or subsidize certain costs using tax savings. Saving for retirement — RRSP contributions — are tax deductible. Child care expenses for working parents — daycare or a nanny — are tax deductible. Retirement planning fees are not tax deductible — unless paid by an employer for an employee. Perhaps this is something Finance Minister Morneau should consider for the next federal budget.
Barrier 3: The idea of retirement planning can bring on strong negative emotions and people may put it off to avoid those emotions.
The retirement planning process could be co-ordinated to coincide with times when someone is likely feeling more positive about their finances. For employers, this might be when an employee has just received a promotion or bonus. These events should also prompt individuals to put some thought to retirement.
For a financial institution or adviser, a good time might be when a client has just received a windfall like an inheritance or asset sale. I can share from experience that clients are turned off by phone calls from the bank branch to come in and talk about investments when there has been a large deposit to a bank account. It comes across as disingenuous and self-serving, whereas a phone call to develop a retirement plan to help make decisions about the windfall may be better received and more beneficial for the client.
Although the Canada Revenue Agency may not be best suited to give retirement advice, along the same vein would be a prompt about RRSPs, TFSAs or retirement statistics when issuing a tax refund above a certain threshold.
Other ideas in the Ontario Securities Commission report include sending prompts on a birthday, particularly a round number birthday; using regret lotteries, where people must participate and complete a retirement plan to be entered to win a prize; and using messages appealing to the ego, such as “you have been selected to receive this free retirement planning tool/resource/service.”
I applaud the OSC for commissioning the behavioural insights team report, and encourage governments, employers, advisers and individuals to consider some of the best practices to engage citizens, employees, clients or yourself to plan for retirement. It can be a daunting task, but with a little nudge in the right direction, everyone can be more motivated to develop a retirement plan.
Jason Heath is a fee-only, advice-only Certified Financial Planner (CFP) at Objective Financial Partners Inc. in Toronto, Ontario. He does not sell any financial products whatsoever.
from Financial Post https://ift.tt/2Bskr4H via IFTTT Blogger Mortgage Tumblr Mortgage Evernote Mortgage Wordpress Mortgage href="https://www.diigo.com/user/gelsi11">Diigo Mortgage
0 notes
Text
A CPP homework help service offers expert guidance and support to students struggling with CPP programming assignments. With experienced tutors and comprehensive coverage of CPP topics, it provides customized solutions tailored to individual requirements. Timely delivery, plagiarism-free work, and 24/7 availability ensure efficient assistance and customer satisfaction.
0 notes
Link
Get thoroughly commented and laid code solutions for your C++ assignment that will get you the grade you deserve.
0 notes
Text


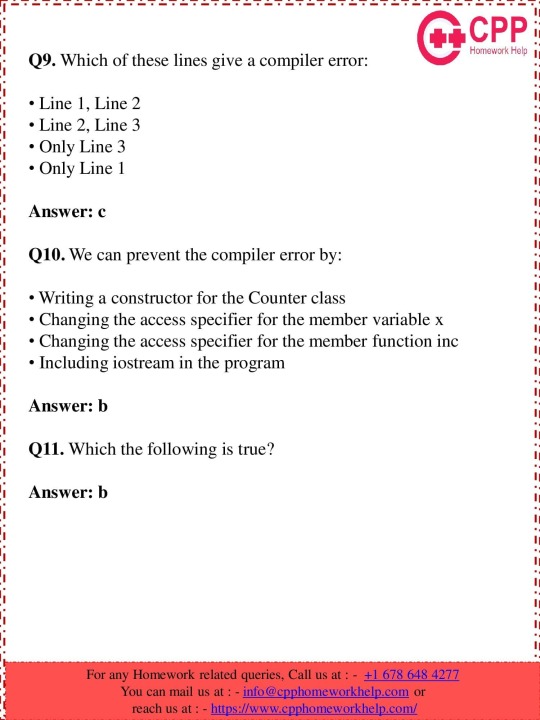
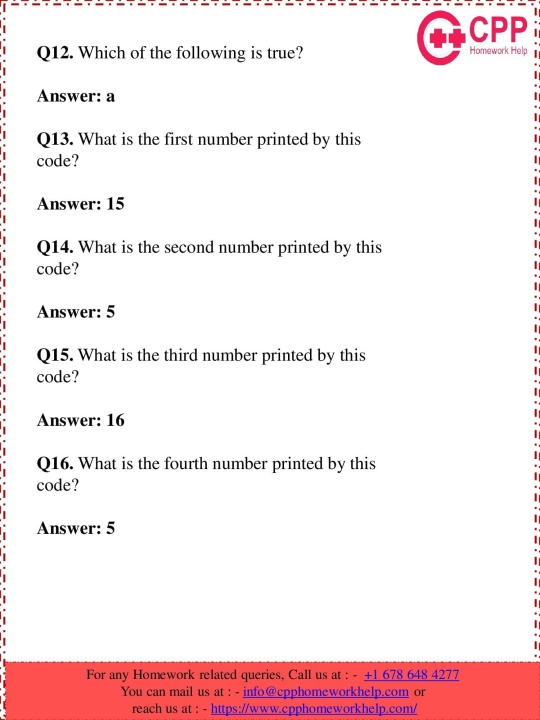
I am Patricia Jones. I love exploring new topics. Academic writing seemed an exciting option for me, after working for many years with cpphomeworkhelp.com. I have assisted many students with their C++ homework. I can proudly say, each student I have served is happy with the quality of the solution that I have provided. I acquired my bachelor's from Rice University, USA.
#cpp homework help#assignment help#homework help#education#tutor#online education#acedemics#university#cpp programming homework help#cpp assignment help#cpp programming assignment help
0 notes
Text






I am Rose Tom. Currently associated with cpphomeworkhelp.com as a CPP tutor. After completing my master's from the University of Brighton, UK. I was in search of an opportunity that would expand my area of knowledge hence I decided to help students with their C++ programming homework. I have written several CPP homework to date to help students overcome numerous difficulties they face.
#cpp assignment help#cpp homework help#assignment help#homework help#education#tutor#cpp programming assignment help#cpp programming homework help#online education#university#acedemics
0 notes
Text







I am Jeremy P. I am a C++ Programming Homework Help Expert at cpphomeworkhelp.com. I hold a Masters in Programming from Loughborough University, UK. I have been helping students with their homework for the past 5 years. I solve homework related to C++ Programming . Visit cpphomeworkhelp.com or email [email protected]. You can also call on +1 678 648 4277 for any assistance with C++ Programming Homework.
#cpp assignment help#cpp homework help#assignment help#homework help#education#tutor#online education#cpp programming assignment help#cpp programming homework help
0 notes
Text






I am Walter G. I am a C++ Programming Homework Help Expert at cpphomeworkhelp.com. I hold a Masters in Programming from Oxford University, UK. I have been helping students with their homework for the past 8 years. I solve homework related to C++ Programming. Visit cpphomeworkhelp.com or email [email protected]. You can also call on +1 678 648 4277 for any assistance with C++ Programming Homework.
#cpp assignment help#cpp homework help#assignment help#homework help#education#tutor#cpp programming assignment help#cpp programming homework help#online education
0 notes
Text





I am Irene M. I am a C++ Programming Homework Help Expert at cpphomeworkhelp.com. I hold a Masters in Programming from California, USA. I have been helping students with their homework for the past 6 years. I solve homework related to C++ Programming. Visit cpphomeworkhelp.com or email [email protected]. You can also call on +1 678 648 4277 for any assistance with C++ Programming Homework.
#cpp assignment help#cpp homework help#assignment help#homework help#education#tutor#cpp programming assignment help#cpp programming homework help#online education
0 notes