#dropzone js laravel
Explore tagged Tumblr posts
Text
0 notes
Text
#websolutionstuff#laravel#laravel9#laravel8#laravel7#php#laravel6#example#jquery#bootstrap#html#file upload#drag and drop#dropzone js
0 notes
Text
#techsolutionstuff#laravel#laravel6#laravel7#laravel 8#php#example#jquery#bootstrap#html#drag and drop#file upload#dropzone js
0 notes
Text
Laravel 7.x, 6.x Tutorial Mengunggah Gambar Dropzone
Laravel 7.x, 6.x Tutorial Mengunggah Gambar Dropzone
Laravel 7 dan 6 mengunggah gambar menggunakan tutorial dropzone js. Dalam tutorial ini, kami ingin membagikan kepada Anda bagaimana Anda dapat menggunakan dropzone untuk mengunggah satu atau beberapa gambar di Laravel. Tutorial contoh ini juga berfungsi dengan laravel 7.x versi.
Tutorial ini menunjukkan kepada Anda hal-hal langkah demi langkah untuk mengunggah gambar menggunakan dropzone di…
View On WordPress
0 notes
Photo
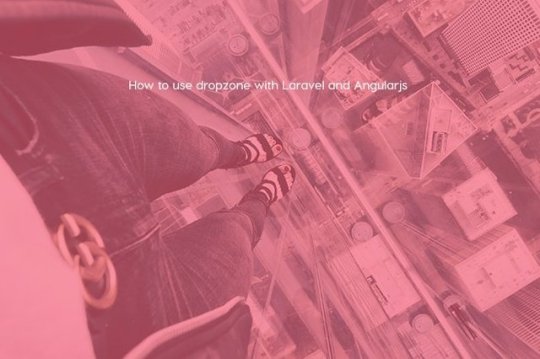
How to use dropzone with Laravel and Angularjs https://t.co/IPhJL0zgub #laravel #php #dev #html5 #js #coder #code #geek #angularjs #coding #developer #vue #vuejs #wordpress #bootstrap #lumen #angular6 https://t.co/aspWlzVFIt
0 notes
Photo
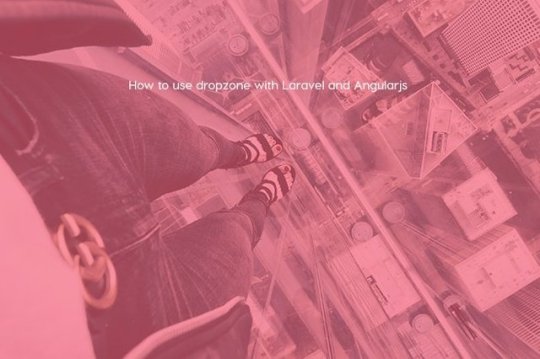
How to use dropzone with Laravel and Angularjs https://t.co/IPhJL0zgub #laravel #php #dev #html5 #js #coder #code #geek #angularjs #coding #developer #vue #vuejs #wordpress #bootstrap #lumen #angular6 https://t.co/aspWlzVFIt #Laravel #PHP
0 notes
Photo
New Post has been published on https://programmingbiters.com/file-uploads-with-laravel-5-and-dropzone-drag-and-drop/
File Uploads with Laravel 5 and Dropzone drag and drop
File uploads with Laravel is incredibly easy, thanks to the powerful file systemabstraction that it comes bundled with. Couple that up with Dropzone’s drag and drop feature, and you have a robust file upload mechanism. Not only these are easy to implement, they provide a great number of features in very few lines of code.
# Setting up the uploads
We’ll create a database table that contains information about each of the uploads. Of course we are not going to store our file in the database itself, rather we’d store only some metadata of the file. So let’s create a schema.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
public function up()
Schema::create(‘uploads’, function (Blueprint $table)
$table->increments(‘id’);
$table->integer(‘user_id’)->unsigned()->index();
$table->string(‘filename’);
$table->bigInteger(‘size’);
$table->softDeletes();
$table->timestamps();
$table->foreign(‘user_id’)->references(‘id’)->on(‘users’)->onDelete(‘cascade’);
);
Here only metadata we are storing are filename and size . filename would be used to store and retrieve the file later. We add a foreign keyuser_id to keep track of who uploaded the file. This can also be used to check the owner of a file. We are also going to use SoftDeletes to mark a file as deleted rather than actually delete the file from storage, when a user deletes a file.
Now, let’s php artisan make:model Upload to create a Upload model for this table.
Next, we define the relationship between a user and an uploaded file – a user may upload many files and each uploaded file will belong to a user. Therefore, let’s define a one-to-many relationship in our User and Upload models. In theUser model:
1
2
3
4
5
6
public function uploads()
return $this->hasMany(Upload::class);
and the inverse relationship is defined in our Upload model:
1
2
3
4
5
6
public function user()
return $this->belongsTo(User::class);
Also while we are at it, let’s update the fillable columns for our Upload model.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
use Illuminate\Database\Eloquent\SoftDeletes;
use App\User;
class Upload extends Model
use SoftDeletes;
protected $fillable = [
‘filename’, ‘size’,
];
public function user()
return $this->belongsTo(User::class);
That’s all! Our Upload model is now ready.
# Setting up dropzone
Now that our uploads backend is ready, let’s setup dropzone for drag and drop file uploads.
First we need to install dropzone, so let’s do a npm install dropzone —save . Next, we need to load the dropzone library. Since we are going to use Laravel Mix to compile our frontend assets, we can load the library withinresources/assets/js/bootstrap.js :
1
2
3
4
5
6
// Dropzone
window.Dropzone = require(‘dropzone’);
Dropzone.autoDiscover = false;
This will set Dropzone to the window object. We are disabling the autoDiscover option, which is used to automatically attach dropzone with any file input, which we do not need.
Now let’s add the styling for this (otherwise it’s going to look uglier than Voldemort’s face, trust me). We can do this in ourresources/assets/sass/app.scss .
1
2
3
4
5
6
7
8
@import “~dropzone/src/dropzone.scss”;
.dropzone
margin-bottom: 20px;
min-height: auto;
All set. So we can now compile the assets using npm run dev .
# Setting up routes
Before we start uploading any files, we need to define some routes. Here we’d create two routes, one for receiving the uploads and one for deleting the uploads, should a user choose to delete one.
1
2
3
4
5
Route::post(‘/upload’, ‘UploadController@store’)->name(‘upload.store’);
Route::delete(‘/upload/upload’, ‘UploadController@destroy’);
Let’s php artisan make:controller UploadController to create the controller. We will use this UploadController to process the uploads in the backend.
# Uploading files
Now that we have pulled in dropzone and attached it to the window object, we need to integrate dropzone within a view to start file uploads. We can simple do this using an id tag.
1
2
3
<div id=“file” class=“dropzone”></div>
class=“dropzone” is used to style the dopzone upload box. We can use the#file to inject dropzone on this element. (tip: it is a best practice in laravel to create javascript snippets in separate partials and then including them in whichever blade template they are needed)
1
2
3
4
5
6
7
8
9
let drop = new Dropzone(‘#file’,
addRemoveLinks: true,
url: ‘ route(‘upload.store‘) ’,
headers:
‘X-CSRF-TOKEN’: document.head.querySelector(‘meta[name=”csrf-token”]’).content
);
We are instantiating the Dropzone class on our #file and then passing in some addition configuration. addRemoveLinks will add a remove link to every uploaded file, so a user can remove a file after uploading it. The url option is used to let dropzone know where to post the uploaded file to, in case we are not using a form. If we are using a form, by default dropzone would use the action attribute of the form as the post url (I’m not quite sure about this though, so please don’t take my words as is, and do correct me if I am wrong). We are using the upload.store route which we have defined earlier.
headers are used to send additional headers to the server. Because we are posting our uploads, we need to send a X–CSRF–TOKEN header, otherwise our app will throw a TokenMismatchException (this is used by laravel to protect our app against CSRF attacks). By default, the meta information in head contains a meta with the name csrf–token . Therefore we select the meta and extract the content which gives us the token .
Dropzone provide’s a huge number of configuration options. Check out the documentation for all the available options. (Also don’t forget to thank the developer of dropzone )
Now that we are able to post files from the frontend, let’s work on our controller to receive and process the uploads on backend.
In the UploadController , let’s define a method storeUploadedFile() which receives a Illuminate\Http\UploadedFile and store all the metadata in our database.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
protected function storeUploadedFile(UploadedFile $uploadedFile)
$upload = new Upload;
$upload->fill([
‘filename’ => $uploadedFile->getClientOriginalName(),
‘size’ => $uploadedFile->getSize(),
]);
$upload->user()->associate(auth()->user());
$upload->save();
return $upload;
getClientOriginalName() gives us the original name of the file that has been uploaded. getSize() returns the size of the uploaded file in bytes. We thenassociate() the upload with the currently logged in user. Finally we save the file and return the model instance.
In the store() method, we accept a Request and then from that request, we extract the file.
1
2
3
4
5
6
public function store(Request $request)
$uploadedFile = $request->file(‘file’);
We are going to store this file in our local filesystem. Of course you can upload it to Amazon S3 or any other cloud storage services and laravel provides an excellent documentation on how to configure different storage services. However, I’m going to store the uploads on our local disk only.
Next we need to save the file metadata like name, size and the user who uploaded it to our database. For this, we can use thestoreUploadedFile() method we implemented above.
1
2
3
$upload = $this->storeUploadedFile($uploadedFile);
This returns us an instance of the Upload model. We can now use this to store the file.
1
2
3
4
5
6
7
Storage::disk(‘local’)->putFileAs(
‘uploads/’ . $request->user()->id,
$uploadedFile,
$upload->filename
);
The Storage facade is used to interact with the disk in which we are going to store the file. Storage::disk(‘local’) gives us an instance of the local disk. The default local storage root is storage/app .
putFileAs() method is used to automatically stream a given file to a storage location. The first argument ‘uploads/’ . $request->user()->id is the upload path. We are creating a new directory uploads and within that directory creating another directory using user’s id and storing the file there, so that all files uploaded by a user goes to the same location. For example, if a user’s id is 7, any file he uploads will be stored at storage/app/uploads/7/ .
The second argument in putAsFile() method is theIlluminate\Http\UploadedFile instance and the last argument is the filename that will be used to store the file. Because at this point, we already have stored the file’s metadata in our database, we can simply get the name of the file by $upload->filename .
At this point, file storing is complete. Finally we send back a JSON response with the uploaded file’s id.
1
2
3
4
5
return response()->json([
‘id’ => $upload->id
]);
That’s all. The file has been stored on our local disk.
# Deleting files
To remove a file, Dropzone provides a removedfile event, which we can listen to and then send a delete request. We will use axios to send the request.
We can register to any dropzone event by calling .on(eventName,callbackFunction) on our dropzone instance. Check this documentation for a list of different dropzone events and when they are triggered.
Now we need to use the id of the uploaded file in order to send the delete request. But from the frontend how could we possibly know what’s the id of a uploaded file? Well, this is where the JSON response from thestore() method is useful. When we uploaded a file successfully, we are sending back the id of the file from the backend. On a successful upload, we can therefore associate this id with the file on the frontend.
1
2
3
4
5
drop.on(‘success’, function(file, response)
file.id = response.id;
);
When a file has been uploaded successfully, dropzone triggers a success event. We can register to this event to get the file instance and theresponse from the backend inside the callback function. We simple assign theresponse.id to file.id . Therefore, for every file that we have successfully uploaded, now we have an identifier associated with it to be used on the frontend. Great!
Now that we have an id associated with each file, deleting files is easy. We listen for removedfile event and then use axios from the global window object to fire a delete request to the backend.
1
2
3
4
5
6
7
8
9
10
11
drop.on(‘removedfile’, function(file)
axios.delete(‘/upload/’ + file.id).catch(function(error)
drop.emit(‘addedfile’,
id: file.id,
name: file.name,
size: file.size,
);
);
);
Notice how we are chaining the catch() method. This to catch any error when removing a file. If an error occurs that prevented the file deletion, we want to add it back so that the user knows the deletion failed and they may try again. We do that simply by calling the emit() method and passing in the details of the file. This will call the default addedfile event handler and add the file back.
Okay. So our frontend is ready to delete files. Let’s start working on thedestroy() controller method.
Because we are injecting the file id on our delete request, we can therefore accept the file we trying to delete , destroy(Upload $upload) , using laravel’s route model binding. The next thing we need to do is verify if the delete request is actually coming from the owner of the file. Let’s create a policy,UploadPolicy for that.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
use App\User, Upload;
use Illuminate\Auth\Access\HandlesAuthorization;
class UploadPolicy
use HandlesAuthorization;
public function touch(User $user, Upload $upload)
return $user->id === $upload->user_id;
In the touch() method, we are checking if the ids of the user who uploaded it and the user who is sending the delete request is same. In our destroy() method, we can now use this touch() method to authorize the request.
1
2
3
$this->authorize(‘touch’, $upload);
Finally we can delete the file.
1
2
3
4
5
6
7
8
public function destroy(Upload $upload)
$this->authorize(‘touch’, $upload);
$upload->delete();
Since we are using SoftDeletes , this will mark the file as deleted in the database.
That’s all folks.
The complete UploadController looks like this.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
<?php
namespace App\Http\Controllers;
use Storage;
use Illuminate\Http\Request;
use Illuminate\Http\UploadedFile;
use App\Upload;
class UploadController extends Controller
public function __construct()
$this->middleware(‘auth’);
public function store(Request $request)
$uploadedFile = $request->file(‘file’);
$upload = $this->storeUploadedFile($uploadedFile);
Storage::disk(‘local’)->putFileAs(
‘uploads/’ . $request->user()->id,
$uploadedFile,
$upload->filename
);
return response()->json([
‘id’ => $upload->id
]);
public function destroy(Upload $upload)
$this->authorize(‘touch’, $upload);
$upload->delete();
protected function storeUploadedFile(UploadedFile $uploadedFile)
$upload = new Upload;
$upload->fill([
‘filename’ => $uploadedFile->getClientOriginalName(),
‘size’ => $uploadedFile->getSize(),
]);
$upload->user()->associate(auth()->user());
$upload->save();
return $upload;
# Conclusion
There can be so many different ways of implementing file uploads. Dropzone’s documentation includes an example section that have quite a few in-depth excellent examples. Don’t forget to check that out!
0 notes
Text
Multiple Image Uploading using Dropzone js in Laravel - Learn Infinity
Multiple Image Uploading using Dropzone js in Laravel – Learn Infinity
[ad_1]
We generally need to do pictures transferring in our laravel application, In this post, I give you case code of how to transfer different pictures utilizing dropzone.js. dropzone.js through we can make picture transferring basically.
Dropzone.js is a jquery module, through this we can choose one or many picture and furthermore with see. After pick picture, we can see the review of the…
View On WordPress
0 notes
Text
ngLaravel - CRUD angulaire et Laravel API REST sur JWT + rôle Permiss... Script Développeur comparatif 2017
New Post has been published on http://www.developpeur.ovh/1330/
ngLaravel - CRUD angulaire et Laravel API REST sur JWT + rôle Permiss...
ngLaravel vue d’ensemble
Yep ngLaravel inclus CRUD (Create, lire, update et delete) basé sur l’API REST (Laravel 5.1.x & 5.3.x) avec AngularJS. Avec ce script, vous pouvez créer facilement une seule Application Web sécurisée. ngLaravel sécurisé en Back-End(Laravel) et en Front-End(AngularJS) issu de JWT. Vous pouvez également utiliser back-end(Laravel) code comme serveur dans l’application mobile.
JWT sont une norme car les informations qu’ils véhiculent sont transmises par l’intermédiaire de JSON. JSON Web jetons travailler dans différents langages de programmation et JWTs sont autonomes et peuvent être passées autour facilement.
dans ce script, vous pouvez définir autorisations personnalisée et puis associez-la au rôle et enfin attribuer de rôle d’utilisateur. Vous pouvez définir un accès à des parcours spécifiques (AngularJS et Laravel API) en permission. Quand une ouverture de session utilisateur, un jeton est généré pour lui. Ce jeton inclut toutes les autorisations utilisateur et son profil. Avec les autorisations incluses dans le jeton, vous pouvez afficher ou masquer certaines sections dans votre view(HTML).
également, vous pouvez utiliser un drag et drop uploader ajax pour téléverser des photos et rien.
nous avons ajouté le module des tâches comme échantillon. Vous pouvez personnaliser pour n’importe quel modual comme produit, article et etc.
exigence
PHP > = 5.5.9
Extension OpenSSL de PHP
PDO PHP Extension
Mbstring PHP Extension
Extension de PHP Tokenizer
version plus récente du compositeur
caractéristiques ngLaravel
Laravel 5.1.x & 5.3.x Base cadre
AngularJS 1.4.8
supplémentaires Bootstrap Admin Template avec 50 + plugins [ApplicationWebunique(YepGolabiAdmin)
connexion / s’inscrire avec l’email de confirmation adresse
réinitialiser le mot de passe
Profil
Web Assistant nglaravel installateur
Ajouter/modifier/supprimer/lecture/recherche/recherche utilisateurs
Ajouter/modifier/supprimer/lecture/recherche/recherche rôles
ajouter/lire/recherche/pagination autorisations utilisateur (disable supprimer & modifier des actions en raison du changement dans la logique du programme)
Ajouter/modifier/supprimer/lecture/recherche/recherche opérationnelle
Ajouter/modifier/supprimer/lecture spéciale catégorie
Ajax upload/supprimer avatar
Ajax multi uploader/supprimer l’image
Unified access permission les deux en back-end et Front-End
Access control API Back-End Laravel par utilisateur permission
définir l’accès pour n’importe quel état ui-route par utilisateur permission
définir l’accès pour toutes les sections du mode html par autorisation de l’utilisateur avec speacial directives
générer fil d’Ariane d’ui-routeur
charger la Bibliothèque CSS & JS comme Base (LazyLoad)
à la demande sur Restangular & UI-routeur & oclazyLoad
Base sur bootstrap 3.x
conception sensible (mobile, table, bureau)
bien Documention ressource vidéo
Golabi Admin – supplémentaires Bootstrap Admin Template Overview
Golabi Admin modèle est un modèle de web basé sur les données d’amorçage. Tous les éléments inclus dans ce modèle de tableau de bord a été développé pour apporter tout le potentiel du HTML5 et Bootstrap et ensemble de nouvelles fonctionnalités (JS et CSS) idéales pour votre prochain tableau de bord admin thème ou admin web app projet.
il est livré avec un tas de plugins tiers et éléments utilisables tels que des boutons, miniatures, objets multimédias et bien plus encore et il a été développé pour tous les types d’applications web comme panneau d’administration personnalisé, tableau de bord admin, application backend, CMS, CRM, site Web de l’entreprise, entreprise, portfolio, blog etc.
Golabi Admin caractéristique
construite sur dernières jQuery 1.11.2
Présentation sensible (ordinateurs de bureau comprimés, appareils mobiles)
RTL version
version
AngularJS version HTML
construit avec nouveau Bootstrap 3.3.x
UI plat avec propre style
SASS & moins fichiers inclus
Affichage Boxed, fluide vue
Fixed-View (Include fixe-en-tête, fix-pied de page, fix-sidebar)
Top-menu disposition
plusieurs encadrés (offcanvas)
Tab Sidebar avec recherche sur demande
Animations CSS3
Web documentation
divers et des styles adaptés de tables et tables de données
Notifiction à l’intérieur de l’onglet
Multiple
panneaux Draggable
petit chat
Sidebar dragable tâche widget
Sidebar contact cutané avec recherche
Sidebar rapetisser mode
Fullscreen mode option
tableau de bord style « design »
typographie
onglet & accordéon vue personnalisée
jsTree vue
jQuery UI thème
liste emboîtable
double liste
Image culture
menu de barre latérale niveau 4
3 bibliothèques graphiques différents
forment validation
formulaire Assistant validation
formulaire plugin (Include icône-sélecteur, masque de sélecteur de dates/temps, pipette, x-modifiables,…)
puissant éditeur
Dropzone file upload multiple
vecteur de multiples uploader de fichier ajax
carte
API Google map
boîte aux lettres (Include composer, liste de courrier, affichage courrier)
Galerie vue
Calendar view (événement Add et remove)
chronologie vue
profil utilisateur vue
Login et Registre vue
2-Step
recherche page
erreur page vérification (400 page d’erreur 500)
et bien d’autres …
ngLaravel – IONIC Client Mobile App
ionique
est un frontal SDK pour le développement d’applications mobiles multi-plateformes. Construit au sommet de l’angulaire, Ionic fournit également une plate-forme d’intégration des services comme les notifications push et analytique. Ionique n’est pas une option alternative à Cordoue, mais plutôt une bibliothèque d’interface utilisateur pour faire un meilleur projet de Cordova. Ionique peut être comparé à un cadre comme Bootstrap ou Fondation, mais cette fois pour Mobile et pas de Web.
sécurité application cliente ionique comme ngLaravel web app est basée sur le jeton à Web JSON (JWT) et vous pouvez utiliser les mêmes autorisations de groupe pour contrôle d’accès dans l’application mobile. Aussi dans cette application mobile vous pouvez vous connecter au périphérique mobile pour obtenir les données comme naturellement.
ici pour télécharger ionique Mobile Client App (.apk) version android nom d’utilisateur par un clic : [email protected] mot de passe : 123456
ionique Mobile Client App caractéristique :
autorisation de contrôle d’accès basé sur Laravel dorsal API
action de CRUD basée sur Laravel dorsal API
CRUD SQLite DB sur appareil Android
prendre photo de caméra Mobile
Barcode Scanner
appareil Mobile vibration
Get device info
envoyer des SMS
Ajouter Contact et se connecter à contacts mobiles
Local push notification
Multi image uploader
Multi sélectionnez auto complet
et plus …
en cours
Upgarde à 5,3 Laravel (Now Available)
enregistrement de l’utilisateur (Now Available)
ajouter le profil utilisateur et Laravel back-end toile installer (Now Available)
Ajouter ionique Mobile App de travailler avec ngLaravel (Now Available)
direction multilingues & changement pour RTL langauage (Now Available)
exportation CSV & XLS (Now Available)
importation CSV & XLS (Now Available)
Change Log
ngLaravel 2.0 (2017-01-08) - mise à niveau Laravel 5.1 à 5.3
ngLaravel 1.7.5 (2016-11-30) - Sweetalert de mise à niveau à la version sweetalert2 v4.2.5 - amélioration pour NG-PLMB - Difficulté certaines questions
ngLaravel 1.7.1 (2016-09-25) - Ajouter l’enregistrement de l’utilisateur - Difficulté active & désactiver utilisateur question
ngLaravel v1.6.1 (2016-09-13) - ajouter un profil de l’utilisateur - Ajouter Assistant de configuration pour ngLaravel dorsal - question Difficulté LTR et RTL dans safari
ngLaravel v1.5.2 (2016-08-09) - Fix namespace questions au serveur linux (ne fait pas de connexion au serveur linux)
ngLaravel v1.5.1 - version ajouter ionique Client ngLaravel - ionique v1.3 - directive de secours ajouter image source - Difficulté Access-Control-Allow-Origin dans laravel-backend
ngLaravel v1.3.6 - Fix recherche en cause liste utilisateur - Fix recherche & par page activer/désactiver la question
ngLaravel v1.3.4 - ajouter la fonctionnalité de langage multi avec changement de direction pour les langues RTL - ajouter et imporove notification - ajouter angulaire charge dynamique locale - ajouter modulaire chargement partiel langue fichier de module
ngLaravel
v1.2.3 - Ajouter cacheFactory - Assistant Ajouter une importation de fichier XLS & CSV - ajouter Exportation XLS & CSV et PDF - améliorer charge page vitesse avec méthode cache - Difficulté tâche supprimer problème comme promis - mot de passe utilisateur Fix en edit mode
Version v1.1.3 - Ajouter preloader dans la page de connexion - ajouter preloader dans stateChange - restriction de version démo Ajouter - Ajouter charger des données avant ouverture page - améliorer la documention et ajouter vidéo tutoriel - numéro d’édition spéciale Difficulté - Fix utilisateur liste aperçu questions - Fix modifier avec multi uploader
[Galeried’images
version 1.0 - version initiale
Voir sur Codecanyon
ngLaravel - CRUD angulaire et Laravel API REST sur JWT + rôle Permiss...
0 notes
Text
#websolutionstuff#laravel#laravel8#laravel7#laravel6#php#jquery#example#bootstrap#html#dropzone#drag and drop#files#file upload#dropzone js
0 notes
Text
Laravel 7.x, 6.x Dropzone Upload Image Tutorial
Laravel 7 and 6 upload images using dropzone js tutorial. In this tutorial, we would love to share with you how you can use the dropzone to upload single or multiple images in Laravel. This example tutorial also works with laravel 7.x version.
This tutorial shows you things step by step for uploading the images using dropzone in laravel.
Laravel Upload Image Using Dropzone js Tutorial With Example
View On WordPress
0 notes