#getdata
Explore tagged Tumblr posts
Text
Handling Asynchronous Responses in JavaScript: A Guide to Returning Data In modern web development, asynchronous operations are a fundamental part of working with JavaScript. Whether you're fetching data from an API, reading a file, or managing timers, JavaScript often needs to wait for a task to complete without freezing the entire application. This is where asynchronous programming shines. However, handling asynchronous responses—and returning the data when needed—can be tricky for newcomers. Over the years, JavaScript has evolved from callback functions to Promises, and now to the more modern and readable async/await syntax. This guide will walk you through the key techniques for handling and retrieving data from asynchronous operations using JavaScript Promises, async/await, and callback functions, complete with code examples and best practices. Understanding Asynchronous JavaScript What Is Asynchronous Programming? Asynchronous programming allows JavaScript to perform long-running tasks (like network requests) without blocking the main thread. This is possible because of the event loop, which handles the execution of asynchronous code after the synchronous code has completed. Common Use Cases Asynchronous operations are used in scenarios such as: Fetching API data (fetch, axios) Reading files (in Node.js with fs.readFile) Timers (setTimeout, setInterval) Database operations Why Synchronous Code Doesn’t Work If JavaScript waited synchronously for every request or operation, your app would freeze. That’s why trying to return data like this fails: function getData() const response = fetch('https://api.example.com/data'); return response.json(); // This throws an error The above won't work because fetch is asynchronous. Let’s dive into how to handle such cases properly. Promises for Asynchronous Responses What Is a Promise? A Promise is an object representing the eventual completion (or failure) of an asynchronous operation. A promise can be: Pending: initial state Fulfilled: operation completed successfully Rejected: operation failed Creating a Promise const fetchData = () => return new Promise((resolve, reject) => setTimeout(() => resolve("Data loaded"); , 1000); ); ; Handling Promises with .then(), .catch(), and .finally() fetchData() .then(data => console.log(data); // "Data loaded" ) .catch(error => console.error("Error:", error); ) .finally(() => console.log("Request completed"); ); Chaining Promises fetch('https://api.example.com/data') .then(response => response.json()) .then(json => console.log("Processed JSON:", json); ) .catch(error => console.error("Fetch error:", error)); Handling Multiple Promises: Promise.all() and Promise.race() Promise.all([ fetch('/data1.json'), fetch('/data2.json') ]).then(responses => return Promise.all(responses.map(res => res.json())); ).then(results => console.log("Both results:", results); ); Promise.race([ fetch('/fast.json'), fetch('/slow.json') ]).then(response => console.log("First resolved:", response.url); ); Async/Await for Asynchronous Responses Introduction to async/await Introduced in ES2017, async/await is syntactic sugar over Promises that makes asynchronous code look synchronous and easier to read. Using async/await async function getData() try const response = await fetch('https://api.example.com/data'); const json = await response.json(); console.log("Data:", json); catch (error) console.error("Error:", error); How async/await Works Internally An async function always returns a Promise. await pauses execution inside the function until the Promise is resolved or rejected. async function example() return "Hello"; example().then(console.log); // Outputs "Hello" Callbacks (Legacy Approach) What Is a Callback? A callback function is passed as an argument to another function and executed later.
This was the earliest method of handling asynchronous code in JavaScript. function loadData(callback) setTimeout(() => callback("Data loaded via callback"); , 1000); loadData(data => console.log(data); ); Callback Hell and Limitations Nested callbacks can quickly become unreadable—a problem known as "callback hell": loadData(data => parseData(data, parsed => saveData(parsed, saved => console.log("All done"); ); ); ); Why Promises and Async/Await Are Better Improved readability Easier error handling Cleaner flow control Handling Different Response Types JSON Data const getJson = async () => const res = await fetch('/data.json'); const json = await res.json(); console.log(json); ; Text Response const getText = async () => const res = await fetch('/message.txt'); const text = await res.text(); console.log(text); ; Binary Data (e.g., Images) const getBlob = async () => const res = await fetch('/image.png'); const blob = await res.blob(); const url = URL.createObjectURL(blob); document.querySelector('img').src = url; ; Error Handling and Best Practices try/catch for Async/Await try const response = await fetch('/invalid-url'); if (!response.ok) throw new Error('Server error'); const data = await response.json(); catch (error) console.error('Fetch failed:', error.message); Promise Rejection Handling fetch('/invalid-url') .then(res => if (!res.ok) throw new Error("Server responded with error"); return res.json(); ) .catch(err => console.error("Promise error:", err); ); Network Error vs. Server Error Network Error: Browser fails to connect to the server. Server Error: Server responds with status 4xx or 5xx. Always check both response.ok and handle exceptions. Conclusion Handling asynchronous responses in JavaScript is crucial for building modern, responsive applications. With tools like Promises and async/await, developers can write cleaner, more manageable code. To recap: Use Promises to handle operations with .then() and .catch(). Prefer async/await for better readability and structured error handling. Understand callbacks for legacy support, but use modern alternatives. Always handle errors carefully to ensure app stability. Understanding and mastering JavaScript asynchronous responses is essential for every developer. Start practicing today to make your apps more efficient and responsive. External References MDN Web Docs: Promises MDN Web Docs: async function JavaScript Event Loop (MDN)
0 notes
Text
Output of Java Program | Set 11
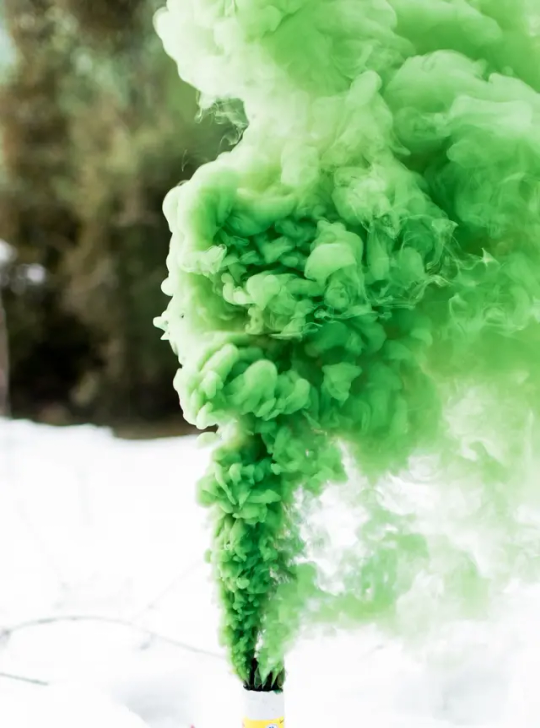
Predict the output of following Java programs: Question 1 : public class Base private int data; public Base() data = 5; public int getData() return this.data; class Derived extends Base private int data; public Derived() data = 6; private int getData() return data; public static void main(String[] args) Derived myData = new Derived(); System.out.println(myData.getData()); a) 6b) 5c) Compile time errord) Run time error Answer (c)Explanation: When overriding a method of superclass, the method declaration in subclass cannot be more restrictive than that […]
0 notes
Text
7 Essential JavaScript Features Every Developer Should Know Early.
JavaScript is the backbone of modern web development. Whether you're just starting out or already have some coding experience, mastering the core features of JavaScript early on can make a big difference in your growth as a developer. These essential features form the building blocks for writing cleaner, faster, and more efficient code.

Here are 7 JavaScript features every developer should get familiar with early in their journey:
Let & Const Before ES6, var was the only way to declare variables. Now, let and const offer better ways to manage variable scope and immutability.
let allows you to declare block-scoped variables.
const is for variables that should not be reassigned.
javascript Copy Edit let count = 10; const name = "JavaScript"; // name = "Python"; // This will throw an error Knowing when to use let vs. const helps prevent bugs and makes code easier to understand.
Arrow Functions Arrow functions offer a concise syntax and automatically bind this, which is useful in callbacks and object methods.
javascript Copy Edit // Traditional function function add(a, b) { return a + b; }
// Arrow function const add = (a, b) => a + b; They’re not just syntactic sugar—they simplify your code and avoid common scope issues.
Template Literals Template literals (${}) make string interpolation more readable and powerful, especially when dealing with dynamic content.
javascript Copy Edit const user = "Alex"; console.log(Hello, ${user}! Welcome back.); No more awkward string concatenation—just cleaner, more intuitive strings.
Destructuring Assignment Destructuring allows you to extract values from objects or arrays and assign them to variables in a single line.
javascript Copy Edit const user = { name: "Sara", age: 25 }; const { name, age } = user; console.log(name); // "Sara" This feature reduces boilerplate and improves clarity when accessing object properties.
Spread and Rest Operators The spread (…) and rest (…) operators may look the same, but they serve different purposes:
Spread: Expands an array or object.
Rest: Collects arguments into an array.
javascript Copy Edit // Spread const arr1 = [1, 2]; const arr2 = […arr1, 3, 4];
// Rest function sum(…numbers) { return numbers.reduce((a, b) => a + b); } Understanding these makes working with arrays and objects more flexible and expressive.
Promises & Async/Await JavaScript is asynchronous by nature. Promises and async/await are the key to writing asynchronous code that reads like synchronous code.
javascript Copy Edit // Promise fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data));
// Async/Await async function getData() { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); } Mastering these will help you handle APIs, databases, and other async operations smoothly.
Array Methods (map, filter, reduce) High-order array methods are essential for transforming and managing data.
javascript Copy Edit const numbers = [1, 2, 3, 4, 5];
// map const doubled = numbers.map(n => n * 2);
// filter const even = numbers.filter(n => n % 2 === 0);
// reduce const sum = numbers.reduce((total, n) => total + n, 0); These methods are clean, efficient, and favored in modern JavaScript codebases.
Final Thoughts Learning these JavaScript features early gives you a solid foundation to write better, more modern code. They’re widely used in frameworks like React, Vue, and Node.js, and understanding them will help you grow faster as a developer.
Start with these, build projects to apply them, and your JavaScript skills will take off.
1 note
·
View note
Text
HarmonyOS NEXT Practical: Waterfall Flow and LazyForeach
Goal: Implement waterfall flow images and text, and load waterfall flow sub items through lazy loading.
Implementation idea:
Create a Card model
Create WaterFlowDataSource data source
Customize WaterFlowVNet Component Custom Components
Implement WaterFlow and LazyForEach loops on the page
WaterFlow The waterfall container is composed of cells separated by rows and columns. Through the container's own arrangement rules, different sized items are arranged tightly from top to bottom, like a waterfall. Only supports FlowItem sub components and supports rendering control types (if/else, ForEach, LazyForEach, and Repeat).
Actual combat: WaterFlowDataSource [code] // An object that implements the iPadOS Source interface for loading data into waterfall components export class WaterFlowDataSource implements IDataSource { private dataArray: Card[] = []; private listeners: DataChangeListener[] = [];
constructor() { this.dataArray.push({ image: $r('app.media.img_1'), imageWidth: 162, imageHeight: 130, text: 'Ice cream is made with carrageenan …', buttonLabel: 'View article' }); this.dataArray.push({ image: $r('app.media.img_2'), imageWidth: '100%', imageHeight: 117, text: 'Is makeup one of your daily esse …', buttonLabel: 'View article' }); this.dataArray.push({ image: $r('app.media.img_3'), imageWidth: '100%', imageHeight: 117, text: 'Coffee is more than just a drink: It’s …', buttonLabel: 'View article' }); this.dataArray.push({ image: $r('app.media.img_4'), imageWidth: 162, imageHeight: 130, text: 'Fashion is a popular style, especially in …', buttonLabel: 'View article' }); this.dataArray.push({ image: $r('app.media.img_5'), imageWidth: '100%', imageHeight: 206, text: 'Argon is a great free UI packag …', buttonLabel: 'View article' }); }
// 获取索引对应的数据 public getData(index: number): Card { return this.dataArray[index]; }
// 通知控制器数据��新加载 notifyDataReload(): void { this.listeners.forEach(listener => { listener.onDataReloaded(); }) }
// 通知控制器数据增加 notifyDataAdd(index: number): void { this.listeners.forEach(listener => { listener.onDataAdd(index); }) }
// 通知控制器数据变化 notifyDataChange(index: number): void { this.listeners.forEach(listener => { listener.onDataChange(index); }) }
// 通知控制器数据删除 notifyDataDelete(index: number): void { this.listeners.forEach(listener => { listener.onDataDelete(index); }) }
// 通知控制器数据位置变化 notifyDataMove(from: number, to: number): void { this.listeners.forEach(listener => { listener.onDataMove(from, to); }) }
//通知控制器数据批量修改 notifyDatasetChange(operations: DataOperation[]): void { this.listeners.forEach(listener => { listener.onDatasetChange(operations); }) }
// 获取数据总数 public totalCount(): number { return this.dataArray.length; }
// 注册改变数据的控制器 registerDataChangeListener(listener: DataChangeListener): void { if (this.listeners.indexOf(listener) < 0) { this.listeners.push(listener); } }
// 注销改变数据的控制器 unregisterDataChangeListener(listener: DataChangeListener): void { const pos = this.listeners.indexOf(listener); if (pos >= 0) { this.listeners.splice(pos, 1); } }
// 增加数据 public add1stItem(card: Card): void { this.dataArray.splice(0, 0, card); this.notifyDataAdd(0); }
// 在数据尾部增加一个元素 public addLastItem(card: Card): void { this.dataArray.splice(this.dataArray.length, 0, card); this.notifyDataAdd(this.dataArray.length - 1); }
public addDemoDataAtLast(): void { this.dataArray.push({ image: $r('app.media.img_1'), imageWidth: 162, imageHeight: 130, text: 'Ice cream is made with carrageenan …', buttonLabel: 'View article' }); this.dataArray.push({ image: $r('app.media.img_2'), imageWidth: '100%', imageHeight: 117, text: 'Is makeup one of your daily esse …', buttonLabel: 'View article' }); this.dataArray.push({ image: $r('app.media.img_3'), imageWidth: '100%', imageHeight: 117, text: 'Coffee is more than just a drink: It’s …', buttonLabel: 'View article' }); this.dataArray.push({ image: $r('app.media.img_4'), imageWidth: 162, imageHeight: 130, text: 'Fashion is a popular style, especially in …', buttonLabel: 'View article' }); this.dataArray.push({ image: $r('app.media.img_5'), imageWidth: '100%', imageHeight: 206, text: 'Argon is a great free UI packag …', buttonLabel: 'View article' }); }
// 在指定索引位置增加一个元素 public addItem(index: number, card: Card): void { this.dataArray.splice(index, 0, card); this.notifyDataAdd(index); }
// 删除第一个元素 public delete1stItem(): void { this.dataArray.splice(0, 1); this.notifyDataDelete(0); }
// 删除第二个元素 public delete2ndItem(): void { this.dataArray.splice(1, 1); this.notifyDataDelete(1); }
// 删除最后一个元素 public deleteLastItem(): void { this.dataArray.splice(-1, 1); this.notifyDataDelete(this.dataArray.length); }
// 在指定索引位置删除一个元素 public deleteItem(index: number): void { this.dataArray.splice(index, 1); this.notifyDataDelete(index); }
// 重新加载数据 public reload(): void { this.dataArray.splice(1, 1); this.dataArray.splice(3, 2); this.notifyDataReload(); } }
export interface Card { image: Resource //图片 imageWidth: Length //图片宽度 imageHeight: Length //图片高度 text: string //文字 buttonLabel: string //按钮文字 } [/code] WaterFlowItemComponent [code] import { Card } from "./WaterFlowDataSource";
// @Reusable @Component export struct WaterFlowItemComponent { @Prop item: Card
// 从复用缓存中加入到组件树之前调用,可在此处更新组件的状态变量以展示正确的内容 aboutToReuse(params: Record) { this.item = params.item; console.info('Reuse item:' + JSON.stringify(this.item)); }
aboutToAppear() { console.info('new item:' + JSON.stringify(this.item)); }
build() { if (this.item.imageWidth == '100%') { Column() { Image(this.item.image) .width(this.item.imageWidth) .height(this.item.imageHeight) Column() { Text(this.item.text) .fontWeight(400) .fontColor('#32325D') .fontSize(14) .lineHeight(18) Text(this.item.buttonLabel) .fontWeight(700) .fontColor('#5E72E4') .fontSize(12) .lineHeight(17) } .width('100%') .padding(12) .layoutWeight(1) .alignItems(HorizontalAlign.Start) .justifyContent(FlexAlign.SpaceBetween) } .width('100%') .height('100%') .alignItems(HorizontalAlign.Start) } else { Row() { Image(this.item.image) .width(this.item.imageWidth) .height(this.item.imageHeight) Column() { Text(this.item.text) .fontWeight(400) .fontColor('#32325D') .fontSize(14) .lineHeight(18) Text(this.item.buttonLabel) .fontWeight(700) .fontColor('#5E72E4') .fontSize(12) .lineHeight(17) } .height('100%') .layoutWeight(1) .alignItems(HorizontalAlign.Start) .padding(12) .justifyContent(FlexAlign.SpaceBetween) } .width('100%') .height('100%') }
} } [/code] WaterFlowDemoPage [code] import { Card, WaterFlowDataSource } from './WaterFlowDataSource'; import { WaterFlowItemComponent } from './WaterFlowItemComponent';
@Entry @Component export struct WaterFlowDemoPage { minSize: number = 80; maxSize: number = 180; fontSize: number = 24; scroller: Scroller = new Scroller(); dataSource: WaterFlowDataSource = new WaterFlowDataSource(); dataCount: number = this.dataSource.totalCount(); private itemHeightArray: number[] = []; @State sections: WaterFlowSections = new WaterFlowSections(); sectionMargin: Margin = { top: 10, left: 20, bottom: 10, right: 20 };
// 设置FlowItem的高度数组 setItemSizeArray() { this.itemHeightArray.push(130); this.itemHeightArray.push(212); this.itemHeightArray.push(212); this.itemHeightArray.push(130); this.itemHeightArray.push(268); }
aboutToAppear() { this.setItemSizeArray(); this.addSectionOptions(true); for (let index = 0; index < 10; index++) { this.dataSource.addDemoDataAtLast(); this.setItemSizeArray(); this.addSectionOptions(); } }
addSectionOptions(isFirstAdd: boolean = false) { this.sections.push({ itemsCount: 1, crossCount: 1, margin: isFirstAdd ? { top: 20, left: 20, bottom: 10, right: 20 } : this.sectionMargin, onGetItemMainSizeByIndex: (index: number) => { return 130; } }) this.sections.push({ itemsCount: 2, crossCount: 2, rowsGap: '20vp', margin: this.sectionMargin, onGetItemMainSizeByIndex: (index: number) => { return 212; } }) this.sections.push({ itemsCount: 1, crossCount: 1, margin: this.sectionMargin, onGetItemMainSizeByIndex: (index: number) => { return 130; } }) this.sections.push({ itemsCount: 1, crossCount: 1, rowsGap: '20vp', columnsGap: '20vp', margin: this.sectionMargin, onGetItemMainSizeByIndex: (index: number) => { return 268; } }) }
build() { Column({ space: 2 }) { WaterFlow({ scroller: this.scroller, sections: this.sections }) { LazyForEach(this.dataSource, (item: Card, index: number) => { FlowItem() { WaterFlowItemComponent({ item: item }) } .width('100%') .backgroundColor(Color.White) .borderRadius(6) .clip(true) }, (item: Card, index: number) => index.toString()) } // .columnsTemplate('1fr 1fr') // 瀑布流使用sections参数时该属性无效 .columnsGap(14) .rowsGap(20) .backgroundColor('#F8F9FE') .width('100%') .height('100%') .layoutWeight(1) } } } [/code]
0 notes
Text
Java Unit Testing: A Comprehensive Guide
Introduction: The Importance of Unit Testing in Java
Java Unit testing is a cornerstone of modern software development, ensuring that individual components of a Java application work as expected. By isolating and testing specific units of code, developers can identify bugs early and maintain higher code quality throughout the development lifecycle.
What is Unit Testing?
Unit testing involves testing individual units of code, such as methods or classes, in isolation to verify their correctness. Each test case validates a specific behavior, ensuring that the code performs as intended under various conditions. This foundational testing practice is critical for building reliable and maintainable Java applications.
Why is Unit Testing Essential in Java Development?
Unit testing in Java helps catch bugs early, improves code quality, and ensures that changes don’t break existing functionality. It enables developers to refactor code with confidence, facilitates collaboration, and supports continuous integration and deployment workflows. Simply put, unit testing lays the groundwork for robust and scalable software.
Setting Up a Unit Testing Environment in Java
Before writing unit tests in Java, you need to set up the necessary tools and frameworks. The most commonly used framework for Java unit testing is JUnit. Here’s how to get started:
Install JUnit or TestNG: Add JUnit as a dependency in your project’s pom.xml (Maven) or build.gradle (Gradle).
Configure Your IDE: Use a Java IDE like IntelliJ IDEA or Eclipse to streamline the testing process with built-in support for JUnit.
Writing Your First Unit Test with JUnit
JUnit is the most popular framework for writing and running unit tests in Java. Let’s walk through a basic example:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
class CalculatorTest {
@Test
void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertEquals(5, result);
}
}
This simple test verifies that the add method in the Calculator class returns the correct sum of two numbers.
Best Practices for Writing Unit Tests in Java
Adhering to best practices ensures that your unit tests are effective, reliable, and maintainable:
Write Small, Isolated Tests: Test one functionality at a time to simplify debugging and ensure accuracy.
Use Meaningful Test Names: Clearly describe the test’s purpose to improve readability.
Avoid External Dependencies: Mock external systems to focus on the unit under test.
Mocking Dependencies with Mockito
Mockito is a powerful library for mocking dependencies, allowing you to test components in isolation. For example:
import org.mockito.Mockito;
import static org.mockito.Mockito.*;
class ServiceTest {
@Test
void testService() {
Database mockDatabase = mock(Database.class);
when(mockDatabase.getData()).thenReturn("Mock Data");
Service service = new Service(mockDatabase);
String result = service.processData();
assertEquals("Processed Mock Data", result);
verify(mockDatabase).getData();
}
}
This test uses Mockito to mock a database dependency, ensuring that the Service class is tested in isolation.
Testing Edge Cases and Error Handling
Effective unit testing involves covering not just the happy paths but also edge cases and error scenarios. For instance:
@Test
void testNullInput() {
Exception exception = assertThrows(IllegalArgumentException.class, () -> {
new Calculator().add(null, 5);
});
assertEquals("Input cannot be null", exception.getMessage());
}
This test ensures that the add method throws an appropriate exception for null inputs.
Running and Automating Unit Tests
Running unit tests frequently is crucial, and automating the process ensures they remain part of your development workflow. Tools like Maven and Gradle allow seamless test execution:
Maven: Use mvn test to run all unit tests.
Gradle: Use ./gradlew test to execute tests in your project.
Integrating these commands into a CI/CD pipeline ensures that tests are run automatically with every code change.
Measuring Code Coverage
Code coverage tools help you measure how much of your codebase is exercised by unit tests. Popular tools include:
JaCoCo: Provides detailed coverage reports and integrates with Maven and Gradle.
Cobertura: Offers insights into tested and untested code paths.
Use these tools to identify gaps in your test coverage and ensure critical paths are thoroughly tested.
Challenges in Unit Testing Java Applications
While unit testing is essential, it comes with its own set of challenges, especially in complex Java applications:
Managing Dependencies: Complex applications often involve numerous dependencies that can be difficult to mock or isolate.
Testing Legacy Code: Legacy codebases may lack modularity, making them harder to test effectively.
Address these challenges by refactoring code, using mocking frameworks, and incrementally increasing test coverage.
Conclusion: Embracing Unit Testing for Robust Java Applications
Unit testing is an indispensable practice for building reliable, maintainable, and bug-free Java applications. By incorporating tools like JUnit and Mockito, following best practices, and addressing challenges head-on, you can ensure that your Java projects meet the highest standards of quality.
0 notes
Text
How to get Product Collection in Magento 2
Hello Everyone,
In this blog, we will learn about how to get Product Collection in Magento 2.
Product Collection means showing the items in your Magento 2 Store when you run the command.
Without wasting your time, let us guide you straight away. Follow the easy step given below to get Product Collection in Magento 2.
STEPS FOR GET PRODUCT COLLECTION IN MAGENTO 2
Step 1: Create Hello.php file
app/code/Vendor/Extension/Block/Hello.php
<?php
namespace Vendor\Extension\Block;
class Hello extends \Magento\Framework\View\Element\Template
{
protected $productFactory;
public function __construct(
\Magento\Backend\Block\Template\Context $context,
\Magento\Catalog\Model\ResourceModel\Product\CollectionFactory $productFactory,
array $data = []
)
{
$this->productFactory = $productFactory;
parent::__construct($context, $data);
}
public function getProductCollection()
{
$collection = $this->productFactory->create();
return $collection;
}
}
Now We Print Product Collection in .phtml file. We call getProductCollection() method from our block.
<?php
$productCollection = $block->getProductCollection();
foreach ($productCollection as $product) {
echo “<pre>”;
print_r($product->getData());
echo “</pre>”;
}
Final Thoughts:
So this was the easiest way which we have told you in this blog. This is how you can get Product Collection in Magento 2. Hope you liked the blog.
So quickly go to the comment box and tell me how you like this blog?
Stay tuned with us on our site to get new updates of Magento.
Thanks for reading and visiting our site.
0 notes
Text
Impression of Anoboy in Anime Streaming Sites and Audience
We have a constantly changing anime industry, moving with the times (technology and half of what every fan approves), influenced by notable figures regarding different movements. Of these voices, Anoboy has become a rising tide that lifts most anime streaming platforms up and also its viewership. The methods that he used for commentary and content creation, helped in shaping how his fans looked at anime through a different perspective hence providing him with the ground-breaking way ahead of streaming platform strategies.
How Anoboy is Shaping the Future of Anime Streaming
The influence Anoboy bestowed on anime streaming platforms confirms his place in the world of otaku. He effectively changed the way we talk about and consume anime commentary, which begat some significant shifts in the behavior of viewers and what streaming platforms see as strategy.
1. Engage and Discoveryunicast [×]arc edittrailerstvSeason 2, EPISODE 1The new way to watch video.
Among the most notable effects that Anoboy has on anime streaming platform is in increasing viewer engagement and discovery. Anoboy, are an online platforms that serve reviews for series obscure as well some hidden gems to the fans through there detailed analysis and collectible content. His suggestions and reviews guide viewers to check out new shows that might have been skipped over, keeping streaming sites working on expanding the field of anime they are willing to put in front of their audiences.
Anoboy often creates in-depth blogs about mainstream and obscure anime, guiding his followers to explore those...getData=url;forest(3,/portfolio/anisong-bikin-rng-gen-m-98-di-marketing-media/,true). It's why certain series are now attracting more traffic and viewership than they might've from the jump, changing how streaming services value their content.
2. Improving Content Curation and Discovery
As we know it, streaming platforms have long been trying to offer the best-personalized content product recommendations and influence from Anoboy EFFECTED how they too had redefined their curation strategy. Anoboy's more nuanced anime analysis and critique ability have proved the necessity of a recommendation algorithm that can be adopted to higher levels. The possibility that platforms are becoming more likely to contain curation lists and suggestions which are auto designed for this sort of in-depth analysis and thematic dialogue has existed for at least as long Anoboy.
Thus, for one instance; anime streaming services have now begun recommending themes or genres regarding the types of videos Anoboy talks about. This suggests users new series based on their tastes in commentaries.
3. Effect on Streaming Service Partnerships
The popularity of Anoboy is also changing the way streaming services think about working with creators. Given the reach and influence of Anoboy, it is no surprise that a number of streaming services are doing promotional campaigns with him as well exclusive content or even live events.
Often such partnerships are accompanied by special promotions or exclusive previews of anime content in the pipeline, which helps to build hype around series — Anoboy having garnered quite a bit of credibility and fanbase among fans. These collaborations allow platforms to draw in new subscribers or beyonden by providing original content and experiences around the most popular figures for example is Anoboy.
4. Setting a bar with both (for viewer expectations and content quality)
We anoboy have raised our standard to provide quality commentary on insightful anime discussion. As a result, most viewers who start engaging with content by anoboy in any way are exposed to higher standards and improved scope of anime-related stuff. This change in viewer perception has nudged the streaming services to put more emphasis on content power as well as innovation.
In terms of more widely available content, many other platforms are opting for HD streaming or improved subtitling — possibly even including bonus features such as behind-the-scenes footage and interviews with the creators. This new demand of higher quality and dynamic content, largely influenced by Anoboy as the catalyst resulted in an overall better streaming experience for those watching anime.
The Broader Anoboy Influence
This ultimately has a much broader and deeper impact compared to individual platforms or viewership trends. More broadly, his style of anime commentary — which has since taken root a bit more widely across the industry and influenced at least some people to think about engaging with my art form less shallowly than is typically considered normal for the ilk — spurred positive cultural movement within our little world here.
1. Fostering A Climate of Rigorous Discussion
Thanks to Anoboy for providing such detailed review and discussion which leads us in discussing this anime when serious discourses is being build by fans around the globe. This has provoked some similarly reasoned responses from other sides of the argument, which in turn affects how anime is reviewed and debated about within broader anime communities.
2. Diverse Representation of Anime
Anoboy covers all the mainstream hits and also, some of its lesser-known series has only helped growing diversity in anime content. This also encouraged streaming platforms to focus on more flexible anime that appeals to all sorts of types and varieties through the fandom.
3. HyperBeard Fosters the Next Wave of Creators
And For you guys who wanna become blog anime or YouTube commentators and are being asked about anoboy again, since AnoBoy has succeeded this can inspirated another younger brother in the age of booming 2019. His impact on upcoming creators is how anime commentary starts to grow into a colorful, moving landscape.
Conclusion
The impact of anoboy on anime streamers and viewers is visible in the way he built a huge fan base for himself. Anoboy, by encouraging audience participation and style curation acts as a bridge in changing how anime is consumed and discussed moving forward with the help of his superior quality video analysis. While Anoboy has already left an indelible mark on the anime industry, his influence can still be felt through waves that continue to develop and change with time.
0 notes
Text
Disclosure of CPU DoS due to malicious P2P message (≤ version 0.19.2)
A malformed GETDATA message could trigger an infinite loop on the receiving node, using 100% of the CPU allocated to this thread and not making further progress on this connection. This issue is considered Low severity. Details Before Bitcoin Core 0.20.0, an attacker (or buggy client, even) could send us a GETDATA message that would cause our net_processing thread to start spinning at 100%, and not make progress processing messages for the attacker peer anymore. It would still make progress processing messages from other peers, so it is just a CPU DoS with low impact beyond that (not making progress for attacker peers is a non-issue). It also increases per-peer long-term memory usage up by 1.5 MB per attacker peer. John Newbery opened PR #18808 to fix this issue by only disclosing the lack of progress. Attribution Credits to John Newbery for finding this bug, responsibly disclosing it and fixing it. Timeline * 2020-04-29 John Newbery opens #18808 * 2020-05-08 John Newbery reports his finding by email * 2020-05-12 #18808 is merged * 2020-06-03 Bitcoin Core version 0.20.0 is released with a fix * 2021-09-13 The last vulnerable Bitcoin Core version (0.19.x) goes EOL * 2024-07-03 Public disclosure. http://dlvr.it/T96bk8
0 notes
Text
BIA660 Assignment 2: Web Scraping solved
Q1. Scrape Book Catalog Scape content of http://books.toscrape.com (http://books.toscrape.com) Write a function getData() to scrape title (see (1) in Figure), rating (see (2) in Figure), price (see (3) in Figure) of all books (i.e. 20 books) listed in the page. For example, the figure shows one book and the corresponding html code. You need to scrape the highlighted content. For star ratings, you…
View On WordPress
0 notes
Text
BEST ONLINE SPORTSBOOK
Are you a cricket fan looking for some exciting betting? Look no further. It is the perfect place for you! Browse through hundreds of bets on thousands of cricket matches and start betting today.
KOHINOOR is a widely available online sports betting site and the most trusted bookie in the country. It has an exclusive deal to run online sports betting in New Hampshire and Oregon, and it is available in many more states. KOHINOOR is the No. 1 sports betting site in several states, including Illinois and Arizona. It also began life as a DFS provider, but it pivoted to online sports betting after the federal ban was declared unconstitutional in 2018. KOHINOOR offers very appealing welcome bonuses and generous odds. Its sportsbook is powered by industry titan SBTech, which Kohinoor purchased in 2020. KOHINOOR also has a very impressive online casino and an NFT marketplace, and its rewards program spans each section of the site. No specific Kohinoor promo code is needed to get its welcome offer.
KOHINOOR is perfect for newcomers to online sports betting, because the website is very simple and user-friendly, and the customer service is strong. It is now available in nine states, having grown quicker and it remains on the expansion trail.
0 notes
Photo

NAS Storage Box Failed ? 📞 +968 963 12346 NOW! Get back the Data Lost in Any Scenarios from Any Model NAS Storage Devices. • Accidentally Delete Files • Operating System Crashes • Damaged/Dead/Crashed Drives • Virus/Ransomware attack • Lost Partitions • Formatted Drives • Raw/Unallocated Partitions • Water/Fire damage Space Recovery, 1st Floor, Azaiba Mall, Azaiba, Muscat https://spacedatarecovery.com [email protected] #synology #qnap #nexgear #drobo #storage #nas #nasstorage #datastorage #veem #storagebox #getdata #datarecovery #photooman #professional #spacedatarecovery #omanmuscat (at Muscat, Oman) https://www.instagram.com/p/CggiWUKKgqP/?igshid=NGJjMDIxMWI=
#synology#qnap#nexgear#drobo#storage#nas#nasstorage#datastorage#veem#storagebox#getdata#datarecovery#photooman#professional#spacedatarecovery#omanmuscat
0 notes
Photo

"Herhangi bir siteden veri çekmenizi kolaylaştıran uygulama: GetData" https://www.hasanzanbak.com/teknoloji/herhangi-bir-siteden-veri-cekmenizi-kolaylastiran-uygulama-getdata/
0 notes
Text
Servico profesional de topografía, fotogrametria, cartografía.
#getdata
#RTK
#fotogrametria
GetData
1 note
·
View note
Text
An Introduction to GoMock: Mocking in Go
In software development, testing is a critical aspect that ensures the quality and reliability of your code. However, when writing tests, you often encounter situations where you need to test a component in isolation, free from the influence of its dependencies. This is where mocking comes into play, and for Go developers, GoMock is one of the most widely used frameworks for this purpose.
In this article, we’ll explore GoMock, a powerful mocking framework for Go (Golang), and demonstrate how to use it effectively in your testing strategy.
What is GoMock?
GoMock is a mocking framework for Go that allows developers to create mock objects for their tests. These mock objects are simulated versions of real objects, enabling you to test your code in isolation by replacing dependencies with mock implementations. This is particularly useful for testing complex interactions between components without relying on actual external systems or dependencies.
Why Use GoMock?
Mocking is essential for several reasons:
Isolation: By mocking dependencies, you can focus on testing the functionality of a specific component without being affected by the behavior of its dependencies.
Speed: Mocked tests typically run faster because they don’t involve actual database connections, network calls, or other time-consuming operations.
Control: With mocks, you have full control over the behavior of the dependencies, allowing you to simulate various scenarios, including edge cases.
Repeatability: Mocks help ensure that tests are repeatable and produce consistent results, even when external conditions change.
GoMock provides a robust solution for implementing mocks in Go, making it easier to test your code effectively.
Getting Started with GoMock
1. Installing GoMock
To get started with GoMock, you need to install the GoMock package and the mockgen tool, which generates mock implementations for your interfaces. You can do this using Go modules:
go get github.com/golang/mock/gomock
go install github.com/golang/mock/mockgen@latest
2. Generating Mocks with mockgen
The mockgen tool is used to generate mock implementations for interfaces. Let’s say you have an interface Database in a package db that you want to mock. You would generate the mock like this:
mockgen -source=db.go -destination=mocks/mock_db.go -package=mocks
This command generates a mock implementation of the Database interface in the mocks package, which you can use in your tests.
3. Writing Tests with GoMock
Once you have generated your mocks, you can use them in your test cases. Here’s an example:
package mypackage
import (
"testing"
"github.com/golang/mock/gomock"
"mypackage/mocks" // Import the package containing the generated mocks
)
func TestMyFunction(t *testing.T) {
// Create a new Gomock controller
ctrl := gomock.NewController(t)
defer ctrl.Finish()
// Create a mock instance of the Database interface
mockDB := mocks.NewMockDatabase(ctrl)
// Set up expectations on the mock
mockDB.EXPECT().GetData("key").Return("value", nil)
// Call the function under test, using the mock as a dependency
result, err := MyFunction(mockDB, "key")
// Assert the results
if err != nil {
t.Fatalf("expected no error, got %v", err)
}
if result != "value" {
t.Fatalf("expected 'value', got %v", result)
}
}
In this example, MyFunction is the function being tested, and it relies on a Database interface. The mock object mockDB is used to simulate the behavior of the real database during the test, ensuring that the test focuses solely on the logic within MyFunction.
4. Setting Expectations with GoMock
One of the key features of GoMock is the ability to set expectations on method calls. You can specify how many times a method should be called, with what arguments, and what it should return. This helps in ensuring that your code interacts with dependencies as expected.
For example:
mockDB.EXPECT().GetData("key").Times(1).Return("value", nil)
This expectation specifies that the GetData method should be called exactly once with the argument "key" and should return "value" and nil as the error.
Best Practices for Using GoMock
To make the most of GoMock, consider the following best practices:
Keep Tests Focused: Use GoMock to isolate the component under test and focus on its logic, rather than testing the behavior of its dependencies.
Avoid Over-Mocking: While mocking is powerful, avoid the temptation to mock too many dependencies, as it can lead to brittle tests that are difficult to maintain.
Clear Expectations: Be explicit about the expectations on your mocks to ensure that your tests are reliable and easy to understand.
Leverage gomock.InOrder: Use gomock.InOrder to enforce the order of method calls when necessary, ensuring that your mocks are used in the expected sequence.
Conclusion
GoMock is an invaluable tool for Go developers, providing the ability to create robust, isolated unit tests by mocking dependencies. By using GoMock effectively, you can ensure that your tests are reliable, repeatable, and focused on the logic of the code under test. Whether you’re testing complex interactions or simply isolating your code from external systems, GoMock offers the flexibility and control needed to maintain high-quality test coverage. By integrating GoMock into your testing strategy, you can enhance the effectiveness of your tests, leading to more reliable and maintainable Go applications.
0 notes
Text
Elefant 2.2.4
Elefant 2.2.4 has been released with a number of bug fixes and improvements.
Click here to download or update.
Introducing JobQueue
This update adds a new JobQueue class which is powered by Pheanstalk/Beanstalkd. This makes it very easy to setup background workers and send tasks to them for processing.
Setup
To set it up, install Beanstalkd via your package manager of choice (apt/yum/brew/port), for example:
$ apt install beanstalkd
Next, after upgrading Elefant to 2.2.4, run Composer update from the root directory of your website to install the Pheanstalk library:
$ cd /path/to/www && composer update
Lastly, edit the [JobQueue] settings in your conf/config.php file to point to your Beanstalkd server:
[JobQueue] backend = beanstalkd host = 127.0.0.1 port = 11300
Usage
Sending a job to be done by a background worker is as easy as:
JobQueue::enqueue ('tube-name', ['data' => '...']);
Writing a worker in Elefant looks like this:
<?php // apps/myapp/handlers/worker.php if (! $this->cli) exit; $page->layout = false; $worker = JobQueue::worker (); $worker->watch ('tube-name'); while ($job = $worker->reserve ()) { $data = json_decode ($job->getData ()); // Process job with $data $worker->delete ($job); }
The above worker can be run via php index.php myapp/worker and can be initialized using your system scheduler, such as systemd.
Note that workers can be written in any language that connects to Beanstalkd and listens for jobs to process.
Other improvements
Added User::require_verification() which extends User::require_login() to require email verification too
Added Auto-include Composer autoloader to Site Settings form so you no longer need to include it manually in a bootstrap.php file
Click here for a full list of changes.
#cms#content management#php cms#php#php framework#framework#frameworks#web framework#web development#open source#free software#updates#news#new releases#beanstalkd#pheanstalk#job queues#background workers#bug fixes
2 notes
·
View notes
Text
Waiting For the First Returned API Result With Promise.any() in JavaScript
Waiting For the First Returned API Result With Promise.any() in JavaScript
Promise.any resolves with the found value when the first promise successfully resolves. Which is useful for taking the fastest returning result from a set of slow or unstable APIs. For example, the below simulates different potentially slow APIs by using some with long timeouts:
function apiA() { return new Promise((resolve, reject) => { setTimeout(() => { resolve({ some: 'data', from: 'A' }); }, 1000); }); } function apiB() { return new Promise((resolve, reject) => { setTimeout(() => { resolve({ some: 'data', from: 'B' }); }, 200); }); } function apiC() { return new Promise((resolve, reject) => { setTimeout(() => { resolve({ some: 'data', from: 'C' }); }, 1500); }); } async function getData() { console.time('Promise.any completion time'); const data = await Promise.any([ apiA(), apiB(), apiC(), ]); console.info('Retrieved', data); console.timeEnd('Promise.any completion time'); // Retrieved { some: 'data', from: 'B' } // Promise.any completion time: 203.451ms (exact time will vary slightly) } getData();
Without that, some level of management code would be needed. Note that compared to Promise.race which is now supported by major browsers and Node.js, this only resolves by the first successful promise. Promise.race also resolves when any process causes an error.
For my example, I provided a simple Polyfill that does not do error handling since it was only for this example:
if (typeof Promise.any !== 'function') { // Only for example purposes. This does not implement the error handling of Promise.any. For a production ready // implementation instead consider libraries like Bluebird: https://github.com/petkaantonov/bluebird Promise.any = function(promises) { let resolved = false; return new Promise((resolve, reject) => { for (const promise of promises) { promise.then((result) => { if (resolved !== true) { resolved = true; resolve(result); } }); } }); } }
This will be necessary since Promise.any is in stage 3 which means that although the syntax is finalized, it is still awaiting implementation in most JS environments. If you need a production version of Promise.any(), it will be available in many Promise libraries like Bluebird: https://github.com/petkaantonov/bluebird
Github Location https://github.com/Jacob-Friesen/obscurejs/blob/master/2019/promiseAny.js
8 notes
·
View notes