#getter and setter methods
Explore tagged Tumblr posts
Link
JavaScript Class Syntax and Object-Oriented Programming Essentials
This article provides a comprehensive overview of JavaScript's class syntax and its various features introduced in ES6 (ES2015). It emphasizes the shift from the traditional function and prototype-based object-oriented programming to the more intuitive and structured class-based approach. The article covers key concepts such as class declaration, constructor methods for initialization, method definitions, instance methods, static methods, inheritance, method overriding, access modifiers, getter and setter methods, and static members.
By explaining the rationale behind using classes and highlighting their advantages, the article helps readers understand how class syntax in JavaScript enhances code organization, reusability, and modularity. It clarifies the distinction between instance methods and static methods, making it easier for developers to grasp their appropriate use cases. Furthermore, the article offers insights into inheritance and method overriding, providing practical examples to demonstrate how class relationships can be established.
In addition, the article delves into advanced topics such as access modifiers, getter and setter methods, and static members, shedding light on encapsulation and shared behavior in classes. Overall, the article equips developers with a solid foundation for utilizing class-based object-oriented programming in JavaScript effectively.
#JavaScript#class syntax#ES6#object-oriented programming#inheritance#access modifiers#getter and setter methods#static members#method overriding#code organization
0 notes
Text
Object-Oriented Programming (OOP) Explaine
Object-Oriented Programming (OOP) is a programming paradigm based on the concept of "objects," which represent real-world entities. Objects combine data (attributes) and functions (methods) into a single unit. OOP promotes code reusability, modularity, and scalability, making it a popular approach in modern software development.
Core Concepts of Object-Oriented Programming
Classes and Objects
Class: A blueprint or template for creating objects. It defines properties (attributes) and behaviors (methods).
Object: An instance of a class. Each object has unique data but follows the structure defined by its
Encapsulations
Encapsulation means bundling data (attributes) and methods that operate on that data within a class. It protects object properties by restricting direct access.
Access to attributes is controlled through getter and setter methods.Example: pythonCopyEditclass Person: def __init__(self, name): self.__name = name # Private attribute def get_name(self): return self.__name person = Person("Alice") print(person.get_name()) # Output: Alice
Inheritance
Inheritance allows a class (child) to inherit properties and methods from another class (parent). It promotes code reuse and hierarchical relationships.Example: pythonCopyEditclass Animal: def speak(self): print("Animal speaks") class Dog(Animal): def speak(self): print("Dog barks") dog = Dog() dog.speak() # Output: Dog barks
Polymorphism
Polymorphism allows methods to have multiple forms. It enables the same function to work with different object types.
Two common types:
Method Overriding (child class redefines parent method).
Method Overloading (same method name, different parameters – not natively supported in Python).Example: pythonCopyEditclass Bird: def sound(self): print("Bird chirps") class Cat: def sound(self): print("Cat meows") def make_sound(animal): animal.sound() make_sound(Bird()) # Output: Bird chirps make_sound(Cat()) # Output: Cat meows
Abstraction
Abstraction hides complex implementation details and shows only the essential features.
In Python, this is achieved using abstract classes and methods (via the abc module).Example: pythonCopyEditfrom abc import ABC, abstractmethod class Shape(ABC): @abstractmethod def area(self): pass class Circle(Shape): def __init__(self, radius): self.radius = radius def area(self): return 3.14 * self.radius * self.radius circle = Circle(5) print(circle.area()) # Output: 78.5
Advantages of Object-Oriented Programming
Code Reusability: Use inheritance to reduce code duplication.
Modularity: Organize code into separate classes, improving readability and maintenance.
Scalability: Easily extend and modify programs as they grow.
Data Security: Protect sensitive data using encapsulation.
Flexibility: Use polymorphism for adaptable and reusable methods.
Real-World Applications of OOP
Software Development: Used in large-scale applications like operating systems, web frameworks, and databases.
Game Development: Objects represent game entities like characters and environments.
Banking Systems: Manage customer accounts, transactions, and security.
E-commerce Platforms: Handle products, users, and payment processing.
Machine Learning: Implement models as objects for efficient training and prediction.
Conclusion
Object-Oriented Programming is a powerful paradigm that enhances software design by using objects, encapsulation, inheritance, polymorphism, and abstraction. It is widely used in various industries to build scalable, maintainable, and efficient applications. Understanding and applying OOP principles is essential for modern software development.
: pythonCopyEdit
class Car: def __init__(self, brand, model): self.brand = brand self.model = model def display_info(self): print(f"Car: {self.brand} {self.model}") my_car = Car("Toyota", "Camry") my_car.display_info() # Output: Car: Toyota Camry
Encapsulation
2 notes
·
View notes
Text
Kotlin: 100 Simple Codes
Kotlin: 100 Simple Codes
beginner-friendly collection of easy-to-understand Kotlin examples.

Each code snippet is designed to help you learn programming concepts step by step, from basic syntax to simple projects. Perfect for students, self-learners, and anyone who wants to practice Kotlin in a fun and practical way.
Codes:
1. Hello World
2. Variables and Constants
3. If-Else Statement
4. When Statement (Switch)
5. For Loop
6. While Loop
7. Functions
8. Return Value from Function
9. Array Example
10. List Example
===
11. Mutable List
12. Map Example
13. Mutable Map
14. Class Example
15. Constructor with Default Value
16. Nullable Variable
17. Safe Call Operator
18. Elvis Operator
19. Data Class
20. Loop with Index
===
21. Lambda Function
22. Higher-Order Function
23. Filter a List
24. Map a List
25. String Interpolation
26. String Templates with Expressions
27. Read-Only vs Mutable List
28. Check Element in List
29. Exception Handling
30. Null Check with let
===
31. For Loop with Step
32. For Loop in Reverse
33. Break in Loop
34. Continue in Loop
35. Check String Empty or Not
36. Compare Two Numbers
37. Array Access by Index
38. Loop Through Map
39. Default Parameters in Function
40. Named Arguments
===
41. Range Check
42. Function Returning Unit
43. Multiple Return Statements
44. Chained Method Calls
45. Function Inside Function
46. Function Expression Syntax
47. Array Size
48. String to Int Conversion
49. Safe String to Int Conversion
50. Repeat Block
===
51. Sealed Class
52. Object Expression (Anonymous Object)
53. Singleton using Object Keyword
54. Extension Function
55. Enum Class
56. Use Enum in When Statement
57. Type Alias
58. Destructuring Declarations
59. Companion Object
60. Simple Interface Implementation
===
61. Abstract Class
62. Lateinit Variable
63. Initialization Block
64. Secondary Constructor
65. Nested Class
66. Inner Class
67. Generic Function
68. Generic Class
69. Custom Getter
70. Custom Setter
===
71. String Equality
72. Loop with Range Until
73. Using Pair
74. Triple Example
75. Check Type with is
76. Smart Cast
77. Type Casting with as
78. Safe Casting with as?
79. Loop Through Characters of String
80. Sum of List
===
81. Min and Max of List
82. Sort List
83. Reverse List
84. Count Items in List
85. All / Any Conditions
86. Check if List is Empty
87. Join List to String
88. Take and Drop
89. Zipping Lists
90. Unzipping Pairs
===
91. Chunked List
92. Windowed List
93. Flatten List
94. FlatMap
95. Remove Duplicates
96. Group By
97. Associate By
98. Measure Execution Time
99. Repeat with Index
100. Create Range and Convert to List
===
0 notes
Text
Exploring Record Classes in Java: The Future of Immutable Data Structures
A record in Java is a special type of class designed specifically for holding immutable data. Introduced in Java 14 as a preview feature and made stable in Java 16, records eliminate the need for writing repetitive boilerplate code while still providing all the essential functionalities of a data model.
Key Characteristics of Java Records
Immutable by Default – Once created, the fields of a record cannot be modified.
Automatic Methods – Java automatically generates equals(), hashCode(), and toString() methods.
Compact Syntax – No need for explicit constructors and getters.
Final Fields – Fields inside a record are implicitly final, meaning they cannot be reassigned.
How to Define a Record Class in Java
Defining a record class is straightforward. You simply declare it using the record keyword instead of class.
Example: Creating a Simple Record
java
Using the Record Class
java
Notice how we access fields using methods like name() and age() instead of traditional getter methods (getName() and getAge()).
Comparing Records vs. Traditional Java Classes
Before records, we had to manually write constructors, getters, setters, and toString() methods for simple data structures.
Traditional Java Class (Without Records)
java
This approach requires extra lines of code and can become even more verbose when dealing with multiple fields.
With records, all of this is reduced to just one line:
java
When to Use Records?
Records are ideal for: ✔ DTOs (Data Transfer Objects) ✔ Immutable Data Representations ✔ Returning Multiple Values from a Method ✔ Reducing Boilerplate Code in Simple Models
Customizing Records: Adding Methods and Static Fields
Though records are immutable, you can still add methods and static fields for additional functionality.
Example: Adding a Custom Method
java
Now you can call circle.area() to calculate the area of a circle.
Using Static Fields in Records
java
Limitations of Java Record Classes
While records are powerful, they do have some limitations: ❌ Cannot Extend Other Classes – Records implicitly extend java.lang.Record, so they cannot inherit from any other class. ❌ Immutable Fields – Fields are final, meaning you cannot modify them after initialization. ❌ Not Suitable for Complex Objects – If your object has behavior (methods that modify state), a traditional class is better.
Conclusion: Are Java Record Classes the Future?
Record classes offer a modern, efficient, and elegant way to work with immutable data structures in Java. By removing repetitive boilerplate code, they improve code readability and maintainability.
If you’re working with data-heavy applications, DTOs, or immutable objects, adopting records is a great way to simplify your Java code while ensuring efficiency.
What’s your experience with Java records? Share your thoughts in the comments! 🚀
FAQs
1. Can I modify fields in a Java record?
No, records are immutable, meaning all fields are final and cannot be changed after object creation.
2. Are Java records faster than regular classes?
Performance-wise, records are similar to normal classes but offer better readability and maintainability due to their compact syntax.
3. Can a record extend another class?
No, records cannot extend any other class as they already extend java.lang.Record. However, they can implement interfaces.
4. How are records different from Lombok’s @Data annotation?
While Lombok’s @Data generates similar boilerplate-free code, it requires an external library. Java records, on the other hand, are built into the language.
5. What Java version supports records?
Records were introduced as a preview feature in Java 14 and became a stable feature in Java 16. For more Info : DevOps with Multi Cloud Training in KPHB
#Java#CoreJava#JavaProgramming#JavaDeveloper#LearnJava#Coding#Programming#Tech#SoftwareDevelopment#ImmutableObjects#JavaRecords#OOP#CleanCode#CodeNewbie#DevLife#BackendDevelopment#Java21#TechBlog#CodeWithMe#100DaysOfCode#CodeSnippet#ProgrammingTips#TechTrends
0 notes
Text
JavaScript 1 🧬 JavaScript Introduction
New Post has been published on https://tuts.kandz.me/javascript-1-%f0%9f%a7%ac-javascript-introduction/
JavaScript 1 🧬 JavaScript Introduction

youtube
a - JavaScript Introduction JavaScript is a versatile interpreted programming language. It was primarily used to add interactivity and dynamic behavior to web pages It runs on web browsers as well as on servers using Node.js You can also create desktop applications using Electron Using React Native, Ionic and other frameworks and libraries you can create mobile application for Android and iOS JS is one of the core technologies of the World Wide Web along with HTML and CSS JS originally designed by Brendan Eich at Netscape in 1995 b - Javascipt Key Features Interactivity → JS allows developers to create interactive web pages that change on user actions Client-Side execution → Running on the client-side(web browsers), reduces the server load Rich Web Applications → It supports complex applications through frameworks (React, Angular, and Vue.js) building single-page applications (SPAs) Cross-Platform Compatibility → While primarily used on browsers, JavaScript can also run in other environments such as Node.js for server-side programming, IoT devices, and more. Event-Driven Programming → JavaScript uses an event-driven model to respond to events triggered by the user or browser actions like mouse clicks, key presses, etc. Rich API → It provides a vast array of built-in functions (APIs) for tasks ranging from manipulating images and videos in real time to accessing hardware features directly through browsers. Dynamic Typing → JavaScript is dynamically typed, which means that variable types are not defined until the code is run and can change during execution. Popularity → It's widely used due to its simplicity and flexibility, making it a cornerstone for both front-end (client-side) and back-end development (using Node.js). c - JavaScript Versions 1/2 ES1 → ECMAScript 1 → 1997 → First release ES2 → ECMAScript 2 → 1998 → Minor changes ES3 → ECMAScript 3 → 1999 → regular expressions, do-while, switch, try/catch ES4 → ECMAScript 4 → Never Released. ES5 → ECMAScript 5 → 2009 → JavaScript strict mode, Multiline strings, String.trim(), Array methods, Object methods, Getters and setters, Trailing commas ES6 → ECMAScript 2015 → 2015 → let and const statements, Map and set objects, Arrow functions, For/of loop, Some array methods, Symbol, Classes, Promises, JavaScript Modules, New Number methods and properties, For/of loop, Spread operator ES7 → ECMAScript 2016 → 2016 → Exponential (**) operator, Array.includes() method ES8 → ECMAScript 2017 → 2017 → Async/await, Object.entries() method, Object.values() method, Object.getOwnPropertyDescriptor() method, string padding d - JavaScript Versions 2/2 ES9 → ECMAScript 2018 → 2018 → Rest object properties, JavaScript shared memory, Promise.finally() method, New features of the RegExp() object ES10 → ECMAScript 2019 → 2019 → String trim.start(), String trim.end(), Array.flat(), Revised Array.sort(), Revised JSON.stringify() / toString(), Object.fromEntries() method ES11 → ECMAScript 2020 → 2020 → Nullish Coalescing Operator (??), BigInt primitive data type ES12 → ECMAScript 2021 → 2021 → String.replaceAll() method, Promise.Any() method ES13 → ECMAScript 2022 → 2022 → static block inside the class, New class features, Top-level await ES14 → ECMAScript 2023 → 2023 → Array findLast() & findLastIndex(), Hashbang Grammer, Symbols as WeakMap keys
0 notes
Text
CS150 - Lab 27/28 (Counts as two labs) Solved
Create classes CatRecord, DogRecord and BirdRecord that inherit from (extend) the PetRecord class located on Canvas. Add an integer variable wingspan to BirdRecord, and a Boolean variable hasLongHair to CatRecord and DogRecord. Create appropriate setter and getter methods for each. Also, create constructors that can set all of the values for a cat, dog or bird by setting the values specific to…
0 notes
Text
Kotlin in Mobile App Development: A Modern Approach to Building Robust Android Applications
In the realm of mobile app development, Kotlin has emerged as a game-changer, particularly for Android development. Since its official adoption by Google as a first-class language for Android in 2017, Kotlin has gained widespread popularity among developers due to its concise syntax, interoperability with Java, and robust features that enhance productivity and code safety. As the demand for high-quality mobile applications continues to grow, Kotlin has positioned itself as a modern, efficient, and future-proof choice for building Android apps.
One of the key advantages of Kotlin is its interoperability with Java, which allows developers to seamlessly integrate Kotlin code into existing Java projects. This feature has been instrumental in Kotlin's rapid adoption, as it enables teams to migrate gradually without the need for a complete rewrite. Kotlin's null safety feature is another standout aspect, addressing one of the most common pitfalls in Java development—null pointer exceptions. By distinguishing between nullable and non-nullable types at the language level, Kotlin significantly reduces the risk of runtime crashes, leading to more stable and reliable applications.
Kotlin's concise syntax is another major draw for developers. Compared to Java, Kotlin requires significantly less boilerplate code, making it easier to read and maintain. Features like data classes, extension functions, and lambda expressions allow developers to achieve more with fewer lines of code. For instance, a data class in Kotlin can replace an entire Java class with getters, setters, equals(), hashCode(), and toString() methods, all in a single line. This conciseness not only speeds up development but also reduces the likelihood of errors.
The rise of Kotlin Multiplatform Mobile (KMM) has further expanded the language's reach beyond Android development. KMM allows developers to share business logic between iOS and Android apps, reducing the need for platform-specific code. While the UI layer remains native to each platform, shared modules written in Kotlin can handle tasks such as networking, data storage, and business logic. This approach not only streamlines development but also ensures consistency across platforms, making it an attractive option for teams looking to optimize their workflows.
Kotlin's integration with modern development tools and frameworks has also contributed to its success. Libraries like Ktor for networking and Room for database management are designed to work seamlessly with Kotlin, offering a more idiomatic and efficient development experience. Additionally, Kotlin's support for coroutines has revolutionized asynchronous programming in Android development. Coroutines simplify the handling of background tasks, such as network requests or database operations, by allowing developers to write asynchronous code in a sequential manner. This eliminates the complexity of callbacks and AsyncTask, making the code more readable and maintainable.
In the context of mobile app architecture, Kotlin aligns well with modern patterns such as Model-View-ViewModel (MVVM) and Model-View-Intent (MVI). These architectures promote separation of concerns, making apps easier to test and maintain. Kotlin's sealed classes and inline functions are particularly useful in implementing these patterns, enabling developers to create more expressive and type-safe code. Furthermore, Kotlin's compatibility with Jetpack Compose, Google's modern toolkit for building native UIs, has opened up new possibilities for declarative UI development, further enhancing the developer experience.
Security is a critical consideration in mobile app development, and Kotlin provides several features to help developers build secure applications. For instance, Kotlin's immutable collections and read-only properties encourage the use of immutable data structures, reducing the risk of unintended side effects. Additionally, Kotlin's support for encryption libraries and secure storage APIs ensures that sensitive data, such as user credentials and payment information, is protected. Developers can also leverage Kotlin's type-safe builders to create secure configurations for network requests and other critical operations.
The future of Kotlin in mobile app development looks promising, with ongoing advancements in the language and its ecosystem. The introduction of Kotlin/Native has expanded its capabilities to include iOS and desktop development, while Kotlin/JS enables developers to target web applications. These developments, combined with the language's growing community and support from major tech companies, suggest that Kotlin will continue to play a pivotal role in the evolution of mobile and cross-platform development.
In conclusion, Kotlin has redefined the landscape of Android app development, offering a modern, efficient, and secure alternative to traditional languages like Java. Its concise syntax, robust features, and interoperability with existing tools have made it a favorite among developers. As the mobile ecosystem continues to evolve, Kotlin's versatility and adaptability ensure that it will remain at the forefront of innovation, empowering developers to build the next generation of mobile applications.
Make order Tg Bot or Mobile app from us: @ChimeraFlowAssistantBot
Our portfolio: https://www.linkedin.com/company/chimeraflow
1 note
·
View note
Text
ID Lab Iddriver.cpp ID.cpp ID.h Solved
Introduction: This lab will create a class named ID which will be used by the main program called IDdriver.cpp. The objective of this lab is to create an object that initializes an 8 digit ID_number and a 9 character full_ID that begins with the character ‘A’ (example: “A12345678”). Declare all the methods and constructors (getters, setters, default constructors) in the .h file while defining…
0 notes
Text
Share tips for improving code quality and maintainability.
1. Follow Java Naming Conventions
Classes: Use PascalCase for class names (e.g., EmployeeDetails).
Methods/Variables: Use camelCase for method and variable names (e.g., calculateSalary).
Constants: Use uppercase letters with underscores for constants (e.g., MAX_LENGTH).
2. Use Proper Object-Oriented Principles
Encapsulation: Make fields private and provide public getters and setters to access them.
Inheritance: Reuse code via inheritance but avoid deep inheritance hierarchies that can create tightly coupled systems.
Polymorphism: Use polymorphism to extend functionalities without changing existing code.
3. Write Clean and Readable Code
Keep Methods Small: Each method should do one thing and do it well. If a method is too long or does too much, break it down into smaller methods.
Avoid Nested Loops/Conditionals: Too many nested loops or conditionals can make code hard to read. Extract logic into separate methods or use design patterns like the Strategy or State pattern.
4. Use Design Patterns
Leverage proven design patterns like Singleton, Factory, Observer, and Strategy to solve common problems in a standardized, maintainable way.
Avoid overcomplicating things; use patterns only when they add clarity and solve a specific problem.
5. Implement Proper Error Handling
Use exceptions appropriately. Don’t overuse them, and catch only the exceptions you can handle.
Ensure that exceptions are logged for better debugging and auditing.
Use custom exceptions to represent domain-specific issues, so they are easier to debug.
6. Utilize Java’s Stream API
The Stream API (introduced in Java 8) helps reduce boilerplate code when performing collection operations like filtering, mapping, and reducing.
It makes code more concise and expressive, which helps with readability and maintainability.
7. Write Unit Tests
Use JUnit and Mockito for unit testing and mocking dependencies.
Write test cases for all critical methods and components to ensure the behavior is as expected.
Use Test-Driven Development (TDD) to ensure code correctness from the start.
8. Use Dependency Injection
Prefer Dependency Injection (DI) for managing object creation and dependencies. This decouples components and makes testing easier (using tools like Spring Framework or Guice).
DI helps to make your classes more modular and improves maintainability.
9. Avoid Code Duplication
Use methods or utility classes to avoid repeating code.
If the same logic is used in multiple places, refactor it into a single reusable method.
10. Use Annotations
Use Java annotations (like @Override, @NotNull, @Entity, etc.) to improve code clarity and reduce boilerplate code.
Annotations help to enforce business logic and constraints without having to manually check them.
11. Leverage IDE Features
Use tools like IntelliJ IDEA or Eclipse to automatically format code and identify potential issues.
Many IDEs have integrated tools for running tests, refactoring code, and applying coding standards, so make full use of these features.
12. Optimize for Performance Without Sacrificing Readability
Only optimize performance when necessary. Premature optimization can lead to complex code that’s difficult to maintain.
Profile your code to identify bottlenecks, but prioritize clarity and maintainability over micro-optimizations.
13. Implement Proper Logging
Use a logging framework like SLF4J with Logback or Log4j2 for logging. This provides a consistent logging mechanism across the application.
Ensure that logs are meaningful, providing information about the application’s state, errors, and flow, but avoid excessive logging that clutters output.
14. Document Your Code
Use JavaDocs to generate documentation for public methods and classes.
Document not just the what but also the why behind critical decisions in the codebase.
15. Keep Your Codebase Modular
Break your project into smaller, well-defined modules, and avoid large monolithic classes.
Use packages to group related classes, ensuring that each class or module has a single responsibility.
16. Use Static Analysis Tools
Integrate tools like Checkstyle, PMD, and SonarQube to enforce coding standards, detect bugs, and ensure code quality.
These tools help you identify code smells and areas where quality can be improved.
0 notes
Text
Top 60 most asked Java interview questions: crack like hack
Top 60 most asked Java interview questions It gives this guide easy navigation through every possible concept which could assist you either as a beginner entering into the tech world or an experienced developer wanting to progress and learn technical questions in java. Although, we have blogs related to becoming java developer full guide , how to become graphic designer in 2025 and Java full stack developer course free : A complete guide check it out.
Core Java Concepts: A refresher course on OOP principles, collections, and exceptions. Advanced Topics: Understanding multithreading, design patterns, Java 8 features including lambda expressions and streams with Java code interview questions. Practical Scenarios: Real-world examples that make you shine during the technical discussions. Interview-Ready: Java code interview questions Solaractical examples and explanations to build unfaltering confidence.
What we have for you ? core java interview question with answers
💡 You will now be brimming with strength, ready to tackle not just the routine questions but also the toughest questions if any arise, impress the hiring managers, and, of course, get through the offer!
👉 So begin your journey to becoming the Java professional everyone wants to have on board.
What is Java? Certainly, Java is a high-level, class-based object-oriented programming language, with minimal implementation dependency. It operates on the principle of “write once, run anywhere.” That is, compiled Java code can be run on all those platforms that support Java without recompilation.”
What are the unique features of Java? -Dynamic -High
Performance -Secure
Robust
Distributed
Multithreaded
Platform-independent
Object-oriented
3.Difference between JDK, JRE, and JVM. -JDK: Java Development Kit: It contains the Java Runtime Environment and an assortment of development tools used for Java application development. -JRE: Java Runtime Environment. This is a part of a computer and thus is not a tool given for Java. It provides the set of libraries and JVM needed to run Java applications. -JVM: Java Virtual Machine. An abstraction of a computer that allows a computer to execute Java programs by converting bytecode into machine-specific code.
4,What do you understand by ClassLoader in Java? ClassLoader in Java is a component of the Java Runtime Environment that is responsible for dynamically loading Java classes into the Java Virtual Machine. A ClassLoader finds and loads the class files at runtime.
5.Explain Object-Oriented Programming (OOP)
OOP is the programming paradigm based on the idea of “objects,” containing both data and code to manipulate that data. Four key important principles:
Encapsulation -Inheritance
Polymorphism
Abstraction
6.What is inheritance in Java?
Inheritance is the process of taking attributes and behaviors from one class to another, It is the mechanism through which a new class (subclass) inherits from an existing one (superclass). Inheritance supports code reusability and creates a relationship between classes, i.e., a superclass-subclass relationship. Top 60 most asked Java interview questions is one of important question
7.Polymorphism in Java?
More simply, polymorphic methods work differently depending on the object invoking them. Thus, polymorphism is of two types: name polymorphism and method overriding.
Compile-time polymorphism (Method Overloading)
Runtime polymorphism (Method Overriding)
8. What is encapsulation in Java?
Certainly, Encapsulation wraps the data (variables) and the code (methods) together as a single unit. Also, Developers achieve this by making the variables private and providing public getter and setter methods. But restricts direct access to some of the object’s components, which can prevent an accident song of data.
9. What is abstraction in Java?
Abstraction refers to the preventing the viewing of the complex implementation details while showing only the essential features of an object. It can be implemented. through abstract classes and interfaces.
10. What is the difference between an abstract class and an interface?
An abstract class is the one with both abstract and concrete methods and can maintain state via instance variables. A class can inherit an abstract class. An interface only has abstract methods (until Java 8, which introduced default and static methods) and cannot maintain state. A class can implement multiple interfaces.
11. What is a constructor in Java?
A constructor is a special method. which is called when an object is created. It has the same name as the class and no return type. It can also be overloaded, meaning that one class can have multiple constructors that can accept different numbers of parameters.
12. What is the difference between method overloading Certainly, Method overloading introduces the same names to multiple methods in the same class. But Method overriding means that a subclass provides a specific implementation. for a method that was already defined in the superclass.
13. What is the ‘this’ keyword in Java?
Although, The ‘this’ keyword refers to the current instance of class. Also It is used to indicate access to class variables and methods, and it helps in distinguishing the class attributes and parameters with identical names. and Top 60 most asked Java interview questions is one of important question
14. What is the ‘super’ keyword in Java?
The ‘super’ keyword refers to the immediate parent class object and can be used to access superclass methods and constructors.
15. A different comparison is the ‘== operator’ and the ‘equals()’ method in Java.
‘== operator’: Reference Comparison. It checks whether both references point to the same object or not.
‘equals()’ method: Compares for equality of the actual contents of the objects.
16. What is a static variable and a static method?
Static Variable: The variable that is shared across all instances of a class. It relates more to the class than to any instance of it.
Static Method: Refers to methods that belong to a class rather than the instance of an object. They do not require an instance of a class to be called.
17. What are Java Collections? Framework is a name given to the entire collection of classes and interfaces forming commonly reusable collection data structures such as lists, sets, queues, and Maps.
18. What is the difference between an ArrayList and a LinkedList in Java?
ArrayList: Certainly, Use dynamic arrays to store elements; it will provide fast random access but will be slow on insertions and deletions, especially in the middle.
LinkedList: Use doubly linked lists to store elements. It provides for faster insertions and deletions, but slower random access.
19. What is a Map in Java? Although, A map is an object that maps keys to values. It does not permit duplicate keys, and each key can map to at most one value worldwide.
20.What is the difference between HashMap and TreeMap in Java?
HashMap: Implements the Map interface using
21.What is the difference between HashSet and TreeSet?
HashSet: Uses a hash table for storage; does not maintain any order of elements.
TreeSet: Implements the NavigableSet interface and uses a red-black tree to maintain elements in sorted order.
22. Explain the differences between List and Set in Java.
List: It allows duplicate elements and maintains insertion order. Set: Does not allow duplicate elements and does not guarantee any particular order.
23. Explain the differences between an array and an ArrayList.
Array: Fixed-size, which can store both primitives and objects. ArrayList: Resizable, storing only objects.
24. What does the final keyword do in Java?
Final Variable: Cannot change its value. Final Method: This Cannot be overridden. Final Class: Cannot be subclassed.
25. What is the difference between String, StringBuilder, and StringBuffer?
String: Immutable, thread-safe. StringBuilder: Mutable, not synchronized, faster than StringBuffer. StringBuffer: Mutable, thread-safe.
26. What is the purpose of the transient keyword in Java?
The transient keyword is used to indicate that a field should not be serialized.
27. What is a volatile keyword in Java?
It ensures all the changes to a variable are visible to all threads. Top 60 most asked Java interview questions is one of important question.
28. What are the differences between synchronized and lock in Java?
Synchronized: Implicit locking mechanism. Lock: Offers more control and flexibility in locking.
29. What is multithreading in Java? Multithreading allows concurrent execution of two or more threads for maximum utilization of CPU.
30. What are the states of a thread in Java?
New
Runnable
Blocked
Waiting
Timed Waiting
Terminated
31. What is the difference between wait(), notify(), and notifyAll()?
wait(): Pauses the thread and releases the lock.
notify(): Wakes up a single thread waiting on the object’s monitor.
notifyAll(): Wakes up all threads waiting on the object’s monitor.
32. What is garbage collection in Java? Garbage collection is the process of automatically reclaiming memory by removing unused objects.
33. What are the types of memory areas allocated by JVM?
Heap
Stack
Method Area
Program Counter Register
Native Method Stack
34. What are the differences between throw and throws?
throw: Used to explicitly throw an exception.
throws: Declares exceptions in the method signature.
35. What is the difference between checked and unchecked exceptions?
Checked Exceptions: Must be handled or declared in the method signature.
Unchecked Exceptions: Runtime exceptions that do not need to be explicitly handled.
36. What is an enum in Java?
Enums are special data types that define the list of constants.
37. What is reflection in Java?
Reflection is the ability to dynamically inspect and modify a class’s behavior during runtime.
38. What is the difference between shallow cloning and deep cloning?
Certainly, Shallow cloning, like cloning, copies the values of all fields without taking into account the objects referred to. But , Deep cloning is like for all fields, a brand new class instance is created at those places. Top 60 most asked Java interview questions is one of important question
39. What are the types of design patterns in Java?
Creational (e.g., Singleton, Factory) Structural (e.g., Adapter, Proxy) Behavioral (e.g., Observer, Strategy)
40. What is the Singleton design pattern?
A design pattern that restricts the instantiation of a class to just one object and provides a global point of access to it.
To know more click here
0 notes
Text
Difference between ES5 and ES6
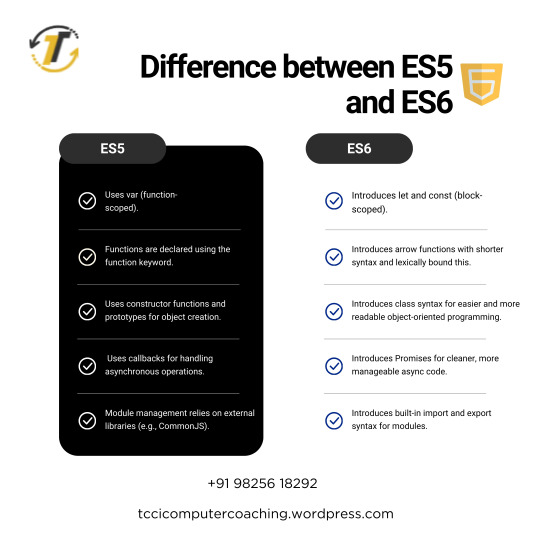
JavaScript is the must need programming language for both web and software engineering, and it keeps on changing with time. All developers should know the difference between ES5 and ES6 because major variations and advancements were made in those versions. ES5 started the foundation and had shown more advanced features in the version of ECMAScript 2015, also known as ES6. At TCCI Computer Coaching Institute, we ensure that each student understands these updates deeply so that they can be ahead in their programming journey.
What is ES5?
ES5, which stands for ECMAScript 5, was launched in 2009. This is the version of JavaScript which was seen to be stable, and it filled some of the most important holes that were there in the earlier versions. Key features of ES5 include:
Strict Mode: Strict mode allows developers to write secure and optimized code because it throws an error for all actions that are allowed in other versions.
JSON Support: ES5 provided support to work with JSON (JavaScript Object Notation), which is an essential feature for data interchange.
Array Methods: It introduced several new array methods, such as forEach(), map(), filter(), and reduce(), that simplify operations on arrays.
Getter/Setter Methods: ES5 allows the use of getter and setter methods, making it possible to control object properties.
What is ES6?
ES6, also commonly referred to as ECMAScript 2015, was a substantial update to the JavaScript language to include many new features as well as additions that make this language more powerful, concise, and much more modern in its expression. Some of its key features include:
Let and Const: There are let and const, added to declare a variable, with let offering better scoping than var, and const having no redefinition.
Arrow Functions: More concise syntax - also preserves this context.
Template Literals: Template literals ease string interpolation and multiline strings, and are much readable and less error-prone.
Classes: Classes, which are new in ES6, make the syntax for creating objects and handling inheritance much simpler than it used to be. It makes JavaScript resemble other more conventional object-oriented programming languages.
Promises: The promise is how asynchronous code works; promises handle success and failure states much easier.
Destructuring: This helps extract values from arrays and objects into separate variables in a simple syntax.
Modules: ES6 provided modules, where JavaScript code could be broken into reusable, maintainable pieces.
Key differences between ES5 and ES6
Variable declarations:
ES5 : Variables are declared using var.
ES6 : let and const keywords were introduced which provide block scoping and better predictability
Functions:
ES5: The function keyword is used to declare functions, and the this context can be a little tricky in some cases.
ES6: Arrow functions offer a more compact syntax and lexically bind the this keyword, avoiding common problems with this.
Object-Oriented Programming:
ES5: JavaScript uses prototype-based inheritance.
ES6: Introduces the class syntax, making object-oriented programming much more intuitive and easier to use.
Asynchronous Programming:
ES5: Callbacks are typically used for handling asynchronous code.
ES6: It introduced promises that made asynchronous operation cleaner.
Modules:
ES5 JavaScript does not natively support modules. Developers will use libraries such as CommonJS or RequireJS.
ES6 Native module support using the keywords import and export, which facilitates code organization and reusability
Template Literals:
ES5 String concatenation is done by using + operators, which can sometimes be error-prone and messy to read.
ES6 Template literals enable multi-line strings and interpolation of strings, enhancing readability.
Why Is It Important to Learn ES6?
At TCCI Computer Coaching Institute, we understand that staying updated with the latest advancements in JavaScript is crucial for your programming career. Learning ES6 is not just about getting familiar with new syntax, but also about adopting best practices that improve the quality and maintainability of your code.
As the JavaScript community shifts towards ES6 as the norm, most of the modern libraries and frameworks are built using the features of ES6. So, mastering ES6 will help you work with the latest technologies like React, Angular, and Node.js. The difference between ES5 and ES6 will also make you a more efficient developer in writing clean, efficient, and scalable code regardless of whether you are a beginner or a seasoned developer.
Learn ES6 with TCCI Computer Coaching Institute
We provide in-depth JavaScript training classes at TCCI Computer Coaching Institute that covers everything, starting from the basic knowledge of ES5 to the latest features added to ES6 and beyond. We ensure you have hands-on practice by giving practical exercise and real-world projects, along with individual guidance.
Learn at your pace with our online and offline classes.
Expert Trainers: Our trainers are industry experts with years of experience in teaching JavaScript and web development.
Real-World Applications: We focus on real-world programming challenges, ensuring that you are well-prepared for the job market.
It is one of the important steps in becoming a good JavaScript developer, as one should master the differences between ES5 and ES6. We provide the right environment and resources to learn and grow as a developer at TCCI Computer Coaching Institute. Join us today and start your journey toward mastering JavaScript!
Location: Ahmedabad, Gujarat
Call now on +91 9825618292
Get information from https://tccicomputercoaching.wordpress.com/
#Best Computer Training Institutes Bopal Ahmedabad#Best Javascript Course Training in Ahmedabad#Difference between ES5 and ES6#ES5 vs ES6#TCCI Computer Coaching Institute
0 notes
Link
JavaScript Class Syntax and Object-Oriented Programming Essentials
This article provides a comprehensive overview of JavaScript's class syntax and its various features introduced in ES6 (ES2015). It emphasizes the shift from the traditional function and prototype-based object-oriented programming to the more intuitive and structured class-based approach. The article covers key concepts such as class declaration, constructor methods for initialization, method definitions, instance methods, static methods, inheritance, method overriding, access modifiers, getter and setter methods, and static members.
By explaining the rationale behind using classes and highlighting their advantages, the article helps readers understand how class syntax in JavaScript enhances code organization, reusability, and modularity. It clarifies the distinction between instance methods and static methods, making it easier for developers to grasp their appropriate use cases. Furthermore, the article offers insights into inheritance and method overriding, providing practical examples to demonstrate how class relationships can be established.
In addition, the article delves into advanced topics such as access modifiers, getter and setter methods, and static members, shedding light on encapsulation and shared behavior in classes. Overall, the article equips developers with a solid foundation for utilizing class-based object-oriented programming in JavaScript effectively.
#JavaScript#class syntax#ES6#object-oriented programming#inheritance#access modifiers#getter and setter methods#static members#method overriding#code organization
0 notes
Text
purecode ai company reviews | Object Handling
Objects in JavaScript offer remarkable versatility. They can be created using object literals, constructor functions, or the Object.create() method. Properties can be accessed using dot notation or bracket notation and added to objects using these notations or through property assignment. Getters and setters can manage property access and assignment with functions.
#Object Handling#purecode software reviews#purecode ai reviews#purecode#purecode company#purecode reviews#purecode ai company reviews
0 notes
Text
Understanding Object-Oriented Programming (OOP) Concepts
Object-Oriented Programming (OOP) is a powerful programming paradigm used in many popular programming languages, including Java, Python, C++, and JavaScript. It helps developers organize code, promote reusability, and manage complexity more effectively. In this post, we'll cover the core concepts of OOP and why they matter.
What is Object-Oriented Programming?
OOP is a style of programming based on the concept of "objects", which are instances of classes. Objects can contain data (attributes) and functions (methods) that operate on the data.
1. Classes and Objects
Class: A blueprint or template for creating objects.
Object: An instance of a class with actual values.
Example in Python:class Car: def __init__(self, brand, model): self.brand = brand self.model = model def drive(self): print(f"The {self.brand} {self.model} is driving.") my_car = Car("Toyota", "Corolla") my_car.drive()
2. Encapsulation
Encapsulation is the bundling of data and methods that operate on that data within one unit (class), and restricting access to some of the object’s components.
Helps prevent external interference and misuse.
Achieved using private variables and getter/setter methods.
3. Inheritance
Inheritance allows one class (child/subclass) to inherit attributes and methods from another (parent/superclass). It promotes code reuse and logical hierarchy.class ElectricCar(Car): def charge(self): print(f"The {self.brand} {self.model} is charging.")
4. Polymorphism
Polymorphism means "many forms". It allows methods to do different things based on the object calling them. This can be achieved through method overriding or overloading.def start(vehicle): vehicle.drive() start(my_car) start(ElectricCar("Tesla", "Model 3"))
5. Abstraction
Abstraction means hiding complex implementation details and showing only essential features. It helps reduce complexity and increases efficiency for the user.
In Python, you can use abstract base classes with the abc module.
Why Use OOP?
Improves code organization and readability
Makes code easier to maintain and extend
Promotes reusability and scalability
Matches real-world modeling for easier design
Languages That Support OOP
Java
Python
C++
C#
JavaScript (with ES6 classes)
Conclusion
Object-Oriented Programming is a foundational concept for any serious programmer. By understanding and applying OOP principles, you can write cleaner, more efficient, and more scalable code. Start with small examples, and soon you'll be building full applications using OOP!
0 notes
Text
How do I create and use classes and objects in Dart?
How do I create and use classes and objects in Dart?
In Dart, creating and using classes and objects follows a similar structure to other object-oriented languages. Here’s a basic overview:
1. Creating a Class
A class is a blueprint for creating objects. It defines properties (variables) and methods (functions) that the objects created from the class will have.
Here’s an example of a simple class:
class Person {
String name;
int age;
Person(this.name, this.age);
void displayInfo() {
print('Name: $name, Age: $age');
}
}
In this class:
name and age are properties (also called fields or attributes).
The constructor Person(this.name, this.age) initializes the properties when the object is created.
displayInfo is a method that displays the person's name and age.
2. Creating an Object
Once you’ve defined a class, you can create objects from it. Objects are instances of the class and have access to the class’s properties and methods.
void main() {
Person person1 = Person('John Doe', 25);
print(person1.name);
person1.displayInfo();
}
3. Private Properties and Methods
In Dart, you can make properties and methods private by prefixing them with an underscore (_).
class Car {
String _model;
Car(this._model);
String getModel() {
return _model;
}
}
4. Getters and Setters
Getters and setters allow controlled access to class properties. Dart supports automatic getters and setters, but you can also define them explicitly.
class Rectangle {
double _width;
double _height;
Rectangle(this._width, this._height);
double get area => _width * _height;
set width(double value) {
_width = value;
}
set height(double value) {
_height = value;
}
}
5. Inheritance
In Dart, a class can inherit properties and methods from another class using the extends keyword.
dart
class Animal {
void makeSound() {
print('Animal makes a sound');
}
}
class Dog extends Animal {
@override
void makeSound() {
print('Dog barks');
}
}
void main() {
Dog dog = Dog();
dog.makeSound(); // Output: Dog barks
}
6. Abstract Classes and Interfaces
An abstract class is a class that cannot be instantiated. You use it as a base class that other classes inherit from. It can contain abstract methods (methods without implementation).
abstract class Shape {
double getArea();
}
class Circle extends Shape {
double radius;
Circle(this.radius);
@override
double getArea() => 3.14 * radius * radius;
}
void main() {
Circle circle = Circle(5);
print(circle.getArea()); // Output: 78.5
}
Summary
Class: A blueprint for creating objects.
Object: An instance of a class.
Constructor: A special method to initialize objects.
Methods: Functions inside a class.
Getters and Setters: Control how you access and set property values.
Inheritance: Reusing and extending functionality from another class.
Abstract Classes: Classes with unimplemented methods, serving as a template for subclasses.
You can use these building blocks to create more complex applications in Dart.
Hire Me: https://www.fiverr.com/s/Gzyjzoz
Facebook Profile: https://www.facebook.com/iamsusmoydutta
---------------------
Medium Profile :https://medium.com/@softcode9x/how-do-i-create-and-use-classes-and-objects-in-dart-2b2a2e39d422
---------------------
Behance Profile: https://www.behance.net/susmoydutta
---------------------
Linkedin Profile: https://www.linkedin.com/in/susmoy-dutta/
------------------
#flutter#softcode9x#susmoydutta#softwaresoftcode9x#FlutterApp#FlutterUi#Flutter#FlutterDeveloper#FlutterFirbase#Amanda#Paula#SusmoyDutta#infoosp#SoftCode9X#SoftCodeNinex#NineSwiftCode#SoftCodeQuick9#9XSoftPro#NineSpeedCode#FastCode9X#NineXSoft#SwiftSoft9X#9XVelocityCode#TurboSoft9#RapidCode9X#NineXAccel#SoftCodeRush9#9XHyperCode#NineXExpress#SoftCode9Jet
0 notes
Text
Code Your Vision into Reality: Java Mastery at Cyber Success Pune
Java programming continues to be a dominant force in the world of programming, offering a rich set of features and versatility for developers. Among its powerful tools, nested and inner classes stand out for their ability to enhance code organization and flexibility. Whether you're just starting your programming journey or looking to sharpen your skills, mastering these features is essential. With Cyber Success explores the concepts behind Java's nested and inner classes, their types, and their importance. Plus, if you’re eager to gain hands-on experience and in-depth knowledge, Cyber Success Institute’s Java course in Pune can guide you toward becoming a proficient Java developer.
Become a Java Expert: With Java Course in Pune at Cyber Success
In Java programming, a nested class is a class defined inside another class. This approach helps developers maintain clean and organized code by logically grouping related tasks. Nested classes enable external and internal classes to work together, reducing redundancy and promoting better code encapsulation. Nested classes can be further divided into static nested classes and inner classes (volatile). While static nested classes do not need instances of the outer class, inner classes always have an instance of the outer class, allowing direct access to their members.
Elevate Your Nested and Inner Classes Skills with Java Classes in Pune
There are many nested, inner-classes in Java. Each serves a specific purpose, allowing developers to manage relationships between classes more effectively. Understanding their implementation is key to writing clean and efficient Java code. Learn the basics of classes to their types with Java Course in Pune at Cyber Success and make your dream of IT career a reality.
These include:
Static Nested Classes: These are statically declared in the outer class and can only access static members of the outer class. It is typically used when the inner class does not need to have instance-level members of the outer class.
Volatile inner classes: These classes must be instances of the outer class. They have access to all areas and channels of the external team, making them ideal for cases where strong communication between the internal and external teams is required.
Local inner classes: Local inner classes are defined in a method or block of code within an outer class. They are accessible only in the form definition, which helps preserve the behavior required for only a small distance.
Anonymous Inner Classes: Anonymous classes are anonymous inner classes and are usually used for temporary operations like event handling. They allow developers to quickly implement interfaces or extend classes without having to use full class definitions.
Elevate Your Classes Potential at Cyber Success with Java Course in Pune with Placement
Nested and inner classes are an integral part of Java’s object-oriented design, and they provide many important benefits for Java developers:
Enhanced Code Organization: By combining related classes, you can make the structure of your code more readable and maintainable. This organization is particularly useful for large projects where the classes are closely related.
Improved Encapsulation: Since there are nested classes inside an outer class, it prevents unnecessary exposure of inner functionality. Adherence to these encapsulation principles contributes to a more secure and robust codebase.
Simplified Access to Outer Class Members: Central classes have access to all external class members, both private and public. This allows developers to eliminate unnecessary getter-setter methods and simplifies communication between the two classes.
Logical Grouping: Nested classes are useful when a class relates only to its outer class. This creates a strong and logical relationship between the classes and makes it easier to follow the rules.
Cleaner Code with Local and Anonymous Classes: This inner class facilitates the process of streamlining the code for specific local operations, such as concise scheduling in event listeners or time against smaller functions reducing the overall complexity of the code.
Code Your Vision into Reality: Achieve Java Mastery with Java Course in Pune at Cyber Success

At The Cyber Success Institute in Pune, India, we offer advanced Java Course in Pune with Placements designed to prepare you with the knowledge and skills needed to succeed in today's technology the rapid development. Our Java Classes in Pune include everything from basic object conversions to advanced topics like nested inner classes, multithreading, collections, and more. By the end of the Java Classes in Pune, you will have a solid foundation in Java and be ready to tackle it with real-world projects.
Expert Instructors: Instructors with extensive experience in Java programming and related technologies. Advanced degrees, industry certifications, and hands-on experience in real industry are required.
Advanced Course: A well-structured course in advanced foundational Java concepts. Topics include object-oriented programming, data structures, algorithms, frameworks such as Java, Spring and Hibernate, and software development best practices.
Free Aptitude Sessions: We believe soft skills are also essential for career development. Other sessions focused on developing problem-solving skills and logical thinking. These seminars are usually designed to help students improve their analytical abilities and excel in technical research.
Weekly Mock Interviews: A routine exercise where students can simulate a real job interview. These sessions provide information on technical questions, coding problems, and behavioral questions, and help students improve their interviewing skills.
Hands-on learning: Practical experience through coding exercises, projects, and labs. Students work on real-world applications, debugging, and coding challenges to develop a solid practical understanding of Java.
100% Recruitment Assistance: We provide 100% placement assistance to our students with their respected Java Course in Pune in securing job opportunities including resume building, interview preparation, and networking with potential employers. This usually involves collaborating with companies and a dedicated recruitment team.
Industry Networking: Opportunities to network with industry professionals through workshops, seminars and networking events. This allows students to build professional networks and gain insight into industry trends. Take the Next Step Toward a Bright Career with Java Classes in Pune Java programming continues to be an important skill for developers, providing opportunities in a variety of industries. By enrolling in the Cyber Success Institute Java course in Pune, you can build a solid foundation in Java and gain advanced knowledge that will set you apart from the competition. Whether you're a beginner looking to start your programming journey, or an experienced programmer aiming to improve your skills, this course is designed to meet your needs to be addressed
Take control of your future and join the best Java training in Pune at CyberSuccess Institute today! Let us help you achieve your career goals and become a Java development expert.
Attend 2 FREE Demo Sessions!
To learn more about the course in detail visit, https://www.cybersuccess.biz/all-courses/java-course-in-pune/
Secure your place at, https://www.cybersuccess.biz/contact-us/
📍 Our Visit: Cyber Success, Asmani Plaza, 1248 A, opp. Cafe Goodluck, Pulachi Wadi, Deccan Gymkhana, Pune, Maharashtra 411004
📞 For more information, call: 9226913502, 9168665644, 7620686761.
#software#education#programming#technology#training#itinstitute#career#software developer#software development#javascript#coding#htmlcoding
0 notes