#hashset examples
Explore tagged Tumblr posts
Text
How are hashCode and equals methods related?
In Java, the hashCode() and equals() methods play a critical role in determining object equality and behavior in hash-based collections like HashMap, HashSet, and Hashtable.
The equals() method is used to compare two objects for logical equality. By default, the equals() method in the Object class compares memory references. However, in most custom classes, this method is overridden to provide meaningful comparison logic—such as comparing object content (fields) rather than memory addresses.
The hashCode() method returns an integer representation of an object’s memory address by default. However, when overriding equals(), it is essential to also override hashCode() to maintain the general contract:
If two objects are equal according to the equals() method, then they must have the same hashCode() value.
Failing to do this can lead to unexpected behavior in collections. For instance, adding two logically equal objects (via equals()) to a HashSet may result in duplicates if hashCode() returns different values for them. This is because hash-based collections first use the hashCode() to find the correct bucket, and then use equals() to compare objects within the same bucket.
Example:
@Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null || getClass() != obj.getClass()) return false; MyClass other = (MyClass) obj; return this.id == other.id; } @Override public int hashCode() { return Objects.hash(id); }
In summary, always override both methods together to ensure correct object behavior in collections. A strong grasp of these concepts is crucial for building reliable applications and is a core topic in any full stack Java developer course.
0 notes
Text
Key Concepts to Review Before Your Java Interview
youtube
Java interviews can be both challenging and rewarding, often acting as a gateway to exciting roles in software development. Whether you're applying for an entry-level position or an advanced role, being well-prepared with core concepts is essential. In this guide, we’ll cover key topics to review before your Java interview, ensuring you're confident and ready to impress. Additionally, don't forget to check out this detailed video guide to strengthen your preparation with visual explanations and code demonstrations.
1. Object-Oriented Programming (OOP) Concepts
Java is known for its robust implementation of OOP principles. Before your interview, make sure to have a firm grasp on:
Classes and Objects: Understand how to create and use objects.
Inheritance: Review how subclasses inherit from superclasses, and when to use inheritance.
Polymorphism: Know the difference between compile-time (method overloading) and runtime polymorphism (method overriding).
Abstraction and Encapsulation: Be prepared to explain how and why they are used in Java.
Interview Tip: Be ready to provide examples of how you’ve used these concepts in your projects or coding exercises.
2. Core Java Concepts
In addition to OOP, there are foundational Java topics you need to master:
Data Types and Variables: Understand primitive types (int, double, char, etc.) and how they differ from non-primitive types.
Control Structures: Revise loops (for, while, do-while), conditional statements (if-else, switch-case), and how they control program flow.
Exception Handling: Know how try, catch, finally, and custom exceptions are used to manage errors in Java.
Collections Framework: Familiarize yourself with classes such as ArrayList, HashSet, HashMap, and their interfaces (List, Set, Map).
Interview Tip: Be prepared to discuss the time and space complexities of different collection types.
3. Java Memory Management
Understanding how Java manages memory can set you apart from other candidates:
Heap vs. Stack Memory: Explain the difference and how Java allocates memory.
Garbage Collection: Understand how it works and how to manage memory leaks.
Memory Leaks: Be prepared to discuss common scenarios where memory leaks may occur and how to avoid them.
Interview Tip: You may be asked how to optimize code for better memory management or to explain how Java’s finalize() method works.
4. Multithreading and Concurrency
With modern applications requiring multi-threading for efficient performance, expect questions on:
Threads and the Runnable Interface: Know how to create and run threads.
Thread Lifecycle: Be aware of thread states and what happens during transitions (e.g., from NEW to RUNNABLE).
Synchronization and Deadlocks: Understand how to use synchronized methods and blocks to manage concurrent access, and how deadlocks occur.
Concurrency Utilities: Review tools like ExecutorService, CountDownLatch, and Semaphore.
Interview Tip: Practice writing simple programs demonstrating thread synchronization and handling race conditions.
5. Java 8 Features and Beyond
Many companies expect candidates to be familiar with Java’s evolution, especially from Java 8 onward:
Lambda Expressions: Know how to write concise code with functional programming.
Streams API: Understand how to use streams for data manipulation and processing.
Optional Class: Learn to use Optional for handling null checks effectively.
Date and Time API: Review java.time package for managing date and time operations.
Interview Tip: Be prepared to solve coding problems using Java 8 features to show you’re up-to-date with recent enhancements.
6. Design Patterns
Java interviews often include questions on how to write clean, efficient, and scalable code:
Singleton Pattern: Know how to implement and when to use it.
Factory Pattern: Understand the basics of creating objects without specifying their exact class.
Observer Pattern: Be familiar with the publish-subscribe mechanism.
Decorator and Strategy Patterns: Understand their practical uses.
Interview Tip: Have examples ready that demonstrate how you’ve used these patterns in your projects.
7. Commonly Asked Coding Problems
Prepare by solving coding problems related to:
String Manipulations: Reverse a string, find duplicates, and check for anagrams.
Array Operations: Find the largest/smallest element, rotate arrays, or merge two sorted arrays.
Linked List Questions: Implement basic operations such as reversal, detecting cycles, and finding the middle element.
Sorting and Searching Algorithms: Review quicksort, mergesort, and binary search implementations.
Interview Tip: Practice on platforms like LeetCode or HackerRank to improve your problem-solving skills under time constraints.
Final Preparation Tips
Mock Interviews: Conduct practice interviews with peers or mentors.
Review Your Code: Ensure your past projects and code snippets are polished and ready to discuss.
Brush Up on Basics: Don’t forget to revise simple concepts, as interviews can include questions on any level of difficulty.
For more in-depth preparation, watch this helpful video that provides practical examples and coding tips to boost your confidence.
With these concepts in mind, you'll be well-equipped to handle any Java interview with poise. Good luck!
0 notes
Text
hi
fizzbuzz reverse string implement stack
convert integer to roman numeral longest palindrome substring
design hashset
Java group by sort – multiple comparators example https://leetcode.com/discuss/interview-question/848202/employee-implementation-online-assessment-hackerrank-how-to-solve
SELECT SUBQUERY1.name FROM (SELECT ID,name, RIGHT(name, 3) AS ExtractString FROM students where marks > 75 ) SUBQUERY1 order by SUBQUERY1.ExtractString ,SUBQUERY1.ID asc ;
SELECT *
FROM CITY
WHERECOUNTRYCODE = 'USA' AND POPULATION > 100000;
Regards
Write a simple lambda in Java to transpose a list of strings long value to a list of long reversed. Input: [“1”,”2”,”3”,”4”,”5”] output: [5,4,3,2,1] 2. Write a Java Program to count the number of words in a string using HashMap.
Sample String str = "Am I A Doing the the coding exercise Am" Data model for the next 3 questions:
Write a simple lambda in Java to transpose a list of strings long value to a list of long reversed. Input: [“1”,”2”,”3”,”4”,”5”] output: [5,4,3,2,1] 2. Write a Java Program to count the number of words in a string using HashMap.
Sample String str = "Am I A Doing the the coding exercise Am" Data model for the next 3 questions:
Customer :
CustomerId : int Name : varchar(255)
Account :
AccountId : int CustomerId : int AccountNumber : varchar(255) Balance : int
Transactions : Transactionid : int AccountId: int TransTimestamp : numeric(19,0) Description : varchar(255) Amount(numeric(19,4)) 3. Write a select query to find the most recent 10 transactions. 4. Write a select query, which, given an customer id, returns all the transactions of that customer. 5. What indexes should be created for the above to run efficiently? CustomerId, AccountId 6. Write a program to sort and ArrayList.
Regards
0 notes
Text
The Ultimate Guide to Java Collection
Java libraries are indispensable tools that streamline development by providing pre-written code for common tasks. "The Ultimate Guide to Java Libraries" explores a myriad of libraries that enhance Java programming, from handling data structures to implementing complex algorithms.
A key feature covered is collections in Java, which offer efficient ways to manage groups of objects, improving code efficiency and readability.
TpointTech is a valuable resource for developers seeking in-depth tutorials and examples on using these libraries effectively. Leveraging these libraries can significantly reduce development time and improve application performance.
Overview of Java Collections
The Java Collections Framework includes interfaces, implementations, and algorithms. The core interfaces include Collection, List, Set, Queue, and Map, each serving different purposes.
Collection Interface:
The root interface of the framework, representing a group of objects known as elements. It is extended by List, Set, and Queue interfaces.
List Interface:
An ordered collection that allows duplicate elements. Common implementations are ArrayList, LinkedList, and Vector. Lists are ideal when you need to access elements by their index.
ArrayList: Resizable array implementation, offering constant-time positional access but slower for insertion and deletion.
LinkedList: Doubly-linked list implementation, providing efficient insertion and deletion but slower access time.
Vector: Synchronized version of ArrayList, rarely used due to performance overhead.
Set Interface:
A collection that does not allow duplicate elements. It models mathematical sets and provides implementations like HashSet, LinkedHashSet, and TreeSet.
HashSet: Uses a hash table for storage, offering constant-time performance for basic operations.
LinkedHashSet: Maintains insertion order, slightly slower than HashSet.
TreeSet: Implements the SortedSet interface, ensuring elements are in ascending order, based on their natural ordering or a specified comparator.
Queue Interface:
Designed for holding elements prior to processing, typically ordered in a FIFO (first-in-first-out) manner. Common implementations include LinkedList, PriorityQueue, and ArrayDeque.
PriorityQueue: Elements are ordered according to their natural ordering or a provided comparator, useful for creating priority-based tasks.
ArrayDeque: Resizable-array implementation of the Deque interface, providing efficient insertion and deletion from both ends.
Map Interface:
Represents a collection of key-value pairs, where each key maps to one value. Popular implementations are HashMap, LinkedHashMap, and TreeMap.
HashMap: Provides constant-time performance for basic operations, assuming a good hash function.
LinkedHashMap: Maintains a doubly-linked list of its entries, preserving the order of insertion.
TreeMap: Implements the SortedMap interface, ensuring keys are in ascending order.
Advantages of Java Collections Framework
Reduces Programming Effort: With a set of ready-made data structures and algorithms, JCF eliminates the need for developers to implement complex data structures from scratch.
Increases Program Speed and Quality: Standardized interfaces and optimized implementations ensure high performance and reliability.
Interoperability: Collections can be easily passed across APIs, reducing the complexity of integration.
Ease of Maintenance: Well-documented and widely-used classes make it easier for developers to maintain and enhance code.
Common Algorithms in JCF
Java Collections Framework includes various algorithms to perform routine tasks, such as sorting, searching, and shuffling. These algorithms are static methods in the Collections utility class.
Sorting: Collections.sort(List list), sorts the specified list into ascending order.
Shuffling: Collections.shuffle(List list), randomly permutes the elements in the list.
Searching: Collections.binarySearch(List> list, T key), performs binary search on a sorted list.
Conclusion
The Java Collections Framework is indispensable for any Java developer. It offers a standardized and efficient way to manage groups of objects, making code more robust and maintainable.
By leveraging the various interfaces and implementations, such as lists, sets, queues, and maps, developers can handle data structures effectively.
Understanding collections in Java, as detailed on resources like TpointTech, is crucial for building high-performance applications. Whether you're a beginner or an experienced developer, mastering Java collections will significantly enhance your programming capabilities.
0 notes
Text
Java Topics Demystified: Everything You Need To Know

Introduction:
Java, a stalwart in the programming world, is a cornerstone for developers due to its robustness, versatility, and platform independence. For beginners and seasoned programmers alike, understanding the core topics in Java can significantly enhance one's ability to create efficient and maintainable code. This article demystifies essential Java topics, providing a comprehensive overview to guide your learning journey. Enrolling in in-depth Java Training In Chennai is a great way for anyone looking to improve their programming abilities to get real-world experience and knowledge relevant to the business.
Object-Oriented Programming (OOP)
At the heart of Java lies Object-Oriented Programming (OOP), a paradigm that organizes software design around data or objects rather than functions and logic. The four fundamental principles of OOP in Java are:
Encapsulation: This is grouping variables (data) and functions (methods) that manipulate the data into a class unit. Encapsulation helps protect data from unauthorised access and modification.
Inheritance: Java allows a class to inherit the properties and methods of another class. This promotes code reuse and establishes a natural hierarchical relationship between classes. For example, a `Car` class can be inherited from a `Vehicle` class.
Polymorphism: Polymorphism enables objects to be treated as instances of their parent class rather than their actual class. The two types of polymorphism in Java are compile-time (method overloading) and runtime (method overriding).
Abstraction: According to this concept, only the object's most important features should be displayed, concealing the intricate implementation details. Java achieves abstraction through the usage of abstract classes and interfaces.
Data Structures And Collections
The Java Collections Framework (JCF) is the aggregate name for Java's extensive collection of built-in data structures. Understanding these data structures is crucial for effective programming.
- List: an arranged grouping that may include duplicate items. Common implementations include `LinkedList` and `ArrayList` classes.
- Set: A collection that does not allow duplicate elements. The `HashSet` and `TreeSet` classes are popular choices.
- Map: a mapping object that prevents duplicate keys and associates keys with values. The implementations `HashMap} and `TreeMap} are commonly used.
These structures help manage and organise data efficiently, enabling faster retrieval and manipulation.
Exception Handling
Exception handling is a technique to manage runtime problems and maintain the program's normal flow. Java provides five keywords for exception handling:
- try: To specify a block of code to be tested for errors.
- catch: To handle the exception if it occurs.
- throw: To explicitly throw an exception.
- throws: To declare an exception that might be thrown by a method.
Proper exception handling makes your code more robust and error-resistant.
Concurrency
Concurrency in Java is achieved through multithreading, where multiple threads run concurrently within a program. Key concepts include:
- Thread Class: Extending the `Thread` class to create a new thread.
- Runnable Interface: Implementing the `Runnable` interface to define the code executed by the thread.
- Synchronisation: Managing the access of multiple threads to shared resources to prevent data inconsistency.
Understanding concurrency is vital for writing efficient, high-performance applications.
Java Development Tools
Mastering Java involves familiarity with development tools and environments that streamline coding, debugging, and deployment processes. Notable tools include:
- Integrated Development Environments (IDEs): Eclipse, IntelliJ IDEA, and NetBeans offer comprehensive support for Java development.
- Build Tools: Maven and Gradle automate project build, dependency management, and more.
- Version Control Systems: Git is essential for collaborative development and version tracking.
- If you want to enhance your programming skills, enrolling in a reputable Software Training Institute In Chennai can provide you with the necessary knowledge and hands-on experience.
Conclusion:
Java's vast ecosystem offers many features that cater to various programming needs. By mastering OOP principles, data structures, exception handling, concurrency, and essential development tools, you can harness the full potential of Java to build robust, scalable, and efficient applications. Maintaining proficiency in this dynamic language requires constant study and practice, whether a beginner or an expert.
0 notes
Text
Java Collections Framework: A Comprehensive Guide
The Java Collection Framework (JCF) is one of the most important features of the Java programming language. It provides a unified framework for representing and managing collections, enabling developers to work more efficiently with data structures. Whether you are a beginner or an experienced Java developer, understanding the Java collection system is important. This comprehensive guide will delve into the basic design and implementation of the Java compilation system, highlighting its importance in modern Java programming. The basics of the Java collections framework At its core, the Java collections in Java with Examples consist of interfaces and classes that define collections. These collections can contain objects and provide functions such as insertion, deletion, and traversal. The main features of JCF include List, Set, and Map. Lists are ordered collections of objects, aggregates are unordered collections without duplicate objects, and maps are key-value pairs. Significant interactions with classes in the Java collection system List Interface: Lists in JCF are implemented by classes like ArrayList and LinkedList. ArrayList provides dynamic arrays, allowing quick access to elements, while LinkedList uses a doubly linked list internally, making insertion and deletion faster Set interfaces: Represent classes such as sets, HashSet and TreeSet, and do not allow duplicate elements. HashSet uses hashing techniques for fast access, while TreeSet maintains objects in sorted order, enabling efficient retrieval. Map Interface: Maps are represented by HashMap and TreeMap. HashMap uses hashing to store key-value pairs and provides a constant-time display for basic processing. TreeMap, on the other hand, maintains elements in a sorted tree structure, enabling operations in logarithmic time. Advantages of Java Collections Framework The concurrent Collections in Java Framework offers several benefits to developers: Re-usability: Pre-implemented classes and interfaces let developers focus on solving specific problems without worrying about downstream data structures Interactivity: Collections in JCF can store object by object, encouraging interactivity and allowing developers to work with multiple data types. Performance: The system is designed to be efficient. The algorithm is implemented in such a way that it is efficient in terms of time and memory consumption. Scalability: JCF supports scalability, allowing developers to handle large amounts of data without worrying about memory limitations. Frequent use of information in the Java collections system Data Storage and Retrieval: Lists, sets, and maps are widely used to store and retrieve data efficiently. Lists are suitable for sorted collections, aggregates for unique elements, and maps for key-value pairs. Algorithm Implementation: Java Collections Framework can be used to implement many algorithms such as search and sort. This simplifies the coding process and reduces the possibility of error. Concurrent control: Classes like ConcurrentHashMap and CopyOnWriteArrayList provide concurrent access to collections, ensuring thread safety in multi-threaded applications. Best practices for Java collections systems with examples 1. List Interface (ArrayList):import java.util.ArrayList;import java.util.List; public class ListExample {public static void main(String args) {List list = new ArrayList(); // Adding elements to the list list.add("Java"); list.add("Python"); list.add("C++"); // Accessing elements using index System.out.println("Element at index 1: " + list.get(1)); // Iterating through the list System.out.println("List elements:"); for (String language : list) { System.out.println(language); } // Removing an element list.remove("Python"); System.out.println("List after removing 'Python': " + list); } } 2. Set Interface (HashSet) import java.util.HashSet;import java.util.Set; public class SetExample {public static void main(String args) {Set set = new HashSet(); // Adding elements to the set set.add("Apple"); set.add("Banana"); set.add("Orange"); // Iterating through the set System.out.println("Set elements:"); for (String fruit : set) { System.out.println(fruit); } // Checking if an element exists System.out.println("Contains 'Apple': " + set.contains("Apple")); // Removing an element set.remove("Banana"); System.out.println("Set after removing 'Banana': " + set); } 3. Map Interface (HashMap):import java.util.HashMap;import java.util.Map; public class MapExample {public static void main(String args) {Map map = new HashMap(); // Adding key-value pairs to the map map.put("Java", 1); map.put("Python", 2); map.put("C++", 3); // Iterating through the map System.out.println("Map elements:"); for (Map.Entry entry : map.entrySet()) { System.out.println(entry.getKey() + ": " + entry.getValue()); } // Checking if a key exists System.out.println("Contains key 'Java': " + map.containsKey("Java")); // Removing a key-value pair map.remove("Python"); System.out.println("Map after removing 'Python': " + map); } Conclusion: In Java programming, the Java collections tutorial framework stands as the cornerstone of efficient data manipulation. Its versatile interfaces and classes empower developers to create complex applications, handling data structures with ease. Understanding the nuances of different types of collections, their functionality, and best practices is important for enabling Java developers aiming to build high-performance, scalable, and error-free applications Java collection concepts has been optimized to enable developers to unlock the full programming capabilities of Java Read the full article
0 notes
Text
"Coding in Java with Confidence: A Step-by-Step Mastery Guide"
Java is one of the most popular and widely used programming languages and platforms, offering a versatile environment for developing and executing programs across various domains. It is renowned for its speed, reliability, and robust security features. Its ease of learning, uncomplicated syntax, and roots in C++ make it accessible to programmers of different backgrounds. Java simplifies memory management through its automatic garbage collector, which efficiently cleans up unused objects.
For Beginners commencing on the Path to Java Mastery, This In-Depth Step-by-Step Guide Serves as Your Companion:
Understanding Java: Before venturing forth, it's imperative to answer fundamental questions about Java, such as its nature, reasons for popularity, and distinctive features. Gain clarity on Java by exploring informative articles that not only provide insights but also guide you on the path to effective learning.
Java Environment: A solid understanding of the environment in which Java operates is essential. Familiarize yourself with the intricacies of this environment, including the Java Virtual Machine (JVM). Discover more about the JVM, its architecture, and operational mechanisms by reading this informative article.
Java Programming Basics: Proficiency in any programming language begins with a strong grasp of its fundamentals. Explore into the basics of Java programming through articles that provide in-depth knowledge in a clear and concise format.
Object-Oriented Programming (OOPs) in Java: Java is a prime example of an object-oriented programming (OOP) language. To master Java, it's essential to understand OOP concepts, which simplify program structure by breaking it down into manageable objects.
Classes and Objects in Java: Classes and objects form the foundational concepts of object-oriented programming in Java. They represent real-world entities and serve as the building blocks for Java programming.
Constructors in Java: To efficiently harness the power of Classes and Objects, a comprehensive understanding of Constructors in Java is indispensable. Constructors play a vital role in initializing an object's state. Much like methods, constructors consist of a set of instructions executed at the time of object creation.
Methods in Java: Methods in Java encompass collections of statements designed to execute specific tasks and return results to the caller. Methods can perform specific tasks without necessarily returning values.
Strings in Java: Strings, defined as arrays of characters in Java, exhibit a unique ease of implementation compared to other programming languages. Even beginners can navigate the intricacies of Java's string handling.
Arrays in Java: Arrays in Java differ significantly in their functionality from those in C/C++.
Collections in Java: Collections in Java encapsulate individual objects into cohesive units. Java's Collection Framework furnishes a comprehensive set of classes and interfaces to facilitate the effective management of object collections.
Generics in Java: Generics in Java bear similarities to templates in C++. They empower the parameterization of methods, classes, and interfaces with types, including user-defined types. Classes such as HashSet, ArrayList, and HashMap effectively leverage generics, accommodating various data types.
Stream API in Java: The Stream API, introduced in Java 8, emerges as a potent tool for processing collections of objects. A stream represents a sequence of objects supporting a range of methods that can be pipelined to achieve specific outcomes.
Exceptions and Exception Handling in Java: Exception handling emerges as a recurring theme throughout the learning journey of Java. Unwanted or unexpected events, termed "EXCEPTIONs," may disrupt the orderly progression of program instructions during runtime.
Regular Expressions (Regex) in Java: While the term "Regular Expression" may appear unfamiliar, its significance in development cannot be overstated.
Multithreading in Java: Multithreading constitutes a distinctive Java feature facilitating concurrent execution of multiple program segments to maximize CPU utilization.
File Handling in Java: Java extends its support for file handling, enabling users to manage files by reading, writing, and engaging in various other file-related operations.
Packages in Java: In Java, packages serve as a mechanism for encapsulating a cluster of classes, sub-packages, and interfaces.
If you're interested in expanding your knowledge of Java, consider exploring ACTE Technologies. Their instructors are highly skilled and adept at teaching. You have the flexibility to learn Java online or in a traditional classroom setting. Java learning program at ACTE Technologies provides certifications and job placement assistance. In summary, mastering Java demands patience, dedication, and consistent practice. Staying committed to enhancing your skills is crucial. With sustained effort, you can become proficient in the language and leverage it to develop diverse applications and projects.
0 notes
Text
How to optimize memory in Java?

Optimizing memory usage in Java training near me is essential to ensure efficient and responsive applications while minimizing the risk of memory-related issues like Out Of Memory Errors. Here are several strategies to optimize memory in Java
Use Data Structures Wisely
Choose the appropriate data structures for your application's needs. Efficient data structures can significantly reduce memory consumption.
For example, use ArrayList when the size is dynamic but known in advance and HashSet or HashMap when you need fast lookup operations.
Minimize Object Creation
Excessive object creation can lead to high memory usage and increased garbage collection overhead. Reuse objects whenever possible.
Consider using object pooling or object reclamation techniques to reduce object churn.
String Handling
Be mindful of string concatenation operations, as they can create many temporary string objects. Use StringBuilder or StringBuffer for efficient string concatenation.
If you have many identical strings, consider using string interning to reuse string instances.
Avoid Memory Leaks
Be cautious about holding references to objects longer than necessary. Ensure that objects are eligible for garbage collection when they are no longer needed.
Use weak references or soft references when appropriate to allow objects to be collected more easily.
Use Primitive Data Types
Whenever possible, use primitive data types (int, float, char, etc.) instead of their object counterparts (Integer, Float, Character, etc.) to save memory.
Array Optimization
Use arrays instead of collections (e.g., ArrayList) when the size is known and fixed, as arrays have a smaller memory overhead.
Be cautious with multi-dimensional arrays, as they can consume more memory than expected due to padding.
Memory Profiling
Use memory profiling tools to identify memory leaks and memory-hungry parts of your application. Tools like VisualVM or YourKit can help pinpoint memory issues.
Garbage Collection Tuning
Tune the garbage collection settings using JVM flags (e.g., -Xmx, -Xms, -XX:MaxHeapFreeRatio, -XX:MinHeapFreeRatio, etc.) to optimize heap memory management.
0 notes
Text
Mastering C# Collections: Enhance Your Coding Skills and Streamline Data Management
As a developer, it is essential to have a solid understanding of data management in programming languages. In C#, collections play a crucial role in efficiently organizing and manipulating data. Collections are containers that allow you to store and retrieve multiple values of the same or different types. They provide powerful ways to manage data, improve code readability, and enhance overall coding skills.
Benefits of using collections in C
Using collections in C# offers several benefits that contribute to better coding practices and streamlined data management. Firstly, collections provide a structured approach to store and organize data, making it easier to access and manipulate specific elements. Unlike traditional arrays, collections offer dynamic resizing, allowing you to add or remove elements as needed, without worrying about size limitations.
Secondly, collections provide a wide range of built-in methods and properties that simplify common data operations. For example, you can easily sort, filter, or search elements within a collection using predefined methods. This saves time and effort in writing custom algorithms for such operations.
Thirdly, collections support type safety, ensuring that you can only store elements of specific types within a collection. This helps prevent runtime errors and enhances code reliability. Additionally, collections allow you to iterate over elements using loops, making it easier to perform batch operations or apply transformations to each element.
Understanding different collection types in C
C# offers a variety of collection types, each designed for specific use cases. Let's explore some of the most commonly used collection types in C# and understand their characteristics:
Arrays: Arrays are the most basic collection type in C#. They provide a fixed-size structure to store elements of the same type. Arrays offer efficient memory allocation and fast access to elements, but they lack dynamic resizing capabilities.
Lists: Lists, represented by the List<T> class, are dynamic collections that can grow or shrink based on the number of elements. They provide methods to add, remove, or modify elements at any position within the list. Lists are widely used due to their flexibility and ease of use.
Dictionaries: Dictionaries, represented by the Dictionary<TKey, TValue> class, store key-value pairs. They enable fast retrieval of values based on a unique key. Dictionaries are ideal for scenarios where you need to quickly access elements by their associated keys.
Sets: Sets, represented by the HashSet<T> class, store unique elements without any specific order. They provide methods to add, remove, or check for the existence of elements efficiently. Sets are useful when you need to perform operations like union, intersection, or difference between multiple collections.
Queues: Queues, represented by the Queue<T> class, follow the First-In-First-Out (FIFO) principle. Elements are added to the end of the queue and removed from the front, maintaining the order of insertion. Queues are commonly used in scenarios where you need to process items in the order of their arrival.
Stacks: Stacks, represented by the Stack<T> class, follow the Last-In-First-Out (LIFO) principle. Elements are added to the top of the stack and removed from the same position. Stacks are useful when you need to implement algorithms like depth-first search or undo/redo functionality.
Exploring C# generic collections
C# also provides a powerful feature called generic collections, which allow you to create strongly typed collections. Generic collections are parameterized with a specific type, ensuring type safety and eliminating the need for explicit type casting. Let's explore some commonly used generic collection types in C#:
List: Generic lists provide the flexibility of dynamically resizing collections while ensuring type safety. You can create a list of any type by specifying the desired type within angle brackets. For example, List<int> represents a list of integers, and List<string> represents a list of strings.
Dictionary: Generic dictionaries store key-value pairs, similar to non-generic dictionaries. However, generic dictionaries provide type safety and better performance. You can specify the types of keys and values when creating a dictionary. For example, Dictionary<string, int> represents a dictionary with string keys and integer values.
HashSet: Generic hash sets store unique elements without any specific order. They provide efficient lookup, insertion, and removal operations. You can create a hash set of any type by specifying the desired type within angle brackets. For example, HashSet<string> represents a hash set of strings.
Queue: Generic queues follow the First-In-First-Out (FIFO) principle, similar to non-generic queues. They ensure type safety and provide methods to enqueue and dequeue elements. You can create a queue of any type by specifying the desired type within angle brackets. For example, Queue<int> represents a queue of integers.
Stack: Generic stacks follow the Last-In-First-Out (LIFO) principle, similar to non-generic stacks. They ensure type safety and provide methods to push and pop elements. You can create a stack of any type by specifying the desired type within angle brackets. For example, Stack<string> represents a stack of strings.
By utilizing generic collections, you can write cleaner and more robust code, eliminating potential runtime errors and enhancing code maintainability.
Sample C# codes for working with collections
To illustrate the usage of collections in C#, let's explore some sample code snippets that demonstrate common operations:
Working with Lists:
List<string> fruits = new List<string>();
fruits.Add("Apple");
fruits.Add("Banana");
fruits.Add("Orange");
Console.WriteLine("Total fruits: " + fruits.Count);
foreach (string fruit in fruits)
{
Console.WriteLine(fruit);
}
if (fruits.Contains("Apple"))
{
Console.WriteLine("Apple is present in the list.");
}
fruits.Remove("Banana");
Console.WriteLine("Total fruits after removing Banana: " + fruits.Count);
Working with Dictionaries:
Dictionary<string, int> ages = new Dictionary<string, int>();
ages.Add("John", 25);
ages.Add("Emily", 30);
ages.Add("Michael", 35);
Console.WriteLine("Age of John: " + ages["John"]);
foreach (KeyValuePair<string, int> entry in ages)
{
Console.WriteLine(entry.Key + ": " + entry.Value);
}
if (ages.ContainsKey("Emily"))
{
Console.WriteLine("Emily's age: " + ages["Emily"]);
}
ages.Remove("Michael");
Console.WriteLine("Total entries after removing Michael: " + ages.Count);
These code snippets demonstrate basic operations like adding elements, iterating over collections, checking for element existence, and removing elements. Modify and experiment with these code snippets to understand the behavior of different collection types and their methods.
Examples of common use cases for collections in C
Collections in C# find applications in various scenarios. Let's explore some common use cases where collections prove to be invaluable:
Data storage and retrieval: Collections provide a convenient way to store and retrieve data. For example, you can use a list to store a collection of customer details, a dictionary to store key-value pairs representing configuration settings, or a queue to manage incoming requests.
Sorting and searching: Collections offer built-in methods for sorting and searching elements. You can easily sort a list of objects based on specific properties or search for elements that meet certain criteria. Collections eliminate the need for writing complex sorting or searching algorithms from scratch.
Batch processing and transformations: Collections allow you to iterate over elements using loops, enabling batch processing and transformations. For example, you can apply a discount to each item in a list, convert a list of strings to uppercase, or filter out elements based on specific conditions.
Efficient memory management: Collections provide dynamic resizing capabilities, ensuring efficient memory utilization. Unlike arrays, which have a fixed size, collections automatically resize themselves based on the number of elements. This prevents unnecessary memory allocation or wastage.
Concurrency and thread safety: Collections in C# offer thread-safe alternatives, ensuring safe access and manipulation of data in multi-threaded environments. For example, the ConcurrentDictionary<TKey, TValue> class provides thread-safe operations for dictionary-like functionality.
By leveraging the power of collections, you can simplify complex data management tasks, improve code readability, and enhance the overall efficiency of your C# applications.
Comparing C# collection vs list
One common question that arises when working with collections in C# is the difference between a collection and a list. While a list is a specific type of collection, there are some key distinctions to consider:
Collections: In C#, the term "collection" refers to a general concept of a container that stores and organizes data. Collections encompass various types like arrays, lists, dictionaries, sets, queues, and stacks. Collections provide a higher-level abstraction for data management and offer a range of operations and properties that can be applied to different scenarios.
List: A list, on the other hand, is a specific type of collection provided by the List<T> class in C#. It offers dynamic resizing capabilities, allowing you to add or remove elements as needed. Lists provide methods to insert, remove, or modify elements at any position within the list. Lists are commonly used due to their flexibility and ease of use.
In summary, a list is a type of collection that offers dynamic resizing and additional methods for element manipulation. Collections, on the other hand, encompass a broader range of container types, each designed for specific use cases.
Best practices for efficient data management using collections
To utilize collections effectively and ensure efficient data management in C#, consider the following best practices:
Choose the appropriate collection type: Select the collection type that best suits your specific use case. Consider factors like data size, performance requirements, element uniqueness, and the need for sorting or searching operations. Choosing the right collection type can significantly impact the efficiency of your code.
Use generics for type safety: Whenever possible, utilize generic collections to ensure type safety. By specifying the type of elements stored in a collection, you can eliminate potential runtime errors and improve code maintainability. Generic collections also eliminate the need for explicit type casting.
Prefer foreach loops for iteration: When iterating over elements in a collection, prefer the foreach loop over traditional indexing with a for loop. Foreach loops provide a more concise syntax and handle underlying details like bounds checking and iteration logic automatically.
Consider performance implications: Be mindful of performance implications, especially when dealing with large data sets. For example, using a List<T> for frequent insertions or removals at the beginning of the list may result in poor performance. In such cases, consider using a LinkedList<T> or other suitable collection type.
Dispose disposable collections: If you are using collections that implement the IDisposable interface, ensure proper disposal to release any unmanaged resources. Wrap the usage of such collections in a using statement or manually call the Dispose() method when you are done working with them.
By following these best practices, you can optimize your code for efficient data management and enhance the overall performance of your C# applications.
Advanced techniques for optimizing collection performance
While collections in C# are designed to provide efficient data management out of the box, there are advanced techniques you can employ to further optimize collection performance:
Preallocate collection size: If you know the approximate number of elements that will be stored in a collection, consider preallocating the size using the constructor or the Capacity property. This eliminates unnecessary resizing operations and improves performance.
Avoid unnecessary boxing and unboxing: Boxing and unboxing operations, where value types are converted to reference types and vice versa, can impact performance. Whenever possible, use generic collections to store value types directly, eliminating the need for boxing and unboxing.
Implement custom equality comparers: If you are working with collections that require custom equality checks, consider implementing custom equality comparers. By providing a specialized comparison logic, you can improve the performance of operations like searching, sorting, or removing elements.
Use parallel processing: In scenarios where you need to perform computationally intensive operations on collection elements, consider utilizing parallel processing techniques. C# provides the Parallel class and related constructs to parallelize operations, taking advantage of multi-core processors.
Profile and optimize: Regularly profile your code to identify performance bottlenecks. Use tools like profilers to measure execution times and memory usage. Once identified, optimize the critical sections of your code by employing appropriate algorithms or data structures.
By employing these advanced techniques, you can further enhance the performance of your C# collections and optimize your code for maximum efficiency.
Conclusion and next steps for mastering C# collections
In this article, we explored the world of C# collections and their significance in enhancing your coding skills and streamlining data management. We discussed the benefits of using collections in C#, understanding different collection types, and explored generic collections for strong typing. We also provided sample code snippets and examples of common use cases for collections.
Furthermore, we compared collections to lists, outlined best practices for efficient data management, and explored advanced techniques for optimizing collection performance. By following these guidelines, you can harness the full power of C# collections and elevate your coding skills to the next level.
To master C# collections, continue practicing with different types of collections, experiment with advanced scenarios, and explore additional features and methods provided by the .NET framework. Keep exploring the vast possibilities offered by collections, and strive to write clean, efficient, and maintainable code.
Start your journey to mastering C# collections today and witness the transformation in your coding skills and data management capabilities.
Next Steps: To further enhance your skills and understanding of C# collections, consider exploring the official Microsoft documentation on .NET collections and data structures. Additionally, practice implementing collections in real-world scenarios and collaborate with fellow developers to exchange ideas and best practices. Happy coding!
0 notes
Text
LinkedHashSet Class in Java With Program Example
LinkedHashet is an implementation class of the Set interface which also extends the HashSet class. It was introduced in Java 1.4 version. It behaves in the same manner as HashSet except that it preserves or maintains the insertion order of elements inserted in the set. In simple words, It is an implementation of the set interface that stores and manipulates an ordered collection of elements. It…
View On WordPress
#collection#collection framework#data structure#HashTable#java#java program#Linked List#LinkedHashSet
0 notes
Text
RADIX scrypto 1
Accounts on the Radix network are actually Scrypto components that hold resource containers and define rules for accessing them.
You can instantiate a new account component in the simulator with the resim new-account
This will give you back the created account’s address, public key and private key.
Additionally, at any time, you can find the current default account with the command resim show-configs.
You can send tokens from the default account to another one by running the command:
resim transfer [amount] [resource_address] [recipient_address]
Let’s verify the balance of the second account. You can query the state of an address with the resim show [address] command.
resim show resource_sim1qyqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqs6d89k
to easily create a boilerplate Scrypto package with basic functionality. To do this, simply type the following command scrypto new-package [package_name].
Directory Structure
At the root of the generated package directory, you should find the src, and tests folders plus a Cargo.toml file.
The tests folder is where you write the integration tests for your package. You can run the tests located in this directory with the scrypto test command.
All packages must have a lib.rs file - which is the starting point of your Scrypto package.
You can think of blueprints and components like classes and objects in object-oriented programming.
When you create a new project with the scrypto new-package command, it creates a Scrypto package. A simple package, like the HelloToken example, will only contain a single blueprint but you could “package” together multiple blueprints that need each-other to work when deploying them on the ledger. That way, you are certain that all your blueprints are deployed at the same time and the platform knows that those blueprints are related to each other.
(즉 하나의 팩키지에 여러개의 블루프린트가 있을수있으며 하나의 팩키지안의 여러 블루프린트는 서로 연관되어있다고 여겨진다)
#[blueprint]. A blueprint definition must contain a struct and an impl section with the name of the blueprint
impl ���락 안에
you will write the functions callable on the blueprint itself and the methods callable on the instantiated components.
It’s important to make a distinction between functions and methods:
Functions can be called on the blueprints and do not have access to any state.
Methods on the other hand are callable on individual components and are able to get data from the state and update or mutate it.
위는 function 의 한 예시
위는 method의 한 예시
&mut self가 전달되면 메소드 가 된다
Safe Types
These types are just like the u8, i8, u16, etc. but they differ in the fact that they panic (and make the transaction fail) if an overflow occurs when doing operations on them. You define these types by using an uppercase U for unsigned numbers and uppercase I for signed numbers:
I8, I16, I32, I64, I128, I256, I384, I512
U8, U16, U32, U64, U128, U256, U384, U512
They also have the benefit of offering 384 bits and 512 bits options instead of a maximum of 128 bits.
Strings
The String type is supported in Scrypto.
Floating points
the Rust types f32 and f64 representing floating point numbers are not supported in Scrypto. Instead, we offer the Decimal and PreciseDecimal types that allow you to represent numbers with a fractional part using fixed point arithmetic (which is deterministic).
you have to wrap the number in quotes (as seen for c and e in the previous example). If you don’t, you will not be able to compile your package because it will detect the use of floating point numbers.
The Decimal type supports a maximum of 18 decimal places -if you need more you should use PreciseDecimal which supports up to 64 decimal places:
Collections
Scrypto supports the basic Rust collections like Vec, HashMap and HashSet. We also provide a new type KeyValueStore which is like a HashMap, but it does not load the full content of the Map until you request it. Here are some examples:
Scrypto Types
The PackageAddress, ComponentAddress and ResourceAddress types are used to work with the different address types. The Scrypto types you will work with the most are the Bucket and Vault types
Creating Custom Types
You create a custom type by writing a struct outside of the blueprint! definition. It is important to remember to add the line #[scrypto(ScryptoCategorize, ScryptoEncode, ScryptoDecode, LegacyDescribe)] before the struct block. This adds, at compile time, methods to the type to make it compatible with the Radix Engine. Here is an example:
To test our blueprint locally we will use resim. You may recall we saw how to use resim to create accounts and transfer tokens in the simulator. By running the resim --help command you will see that you can do much more with resim. In our case, we will use it to publish our package to the local simulator, instantiate our component, and call a method to interact with it.
To ensure we have a clean slate to begin with let’s run resim reset and then generate a new default account by running resim new-account be sure to save the account address as we will want to inspect it later. You can save this as an environment variable for convenience or just create a file for your own reference to copy and paste.
The next step is to build and deploy the package. You can do that with the following command: resim publish . (make sure you are in the hello_token directory!).
To create an instance of our deployed blueprint we will use the package address that we just received to instantiate our component by calling the instantiate_hello function on the Hello blueprint by running
resim call-function [package_address] [blueprint_name] [function]
which will look something like this:
resim call-function package_sim1qxxkyjgkyryztmtlsypzd8244xkvqeln32aqdersgk3szcwxzq Hello instantiate_hello
After running the call-function command above, you will get various information about the transaction that was executed.
Now that we have an instantiated Hello component, all the methods defined by its blueprint are exposed to us and callable. We are going to call the free_token method on it with a resim command resim call-method [component_address] [method]
token도 resource 중의 한가지 이다
Resources are built into the platform
Resources on Radix need to always be placed in some kind of resource containers. Resource containers, as the name suggest, hold resources. Each resource container can only hold one type of resource and the purpose of these resource containers are to properly move, secure, and account for the resources that are being transacted. There are two types of resource containers: Bucket and Vault.
Bucket - Buckets are temporary containers and are used to move resources during a transaction; therefore, only exist in the duration of the transaction.
Vault - Vaults are permanent containers and at the end of each transaction, all resources must be stored in a Vault.
Scrypto offers a handful of utilities to conveniently create and manage resources.
ResourceBuilder - The ResourceBuilder is used to create Fungible and NonFungible resources.
ResourceManager - When a resource is created, a ResourceManager is also created to manage the resource.
The ResourceBuilder is a Scrypto utility that we use to create Fungible and NonFungible resources. Below is a table summarizing the methods provided by the ResourceBuilder for Fungible resources.
Notice that we have fields such as color and rarity. These values are a selection of enums and if you're not familiar with enums, the Rust handbook provides a section on enums.
Creating a NonFungible Resource
Creating a NonFungible resource is a little different from creating a Fungible resource as we saw in the earlier section. To give some context, when we create a NonFungible resource, the collection of the NonFungible resource will have one ResourceAddress. However, each individual NonFungible that we decide to mint will have its own unique identifiers called NonFungibleLocalId. We have an option of data types we can declare to be our NonFungibleLocalId, but it is a requirement to declare a type.
아래는 integer를 id 로 사용하는 경우이다
Creating NFTs with a Mutable Supply
You can create NFTs with no specified initial supply. To do so, we would need to create a badge that will be held by the component Vault.
Viewing, Utilizing, and Mutating NonFungibleData
full example code
0 notes
Text
Set, HashSet, LinkedHashSet, and TreeSet in Java with Sample Programs | Java Collections
Set, HashSet, LinkedHashSet, and TreeSet in Java with Sample Programs | Java Collections
Get a nearer glimpse at Established, HashSet, LinkedHashSet, and TreeSet in Java with sample systems. [Video] by · Sep. 28, 21 · Java Zone · Presentation Resource url
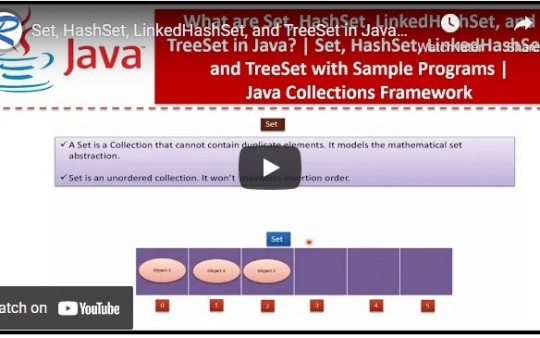
View On WordPress
0 notes
Text
Top Java Interview Questions You Should Know
Preparing for a Java interview can be daunting, especially when you're unsure of what to expect. Mastering common Java questions is crucial for making a lasting impression. This blog covers the top Java interview questions you should know and provides tips for answering them effectively. For a more interactive learning experience, check out this Java interview preparation video, which breaks down key concepts and interview strategies.
1. What is Java?
Answer: Java is a high-level, object-oriented programming language developed by Sun Microsystems (now owned by Oracle). It is designed to have as few implementation dependencies as possible, allowing developers to write code that runs on all platforms supporting Java without the need for recompilation.
Pro Tip: Mention the "write once, run anywhere" (WORA) principle during your interview to emphasize your understanding of Java’s cross-platform capabilities.
2. What is the Difference Between JDK, JRE, and JVM?
Answer:
JDK (Java Development Kit): Contains tools for developing Java applications, including the JRE and compilers.
JRE (Java Runtime Environment): A subset of JDK, containing libraries and components required to run Java applications.
JVM (Java Virtual Machine): The part of the JRE responsible for executing Java bytecode on different platforms.
Pro Tip: Explain how these components interact to demonstrate a deeper understanding of Java's execution process.
3. Explain OOP Principles in Java
Answer: Java is based on four main principles of Object-Oriented Programming (OOP):
Encapsulation: Bundling data and methods that operate on the data within one unit (class).
Inheritance: Creating a new class from an existing class to promote code reuse.
Polymorphism: The ability of a method or function to behave differently based on the object calling it.
Abstraction: Hiding complex implementation details and showing only the necessary features.
Pro Tip: Use a real-world example to illustrate these principles for better impact.
4. What are Constructors in Java?
Answer: Constructors are special methods used to initialize objects in Java. They have the same name as the class and do not have a return type. There are two types:
Default Constructor: Automatically created if no other constructors are defined.
Parameterized Constructor: Accepts arguments to initialize an object with specific values.
Pro Tip: Highlight the differences between constructors and regular methods, and explain constructor overloading.
5. What is the Difference Between == and .equals() in Java?
Answer:
==: Used to compare primitive data types or check if two object references point to the same memory location.
.equals(): Used to compare the content within objects. This method should be overridden for custom comparison logic in classes.
Pro Tip: Demonstrating this concept with code snippets can be a game-changer in your interview.
6. What are Java Collections?
Answer: The Java Collections Framework (JCF) provides a set of classes and interfaces to handle collections of objects. Commonly used collections include:
List (e.g., ArrayList, LinkedList)
Set (e.g., HashSet, TreeSet)
Map (e.g., HashMap, TreeMap)
Pro Tip: Be prepared to discuss the performance differences between various collections and when to use each.
7. What is Exception Handling in Java?
Answer: Exception handling in Java involves managing runtime errors to maintain normal program flow. The main keywords used are:
try: Block to wrap code that might throw an exception.
catch: Block to handle the exception.
finally: Block that always executes, used for cleanup code.
throw and throws: Used to manually throw an exception and indicate that a method may throw an exception, respectively.
Pro Tip: Discuss custom exceptions and when it is appropriate to create them for better code design.
8. What is Multithreading in Java?
Answer: Multithreading is a feature in Java that allows concurrent execution of two or more threads. It is useful for performing multiple tasks simultaneously within a program.
Pro Tip: Familiarize yourself with the Thread class and Runnable interface. Highlight synchronization and thread-safe practices to show advanced understanding.
9. What are Lambda Expressions in Java?
Answer: Introduced in Java 8, lambda expressions provide a concise way to implement functional interfaces. They enable writing cleaner, more readable code for single-method interfaces (e.g., using a lambda to sort a list).
Example:
java
Copy code
List<String> list = Arrays.asList("apple", "banana", "cherry");
list.sort((a, b) -> a.compareTo(b));
Pro Tip: Mention how lambda expressions contribute to functional programming in Java.
10. What is the Significance of the final Keyword?
Answer: The final keyword can be used with variables, methods, and classes to restrict their usage:
Variables: Makes the variable constant.
Methods: Prevents method overriding.
Classes: Prevents inheritance.
Pro Tip: Explain how using final can improve security and design integrity in your applications.
Conclusion
Reviewing these questions and understanding their answers can prepare you for technical interviews. For additional explanations and examples, check out this detailed Java interview preparation video.
youtube
0 notes
Conversation
Goal-Oriented Behaviours in Artificial Intelligence for Games
Often times, game developers will want to create an AI unit that is looking to accomplish a specific goal or set of goals. In games such as Hyrule Warriors, AI characters attempt to accomplish any given goal, stopping along the way to fight any enemies that hinder their progress. In this case, each character is given an end “destination”, then runs through a Finite State Machine (FSM) to determine when an enemy is prevent the character from progressing. For something as simple as “go to this location, stopping to kill enemies along the way”, FSMs work extremely well. They’re relatively simple to program, relatively efficient for these small-scale projects, and tend to work really well for multiple units that run the same functionality. However, in games that are constantly expanding and have to implement new features, such as F.E.A.R., the AI units also need to be able to adapt. This is where Goal Oriented Behaviours (GOB) come in.
GOBs take FSMs, which have defined entry and exit transitions between two (static) states and completely decouple the code tying any two states together. As such, each “state” (which will now be referred to as an “action”) has preconditions that are required in order to run and effects that are activated once the action has been completed. In implementing the system this way, we can easily add or remove features as we see fit, meaning that the system can be easily expanded for future development without potentially destroying the pre-existing framework. Orkin describes this process that F.E.A.R. uses perfectly, mentioning the
To begin with, every action shares a few characteristics. With this in mind, creating a singular abstract base class that each action will stem from makes the most sense. Each action will have some form of target, which is normally a location where the action will be performed. Additionally, each action should contain a cost (although it’s not necessary) and a handful of abstract functions, such as checking if an action needs to be within a defined range, if the action can be run, and a function to reset the action once it’s been completed. Finally, the base class should also contain a definition for functions to add preconditions and effects. To store each precondition and effect, I’d recommend using HashSets of KeyValuePairs or . It’s an extremely simple way of handling expanding lists of complicated data and works relatively efficiently.
Each class should also be a subclass of the base action class and implement the abstract functions defined above. Additionally, each action's constructors should add each precondition and effect that action requires and completes. With that out of the way, we can now create our behaviour tracker.
GOBs work exceptionally well when used as a state within a FSM. As such, we can create a “GOBState” class, which basically begins running the GOB. This state class knows the other necessary states of the FSM (move, idle, perform), the set of available actions, and an empty queue that will contain the list of actions to execute. When a specific state is to be executed, the GOBState class moves to a “Planner” class, which determines how the AI’s current goal will be achieved. Similar to most pathfinding algorithms, it takes cost into account, determining the cheapest way to accomplish the goal. The algorithm begins by finding the goal within a created tree of possible paths. Afterward, it navigates the tree to the highest node, then sends the result to the GOBState class as a Queue, which will then execute the given commands. The tree itself is created by putting each effect as a leaf, then adding the actions that cause those effects as a parent of that leaf.
The best example of this method can be viewed through Brent Owens’ demo, where multiple AI units have various actions they have to achieve, with their main objective being to collect resources, deposit them, and repair/obtain tools if deemed necessary. A lot of the understanding comes from the article (shown below), so I would highly recommend diving through both his demo and post for extra clarification.
References
Chaudhari, Vedant. “Goal Oriented Action Planning.” Medium, Medium, 12 Dec. 2017, https://medium.com/@vedantchaudhari/goal-oriented-action-planning-34035ed40d0b.
Orkin, Jeff. Three States and a Plan; The A.I. of F.E.A.R. 2006, http://alumni.media.mit.edu/~jorkin/gdc2006_orkin_jeff_fear.pdf.
Owens, Brent. “Goal Oriented Action Planning for a Smarter AI.” Game Development Envato Tuts , 23 Apr. 2014, https://gamedevelopment.tutsplus.com/tutorials/goal-oriented-action-planning-for-a-smarter-ai--cms-20793.
1 note
·
View note
Text
Complete Core Java Topics List | From Basic to Advanced Level [2021]
Attention Dear Reader, In this article, I will provide you a Complete Core Java Topics List & All concepts of java with step by step. How to learn java, when you need to start, what is the requirement to learn java, from where to start learning java,and Tools to Understand Java code. every basic thing I will include in this List of java.
core java topics list Every beginner is a little bit confused, at the starting point before learning any programming language. But you no need to worry about it. Our aim to teach you to become a good programmer. In below you can start with lesson 1 or find out the topics manually. it’s your choice.
Complete Core Java Topics List.
Chapter. 1Full Basic Introduction of java.1.1What is java?1.2Features if java?1.3What is Software?1.4What are Programs?1.5Need to know about Platforms.1.6Object Orientation.1.7What are objects in java?1.8Class in java.1.9Keywords or Reserved words of java.1.10Identifiers in java.1.11Naming convention / coding Standard.1.12Association (Has-A Relationship).1.13Aggregation and Composition in java.1.14Java Compilation process.1.15WORA Architecture of java.1.16What is JVM, JRE and JDK in java?Chapter. 2Classes and Objects in Detail2.1How to create Class?2.2How to Create Objects?2.3Different ways to create objects in class.2.4object Reference / object Address.2.5Object States and Behavior.2.6Object Creation & Direct Initialization.2.7Object initialization using Reference.Chapter. 3Data Types in Java.3.1Java Primitive Data Types.3.2Java Non-Primitive Data Types.Chapter. 4Instance Method.4.1Types of methods in java.4.2Rules of Methods.4.3How to use methods in a class.Chapter. 5Class Loading in Java?5.1What is Class Loading.5.2Class Loading Steps.5.3Program Flow explanation.5.4"this" Keyword in java?Chapter. 6Variables in java.6.1Types of Variables.6.2Scope of variables.6.3Variable initialization.Chapter. 7Variable Shadowing in Java?Chapter. 8 Constructors in java.8.1Rules for creating java Constructors.8.2Types of Construstors.8.3Difference between Constructor and method in java.Chapter. 9Categories of methods in java.9.1Abstract Method in java.9.2Concrete Method in java.Chapter. 10Overloading in java.10.1Types if Overloading.10.2Advantages and Disadvantages of overloading.10.3Can we overload main( ) method in java?10.4Constructor Overloading in java.Chapter. 11Inheritance in java?11.1Types of Inheritance.11.2Terms used in inheritance.11.3The syntax of java inheritance.11.4How to use Inheritance in java/Chapter. 12Overriding in java.12.1Usage of java method Overriding.12.2Rules of method Overriding.12.3Importance of access modifiers in overriding.12.4Real example of method Overriding.12.5Difference between method overriding and overloading java.12.6"Super" keyword in java.Chapter. 13Constructor chaining in java.13.1Generalization in java with Example.Chapter. 14Type-Casting in java.14.1Primitive and Non-Primitive Casting in java.14.2Data Widening and Data Narrowing in java.14.3Up-Casting in java.14.4Down-Casting in java.14.5Characteristics of Up-Casting.Chapter. 15Garbage Collection.15.1Advantages of Garbage Collection.15.2How can an object be unreferanced in java?15.3Some examples of Garbage Collection in java.Chapter. 16Wrapper Class in java.16.1Uses of wrapper class in java.16.2How to Convert Primitive form to object form.16.3How to Convert String form to Primitive form.16.4Learn public static xxx parsexxx(Strings srop).16.5Autoboxing in java.16.6Unboxing in java.Chapter. 17Polymorphism in java?17.1Compile time polymorphism.17.2Runtime Polymorphism.Chapter. 18Packages in java?18.1Advantages of Packages in java.18.2Standard Package structure if java Program.18.3How to access package from another package?18.4Inbuilt package in java?18.5What are Access Modifiers In java.18.6Types of Access Modifires.Chapter. 19Encapsulation in java.19.1Advantages of Encapsulation in java?19.2Java Bean Specification or Guidelines.Chapter. 20Abstraction in java.20.1What are abstract methods in java?20.2Abstract Class in java?20.3Difference between Abstract class and Concrete class.20.4Similarities between Abstract and Concrete class?20.5Interface in java?20.6Why use java interface?20.7How to declare an interface in java?20.8Types of Interface in java?20.9What are the uses of Abstraction?20.10Purpose or Advantages of Abstraction.Chapter. 21Collection Framework in java?21.1What is collection in java?21.2What is framework in java?21.3Advantages of collection over an array in java.21.4Collection Framework Hierarchy in java.21.5Generics in java?21.6List Interface in java.21.7Methods of List Interfae in java.21.8ArrayList in java?21.9Vector in java.21.10Difference between ArrayList and Vector.21.11LinkedList in Java.21.12Difference between ArrayList and LinkedList.21.13Iterating Data from List.21.14foreach Loop in java.21.15Difference between for loop and foreach loop.21.16Set Interface in java.21.17Has-based Collection in java.21.18What are HashSet in java?21.19What are LinkedHashSet in java?21.20What are TreeSet in Java?21.21Iterator interface in java.21.22Iterator Methods in java.21.23ListIterator interface in java.Chapter. 22What are Map in java?22.1Map interface method in java.22.2What are HashMap in java?22.3What are LinkedHashMap in java?22.4What are TreeMap in java?22.5HashTable in java?22.6ShortedMap in java?22.7Difference between HashMap and Hashtable in java?22.8Comparable and Comparator in java?22.9Where Comparable and Comparator is used in java?22.10What is Comparable and Comparator interface in java?Chapter. 23Exception Handling in Java.23.1Exception Hierarchy in java.23.2Checked and Unchecked Exception in java?23.3Java Try Block?23.4java catch Block?23.5Multi-catch block in java?23.6Sequence of catch Block in java?23.7Finally Block in java?23.8"throws" keyword in java?23.9"throw" keyword in java?23.10Difference between throw and throws in java.23.11Custom and user defined exception in java?23.12Difference between final, finally and finalize in java?Chapter. 24Other Advanced Topics24.1Advanced Programming Java Concepts"👆This is the Complete Core Java Topics List"
How to Learn Java Step by Step?
We know that java is the most popular programming language on the internet. and also know this is the most important language for Android Development. with the help of java, we can develop an Android application. All over the topics will helps you to understated the basic concepts to learn java step by step. which helps you to understand the structure of any application backend code for app development. we recommended you to do more practice of all concepts and topics list. which is given over to becoming a good java developer. Must Read: What is Java, Full Introduction of Java for Beginners with Examples?
What is a Programming Language?
In short, Any software language. which is used in order to build a program is called a programming language. Java is also an object-oriented programming language. using that we can develop any type of software or web application. Is it easy to learn Java?
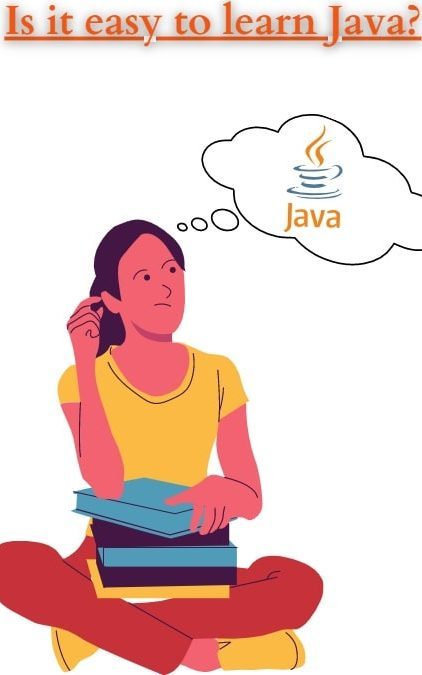
Is it easy to learn Java? My answer is "yes". Java is a beginner-friendly programming language. Because Every beginner easily learns java programming language. that means you don't need to give much effort to learn. you can start from a lower level to a higher level. Also, it provides you a user-friendly environment to develop software for clients. it has a garbage collector, which helps a lot to manage the memory space of your system for better performance. Tools to Understand Java Code. The market has lots of tools for programming. as a beginner, I will be recommending some Cool tools for Java Programming, which helps you to write and execute codes easily. 1. Eclipse: This is one of my favorite tools for practicing java codes. it will provide an integrated development environment for Java. Eclipse proved us so many interesting & modern features, modeling tools, testing tools. and frameworks development environment.
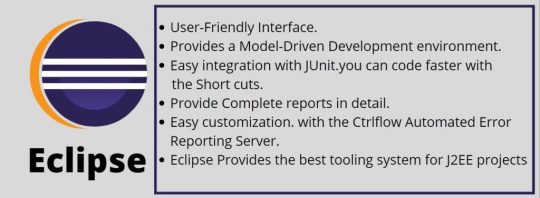
this Tools helps you to Understand Java Code. Here is Some Features of Eclipse: - User-Friendly Interface. - Provides a Model-Driven Development environment. - Easy integration with JUnit. - you can code faster with the Short-cuts. - Provide Complete reports in detail. - easy customization. with the Ctrlflow Automated Error Reporting Server. - Eclipse Provides the best tooling system for J2EE projects. - You can Download free. 2. JUnit: it is an open source unit for testing tool for Java programs. It is test-driven development and software deployment.

this Tools helps you to Understand Java Code. Here is Some Features of JUnit: - Prepare for input data and setup. - Some creation of fake objects. - Automatic Loading databases with a specific set of data - offers annotations, that test classes fixture, that's run before or after every test - support for write and execute tests on the platform. - Have some annotations to identify test methods - we can write codes faster, which increases the quality of codes and practices. - You can Download free Also Read: How to Download and Install Java JDK 15 in Windows 10 ? How longs to learn java? Every beginner student had pinged this question. How longs to learn java? now I will tell you according to the research. the speed of learning technologies and related subjects depends on the regularity of studies and the initial capacity of every student. I know you can do anything with your initial capacity level. but the regular study is a big responsibility of every student. I will recommend practicing daily to improve your programming skills and java concepts knowledge. and get stay motivated with your goals. definitely it will help you to get your first job. Note:- if any person has a little bit of programming knowledge, honestly it would take around 1 month to learn the basic concepts of java. if you are from a non-programming background but have a good knowledge of basic mathematics. definitely, you can also learn and understand fast all the concepts of java. it will all depend on your problem-solving skills and understanding concepts. Conclusions: At the starting of the article, I will provide you a complete core java topics list. it will help you to understand, where you need start, this is the basic step for a beginner. and also provides some tools for your practicing. I hope now you can start lean java with you motivation. Admin Word:- In this article, I provided A Complete Core Java Topics List. and also give your all pinged questions answers such as How to Learn Java Step by Step?, What is a Programming Language, Is it easy to learn Java, How longs to learn java. Also provides some free Tools to Understand Java Code which helps to Learn Java Step by Step. if you have any questions regarding this article please feel free to ask in the comment below of this article. Read the full article
0 notes
Text
HashSet Class in Java With Program Example
Set interface doesn’t provide any additional method, as a result, implementation classes use only collection interface methods. HashSet is an implementing class of Set interface and it represents Hash Table as its underlying data structure. It is a collection of unordered unique elements that don’t allow duplicates. It also doesn’t preserve insertion order as it uses hash code to store an…
View On WordPress
#collection#Collection interface#Hash Table#HashSet#HashSet class#java#java program#Set#Set interface
0 notes