#How to optimize memory in Java?
Explore tagged Tumblr posts
Text
How to optimize memory in Java?

Optimizing memory usage in Java training near me is essential to ensure efficient and responsive applications while minimizing the risk of memory-related issues like Out Of Memory Errors. Here are several strategies to optimize memory in Java
Use Data Structures Wisely
Choose the appropriate data structures for your application's needs. Efficient data structures can significantly reduce memory consumption.
For example, use ArrayList when the size is dynamic but known in advance and HashSet or HashMap when you need fast lookup operations.
Minimize Object Creation
Excessive object creation can lead to high memory usage and increased garbage collection overhead. Reuse objects whenever possible.
Consider using object pooling or object reclamation techniques to reduce object churn.
String Handling
Be mindful of string concatenation operations, as they can create many temporary string objects. Use StringBuilder or StringBuffer for efficient string concatenation.
If you have many identical strings, consider using string interning to reuse string instances.
Avoid Memory Leaks
Be cautious about holding references to objects longer than necessary. Ensure that objects are eligible for garbage collection when they are no longer needed.
Use weak references or soft references when appropriate to allow objects to be collected more easily.
Use Primitive Data Types
Whenever possible, use primitive data types (int, float, char, etc.) instead of their object counterparts (Integer, Float, Character, etc.) to save memory.
Array Optimization
Use arrays instead of collections (e.g., ArrayList) when the size is known and fixed, as arrays have a smaller memory overhead.
Be cautious with multi-dimensional arrays, as they can consume more memory than expected due to padding.
Memory Profiling
Use memory profiling tools to identify memory leaks and memory-hungry parts of your application. Tools like VisualVM or YourKit can help pinpoint memory issues.
Garbage Collection Tuning
Tune the garbage collection settings using JVM flags (e.g., -Xmx, -Xms, -XX:MaxHeapFreeRatio, -XX:MinHeapFreeRatio, etc.) to optimize heap memory management.
0 notes
Text
Mastering Data Structures: A Comprehensive Course for Beginners
Data structures are one of the foundational concepts in computer science and software development. Mastering data structures is essential for anyone looking to pursue a career in programming, software engineering, or computer science. This article will explore the importance of a Data Structure Course, what it covers, and how it can help you excel in coding challenges and interviews.
1. What Is a Data Structure Course?
A Data Structure Course teaches students about the various ways data can be organized, stored, and manipulated efficiently. These structures are crucial for solving complex problems and optimizing the performance of applications. The course generally covers theoretical concepts along with practical applications using programming languages like C++, Java, or Python.
By the end of the course, students will gain proficiency in selecting the right data structure for different problem types, improving their problem-solving abilities.
2. Why Take a Data Structure Course?
Learning data structures is vital for both beginners and experienced developers. Here are some key reasons to enroll in a Data Structure Course:
a) Essential for Coding Interviews
Companies like Google, Amazon, and Facebook focus heavily on data structures in their coding interviews. A solid understanding of data structures is essential to pass these interviews successfully. Employers assess your problem-solving skills, and your knowledge of data structures can set you apart from other candidates.
b) Improves Problem-Solving Skills
With the right data structure knowledge, you can solve real-world problems more efficiently. A well-designed data structure leads to faster algorithms, which is critical when handling large datasets or working on performance-sensitive applications.
c) Boosts Programming Competency
A good grasp of data structures makes coding more intuitive. Whether you are developing an app, building a website, or working on software tools, understanding how to work with different data structures will help you write clean and efficient code.
3. Key Topics Covered in a Data Structure Course
A Data Structure Course typically spans a range of topics designed to teach students how to use and implement different structures. Below are some key topics you will encounter:
a) Arrays and Linked Lists
Arrays are one of the most basic data structures. A Data Structure Course will teach you how to use arrays for storing and accessing data in contiguous memory locations. Linked lists, on the other hand, involve nodes that hold data and pointers to the next node. Students will learn the differences, advantages, and disadvantages of both structures.
b) Stacks and Queues
Stacks and queues are fundamental data structures used to store and retrieve data in a specific order. A Data Structure Course will cover the LIFO (Last In, First Out) principle for stacks and FIFO (First In, First Out) for queues, explaining their use in various algorithms and applications like web browsers and task scheduling.
c) Trees and Graphs
Trees and graphs are hierarchical structures used in organizing data. A Data Structure Course teaches how trees, such as binary trees, binary search trees (BST), and AVL trees, are used in organizing hierarchical data. Graphs are important for representing relationships between entities, such as in social networks, and are used in algorithms like Dijkstra's and BFS/DFS.
d) Hashing
Hashing is a technique used to convert a given key into an index in an array. A Data Structure Course will cover hash tables, hash maps, and collision resolution techniques, which are crucial for fast data retrieval and manipulation.
e) Sorting and Searching Algorithms
Sorting and searching are essential operations for working with data. A Data Structure Course provides a detailed study of algorithms like quicksort, merge sort, and binary search. Understanding these algorithms and how they interact with data structures can help you optimize solutions to various problems.
4. Practical Benefits of Enrolling in a Data Structure Course
a) Hands-on Experience
A Data Structure Course typically includes plenty of coding exercises, allowing students to implement data structures and algorithms from scratch. This hands-on experience is invaluable when applying concepts to real-world problems.
b) Critical Thinking and Efficiency
Data structures are all about optimizing efficiency. By learning the most effective ways to store and manipulate data, students improve their critical thinking skills, which are essential in programming. Selecting the right data structure for a problem can drastically reduce time and space complexity.
c) Better Understanding of Memory Management
Understanding how data is stored and accessed in memory is crucial for writing efficient code. A Data Structure Course will help you gain insights into memory management, pointers, and references, which are important concepts, especially in languages like C and C++.
5. Best Programming Languages for Data Structure Courses
While many programming languages can be used to teach data structures, some are particularly well-suited due to their memory management capabilities and ease of implementation. Some popular programming languages used in Data Structure Courses include:
C++: Offers low-level memory management and is perfect for teaching data structures.
Java: Widely used for teaching object-oriented principles and offers a rich set of libraries for implementing data structures.
Python: Known for its simplicity and ease of use, Python is great for beginners, though it may not offer the same level of control over memory as C++.
6. How to Choose the Right Data Structure Course?
Selecting the right Data Structure Course depends on several factors such as your learning goals, background, and preferred learning style. Consider the following when choosing:
a) Course Content and Curriculum
Make sure the course covers the topics you are interested in and aligns with your learning objectives. A comprehensive Data Structure Course should provide a balance between theory and practical coding exercises.
b) Instructor Expertise
Look for courses taught by experienced instructors who have a solid background in computer science and software development.
c) Course Reviews and Ratings
Reviews and ratings from other students can provide valuable insights into the courseâs quality and how well it prepares you for real-world applications.
7. Conclusion: Unlock Your Coding Potential with a Data Structure Course
In conclusion, a Data Structure Course is an essential investment for anyone serious about pursuing a career in software development or computer science. It equips you with the tools and skills to optimize your code, solve problems more efficiently, and excel in technical interviews. Whether you're a beginner or looking to strengthen your existing knowledge, a well-structured course can help you unlock your full coding potential.
By mastering data structures, you are not only preparing for interviews but also becoming a better programmer who can tackle complex challenges with ease.
3 notes
¡
View notes
Text
Understanding Java Data Types: A Comprehensive Guide
Java, one of the most widely used programming languages, is known for its portability, security, and rich set of features. At the core of Java programming are data types, which define the nature of data that can be stored and manipulated within a program. Understanding data types is crucial for effective programming, as they determine how data is stored, how much memory it occupies, and the operations that can be performed on that data.
What are Data Types?
In programming, data types specify the type of data that a variable can hold. They provide a way to classify data into different categories based on their characteristics and operations. Java categorizes data types into two main groups:
1. Primitive Data Types
2. Reference Data Types
Why Use Data Types?
1. Memory Management: Different data types require different amounts of memory. By choosing the appropriate data type, you can optimize memory usage, which is particularly important in resource-constrained environments.
2. Type Safety: Using data types helps catch errors at compile time, reducing runtime errors. Java is a statically typed language, meaning that type checks are performed during compilation.
3. Code Clarity: Specifying data types makes the code more readable and understandable. It allows other developers (or your future self) to quickly grasp the intended use of variables.
4. Performance Optimization: Certain data types can enhance performance, especially when dealing with large datasets or intensive calculations. For example, using int instead of long can speed up operations when the range of int is sufficient.
5. Defining Operations: Different data types support different operations. For example, you cannot perform mathematical operations on a String data type without converting it to a numeric type.
When and Where to Use Data Types?
1. Choosing Primitive Data Types:
Use int when you need a whole number without a decimal, such as counting items.
Use double for fractional numbers where precision is essential, like financial calculations.
Use char when you need to store a single character, such as a letter or symbol.
Use boolean when you need to represent true/false conditions, like in conditional statements.
2. Choosing Reference Data Types:
Use String for any textual data, such as names, messages, or file paths.
Use Arrays when you need to store multiple values of the same type, such as a list of scores or names.
Use Custom Classes to represent complex data structures that include multiple properties and behaviors. For example, a Car class can encapsulate attributes like model, year, and methods for actions like starting or stopping the car.
1. Primitive Data Types
Primitive data types are the most basic data types built into the Java language. They serve as the building blocks for data manipulation in Java. There are eight primitive data types:
Examples of Primitive Data Types
1. Byte Example
byte age = 25; System.out.println(âAge: â + age);
2. Short Example
short temperature = -5; System.out.println(âTemperature: â + temperature);
3. Int Example
int population = 1000000; System.out.println(âPopulation: â + population);
4. Long Example
long distanceToMoon = 384400000L; // in meters System.out.println(âDistance to Moon: â + distanceToMoon);
5. Float Example
float pi = 3.14f; System.out.println(âValue of Pi: â + pi);
6. Double Example
double gravitationalConstant = 9.81; // m/s^2 System.out.println(âGravitational Constant: â + gravitationalConstant);
7. Char Example
char initial = âJâ; System.out.println(âInitial: â + initial);
8. Boolean Example
boolean isJavaFun = true; System.out.println(âIs Java Fun? â + isJavaFun);
2. Reference Data Types
Reference data types, unlike primitive data types, refer to objects and are created using classes. Reference data types are not defined by a fixed size; they can store complex data structures such as arrays, strings, and user-defined classes. The most common reference data types include:
Strings: A sequence of characters.
Arrays: A collection of similar data types.
Classes: User-defined data types.
Examples of Reference Data Types
1. String Example
String greeting = âHello, World!â; System.out.println(greeting);
2. Array Example
int[] numbers = {1, 2, 3, 4, 5}; System.out.println(âFirst Number: â + numbers[0]);
3. Class Example
class Car { Â Â Â String model; Â Â Â int year;
   Car(String m, int y) {        model = m;        year = y;    } }
public class Main { Â Â Â public static void main(String[] args) { Â Â Â Â Â Â Â Car car1 = new Car(âToyotaâ, 2020); Â Â Â Â Â Â Â System.out.println(âCar Model: â + car1.model + â, Year: â + car1.year); Â Â Â } }
Type Conversion
In Java, type conversion refers to converting a variable from one data type to another. This can happen in two ways:
1. Widening Conversion: Automatically converting a smaller data type to a larger data type (e.g., int to long). This is done implicitly by the Java compiler.
int num = 100; long longNum = num; // Widening conversion
2. Narrowing Conversion: Manually converting a larger data type to a smaller data type (e.g., double to int). This requires explicit casting.
double decimalNum = 9.99; int intNum = (int) decimalNum; // Narrowing conversion
Conclusion
Understanding data types in Java is fundamental for effective programming. It not only helps in managing memory but also enables programmers to manipulate data efficiently. Javaâs robust type system, consisting of both primitive and reference data types, provides flexibility and efficiency in application development. By carefully selecting data types, developers can optimize performance, ensure type safety, and maintain code clarity.
By mastering data types, youâll greatly enhance your ability to write efficient, reliable, and maintainable Java programs, setting a strong foundation for your journey as a Java developer.
3 notes
¡
View notes
Text
Accelerate Your Java Journey: Tips and Strategies For Rapid Learning
Java, renowned for its versatility and widespread use in the software development realm, offers an exciting and rewarding journey for programmers of all levels. Whether you're an aspiring coder stepping into the world of programming or a seasoned developer eager to add Java to your skill set, this programming language holds the promise of empowering you with valuable capabilities.
But learning Java isn't just about mastering the syntax and libraries; it's about embracing a mindset of problem-solving, creativity, and adaptability. As we navigate the rich landscape of Java's features, libraries, and best practices, keep in mind that your commitment to continuous learning and your passion for programming will be the driving forces behind your success.
So, fasten your seatbelt as we embark on a voyage into the world of Java programming. Along the way, we'll discover the resources, strategies, and insights that will empower you to unlock the full potential of this versatile language. The journey to Java mastery awaits, and we're here to guide you every step of the way.
1. Starting with the Building Blocks: Mastering the Basics
Every great journey begins with the first step, and in the world of Java, that means mastering the fundamentals. Start by immersing yourself in the basics, which include variables, data types, operators, and control structures like loops and conditionals. There's a wealth of online tutorials, textbooks, and courses that can provide you with a rock-solid foundation in these core concepts. Take your time to grasp these fundamentals thoroughly, as they will serve as the bedrock of your Java expertise.
2. The Power of Object-Oriented Programming (OOP)
Java is renowned for its adherence to the principles of Object-Oriented Programming (OOP). To unlock the true potential of Java, invest time in understanding OOP principles such as encapsulation, inheritance, polymorphism, and abstraction. These concepts are at the heart of Java's design and are essential for writing clean, maintainable, and efficient code. A deep understanding of OOP will set you on the path to becoming a Java maestro.
3. Practice Makes Perfect: The Art of Consistency
In the realm of programming, consistent practice is the key to improvement. Make it a habit to write Java code regularly. Start with small projects that align with your current skill level, and gradually work your way up to more complex endeavors. Experiment with different aspects of Java programming, from console applications to graphical user interfaces. Platforms like LeetCode, HackerRank, and Codecademy offer a plethora of coding challenges that can sharpen your skills and provide practical experience.
4. Harnessing Java's Vast Standard Library
Java boasts a vast standard library filled with pre-built classes and methods that cover a wide range of functionalities. Familiarize yourself with these libraries, as they can be invaluable time-savers when developing applications. Whether you're working on file manipulation, network communication, or user interface design, Java's standard library likely has a class or method that can simplify your task. A deep understanding of these resources will make you a more efficient and productive Java developer.
5. Memory Management: The Art of Efficiency
Java uses garbage collection, an automatic memory management technique. To write efficient Java code, it's crucial to understand how memory is allocated and deallocated in Java. This knowledge not only helps you avoid memory leaks but also allows you to optimize your code for better performance. Dive into memory management principles, learn about object references, and explore strategies for memory optimization.
6. Building Real-World Projects: Learning by Doing
While theory is essential, practical application is where true mastery is achieved. One of the most effective ways to learn Java is by building real-world projects. Start with modest undertakings that align with your skill level and gradually progress to more ambitious ventures. Java provides a versatile platform for application development, allowing you to create desktop applications using JavaFX, web applications with Spring Boot, or Android apps with Android Studio. The hands-on experience gained from project development is invaluable and will solidify your Java skills.
7. The Wisdom of Java Books
In the world of Java programming, books are a treasure trove of knowledge and best practices. Consider delving into Java literature, including titles like "Effective Java" by Joshua Bloch and "Java: The Complete Reference" by Herbert Schildt. These books offer in-depth insights into Java's intricacies and provide guidance on writing efficient and maintainable code.
8. Online Courses and Tutorials: Structured Learning
Online courses and tutorials from reputable platforms like Coursera, Udemy, edX, and, notably, ACTE Technologies, offer structured and guided learning experiences. These courses often feature video lectures, homework assignments, quizzes, and exams that reinforce your learning. ACTE Technologies, a renowned provider of IT training and certification programs, offers top-notch Java courses with expert instructors and a comprehensive curriculum designed to equip you with the skills needed for success.
9. Engaging with the Java Community
Java has a thriving online community of developers, programmers, and enthusiasts. Join Java forums and participate in online communities like Stack Overflow, Reddit's r/java, and Java-specific LinkedIn groups. Engaging with the community can help you get answers to your questions, gain insights from experienced developers, and stay updated on the latest trends and developments in the Java world.
10. Staying on the Cutting Edge
Java is a dynamic language that evolves over time. To stay ahead in the Java programming landscape, it's essential to keep abreast of the latest Java versions and features. Follow blogs, subscribe to newsletters, and connect with social media accounts dedicated to Java programming. Being up-to-date with the latest advancements ensures that you can leverage the full power of Java in your projects.
In conclusion, embarking on the journey to learn Java is an exciting and rewarding endeavor. It's a language with immense versatility and widespread use, making it a valuable skill in today's tech-driven world. To accelerate your learning and ensure you're equipped with the knowledge and expertise needed to excel in Java programming, consider enrolling in Java courses at ACTE Technologies.
ACTE Technologies stands as a reputable provider of IT training and certification programs, offering expert-led courses designed to build a strong foundation and advance your Java skills. With their guidance and structured learning approach, you can unlock the full potential of Java and embark on a successful programming career. Don't miss the opportunity to learn Java with ACTE Technologies and fast-track your path to mastery. The world of Java programming awaits your expertise and innovation.
8 notes
¡
View notes
Text
Fun with Flutter & Kotlin: A Beginner's Guide
Embark on the dynamic journey of cross-platform app development with the seamless integration of Flutter and Kotlin. This guide unveils key aspects for beginners, ensuring a smooth introduction to creating your first Flutter + Kotlin app.
Introduction
Discover the perfect synergy between Flutter, Google's UI toolkit, and Kotlin, a modern programming language, setting the stage for efficient cross-platform development.
Understanding Flutter
What is Flutter?: A Dart-powered framework simplifying cross-platform development.
Key Features: Real-time updates with Hot Reload and a rich widget library for intuitive UI development.
Setting Up Flutter: A user-friendly guide for installing Flutter SDK and configuring the development environment.
Dive into Kotlin
Introduction: Exploring Kotlin's origins, its role in mobile development, and interoperability with Java.
Setting Up Kotlin for Flutter: Seamless integration guidance for a harmonious development experience.
Building Your First Flutter + Kotlin App
Project Structure Overview: Breakdown of components and files within a Flutter + Kotlin project.
Creating UI with Flutter: Leveraging the widget system for visually appealing interfaces.
Adding Functionality with Kotlin: Integrating Kotlin code seamlessly for enhanced functionality.
Navigating Through Flutter and Kotlin
Navigation Basics: Demystifying navigation within a Flutter app.
Kotlin's Role in Navigation: Enhancing navigation functionalities with Kotlin code.
Debugging and Testing
Debugging Techniques in Flutter: Navigating common challenges with Flutter DevTools.
Testing Strategies with Kotlin: Effective unit testing guidance in Kotlin.
Optimization and Performance
Flutter Performance Tips: Managing widget rebuilds and optimizing state management.
Kotlin's Performance Contribution: Enhancing app performance through efficient coding and memory management.
Advanced Concepts
State Management in Flutter: Insights into advanced options for efficient state management.
Advanced Kotlin Features: Exploration of Kotlin's advanced features, including coroutines and concurrency.
Deployment and Publishing
Preparing Your App: Steps for building and securing an app for deployment.
Publishing on App Stores: Navigating submission processes for Google Play and App Store.
Troubleshooting and Common Issues
Flutter Troubleshooting: Strategies for addressing common issues and handling errors.
Kotlin-Specific Challenges: Identifying and overcoming challenges specific to Kotlin in Flutter projects.
Community and Resources
Joining Flutter Communities: Encouragement to connect through online forums and groups.
Kotlin Resources for Beginners: A curated list of tutorials and documentation for Kotlin learners.
This is the short description for flutter and kotlin. Check out the full descriptive blog for flutter vs Kotlin.
Conclusion
Summarizing key learnings, this guide encourages continued exploration of Flutter and Kotlin's potential in cross-platform app development. If you are a business owner and want your app ready but you are still not sure about which platform you should go for either flutter or kotlin. Here at Eitbiz you will find the best experts who will guide you to the best platform according to your business or service. Check out how we create and help businesses with our flutter app development and kotlin app development.
2 notes
¡
View notes
Text
Inside the World of Full Stack Development: Crafting Seamless Digital Experiences
In todayâs fast-paced digital age, the demand for adaptable, versatile developers has reached an all-time high. As businesses continue to evolve in a technology-driven landscape, the role of full stack developers has emerged as a pivotal force in shaping seamless digital experiences. From the front-end visuals to the back-end functionality, these professionals orchestrate entire applications with precision and efficiency.
But what does it really mean to live inside the world of full stack development?
Understanding the Full Stack Ecosystem
Full stack development refers to the ability to work on both the front end and back end of web and software applications. While front-end development focuses on user interface (UI) and user experience (UX), the back end includes server logic, databases, APIs, and integration systems.
To craft a seamless digital experience, a full stack developer must have a working command over multiple layers of technology. A few core components include:
Front-end technologies such as HTML, CSS, JavaScript, React, or Angular.
Back-end development using tools like Node.js, Python, Ruby, or Java.
Database management with MySQL, MongoDB, or PostgreSQL.
Version control systems like Git for code management.
Server and deployment practices using Docker, Kubernetes, or AWS.
But mastering tools isnât enough. What truly sets apart todayâs developers is how they learn and apply these skills in real-time environments.
Project-Based Full Stack Learning: The Key to Practical Expertise
Traditional learning models often focus too much on theory. But in the evolving tech ecosystem, practical exposure wins the race. This is where project-based full stack learning steps in.
Instead of merely learning syntax or reading documentation, learners build actual applications that reflect real-world use cases. This method:
Encourages hands-on coding from day one.
Teaches students how different components interact in a live environment.
Helps learners grasp error handling, debugging, and optimization organically.
Boosts confidence and provides a portfolio to showcase in interviews.
In short, it bridges the gap between conceptual understanding and workplace application.
Solving Real-World Challenges with Java
A core part of being a well-rounded full stack developer is problem-solving. And Java, being one of the most stable and widely-used programming languages, plays a crucial role in that journey.
Real-time problem-solving with Java introduces developers to scenarios where high-performance, secure, and scalable systems are required. Think of things like:
Building payment gateways
Developing REST APIs for e-commerce platforms
Creating server-side logic for mobile applications
Ensuring thread safety and memory management in multi-user systems
Using Java in full stack development isnât just about writing back-end logic; itâs about integrating robust performance and security within scalable architectures. And when these solutions are executed in real-time, they provide a rich learning ground for both novices and seasoned developers.
Why Full Stack Development Matters Today
Crafting seamless digital experiences isn't simply about attractive interfaces. It's about delivering responsive, secure, and optimized platforms that feel effortless to users.
Hereâs why full stack development has become a cornerstone in the tech world:
Efficiency: One person can handle both front and back-end, reducing development time and communication gaps.
Flexibility: Developers can switch roles depending on project needs.
Comprehension: Better understanding of how components interact improves debugging and integration.
Value: Companies save costs while ensuring faster delivery and consistency.
In fact, many startups and small businesses now prefer hiring full stack developers over segmented teams, as they can iterate rapidly and pivot when needed.
Building a Career Inside the Full Stack World
To thrive in this space, aspiring developers must combine technical skills with the right mindset. Hereâs what helps:
Focus on end-to-end project development. Not just coding snippets, but building from concept to deployment.
Practice debugging in live environments. Mistakes are your best teachers.
Engage in real-time problem-solving with Java and other back-end tools.
Join developer communities. Platforms like GitHub, Stack Overflow, and Dev.to offer immense collaborative learning.
Stay updated. The tech world evolves fast â full stack developers must keep up.
Top Skills Every Full Stack Developer Should Master
HTML5, CSS3, JavaScript (ES6+)
Front-end frameworks like React, Vue, or Angular
Back-end platforms like Node.js, Java Spring Boot, or Express.js
Databases: SQL & NoSQL
Version Control: Git & GitHub
Web Hosting & Deployment: Heroku, AWS, Netlify
Soft Skills: Communication, Time Management, and Critical Thinking
Final Thoughts
Inside the world of full stack development, the journey is as important as the destination. From learning through project-based full stack learning modules to encountering real-time problem-solving with Java, the process transforms a beginner into a professional equipped to handle dynamic digital challenges.
Crafting seamless digital experiences isnât just about code â itâs about vision, innovation, and adaptability. Full stack developers are not just builders of websites or apps; they are the architects of digital transformation.
Whether you're a curious beginner or a tech enthusiast looking to upskill, stepping into this world is a decision that will shape not just your career, but the way you understand and influence technology.
0 notes
Text
Unlock the Power of Code: 2025 Rust Programming for Beginners
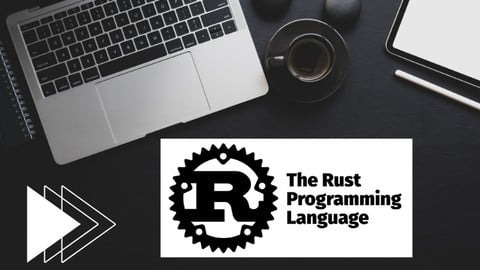
In 2025, the world of programming continues to evolve rapidlyâand if you're searching for a powerful, fast, and reliable language thatâs growing in popularity, Rust should be on your radar. Whether you're just starting out or transitioning from another language, Rust Programming for Beginners is the perfect place to begin your journey into systems-level programming, the safe way.
Rust isnât just another programming languageâitâs a revolution. Built with safety, concurrency, and performance at its core, Rust is used by developers at Google, Microsoft, Dropbox, Amazon, and many top-tier companies. If you want a future-proof skill that employers are actively hiring for, learning Rust in 2025 is a smart move.
Why Rust? What Makes It So Special?
Rust was designed to solve real-world programming problems that other languages struggle with. It combines the speed and control of C and C++ with memory safetyâwithout the need for a garbage collector. This makes it ideal for system-level development, embedded systems, and even web development using tools like WebAssembly.
Hereâs why Rust is getting the spotlight in 2025:
Memory Safety Without Garbage Collection: Rustâs ownership model ensures that you donât deal with memory leaks or dangling pointers.
Blazing Fast Performance: Rust code is compiled directly to machine code and optimized for speed.
Concurrency Made Safe: Rustâs approach to multithreading ensures thread safety without sacrificing performance.
Backed by Industry Leaders: Companies like Mozilla, Meta, and AWS use Rust in production.
Growing Community & Ecosystem: Rustâs tooling (Cargo, Clippy, Rustfmt) is modern and developer-friendly.
Who Should Learn Rust in 2025?
If you're wondering whether Rust is right for you, the answer is yesâespecially if:
You're a complete beginner with an interest in programming.
Youâre a software developer wanting to explore systems programming.
You come from Python, JavaScript, or Java and want a high-performance language.
Youâre interested in game development, embedded systems, or WebAssembly.
You want to level up your coding interview game with low-level problem-solving.
No matter your background, you can learn Rust from scratch with the right guidance. Thatâs where this top-rated beginner course comes in.
Introducing the Best Course: Rust Programming for Beginners
If you're serious about mastering Rust, donât waste hours jumping from blog to blog or piecing together fragmented tutorials. The Rust Programming for Beginners course offers structured, beginner-friendly training that walks you through everything from basics to hands-on projects.
đ Course Highlights:
Absolute Beginner-Friendly: No prior programming experience needed.
Step-by-Step Lessons: From variables to control flow, functions to ownership.
Real Projects: Build actual applications to solidify your knowledge.
Lifetime Access: Learn at your own pace, and revisit whenever needed.
Quizzes and Practice Exercises: Test your knowledge as you go.
Certificate of Completion: Showcase your skills on your resume or LinkedIn.
Whether youâre just dabbling or fully committing to Rust, this course makes sure you're not just learningâbut actually understanding.
What You'll Learn: From "Hello, World!" to Building Real Projects
This course truly covers everything you need to become productive with Rust. Hereâs a quick peek at the modules:
1. Introduction to Rust
History of Rust
Setting up your environment
Understanding Cargo (Rustâs package manager)
2. Basic Programming Concepts
Variables and data types
Functions and control flow
Loops and conditionals
3. Ownership, Borrowing, and Lifetimes
The heart of Rustâs memory safety
Avoiding bugs at compile time
How Rust manages memory differently than other languages
4. Structs and Enums
Data structures in Rust
When to use structs vs. enums
Pattern matching with match
5. Collections and Error Handling
Vectors, HashMaps, and Strings
Option and Result types
Handling errors the Rust way
6. Working with Modules and Crates
Organizing your code
Using external libraries from crates.io
7. Project-Based Learning
Build your own command-line tools
Create a mini web scraper
Start a simple API with Rocket or Actix-web
What Makes This Course Different?
With hundreds of Rust tutorials online, why should you choose this course? Hereâs the difference:
â
Hands-On Approach â You wonât just watch videos; youâll build real things. â
Beginner-Focused â Complex topics are explained simply, with clear visuals. â
Updated for 2025 â Content is fresh, aligned with the latest Rust updates. â
Trusted Platform â Hosted on Udemy, promoted by Korshub, and trusted by thousands.
Plus, the instructor is known for teaching with clarity, warmth, and energyâmaking learning Rust genuinely fun.
Real-World Applications of Rust
Wondering what you can actually do with Rust after completing this course?
Here are just a few career paths and domains where Rust is making waves:
đ ď¸ System-Level Development
Rust is excellent for writing OS-level code, drivers, and performance-critical tools.
đ Web Development with WebAssembly
Rust compiles to WebAssembly (WASM), letting you build blazing-fast web apps.
đŽ Game Development
Rustâs performance and safety features make it a great fit for modern game engines.
đ Cybersecurity Tools
Rustâs memory safety makes it ideal for building secure applications like firewalls or scanning tools.
đ Embedded Systems and IoT
Rust runs on small devices, allowing you to build firmware and IoT projects with confidence.
Testimonials from Learners Like You
âI was completely new to programming, and this course helped me grasp core concepts fast. Rust seemed intimidating until now!â â Ankit, Student
âAfter taking this course, I landed an internship where they use Rust in production. Couldnât have done it without this course!â â Priya, Junior Developer
âThe project sections made everything click. I finally feel confident with a low-level language like Rust.â â Kevin, Freelance Dev
Whatâs Next After You Complete the Course?
Learning Rust is a journeyâand this course is your foundation. After completing Rust Programming for Beginners, you can:
Dive into intermediate topics like async programming and concurrency.
Contribute to open-source Rust projects on GitHub.
Build performance-critical applications from scratch.
Start freelancing or apply for Rust developer jobs.
And guess what? Rust developers are in high demand in 2025âwith salaries averaging $100,000+ globally. So, this isnât just a learning investmentâitâs a career move.
Final Thoughts: Is Rust the Right Choice for You?
If you're looking for a language that combines power, performance, and safety, then Rust is the future. Whether you're planning to become a professional developer or youâre just a curious learner, starting with Rust in 2025 puts you ahead of the curve.
The best part? You donât have to figure it all out alone.
The Rust Programming for Beginners course provides everything you need to build your skills step-by-step, gain confidence, and start building exciting projects from day one.
So, donât wait. Learn Rust now and be future-ready.
0 notes
Text
AOSP Architecture Explained: A Practical Guide for Android DevelopersÂ
In this blog, we delve into the architecture of the Android Open Source Project (AOSP), breaking down each layer of the software stack. From the Linux Kernel to Applications, we explore the functionalities and responsibilities of each component. Whether you're customizing ROMs or developing hardware-specific solutions, understanding the AOSP architecture is crucial.Â
The Android Open Source Project (AOSP) serves as the foundation for the Android operating system, offering a comprehensive software stack that enables developers to create a consistent user experience across various devices. Understanding the AOSP architecture is essential for developers aiming to build custom Android builds or integrate deeply with system components.Â
Overview of the AOSP Software StackÂ
The AOSP software stack is organized into several layers, each responsible for specific functionalities:Â
Linux Kernel: At the base, the Linux Kernel manages core system services such as process management, memory management, and hardware drivers. It acts as an abstraction layer between the hardware and the rest of the software stack.Â
Hardware Abstraction Layer (HAL): HAL provides standard interfaces that expose device hardware capabilities to the higher-level Java API framework. This allows Android to be agnostic about lower-level driver implementations.Â
System Services and Daemons: These are background processes that provide core system functionalities like power management, telephony, and media playback. They facilitate communication between the HAL and the Android Runtime.Â
Android Runtime (ART): ART is the managed runtime used by applications and some system services. It includes a set of core libraries and handles tasks like memory management, garbage collection, and bytecode execution.Â
System APIs: These APIs provide the necessary interfaces for applications to interact with the underlying hardware and system services, enabling functionalities like location services, telephony, and sensor management.Â
Android Framework: The framework offers a rich set of APIs that developers use to build applications. It includes components like Activity Manager, Window Manager, and Content Providers, which manage the user interface and application resources.Â
Applications: At the top layer, applications include both native apps provided by the device manufacturer and third-party apps installed by users. These apps interact with the Android Framework to perform their functions.Â
Importance for DevelopersÂ
Understanding the AOSP architecture is vital for several reasons:Â
Customization: For developers building custom ROMs or tailoring Android for specific hardware, knowledge of each layer allows for effective customization and optimization.Â
Performance Optimization: Identifying and addressing performance bottlenecks requires a deep understanding of how different layers interact and where potential issues may arise.Â
Scalability: Proper utilization of the Android Framework and System APIs ensures that applications are scalable and maintain compatibility across various devices and Android versions.Â
Security: Awareness of the interactions between privileged and system-level components is crucial for developing secure applications and protecting user data.Â
ConclusionÂ
The AOSP software stack is a meticulously designed architecture that harmonizes hardware and software components to deliver a seamless user experience. For developers, mastering this architecture is key to unlocking the full potential of Android, whether it's for application development, system customization, or hardware integration.Â
If you're looking to leverage AOSP for your projects, consider partnering with industry leaders like Silicon Signals. Recognized among the top 10 BSP and AOSP service companies, Silicon Signals offers expert services in Android BSP development, custom Android solutions, and more. Their team excels in delivering tailored solutions that meet the evolving demands of the industry. Silicon SignalsÂ
Ready to bring your Android project to life? Reach out to Silicon Signals at [email protected] for a free consultation.Â
#embeddedtechnology#embeddedsoftware#embeddedsystems#linux kernel#androidbsp#linuxdebugging#android#aosp#androidopensource
0 notes
Text
What to Expect During Your MCA Journey: From Code to Career
The Master of Computer Applications (MCA) is more than just a postgraduate degreeâit bridges foundational computing knowledge and a dynamic career in the tech industry. For students coming from BCA, BSc Computer Science, or even non-IT backgrounds, MCA offers a structured path to mastering modern technologies and stepping confidently into software development, data science, cybersecurity, and beyond.
As tech industries evolve rapidly, knowing what to expect during your MCA program can help you prepare and make the most of the opportunity. Whether youâre eyeing a development job, a role in IT consulting, or a future in tech entrepreneurship, hereâs a roadmap of what your MCA journey will involve.
A Deep Dive into Programming and Problem Solving
In the first year, youâll revisit programming basicsâbut at a more advanced level. Languages like C, C++, Java, and Python become foundational tools. Youâll work on algorithms, data structures, and problem-solving techniques that form the backbone of any successful software career.
Expect a strong focus on writing efficient, scalable code and understanding how logic drives every tech solutionâfrom simple applications to enterprise systems.
Exposure to Core Computer Science Concepts
Beyond programming, your coursework will cover the fundamentals of computer science, including:
Operating systems
Database management systems
Computer networks
Software engineering
Web technologies
Object-oriented programming
This knowledge helps students develop systems thinking and learn how to build, manage, and optimize large-scale software applications.
Electives in Emerging Tech Areas
Most modern MCA programs offer electives that align with current industry trends. Depending on the institution, you might study:
Artificial Intelligence and Machine Learning
Data Science and Big Data Analytics
Cloud Computing
Cybersecurity and Ethical Hacking
Mobile Application Development
Internet of Things (IoT)
These specializations allow you to align your interests with future career paths and gain a competitive edge in the job market.
Hands-On Learning through Projects and Labs
MCA is a practice-driven course. Youâll spend considerable time in labs working on programming assignments, web development tasks, and database applications. Mini-projects and hackathons help reinforce classroom learning and promote problem-solving under real-world constraints.
Your final year will likely include a capstone project or internship, giving you direct exposure to working in a professional tech environmentâan experience that often leads to job offers.
Building Soft Skills and Teamwork
Technical skills alone arenât enough. Youâll also develop communication, presentation, and teamwork skills, which are vital in any tech organization. Group projects, seminars, and role-based assignments teach you how to work collaboratively, manage time, and handle project dynamicsâall of which prepare you for real industry scenarios.
Industry Readiness and Career Support
Top MCA colleges in Mangalore integrate placement training into their curriculum. This includes resume-building workshops, coding test preparation, mock interviews, and personality development sessions.
Many colleges also invite IT companies for campus recruitment drives, helping students land roles in software development, system administration, IT consulting, cloud services, and data analytics.
Why Mangalore is a Smart Choice for MCA Aspirants
Mangalore is emerging as an educational hub with a growing presence of tech startups and IT services. It offers a mix of strong academic institutions and a peaceful learning environment, making it an ideal destination for postgraduate students.
Students researching MCA colleges in Mangalore will find programs that combine technical depth, practical exposure, and excellent placement support.
Nitte Mahalinga Adyantaya Memorial Institute of Technology â Shaping Future-Ready Tech Professionals
Among the leading MCA colleges in Mangalore, Nitte Mahalinga Adyantaya Memorial Institute of Technology (NMAMIT) offers a comprehensive MCA program designed to bridge academic learning with real-world application. The curriculum emphasizes core computing skills, emerging technologies, and industry-relevant training. With experienced faculty, well-equipped labs, and strong industry linkages, NMAMIT prepares students for roles in software development, data analytics, AI, cybersecurity, and more.
Through project-based learning, internships, and placement support, NMAMIT ensures that students graduate with the confidence and capabilities needed to thrive in todayâs competitive tech landscape.
1 note
¡
View note
Text
The Ultimate Roadmap to Web Development â Coding Brushup
In today's digital world, web development is more than just writing codeâit's about creating fast, user-friendly, and secure applications that solve real-world problems. Whether you're a beginner trying to understand where to start or an experienced developer brushing up on your skills, this ultimate roadmap will guide you through everything you need to know. This blog also offers a coding brushup for Java programming, shares Java coding best practices, and outlines what it takes to become a proficient Java full stack developer.
Why Web Development Is More Relevant Than Ever
The demand for web developers continues to soar as businesses shift their presence online. According to recent industry data, the global software development market is expected to reach $1.4 trillion by 2027. A well-defined roadmap is crucial to navigate this fast-growing field effectively, especially if you're aiming for a career as a Java full stack developer.
Phase 1: The Basics â Understanding Web Development
Web development is broadly divided into three categories:
Frontend Development: What users interact with directly.
Backend Development: The server-side logic that powers applications.
Full Stack Development: A combination of both frontend and backend skills.
To start your journey, get a solid grasp of:
HTML â Structure of the web
CSS â Styling and responsiveness
JavaScript â Interactivity and functionality
These are essential even if you're focusing on Java full stack development, as modern developers are expected to understand how frontend and backend integrate.
Phase 2: Dive Deeper â Backend Development with Java
Java remains one of the most robust and secure languages for backend development. Itâs widely used in enterprise-level applications, making it an essential skill for aspiring Java full stack developers.
Why Choose Java?
Platform independence via the JVM (Java Virtual Machine)
Strong memory management
Rich APIs and open-source libraries
Large and active community
Scalable and secure
If you're doing a coding brushup for Java programming, focus on mastering the core concepts:
OOP (Object-Oriented Programming)
Exception Handling
Multithreading
Collections Framework
File I/O
JDBC (Java Database Connectivity)
Java Coding Best Practices for Web Development
To write efficient and maintainable code, follow these Java coding best practices:
Use meaningful variable names: Improves readability and maintainability.
Follow design patterns: Apply Singleton, Factory, and MVC to structure your application.
Avoid hardcoding: Always use constants or configuration files.
Use Java Streams and Lambda expressions: They improve performance and readability.
Write unit tests: Use JUnit and Mockito for test-driven development.
Handle exceptions properly: Always use specific catch blocks and avoid empty catch statements.
Optimize database access: Use ORM tools like Hibernate to manage database operations.
Keep methods short and focused: One method should serve one purpose.
Use dependency injection: Leverage frameworks like Spring to decouple components.
Document your code: JavaDoc is essential for long-term project scalability.
A coding brushup for Java programming should reinforce these principles to ensure code quality and performance.
Phase 3: Frameworks and Tools for Java Full Stack Developers
As a full stack developer, you'll need to work with various tools and frameworks. Hereâs what your tech stack might include:
Frontend:
HTML5, CSS3, JavaScript
React.js or Angular: Popular JavaScript frameworks
Bootstrap or Tailwind CSS: For responsive design
Backend:
Java with Spring Boot: Most preferred for building REST APIs
Hibernate: ORM tool to manage database operations
Maven/Gradle: For project management and builds
Database:
MySQL, PostgreSQL, or MongoDB
Version Control:
Git & GitHub
DevOps (Optional for advanced full stack developers):
Docker
Jenkins
Kubernetes
AWS or Azure
Learning to integrate these tools efficiently is key to becoming a competent Java full stack developer.
Phase 4: Projects & Portfolio â Putting Knowledge Into Practice
Practical experience is critical. Try building projects that demonstrate both frontend and backend integration.
Project Ideas:
Online Bookstore
Job Portal
E-commerce Website
Blog Platform with User Authentication
Incorporate Java coding best practices into every project. Use GitHub to showcase your code and document the learning process. This builds credibility and demonstrates your expertise.
Phase 5: Stay Updated & Continue Your Coding Brushup
Technology evolves rapidly. A coding brushup for Java programming should be a recurring part of your development cycle. Hereâs how to stay sharp:
Follow Java-related GitHub repositories and blogs.
Contribute to open-source Java projects.
Take part in coding challenges on platforms like HackerRank or LeetCode.
Subscribe to newsletters like JavaWorld, InfoQ, or Baeldung.
By doing so, youâll stay in sync with the latest in the Java full stack developer world.
Conclusion
Web development is a constantly evolving field that offers tremendous career opportunities. Whether you're looking to enter the tech industry or grow as a seasoned developer, following a structured roadmap can make your journey smoother and more impactful. Java remains a cornerstone in backend development, and by following Java coding best practices, engaging in regular coding brushup for Java programming, and mastering both frontend and backend skills, you can carve your path as a successful Java full stack developer.
Start today. Keep coding. Stay curious.
0 notes
Text
Understanding Java: A Comprehensive Guide for Beginners and Pros
In the ever-expanding landscape of programming languages, Java stands as a stalwart, renowned for its versatility, reliability, and scalability. Whether you're an aspiring coder taking your first steps into the world of programming or a seasoned developer looking to broaden your skill set, Java presents a vast and captivating journey of discovery. This comprehensive guide aims to provide you with a roadmap for mastering Java effectively, offering insights, strategies, and resources to empower your learning process.
Java's prominence in the tech industry cannot be overstated. Its cross-platform compatibility, robustness, and extensive libraries make it a top choice for a wide range of applications, from web development to mobile app creation and enterprise-grade software solutions. With Java as your programming language of choice, you're embarking on a learning journey that can open doors to diverse and rewarding opportunities in the software development field.
Whether you're just beginning your Java journey or seeking to enhance your existing skills, this guide is your compass, pointing you toward the resources and strategies that will enable you to thrive in the world of Java development. So, let's embark on this adventure together, as we unlock the power of Java and take your coding skills to new heights.
The Fundamentals: Starting with the Basics
At the heart of mastering Java lies a strong grasp of its fundamentals. To begin your Java journey, you must dive into the basics. Start by understanding variables, data types, operators, and control structures, including loops and conditionals. These are the building blocks of Java programming and serve as your foundation. Fortunately, there's a wealth of online tutorials, textbooks, and courses available to help you comprehend these essential concepts.
The Power of Object-Oriented Programming (OOP)
Java is often celebrated for its object-oriented programming (OOP) paradigm. Delve into the world of OOP, where concepts like encapsulation, inheritance, polymorphism, and abstraction are fundamental. These principles are crucial for writing clean, maintainable, and scalable Java code. A strong grasp of OOP sets you on the path to becoming a proficient Java developer.
Practice Makes Perfect: The Art of Coding
Programming is a skill that thrives with practice. To truly master Java, you must write code regularly. Start with small projects and gradually work your way up to more complex challenges. Experiment with different aspects of Java, refine your coding style, and embrace the iterative process of development. Platforms such as LeetCode, HackerRank, and Codecademy offer a plethora of coding challenges that can sharpen your skills and boost your confidence.
Harnessing the Java Ecosystem: APIs and Libraries
Java boasts a vast standard library, replete with pre-built classes and methods. Familiarize yourself with these libraries as they can significantly streamline your development process. By leveraging these resources, you can save time and effort when building applications. Whether you're developing desktop applications with JavaFX, web solutions with Spring Boot, or mobile apps using Android Studio, understanding the Java ecosystem is indispensable.
Memory Management: The Key to Efficiency
Java employs automatic memory management through garbage collection. To optimize performance and prevent memory leaks, it's essential to grasp how memory is allocated and deallocated in Java. Understanding the intricacies of memory management is a hallmark of a proficient Java developer.
Building Real-World Projects
Theory alone won't make you a Java expert. Building practical applications is one of the most effective ways to learn Java. Start with modest projects and gradually increase their complexity. This hands-on approach not only reinforces your understanding but also equips you with real-world experience. Develop desktop applications with JavaFX for intuitive user interfaces, craft robust web applications using Spring Boot, or dive into the world of mobile app development with Android Studio. The projects you undertake will serve as a testament to your Java prowess.
The Power of Java Books: In-Depth Knowledge
Consider supplementing your practical experience with authoritative Java books. "Effective Java" by Joshua Bloch and "Java: The Complete Reference" by Herbert Schildt are highly recommended. These books provide in-depth knowledge, best practices, and insights from seasoned Java experts. They are invaluable resources for expanding your understanding of Java.
Enriching Your Learning Journey: Online Courses and Tutorials
Online courses from reputable platforms like Coursera, Udemy, edX, and ACTE Technologies can accelerate your Java learning journey. These courses offer a structured approach, with video lectures, assignments, and quizzes to reinforce your knowledge. ACTE Technologies, in particular, stands out for its expert instructors and comprehensive curriculum, providing you with valuable insights into the world of Java development.
Engaging with the Community: Online Forums and Communities
Joining Java forums and communities is an excellent way to complement your learning. Platforms like Stack Overflow, Reddit's r/java, and Java-specific LinkedIn groups provide a space to ask questions, seek guidance, and share your knowledge. Engaging with the Java community not only helps you find answers to your queries but also keeps you updated on the latest industry trends and best practices.
Staying Current: Java's Evolution
Java is a dynamic language that evolves over time. To remain at the forefront of Java development, it's essential to stay updated with the latest Java versions and features. Follow industry-related blogs, newsletters, and social media accounts. Keeping abreast of these changes ensures that your Java skills remain relevant and in demand.
In conclusion, embarking on the journey to master Java is an exciting and rewarding endeavor that demands dedication and continuous learning. The world of Java programming offers a multitude of opportunities, from web development to mobile app creation and enterprise solutions. By following the comprehensive roadmap outlined in this blog, you can pave the way to becoming a proficient Java developer.
Remember, learning from reputable sources such as ACTE Technologies can provide you with the knowledge and expertise needed to excel in Java programming. ACTE Technologies' exemplary Java courses, led by expert instructors and featuring a comprehensive curriculum, can help you establish a strong foundation and advance your Java skills. As you embark on your Java learning journey, may your passion for coding and commitment to excellence lead you to success. Good luck!
8 notes
¡
View notes
Text
Red Hat Summit 2025: Microsoft Drives into Cloud Innovation

Microsoft at Red Hat Summit 2025
Microsoft is thrilled to announce that it will be a platinum sponsor of Red Hat Summit 2025, an IT community favourite. IT professionals can learn, collaborate, and build new technologies from the datacenter, public cloud, edge, and beyond at Red Hat Summit 2025, a major enterprise open source event. Microsoft's partnership with Red Hat is likely to be a highlight this year, displaying collaboration's power and inventive solutions.
This partnership has changed how organisations operate and serve customers throughout time. Red Hat's open-source leadership and Microsoft's cloud knowledge synergise to advance technology and help companies.
Red Hat's seamless integration with Microsoft Azure is a major benefit of the alliance. These connections let customers build, launch, and manage apps on a stable and flexible platform. Azure and Red Hat offer several tools for system modernisation and cloud-native app development. Red Hat OpenShift on Azure's scalability and security lets companies deploy containerised apps. Azure Red Hat Enterprise Linux is trustworthy for mission-critical apps.
Attend Red Hat Summit 2025 to learn about these technologies. Red Hat and Azure will benefit from Microsoft and Red Hat's new capabilities and integrations. These improvements in security and performance aim to meet organisations' digital needs.
WSL RHEL
This lets Red Hat Enterprise Linux use Microsoft Subsystem for Linux. WSL lets creators run Linux on Windows. RHEL for WSL lets developers run RHEL on Windows without a VM. With a free Red Hat Developer membership, developers may install the latest RHEL WSL image on their Windows PC and run Windows and RHEL concurrently.
Red Hat OpenShift Azure
Red Hat and Microsoft are enhancing security with Confidential Containers on Azure Red Hat OpenShift, available in public preview. Memory encryption and secure execution environments provide hardware-level workload security for healthcare and financial compliance. Enterprises may move from static service principals to dynamic, token-based credentials with Azure Red Hat OpenShift's managed identity in public preview.
Reduced operational complexity and security concerns enable container platform implementation in regulated environments. Azure Red Hat OpenShift has reached Spain's Central region and plans to expand to Microsoft Azure Government (MAG) and UAE Central by Q2 2025. Ddsv5 instance performance optimisation, enterprise-grade cluster-wide proxy, and OpenShift 4.16 compatibility are added. Red Hat OpenShift Virtualisation on Azure is also entering public preview, allowing customers to unify container and virtual machine administration on a single platform and speed up VM migration to Azure without restructuring.
RHEL landing area
Deploying, scaling, and administering RHEL instances on Azure uses Azure-specific system images. A landing zone lesson. Red Hat Satellite and Satellite Capsule automate software lifecycle and provide timely updates. Azure's on-demand capacity reservations ensure reliable availability in Azure regions, improving BCDR. Optimised identity management infrastructure deployments decrease replication failures and reduce latencies.
Azure Migrate application awareness and wave planning
By delivering technical and commercial insights for the whole application and categorising dependent resources into waves, the new application-aware methodology lets you pick Azure targets and tooling. A collection of dependent applications should be transferred to Azure for optimum cost and performance.
JBossEAP on AppService
Red Hat and Microsoft developed and maintain JBoss EAP on App Service, a managed tool for running business Java applications efficiently. Microsoft Azure recently made substantial changes to make JBoss EAP on App Service more inexpensive. JBoss EAP 8 offers a free tier, memory-optimized SKUs, and 60%+ license price reductions for Make monthly payments subscriptions and the soon-to-be-released Bring-Your-Own-Subscription to App Service.
JBoss EAP on Azure VMs
JBoss EAP on Azure Virtual Machines is currently GA with dependable solutions. Microsoft and Red Hat develop and maintain solutions. Automation templates for most basic resource provisioning tasks are available through the Azure Portal. The solutions include Azure Marketplace JBoss EAP VM images.
Red Hat Summit 2025 expectations
Red Hat Summit 2025 should be enjoyable with seminars, workshops, and presentations. Microsoft will offer professional opinions on many subjects. Unique announcements and product debuts may shape technology.
This is a rare chance to network with executives and discuss future projects. Mission: digital business success through innovation. Azure delivers the greatest technology and service to its customers.
Read about Red Hat on Azure
Explore Red Hat and Microsoft's cutting-edge solutions. Register today to attend the conference and chat to their specialists about how their cooperation may aid your organisation.
#RedHatSummit2025#RedHatSummit#AzureRedHatOpenShift#RedHat#RedHatEnterprise#RedHatEnterpriseLinux#technology#technologynews#TechNews#news#govindhtech
1 note
¡
View note
Text
Ultimate Guide to Python Compiler
When diving into the world of Python programming, understanding how Python code is executed is crucial for anyone looking to develop, test, or optimize their applications. This process involves using a Python compiler, a vital tool for transforming human-readable Python code into machine-readable instructions. But what exactly is a Python compiler, how does it work, and why is it so important? This guide will break it all down for you in detail, covering everything from the basic principles to advanced usage.
What is a Python Compiler?
A Python compiler is a  software tool that translates Python code (written in a human-readable form) into machine code or bytecode that the  computer can execute. Unlike languages like C or Java, Python is primarily an interpreted language, which means the code is executed line by line by an interpreter. However, Python compilers and interpreters often work together to convert the source code into something that can run on your system.
Difference Between Compiler and Interpreter
Before we delve deeper into Python compilers, itâs important to understand the distinction between a compiler and an interpreter. A compiler translates the entire source code into a machine-readable format at once, before execution begins. Once compiled, the program can be executed multiple times without needing to recompile.
On the other hand, an interpreter processes the source code line by line, converting each line into machine code and executing it immediately. Python, as a high-level language, uses both techniques: it compiles the Python code into an intermediate form (called bytecode) and then interprets that bytecode.
How Does the Python Compiler Work?
The Python compiler is an essential part of the Python runtime environment. When you write Python code, it first undergoes a compilation process before execution. Hereâs a step-by-step look at how it works:
1. Source Code Parsing
The process starts when the Python source code (.py file) is written. The Python interpreter reads this code, parsing it into a data structure called an Abstract Syntax Tree (AST). The AST is a hierarchical tree-like representation of the Python code, breaking it down into different components like variables, functions, loops, and classes.
2. Compilation to Bytecode
After parsing the source code, the Python interpreter uses a compiler to convert the AST into bytecode. This bytecode is a lower-level representation of the source code that is platform-independent, meaning it can run on any machine that has a Python interpreter. Bytecode is not human-readable and acts as an intermediate step between the high-level source code and the machine code that runs on the hardware.
The bytecode generated is saved in .pyc (Python Compiled) files, which are stored in a special directory named __pycache__. When you run a Python program, if a compiled .pyc file is already available, Python uses it to speed up the startup process. If not, it re-compiles the source code.
3. Execution by the Python Virtual Machine (PVM)
After the bytecode is generated, it is sent to the Python Virtual Machine (PVM), which executes the bytecode instructions. The PVM is an interpreter that reads and runs the bytecode, line by line. It communicates with the operating system to perform tasks such as memory allocation, input/output operations, and managing hardware resources.
This combination of bytecode compilation and interpretation is what allows Python to run efficiently on various platforms, without needing separate versions of the program for different operating systems.
Why is a Python Compiler Important?
Using a Python compiler offers several benefits to developers and users alike. Here are a few reasons why a Python compiler is so important in the programming ecosystem:
1. Portability
Since Python compiles to bytecode, itâs not tied to a specific operating system or hardware. This allows Python programs to run on different platforms without modification, making it an ideal language for cross-platform development. Once the code is compiled into bytecode, the same .pyc file can be executed on any machine that has a compatible Python interpreter installed.
2. Faster Execution
Although Python is an interpreted language, compiling Python code to bytecode allows for faster execution compared to direct interpretation. Bytecode is more efficient for the interpreter to execute, reducing the overhead of processing the raw source code each time the program runs. It also helps improve performance for larger and more complex applications.
3. Error Detection
By using a Python compiler, errors in the code can be detected earlier in the development process. The compilation step checks for syntax and other issues, alerting the developer before the program is even executed. This reduces the chances of runtime errors, making the development process smoother and more reliable.
4. Optimizations
Some compilers provide optimizations during the compilation process, which can improve the overall performance of the Python program. Although Python is a high-level language, there are still opportunities to make certain parts of the program run faster. These optimizations can include techniques like constant folding, loop unrolling, and more.
Types of Python Compilers
While the official Python implementation, CPython, uses a standard Python compiler to generate bytecode, there are alternative compilers and implementations available. Here are a few examples:
1. CPython
CPython is the most commonly used Python implementation. It is the default compiler for Python, written in C, and is the reference implementation for the language. When you install Python from the official website, youâre installing CPython. This compiler converts Python code into bytecode and then uses the PVM to execute it.
2. PyPy
PyPy is an alternative implementation of Python that features a Just-In-Time (JIT) compiler. JIT compilers generate machine code at runtime, which can significantly speed up execution. PyPy is especially useful for long-running Python applications that require high performance. It is highly compatible with CPython, meaning most Python code runs without modification on PyPy.
3. Cython
Cython is a superset of Python that allows you to write Python code that is compiled into C code. Cython enables Python programs to achieve performance close to that of C while retaining Pythonâs simplicity. It is commonly used when optimizing computationally intensive parts of a Python program.
4. Jython
Jython is a Python compiler written in Java that allows Python code to be compiled into Java bytecode. This enables Python programs to run on the Java Virtual Machine (JVM), making it easier to integrate with Java libraries and tools.
5. IronPython
IronPython is an implementation of Python for the .NET framework. It compiles Python code into .NET Intermediate Language (IL), enabling it to run on the .NET runtime. IronPython allows Python developers to access .NET libraries and integrate with other .NET languages.
Python Compiler vs. Interpreter: Whatâs the Difference?
While both compilers and interpreters serve the same fundamental purposeâturning source code into machine-executable codeâthere are distinct differences between them. Here are the key differences:
Compilation Process
Compiler: Translates the entire source code into machine code before execution. Once compiled, the program can be executed multiple times without recompilation.
Interpreter: Translates and executes source code line by line. No separate executable file is created; execution happens on the fly.
Execution Speed
Compiler: Generally faster during execution, as the code is already compiled into machine code.
Interpreter: Slower, as each line is parsed and executed individually.
Error Detection
Compiler: Detects syntax and other errors before the program starts executing. All errors must be fixed before running the program.
Interpreter: Detects errors as the code is executed. Errors can happen at any point during execution.
Conclusion
The Python compiler plays a crucial role in the Python programming language by converting source code into machine-readable bytecode. Whether youâre using the default CPython implementation, exploring the performance improvements with PyPy, or enhancing Python with Cython, understanding how compilers work is essential for optimizing and running Python code effectively.
The compilation process, which includes parsing, bytecode generation, and execution, offers various benefits like portability, faster execution, error detection, and optimizations. By choosing the right compiler and understanding how they operate, you can significantly improve both the performance and efficiency of your Python applications.
Now that you have a comprehensive understanding of how Python compilers work, youâre equipped with the knowledge to leverage them in your development workflow, whether youâre a beginner or a seasoned developer.
0 notes
Text
Integrating Native Modules in Hybrid Apps: A Step-by-Step GuideÂ

In today's rapidly evolving tech landscape, hybrid app development has gained immense popularity due to its cost-effectiveness and cross-platform capabilities. Businesses increasingly prefer hybrid frameworks like React Native, Flutter, and Ionic to streamline development without sacrificing user experience. However, while hybrid apps offer flexibility, they sometimes fall short in accessing device-specific features efficiently. Thatâs where integrating native modules becomes essential.Â
This blog walks you through the step-by-step process of integrating native modules in hybrid apps to enhance performance and functionality without losing the benefits of cross-platform development.Â
Why Integrate Native Modules?Â
Hybrid apps are built using web technologies, but when it comes to device-level capabilitiesâlike accessing the camera, Bluetooth, sensors, or high-performance animationsâweb code might not be enough. Native modules bridge this gap by allowing developers to write platform-specific code (Java/Kotlin for Android, Swift/Objective-C for iOS) and call it from the hybrid layer.Â
This approach improves app performance, unlocks advanced device features, and delivers a more native-like experience to users.Â
Step-by-Step Guide to Integrating Native ModulesÂ
1. Identify the RequirementÂ
Before jumping into coding, evaluate what native functionality is needed. For example:Â
Accessing device sensors (accelerometer, gyroscope)Â
Background tasksÂ
Complex animations or gesturesÂ
Bluetooth integrationsÂ
Once the need is identified, determine if existing plugins or libraries support it. If not, proceed to write your own native module.Â
2. Set Up the Native EnvironmentÂ
Depending on your hybrid framework, youâll need to set up native development environments:Â
React Native: Android Studio + XcodeÂ
Flutter: Android Studio + XcodeÂ
Ionic/Cordova: Node.js, Android SDK, Xcode, and relevant CLI toolsÂ
Make sure your environment is correctly configured for both Android and iOS builds.Â
3. Write Native CodeÂ
Now comes the core part: writing native code.Â
Android: Create a new Java or Kotlin class that extends the appropriate module class.Â
iOS: Create a Swift or Objective-C class implementing the required bridge protocols.Â
Ensure this native module exposes methods or events you want to use in your hybrid code.Â
4. Bridge Native Module to Hybrid CodeÂ
Use your hybrid frameworkâs bridging mechanism:Â
React Native: Use the NativeModules API to expose native functions to JavaScript.Â
Flutter: Use MethodChannels to communicate between Dart and native code.Â
Ionic: Use Capacitor or Cordova plugins to integrate native functionality.Â
This is the layer where your JavaScript/Dart/TypeScript code talks directly to your native module.Â
5. Testing Across PlatformsÂ
After successful integration, test the functionality on both Android and iOS devices. Ensure consistent behavior and handle any platform-specific nuances.Â
Pro Tip: If you're unsure how your budget aligns with these technical enhancements, use a mobile app cost calculator to estimate expenses before diving into native module integration.Â
6. Handle Errors and Platform LimitationsÂ
Itâs important to gracefully handle scenarios where a feature is not available on one platform. Build fallbacks or conditional code execution based on the OS or device capabilities.Â
7. Optimize and MaintainÂ
Regularly update native modules to align with OS updates. Unmaintained native code can lead to app crashes, rejections from app stores, or security vulnerabilities.Â
Best PracticesÂ
Keep the native code modular and well-documented.Â
Avoid bloating your hybrid app with too many native modules unless necessary.Â
Leverage community-tested plugins when possible, but vet them for security and updates.Â
Monitor performance to ensure that native modules are not negatively impacting app speed or memory usage.Â
Real-World Use CaseÂ
Imagine a fitness app that requires real-time motion tracking. A hybrid app alone may lag in accurately capturing movement, but by integrating a native module to tap directly into accelerometer and gyroscope sensors, you can dramatically improve tracking accuracy and responsiveness.Â
This is just one of many scenarios where hybrid apps shine brighter when empowered with native capabilities.Â
Book an Appointment with Our ExpertsÂ
Integrating native modules can be tricky without deep knowledge of both mobile platforms. If you're unsure where to start or how to do it right, Book an Appointment with our experienced mobile developers today. We'll help you tailor the perfect strategy for your app.Â
ConclusionÂ
As hybrid app frameworks evolve, the need to blend native capabilities with cross-platform logic will only grow. By understanding and implementing native modules, developers can create high-performing, feature-rich apps without going fully native.Â
Looking to upgrade your hybrid application or start a new project from scratch? Our team offers top-notch hybrid app development services tailored to your business needs.Â
0 notes
Text
Why Learning Rust Programming is the Best Decision for Your Developer Journey

If you're a beginner exploring which programming language to learn, chances are you've come across Python, JavaScript, and even C++. But have you heard about Rust? Not only is it one of the most modern, fast, and memory-safe programming languages out there, but itâs also quickly climbing the ranks among the developer community.
So why are so many developers â including beginners â switching to Rust? The answer lies in its performance, security, and simplicity, all packed into one elegant language.
In this post, we'll explore the core concepts of Rust programming for beginners, what makes it special, and why you should seriously consider learning it to future-proof your coding career.
đ What is Rust, and Why is Everyone Talking About It?
Rust is a statically typed, compiled programming language designed for performance and safety, especially when it comes to concurrent programming. Developed by Mozilla, it's been lauded for combining the power of C and C++ with memory safety without needing a garbage collector.
This means Rust is:
Fast â as fast as C or C++.
Safe â it prevents memory-related bugs before they happen.
Reliable â no more segmentation faults or thread safety issues.
Loved â itâs consistently ranked #1 in Stack Overflowâs Developer Survey as the âmost loved programming language.â
The beauty of Rust lies in its balance â high performance without the fear of memory bugs. That's a dream come true for developers, especially those who are just starting.
đ° Why Rust is Perfect for Beginners
At first glance, Rust might look intimidating. Its syntax is different, its compiler is strict, and the learning curve can feel steep. But thatâs actually what makes it so perfect for beginners.
Here's why:
1. Rust Teaches You to Code the Right Way
The Rust compiler doesnât just throw errors â it guides you. Think of it as a helpful teacher. You'll get suggestions, explanations, and documentation that lead you to write clean, efficient, and safe code.
2. Memory Safety Without Garbage Collection
Unlike languages like Java or Python, Rust does not rely on a garbage collector. Instead, it uses a unique ownership model to manage memory safely and efficiently â a game-changer when it comes to system-level programming.
3. Fantastic Tooling
Tools like cargo (Rust's package manager and build system) and rustfmt (code formatter) make development easier and more organized. Even setting up a project feels seamless and beginner-friendly.
4. Growing Community & Learning Resources
The Rust community is known for being incredibly welcoming. Youâll find tons of resources â documentation, tutorials, forums â and supportive people who are happy to help.
And for those wanting to learn with structure, this Rust Programming for Beginners course is the perfect start. It's beginner-friendly and walks you through everything step-by-step, even if you're totally new to coding.
đ§ Key Concepts Youâll Learn as a Beginner in Rust
Getting started with Rust is more about understanding its core concepts than just memorizing syntax. Here are the most important building blocks youâll come across:
â
Ownership & Borrowing
This is Rustâs secret sauce. The ownership system ensures memory is safely managed without needing a garbage collector. Once you understand how variables own their data, youâll be amazed at how much it simplifies debugging and optimization.
â
Pattern Matching
Rust has powerful pattern matching using the match keyword. Itâs clean, readable, and far more expressive than traditional if/else logic in other languages.
â
Enums & Option/Result Types
Rust handles errors gracefully and safely with the Result and Option types. No more null references or unexpected crashes!
â
Lifetimes
They sound scary, but lifetimes are Rustâs way of making sure references live just long enough â not too little, not too long. And yes, the compiler will help you here too.
â
Traits & Generics
Want to write code thatâs flexible and reusable? Traits and generics in Rust help you do just that â without the bloat or confusion.
đŠâđť Real-World Applications of Rust
So where can you actually use Rust? Here's where it shines:
Operating Systems â It's being used in parts of Windows and Linux!
WebAssembly â You can compile Rust into WebAssembly to run in browsers.
Game Development â Thanks to its speed and memory safety.
CLI Tools â Many popular command-line tools are written in Rust.
Blockchain & Cryptography â Its memory safety makes it perfect for secure applications.
Embedded Systems â With minimal overhead, Rust works great on microcontrollers.
⨠What Makes Rust Different from Other Languages?
Letâs compare Rust briefly to other popular languages beginners usually learn: LanguagePerformanceMemory SafetyBeginner FriendlyCommunity SupportPythonđĄ Moderateđ˘ Safeđ˘ Veryđ˘ HighJavađ˘ Goodđ˘ Safeđ˘ Highđ˘ HighC++đ˘ Fastđ´ Riskyđ´ Steepđ˘ HighRustđ˘ Fastđ˘ Very SafeđĄ Moderateđ˘ Growing Fast
Rust finds the perfect middle ground. It gives you the performance of C++ and the safety of Java or Python â with modern tooling and cleaner syntax.
đŻ Who Should Learn Rust?
Rust is ideal for:
Absolute beginners who want to build a solid foundation.
Web developers curious about system-level performance.
Backend developers who want to write faster APIs.
Game developers interested in high-performance engines.
Security-conscious developers working in finance, healthcare, or blockchain.
IoT developers needing low-level control with safety.
In fact, learning Rust gives you an edge in both system-level and application-level programming â something few languages can offer.
đ§ How to Start Learning Rust (Without Feeling Overwhelmed)
Here's a simple roadmap you can follow:
Install Rust using rustup â the official installer.
Learn the basics of ownership, types, and control flow.
Build small CLI projects (like a to-do app or file parser).
Explore crates (Rustâs libraries) and use them in your projects.
Take a structured course, like the Rust Programming for Beginners, which covers all core concepts with practical examples.
Join the community â forums, Discord, Reddit, GitHub.
Keep experimenting â build, fail, learn, repeat!
đĄ Tips to Stay Motivated While Learning Rust
Break it down: Rust has a learning curve. Focus on one concept at a time.
Practice > Theory: Build small, real projects to reinforce your learning.
Use online playgrounds: You donât always need a full setup â try play.rust-lang.org to write and run code in your browser.
Follow Rustaceans (Rust developers) on social platforms to stay updated and inspired.
Celebrate your wins: Even if you understand ownership for the first time â thatâs huge!
đ§° Tools and Resources to Support Your Rust Learning Journey
The Rust Book â Free and official Rust guide online.
Rustlings â Hands-on small exercises to practice Rust syntax.
Crates.io â Explore and use open-source libraries.
Rust Analyzer â IDE plugin to make coding in Rust easier and smarter.
VS Code â Pair it with Rust plugins for a smooth dev experience.
But if you want all this knowledge structured and simplified for a beginner, the Rust Programming for Beginners course is hands-down the easiest way to start.
đ The Future of Rust: Why Youâre Getting in Early
Tech giants like Microsoft, Google, Amazon, and Dropbox are all investing in Rust. Major software like Firefox, Cloudflare, and even parts of Android OS use it under the hood.
Learning Rust today is like learning Python in 2009 â it sets you up to be ahead of the curve. And because so few people truly master it, Rust developers are in high demand.
đ Final Thoughts: Start Today, Build Smarter Tomorrow
Rust is not just another programming language. Itâs a movement toward safer, faster, and more reliable code. Whether youâre aiming to become a system developer, improve your backend skills, or build next-gen applications, Rust will be your secret weapon.
So if youâre a beginner ready to start coding with confidence, explore the Rust Programming for Beginners course and kickstart your journey today.
Remember â every expert was once a beginner. And the best time to start learning Rust is now. đ
0 notes
Text
Best Practices for Writing Efficient Java Code
Starting your journey with the best Java training in Hyderabad can be the game-changer you need to become a confident and efficient Java developer. Quality training helps you not just understand Java syntax, but also teaches you how to write optimized, clean, and professional-grade code that performs well in real-world applications.
1. Keep Loops Simple and Efficient
One of the easiest ways to boost code performance is by refining your loops. Avoid deep nesting and eliminate redundant iterations. Use enhanced for-each loops when working with collections, and prefer StringBuilder for string manipulation inside loops to save memory and processing time.
2. Take Advantage of Java Libraries
Java offers a wide range of reliable, built-in libraries. Rather than creating custom functions for common tasks, make use of these tried-and-tested tools. Theyâre not only optimized for performance but also help in writing less code with fewer bugs.
3. Stick to Core OOP Concepts
Writing efficient Java code starts with designing well-structured programs. Apply object-oriented principles like abstraction, inheritance, and polymorphism to build scalable and reusable components. Break down larger problems into smaller, manageable classes for better code organization.
4. Handle Resources Smartly
Improper handling of system resources can cause memory leaks. Always close files, streams, and database connections after use. The try-with-resources statement in Java is a great way to manage and close resources automatically.
5. Write Clean, Readable Code
Readable code is maintainable code. Use clear and descriptive names for variables and methods, and maintain consistent formatting. Add comments where necessary to make the logic easy to follow for future developersâor yourself.
Conclusion
It takes time and the right guidance to become proficient in Java. If you're serious about building a career in software development, choose SSSIT Computer Educationâa leading institute for Java training in Hyderabad. With expert mentors and practical learning, youâll gain the skills to write top-quality, efficient Java code.
1 note
¡
View note