#laravel echo
Explore tagged Tumblr posts
Text
How to Prevent Cross-Site Script Inclusion (XSSI) Vulnerabilities in Laravel
Introduction
Cross-Site Script Inclusion (XSSI) is a significant security vulnerability that allows attackers to include malicious scripts in a web application. These scripts can be executed in the context of a user’s session, leading to data theft or unauthorized actions.

In this post, we’ll explore what XSSI is, how it impacts Laravel applications, and practical steps you can take to secure your app.
What is Cross-Site Script Inclusion (XSSI)?
XSSI occurs when a web application exposes sensitive data within scripts or includes external scripts from untrusted sources. Attackers can exploit this by injecting malicious scripts that execute within the user’s browser. This can lead to unauthorized access to sensitive data and potentially compromise the entire application.
Identifying XSSI Vulnerabilities in Laravel
To prevent XSSI, start by identifying potential vulnerabilities in your Laravel application:
Review Data Endpoints: Ensure that any API or data endpoint returns the appropriate Content-Type headers to prevent the browser from interpreting data as executable code.
Inspect Script Inclusions: Make sure that only trusted scripts are included and that no sensitive data is embedded within these scripts.
Use Security Scanners: Utilize tools like our Website Vulnerability Scanner to analyze your app for potential XSSI vulnerabilities and get detailed reports.

Screenshot of the free tools webpage where you can access security assessment tools.
Mitigating XSSI Vulnerabilities in Laravel
Let’s explore some practical steps you can take to mitigate XSSI risks in Laravel.
1. Set Correct Content-Type Headers
Make sure that any endpoint returning JSON or other data formats sets the correct Content-Type header to prevent browsers from interpreting responses as executable scripts.
Example:
return response()->json($data);
Laravel’s response()->json() method automatically sets the correct header, which is a simple and effective way to prevent XSSI.
2. Avoid Including Sensitive Data in Scripts
Never expose sensitive data directly within scripts. Instead, return data securely through API endpoints.
Insecure Approach
echo "<script>var userData = {$userData};</script>";
Secure Approach:
return response()->json(['userData' => $userData]);
This method ensures that sensitive data is not embedded within client-side scripts.
3. Implement Content Security Policy (CSP)
A Content Security Policy (CSP) helps mitigate XSSI by restricting which external sources can serve scripts.
Example:
Content-Security-Policy: script-src 'self' https://trusted.cdn.com;
This allows scripts to load only from your trusted sources, minimizing the risk of malicious script inclusion.
4. Validate and Sanitize User Inputs
Always validate and sanitize user inputs to prevent malicious data from being processed or included in scripts.
Example:
$request->validate([ 'inputField' => 'required|string|max:255', ]);
Laravel’s built-in validation mechanisms help ensure that only expected, safe data is processed.
5. Regular Security Assessments
Conduct regular security assessments to proactively identify potential vulnerabilities. Tools like our free Website Security Scanner can provide detailed insights into areas that need attention.

An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities.
Conclusion
Preventing Cross-Site Script Inclusion (XSSI) vulnerabilities in your Laravel applications is essential for safeguarding your users and maintaining trust. By following best practices like setting proper content-type headers, avoiding sensitive data exposure, implementing CSP, validating inputs, and regularly assessing your app’s security, you can significantly reduce the risk of XSSI attacks.
Stay proactive and secure your Laravel applications from XSSI threats today!
For more insights into securing your Laravel applications, visit our blog at Pentest Testing Corp.
3 notes
·
View notes
Text
Fluent CLI в PHP: Создаём консольные команды с __call и никаких танцев с бубном
В мире разработки часто возникает потребность в работе с командной строкой — будь то выполнение системных команд, обработка их вывода или работа с различными кодировками. Столкнувшис�� с такими задачами, программисты часто начинают искать готовые решения, чтобы сэкономить время и избежать повторения однотипного кода.
Именно для таких случаев была разработана библиотека PhpFluentConsole — простое и гибкое решение для работы с командной строкой, с поддержкой кодировок и множеством полезных фич для работы с выводом команд.
Зачем нужна эта библиотека?
PhpFluentConsole не заменяет существующие библиотеки, а выступает как легковесная основа для гибкого построения CLI-запросов. Её цель — упростить интеграцию системных команд без необходимости изучать объёмную документацию или вникать в сложный API.
Основная цель библиотеки — упрощение и автоматизация выполнения команд, а также обработка вывода, что делает её удобной для разработчиков, которые хотят создавать свои собственные решения на основе этой библиотеки.
Преимущества использования
Гибкость и удобство:
Библиотека поддерживает текучий интерфейс для построения команд, что делает код максимально читаемым и лаконичным. Пример:$cli = new ConsoleRunner() ->setCommand('echo') ->addKey('Hello, World!')
Поддержка кодировок:
Для работы с кириллицей и другими символами, библиотека включает поддержку различных кодировок, таких как CP866, UTF-8, CP1251, и других. Это особенно важно при работе в windows-среде, где часто возникают проблемы с выводом текста в стандартных кодировках, что так же может затруднить обработку вывода с помощью регулярных выражений. Пример:$cli = new ConsoleRunner() ->setCommand('dir') ->encoding('866')
Обработка ошибок и кодов возврата:
Библиотека позволяет получать код возврата после выполнения команды и искать ошибки в выводе с помощью регулярных выражений. Это важно, если вам нужно удостовериться, что команда завершилась успешно или отловить ошибку в выводе. Пример:if (!$cli->run()) { echo "Ошибка выполнения команды!" . $cli->getReturnCode(); }
Поиск совпадений по паттернам:
Вы можете искать совпадения в выводе команды, используя регулярные выражения. Это полезно, если нужно извлечь конкретную информацию из вывода команд, например, лог-файлов или диагностики системы. Пример:if ($cli->run()) { $matches = $cli->getMatches('/Error/'); print_r($matches); }
Стандартный вывод:
Если нам просто нужно получить массив строк. Пример:if ($cli->run()) { print_r($cli->getOutput()); }
Установка
composer require mikhailovlab/php-fluent-console
Описание методов
Устанавливает команду для выполнения.public function setCommand(string $cmd): self
Добавляет аргумент или часть команды.public function addKey(string $key): self
Устанавливает кодировку для вывода. Например, '866' для отображения кириллицы в Windows.public function encoding(?string $encoding = null): self
Устанавливает флаг, что нужно выполнить обратную конвертацию кодировки. Метод полезен для возврата вывода в исходной кодировке для работы с cmd.public function decoding(): self
Возвращает текущую команду.public function getCommand(): string
Выполняет команду и возвращает true, если код возврата равен 0.public function run(): bool
Возвращает вывод после выполнения команды.public function getOutput(): array
Возвращает код возврата выполнения команды.public function getReturnCode(): int
Проверяет вывод на наличие ошибки по регулярному выражению.public function hasError(string $pattern): bool
Возвращает все строки вывода, совпавшие с регулярным выражением.public function getMatches(string|array $patterns): array
Примеры
Проверять функционал будем на примере фреймворка Laravel 11, но так же можно использовать любой другой, это непринципиально:
Пример 1: Получаем IP адрес в операционной системе windows
$cli = new ConsoleRunner() ->setCommand('ipconfig') ->addKey('/all') ->encoding('866'); //ожидаем вывод с кириллицей if ($cli->run()) { dd($cli->getOutput()); }
Вывод:array:59 [▼ // app\Http\Controllers\TestController.php:21 0 => "" 1 => "Настройка протокола IP для Windows" 2 => "" 3 => " Имя компьютера . . . . . . . . . : DESKTOP-HRTB4N9" 4 => " Основной DNS-суффикс . . . . . . :" 5 => " Тип узла. . . . . . . . . . . . . : Гибридный" 6 => " IP-маршрутизация включена . . . . : Нет" 7 => " WINS-прокси включен . . . . . . . : Нет" 8 => " Порядок просмотра суффиксов DNS . : lan" 9 => "" 10 => "Адаптер Ethernet Ethernet 2:" ...
Кириллица отображается корректно, можно двигаться дальше.
Пример 2: Получаем список контейнеров с электронными подписями
$cli = new ConsoleRunner() ->setCommand('csptest') ->addKey('-keyset') ->addKey('-enum_cont') ->addKey('-verifycontext') ->addKey('-fqcn'); if ($cli->run()) { dd($cli->getMatches('#\\\\.*#')); } $pattern = '/\[ErrorCode:\s*(0x[0-9A-Fa-f]+)\]/'; dd('Error code: ' . $cli->getMatches($pattern)[0]);
Вывод:array:1 [▼ // app\Http\Controllers\TestController.php:23 0 => "\\.\REGISTRY\d58fe6c13-d917-2a53-8e9c-8c4b8158220-test" ]
В принципе работает, но если мы собираемся разрабатывать свою библиотеку, такой подход может показаться избыточным.
Пример 3: Расширяем базовый класс
Мы можем наследоваться от базового класса ConsoleRunner и вместо указания методов через addKey, вызывать их динамически, используя магический метод __call. Как правило, в консольных утилитах мы можем получить список всех доступных методов и аргументов используя ключ -help и добавить их в массив, что бы исключить ошибки, т.к. при несовпадении будет выброшено исключение.class customRunner extends ConsoleRunner { private $methods = [ 'keyset', 'enum_cont', 'verifycontext', 'fqcn' ]; public function __call(string $name, array $arguments): self { if (in_array($name, $this->methods)) { $this->addKey('-' . $name); if (!empty($arguments)) { foreach ($arguments as $arg) { $this->addKey((string) $arg); } } return $this; } throw new \BadMethodCallException("Method $name is not supported"); } } try{ $cli = new customRunner() ->setCommand('csptest') ->keyset() ->enum_cont() ->verifycontext() ->fqcn(); if ($cli->run()) { dd($cli->getMatches('#\\\\.*#')); } $pattern = '/\[ErrorCode:\s*(0x[0-9A-Fa-f]+)\]/'; dd('Error code: ' . $cli->getMatches($pattern)[0]); }catch (Exception $e){ dd($e->getMessage()); }
Вывод:array:1 [▼ // app\Http\Controllers\TestController.php:23 0 => "\\.\REGISTRY\d58fe6c13-d917-2a53-8e9c-8c4b8158220-test" ]
Мы можем перенести логику и обработку вывода в отдельные методы, что бы сделать код еще более лаконичным.try{ $containers = new customRunner() ->getContainers() ->run(); dd($containers); }catch (Exception $e){ dd($e->getMessage()); }
Таким образом мы можем наследоваться от базового класса и создавать свои классы-обертки, в которых будем описывать методы и обрабатывать вывод.
Заключение
PhpFluentConsole — это удобный и гибкий инструмент для работы с командной строкой. Он позволяет строить команды через простой и понятный интерфейс, поддерживает работу с кодировками, предоставляет возможности для обработки ошибок и извлечения данных из вывода команд. Библиотека является отличной основой для создания более сложных решений или даже для разработки других библиотек на её основе, таких как CryptoProBuilder, о которой я расскажу в следующей статье.
0 notes
Text
Complete Guide to Hiring Laravel Developers: Tips and Best Practices
Introduction
In the dynamic world of network development, it is necessary and challenging to find talented Laravel developers. A popular PHP network has been observed for frameworks, Laravel, its pure syntax, powerful features and strong developer communities. Leasing the right Laravel developers is important to secure the success of your web projects.
In this comprehensive manual, we can pressure you through the process of hiring Laravel developers, who will cover the entirety from defining their necessities to successfully comparing candidates.
Why Laravel Is the Go-To Framework for Modern Web Development
Introduction to Laravel and Its Popularity
An elegant and powerful PHP structure, Laravel has greatly affected the net development with its expressive syntax and strong properties. The extensive popularity comes from the opportunity to streamline general network development features such as authentication, routing and improving cache, so developers can focus on creating unique application features. This efficiency makes it a preferred alternative to companies and developers, which continuously improves the demand for skilled Laravel professionals.
Benefits of Using Laravel for Scalable Projects
Laravel provides many benefits, making it an excellent choice to create scalable and maintenance web applications:
MVC Architecture:Its Model-Vs-Controller (MVC) Architectural patterns sell prepared, modular and reformulated code to simplify the manipulation and scaling of architectural styles and complex projects.
Built-in Features: Laravel comes tool without-of-the-field functionalities like authentication, authorization, and API support, substantially accelerating development time.
Enhanced Security: It gives sturdy security facilities along with SQL injection, Cross-Site Request Founder (CSRF) and protection against Cross-Site Scripting (XSS), which guarantees information integrity and user safety.
Artisan CLI: Powerful command line interface (CLI), Artisan, Database Migration and Code Generation automates repetitive capabilities, promotes the developer's productivity.
Vast Ecosystem and Community: Laravel boasts a huge and lively network, offering sizable documentation, tutorials, and a wealth of organized-to-use applications, which simplifies development and troubleshooting.
Modern Trends Compatibility: Laravel is continuously evolving to help cutting-edge development tendencies like microservices shape, serverless deployments (through Laravel Vapor), AI integration, real-time packages (with Laravel Echo and WebSockets), and headless CMS setups.
Why Businesses Prefer to Hire Laravel Developers
Businesses frequently pick to hire Laravel developers because of the framework's performance, safety, and scalability. Laravel enables quicker improvement cycles, thereby lowering the time-to-market for emblem spanking new applications. Its easy code and well-defined architectural styles result in less difficult upkeep and destiny improvements, offering a robust return on funding. Furthermore, the lively community useful resource ensures that builders can speedy find out answers and cling to great practices, further streamlining project execution.
When Should You Hire a Laravel Developer?
Signs You Need a Dedicated Laravel Developer
If you understand any of the following signs, you can need to hire a Laravel developer
Increasing Project Complexity: Your present day team is suffering to deal with the growing complexity of your web utility.
Slow Development Pace: The growth cycles are increased, and the rollout of new functions is dull.
Performance Issues: Your current application met the hedges or challenges of scalability.
Security Concerns: You need increased security measures and comply with modern security standards online.
Lack of Specialized Expertise: Your in-house team lacks specific larval knowledge or experience with advanced functions such as larvae Octane or integrated AI/ML.
Project Types Ideal for Laravel Development
The versatility of the laravel is suitable for a wide range of projects, including:
Custom Web Applications: Construction of unique business equipment, CRM or ERP that fits specific requirements.
E-commerce Platforms: Development of scalable online stores with strong payment ports and complex product management.
Content Management Systems (CMS): Flexible production, user-friendly and easy to manage material platforms including headless CMS implementation.
APIs and Backend Services: Mobile applications, applications with one-position (SPA) and powerful backing crafts for third-party integration.
SaaS Applications: Develop software program-e-service answers with multi-tarm-e-provider that require high scalability and strong user management.
In-House vs. Outsourced Laravel Development
When considering how to hire Laravel developers, you mainly have two strategic options:
In-House Development:This involves hiring full -time employees to work directly in your organization. It provides high control and direct communication, but often comes with high overhead costs (salaries, profits, office space) and can be a slow process of recruitment.
Outsourced Development: IT forces partnership with external experts or agencies.The options include hiring freelancers, operating with a committed improvement agency or deciding on an offshore team. Outsourcing provides flexibility, get right of entry to to a global abilities pool, and regularly provides extensive fee financial savings.
How to Hire Laravel Developers: A Step-by-Step Process
Define Your Project Requirements Clearly
It is important to define your project well before you go ahead to hire the laravel developers in India talent. It also includes:
Project Goals and Objectives: Clearly explain what you want to achieve with the application.
Key Features and Functionalities: List all the necessary features and desired user streams.
Technical Specifications: preferred technical stacks, essential integration (eg payment port, external API), and expanded expectations of performance.
Timeline and Budget: Establish realistic time constraints and benefit from the right financial resources. A clear scope of the project will largely help to identify proper developers with accurate skills and necessary experience.
Decide Between Hiring Freelancers, Agencies, or Offshore Teams
The alternative between these models depends on the scope of the project, budget and desired control level:
Freelancers: Ideal for small projects, specific tasks, or when you need rapid changes for well -defined tasks. They offer flexibility and competitive prices, but long -term support and frequent accessibility can be an idea.
Agencies: A complete team, best suited for complex projects that require extensive project management and underlying quality assurance procedures. They usually come with a prize, but give a more structured approach.
Offshore Teams:A very popular choice to achieve cost-effectiveness and access a large global talent pool. It may include dedicated teams from areas known for strong technical talents such as India.
Why Many Businesses Hire Laravel Developers in India
Many companies choose to hire Laravel developers in India because of the country's talented, English -speaking developers and sufficient pools with very competitive prices. Indian Laravel developers regularly have widespread experiences in one-of-a-kind industries and are professional in running with international customers, making them a strategic alternative for outsourcing.
Benefits of Hiring Offshore Laravel Developers
Hiring an offshore Laravel developer or dedicated team provides many compelling benefits:
Cost-Effectiveness: Significant cost savings compared to employment in areas with high life costs often reduce growth expenses by a sufficient margin.
Access to Global Talent: Take advantage of a wide talent pool so you can find very specific developers with niche skills that can be locally rare.
Time Zone Advantages: Can enable development around the clock, where the work continues even after the local team's commercial hours potentially accelerates the project.
Scalability and Flexibility: Local employment -affiliated complications and the needs of ups and downs without overhead give the agility to easily score your team up or down.
Evaluate Technical Skills and Experience
When you set out to hire Laravel developers, consider their skills well in the following areas:
PHP and Laravel Framework: PHP Fundamental, Object-Oriented Programming (OOP) The deep understanding of the principles, and specialized in the main components of the laravel
MVC Architecture:A sturdy grasp of the Model-View-Controller layout sample and a way to correctly enforce it within Laravel.
Database Management: Extensive revel in relational databases like MySQL, PostgreSQL, and potentially NoSQL databases like MongoDB, consisting of database design, optimization, and query writing.
RESTful APIs: The capability to layout, construct, and devour RESTful APIs for seamless records exchange and integration with different systems.
Version Control:Proficiency with Git and collaborative structures which include GitHub, GitLab, or Bitbucket.
Front-end Technologies: Familiarity with HTML, CSS, JavaScript, and preferably revel in with present day JavaScript frameworks like Vue.Js (Laravel's default front-stop framework), React, or Angular.
Testing: Experience with PHPUnit for unit and characteristic checking out, and a commitment to writing testable code.
Conduct Technical Interviews and Code Assessments
In addition to reviewing the CV, the operation of complete technical evaluation of the laravel importance is:
Technical Interviews: Ask questions that examine their understanding of Laravel concepts, their attitude to problems and specific experiences from previous projects. Ask about their knowledge of newer largest updates and trends (eg largest octane, live wire).
Code Assessments/Live Coding: Provide a practical coding challenge or a small home project. This allows you to evaluate their actual coding skills, best practice, code quality and ability to meet specified requirements.
Portfolio Review: Carefully examine their previous work. Look for different projects that demonstrate their ability to handle complexity, create scalable applications and integrate different systems.
Where to Find and Hire Laravel Developers
Top Platforms to Hire Laravel Developers
Freelance Platforms: Popular options include Upwork, Fiver and Top tier (top-races known for their hard Beating process).
Job Boards: LinkedIn, General Job Tablets such as Real and Glassdoor, and professional network pages as a specialized technical job board.
Developer Communities: Github, Stack Overflow and Laravel Specific Forums (e.g. Laravel.io, Laracasts) can be an excellent source for finding the developers active and experts on platforms such as Laravel.io, Laracasts.
Specialized Agencies: recruitment agencies or development companies that are experts in larva development and growth of employees can provide talent before reviewing and extensive recruitment solutions.
Should You Hire Laravel Developers in India?
Yes, hiring Laravel developers in India is a very viable and often beneficial alternative for businesses. India provides a strong balance between cost -effectiveness and quality. The country's strong IT education system produces a large number of talented people, many of whom have experience working with international customers and understanding the method of the global project. In addition, many Indian development companies offer extensive services including project management and quality assurance.
Pros and Cons of Hiring Through Freelance Platforms
Pros:
Cost-Effective: Often cheaper than attractive agencies that create their own employment.
Flexibility: For quick -time period initiatives or specific responsibilities, clean agility to scale your group provides up or down.
Diverse Talent Pool: Access to a wide variety of developers with exceptional understanding and global approach..
Cons:
Less Control: Internal teams or dedicated agency models can pay less direct inspection and dedicated attention.
Communication Challenges:The difference and relevant language barriers in the field require potential, active management.
Commitment Issues:Freelancers can control several projects at the identical time, that may potentially affect their committed attention in your project.
Long-Term Support: Securing consistent prolonged-time period manual or ongoing preservation from man or woman freelancers can sometimes be challenging.
Key Skills to Look for in a Laravel Developer
Must-Have Technical Skills (PHP, Laravel, MVC, APIs)
As highlighted, middle technical skills are foundational. Ensure applicants demonstrate robust proficiency in:
PHP: A thorough facts of present day PHP versions, together with object-oriented programming (OOP), design styles, and outstanding practices.
Laravel Framework: Expertise in all components of the Laravel framework, which incorporates routing, controllers, Eloquent ORM, migrations, queues, middleware, broadcasting, and Blade templating.
MVC Architecture: The potential to efficiently form packages adhering to the Model-View-Controller layout pattern for maintainability and scalability.
APIs: Extensive experience in each building sturdy RESTful APIs and integrating with third-party APIs. Familiarity with GraphQL can be a significant plus.
Database Knowledge: Strong command of SQL, database layout principles, question optimization, and enjoyment with applicable database structures.
Front-end Integration: Competence in integrating Laravel with the front-give up generation and JavaScript frameworks, in particular Vue.Js or Livewire.
Soft Skills: Communication, Collaboration, and Problem-Solving
Technical prowess is vital, but gentle capabilities are similarly essential for group concord and project success. Look for:
Communication: Clear, concise, and effective verbal and written communication talents. This is especially important when you lease offshore Laravel developer groups to bridge geographical distances..
Collaboration: The skills to seamlessly interior a team's environment, make use of collaborative equipment (e.G., Jira, Trello, Slack), and actively participate in code reviews.
Problem-Solving:Strong analytical abilities to speedy understand complicated troubles, debug efficiently, and devise revolutionary, efficient answers.
Adaptability: Openness to mastering the new generation, adopting evolving satisfactory practices, and adapting to adjustments in venture necessities or priorities.
Industry Experience and Portfolio Evaluation
Relevant Industry Experience: Assess if the developer has prior experience running on tasks much like yours or within your specific enterprise area. This can frequently translate to a quicker expertise of enterprise needs and commonplace demanding situations.
Portfolio: A nicely-curated portfolio showcasing diverse tasks, clean code, and a successful real-global implementation is a robust indicator of a developer's talents. Pay attention to the complexity of the projects, their function in every, and how they contributed to the answer.
Cost of Hiring Laravel Developers
Global Laravel Developer Rates
The fee to hire Laravel developers varies appreciably based totally totally on factors at the side of vicinity, stage of experience, and the selected engagement version (freelancer, agency, or dedicated team). As of 2025:
North America/Western Europe: Typically the best fees, starting from $70-$100 fifty in line with hours for mid to senior-stage developers.
Eastern Europe: Mid-variety costs, regularly among $30-$60 per hour.
South Asia (e.g., India): Generally the maximum value-effective, with fees from $15-$forty in line with an hour for experienced skills. It's crucial to note that senior developers and people with relatively specialised abilities (e.G., DevOps for Laravel, AI/ML integration) will command better quotes irrespective of vicinity.
Why Hiring Laravel Developers in India Is Cost-Effective
When you choose to hire Laravel developer in India, you can comprehend sizable fee financial savings without always compromising on satisfaction. The lower price of residing and operational expenses in India without delay translate to extra aggressive hourly or challenge quotes. Despite the decreased value, India possesses a big and growing pool of exceptionally skilled Laravel professionals with strong technical backgrounds and massive revel in working with diverse global clients.
How to Balance Budget and Quality When Hiring Offshore
To correctly balance cost and exceptional while you hire offshore Laravel developer teams:
Clear and Detailed Requirements: Well-described undertaking necessities and popularity standards save you scope creep and make sure the offshore group grants exactly what is wanted, minimizing rework.
Thorough Vetting Process: Do not pass comprehensive technical assessments, more than one rounds of interviews, and rigorous portfolio reviews, even if thinking about price-effective offshore options.
Start Small with a Pilot Project:Consider beginning a smaller, nicely-scoped trial assignment to evaluate the group's capabilities, communique effectiveness, and usual health before dedicating Laravel , lengthy-term engagement.
Look for Transparency: Partner with carriers who provide transparent pricing fashions, provide ordinary development updates, and maintain clean, proactive verbal exchange channels in the course of the project lifecycle.
Best Practices to Hire Laravel Developers Successfully
Write a Clear Job Description
A precise, specific, and attractive process description is crucial for attracting the proper candidates. Ensure it sincerely specifies:
Role and Responsibilities: Define the everyday responsibilities and prevalent expectancies for the position.
Required Skills: List each the critical technical abilities and ideal tender skills.
Project Details: Briefly describe the type of initiatives the developer can be working on and the commercial enterprise impact.
Compensation and Benefits:Outline the revenue range, any perks, and the running model (e.G., faraway, hybrid, in-workplace, contract).
Use Technical Assessments and Trial Tasks
As previously emphasised, sensible assessments are crucial for validating a candidate's talents. Beyond theoretical expertise, technical tests or small trial responsibilities show a developer's capability to jot down easy, efficient, nicely-examined, and maintainable code in a real-international situation. This also allows checking their hassle-fixing technique and adherence to coding standards.
Protect Your IP with Contracts and NDAs
Safeguarding your highbrow assets (IP) and touchy enterprise records is paramount. Implement robust felony files such as:
Non-Disclosure Agreements (NDAs): Ensure the confidentiality of proprietary statistics, exchange secrets, and venture information.
Intellectual Property Assignment Agreements:Clearly outline the ownership of all code, designs, and some other property advanced in the course of the engagement. These felony protections are particularly important while you lease offshore Laravel builders or have interaction with freelancers.
Foster Long-Term Relationships with Talented Developers
Building enduring relationships with your Laravel developers, whether they're in-residence or outsourced, yields sizable blessings. Provide opportunities for professional increase, provide truthful and competitive compensation, and cultivate a supportive and collaborative working environment. Engaged and valued developers are much more likely to supply first-rate paintings, remain devoted to your initiatives, and become useful long-time period assets for your enterprise.
Common Mistakes to Avoid When You Hire a Laravel Developer
Ignoring Communication Skills
A frequent pitfall is focusing entirely on a candidate's technical competencies even as overlooking their communication skills. Poor conversation can result in commonplace misunderstandings, assignment delays, rework, and a miles less efficient and collaborative group surroundings, regardless of how technically talented a developer can be. Always verify their capacity to articulate ideas really, ask pertinent questions, and provide positive comments.
Focusing Only on Cost
While price-effectiveness is a considerable benefit, mainly when you recall alternatives to hire Laravel developers in India, creating a hiring choice based entirely on the bottom rate can frequently show to be a false economic system. Extremely low quotes may indicate compromised excellent, overlooked closing dates, a loss of enjoyment, or insufficient guidance, doubtlessly costing you extra in terms of misplaced time, transform, and in the long run, a subpar product. Prioritize typical cost, verified understanding, and reliability over more price.
Skipping Background or Code Quality Checks
Never bypass vital due diligence. Always affirm references, thoroughly review past projects, and conduct rigorous code nice checks on sample paintings or in the course of technical tests.This allows verifying a developer's claimed capabilities, guarantees their adherence to enterprise nice practices, and minimizes the threat of collecting technical debt for your project, which may be high-priced to rectify later.
Final Thoughts: Making the Right Hire for Your Laravel Project
Hiring the right Laravel developer or development team is a strategic decision that can determine the depth of success and the path of your web project. By understanding the strong characteristics of the largest, carefully defined the specific needs of your project, and following a structured and comprehensive recruitment process, you can safely identify and on board talented individuals who will bring your vision to the fruit. Whether you choose to hireLaravel developers locally, associate with a particular agency, or strategically, choose us for the hiring offshore Laravel development teams for cost-willing and access to various global talents, a thoughtful and hard-working attitude will ensure you make the optimal hire for your Laravel project.
Know more>>https://medium.com/@ridh57837/complete-guide-to-hiring-laravel-developers-tips-and-best-practices-f81a501a60dc
#hire laravel developer#hire laravel developers#hire laravel developers india#hire offshore laravel developer#hire laravel developer in india
0 notes
Text
Complete PHP Tutorial: Learn PHP from Scratch in 7 Days
Are you looking to learn backend web development and build dynamic websites with real functionality? You’re in the right place. Welcome to the Complete PHP Tutorial: Learn PHP from Scratch in 7 Days — a practical, beginner-friendly guide designed to help you master the fundamentals of PHP in just one week.
PHP, or Hypertext Preprocessor, is one of the most widely used server-side scripting languages on the web. It powers everything from small blogs to large-scale websites like Facebook and WordPress. Learning PHP opens up the door to back-end development, content management systems, and full-stack programming. Whether you're a complete beginner or have some experience with HTML/CSS, this tutorial is structured to help you learn PHP step by step with real-world examples.
Why Learn PHP?
Before diving into the tutorial, let’s understand why PHP is still relevant and worth learning in 2025:
Beginner-friendly: Easy syntax and wide support.
Open-source: Free to use with strong community support.
Cross-platform: Runs on Windows, macOS, Linux, and integrates with most servers.
Database integration: Works seamlessly with MySQL and other databases.
In-demand: Still heavily used in CMS platforms like WordPress, Joomla, and Drupal.
If you want to build contact forms, login systems, e-commerce platforms, or data-driven applications, PHP is a great place to start.
Day-by-Day Breakdown: Learn PHP from Scratch in 7 Days
Day 1: Introduction to PHP & Setup
Start by setting up your environment:
Install XAMPP or MAMP to create a local server.
Create your first .php file.
Learn how to embed PHP inside HTML.
Example:
<?php echo "Hello, PHP!"; ?>
What you’ll learn:
How PHP works on the server
Running PHP in your browser
Basic syntax and echo statement
Day 2: Variables, Data Types & Constants
Dive into PHP variables and data types:
$name = "John"; $age = 25; $is_student = true;
Key concepts:
Variable declaration and naming
Data types: String, Integer, Float, Boolean, Array
Constants and predefined variables ($_SERVER, $_GET, $_POST)
Day 3: Operators, Conditions & Control Flow
Learn how to make decisions in PHP:
if ($age > 18) { echo "You are an adult."; } else { echo "You are underage."; }
Topics covered:
Arithmetic, comparison, and logical operators
If-else, switch-case
Nesting conditions and best practices
Day 4: Loops and Arrays
Understand loops to perform repetitive tasks:
$fruits = ["Apple", "Banana", "Cherry"]; foreach ($fruits as $fruit) { echo $fruit. "<br>"; }
Learn about:
for, while, do...while, and foreach loops
Arrays: indexed, associative, and multidimensional
Array functions (count(), array_push(), etc.)
Day 5: Functions & Form Handling
Start writing reusable code and learn how to process user input from forms:
function greet($name) { return "Hello, $name!"; }
Skills you gain:
Defining and calling functions
Passing parameters and returning values
Handling HTML form data with $_POST and $_GET
Form validation and basic security tips
Day 6: Working with Files & Sessions
Build applications that remember users and work with files:
session_start(); $_SESSION["username"] = "admin";
Topics included:
File handling (fopen, fwrite, fread, etc.)
Reading and writing text files
Sessions and cookies
Login system basics using session variables
Day 7: PHP & MySQL – Database Connectivity
On the final day, you’ll connect PHP to a database and build a mini CRUD app:
$conn = new mysqli("localhost", "root", "", "mydatabase");
Learn how to:
Connect PHP to a MySQL database
Create and execute SQL queries
Insert, read, update, and delete (CRUD operations)
Display database data in HTML tables
Bonus Tips for Mastering PHP
Practice by building mini-projects (login form, guest book, blog)
Read official documentation at php.net
Use tools like phpMyAdmin to manage databases visually
Try MVC frameworks like Laravel or CodeIgniter once you're confident with core PHP
What You’ll Be Able to Build After This PHP Tutorial
After following this 7-day PHP tutorial, you’ll be able to:
Create dynamic web pages
Handle form submissions
Work with databases
Manage sessions and users
Understand the logic behind content management systems (CMS)
This gives you the foundation to become a full-stack developer, or even specialize in backend development using PHP and MySQL.
Final Thoughts
Learning PHP doesn’t have to be difficult or time-consuming. With the Complete PHP Tutorial: Learn PHP from Scratch in 7 Days, you’re taking a focused, structured path toward web development success. You’ll learn all the core concepts through clear explanations and hands-on examples that prepare you for real-world projects.
Whether you’re a student, freelancer, or aspiring developer, PHP remains a powerful and valuable skill to add to your web development toolkit.
So open up your code editor, start typing your first <?php ... ?> block, and begin your journey to building dynamic, powerful web applications — one day at a time.

0 notes
Text
Best Laravel Development Services for Fintech App Security & Speed
In 2025, the fintech sector is booming like never before. From digital wallets and neobanks to loan management systems and investment platforms, the demand for secure, fast, and scalable applications is skyrocketing. Behind many of these high-performing platforms lies one key technology: Laravel development services.
Laravel is a PHP-based web framework known for its elegant syntax, built-in security features, and flexibility. It has quickly become a go-to solution for fintech companies looking to build robust and future-ready apps.
In this blog, we’ll dive deep into why Laravel development services are the best choice for fintech applications, especially when it comes to security and speed. We’ll also answer key FAQs to help you make an informed decision.
Why Laravel is the Smart Choice for Fintech Development
1. Bank-Grade Security
Security is non-negotiable in fintech. Laravel offers features like:
CSRF protection
Encrypted password hashing (Bcrypt and Argon2)
SQL injection prevention
Two-factor authentication integrations
Secure session handling
When you hire expert Laravel development services, you ensure that your fintech app is guarded against common cyber threats and vulnerabilities.
2. Speed & Performance Optimization
In fintech, milliseconds matter. Laravel is designed for high performance. With features like:
Built-in caching with Redis or Memcached
Lazy loading of data
Queues for background processing
Lightweight Blade templating engine
Laravel apps are optimized to run fast and efficiently, even with complex data and multiple users.
3. Modular & Scalable Structure
Fintech startups need to evolve quickly. Laravel’s modular architecture allows developers to add new features without rewriting the whole app. Need to add payment gateways, KYC verification, or investment tracking? Laravel makes it easier and more maintainable.
4. API-Ready Backend
Most fintech apps need strong API support for mobile apps, third-party services, or internal dashboards. Laravel offers:
RESTful routing
API authentication with Laravel Sanctum or Passport
Seamless data exchange in JSON format
This makes Laravel development services ideal for creating flexible, API-first applications.
5. Developer Ecosystem & Community
Laravel has one of the strongest developer communities, which means:
Quick access to pre-built packages (e.g., for payments, SMS alerts, OTP login)
Frequent updates and support
Access to Laravel Nova, Horizon, and Echo for admin panels, job queues, and real-time data respectively
This helps fintech businesses reduce time-to-market and focus on innovation.
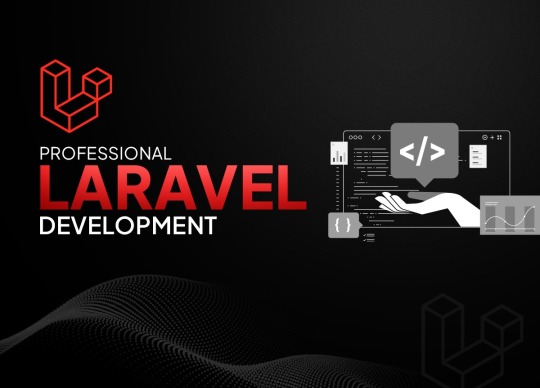
Real-World Use Case: Laravel in Fintech
A Canadian lending startup partnered with a Laravel development services provider to build a loan origination platform. The app included borrower onboarding, KYC checks, EMI tracking, and real-time risk analysis. Using Laravel:
The app handled over 10,000 users in the first 3 months.
Page load times were under 1 second even during peak hours.
The system passed a third-party penetration test with zero critical vulnerabilities.
Key Laravel Features That Fintech Businesses Love
Feature
Why It Matters for Fintech
Blade Templates
Speeds up frontend UI without complex JS
Laravel Sanctum
Easy API token management for mobile apps
Laravel Queue System
Handles transactions, notifications in background
Migration System
Helps keep track of database changes easily
Test Automation Support
Essential for secure and bug-free releases
How to Choose the Right Laravel Development Services
Here are 5 tips to find the best Laravel team for your fintech project:
Check for Security Expertise: Ask how they handle encryption, SSL, and data privacy.
Look for Fintech Experience: Have they built apps in finance, banking, or insurance?
Ask About Performance Tuning: Do they use Redis, CDN, or job queues?
Review Client Testimonials: Look for real business results and successful launches.
Support & Maintenance: Fintech apps need ongoing updates. Make sure they offer it.
FAQs: Laravel Development Services for Fintech
Q1: Can Laravel handle sensitive financial data securely?
Yes. Laravel offers built-in tools for encryption, secure session handling, and protection against OWASP top 10 vulnerabilities. Many fintech platforms successfully use Laravel.
Q2: Is Laravel fast enough for real-time fintech applications?
Absolutely. With caching, queues, and efficient routing, Laravel delivers low-latency responses. For real-time data (like trading apps), Laravel Echo and WebSockets can be used.
Q3: Can Laravel be used for mobile fintech apps?
Yes. Laravel is commonly used as a backend for mobile apps (using Flutter, React Native, or native frameworks). Laravel APIs are easy to connect with mobile frontends.
Final Thoughts
In the fintech world, the margin for error is razor-thin. Security breaches or slow load times can lead to user loss and legal trouble. That’s why choosing the right tech stack and more importantly, the right development team is crucial.
With Laravel, you get a framework that’s powerful, secure, and scalable. By partnering with professional Laravel development services, fintech companies can:
Launch secure and lightning-fast apps
Stay compliant with global standards
Scale features and users effortlessly
Beat the competition in speed and reliability
So, if you're planning to build or upgrade your fintech platform in 2025, now is the perfect time to invest in trusted Laravel development services.
0 notes
Text
Exploring Laravel’s Ecosystem: Top Tools and Packages You Should Use

Laravel has become one of the most popular PHP frameworks due to its elegant syntax, robust features, and a thriving ecosystem. If you're working with Laravel or considering it for your next project, exploring its extensive range of tools and packages is crucial to optimizing your development process. A Laravel Development Company can help you unlock the full potential of Laravel by implementing these tools effectively. Whether you're building a small website or a complex web application, understanding the tools available within Laravel's ecosystem can significantly improve both the development speed and the performance of your project.
The Laravel Ecosystem: A Treasure Trove of Tools
Laravel is not just a framework; it’s an entire ecosystem with various tools and packages that simplify everything from authentication to deployment. Laravel’s ecosystem is known for its simplicity, scalability, and ability to integrate with a variety of technologies. It’s designed to streamline development, making it easier for developers to build web applications quickly and efficiently.
One of the standout features of Laravel is Laravel Forge, a tool that simplifies server management, allowing developers to deploy applications with ease. Forge provides a robust solution for provisioning servers, configuring SSL, and monitoring server health. This tool eliminates the hassle of manual server management, enabling developers to focus on writing clean and efficient code.
Top Laravel Tools and Packages to Boost Your Productivity
Here are some essential tools and packages that you should consider when working with Laravel:
1. Laravel Nova
Nova is a beautifully designed administration panel for Laravel applications. It provides a clean and intuitive interface for managing the data in your application. Nova allows you to create custom dashboards, manage resources, and build complex relationships between different parts of your app.
It is ideal for Laravel developers who want to create powerful and customized admin panels without reinventing the wheel. As a package, Nova offers a flexible and highly configurable UI that can be tailored to fit your business needs.
2. Laravel Echo
For applications that require real-time features like notifications, chat, or activity feeds, Laravel Echo is an essential tool. Echo makes it easy to broadcast events from your application to clients in real time. It integrates seamlessly with WebSockets, so you can push updates to users without requiring them to refresh the page.
If your app demands live updates, whether for a messaging system, live notifications, or any other feature requiring real-time data, Echo is a must-have tool in your Laravel toolkit.
3. Laravel Passport
OAuth2 authentication is a common feature for many modern applications, especially those that require API-based access. Laravel Passport is a full OAuth2 server implementation for Laravel, providing a secure and straightforward way to manage API authentication.
Passport makes it simple to issue access tokens for your API and protect routes with OAuth2 security. It’s an essential package for developers building large-scale applications with API-driven architectures.
4. Laravel Horizon
Managing queues and jobs is a significant part of building scalable applications. Laravel Horizon is a powerful queue manager that provides a beautiful dashboard for monitoring and managing your queues. With Horizon, you can track job throughput, failures, and other crucial metrics that help ensure your queue system runs smoothly.
Horizon is particularly useful for applications that need to handle a high volume of tasks or background processes, such as processing payments or sending emails.
5. Laravel Mix
When it comes to asset compilation, Laravel Mix simplifies front-end workflow. Mix provides a clean API for defining Webpack build steps for your Laravel application, making it easier to manage CSS, JavaScript, and other assets.
With its seamless integration into Laravel, Mix allows you to compile and minify your assets with ease, helping you improve the performance and user experience of your application.
6. Spatie Packages
Spatie is a renowned company within the Laravel community that has created a wide array of useful packages. Some of their most popular packages include Laravel Permission for role-based access control, Laravel Media Library for handling file uploads, and Laravel Activitylog for tracking user activity.
Spatie’s tools are known for their reliability and ease of use, making them excellent choices for developers who want to extend Laravel’s functionality without reinventing the wheel.
7. Laravel Scout
If your application needs full-text search capabilities, Laravel Scout is the go-to solution. Scout provides a simple, driver-based solution for adding full-text search to your models. It works with several popular search engines like Algolia and TNTSearch.
Using Scout, you can easily implement robust search functionality in your Laravel application without having to worry about the complexities of managing search indexes and queries.
Considering Mobile App Development? Don’t Forget the Cost
If you're planning to take your Laravel web application to the mobile platform, integrating a mobile app into your Laravel project is becoming increasingly popular. However, before diving into mobile app development, it's important to consider the mobile app cost calculator to understand the expenses involved.
Building a mobile app can range from a few thousand dollars to hundreds of thousands, depending on the features, platforms (iOS/Android), and the complexity of the app. A mobile app cost calculator can give you a rough estimate of the costs based on your desired features and functionalities, helping you make informed decisions about your project’s budget and scope.
If you’re unsure of how to proceed with your Laravel app and mobile development strategy, it’s always a good idea to consult a Laravel Development Company for expert advice and support.
If you're interested in exploring the benefits of Laravel development services for your business, we encourage you to book an appointment with our team of experts. Book an Appointment
Conclusion
The Laravel ecosystem is rich with tools and packages designed to streamline development, enhance functionality, and improve the overall user experience. From real-time events with Laravel Echo to managing queues with Laravel Horizon, these tools can help you build robust applications more efficiently.
If you’re looking for expert guidance or need help with your next project, consider leveraging Laravel App Development Services to ensure you’re utilizing the full power of the Laravel ecosystem. By working with professionals, you can save time and focus on what matters most—creating outstanding web applications.
0 notes
Text
Expert Guide to Hiring Freelancer Laravel Developers in Ahmedabad for E-commerce Development
In the dynamic world of e-commerce, finding the right talent for development is crucial. Laravel, a popular PHP framework, offers robust solutions for building scalable and secure e-commerce platforms. If you’re based in Ahmedabad and looking to hire freelancer Laravel developers, this guide will provide you with essential insights and steps to ensure you choose the right professionals for your project. We'll explore the hiring process, key considerations, and how partnering with experts like i-Quall can make a significant difference.
When embarking on an e-commerce project, choosing the right framework and developers can significantly impact your success. Laravel stands out for its elegant syntax, extensive features, and strong community support, making it an excellent choice for e-commerce development. Ahmedabad, a city renowned for its thriving tech scene, offers a pool of talented freelancer Laravel developers. However, finding the right freelancer requires careful consideration and a strategic approach. This guide delves into the process of hiring freelancer Laravel developers in Ahmedabad, providing you with actionable steps and tips to ensure your e-commerce project’s success.
Define Your E-commerce Requirements: Before starting your search for freelancer Laravel developers, clearly outline your e-commerce project's requirements. Consider the features you need, such as product management, payment gateways, and user authentication.
Search for Qualified Freelancers: Look for freelancer Laravel developers with a proven track record in e-commerce projects. Platforms like Upwork, Freelancer, and LinkedIn can be useful in finding professionals with the right skills.
Evaluate Portfolios and Experience: Review the portfolios of potential candidates to assess their experience with Laravel and e-commerce projects. Look for developers who have successfully completed similar projects.
Conduct Technical Interviews: To ensure the developers possess the necessary skills, conduct technical interviews. Ask about their experience with Laravel, their approach to problem-solving, and their understanding of e-commerce best practices.
Check References and Reviews: Contact previous clients to get feedback on the freelancer's work ethic, reliability, and overall performance. Positive references can be a good indicator of a developer’s ability to deliver quality results.
Discuss Project Timelines and Budget: Clearly define the project timeline and budget with the freelancer. Ensure that both parties agree on deliverables, milestones, and payment terms to avoid misunderstandings later.
Start with a Small Project: Consider starting with a smaller project or a trial phase to evaluate the freelancer’s capabilities before committing to a larger, long-term engagement.
Understanding the Laravel framework and its capabilities is essential when hiring freelancer developers. Laravel provides a range of tools and features specifically designed for e-commerce, such as:
Eloquent ORM: Facilitates database management with an elegant syntax.
Blade Templating Engine: Allows for clean and reusable templates.
Laravel Echo: Supports real-time event broadcasting, which can enhance user experience.
Laravel Passport: Provides an easy way to implement API authentication.
Familiarity with these features can help you assess whether a freelancer has the necessary expertise to develop a robust e-commerce platform.
Imagine you’re launching an online store for handmade crafts and need a customized e-commerce platform built with Laravel. By hiring a freelancer Laravel developer in Ahmedabad, you find a professional with experience in creating similar platforms. They use Laravel’s built-in features to develop a secure and scalable e-commerce site that integrates smoothly with payment gateways and provides an intuitive user experience. This tailored approach ensures that your project meets your specific needs and stands out in the competitive e-commerce market.
When looking for top-tier freelancer Laravel developers, partnering with a reputable agency like i-Quall can offer additional advantages. i-Quall specializes in providing skilled Laravel developers who are well-versed in e-commerce development. Their expertise ensures that your project is handled professionally, from initial planning to final deployment. By choosing i-Quall, you benefit from their comprehensive understanding of Laravel and their commitment to delivering high-quality solutions tailored to your e-commerce needs.
Conclusion
Hiring freelancer Laravel developers in Ahmedabad for e-commerce development can be a game-changer for your business. By following the outlined steps and leveraging the expertise of professionals like i-Quall Infoweb, you can ensure that your e-commerce platform is built to high standards, offering a seamless experience for your customers. Investing time in finding the right developer will pay off in the long run, helping you create a successful and scalable online presence.
URL : https://www.i-quall.com/ahmedabad/freelancer-laravel-developers-ahmedabad-for-e-commerce-development/
0 notes
Text
Why Use Laravel to Develop Faster Web-Based Apps?
In the fast-evolving world of web development, choosing the right framework is crucial for building efficient, scalable, and high-performance applications. Laravel, a PHP framework, has emerged as a popular choice among developers for creating robust web-based apps quickly. Here’s why Laravel stands out and why you should consider it for your next web development project.

Elegant Syntax and Readability
Laravel is known for its elegant syntax that is easy to understand and write. This readability reduces the learning curve for new developers and enhances productivity for experienced ones. The clean and expressive syntax facilitates easier code maintenance and debugging, which is crucial for developing complex applications swiftly.
MVC Architecture
Laravel follows the Model-View-Controller (MVC) architectural pattern, which separates the business logic, presentation, and data layers. This separation simplifies the development process, making it easier to manage and scale applications. The MVC architecture also enhances performance and ensures that your web apps are both robust and maintainable.
Comprehensive Documentation
One of Laravel’s strong points is its comprehensive and well-structured documentation. Whether you’re a beginner or an advanced developer, Laravel’s documentation provides detailed guides, examples, and API references. This extensive documentation helps in speeding up the development process by providing clear instructions and solutions to common problems.
Built-in Authentication and Authorization
Laravel offers built-in tools for implementing authentication and authorization, saving developers significant time. The framework provides a simple and secure way to manage user authentication, including login, registration, password resets, and email verification. These out-of-the-box features help in quickly setting up secure user access in web applications.
Eloquent ORM
Laravel’s Eloquent ORM (Object-Relational Mapping) makes interacting with databases straightforward and intuitive. Eloquent provides a beautiful and easy-to-use ActiveRecord implementation for working with your database. This allows developers to perform database operations using simple, expressive syntax, reducing the time and effort needed to manage database interactions.
Artisan Command-Line Interface
Laravel comes with Artisan, a powerful command-line interface that provides a range of helpful commands for common tasks during development. From database migrations and seedings to creating boilerplate code, Artisan streamlines the development workflow, enabling developers to focus on building features rather than repetitive tasks.
Blade Templating Engine
Laravel’s Blade templating engine is simple yet powerful, allowing developers to create dynamic and reusable templates with ease. Blade’s lightweight syntax and template inheritance features help in building complex layouts quickly and efficiently. This templating engine enhances productivity by reducing the amount of boilerplate code and promoting code reuse.
Robust Ecosystem and Community Support
Laravel boasts a robust ecosystem with numerous packages and tools that extend its functionality. From Laravel Echo for real-time events to Laravel Passport for API authentication, the ecosystem offers solutions for various development needs. Additionally, Laravel has a vibrant community of developers who contribute to its continuous improvement, provide support, and share knowledge through forums, tutorials, and conferences.
Testing and Debugging Tools
Laravel places a strong emphasis on testing, with built-in support for PHPUnit and various testing methods. This focus on testing ensures that applications are reliable and bug-free. Laravel also offers debugging tools like Laravel Telescope, which provides insights into the application's requests, exceptions, and database queries, making it easier to identify and fix issues promptly.
Scalability and Performance Optimization
Laravel is designed with scalability in mind. Whether you’re building a small web app or a large enterprise solution, Laravel’s modular architecture and performance optimization features ensure that your application can handle increased traffic and complex operations efficiently. Laravel's caching, session management, and queueing system contribute to faster load times and improved application performance.
Conclusion
Laravel is a powerful and versatile framework that accelerates the development of web-based applications. Its elegant syntax, robust features, and supportive community make it an excellent choice for developers looking to build high-performance and scalable applications quickly. By leveraging Laravel’s capabilities, you can streamline your development process, reduce time-to-market, and deliver exceptional web-based apps that meet modern standards.
By using Laravel, you not only enhance your productivity but also ensure that your applications are secure, maintainable, and future-proof. So, if you’re planning to develop a web-based app, consider Laravel for a faster, efficient, and enjoyable development experience.
#web development#perception system#web portal development#software development#cloud consulting#web design#ecommerce development#it services#mobile app development#perception systemarketing
0 notes
Text
Back-End Frameworks: How to Make the Right Choice for Your Web Development
The web development world thrives on powerful tools. Back-end frameworks are some of the most crucial in a developer's arsenal. But with a vast array of options available, choosing the right one can feel overwhelming. Fear not, fellow coder! This guide will equip you to make an informed decision and select the perfect back-end framework for your next web application.
Understand Your Project Requirements
Before diving into the specifics of each framework, it’s essential to clearly define your project requirements. Consider the following questions:
What is the scope of your project? Large-scale enterprise applications have different needs compared to small, simple websites.
What are your performance requirements? High-traffic applications may require a framework known for its performance and scalability.
What is your timeline and budget? Some frameworks may require more development time and resources than others.
Choosing the Right Framework: Key Considerations
Now that you understand the power of back-end frameworks, let's explore the factors to consider when making your choice:
Project Requirements: What kind of web application are you building? A simple blog will have different needs than a complex e-commerce platform. Analyze your project's specific functionalities and choose a framework that can handle them effectively.
Team Expertise: Consider your development team's skills and experience. Opt for a framework that aligns with their knowledge base to ensure a smooth development process. Learning a new framework can be time-consuming, so factor that into your project timeline.
Performance and Scalability: How much traffic do you anticipate your application receiving? Choose a framework known for its performance and ability to scale efficiently as your user base grows.
Security: Web security is paramount. Select a framework with robust security features built-in to safeguard your application from potential threats.
Community and Support: A strong community and ample support resources can be invaluable during Web development. Look for a framework with a large and active community where you can find solutions and ask questions.
2. Consider Language and Ecosystem
The programming language you are most comfortable with or that your team specializes in can significantly influence your choice of framework. Here are some popular languages and their associated frameworks:
JavaScript/TypeScript: Node.js with Express.js, NestJS
Python: Django, Flask
Ruby: Ruby on Rails
Java: Spring Boot
PHP: Laravel, Symfony
Go: Gin, Echo
Each language and framework has its own ecosystem, including libraries, tools, and community support, which can significantly impact development productivity.
Popular Back-End Frameworks: A Glimpse
The world of back-end frameworks is vast, but here are a few of the most popular options, each with its own strengths:
Django (Python): Known for its rapid development features, clean syntax, and extensive community support, Django excels at building complex web applications.
Laravel (PHP): A powerful framework offering a secure foundation for web applications. Laravel is particularly user-friendly for developers with a background in PHP.
Ruby on Rails (Ruby): This convention-over-configuration framework streamlines development and is renowned for its developer happiness focus.
Spring (Java): A mature and versatile framework well-suited for large-scale enterprise applications. Spring offers a robust ecosystem of tools and libraries.
Express.js (JavaScript): A lightweight and flexible framework for building web applications and APIs with Node.js. Express.js allows for a high degree of customization.
Selecting the right back-end framework for your web development project involves balancing several factors, including project requirements, language preferences, performance needs, security, community support, flexibility, and development speed.
Choose Umano Logic for Your Web Development Success
At Umano Logic, we help businesses make smart technology choices. As a top web development company in Canada, our expert team can guide you in selecting and implementing the best back-end framework for your project. Reach out to us today to learn how we can help you achieve your goals and propel your business forward.
Contact us today !!
#Web development company in Edmonton#Dedicated web developers in CA#Web app development Service Canada#software development Canada
0 notes
Text
🚀 Prevent Buffer Overflow in Symfony: Best Practices & Code Examples
Buffer overflow vulnerabilities can have devastating consequences on your web applications — allowing attackers to execute arbitrary code, crash your server, or even take over your system. Symfony, a robust PHP framework, provides developers the tools to write secure applications, but it’s still your responsibility to implement proper safeguards.

In this guide, you’ll learn how to prevent buffer overflow in Symfony, with secure coding examples, links to our website vulnerability scanner online free, and tips to keep your apps safe.
🔍 Why Buffer Overflows Happen
Buffer overflows occur when an application writes more data into a buffer (memory area) than it was designed to hold. In PHP (and thus Symfony), they’re less common than in C, but they can still manifest through:
Unvalidated input written into files or memory
Large POST payloads overwhelming your scripts
Poorly handled binary data or file uploads
A proactive developer can prevent them by implementing input validation, limits, and proper handling of user data.
🧰 Use Our Free Website Security Checker Tool
Before diving deeper, check if your website is already vulnerable. We built a free, fast, and easy-to-use Website Vulnerability Scanner:

Screenshot of the free tools webpage where you can access security assessment tools.
We even provide a downloadable vulnerability assessment report to check Website Vulnerability when you scan your site:
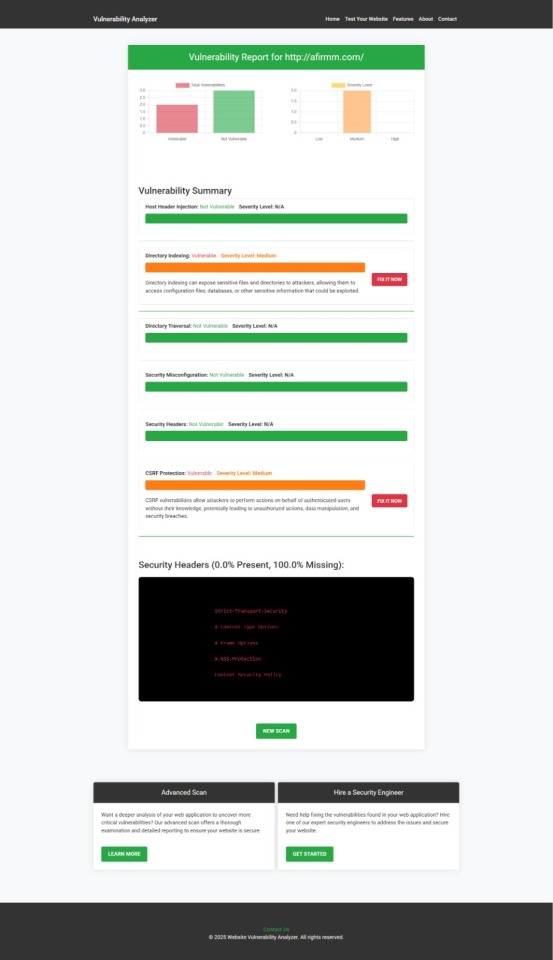
An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities.
Don’t launch another feature before checking your site!
🛡️ How to Prevent Buffer Overflows in Symfony
1️⃣ Limit User Input Size
Symfony provides a robust validation component. Always validate and constrain incoming data. For example:
use Symfony\Component\Validator\Constraints as Assert; class UserInput { /** * @Assert\Length( * max = 255, * maxMessage = "Input too long, maximum is {{ limit }} characters" * ) */ private $comment; }
Here, any user comment longer than 255 characters will fail validation.
2️⃣ Use ini_set() to Limit POST and Upload Sizes
In your Symfony app (often via config/packages/framework.yaml), ensure PHP limits large payloads:
framework: http_method_override: false session: handler_id: null parameters: upload_max_filesize: 2M post_max_size: 8M
You can also enforce limits in your .htaccess or php.ini:
post_max_size = 8M upload_max_filesize = 2M
3️⃣ Use Streams for Large Files
When handling file uploads, avoid loading entire files into memory. Use Symfony’s StreamedResponse:
use Symfony\Component\HttpFoundation\StreamedResponse; $response = new StreamedResponse(function () { $handle = fopen('/path/to/largefile', 'rb'); while (!feof($handle)) { echo fread($handle, 1024); } fclose($handle); }); $response->send();
This prevents memory exhaustion (and possible overflow scenarios).
4️⃣ Filter Binary Data
If your app processes binary payloads (e.g., images, files), validate file type and size explicitly. Symfony’s File constraint helps:
use Symfony\Component\Validator\Constraints\File; class UploadModel { /** * @Assert\File( * maxSize = "2M", * mimeTypes = {"image/png", "image/jpeg"} * ) */ public $uploadedFile; }
💡 Learn More on Our Blog
For more security tips and coding best practices, visit our blog: 📖 https://www.pentesttesting.com/blog/
We regularly publish tutorials and in-depth guides for Symfony, Laravel, React, and beyond.
🔗 Related Services We Offer
✅ Web Application Penetration Testing
If you want professionals to test your Symfony application, check our dedicated service: 👉 https://www.pentesttesting.com/web-app-penetration-testing-services/
We’ll identify vulnerabilities and help you patch them fast.
🤝 Offer Cybersecurity Services to Your Clients
Are you an agency? We offer white-label cybersecurity services: 👉 https://www.pentesttesting.com/offer-cybersecurity-service-to-your-client/
Strengthen your portfolio and keep your clients safe under your brand.
📬 Stay Updated
Get the latest insights on application security by subscribing to our LinkedIn newsletter: 🔗 Subscribe on LinkedIn
👏 Final Thoughts
Buffer overflows in Symfony apps may not be as obvious as in low-level languages, but they still pose a risk when handling untrusted, oversized, or improperly validated input. By validating, limiting, and streaming data properly — and running regular security checks — you can keep your application robust and secure.
Try our free vulnerability scanner today: 👉 https://free.pentesttesting.com/
Have questions? Drop them in the comments or DM me!
1 note
·
View note
Text
Core PHP vs Laravel – Which to Choose?
Choosing between Core PHP and Laravel for developing web applications is a critical decision that developers and project managers face. This blog aims to explore the unique aspects of each, current trends in 2024, and how new technologies influence the choice between the two. We'll dive into the differences between core php and Laravel ecosystems, performance, ease of use, community support, and how they align with the latest technological advancements.
Introduction to Core PHP and Laravel
Core PHP refers to PHP in its raw form, without any additional libraries or frameworks. It gives developers full control over the code they write, making it a powerful option for creating web applications from scratch. On the other hand, Laravel is a PHP framework that provides a structured way of developing applications. It comes with a set of tools and libraries designed to simplify common tasks, such as routing, sessions, caching, and authentication, thereby speeding up the development process for any business looking to hire php developers.
Unique Aspects of Core PHP and Laravel
Core PHP:
Flexibility and Control: Offers complete freedom to write custom functions and logic tailored to specific project requirements.
Performance: Without the overhead of a framework, Core PHP can perform faster in scenarios where the codebase is optimized and well-written.
Learning Curve: Learning Core PHP is essential for understanding the fundamentals of web development, making it a valuable skill for developers.
Laravel:
Ecosystem and Tools: Laravel boasts an extensive ecosystem, including Laravel Vapor for serverless deployment, Laravel Nova for administration panels, and Laravel Echo for real-time events.
MVC Architecture: Promotes the use of Model-View-Controller architecture, which helps in organizing code better and makes it more maintainable.
Blade Templating Engine: Laravel’s Blade templating engine simplifies tasks like data formatting and layout management without slowing down application performance.
Trends in 2024
Headless and Microservices Architectures: There's a growing trend towards using headless CMSes and microservices architectures. Laravel is particularly well-suited for this trend due to its ability to act as a backend service communicating through APIs.
Serverless Computing: The rise of serverless computing has made frameworks like Laravel more attractive due to their compatibility with cloud functions and scalability.
AI and Machine Learning Integration: Both Core PHP and Laravel are seeing libraries and tools that facilitate the integration of AI and machine learning functionalities into web applications.
New Technologies Influencing PHP Development
Containerization: Docker and Kubernetes are becoming standard in deployment workflows. Laravel Sail provides a simple command-line interface for managing Docker containers, making Laravel applications easier to deploy and scale.
WebSockets for Real-Time Apps: Technologies like Laravel Echo allow developers to easily implement real-time features in their applications, such as live chats and notifications.
API-First Development: The need for mobile and single-page applications has pushed the adoption of API-first development. Laravel excels with its Lumen micro-framework for creating lightning-fast APIs.
Performance and Scalability
Performance and scalability are crucial factors in choosing between Core PHP and Laravel. While Core PHP may offer raw performance benefits, Laravel's ecosystem contains tools and practices, such as caching and queue management, that help in achieving high scalability and performance for larger applications.
Community Support and Resources
Laravel enjoys robust community support, with a wealth of tutorials, forums, and third-party packages available. Core PHP, being the foundation, also has a vast amount of documentation and community forums. The choice might depend on the type of support and resources a developer is comfortable working with.
PHP 8,3 vs Laravel 10
Comparing the latest versions of PHP (8.3) and Laravel (10) reveals distinct advancements tailored to their respective ecosystems. PHP 8.3 brings enhancements such as Typed Class Constants, dynamic class constant and Enum member fetch support, along with the introduction of new functions like json_validate() and mb_str_pad(), aimed at improving the language's robustness and developer experience. The addition of the #[\Override] attribute further emphasizes PHP's commitment to cleaner code and better inheritance management. On the other side, Laravel 10 updates its arsenal with support for its latest version across various official packages including Breeze, Cashier Stripe, Dusk, Horizon, and others, ensuring a seamless integration and enhanced developer toolkit. These updates focus on enriching Laravel's ecosystem, providing more out-of-the-box features, and improving the development process for web applications. While PHP 8.3 focuses on language level improvements and new functionalities for a broader range of PHP applications, Laravel 10 hones in on refining the framework's capabilities and ecosystem, making web development more efficient and scalable.
Conclusion
The decision between Core PHP and Laravel comes down to the project's specific requirements, the top PHP development companies and their team's expertise, and the desired scalability and performance characteristics. For projects that require rapid development with a structured approach, Laravel stands out with its comprehensive ecosystem and tools. Core PHP remains unbeatable for projects requiring custom solutions with minimal overhead.
In 2024, the trends towards serverless computing, microservices, and API-first development are shaping the PHP development services landscape. Laravel's alignment with these trends makes it a compelling choice for modern web applications. However, understanding Core PHP remains fundamental for any PHP developer, offering unparalleled flexibility and control over web development projects.
Embracing new technologies and staying abreast of trends is crucial, whether choosing Core PHP for its directness and speed or Laravel for its rich features and scalability. The ultimate goal is to deliver efficient, maintainable, and scalable web applications that meet the evolving needs of users and businesses alike.
0 notes
Text
Building Chat Applications with Laravel Echo: A Guide to Real-Time Interactivity
In the ever-evolving landscape of web development, the demand for real-time communication features has surged, giving rise to the need for robust and efficient solutions. Laravel Echo, a powerful tool integrated into the Laravel framework, emerges as a key player in facilitating real-time interactions, particularly in the realm of chat applications. A leading web development company in Kolkata provides professional services on building chat applications with Laravel Echo.
In this comprehensive guide, we'll explore the fundamentals of Laravel Echo, its integration with Laravel Broadcasting, and how developers can leverage this dynamic duo to build engaging and responsive chat applications.
Understanding Laravel Echo and Broadcasting
Laravel Echo: The Voice of Real-Time Web Applications
Introduction to Laravel Echo:
Laravel Echo is a JavaScript library that simplifies the implementation of real-time features in web applications. It serves as the bridge between your Laravel back end and the front end, allowing seamless communication and data synchronization.
Features and Benefits:
Event Listening: Laravel Echo enables the listening for specific events broadcasted by the server, such as new messages or user updates.
Broadcasting Channels: It provides a straightforward way to subscribe to and broadcast events on channels, facilitating organized communication.
WebSocket Integration: Laravel Echo seamlessly integrates with WebSocket protocols, ensuring low-latency, real-time updates.
Laravel Broadcasting: Broadcasting Events to Multiple Clients
Introduction to Laravel Broadcasting:
Laravel Broadcasting is the Laravel framework's built-in solution for real-time event broadcasting. It utilizes various broadcasting drivers, including Pusher, Redis, and more, to broadcast events to multiple clients simultaneously.
Event Broadcasting Workflow:
Event Creation: Define events that should trigger real-time communication, such as a new chat message or user activity.
Event Broadcasting: Laravel Broadcasting broadcasts these events to subscribed clients through a selected broadcasting driver.
Client-Side Listening: Laravel Echo on the client side listens for these events, triggering actions based on the received data.
Building a Real-Time Chat Application with Laravel Echo
Unveiling the synergy of Laravel Echo and Laravel Broadcasting, this guide empowers developers to craft engaging chat experiences that transcend traditional communication boundaries.
From setting up real-time events to implementing presence channels and optimizing the chat interface, join us in exploring the intricacies of Laravel Echo as we bring the immediacy of real-time interactions to the forefront of web development.
Setting Up Laravel Broadcasting:
Installation and Configuration
Install Laravel Echo and a broadcasting driver of your choice (e.g., Pusher, Redis).
Configure Laravel to use the chosen broadcasting driver in the broadcasting.php configuration file.
Creating Chat Events
Event Creation:
Generate an event class for chat messages using the php artisan make:event command.
Define the necessary properties within the event class, such as the sender, message content, and timestamp.
Broadcasting the Event:
Implement the ShouldBroadcast interface in the event class to indicate that it should be broadcast.
Laravel automatically handles broadcasting the event when triggered.
Broadcasting Chat Messages
Server-Side Broadcasting:
When a new chat message is created, broadcast the chat event using Laravel's event system.
Laravel Broadcasting takes care of pushing the event data to the selected broadcasting driver.
Client-Side Listening:
On the client side, use Laravel Echo to listen for the chat event.
Define actions to take when the event is received, such as updating the chat interface with the new message.
Implementing Presence Channels
Introduction to Presence Channels:
Presence channels in Laravel allow you to track the presence of users in real-time.
Useful for displaying online users, typing indicators, or other presence-related features in a chat application.
Setting Up Presence Channels:
Define presence channels in Laravel Echo and configure the back end to authorize users to join these channels.
Update the front end to display real-time presence information.
Optimizing and Enhancing the Chat Experience
Discover how to seamlessly integrate real-time features, implement presence channels, and employ innovative strategies. It helps developers create a dynamic and immersive chat environment that goes beyond conventional expectations, providing users with an enriched and interactive communication experience.
Authentication and Authorization
User Authentication:
Authenticate users within your Laravel application.
Laravel Echo automatically binds the authenticated user's ID to the broadcasted events.
Authorization for Channels:
Implement channel authorization to ensure that users can only access channels they are authorized to join.
Laravel Echo provides mechanisms to handle this authorization seamlessly.
Implementing Typing Indicators
Real-Time Typing Notifications:
Extend the chat application by implementing real-time typing indicators.
Broadcast typing events when users start or stop typing, updating the interface for all connected users.
Storing and Retrieving Chat History
Database Integration:
Store chat messages in the database to ensure persistence and enable retrieval of chat history.
Implement methods to fetch and display chat history on the client side.
Security Considerations
Secure Broadcasting:
Implement secure practices, such as using HTTPS and securing broadcasting channels, to ensure the confidentiality and integrity of transmitted data.
Data Validation:
Implement robust validation for incoming chat messages to prevent security vulnerabilities.
Conclusion: Elevating Interactivity with Laravel Echo
In conclusion, Laravel Echo emerges as a game-changer for developers seeking to enhance their web applications with real-time interactivity. Whether you're building a chat application, collaborative tools, or live update features, Laravel Echo, in tandem with Laravel Broadcasting, provides a seamless and efficient solution. By following the outlined steps and considerations, developers can empower their applications with the dynamic responsiveness users crave in today's digital landscape. A leading web development company in Kolkata also helps build chat applications with Laravel Echo. Laravel Echo opens the door to a new era of user engagement, where real-time communication is not just a feature but a cornerstone of modern web development.
0 notes
Text
Laravel Beyond Basics: Unveiling Advanced Techniques for Unparalleled App Performance
Laravel Beyond Basics: Unveiling Advanced Techniques for Unparalleled App Performance
In the rapidly evolving landscape of web application development, staying ahead of the curve is crucial for any Laravel app development company. As the demand for sophisticated and high-performing applications grows, mastering advanced techniques in Laravel becomes a necessity rather than a luxury. In this comprehensive guide, we, at Wama Technology, a leading Laravel app development company in the USA, delve into the realm of advanced Laravel techniques that promise to elevate your app's performance to new heights.
Introduction: Enhancing App Performance with Advanced Laravel Techniques
Laravel, renowned for its elegant syntax and developer-friendly features, has established itself as a go-to framework for building robust web applications. While its basics can yield impressive results, the true potential of Laravel lies in its advanced capabilities that can significantly boost app performance.
Optimizing Database Queries for Lightning-Fast Results
At Wama Technology, our Laravel app developers understand the critical role that database queries play in determining an application's speed and efficiency. To achieve optimal performance, we emphasize advanced database optimization techniques. Utilizing Laravel's Eloquent ORM, we not only simplify database interactions but also fine-tune queries for faster execution. By employing features like query caching, database indexing, and eager loading, we ensure that your application retrieves and presents data with lightning-fast speed.
Caching Strategies for Seamless User Experiences
In the realm of Laravel application development, caching emerges as a powerful tool for reducing response times and enhancing user experiences. Our Laravel app development team implements advanced caching strategies such as opcode caching, query caching, and content caching. Leveraging Laravel's built-in caching mechanisms and integrating tools like Redis, we help your app deliver dynamic content with the responsiveness of static pages, ensuring that users stay engaged and satisfied.
Microservices Architecture for Scalability and Performance
Modern web applications demand scalability to accommodate growing user bases and changing requirements. Our Laravel app development company in the USA specializes in implementing a microservices architecture using Laravel Lumen—a lightweight and efficient micro-framework by Laravel. By breaking down your application into smaller, independent services, we ensure better resource utilization, improved fault tolerance, and enhanced overall performance.
Harnessing Queues for Asynchronous Processing
Unpredictable processing times, such as sending emails, processing payments, or generating reports, can hinder your application's responsiveness. At Wama Technology, we harness Laravel's advanced queueing system to delegate time-consuming tasks to background processes. By ensuring asynchronous execution, we free up resources for more critical tasks, resulting in a smoother user experience and reduced latency.
Real-time Interactions with WebSockets
The demand for real-time user interactions has never been higher. Whether it's live chats, notifications, or collaborative tools, users expect seamless real-time experiences. To meet these expectations, our Laravel app developers employ WebSockets—a cutting-edge technology that facilitates two-way communication between clients and servers. By integrating packages like Laravel Echo and Pusher, we empower your application to deliver real-time updates and notifications, enriching user engagement and satisfaction.
Conclusion: Elevate Your Laravel App Performance with Wama Technology
In a competitive digital landscape, delivering high-performance web applications is non-negotiable. As a premier Laravel app development company in the USA, Wama Technology is committed to pushing the boundaries of what Laravel can achieve. By harnessing advanced techniques like optimized database queries, caching strategies, microservices architecture, queues, and real-time interactions, we ensure that your Laravel app not only meets but exceeds user expectations.
If you're ready to take your Laravel app application development to the next level, partner with Wama Technology today. Our seasoned Laravel app developers are equipped with the knowledge, experience, and dedication to transform your vision into a high-performing reality. Contact us to explore the limitless possibilities of Laravel beyond the basics.
0 notes
Photo

Herramientas para Laravel que debes conocer En este vídeo comparto contigo todas de las herramientas que utilizo en mis proyectos Laravel y te explico para qué sirve cada una de ellas y cómo pueden ayudarte en tu día a día. 🔥 Accede a la entrada de blog en Cursosdesarrolloweb 🔥 source
#herramientas laravel#Laravel#laravel 5#laravel 6#laravel 7#laravel 8#laravel alpinejs#laravel broadcasting#laravel cashier#laravel dusk#laravel echo#laravel envoy#laravel forge#laravel from scratch#laravel hyn#laravel inertia#laravel jetstream#laravel livewire#laravel passport#Laravel Sanctum#laravel socialite#laravel tenancy#laravel valet#laravel vue#php
0 notes
Text
The Role of Laravel in Modern Web Development: Trends to Watch in 2025

In the rapidly evolving landscape of web development, Laravel continues to stand out as one of the most powerful and popular frameworks available to developers. As Laravel development companies around the globe refine their strategies and techniques, the framework's adaptability and robust features make it a preferred choice for building complex, scalable, and high-performance web applications. In 2025, Laravel is expected to remain at the forefront of web development trends, offering advanced features and capabilities that cater to the growing needs of developers, businesses, and end-users alike. This article explores how Laravel is shaping modern web development, highlighting key trends and features that are likely to dominate in 2025.
1. Increased Focus on Developer Productivity
One of the key reasons Laravel remains a popular choice among developers is its focus on productivity. With tools like Laravel Forge, Laravel Envoyer, and Laravel Nova, developers can significantly streamline deployment, configuration, and maintenance tasks. As Laravel development companies continue to emphasize ease of use, the framework is expected to improve even further with updates that automate more complex tasks, leaving developers to focus on building and scaling their applications.
For instance, Laravel’s Blade templating engine provides developers with a simple yet powerful way to manage views and layouts. The expressive syntax allows for rapid development while maintaining clean, readable code. In addition, the framework’s built-in features such as authentication, routing, and sessions allow developers to avoid reinventing the wheel, leading to faster development cycles. In 2025, Laravel will likely push the envelope on these productivity-enhancing tools, making it even more attractive for developers seeking to maximize efficiency.
2. Enhanced Ecosystem and Integration with Third-Party Tools
Laravel’s ecosystem is one of the most extensive in the world of web development. From Laravel Horizon for managing queues to Laravel Echo for real-time events, Laravel provides a comprehensive suite of tools to enhance its core framework. In the coming years, expect to see even more integrations and expansions of the Laravel ecosystem, providing developers with everything they need to build feature-rich applications quickly.
One of the prominent trends in 2025 will be the increased focus on seamless integrations with other technologies, such as cloud services and third-party APIs. As the demand for hybrid applications grows, Laravel’s flexibility in integrating with various services will allow it to stay competitive and relevant. With advanced integration capabilities, developers can easily embed services like Stripe for payments, Twilio for messaging, and other essential tools, ensuring that their applications are both functional and scalable.
3. Improved Mobile App Integration and API Development
In 2025, the integration of Laravel with mobile apps will be more important than ever. Laravel’s robust API capabilities, especially when combined with RESTful APIs and GraphQL, make it an ideal backend solution for mobile applications. As mobile-first design continues to dominate, developers will need more efficient and streamlined methods of connecting web applications with mobile apps. Laravel’s ability to serve as a backend for mobile applications is expected to be a critical trend, offering flexibility and scalability for both startups and large enterprises.
With mobile apps becoming increasingly crucial for businesses, the demand for Laravel development services that cater to mobile integration will grow substantially. This is where frameworks like Laravel’s Passport, which simplifies API authentication, will play a key role. Developers will continue to leverage these powerful tools to build smooth and responsive mobile apps with Laravel at the backend.
4. Serverless and Cloud-Native Architectures
Cloud computing has been on the rise for years, but 2025 will see an even stronger shift toward serverless and cloud-native architectures. Laravel’s ability to integrate seamlessly with popular cloud platforms like AWS and Google Cloud makes it a top choice for companies looking to build cloud-native applications. Laravel’s Forge and Envoyer tools already provide powerful tools for server management, and as the demand for serverless architectures increases, Laravel is expected to adapt to these changes.
Serverless computing allows developers to focus more on code and less on infrastructure, which is especially useful for Laravel developers. For instance, Laravel developers will be able to deploy apps without worrying about maintaining servers or scaling resources manually. In 2025, this trend will continue to gain traction as businesses move to serverless models for efficiency, cost reduction, and scalability.
5. Increased Use of AI and Automation
As AI and machine learning technologies continue to make waves in various industries, Laravel developers are beginning to embrace these technologies to automate certain processes within web applications. From chatbots and intelligent recommendation engines to predictive analytics, AI-powered tools are poised to revolutionize the way Laravel is used for web development.
Laravel developers will increasingly integrate AI tools like TensorFlow or OpenAI into their projects. Additionally, the automation of repetitive tasks, such as data entry or user verification, will become a common practice. This integration will not only improve the user experience but also drive operational efficiency, allowing businesses to reduce costs and improve service delivery. Laravel’s flexible architecture will enable developers to integrate these tools into their web applications smoothly.
6. Mobile App Cost Calculator Integration
As businesses continue to explore mobile app development, they increasingly look for tools that can help them estimate the cost of building a mobile app. In 2025, one trend that will gain traction is the use of mobile app cost calculators in Laravel applications. These calculators will allow potential clients or businesses to estimate the cost of developing a mobile app based on various parameters like features, design, platform, and complexity.
By integrating these calculators into Laravel applications, businesses can provide their clients with instant, transparent pricing, improving the overall customer experience. This trend is likely to grow as businesses strive to provide more user-friendly and efficient solutions for app development.
7. Improved Security and Data Privacy
Security is always a top priority for developers, and Laravel has always offered a robust set of features for protecting web applications. In 2025, developers will continue to see improvements in Laravel’s security features, especially as concerns over data privacy grow globally. With advancements in encryption techniques, two-factor authentication, and protection against common vulnerabilities (such as SQL injection and cross-site scripting), Laravel will remain a secure choice for building applications that handle sensitive data.
As businesses continue to adapt to new regulations, such as GDPR and CCPA, Laravel’s ability to incorporate compliance-focused features will make it an essential framework for companies operating in regulated industries.
Conclusion
As we look to 2025, it is clear that Laravel will remain a cornerstone in modern web development, driving innovation and meeting the growing needs of businesses and developers. From enhanced productivity features and AI integration to serverless architectures and mobile app integration, Laravel will continue to evolve and provide powerful tools for web developers. As businesses seek to leverage the best of Laravel’s offerings, turning to Laravel development services will be essential in building scalable, secure, and feature-rich applications that cater to the needs of today’s users.
0 notes
Link
1 note
·
View note