#reactdom
Explore tagged Tumblr posts
Text
Integrating Redux Toolkit into Your React TypeScript App: A Comprehensive Guide
It is essential to keep up with the latest tools and technologies in the field of front-end web development. One such tool that has gained popularity among developers is Redux Toolkit. Redux Toolkit is a package that simplifies the process of managing state in React applications, especially when using TypeScript.
In this blog, we'll guide you through the process of integrating the Redux Toolkit into your React TypeScript app, ensuring a smoother and more efficient development experience.
What is Redux Toolkit?
Redux Toolkit is a package that provides a set of tools and best practices for managing state in Redux applications. It includes utilities such as configureStore, which simplifies the setup of a Redux store, as well as createSlice, which allows you to define Redux slices with less boilerplate code. Redux Toolkit also includes middleware such as redux-thunk, which enables you to write asynchronous logic in your Redux reducers.
Benefits for Developers and Users:
Simplified State Management:
Redux Toolkit simplifies state management, ensuring a more predictable application state for a smoother user experience.
Improved Code Organization:
Encourages a structured approach to code organization, enhancing readability and maintainability.
Enhanced Debugging:
Includes Redux DevTools for real-time inspection and debugging of state changes.
Streamlined Asynchronous Actions:
Simplifies handling of asynchronous actions like API calls, improving performance.
Scalability:
Designed to scale with the application's complexity, maintaining performance and maintainability.
Type Safety:
Provides TypeScript integration for type safety, reducing runtime errors, and improving code quality.
Step 1: Installing Redux Toolkit
The first step in integrating the Redux Toolkit into your React TypeScript app is to install the package. You can use npm or yarn for this:
npm install @reduxjs/toolkit
or
yarn add @reduxjs/toolkit
Step 2: Setting Up the Redux Store
Next, you'll need to set up the Redux store in your application. Create a new file called store.ts and define your Redux store using the ‘configureStore’ function from Redux Toolkit:
import { configureStore } from '@reduxjs/toolkit';
import rootReducer from './reducers';
const store = configureStore({
reducer: rootReducer,
});
export default store;
Step 3: Creating Redux Slices
Redux Toolkit allows you to define Redux slices using the createSlice function. A slice is a collection of Redux reducers and actions that are related to a specific feature or part of your application. Here's an example of how you can create a slice for managing a user's authentication state:
import { createSlice } from '@reduxjs/toolkit';
const authSlice = createSlice({
name: 'auth',
initialState: {
isAuthenticated: false,
user: null,
},
reducers: {
login(state, action) {
state.isAuthenticated = true;
state.user = action.payload;
},
logout(state) {
state.isAuthenticated = false;
state.user = null;
},
},
});
export const { login, logout } = authSlice.actions;
export default authSlice.reducer;
Step 4: Connecting Redux to Your React Components
Finally, you'll need to connect your Redux store to your React components using the Provider component from the react-redux package. Use the Provider component to wrap your root component and pass your Redux store as a prop:
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import store from './store';
import App from './App';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
Integrating the Redux Toolkit into your React TypeScript app can help you manage state more effectively and improve the overall development experience. By following the steps outlined in this guide, you'll be able to seamlessly integrate Redux Toolkit into your app and take advantage of its powerful features. Remember to stay updated with the latest developments in front-end web development, as new tools and technologies are constantly emerging.
Ready to elevate your front-end services? For professional front-end services that will boost your app, get in touch with a front-end web development company right now. https://www.pravaahconsulting.com/front-end-development
0 notes
Text
How to Redirect URLs in ReactJS
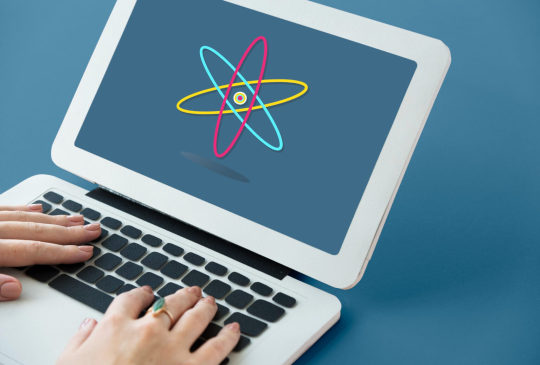
Redirecting URLs is a common task in web development, and ReactJS provides several methods to achieve this. In this blog post, we will explore how to redirect URLs in a React application and provide a practical example to illustrate each method.
Method 1: Using React Router
React Router is a popular library for handling routing in React applications. It offers a convenient way to navigate and redirect URLs. Here’s how you can use it:
Step 1: Install React Router
If you haven’t already, install React Router in your project:
npm install react-router-dom
Step 2: Import and Set Up BrowserRouter
In your index.js or App.js, import BrowserRouter and wrap your entire application with it:
import React from 'react';
import ReactDOM from 'react-dom';
import { BrowserRouter } from 'react-router-dom';
import App from './App';
ReactDOM.render(
<BrowserRouter>
<App />
</BrowserRouter>,
document.getElementById('root')
);
Step 3: Redirecting with <Redirect>
In your React component, you can use the <Redirect> component from React Router to redirect to a new URL:
import React from 'react';
import { Redirect } from 'react-router-dom';
function MyComponent() {
// Redirect to '/new-url' when a certain condition is met
if (someCondition) {
return <Redirect to="/new-url" />;
}
return (
// Your component content
);
}
export default MyComponent;
Method 2: Using window.location
If you need to perform a simple URL redirect without the need for React Router, you can use the window.location object:
import React from 'react';
function MyComponent() {
// Redirect to '/new-url'
if (someCondition) {
window.location.href = '/new-url';
}
return (
// Your component content
);
}
export default MyComponent;
Practical Example:
Let’s create a practical example using React Router for URL redirection:
import React from 'react';
import { BrowserRouter, Route, Redirect, Switch } from 'react-router-dom';
function App() {
return (
<BrowserRouter>
<Switch>
<Route exact path="/" render={() => <Redirect to="/home" />} />
<Route path="/home" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
<Route path="*" component={NotFound} />
</Switch>
</BrowserRouter>
);
}
function Home() {
return <h1>Welcome to the Home Page!</h1>;
}
function About() {
return <h1>Learn more about us on the About Page.</h1>;
}
function Contact() {
return <h1>Contact us on the Contact Page.</h1>;
}
function NotFound() {
return <h1>404 - Page not found</h1>;
}
export default App;
In this example, we use React Router to handle different routes, and we set up a redirect from the root URL (/) to the /home URL.
Conclusion:
Redirecting URLs in ReactJS can be achieved using various methods. React Router provides a powerful and flexible way to handle routing and redirection within your React applications. Whether you prefer using React Router or simple JavaScript for redirection, these methods will help you efficiently navigate and redirect users to different parts of your application.
If you have any questions or need further assistance, please don’t hesitate to contact us for more details. We’re here to support your React development journey.
Follow The React Company for more details.
0 notes
Text
Getting Started with React: Building Your First React Application
React, frequently appertained to as React.js or ReactJS, is an open-source JavaScript library created by Facebook for structure stoner interfaces. It's an important tool that has revolutionized the way web operations are developed. In this preface, we'll explore what React is, why it's so popular, the abecedarian generalities behind this library, who uses it, and when it was released.
The use of a virtual DOM in React enhances rendering, performing briskly and more effectively performance in comparison to traditional DOM manipulation ways. Its concentration on performance and reusability has made Reply a popular choice among inventors. In this companion, we'll go through the process of setting up an introductory React design and creating your first React element.
In the world of web development, React has had a profound impact, leading to the development of colourful libraries and tools that round and enhance its capabilities. Reply is used in combination with other technologies similar as React Router for routing, Redux for state operation, and colourful figure tools for creating important and interactive web operations. The reply is also frequently used to produce applicable UI factors, and it follows a declarative and element-grounded approach. One of the crucial features of React is its capability to efficiently modernize and render stoner interfaces, thanks to its virtual DOM (Document Object Model) medium, which minimizes direct commerce with the factual DOM and enhances performance.
Prerequisites
Before we start, make sure that you have the following tools installed in the system:
Node.js: React requires Node.js, a JavaScript runtime, to work. If it’s not available you can download from the sanctioned website.
npm (Node Package Manager): It comes bundled with Node.js, so there's no need to install it separately.
Setting up Your React Project
Let's start by creating a new React project. React provides a tool called "Create React App" that simplifies the setup process.
npx create-react-app my-react-app
Replace "my-react-app" with the desired name for the project. This command will create a new directory with the project's structure and install all the necessary dependencies.
Running Your React Application
After the project is created, then navigate to the project folder as follows:
cd my-react-app
To start the React application, run the command as follows:
npm start
This command will start the development server, and your React application will be available at ‘http://localhost:3000’ in your web browser.
Creating Your First React Component
Now your React project is up and running, let's create a simple React component. Open the src directory in your project folder and locate the App.js file. This is the default component that gets displayed in your application.
Let's modify the App.js file to create a basic component:
jsx
import React from 'react';
function App() {
return (
<div>
<h1>Welcome to My First React App</h1>
<p>This is a simple React component.</p>
<p>We will see how to create the React Application</p>
</div>
);
}
export default App;
In this example, we have created a functional component called App. It returns JSX (JavaScript XML), which defines the structure and content of the component.
Displaying Your Component
To display your new component, open the src/index.js file in your project folder. Modify it to look like this:
jsx
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
Now, save your changes, and you'll see your component being rendered in the browser.
You've successfully set up a React project and created your first React component. You can continue building your application by adding more components. React component-based architecture provides a flexible and efficient way to create complex user interfaces.
Conclusion
In this article, we have covered the basics of getting started with React. You've set up a new React project, created a simple component, and displayed it in a web application. Now you're ready to explore more advanced concepts and build interactive and dynamic user interfaces with React.
Credits- Komal Waghmare
MetricsViews Pvt. Ltd.
MetricsViews specializes in building a solid DevOps strategy with cloud-native including AWS, GCP, Azure, Salesforce, and many more. We excel in microservice adoption, CI/CD, Orchestration, and Provisioning of Infrastructure - with Smart DevOps tools like Terraform, and CloudFormation on the cloud.
0 notes
Text
¿Cómo crear un app en React js?
Aquí está una explicación más detallada para crear una aplicación React simple desde cero:
1. Crear una carpeta de proyecto y abrir una terminal en esa carpeta.
2. Ejecutar `npm init -y` para inicializar un proyecto de Node. Esto creará un archivo package.json con la configuración básica.
3. Instalar React ejecutando `npm install react react-dom`. Esto instalará React y ReactDOM como dependencias del proyecto.
4. Crear un archivo index.html con un div root para renderizar la aplicación React:
```html <!DOCTYPE html> <html> <head> <title>My App</title> </head> <body> <div id="root"></div> </body> </html> ```
5. Crear un componente App.js que contendrá la UI de la aplicación:
```jsx // App.js import React from 'react';
function App() { return <h1>Mi Aplicación React</h1>; }
export default App; ```
6. Crear un archivo index.js que se encargará de renderizar el componente App en el div root:
```js // index.js
import React from 'react'; import ReactDOM from 'react-dom'; import App from './App';
ReactDOM.render(<App />, document.getElementById('root')); ```
7. Ejecutar la aplicación con `npm start` y acceder a index.html. Debería mostrar el título renderizado por App.js.
8. A partir de aquí puedes crear más componentes y añadir routing, estados, etc.
Así se crea una aplicación React muy básica configurando Webpack y Babel para empaquetar el código. ¡Espero que esto ayude! Si tienes alguna otra duda no dudes en preguntar.
1 note
·
View note
Text
The React UI Library Landscape in 2023: A Guide for Enterprise Developers
React has transformed frontend development since Facebook open-sourced it over a decade ago. One key driver of React's meteoric rise has been its extensive ecosystem of third-party UI component libraries.
With thousands of React libraries to evaluate, how do enterprise developers identify the most valuable options? This guide explains why UI libraries are critical for enterprise React apps and highlights the top libraries for 2023 based on real-world traction and impact.
The Power of UI Component Libraries
UI libraries provide several crucial advantages:
Accelerated Development
Like getting a completed Lego set to build a new spaceship rather than assembling each brick from scratch, pre-built UI components allow you to skip repetitive work on buttons, menus, etc so you can focus on your unique product needs. Wired-together elements kickstart development so you build new features faster.
Consistent Look and Feel
Ever notice how Apple products seamlessly work together? From iPhones syncing smoothly with Macbooks, established design systems minimize custom work and provide a unified UI language. Thoughtfully designed components naturally fit together out-of-the-box.
Best Practices Baked In
Vetted libraries incorporate crucial capabilities like accessibility, internationalization, and responsiveness so you don’t have to reinvent the wheel. It's like getting a cake recipe from a master chef versus guessing at measurements.
Cross-Platform Flexibility
Many libraries offer native equivalents via React Native for web, iOS, and Android code reuse. Write once, run anywhere - like magic!
Battle-tested Solutions
Popular libraries represent solutions refined over years of real-world usage, avoiding the pitfalls of a homegrown approach. Why build your own car when you can buy a proven Honda?
Top 5 React UI Libraries for Enterprises
Now let's explore the top React UI libraries for enterprises based on community adoption, documentation quality, and real-world performance.
1. Material UI
Overview: The most complete implementation of Google's Material Design specification in React. Includes all core components like buttons, menus, text fields, grids, and more needed to build compliant Material apps.
With 5M+ downloads per month, Material UI provides everything you need for professional Material Design in React. Like getting a master's toolbox for carpentry, Material UI equips you with meticulously crafted components aligned to Material principles so you can build quality UIs faster.
Key Benefits:
Extremely customizable visual themes let you match your brand identity. Make Material Design your own.
Components rigorously follow Material design standards for professional polish out of the box.
Strong focus on accessibility with built-in ARIA roles, dark mode, RTL support, and more.
Example Apps: T-Mobile, Toyota, IBM Cloud, TripAdvisor, Docker
Learn More: Material UI Documentation
2. React Bootstrap
Overview: A complete rebuild of Bootstrap's CSS framework and components for React apps. Includes all the flagship Bootstrap components like grid system, forms, buttons, navigation, and more optimized for use in React.
If you know Bootstrap, you already know React Bootstrap. Like upgrading your old car with a new engine and accessories, React Bootstrap will be instantly familiar. Modernizes and supercharges your Bootstrap knowledge to build React apps faster.
Key Benefits:
No need to learn new patterns - build React apps with familiar Bootstrap conventions.
Huge time savings reusing your team's existing Bootstrap knowledge and code.
Integrates with Create React App and ReactDOM for painless startup configuration.
Extensive custom theming support adapts Bootstrap styling to your brand.
Example Apps: Twitter, Under Armour, Trivago, Glassdoor, Wix
Learn More: React Bootstrap Documentation
3. Ant Design
Overview: An enterprise-grade React UI library for building elegant, data-rich applications. Offers strong default theme, out-of-box accessibility, and powerful data components like tables, trees, and dashboards.
If Material Design feels too rigid and Bootstrap too basic, Ant Design hits the sweet spot between design-led and developer-focused. Originating from Chinese e-commerce giant Alibaba, Ant Design combines stunning visuals with robust customization capabilities suited to complex business apps.
Key Benefits:
Sophisticated data visualization components for crafting interactive analytics and reporting.
Robust theming customization with variable-based approach similar to SASS/LESS.
Internationalized by default with 30+ language packs available.
Thoughtful interactions like drag-and-drop table rows make UIs dynamic.
Example Apps: Alibaba, Bilibili, Affirm, Grab, PicnicHealth
Learn More: Ant Design Documentation
4. Chakra UI
Overview: A modular, customizable React component library designed for building accessible, lightweight UIs. Components expose style props for complete CSS-in-JS control.
If you value fine-grained control over your UI styling, Chakra UI delivers a lean yet customizable component toolkit. Inspired by sites like Twitch and GitHub, Chakra focuses on flexibility with its modular CSS-in-JS approach while providing tight accessibility compliance.
Key Benefits:
Elegant out-of-the-box theme minimizes need to write custom styles.
Flexible CSS-in-JS styling and component composition enable endless customization.
Focus on accessibility with screen reader support, WAI-ARIA compliance, dark mode.
Components designed for great page performance and small bundle sizes.
Example Apps: LYX, Plausible Analytics, Swapcard, Replit, Figma
Learn More: Chakra UI Documentation
5. BlueprintJS
Overview: A React UI toolkit for the web focused on enterprise applications needing complex data visualizations, tables, charts, pickers and more.
For building dense data-rich interfaces with React, BlueprintJS provides a refined UI palette that looks great out-of-the-box. Originally created by Palantir, Blueprint includes robust support for crafting complex analytical applications with its broad selection of polished charts, pickers, and data components.
Key Benefits:
Elegant visual style language optimized for dense data UIs.
Robust theming and stylesheet customization options.
Components rigorously tested across modern browsers for reliability.
Integrates with React, Angular, Vue, and plain JavaScript apps.
Example Apps: Spotify, Netflix, Airbnb, IBM Watson, CircleCI
Learn More: BlueprintJS Documentation
And So Much More...
While this post covers 5 recommended libraries, there are many more excellent options like React Strap, Grommet, Elemental UI, and PrimeReact. The React ecosystem expands daily, with abundant libraries to enhance your next project.
When evaluating options, consider your constraints around design, speed, platforms, and existing tech stack. Opt for established libraries with growing communities over one-off solutions.
Ready to Build with React?
React UI libraries enable enterprises to build world-class software faster. Hopefully this guide has provided a useful introduction to the React ecosystem and top component libraries for enterprise developers.
Here at FullStackDevelopers.co, we specialize in connecting great enterprises with expert React and JavaScript developers to turn ambitious visions into reality. If your team needs help on an upcoming React project, get in touch to start a conversation and take your skills to the next level. The right talents could be just a few clicks away!
1 note
·
View note
Text
The React UI Library Landscape in 2023: A Guide for Enterprise Developers
React has transformed frontend development since Facebook open-sourced it over a decade ago. One key driver of React's meteoric rise has been its extensive ecosystem of third-party UI component libraries.
With thousands of React libraries to evaluate, how do enterprise developers identify the most valuable options? This guide explains why UI libraries are critical for enterprise React apps and highlights the top libraries for 2023 based on real-world traction and impact.
The Power of UI Component Libraries
UI libraries provide several crucial advantages:
Accelerated Development
Like getting a completed Lego set to build a new spaceship rather than assembling each brick from scratch, pre-built UI components allow you to skip repetitive work on buttons, menus, etc so you can focus on your unique product needs. Wired-together elements kickstart development so you build new features faster.
Consistent Look and Feel
Ever notice how Apple products seamlessly work together? From iPhones syncing smoothly with Macbooks, established design systems minimize custom work and provide a unified UI language. Thoughtfully designed components naturally fit together out-of-the-box.
Best Practices Baked In
Vetted libraries incorporate crucial capabilities like accessibility, internationalization, and responsiveness so you don’t have to reinvent the wheel. It's like getting a cake recipe from a master chef versus guessing at measurements.
Cross-Platform Flexibility
Many libraries offer native equivalents via React Native for web, iOS, and Android code reuse. Write once, run anywhere - like magic!
Battle-tested Solutions
Popular libraries represent solutions refined over years of real-world usage, avoiding the pitfalls of a homegrown approach. Why build your own car when you can buy a proven Honda?
Top 5 React UI Libraries for Enterprises
Now let's explore the top React UI libraries for enterprises based on community adoption, documentation quality, and real-world performance.
1. Material UI
Overview: The most complete implementation of Google's Material Design specification in React. Includes all core components like buttons, menus, text fields, grids, and more needed to build compliant Material apps.
With 5M+ downloads per month, Material UI provides everything you need for professional Material Design in React. Like getting a master's toolbox for carpentry, Material UI equips you with meticulously crafted components aligned to Material principles so you can build quality UIs faster.
Key Benefits:
Extremely customizable visual themes let you match your brand identity. Make Material Design your own.
Components rigorously follow Material design standards for professional polish out of the box.
Strong focus on accessibility with built-in ARIA roles, dark mode, RTL support, and more.
Example Apps: T-Mobile, Toyota, IBM Cloud, TripAdvisor, Docker
Learn More: Material UI Documentation
2. React Bootstrap
Overview: A complete rebuild of Bootstrap's CSS framework and components for React apps. Includes all the flagship Bootstrap components like grid system, forms, buttons, navigation, and more optimized for use in React.
If you know Bootstrap, you already know React Bootstrap. Like upgrading your old car with a new engine and accessories, React Bootstrap will be instantly familiar. Modernizes and supercharges your Bootstrap knowledge to build React apps faster.
Key Benefits:
No need to learn new patterns - build React apps with familiar Bootstrap conventions.
Huge time savings reusing your team's existing Bootstrap knowledge and code.
Integrates with Create React App and ReactDOM for painless startup configuration.
Extensive custom theming support adapts Bootstrap styling to your brand.
Example Apps: Twitter, Under Armour, Trivago, Glassdoor, Wix
Learn More: React Bootstrap Documentation
3. Ant Design
Overview: An enterprise-grade React UI library for building elegant, data-rich applications. Offers strong default theme, out-of-box accessibility, and powerful data components like tables, trees, and dashboards.
If Material Design feels too rigid and Bootstrap too basic, Ant Design hits the sweet spot between design-led and developer-focused. Originating from Chinese e-commerce giant Alibaba, Ant Design combines stunning visuals with robust customization capabilities suited to complex business apps.
Key Benefits:
Sophisticated data visualization components for crafting interactive analytics and reporting.
Robust theming customization with variable-based approach similar to SASS/LESS.
Internationalized by default with 30+ language packs available.
Thoughtful interactions like drag-and-drop table rows make UIs dynamic.
Example Apps: Alibaba, Bilibili, Affirm, Grab, PicnicHealth
Learn More: Ant Design Documentation
4. Chakra UI
Overview: A modular, customizable React component library designed for building accessible, lightweight UIs. Components expose style props for complete CSS-in-JS control.
If you value fine-grained control over your UI styling, Chakra UI delivers a lean yet customizable component toolkit. Inspired by sites like Twitch and GitHub, Chakra focuses on flexibility with its modular CSS-in-JS approach while providing tight accessibility compliance.
Key Benefits:
Elegant out-of-the-box theme minimizes need to write custom styles.
Flexible CSS-in-JS styling and component composition enable endless customization.
Focus on accessibility with screen reader support, WAI-ARIA compliance, dark mode.
Components designed for great page performance and small bundle sizes.
Example Apps: LYX, Plausible Analytics, Swapcard, Replit, Figma
Learn More: Chakra UI Documentation
5. BlueprintJS
Overview: A React UI toolkit for the web focused on enterprise applications needing complex data visualizations, tables, charts, pickers and more.
For building dense data-rich interfaces with React, BlueprintJS provides a refined UI palette that looks great out-of-the-box. Originally created by Palantir, Blueprint includes robust support for crafting complex analytical applications with its broad selection of polished charts, pickers, and data components.
Key Benefits:
Elegant visual style language optimized for dense data UIs.
Robust theming and stylesheet customization options.
Components rigorously tested across modern browsers for reliability.
Integrates with React, Angular, Vue, and plain JavaScript apps.
Example Apps: Spotify, Netflix, Airbnb, IBM Watson, CircleCI
Learn More: BlueprintJS Documentation
And So Much More...
While this post covers 5 recommended libraries, there are many more excellent options like React Strap, Grommet, Elemental UI, and PrimeReact. The React ecosystem expands daily, with abundant libraries to enhance your next project.
When evaluating options, consider your constraints around design, speed, platforms, and existing tech stack. Opt for established libraries with growing communities over one-off solutions.
Ready to Build with React?
React UI libraries enable enterprises to build world-class software faster. Hopefully this guide has provided a useful introduction to the React ecosystem and top component libraries for enterprise developers.
Here at FullStackDevelopers.co, we specialize in connecting great enterprises with expert React and JavaScript developers to turn ambitious visions into reality. If your team needs help on an upcoming React project, get in touch to start a conversation and take your skills to the next level. The right talents could be just a few clicks away!
1 note
·
View note
Photo
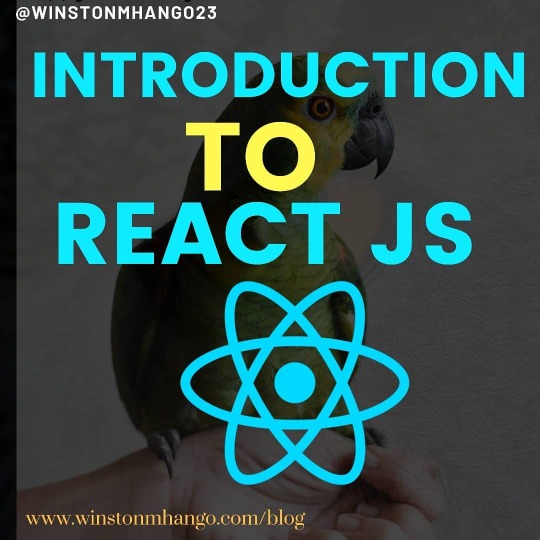
Introduction to React JS React is one of the most famous JavaScript libraries used for building complex user interfaces. Created by Facebook,the library is used of now,reportedly used by 8798 top fortune companies including Facebook itself,Twitter, Netflix, Uber, Airbnb and others. To be honest,it has an intimidating syntax if you look at it from a distance (especially when your JavaScript skills are not strong enough ). But the reality is that React is very simple.Just build your es6 skills.That will be it.Once you start, you will feel like coding in native Javascript!! All the library is doing is creating and manipulating DOM elements in a smarter way by using the virtual DOM🤗🤗. In this new slides series on Introduction to React JS,I will be showing how easy it is to develop react applications. This slide set gives a brief introduction of the library and how it works. Enjoy the reading and be there for more #javascriptlibraries #reactjs #reactvirtualDOM #htDOM #react.rendermethod #reactDOM.rendermethod #reactcreateapp #jsx #reactjsx (at Lilongwe, Malawi) https://www.instagram.com/p/B9b78SKhquY/?igshid=1lowi8kdwf8zx
0 notes
Video
youtube
ReactJS Tutorials in Hindi | Environment Setup | CDN Links | Part-2
0 notes
Text
React JS.NET - clientside errors with Webpack
If you are using using React JS.NET versions 3 or 4 in combination with Webpack 4 and Babel you will may come across an error where a serverside rendered component will not have working events on the clientside. In this scenario the @Html.ReactWithInit() function is not rendering the ReactDom.Hydrate clientside script. A workaround is to use script initialisation per component rather than a global one for all components. So rather than using @Html.React() to place a component in a MVC View i'm using @Html.ReactWithInit(). The only other difference is that your React and clientside javascript needs to be declared in your document head (i.e. before your components). A lot of the examples online for ReactJS.Net are out of date and use Webpack 1 (such as https://github.com/reactjs/React.NET/tree/master/src/React.Sample.Webpack), so keep this in mind when setting up a new project.
0 notes
Text
react tutorial by programming by mosh
Wrap code in () in return to return multiple line js code
function ListGroup() {
return(
<ul className="list-group">
<li classNa me="list-group-item">An item</li>
<li className="list-group-item">A second item</li>
<li className="list-group-item">A third item</li>
<li className="list-group-item">A fourth item</li>
<li className="list-group-item">And a fifth one</li>
</ul>);
}
export default ListGroup;
React Fragment
is a feature in React that allows you to return multiple elements from a React component by allowing you to group a list of children without adding extra nodes to the DOM.
import
import { Fragment } from "react";
Syntax
<Fragment></Fragment>
or
<></> empty tags
Wrapping js code inside jsx is not possible to use it we use { } Only react components and html code inside jsx is allowed.
import { Fragment } from "react";
function ListGroup() {
const items = ["tokyo", "Delhi", "texas", "toronto", "vancouver"];
return(
<>
<ul className="list-group">
{ items.map((item)=><li className="list-group-item" key={item}>{item}</li> )}
// green item is dynamic element so it's wrapped in {}
</ul>
</>
);
}
export default ListGroup;
expressions
'true' && 'mosh' // mosh
'true' && 1 // 1
result is second value always when first is true
'false' && 1
result is false
HOW DOES IT KNOW INDEX VARIABLE INSIDE map function second variable is index?
import { Fragment } from "react";
function ListGroup() {
const items = ["tokyo", "Delhi", "texas", "toronto", "vancouver"];
return(
<>
<h1>List</h1>
<ul className="list-group">
{ items.map((item, index)=><li className="list-group-item" key={item} onClick={()=>console.log(item, index)} >{item}</li> )}
</ul>
</>
);
}
export default ListGroup;
text in the red. because syntax of map is:
map(function (element, index, array) { /* … */ }, thisArg)
useState
syntax
const [state, setState] = useState(initialValue); state is data that changes
initial value is something you initialize and
setState is changed value
state is previous state that will be changed.
code
import { useState } from "react";import ReactDOM from "react-dom/client";
function FavoriteColor() { const [color, setColor] = useState("red");
return ( <> My favorite color is {color}!
setColor("blue")} >Blue ) }
const root = ReactDOM.createRoot(document.getElementById('root')); root.render();
Props
props are input to the component
Step 1:
interface Props{
}
this red Props can also be named after component like ListGroupProps{
}
Step 2
after this just like function arguments write these in component ( )
function ListGroup({items, heading}: Props ){
}
Step 3:
in app.tsx
function App(){
let items = ["tokyo", "Delhi", "texas", "toronto", "vancouver"];
return <div><ListGroup items={items} heading="cities"/></div>;
}
green text was pasted from ListGroup.tsx
mention the props from the ListGroup.tsx in tag of component in app.tsx just like red text.
we could write heading="cities" like heading={"cities"} also but it is unnecessary.
0 notes
Text
8 Arguments for Learning React js
8 Arguments for Learning React js
One of the most widely used JavaScript frameworks for creating dynamic and quick online apps is React.
React is simple to utilize in new or existing applications, making it accessible to JavaScript developers of all skill levels.
In its relatively brief existence, React has swiftly established itself as a developer favorite, competing with other JavaScript frameworks like Angular and Vue.js.
Simple Learning Curve 1.
As a JavaScript developer, learning React is a simple and stress-free process. React's syntax and structure are quite close to ordinary JavaScript because it is a JavaScript framework. Your React application can use any JavaScript notion or functionality. As a result, you may quickly embrace React and create your first web application with it.
Additionally, switching your application from a language like jQuery to React is simple. For beginner JavaScript developers, the React team's thorough documentation, fantastic online courses, and simple access to resources will help you become familiar with all the key ideas required to become a well-rounded React developer.
2. Lightweight Virtual DOM
Although DOM operations are speedy and light, re-rendering in response to data updates is typically expensive. React does not render UI elements using the browser's actual DOM, in contrast to the majority of other front-end JavaScript frameworks. The ReactDOM library, which manages how the rendering update will take place, is used by React.
React DOM renders views using a Virtual DOM technique. It keeps a virtual copy of the UI modifications and later updates the DOM of the browser. By simply updating what is required when a change occurs as opposed to rebuilding the complete program, a virtual DOM will improve view updates.
3. Component Organization
A component is a JavaScript class or function at its most fundamental level. They take input values, or "props," and return particular user interface components in the form of React components. They enable programmers to separate user interfaces into distinct chunks that they can combine to create more complex user interfaces.
You may create dynamic web apps using the reusable components found in the React library. The design interface of every React application combines components to create more sophisticated ones, which collectively make up a component tree. By reusing those same components, React's component structure enables you to keep your web app's design consistent while extending its code base.
4. Good Performance
Fast and dependable applications made with React. React apps are created in a variety of ways as a result of which this performance success is achieved.
The application does not have to re-render the entire page every time a component's state value changes thanks to React's lightweight virtual DOM technology. As the user interacts with the web app, React will maintain perfect sync between the virtual and actual DOMs, updating the latter as appropriate. This lessens the strain on the browser and improves user experience.
React is incredibly adaptable, therefore by adhering to React best practices, you may enhance the performance of your online application. You may build React code that is better and more organized by using these techniques.
5. React DevTools Make Debugging Simple
You can debug React projects using the browser extension React Developer Tools. When executing a development build, you can analyze React components inside your web application to find performance problems. Inside the React component tree, you may also inspect component props, states, and hooks. This makes debugging your React applications simpler, giving you more time to concentrate on the essential components of your program. For browsers like Safari, React Dev Tools is available as an npm package.
6. Shorter Development Period
You can ship your applications more quickly by using React while creating web applications.
React is a well-liked and reliable framework, therefore there are many open-source APIs and tools to speed up development and assist you in scaling through complicated setups. Developers save time thanks to React's component reuse because they don't have to waste time creating the same components every time they want to utilize them.
Additionally, React uses a declarative method rather than an imperative one to handle state in web apps. This implies that instead of you having to code the intricate logic yourself, React will take care of the management and representation of the state in your application.
7. Low Cost of Development
The time it takes to create a web application directly relates to how much it costs to produce that web application. A standard functioning React web application may be built in a relatively short amount of time, which results in decreased development costs. React Native, a cross-platform mobile programming framework may also be learned with React. As a result, you will be able to convert your online applications into cross-platform mobile applications using your existing React knowledge.
All of these enable you to cut costs while maximizing efficiency.
8. Active Neighborhood Support
React's the vibrant community is one of the factors that make it such a popular JavaScript framework for creating online apps. The first day of React's availability was May 29, 2013. Since it was released, there has been a significant increase in both developer adoption and community size.
React is the second-most popular framework among developers worldwide, according to Statista.
0 notes
Text
SPFX themes
how to make spfx obey themes
build your app component class
build app component props interface
add the following to the props interface import { IReadonlyTheme } from '@microsoft/sp-component-base';
and add a property to store the theme
themeVariant: IReadonlyTheme | undefined;
add the following to the webPart props interface import { IReadonlyTheme } from '@microsoft/sp-component-base';
and add a property to store the theme themeVariant: this._themeVariant
add the following imports to your webPart class<span>import</span><span> { </span><span>ThemeProvider</span><span>, </span><span>ThemeChangedEventArgs</span><span>, </span><span>IReadonlyTheme</span><span> } </span><span>from</span><span> </span><span>'@microsoft/sp-component-base'</span><span>;</span>
ensure that in your render you have set the themeVariant like so <span> </span><span>public</span><span> </span><span>render</span><span>(): </span><span>void</span><span> {</span><br><span> </span><span>const</span><span> </span><span>element</span><span>: </span><span>React</span><span>.</span><span>ReactElement</span><span><</span><span>ISpfxWeeklyQuestionnaireAppProps</span><span>> = </span><span>React</span><span>.</span><span>createElement</span><span>(</span><br><span> </span><span>SpfxWeeklyQuestionnaireApp</span><span>,</span><br><span> {</span><br><span> </span><span>listTitle</span><span>:</span><span> </span><span>this</span><span>.</span><span>properties</span><span>.</span><span>title</span><span>,</span><br><span> </span><span>ctx</span><span>:</span><span> </span><span>this</span><span>.</span><span>context</span><span>,</span><br><span> </span><span>themeVariant</span><span>:</span><span> </span><span>this</span><span>.</span><span>_themeVariant</span><br><span> }</span><br><span> );</span><br><span> </span><span>ReactDom</span><span>.</span><span>render</span><span>(</span><span>element</span><span>, </span><span>this</span><span>.</span><span>domElement</span><span>);</span><br><span> }</span>
add the following to end of your webPart class <span> </span><span>private</span><span> </span><span>_themeProvider</span><span>: </span><span>ThemeProvider</span><span>;</span><br><span> </span><span>private</span><span> </span><span>_themeVariant</span><span>: </span><span>IReadonlyTheme</span><span> | </span><span>undefined</span><span>;</span><br><br><span> </span><span>protected</span><span> </span><span>onInit</span><span>(): </span><span>Promise</span><span><</span><span>void</span><span>> {</span><br><span> </span><span>this</span><span>.</span><span>_themeProvider</span><span> = </span><span>this</span><span>.</span><span>context</span><span>.</span><span>serviceScope</span><span>.</span><span>consume</span><span>(</span><span>ThemeProvider</span><span>.</span><span>serviceKey</span><span>);</span><br><span> </span><span>this</span><span>.</span><span>_themeVariant</span><span> = </span><span>this</span><span>.</span><span>_themeProvider</span><span>.</span><span>tryGetTheme</span><span>();</span><br><span> </span><span>this</span><span>.</span><span>_themeProvider</span><span>.</span><span>themeChangedEvent</span><span>.</span><span>add</span><span>(</span><span>this</span><span>,</span><span>this</span><span>.</span><span>_handleThemeChangedEvent</span><span>);</span><br><span> </span><span>return</span><span> </span><span>super</span><span>.</span><span>onInit</span><span>();</span><br><span> }</span><br><br><span> </span><span>private</span><span> </span><span>_handleThemeChangedEvent</span><span>(</span><span>args</span><span>: </span><span>ThemeChangedEventArgs</span><span>): </span><span>void</span><span> {</span><br><span> </span><span>this</span><span>.</span><span>_themeVariant</span><span>=</span><span>args</span><span>.</span><span>theme</span><span>;</span><br><span> </span><span>this</span><span>.</span><span>render</span><span>();</span><br><span> }</span>
0 notes
Text
What to Choose Between Node.js and React.js?

Javascript is one of the most popular languages. Node.js and React.js is two most popular frameworks. Sometime developer face struggle to use these frameworks. When it comes to comparison between the both platforms Node.js is a back-end framework, and React.js is used for developing user interfaces. Both are gaining special attention nowadays with their some advantages and disadvantages. If you want to know which one best for you, you need to read this post to choose the better option.
Read out below:
Node.js:
For the purpose of running JavaScript code outside of a browser, Node.js is an open-source, cross-platform runtime environment. Also you need to consider that Node.js is not a framework and also it is not a programming language. Most of the newbie developers are facing trouble to understand that it is a framework or a programming language. It is used for back-end services like APIs like Mobile App or Web App.
Key features
From many other programming languages it is use to create back-end services that makes the Node.js is a unique platform to work on. These are some Node.js features:
· It is very agile to develop simple to use and can be used for prototyping.
· It offers the quick and scalable services.
· It uses JavaScript throughout, to make it simple for a JavaScript programmer.
ReactJS:
ReactJS is an open-source to build a single page interface.
It is an efficient, flexible, declarative platform that allows the users to create reusable UI components. Additionally, it serves as a foundation for complicated, one-page interactive web projects and react components that are very challenging to reuse. React's virtual DOM technique requires creating lengthy, inaccurate code. Each component in a React application is in charge of rendering a discrete chunk of reusable HTML. Simple building blocks can be used to create complex applications because to the ability to nest components inside of one another.
Key features
· It is clean or more consistent source code.
· Open-source libraries have a larger ecosystem.
· Its nature is non-blocking or asynchronous.
· The following are some traits that set React.js apart from other programming languages:
· Also its components feature has reusable code, which makes it easy to understand and use as well.
· The React package's JSX (JavaScript XML) syntax is an HTML-like syntax that converts to JavaScript calls.
· Reusable React components with their own logic and controls are helpful when working on bigger projects.
· You have more control over your application when using one-way data binding.
· The ReactDOM package is used by the virtual DOM, which theoretically or virtually represents the user interface and keeps it in memory while synchronising with the real DOM.
· Because virtual components are present, DOM performs more quickly and smoothly.
Conclusion
If you are looking for creating server-side web application, like an online streaming platform, Node.js can be an excellent framework to use. Instead if you want to create a project with changing states like dynamic buttons, inputs, and so on, React.js can be the choice to work on. Also you can utilise both frameworks for single project.
Rather if you are looking for professional Node.js Development Services you can choose our Node.js Development Company for the best result.
#Node.js Development Company#Node.js Development#Node.js Development Services#Node.js Development Company India#Node.js Development Company USA#Node.js Developer#Node.js#Best Node.js Development Company
0 notes
Text
React script unpkg

#React script unpkg how to#
#React script unpkg how to#
At the time of this writing, that’s what the React docs show you how to do it for basic examples, i.e.: ReactDOM. Granted, you can author in JSX and ship it directly to the browser. (Once we get stuff like import maps you wouldn’t even have to do this.) I import the libraries in one place and export them for use in other files. For example, having a deps.js file makes it so I don’t have to write out those long CDN URLs all the time. Then my index.js file: import ` īut as I mentioned, I like splitting the JavaScript out into individual modules because it helps me expand and grow the functionality over time. If it becomes viable longer term, I then consider factoring in a build process, removing my dependence on React, etc.įirst, I have my root HTML file ( index.html): Title of Your Page This is a great place to start with an idea. The code I write is the code that ships to the browser. It allows me to leverage React and write JSX-like syntax without any dependence on a build tool. Ok, so, with that context in mind, I have this little boilerplate I’ve pieced together from a couple different sources (big thanks to the preact docs). computer-“can I get this working?”-and I find that I’ll move heaven and earth just to see something work only to realize that what I wanted to do was building something usable in a browser, not optimize and streamline my “DX”. It’s like I immediately forget that I was trying to build something useful and instead it becomes a fight of nerd vs. The moment configurations, build processes, and what not get involved, those become the focus of my attention. Using a framework like React can make doing that incredibly easy-as long as there is no build tooling involved. I find myself quite often needing to prototype something really quick, sometimes even build an “MVP” of something I can put out into the world for feedback. First off: this is mostly a reference for myself.

0 notes
Text
10 Reasons You Must Know About ReactJS Development Before Using It
A well-liked JavaScript library for web development is called React. Different names for ReactJS include React.js, ReactJS, and React. React JS is also used by a lot of big businesses today (like Netflix and Instagram, to name a couple). This framework has many advantages over other frameworks, and it has consistently ranked in the top 10 programming languages according to various language ranking indices for the past few years.
ReactJS: Why?What reasons do people choose it?
All in all, what are the top advantages ReactJs Development Company can get from utilizing React in their activities and top motivations to pick?
Part Style Architecture - ReactJS is Component Style Architecture, and that is where the fate of the web is. This Architecture has additionally helped the Java engineer local area to relocate to ReactJS with less erosion.
Less development time and better quality - React permits you to compose perfect and particular code, breaking the task into discrete parts. So we can reuse code, and which will expand the efficiency of developers and ReactJS development Services.
Elite execution of your application - ReactJS primary element i.e Virtual DOM (in which portrayal of UI parts is kept in memory and afterward adjusted with genuine DOM by library like ReactDOM) that outcomes in superior execution of the application and gives better client experience
Solid people group - Being made by Facebook, the library is as yet kept up with by the company as well as by free benefactors around the world. Because of the local area and strong corporate help, React is a dependable and modern innovation. Since the local area is developing quickly, it will be simpler to employ reactjs developers.
Adaptable and simple to keep up with - React code is not difficult to refresh and keep up with because of its secluded construction. React projects are amazingly adaptable and can be scaled without any problem. It can assist you with setting aside time and cash over the long haul altogether.
Some outsider parts - Since React has major areas of strength for a local area, there are some outsider open source parts accessible which we can use in our venture.
Simple progress to React Native - React Native is a versatile application development system, in view of React. In this way, it is easy for ReactJS developers to change to React Native and make versatile applications with a local look and feel. We can reuse a few parts created in React web application in a React Native portable application (for the most part business rationale), which further develops the development speed.
It's not difficult to learn - React is a lot more straightforward to learn than to other famous frontend structures like AngularJS Development and VueJS Development. It's one of the fundamental justifications for why React acquired such a lot of ubiquity in so brief period. It assists businesses with rapidly assembling their undertakings.
Make react-application - React gives make react-application, which in all actuality do all underlying truly difficult work of setting up setup and assists us with zeroing in on code and not toolchain. This order in React introduces the conditions expected to assemble your undertaking and furthermore created an underlying task structure. By running this order, we can rapidly begin with new undertakings.
It assists with building rich UIs - Today, the nature of the UI in an application assumes a significant part. Ineffectively planned UIs bring down the possibilities of an application to succeed. However, in the event that an application has excellent UI, there are better opportunities for clients to very much want to utilize the application. React licenses building such top notch, rich UIs through its parts
It permits composing custom parts - React accompanies JSX, a linguistic structure expansion, which makes it conceivable to compose your parts. These parts acknowledge HTML citing and furthermore makes all subcomponent delivering a brilliant encounter for developers.
With this, my blog post has finally come to a conclusion. We believe you now understand why React JS is an excellent front-end framework.
React is a top-notch framework that is deserving of your attention, just as Angular.
What then are you still holding out for?
Hire React JS developer now to get going!
And keep in mind that working with the finest iSyncEvolution LLP firm is essential to creating a successful React JS app. Therefore, get in contact with us right away to schedule a project consultation.
0 notes
Text
React is a JavaScript library that helps developers build web and mobile apps. It is one of the most popular front-end libraries in use today. React has two main components - React.js and ReactDOM. The former provides the core framework for a developer to work with while the latter provides a way to render the react UI on the browser or mobile app screen. React best practices are important to follow if you want your app to be successful and stable. Some of these include
Use JSX for your markup
Prefer ES6 over ES5
Use JSX for rendering
Keep stateless components in a separate file
0 notes