#types of inheritance in java
Explore tagged Tumblr posts
Text
Understanding Inheritance in Java: Exploring Types and Examples
In the world of Java programming, inheritance is a powerful concept that allows developers to create a relationship between classes, fostering code reuse and organization. In this blog, we'll delve into what inheritance is in Java, explore its types, and provide practical examples to make it all crystal clear.
What is Inheritance in Java?
Inheritance is a key pillar of Object-Oriented Programming (OOP), enabling a class to inherit properties and behaviors from another class. The class granting the inheritance is known as the superclass or parent class, and the class receiving the inheritance is the subclass or child class. This mechanism promotes the reuse of code and establishes a hierarchy among classes.
Types of Inheritance in Java:
1. Single Inheritance:
In single inheritance, a subclass inherits from only one superclass. Let's illustrate this with a simple example:
Here, Dog is the subclass inheriting from the Animal superclass. The Dog class can access the eat() method from the Animal class.
2. Multiple Inheritance (Through Interfaces):
Java doesn't support multiple inheritance for classes, but it does support it through interfaces. Consider the following:
Here, the Human class implements both the Walkable and Swimmable interfaces, achieving a form of multiple inheritance.
3. Multilevel Inheritance:
In multilevel inheritance, a class inherits from another class, and then another class inherits from the second class, forming a chain. Let's see an example:
In this scenario, C inherits from B, and B inherits from A.
4. Hierarchical Inheritance:
In hierarchical inheritance, multiple classes inherit from a single superclass, creating a hierarchy. Consider the following:
Here, both Circle and Rectangle inherit from the Shape superclass.
Benefits of Inheritance:
Code Reusability: Inheritance allows you to reuse code from existing classes, minimizing redundancy.
Method Overriding: Subclasses can provide specific implementations for methods inherited from the superclass.
Polymorphism: Objects of the subclass can be treated as objects of the superclass, enhancing flexibility.
Modularity: Inheritance aids in creating modular and easily understandable code by organizing classes into a hierarchical structure.
Conclusion
In conclusion, understanding inheritance in Java is fundamental to writing efficient and organized code. Whether you're a beginner or an experienced developer, incorporating inheritance into your Java programming skills can significantly enhance your ability to create robust and maintainable applications.
Get to Know more: https://analyticsjobs.in/question/can-you-please-tell-me-what-are-the-various-types-of-inheritance-in-java/
#java#inheritance in java#learn java#analyticsjobs#what is inheritance#types of inheritance#elearning#coding#programming
0 notes
Text
Good Code is Boring
Daily Blogs 358 - Oct 28th, 12.024
Something I started to notice and think about, is how much most good code is kinda boring.
Clever Code
Go (or "Golang" for SEO friendliness) is my third or fourth programming language that I learned, and it is somewhat a new paradigm for me.
My first language was Java, famous for its Object-Oriented Programming (OOP) paradigms and features. I learned it for game development, which is somewhat okay with Java, and to be honest, I hardly remember how it was. However, I learned from others how much OOP can get out of control and be a nightmare with inheritance inside inheritance inside inheritance.
And then I learned JavaScript after some years... fucking god. But being honest, in the start JS was a blast, and I still think it is a good language... for the browser. If you start to go outside from the standard vanilla JavaScript, things start to be clever. In an engineering view, the ecosystem is really powerful, things such as JSX and all the frameworks that use it, the compilers for Vue and Svelte, and the whole bundling, and splitting, and transpiling of Rollup, ESBuild, Vite and using TypeScript, to compile a language to another, that will have a build process, all of this, for an interpreted language... it is a marvel of engineering, but it is just too much.
Finally, I learned Rust... which I kinda like it. I didn't really make a big project with it, just a small CLI for manipulating markdown, which was nice and when I found a good solution for converting Markdown AST to NPF it was a big hit of dopamine because it was really elegant. However, nowadays, I do feel like it is having the same problems of JavaScript. Macros are a good feature, but end up being the go-to solution when you simply can't make the code "look pretty"; or having to use a library to anything a little more complex; or having to deal with lifetimes. And if you want to do anything a little more complex "the Rust way", you will easily do head to head with a wall of skill-issues. I still love it and its complexity, and for things like compiler and transpilers it feels like a good shot.
Going Go
This year I started to learn Go (or "Golang" for SEO friendliness), and it has being kinda awesome.
Go is kinda like Python in its learning curve, and it is somewhat like C but without all the needing of handling memory and needing to create complex data structured from scratch. And I have never really loved it, but never really hated it, since it is mostly just boring and simple.
There are no macros or magic syntax. No pattern matching on types, since you can just use a switch statement. You don't have to worry a lot about packages, since the standard library will cover you up to 80% of features. If you need a package, you don't need to worry about a centralized registry to upload and the security vulnerability of a single failure point, all packages are just Git repositories that you import and that's it. And no file management, since it just uses the file system for packages and imports.
And it feels like Go pretty much made all the obvious decisions that make sense, and you mostly never question or care about them, because they don't annoy you. The syntax doesn't get into your way. And in the end you just end up comparing to other languages' features, saying to yourself "man... we could save some lines here" knowing damn well it's not worth it. It's boring.
You write code, make your feature be completed in some hours, and compile it with go build. And run the binary, and it's fast.
Going Simple
And writing Go kinda opened a new passion in programming for me.
Coming from JavaScript and Rust really made me be costumed with complexity, and going now to Go really is making me value simplicity and having the less moving parts are possible.
I am becoming more aware from installing dependencies, checking to see their dependencies, to be sure that I'm not putting 100 projects under my own. And when I need something more complex but specific, just copy-and-paste it and put the proper license and notice of it, no need to install a whole project. All other necessities I just write my own version, since most of the time it can be simpler, a learning opportunity, and a better solution for your specific problem. With Go I just need go build to build my project, and when I need JavaScript, I just fucking write it and that's it, no TypeScript (JSDoc covers 99% of the use cases for TS), just write JS for the browser, check if what you're using is supported by modern browsers, and serve them as-is.
Doing this is really opening some opportunities to learn how to implement solutions, instead of just using libraries or cumbersome language features to implement it, since I mostly read from source-code of said libraries and implement the concept myself. Not only this, but this is really making me appreciate more standards and tooling, both from languages and from ecosystem (such as web standards), since I can just follow them and have things work easily with the outside world.
The evolution
And I kinda already feel like this is making me a better developer overhaul. I knew that with an interesting experiment I made.
One of my first actual projects was, of course, a to-do app. I wrote it in Vue using Nuxt, and it was great not-gonna-lie, Nuxt and Vue are awesome frameworks and still one of my favorites, but damn well it was overkill for a to-do app. Looking back... more than 30k lines of code for this app is just too much.
And that's what I thought around the start of this year, which is why I made an experiment, creating a to-do app in just one HTML file, using AlpineJS and PicoCSS.
The file ended up having just 350 files.
Today's artists & creative things Music: Torna a casa - by Måneskin
© 2024 Gustavo "Guz" L. de Mello. Licensed under CC BY-SA 4.0
4 notes
·
View notes
Text
Mastering Java: Your Comprehensive Guide to Programming Excellence
Embarking on the journey of mastering Java is akin to entering a realm of endless possibilities. Java, a versatile and widely-utilized programming language, offers a broad spectrum of applications, from crafting web and mobile applications to powering robust enterprise systems. Whether you are a novice in the realm of coding or a seasoned programmer looking to broaden your skill set, the path to proficiency in Java is an exciting one.
In this comprehensive guide, we will be your guiding light through the intricacies of Java, starting from the foundational basics and progressing to the more advanced aspects of the language. Our objective is to equip you with the knowledge and skills that form a robust and unshakable foundation for your journey into the vibrant world of Java. Fasten your seatbelt as we embark on this exhilarating exploration, charting a course that will empower you to thrive in the ever-evolving landscape of software development.
Here's a 8-step guide to effectively learn Java
Step 1: Setting Up Your Development Environment
Your journey to becoming a proficient Java developer commences with setting up your development environment. The essential components are the Java Development Kit (JDK) and an Integrated Development Environment (IDE) like Eclipse or IntelliJ IDEA. These tools aren't just convenient; they're the gears that will drive your Java programming endeavors. They streamline the coding process, provide useful features, and offer an organized workspace, making your coding experience efficient and enjoyable.
Step 2: The Foundation - Learning the Basics
With your development environment ready, it's time to delve into the fundamental building blocks of Java. Begin by acquainting yourself with data types, variables, operators, and control structures. These are the nuts and bolts of the language, and a solid grasp of these concepts is essential. You'll find an abundance of online tutorials and beginner-friendly Java books to assist you at this stage.
Step 3: Navigating the World of Object-Oriented Programming (OOP)
The object-oriented programming (OOP) approach is well known in Java. To harness the true power of Java, immerse yourself in the world of OOP. Understand the concepts of classes, objects, inheritance, encapsulation, and polymorphism. This knowledge forms the bedrock of Java programming and enables you to design efficient, organized, and scalable code.
Step 4: Mastering Data Structures and Algorithms
Data structures (such as arrays, lists, and sets) and algorithms are the secret sauce behind solving real-world problems efficiently. As you progress, dive into the world of data structures and algorithms. These are the tools that will empower you to handle complex tasks and optimize your code. They're your go-to assets for creating efficient and responsive applications.
Step 5: The Art of Exception Handling
Java boasts a robust exception-handling mechanism. Understanding how to handle exceptions properly is not just an add-on skill; it's a vital aspect of writing reliable code. Exception handling ensures that your code gracefully manages unexpected situations, preventing crashes and delivering a seamless user experience.
Step 6: Exploring Input and Output Operations
In this step, you'll explore the realm of input and output (I/O) operations. Mastering I/O is crucial for reading and writing files, as well as interacting with users. You'll gain the ability to build applications that can efficiently process data and communicate effectively with users.
Step 7: Conquering Multi tasking
Java's support for multi tasking is a significant advantage. Understanding how to manage threads and synchronize their actions is vital for creating concurrent applications. Multithreading is the key to developing software that can handle multiple tasks simultaneously, making your applications responsive and scalable.
Step 8: Building Projects and Real-World Practice
Theory is only as valuable as its practical application. The final step involves applying what you've learned by building small projects. These projects serve as a proving ground for your skills and provide valuable additions to your portfolio. Whether it's a simple application or a more complex project, the act of building is where the real learning takes place.
As you step into this vibrant realm of Java, remember that continuous learning is the key to staying relevant and effective in the ever-evolving field of software development. Be open to exploring diverse applications, from web development to mobile apps and enterprise solutions, and never underestimate the power of hands-on practice. Building projects, no matter how small, will solidify your knowledge and boost your confidence.
In your quest to master Java, ACTE Technologies stands as a valuable ally. Their expert guidance and comprehensive training programs will sharpen your skills, boost your confidence, and pave the way for a rewarding career in software development. Whether you're embarking on your Java journey or looking to take your skills to the next level, ACTE Technologies offers the resources and support you need to thrive in the world of Java programming.
So, with Java as your trusty companion, and ACTE Technologies as your guide, the possibilities are boundless. Your journey is just beginning, and the world of software development awaits your innovation and expertise. Best of luck on your path to mastering Java!
9 notes
·
View notes
Text
Understanding Object-Oriented Programming and OOPs Concepts in Java
Object-oriented programming (OOP) is a paradigm that has revolutionized software development by organizing code around the concept of objects. Java, a widely used programming language, embraces the principles of OOP to provide a robust and flexible platform for developing scalable and maintainable applications. In this article, we will delve into the fundamental concepts of Object-Oriented Programming and explore how they are implemented in Java.
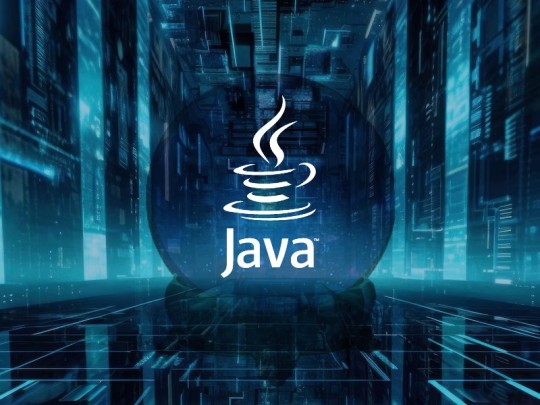
Object-Oriented Programming:
At its core, Object-Oriented Programming is centered on the idea of encapsulating data and behavior into objects. An object is a self-contained unit that represents a real-world entity, combining data and the operations that can be performed on that data. This approach enhances code modularity, and reusability, and makes it easier to understand and maintain.
Four Pillars of Object-Oriented Programming:
Encapsulation: Encapsulation involves bundling data (attributes) and methods (functions) that operate on the data within a single unit, i.e., an object. This encapsulation shields the internal implementation details from the outside world, promoting information hiding and reducing complexity.
Abstraction: Abstraction is the process of simplifying complex systems by modeling classes based on essential properties. In Java, abstraction is achieved through abstract classes and interfaces. Abstract classes define common characteristics for a group of related classes, while interfaces declare a set of methods that must be implemented by the classes that implement the interface.
Inheritance: Inheritance is a mechanism that allows a new class (subclass or derived class) to inherit properties and behaviors of an existing class (superclass or base class). This promotes code reuse and establishes a hierarchy, facilitating the creation of specialized classes while maintaining a common base.
Polymorphism: Polymorphism allows objects of different types to be treated as objects of a common type. This is achieved through method overloading and method overriding. Method overloading involves defining multiple methods with the same name but different parameters within a class, while method overriding allows a subclass to provide a specific implementation of a method that is already defined in its superclass.
Java Implementation of OOP Concepts:
Classes and Objects: In Java, a class is a blueprint for creating objects. It defines the attributes and methods that the objects of the class will have. Objects are instances of classes, and each object has its own set of attributes and methods. Classes in Java encapsulate data and behavior, fostering the principles of encapsulation and abstraction.
Abstraction in Java: Abstraction in Java is achieved through abstract classes and interfaces. Abstract classes can have abstract methods (methods without a body) that must be implemented by their subclasses. Interfaces declare a set of methods that must be implemented by any class that implements the interface, promoting a higher level of abstraction.
Inheritance in Java: Java supports single and multiple inheritances through classes and interfaces. Subclasses in Java can inherit attributes and methods from a superclass using the extends keyword for classes and the implements keyword for interfaces. Inheritance enhances code reuse and allows the creation of specialized classes while maintaining a common base.
Polymorphism in Java: Polymorphism in Java is manifested through method overloading and overriding. Method overloading allows a class to define multiple methods with the same name but different parameters. Method overriding occurs when a subclass provides a specific implementation for a method that is already defined in its superclass. This enables the use of a common interface for different types of objects.
Final Thoughts:
Object-oriented programming and its concepts form the foundation of modern software development. Java, with its robust support for OOP, empowers developers to create scalable, modular, and maintainable applications. Understanding the principles of encapsulation, abstraction, inheritance, and polymorphism is crucial for harnessing the full potential of OOPs concepts in Java. As you continue your journey in software development, a solid grasp of these concepts will be invaluable in designing efficient and effective solutions.
#javascript#javaprogramming#java online training#oops concepts in java#object oriented programming#education#technology#study blog#software#it#object oriented ontology#java course
3 notes
·
View notes
Text
Computer Language
Computer languages, also known as programming languages, are formal languages used to communicate instructions to a computer. These instructions are written in a syntax that computers can understand and execute. There are numerous programming languages, each with its own syntax, semantics, and purpose. Here are some of the main types of programming languages:
1.Low-Level Languages:
Machine Language: This is the lowest level of programming language, consisting of binary code (0s and 1s) that directly corresponds to instructions executed by the computer's hardware. It is specific to the computer's architecture.
Assembly Language: Assembly language uses mnemonic codes to represent machine instructions. It is a human-readable form of machine language and closely tied to the computer's hardware architecture
2.High-Level Languages:
Procedural Languages: Procedural languages, such as C, Pascal, and BASIC, focus on defining sequences of steps or procedures to perform tasks. They use constructs like loops, conditionals, and subroutines.
Object-Oriented Languages: Object-oriented languages, like Java, C++, and Python, organize code around objects, which are instances of classes containing data and methods. They emphasize concepts like encapsulation, inheritance, and polymorphism.
Functional Languages: Functional languages, such as Haskell, Lisp, and Erlang, treat computation as the evaluation of mathematical functions. They emphasize immutable data and higher-order functions.
Scripting Languages: Scripting languages, like JavaScript, PHP, and Ruby, are designed for automating tasks, building web applications, and gluing together different software components. They typically have dynamic typing and are interpreted rather than compiled.
Domain-Specific Languages (DSLs): DSLs are specialized languages tailored to a specific domain or problem space. Examples include SQL for database querying, HTML/CSS for web development, and MATLAB for numerical computation.
3.Other Types:
Markup Languages: Markup languages, such as HTML, XML, and Markdown, are used to annotate text with formatting instructions. They are not programming languages in the traditional sense but are essential for structuring and presenting data.
Query Languages: Query languages, like SQL (Structured Query Language), are used to interact with databases by retrieving, manipulating, and managing data.
Constraint Programming Languages: Constraint programming languages, such as Prolog, focus on specifying constraints and relationships among variables to solve combinatorial optimization problems.
2 notes
·
View notes
Text
Mastering C++: The Complete Programming Guide from Beginner to Advanced

C++ isn't just another programming language. It’s the powerhouse behind many of the tools, games, apps, and operating systems we use every day. Whether you're dreaming of becoming a game developer, software engineer, or embedded systems expert, learning C++ can open doors to some of the most rewarding tech careers.
But where do you start? And how do you go from writing your first “Hello World” to building real-world applications?
Let’s walk through the journey of mastering this versatile language—and yes, you can do it even if you're a total beginner.
Why Learn C++ Programming in 2025?
If you're wondering whether C++ is still relevant in today’s tech world, the answer is a big YES. From performance-critical applications to systems-level software, C++ continues to dominate industries like:
Game Development (think Unreal Engine)
Operating Systems (Windows, parts of macOS and Linux)
Finance & Trading Platforms (where milliseconds matter)
Embedded Systems (IoT, automotive software, robotics)
Backend Programming (when speed and efficiency are vital)
Its flexibility and power give developers deep control over hardware and system resources, which is why it's still the go-to for high-performance applications.
Who Should Learn C++?
Whether you’re a college student, an aspiring developer, or someone looking to level up your programming skills, C++ is an excellent language to learn. Unlike some modern high-level languages, C++ teaches you the foundations of computing—like memory management, pointers, data structures, and algorithms.
This foundational knowledge will not only help you in C++ but also in other languages like Python, Java, or even Rust later on.
What You’ll Learn from a Quality C++ Course
The best way to learn C++ isn’t just reading textbooks or copying code snippets. You need a structured approach that starts from the basics and gradually builds up to advanced concepts—and teaches you to think like a programmer.
A great course will cover:
✅ Beginner Topics
Setting up your development environment (Windows/Mac/Linux)
Writing your first C++ program
Understanding variables, data types, and input/output
Mastering conditionals and loops
✅ Intermediate Topics
Working with functions and arrays
Diving into object-oriented programming (classes, inheritance, polymorphism)
File handling in C++
Exception handling and debugging
✅ Advanced Topics
Pointers, memory allocation, and reference variables
Templates and Standard Template Library (STL)
Data structures: linked lists, stacks, queues, trees
Advanced projects like calculators, games, and simulations
And what’s even better? You don’t need to search around the internet to find the right course. The entire learning journey is now bundled in one comprehensive online course.
Check out this must-have program: 👉 Learn C++ Programming - Beginner to Advanced !
This course not only teaches you how to code in C++ but also guides you to apply your skills in practical, real-world projects. It’s the ideal roadmap for anyone serious about programming.
How Long Does It Take to Learn C++?
Let’s be real—C++ has a steeper learning curve than some beginner languages like Python. But here’s the good news: with the right course and consistent practice, you can go from zero to job-ready in a few months.
Here’s a rough breakdown:
0–1 month: Master the basics (syntax, loops, conditionals)
2–3 months: Dive into object-oriented programming and functions
3–5 months: Tackle advanced topics like memory management, data structures, and real-world projects
Of course, your pace may vary. But the key? Stay consistent and build projects as you go. The more you code, the faster you'll learn.
What Makes This C++ Course Different?
There are tons of tutorials out there. So, why choose this one?
Because it’s designed to guide you through every stage, even if you’ve never written a line of code before. By the end of the course, you’ll not only understand how C++ works but also how to think like a software engineer.
What makes this course a favorite?
🎯 Clear, step-by-step explanations
💻 Practical coding exercises and quizzes
🧠 Real-world projects to test your skills
📜 Certification to add to your resume
🔁 Lifetime access so you can learn at your own pace
It’s more than just a video series—it’s a complete learning experience crafted for beginners and intermediate learners alike.
Ready to dive in? Start now with Learn C++ Programming - Beginner to Advanced !
Career Opportunities After Learning C++
Once you've got a solid handle on C++, the job possibilities are endless. Here are some roles where your skills will shine:
Software Developer
Game Programmer
Embedded Systems Engineer
Backend Developer
Robotics Programmer
Quantitative Analyst (Quant Developer)
Companies like Google, Microsoft, IBM, and Intel constantly look for developers with strong C++ skills. Plus, if you're planning to pursue competitive programming or crack coding interviews at FAANG companies, mastering C++ gives you a major edge.
Tips to Succeed While Learning C++
Here are some handy tips to make your C++ journey smoother:
Practice daily: Coding is a skill—you get better the more you do it.
Build small projects: Start with calculators, number games, or basic apps.
Don’t skip the hard parts: Concepts like pointers and memory management are tough but essential.
Join online forums or groups: Learning with others can help you stay motivated.
Keep revising: Going back and reviewing tricky topics can solidify your understanding.
And above all—be patient with yourself. Every programmer started where you are now.
Final Thoughts: Start Your C++ Journey Today
C++ might look intimidating at first glance, but once you get started, it’s actually fun and deeply rewarding. Whether you're coding games, controlling robots, or building applications from scratch, this language gives you the tools to create magic with code.
If you’re serious about becoming a confident, job-ready programmer, don’t miss out on this opportunity to learn C++ programming from beginner to advanced in a structured, hands-on way.
👉 Enroll today: Learn C++ Programming - Beginner to Advanced ! Take the first step towards your programming future. The journey starts with one click.
0 notes
Text
SortedSet contains() method in Java with Examples

The contains() method is used to check whether a specific element is present in the SortedSet or not. So basically it is used to check if a SortedSet contains any particular element. Syntax: boolean contains(Object element) Parameters: The parameter element is of the type of SortedSet. This is the element that needs to be tested if it is present in the set or not. Return Value: The method returns true if the element is present in the set else return False. Note: The contains() method in SortedSet is inherited from the Set interface in Java. Below program illustrate the Java.util.Set.contains() […]
0 notes
Text
Java in Action — Top 3 Use Cases

Software development is a dynamic industry that evolves promptly to deliver the best tech to businesses. The introduction of programming languages brought a significant change in the industry. This is because it allowed businesses to bring them online with the help of the internet.
One of the programming languages that was introduced is Java.
In the mid-1990s, Java’s inception took place, and since then, it has been a preferred choice of developers and businesses. Research shows that in 2024, Java ranked as the third most used programming language after Python and JavaScript.
Java is a highly adaptable language, ideal for developing scalable enterprise software and performance-driven mobile applications. This is because it is a flexible, reliable, and secure language.
In this blog, we’ll explore the top 3 Java use cases that highlight the language’s practical power in real-world scenarios. Let’s dive in!
Why Java?
There are certain reasons why Java is preferred to date, apart from its versatility. Let’s discuss them briefly:
1. Object-Oriented Programming (OOP): Java is based on OOP principles, which include concepts such as classes, objects, inheritance, encapsulation, and polymorphism. These principles facilitate the creation of code that is structured, reusable, and maintainable over time.
2. Platform Independence: Java follows the “write once, run anywhere” philosophy, allowing code to be compiled into bytecode. Once compiled into bytecode, Java programs are executed by the Java Virtual Machine (JVM), ensuring platform independence across systems like Windows, Linux, and macOS.
3. Simplicity: Java offers a simpler learning curve compared to C or C++, making it a popular choice for beginners. It avoids complex features like pointers and multiple inheritance, which enhances code readability and maintainability.
4. Robustness: Java’s built-in features, including automatic memory management, structured exception handling, and rigorous type checking, make it a trusted choice for enterprise software development.
5. Security: Java offers enhanced security through its bytecode verification, security manager, and lack of direct pointer access. This reduces the risk of memory or data corruption by malicious code.
6. High Performance: Java achieves high performance through its bytecode, JVM optimizations, and built-in features like garbage collection.
7. Multithreading: Java supports multithreading, allowing multiple tasks to run concurrently within a single program, which improves efficiency and responsiveness.
8. Distributed Computing: Java’s networking capabilities and support for distributed computing make it well-suited for building applications that operate across multiple computers on a network.
9. Dynamic: Java is considered a dynamic language because it supports features like dynamic proxies and reflection, allowing for flexible and adaptable code.
10. Interpreted: Java is an interpreted language, meaning the code is compiled into bytecode, which the JVM interprets at runtime. This enables Java code to run seamlessly across different platforms without modification.
11. Architecture-Neutral: Java’s architecture-neutral design enables it to run unmodified on any hardware and operating system.
Now that you know the features of Java, let’s discuss some of its use cases.
Top 3 Use Cases of Java
Here you will learn the use of Java in enterprise applications, Android applications, big data, and distributed systems. Let’s dive in.
Enterprise Application Development
Java stands as a dominant technology for building scalable, secure enterprise solutions. Whether it is a financial institution or a healthcare provider, Java is the cornerstone of numerous enterprise-critical systems.
Why Enterprises Choose Java?
Java’s power lies in its scalability, dependability, and comprehensive frameworks like Jakarta EE and Spring.
These tools empower developers to build modular, maintainable, and high-performance business applications.
Key Features of Java for Enterprises
Here are some features of Java that benefit enterprises:
JVM-based Scalability
Java’s robust architecture enables exceptional scalability, allowing applications to handle millions of transactions seamlessly. This is crucial for enterprises needing reliability and performance during peak loads.
Spring Boot and Spring Cloud
Spring Boot and Spring Cloud enable the creation of flexible and efficient microservices, promoting modular design and rapid deployment for easy application maintenance.
Comprehensive Security APIs
Java provides essential security APIs and enterprise-grade authentication tools to protect sensitive data and guard against threats, making security a top priority for enterprises.
Seamless Database Integration
JDBC, Hibernate, and JPA enable Java to efficiently connect with databases, allowing enterprises to dynamically manage data and perform complex queries for data-driven applications.
Real-World Examples
Banking Sector: Global banks like HSBC and Citibank use Java to power secure backend operations, fraud detection systems, and online transaction platforms.
Healthcare: Java is used for managing patient data, scheduling, and claims processing in HIPAA-compliant applications.
Retail and ERP: Java helps power large-scale inventory systems, logistics management, and customer service platforms.
Android Application Development
Java has held its ground in Android development despite the rise of Kotlin. Java has been the original language of Android app development, and thus it continues to facilitate numerous mobile applications globally.
Why Java for Android?
Here are some reasons why Java is used for Android:
Vast legacy codebases and rich libraries developed in Java underpin many critical systems.
Android SDK is largely Java-based.
Strong IDE support (e.g., Android Studio).
While Kotlin offers a modern syntax, Java is still the go-to for many developers, especially those new to mobile app development or maintaining existing apps.
Features of Java for Android
Memory Management: Java’s garbage collection helps maintain a smooth performance.
Exception Handling: Enhances error management to prevent application crashes.
Multithreading: It is essential for background tasks such as downloading files or syncing data.
Popular Java-Based Android Apps
Twitter (legacy versions): Java-powered backend and UI rendering.
Spotify: Music streaming giant used Java extensively during its Android development journey.
Big Data and Distributed Systems
Java plays a vital role in big data and distributed computing due to its performance, portability, and multithreading abilities. It acts as a keystone for many tools in the Big Data ecosystem.
Why Java in Big Data?
Many of the widely used big data libraries, like Apache Hadoop, Apache Spark, and Apache Kafka, are either written in Java or utilise Java APIs. This is because Java’s efficient memory handling and thread management make it suitable for processing huge data sets.
Features of Java for Big Data Systems
Concurrency support for handling parallel data streams.
Seamlessly integrates with big data technologies and NoSQL databases like Cassandra, HBase, and MongoDB.
Platform-independent for easy deployment in cloud-native environments.
Real-World Applications
Netflix: Uses Java for its real-time data ingestion and processing pipeline with Kafka and Apache Flink.
LinkedIn: Heavily relies on Java for handling billions of data events daily.
Telecom: Real-time call analysis and network monitoring platforms are often built using Java.
Conclusion
Java is the leading choice in modern development, from enterprise platforms to mobile apps and big data pipelines. Its ability to meet business and developer needs stems from its popularity. Moreover, it offers features like platform independence, security, scalability, and extensive libraries. Thus, whether required by a developer or a business, Java is a smart and future-ready programming language.
If you want to bring this versatile programming language to your business’s tech stack, contact IT companies like BestPeers. They will help you create seamless and functional web applications that are secure and scalable using Java.
0 notes
Text
Java Interview Questions and Answers: Your Ultimate Preparation Guide

That’s why we’ve created "Java Interview Questions and Answers: Your Ultimate Preparation Guide" to help you get fully prepared and stand out from the competition.
Java remains one of the most widely used programming languages across the tech industry. From building enterprise-grade applications to Android development and cloud-based systems, Java is a powerful, object-oriented language that has stood the test of time. As a result, Java continues to be a core requirement in thousands of job listings globally, and technical interviews often focus heavily on Java fundamentals, coding practices, and real-world problem-solving.
This guide offers a comprehensive breakdown of the most commonly asked Java interview questions, along with expert-level answers that explain not just the what, but the why—helping you build a strong conceptual foundation.
Why This Guide Matters
"Java Interview Questions and Answers: Your Ultimate Preparation Guide" is designed to equip you with the most relevant, up-to-date, and frequently asked questions across various job roles and experience levels. Whether you're a fresher just entering the field or a seasoned Java developer with years of experience, the questions included in this guide cover all the core areas expected in a Java interview.
With structured answers, real-world examples, and technical explanations, this guide helps you understand each topic in depth—so you’re not just memorizing, but truly learning.
Key Topics Covered in This Guide
Here are the primary categories of Java interview questions and answers covered in this ultimate preparation guide:
1. Core Java Basics
These questions test your fundamental knowledge of Java, including syntax, control structures, and data types. Examples include:
What are the main features of Java?
What is the difference between JDK, JRE, and JVM?
Explain the concept of platform independence in Java.
2. Object-Oriented Programming (OOP) in Java
As Java is built around the OOP paradigm, interviewers often assess your grasp of these principles:
What is encapsulation, and why is it important?
Explain inheritance with examples.
What is polymorphism, and how is it implemented in Java?
3. Exception Handling
Proper exception handling is critical in robust Java applications. Common questions include:
What is the difference between checked and unchecked exceptions?
How do try, catch, finally, and throw work together?
What is the purpose of custom exceptions?
4. Collections Framework
This is a favorite topic in Java interviews due to its practical importance:
What is the difference between ArrayList and LinkedList?
How does HashMap work internally?
What are the differences between Set, List, and Map?
5. Multithreading and Concurrency
Java supports concurrent programming, and questions in this category test your knowledge of threading concepts:
What is a thread in Java?
Explain the differences between Runnable and Thread.
How do you avoid thread-safety issues in Java applications?
6. Java 8 and Beyond
Modern Java versions introduced features like lambdas, streams, and functional programming:
What are lambda expressions?
How do you use the Stream API in Java 8?
What is the difference between Optional and null?
7. JVM Internals and Memory Management
Senior-level candidates are often expected to understand how Java works under the hood:
How does garbage collection work in Java?
What are the different memory areas in JVM?
How can memory leaks be detected and avoided?
8. Design Patterns and Best Practices
To demonstrate architectural thinking, candidates may be asked:
What is the Singleton pattern and how do you implement it?
Explain the Factory and Observer patterns.
What are SOLID principles in Java programming?
Sample Questions from the Guide
Here are a few samples from "Java Interview Questions and Answers: Your Ultimate Preparation Guide":
1: What is the difference between ‘==’ and .equals() in Java? Ans: == checks reference equality, meaning whether two references point to the same object. .equals() checks logical equality, meaning whether two objects have the same value. For example, two different String objects with the same value will return true using .equals() but false using ==.
2: What is a HashMap, and how does it work internally? Ans: A HashMap stores key-value pairs. It uses a hash function to compute an index where the value should be stored in an array. If multiple keys hash to the same index, Java handles collisions using a linked list or a balanced tree (as of Java 8).
3: How does Java achieve platform independence? Ans: Java code is compiled into bytecode by the Java compiler. This bytecode is platform-independent and can be executed by the Java Virtual Machine (JVM), which is available on multiple operating systems.
How to Use This Guide for Effective Interview Prep
To get the most out of "Java Interview Questions and Answers: Your Ultimate Preparation Guide", follow these steps:
Study the concepts – Don’t just read the answers; understand the reasoning behind them.
Practice coding – Use platforms like HackerRank, LeetCode, or Codeforces to apply Java in real coding problems.
Mock interviews – Simulate real interview scenarios with peers or mentors to practice verbalizing your thoughts.
Build small projects – Implement real-world solutions to solidify your understanding of Java concepts.
Keep learning – Stay up-to-date with Java updates and community discussions to stay ahead of the curve.
Conclusion
Preparation is key to succeeding in a Java interview, and "Java Interview Questions and Answers: Your Ultimate Preparation Guide" is your all-in-one resource for that journey. By mastering the topics covered in this guide, you'll gain the confidence and knowledge needed to impress your interviewers and secure your desired role in the tech industry.
0 notes
Text
Crack the Code: Why Learning Java Is Still One of the Smartest Career Moves in 2025
In a world of constantly changing tech trends—where Python, Kotlin, and JavaScript dominate discussions—Java continues to stand strong. And if you're a student or fresher looking to enter the tech industry, learning Java might just be your smartest investment yet.
Why? Because Java is everywhere. From Android apps to enterprise systems, banking software to back-end platforms—Java powers millions of applications used daily. And the demand for skilled Java developers isn't just staying steady; it's growing.
In 2025, Java remains a gateway to building a robust, long-lasting career in software development. And thanks to platforms like Beep, students now have access to hands-on, Java programming courses for beginners that are affordable, practical, and job-oriented.
Why Java Still Rules the Backend World
Some people wrongly assume Java is “old school.” But ask any senior developer, and you’ll hear the same thing: Java is battle-tested, secure, and versatile.
Here’s why companies continue to prefer Java:
Scalability: Perfect for high-traffic apps and large databases
Platform independence: “Write once, run anywhere” is still relevant
Community support: Millions of developers worldwide
Enterprise adoption: Banks, telecoms, logistics firms, and even startups love Java’s stability
Whether you're building a mobile app or designing a cloud-based ERP, Java offers the tools to scale and succeed.
What Makes Java Perfect for Beginners
You don’t need to be an expert to start with Java. In fact, many colleges use Java as a foundation for teaching object-oriented programming (OOP).
As a beginner, you’ll gain core skills that apply across languages:
Variables, data types, control structures
Classes, objects, inheritance, polymorphism
File handling, exception management
Basic UI development using JavaFX or Swing
Introduction to frameworks like Spring (as you advance)
This foundation makes it easier to switch to more specialized stacks later (like Android or Spring Boot) or even pick up other languages like Python or C#.
Where to Start Learning Java the Right Way
While YouTube and free tutorials are good for browsing, structured learning is better for job-readiness. That’s why Beep offers a beginner-friendly Java programming course that’s designed specifically for students and freshers.
What makes this course ideal:
It covers both basic and intermediate concepts
You build real-world projects along the way
You learn how Java is used in interviews and job scenarios
You get certified upon completion—great for your resume
It’s flexible and can be completed alongside college or internship schedules
And if you’re aiming for backend developer jobs, this certification is a strong step in the right direction.
How Java Helps You Land Jobs Faster
Hiring managers love candidates who know Java for one simple reason—it’s practical.
Java-trained freshers can apply for roles like:
Junior Software Developer
Backend Developer
QA Engineer (Automation Testing)
Android App Developer
Support Engineer (Java-based systems)
These roles often mention Java and SQL as core requirements, making it easier for you to stand out if you’ve completed a course and built some small projects.
Explore the latest jobs for freshers in India on Beep that list Java among the top preferred skills.
Build Projects, Not Just Skills
To truly master Java—and get noticed—you need to build and share your work. Here are some beginner-friendly project ideas:
Student registration portal
Simple inventory management system
Expense tracker
Quiz game using JavaFX
File encryption/decryption tool
Host these on GitHub and add them to your resume. Recruiters love seeing what you’ve created, not just what you’ve studied.
What About Java vs. Python?
This is a common question among freshers: Should I learn Java or Python?
The answer: learn based on your goals.
Want to work in data science or AI? Python is ideal.
Want to build robust applications, Android apps, or work in enterprise systems? Java is your best bet.
Also, once you understand Java, learning Python becomes easier. So why not start with the tougher but more rewarding path?
How to Prepare for Java Interviews
Once you’ve got the basics down and completed a project or two, start preparing for interviews with:
Practice problems on platforms like LeetCode or HackerRank
Study key Java topics: Collections, OOP principles, exception handling
Learn basic SQL (many Java jobs also require DB interaction)
Brush up on scenario-based questions
You can also check out Beep’s resources for interview prep alongside your course content.
Final Thoughts: Learn Once, Earn Always
Learning Java isn’t just about getting your first job—it’s about building a lifelong skill. Java has been around for over two decades, and it’s not going anywhere. From web to mobile to enterprise, Java developers are always in demand.
So if you're ready to start your tech journey, don't chase trends. Build a solid base. Start with the best Java course for beginners, practice consistently, and apply with confidence. Because a well-written Java application—and resume—can open more doors than you think.
0 notes
Text
From Novice to Java Pro: A Step-by-Step Learning Journey
Java programming, renowned for its platform independence and versatility, is a sought-after language in the world of software development. It's the language behind countless web applications, mobile apps, game development, and more. This blog serves as your comprehensive guide to embarking on a journey to learn and master Java programming. We'll walk you through the basics of programming, Java's syntax and structure, the essential tools and libraries, and the importance of practice.
The Path to Java Proficiency:
1. Understand the Basics of Programming:
Prior Experience: If you're new to programming, it's crucial to start with the foundational concepts. Variables, data types, control structures (if-else, loops), and functions are universal principles that you need to understand before delving into any programming language, including Java.
What to Learn: Begin by grasping the basics of programming logic and problem-solving. Familiarize yourself with concepts like variables (to store data), data types (to specify the kind of data), and control structures (to make decisions and loop through actions). These concepts serve as the building blocks for Java programming.
2. Java Syntax and Structure:
Prior Experience: As you delve into Java, you'll find that it's an object-oriented language with its unique syntax and structure. While prior programming experience is beneficial, it's not a prerequisite. You can start with Java as your first language.
What to Learn: Java introduces you to classes and objects. Classes are blueprints for creating objects, which are instances of classes. Java is known for its object-oriented nature, where you'll encounter concepts like inheritance (creating new classes based on existing ones), polymorphism (objects of different classes can be treated as objects of the same class), encapsulation (data hiding), and abstraction (simplifying complex reality by modeling classes based on real-world objects).
3. Get Your Hands on a Compiler and IDE:
Prior Experience: No prior experience is required to set up your Java development environment.
What to Learn: To start writing and running Java code, you'll need to install a Java Development Kit (JDK), which includes the Java compiler (javac). This kit allows you to compile your source code into bytecode that the Java Virtual Machine (JVM) can execute. Additionally, install an Integrated Development Environment (IDE) like Eclipse, IntelliJ IDEA, or NetBeans. These IDEs provide a user-friendly interface for coding, debugging, and managing your Java projects.
4. Learn the Java Standard Library:
Prior Experience: No specific prior experience is necessary, but familiarity with basic programming concepts is beneficial.
What to Learn: Java's power lies in its extensive standard library. This library includes pre-built classes and packages that simplify common programming tasks. For example, you can utilize classes in the java.io package to handle file input and output. You'll explore the java.lang package, which provides fundamental classes like String and Integer. Understanding this library is crucial for performing operations like file handling, string manipulation, and working with data structures.
5. Practice, Practice, Practice:
Prior Experience: No prior experience is required. This stage is suitable for beginners and those with basic programming knowledge.
What to Learn: The key to mastering Java or any programming language is practice. Apply the knowledge you've gained by working on small coding projects. Start with simple programs and gradually move on to more complex tasks. Solving programming challenges, such as those on websites like LeetCode or HackerRank, is an excellent way to put your skills to the test. Build simple applications to get hands-on experience in real-world scenarios.
6. Join Java Communities:
Prior Experience: No prior experience is needed to join Java communities.
What to Learn: Engage with Java communities and forums, such as Stack Overflow or Reddit's r/java. These platforms provide a space to ask questions, seek advice, and learn from experienced Java developers. You can also contribute by answering questions and sharing your knowledge.
7. Enroll in Java Courses:
Prior Experience: Enrolling in Java courses is suitable for learners of all levels, from beginners to advanced users.
What to Learn: ACTE Technologies offers comprehensive Java training programs that cater to a diverse range of learners. These programs are designed to provide hands-on experience and real-world examples, ensuring that you gain practical skills in Java programming.
In your journey to master Java programming, structured training plays a pivotal role. ACTE Technologies is a trusted partner, offering Java training programs for learners at all levels. Whether you're a beginner taking your first steps in Java or an experienced programmer seeking to expand your skill set, they can provide the guidance and resources needed to excel in the world of Java development. Consider exploring their Java courses to kickstart or enhance your Java programming journey. Java programming is a versatile and essential language, and mastering it is a rewarding journey. With dedication, practice, and structured training, you can become a proficient Java developer. Institutions like ACTE Technologies offer valuable resources and courses to accelerate your learning process, making your journey to Java mastery even more efficient.
7 notes
·
View notes
Text
Mastering Java: Key Concepts for Intermediate and Advanced Learners
If you're enrolled in java full stack training in Hyderabad, it's important to move beyond the basics and explore Java’s more advanced features. Mastering these key concepts will help you build strong, efficient, and professional applications that meet real-world industry demands.
1. Deep Understanding of OOP (Object-Oriented Programming)
Java is built on OOP principles. At the intermediate and advanced levels, you should know how to apply:
Abstraction – A class or interface can be abstracted to hide implementation details.
Encapsulation – protecting data by making variables private and using getter/setter methods.
Inheritance – allowing one class to inherit from another to reuse code.
Polymorphism – writing one method that behaves differently based on object type.
2. Exception Handling and Generics
Good Java developers write code that handles errors gracefully. You should be able to:
Use try-catch-finally blocks and create custom exceptions.
Work with Generics to make your code more flexible and type-safe, especially when dealing with collections.
3. Collections and Stream API
Java’s Collections Framework (like ArrayList, HashMap, HashSet) is essential for handling data. With Streams and Lambda expressions, you can process data more efficiently and write shorter, cleaner code.
4. Multithreading and Concurrency
Advanced Java includes running multiple tasks at the same time (multithreading). Java provides tools like Thread, ExecutorService, and Future to build responsive and scalable applications.
Conclusion
Mastering these core concepts is essential to becoming a Java developer. If you're looking for expert guidance and hands-on training, Monopoly IT Solutions Pvt. Ltd. offers the best industry-focused programs in Hyderabad to help you grow your Java career with confidence.
#java full stack training#java full stack training in hyderabad#java full stack training in kphb#java full stack developer training
0 notes
Text
Java Developer Roadmap: How to Become a Professional in 6 Months
If you're already building a strong tech foundation at the Best Dot Net Training Institute in Hyderabad, you’re in a great position to expand your skills. Java remains one of the most in-demand programming languages in 2025, and with the right roadmap, you can become a professional Java developer in just six months.
Month 1: Core Java Basics
Start with understanding the fundamentals—data types, loops, conditionals, arrays, and object-oriented programming (OOP) concepts like classes, objects, inheritance, and polymorphism. Practice writing simple programs daily.
Month 2: Advanced Java Concepts
Move on to more complex topics like exception handling, collections framework, generics, multithreading, and file I/O operations. Build small projects like a student management system or a calculator.
Month 3: JDBC and Database Integration
Learn how to connect Java applications with databases using JDBC (Java Database Connectivity). Practice CRUD operations with MySQL or Oracle databases.
Month 4: Web Development with Servlets and JSP
Dive into web technologies. Learn how to create dynamic web applications using Servlets, JSP (JavaServer Pages), and basic HTML/CSS. This will help you understand client-server architecture.
Month 5: Frameworks – Spring and Hibernate
Master popular frameworks like Spring Core, Spring Boot, and Hibernate ORM. These are essential for real-world enterprise applications and will make your profile job-ready.
Month 6: Build Projects and Prepare for Interviews
Create at least 2–3 full-stack projects, such as a library management system or an e-commerce app. Simultaneously, start preparing for technical interviews by practicing DSA and common Java questions.
Conclusion
Becoming a professional Java developer in six months is possible with the right focus and guidance. To accelerate your journey, consider training at SSS IT Computer Education, where practical learning meets expert mentorship.
0 notes
Text
Boost Your Tech Career with a Comprehensive Java Training and Internship Program
In today’s fast-paced digital world, programming skills are more than just an added advantage—they are essential. Among the myriad programming languages, Java stands out as one of the most robust, versatile, and in-demand languages used across industries, from mobile apps to enterprise-level software. If you're a student, fresher, or even a working professional looking to transition into software development, enrolling in a Java training and internship program can be your golden ticket to a successful IT career.
Why Learn Java?
Java is one of the oldest and most powerful programming languages still actively used today. It runs on billions of devices and serves as the backbone of many enterprise systems, Android apps, web servers, and more. Here's why Java continues to be a top choice for developers and organizations:
Platform Independence: Java is a "write once, run anywhere" language, meaning code written in Java can run on any system with a Java Virtual Machine (JVM).
Strong Community Support: With millions of developers worldwide, help, documentation, and updates are always accessible.
Versatile Applications: From banking software to gaming applications and Android development, Java is everywhere.
High Demand: Companies across the globe constantly look for skilled Java developers, making it a highly employable skill.
What is a Java Training and Internship All About?
A Java training and internship is a structured learning program designed to teach both foundational and advanced concepts in Java programming, followed by real-time industry exposure through internships.
This program typically includes:
In-Depth Java Training: Covering topics such as Core Java, OOPs (Object-Oriented Programming), Collections Framework, Exception Handling, Multithreading, JDBC, and GUI programming.
Advanced Java Concepts: Including Servlets, JSP (JavaServer Pages), Spring Framework, Hibernate, RESTful APIs, and Microservices.
Project Development: Hands-on development of live projects to implement what you’ve learned.
Internship Experience: Working with a tech company or development team to gain practical, real-world experience.
Certification: A recognized certificate to showcase your skills to future employers.
Key Skills You’ll Learn
Participating in a Java training and internship program can help you master several critical skills that are valuable in the IT industry. These include:
1. Programming Fundamentals
You will start with understanding how Java works, its syntax, data types, variables, operators, and flow control statements.
2. Object-Oriented Programming (OOP)
Java is an object-oriented language. You'll learn about classes, objects, inheritance, polymorphism, encapsulation, and abstraction—concepts that form the base of modern software development.
3. Database Integration
Using JDBC and ORM tools like Hibernate, you’ll learn how Java applications interact with databases such as MySQL, Oracle, or PostgreSQL.
4. Web Development
You'll gain skills in building Java-based web applications using Servlets, JSP, and frameworks like Spring MVC or Spring Boot.
5. Debugging and Testing
Understand how to debug Java applications, write unit tests using JUnit, and ensure that your code is reliable and efficient.
6. Project Management Tools
Gain experience using Git, GitHub, Maven, and other tools commonly used in development environments.
Benefits of Joining a Java Training and Internship Program
Enrolling in such a program offers multiple advantages beyond just learning how to code.
✅ Structured Learning
The course is designed by experts and follows a curriculum that gradually takes you from beginner to advanced level.
✅ Hands-on Experience
Working on live projects and real-world problems enhances your problem-solving ability and builds your portfolio.
✅ Professional Mentorship
Access to experienced mentors and instructors helps clarify doubts, understand complex topics, and receive valuable career guidance.
✅ Resume Building
The internship experience, along with a certificate of completion, significantly boosts your resume and makes you stand out to employers.
✅ Job Opportunities
Many internship programs have placement assistance or allow top performers to get absorbed into the company itself.
Who Should Join?
This program is suitable for:
Students in B.Tech/BCA/MCA or other CS-related streams who want to strengthen their programming base.
Fresh graduates looking to land their first job in software development.
Working professionals aiming to shift from non-tech roles or other languages into Java-based development.
Freelancers and entrepreneurs who want to build Java-based applications independently.
What a Typical Training and Internship Program Includes
While the details may vary by provider, a good Java training and internship program typically offers:
Component
Details
Duration
8 to 16 weeks
Mode
Online / Offline / Hybrid
Learning Hours
2-3 hours/day or weekend batches
Tools Covered
IntelliJ IDEA, Eclipse, GitHub, MySQL, Maven
Projects
E-commerce website, Online Exam Portal, Chat App, etc.
Certification
Yes – with performance evaluation
Placement Support
Resume building, mock interviews, job referrals
How to Choose the Right Program?
When selecting a Java training and internship program, consider the following:
Reputation of the Institution: Choose a training center or ed-tech platform with positive reviews and a proven track record.
Curriculum Quality: Ensure that the course covers both Core and Advanced Java, along with real-time projects.
Internship Opportunities: Look for programs that offer internships with reputed companies.
Post-training Support: Some programs offer job placement help or career counseling—this is a big plus.
Affordability: Check if the program is within your budget and whether it offers EMI or scholarship options.
Real Career Impact
Those who complete the training and internship successfully often find career paths such as:
Java Developer
Backend Developer
Android Developer (Java-based)
Software Engineer
Full Stack Developer
Technical Consultant
With time and experience, you can grow into roles like Team Lead, Solution Architect, or even Product Manager in IT companies.
Final Thoughts
Investing your time and effort in a structured learning program can transform your career path. Java is more than just a programming language—it's a career catalyst. Whether you want to build enterprise software, Android applications, or simply add a valuable skill to your resume, a Java training and internship program provides the knowledge, experience
0 notes
Text
Unlock Your Programming Potential with the 2025 Core JAVA Bootcamp from Zero to Hero!!

If you’ve ever felt that learning to code in Java was too intimidating or just didn’t know where to begin, we’ve got good news. There’s now a clear, exciting, and structured path to mastering one of the world’s most in-demand programming languages — and you don’t need a tech degree to get started.
Whether you’re looking to launch a career in software development, build Android apps, or simply learn how programming works, the 2025 Core JAVA Bootcamp from Zero to Hero !! is your perfect launchpad.
Let’s explore what makes this bootcamp your best bet for mastering Java and transforming your tech journey in 2025.
Why Java? Why Now?
Java is everywhere. From enterprise software powering Fortune 500 companies to Android apps on billions of devices, Java remains a foundational technology across industries. It’s trusted, flexible, and incredibly versatile.
Still not convinced? Here are a few compelling reasons to dive into Java in 2025:
High Demand for Java Developers: Companies around the globe continue to seek skilled Java developers for both backend and full-stack roles.
Strong Earning Potential: Java developers are among the top-paid software engineers globally.
Massive Community Support: With millions of Java developers worldwide, help is always a click away.
Platform Independence: Java runs on everything from laptops to game consoles, thanks to its “write once, run anywhere” design.
Learning Java in 2025 isn’t just relevant — it’s a smart career move.
What Makes the 2025 Core JAVA Bootcamp Stand Out?
Let’s be real. There are thousands of Java tutorials floating around. Some are outdated. Some are too basic. Others are too complex. What you need is a structured, well-paced, hands-on course that takes you from zero to hero — without the fluff.
That’s where the 2025 Core JAVA Bootcamp from Zero to Hero !! shines.
Here’s why:
✅ Beginner-Friendly Start
This bootcamp assumes no prior coding experience. You’ll start from the very basics — understanding what Java is, how to install it, and writing your very first “Hello World” program.
✅ Hands-On Projects
Theory is good. Practice is better. This bootcamp is packed with real-world mini-projects and coding challenges that help cement every concept.
✅ Up-to-Date Curriculum
Java evolves — and so does this course. The curriculum is aligned with the latest industry practices, including updates for Java 17 and beyond.
✅ Learn at Your Own Pace
Whether you’re a busy professional, student, or a stay-at-home parent making a career switch, the course fits your schedule. You control the pace.
✅ Lifetime Access
Once you enroll, you get lifetime access, meaning you can revisit lessons anytime — especially helpful during job interviews or future projects.
What You’ll Learn Inside the Bootcamp
Here's a peek into what you'll master during the bootcamp:
Java Basics: Data types, variables, operators, and control flow
Object-Oriented Programming: Classes, objects, inheritance, polymorphism, encapsulation, and abstraction
Error Handling: Try-catch blocks, custom exceptions, and debugging tips
Collections Framework: Lists, Sets, Maps, and the power of generics
File I/O and Streams: Reading and writing data like a pro
Multithreading: Understand concurrency and build efficient applications
Java 8+ Features: Lambda expressions, streams API, and functional programming
Simple Game Development: Put theory into practice with a beginner-friendly game project
Database Connectivity (JDBC): Connecting Java apps with MySQL databases
Mini Projects: Including a calculator app, to-do list, contact manager, and more
The 2025 Core JAVA Bootcamp from Zero to Hero !! takes you from baby steps to building your own Java applications — confidently and independently.
Who Should Take This Course?
This bootcamp is designed with clarity and community in mind. You’ll feel guided, supported, and empowered no matter your background.
It’s perfect for:
📌 Absolute beginners with no programming experience
📌 College students looking to level up their skills
📌 Working professionals exploring a career in software development
📌 Aspiring Android developers
📌 Anyone who wants to build a solid foundation in Java
Benefits Beyond the Code
Here’s the thing — it’s not just about learning Java. It’s about building confidence, opening career doors, and future-proofing your skill set.
✅ Boost Your Resume
Adding Java and project work to your portfolio makes you instantly more attractive to employers. You'll not only speak their language — you’ll code in it.
✅ Prep for Interviews
The bootcamp covers essential Java topics often asked in coding interviews. You’ll be better prepared for technical rounds, coding challenges, and whiteboard sessions.
✅ Launch Freelance Work
Once you complete the course, you’ll be ready to take on small Java projects — offering your services on freelance platforms or building your own apps.
Success Stories from Previous Students
Past learners of this course have gone on to land developer jobs, crack interviews at top tech firms, and even start their own freelance businesses.
Here’s what students are saying:
“I came from a non-technical background, but this course made learning Java surprisingly easy. The examples were practical, and I now feel confident enough to apply for Java developer roles.” — Riya M.
“The project-based approach really helped. I didn’t just watch videos — I built things, and that’s what made the knowledge stick.” — Daniel T.
You could be the next success story.
Learning Java in 2025: The Right Time Is Now
There’s never been a better time to start learning Java:
🚀 The job market is booming for Java developers 📈 Companies are seeking scalable, backend solutions — and Java is the answer 🤖 Technologies like machine learning, big data, and enterprise cloud still lean on Java-based solutions
Whether you’re learning for fun, career growth, or personal satisfaction — the 2025 Core JAVA Bootcamp from Zero to Hero !! has the roadmap to take you there.
What You’ll Need to Get Started
Nothing fancy. Just:
A laptop or desktop
Internet connection
A curious mind
A willingness to learn and practice
You don’t need any prior programming experience — just a desire to grow and an hour or two each day to dedicate to learning.
Let’s Talk About Certification
On completion, you’ll receive a certificate that you can showcase on LinkedIn, your resume, or even frame on your wall.
While certification is just one part of the equation, it’s a great way to validate your skills — especially when applying for jobs or bidding on freelance gigs.
What’s the Investment?
Here’s the best part — you don’t need to spend thousands of dollars or attend a coding bootcamp in-person.
You get access to all this learning — plus future updates, support, and community — at a fraction of the cost.
And remember: it’s not just an expense, it’s an investment in your future.
Final Thoughts: You’re Closer Than You Think
Learning to code in Java doesn’t have to be confusing or frustrating. With the right course, guidance, and practice, anyone can learn — including you.
The 2025 Core JAVA Bootcamp from Zero to Hero !! breaks it down into digestible lessons, real-life projects, and fun challenges — making it easy and enjoyable to stay motivated and on track.
So if you’re ready to finally conquer Java and take a big step toward your tech career or passion project, this is your moment.
👉 Start your journey from Zero to Hero today.
0 notes
Text
Find the Best C++ Course Online with Certification and Easy to Follow Lessons

If you want to learn computer programming from the very beginning, then doing a C++ programming course can be a really good step. C++ is one of those programming languages that has been around for a long time and is still used in many areas like making computer software, designing games, and even building robots.
In this blog, you will get to know everything in detail about the C++ course — why it is useful, what topics you will study in it, what job options are available after learning it, and how you can begin learning it using the right tools and support.
What is a C++ Programming Course?
A C++ programming course is a learning program that helps you understand how to create computer programs using the C++ language. It is specially made for those who are new to programming or those who already know a little and want to learn more.
In this course, you will be taught how to write simple codes, find solutions to programming problems, and understand the basic ideas of how object-based programming works — which is a way of building programs by using small blocks called “objects.”
Why should you learn C++ in 2025?
Choosing to learn C++ in 2025 is a really good idea for several simple reasons. C++ is still used a lot by many companies. Big companies pick C++ when they want to make software that works quickly and does not break easily. If you want a job in IT, making software, or even working with artificial intelligence, knowing C++ will help you stand out. Also, C++ is the starting point for many other programming languages. After you learn C++, it becomes much simpler to pick up other languages like Java or Python.
Who can join a C++ Programming Course?
If you like computers or want to learn about technology, you can join a C++ programming course. You do not need to be a computer expert or have any special background. Many students take a C++ course after finishing class 12 because it helps them start learning programming early. People who already have jobs but want to learn new things or change their career can also join a C++ course.
If you are a student, you can find C++ courses made just for students, so learning is easy for you. There are also many good C++ courses online in 2025 for all levels, whether you are just starting or already know some programming.
What Will You Learn in a C++ Course? (Syllabus Overview)
When you join a C++ course made for beginners, you will learn all the main things that help you start coding easily. Here’s what is usually taught in such a course:
A simple explanation of what C++ is and where it came from
How to install and start using the C++ software on your computer
Writing your first basic program in C++
Learning about variables, data types, and math symbols (operators)
How to use loops and “if-else” conditions to make decisions in code
What functions are, and how to use them in different programs
Understanding arrays, how to use strings, and what pointers do
Learning the basics of object oriented programming in C++, like how classes and objects work, and things like inheritance and polymorphism
How to work with files, and how to handle errors in programs
Doing small projects and exercises to check what you’ve learned
Most C++ courses also come with a C++ programming tutorial step by step, which means you don’t just read — you also practice. This kind of hands-on method helps you remember and understand better.
Career Opportunities After Learning C++
When you finish a C++ programming course, you will have many job options. C++ is used in lots of different fields, so you can find work in many kinds of jobs. Some common jobs you can get are:
Software Developer
Game Developer
System Programmer
Embedded Systems Engineer
Data Analyst
Robotics Programmer
Having a job in C++ programming is safe and can help you grow in your career. Many companies want people who know C++. You can also choose to work for yourself as a freelancer or even start your own software business.
C++ Course Duration and Fees
How long a C++ course takes and how much it costs depends on where you study and which course you pick. Most beginner courses are between 1 and 3 months long. If you go for a bigger or more advanced course, it might take more time. The fees are different for each course. Some websites let you learn the full C++ course in English for free, but some may ask for a small payment for a C++ certification course.
It is smart to look at different courses and see which one is best for your budget and the way you like to learn. Many places also give discounts or scholarships to students.
Best Tools and Software for Practicing C++
If you want to practice C++, you should use some good tools. Here are some well-known choices:
Code::Blocks: This tool is friendly for people who are just starting. It is not hard to use.
Dev C++: This one is simple and does not use much space on your computer.
Visual Studio: This software is strong and many experts use it for their work.
Online compilers: There are websites like Online GDB and Repl.it where you can write and check your C++ code online. You do not have to put any software on your computer to use these sites.
With these tools, you can easily learn from a C++ programming tutorial step by step and practice everything you study.
Expected Salary After Completing a C++ Course
A big reason many people pick a C++ programming course is because they can get a good salary. After you finish your course, your starting pay is often more than many other jobs. In India, if you are new, you can earn about ₹3 to ₹6 lakhs each year. If you have more experience, you can get paid even more.
If you get a job in another country, your salary can be much higher. As you keep working and learning, your pay will go up when you get bigger and better jobs. After a C++ course, you can find jobs in both private companies and government offices.
Tips and Resources to Learn C++ Easily
If you want to learn C++ in a simple way, try these tips:
- Try to write code every day, even if you only have 30 minutes. - Watch simple video lessons and do the steps shown in the videos. - Join online groups or forums where you can ask questions and talk about what you are learning. - Work on small, easy projects to use what you have learned. - Do quizzes and small tests to see how much you understand.
There are lots of helpful things online, like a C++ full course in English, video lessons, and e-books. You can also learn C++ online for free and get a certificate from good websites.
How to Join a C++ Programming Course with IID
If you want to start learning C++ and are searching for a good place, the Institute for Industrial Development (IID) is a very good option. IID has a C++ certification course that is made for beginners and teaches you all the important things you need to know. You can sign up for the course online, join live classes, and get help from expert teachers. IID gives you study materials, assignments to practice, and a certificate that is recognized when you finish the course. You can learn at your own speed with IID, and if you ever have questions or need help, support is always available. This makes learning C++ with IID easy and comfortable.
Conclusion
In 2025, taking a C++ programming course is still a great way to begin a career in technology. C++ is not hard to learn, many companies need people who know it, and it can help you get many different jobs. It does not matter if you are a student, someone working, or just interested in programming-learning C++ will give you a strong base for your future. There are lots of resources and online courses you can use, so now is the perfect time to start learning C++. Take the first step, join a course, and open up many new opportunities for yourself with C++.
#c++#c++ programming#programming languages#c language#learningc++#prgrammingcourse#career#industrialcourse#businesscourses#businesscouse#professionalcourses#entrepreneurcourse#iidcourses#onlinecourse#onlinecourses#professionalcourse
0 notes