#Base UI Component Structure
Explore tagged Tumblr posts
Text
For the #PlayStation7 UI framework, we can start with a skeleton of the structure that allows for intuitive navigation, personalization, accessibility features, interactive tutorials, and feedback mechanisms. This foundation will give us a clear and modular layout for managing and expanding each component.
I’ll break down each area and propose a code structure for a scalable and flexible UI system. Since this is for a game console, it would likely be built using a high-performance front-end framework like React or Unity UI Toolkit if we want C# for seamless integration. I’ll draft this in React with TypeScript for scalability and reusability.
1. Base UI Component Structure
The UI can be organized in a hierarchy of components that handle each aspect:
// App.tsx - Main entry point
import React from 'react';
import { NavigationMenu } from './components/NavigationMenu';
import { Personalization } from './components/Personalization';
import { Accessibility } from './components/Accessibility';
import { Tutorials } from './components/Tutorials';
import { Feedback } from './components/Feedback';
export const App: React.FC = () => {
return (
<div className="ps7-ui">
<NavigationMenu />
<Personalization />
<Accessibility />
<Tutorials />
<Feedback />
</div>
);
};
Each component would encapsulate its own logic and state, allowing for modular updates and improved maintainability.
2. Intuitive Navigation
The NavigationMenu component could use React Router to manage routes for easy navigation between games, apps, and settings. Quick access can be achieved using a menu structure that includes hotkeys or icons.
// components/NavigationMenu.tsx
import React from 'react';
import { Link } from 'react-router-dom';
export const NavigationMenu: React.FC = () => {
return (
<nav className="navigation-menu">
<Link to="/home">Home</Link>
<Link to="/games">Games</Link>
<Link to="/apps">Apps</Link>
<Link to="/settings">Settings</Link>
</nav>
);
};
3. Personalization Options
The Personalization component could offer theme and layout options. We can use context for storing and accessing user preferences globally.
// components/Personalization.tsx
import React, { useState, useContext } from 'react';
import { UserContext } from '../context/UserContext';
export const Personalization: React.FC = () => {
const { userPreferences, setUserPreferences } = useContext(UserContext);
const handleThemeChange = (theme: string) => {
setUserPreferences({ ...userPreferences, theme });
};
return (
<div className="personalization">
<h3>Customize Your Experience</h3>
<select onChange={(e) => handleThemeChange(e.target.value)}>
<option value="dark">Dark</option>
<option value="light">Light</option>
</select>
</div>
);
};
4. Accessibility Features
The Accessibility component includes support for voice commands, color blindness options, and mobility support. We can add accessibility settings to UserContext.
// components/Accessibility.tsx
import React, { useContext } from 'react';
import { UserContext } from '../context/UserContext';
export const Accessibility: React.FC = () => {
const { accessibilityOptions, setAccessibilityOptions } = useContext(UserContext);
const toggleVoiceCommands = () => {
setAccessibilityOptions({ ...accessibilityOptions, voiceCommands: !accessibilityOptions.voiceCommands });
};
return (
<div className="accessibility">
<h3>Accessibility Settings</h3>
<label>
Voice Commands
<input type="checkbox" checked={accessibilityOptions.voiceCommands} onChange={toggleVoiceCommands} />
</label>
</div>
);
};
5. Interactive Tutorials
The Tutorials component will guide new users through the setup. You can create individual tutorial steps as components or objects within an array.
// components/Tutorials.tsx
import React, { useState } from 'react';
export const Tutorials: React.FC = () => {
const [currentStep, setCurrentStep] = useState(0);
const tutorialSteps = [
"Welcome to PlayStation 7",
"How to Navigate",
"Personalization Settings",
"Feedback and Support",
];
return (
<div className="tutorials">
<h3>{tutorialSteps[currentStep]}</h3>
<button onClick={() => setCurrentStep((prev) => (prev + 1) % tutorialSteps.length)}>Next</button>
</div>
);
};
6. Feedback Mechanisms
The Feedback component enables users to submit feedback. This could involve a backend integration for storing data and using WebSocket for real-time updates.
// components/Feedback.tsx
import React, { useState } from 'react';
export const Feedback: React.FC = () => {
const [feedback, setFeedback] = useState("");
const handleSubmit = () => {
// Logic to send feedback to the server
console.log("Feedback submitted:", feedback);
};
return (
<div className="feedback">
<h3>Give Us Your Feedback</h3>
<textarea value={feedback} onChange={(e) => setFeedback(e.target.value)} />
<button onClick={handleSubmit}>Submit</button>
</div>
);
};
7. Context for Global State
A UserContext can hold user preferences, accessibility settings, and other globally accessible states, making the app reactive and user-tailored.
// context/UserContext.tsx
import React, { createContext, useState } from 'react';
interface UserPreferences {
theme: string;
layout: string;
}
interface AccessibilityOptions {
voiceCommands: boolean;
colorBlindMode: boolean;
}
interface UserContextProps {
userPreferences: UserPreferences;
setUserPreferences: React.Dispatch<React.SetStateAction<UserPreferences>>;
accessibilityOptions: AccessibilityOptions;
setAccessibilityOptions: React.Dispatch<React.SetStateAction<AccessibilityOptions>>;
}
export const UserContext = createContext<UserContextProps>({} as UserContextProps);
export const UserProvider: React.FC = ({ children }) => {
const [userPreferences, setUserPreferences] = useState<UserPreferences>({ theme: 'dark', layout: 'grid' });
const [accessibilityOptions, setAccessibilityOptions] = useState<AccessibilityOptions>({ voiceCommands: false, colorBlindMode: false });
return (
<UserContext.Provider value={{ userPreferences, setUserPreferences, accessibilityOptions, setAccessibilityOptions }}>
{children}
</UserContext.Provider>
);
};
Wrapping Up
By organizing the code into components and using context, this framework can be expanded for future updates and personalized settings, all without sacrificing modularity. This structure allows each branch of the UI to evolve independently, providing a scalable, interactive, and customizable experience for #PlayStation7 users.
Would you like to explore one of these sections in even more detail, or should I add more code to specific functionalities?
0 notes
Text
React Native Auto Code Application Development: The Smart Way to Build Mobile Apps
Introduction to React Native Auto Code Application Development
React Native auto code application development is changing the way mobile apps are built. Developers now use intelligent automation tools to generate code quickly and accurately. This speeds up development, reduces errors, and improves the overall quality of applications.
Auto code development with React Native is ideal for startups, agencies, and enterprises aiming to deliver high-quality apps fast.
youtube
Benefits of React Native Auto Code Application Development
1. Speed Up Development Cycles Automation tools handle repetitive tasks instantly. Developers can focus on building unique features rather than wasting time on boilerplate code.
2. Maintain Consistent Code Quality Auto-generated code follows consistent standards. This consistency improves code readability and eases future maintenance.
3. Reduce Human Errors By automating setup and structure, auto code tools minimize the chances of introducing bugs during the initial stages.
4. Enhance Developer Productivity Developers spend more time solving real problems and less time writing repetitive components.
5. Cost-Effective App Creation Faster development with fewer mistakes leads to lower project costs. This allows businesses to scale faster without ballooning budgets.
Popular Tools for React Native Auto Code Development
Ignite CLI Ignite offers pre-configured templates and plugins, making it easy to set up scalable projects instantly.
Hygen Hygen is a simple yet powerful code generator that helps teams maintain consistent coding standards with minimal setup.
Plop.js Plop.js allows developers to create custom generators for components, screens, and more, saving hours on manual coding.
Draftbit Draftbit provides a visual builder for React Native apps. Developers can create screens visually and export production-ready code.
Steps to Implement Auto Code in Your React Native Project
Step 1: Select the right auto code tools based on your project size and complexity. Step 2: Install and configure the tools within your development environment. Step 3: Create templates for commonly used components and screens. Step 4: Generate code structures automatically with simple commands. Step 5: Customize and enhance the generated code to meet unique business needs.
Following a structured approach ensures that automation becomes an asset, not a liability.
Challenges of Auto Code Application Development
1. Initial Learning Curve Some auto code tools require initial time investment for setup and training.
2. Over-Template Dependency Too much reliance on templates can sometimes limit creativity and flexibility.
3. Need for Regular Updates Auto code tools need constant updates to stay compatible with the latest React Native versions.
Developers can overcome these challenges with proper training and proactive tool management.
Best Practices for React Native Auto Code Development
- Use Automation for Repetitive Tasks Only Rely on auto code tools for repetitive elements but custom-build critical business logic manually.
- Keep Templates Updated Ensure all code generation templates are updated regularly to match new coding practices.
- Conduct Regular Code Reviews Even auto-generated code should undergo strict code reviews to maintain quality standards.
- Train Developers Continually Keep teams updated with the latest automation practices and tool updates for maximum efficiency.
Future of React Native Auto Code Application Development
The future points toward more AI-driven automation. Smart assistants will suggest code, build UI components, and even test functionalities automatically. React Native developers who embrace automation today will gain a competitive edge tomorrow.
Innovation combined with automation will define the next era of mobile app development.
Conclusion
React Native auto code application development offers a smarter, faster, and more efficient path to building world-class mobile apps. It helps developers minimize repetitive work, deliver projects quicker, and ensure consistent quality across platforms.
By integrating automation thoughtfully, businesses can create better apps and reach the market faster. React Native auto code development isn't just the future—it's the present.
Read More:
Ai Auto code
AI Wave maker
Rapid low code application development platform
Low code platform Enterprise software for application development
Low code application development platform or Low code platform for application development
What is Low code app development platforms
Composable low code isvs
Java-based low code platform
Composable isvs
RAD studio-Rapid application development software platform
APAAS-application platform as a service
Cloud Low code application development platform
Legacy application modernization solutions
React-native cross-platform mobile application development platform
Compare Wavemaker vs Outsystems vs mendix vs power apps — low code alternatives and its pricing
New and fast application development platform
Rapid application development model or RAD model
Low-code for consumable Banking and financial Low-code platform solutions
Internal api vs external apis
Rapid application development vs SDLC Platform
Custom Enterprise low code application development platform
Legacy enterprise application Modernization Platform
Embedded banking and Finance, Low-Code and the Emerging Face of Adaptability
BAAS- Low code Banking as a service
Composable Low code banking solutions
Telecom low code platform
Alternative to Xamarin and Cordova
Wavemaker Low code
Legacy application modernization platform
Cross-Platform React Native Mobile App Development Platform
2 notes
·
View notes
Text
What’s new in React?
React is a continuously evolving library in the ever-changing web development landscape. As you embark on your journey to learn and master React, it’s important to understand the evolution of the library and its updates over time.
One of the advantages of React is that its core API has remained relatively stable in recent years. This provides a sense of continuity and allows developers to leverage their knowledge from previous versions. The conceptual foundation of React has remained intact, meaning that the skills acquired three or five years ago can still be applied today. Let’s take a step back and trace the history of React from its early versions to the recent ones. From React 0.x to React 18, numerous pivotal changes and enhancements have been made as follows: 1. React 0.14: In this version, the introduction of functional components allowed developers to utilize functions as components, simplifying the creation of basic UI elements. At that time, no one knew that now we would write only functional components and almost completely abandon class-based components.
2. React 15: With a new versioning scheme, the next update of React 15 brought a complete overhaul of the internal architecture, resulting in improved performance and stability.
3. React 16: This version, however, stands as one of the most notable releases in React’s history. It introduced hooks,a revolutionary concept that enables developers to use state and other React features without the need for class components. Hooks make code simpler and more readable, transforming the way developers write components.Additionally, React 16 introduced Fiber, a new reconciliation mechanism that significantly improved performance, especially when dealing with animations and complex UI structures.
4. React 17: This version focused on updating and maintaining compatibility with previous versions. It introduced a new JSX transform system.
5. React 18: This is the latest stable release, which continues the trajectory of improvement and emphasizes performance enhancements and additional features, such as the automatic batching of renders, state transitions, server components, and streaming server-side rendering.
Setting up a new React project There are several ways to create a React project when you are getting started. In this section, let's explore three common approaches: • Using web bundlers • Using frameworks • Using an online code editor
Using web bundlers Using a web bundler is an efficient way to create React projects, especially if you are building a Single-Page Application (SPA). Vite is known for its remarkable speed and ease of setup and use.
Using frameworks For real-world and commercial projects, it is recommended to use frameworks built on top of React. These frameworks provide additional features out of the box, such as routing and asset management (images, SVG files, fonts, etc.). They also guide you in organizing your project structure effectively, as frameworks often enforce specific file organization rules. Some popular React frameworks include Next.js, Gatsby, and Remix.
Online code editors Online code editors combine the advantages of web bundlers and frameworks but allow you to set up your React development environment in the cloud or right inside of the browser. This eliminates the need to install anything on your machine and lets you write and explore React code directly in your browser. While there are various online code editors available, some of the most popular options include CodeSandbox, StackBlitz, and Replit. These platforms provide a user-friendly interface and allow you to create, share, and collaborate on React projects without any local setup.To get started with an online code editor, you don’t even need an account. Simply follow this link on your browser:(https://codesandbox.io/p/sandbox/react-new?utm_source=dotnew). In a few seconds, you will see that CodeSandbox is ready to work with a template project, and a live preview of the editor is available directly in the browser tab. If you want to save your changes, then you need to create an account.Using online code editors is a convenient way to learn and experiment with React, especially if you prefer a browser-based development environment.
Reference material: React and React Native
2 notes
·
View notes
Text
Frontend Frameworks for Web Development
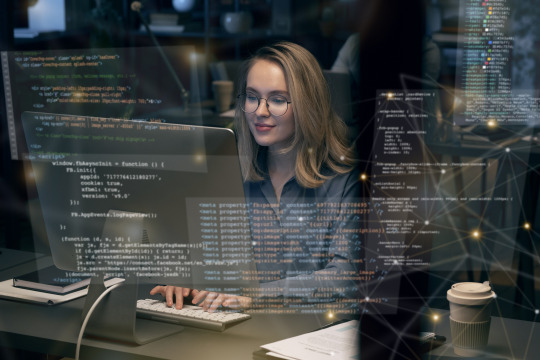
Frontend Frameworks for Web Development, creating captivating and user-friendly websites and web applications is essential for businesses to thrive. With the increasing demand for dynamic and interactive web experiences, frontend development has become more crucial than ever.
To meet these demands efficiently, developers rely on frontend frameworks, which streamline the development process and enhance productivity.
In this comprehensive guide, we'll explore the world of frontend frameworks for web development, covering everything from key factors to consider when choosing a framework to the top options available in India.
Overview of Frontend Frameworks for Web Development
Frontend frameworks are collections of pre-written code, libraries, and tools that expedite the process of building user interfaces for websites and web applications.
These frameworks provide developers with a structured approach to frontend development, offering ready-made components, templates, and utilities to streamline common tasks.
By leveraging frontend frameworks, developers can achieve consistency, maintainability, and scalability in their projects while focusing more on functionality and user experience.
These frameworks often follow the principles of modularization and component-based architecture, facilitating code reuse and making development more efficient.
Key Factors to Consider in a Frontend Frameworks for Web Development
When choosing a frontend framework for web development, several key factors should be considered:
Community Support: Opt for frameworks with active and robust communities. A strong community ensures ongoing support, frequent updates, and a wealth of resources such as documentation, tutorials, and plugins.
Performance: Evaluate the performance metrics of the framework, including page load times, rendering speed, and resource utilization. A lightweight and efficient framework can significantly impact the user experience.
Flexibility and Customization: Assess the framework's flexibility in accommodating project requirements and its customization options. Look for frameworks that allow developers to tailor components and styles to suit specific design needs.
Learning Curve: Consider the learning curve associated with the framework, especially if you're working with a team of developers with varying skill levels. Choose a framework that aligns with your team's expertise and resources.
Compatibility and Browser Support: Ensure that the framework is compatible with a wide range of browsers and devices, particularly if your target audience includes users with diverse preferences and devices.
Updates and Maintenance: Check the framework's update frequency and long-term maintenance plans. Regular updates and proactive maintenance are essential for addressing security vulnerabilities and compatibility issues.
7 Best Frontend Frameworks for Web Development in India
Now, let's explore some of the top Frontend Frameworks for Web Development widely used by developers in India:
React.js: Developed by Facebook, React.js is a popular JavaScript library for building user interfaces. It emphasizes component-based architecture and virtual DOM for efficient rendering. React's ecosystem includes tools like React Router for routing and Redux for state management.
Angular: Backed by Google, Angular is a comprehensive frontend framework for building robust web applications. It provides features such as two-way data binding, dependency injection, and modular development. Angular offers a full-fledged ecosystem with Angular CLI for project scaffolding and Angular Material for UI components.
Vue.js: Vue.js is an advanced JavaScript framework known for its simplicity and flexibility. It allows developers to incrementally adopt its features and integrate it into existing projects easily. Vue.js offers reactive data binding, virtual DOM, and a rich ecosystem of plugins and components.
Bootstrap: Bootstrap is a popular CSS framework for building responsive and mobile-first websites. It provides a grid system, pre-styled components, and responsive utilities, allowing developers to create sleek and consistent designs quickly. Bootstrap is highly customizable and offers extensive documentation and community support.
Svelte: Svelte is a relatively new frontend framework that focuses on compiling components at build time rather than runtime. This approach results in highly optimized and lightweight web applications. Svelte's simplicity and performance make it an attractive choice for developers seeking efficiency and speed.
Tailwind CSS: Tailwind CSS is a utility-first CSS framework that provides a set of low-level utility classes for building custom designs. It offers a highly customizable and expressive approach to styling, enabling developers to create unique and responsive interfaces without writing custom CSS. Tailwind CSS is gaining popularity for its developer-friendly workflow and rapid prototyping capabilities.
Foundation: Foundation is a responsive front-end framework developed by ZURB, known for its modular and customizable nature. It offers a comprehensive set of CSS and JavaScript components, as well as a robust grid system and a variety of UI elements. Foundation is well-suited for building modern and accessible web projects.
Conclusion for Web Development
Choosing the right Frontend Frameworks for Web Development is crucial to the success of your website development. Consider factors such as community support, performance, flexibility, and compatibility when evaluating different frameworks. Each framework has its strengths and weaknesses, so assess your project requirements and development preferences carefully before making a decision.
Whether you opt for React.js, Angular, Vue.js, or any other frontend framework, prioritize learning and mastering the chosen tool to maximize its potential and deliver exceptional web experiences. Keep abreast of new developments, best practices, and emerging trends in frontend development to stay ahead in this ever-evolving field.
FAQs for Web Development
Q: Which frontend framework is best for beginners?
A: Vue.js and React.js are often recommended for beginners due to their relatively gentle learning curves and extensive documentation.
Q: How do I choose between Angular and React for my project?
A: Consider factors such as project requirements, team expertise, and ecosystem preferences. Angular offers a comprehensive solution with built-in features, while React provides more flexibility and a vibrant ecosystem.
Q: Are frontend frameworks necessary for web development?
A: While not strictly necessary, frontend frameworks greatly simplify and expedite the web development process, especially for complex and dynamic projects. They provide structure, consistency, and efficiency, ultimately enhancing productivity and user experience.
Q: Can I use multiple frontend frameworks in the same project?
A: While technically possible, using multiple frontend frameworks in the same project can lead to complexity, conflicts, and maintenance challenges. It's generally advisable to stick to a single framework to maintain code consistency and streamline development.
More Details
Email: [email protected]
Website: https://censoware.com/
#web development#website development#software development#web developers#pythonprogramming#phpdeveloper#javascriptdeveloper#mern stack development#mobile app development
2 notes
·
View notes
Text
Maximizing Test Effectiveness: Unraveling the Advantages of Selenium in Web Testing
Introduction: Selenium, a prominent automation testing framework, has redefined the landscape of web application testing. Renowned for its versatility, user-friendliness, and robust capabilities, Selenium is an indispensable asset for professionals engaged in software testing. In this discourse, we explore the manifold advantages of utilizing Selenium for web testing and elucidate how it empowers testers to achieve optimal outcomes.
1. Precision and Dependability: Selenium provides precise and dependable element identification, enabling testers to accurately pinpoint and interact with web elements on a page. Leveraging unique attributes such as IDs, classes, or XPath expressions, Selenium ensures tests execute consistently and yield reliable results across various browsers and environments.
2. Enhanced Test Stability: Selenium bolsters test stability by mitigating the impact of changes in the application under test. Selenium locators are adept at withstanding alterations in page layout or structure, ensuring tests remain stable and impervious to minor UI modifications. This stability is pivotal for upholding the integrity of test suites and ensuring accurate validation of application functionality.
3. Improved Test Efficiency: Leveraging Selenium for web testing enhances efficiency and productivity by automating repetitive testing tasks. By automating routine test cases, testers can allocate their time and effort toward more intricate scenarios and exploratory testing, thereby expediting the overall testing process and reducing time-to-market for web applications.
4. Cross-Browser Compatibility: A standout feature of Selenium is its cross-browser compatibility, facilitating test execution across diverse web browsers with minimal effort. Selenium WebDriver seamlessly supports popular browsers like Chrome, Firefox, Safari, and Edge, ensuring consistent test execution and compatibility across varied browser environments.
5. Seamless Integration with Testing Frameworks: Selenium seamlessly integrates with a myriad of testing frameworks, enabling testers to harness their preferred tools and methodologies. Whether utilizing TestNG, JUnit, or NUnit, Selenium can be effortlessly integrated into existing testing workflows, affording flexibility and customization options tailored to the needs of individual testing teams.
6. Scalability and Reusability: Selenium advocates scalability and reusability through its modular test design and component-based architecture. Test scripts and components can be modularized and repurposed across multiple test cases, curbing duplication of effort and maintenance overhead. This modular approach fosters test maintainability and facilitates the creation of robust, scalable test suites.
7. Cost-Effectiveness: By automating web testing tasks, Selenium aids organizations in trimming manual testing efforts and associated costs. Automated tests can be executed repeatedly sans additional expenses, resulting in long-term cost savings and heightened return on investment. Additionally, Selenium's open-source nature obviates licensing fees, rendering it a cost-effective choice for organizations of all sizes.
8. Comprehensive Test Coverage: Selenium empowers testers to attain comprehensive test coverage by supporting a gamut of testing scenarios and workflows. From functional and regression testing to performance and compatibility testing, Selenium caters to diverse testing requirements, ensuring thorough validation of web applications across various dimensions.
In summation, Selenium offers a plethora of advantages for web testing, encompassing precision and reliability, enhanced test stability, improved efficiency, cross-browser compatibility, seamless integration, scalability, cost-effectiveness, and comprehensive test coverage. By harnessing the power of Selenium, testers can streamline their testing endeavors, augment test coverage, and deliver high-quality web applications that align with the expectations of end-users and stakeholders alike.
2 notes
·
View notes
Text
React training in hyderabad
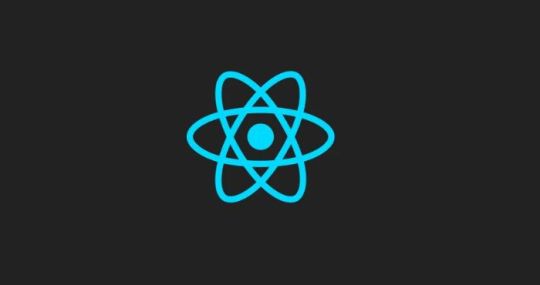
Introduction to React JS
React is like the carpenter’s toolbox for building web interfaces. Created by the folks at Facebook, it’s a set of tools that makes crafting interactive and dynamic websites a whole lot easier. Imagine it as a set of magic building blocks that help developers create sleek, responsive, and engaging front-end applications. Since its debut in 2013, React has become a favorite among web developers, kind of like the go-to tool when you want to make your website not just look good but also feel lively and interactive. It’s a bit like the secret sauce behind many of the awesome websites you use every day.
Features of React JS
Declarative Syntax: React uses a declarative syntax, allowing developers to describe the desired outcome, and React takes care of the underlying logic to achieve that outcome. This makes the code more predictable and easier to understand.
2. Component-Based Architecture: React follows a component-based architecture where the UI is broken down into reusable components. Each component manages its own state and can be composed to build complex user interfaces.
3. Virtual DOM: React uses a virtual DOM to improve performance. Instead of directly manipulating the actual DOM, React creates a virtual representation of it in memory and updates only the parts of the actual DOM that have changed. This minimizes the number of DOM manipulations, resulting in faster updates.
4. JSX (JavaScript XML): React uses JSX, a syntax extension for JavaScript that allows you to write HTML elements and components in a syntax similar to XML or HTML. JSX makes the code more readable and helps with the integration of UI components.
Components in React
In React, think of components as building blocks for your user interface — they’re like Lego pieces that you can assemble to create your application. These components are self-contained and can be reused, making it easier to manage and organize your user interface. It’s as if you’re constructing your application with Lego bricks, where each brick represents a specific part of your user interface.
This modular approach simplifies the development process and encourages a more flexible and maintainable code structure.
Dumb components: Think of these components as the friendly faces you see in a store’s display window. They’re there to catch your eye and make everything look inviting. These components are all about the visual appeal, like the welcoming decor of a shop, without getting into the technical details or behind-the-scenes work.
2. Smart components:Think of these components as the wise decision-makers. They not only handle the important business details but also decide when and how things should appear on the screen. It’s like having an event planner for your app — they manage the behind-the-scenes work and ensure everything shows up at just the right time and in the best way possible.
NOTE 🤓:These components can come to life either as classes or functions. They’re adaptable, like a versatile tool that can be crafted in different ways based on your needs.
State of a component
In the world of React, think of the state as a component’s personal notebook — it’s where the component keeps track of information that can change over time. This information might shift based on how users interact with the component or how the outside world reacts to it. Whether the component is a classic novel (a class) or a snappy note (a function), it handles its state in its own unique way. What’s really neat is that when this internal state undergoes a change, it’s like the component automatically freshens up, updating its look without any fuss — kind of like a quick, seamless makeover happening in the background.
Properties of a component
In React, components communicate with each other through a feature called “Props.” It’s like sharing notes or gifts between them, but here’s the catch: the communication is a one-way street, flowing strictly from a parent component to its child. Imagine it as a parent passing a sealed letter to their child. What’s interesting is that these messages, or props, are unchangeable once delivered. It’s akin to sending a secure package — the information remains intact, ensuring a clear and organized flow of data between React components.
Life cycle of a component
Components in React have a lifecycle, and it’s like understanding the natural flow of a component’s journey. This lifecycle serves as our guide, allowing us to make smart decisions at different points in the component’s existence. It’s a bit like knowing when to take specific actions, such as making an HTTP request or tidying up the user interface.
componentDidMount: Think of this as the behind-the-scenes moment when the component takes its place on the UI stage for the first time.
componentDidUpdate:Picture this as the component’s way of adapting and evolving — a sort of behind-the-scenes dance that happens when the component experiences a change in its mood or receives something new to work with.
componentWillUnmount: Function executed when the component is unmounted from the UI.
React Hooks are a set of functions that were introduced in React 16.8 to enable the use of state and other React features in functional components. Before the introduction of hooks, state and lifecycle methods were primarily associated with class components. Hooks allow functional components to have state, lifecycle features, and more, making them a powerful and concise alternative to class components.
The most commonly used React Hooks include:
use State: Enables functional components to manage state.
2. use Effect: Provides a way to perform side effects in functional components, similar to component DidMount and component DidUpdate in class components.
3. use Context: Allows functional components to subscribe to React context without introducing a nested component.
4. use Reducer: An alternative to use State for managing more complex state logic in functional components.
5. use Callback and use Memo: Optimize performance by memoizing functions and values to prevent unnecessary re-renders.
Hello world with create react app
Create React App is a ready-to-go setup designed for building React applications. It works seamlessly with Node version 14.0.0 or higher and npm version 5.6 or higher. To kickstart a new project, simply run the following commands in your terminal:
“npx create-react-app your-project-name”
Most used add-on libraries in React JS
1. Redux: A predictable state container for managing the state of your application in a more organized and scalable way.
2. React Router: Provides navigation and routing functionalities for React applications, allowing you to create dynamic and SPA (Single Page Application) experiences.
3. Axios: A promise-based HTTP client that simplifies making HTTP requests in React applications.
4. Styled-components: Enables writing CSS directly in your JavaScript files using tagged template literals, promoting component-based styling.
5. Material-UI: A popular React component library that implements Google’s Material Design, offering a set of pre-designed and customizable components.
6. Formik: A form management library that simplifies form building, validation, and handling form submissions.
7. React Query: A library for managing, caching, and syncing asynchronous data in React applications, making it easier to work with API calls and data fetching.
8. Chakra UI: A component library for React that provides a set of accessible and customizable UI components.
9. React Helmet: Allows manipulation of the document head, useful for managing meta tags, titles, and other document head elements.
10. React-Bootstrap: Integrates the Bootstrap CSS framework with React components, providing a set of responsive and customizable UI elements.
Recursos React JS
React Official Website: Explore the heart of React at React official websites. Immerse yourself in comprehensive documentation, tutorials, and fundamental concepts that form the backbone of React development.
React Blog: Stay updated on the latest in React by checking out the React Blog. Dive into news, official articles, and insightful posts that illuminate the evolving world of React development.
Thinking in React: Embark on your React journey by embracing the philosophy of “Thinking in React.” Learn how to kickstart your understanding by focusing on the core concept of thinking in components. The journey begins with a guide to getting started thinking in components. This course is designed to provide students with a solid understanding of the architecture and functionality of MuleSoft’s integration platform.
3 notes
·
View notes
Text
React JS
Unleashing the Power of User Interfaces: A Guide to React.js
In the ever-evolving landscape of web development, creating interactive and dynamic user interfaces is crucial to delivering engaging online experiences. One technology that has risen to prominence in this domain is React.js. Developed by Facebook, React.js has transformed the way developers build web applications by providing a powerful framework for building modular and reusable UI components. In this blog post, we'll delve into the world of React.js, exploring its core concepts, benefits, and practical applications.
Understanding React.js:
At its heart, React.js is a JavaScript library that focuses on the efficient rendering of user interfaces. It employs a declarative approach to building UI components, where developers define how the interface should look at any given point in time, and React takes care of efficiently updating and rendering the UI when data changes.
Key Concepts:
Components: In React, user interfaces are constructed using components - self-contained, reusable building blocks that encapsulate both UI elements and the logic to manage their behavior. Components can be nested within each other, forming a tree-like structure that represents the entire user interface.
Virtual DOM: One of the most powerful features of React is its Virtual DOM. Rather than directly manipulating the actual DOM, React creates a lightweight virtual representation of the DOM in memory. When there are changes in the data or state of a component, React calculates the difference (also known as the "diff") between the previous and current virtual DOM states, and then applies only the necessary updates to the actual DOM. This process greatly improves performance and minimizes unnecessary re-rendering.
State and Props: State represents the dynamic data that can change within a component. Props (short for properties) are immutable data that are passed from parent to child components, allowing for dynamic content and customization.
Lifecycle Methods: React components have a lifecycle, consisting of phases from initialization to rendering, updating, and unmounting. Developers can tap into these lifecycle methods to control behavior at various points in a component's existence.
Hooks: Introduced in React 16.8, hooks are functions that allow developers to add state and lifecycle features to functional components. This enables a more concise and readable code structure, promoting the use of functional components over class components.
Benefits of Using React.js:
Modularity and Reusability: React's component-based architecture promotes modular development. Components can be reused across different parts of an application or even shared between projects, leading to more efficient development and easier maintenance.
Performance: By employing the Virtual DOM and selective rendering, React minimizes the performance overhead of constantly updating the actual DOM. This results in faster, smoother user interfaces, even for complex applications.
Community and Ecosystem: React has a vast and active community that contributes to its ecosystem. This means there are numerous libraries, tools, and resources available to enhance and streamline the development process.
SEO-Friendly: React applications can be rendered on the server side, improving search engine optimization (SEO) by providing search engines with a fully rendered HTML page.
Practical Applications:
React.js finds applications in a wide range of projects, from simple web apps to complex enterprise-level solutions. Some common use cases include:
Single-Page Applications (SPAs): React excels in building SPAs where fluid user experiences are key. Platforms like Facebook and Instagram use React to power their dynamic interfaces.
E-Commerce Websites: React's modular nature is beneficial for e-commerce sites, allowing developers to create reusable components for product listings, carts, and checkout processes.
Data Dashboards: React is suitable for building data visualization dashboards that require real-time updates and interactive charts.
Mobile Applications: React Native, an extension of React.js, is used to develop cross-platform mobile applications with a native-like experience.
In Conclusion:
React.js has revolutionized the way web applications are developed by providing a powerful set of tools for building modular, efficient, and interactive user interfaces. Its component-based architecture, Virtual DOM, and rich ecosystem make it a top choice for developers looking to create modern and engaging web experiences. Whether you're building a personal project or a complex enterprise application, React.js is a valuable technology to have in your toolkit. So, embrace the power of React.js and embark on a journey to create stunning user interfaces that captivate and delight your audience.
6 notes
·
View notes
Text
Software Development: Essential Terms for Beginners to Know
Certainly, here are some essential terms related to software development that beginners, including software developers in India, should know:
Algorithm: A step-by-step set of instructions to solve a specific problem or perform a task, often used in programming and data processing.
Code: The written instructions in a programming language that computers can understand and execute.
Programming Language: A formal language used to write computer programs, like Python, Java, C++, etc.
IDE (Integrated Development Environment): A software suite that combines code editor, debugger, and compiler tools to streamline the software development process.
Version Control: The management of changes to source code over time, allowing multiple developers to collaborate on a project without conflicts.
Git: A popular distributed version control system used to track changes in source code during software development.
Repository: A storage location for version-controlled source code and related files, often hosted on platforms like GitHub or GitLab.
Debugging: The process of identifying and fixing errors or bugs in software code.
API (Application Programming Interface): A set of protocols and tools for building software applications. It specifies how different software components should interact.
Framework: A pre-built set of tools, libraries, and conventions that simplifies the development of specific types of software applications.
Database: A structured collection of data that can be accessed, managed, and updated. Examples include MySQL, PostgreSQL, and MongoDB.
Frontend: The user-facing part of a software application, typically involving the user interface (UI) and user experience (UX) design.
Backend: The server-side part of a software application that handles data processing, database interactions, and business logic.
API Endpoint: A specific URL where an API can be accessed, allowing applications to communicate with each other.
Deployment: The process of making a software application available for use, typically on a server or a cloud platform.
DevOps (Development and Operations): A set of practices that aim to automate and integrate the processes of software development and IT operations.
Agile: A project management and development approach that emphasizes iterative and collaborative work, adapting to changes throughout the development cycle.
Scrum: An Agile framework that divides work into time-boxed iterations called sprints and emphasizes collaboration and adaptability.
User Story: A simple description of a feature from the user's perspective, often used in Agile methodologies.
Continuous Integration (CI) / Continuous Deployment (CD): Practices that involve automatically integrating code changes and deploying new versions of software frequently and reliably.
Sprint: A fixed time period (usually 1-4 weeks) in Agile development during which a specific set of tasks or features are worked on.
Algorithm Complexity: The measurement of how much time or memory an algorithm requires to solve a problem based on its input size.
Full Stack Developer: A developer who is proficient in both frontend and backend development.
Responsive Design: Designing software interfaces that adapt and display well on various screen sizes and devices.
Open Source: Software that is made available with its source code, allowing anyone to view, modify, and distribute it.
These terms provide a foundational understanding of software development concepts for beginners, including software developers in India.
#software app#software development#software developers#software development in India#Indian software developers
3 notes
·
View notes
Text
Jexcore Infotech – India’s Premium Customized Website Design Company in Ahmedabad

A website is a business’s front entryway for possibilities. It is one of the indispensable advertising resources from which visitors can find out about your business. A website with a viable UI can draw more customers and get more conversions or business deals.
Even though a theme-based website can be set up rapidly and is cost-proficient however frequently misses the mark on functionalities. However Customized website Design offers greater adaptability. Giving your business a decent start is basic and sufficiently expert. Anyway, what rehearses do you take on to captivate possible visitors? How about we comprehend from website Design specialist to you?
Jexcore Infotech, a top website design company in Ahmedabad, makes websites that dazzle possibilities in the primary case. Here are the prescribed procedures we follow to help you to boost your web presence.
#1 Audience Centric Website Design
A website represents an organization or company, its related services and products, and eventually the brand. Hence, we focus on planning a website that looks outwardly clean and proficient. We redo your website in view of your audience’s necessities and remember your offers in mind. Our web design methodology incorporates white spaces, cleaned-up designs, quality product or service images, and engaging illustrations that let your image hang out in digital space.
Our expert team leader Kalpesh Patel at Jexcore Infotech can execute straightforward and popular website architectures for your brand image and impact web clients to interface with items/services with no aversion.
We try not to make a conjured or cluttered website: When a client comes to the site, he requires a couple of moments to choose whether to investigate more or leave. A cluttered website page with different varieties and blocked text styles can make a webpage outwardly confounding. Going against the norm, a very organized plan structure enhances clients’ web insight and permits them where they need to go.
We utilize decent colour tones: Irregular and strong varieties can make a site look ungainly. To make it a winsome site, we utilize quiet and muffled colours thinking about your brand logo and company’s vision and guarantee that the site looks spotless and minimal.
#2 Easily Readable Content
Readability or Intelligibility is one of the critical components of web content, significant for further developing webpage web crawler will index. It is a robust website design and SEO practice that makes content simple to grasp by the ideal audience. Regardless of how great the site content is, assuming the comprehensibility is poor, it can affect the website ranking in SERP.
Jexcore Infotech has an expert team that works in making fascinating web content and guarantees that it is discernible for both audience and SERP. Here are a few hints that make your web content decipherable.
Short Paragraphs: We make significant sentences by keeping short paragraphs – it gives pursuers a chance to take in the data without being overpowered at its length. Also, it causes readers to comprehend the message right away.
Text style Selection: For a business, textual style concludes whether it is not difficult to peruse the site’s content. It keeps the site’s appearance basic and satisfactory to the viewers.
#3 Mobile Friendly Web Design
To a new survey, in the final quarter of 2021, mobile phones produced 54.4% of worldwide web traffic (Source – google trends).
Most visitors that don’t find a site viable with smartphones will not prescribe it to other people. More Indians are perusing and shopping on smartphones and tablets, and they lean toward a site that functions admirably on their cell phone. We make responsive website designs so your site stays high in front of competitors in the business.
Jexcore Infotech is a website design company in Ahmedabad with mastery in making responsive first website design. We guarantee that you don’t miss guests coming through mobile. Here are the systems that work on visitors’ insight as well as SEO positioning.
Enhance Site for Mobile: We offer an incredible experience to clients by streamlining the website content with the goal that it fits into the client’s gadget screen.
Further develop Functionality: Our designer makes a site that is responsive in every screen type. Besides, we streamline pictures, recordings, and visuals to further develop site page loading speed.
Jexcore Infotech – India’s Premium Digital Marketing Company
Jexcore Infotech is appraised as one of the top website design company in India by a few review websites. We have a reasonable team of award-winning website specialists and designers that can assist in increasing business revenue and income with their masterful strategy plans.
We are a one-stop solution for our client’s digital marketing needs. We have expert digital marketers who had great experience to deliver results in line with your vision. We offer services, including SMO, SEO, SEM, PPC Ads, and web developing and designing.
#marketing#socialmediamarketing#digitalmarketing#branding#contentstrategy#jexcore#marketingagency#marketingdigital#marketingservices#digital marketing
3 notes
·
View notes
Text
React vs Vue vs Angular: Which One Should You Use in 2025
Overview: (React)
React continues to dominate the frontend development world in 2025, offering developers unmatched flexibility, performance, and community support. Built and maintained by Meta (formerly Facebook), React has matured into a robust UI library that startups and tech giants use.
What Is React?
React is an open-source JavaScript library designed for building fast, interactive user interfaces, primarily for single-page applications (SPAs). It's focused on the "View" layer of web apps, allowing developers to build encapsulated, reusable components that manage their state.
With the release of React 18 and innovations like Concurrent Rendering and Server Components, React now supports smoother UI updates and optimized server-side rendering, making it more future-ready than ever.
Key Aspects
Component-Based Architecture: React's modular, reusable component structure makes it ideal for building scalable UIs with consistent patterns.
Blazing-Fast UI Updates: Thanks to the virtual DOM, React efficiently updates only what's changed, ensuring smooth performance even in complex apps.
Hooks and Functional Components: With modern features like React Hooks, developers can manage state and lifecycle behavior cleanly in functional components—there is no need for class-based syntax.
Concurrent Rendering: React 18 introduced Concurrent Mode, improving performance by rendering background updates without blocking the main thread.
Massive Ecosystem: From Next.js for SSR to React Native for mobile apps, React integrates with an enormous ecosystem of tools, libraries, and extensions.
Overview (Aue)
Vue.js continues to be a strong contender in the frontend framework space in 2025, primarily for developers and teams seeking simplicity without sacrificing power. Created by Evan You, Vue has grown into a mature framework known for its clean syntax, detailed documentation, and ease of integration.
What Is Vue?
Vue is a progressive JavaScript framework for building web user interfaces. Its design philosophy emphasizes incrementality—you can use Vue for a small feature on a page or scale it up into a full-fledged single-page application (SPA).
With Vue 3 and the Composition API, Vue has evolved to offer better modularity, TypeScript support, and reusability of logic across components.
Key Aspects
Lightweight and Fast: Vue has a small footprint and delivers high performance out of the box. It's fast to load, compile, and render, making it an excellent choice for performance-sensitive projects.
Simple Integration: Vue can be dropped into existing projects or used as a complete app framework. It works well with legacy systems and new apps alike.
Easy to Learn: Vue's gentle learning curve and readable syntax make it a top choice for beginners and teams with mixed skill levels.
Composition API: The Composition API in Vue 3 allows for better code reuse and more scalable application architecture, similar to React's hooks.
Overview (Angular)
Angular, developed and maintained by Google, remains a top choice for enterprise-level applications in 2025. As a fully integrated framework, Angular provides all the tools a development team needs to build large-scale, maintainable apps out of the box.
What Is Angular?
Angular is a TypeScript-based frontend framework that includes built-in solutions for routing, state management, HTTP communication, form handling, and more. Unlike React or Vue, Angular is opinionated and follows strict architectural patterns.
Angular 17 (and beyond) introduces Signals, a new reactive system designed to improve state management and performance by offering more predictable reactivity.
Key Aspects:
All-in-One Framework: Angular offers everything you need—from routing to testing—without needing third-party libraries. This consistency is great for large teams.
Strong Typing with TypeScript: TypeScript is the default language in Angular, making it ideal for teams that prioritize type safety and tooling.
Ideal for Enterprises: With its structured architecture, dependency injection, and modular system, Angular is built for scalability, maintainability, and long-term project health.
Improved Performance: Angular 17 introduces Signals, improving reactive programming, rendering speed, and resource efficiency.
Angular Drawbacks
A steep learning curve due to its complex concepts like decorators, DI, zones, etc.
More verbose code compared to Vue and React.
Slower adoption in smaller teams and startups.
Which One Should Use
If you're looking for simplicity and speed, especially as a solo developer or on smaller projects, Vue.js is your best bet. Its gentle learning curve and clean syntax make it ideal for quick development and maintainable code.
For scalable, dynamic applications, React strikes the perfect balance. It offers flexibility, a vast ecosystem, and strong community support, making it a top choice for startups, SaaS products, and projects that may evolve over time.
If you're building large-scale, enterprise-grade apps, Angular provides everything out of the box—routing, forms, state management—with a highly structured approach. It's TypeScript-first and built for long-term maintainability across large teams.
In short:
Choose Vue for ease and speed.
Choose React for flexibility and modern workflows.
Choose Angular for structure and enterprise power.
#bbc marketing in prayagraj#seo services in prayagraj#google ads services in prayagraj#digital marketing agency in prayagraj
0 notes
Text
The Modern Full Stack Toolkit: What You Really Need
The role of a full stack web developer is more dynamic than ever. With the rapid evolution of technology, staying up-to-date with the latest tools and frameworks is essential. The modern full stack toolkit combines a wide range of technologies, from front-end to back-end development, enabling developers to create robust, efficient, and interactive web applications. Understanding what tools to use is key to building successful web applications, and knowing how to use them simultaneously is equally important.
What Does a Full Stack Web Developer Do?
A full stack web developer is responsible for developing both the client and server sides of web applications. This includes the user interface (UI), as well as the underlying database and server architecture. A typical full stack developer needs to be proficient in various technologies, ranging from HTML, CSS, and JavaScript to server-side languages and databases.
The role requires both a creative and analytical mindset, as developers must consider not only the aesthetic aspects but also performance, scalability, and security. A strong understanding of both front-end and back-end frameworks allows full stack developers to create seamless user experiences while maintaining backend integrity.
Key Components of the Modern Full Stack Toolkit
To excel in this multifaceted role, a full stack web developer needs a toolkit that covers all aspects of development. Here’s a breakdown of the modern tools that developers use simultaneously to build effective web applications:
1. Front-End Development Tools
The front end is everything the user interacts with directly. A good full stack developer must master the following front-end technologies:
HTML5 & CSS3: The building blocks of the web, allowing you to structure content and design the visual layout.
JavaScript: This programming language adds interactivity to your website, allowing for dynamic content and real-time updates.
React.js: A popular front-end JavaScript library developed by Facebook, enabling the creation of responsive, component-based user interfaces.
Vue.js: Another framework for building user interfaces, known for its simplicity and flexibility.
Bootstrap: A front-end framework for developing responsive, mobile-first websites quickly.
2. Back-End Development Tools
The back end of a web application is what powers the user-facing side. It involves handling data storage, user authentication, and communication between the front end and the database. The following tools are essential for the back-end of any modern web application:
Node.js: A JavaScript runtime environment that allows you to run JavaScript on the server side, enabling you to use the same language for both the front end and back end.
Express.js: A minimal and flexible Node.js web application framework that simplifies routing and handling server-side logic.
Django: A high-level Python framework for rapid development of secure and maintainable websites.
Ruby on Rails: A robust framework for building full-featured web applications using Ruby, known for its convention over configuration approach.
3. Databases
No web application is complete without a robust database. A full stack developer should be proficient in working with databases to store and retrieve application data:
MongoDB: A NoSQL database that stores data in JSON-like format, ideal for handling large volumes of unstructured data.
MySQL: A traditional relational database management system (RDBMS) that uses SQL to query data and is well-suited for structured data.
PostgreSQL: An advanced open-source relational database with a focus on extensibility and standards compliance.
4. Version Control
Collaboration is a crucial part of the development process, and version control is a must-have tool for any developer. Git, the most popular version control system, allows developers to track changes in code, collaborate with others, and maintain project histories.
GitHub: A platform that hosts Git repositories, enabling developers to collaborate, share code, and deploy applications easily.
5. DevOps Tools
DevOps tools help manage the infrastructure and deployment pipelines, ensuring continuous integration and continuous delivery (CI/CD). These tools streamline the development process and allow for faster, more reliable releases.
Docker: A containerization platform that allows developers to package applications and their dependencies into a standardized unit for development, testing, and deployment.
Kubernetes: A container orchestration tool that automates the deployment, scaling, and management of containerized applications.
6. Testing Tools
To ensure the quality of your application, a full stack web developer must also implement rigorous testing at every stage of the development cycle.
Jest: A JavaScript testing framework for ensuring that your front-end and back-end code works as expected.
Mocha: A feature-rich JavaScript test framework that works well with Node.js applications.
The Importance of Integration and Simultaneous Use
Being proficient in the individual components of full stack development is important, but the real challenge lies in using these tools simultaneously. A full stack web developer must understand how to integrate various front-end and back-end technologies into a cohesive whole.
For example, a full stack web developer working with React.js on the front end might use Node.js and Express on the back end while integrating MongoDB as the database. The developer needs to ensure smooth communication between these components and optimize performance across all layers of the application.
Conclusion
The modern full stack web developer must be equipped with a broad set of tools that spans front-end, back-end, databases, and deployment. The key to success lies not only in mastering individual technologies but in integrating them simultaneously to build seamless, high-performance applications. By leveraging the right tools and frameworks, a full stack web developer can ensure that they stay ahead in the fast-paced world of web development.
0 notes
Text
React vs Vue vs Angular: Which One Should You Use in 2025
Overview: (React)
React continues to dominate the frontend development world in 2025, offering developers unmatched flexibility, performance, and community support. Built and maintained by Meta (formerly Facebook), React has matured into a robust UI library that startups and tech giants use.
What Is React?
React is an open-source JavaScript library designed for building fast, interactive user interfaces, primarily for single-page applications (SPAs). It's focused on the "View" layer of web apps, allowing developers to build encapsulated, reusable components that manage their state.
With the release of React 18 and innovations like Concurrent Rendering and Server Components, React now supports smoother UI updates and optimized server-side rendering, making it more future-ready than ever.
Key Aspects
Component-Based Architecture: React's modular, reusable component structure makes it ideal for building scalable UIs with consistent patterns.
Blazing-Fast UI Updates: Thanks to the virtual DOM, React efficiently updates only what's changed, ensuring smooth performance even in complex apps.
Hooks and Functional Components: With modern features like React Hooks, developers can manage state and lifecycle behavior cleanly in functional components—there is no need for class-based syntax.
Concurrent Rendering: React 18 introduced Concurrent Mode, improving performance by rendering background updates without blocking the main thread.
Massive Ecosystem: From Next.js for SSR to React Native for mobile apps, React integrates with an enormous ecosystem of tools, libraries, and extensions.
Code: App.jsx
Import React from 'react';
function App() {
return (
<div>
<h1>Hello, World! </h1>
</div>
);
}
export default App;
Entry Point: main.jsx
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App.jsx';
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<App />
</React.StrictMode>
);
HTML Template: index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>React App</title>
</head>
<body>
<div id="root"></div>
<script type="module" src="/main.jsx"></script>
</body>
</html>
Overview (Aue)
Vue.js continues to be a strong contender in the frontend framework space in 2025, primarily for developers and teams seeking simplicity without sacrificing power. Created by Evan You, Vue has grown into a mature framework known for its clean syntax, detailed documentation, and ease of integration.
What Is Vue?
Vue is a progressive JavaScript framework for building web user interfaces. Its design philosophy emphasizes incrementality—you can use Vue for a small feature on a page or scale it up into a full-fledged single-page application (SPA).
With Vue 3 and the Composition API, Vue has evolved to offer better modularity, TypeScript support, and reusability of logic across components.
Key Aspects
Lightweight and Fast: Vue has a small footprint and delivers high performance out of the box. It's fast to load, compile, and render, making it an excellent choice for performance-sensitive projects.
Simple Integration: Vue can be dropped into existing projects or used as a complete app framework. It works well with legacy systems and new apps alike.
Easy to Learn: Vue's gentle learning curve and readable syntax make it a top choice for beginners and teams with mixed skill levels.
Composition API: The Composition API in Vue 3 allows for better code reuse and more scalable application architecture, similar to React's hooks.
Code: App.vue
<template>
<div>
<h1>Hello, World! </h1>
</div>
</template>
<script setup>
</script>
<style scoped>
h1 {
color: #42b983;
}
</style>
Entry Point: main.js
import { createApp } from 'vue';
import App from './App.vue';
createApp(App).mount('#app');
HTML Template: index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>Vue App</title>
</head>
<body>
<div id="app"></div>
<script type="module" src="/main.js"></script>
</body>
</html>
Overview (Angular)
Angular, developed and maintained by Google, remains a top choice for enterprise-level applications in 2025. As a fully integrated framework, Angular provides all the tools a development team needs to build large-scale, maintainable apps out of the box.
What Is Angular?
Angular is a TypeScript-based frontend framework that includes built-in solutions for routing, state management, HTTP communication, form handling, and more. Unlike React or Vue, Angular is opinionated and follows strict architectural patterns.
Angular 17 (and beyond) introduces Signals, a new reactive system designed to improve state management and performance by offering more predictable reactivity.
Key Aspects:
All-in-One Framework: Angular offers everything you need—from routing to testing—without needing third-party libraries. This consistency is great for large teams.
Strong Typing with TypeScript: TypeScript is the default language in Angular, making it ideal for teams that prioritize type safety and tooling.
Ideal for Enterprises: With its structured architecture, dependency injection, and modular system, Angular is built for scalability, maintainability, and long-term project health.
Improved Performance: Angular 17 introduces Signals, improving reactive programming, rendering speed, and resource efficiency.
Angular Drawbacks
A steep learning curve due to its complex concepts like decorators, DI, zones, etc.
More verbose code compared to Vue and React.
Slower adoption in smaller teams and startups.
Project Setup:
bash
Copy
Edit
ng new hello-world-app
cd hello-world-app
ng serve
Component: app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `<h1>Hello, World! </h1>`,
styles: [`h1 { color: #dd0031; }`]
})
export class AppComponent {}
Module: app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
bootstrap: [AppComponent]
})
export class AppModule {}
Entry Point: main.ts
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';
import { AppModule } from './app/app.module';
platformBrowserDynamic().bootstrapModule(AppModule)
.catch(err => console.error(err));
Which One Should Use
If you're looking for simplicity and speed, especially as a solo developer or on smaller projects, Vue.js is your best bet. Its gentle learning curve and clean syntax make it ideal for quick development and maintainable code.
For scalable, dynamic applications, React strikes the perfect balance. It offers flexibility, a vast ecosystem, and strong community support, making it a top choice for startups, SaaS products, and projects that may evolve over time.
If you're building large-scale, enterprise-grade apps, Angular provides everything out of the box—routing, forms, state management—with a highly structured approach. It's TypeScript-first and built for long-term maintainability across large teams.
In short:
Choose Vue for ease and speed.
Choose React for flexibility and modern workflows.
Choose Angular for structure and enterprise power.
0 notes
Text
Choosing the Right Path: Adobe Commerce Development Services, Ecommerce Development Services, and Shopify Development Agency
As digital commerce continues to expand, businesses are faced with critical decisions about how to build and manage their online stores. The choices often boil down to selecting the right platform and the right development support. This article explores three key segments in today’s ecommerce ecosystem—Adobe Commerce Development Services, general ecommerce development services, and selecting a Shopify Development Agency—to help businesses make informed decisions based on their specific needs and resources.
Understanding Adobe Commerce Development Services
Adobe Commerce, formerly known as Magento Commerce, is a powerful open-source ecommerce platform that offers high flexibility, scalability, and advanced customization options. It is best suited for medium to large enterprises with complex product catalogs and advanced operational requirements.
Key Features of Adobe Commerce:
Customizable architecture: Allows tailored solutions for complex product configurations.
Multi-store management: Operate multiple brands or regions from one backend.
Advanced pricing and promotions: Set rules based on customer groups, quantities, or time frames.
Integrated B2B tools: Quote management, bulk ordering, and custom catalogs.
Third-party integrations: Seamlessly connects with CRMs, ERPs, and payment gateways.
When to Choose Adobe Commerce:
You require a tailored ecommerce solution with unique workflows.
Your business needs strong internationalization or multi-brand management.
You have in-house or external development support to manage a more technical platform.
What Ecommerce Development Services Typically Include
The term ecommerce development services encompasses a range of support required to plan, build, launch, and maintain an online store—regardless of the platform. These services are essential whether a company is just starting or optimizing an existing online business.
Core Components:
Platform selection: Guidance on whether to use Shopify, Adobe Commerce, WooCommerce, etc.
UX/UI design: Designing user-friendly interfaces that improve conversion rates.
Custom development: Adding functionality specific to the business (e.g., custom product configurators).
Integration: Connecting ecommerce platforms to third-party tools like logistics, inventory, or accounting software.
Testing and quality assurance: Ensuring the site works across devices, browsers, and use cases.
Ongoing maintenance: Monitoring performance, updates, and fixing issues as they arise.
Benefits of Comprehensive Ecommerce Services:
A structured approach to launch and scalability.
Support for both frontend and backend development.
Greater alignment between business goals and platform capabilities.
Choosing a Shopify Development Agency
For businesses seeking simplicity, speed, and ease of use, Shopify is often the platform of choice. A Shopify Development Agency specializes in setting up, customizing, and managing stores on the Shopify ecosystem. It’s ideal for small to medium-sized businesses, or even enterprise-level brands looking for a cloud-based solution.
What Shopify Agencies Offer:
Store setup and theme customization: Based on existing themes or fully custom designs.
App integration: Adding functionality through vetted Shopify apps or custom app development.
Performance optimization: Improving speed, SEO readiness, and mobile usability.
Migration support: Moving from another platform to Shopify with minimal downtime.
Training and documentation: Helping clients manage their store post-launch.
Advantages of Shopify:
Hosted solution with built-in security and updates.
Faster time-to-market with less technical overhead.
Suitable for both D2C and B2B with plugins and minor customization.
Platform Comparison: Adobe Commerce vs. Shopify
Hosting
Adobe Commerce: Self-hosted or Adobe Commerce Cloud (requires server management)
Shopify: Fully hosted and managed by Shopify
Customization
Adobe Commerce: Highly customizable (open-source codebase)
Shopify: Limited to theme and app-based customization
Scalability
Adobe Commerce: Excellent for large product catalogs and enterprise needs
Shopify: Scalable via Shopify Plus, but less flexible for complex architectures
Time to Deploy
Adobe Commerce: Longer development and setup time
Shopify: Quick setup, faster time-to-market
Technical Expertise
Adobe Commerce: Requires experienced developers and ongoing maintenance
Shopify: Minimal technical knowledge needed for basic setup
Cost
Adobe Commerce: Higher initial and maintenance costs (development, hosting, updates)
Shopify: Predictable monthly pricing with lower upfront costs
Third-Party Integration
Adobe Commerce: Seamless with ERP, CRM, and custom workflows
Shopify: Integrates well via apps, though some limitations for large enterprise tools
Multi-store Capabilities
Adobe Commerce: Supports multi-store, multi-language, multi-currency natively
Shopify: Limited without Shopify Plus or workarounds
B2B Features
Adobe Commerce: Built-in B2B features (quotes, company accounts, tiered pricing)
Shopify: Requires Shopify Plus and additional apps for B2B functionality
SEO Control
Adobe Commerce: Advanced SEO capabilities with full control
Shopify: Good out-of-the-box SEO, but limited URL and structure customization
Each platform has its strengths. Adobe Commerce is preferred when advanced functionality and control are necessary, while Shopify is ideal for businesses wanting an all-in-one platform that’s easier to manage with fewer technical requirements.
Selecting the Right Development Partner
Choosing the right development support—whether it’s an Adobe Commerce Development Services provider, general ecommerce development services, or a Shopify Development Agency—should be based on both technical needs and business strategy.
Key Considerations:
Platform experience: Does the team have demonstrated success with the platform in question?
Project scope: Is the agency equipped to handle your long-term goals, not just the initial launch?
Support structure: What does post-launch support include? Is it reactive or proactive?
Budget alignment: Are the service levels matched with your operational budget?
Communication clarity: Does the partner provide transparent project timelines, milestones, and responsibilities?
One example of a company offering tailored services across platforms is Webiators, known for its balanced approach to technical excellence and practical implementation across ecommerce platforms.
Conclusion
Navigating the ecommerce development landscape requires careful planning. Whether you need highly flexible Adobe Commerce Development Services, broad ecommerce development services, or a specialized Shopify Development Agency, the choice should align with your business's growth stage, technical capacity, and long-term vision.
By understanding the core features and benefits of each platform and development approach, businesses can make informed decisions that support sustainable growth and operational stability—without unnecessary complexity.
0 notes
Text
Specialized Full-Stack Development Services Company

Having a robust online presence and useful web or mobile applications is now necessary in a world that prioritizes digital technology. Developing scalable and effective software solutions is essential for expansion and success, regardless of the size of your company—startup, mid-size, or large enterprise. A specialist full-stack development services provider can help in this situation by providing comprehensive, end-to-end development that addresses each layer of a digital product.
What Is Full-Stack Development?
Full- mound development refers to the capacity to construct programs that are both customer- side and garçon- side. A full- mound inventor or platoon handles everything from stoner interfaces and stoner experience( UI/ UX) to garçon sense, databases, APIs, and structure.
Front- end technologies include HTML, CSS, JavaScript, React, Angular, and Vue.js. Back- end development generally uses Node.js, Python, PHP, Ruby on Rails, Java, databases like MySQL and MongoDB, and deployment tools like AWS or Docker.
Why Choose a Specialized Full-Stack Development Company?
A specialized full-stack development services company, as opposed to generalist firms, offers deep expertise, streamlined development processes, and a strategic focus catered to your company's particular needs. The following are the main justifications for selecting such a partner:
1. Comprehensive Development Services
A full-stack business provides a one-stop shop for all of your development requirements. Everystage of the software development lifecycle—from design and wireframing to coding, testing, and deployment—is managed with ease. Compared to hiring different teams for different components, this guarantees a quicker turnaround, better integration, and lower costs.
2. Scalable Architecture
As business needs change, so should your technology. Your application can manage increasing traffic, users, and data because a specialized company builds systems with scalability in mind. They make sure your application can grow with your company, whether that means optimizing databases or increasing server capacity.
3. Secure Code and Infrastructure
Application developers should take cybersecurity very seriously. To protect your digital assets and sensitive client data, specialized teams use best practices like data encryption, secure authentication, role-based access control, and frequent code audits.
4. High-Performance Applications
The user experience depends heavily on responsiveness and speed. In order to improve loading times, lower latency, and guarantee effective backend processing, full-stack developers optimize performance on both ends. Higher retention and satisfaction are the results of seamless and interesting user interactions.
5. Customized Solutions
Every business is unique. A specialized full-stack business takes the time to learn about your unique objectives, market obstacles, and client requirements. They then create a customized solution that precisely matches your vision, be it a mobile app, SaaS product, eCommerce platform, or CRM system.
6. Agile and Collaborative Development
These businesses usually use Scrum or Agile approaches, which guarantee ongoing cooperation, openness, and iterative development. Throughout the project, you receive frequent updates, demos, and the freedom to swiftly incorporate feedback.
Core Services Offered
A specialized full-stack development services company often provides:
Custom Web Application Development
Mobile App Development (iOS, Android, Cross-platform)
UI/UX Design
Backend & Database Development
API Development & Integration
Cloud Infrastructure & DevOps
Maintenance and Support Services
Industries Served
These companies serve diverse industries including:
Healthcare
E-commerce
Finance
Real Estate
Education
Logistics
SaaS & Tech Startups
Conclusion
Any company looking to build strong, dependable, and scalable digital solutions would be wise to collaborate with a specialized full-stack development services provider. They accurately and quickly realize your ideas thanks to their proficiency in both front-end and back-end development.
A full-stack partner guarantees that you have everything you require, in one location, with unparalleled efficiency, whether you're introducing a new product, digitizing internal procedures, or updating legacy systems.
Source: https://silverspaceinc.com/full-stack-development-services/
0 notes
Text
Powering the Future of On-Demand Entertainment with Advanced OTT APP Development by ideyaLabs

OTT APP Development: Defining the New Standard in Streaming Services
The demand for seamless online streaming escalates every day. Users expect content at their fingertips through innovative and reliable platforms. Businesses need to deliver a captivating digital experience from start to finish. ideyaLabs consistently shapes this demand by offering futuristic OTT APP Development solutions for ambitious companies. Every project merge cutting-edge technology with a user-focused approach. These applications handle massive content libraries with ease while delivering a fast, engaging experience on any device.
Why Businesses Prioritize Professional OTT Platforms
Content delivery requires structure, scalability, and stability. Traditional media channels no longer suffice in an on-the-go society. ideyaLabs bridges this gap for brands that strive for a strong digital presence. With OTT APP Development, brands reach viewers on smartphones, smart TVs, tablets, and desktops. Feature-rich portals meet audiences wherever they prefer to watch. The scalability ensures seamless growth as subscriber bases expand. Each deployment prioritizes uptime, security, and rapid content delivery.
Key Components of Modern OTT APP Development
Successful OTT platforms thrive on advanced features and robust performance. ideyaLabs focuses on these core pillars:
Multi-Device Compatibility Applications function flawlessly across Android, iOS, smart TVs, web browsers, and game consoles. The interface adapts to different screen formats. This approach broadens the platform’s reach and increases viewer engagement.
Rich Content Management Systems (CMS) Manage vast digital libraries securely. Content managers benefit from categorization, tagging, scheduling, and rapid publishing tools. These systems allow for flexible monetization strategies like subscriptions, pay-per-view, or ad-supported streaming.
High-Fidelity Video Streaming Viewers get buffer-free access to HD and 4K streams. Adaptive bitrate streaming allows uninterrupted viewing even on fluctuating networks.
Personalized User Experience AI-powered recommendations help retain subscribers. The interface highlights trending shows, personalized watchlists, and thematic collections.
Secure Authentication and DRM Protect intellectual property with enterprise-grade Digital Rights Management (DRM), multi-factor authentication, and encrypted streams. Secure payment gateways build user trust.
Benefits of Choosing ideyaLabs for OTT APP Development
Companies partnering with ideyaLabs secure a competitive edge. ideyaLabs delivers:
End-to-end project management
Agile development methodologies
Custom branding and UX/UI design
Reliable post-launch maintenance
Unique features set ideyaLabs apart:
Modular architecture simplifies feature updates.
Scalable cloud infrastructure manages viral spikes in traffic.
Integrated analytics provide real-time insights into user behavior and content performance.
Innovations in Live Streaming and Video-On-Demand
Brands bring events, sports, and entertainment into living rooms around the world. ideyaLabs equips OTT platforms with live streaming modules that support high-traffic broadcasts. Low-latency protocols enable instant interaction and real-time experiences. Video-on-demand solutions ensure content is available at the click of a button. Integrated search, fast load times, and smart recommendations maximize viewer retention.
Monetizing OTT Platforms for Sustained Revenue Growth
Effective monetization turns content into consistent revenue. ideyaLabs enables seamless integration of various models:
Subscription Video On Demand (SVOD): Offer flexible tiers and packages.
Transactional Video On Demand (TVOD): Let viewers rent or buy content.
Advertising-Based Video On Demand (AVOD): Include targeted, non-intrusive ads for broader reach.
Brand partners choose the best mix for their audience. Built-in analytics help refine strategies for higher conversion and longer retention.
Data Security and Compliance: A Core Focus
Safeguarding user data remains crucial in today’s digital ecosystem. ideyaLabs implements multi-layered security protocols:
End-to-end encryption
GDPR and regional compliance
Access controls and regular audits
This proactive approach gives users peace of mind with every interaction.
Elevating User Engagement with Advanced Features
Successful OTT APP Development prioritizes unique features that spark interaction. ideyaLabs delivers:
Social media sharing
Watch parties and synchronized streaming
Multi-language support and subtitles
Seamless switching between devices
These features enhance satisfaction and keep viewers coming back.
The ideyaLabs Approach to Scalable OTT Growth
Future-focused solutions adapt as audiences grow. ideyaLabs architects each project for:
Effortless scalability with cloud-native technologies
Automated load balancing for uninterrupted streaming
Modular feature rollout for phased updates
This foundation guarantees performance during traffic spikes, major premieres, or viral content surges.
Seamless Launch and Ongoing Support for OTT Success
Launching an OTT platform becomes seamless with ideyaLabs managing the process. The team assists in:
App store listings and compliance
Ongoing bug fixes and performance optimization
Custom analytics dashboard integration
Post-launch support ensures the platform evolves with user needs and market trends.
Leading the New Age of Digital Entertainment with OTT APP Development
Enterprises understand that the future belongs to flexible, scalable, and personalized content delivery. ideyaLabs empowers brands to claim their stake in this digital landscape. The focus centers around intuitive interfaces, rapid streaming, global accessibility, and long-term scalability. With expert OTT APP Development, every client stands ready to lead in the competitive entertainment sector.
Build the Ultimate OTT Experience with ideyaLabs
OTT APP Development shapes the future of entertainment. ideyaLabs offers the expertise, vision, and technology needed to deliver a world-class digital streaming solution. Every project combines reliability, creativity, and growth potential. Businesses ready to invest in the next era of streaming choose ideyaLabs as their trusted partner.
Contact ideyaLabs to Launch Your OTT Journey
Ready to take the leap into the on-demand entertainment market? ideyaLabs transforms ideas into powerful OTT platforms. Leverage expert OTT APP Development and become a leader in digital content delivery. Reach your audience. Grow your brand. Shape the future with ideyaLabs.
0 notes
Text
Master Web Development with a MERN Full Stack Development Course

In today’s tech-driven world, web development is no longer just a niche skill—it’s a career-defining asset. As businesses transition to digital platforms, the demand for full stack developers has skyrocketed. One of the most efficient and popular stacks used by developers worldwide is the MERN stack. If you're aiming to become a proficient web developer, enrolling in a MERN full stack development course is a smart and future-proof investment.
What is the MERN Stack?
MERN stands for MongoDB, Express.js, React.js, and Node.js—a powerful combination of technologies used to build high-performing, scalable, and dynamic web applications. Here’s a quick breakdown:
MongoDB: A flexible, document-based NoSQL database that stores data in JSON format.
Express.js: A minimalist web framework for Node.js used for building APIs and server-side applications.
React.js: A front-end JavaScript library for creating interactive user interfaces with reusable components.
Node.js: A JavaScript runtime environment that enables server-side execution of code.
Together, these technologies allow developers to build modern web applications entirely in JavaScript—from the front-end UI to the back-end database.
Why Choose a MERN Full Stack Development Course?
Opting for a MERN full stack development course offers several key benefits:
1. Learn the Most In-Demand Technologies
The MERN stack is widely adopted by startups, tech companies, and large enterprises. Learning these tools ensures you're equipped with skills that are relevant in the job market.
2. Work with Real-World Projects
Most courses are project-based, meaning you’ll build applications like e-commerce platforms, social networks, blog sites, and more. These projects help you build a strong portfolio to showcase to potential employers.
3. Master End-to-End Development
With the MERN stack, you learn both client-side and server-side development. You'll be able to create full-featured apps—handling everything from UI design to server logic and database integration.
4. Career Growth and Salary Potential
MERN developers are among the highest-paid web developers. Companies prefer hiring full stack developers who can handle both front-end and back-end tasks, saving them time and resources.
What Will You Learn in a MERN Full Stack Development Course?
A well-structured course generally includes:
✅ Front-End Development
HTML5, CSS3, JavaScript (ES6+)
React.js with Hooks, Context API, Redux
Component-based UI development
Responsive design and user experience
✅ Back-End Development
Node.js fundamentals
Building APIs with Express.js
Authentication using JWT or OAuth
File handling, middleware, routing
✅ Database Integration
MongoDB basics and advanced features
Mongoose for schema modeling
Data validation, relationships, and queries
✅ DevOps and Deployment
Git & GitHub for version control
Hosting on platforms like Heroku, Vercel, Netlify
Environment variables and production readiness
Continuous Integration & Deployment (CI/CD)
Additional Benefits of a MERN Full Stack Development Course
Community Support: Join Discord groups, forums, and alumni networks for peer-to-peer learning.
Mentorship: Access to experienced instructors who guide you through concepts and project work.
Career Services: Resume reviews, mock interviews, and job placement support in premium courses.
Certification: Earn a certificate to showcase your skills to employers and clients.
Who is This Course For?
Beginners looking to start a career in web development
Computer Science students who want to build a strong portfolio
Software developers transitioning into full stack roles
Freelancers and entrepreneurs aiming to build complete web apps on their own
Final Thoughts
A mern full stack development course is more than just a learning program—it’s your entry point into the thriving world of full stack web development. Whether you're aiming to land your first tech job, build your own startup, or freelance with confidence, mastering the MERN stack equips you with the tools to succeed.
Start building real-world applications, gain hands-on experience, and unlock exciting career opportunities today with a mern full stack development course
0 notes