#JavaScript library designed
Explore tagged Tumblr posts
Text
React vs Vue vs Angular: Which One Should You Use in 2025
Overview: (React)
React continues to dominate the frontend development world in 2025, offering developers unmatched flexibility, performance, and community support. Built and maintained by Meta (formerly Facebook), React has matured into a robust UI library that startups and tech giants use.
What Is React?
React is an open-source JavaScript library designed for building fast, interactive user interfaces, primarily for single-page applications (SPAs). It's focused on the "View" layer of web apps, allowing developers to build encapsulated, reusable components that manage their state.
With the release of React 18 and innovations like Concurrent Rendering and Server Components, React now supports smoother UI updates and optimized server-side rendering, making it more future-ready than ever.
Key Aspects
Component-Based Architecture: React's modular, reusable component structure makes it ideal for building scalable UIs with consistent patterns.
Blazing-Fast UI Updates: Thanks to the virtual DOM, React efficiently updates only what's changed, ensuring smooth performance even in complex apps.
Hooks and Functional Components: With modern features like React Hooks, developers can manage state and lifecycle behavior cleanly in functional components—there is no need for class-based syntax.
Concurrent Rendering: React 18 introduced Concurrent Mode, improving performance by rendering background updates without blocking the main thread.
Massive Ecosystem: From Next.js for SSR to React Native for mobile apps, React integrates with an enormous ecosystem of tools, libraries, and extensions.
Code: App.jsx
Import React from 'react';
function App() {
return (
<div>
<h1>Hello, World! </h1>
</div>
);
}
export default App;
Entry Point: main.jsx
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App.jsx';
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<App />
</React.StrictMode>
);
HTML Template: index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>React App</title>
</head>
<body>
<div id="root"></div>
<script type="module" src="/main.jsx"></script>
</body>
</html>
Overview (Aue)
Vue.js continues to be a strong contender in the frontend framework space in 2025, primarily for developers and teams seeking simplicity without sacrificing power. Created by Evan You, Vue has grown into a mature framework known for its clean syntax, detailed documentation, and ease of integration.
What Is Vue?
Vue is a progressive JavaScript framework for building web user interfaces. Its design philosophy emphasizes incrementality—you can use Vue for a small feature on a page or scale it up into a full-fledged single-page application (SPA).
With Vue 3 and the Composition API, Vue has evolved to offer better modularity, TypeScript support, and reusability of logic across components.
Key Aspects
Lightweight and Fast: Vue has a small footprint and delivers high performance out of the box. It's fast to load, compile, and render, making it an excellent choice for performance-sensitive projects.
Simple Integration: Vue can be dropped into existing projects or used as a complete app framework. It works well with legacy systems and new apps alike.
Easy to Learn: Vue's gentle learning curve and readable syntax make it a top choice for beginners and teams with mixed skill levels.
Composition API: The Composition API in Vue 3 allows for better code reuse and more scalable application architecture, similar to React's hooks.
Code: App.vue
<template>
<div>
<h1>Hello, World! </h1>
</div>
</template>
<script setup>
</script>
<style scoped>
h1 {
color: #42b983;
}
</style>
Entry Point: main.js
import { createApp } from 'vue';
import App from './App.vue';
createApp(App).mount('#app');
HTML Template: index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>Vue App</title>
</head>
<body>
<div id="app"></div>
<script type="module" src="/main.js"></script>
</body>
</html>
Overview (Angular)
Angular, developed and maintained by Google, remains a top choice for enterprise-level applications in 2025. As a fully integrated framework, Angular provides all the tools a development team needs to build large-scale, maintainable apps out of the box.
What Is Angular?
Angular is a TypeScript-based frontend framework that includes built-in solutions for routing, state management, HTTP communication, form handling, and more. Unlike React or Vue, Angular is opinionated and follows strict architectural patterns.
Angular 17 (and beyond) introduces Signals, a new reactive system designed to improve state management and performance by offering more predictable reactivity.
Key Aspects:
All-in-One Framework: Angular offers everything you need—from routing to testing—without needing third-party libraries. This consistency is great for large teams.
Strong Typing with TypeScript: TypeScript is the default language in Angular, making it ideal for teams that prioritize type safety and tooling.
Ideal for Enterprises: With its structured architecture, dependency injection, and modular system, Angular is built for scalability, maintainability, and long-term project health.
Improved Performance: Angular 17 introduces Signals, improving reactive programming, rendering speed, and resource efficiency.
Angular Drawbacks
A steep learning curve due to its complex concepts like decorators, DI, zones, etc.
More verbose code compared to Vue and React.
Slower adoption in smaller teams and startups.
Project Setup:
bash
Copy
Edit
ng new hello-world-app
cd hello-world-app
ng serve
Component: app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `<h1>Hello, World! </h1>`,
styles: [`h1 { color: #dd0031; }`]
})
export class AppComponent {}
Module: app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
bootstrap: [AppComponent]
})
export class AppModule {}
Entry Point: main.ts
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';
import { AppModule } from './app/app.module';
platformBrowserDynamic().bootstrapModule(AppModule)
.catch(err => console.error(err));
Which One Should Use
If you're looking for simplicity and speed, especially as a solo developer or on smaller projects, Vue.js is your best bet. Its gentle learning curve and clean syntax make it ideal for quick development and maintainable code.
For scalable, dynamic applications, React strikes the perfect balance. It offers flexibility, a vast ecosystem, and strong community support, making it a top choice for startups, SaaS products, and projects that may evolve over time.
If you're building large-scale, enterprise-grade apps, Angular provides everything out of the box—routing, forms, state management—with a highly structured approach. It's TypeScript-first and built for long-term maintainability across large teams.
In short:
Choose Vue for ease and speed.
Choose React for flexibility and modern workflows.
Choose Angular for structure and enterprise power.
0 notes
Text
How can I control render blocking in an HTML and React.js application? Render blocking can significantly impact the performance of your HTML and React.js application, slowing down the initial load time and user experience. It occurs when the browser is prevented from rendering the page until certain resources, like scripts or stylesheets, are loaded and executed. To control render blocking, you can employ various techniques and optimizations. Let's explore some of them with code examples.
#libraries#web design#website#reactjs#web development#web developers#html css#ui ux design#tumblr ui#figma#blue archive#responsivedesign#responsive website#javascript#coding#developer#code#software#php script#php programming#phpdevelopment#software development#developers#php#php framework#jquery
17 notes
·
View notes
Text
Non-techical people making technical decisions is how you get 6× as many developer hired to write the frontend website code as the number of developers in the entire infrastructure team.
#codeblr#progblr#then when velocity is slow they hire another frontend developer#you know how little you value ux designers?#you should value frontend developers that much#thats how many you need to hire#also value ux more my guy#its actually good if blind people can use your website#even though it makes features come out “slower” and you cant see what changed in the website#your team should comprise of mostly backend developers#i say frontend but technically were “fullstack” developers#my hot take is that “fullstack” is just a fancier word for frontend#we write javascript and just enough of the serverside to call the apis/libraries that the real backend developers write#“Fullstack” “engineer” lmao gimme a break#That said “fullstack senior engineer” is definitely on my resume
1 note
·
View note
Text
𝐂𝐇𝐈𝐀𝐑𝐎𝐒𝐂𝐔𝐑𝐎 is a text generator designed specifically for roleplayers using discord. It helps you format your text with ease, ready to be used in Discord, making your roleplay posts stand out. This is my first attempt at a generator. If it has any bugs feel free to dm or inbox me. Please, like or reblog if it helps with your interactions.
› 𝐅𝐄𝐀𝐓𝐔𝐑𝐄𝐒 :
Bold, Italic, Strikethrough, and Underline › Want to make something bold or italic? You can click the B, I, S, or U buttons to quickly format your text while typing in the editor.
Double-Spaced Text › Enable the Double Space checkbox, and it will automatically add extra spaces between words.
Markdown-Ready Text › Once you're done typing and formatting your text, click the Generate Discord Format button. Your text will be converted into Discord's markdown syntax, ready to copy and paste into your chat!
Copy to Clipboard › After generating your formatted text, you can quickly copy it to your clipboard with a single click.
This tool uses some simple coding magic behind the scenes. Built using Quill.js, a text editor library, and some custom JavaScript, it allows you to format text in real time. When you apply styles (like bold or italic), it changes the text instantly, and when you hit Generate, it converts it into the markdown format used in Discord.
669 notes
·
View notes
Text




COMPUTER FRIENDS
ALL MY FRIENDS LIVE IN MY COMPUTER
GO MAKE FRIENDS 🫵🫵🫵
5 notes
·
View notes
Text
Cyberpunk Buttons with JavaScript: Featurastic UI
Featurastic UI Buttons is a JavaScript library that helps you create modern, responsive, cyberpunk-style buttons for web applications. It currently comes with five button variants (primary, success, secondary, error, and netural) with customizable styles and animations. The library generates unique web buttons through geometric shapes and glowing effects. Each button has an angled cut design with…
7 notes
·
View notes
Text
Funding FujoCoded: Stretch Goals!
It’s time! With our first goal met (���� thank you!), let’s talk about stretch goals. We have quite a few planned, so we're going to go through them one by one and explain what they are and why we chose them!
Before we go down the list, here's something fun:
Sticker Unlock: At 45 backers, we also unlocked one more sticker!
The goal of our campaign is to cover business expenses most of all. The unlocked content is an extra token of gratitude for your support that also helps us meet our own targets!
With that said, let's get to our stretch goals...
$4,000: "That's Why I Ship On Company Time" Ao3 Sticker
At $4,000 we'll unlock one more sticker design that you can add to your collection!
Our first version of this "shipping" sticker features VSCode and a terminal, but there's more than one type of shipping... here's to the other one!
$5,000: "Using NPM with Javascript" Article
Next up, we have our first article. Our plan is to add an Articles section to @fujowebdev where we'll collect simple, free guides to help beginners get past the roadblocks we see them encounter!
This first one will cover the basics of NPM, a core element of modern JavaScript!
"How do I install this JavaScript library? How do I run this open source JavaScript project? How can I get started creating my blog using a tool like @astrodotbuild?" are some of the most common questions we get in our Fandom Coders server.
Let's give *everyone* the answer!
$6,000: Offering Website Art Prints
Next up, we'll turn the excellent art on our website into prints! These will be (probably) 8x10-sized art prints that will look amazing without breaking the bank. Full specs soon!
...and speaking of the site, you have tried moving the windows, right?
$7,000: "Catching Up With Terminal" Article
Next, another common issue for beginner developers: how to start learning how to handle the Terminal.
This will require some research to determine the major roadblocks, which is how our project operates: active learning from those going through it all!
$8,000: "Crucial Confrontations" Article
And last (for now), something very dear to us: an article extracting some wisdom from the book "Crucial Confrontations": https://www.amazon.com/Crucial-Confrontations-Resolving-Promises-Expectations/dp/0071446524
This may seem like an unusual choice, but it highlights how our teaching goals go beyond programming to cover collaboration!
After years of working within our community, we repeatedly found that developing effective communication and confrontation skills helps our collaborators thrive. Unfortunately, the world doesn't teach us how to effectively (but kindly) hold each other accountable.
Some of our most involved collaborators have read this book and found the tools within it transformative. Given this experience, we deeply believe that making some of this wisdom easily accessible (without having to read the full book) will allow all of us to collaborate better!
If we can reach $8,000, this will enable us to test this hypothesis and learn how teaching soft skills beyond programming influences what we're able to achieve! It's a bold idea, but we're excited to see how it turns out in practice.
Help us make it there!
And that's all...for now!
If you want to hop on Twitch right now, you can join us as we put some extra polish on our shiny new FujoCoded website.
And remember, you can back our campaign here to help us achieve these goals and more:
23 notes
·
View notes
Text
I noticed a Pokerogue post on my tumblr…
My brother mods that.
Here are some tools he made:
Legendary calendar
He is so cool and talented ^^
8 notes
·
View notes
Text
I made a game in p5js about the last time I was in my old apartment following the end of my marriage. It's meant to be played in browser using a laptop or desktop. Some information is found in the code itself
5 notes
·
View notes
Text
One of the neat consequences of the design of the language I'm working on with operatives instead of macros and a modular categorical semantics, is that everything that would be a keyword or special operator in other languages can be an operative in ours, which means they can be replaced and changed and modified in user code. This means that in this language the problem of two different dependencies of the same project wanting to use different versions of the language reduces to the problem of different dependencies wanting to use different libraries, and no system of complicated features pragmas or standard command line arguments that grow and grow and grow over time is necessary. Instead we can just have different versions of the syntax as ordinary libraries, versioned, polyfilled, dependency injected. Similarly, the formally meaningful modular semantics system means that we can add and remove features without that being a global decision; an old library that uses an old semantics can be transported to a new semantics by a functor, and we can prove that the functor implements all the axioms of the original semantics in terms of the new semantics, and then the old library will work just like a native library on the new semantics, with all its types, code, proofs and properties available for use and to the optimizer. A library written next year and proven correct should still run correctly in 300 years with no maintenance specific to that library. The compiler will of course need maintenance to keep it running on new hardware generations and adopt new technology.
But this isn't just a speculative future thing. Right now, modular semantics are useful in writing a project that works on multiple targets. For example, it might be useful to write a library that does some math which can work when compiled to JavaScript, wasm, or native. These targets have huge differences between them, but with modular semantics it's possible to just write using the minimal set of primitives needed, working from abstract high level collection data structures and math operations, and then any project that works in a specific system can just request a version of your package transported to their system, and all the high level data structures and properties will be filled in with whatever their platform uses to interop at full native speed. This also works in reverse; code that runs in a webpage using webgpu can have different primitives available based on which device they will run on, and not only share common libraries and types between them but also use target specific features like garbage collection or warp level parallel operators, even if the code is mixed in a single file to collect both facets of the implementation of a specific feature, and the compiler will give a nice type error if one of them gets used in the wrong place.
6 notes
·
View notes
Text
What is Mern stack And Its importance? Before that I will Tell you the best institute for Mern stack course in Chandigarh.
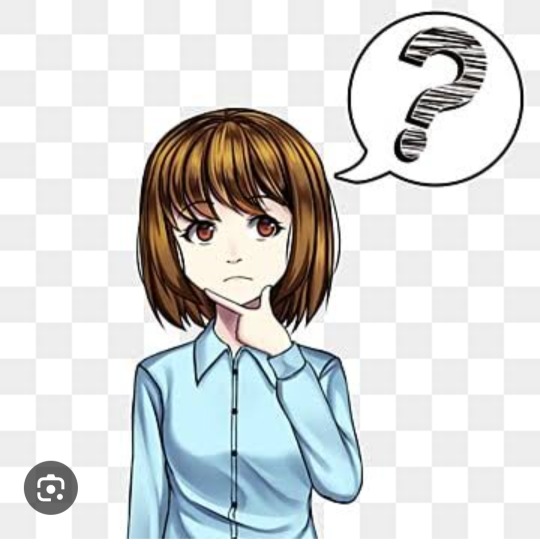
What is Mern stack?
MERN Stack is a popular JavaScript-based technology stack used for building full-stack web applications. It consists of four key technologies:
MongoDB: A NoSQL database that stores data in a flexible, JSON-like format.
Express.js: A lightweight and fast backend framework for Node.js.
React.js: A front-end JavaScript library for building user interfaces.
Node.js: A runtime environment that allows JavaScript to run on the server side.
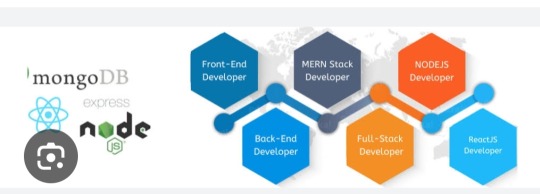
Importance of Mern Stack :
Full-Stack JavaScript – Uses JavaScript for frontend and backend, simplifying development.
High Performance – Node.js ensures fast, scalable applications.
Cost-Effective – Open-source, reducing development costs.
Rapid Development – React’s reusable components speed up UI building.
Flexibility – Suitable for web apps, SPAs, eCommerce, and real-time applications.
Scalability – MongoDB handles large data efficiently.
Strong Community Support – Large developer base ensures continuous updates and support.
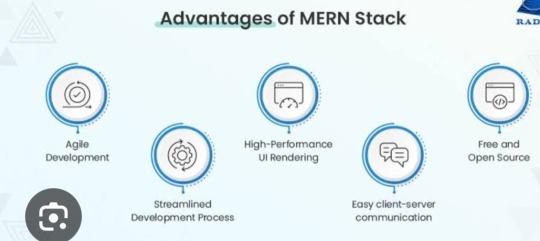
Now i will tell you the best institute for Mern stack course in Chandigarh .
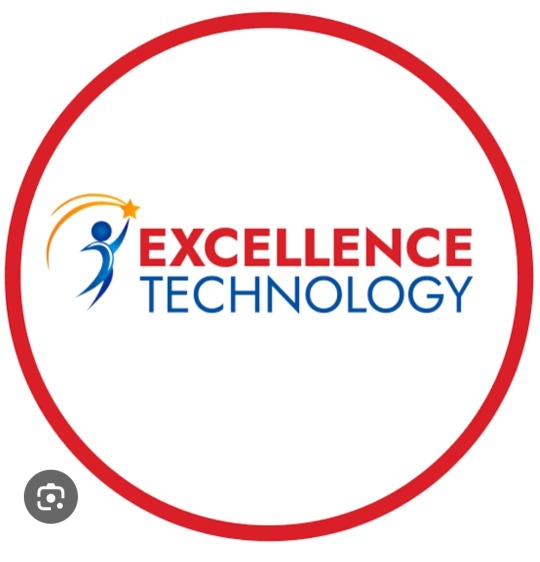
Excellence Technology is a leading EdTech (Educational technology) company dedicated to empowering individuals with cutting -edge IT skills and bridging the gap between education and industry demands. Specializing in IT training ,carrer development, and placement assistance ,the company equipts learners with the technical expertise and practical experience needed to thrive in today's competitive tech landscape. We provide IT courses like python ,Full stack Development, Web Design ,Graphic Design and Digital Marketing.
Contact Us for more details: 93177-88822
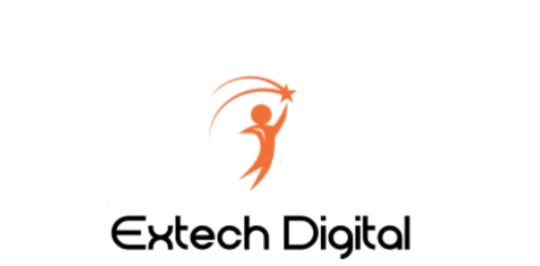
Extech Digital is a leading software development company dedicated to empowering individuals with cutting -edge IT skills and bridging the gap between education and industry demands. Specializing in IT training ,carrer development, and placement assistance ,the company equipt learners with the technical expertise and practical experience needed to thrive in today's competitive tech landscape. e provide IT courses like Python ,Full stack Development, Web Design ,Graphic Design and Digital Marketing.
Contact Us for more details: 93177-88822
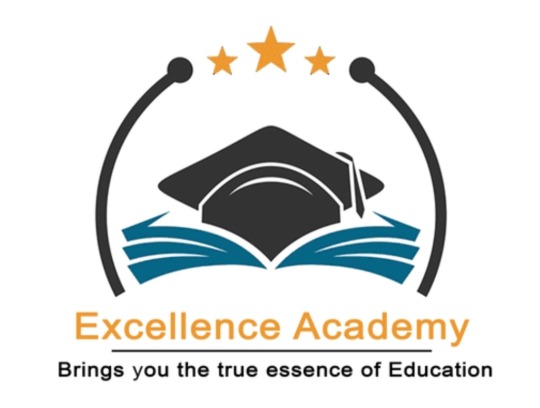
Excellence academy is a leading software development company dedicated to empowering individual with cutting edge IT skills and bridging the gap between education and industry demands.specializing in IT training, career development, and placement assistance, the company equits learners with the technical expertise and practical experience needed to thrive in today's landscape. We provide IT courses like python, full stack development,Web design, and Digital marketing.
Contact Us for more details: 93177-88822
About Author
Nikita Thakur
Mern stack AI Developer/ 2+ years of experience
Excellence technology
Professional summary
Nikita thakur is a skilled MERN Stack AI Developer with over 2 years of experience at Excellence Technology. Proficient in MongoDB, Express.js, React.js, and Node.js, she integrates AI solutions to build scalable, high-performance web applications. Nikita excels in developing innovative solutions, enhancing user experiences, and driving business growth through technology.
2 notes
·
View notes
Text
My Journey to Becoming a Frontend Developer
In today’s fast paced digital world, frontend development has become the cornerstone of delivering exceptional user experiences. From seamless web app navigation to visually stunning interfaces, a frontend developer brings ideas to life.
Why I Want to Be a Frontend Developer and How HNG Will Help Me Achieve My Goals
My journey to becoming a frontend developer is fueled by a passion for creativity, problem solving, and the desire to create applications that users love. Joining HNG internship bootcamp as a frontend developer is the perfect opportunity to sharpen my skills and gain hands on experience while contributing to real world applications.
My Motivation for Becoming a Frontend Developer
Ever since I first interacted with web technologies, I’ve been fascinated by the magic of turning code into functional and beautiful interfaces. It’s amazing how a few lines of HTML, CSS, and JavaScript can create immersive websites and applications that millions of people rely on daily.
Another reason I gravitate toward frontend development is its user centric nature. I love the idea of creating intuitive and visually appealing designs that make people’s lives easier. I’m driven by the opportunity to make technology accessible and enjoyable for everyone.
How HNG Will Help Me Grow in the Field
The HNG internship bootcamp is a game changer for aspiring developers like me. One of the most significant challenges in the tech world is bridging the gap between theoretical knowledge and real world application. HNG provides the perfect platform to tackle this by immersing participants in a fast paced, project driven environment.
Here’s how I believe HNG will accelerate my growth:
Hands On Experience: HNG’s focus on building real life applications aligns perfectly with my goal to learn by doing. By collaborating with a team to tackle real world challenges, I will develop technical skills that can’t be learned in isolation.
Mentorship and Guidance: HNG’s experienced mentors will provide valuable insights and feedback, helping me refine my coding skills, improve my design thinking, and understand industry best practices.
Exposure to Modern Tools and Frameworks: The bootcamp emphasizes modern frontend technologies. This aligns with my goal of mastering the tools that drive innovation in the industry.
Networking Opportunities: Being part of a vibrant community of like minded developers and industry experts at HNG will expand my professional network and open doors for future opportunities.
My Goals for the Internship and How I Plan to Achieve Them
During my time at HNG, my primary goal is to become a finalist in the frontend track and I plan to achieve this through other key goals such as:
Master Frontend Fundamentals: I aim to strengthen my knowledge of HTML, CSS, JavaScript, and popular libraries like React. I plan to achieve this by actively participating in all coding sessions and tasks, seeking feedback, and consistently practicing.
Contribute to Real World Projects: One of my key goals is to contribute meaningfully to the applications we build at HNG. By collaborating with team mates, meeting deadlines, and embracing challenges, I hope to add value to every project as I move up the ladder.
Enhance Problem Solving Skills: Frontend development often involves debugging and optimizing code. I will approach every challenge as an opportunity to learn and improve my critical thinking.
Develop a Strong Portfolio: By the end of the internship, I want to have a portfolio showcasing my contributions to real world applications, demonstrating my skills to potential employers that require the service of an experienced JavaScript and React developer.
Conclusion
Becoming a frontend developer is not just a career choice for me, it’s a passion that aligns with my creative and technical interests. The HNG internship bootcamp provides a unique opportunity to accelerate my growth, gain hands on experience, and prepare for the dynamic world of frontend development. With a clear vision of my goals and a commitment to learning, I’m excited to embark on this journey and make the most of this incredible opportunity at HNG.
3 notes
·
View notes
Text
I might've done a thing in my programming class- I swear I will II-ify everything I can
I couldn't just make this and not share it, so here, have fun, make Lightbulb swear, idk
#inanimate insanity#ii lightbulb#I would've done/messed with it more#but I had to get it turned in at some point#idk what else to tag this#programming#im sorry programmers#she's yapping /positive
5 notes
·
View notes
Text
JavaScript
Introduction to JavaScript Basics
JavaScript (JS) is one of the core technologies of the web, alongside HTML and CSS. It is a powerful, lightweight, and versatile scripting language that allows developers to create interactive and dynamic content on web pages. Whether you're a beginner or someone brushing up on their knowledge, understanding the basics of JavaScript is essential for modern web development.
What is JavaScript?
JavaScript is a client-side scripting language, meaning it is primarily executed in the user's web browser without needing a server. It's also used as a server-side language through platforms like Node.js. JavaScript enables developers to implement complex features such as real-time updates, interactive forms, and animations.
Key Features of JavaScript
Interactivity: JavaScript adds life to web pages by enabling interactivity, such as buttons, forms, and animations.
Versatility: It works on almost every platform and is compatible with most modern browsers.
Asynchronous Programming: JavaScript handles tasks like fetching data from servers without reloading a web page.
Extensive Libraries and Frameworks: Frameworks like React, Angular, and Vue make it even more powerful.
JavaScript Basics You Should Know
1. Variables
Variables store data that can be used and manipulated later. In JavaScript, there are three ways to declare variables:
var (old way, avoid using in modern JS)
let (block-scoped variable)
const (constant variable that cannot be reassigned)
Example:
javascript
Copy code
let name = "John"; // can be reassigned const age = 25; // cannot be reassigned
2. Data Types
JavaScript supports several data types:
String: Text data (e.g., "Hello, World!")
Number: Numeric values (e.g., 123, 3.14)
Boolean: True or false values (true, false)
Object: Complex data (e.g., { key: "value" })
Array: List of items (e.g., [1, 2, 3])
Undefined: A variable declared but not assigned a value
Null: Intentional absence of value
Example:
javascript
Copy code
let isLoggedIn = true; // Boolean let items = ["Apple", "Banana", "Cherry"]; // Array
3. Functions
Functions are reusable blocks of code that perform a task.
Example:
javascript
Copy code
function greet(name) { return `Hello, ${name}!`; } console.log(greet("Alice")); // Output: Hello, Alice!
4. Control Structures
JavaScript supports conditions and loops to control program flow:
If-Else Statements:
javascript
Copy code
if (age > 18) { console.log("You are an adult."); } else { console.log("You are a minor."); }
Loops:
javascript
Copy code
for (let i = 0; i < 5; i++) { console.log(i); }
5. DOM Manipulation
JavaScript can interact with and modify the Document Object Model (DOM), which represents the structure of a web page.
Example:
javascript
Copy code
document.getElementById("btn").addEventListener("click", () => { alert("Button clicked!"); });
Visit 1
mysite
Conclusion
JavaScript is an essential skill for web developers. By mastering its basics, you can create dynamic and interactive websites that provide an excellent user experience. As you progress, you can explore advanced concepts like asynchronous programming, object-oriented design, and popular JavaScript frameworks. Keep practicing, and you'll unlock the true power of JavaScript!
2 notes
·
View notes
Text
🌐 Top 10 Tools Every Web Developer Should Know in 2024 🚀
Hey, guys👋 If you're diving into the world of web development or looking to level up your skills, here are the top 10 tools you need to check out this year:
Visual Studio Code - The ultimate code editor with tons of extensions to boost your productivity. 💻✨
GitHub - Manage your code and collaborate with others seamlessly. 🛠️🤝
Bootstrap - Design responsive websites quickly with this popular CSS framework. 📱🎨
Figma - Collaborate on UI/UX designs in real-time with this powerful design tool. 🖌️👥
Node.js - Build scalable server-side applications using JavaScript. 🌐🔧
Webpack - Optimize your JavaScript files and manage dependencies efficiently. ⚙️📦
React.js - Create interactive UIs with this widely-used JavaScript library. ⚛️🔍
Sass - Write more maintainable CSS with features like variables and mixins. 🧩📝
Postman - Test APIs and ensure everything runs smoothly. 🔍💡
Jira - Track tasks and manage agile workflows for smooth project management. 📊📅
These tools can supercharge your development process and help you build amazing web applications. For those looking to take their projects to the next level, partnering with a web application development agency could be the key to unlocking even more potential. 🚀💼
#WebDevelopment#Coding#DeveloperTools#VisualStudioCode#GitHub#Bootstrap#Figma#NodeJS#Webpack#ReactJS#Sass#Postman#Jira#TechTips#WebApplicationDevelopment
3 notes
·
View notes
Text
The Roadmap to Full Stack Developer Proficiency: A Comprehensive Guide
Embarking on the journey to becoming a full stack developer is an exhilarating endeavor filled with growth and challenges. Whether you're taking your first steps or seeking to elevate your skills, understanding the path ahead is crucial. In this detailed roadmap, we'll outline the stages of mastering full stack development, exploring essential milestones, competencies, and strategies to guide you through this enriching career journey.
Beginning the Journey: Novice Phase (0-6 Months)
As a novice, you're entering the realm of programming with a fresh perspective and eagerness to learn. This initial phase sets the groundwork for your progression as a full stack developer.
Grasping Programming Fundamentals:
Your journey commences with grasping the foundational elements of programming languages like HTML, CSS, and JavaScript. These are the cornerstone of web development and are essential for crafting dynamic and interactive web applications.
Familiarizing with Basic Data Structures and Algorithms:
To develop proficiency in programming, understanding fundamental data structures such as arrays, objects, and linked lists, along with algorithms like sorting and searching, is imperative. These concepts form the backbone of problem-solving in software development.
Exploring Essential Web Development Concepts:
During this phase, you'll delve into crucial web development concepts like client-server architecture, HTTP protocol, and the Document Object Model (DOM). Acquiring insights into the underlying mechanisms of web applications lays a strong foundation for tackling more intricate projects.
Advancing Forward: Intermediate Stage (6 Months - 2 Years)
As you progress beyond the basics, you'll transition into the intermediate stage, where you'll deepen your understanding and skills across various facets of full stack development.
Venturing into Backend Development:
In the intermediate stage, you'll venture into backend development, honing your proficiency in server-side languages like Node.js, Python, or Java. Here, you'll learn to construct robust server-side applications, manage data storage and retrieval, and implement authentication and authorization mechanisms.
Mastering Database Management:
A pivotal aspect of backend development is comprehending databases. You'll delve into relational databases like MySQL and PostgreSQL, as well as NoSQL databases like MongoDB. Proficiency in database management systems and design principles enables the creation of scalable and efficient applications.
Exploring Frontend Frameworks and Libraries:
In addition to backend development, you'll deepen your expertise in frontend technologies. You'll explore prominent frameworks and libraries such as React, Angular, or Vue.js, streamlining the creation of interactive and responsive user interfaces.
Learning Version Control with Git:
Version control is indispensable for collaborative software development. During this phase, you'll familiarize yourself with Git, a distributed version control system, to manage your codebase, track changes, and collaborate effectively with fellow developers.
Achieving Mastery: Advanced Phase (2+ Years)
As you ascend in your journey, you'll enter the advanced phase of full stack development, where you'll refine your skills, tackle intricate challenges, and delve into specialized domains of interest.
Designing Scalable Systems:
In the advanced stage, focus shifts to designing scalable systems capable of managing substantial volumes of traffic and data. You'll explore design patterns, scalability methodologies, and cloud computing platforms like AWS, Azure, or Google Cloud.
Embracing DevOps Practices:
DevOps practices play a pivotal role in contemporary software development. You'll delve into continuous integration and continuous deployment (CI/CD) pipelines, infrastructure as code (IaC), and containerization technologies such as Docker and Kubernetes.
Specializing in Niche Areas:
With experience, you may opt to specialize in specific domains of full stack development, whether it's frontend or backend development, mobile app development, or DevOps. Specialization enables you to deepen your expertise and pursue career avenues aligned with your passions and strengths.
Conclusion:
Becoming a proficient full stack developer is a transformative journey that demands dedication, resilience, and perpetual learning. By following the roadmap outlined in this guide and maintaining a curious and adaptable mindset, you'll navigate the complexities and opportunities inherent in the realm of full stack development. Remember, mastery isn't merely about acquiring technical skills but also about fostering collaboration, embracing innovation, and contributing meaningfully to the ever-evolving landscape of technology.
#full stack developer#education#information#full stack web development#front end development#frameworks#web development#backend#full stack developer course#technology
9 notes
·
View notes