#Python development
Explore tagged Tumblr posts
Text
Discover the top 10 Python libraries every developer should know to enhance productivity and efficiency in web development and data science. These libraries, including NumPy, Pandas, and TensorFlow, provide powerful tools for data manipulation, analysis, and machine learning, making Python an indispensable language for modern development tasks.
#Python Development Services#Python Development Company#Python Development#python#best web development company in usa
2 notes
·
View notes
Text
How you can use python for data wrangling and analysis
Python is a powerful and versatile programming language that can be used for various purposes, such as web development, data science, machine learning, automation, and more. One of the most popular applications of Python is data analysis, which involves processing, cleaning, manipulating, and visualizing data to gain insights and make decisions.
In this article, we will introduce some of the basic concepts and techniques of data analysis using Python, focusing on the data wrangling and analysis process. Data wrangling is the process of transforming raw data into a more suitable format for analysis, while data analysis is the process of applying statistical methods and tools to explore, summarize, and interpret data.
To perform data wrangling and analysis with Python, we will use two of the most widely used libraries: Pandas and NumPy. Pandas is a library that provides high-performance data structures and operations for manipulating tabular data, such as Series and DataFrame. NumPy is a library that provides fast and efficient numerical computations on multidimensional arrays, such as ndarray.
We will also use some other libraries that are useful for data analysis, such as Matplotlib and Seaborn for data visualization, SciPy for scientific computing, and Scikit-learn for machine learning.
To follow along with this article, you will need to have Python 3.6 or higher installed on your computer, as well as the libraries mentioned above. You can install them using pip or conda commands. You will also need a code editor or an interactive environment, such as Jupyter Notebook or Google Colab.
Let’s get started with some examples of data wrangling and analysis with Python.
Example 1: Analyzing COVID-19 Data
In this example, we will use Python to analyze the COVID-19 data from the World Health Organization (WHO). The data contains the daily situation reports of confirmed cases and deaths by country from January 21, 2020 to October 23, 2023. You can download the data from here.
First, we need to import the libraries that we will use:import pandas as pd import numpy as np import matplotlib.pyplot as plt import seaborn as sns
Next, we need to load the data into a Pandas DataFrame:df = pd.read_csv('WHO-COVID-19-global-data.csv')
We can use the head() method to see the first five rows of the DataFrame:df.head()
Date_reportedCountry_codeCountryWHO_regionNew_casesCumulative_casesNew_deathsCumulative_deaths2020–01–21AFAfghanistanEMRO00002020–01–22AFAfghanistanEMRO00002020–01–23AFAfghanistanEMRO00002020–01–24AFAfghanistanEMRO00002020–01–25AFAfghanistanEMRO0000
We can use the info() method to see some basic information about the DataFrame, such as the number of rows and columns, the data types of each column, and the memory usage:df.info()
Output:
RangeIndex: 163800 entries, 0 to 163799 Data columns (total 8 columns): # Column Non-Null Count Dtype — — — — — — — — — — — — — — — 0 Date_reported 163800 non-null object 1 Country_code 162900 non-null object 2 Country 163800 non-null object 3 WHO_region 163800 non-null object 4 New_cases 163800 non-null int64 5 Cumulative_cases 163800 non-null int64 6 New_deaths 163800 non-null int64 7 Cumulative_deaths 163800 non-null int64 dtypes: int64(4), object(4) memory usage: 10.0+ MB “><class 'pandas.core.frame.DataFrame'> RangeIndex: 163800 entries, 0 to 163799 Data columns (total 8 columns): # Column Non-Null Count Dtype --- ------ -------------- ----- 0 Date_reported 163800 non-null object 1 Country_code 162900 non-null object 2 Country 163800 non-null object 3 WHO_region 163800 non-null object 4 New_cases 163800 non-null int64 5 Cumulative_cases 163800 non-null int64 6 New_deaths 163800 non-null int64 7 Cumulative_deaths 163800 non-null int64 dtypes: int64(4), object(4) memory usage: 10.0+ MB
We can see that there are some missing values in the Country_code column. We can use the isnull() method to check which rows have missing values:df[df.Country_code.isnull()]
Output:
Date_reportedCountry_codeCountryWHO_regionNew_casesCumulative_casesNew_deathsCumulative_deaths2020–01–21NaNInternational conveyance (Diamond Princess)WPRO00002020–01–22NaNInternational conveyance (Diamond Princess)WPRO0000……………………2023–10–22NaNInternational conveyance (Diamond Princess)WPRO07120132023–10–23NaNInternational conveyance (Diamond Princess)WPRO0712013
We can see that the missing values are from the rows that correspond to the International conveyance (Diamond Princess), which is a cruise ship that had a COVID-19 outbreak in early 2020. Since this is not a country, we can either drop these rows or assign them a unique code, such as ‘IC’. For simplicity, we will drop these rows using the dropna() method:df = df.dropna()
We can also check the data types of each column using the dtypes attribute:df.dtypes
Output:Date_reported object Country_code object Country object WHO_region object New_cases int64 Cumulative_cases int64 New_deaths int64 Cumulative_deaths int64 dtype: object
We can see that the Date_reported column is of type object, which means it is stored as a string. However, we want to work with dates as a datetime type, which allows us to perform date-related operations and calculations. We can use the to_datetime() function to convert the column to a datetime type:df.Date_reported = pd.to_datetime(df.Date_reported)
We can also use the describe() method to get some summary statistics of the numerical columns, such as the mean, standard deviation, minimum, maximum, and quartiles:df.describe()
Output:
New_casesCumulative_casesNew_deathsCumulative_deathscount162900.000000162900.000000162900.000000162900.000000mean1138.300062116955.14016023.4867892647.346237std6631.825489665728.383017137.25601215435.833525min-32952.000000–32952.000000–1918.000000–1918.00000025%-1.000000–1.000000–1.000000–1.00000050%-1.000000–1.000000–1.000000–1.00000075%-1.000000–1.000000–1.000000–1.000000max -1 -1 -1 -1
We can see that there are some negative values in the New_cases, Cumulative_cases, New_deaths, and Cumulative_deaths columns, which are likely due to data errors or corrections. We can use the replace() method to replace these values with zero:df = df.replace(-1,0)
Now that we have cleaned and prepared the data, we can start to analyze it and answer some questions, such as:
Which countries have the highest number of cumulative cases and deaths?
How has the pandemic evolved over time in different regions and countries?
What is the current situation of the pandemic in India?
To answer these questions, we will use some of the methods and attributes of Pandas DataFrame, such as:
groupby() : This method allows us to group the data by one or more columns and apply aggregation functions, such as sum, mean, count, etc., to each group.
sort_values() : This method allows us to sort the data by one or more
loc[] : This attribute allows us to select a subset of the data by labels or conditions.
plot() : This method allows us to create various types of plots from the data, such as line, bar, pie, scatter, etc.
If you want to learn Python from scratch must checkout e-Tuitions to learn Python online, They can teach you Python and other coding language also they have some of the best teachers for their students and most important thing you can also Book Free Demo for any class just goo and get your free demo.
#python#coding#programming#programming languages#python tips#python learning#python programming#python development
2 notes
·
View notes
Text
Advanced Python: Exploring Beyond the Basics
Python has rapidly grown from a beginner-friendly scripting language to a powerful tool driving modern software development, data science, machine learning, and automation. If you’ve mastered the basics—variables, loops, and functions—it’s time to elevate your skills and dive into the more advanced aspects of Python that can truly set you apart.
Why Go Beyond Basics?
Understanding advanced Python concepts not only improves code efficiency but also opens doors to complex problem-solving, cleaner design patterns, and better performance. These skills are crucial for developers aiming to work on scalable applications or contribute to open-source projects.
Key Areas to Explore in Advanced Python
Let’s look at some crucial areas that you should focus on once you’re past the fundamentals:
Decorators and Generators
Decorators enable altering a function's behaviour without modifying its original code. They are essential for clean and reusable code, especially in frameworks like Flask or Django.
Generators help manage memory efficiently by yielding data instead of returning it all at once. They're ideal for working with large datasets or real-time data streams.
Context Managers
Effectively using the with statement ensures efficient management of resources such as file streams and database connections. Custom context managers using enter() and exit() methods ensure better control and resource handling.
Metaclasses
A powerful but less commonly used feature, metaclasses let you control the behaviour of class creation. They are typically used in frameworks and libraries where dynamic class generation is needed.
Concurrency and Parallelism
Python offers several ways to execute code concurrently:
Threading: Best for I/O-bound tasks
Multiprocessing: Suitable for CPU-bound operations
AsyncIO: Ideal for writing scalable asynchronous code
Understanding when and how to use these can greatly improve application performance.
Type Hinting and Annotations
As codebases grow, readability and maintenance become crucial. Type hints (introduced in PEP 484) enhance code clarity and enable better support from tools like linters and IDEs.
Data Classes
Introduced in Python 3.7, data classes reduce boilerplate code when creating classes to store data. They automatically generate init, repr, and eq methods, making code cleaner and more readable.
Advanced Built-in Functions and Libraries
Beyond map(), filter(), and reduce(), Python’s standard library includes powerful modules like itertools, functools, and collections that can help write efficient and expressive code.
Real-World Applications
Understanding these advanced concepts is more than just theory—it translates into practical benefits:
Writing efficient machine learning pipelines
Developing high-performance APIs
Creating complex data analysis workflows
Building automation tools for businesses
Keep Practicing and Learning
Advancing in Python is a continuous process. Real growth happens through hands-on practice, building projects, and solving real-world problems. If you're based in Maharashtra and looking to sharpen your skills with expert guidance, enrolling in a Python training in Thane can be a smart move to fast-track your progress and connect with a community of passionate developers.
The journey from beginner to advanced Python developer is filled with exciting challenges and rewarding milestones. By diving into advanced topics and consistently practicing, you’ll not only become a better coder but also enhance your career prospects in today’s tech-driven world.
At DataMites Institute, we take pride in being a leading provider of practical, accessible, and high-quality training in the fast-evolving world of analytics. Our curriculum is designed to be industry-ready, covering diverse domains such as Data Science, Machine Learning, Data Mining, Tableau, Text Mining, Python Programming, Deep Learning, and Minitab. We offer globally recognized certifications, hands-on learning experiences, and expert mentorship to ensure our learners are well-prepared for success. DataMites also provides both online and offline training options to suit different learning preferences. We aim to empower individuals with the expertise and assurance required to excel in the modern data-centric world.
#python certification#python course#python training#python#pythonprogramming#python developers#python development#python skills#python programming language#python institute#python institute in india#python course in India#python training in india#python course in Thane#python training in thane#online python course#online python course in India
0 notes
Text
Machine learning with Python has grown rapidly, thanks to its clear syntax and powerful tools. If you're planning to build ML models, choosing the right libraries can save you a lot of time and help you get better results. Here are some of the top Python libraries for ML that are commonly used by developers and data scientists:
#top Python libraries for ML#machine learning in python#Python development#Best python libraries for ml
0 notes
Text
IDS is a software system designed and implemented as a dashboard application to gather system status, network statistics and application logs of different systems and analyze them.
#Custom software development company#python development company#python development#python development services#python company
0 notes
Text
Your Gateway to Expert Python Development Classes
In today’s tech-driven world, programming is no longer just a skill — it’s a necessity. Among the many languages dominating the industry, Python stands out as one of the most versatile, powerful, and beginner-friendly options. At Fusion Software Institute, we understand the importance of equipping aspiring developers with the right tools, which is why we offer industry-focused Python development classes tailored for learners at all levels.
Read more: Your Gateway to Expert Python Development Classes
Python has become the go-to language for web development, data science, artificial intelligence, automation, and more. Whether you’re a student, a professional looking to switch careers, or a hobbyist eager to build your own projects, mastering Python can open a world of opportunities. Our classes are structured to provide hands-on experience, practical knowledge, and real-world application of Python principles.
What sets our Python development classes apart is our personalized teaching approach. We maintain small batch sizes to ensure individual attention, and our instructors bring years of professional experience to the classroom. From basic syntax and data structures to advanced topics like Django, Flask, machine learning, and API integration, our curriculum is regularly updated to reflect the latest industry trends.
In addition to technical training, we emphasize project-based learning. Students at Fusion Software Institute graduate with not only theoretical knowledge but also a portfolio of completed projects that demonstrate their skills to potential employers. We also offer placement assistance, resume-building sessions, and mock interviews to help you confidently step into the job market.
If you’re ready to take your programming skills to the next level, there’s no better time to start. Python is in high demand across industries, and with expert guidance, you can harness its full potential.
Start your journey with Fusion Software Institute today and unlock endless career opportunities through our expert-led Python development classes.
Visit here: https://www.fusion-institute.com/courses/python-development
#Python Development Classes#Python Development#Python Development Courses#Python Development training
0 notes
Text
Businesses are searching for top-tier development partners who can transform ideas into scalable solutions, as Python continues to rule the software development industry. The top ten Python development firms that are expected to have significant growth in 2025 are highlighted in this blog. These companies are distinguished by their technical know-how, inventiveness, client satisfaction, and solutions that are prepared for the future. This list will assist you in finding the ideal Python partner, regardless of whether you're a corporation planning your next big digital product or a startup wanting to develop your first app.
0 notes
Text
Elevate Your Business with Python Development Services
Leverage Python development services to build scalable, secure, and high-performance applications. Discover how Python can transform your business. Read more!
0 notes
Text
Python Development Services | Bitcot
Bitcot helps businesses build fast and scalable applications with expert Python development. Your business might need a web application, a back-end system, or automation, but our developers deliver efficient and stable code that is particular to your specifications. We strive for clean development principles in order to deliver smooth execution and easy maintenance.
Let's build something incredible together. Learn more about our Python development services here: https://www.bitcot.com/python-development-services/

0 notes
Text
Python Development Company in New York
LDS Engineers – The Leading Python Development Company in New York, when it comes to Python development, LDS Engineers is a famous and relied-on name in New York. We focus on Python software program development and provide remarkable solutions to corporations across various industries. With years of experience in the discipline, we've correctly added custom net packages, dynamic websites, and computing device programs to customers throughout the USA, UK, India, and Australia.
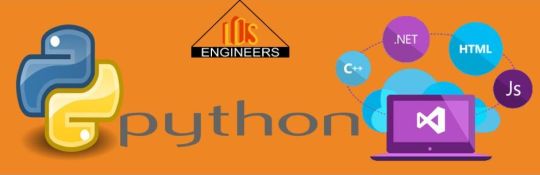
Why Choose Python for Development?
Python is one of the maximum popular and flexible programming languages in the global these days. Businesses choose Python due to the fact it's miles:
Fast and efficient: Python offers brief development, which saves money and time.
Flexible: It may be used for net development, software program programs, artificial intelligence (AI), system learning, and more.
Reliable: Python packages run smoothly and offer amazing performance.
Secure: It comes with built-in protection capabilities, making it a first-rate desire for developing business packages.
At LDS Engineers, we make the maximum of Python’s capabilities to construct sturdy, scalable, and value-powerful applications for our clients.
Our Python Development Services
We provide a wide variety of Python development offerings, such as:
Python Web Development
We increase dynamic and feature-wealthy websites using Python frameworks like Django and Flask. Our websites are consumer-pleasant, responsive, and SEO-optimized.
Custom Python Software Development
Whether you want a custom business utility, CRM, or ERP software, our crew can create a powerful Python-primarily based solution tailor-made to your wishes.
Python Mobile App Development
We build cross-platform cell programs with the usage of Python, ensuring an unbroken experience for both Android and iOS users.
AI and Machine Learning Solutions
Python is widely used for synthetic intelligence and machine-mastering applications. Our group focuses on developing AI-powered solutions for companies.
Data Science and Analytics
We help businesses analyze and visualize statistics the usage of Python-based totally equipment, making it simpler to make knowledgeable business decisions.
Python API Development and Integration
We increase and integrate APIs to beautify the functionality of your Python packages.
Support and Maintenance
Our job doesn’t quit with development. We provide ongoing support, updates, and renovation to make certain that your Python software runs easily.
Why Choose LDS Engineers?
With years of experience in Python development, LDS Engineers sticks out as a dependent Python development organization in New York. Here’s why businesses decide to work with us:
Expert Team: Our group consists of professional Python developers with experience in diverse industries.
Custom Solutions: We tailor our Python development services to fulfill your enterprise's specific needs.
Cost-Effective Services: We deliver extraordinary Python programs at aggressive charges.
On-Time Delivery: We value a while and make sure that each initiative is finished within time limits.
Global Presence: We provide Python development offerings not just in the US but also within the UK, India, and Australia.
Let’s Build Your Next Python Project!
If you’re looking for a reliable Python development services in New York, appearance is not in addition to LDS Engineers. Whether you want a website, cell app, AI answer, or business utility, we are here to help.
Contact us nowadays to discuss your task and get a custom Python answer that suits your business wishes!
python development, python software development, python software development services, python web application development, python website development, python development, python development in us, python development company.
#python development#python software development#python software development services#python web application development#python website development#python development in us#python development company#newyork#nyc#newyorkcity#usa#photography#art#explorepage#rap#trending
0 notes
Text
Top 10 Projects to Build as a Python Full Stack Developer
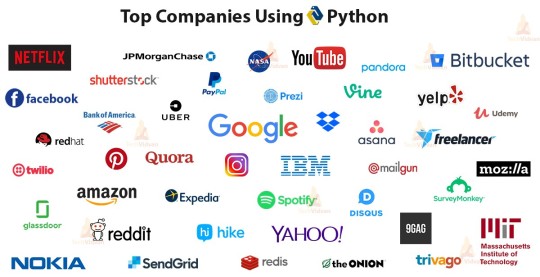
Building real-world projects is one of the best ways to master Python Full Stack Development. Whether you are a recent graduate, an aspiring developer, or a professional looking to enhance your skills, working on these projects will help you gain hands-on experience and showcase your abilities to potential employers. Below, we outline 10 practical and impactful project ideas to help you grow as a Python Full Stack Developer.
1. Blog Platform
Overview: Create a blogging platform where users can register, log in, create, edit, and delete blog posts. Include features like user authentication, categories, and comments. Technologies Involved: Django for backend, HTML/CSS for frontend, SQLite or PostgreSQL for database. Skills Gained: Authentication systems, CRUD operations, and database integration. Challenges: Implementing a secure login system and designing a user-friendly UI.
2. E-Commerce Website
Overview: Build an e-commerce platform where users can browse products, add them to a cart, and checkout with payment integration. Technologies Involved: Django or Flask for backend, React for frontend, Stripe or PayPal for payment gateway. Skills Gained: API integration, session management, and frontend-backend communication. Challenges: Implementing payment gateways and managing inventory.
3. To-Do List Application
Overview: Develop a simple to-do list app where users can create, edit, and delete tasks. Add features like due dates and priority levels. Technologies Involved: Flask for backend, Bootstrap for frontend, SQLite for database. Skills Gained: RESTful APIs, data validation, and responsive design. Challenges: Ensuring smooth user experience across devices.
4. Social Media Dashboard
Overview: Create a dashboard where users can view and manage posts, followers, and analytics from multiple social media platforms. Technologies Involved: Django REST Framework (DRF), React, and Chart.js for data visualization. Skills Gained: API handling, data visualization, and advanced JavaScript frameworks. Challenges: Integrating third-party APIs and managing data consistency.
5. Online Forum
Overview: Develop a discussion forum where users can create threads, reply to posts, and vote on responses. Technologies Involved: Flask for backend, PostgreSQL for database, and Materialize for frontend. Skills Gained: Managing user-generated content, search functionality, and thread management. Challenges: Moderating content and implementing a voting system.
6. Real-Time Chat Application
Overview: Build a real-time chat application with group and private messaging features. Technologies Involved: Django Channels, WebSockets, and Redis. Skills Gained: Real-time communication, WebSocket programming, and performance optimization. Challenges: Managing real-time data and ensuring security in messaging.
7. Weather Forecast App
Overview: Create a weather application where users can search for the current weather and forecasts for their location. Technologies Involved: Flask, OpenWeather API, and Bootstrap. Skills Gained: API consumption, error handling, and responsive design. Challenges: Handling API limits and designing an intuitive UI.
8. Portfolio Website
Overview: Build a personal portfolio website showcasing your projects, resume, and contact information. Technologies Involved: Django or Flask for backend, HTML/CSS/JavaScript for frontend. Skills Gained: Deployment, personal branding, and responsive design. Challenges: Optimizing for performance and SEO.
9. Online Examination System
Overview: Develop a platform where instructors can create exams, and students can take them with automated grading. Technologies Involved: Django, React, and PostgreSQL. Skills Gained: Role-based access control, real-time grading, and database design. Challenges: Ensuring scalability and handling multiple concurrent users.
10. Expense Tracker
Overview: Create an expense tracker that allows users to log, categorize, and visualize their expenses. Technologies Involved: Flask, Chart.js, and SQLite. Skills Gained: Data visualization, CRUD operations, and user management. Challenges: Designing effective visualizations and managing user data securely.
Conclusion
These projects not only help you practice Full Stack Development but also serve as a testament to your skills when showcased in your portfolio. Start with simpler projects and gradually move to complex ones as you build your expertise.
If you want to master Python Full Stack Development and work on real-world projects, Syntax Minds is here to help!
Address: Flat No.202, 2nd Floor, Vanijya Complex, Beside VRK Silks, KPHB, Hyderabad - 500085 Phone: 9642000668, 9642000669 Email: [email protected]
#artificial intelligence#data science#deep learning#machine learning#data scientist#data analytics#Python#Python Development#Full Stack Development
0 notes
Text
Top 10 Sites to Hire Python Developers
Choosing the right Python development partner is crucial for the life streaming of your project. In any kind of business, it is now crucial to have a team of trusted Python developers, especially in such a competitive technologically advanced world. The need for Python developers arises in cases where you would like to build fabulous web applications, implement sophisticated intelligent business processes and procedures or design and develop various types of software solutions.
0 notes
Text
BEGINNING YOUR CAREER IN PYTHON : COMPREHENSIVE GUIDE
Starting your career in Python can be a rewarding endeavor; indeed, this is due to its versatility and demand in various fields. Therefore, here’s a comprehensive guide to help you navigate your journey from a beginner to a proficient Python developer.
1. Understanding Python Basics
What is Python basics?
A high-level, interpreted programming language, notably known for its readability and simplicity.
Installation
"First, download and install Python from the official website.
Then, set up an IDE (Integrated Development Environment) like PyCharm, VSCode, or Jupyter Notebook."
2. Deepening Your Knowledge
3. Exploring Libraries and Frameworks
4. Hands-On Practice
5. Version Control
1 note
·
View note
Text
Machine learning is revolutionizing industries with its power to turn data into actionable insights. If you're diving into this field, you need to be familiar with the right tools to build, train, and deploy your models efficiently. Here’s a look at the Top Python Libraries For Machine Learning that every aspiring data scientist or engineer should know about.
0 notes
Text
Need a Python expert? I offer freelance Python development for web apps, data processing, and automation. With strong experience in Django, Flask, and data analysis, I provide efficient, scalable solutions tailored to your needs. Let’s bring your project to life with robust Python development. more information visit my website : www.karandeeparora.com
#web services#python developers#data processing#django#data analysis#flask#python development#automation
0 notes
Text
Error Handling in Python: Try, Except, and More
Python, like many programming languages, uses error handling to catch and manage exceptions, preventing your programs from crashing. By implementing proper error handling, you can ensure smoother user experiences and more maintainable code. In this blog, we will dive into the basics of handling errors in Python using the try, except, finally, and else blocks.
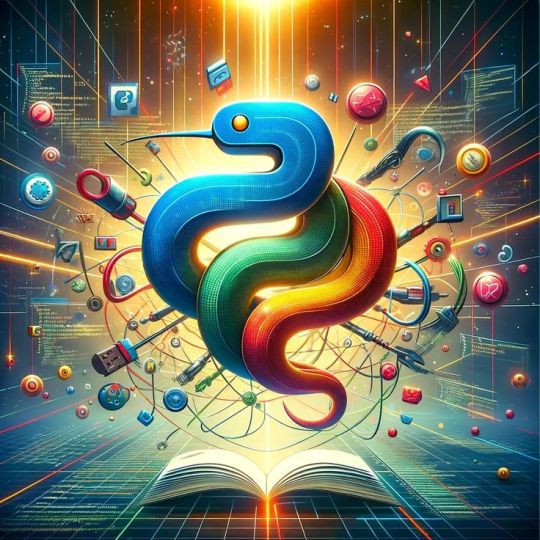
Basics of Error Handling in Python
Errors or exceptions in Python come in many forms: ValueError, ZeroDivisionError, FileNotFoundError, etc. The simplest way to handle these errors is by using a try and except block.
try:
x = 10 / 0
except ZeroDivisionError:
print("You can't divide by zero!")
In this example, the code inside the try block attempts to divide by zero, which would normally cause a crash. However, with except, the program catches the ZeroDivisionError and prints a user-friendly message.
Multiple Exceptions
You can handle multiple exceptions by specifying them in the except block or by using separate blocks.
try:
x = int(input("Enter a number: "))
result = 10 / x
except ValueError:
print("You must enter a valid number!")
except ZeroDivisionError:
print("You can't divide by zero!")
Here, if the user enters something that is not a number, the ValueError will be caught. If they enter zero, the ZeroDivisionError will be caught. Multiple except blocks allow for more granular error handling.
Using else and finally
Python allows for even more control using else and finally. The else block executes only if no exceptions are raised, while the finally block always runs, regardless of whether an exception occurs.
try:
file = open('data.txt', 'r')
content = file.read()
except FileNotFoundError:
print("The file does not exist.")
else:
print("File read successfully!")
finally:
print("Closing file.")
file.close()
In this example, the else block runs if no exception occurs during file opening and reading. Regardless of the outcome, the finally block ensures that the file is closed.
Custom Exceptions
You can also define your own exceptions by subclassing Python’s built-in Exception class. This is useful when you need more specific error reporting for custom scenarios.
class NegativeNumberError(Exception):
pass
def check_positive(number):
if number < 0:
raise NegativeNumberError("Negative numbers are not allowed!")
In this case, a custom exception is raised if the number is negative, offering precise control over error conditions.
Conclusion
Error handling is a critical part of Python programming, making your code robust and user-friendly. By mastering try, except, else, and finally, you can prevent unexpected crashes and create a smoother user experience.
Want to learn more about Python? Enroll in our Python for Beginners course now and master error handling, data structures, and more!
0 notes