#codingtips
Explore tagged Tumblr posts
Text
"Introduction to Algorithms" by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein (often referred to as CLRS) is one of the most comprehensive and widely used textbooks on algorithms. It provides a deep dive into the design, analysis, and implementation of algorithms, making it an essential resource for students, programmers, and computer science enthusiasts. Below is a step-by-step breakdown of the outcomes you can expect from studying this book:
#Algorithms#ComputerScience#CS#TechBooks#SoftwareDevelopment#DataStructures#AlgorithmDesign#Programming#Coding#TechLearning#AlgorithmAnalysis#ComputerScienceBooks#DataStructuresAndAlgorithms#AlgorithmOptimization#ProgrammingLanguages#AlgorithmTheory#TechEducation#SoftwareEngineering#ProblemSolving#CodingTips#AlgorithmicThinking#CSBooks#TechTutorial#AlgorithmicDesign#AlgorithmImplementation
0 notes
Text
📚 Comparing Java Collections: Which Data Structure Should You Use?
If you're diving into Core Java, one thing you'll definitely bump into is the Java Collections Framework. From storing a list of names to mapping users with IDs, collections are everywhere. But with all the options like List, Set, Map, and Queue—how do you know which one to pick? 🤯
Don’t worry, I’ve got you covered. Let’s break it down in simple terms, so you can make smart choices for your next Java project.
🔍 What Are Java Collections, Anyway?
The Java Collection Framework is like a big toolbox. Each tool (or data structure) helps you organize and manage your data in a specific way.
Here's the quick lowdown:
List – Ordered, allows duplicates
Set – Unordered, no duplicates
Map – Key-value pairs, keys are unique
Queue – First-In-First-Out (FIFO), or by priority
📌 When to Use What? Let’s Compare!
📝 List – Perfect for Ordered Data
Wanna keep things in order and allow duplicates? Go with a List.
Popular Types:
ArrayList – Fast for reading, not so much for deleting/inserting
LinkedList – Good for frequent insert/delete
Vector – Thread-safe but kinda slow
Stack – Classic LIFO (Last In, First Out)
Use it when:
You want to access elements by index
Duplicates are allowed
Order matters
Code Snippet:
java
🚫 Set – When You Want Only Unique Stuff
No duplicates allowed here! A Set is your go-to when you want clean, unique data.
Popular Types:
HashSet – Super fast, no order
LinkedHashSet – Keeps order
TreeSet – Sorted, but a bit slower
Use it when:
You care about uniqueness
You don’t mind the order (unless using LinkedHashSet)
You want to avoid duplication issues
Code Snippet:
java
🧭 Map – Key-Value Power Couple
Think of a Map like a dictionary. You look up values by their unique keys.
Popular Types:
HashMap – Fastest, not ordered
LinkedHashMap – Keeps insertion order
TreeMap – Sorted keys
ConcurrentHashMap – Thread-safe (great for multi-threaded apps)
Use it when:
You need to pair keys with values
You want fast data retrieval by key
Each key should be unique
Code Snippet:
java
⏳ Queue – For First-Come-First-Serve Vibes
Need to process tasks or requests in order? Use a Queue. It follows FIFO, unless you're working with priorities.
Popular Types:
LinkedList (as Queue) – Classic FIFO
PriorityQueue – Sorted based on priority
ArrayDeque – No capacity limit, faster than LinkedList
ConcurrentLinkedQueue – Thread-safe version
Use it when:
You’re dealing with task scheduling
You want elements processed in the order they come
You need to simulate real-life queues (like print jobs or tasks)
Code Snippet:
java
🧠 Cheat Sheet: Pick Your Collection Wisely
⚙️ Performance Talk: Behind the Scenes
💡 Real-Life Use Cases
Use ArrayList for menu options or dynamic lists.
Use HashSet for email lists to avoid duplicates.
Use HashMap for storing user profiles with IDs.
Use Queue for task managers or background jobs.
🚀 Final Thoughts: Choose Smart, Code Smarter
When you're working with Java Collections, there’s no one-size-fits-all. Pick your structure based on:
What kind of data you’re working with
Whether duplicates or order matter
Performance needs
The better you match the collection to your use case, the cleaner and faster your code will be. Simple as that. 💥
Got questions? Or maybe a favorite Java collection of your own? Drop a comment or reblog and let’s chat! ☕💻
If you'd like me to write a follow-up on concurrent collections, sorting algorithms, or Java 21 updates, just say the word!
✌️ Keep coding, keep learning! For More Info : Core Java Training in KPHB For UpComing Batches : https://linktr.ee/NIT_Training
#Java#CoreJava#JavaProgramming#JavaCollections#DataStructures#CodingTips#DeveloperLife#LearnJava#ProgrammingBlog#TechBlog#SoftwareEngineering#JavaTutorial#CodeNewbie#JavaList#JavaSet#JavaMap#JavaQueue#CleanCode#ObjectOrientedProgramming#BackendDevelopment#ProgrammingBasics
0 notes
Text
Just starting your web dev journey?🚀
Don't get overwhelmed!
TechySchools makes it easy to understand the basics. Remember, HTML gives structure, CSS adds style, and JavaScript brings it to life! Ready to learn more?
Check out TechySchools!
0 notes
Text
Finding the right balance between deep focus and team collaboration can be a challenge for developers. 🧑💻 Too many meetings? Constant interruptions? Learn how to stay productive without losing touch with your team!
With Teamcamp, you can streamline communication, minimize distractions, and keep your projects on track—all while maintaining deep focus. 🚀
💬 How do you manage deep work and collaboration? Share your thoughts in the comments!
#SoftwareDevelopment#DeepWork#ProductivityHacks#DevCommunity#TechLife#ProjectManagement#CodingTips#DeveloperMindset#Teamwork#AgileDevelopment#SoftwareEngineering#FocusTime
0 notes
Text
🚀 5 Python Full Stack Tricks You Must Know!
✅ Use DRF for APIs – Simplifies API dev with built-in auth & serialization.
✅ Dockerize Your App – Deploy anywhere with ease.
✅ Secure APIs with JWT – Strong authentication with JSON Web Tokens.
✅ Use Lazy Loading (React) – Boost performance by loading components on demand.
💡 Follow @pythonfullstackmasters for more tips!
🔥 Drop a 🔥 if you found this useful!
#PythonFullStack#Django#ReactJS#WebDev#FullStackTips#FullStackDeveloper#PythonDeveloper#WebDevelopment#CodingTips#ProgrammersLife#MERNvsPERN#FullStackLife#WebDesign#PythonTricks#SoftwareDeveloper#CodeNewbie
0 notes
Text
CSS Questions & Answers – Styling Tables
#quizsquestion#CSS#WebDesign#StylingTables#FrontendDevelopment#WebDevelopment#CSSQuestions#CodingTips#WebDesignTips#TableStyling#LearnCSS
0 notes
Text
🌟 Git Branch Best Practices: A Must-Know Guide for Developers! 🌟
Ever struggled with messy repositories, merge conflicts, or lost code? 🤯 Managing Git branches effectively is the key to smoother collaboration and efficient development!
💡 In our latest guide, we break down: ✅ Why Git branching strategies matter ✅ Different Git workflows (Git Flow, GitHub Flow, Trunk-Based) ✅ Naming conventions to keep your repo organized ✅ Best practices for branch creation, merging, and deletion
🚀 Level up your Git game today! Read the full blog here: https://techronixz.com/blogs/git-branch-best-practices-complete-guide
💬 Which Git strategy do you prefer? Drop your thoughts in the comments! 💻✨
0 notes
Text
#WebDevelopment#AppDevelopment#TechSolutions#SoftwareEngineering#WebDevChallenges#CodingTips#ProgrammingLife#DevCommunity#TechInnovation#WebApp
0 notes
Text
Handling Android 13+ Notification Permissions in React Native
Learn how to request and manage notification permissions in React Native for Android 13+ using best practices. Ensure a smooth user experience with the right implementation.
#ReactNative#Android13#MobileDevelopment#PushNotifications#AppPermissions#AndroidDev#CodingTips#HireReactNativeDevelopers
0 notes
Text
Efficient Time Control in Toggle Timer for Better Accuracy
#elections#ToggleTimer#TimeManagement#TimerControl#CodingTips#Developer#SoftwareEngineering#TechInnovation#Automation#Efficiency#programming
0 notes
Text

🚀 Guidewire Performance Tips! 🚀
Boost your Guidewire efficiency with these 5 pro tips:
✅ Optimize Queries – Fetch only what’s needed. ✅ Minimize PCF Reloads – Avoid unnecessary UI refreshes. ✅ Use Batch Processing – Handle large data smartly. ✅ Optimize Gosu Code – Write clean & efficient logic. ✅ Enable Logging & Monitoring – Track performance issues early.
📍 Learn more: www.guidewiremasters.in 📞 +91 9885118899
✨ JOIN NOW & Upgrade Your Skills! 💡💻
0 notes
Text
TYPO3 CLI Commands Explained – How to Manage Your Website Faster
Introduction
TYPO3 websites require regular maintenance tasks such as clearing cache, updating extensions, and managing users. Doing this manually in the backend takes time and effort. TYPO3 CLI commands allow you to perform these tasks quickly using the command line. This guide will cover the most useful CLI commands for TYPO3 website administrators.

Why You Should Use TYPO3 CLI Commands
Using CLI commands instead of the backend interface has major benefits:
Faster execution – A single command replaces multiple clicks in the backend.
Fewer errors – Direct commands reduce human mistakes.
Automation – CLI commands can be included in scripts to handle repetitive tasks.
More control – Advanced commands offer features not available in the backend.
How to Access TYPO3 CLI
Step 1: Open the Terminal and Navigate to TYPO3 Directory
Before using CLI commands, go to your TYPO3 installation folder.
Type: cd /path/to/typo3
Step 2: View All Available Commands
To list all commands, type: vendor/bin/typo3
Important TYPO3 CLI Commands
Clear Cache Remove old cache data to refresh the site: vendor/bin/typo3 cache:flush
List Installed Extensions View all installed TYPO3 extensions: vendor/bin/typo3 extension:list
Enable or Disable Extensions Activate an extension: vendor/bin/typo3 extension:activate extension_key Deactivate an extension: vendor/bin/typo3 extension:deactivate extension_key
Reset a TYPO3 Backend User’s Password If a user is locked out, reset their password: vendor/bin/typo3 backend:resetpassword username
Create a New TYPO3 Admin User To add a new backend admin: vendor/bin/typo3 backend:user:create --username=newuser --password=newpassword [email protected]
Update Language Packs Refresh TYPO3’s translation files: vendor/bin/typo3 language:update
List All TYPO3 Websites To see all configured websites: vendor/bin/typo3 site:list
Advanced TYPO3 CLI Commands
Update Database Schema
For database updates after installing an extension, run: vendor/bin/typo3 database:updateschema
Execute Scheduled Tasks
Manually run TYPO3’s scheduler: vendor/bin/typo3 scheduler:run
Real-Life Examples of Using TYPO3 CLI
Clear cache quickly after making changes Instead of navigating the backend, simply run: vendor/bin/typo3 cache:flush
Reset a forgotten backend user password If a user is locked out, type: vendor/bin/typo3 backend:resetpassword admin
Automatically update all language files Keep translations up to date by running: vendor/bin/typo3 language:update
Final Thoughts
TYPO3 CLI commands are powerful tools for website administrators and developers. They save time, reduce errors, and make website management more efficient.
By learning and using these commands, you can streamline your TYPO3 workflow and manage websites like a pro! 🚀
0 notes
Text
3/3
Core Java: A Beginner’s Guide to Java Programming
Java has stood the test of time as one of the most widely used programming languages. From building desktop applications to powering large-scale enterprise systems, its versatility has made it indispensable. If you're just stepping into the world of programming, learning Core Java is an excellent place to start.
What is Core Java?
Core Java refers to the fundamental aspects of Java programming, forming the backbone of the language. It encompasses essential concepts such as object-oriented programming (OOP), memory management, exception handling, and multi-threading. Unlike Advanced Java, which includes frameworks like Spring and Hibernate, Core Java focuses on the basic building blocks needed to write efficient and scalable applications.
Why Learn Core Java?
Before diving into web or enterprise development, mastering Core Java equips you with:
A solid foundation in OOP principles – Essential for writing clean, reusable, and modular code.
Platform independence – Java’s “Write Once, Run Anywhere” philosophy allows applications to run on multiple platforms without modification.
Memory management – Java handles memory allocation and garbage collection efficiently.
Robust exception handling – Prevents unexpected crashes and improves software reliability.
Multi-threading capabilities – Enables efficient execution of concurrent tasks.
Setting Up Java
To start coding in Java, follow these simple steps:
Install Java Development Kit (JDK) – Download the latest version from Oracle’s official website.
Set up an Integrated Development Environment (IDE) – Popular choices include:
IntelliJ IDEA – A feature-rich, user-friendly IDE.
Eclipse – A powerful, open-source Java IDE.
NetBeans – Great for beginners due to its built-in tools.
Verify the installation by running: shCopyEditjava -version This command confirms that Java is installed and accessible.
Understanding the Basics of Java
1. Hello World Program
The classic first step in any language is printing "Hello, World!" to the console:
java
CopyEdit
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
public class HelloWorld – Defines a class named HelloWorld.
public static void main(String[] args) – The entry point of any Java program.
System.out.println() – Prints text to the console.
2. Variables and Data Types
Java has various data types:
java
CopyEdit
int age = 25; double price = 99.99; char letter = 'A'; boolean isJavaFun = true; String message = "Java is awesome!";
Each type serves a distinct purpose, from storing numbers to handling text.
3. Control Flow Statements
Decisions and loops are integral to programming:
java
CopyEdit
if (age > 18) { System.out.println("You are an adult."); } else { System.out.println("You are a minor."); }
Loops enable repeated execution:
java
CopyEdit
for (int i = 0; i < 5; i++) { System.out.println("Iteration: " + i); }
4. Object-Oriented Programming (OOP)
Java follows OOP principles, including:
Encapsulation – Data hiding through private fields and public methods.
Inheritance – Reusing code through parent-child class relationships.
Polymorphism – Methods behaving differently based on input.
Abstraction – Hiding implementation details and exposing only necessary functionality.
Example:
java
CopyEdit
class Animal { void makeSound() { System.out.println("Animal makes a sound"); } } class Dog extends Animal { void makeSound() { System.out.println("Dog barks"); } } public class Test { public static void main(String[] args) { Animal myDog = new Dog(); myDog.makeSound(); // Output: Dog barks } }
Next Steps in Java Learning
Once you've grasped Core Java, you can explore:
Collections Framework – Handling groups of objects efficiently.
Multi-threading – Running tasks in parallel for improved performance.
File Handling – Reading and writing files.
JDBC – Connecting Java applications to databases.
Final Thoughts
Java continues to be a powerhouse in software development, with Core Java serving as the gateway to mastering the language. Whether you're aiming to build Android apps, enterprise solutions, or cloud-based systems, a strong grasp of Core Java will set you on the path to success.
Feedback Time!
What would you like to improve in this article? A) Add more beginner-friendly examples B) Include more advanced topics C) Simplify explanations D) Provide additional resources for learning Java E) It’s perfect as is!
Comment Below
#Java#CoreJava#JavaProgramming#CodingForBeginners#LearnToCode#Programming#Tech#SoftwareDevelopment#OOP#CodeNewbie#TechEducation#JavaDeveloper#ProgrammingBasics#CodingTips#DeveloperLife
0 notes
Text
Coding Made Fun: Discover the World of Web Development
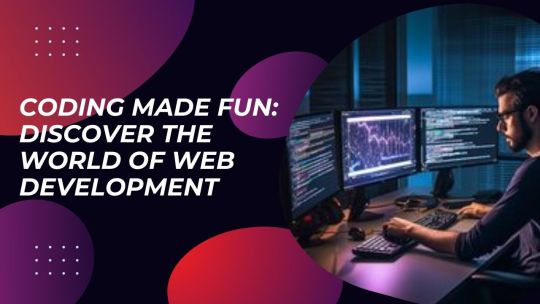
In today’s digital age, coding has become one of the most essential skills to master, and web development is at the heart of it all. From creating stunning websites to building complex web applications, web development allows you to bring your ideas to life on the internet. For students and beginners, it might seem overwhelming at first, but with the right approach and guidance—such as enrolling in web development classes in Yamuna Vihar—coding can become an enjoyable and rewarding journey.
Why Learn Web Development?
Unleash Your Creativity Web development is a blend of logic and creativity. Whether it’s designing visually appealing websites or crafting interactive features, this field lets you showcase your artistic side while solving real-world problems.
High Demand for Skills Every business today needs an online presence, and web developers are at the forefront of this digital transformation. Skilled developers are in high demand across industries, offering lucrative job opportunities.
Versatility and Growth Web development isn’t just limited to coding. You’ll learn about design principles, user experience (UX), and even backend operations like database management. This versatility opens doors to diverse career paths.
Build While You Learn One of the best parts about web development is that you can create projects right from day one. Whether it’s a personal blog, a portfolio website, or even a small e-commerce platform, each project helps you gain practical knowledge and boost your confidence.
What Does Web Development Involve?
Web development can be divided into three main areas:
Frontend Development This focuses on the visible parts of a website—the design, layout, and user interaction. Tools like HTML, CSS, and JavaScript are the building blocks of frontend development.
Backend Development The backend is what powers a website behind the scenes. It involves server-side programming, database management, and application logic. Languages like Python, PHP, and frameworks like Node.js are commonly used.
Full-Stack Development A combination of both frontend and backend, full-stack developers can handle all aspects of web development, making them highly valuable in the industry.
How to Make Coding Fun
Start Small Don’t aim for perfection right away. Begin with simple projects like a to-do list app or a personal website.
Engage with the Community Join online forums or attend coding meetups. Collaborating with others can make learning more enjoyable and expose you to different perspectives.
Learn with Guidance If you’re new to coding, enrolling in a web development coaching centre in Yamuna Vihar can provide structured learning and mentorship, helping you avoid common pitfalls.
Experiment and Explore The beauty of coding is that there are no limits. Try new libraries, experiment with design ideas, and explore the endless possibilities of web development.
Where to Learn?
For those ready to dive into the coding world, professional courses are a great way to get started. Enroll in a web development training institute in Uttam Nagar or similar institutes near you. These programs offer hands-on experience, teaching you everything from the basics to advanced concepts in web development.
Conclusion
Web development is more than just coding—it’s about creating experiences, solving problems, and expressing ideas through technology. Whether you want to build your dream website or kickstart a tech career, web development offers endless possibilities. Begin your journey today by exploring web development in training institute Uttam Nagar With dedication and the right guidance, coding can indeed be fun and your gateway to a bright future!
Suggested Links
Full Stack Web Development Training Institute
Java Full Stack Developer Course
Web Development coaching centre
#coding#codingtips#web development#webdesign#Web Development training#Web Development training Institute in Yamuna vihar#web development course#wordpress development#website design
0 notes
Text
Struggling to keep your software development projects on track? You're not alone! 🚀 Learn how to cut through the chaos, improve collaboration, and deliver projects smoothly. Master the art of efficient project management today! Teamcamp makes project management effortless with intuitive tools designed for developers. Try it out and keep your workflow organized!
💬 What’s your biggest challenge in managing projects? Let’s discuss in the comments!
#SoftwareDevelopment#ProjectManagement#DevCommunity#TechLeadership#AgileDevelopment#SoftwareEngineering#TechBlog#DeveloperLife#CodingTips#Scrum#Productivity#Programming#DevLife
1 note
·
View note
Text
🚀 Python Full Stack Tips & Tricks! 🚀
Boost your Full Stack skills with these key insights:
✅ Choose the Right Tech Stack ✅ Build Scalable REST APIs (Django/Flask) ✅ Optimize with Caching & ORM ✅ Ensure Smooth Frontend-Backend Integration ✅ Follow Best Deployment & Security Practices
💡 Ready to level up? Drop a 🚀 in the comments!
📩 Contact us: pythonfullstackmasters.in 📧 [email protected] 📞 +91 97049 44488
0 notes