#db2 questions for interview
Explore tagged Tumblr posts
Text
Java Database Connectivity API contains commonly asked Java interview questions. A good understanding of JDBC API is required to understand and leverage many powerful features of Java technology. Here are few important practical questions and answers which can be asked in a Core Java JDBC interview. Most of the java developers are required to use JDBC API in some type of application. Though its really common, not many people understand the real depth of this powerful java API. Dozens of relational databases are seamlessly connected using java due to the simplicity of this API. To name a few Oracle, MySQL, Postgres and MS SQL are some popular ones. This article is going to cover a lot of general questions and some of the really in-depth ones to. Java Interview Preparation Tips Part 0: Things You Must Know For a Java Interview Part 1: Core Java Interview Questions Part 2: JDBC Interview Questions Part 3: Collections Framework Interview Questions Part 4: Threading Interview Questions Part 5: Serialization Interview Questions Part 6: Classpath Related Questions Part 7: Java Architect Scalability Questions What are available drivers in JDBC? JDBC technology drivers fit into one of four categories: A JDBC-ODBC bridge provides JDBC API access via one or more ODBC drivers. Note that some ODBC native code and in many cases native database client code must be loaded on each client machine that uses this type of driver. Hence, this kind of driver is generally most appropriate when automatic installation and downloading of a Java technology application is not important. A native-API partly Java technology-enabled driver converts JDBC calls into calls on the client API for Oracle, Sybase, Informix, DB2, or other DBMS. Note that, like the bridge driver, this style of driver requires that some binary code be loaded on each client machine. A net-protocol fully Java technology-enabled driver translates JDBC API calls into a DBMS-independent net protocol which is then translated to a DBMS protocol by a server. This net server middleware is able to connect all of its Java technology-based clients to many different databases. The specific protocol used depends on the vendor. In general, this is the most flexible JDBC API alternative. It is likely that all vendors of this solution will provide products suitable for Intranet use. In order for these products to also support Internet access they must handle the additional requirements for security, access through firewalls, etc., that the Web imposes. Several vendors are adding JDBC technology-based drivers to their existing database middleware products. A native-protocol fully Java technology-enabled driver converts JDBC technology calls into the network protocol used by DBMSs directly. This allows a direct call from the client machine to the DBMS server and is a practical solution for Intranet access. Since many of these protocols are proprietary the database vendors themselves will be the primary source for this style of driver. Several database vendors have these in progress. What are the types of statements in JDBC? the JDBC API has 3 Interfaces, (1. Statement, 2. PreparedStatement, 3. CallableStatement ). The key features of these are as follows: Statement This interface is used for executing a static SQL statement and returning the results it produces. The object of Statement class can be created using Connection.createStatement() method. PreparedStatement A SQL statement is pre-compiled and stored in a PreparedStatement object. This object can then be used to efficiently execute this statement multiple times. The object of PreparedStatement class can be created using Connection.prepareStatement() method. This extends Statement interface. CallableStatement This interface is used to execute SQL stored procedures. This extends PreparedStatement interface. The object of CallableStatement class can be created using Connection.prepareCall() method.
What is a stored procedure? How to call stored procedure using JDBC API? Stored procedure is a group of SQL statements that forms a logical unit and performs a particular task. Stored Procedures are used to encapsulate a set of operations or queries to execute on database. Stored procedures can be compiled and executed with different parameters and results and may have any combination of input/output parameters. Stored procedures can be called using CallableStatement class in JDBC API. Below code snippet shows how this can be achieved. CallableStatement cs = con.prepareCall("call MY_STORED_PROC_NAME"); ResultSet rs = cs.executeQuery(); What is Connection pooling? What are the advantages of using a connection pool? Connection Pooling is a technique used for sharing the server resources among requested clients. It was pioneered by database vendors to allow multiple clients to share a cached set of connection objects that provides access to a database. Getting connection and disconnecting are costly operation, which affects the application performance, so we should avoid creating multiple connection during multiple database interactions. A pool contains set of Database connections which are already connected, and any client who wants to use it can take it from pool and when done with using it can be returned back to the pool. Apart from performance this also saves you resources as there may be limited database connections available for your application. How to do database connection using JDBC thin driver ? This is one of the most commonly asked questions from JDBC fundamentals, and knowing all the steps of JDBC connection is important. import java.sql.*; class JDBCTest public static void main (String args []) throws Exception //Load driver class Class.forName ("oracle.jdbc.driver.OracleDriver"); //Create connection Connection conn = DriverManager.getConnection ("jdbc:oracle:thin:@hostname:1526:testdb", "scott", "tiger"); // @machineName:port:SID, userid, password Statement stmt = conn.createStatement(); ResultSet rs = stmt.executeQuery("select 'Hi' from dual"); while (rs.next()) System.out.println (rs.getString(1)); // Print col 1 => Hi stmt.close(); What does Class.forName() method do? Method forName() is a static method of java.lang.Class. This can be used to dynamically load a class at run-time. Class.forName() loads the class if its not already loaded. It also executes the static block of loaded class. Then this method returns an instance of the loaded class. So a call to Class.forName('MyClass') is going to do following - Load the class MyClass. - Execute any static block code of MyClass. - Return an instance of MyClass. JDBC Driver loading using Class.forName is a good example of best use of this method. The driver loading is done like this Class.forName("org.mysql.Driver"); All JDBC Drivers have a static block that registers itself with DriverManager and DriverManager has static initializer method registerDriver() which can be called in a static blocks of Driver class. A MySQL JDBC Driver has a static initializer which looks like this: static try java.sql.DriverManager.registerDriver(new Driver()); catch (SQLException E) throw new RuntimeException("Can't register driver!"); Class.forName() loads driver class and executes the static block and the Driver registers itself with the DriverManager. Which one will you use Statement or PreparedStatement? Or Which one to use when (Statement/PreparedStatement)? Compare PreparedStatement vs Statement. By Java API definitions: Statement is a object used for executing a static SQL statement and returning the results it produces. PreparedStatement is a SQL statement which is precompiled and stored in a PreparedStatement object. This object can then be used to efficiently execute this statement multiple times. There are few advantages of using PreparedStatements over Statements
Since its pre-compiled, Executing the same query multiple times in loop, binding different parameter values each time is faster. (What does pre-compiled statement means? The prepared statement(pre-compiled) concept is not specific to Java, it is a database concept. Statement precompiling means: when you execute a SQL query, database server will prepare a execution plan before executing the actual query, this execution plan will be cached at database server for further execution.) In PreparedStatement the setDate()/setString() methods can be used to escape dates and strings properly, in a database-independent way. SQL injection attacks on a system are virtually impossible when using PreparedStatements. What does setAutoCommit(false) do? A JDBC connection is created in auto-commit mode by default. This means that each individual SQL statement is treated as a transaction and will be automatically committed as soon as it is executed. If you require two or more statements to be grouped into a transaction then you need to disable auto-commit mode using below command con.setAutoCommit(false); Once auto-commit mode is disabled, no SQL statements will be committed until you explicitly call the commit method. A Simple transaction with use of autocommit flag is demonstrated below. con.setAutoCommit(false); PreparedStatement updateStmt = con.prepareStatement( "UPDATE EMPLOYEE SET SALARY = ? WHERE EMP_NAME LIKE ?"); updateStmt.setInt(1, 5000); updateSales.setString(2, "Jack"); updateStmt.executeUpdate(); updateStmt.setInt(1, 6000); updateSales.setString(2, "Tom"); updateStmt.executeUpdate(); con.commit(); con.setAutoCommit(true); What are database warnings and How can I handle database warnings in JDBC? Warnings are issued by database to notify user of a problem which may not be very severe. Database warnings do not stop the execution of SQL statements. In JDBC SQLWarning is an exception that provides information on database access warnings. Warnings are silently chained to the object whose method caused it to be reported. Warnings may be retrieved from Connection, Statement, and ResultSet objects. Handling SQLWarning from connection object //Retrieving warning from connection object SQLWarning warning = conn.getWarnings(); //Retrieving next warning from warning object itself SQLWarning nextWarning = warning.getNextWarning(); //Clear all warnings reported for this Connection object. conn.clearWarnings(); Handling SQLWarning from Statement object //Retrieving warning from statement object stmt.getWarnings(); //Retrieving next warning from warning object itself SQLWarning nextWarning = warning.getNextWarning(); //Clear all warnings reported for this Statement object. stmt.clearWarnings(); Handling SQLWarning from ResultSet object //Retrieving warning from resultset object rs.getWarnings(); //Retrieving next warning from warning object itself SQLWarning nextWarning = warning.getNextWarning(); //Clear all warnings reported for this resultset object. rs.clearWarnings(); The call to getWarnings() method in any of above way retrieves the first warning reported by calls on this object. If there is more than one warning, subsequent warnings will be chained to the first one and can be retrieved by calling the method SQLWarning.getNextWarning on the warning that was retrieved previously. A call to clearWarnings() method clears all warnings reported for this object. After a call to this method, the method getWarnings returns null until a new warning is reported for this object. Trying to call getWarning() on a connection after it has been closed will cause an SQLException to be thrown. Similarly, trying to retrieve a warning on a statement after it has been closed or on a result set after it has been closed will cause an SQLException to be thrown. Note that closing a statement also closes a result set that it might have produced. What is Metadata and why should I use it?
JDBC API has 2 Metadata interfaces DatabaseMetaData & ResultSetMetaData. The DatabaseMetaData provides Comprehensive information about the database as a whole. This interface is implemented by driver vendors to let users know the capabilities of a Database Management System (DBMS) in combination with the driver based on JDBC technology ("JDBC driver") that is used with it. Below is a sample code which demonstrates how we can use the DatabaseMetaData DatabaseMetaData md = conn.getMetaData(); System.out.println("Database Name: " + md.getDatabaseProductName()); System.out.println("Database Version: " + md.getDatabaseProductVersion()); System.out.println("Driver Name: " + md.getDriverName()); System.out.println("Driver Version: " + md.getDriverVersion()); The ResultSetMetaData is an object that can be used to get information about the types and properties of the columns in a ResultSet object. Use DatabaseMetaData to find information about your database, such as its capabilities and structure. Use ResultSetMetaData to find information about the results of an SQL query, such as size and types of columns. Below a sample code which demonstrates how we can use the ResultSetMetaData ResultSet rs = stmt.executeQuery("SELECT a, b, c FROM TABLE2"); ResultSetMetaData rsmd = rs.getMetaData(); int numberOfColumns = rsmd.getColumnCount(); boolean b = rsmd.isSearchable(1); What is RowSet? or What is the difference between RowSet and ResultSet? or Why do we need RowSet? or What are the advantages of using RowSet over ResultSet? RowSet is a interface that adds support to the JDBC API for the JavaBeans component model. A rowset, which can be used as a JavaBeans component in a visual Bean development environment, can be created and configured at design time and executed at run time. The RowSet interface provides a set of JavaBeans properties that allow a RowSet instance to be configured to connect to a JDBC data source and read some data from the data source. A group of setter methods (setInt, setBytes, setString, and so on) provide a way to pass input parameters to a rowset's command property. This command is the SQL query the rowset uses when it gets its data from a relational database, which is generally the case. Rowsets are easy to use since the RowSet interface extends the standard java.sql.ResultSet interface so it has all the methods of ResultSet. There are two clear advantages of using RowSet over ResultSet RowSet makes it possible to use the ResultSet object as a JavaBeans component. As a consequence, a result set can, for example, be a component in a Swing application. RowSet be used to make a ResultSet object scrollable and updatable. All RowSet objects are by default scrollable and updatable. If the driver and database being used do not support scrolling and/or updating of result sets, an application can populate a RowSet object implementation (e.g. JdbcRowSet) with the data of a ResultSet object and then operate on the RowSet object as if it were the ResultSet object. What is a connected RowSet? or What is the difference between connected RowSet and disconnected RowSet? or Connected vs Disconnected RowSet, which one should I use and when? Connected RowSet A RowSet object may make a connection with a data source and maintain that connection throughout its life cycle, in which case it is called a connected rowset. A rowset may also make a connection with a data source, get data from it, and then close the connection. Such a rowset is called a disconnected rowset. A disconnected rowset may make changes to its data while it is disconnected and then send the changes back to the original source of the data, but it must reestablish a connection to do so. Example of Connected RowSet: A JdbcRowSet object is a example of connected RowSet, which means it continually maintains its connection to a database using a JDBC technology-enabled driver. Disconnected RowSet A disconnected rowset may have a reader (a RowSetReader object) and a writer (a RowSetWriter object) associated with it.
The reader may be implemented in many different ways to populate a rowset with data, including getting data from a non-relational data source. The writer can also be implemented in many different ways to propagate changes made to the rowset's data back to the underlying data source. Example of Disconnected RowSet: A CachedRowSet object is a example of disconnected rowset, which means that it makes use of a connection to its data source only briefly. It connects to its data source while it is reading data to populate itself with rows and again while it is propagating changes back to its underlying data source. The rest of the time, a CachedRowSet object is disconnected, including while its data is being modified. Being disconnected makes a RowSet object much leaner and therefore much easier to pass to another component. For example, a disconnected RowSet object can be serialized and passed over the wire to a thin client such as a personal digital assistant (PDA). What is the benefit of having JdbcRowSet implementation? Why do we need a JdbcRowSet like wrapper around ResultSet? The JdbcRowSet implementation is a wrapper around a ResultSet object that has following advantages over ResultSet This implementation makes it possible to use the ResultSet object as a JavaBeans component. A JdbcRowSet can be used as a JavaBeans component in a visual Bean development environment, can be created and configured at design time and executed at run time. It can be used to make a ResultSet object scrollable and updatable. All RowSet objects are by default scrollable and updatable. If the driver and database being used do not support scrolling and/or updating of result sets, an application can populate a JdbcRowSet object with the data of a ResultSet object and then operate on the JdbcRowSet object as if it were the ResultSet object. Can you think of a questions which is not part of this post? Please don't forget to share it with me in comments section & I will try to include it in the list.
0 notes
Text
Question 69: How can I create a pipeline to copy data from an on-premises DB2 database to Azure Table Storage using Azure Data Factory?
Interview Questions on Azure Data Factory Development: #interview, #interviewquestions, #Microsoft, #azure, #adf , #eswarstechworld, #azuredataengineer, #development, #azuredatafactory , #azuredeveloper
Azure Data Factory is a cloud-based data integration service that allows you to create and manage data-driven workflows. In this scenario, the pipeline is designed to extract data from an on-premises DB2 database and load it into Azure Table Storage. Categories: The pipeline to copy data from an on-premises DB2 database to Azure Table Storage falls under the data integration and data movement…

View On WordPress
#adf#azure#azuredataengineer#azuredatafactory#azuredeveloper#development#eswarstechworld#interview#interviewquestions#Microsoft
0 notes
Text
db2 questions for interview
complete notes on db2, complete tutorials on db2, db2 notes, db2 questions asked in companies, db2 questions asked in interview, db2 questions asked in mnc, db2 questions for interview, db2 tutorials, faq for db2, faq on db2, interview questions on db2, latest interview questions on db2, most asked db2 interview questions, notes on db2, rapid fire on db2, rapid fire questions on db2, top interview questions on db2, tutorials on db2, updated interview questions answers on db2, db2 interview questions
0 notes
Text
Sql Interview Questions
If a WHERE clause is used in cross join after that the inquiry will certainly function like an INTERNAL SIGN UP WITH. A DISTINCT restraint ensures that all values in a column are various. This supplies uniqueness for the column and also assists identify each row distinctively. It promotes you to manipulate the data stored in the tables by using relational drivers. Instances of the relational data source administration system are Microsoft Gain access to, MySQL, SQLServer, Oracle database, etc. One-of-a-kind crucial restriction uniquely identifies each document in the data source. https://geekinterview.net This vital provides uniqueness for the column or set of columns. A database arrow is a control framework that permits traversal of documents in a data source. Cursors, on top of that, promotes handling after traversal, such as access, addition as well as deletion of database documents. They can be considered as a reminder to one row in a set of rows. An alias is a feature of SQL that is sustained by a lot of, otherwise all, RDBMSs. It is a temporary name designated to the table or table column for the purpose of a specific SQL inquiry. Furthermore, aliasing can be employed as an obfuscation technique to protect the actual names of database fields. A table pen name is also called a relationship name. students; Non-unique indexes, on the other hand, are not utilized to enforce restrictions on the tables with which they are linked. Rather, non-unique indexes are made use of solely to improve query performance by keeping a sorted order of data worths that are used regularly. A database index is a data structure that offers quick lookup of data in a column or columns of a table. It boosts the speed of operations accessing data from a data source table at the cost of additional creates as well as memory to keep the index information framework. Prospects are most likely to be asked standard SQL interview concerns to progress degree SQL concerns relying on their experience and different other aspects. The listed below list covers all the SQL meeting concerns for betters in addition to SQL interview questions for knowledgeable degree candidates as well as some SQL question meeting concerns. SQL provision helps to restrict the outcome set by offering a problem to the query. A clause assists to filter the rows from the entire set of records. Our SQL Meeting Questions blog site is the one-stop source where you can improve your meeting prep work. It has a set of leading 65 concerns which an interviewer intends to ask throughout an interview procedure. Unlike primary vital, there can be numerous distinct restrictions specified per table. The code syntax for UNIQUE is rather comparable to that of PRIMARY SECRET and can be utilized mutually. A lot of modern data source management systems like MySQL, Microsoft SQL Server, Oracle, IBM DB2 as well as Amazon Redshift are based upon RDBMS. SQL clause is specified to restrict the result set by providing problem to the query. This typically filterings system some rows from the whole collection of records. Cross join can be defined as a cartesian product of both tables included in the join. The table after sign up with contains the same number of rows as in the cross-product of number of rows in the two tables. Self-join is set to be query made use of to contrast to itself. This is utilized to contrast values in a column with other worths in the exact same column in the same table. PEN NAME ES can be utilized for the same table contrast. This is a key words used to inquire information from even more tables based upon the partnership between the areas of the tables. A international trick is one table which can be associated with the main key of another table. Partnership requires to be produced in between 2 tables by referencing international key with the main key of another table. A Distinct essential restraint uniquely recognized each record in the data source. It begins with the fundamental SQL interview questions and later on remains to sophisticated inquiries based on your conversations and also solutions. These SQL Interview concerns will aid you with various knowledge levels to reap the optimum take advantage of this blog. A table has a specified number of the column called fields however can have any kind of variety of rows which is called the document. So, the columns in the table of the database are called the fields and they represent the feature or attributes of the entity in the document. Rows below describes the tuples which stand for the easy data item and also columns are the quality of the information products existing particularly row. Columns can classify as vertical, as well as Rows are straight. There is provided sql meeting questions and also responses that has actually been asked in lots of business. For PL/SQL interview questions, visit our following web page. A view can have information from several tables integrated, as well as it depends on the connection. Views are used to apply security system in the SQL Server. The sight of the database is the searchable object we can use a inquiry to browse the view as we use for the table. RDBMS means Relational Database Monitoring System. It is a data source administration system based upon a relational version. RDBMS stores the data right into the collection of tables and also links those table using the relational drivers easily whenever called for. This provides uniqueness for the column or set of columns. A table is a collection of information that are organized in a version with Columns and Rows. Columns can be categorized as vertical, as well as Rows are straight. A table has specified number of column called areas but can have any kind of number of rows which is called record. RDBMS save the data into the collection of tables, which is connected by common areas between the columns of the table. It also provides relational operators to manipulate the information stored into the tables. Adhering to is a curated listing of SQL interview concerns as well as answers, which are most likely to be asked during the SQL meeting.

1 note
·
View note
Text
Business Analyst Finance Domain Sample Resume
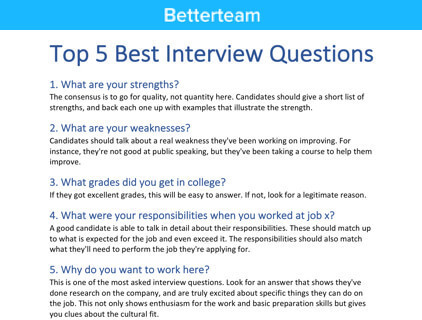
This is just a sample Business Analyst resume for freshers as well as for experienced job seekers in Finance domain of business analyst or system analyst. While this is only a sample resume, please use this only for reference purpose, do not copy the same client names or job duties for your own purpose. Always make your own resume with genuine experience.
Name: Justin Megha
Ph no: XXXXXXX
your email here.
Business Analyst, Business Systems Analyst
SUMMARY
Accomplished in Business Analysis, System Analysis, Quality Analysis and Project Management with extensive experience in business products, operations and Information Technology on the capital markets space specializing in Finance such as Trading, Fixed Income, Equities, Bonds, Derivatives(Swaps, Options, etc) and Mortgage with sound knowledge of broad range of financial instruments. Over 11+ Years of proven track record as value-adding, delivery-loaded project hardened professional with hands-on expertise spanning in System Analysis, Architecting Financial applications, Data warehousing, Data Migrations, Data Processing, ERP applications, SOX Implementation and Process Compliance Projects. Accomplishments in analysis of large-scale business systems, Project Charters, Business Requirement Documents, Business Overview Documents, Authoring Narrative Use Cases, Functional Specifications, and Technical Specifications, data warehousing, reporting and testing plans. Expertise in creating UML based Modelling views like Activity/ Use Case/Data Flow/Business Flow /Navigational Flow/Wire Frame diagrams using Rational Products & MS Visio. Proficient as long time liaison between business and technology with competence in Full Life Cycle of System (SLC) development with Waterfall, Agile, RUP methodology, IT Auditing and SOX Concepts as well as broad cross-functional experiences leveraging multiple frameworks. Extensively worked with the On-site and Off-shore Quality Assurance Groups by assisting the QA team to perform Black Box /GUI testing/ Functionality /Regression /System /Unit/Stress /Performance/ UAT's. Facilitated change management across entire process from project conceptualization to testing through project delivery, Software Development & Implementation Management in diverse business & technical environments, with demonstrated leadership abilities. EDUCATION
Post Graduate Diploma (in Business Administration), USA Master's Degree (in Computer Applications), Bachelor's Degree (in Commerce), TECHNICAL SKILLS
Documentation Tools UML, MS Office (Word, Excel, Power Point, Project), MS Visio, Erwin
SDLC Methodologies Waterfall, Iterative, Rational Unified Process (RUP), Spiral, Agile
Modeling Tools UML, MS Visio, Erwin, Power Designer, Metastrom Provision
Reporting Tools Business Objects X IR2, Crystal Reports, MS Office Suite
QA Tools Quality Center, Test Director, Win Runner, Load Runner, QTP, Rational Requisite Pro, Bugzilla, Clear Quest
Languages Java, VB, SQL, HTML, XML, UML, ASP, JSP
Databases & OS MS SQL Server, Oracle 10g, DB2, MS Access on Windows XP / 2000, Unix
Version Control Rational Clear Case, Visual Source Safe
PROFESSIONAL EXPERIENCE
SERVICE MASTER, Memphis, TN June 08 - Till Date
Senior Business Analyst
Terminix has approximately 800 customer service agents that reside in our branches in addition to approximately 150 agents in a centralized call center in Memphis, TN. Terminix customer service agents receive approximately 25 million calls from customers each year. Many of these customer's questions are not answered or their problems are not resolved on the first call. Currently these agents use an AS/400 based custom developed system called Mission to answer customer inquiries into branches and the Customer Communication Center. Mission - Terminix's operation system - provides functionality for sales, field service (routing & scheduling, work order management), accounts receivable, and payroll. This system is designed modularly and is difficult to navigate for customer service agents needing to assist the customer quickly and knowledgeably. The amount of effort and time needed to train a customer service representative using the Mission system is high. This combined with low agent and customer retention is costly.
Customer Service Console enables Customer Service Associates to provide consistent, enhanced service experience, support to the Customers across the Organization. CSC is aimed at providing easy navigation, easy learning process, reduced call time and first call resolution.
Responsibilities
Assisted in creating Project Plan, Road Map. Designed Requirements Planning and Management document. Performed Enterprise Analysis and actively participated in buying Tool Licenses. Identified subject-matter experts and drove the requirements gathering process through approval of the documents that convey their needs to management, developers, and quality assurance team. Performed technical project consultation, initiation, collection and documentation of client business and functional requirements, solution alternatives, functional design, testing and implementation support. Requirements Elicitation, Analysis, Communication, and Validation according to Six Sigma Standards. Captured Business Process Flows and Reengineered Process to achieve maximum outputs. Captured As-Is Process, designed TO-BE Process and performed Gap Analysis Developed and updated functional use cases and conducted business process modeling (PROVISION) to explain business requirements to development and QA teams. Created Business Requirements Documents, Functional and Software Requirements Specification Documents. Performed Requirements Elicitation through Use Cases, one to one meetings, Affinity Exercises, SIPOC's. Gathered and documented Use Cases, Business Rules, created and maintained Requirements/Test Traceability Matrices. Client: The Dun & Bradstreet Corporation, Parsippany, NJ May' 2007 - Oct' 2007
Profile: Sr. Financial Business Analyst/ Systems Analyst.
Project Profile (1): D&B is the world's leading source of commercial information and insight on businesses. The Point of Arrival Project and the Data Maintenance (DM) Project are the future applications of the company that the company would transit into, providing an effective method & efficient report generation system for D&B's clients to be able purchase reports about companies they are trying to do business.
Project Profile (2): The overall purpose of this project was building a Self Awareness System(SAS) for the business community for buying SAS products and a Payment system was built for SAS. The system would provide certain combination of products (reports) for Self Monitoring report as a foundation for managing a company's credit.
Responsibilities:
Conducted GAP Analysis and documented the current state and future state, after understanding the Vision from the Business Group and the Technology Group. Conducted interviews with Process Owners, Administrators and Functional Heads to gather audit-related information and facilitated meetings to explain the impacts and effects of SOX compliance. Played an active and lead role in gathering, analyzing and documenting the Business Requirements, the business rules and Technical Requirements from the Business Group and the Technological Group. Co - Authored and prepared Graphical depictions of Narrative Use Cases, created UML Models such as Use Case Diagrams, Activity Diagrams and Flow Diagrams using MS Visio throughout the Agile methodology Documented the Business Requirement Document to get a better understanding of client's business processes of both the projects using the Agile methodology. Facilitating JRP and JAD sessions, brain storming sessions with the Business Group and the Technology Group. Documented the Requirement traceability matrix (RTM) and conducted UML Modelling such as creating Activity Diagrams, Flow Diagrams using MS Visio. Analysed test data to detect significant findings and recommended corrective measures Co-Managed the Change Control process for the entire project as a whole by facilitating group meetings, one-on-one interview sessions and email correspondence with work stream owners to discuss the impact of Change Request on the project. Worked with the Project Lead in setting realistic project expectations and in evaluating the impact of changes on the organization and plans accordingly and conducted project related presentations. Co-oordinated with the off shore QA Team members to explain and develop the Test Plans, Test cases, Test and Evaluation strategy and methods for unit testing, functional testing and usability testing Environment: Windows XP/2000, SOX, Sharepoint, SQL, MS Visio, Oracle, MS Office Suite, Mercury ITG, Mercury Quality Center, XML, XHTML, Java, J2EE.
GATEWAY COMPUTERS, Irvine, CA, Jan 06 - Mar 07
Business Analyst
At Gateway, a Leading Computer, Laptop and Accessory Manufacturer, was involved in two projects,
Order Capture Application: Objective of this Project is to Develop Various Mediums of Sales with a Centralized Catalog. This project involves wide exposure towards Requirement Analysis, Creating, Executing and Maintaining of Test plans and Test Cases. Mentored and trained staff about Tech Guide & Company Standards; Gateway reporting system: was developed with Business Objects running against Oracle data warehouse with Sales, Inventory, and HR Data Marts. This DW serves the different needs of Sales Personnel and Management. Involved in the development of it utilized Full Client reports and Web Intelligence to deliver analytics to the Contract Administration group and Pricing groups. Reporting data mart included Wholesaler Sales, Contract Sales and Rebates data.
Responsibilities:
Product Manager for Enterprise Level Order Entry Systems - Phone, B2B, Gateway.com and Cataloging System. Modeled the Sales Order Entry process to eliminate bottleneck process steps using ERWIN. Adhered and practiced RUP for implementing software development life cycle. Gathered Requirements from different sources like Stakeholders, Documentation, Corporate Goals, Existing Systems, and Subject Matter Experts by conducting Workshops, Interviews, Use Cases, Prototypes, Reading Documents, Market Analysis, Observations Created Functional Requirement Specification documents - which include UMLUse case diagrams, Scenarios, activity, work Flow diagrams and data mapping. Process and Data modeling with MS VISIO. Worked with Technical Team to create Business Services (Web Services) that Application could leverage using SOA, to create System Architecture and CDM for common order platform. Designed Payment Authorization (Credit Card, Net Terms, and Pay Pal) for the transaction/order entry systems. Implemented A/B Testing, Customer Feedback Functionality to Gateway.com Worked with the DW, ETL teams to create Order entry systems Business Objects reports. (Full Client, Web I) Worked in a cross functional team of Business, Architects and Developers to implement new features. Program Managed Enterprise Order Entry Systems - Development and Deployment Schedule. Developed and maintained User Manuals, Application Documentation Manual, on Share Point tool. Created Test Plansand Test Strategies to define the Objective and Approach of testing. Used Quality Center to track and report system defects and bug fixes. Written modification requests for the bugs in the application and helped developers to track and resolve the problems. Developed and Executed Manual, Automated Functional, GUI, Regression, UAT Test cases using QTP. Gathered, documented and executed Requirements-based, Business process (workflow/user scenario), Data driven test cases for User Acceptance Testing. Created Test Matrix, Used Quality Center for Test Management, track & report system defects and bug fixes. Performed Load, stress Testing's & Analyzed Performance, Response Times. Designed approach, developed visual scripts in order to test client & server side performance under various conditions to identify bottlenecks. Created / developed SQL Queries (TOAD) with several parameters for Backend/DB testing Conducted meetings for project status, issue identification, and parent task review, Progress Reporting. AMC MORTGAGE SERVICES, CA, USA Oct 04 - Dec 05
Business Analyst
The primary objective of this project is to replace the existing Internal Facing Client / Server Applications with a Web enabled Application System, which can be used across all the Business Channels. This project involves wide exposure towards Requirement Analysis, Creating, Executing and Maintaining of Test plans and Test Cases. Demands understanding and testing of Data Warehouse and Data Marts, thorough knowledge of ETL and Reporting, Enhancement of the Legacy System covered all of the business requirements related to Valuations from maintaining the panel of appraisers to ordering, receiving, and reviewing the valuations.
Responsibilities:
Gathered Analyzed, Validated, and Managed and documented the stated Requirements. Interacted with users for verifying requirements, managing change control process, updating existing documentation. Created Functional Requirement Specification documents - that include UML Use case diagrams, scenarios, activity diagrams and data mapping. Provided End User Consulting on Functionality and Business Process. Acted as a client liaison to review priorities and manage the overall client queue. Provided consultation services to clients, technicians and internal departments on basic to intricate functions of the applications. Identified business directions & objectives that may influence the required data and application architectures. Defined, prioritized business requirements, Determine which business subject areas provide the most needed information; prioritize and sequence implementation projects accordingly. Provide relevant test scenarios for the testing team. Work with test team to develop system integration test scripts and ensure the testing results correspond to the business expectations. Used Test Director, QTP, Load Runner for Test management, Functional, GUI, Performance, Stress Testing Perform Data Validation, Data Integration and Backend/DB testing using SQL Queries manually. Created Test input requirements and prepared the test data for data driven testing. Mentored, trained staff about Tech Guide & Company Standards. Set-up and Coordinate Onsite offshore teams, Conduct Knowledge Transfer sessions to the offshore team. Lloyds Bank, UK Aug 03 - Sept 04 Business Analyst Lloyds TSB is leader in Business, Personal and Corporate Banking. Noted financial provider for millions of customers with the financial resources to meet and manage their credit needs and to achieve their financial goals. The Project involves an applicant Information System, Loan Appraisal and Loan Sanction, Legal, Disbursements, Accounts, MIS and Report Modules of a Housing Finance System and Enhancements for their Internet Banking.
Responsibilities:
Translated stakeholder requirements into various documentation deliverables such as functional specifications, use cases, workflow / process diagrams, data flow / data model diagrams. Produced functional specifications and led weekly meetings with developers and business units to discuss outstanding technical issues and deadlines that had to be met. Coordinated project activities between clients and internal groups and information technology, including project portfolio management and project pipeline planning. Provided functional expertise to developers during the technical design and construction phases of the project. Documented and analyzed business workflows and processes. Present the studies to the client for approval Participated in Universe development - planning, designing, Building, distribution, and maintenance phases. Designed and developed Universes by defining Joins, Cardinalities between the tables. Created UML use case, activity diagrams for the interaction between report analyst and the reporting systems. Successfully implemented BPR and achieved improved Performance, Reduced Time and Cost. Developed test plans and scripts; performed client testing for routine to complex processes to ensure proper system functioning. Worked closely with UAT Testers and End Users during system validation, User Acceptance Testing to expose functionality/business logic problems that unit testing and system testing have missed out. Participated in Integration, System, Regression, Performance, and UAT - Using TD, WR, Load Runner Participated in defect review meetings with the team members. Worked closely with the project manager to record, track, prioritize and close bugs. Used CVS to maintain versions between various stages of SDLC. Client: A.G. Edwards, St. Louis, MO May' 2005 - Feb' 2006
Profile: Sr. Business Analyst/System Analyst
Project Profile: A.G. Edwards is a full service Trading based brokerage firm in Internet-based futures, options and forex brokerage. This site allows Users (Financial Representative) to trade online. The main features of this site were: Users can open new account online to trade equitiies, bonds, derivatives and forex with the Trading system using DTCC's applications as a Clearing House agent. The user will get real-time streaming quotes for the currency pairs they selected, their current position in the forex market, summary of work orders, payments and current money balances, P & L Accounts and available trading power, all continuously updating in real time via live quotes. The site also facilitates users to Place, Change and Cancel an Entry Order, Placing a Market Order, Place/Modify/Delete/Close a Stop Loss Limit on an Open Position.
Responsibilities:
Gathered Business requirements pertaining to Trading, equities and Fixed Incomes like bonds, converted the same into functional requirements by implementing the RUP methodology and authored the same in Business Requirement Document (BRD). Designed and developed all Narrative Use Cases and conducted UML modeling like created Use Case Diagrams, Process Flow Diagrams and Activity Diagrams using MS Visio. Implemented the entire Rational Unified Process (RUP) methodology of application development with its various workflows, artifacts and activities. Developed business process models in RUP to document existing and future business processes. Established a business Analysis methodology around the Rational Unified Process. Analyzed user requirements, attended Change Request meetings to document changes and implemented procedures to test changes. Assisted in developing project timelines/deliverables/strategies for effective project management. Evaluated existing practices of storing and handling important financial data for compliance. Involved in developing the test strategy and assisted in developed Test scenarios, test conditions and test cases Partnered with the technical Business Analyst Interview questions areas in the research, resolution of system and User Acceptance Testing (UAT).
1 note
·
View note
Text
Sql Interview Questions
If a WHERE provision is used in cross join after that the inquiry will certainly work like an INNER SIGN UP WITH. A UNIQUE constraint makes sure that all values in a column are various. This provides uniqueness for the column and aids recognize each row distinctively. It promotes you to control the information kept in the tables by utilizing relational operators. Examples of the relational database management system are Microsoft Gain access to, MySQL, SQLServer, Oracle database, etc. One-of-a-kind crucial constraint distinctly identifies each record in the data source. This crucial offers uniqueness for the column or collection of columns. A database cursor is a control structure that enables traversal of records in a database. Cursors, furthermore, facilitates handling after traversal, such as retrieval, enhancement and also removal of database records. They can be viewed as a guideline to one row in a collection of rows. An pen names is a attribute of SQL that is supported by most, if not all, RDBMSs. It is a temporary name assigned to the table or table column for the function of a particular SQL inquiry. In addition, aliasing can be employed as an obfuscation method to protect the real names of database areas. A table pen name is also called a relationship name. trainees; Non-unique indexes, on the other hand, are not made use of to impose restrictions on the tables with which they are associated. Instead, non-unique indexes are utilized exclusively to boost query performance by preserving a sorted order of information values that are used often. A database index is a information structure that provides quick lookup of information in a column or columns of a table. It boosts the speed of operations accessing data from a data source table at the expense of additional creates and memory to preserve the index data framework. Candidates are most likely to be asked standard SQL meeting questions to advance degree SQL questions depending upon their experience as well as numerous other aspects. The listed below list covers all the SQL meeting questions for betters along with SQL meeting concerns for seasoned level candidates and also some SQL inquiry meeting concerns. SQL stipulation helps to limit the result established by offering a condition to the question. A stipulation aids to filter the rows from the whole set of records. Our SQL Interview Questions blog site is the one-stop source from where you can boost your meeting prep work. It has a collection of leading 65 concerns which an recruiter intends to ask throughout an interview process. Unlike https://geekinterview.net , there can be numerous unique restraints defined per table. The code syntax for UNIQUE is rather similar to that of PRIMARY SECRET and can be made use of mutually. The majority of modern data source monitoring systems like MySQL, Microsoft SQL Web Server, Oracle, IBM DB2 and Amazon.com Redshift are based upon RDBMS. SQL provision is defined to limit the outcome established by giving condition to the inquiry. This usually filterings system some rows from the entire set of records. Cross join can be specified as a cartesian product of the two tables included in the join. The table after join contains the same variety of rows as in the cross-product of number of rows in both tables. Self-join is readied to be query made use of to contrast to itself. This is made use of to compare worths in a column with other values in the exact same column in the exact same table. ALIAS ES can be made use of for the very same table comparison. This is a search phrase made use of to quiz information from even more tables based upon the connection between the areas of the tables. A foreign trick is one table which can be connected to the primary trick of one more table. Relationship requires to be produced in between 2 tables by referencing international key with the main secret of one more table. A Unique vital restraint distinctly recognized each record in the data source. It starts with the basic SQL meeting questions as well as later continues to sophisticated concerns based on your conversations and also responses. These SQL Meeting inquiries will assist you with different knowledge levels to gain the optimum gain from this blog site. A table has a defined number of the column called areas however can have any type of variety of rows which is referred to as the document. So, the columns in the table of the data source are known as the fields and also they stand for the quality or characteristics of the entity in the document. Rows right here refers to the tuples which stand for the simple information item as well as columns are the quality of the information items existing in particular row. Columns can classify as vertical, and also Rows are horizontal. There is provided sql meeting questions and responses that has actually been asked in lots of firms. For PL/SQL meeting questions, see our next page.
A sight can have information from several tables integrated, and it relies on the connection. Views are used to use security device in the SQL Server. The sight of the database is the searchable item we can make use of a inquiry to look the deem we utilize for the table. RDBMS stands for Relational Data source Management System. It is a data source administration system based on a relational model. RDBMS stores the information right into the collection of tables as well as links those table using the relational operators conveniently whenever needed. This offers uniqueness for the column or collection of columns. go to my blog is a collection of data that are organized in a design with Columns as well as Rows. https://bit.ly/3tmWIsh can be categorized as vertical, as well as Rows are straight. A table has specified number of column called areas however can have any kind of variety of rows which is called document. RDBMS save the data into the collection of tables, which is connected by typical areas between the columns of the table. https://tinyurl.com/c7k3vf9t provides relational drivers to control the information saved into the tables. Complying with is a curated checklist of SQL meeting inquiries and also responses, which are likely to be asked throughout the SQL interview.
0 notes
Text
Sql Interview Questions
If a WHERE condition is made use of in cross sign up with after that the query will work like an INNER SIGN UP WITH. A UNIQUE restriction ensures that all worths in a column are different. This gives uniqueness for the column as well as helps determine each row distinctively. It promotes you to manipulate the information saved in the tables by using relational drivers. Examples of the relational data source monitoring system are Microsoft Access, MySQL, SQLServer, Oracle data source, and so on. Distinct vital constraint uniquely identifies each record in the data source. This crucial supplies uniqueness for the column or collection of columns. A database cursor is a control structure that allows for traversal of documents in a database. Cursors, additionally, assists in handling after traversal, such as retrieval, enhancement and deletion of database records. They can be viewed as a reminder to one row in a collection of rows. An pen names is a attribute of SQL that is supported by many, if not all, RDBMSs. It is a temporary name designated to the table or table column for the objective of a specific SQL inquiry. In look at this site , aliasing can be used as an obfuscation method to safeguard the actual names of database areas. A table alias is likewise called a connection name. pupils; Non-unique indexes, on the other hand, are not made use of to implement constraints on the tables with which they are linked. Rather, non-unique indexes are made use of solely to enhance inquiry performance by maintaining a arranged order of information worths that are used frequently. A database index is a data framework that supplies fast lookup of information in a column or columns of a table. It boosts the rate of procedures accessing information from a database table at the price of extra creates as well as memory to keep the index data structure. Candidates are most likely to be asked basic SQL interview concerns to progress level SQL concerns depending upon their experience and numerous other variables. The listed below listing covers all the SQL meeting questions for betters as well as SQL interview concerns for experienced degree candidates and some SQL query meeting concerns. SQL condition helps to restrict the outcome established by providing a problem to the query. A stipulation helps to filter the rows from the entire set of documents. Our SQL Meeting Questions blog is the one-stop source where you can increase your interview preparation. It has a collection of leading 65 concerns which an recruiter prepares to ask during an interview procedure. Unlike main vital, there can be several distinct constraints defined per table. The code syntax for UNIQUE is quite similar to that of PRIMARY TRICK and can be made use of interchangeably. A lot of modern data source administration systems like MySQL, Microsoft SQL Server, Oracle, IBM DB2 and also Amazon.com Redshift are based on RDBMS. SQL provision is specified to restrict the result established by providing problem to the query. This normally filters some rows from the whole collection of records. Cross join can be specified as a cartesian item of the two tables consisted of in the sign up with. The table after sign up with includes the exact same number of rows as in the cross-product of number of rows in both tables. Self-join is readied to be query utilized to compare to itself. https://tinyurl.com/c7k3vf9t is made use of to compare values in a column with other worths in the exact same column in the exact same table. PEN NAME ES can be made use of for the same table comparison. This is a key words made use of to quiz information from more tables based on the partnership between the areas of the tables. A international trick is one table which can be connected to the primary trick of another table. Relationship requires to be produced in between 2 tables by referencing foreign secret with the main secret of another table. A Unique crucial restriction distinctively determined each document in the database. It starts with the standard SQL interview concerns and later on remains to innovative concerns based on your conversations and solutions. These SQL Interview inquiries will certainly help you with various competence levels to reap the optimum take advantage of this blog site. A table consists of a specified number of the column called areas yet can have any kind of variety of rows which is referred to as the record. So, the columns in the table of the database are known as the areas and they stand for the characteristic or features of the entity in the record. Rows here describes the tuples which stand for the easy data thing and columns are the characteristic of the data products present particularly row. Columns can classify as upright, and Rows are straight. There is given sql interview concerns and also answers that has been asked in several firms. For PL/SQL interview concerns, see our following page. A sight can have data from one or more tables incorporated, and it relies on the relationship. https://geekinterview.net are used to use safety mechanism in the SQL Web server. The sight of the database is the searchable object we can utilize a question to browse the deem we make use of for the table. RDBMS represents Relational Database Administration System. It is a data source management system based upon a relational model. RDBMS shops the data into the collection of tables and also links those table utilizing the relational drivers conveniently whenever needed. This supplies individuality for the column or set of columns. A table is a set of information that are arranged in a version with Columns and Rows. Columns can be classified as vertical, as well as Rows are horizontal. A table has actually defined number of column called fields but can have any kind of number of rows which is called document. RDBMS keep the data into the collection of tables, which is connected by typical areas in between the columns of the table. It likewise provides relational operators to control the data kept right into the tables. Following is a curated checklist of SQL meeting inquiries and responses, which are most likely to be asked throughout the SQL interview.
0 notes
Text
Sql Meeting Questions
If a IN WHICH stipulation is used in cross join after that the inquiry will certainly work like an INNER JOIN. A DISTINCT restriction ensures that all values in a column are different. This gives originality for the column and helps identify each row uniquely. It facilitates you to adjust the data stored in the tables by using relational drivers. Instances of the relational data source administration system are Microsoft Gain access to, MySQL, SQLServer, Oracle database, and so on. Unique key restriction distinctly identifies each document in the data source. This vital supplies individuality for the column or set of columns. A data source arrow is a control framework that enables traversal of documents in a data source. Cursors, furthermore, helps with handling after traversal, such as access, enhancement and also deletion of data source documents. They can be deemed a pointer to one row in a set of rows. An alias is a function of SQL that is sustained by many, if not all, RDBMSs. It is a short-lived name assigned to the table or table column for the objective of a particular SQL question. In addition, aliasing can be used as an obfuscation technique to protect the genuine names of database fields. A table pen name is additionally called a connection name. trainees; Non-unique indexes, on the other hand, are not used to apply restraints on the tables with which they are connected. Rather, non-unique indexes are used solely to improve inquiry efficiency by keeping a arranged order of information worths that are made use of frequently. A database index is a data framework that offers fast lookup of data in a column or columns of a table. It boosts the speed of operations accessing data from a database table at the price of added writes as well as memory to maintain the index information structure. Candidates are most likely to be asked fundamental SQL interview concerns to advance level SQL inquiries depending upon their experience and also numerous other aspects. The listed below list covers all the SQL meeting questions for betters as well as SQL interview inquiries for knowledgeable degree candidates and also some SQL query meeting inquiries. SQL clause helps to restrict the outcome set by offering a problem to the inquiry. A clause helps to filter the rows from the entire set of documents. Our SQL Meeting Questions blog site is the one-stop source from where you can boost your interview preparation. It has a collection of leading 65 questions which an recruiter intends to ask during an meeting procedure. Unlike primary essential, there can be several one-of-a-kind restraints defined per table. The code syntax for UNIQUE is quite similar to that of PRIMARY TRICK and also can be made use of mutually. The majority of modern database management systems like MySQL, Microsoft SQL Server, Oracle, IBM DB2 as well as Amazon Redshift are based upon RDBMS. SQL provision is defined to limit the result established by offering condition to the inquiry. https://tinyurl.com/c7k3vf9t from the whole set of documents. Cross join can be defined as a cartesian item of the two tables included in the join. The table after join contains the exact same variety of rows as in the cross-product of variety of rows in the two tables. Self-join is set to be query utilized to contrast to itself. This is used to contrast worths in a column with other values in the very same column in the very same table. PEN NAME ES can be made use of for the exact same table comparison. This is a key phrase utilized to quiz data from more tables based upon the relationship in between the areas of the tables. A international secret is one table which can be associated with the main secret of one more table. Relationship requires to be produced in between two tables by referencing international trick with the main secret of an additional table. A Distinct vital restraint distinctively identified each record in the data source. It starts with the fundamental SQL interview inquiries and also later on continues to sophisticated concerns based upon your conversations and also answers. These SQL Meeting questions will certainly assist you with different competence levels to enjoy the maximum gain from this blog site. A table has a specified number of the column called areas however can have any kind of variety of rows which is referred to as the document. So, webpage in the table of the data source are known as the fields and also they represent the attribute or qualities of the entity in the document. Rows below refers to the tuples which represent the simple information product as well as columns are the characteristic of the information products present in particular row. Columns can categorize as upright, as well as Rows are horizontal. There is given sql interview inquiries as well as solutions that has actually been asked in many companies. For PL/SQL interview questions, see our following page. A sight can have information from several tables incorporated, and it depends on the partnership. Views are made use of to apply safety and security system in the SQL Server. The sight of the database is the searchable things we can use a inquiry to search the consider as we make use of for the table. RDBMS represents Relational Database Administration System. It is a database management system based upon a relational model. RDBMS stores the data right into the collection of tables as well as links those table utilizing the relational drivers conveniently whenever called for.
This provides originality for the column or collection of columns. facebook sql interview questions is a set of data that are organized in a model with Columns and Rows. https://geekinterview.net can be classified as upright, as well as Rows are straight. A table has specified variety of column called fields however can have any number of rows which is called document. RDBMS store the information right into the collection of tables, which is associated by common areas between the columns of the table. It also gives relational operators to control the data stored into the tables. Complying with is a curated list of SQL interview inquiries and solutions, which are most likely to be asked during the SQL meeting.
0 notes
Text
Question 68: How can I create a pipeline to copy data from an on-premises DB2 database to Azure Data Lake Storage using Azure Data Factory?
Interview Questions on Azure Data Factory Development: #interview, #interviewquestions, #Microsoft, #azure, #adf , #eswarstechworld, #azuredataengineer, #development, #azuredatafactory , #azuredeveloper
Azure Data Factory is a cloud-based data integration service that enables you to create and manage data-driven workflows. In this scenario, the pipeline is designed to extract data from an on-premises DB2 database and load it into Azure Data Lake Storage. Categories: The pipeline to copy data from an on-premises DB2 database to Azure Data Lake Storage falls under the data integration and data…

View On WordPress
#adf#azure#azuredataengineer#azuredatafactory#azuredeveloper#development#eswarstechworld#interview#interviewquestions#Microsoft
0 notes
Text
db2 questions asked in interview
complete notes on db2, complete tutorials on db2, db2 notes, db2 questions asked in companies, db2 questions asked in interview, db2 questions asked in mnc, db2 questions for interview, db2 tutorials, faq for db2, faq on db2, interview questions on db2, latest interview questions on db2, most asked db2 interview questions, notes on db2, rapid fire on db2, rapid fire questions on db2, top interview questions on db2, tutorials on db2, updated interview questions answers on db2, db2 interview questions
0 notes
Text
Top 10 Data Analytics Tools
The developing interest and significance of information examination in the market have produced numerous openings around the world. It turns out to be marginally difficult to waitlist the top information investigation apparatuses as the Data structure and algorithm are more well known, easy to understand and execution situated than the paid variant. There are many open source apparatuses which doesn't need a lot/any coding and figures out how to convey preferable outcomes over paid renditions for example - R programming in information mining and Tableau public, Python in information representation. The following is the rundown of top 10 of information investigation apparatuses, both open source and paid rendition, in view of their fame, learning and execution.
1. R Programming
R is the main investigation apparatus in the business and generally utilized for measurements and information demonstrating. It can without much of a stretch control your information and present in an unexpected way. It has surpassed SAS from multiple points of view like limit of information, execution and result. R incorporates and runs on a wide assortment of stages viz - UNIX, Windows and MacOS. It has 11,556 bundles and permits you to peruse the bundles by classifications. R likewise gives apparatuses to consequently introduce all bundles according to client necessity, which can likewise be very much gathered with Big information.
2. Scene Public:
Scene Public is a free programming that interfaces any information source be it corporate Data Warehouse, Microsoft Excel or electronic information, and makes information representations, maps, dashboards and so on with ongoing updates introducing on web. They can likewise be shared through web-based media or with the customer. It permits the admittance to download the document in various arrangements. On the off chance that you need to see the force of scene, we should have excellent information source. Scene's Big Data abilities makes them significant and one can dissect and envision information better than some other information perception programming on the lookout.

3. Python
Python is an article arranged scripting language which is not difficult to peruse, compose, keep up and is a free open-source apparatus. It was created by Guido van Rossum in late 1980's which upholds both useful and organized programming strategies.
Python is not difficult to learn as it is fundamentally the same as JavaScript, Ruby, and PHP. Likewise, Python has excellent AI libraries viz. Scikitlearn, Theano, Tensorflow and Keras. Another significant component of Python is that it very well may be amassed on any stage like SQL worker, a MongoDB information base or JSON. Python can likewise deal with text information quite well.
4. SAS
Sas is a programming climate and language for information control and an innovator in examination, created by the SAS Institute in 1966 and further created in 1980's and 1990's. SAS is effectively open, managable and can dissect information from any sources. SAS presented an enormous arrangement of items in 2011 for client insight and various SAS modules for web, online media and showcasing examination that is generally utilized for profiling clients and possibilities. It can likewise foresee their practices, oversee, and advance interchanges.
5. Apache Spark
The University of California, Berkeley's AMP Lab, created Apache in 2009. Apache Spark is a quick enormous scope information preparing motor and executes applications in Hadoop groups multiple times quicker in memory and multiple times quicker on circle. Flash is based on information science and its idea makes information science easy. Sparkle is additionally well known for information pipelines and AI models improvement.
Sparkle additionally incorporates a library - MLlib, that gives a reformist arrangement of machine calculations for dreary information science strategies like Classification, Regression, Collaborative Filtering, Clustering, and so on
6. Dominate
Dominate is an essential, mainstream and generally utilized scientific apparatus practically on the whole businesses. Regardless of whether you are a specialist in Sas, R or Tableau, you will in any case have to utilize Excel. Dominate becomes significant when there is a prerequisite of examination on the customer's interior information. It breaks down the intricate errand that sums up the information with a review of rotate tables that helps in separating the information according to customer necessity. Dominate has the development business examination alternative which helps in demonstrating capacities which have prebuilt choices like programmed relationship recognition, a making of DAX measures and time gathering.
7. RapidMiner:
RapidMiner is an incredible coordinated information science stage created by the very organization that performs prescient examination and other progressed investigation like information mining, text examination, AI and visual examination with no programming. RapidMiner can join with any information source types, including Access, Excel, Microsoft SQL, Tera information, Oracle, Sybase, IBM DB2, Ingres, MySQL, IBM SPSS, Dbase and so on The apparatus is exceptionally amazing that can create examination dependent on genuine information change settings, for example you can handle the organizations and informational indexes for prescient investigation.
8. KNIME
KNIME Developed in January 2004 by a group of programmers at University of Konstanz. KNIME is driving open source, detailing, and incorporated examination apparatuses that permit you to break down and model the information through visual programming, it coordinates different segments for information mining and AI by means of its measured information pipelining idea.
9. QlikView
QlikView has numerous one of a kind highlights like protected innovation and has in-memory information handling, which executes the outcome extremely quick to the end clients and stores the information in the actual report. Information relationship in QlikView is consequently kept up and can be packed to practically 10% from its unique size. Information relationship is envisioned utilizing colors - a particular tone is given to related information and another tone for non-related information.
10. Splunk:
Splunk is an apparatus that examines and search the machine-created information. Splunk pulls all content based log information and gives a basic method to look through it, a client can pull on the whole sort of information, and play out such a fascinating measurable investigation on it, and present it in various organizations.
For More Details, Visit Us:
data structures in java
system design interview questions
Google interview questions
cracking the coding interview python
0 notes
Text
300+ TOP IBM JCL Interview Questions and Answers
IBM JCL Interview Questions for freshers experienced :-
1. What is JCL? JCL stands for Job Control Language. It is the command language of Multiple Virtual Storage (MVS). It is the normally used in Operating System in the IBM Mainframe computers. 2. What is the use of JCL? It is used for the deleting creating, the Data Sets, GDG’S and VSAM clusters. It is used for comparing the files and PDS members It is used for compiling and executing the programs which includes batch programs as well It is used for merging and sorting of file data 3.How many extents are possible for a sequential file ? For a VSAM file? 16 extents on a volume for a sequential file and 123 for a VSAM file. 4.What does a disposition of (NEW,CATLG,DELETE) mean? That this is a new dataset and needs to be allocated, to CATLG the dataset if the step is successful and to delete the dataset if the step abends. 5.What does a disposition of (NEW,CATLG,KEEP) mean? That this is a new dataset and needs to be allocated, to CATLG the dataset if the step is successful and to KEEP but not CATLG the dataset if the step abends. Thus if the step abends, the dataset would not be catalogued and we would need to supply the vol. ser the next time we refer to it. 6.How do you access a file that had a disposition of KEEP? Need to supply volume serial no. VOL=SER=xxxx. 7.What does a disposition of (MOD,DELETE,DELETE) mean ? The MOD will cause the dataset to be created (if it does not exist), and then the two DELETEs will cause the dataset to be deleted whether the step abends or not. This disposition is used to clear out a dataset at the beginning of a job. 8.What is the DD statement for a output file? Unless allocated earlier, will have the foll parameters: DISP=(NEW,CATLG,DELETE), UNIT , SPACE & DCB . 9.What do you do if you do not want to keep all the space allocated to a dataset? Specify the parameter RLSE ( release ) in the SPACE e.g. SPACE=(CYL,(50,50),RLSE) 10.What is DISP=(NEW,PASS,DELETE)? This is a new file and create it, if the step terminates normally, pass it to the subsequent steps and if step abends, delete it. This dataset will not exist beyond the JCL.
IBM JCL Interview Questions 11.How do you create a temporary dataset? Where will you use them? Temporary datasets can be created either by not specifying any DSNAME or by specifying the temporary file indicator as in DSN=&&TEMP. We use them to carry the output of one step to another step in the same job. The dataset will not be retained once the job completes. 12.How do you restart a proc from a particular step? In job card, specify RESTART=procstep.stepname where procstep = name of the jcl step that invoked the proc and stepname = name of the proc step where you want execution to start 13.How do you skip a particular step in a proc/JOB? Can use either condition codes or use the jcl control statement IF (only in ESA JCL) 14.A PROC has five steps. Step 3 has a condition code. How can you override/nullify this condition code? Provide the override on the EXEC stmt in the JCL as follows: //STEP001 EXEC procname,COND.stepname=value All parameters on an EXEC stmt in the proc such as COND, PARM have to be overridden like this. 15.How do you override a specific DDNAME/SYSIN in PROC from a JCL? // DSN=... 16.What is NOTCAT ? This is an MVS message indicating that a duplicate catalog entry exists. E.g., if you already have a dataset with dsn = 'xxxx.yyyy' and u try to create one with disp new,catlg, you would get this error. the program open and write would go through and at the end of the step the system would try to put it in the system catalog. at this point since an entry already exists the catlg would fail and give this message. you can fix the problem by deleting/uncataloging the first data set and going to the volume where the new dataset exists(this info is in the msglog of the job) and cataloging it. 17.What is 'S0C7' abend? Caused by invalid data in a numeric field. 18.What is a S0C4 error ? Storage violation error - can be due to various reasons. e.g.: READING a file that is not open, invalid address referenced due to subscript error. 19.What are SD37, SB37, SE37 abends? All indicate dataset out of space. SD37 - no secondary allocation was specified. SB37 - end of vol. and no further volumes specified. SE37 - Max. of 16 extents already allocated. 20.What is S322 abend ? Indicates a time out abend. Your program has taken more CPU time than the default limit for the job class. Could indicate an infinite loop. 21.Why do you want to specify the REGION parameter in a JCL step? To override the REGION defined at the JOB card level. REGION specifies the max region size. REGION=0K or 0M or omitting REGION means no limit will be applied. 22.What does the TIME parameter signify ? What does TIME=1440 mean ? TIME parameter can be used to overcome S322 abends for programs that genuinely need more CPU time. TIME=1440 means no CPU time limit is to be applied to this step. 23.What is COND=EVEN ? Means execute this step even if any of the previous steps, terminated abnormally. 24.What is COND=ONLY ? Means execute this step only if any of the previous steps, terminated abnormally. 25.How do you check the syntax of a JCL without running it? TYPERUN=SCAN on the JOB card or use JSCAN. 26.What does IEBGENER do? Used to copy one QSAM file to another. Source dataset should be described using SYSUT1 ddname. Destination dataset should be decribed using SYSUT2. IEBGENR can also do some reformatting of data by supplying control cards via SYSIN. 27.How do you send the output of a COBOL program to a member of a PDS? Code the DSN as pds(member) with a DISP of SHR. The disp applies to the pds and not to a specific member. 28.I have multiple jobs ( JCLs with several JOB cards ) in a member. What happens if I submit it? Multiple jobs are submitted (as many jobs as the number of JOB cards). 29.I have a COBOL program that ACCEPTs some input data. How do you code the JCL statment for this? ( How do you code instream data in a JCL? ) //SYSIN DD* input data input data /* 30.Can you code instream data in a PROC ? No. 31. How do you overcome this limitation ? One way is to code SYSIN DD DUMMY in the PROC, and then override this from the JCL with instream data. 32.How do you run a COBOL batch program from a JCL? How do you run a COBOL/DB2 program? To run a non DB2 program, //STEP001 EXEC PGM=MYPROG To run a DB2 program, //STEP001 EXEC PGM=IKJEFT01 //SYSTSIN DD * DSN SYSTEM(....) RUN PROGRAM(MYPROG) PLAN(.....) LIB(....) PARMS(...) /* 33.What is STEPLIB, JOBLIB? What is it used for? Specifies that the private library (or libraries) specified should be searched before the default system libraries in order to locate a program to be executed. STEPLIB applies only to the particular step, JOBLIB to all steps in the job. 34.What is order of searching of the libraries in a JCL? First any private libraries as specified in the STEPLIB or JOBLIB, then the system libraries such as SYS1.LINKLIB. The system libraries are specified in the linklist. 35.What happens if both JOBLIB & STEPLIB is specified ? JOBLIB is ignored. 36.When you specify mutiple datasets in a JOBLIB or STEPLIB, what factor determines the order? The library with the largest block size should be the first one. 37.How to change default proclib ? //ABCD JCLLIB ORDER=(ME.MYPROCLIB,SYS1.PROCLIB) 38.The disp in the JCL is MOD and the program opens the file in OUTPUT mode. What happens ? The disp in the JCL is SHR and the pgm opens the file in EXTEND mode. What happens ? Records will be written to end of file (append) when a WRITE is done in both cases. 39.What are the valid DSORG values ? PS - QSAM, PO - Partitioned, IS - ISAM 40.What are the differences between JES2 & JES3 ? JES3 allocates datasets for all the steps before the job is scheduled. In JES2, allocation of datasets required by a step are done only just before the step executes. 41. What are the differences between JES2 & JES3 ? JES3 allocates Data Sets for all the steps before the job is scheduled. In JES2, allocation of Data Sets Required by a step are done only just before the step executes. JOB /EXEC/DD ALL PARAMETERS JOBLIB/STEPLIB PROCEDURES, PARAMETERS PASSING CONDITION VARIABLES ABEND CODES. 42. What are the kinds of job control statements? The JOB, EXEC and DD statement 43. What is the meaning of keyword in JCL?What is its opposite? A keyword in a JCL statement may appear in different places and is recognized by its name, eg MSGCLASS in the JOB statement The opposite is positional words, where their meaning is based on their position in the statement, eg in the DISP keyword the =(NEW,CATLG,DELETE) meaning are based on first, second and third position. 44. Describe the JOB statement, its meaning, syntax and significant keywords.? The JOB statement is the first in a JCL stream Its format is // jobname, keyword JOB, accounting information in brackets and keywords, MSGCLASS, MSGLEVEL, NOTIFIY, CLASS, etc . 45. Describe the EXEC statement, its meaning, syntax and keywords.? The EXEC statement identifies the program to be executed via a PGM=program name keyword Its format is //jobname EXEC PGM=program name The PARM= keyword can be used to pass external values to the executing program. 46. Describe the DD statement, its meaning, syntax and keywords.? The DD statement links the external Data Set name (DSN) to the DDNAME coded within the executing program It links the File names within the program code to the File names know to the MVS operating system The syntax is // ddname DD DSN=Data Set name Other keywords after DSN are DISP, DCB, SPACE, etc . 47. What is a PROC?What is the difference between an instream and a catalogued PROC? PROC stands for procedure It is 'canned' JCL invoked by a PROC statement An instream PROC is presented within the JCL; a catalogued PROC is referenced from a proclib partitioned Data Set. 48. What is the difference between a symbolic and an override in executing a PROC? A symbolic is a PROC placeholder; the value for the symbolic is supplied when the PROC is invoked, eg &symbol=value An override replaces the PROC's statement with another one; it substitutes for the entire statement 49. What is RESTART? How is it invoked? A RESTART is a JOB statement keyword It is used to restart the job at a specified s step rather than at the beginning 50. What is a GDG? How is it referenced?How is it defined? What is a MODELDSCB? GDG stands for generation data group It is a Data Set with versions that can be referenced absolutely or relatively It is defined by an IDCAMS define generation datagroup execution 51.What is primary allocation for a dataset? The space allocated when the dataset is first created. 52. Explain in ways data can be passed to a COBOL program from JCL? Data can be passed to a COBOL program from JCL through Files SYSIN DD statement PARM parameter 53.What is the difference between primary and secondary allocations for a dataset? Secondary allocation is done when more space is required than what has already been allocated. IBM JCL Questions and Answers Pdf Download Read the full article
0 notes
Text
Sql Interview Questions
If a WHERE stipulation is made use of in cross join then the inquiry will function like an INNER JOIN. A SPECIAL restriction guarantees that all worths in a column are different. This supplies originality for the column and also aids identify each row distinctly. It promotes you to manipulate the information kept in the tables by utilizing relational drivers. Instances of the relational data source monitoring system are Microsoft Access, MySQL, SQLServer, Oracle database, and so on. Unique vital restraint distinctly identifies each document in the data source. This vital supplies individuality for the column or collection of columns. A database cursor is a control framework that allows for traversal of documents in a data source. Cursors, in addition, facilitates handling after traversal, such as access, enhancement and also deletion of database records. They can be viewed as a reminder to one row in a collection of rows. An alias is a attribute of SQL that is sustained by a lot of, otherwise all, RDBMSs. It is a momentary name appointed to the table or table column for the objective of a specific SQL inquiry. Additionally, aliasing can be used as an obfuscation strategy to protect the real names of data source fields. A table pen name is additionally called a connection name.
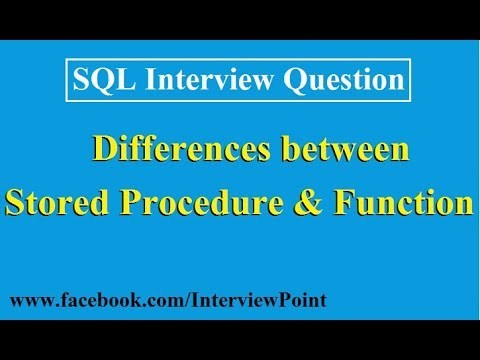
pupils; Non-unique indexes, on the other hand, are not made use of to impose restraints on the tables with which they are associated. Rather, non-unique indexes are made use of only to enhance query efficiency by maintaining a sorted order of data values that are made use of regularly. A data source index is a information framework that provides quick lookup of information in a column or columns of a table. It improves the speed of procedures accessing information from a database table at the price of added creates and memory to preserve the index data structure. Prospects are likely to be asked basic SQL interview questions to advance degree SQL concerns relying on their experience and also different other elements. The below list covers all the SQL interview concerns for betters as well as SQL meeting concerns for knowledgeable degree candidates as well as some SQL query interview questions. SQL clause helps to limit the result set by providing a condition to the question. A clause helps to filter the rows from the whole collection of records. Our SQL Meeting Questions blog is the one-stop resource from where you can improve your meeting prep work. It has a collection of leading 65 concerns which an interviewer intends to ask during an interview procedure. Unlike main crucial, there can be multiple one-of-a-kind restraints specified per table. The code syntax for UNIQUE is quite comparable to that of PRIMARY SECRET and also can be utilized interchangeably. The majority of modern database monitoring systems like MySQL, Microsoft SQL Server, Oracle, IBM DB2 as well as Amazon Redshift are based upon RDBMS. SQL stipulation is specified to restrict the outcome set by providing problem to the query. This typically filters some rows from the entire collection of documents. Cross sign up with can be defined as a cartesian item of the two tables included in the join. https://bit.ly/3tmWIsh after sign up with has the exact same variety of rows as in the cross-product of number of rows in the two tables. Self-join is readied to be query utilized to compare to itself. This is utilized to compare values in a column with various other worths in the exact same column in the same table. https://geekinterview.net can be made use of for the very same table contrast. This is a key words utilized to inquire information from more tables based on the partnership between the fields of the tables. A foreign secret is one table which can be associated with the main key of an additional table. Partnership requires to be produced in between 2 tables by referencing foreign secret with the primary secret of one more table. A Distinct crucial constraint uniquely identified each record in the data source. It starts with the fundamental SQL interview inquiries as well as later on remains to sophisticated inquiries based upon your discussions and solutions. These SQL Interview concerns will assist you with different proficiency levels to reap the maximum gain from this blog. A table contains a specified variety of the column called fields but can have any type of number of rows which is known as the record. So, the columns in the table of the data source are known as the fields and also they represent the feature or attributes of the entity in the document. Rows right here refers to the tuples which represent the simple data thing and columns are the quality of the data things present specifically row. Columns can categorize as upright, and also Rows are horizontal. There is offered sql meeting inquiries as well as responses that has been asked in many firms. For PL/SQL interview concerns, see our following web page. A sight can have information from several tables integrated, as well as it relies on the connection. https://tinyurl.com/c7k3vf9t are utilized to use safety and security mechanism in the SQL Web server. The sight of the database is the searchable things we can utilize a question to search the consider as we utilize for the table. RDBMS means Relational Database Monitoring System. It is a database monitoring system based upon a relational version. RDBMS stores the information right into the collection of tables and also links those table utilizing the relational drivers conveniently whenever called for. This gives uniqueness for the column or set of columns. A table is a set of information that are arranged in a design with Columns and Rows. https://is.gd/snW9y3 can be classified as upright, and also Rows are straight. A table has actually defined number of column called fields yet can have any kind of variety of rows which is called document. RDBMS store the information into the collection of tables, which is related by typical areas in between the columns of the table. It likewise offers relational drivers to control the information stored into the tables. Adhering to is a curated listing of SQL interview concerns as well as responses, which are most likely to be asked throughout the SQL interview.
0 notes
Text
Question 67: How can I create a pipeline to copy data from an on-premises DB2 database to Azure Blob Storage using Azure Data Factory?
Interview Questions on Azure Data Factory Development: #interview, #interviewquestions, #Microsoft, #azure, #adf , #eswarstechworld, #azuredataengineer, #development, #azuredatafactory , #azuredeveloper
Azure Data Factory is a cloud-based data integration service that enables you to create and manage data-driven workflows. In this scenario, the pipeline is designed to extract data from an on-premises DB2 database and load it into Azure Blob Storage. Categories: The pipeline to copy data from an on-premises DB2 database to Azure Blob Storage falls under the data integration and data movement…

View On WordPress
#adf#azure#azuredataengineer#azuredatafactory#azuredeveloper#development#eswarstechworld#interview#interviewquestions#Microsoft
0 notes
Text
db2 interview questions
complete notes on db2, complete tutorials on db2, db2 notes, db2 questions asked in companies, db2 questions asked in interview, db2 questions asked in mnc, db2 questions for interview, db2 tutorials, faq for db2, faq on db2, interview questions on db2, latest interview questions on db2, most asked db2 interview questions, notes on db2, rapid fire on db2, rapid fire questions on db2, top interview questions on db2, tutorials on db2, updated interview questions answers on db2, db2 interview questions
0 notes
Link
0 notes