#javascripttricks
Explore tagged Tumblr posts
Video
youtube
Stop Using Console Log | Console Table Method | Powerful Console JavaScr...
#youtube#JavaScript Debugging DataVisualization WebDevelopment CodingTips JavaScriptTricks Programming WebDev ConsoleTable JavaScriptMethods CodeOrga
0 notes
Text
10 JavaScript Tricks Every Developer Should Know
JavaScript is a tremendously powerful language, yet understanding its behavior can be difficult. Today I'll show you several JavaScript tricks for optimizing your JavaScript code. It will also assist you in writing clean code and optimizing your programming time. Use these JavaScript tricks to optimize your JavaScript code.
1. Resize or Empty an Array Using array.length
When we wish to resize an array, we basically overwrite its length: let array = ; console.log(array.length); // returns the length as 10 array.length = 4; console.log(array.length); // returns the length as 4 console.log(array); // returns
2. Flatten the multidimensional array
Using the spread operator, we may flatten a multidimensional array. var entries = , , 9]; var flat_entries = .concat(...entries); //
3. Getting the last item in the array
Using a negative number as the parameter allows us to retrieve the last piece from the array, which is another clever JavaScript tricks. var array = ; console.log(array.slice(-1)); // console.log(array.slice(-2)); // console.log(array.slice(-3)); //
4. Swap two variables without the third variable
Using the third variable, we can easily swap two variables. However, adding a variable requires adding memory. Additionally, memory can be optimized with just two variables. let x = 1; let y = 2; = ; // x = 2, y = 1
5. Merging two arrays by optimizing memory
Using the array.concat() function, we can simply combine two arrays if necessary. var array1 = ; var array2 = ; console.log(array1.concat(array2)); // ; The best option for tiny arrays is this. However, if we need to merge big arrays, this strategy is completely inadequate. Why so? The array is the cause. concat() function on large arrays will consume a lot of memory while creating the new merged array. There is no need for additional memory in this situation because we can use the array.push.apply(array1, array2) function to merge the second array into the first one. By doing this, we may use less memory. var array1 = ; var array2 = ; console.log(array1.push.apply(array1, array2)); // ;
6. Instead of plain delete use splice
This could be the most effective JavaScript trick for simplifying JavaScript code. It also accelerates your code. Why shouldn't we use delete? Because it only deletes the object property and does not reindex or update the length of the array, leaving undefined values. Basically, it takes a very long time to execute. Splicing rather than deleting is a smart technique because it removes some null/undefined space from your code. Array = Array.splice(0, 2) Result: myArray
7. We should use a switch…case instead of if/else
The switch…case is always overlooked. The switch…case and if/else statements accomplish nearly identical tasks, however the switch…case statement executes faster than the if/else statement! Why is this the case? The if/else statement compares on average to get to the proper clause, but the switch statement is essentially a lookup table with known options.
8. Break the loop as soon as possible
Look for situations in which it is not necessary to complete every iteration of a loop. For example, if we are looking for a specific value and find it, future rounds are superfluous. As a result, we need use a break statement to end the loop's execution:
9. Reduce the chance of memory leaks
JavaScript relies heavily on memory management. If you do not correctly manage memory, memory will leak and cause your software to crash. A memory leak can occur for a variety of reasons. Some of the reasons are: unintentionally using global variables, forgetting about timers or callbacks, unneeded cache, and so on.
10. Understanding Big O notation
This is a fundamental yet underappreciated topic. If you master the Big O notation, you will quickly understand why some of your functions run faster and others use less memory. So, before getting into frameworks and other advanced topics, master this essential concept. Thank you for your time. If you found this helpful, don't forget to share your JavaScript tricks with us. Read the full article
0 notes
Photo

The Caesor Cipher Algorithms The Caesor Cipher algorithms is an opening to the world of cryptography...where you meet and take up a challenge to secure sensitive data. History has it that it was used by the famous Roman Empire known as Julius Caesor to send encrypted messages to his generals to hide sensitive communication from enemies. Well, today it is applied as a basis for a number of encryption algorithms including the famous RTO13 and others. In my next slide set we take a look at the RTO13. For the time being,take a look at how the Caesor Cipher Algorithms work. Enjoy the reading #javascriptalgorithms #encryption #decryption #caesorcipher #rto13 #javascripttricks #programingchallenge (at Lilongwe, Malawi) https://www.instagram.com/p/CFFjDNPh_6_/?utm_medium=tumblr
0 notes
Photo
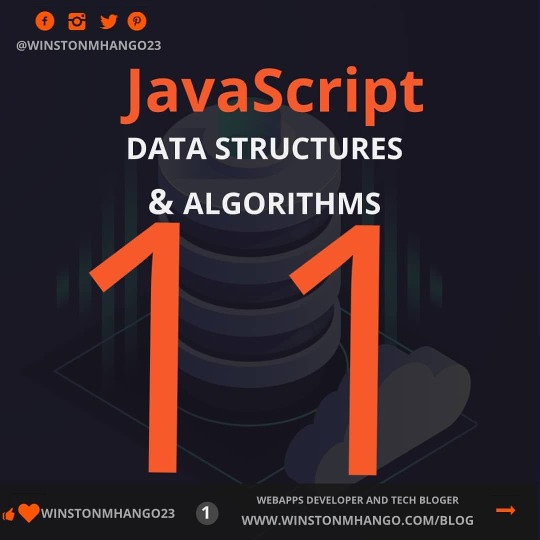
The Caesor Cipher Algorithms The Caesor Cipher algorithms is an opening to the world of cryptography...where you meet and take up a challenge to secure sensitive data. History has it that it was used by the famous Roman Empire known as Julius Caesor to send encrypted messages to his generals to hide sensitive communication from enemies. Well, today it is applied as a basis for a number of encryption algorithms including the famous RTO13 and others. In my next slide set we take a look at the RTO13. For the time being,take a look at how the Caesor Cipher Algorithms work. Enjoy the reading #javascriptalgorithms #encryption #decryption #caesorcipher #rto13 #javascripttricks #programingchallenge (at Lilongwe, Malawi) https://www.instagram.com/p/CFFjDNPh_6_/?igshid=892rebyth143
0 notes
Text
Design and Build Your Own #JavaScript Library: #JavaScriptTips & #JavaScriptTricks https://t.co/xYK3snbxGh #JS
Design and Build Your Own #JavaScript Library: #JavaScriptTips & #JavaScriptTricks https://t.co/xYK3snbxGh #JS
— Macronimous.com (@macronimous) April 15, 2018
from Twitter https://twitter.com/macronimous April 15, 2018 at 07:45PM via IFTTT
0 notes
Photo

Finding most recurring character in a string part 2 In our yesterday's post,we used object method to find most recurring character in a string,in this part we use the Array method. For the most part, you should be familiar with the first few slides,but focus on the last few #javascripttricks #algorithms #datastructures (at Lilongwe, Malawi) https://www.instagram.com/p/CELOa8Whyjo/?utm_medium=tumblr
0 notes
Photo

Finding most recurring character in a string part 2 In our yesterday's post,we used object method to find most recurring character in a string,in this part we use the Array method. For the most part, you should be familiar with the first few slides,but focus on the last few #javascripttricks #algorithms #datastructures (at Lilongwe, Malawi) https://www.instagram.com/p/CELOa8Whyjo/?igshid=1vp8dzrrdn7as
0 notes
Photo
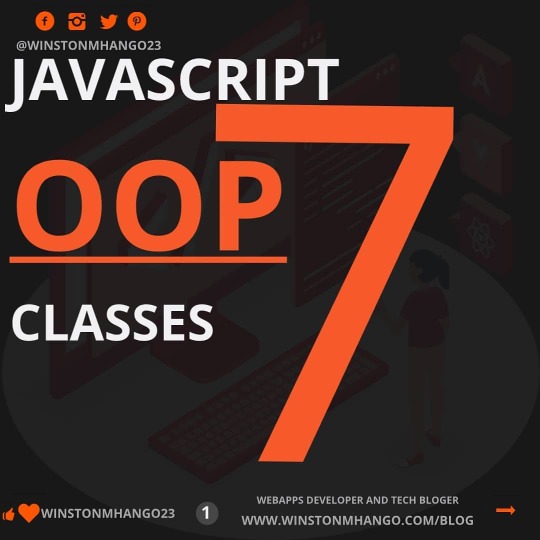
OOP JavaScript : Classes are not classes in JavaScript 👩💻👴🏻👴🏻. Let's take a look what exactly classes are in Javascript now that up to this 7th slides set agree that Javascript is a prototype based Object Oriented Programming language. Yes,they are not classes,but functions behind the scene.They are classes and work classically on the surface. Let's dive in at take a look. #javascripttricks #javascriptobjects #classexpressions #constructorfunctions #objectorientedprogramming #prototypes #prototypeinheritance (at Lilongwe, Malawi) https://www.instagram.com/p/CCJYdaIBoDr/?igshid=4avmysv51v7s
#javascripttricks#javascriptobjects#classexpressions#constructorfunctions#objectorientedprogramming#prototypes#prototypeinheritance
0 notes
Photo

Implementing OOP inheritance using constructor functions in Javascript. So far we have seen that JavaScript is a prototype based programming language but can also implement pretty well the OOP principles. In this slides set we are looking at how constructor functions work behind the scenes to get away with the implementation of inheritance in Javascript. We look at how to construct a constructor function as a blue print,create instances from it as well as challenges associated with constructors and a variety of work arounds. Enjoy the reading #constructorfunctions #inheritance #javascriptoop #javascripttricks (at Lilongwe, Malawi) https://www.instagram.com/p/CB-339GBvo8/?igshid=1fr3aajo254xg
0 notes
Photo
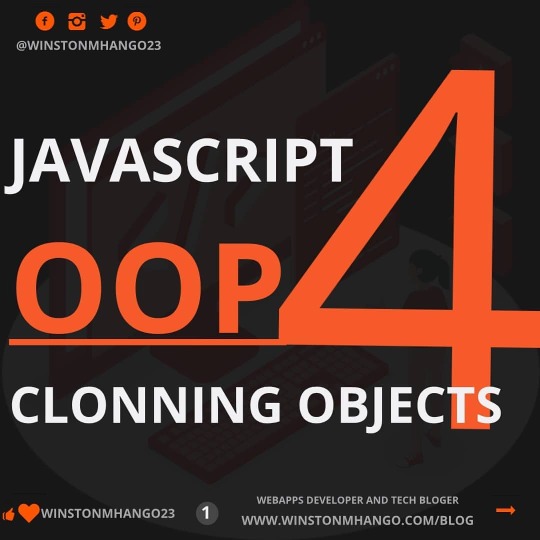
JavaScript OOP: Bypassing the [[prototype]] object to link and inherit properties and methods from our custom objects. This time around I need to take you into alitle bit deep waters down the Javascript language.If you followed my last three slide sets on OOP JavaScript series,this part will not be difficult to follow. What we are doing here is making a custom object the prototype (blue print) from which other objects can inherit properties and methods. This actually sets some basis for some next lessons in this series. Enjoy the reading #javascripttricks #javascriptdatatypes #javascriptstatements #javascripproperties #javascriptmethods #javascriptprototypes #javascriptinheritance #prototypes #prototypechain (at Lilongwe, Malawi) https://www.instagram.com/p/CBfRg0fhVaL/?igshid=i83hykb82isc
#javascripttricks#javascriptdatatypes#javascriptstatements#javascripproperties#javascriptmethods#javascriptprototypes#javascriptinheritance#prototypes#prototypechain
0 notes
Photo
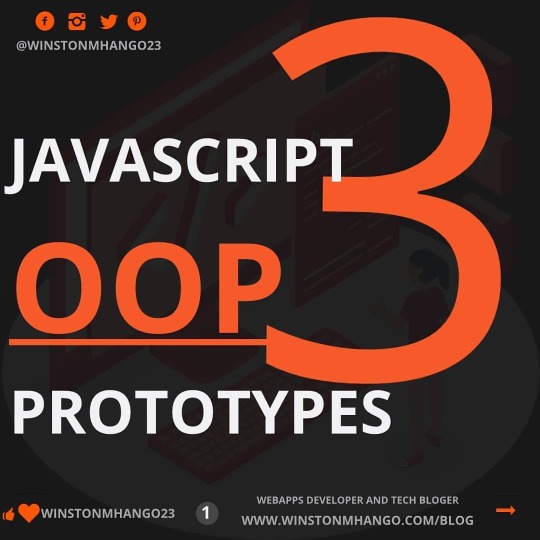
JavaScript OOP:The prototype and prototype chain. In this part we will be looking at the prototype object and prototype chain. The knowledge is very fundamental to understanding how Javascript works and is the very key to understanding prototypal inheritance. Working our way down to understanding the constructors and down to understanding that a class in Javascript is nothing but a function depends very much on the Basics of prototype knowledge. Enjoy the reading #javascripttricks #javascriptinheritance #prototupeinheritance #prototypes (at Lilongwe, Malawi) https://www.instagram.com/p/CBOkpfPhdMu/?igshid=1jrdh6mzgtjtl
0 notes
Photo

Tricks for Quickly understanding JavaScript concepts. I learnt and grasped the ins-and-outs of python in about 2 months 😎😎😀. For JavaScript,it was a completely different story ����🤣. You think you understand it now until stuff like hoisting, lexical environment,closures,callbacks,IIFE,then data structures pop-in. Then, you are like what@#$.Then you are forced to either give up or copy smart coders' code as you go until you find peace with it all. But hey,my trick was to learn JAVA😙.Ahhnha! Yes,not javascript,Java.I got a few ideas,then developed some tricks of my own after a long list of reads from a bunch of 200s,if not a thousand paged book volumes on javascript coupled with YouTube tutorials...bakey... the new Boston...w3schools... In this short slides tutorial,I show you one of the many tricks to avoid going that lane. Enjoy the reading #javascript #javascripttricks #javascriptobjects #javascriptarrays #javascriptnumbers #javascriptdate #javascriptfunctions #javascriptlists (at Lilongwe, Malawi) https://www.instagram.com/p/B8n05u_Bxk3/?igshid=1qeegm832yf6z
#javascript#javascripttricks#javascriptobjects#javascriptarrays#javascriptnumbers#javascriptdate#javascriptfunctions#javascriptlists
0 notes
Text
Design and Build Your Own #JavaScript Library: #JavaScriptTips & #JavaScriptTricks https://t.co/pXZoqXIboR #JS
Design and Build Your Own #JavaScript Library: #JavaScriptTips & #JavaScriptTricks https://t.co/pXZoqXIboR #JS
— Macronimous.com (@macronimous) December 12, 2017
from Twitter https://twitter.com/macronimous December 13, 2017 at 05:08AM via IFTTT
0 notes
Text
Design and Build Your Own #JavaScript Library: #JavaScriptTips & #JavaScriptTricks https://t.co/Q4bcomv4tz #JS
Design and Build Your Own #JavaScript Library: #JavaScriptTips & #JavaScriptTricks https://t.co/Q4bcomv4tz #JS
— Macronimous.com (@macronimous) November 10, 2017
from Twitter https://twitter.com/macronimous November 10, 2017 at 06:27PM via IFTTT
0 notes
Text
Design and Build Your Own #JavaScript Library: #JavaScriptTips & #JavaScriptTricks https://t.co/wZZKhmto3A #JS
Design and Build Your Own #JavaScript Library: #JavaScriptTips & #JavaScriptTricks https://t.co/wZZKhmto3A #JS
— Macronimous.com (@macronimous) July 5, 2017
from Twitter https://twitter.com/macronimous July 05, 2017 at 10:30PM via IFTTT
0 notes
Text
Design and Build Your Own #JavaScript Library: #JavaScriptTips & #JavaScriptTricks https://t.co/DDGZX2ThDA #JS
Design and Build Your Own #JavaScript Library: #JavaScriptTips & #JavaScriptTricks https://t.co/DDGZX2ThDA #JS
— Macronimous.com (@macronimous) July 4, 2017
from Twitter https://twitter.com/macronimous July 05, 2017 at 12:37AM via IFTTT
0 notes