#prototypechain
Explore tagged Tumblr posts
Photo
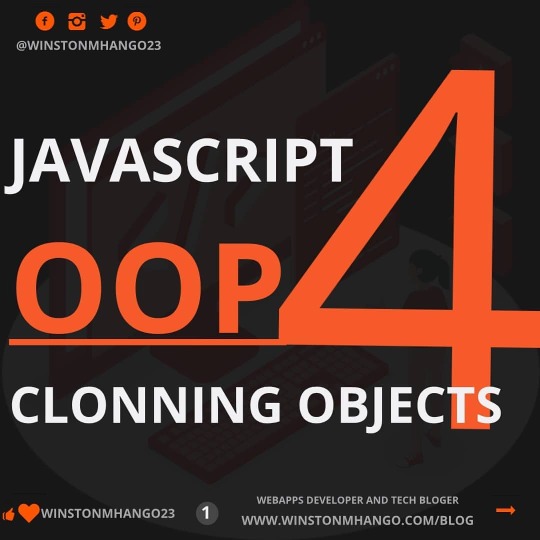
JavaScript OOP: Bypassing the [[prototype]] object to link and inherit properties and methods from our custom objects. This time around I need to take you into alitle bit deep waters down the Javascript language.If you followed my last three slide sets on OOP JavaScript series,this part will not be difficult to follow. What we are doing here is making a custom object the prototype (blue print) from which other objects can inherit properties and methods. This actually sets some basis for some next lessons in this series. Enjoy the reading #javascripttricks #javascriptdatatypes #javascriptstatements #javascripproperties #javascriptmethods #javascriptprototypes #javascriptinheritance #prototypes #prototypechain (at Lilongwe, Malawi) https://www.instagram.com/p/CBfRg0fhVaL/?igshid=i83hykb82isc
#javascripttricks#javascriptdatatypes#javascriptstatements#javascripproperties#javascriptmethods#javascriptprototypes#javascriptinheritance#prototypes#prototypechain
0 notes
Text
What is prototype and prototype chaining in JavaScript
In JavaScript, a prototype is an object from which another object is inherited. When I was using console.log() for an object in the browser console, that is when I first encountered the word "prototype" in JavaScript. After seeing my own properties, which I had purposefully constructed, I continued with my debugging. Since I hadn't made it and assumed it had something to do with the language and wasn't worth worrying about for now, I had entirely disregarded the prototype object that was displayed in the console. Everyone who has experience with class-based object-oriented design, where classes are clearly defined together with their attributes and functions, encounters this problem. But JavaScript is distinct from class-based languages. The prototype-based language JavaScript has a very dynamic nature. JavaScript allows you to start with an empty object and continuing adding properties dynamically. In contrast, class-based languages require that the characteristics be defined explicitly prior to compilation. You could believe that JavaScript already uses the class keyword from ES2015, but classes in JavaScript still operate on a prototype-based model. However, now that JavaScript is widely used for front-end and back-end development, it is crucial for us to comprehend the language features of prototype and prototype chaining in JavaScript. In our last piece, we covered the numerous approaches to creating a JavaScript object. Check out the article by clicking this link: Creating Objects with JavaScript. What is a prototype and prototype chaining in JavaScript will be explained in this post.
Prototype
In JavaScript, a prototype is an object from which another object is inherited. In class-based languages, a class is the container for all of the methods and attributes that have been defined for the class. The public methods and attributes defined inside the class are accessible to the newly formed object when the object of the class is created. Prototype and the class that serves as the model for JavaScript objects are extremely similar. In addition to its own properties and methods, the newly produced JavaScript object has access to all of the properties and methods of the prototype from which it was constructed. Due to JavaScript's dynamic nature, we can also dynamically add properties to the prototype object. This would entail adding a property or behavior to the base class at runtime so that the properties added dynamically to the prototype object are accessible to all newly produced objects. function Student(name,age){ this.name = name; this.age = age; } let student1 = new Student('John',32) let student2 = new Student('Mary',32) console.log(student1); console.log(student2); student1.sports = 'Cricket'; Student.prototype.gender = null let student3 = new Student('Shailesh',26)
Prototype Chaining
JavaScript uses prototypes as its inheritance mechanism. An item's prototype would also include the actual thing. This goes on until there is no longer a prototype object at the top level. In JavaScript, this is referred to as prototype chaining or prototype chain. The prototype objects' defined properties are also available to the object instance. And it is for this reason that we can access attributes that we have not explicitly defined on an object since they are accessible through inheritance via prototype chaining. Any time we attempt to access a property of an object, the object's own property is examined first. If the property is missing from the own property, it is scanned for in the prototype object. This continues until the requested attribute is obtained or the prototype chain ends with an undefined result.
Performance Effects of Prototype Chaining
The overall time to search up a property that is at a higher level of prototype chaining may have a detrimental impact on performance in the code where performance is of the utmost concern due to the various levels of prototype chaining. Additionally, there may be instances where we attempt to search up a property that does not exist, but since the property must be looked up on the prototype chain until we reach the end, we risk wasting time in the lookup process. The function hasOwnProperty() function, which all objects inherit from Object.prototype in order to limit the lookup to a specific level, can be used to prevent such a circumstance. The property name we are looking for is passed to function hasOwnProperty(), which then returns true or false depending on whether the property was located or not. Keep in mind that a JavaScript object's properties can be accessed as well as its functions. function Student(name,age){ this.name = name; this.age = age; } const student = new Student('Shailesh',32) console.log(student) The prototype object of the Student function Object() constructor, which also has a prototype object descended from the Object function Object() constructor, which does not have a prototype object since Object is the top level of the prototype chain, is the first level of the prototype chain as seen in the image above. By using Object.getPrototypeOf or the proto property of the object instance, we may retrieve the prototype of the object instance (obj) The following methods can be used to obtain the student object prototype in the example above: - Object.getPrototypeOf(student) - student.__proto__ - Student.prototype The preceding statements would all produce the same output when executed in the console, but if we tried Object.getPrototypeOf(Student) in the console, the output would differ. Instead of the object instance student, Tt would return the prototype of the function Object() { } function student. I hope you have a basic understanding of - - How is class-based object oriented design different from JavaScript. - Describe a prototype. - What does JavaScript's prototype chaining or prototype chain mean? - Performance Effects of Prototype Chaining I hope you will like the content and it will help you to learn What is prototype and prototype chaining in JavaScript If you like this content, do share. Read the full article
0 notes
Text
Week 2, Day 6
Today i began JavaScript Koans and reached test 77. While the majority of the work didn’t pose much of a challenge, i did stumble across the concept of the prototype chain. I don’t know that i’ll be any clearer in my explanation than the following extract from the MDN:
// Let's assume we have object o, with its own properties a and b: // {a: 1, b: 2} // o.[[Prototype]] has properties b and c: // {b: 3, c: 4} // Finally, o.[[Prototype]].[[Prototype]] is null. // This is the end of the prototype chain as null, // by definition, has no [[Prototype]]. // Thus, the full prototype chain looks like: // {a:1, b:2} ---> {b:3, c:4} ---> null console.log(o.a); // 1 // Is there an 'a' own property on o? Yes, and its value is 1. console.log(o.b); // 2 // Is there a 'b' own property on o? Yes, and its value is 2. // The prototype also has a 'b' property, but it's not visited. // This is called "property shadowing" console.log(o.c); // 4 // Is there a 'c' own property on o? No, check its prototype. // Is there a 'c' own property on o.[[Prototype]]? Yes, its value is 4. console.log(o.d); // undefined // Is there a 'd' own property on o? No, check its prototype. // Is there a 'd' own property on o.[[Prototype]]? No, check its prototype. // o.[[Prototype]].[[Prototype]] is null, stop searching, // no property found, return undefined
Towards the end of the night i began reading up on angularJS - a very popular javascript framework that everyone is going crazy over. My plan is to implement the ga-bank app as an angular app to get experience with the framework and see what the excitement is all about.
I have been using two main resources to study up on angularJS:
egghead.io
thinkster.io
0 notes