#laravel post request from controller
Explore tagged Tumblr posts
Text
Detect SSRF in Symfony Apps – Free Security Checker
Server-Side Request Forgery (SSRF) is a critical web application vulnerability that occurs when an attacker is able to make the server perform unintended requests to internal or external systems. Symfony, while secure by design, can still be vulnerable to SSRF when insecure coding patterns are used.

In this post, we'll break down how SSRF works in Symfony, show coding examples, and share how you can detect it using our website vulnerability scanner online free.
🚨 What is SSRF and Why is it Dangerous?
SSRF occurs when a web server is tricked into sending a request to an unintended destination, including internal services like localhost, cloud metadata endpoints, or other restricted resources.
Impact of SSRF:
Internal network scanning
Accessing cloud instance metadata (AWS/GCP/Azure)
Bypassing IP-based authentication
🧑💻 SSRF Vulnerability in Symfony: Example
Here’s a simplified example in a Symfony controller where SSRF could be introduced:
// src/Controller/RequestController.php namespace App\Controller; use Symfony\Component\HttpFoundation\Request; use Symfony\Component\HttpFoundation\Response; use Symfony\Bundle\FrameworkBundle\Controller\AbstractController; class RequestController extends AbstractController { public function fetch(Request $request): Response { $url = $request->query->get('url'); // Unvalidated user input! // SSRF vulnerability: external requests with unsanitized user input $content = file_get_contents($url); return new Response($content); } }
⚠️ What’s wrong here?
The $url parameter comes directly from user input and is passed to file_get_contents() with no validation. This allows attackers to make arbitrary requests through your server.
✅ How to Fix It
Use a whitelist approach:
$allowedHosts = ['example.com', 'api.example.com']; $parsedUrl = parse_url($url); if (!in_array($parsedUrl['host'], $allowedHosts)) { throw new \Exception("Disallowed URL"); }
Better yet, avoid allowing user-defined URLs entirely unless absolutely necessary. Always sanitize and validate any user input that affects backend requests.
🧪 Test Your Site for SSRF and Other Vulnerabilities
Our free website vulnerability scanner helps you find SSRF and many other issues instantly. No sign-up required.
📷 Screenshot of the tool homepage

Screenshot of the free tools webpage where you can access security assessment tools.
Just input your domain and get a detailed vulnerability assessment to check Website Vulnerability in seconds.
📷 Screenshot of a generated vulnerability report

An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities.
🔗 Try Our Free Website Security Checker
Go to https://free.pentesttesting.com and check your site for SSRF and dozens of other vulnerabilities.
💡 Real-World SSRF Exploitation: Metadata Services
In many cloud setups (like AWS), SSRF is used to access instance metadata services, such as:
curl http://169.254.169.254/latest/meta-data/
If the Symfony app allows attackers to proxy requests through the server, they can potentially leak AWS credentials!
🔍 Symfony + SSRF in HTTP Client
If you're using the HttpClient component:
use Symfony\Contracts\HttpClient\HttpClientInterface; public function __construct(HttpClientInterface $client) { $this->client = $client; } public function fetchUrl(string $url) { $response = $this->client->request('GET', $url); return $response->getContent(); }
Danger: Still vulnerable if $url is user-controlled.
Mitigation:
Validate and sanitize the input
Use allowlists
Block private IPs like 127.0.0.1, 169.254.169.254, etc.
📘 Learn More from Our Cybersecurity Blog
Visit the Pentest Testing Blog for in-depth guides on web application security, including Symfony, Laravel, and Node.js vulnerabilities.
🚀 New: Web App Penetration Testing Services
Want professional help?
Check out our Web Application Penetration Testing Services for in-depth manual testing, compliance audits, and security consulting by certified experts.
📣 Conclusion
SSRF is a silent killer when left unchecked. Symfony developers must avoid directly using unvalidated input for server-side HTTP requests. You can:
Use input validation
Use whitelists
Block internal IP access
Test your applications regularly
👉 Scan your site now for Website Security check with our free security tool.
Stay secure. Stay informed. Follow us at https://www.pentesttesting.com/blog/ for more tips!
#cyber security#cybersecurity#data security#pentesting#security#php#the security breach show#coding#symfony
1 note
·
View note
Text
Laravel customized portal development services
Building Scalable Custom Portals with Laravel
Laravel is one of the most popular PHP frameworks, offering a clean and elegant syntax while providing powerful tools to develop scalable, custom portals. The key features that make Laravel particularly effective in building dynamic, flexible portals for diverse business needs include Eloquent ORM, Blade templating engine, and Laravel Mix.
Eloquent ORM is a beautiful and robust implementation of the ActiveRecord pattern in Laravel, making database interaction very simple. Developers need not write complicated SQL queries to interact with the database; they can use simple PHP syntax for the same purpose, ensuring the development process is efficient and free from errors. This is very helpful in developing scalable portals, where the user base and data can be managed very smoothly as the user base grows. With one-to-many, many-to-many, and polymorphic built-in relationships, Eloquent provides a smooth solution for complex data relationships.
Blade is Laravel's templating engine that helps make dynamic and reusable views by increasing efficiency. Blade is very easy to use and has powerful features like template inheritance, conditional statements, and loops, through which people can easily build robust and user-friendly front-end interfaces for their portals. This ability to organize and reuse layouts makes the development process faster and more manageable.
Laravel Mix is a wrapper around Webpack that makes the management of assets such as CSS, JavaScript, and images easier. The developer can compile, minify, and version assets to ensure that the portal performs well and is optimized for performance and scalability. As portals grow in complexity, using Laravel Mix ensures that the front-end assets are properly compiled and organized, contributing to faster load times and a smoother user experience.
Improving Security in Laravel-Based Portals
Security is a critical aspect when developing custom portals, especially as they handle sensitive user information and business data. Laravel offers a robust suite of built-in security features to safeguard your portals against various threats.
Authentication and Authorization are essential to ensure only authorized users can access certain areas of the portal. Laravel provides an out-of-the-box authentication system, including registration, login, password reset, and email verification. You can extend and customize this system based on specific business requirements.
Laravel's authorization feature permits you to control access to different parts of the portal using gates and policies. Gates provide the "closure-based" simple approach for determining if a user may perform a certain action, whereas policies are classes that group related authorization logic.
Encryption is handled automatically in Laravel. All sensitive data, including passwords, are securely encrypted using industry-standard algorithms. Laravel’s built-in support for bcrypt and Argon2 hashing algorithms ensures that even if the database is compromised, user passwords remain safe.
Third, it ensures protection against other common vulnerabilities, which include Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), and SQL injection attacks. CSRF is enabled by default in Laravel and generates a token for each active user session that validates requests as coming from the same domain. It automatically applies XSS protection through built-in escaping mechanisms in Blade views.
Integrating Third-Party APIs in Laravel Portals
Integration of third-party APIs in custom portals can be highly beneficial for their functionality. Whether it is a payment gateway, data synchronization, or social media integration, Laravel provides an easy and efficient way to integrate with external services.
Laravel's HTTP Client, based on Guzzle, provides a simple and expressive interface to make API requests. You can send GET, POST, PUT, and DELETE requests to external services, handle responses, and manage errors. Laravel makes it seamless to interact with RESTful APIs by handling JSON responses out of the box and offering methods to parse and manipulate data efficiently.
For example, integrating a payment gateway such as Stripe or PayPal is pretty easy with the help of tools from Laravel. Through setting routes and controllers for handling API requests, you will easily enable your users to carry out smooth transactions. This means the payment process is secure and reliable.
The Jobs and Queues feature of Laravel can also be used for managing API integrations that are asynchronous in nature. This will be helpful in case of data syncing or other tasks that might take time to process, keeping the portal responsive even during complex interactions.
In business solutions such as Config Infotech, the integration of APIs for data management or collaboration tools can optimize operations and improve the overall functionality of the portal, allowing businesses to stay competitive and agile.
In a nutshell, Laravel is a powerful framework that allows developers to build scalable, secure, and highly functional custom portals. With its built-in features such as Eloquent ORM, Blade templating, and Laravel Mix, developers can create portals that are not only efficient but also maintainable as the business grows. Its focus on security, combined with its flexibility to integrate third-party APIs, makes it a top choice for building robust, enterprise-level portals.
0 notes
Text
Laravel customized portal development services
Laravel, one of the most widely-used PHP frameworks today, features an elegant syntax combined with a more powerful approach towards developing scalable and custom portals. The salient features of why Laravel has really been a particularly effective one while building dynamic yet flexible portals, catering to varying business needs are Eloquent ORM, Blade Templating Engine and Laravel Mix.
Eloquent ORM is such an elegant and powerful implementation of ActiveRecord in Laravel, making it easy to interact with databases. All complicated SQL queries are avoided by developers as they can relate to the database using simple PHP syntax that keeps the development process efficient and error-free. It is particularly helpful for constructing scalable portals because it can easily manage operations that might otherwise be complex when handling increasing user bases and data volumes. With one-to-many, many-to-many, and polymorphic relationships built in, Eloquent takes care of complex data relationships.
Blade is Laravel's templating engine that increases the efficiency of making dynamic and reusable views. It is simple to use and includes powerful features like template inheritance, conditional statements, and loops. It helps make the building of robust and user-friendly front-end interfaces for portals easier. Its ability to organize and reuse layouts makes the development process faster and more manageable.
Laravel Mix is a wrapper around Webpack that makes it easier to manage assets like CSS, JavaScript, and images. Developers can compile, minify, and version assets so that the portal will perform well and be optimized for performance and scalability. The more complex the portal, the more important it is to ensure that front-end assets are properly compiled and organized so that load times are faster and the user experience is smoother.
Improving Security in Laravel-Based Portals
Security is an important factor in developing custom portals, as they deal with sensitive user information and business data. Laravel has a robust suite of built-in security features to protect your portals from various threats.
The key to allowing only authorized users access to some sections of the portal is Authentication and Authorization. Laravel provides a very comprehensive system of authentication that is ready out of the box for registration, login, password reset, and email verification. All these things can be extended or customized as per specific business requirements.
Controls access to different parts of the portal using gates and policies. Gates can offer a simple closure-based approach to how you determine if a given user can perform a certain action, while policies are classes that group related authorization logic.
Laravel automatically handles encryption. All other sensitive data, including passwords, are encrypted using industry-standard algorithms. In Laravel, the built-in bcrypt and Argon2 hashing algorithms ensure that even in the event of a database breach, passwords for the users cannot be compromised.
It further protects against the most common attacks, including XSS, CSRF, and SQL injection attacks. CSRF protection is enabled by default in Laravel, generating a token for each session that may be active for an authenticated user. This token then validates whether requests originate from the same domain. Protection from XSS, in turn, is automatically applied in Blade views through Laravel's built-in escaping mechanisms.
Including Third-Party APIs in Laravel Portals
Integrating third-party APIs into custom portals can greatly enhance their functionality. Whether it's for payment gateways, data synchronization, or social media integrations, Laravel provides an easy and efficient way to integrate with external services.
Laravel's HTTP Client, which is built on top of Guzzle, provides a simple and expressive way to create API requests. You can send GET, POST, PUT and DELETE requests against other services, handle the response, and manage errors. Laravel provides an extremely easy mechanism to work with RESTful APIs by supporting JSON responses and giving you methods that parse and manipulate data in an efficient way.
It becomes pretty easy, for instance, to integrate the payment gateway if you are working with Stripe or PayPal using the built-in tools in Laravel. With routes and controllers set up to handle the API requests, you can give your users an easy and frictionless transaction with security and reliability.
Additionally, Laravel’s Jobs and Queues feature can be utilized to manage API integrations that require asynchronous processing. This is useful when integrating data syncing or tasks that might take time to process, ensuring the portal remains responsive even during complex interactions.
For business solutions like Config Infotech, integrating APIs for data management or collaboration tools can optimize operations and improve overall portal functionality, enabling businesses to remain competitive and agile.
Summing up, Laravel is a very powerful framework, enabling developers to build scalable, secure, and highly functional custom portals, thus helping in creating portals that are not only efficient but also maintainable as the business grows. In addition, having a focus on security, with considerable flexibility in integrating third-party APIs, it will be one of the top choices for building robust enterprise-level portals.
0 notes
Text
Top 5 Tips for Establishing Robust Security Protocols in Laravel
Introduction
Laravel, a popular PHP framework known for its elegance and simplicity, provides a robust foundation for building secure applications through Security Protocols in Laravel. In the ever-evolving world of web development, Security Protocols in Laravel stand as a critical pillar that sustains the integrity and trustworthiness of applications.

Establishing robust Security Protocols in Laravel is crucial for protecting your application from potential threats and vulnerabilities. However, ensuring optimal security takes more than simply implementing the basic features of Laravel. It requires a proactive approach to establish and maintain comprehensive Security Protocols in Laravel for maximum protection.
Laravel, being a highly popular PHP framework, offers a variety of built-in features and practices to enhance security. Here are some essential tips to help you fortify your Laravel applications.
Essential Tips

Establishing robust security protocols in Laravel is crucial for protecting your application and its users from a wide array of cyber threats. Ensuring strong security practices is essential for safeguarding sensitive data, maintaining the integrity of your system, and building trust with your users.
Businesses should ideally make the smart decision to outsource their Laravel requirements and hire Laravel developers. This is a good way to ensure they build a secure solution.
The top 5 tips for establishing robust security protocols in Laravel are:
Implement basic Laravel security
Authentication and Authorization
Laravel Security Packages
Following Best practices
Security Testing in Laravel
Here it is in more detail:
Implement basic Laravel security:
Implement basic security measures in Laravel to protect your application from common vulnerabilities. Laravel provides built-in tools and best practices for improving application security:
Environment Configuration Security: The .env file stores sensitive configuration data such as database credentials, API keys, and encryption keys. This file should never be exposed in version control (add .env to your .gitignore file). Setting APP_DEBUG=false in production prevents exposing detailed error messages that might give attackers valuable information about the system. Recommended settings for the production .env file:
Code
APP_DEBUG=false
APP_ENV=production
Use HTTPS (SSL/TLS):
Ensure your Laravel application is served over HTTPS in production to protect data in transit. Force HTTPS across the application by adding middleware. Apply this middleware globally to ensure the application always redirects HTTP traffic to HTTPS:
Code
// App\Http\Middleware\RedirectIfNotHttps.php
public function handle($request, Closure $next)
{
if (!$request->secure()) {
return redirect()->secure($request->getRequestUri());
}
return $next($request);
}
Cross-Site Request Forgery (CSRF) Protection:
Laravel has CSRF protection enabled by default for all POST, PUT, PATCH, and DELETE requests. Add the @csrf directive to your forms to protect against CSRF attacks. If you need to exempt specific routes from CSRF verification (though not recommended), you can define them in the VerifyCsrfToken middleware:
CODE
<form method="POST" action="/submit">
@csrf
<input type="text" name="name">
<button type="submit">Submit</button>
</form>
SQL Injection Protection:
Laravel’s query builder and Eloquent ORM automatically use parameter binding to protect against SQL injection. For example, avoid using raw queries:
CODE
// Instead of this (not secure):
DB::select("SELECT * FROM users WHERE email = '$email'");
//Use parameter binding (secure):
DB::select("SELECT * FROM users WHERE email = ?", [$email]);
When using Eloquent or the query builder, parameterized queries are automatically handled:
CODE
User::where('email', $email)->first();
Cross-Site Scripting (XSS) Protection:
Laravel automatically escapes output to prevent XSS attacks. Always use Blade templating to display variables in your views. Never trust user input and always sanitize or escape it before outputting it in views:
CODE
<!-- Safe output escaping -->
{{ $user->name }}
If you need to display raw HTML (be cautious), use the {!! !!} syntax:
CODE
{!! $user->bio !!}
Rate Limiting:
Laravel provides built-in support for rate-limiting API routes to prevent brute-force attacks. You can define rate limits using the throttle middleware. This example limits a user to 60 requests per minute for the /login route:
CODE
Route::middleware(['throttle:60,1'])->group(function () {
Route::post('/login', 'AuthController@login');
});
Security Headers:
Add essential security headers like Content-Security-Policy, X-Frame-Options, Strict-Transport-Security, etc., using middleware. You can use the Laravel Secure Headers package to automatically add security headers to your Laravel app.
Disable Directory Indexing: Ensure directory listing is disabled on your web server. In Apache, you can add this to your .htaccess file:
CODE
Options -Indexes
File Upload Security: Always validate and sanitize file uploads. This ensures that uploaded files are limited to specific types and size restrictions. Store uploaded files in a safe directory and avoid executing them:
CODE
$request->validate([
'file' => 'required|mimes:jpg,png,pdf|max:2048',
]);
Logging:
Ensure proper logging of security-related events, such as failed logins or unauthorized access attempts. Laravel’s logging feature is configured in config/logging.php and can help track suspicious activity.
Sanitize User Input: Use Laravel’s built-in validation to sanitize user input:
CODE
$validated = $request->validate([
'name' => 'required|string|max:255',
'email' => 'required|email',
]);
Error and Exception Handling: Configure Laravel's error handling to log errors without displaying sensitive configuration values to the end user. Implement a comprehensive logging strategy that includes file logging, email notifications, and even SMS alerts for critical issues.
Keep Laravel and Packages Up-to-Date: Install security patches when they become available. They include security patches that fix vulnerabilities discovered since the last version. The updates can also introduce new security features and improvements that enhance the overall security posture of your application. Use composer update regularly to update Laravel and its dependencies. Subscribe to Laravel and security community newsletters to stay informed about critical updates.
Secure Data Handling: Use Laravel’s powerful validation rules to ensure all incoming data is of expected form and content. Sanitize inputs to prevent SQL injection and cross-site scripting (XSS) attacks. Encrypt sensitive data in your database using Laravel’s built-in encryption facilities. Use HTTPS to encrypt data transmitted between the client and server, configuring SSL/TLS on your web server.
Authentication and Authorization:
It uses built-in authentication and authorization features to ensure that only authorized users can access certain parts of your application. It also introduces enhanced security features, building on its already strong foundation to ensure developers can build highly secure applications.
Authentication: Use Laravel's Auth system to handle user authentication. Utilize Laravel's built-in features, such as Laravel Breeze, Jetstream, or Fortify, to manage authentication securely. Implement two-factor authentication (2FA) to add an extra layer of security.
Authorization: You can use gates and policies for role-based or resource-based authorization. They handle the authorization logic. It requires the user roles and permissions to be clearly defined and enforced at both server-side and client-side levels.
Built-In Encryption: Laravel 11 offers powerful encryption using the AES-256 and AES-128 algorithms. This ensures that sensitive data like passwords, tokens, and user information are securely encrypted both in storage and during transmission.
Automatic Password Hashing: Laravel 11 continues to provide strong password hashing using the bcrypt and argon2 algorithms. This prevents passwords from being stored in plain text and offers protection against brute-force attacks.
Two-Factor Authentication (2FA): With the growing need for enhanced security, Laravel 11 supports two-factor authentication (2FA) for user logins, adding an extra layer of security beyond the standard username and password.
Laravel Security Packages:
Laravel packages offer various features, including enhanced security. Regular updates provide security patches, new features, and improvements. Run composer update regularly to update Laravel and its dependencies, keeping security packages up to date. Below are top security packages for Laravel:
Laravel Security: Enhances password hashing, key derivation, encryption, and signature features.
Security Headers: Adds security-related HTTP headers to protect against attacks such as XSS.
Laravel ACL (Access Control List): Simplifies the implementation of role-based access control (RBAC) and permissions in your Laravel app.
Laravel Passport: Provides OAuth2 server implementation for API authentication.
Laravel Sanctum: Provides a lightweight solution for token-based API authentication and SPA (Single Page Application) authentication.
Laravel Shield: A security package that provides rate-limiting features, IP whitelisting, and more to protect Laravel applications.
Laravel Honeypot: Adds a honeypot field to your forms to detect and block bots and spam submissions.
Laravel Firewall: Provides a firewall layer for filtering requests based on IP address, allowing you to block malicious traffic.
Laravel CipherSweet: Provides searchable encryption for sensitive data in Laravel applications.
Laravel Recaptcha: Integrates Google's reCAPTCHA into your Laravel forms to prevent spam and bots.
Security Checker: Scans your composer dependencies for known vulnerabilities and provides warnings if any are found.
Spatie Laravel Permissions: Allows easy handling of user roles and permissions within Laravel applications.
Laravel Trusted Proxy: Manages trusted proxies in Laravel, ensuring correct handling of requests behind proxies or load balancers.
Laravel Audit: Tracks user activities and stores an audit log of changes made to your models, adding transparency and accountability.
Laravel Security Component: This package is useful in securing roles and objects. It does so by integrating the Symfony security core into Laravel. It helps verify the role-based privileges, enhancing security validations using voters.
Best practices:
It is vital to keep in mind that security is an ongoing process. Follow these security best practices to enhance the security of your Laravel applications:
Limit access to sensitive data: Limit access to sensitive data, such as credit card numbers or passwords, to only those who need it. Use Laravel's built-in encryption features to protect sensitive data.
Use a Web Application Firewall (WAF): Use a WAF, such as Laravel's built-in WAF or a third-party WAF, to protect your application from common web attacks.
Implement a secure development lifecycle: Implement a secure development lifecycle to ensure security is integrated into every stage of the development process. Use security testing and code reviews to identify security issues early in the development process.
Prevent SQL injection by avoiding raw queries: It is not uncommon for developers to use raw queries, which tends to leave the code vulnerable to attacks. The main reason Laravel has provided a framework for this purpose is because it uses PDO binding to prevent SQL injection attacks. It does not allow variables to be passed to the database without validation. Raw query issues are prevented by the experts with high-level coding. However, it is not advisable to use raw queries.
Monitor for Vulnerabilities: New security vulnerabilities are constantly being discovered. Regularly scan your dependencies using tools like Laravel Security Checker or Snyk to detect vulnerabilities:
CODE
composer requires enlighten/security-checker --dev
php artisan security:check
Security Testing in Laravel:
Automated security testing in Laravel is an essential practice for ensuring that your web application is secure from vulnerabilities throughout the development lifecycle. Laravel, being a PHP framework, has its own security features, but combining these with automated testing tools ensures that security is continuously enforced as the application evolves. Here’s how automated security testing can be implemente in Laravel:
Integration of Static Application Security Testing (SAST)
Tools: Tools like SonarQube, PHPStan, Psalm, or RIPS can perform static analysis on your Laravel codebase. These tools help catch vulnerabilities like SQL injection, cross-site scripting (XSS), insecure data storage, and more.
Automation: Integrate these tools into your CI/CD pipeline to automatically scan code after every commit. This ensures developers are notified of potential security issues early in the development process.
Dynamic Application Security Testing (DAST)
Tools: Tools like OWASP ZAP or Burp Suite are used to run automated tests on running Laravel applications. They look for vulnerabilities by interacting with the app’s endpoints and attempting common attacks (e.g., XSS, SQL injection).
Automation: These tools can be configured to run during automated testing in the CI/CD pipeline. They test the application from an attacker’s perspective, catching vulnerabilities in real-world scenarios.
Dependency Scanning
Tools: Laravel applications often use third-party packages through Composer. Tools like Snyk, Dependenbot, or PHP Security Advisories scan your Composer dependencies for known security vulnerabilities.
Automation: Use these tools to automatically scan and notify the team of insecure or outdated packages. This can be integrate into the pipeline to prevent deploying vulnerable libraries.
Unit and Integration Testing for Security
Laravel’s testing capabilities (using PHPUnit) allow you to write unit tests and integration tests that can focus on security-critical parts of the application:
SQL Injection: Test database queries for SQL injection vulnerabilities by ensuring proper Use of Laravel's query builder and Eloquent ORM.
Cross-Site Request Forgery (CSRF): Laravel includes CSRF protection by default, but testing can ensure that these protections work correctly across your application.
Authentication and Authorization: Test your authentication mechanisms (login, registration) and authorization policies to ensure they work securely.
Input Validation: Ensure input validation rules are apply correctly to prevent attacks like file uploads or malicious input.
Custom Security Middleware Testing: Laravel allows you to create custom middleware to handle security concerns (e.g., rate-limiting, IP blocking, additional encryption). Automated tests can validate that the middleware works as intended and provides the desired protection.
Test Examples:
Ensure API endpoints are correctly rate-limited.
Validate that middleware for enforcing HTTPS or input sanitation is apply correctly. Continuous Integration/Continuous Deployment (CI/CD) Pipeline
Integrate security testing into your CI/CD pipeline to automatically test for vulnerabilities in every commit, build, or deployment.
Tools: Jenkins, GitHub Actions, GitLab CI, and CircleCI can be use to orchestrate security tests like SAST, DAST, and dependency scanning as part of your deployment process. You can configure automated alerts to flag security issues early in development.
Automated Security Testing for APIs
API Vulnerabilities: APIs are often vulnerable to attacks like rate-limiting bypass, broken authentication, and sensitive data exposure. Automated API security testing tools such as Postman or Insomnia combine with security test suites can be use to test API endpoints.
Laravel Passport/Sanctum: If you're using Laravel Passport or Sanctum for API authentication, ensure you write tests that validate proper Use of OAuth tokens or personal access tokens.
Configuration and Environment Security Testing:
Laravel’s configuration files (.env, config/) are critical for securing the application. Automated tests can ensure:
Sensitive Data: No sensitive data like API keys or passwords are hard-coded or exposed.
Debugging Mode: The application does not have debugging mode enabled in production environments (APP_DEBUG=false).
Encryption: Proper encryption configurations are applied (e.g., for user passwords, sessions, and sensitive data).
Database Security Testing
Testing Queries: Validate that all database queries are properly parameterized to prevent SQL injection.
Data Encryption: Ensure that sensitive data is encrypted at rest (e.g., user passwords with bcrypt or argon2 hashing algorithms).
Test for Common Laravel Security Risks:
Test the application for specific security best practices:
CSRF Protection: Laravel’s default CSRF protection must be tested, especially in form submissions.
SQL Injection: Use automated testing to ensure Eloquent and Query Builder are used safely without risking SQL injections.
Session Hijacking: Automated tests can check whether secure sessions are implemented using HTTPS and cookies are flagged with the Secure and HttpOnly attributes.
Security headers validation: Whether the correct HTTP security headers (e.g., Content-Security-Policy, X-Content-Type-Options, Strict-Transport-Security) are implemente can be valid by automated security tools. Tools like securityheaders.io or Laravel Security Headers package can help enforce these headers.
Laravel Development Services From Acquaint Softtech

Business can benefit from turning to the professionals when it comes to developing a secure application. They have the expertise and experience to develop cutting-edge solutions.
Laravel Partners are firms with specialised skill and resources to develop next-generation solutions. They also have knowledge on how to develop secure solutions. Opting to hire such a Laravel development company can help businesses gain the upper edge.
Looking for secure Laravel development? Acquaint Softtech has you covered! The expert team at Acquaint Softtech will ensure your Laravel application is protecte with top-notch security protocols to guard against threats and vulnerabilities. From data encryption to secure authentication, we take every step to keep your app safe.
Acquaint Softtech is a Laravel partner, one of the few in Asia. We are a well-established software development outsourcing company in India with over 10 years of experience. In fact, we have already delivered over 5000 projects successfully worldwide.
Hire remote developers from here for highly secure applications. Our team of dedicated Laravel developers have both the experience and skill to build flawless solutions. Besides which we also have a dedicated QA team to build a state-of-the-art solution.
Conclusion
Security in Laravel, including Security Protocols in Laravel, is not just a feature but a continuous practice that should be integrate into every stage of application development and maintenance. By adhering to these tips and continually updating your knowledge and practices in response to emerging threats, you can significantly enhance the security of your Laravel applications.
Remember, effective security is about layers; implementing multiple security measures and Security Protocols in Laravel enhances the overall security of your application, making it harder for attackers to breach.
0 notes
Text
Why Choose a Laravel Development Company for Your PHP Website Development Needs
In the dynamic world of web development, selecting the right framework and the right development partner is crucial to the success of your project. As businesses increasingly turn to digital solutions to drive growth and efficiency, the demand for robust and scalable web applications has never been higher. This is where a Laravel development company can make a significant impact, especially when you are looking to leverage the power of PHP.
What Makes Laravel Stand Out in PHP Website Development?
Laravel has emerged as one of the most popular PHP frameworks for web development. Its elegance, simplicity, and functionality set it apart from other PHP frameworks. Here are some reasons why Laravel is preferred by many PHP development companies:
MVC Architecture: Laravel's Model-View-Controller (MVC) architecture ensures a separation of concerns, which enhances the application's scalability and maintainability.
Elegant Syntax: Laravel provides an expressive, elegant syntax which makes web development enjoyable for developers. This results in clean, readable code which is easier to maintain and extend.
Built-in Tools: Laravel comes with a host of built-in tools such as Artisan (a command-line tool), Eloquent ORM (Object-Relational Mapping), and Blade (a powerful templating engine). These tools streamline the development process and enhance productivity.
Security: Laravel includes robust security features out-of-the-box, including protection against common vulnerabilities like SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
Community and Support: Laravel has a large, active community which means ample resources, tutorials, and third-party packages are available to extend its functionality.
Why Partner with a Laravel Development Company?
When you decide to build a PHP-based website, choosing a Laravel development company can provide numerous advantages:
Expertise: Laravel development companies specialize in this framework and bring a wealth of experience and knowledge to your project. They can provide insights and best practices that individual developers might overlook.
Customized Solutions: A professional Laravel development company can tailor the framework to meet your specific business needs, ensuring that your website or application is not only functional but also optimized for performance and user experience.
Efficient Project Management: These companies typically have established processes and project management methodologies in place, ensuring that your project is delivered on time and within budget.
Ongoing Support and Maintenance: Post-launch support is critical for any web application. A reliable development company will offer ongoing maintenance and support services to keep your application running smoothly and securely.
The Role of a PHP Development Company
While Laravel is a standout framework, it’s essential to recognize the broader capabilities of a PHP development company. These companies offer comprehensive services that go beyond just one framework. Here’s how a PHP development company can support your web development needs:
Versatility: PHP development companies are proficient in various PHP frameworks including Laravel, Symfony, CodeIgniter, and more. This allows them to recommend and use the best framework suited to your project requirements.
Full-Stack Development: These companies often provide full-stack development services, covering both front-end and back-end development, ensuring a cohesive and fully integrated application.
Custom PHP Solutions: Whether you need a custom content management system, an e-commerce platform, or a complex web application, PHP development companies have the expertise to deliver tailored solutions.
Integration Services: PHP development companies can integrate your web application with other systems and APIs, enhancing functionality and ensuring seamless operations across your business processes.
Conclusion
Choosing the right development partner is as crucial as choosing the right technology. A Laravel development company brings specialized expertise in one of the best PHP frameworks, ensuring your web application is built with efficiency, security, and scalability in mind. Meanwhile, a PHP development company offers a broad spectrum of services, providing flexibility and versatility to meet diverse development needs.
Investing in professional development services can significantly elevate your project, providing you with a robust, reliable, and high-performing web application that drives business success. Whether you're looking to build a new website or upgrade an existing one, leveraging the expertise of experienced developers is a strategic move that pays off in the long run.
0 notes
Text
Securing Your Laravel App: Latest Best Practices and Threat Protection
In the ever-evolving world of web development, Laravel has emerged as one of the most popular PHP frameworks. Its elegant syntax, robust features, and vibrant community make it a top choice for many developers. However, as with any technology, ensuring the security of your Laravel application is paramount. In this blog post, we will delve into the latest best practices for securing your Laravel app and protecting it against threats.
Why Security Matters in Laravel Development
Before we dive into the specifics, let’s take a moment to understand why security is so crucial in Laravel development. As the digital landscape expands, so does the potential for security breaches. These breaches can lead to data loss, unauthorized access, and even severe financial implications. Therefore, whether you’re a seasoned Laravel developer or a business looking to hire a Laravel developer, understanding and implementing security best practices is non-negotiable.
Laravel Security Best Practices
1. Keep Your Laravel Version Updated
One of the simplest yet most effective ways to secure your Laravel app is by keeping your Laravel version updated. Each new version of Laravel comes with security patches and updates that fix known vulnerabilities.
2. Use HTTPS
Using HTTPS instead of HTTP ensures that all communication between your server and your users is encrypted. This encryption makes it much harder for attackers to access sensitive information.
3. Validate and Sanitize Data
Always validate and sanitize data coming from users or third-party sources. Laravel provides several ways to validate data, including form request validation, manual validation, and even validation rules.
4. Use Laravel’s Built-In Authentication
Laravel comes with built-in authentication scaffolding, which provides a secure way to handle user authentication. Using this feature can save you from the potential security risks of building your own authentication system.
5. Limit and Monitor Access
Implement role-based access control (RBAC) to limit who can access what resources in your application. Also, monitor your application for any unusual activity.
Threat Protection in Laravel
Protecting your Laravel app from threats involves being aware of the common types of attacks and knowing how to prevent them. Here are a few threats you should be aware of:
1. SQL Injection
SQL injection involves an attacker manipulating your SQL queries to gain unauthorized access or make unauthorized changes to your database. Laravel’s Eloquent ORM uses PDO parameter binding, which protects your application from SQL injection.
2. Cross-Site Scripting (XSS)
XSS attacks involve an attacker injecting malicious scripts into your web pages, which are then run by your users’ browsers. Laravel’s Blade templating engine automatically escapes output, protecting your application from XSS attacks.
3. Cross-Site Request Forgery (CSRF)
CSRF attacks trick your users into performing actions without their knowledge or consent. Laravel includes built-in protection against CSRF attacks.
Conclusion
Securing your Laravel application involves a combination of staying updated with the latest Laravel version, using built-in Laravel features, and being aware of common threats. Whether you’re a Laravel developer or a Laravel development company, following these best practices will go a long way in ensuring the security of your Laravel application.
Remember, security is not a one-time task but an ongoing process. Stay vigilant, stay updated, and most importantly, stay secure.
FAQs on Securing Your Laravel App: Latest Best Practices and Threat Protection**
Q: Why is securing my Laravel app important?
- A: Securing your Laravel app is crucial to protect sensitive data, prevent unauthorized access, and safeguard against potential cyber threats. It ensures the integrity and reliability of your application.
Q: What are the latest best practices for securing a Laravel app?
- A: Some best practices include keeping Laravel and its dependencies up-to-date, implementing strong authentication mechanisms, securing sensitive routes, using HTTPS, and regularly auditing and monitoring your application for potential vulnerabilities.
Q: How can I keep my Laravel framework and dependencies updated?
- A: Use Composer to manage your project dependencies and regularly update Laravel by running `composer update`. Stay informed about security releases and apply them promptly.
Q: What authentication mechanisms should I use to secure my Laravel app?
- A: Laravel provides built-in authentication features. Implement multi-factor authentication (MFA), use strong password policies, and consider OAuth or social media logins for additional security layers.
Q: How do I secure sensitive data in my Laravel app?
- A: Employ encryption for sensitive data using Laravel's encryption features. Avoid storing sensitive information in plain text and utilize secure methods like Laravel's Eloquent ORM for database operations.
Q: Is HTTPS necessary for my Laravel app?
- A: Yes, using HTTPS is crucial. It encrypts data in transit, preventing eavesdropping and man-in-the-middle attacks. Obtain an SSL certificate and configure your Laravel app to use HTTPS.
Q: How can I protect against common web application security threats?
- A: Implement input validation, use Laravel's built-in protection against Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF), and sanitize user inputs to prevent SQL injection.
Q: Should I use Laravel's built-in security features or implement additional security packages?
- A: Laravel offers robust security features, but depending on your specific requirements, you may consider additional security packages like Laravel Security Package or Laravel Fortify for enhanced protection.
Q: How can I monitor my Laravel app for security vulnerabilities?
- A: Regularly conduct security audits, use security scanning tools, and implement logging and monitoring solutions to detect and respond to any suspicious activities or potential threats.
Q: What steps can I take to recover my Laravel app in case of a security breach?
- A: Have a well-defined incident response plan, regularly back up your data, and ensure you can quickly restore your application to a secure state. Additionally, notify users of any security incidents and prompt them to update passwords if necessary.
0 notes
Text
Elevating Web Development with a Leading Laravel Development Company in the USA
In the dynamic landscape of Laravel website development, choosing the right framework is paramount to ensuring the success and efficiency of your projects. One such powerhouse in the realm of PHP frameworks is Laravel. Renowned for its elegant syntax, developer-friendly tools, and robust features, Laravel has become the go-to choice for web developers worldwide.
Understanding Laravel's Allure
In this blog post, we will explore the merits of Laravel and shine a spotlight on a top-tier Laravel Development Company in the USA that is revolutionizing web development.
1. Elegant Syntax and Readability:
Laravel stands out with its clean and expressive syntax, making code not only functional but also a joy to read. This readability is crucial for collaborative projects, ensuring that developers can easily understand and contribute to the codebase.
2. Blade Templating Engine:
Laravel incorporates the Blade templating engine, offering a simple yet powerful templating system. Blade templates facilitate the creation of dynamic content, enabling developers to seamlessly integrate logic into their views.
3. Artisan Console:
Laravel comes equipped with Artisan, a powerful command-line tool that automates various tasks, from database migrations to generating boilerplate code. This feature enhances developer productivity by eliminating repetitive manual tasks.
4. Eloquent ORM:
Eloquent, Laravel's Object-Relational Mapping (ORM) system, simplifies database interactions. With an expressive syntax, developers can interact with databases using PHP rather than SQL, streamlining the data manipulation process.
5. Middleware for HTTP Requests:
Laravel's middleware enables developers to filter HTTP requests entering their application. This functionality allows for the creation of custom middleware, enhancing security and controlling access to certain features.
6. Robust Authentication and Authorization:
Laravel provides a comprehensive authentication system out of the box. This system simplifies the implementation of secure user authentication, including features like user registration, password reset, and role-based access control.
Laravel Development Company in the USA: Pioneering Excellence
In the competitive landscape of web development services, one company stands out as a trailblazer in Laravel website development – Iwebnext. With a solid reputation for delivering cutting-edge solutions, Iwebnext has become a trusted partner for businesses seeking top-notch web applications.
1. Expertise and Experience:
Iwebnext boasts a team of highly skilled Laravel developers with years of hands-on experience. Their expertise extends across a spectrum of industries, allowing them to tailor solutions that meet the unique needs of clients.
2. Customized Solutions:
Understanding that one size does not fit all, Iwebnext prides itself on delivering bespoke Laravel solutions. Whether it's e-commerce platforms, content management systems, or enterprise-level applications, they have the proficiency to craft tailored solutions that align with client objectives.
3. Agile Development Approach:
Embracing an agile development methodology, Iwebnext ensures flexibility and adaptability throughout the project lifecycle. This approach facilitates regular client feedback, quick iterations, and the ability to respond swiftly to changing requirements.
4. Scalability and Performance Optimization:
Iwebnext recognizes the importance of scalability in today's rapidly evolving digital landscape. Their Laravel solutions are not only feature-rich but also optimized for performance, ensuring a seamless user experience even as the application scales.
5. Transparent Communication and Project Management:
Open and transparent communication is at the core of Iwebnext's values. Clients are kept informed at every stage of the development process, and the use of robust project management tools ensures timely deliveries without compromising quality.
Conclusion: Elevate Your Web Development with Laravel
In conclusion, Laravel continues to redefine the standards of PHP web development, offering a perfect blend of elegance, functionality, and efficiency. Providing unparalleled services with Laravel development company in USA, Iwebnext stands as a beacon of excellence, ready to transform ideas into reality. Harness the power of Laravel and partner with a company that not only understands the framework inside out but is also dedicated to delivering exceptional results. Your journey to web development excellence begins with the right framework and the right partners – choose Laravel, choose Iwebnext.
#Laravel Website Development#Laravel Development#Website Development Company#Best Website Development Company
0 notes
Text
Unleashing the Power of Laravel: 5 Advantages of Web Development
Introduction:
In the dynamic world of web development, choosing the right framework is crucial for building robust and scalable applications. Laravel, a PHP web application framework, has gained immense popularity for its elegant syntax, expressive features, and developer-friendly environment. In this blog post, we'll explore five key advantages of web development with Laravel.
Laravel comes equipped with Eloquent ORM, a powerful and intuitive database manipulation tool. Eloquent allows developers to interact with databases using object-oriented syntax, making it easier to manage and query database records. This elegant ORM simplifies complex database operations and reduces the amount of boilerplate code typically associated with database interactions. With its expressive syntax, developers can focus on building features rather than getting bogged down by database intricacies.
Laravel's Artisan Console is a command-line interface that simplifies various development tasks. From generating boilerplate code to managing database migrations and running unit tests, Artisan automates many routine processes, enhancing developer productivity. With simple commands, developers can create controllers, models, and migrations, speeding up the development process and ensuring a standardized codebase. This feature promotes consistency and helps developers adhere to best practices throughout the project.
Laravel's Blade templating engine provides a powerful yet simple way to create dynamic, reusable, and efficient views. With Blade, developers can write templates using straightforward syntax, including control structures and template inheritance. This templating engine promotes code organization and reusability, allowing developers to build complex layouts without sacrificing readability. Blade also supports the inclusion of plain PHP code when needed, providing flexibility for developers who may be familiar with PHP.
Middleware plays a crucial role in handling HTTP requests and responses in web applications. Laravel offers a robust middleware system that allows developers to filter HTTP requests entering the application. This enables developers to add custom logic before or after a request is handled, enhancing security, authentication, and request handling processes. With middleware, developers can implement features like authentication checks, logging, and CORS (Cross-Origin Resource Sharing) handling, ensuring a secure and seamless user experience.
Laravel benefits from a vibrant and active community of developers who contribute to its growth and evolution. The Laravel ecosystem includes a wide range of packages and extensions that can be easily integrated into projects. From authentication packages to third-party APIs, Laravel's ecosystem simplifies complex tasks, reducing development time and effort. The extensive documentation and community support ensure that developers can find solutions to common issues and stay updated with the latest best practices.
Conclusion:
In conclusion, Laravel stands out as a powerful and versatile framework for web development, offering a range of features that streamline the development process. From the expressive Eloquent ORM to the convenient Artisan Console and the flexible Blade templating engine, Laravel provides a developer-friendly environment for building robust and scalable web applications. Additionally, the middleware system and the supportive community contribute to the framework's success, making it a top choice for developers seeking efficiency and elegance in their web development company projects.
0 notes
Text
XML Injection in Laravel: Prevention & Secure Coding 🚀
Introduction
XML Injection in Laravel is a critical web security flaw that occurs when attackers manipulate XML input to exploit applications. This vulnerability can lead to data exposure, denial-of-service (DoS) attacks, and even remote code execution in severe cases.

In this post, we will explore what XML Injection is, how it affects Laravel applications, and most importantly, how to prevent it using secure coding practices. We will also show how our Website Vulnerability Scanner can detect vulnerabilities like XML Injection.
What is XML Injection?
XML Injection happens when an application improperly processes XML input, allowing attackers to inject malicious XML data. This can lead to:
Data theft – Attackers can access unauthorized data.
DoS attacks – Malicious XML can crash the application.
Code execution – If poorly configured, it can lead to executing arbitrary commands.
🔍 Example of an XML Injection Attack
Let's consider a Laravel-based ERP system that takes XML input from users:
<?xml version="1.0" encoding="UTF-8"?> <user> <name>John</name> <password>12345</password> </user>
An attacker can inject malicious data to extract sensitive information:
<?xml version="1.0" encoding="UTF-8"?> <user> <name>John</name> <password>12345</password> <role>&exfiltrate;</role> </user>
If the application does not sanitize the input, it may process this malicious XML and expose sensitive data.
How XML Injection Works in Laravel
Laravel applications often use XML parsing functions, and if improperly configured, they may be susceptible to XML Injection.
Consider the following Laravel controller that parses XML input:
use Illuminate\Http\Request; use SimpleXMLElement; class UserController extends Controller { public function store(Request $request) { $xmlData = $request->getContent(); $xml = new SimpleXMLElement($xmlData); $name = $xml->name; $password = $xml->password; return response()->json(['message' => "User $name created"]); } }
🚨 The Problem
The SimpleXMLElement class does not prevent external entity attacks (XXE).
Malicious users can inject XML entities to read sensitive files like /etc/passwd.
How to Prevent XML Injection in Laravel
✅ 1. Disable External Entity Processing (XXE)
Modify XML parsing with libxml_disable_entity_loader() to prevent external entity attacks:
use Illuminate\Http\Request; use SimpleXMLElement; class SecureUserController extends Controller { public function store(Request $request) { $xmlData = $request->getContent(); // Secure XML parsing $xml = new SimpleXMLElement($xmlData, LIBXML_NOENT | LIBXML_DTDLOAD); $name = $xml->name; $password = $xml->password; return response()->json(['message' => "User $name created securely"]); } }
✅ 2. Use JSON Instead of XML
If possible, avoid XML altogether and use JSON, which is less prone to injection attacks:
use Illuminate\Http\Request; class SecureUserController extends Controller { public function store(Request $request) { $validatedData = $request->validate([ 'name' => 'required|string', 'password' => 'required|string|min:6' ]); return response()->json(['message' => "User {$validatedData['name']} created securely"]); } }
✅ 3. Implement Laravel’s Built-in Validation
Always validate and sanitize user inputs using Laravel's built-in validation methods:
$request->validate([ 'xmlData' => 'required|string|max:5000' ]);
Check Your Laravel Website for XML Injection
🚀 You can test your Laravel application for vulnerabilities like XML Injection using our Free Website Security Scanner.
📸 Screenshot of Free Tool Webpage

Screenshot of the free tools webpage where you can access security assessment tools.
How It Works: 1️⃣ Enter your website URL. 2️⃣ Click "Start Test". 3️⃣ Get a full vulnerability report in seconds!
📸 Example of a Security Report to check Website Vulnerability

An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities.
Final Thoughts
XML Injection in Laravel can lead to data breaches and security exploits if not handled properly. Following secure coding practices such as disabling external entities, using JSON, and validating input data can effectively prevent XML Injection attacks.
🔗 Check out more security-related articles on our blog: Pentest Testing Blog
💡 Have you checked your website for vulnerabilities? Run a free security scan now at Website Security Checker.
🔥 Stay secure, keep coding safe! 🔥
1 note
·
View note
Text
Laravel customized portal development services
Building Scalable Custom Portals with Laravel
Laravel is one of the most popular PHP frameworks, offering a clean and elegant syntax while providing powerful tools to develop scalable, custom portals. The key features that make Laravel particularly effective in building dynamic, flexible portals for diverse business needs include Eloquent ORM, Blade templating engine, and Laravel Mix.
Eloquent ORM is a beautiful and robust implementation of the ActiveRecord pattern in Laravel, making database interaction very simple. Developers need not write complicated SQL queries to interact with the database; they can use simple PHP syntax for the same purpose, ensuring the development process is efficient and free from errors. This is very helpful in developing scalable portals, where the user base and data can be managed very smoothly as the user base grows. With one-to-many, many-to-many, and polymorphic built-in relationships, Eloquent provides a smooth solution for complex data relationships.
Blade is Laravel's templating engine that helps make dynamic and reusable views by increasing efficiency. Blade is very easy to use and has powerful features like template inheritance, conditional statements, and loops, through which people can easily build robust and user-friendly front-end interfaces for their portals. This ability to organize and reuse layouts makes the development process faster and more manageable.
Laravel Mix is a wrapper around Webpack that makes the management of assets such as CSS, JavaScript, and images easier. The developer can compile, minify, and version assets to ensure that the portal performs well and is optimized for performance and scalability. As portals grow in complexity, using Laravel Mix ensures that the front-end assets are properly compiled and organized, contributing to faster load times and a smoother user experience.
Improving Security in Laravel-Based Portals
Security is a critical aspect when developing custom portals, especially as they handle sensitive user information and business data. Laravel offers a robust suite of built-in security features to safeguard your portals against various threats.
Authentication and Authorization are essential to ensure only authorized users can access certain areas of the portal. Laravel provides an out-of-the-box authentication system, including registration, login, password reset, and email verification. You can extend and customize this system based on specific business requirements.
Laravel's authorization feature permits you to control access to different parts of the portal using gates and policies. Gates provide the "closure-based" simple approach for determining if a user may perform a certain action, whereas policies are classes that group related authorization logic.
Encryption is handled automatically in Laravel. All sensitive data, including passwords, are securely encrypted using industry-standard algorithms. Laravel’s built-in support for bcrypt and Argon2 hashing algorithms ensures that even if the database is compromised, user passwords remain safe.
Third, it ensures protection against other common vulnerabilities, which include Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), and SQL injection attacks. CSRF is enabled by default in Laravel and generates a token for each active user session that validates requests as coming from the same domain. It automatically applies XSS protection through built-in escaping mechanisms in Blade views.
Integrating Third-Party APIs in Laravel Portals
Integration of third-party APIs in custom portals can be highly beneficial for their functionality. Whether it is a payment gateway, data synchronization, or social media integration, Laravel provides an easy and efficient way to integrate with external services.
Laravel's HTTP Client, based on Guzzle, provides a simple and expressive interface to make API requests. You can send GET, POST, PUT, and DELETE requests to external services, handle responses, and manage errors. Laravel makes it seamless to interact with RESTful APIs by handling JSON responses out of the box and offering methods to parse and manipulate data efficiently.
For example, integrating a payment gateway such as Stripe or PayPal is pretty easy with the help of tools from Laravel. Through setting routes and controllers for handling API requests, you will easily enable your users to carry out smooth transactions. This means the payment process is secure and reliable.
The Jobs and Queues feature of Laravel can also be used for managing API integrations that are asynchronous in nature. This will be helpful in case of data syncing or other tasks that might take time to process, keeping the portal responsive even during complex interactions.
In business solutions such as Config Infotech, the integration of APIs for data management or collaboration tools can optimize operations and improve the overall functionality of the portal, allowing businesses to stay competitive and agile.
In a nutshell, Laravel is a powerful framework that allows developers to build scalable, secure, and highly functional custom portals. With its built-in features such as Eloquent ORM, Blade templating, and Laravel Mix, developers can create portals that are not only efficient but also maintainable as the business grows. Its focus on security, combined with its flexibility to integrate third-party APIs, makes it a top choice for building robust, enterprise-level portals.
0 notes
Text
Fundamentals of Laravel Application Architecture
In the world of web development, creating robust and scalable applications requires a well-thought-out architecture. Laravel, a popular PHP framework, offers developers a solid foundation for building applications that are not only efficient but also maintainable over time. In this blog post, we'll delve into the fundamentals of Laravel application architecture and explore how it contributes to the success of your projects.
1. Model-View-Controller (MVC) Architecture:
Laravel follows the Model-View-Controller (MVC) architectural pattern, which separates the application into three key components: Models, Views, and Controllers. This separation enhances code organization, promotes reusability, and makes maintenance a breeze. Models handle data logic, Views manage presentation, and Controllers handle user interaction and orchestration of data flow.
2. Routing:
Routing plays a pivotal role in Laravel's architecture. Routes define how incoming requests should be handled. Laravel's expressive routing system allows you to define routes in a clear and structured manner, enhancing the readability and maintainability of your code.
3. Middleware:
Middleware in Laravel provides a way to filter HTTP requests entering your application. This powerful feature allows you to perform tasks like authentication, logging, and more before the request reaches your application's routes. Middleware helps keep your codebase clean by separating cross-cutting concerns from your core application logic.
4. Eloquent ORM:
Laravel's Eloquent ORM (Object-Relational Mapping) simplifies database interaction by allowing you to work with databases using intuitive, object-oriented syntax. Eloquent models represent database tables and enable you to perform operations like querying, inserting, updating, and deleting records in a convenient and elegant manner.
5. Service Providers:
Service providers are a key part of Laravel's architecture. They help register and boot various components of the application, such as providers for database connections, authentication, and more. Service providers contribute to the modularity and flexibility of your application, making it easier to swap out components or add new ones as needed.
6. Dependency Injection:
Laravel encourages the use of dependency injection to manage the flow of dependencies within your application. This promotes loose coupling between components, making your codebase more maintainable and testable. Dependency injection is especially beneficial when writing unit tests, as it allows you to easily mock and test components in isolation.
7. Blade Templating Engine:
Blade is Laravel's templating engine, providing a simple and expressive way to create dynamic views. Blade templates allow you to embed PHP code within your HTML while maintaining clean and readable syntax. This separation of concerns between code and presentation contributes to the maintainability of your application's frontend.
8. Artisan CLI:
Laravel's Artisan command-line interface simplifies various development tasks, from generating boilerplate code to performing database migrations and running tests. Artisan commands contribute to a streamlined development workflow, enhancing productivity and reducing the potential for human error.
9. Queues and Jobs:
Laravel's built-in support for queues and jobs enables you to offload time-consuming tasks to background workers, enhancing the responsiveness of your application. This architecture helps maintain a smooth user experience by preventing long-running tasks from blocking the main application thread.
In conclusion, the fundamentals of Laravel application architecture revolve around the principles of separation of concerns, modularity, and maintainability. By following the Model-View-Controller pattern, leveraging powerful features like Eloquent ORM and middleware, and adhering to best practices such as dependency injection, Laravel empowers developers to create sophisticated and scalable web applications. Whether you're building a small project or a complex enterprise application, understanding and applying these architectural concepts can greatly contribute to the success of your Laravel endeavors.
0 notes
Text
Laravel Vs. Codeigniter: A Head-to-Head Comparison?
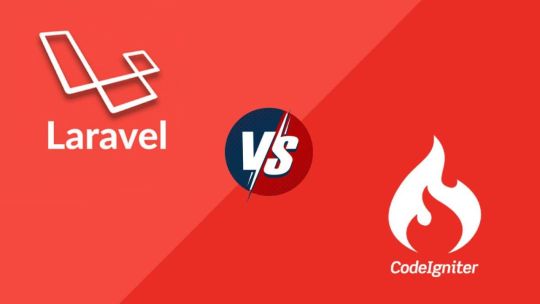
PHP framework's undisputed sovereignty in the digital landscape is known to all and sundry. That pretty much explains why it is the most sought-after framework for the development of cutting-edge and enterprise-grade web applications.
Laravel and Codeigniter are two such PHP frameworks that are widely used by developers across the globe. And if you are looking to build a web application and wondering which framework to go for, this post is for you.
We have compared the two frameworks on various factors including performance, community support, usage statistics, ease-of-use, database support, and scalability to name a few.
Let's dive right in!
Laravel Framework at a Glance
Written in PHP and based on Symfony, Laravel is an open-source framework that is simply outstanding. Being a server-side framework Laravel can be counted on for building robust web applications with a completely customized back-end and pre-defined architecture.
You can also develop web applications with full-stack apps and expect flawless server-side handling of routing, templating, and HTML authentication to name a few.
How popular is Laravel?
Laravel's market share stands at 0.37% which is significantly good. It has got more than 63 thousand Github stars with approximately 21 thousand live projects. Laravel became popular for web development as it supports MVC (model view controller) patterns. It comes with a built-in module and contains eloquent ORM.
No wonder, it is preferred by the biggest of brands. To give you an idea, below are listed some of the most renowned Laravel-based web applications.
Asgard CMS
Laracasts
Barchart
World Walking
Laravel is considered ideal for:
Web management systems involving stock trading
Multi-language support CMS?
E-learning web apps
Web applications based on SaaS
Web apps with rewards and recognition features
On-demand streaming web apps
Why Laravel framework for web app development?
Let?s take a look at some of the major features of the Laravel framework.
Excellent templating system
Laravel uses Blade- a templating engine possessing immeasurable power to format complicated layouts and data with easy navigation. Besides, developers can add new modules and/or features without having to make any modifications to the core part.
Routing
Another noteworthy feature of Laravel is reverse routing. This feature facilitates the automatic creation of URIs that stands for Unique Resource Identifiers.
Automated testing
Testing becomes a less time-consuming task with Laravel as it has expressive testing methods with a PHP Unit simulating user behavior. Through this feature requests are made to the application's functions and the majority of your testing load is cut-off.
Apart from these features, the Laravel framework is also known for automation with Artisan CLI.
There are a few cons of the Laravel framework. For instance, it is lightweight and due to this reason, there is excessive congestion of database queries in the backend; however, it can be easily taken care of if you hire professional Laravel developers from a reputed software development company such as Citta Solutions.
An Introduction to Codeigniter Framework
Codeigniter is another robust PHP framework that is famous for its minimum digital footprint. It is ideal for web application developers looking for a straightforward and less complicated framework with a rich toolkit.
This framework came into existence in 2006 and caught on owing to the degree of freedom it gives to web developers. It has absolutely no reliance on the MVC pattern and since it facilitates 3rd party integration with sheer ease you can achieve the most complex functionalities quite easily.
Codeigniter's popularity at a glance
With more than 1,410,088 websites across 39 countries, Codeigniter has 17.7 thousand stars on Github. And if you are looking to create a web application on Codeigniter, you must consider a few factors before making a decision.
Benefits of Codeigniter
User-friendly interface
Codeigniter offers an exceptional UI and makes for a good pick if you are looking to create responsive websites or feature-rich web apps.
Security
Make security protocols with sheer ease and take application customization to another level with Codeigniter.
Modularity
Codeigniter is known for its expression engine that enables developers to make the most of built-in authentication. Modular applications are a strong point of this framework.
There are a few downsides of Codeigniter too. It has unstable code maintainability and there are lesser updates that have an impact on the growth and development aspect of it.
Summing it up
Both Laravel and Codeigniter are popular PHP frameworks with their own set of advantages and a few downsides. We highly recommend you take into account your development needs and consult a reliable Laravel development company such as Citta Solutions.
If you have any queries about Laravel or Codeigniter, feel free to touch base with us and we will answer all your queries.
0 notes
Text
Building a RESTful API with Laravel: Best practices and tools
Introduction
Are you looking to hire remote developers for your team? In today's globalized world, diversity and inclusion are crucial for the success of any organization. This blog aims to provide effective strategies for building a diverse and inclusive remote development team. You can foster creativity, innovation, and better problem-solving by embracing differences in backgrounds, experiences, and perspectives. So, let's explore how you can attract and retain a diverse talent pool and create a welcoming environment for everyone, regardless of location or background.
Understanding RESTful API Development
Restful API Development, including hiring remote developers, is crucial to building web applications. In this section, we will explore the fundamentals of RESTful architecture and its principles while discussing the benefits of using RESTful APIs for web application development with remote developers.
Fundamentals of RESTful Architecture:
REST stands for Representational State Transfer, an architectural style for designing networked applications.
RESTful architecture is based on a client-server model, where the client sends requests to the server, and the server responds with the requested data.
RESTful APIs use HTTP (Hypertext Transfer Protocol) as the communication protocol, leveraging its methods such as GET, POST, PUT, and DELETE.
Principles of RESTful Architecture:
Stateless: Each request from the client to the server must contain all the necessary information to understand and process the request. The server retains no information about the client's state between requests. Example: When a user logs in to a web application, the server doesn't store the user's session information. Instead, the client includes the authentication token in each request.
Uniform Interface: RESTful APIs have standardized methods and conventions, making them easy to understand and interact with. Example: Using HTTP methods like GET to retrieve data, POST to create new resources, PUT to update existing resources, and DELETE to remove resources.
Resource-based: RESTful APIs expose resources as URLs (Uniform Resource Locators) that can be accessed and manipulated using HTTP. Example: A web application's user management system may expose resources such as /users, /users/{id}, where {id} represents a specific user.
Benefits of using RESTful APIs for web application development:
Simplicity: RESTful APIs are easy to understand and use. Their standardized interface and use of HTTP methods make them accessible to developers.
Scalability: RESTful APIs allow for scalable architectures, as they can be designed to handle many concurrent requests.
Platform independence: RESTful APIs can be used with any programming language or platform that supports HTTP, making them versatile and widely applicable.
Interoperability: RESTful APIs enable communication between different systems and services, facilitating integration and collaboration.
Caching: RESTful APIs leverage HTTP caching mechanisms, enabling efficient caching of responses and reducing server load.
Setting Up Laravel for API Development
Laravel Basics and Features:
Laravel is a popular PHP framework for web application development.
It follows the Model-View-Controller (MVC) architectural pattern, making it easy to organize code and separate concerns.
Laravel provides a wide range of features, including routing, database ORM (Object-Relational Mapping), caching, session management, and authentication.
Installation and Setup of Laravel:
To get started, make sure you have PHP and Composer installed on your system.
Open your terminal or command prompt and run the following command to install Laravel globally: composer global require laravel/installer.
Once the installation is complete, you can create a new Laravel project by running: laravel new project-name.
Change to the project directory: cd project-name.
Start the local development server: php artisan serve.
Configurations and Dependencies for API Development:
Laravel provides a convenient way to build APIs with its built-in tools and packages.
To configure your API development environment, you may need to:
Set up your database connection in the .env file.
Configure routes in the routes/api.php file.
Install any necessary dependencies using Composer. For example, you can use the following command to install the Laravel Passport package for API authentication: composer require laravel/passport.
Creating a New Laravel Project and Database Configuration:
Open your terminal or command prompt and navigate to the desired directory where you want to create your project.
Run the command: laravel new project-name to create a new Laravel project.
Navigate into the project directory: cd project-name.
Open the .env file and configure your database connection details, such as database name, username, and password.
Create a new database that matches your configuration.
Migrate the database tables by running: php artisan migrate.
You can now start building your APIs using Laravel.
Example: Let's say you want to hire a remote developer to create an API for a blog application. You can use Laravel to handle routing, database operations, and authentication. By following the steps mentioned above, you can set up your Laravel project, configure the database connection, and start building your APIs for creating, updating, and fetching blog posts.
Remember to install any additional dependencies as needed, and leverage Laravel's features like middleware, validation, and error handling to enhance your API development process.
Building a RESTful API with Laravel
Designing and structuring a RESTful API:
Understand REST principles:
REST (Representational State Transfer) is an architectural style for designing networked applications.
It uses HTTP methods (GET, POST, PUT, DELETE) to perform CRUD operations on resources.
RESTful APIs are stateless and rely on a client-server communication model.
Identify the resources:
Determine the data entities or resources that the API will expose.
Each resource should have a unique identifier, such as a URL endpoint.
Define the endpoints:
Map each resource to specific API endpoints.
Use meaningful and descriptive URL paths for each endpoint.
Choose appropriate HTTP methods:
Use GET to retrieve resource representations.
Use POST to create new resources.
Use PUT or PATCH to update existing resources.
Use DELETE to remove resources.
Design response formats:
Decide on the format for API responses, such as JSON or XML.
Ensure consistent and well-structured response payloads.
Creating API endpoints for CRUD operations using Laravel's routing system:
Install Laravel:
Use the Laravel installer or Composer to set up a new Laravel project.
Define routes:
Open the routes/api.php file in your Laravel project.
Define routes using the Route facade and specify the HTTP method, URL endpoint, and associated controller method.
Create controllers:
Generate controllers using Laravel's Artisan command-line tool.
Controllers handle the logic for processing API requests and returning responses.
Implement CRUD operations:
Use Laravel's Eloquent ORM or database query builder to interact with the data within the controller methods.
Use the appropriate HTTP methods and route parameters to perform the desired CRUD operations.
Return responses:
Format the data into the desired response format, such as JSON.
Use Laravel's response() function to send the response back to the client.
Following these steps, you can hire a remote developer to design and structure a RESTful API using Laravel's routing system. This allows you to create API endpoints for performing CRUD operations on your resources efficiently and effectively…
Best Practices and Tools for Laravel API Development
Developing a robust and scalable API with Laravel Development requires following best practices, utilizing the right tools, and considering hiring a remote developer. Here are some essential guidelines and popular API development tools in the Laravel ecosystem.
Best Practices for Laravel API Development:
Versioning: Implement API versioning to manage changes and ensure backward compatibility. Use a versioning strategy like URI-based (e.g., /v1/users) or header-based (e.g., Accept: application/vnd.myapp.v1+json) to distinguish between different API versions.
Authentication and Authorization: Secure your API by implementing authentication and authorization mechanisms. Laravel supports popular authentication methods like JWT (JSON Web Tokens), OAuth, and Passport. Choose the appropriate method based on your application's requirements.
Validation: To ensure data integrity and enforce business rules, validate incoming API requests. Laravel offers powerful validation features using Request objects and validation rules. Use these features to validate input data and return appropriate error responses.
Error Handling: Implement proper error handling and responses for your API. Use consistent error formats (e.g., JSON: API) and provide meaningful error messages. Laravel's exception-handling system allows you to catch and handle exceptions gracefully.
Caching: Utilize caching to improve API performance and reduce database queries. Laravel provides a flexible caching system supporting various cache drivers like Redis and Memcached. Cache frequently accessed data or responses to enhance response times.
Testing: Write comprehensive unit and integration tests to ensure API functionality and prevent regressions. Laravel's testing framework simplifies the process of writing and executing tests. Test various scenarios, including success cases, edge cases, and error conditions.
Popular Tools and Packages for Laravel API Development:
Laravel Sanctum: A lightweight package for API authentication using tokens, cookies, or SPA (Single Page Application) authentication. It simplifies API authentication and integrates well with Laravel's authentication system.
Laravel Telescope: A debugging and profiling tool that provides insights into your API's performance, queries, exceptions, and more. It helps you optimize and monitor your API during development.
Laravel Dusk: A browser automation and testing tool designed for Laravel applications. It allows you to write end-to-end tests for your API, simulating user interactions and verifying functionality.
Fractal: A package for transforming API responses into a consistent and structured format. Fractal simplifies the transformation of complex data structures into JSON or other response formats, making it easier to build robust APIs.
Conclusion
In conclusion, building a RESTful API with Laravel involves implementing best practices and utilizing the right tools. Following these guidelines can create a robust and efficient API that meets industry standards. Remember, hiring experienced developers is crucial to ensure the success of your project. If you want to expand your team, consider hiring remote developers who can contribute their expertise anywhere in the world. With the right talent and resources, you can build a powerful API that supports your application's needs.
1 note
·
View note
Text
Laravel is a popular PHP framework that provides a robust routing system for building web applications. The routing system in Laravel allows you to define how incoming HTTPrequests should be handled and mapped to specific controllers and actions within your application. To get started with the routing system in Laravel, follow these steps: Routes File: Open the routes/web.php file in your Laravel application. This file contains the route definitions for handling web requests. You can define your routes here using the Route facade. Basic Route: A basic route definition consists of an HTTP verb (e.g., GET, POST) and a URL pattern. For example, to handle a GET request to the root of your application, you can define a route like this: phpCopy code Route::get('/', function () return 'Hello, World!'; ); Route Parameters: You can define route parameters by wrapping them in curly braces within the URL pattern. For example, to capture a user ID in the URL, you can define a route like this: phpCopy code Route::get('/user/id', function ($id) return 'User ID: ' . $id; ); Named Routes: You can assign names to your routes using the name method. Named routes are useful when generating URLs or redirecting to a specific route. For example: phpCopy code Route::get('/user/id', function ($id) return 'User ID: ' . $id; )->name('user.profile'); Route Groups: You can group related routes together using the prefix and middleware methods. The prefix method allows you to add a common prefix to a group of routes, while the middleware method allows you to apply middleware to the routes within the group. For example: phpCopy code Route::prefix('admin')->middleware('auth')->group(function () Route::get('/dashboard', function () return 'Admin Dashboard'; ); // Other admin routes... ); Route Controllers: Instead of using anonymous functions as route callbacks, you can also route to controller actions. First, create a controller using the php artisan make:controller command. Then, you can define a route that points to a specific controller and action. For example: phpCopy code Route::get('/user/id', 'UserController@show'); In the above example, the show method of the UserController will be called when a GET request is made to the specified URL. Route Model Binding: Laravel's routing system also supports route model binding, which automatically injects model instances into your controller actions. For example, if you have a User model, you can define a route like this: phpCopy code Route::get('/user/user', function (User $user) return $user; ); In this case, Laravel will automatically fetch the user with the corresponding ID from the database and pass it to the route callback. These are some of the key concepts of Laravel routing system. By using these techniques, you can define flexible and powerful routes to handle various HTTP requests in your Laravel application. Remember to refer to the official Laravel documentation for more details and advanced routing features: https://laravel.com/docs/routing
0 notes
Text
My Own Blog by Laravel(1)
Make my own blog with Laravel!!
Hi guys, I will make my own blog by Laravel. I'm a Japanese cook in BC. But I don't work now because of COVID-19. So I have much time now. That's why I have started to learn Laravel. I'm not a good English writer. But I will do my best in English. Please correct my English if you would notice any wrong expressions. Thank you!
Anyway, I will post about making a blog by Laravel for a while. Let's get started!
All we have to do
Install Laravel
Create a Project
Database Setting
Migration
Create Models
Seeding
Routing
Make Controllers
Make Views
Agenda
Today's agenda is
Install Laravel
Create a Project
Database Setting
Migration
Create Models
Seeding
Install Laravel
Laravel utilizes Composer to manage its dependencies. So install Composer first if you have not installed Composer yet. Ok, now you can install Laravel using Composer.
% composer global require Laravel/installer
Here we go. So next step is to create a project named blog!
Create a project
Creating a project in Laravel is super easy. Just type a command like below.
% laravel new blog
That's it. So easy. That command bring every dependencies automatically. And you move to blog directory.
% cd blog
Now you can use a new command called 'artisan'. It's a command used for Laravel. For example, you can start server with this command.
% php artisan serve
Do you see command line like this?
% php artisan serve ~/workspace/blog Laravel development server started: http://127.0.0.1:8000 [Mon Apr 20 09:20:56 2020] PHP 7.4.5 Development Server (http://127.0.0.1:8000) started
You can access localhost:8000 to see the Laravel's welcome page! If you want to know the other arguments of artisan, just type like this.
% php artisan list
Then you can see all artisan commands. You can also display the commands for a specific namespace like this.
% php artisan list dusk ~/workspace/blog Laravel Framework 7.6.2 Usage: command [options] [arguments] Options: -h, --help Display this help message -q, --quiet Do not output any message -V, --version Display this application version --ansi Force ANSI output --no-ansi Disable ANSI output -n, --no-interaction Do not ask any interactive question --env[=ENV] The environment the command should run under -v|vv|vvv, --verbose Increase the verbosity of messages: 1 for normal output, 2 for more verbose output and 3 for debug Available commands for the "dusk" namespace: dusk:chrome-driver Install the ChromeDriver binary dusk:component Create a new Dusk component class dusk:fails Run the failing Dusk tests from the last run and stop on failure dusk:install Install Dusk into the application dusk:make Create a new Dusk test class dusk:page Create a new Dusk page class
So let's go to next step!
Database setting
Open .env located under root directory. And edit around DB setting.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=blog DB_USERNAME=root DB_PASSWORD=
Depends on your database. I use MySQL and I already create database named blog in MySQL. You should create user for only this project when you deploy.
Migration
Laravel supplies the migration system. It allow you to control database using php code. For example, when you want to create database, type the command like this.
% php artisan make:migration create_posts_table
You can see a new migration file database/migrations/xxxx_xx_xx_xxxxxx_create_posts_table.php. Write down columns you need in the function called up() and write down columns you want to delete in down(). Edit it.
public function up() { Schema::create('posts', function (Blueprint $table) { $table->increments('id'); $table->boolean('published'); $table->string('title'); $table->longText('body'); $table->string('tag')->nullable(); $table->timestamps(); }); }
It's ready! Execute this command.
% php artisan migrate
Here we go! Now you got some tables with columns! Let's check them out in MySQL console.
% mysql -uroot
And check tables and columns.
mysql> use blog; Reading table information for completion of table and column names You can turn off this feature to get a quicker startup with -A Database changed mysql> show tables; +----------------+ | Tables_in_blog | +----------------+ | failed_jobs | | migrations | | posts | | users | +----------------+ 4 rows in set (0.01 sec) mysql> desc posts; +------------+------------------+------+-----+---------+----------------+ | Field | Type | Null | Key | Default | Extra | +------------+------------------+------+-----+---------+----------------+ | id | int(10) unsigned | NO | PRI | NULL | auto_increment | | published | tinyint(1) | NO | | NULL | | | title | varchar(191) | NO | | NULL | | | body | longtext | NO | | NULL | | | tag | varchar(191) | YES | | NULL | | | created_at | timestamp | YES | | NULL | | | updated_at | timestamp | YES | | NULL | | | user_id | int(11) | NO | MUL | NULL | | +------------+------------------+------+-----+---------+----------------+ 8 rows in set (0.01 sec)
Good! You could create tables and columns by php. Next step is Create Model.
Create Model
Laravel Framework is MVC application model. MVC is Model, View and Controller. Application works with each role. View works for display to browsers. Controller works as a playmaker. It receives request from router and access databases to get some datas and pass the datas to views. Model connects to the database and gets, inserts, updates or deletes datas.
Now you create a Model.
% php artisan make:model Post
Then you will see the new Post model under app/Post.php.
<?php namespace App; use Illuminate\Database\Eloquent\Model; class Post extends Model { // }
This model has no code. But it's ok. You can leave it for now.
About a model name
A model name is important. A model connects to table of the database with the rule of the name. If you have a posts table, Post model is mapped with posts table automatically.
Seeding
Seeding is very useful function for preparing test datas or master datas. You can use it easily. All you need is just 1. making seeder file and 2. executing it. Let's do that.
Making seeder files
% php artisan make:seeder BooksTableSeeder Seeder created successfully.
Edit seeder files
public function run() { DB::table('posts')->truncate(); $posts = [ [ 'published' => true, 'title' => 'The First Post', 'body' => '1st Lorem ipsum...', 'tag' => 'laravel', 'user_id' => 1 ], [ 'published' => true, 'title' => 'The Second Post', 'body' => '2nd Lorem ipsum dolor sit amet...', 'tag' => 'shiba-inu', 'user_id' => 1 ], [ 'published' => false, 'title' => 'The Third Post', 'body' => '3rd Lorem ipsum dolor sit ...', 'tag' => 'laravel', 'user_id' => 1 ] ]; foreach($posts as $post) { \App\Post::create($post); } }
And edit DatabaseSeeder.php file.
public function run() { // $this->call(UserSeeder::class); $this->call(PostsTableSeeder::class); }
Execute seegding
% php artisan db:seed Seeding: PostsTableSeeder Database seeding completed successfully.
Sweet. Let's check out database.
mysql> select * from posts; +----+-----------+-----------------+-----------------------------------+---------------------------------+---------------------+---------+ | id | published | title | body | tag | created_at | updated_at | user_id | +----+-----------+-----------------+-----------------------------------+-----------+---------------------+---------------------+---------+ | 1 | 1 | The First Post | 1st Lorem ipsum... | laravel | 2020-04-19 19:16:18 | 2020-04-19 19:16:18 | 1 | | 2 | 1 | The Second Post | 2nd Lorem ipsum dolor sit amet... | shiba-inu | 2020-04-19 19:16:18 | 2020-04-19 19:16:18 | 1 | | 3 | 0 | The Third Post | 3rd Lorem ipsum dolor sit ... | laravel | 2020-04-19 19:16:18 | 2020-04-19 19:16:18 | 1 | +----+-----------+-----------------+-----------------------------------+-----------+---------------------+---------------------+---------+ 3 rows in set (0.00 sec)
Perfect! Now we can go next step!
So, see you next time!
References
Installation - Laravel - The PHP Framework For Web Artisans
1 note
·
View note
Text
Laravel 5.8 Post Form Data From View to Controller Using Ajax
[ad_1] In this laravel tutorial I have explained how can we post form data from view to controller Using ajax in Laravel 5.8. I also explained how can we validate the form.
#Laravel5.8 #Laravel
1 – Create Controller (0:25) 2 – Create Route (1:05) 3 – Include jQuery for Ajax Function (7:40) 3 – Add Server Side Validation (17:02)
This laravel tutorial video is very good for beginners where they…
View On WordPress
#get data in controller laravel#how to pass form value to controller in laravel#how to send data from form to database in laravel#how to send form data to controller in laravel#Laravel#laravel pass data from view to controller#laravel pass request to controller#laravel post request from controller#laravel post route#laravel tutorial#laravel tutorial for beginners step by step#pass form data to controller laravel#send post data to controller laravel#view to controller laravel
0 notes