#what are tuples in python
Explore tagged Tumblr posts
Text
Python Operator Basics
x or y - Logical or (y is evaluated only if x is false)
lambda args: expression - Anonymous functions (I don't know what the fuck is this, I have to look into it)
x and y - Logical and (Y is evaluated only if x is true)
<, <=, >, >=, ==, <>, != - comparison tests
is, is not - identity tests
in, in not - membership tests
x | y - bitwise or (I still have to learn bitwise operation)
x^y - bitwise exclusive or (I read this somewhere now I don't remember it)
x&y - bitwise and (again I have to read bitwise operations)
x<;<y, x>>y - shift x left or right by y bits
x+y, x-y - addition/concatenation , subtraction
x*y, x/y, x%y - multiplication/repetition, division, remainder/format (i don't know what format is this? should ask bard)
-x, +x, ~x - unary negation, identity(what identity?), bitwise complement (complement?)
x[i], x[i:j], x.y, x(...) - indexing, slicing, qualification (i think its member access), function call
(...), [...], {...} `...` - Tuple , list dictionary , conversion to string
#kumar's python study notes#study notes#study blog#coding#programmer#programming#python#studyblr#codeblr#progblr
67 notes
·
View notes
Text
What is Data Structure in Python?
Summary: Explore what data structure in Python is, including built-in types like lists, tuples, dictionaries, and sets, as well as advanced structures such as queues and trees. Understanding these can optimize performance and data handling.
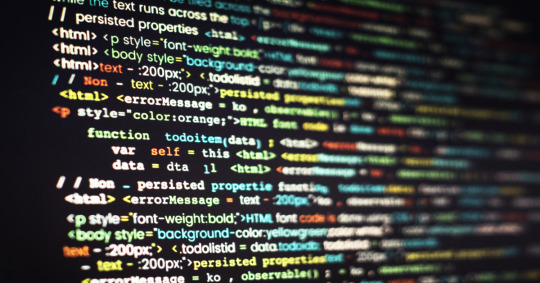
Introduction
Data structures are fundamental in programming, organizing and managing data efficiently for optimal performance. Understanding "What is data structure in Python" is crucial for developers to write effective and efficient code. Python, a versatile language, offers a range of built-in and advanced data structures that cater to various needs.
This blog aims to explore the different data structures available in Python, their uses, and how to choose the right one for your tasks. By delving into Python’s data structures, you'll enhance your ability to handle data and solve complex problems effectively.
What are Data Structures?
Data structures are organizational frameworks that enable programmers to store, manage, and retrieve data efficiently. They define the way data is arranged in memory and dictate the operations that can be performed on that data. In essence, data structures are the building blocks of programming that allow you to handle data systematically.
Importance and Role in Organizing Data
Data structures play a critical role in organizing and managing data. By selecting the appropriate data structure, you can optimize performance and efficiency in your applications. For example, using lists allows for dynamic sizing and easy element access, while dictionaries offer quick lookups with key-value pairs.
Data structures also influence the complexity of algorithms, affecting the speed and resource consumption of data processing tasks.
In programming, choosing the right data structure is crucial for solving problems effectively. It directly impacts the efficiency of algorithms, the speed of data retrieval, and the overall performance of your code. Understanding various data structures and their applications helps in writing optimized and scalable programs, making data handling more efficient and effective.
Read: Importance of Python Programming: Real-Time Applications.
Types of Data Structures in Python
Python offers a range of built-in data structures that provide powerful tools for managing and organizing data. These structures are integral to Python programming, each serving unique purposes and offering various functionalities.
Lists
Lists in Python are versatile, ordered collections that can hold items of any data type. Defined using square brackets [], lists support various operations. You can easily add items using the append() method, remove items with remove(), and extract slices with slicing syntax (e.g., list[1:3]). Lists are mutable, allowing changes to their contents after creation.
Tuples
Tuples are similar to lists but immutable. Defined using parentheses (), tuples cannot be altered once created. This immutability makes tuples ideal for storing fixed collections of items, such as coordinates or function arguments. Tuples are often used when data integrity is crucial, and their immutability helps in maintaining consistent data throughout a program.
Dictionaries
Dictionaries store data in key-value pairs, where each key is unique. Defined with curly braces {}, dictionaries provide quick access to values based on their keys. Common operations include retrieving values with the get() method and updating entries using the update() method. Dictionaries are ideal for scenarios requiring fast lookups and efficient data retrieval.
Sets
Sets are unordered collections of unique elements, defined using curly braces {} or the set() function. Sets automatically handle duplicate entries by removing them, which ensures that each element is unique. Key operations include union (combining sets) and intersection (finding common elements). Sets are particularly useful for membership testing and eliminating duplicates from collections.
Each of these data structures has distinct characteristics and use cases, enabling Python developers to select the most appropriate structure based on their needs.
Explore: Pattern Programming in Python: A Beginner’s Guide.
Advanced Data Structures
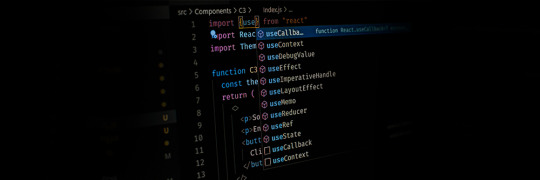
In advanced programming, choosing the right data structure can significantly impact the performance and efficiency of an application. This section explores some essential advanced data structures in Python, their definitions, use cases, and implementations.
Queues
A queue is a linear data structure that follows the First In, First Out (FIFO) principle. Elements are added at one end (the rear) and removed from the other end (the front).
This makes queues ideal for scenarios where you need to manage tasks in the order they arrive, such as task scheduling or handling requests in a server. In Python, you can implement a queue using collections.deque, which provides an efficient way to append and pop elements from both ends.
Stacks
Stacks operate on the Last In, First Out (LIFO) principle. This means the last element added is the first one to be removed. Stacks are useful for managing function calls, undo mechanisms in applications, and parsing expressions.
In Python, you can implement a stack using a list, with append() and pop() methods to handle elements. Alternatively, collections.deque can also be used for stack operations, offering efficient append and pop operations.
Linked Lists
A linked list is a data structure consisting of nodes, where each node contains a value and a reference (or link) to the next node in the sequence. Linked lists allow for efficient insertions and deletions compared to arrays.
A singly linked list has nodes with a single reference to the next node. Basic operations include traversing the list, inserting new nodes, and deleting existing ones. While Python does not have a built-in linked list implementation, you can create one using custom classes.
Trees
Trees are hierarchical data structures with a root node and child nodes forming a parent-child relationship. They are useful for representing hierarchical data, such as file systems or organizational structures.
Common types include binary trees, where each node has up to two children, and binary search trees, where nodes are arranged in a way that facilitates fast lookups, insertions, and deletions.
Graphs
Graphs consist of nodes (or vertices) connected by edges. They are used to represent relationships between entities, such as social networks or transportation systems. Graphs can be represented using an adjacency matrix or an adjacency list.
The adjacency matrix is a 2D array where each cell indicates the presence or absence of an edge, while the adjacency list maintains a list of edges for each node.
See: Types of Programming Paradigms in Python You Should Know.
Choosing the Right Data Structure
Selecting the appropriate data structure is crucial for optimizing performance and ensuring efficient data management. Each data structure has its strengths and is suited to different scenarios. Here’s how to make the right choice:
Factors to Consider
When choosing a data structure, consider performance, complexity, and specific use cases. Performance involves understanding time and space complexity, which impacts how quickly data can be accessed or modified. For example, lists and tuples offer quick access but differ in mutability.
Tuples are immutable and thus faster for read-only operations, while lists allow for dynamic changes.
Use Cases for Data Structures:
Lists are versatile and ideal for ordered collections of items where frequent updates are needed.
Tuples are perfect for fixed collections of items, providing an immutable structure for data that doesn’t change.
Dictionaries excel in scenarios requiring quick lookups and key-value pairs, making them ideal for managing and retrieving data efficiently.
Sets are used when you need to ensure uniqueness and perform operations like intersections and unions efficiently.
Queues and stacks are used for scenarios needing FIFO (First In, First Out) and LIFO (Last In, First Out) operations, respectively.
Choosing the right data structure based on these factors helps streamline operations and enhance program efficiency.
Check: R Programming vs. Python: A Comparison for Data Science.
Frequently Asked Questions
What is a data structure in Python?
A data structure in Python is an organizational framework that defines how data is stored, managed, and accessed. Python offers built-in structures like lists, tuples, dictionaries, and sets, each serving different purposes and optimizing performance for various tasks.
Why are data structures important in Python?
Data structures are crucial in Python as they impact how efficiently data is managed and accessed. Choosing the right structure, such as lists for dynamic data or dictionaries for fast lookups, directly affects the performance and efficiency of your code.
What are advanced data structures in Python?
Advanced data structures in Python include queues, stacks, linked lists, trees, and graphs. These structures handle complex data management tasks and improve performance for specific operations, such as managing tasks or representing hierarchical relationships.
Conclusion
Understanding "What is data structure in Python" is essential for effective programming. By mastering Python's data structures, from basic lists and dictionaries to advanced queues and trees, developers can optimize data management, enhance performance, and solve complex problems efficiently.
Selecting the appropriate data structure based on your needs will lead to more efficient and scalable code.
#What is Data Structure in Python?#Data Structure in Python#data structures#data structure in python#python#python frameworks#python programming#data science
6 notes
·
View notes
Text
What is Python, How to Learn Python?
What is Python?
Python is a high-level, interpreted programming language known for its simplicity and readability. It is widely used in various fields like: ✅ Web Development (Django, Flask) ✅ Data Science & Machine Learning (Pandas, NumPy, TensorFlow) ✅ Automation & Scripting (Web scraping, File automation) ✅ Game Development (Pygame) ✅ Cybersecurity & Ethical Hacking ✅ Embedded Systems & IoT (MicroPython)
Python is beginner-friendly because of its easy-to-read syntax, large community, and vast library support.
How Long Does It Take to Learn Python?
The time required to learn Python depends on your goals and background. Here’s a general breakdown:
1. Basics of Python (1-2 months)
If you spend 1-2 hours daily, you can master:
Variables, Data Types, Operators
Loops & Conditionals
Functions & Modules
Lists, Tuples, Dictionaries
File Handling
Basic Object-Oriented Programming (OOP)
2. Intermediate Level (2-4 months)
Once comfortable with basics, focus on:
Advanced OOP concepts
Exception Handling
Working with APIs & Web Scraping
Database handling (SQL, SQLite)
Python Libraries (Requests, Pandas, NumPy)
Small real-world projects
3. Advanced Python & Specialization (6+ months)
If you want to go pro, specialize in:
Data Science & Machine Learning (Matplotlib, Scikit-Learn, TensorFlow)
Web Development (Django, Flask)
Automation & Scripting
Cybersecurity & Ethical Hacking
Learning Plan Based on Your Goal
📌 Casual Learning – 3-6 months (for automation, scripting, or general knowledge) 📌 Professional Development – 6-12 months (for jobs in software, data science, etc.) 📌 Deep Mastery – 1-2 years (for AI, ML, complex projects, research)
Scope @ NareshIT:
At NareshIT’s Python application Development program you will be able to get the extensive hands-on training in front-end, middleware, and back-end technology.
It skilled you along with phase-end and capstone projects based on real business scenarios.
Here you learn the concepts from leading industry experts with content structured to ensure industrial relevance.
An end-to-end application with exciting features
Earn an industry-recognized course completion certificate.
For more details:
#classroom#python#education#learning#teaching#institute#marketing#study motivation#studying#onlinetraining
2 notes
·
View notes
Text
RenPy: Defining Characters
One of the first things RenPy does upon initialization (the boot-up period before the start label executes the game) is read your custom-made character strings to determine what character dialogue will look like.
Although defining usually takes place under the init block, I've chosen to make a separate pre-start label for organization purposes. Really, any time is fine as long as you make sure the pre-start label runs before the game actually executes, or else you're going to encounter errors.
Let's take a look at the code piece by piece.
$ a = Person(Character("Arthur", who_color="#F4DFB8", who_outlines=[( 3, "#2B0800", 0, 2 )], what_outlines=[( 3, "#2B0800", 0, 2 )], what_color="#F4DFB8", who_font="riseofkingdom.ttf", what_font="junicode.ttf", ctc="ctc", ctc_position="fixed", what_prefix='"', what_suffix='"'), "Arthur", "images/arthurtemp1.png") $ is a common symbol used by both Python and RenPy to define custom-made variables. Here in the pre-start label section of our script, we're using it to define our characters.
For the sake of propriety, it's probably better to define characters using define, but for ease of use, I've chosen $ instead.
It would be tiresome to have to write "Arthur" every time I wanted to call him in the script. Luckily, by assigning these parameters before initialization, RenPy will read "a" as Arthur.
Most scripts will suffice with assigning a Character object class to your character. If you open the script for The Tutorial, you'll find a basic string that looks like this: $ e = Character("Eileen") As you can see in my batch of code, however, I've done something different by nestling the Character object class within a Person object class. The reason why will become apparent in future posts.
For now, let's focus on the fundamentals.
---
who_color tells RenPy the color of the character's name, determined by a hexadecimal code (either three, four, or six digits). Its sister parameter what_color tells RenPy the color of a character's dialogue text.
If no values are given for these parameters, RenPy will look at your project's GUI file to determine them.
---
who_font tells RenPy the kind of font you want to use for your character's name, and likewise, what_font determines the font you want to use for your character's dialogue.
Note that these fonts do not have to match. They can be whatever font you wish.
The size of character names and dialogue text can be customized in the GUI file of your project:
---
who_outlines=[( 3, "#2B0800", 0, 2 )], what_outlines=[( 3, "#2B0800", 0, 2 )]
who_outlines and what_outlines add outlines or drop shadows to your text (character name and character dialogue, respectively). This string is expressed as a tuple, or four values enclosed by parentheses.
The first value expresses the width of the shadow in pixels. The second value is a hexadecimal value for your chosen color. The third value offsets the shadow along the X-axis (pixels to the right or left of the text). Because it's set to 0, my drop shadows do not appear to the right or the left of the text. The fourth value offsets the shadow along the Y-axis (pixels beneath/above the text). In this case, shadows appear 2 pixels beneath the text.
My outlines are a bit hard to see because they're only 3 pixels wide and 2 pixels offset.
---
Font files RenPy recognizes TrueType font files. You can download TTF fonts for free online - just be sure to unzip them and put them in your game folder.
If you intend to monetize your project, you absolutely need to make certain your fonts are royalty-free or, ideally, public domain. Most font families come with licenses telling you whether they are free use.
To be on the safe side, I would put the following code before the start label in your script, just so RenPy knows which files to look for:
init:
define config.preload_fonts = ["fontname1.ttf", "fontname2.ttf", "fontname3.ttf"]
---
ctc stands for "click to continue." It's a small icon commonly seen in visual novels, usually in one corner of the text box, that indicates the player has reached the end of a line. It's called "click to continue" because the program waits for the reader to interact to continue. To make a custom ctc icon, make a small drawing in an art program and save the image to your GUI folder. As seen above, I'm using a tiny moon as the ctc in my current project. ctc_position="fixed" means the ctc icon will stay rooted in whatever place you specify in the code. Like with most everything else in RenPy, you can apply transforms to the ctc if you so wish. Fun fact: because the ctc is determined on a character-by-character basis in initialization, you can give different characters custom ctcs!
---
what_prefix="" and what_suffix="" add scare quotes to the beginning and end of a character's dialogue.
One thing you'll notice as you work with RenPy is that "the computer is stupid." That is to say, the program will not execute code you don't explicitly spell out. Things which seem intuitive and a given to us are not interpreted by the program unless you write them into the code.
That is why, in this case, you need to specify both prefix and suffix, otherwise RenPy may begin lines of dialogue with " but not end with ", or vice-versa.
Note that unless you apply these parameters to the narrator character, ADV and NVL narration will not have them.
---
** Note: the next two tags following these ones are extraneous and therefore ignored.
9 notes
·
View notes
Text
Beginner’s Guide to Data Science: What You’ll Learn at Advanto
If you're looking to break into the world of data, the Data Science Course in Pune offered by Advanto Software is your perfect starting point. Designed for complete beginners and career-switchers, this course equips you with all the essential skills needed to thrive in today’s data-driven world.
In this beginner’s guide, we'll walk you through what data science really is, why it matters, and most importantly, what you’ll learn at Advanto that makes our course stand out in Pune’s competitive tech landscape.
What is Data Science?
Data Science is the art and science of using data to drive decision-making. It involves collecting, cleaning, analyzing, and interpreting data to uncover patterns and insights that can help businesses make smarter decisions. From predicting customer behavior to optimizing supply chains, data science is everywhere.
If you're fascinated by numbers, patterns, and technology, then a Data Science Course in Pune is a fantastic career move.
Why Choose Advanto for Your Data Science Training?
At Advanto, we believe in practical learning. Our Data Science Course in Pune combines theoretical foundations with hands-on experience so that students don’t just learn — they apply. Whether you're from a coding background or completely new to tech, our structured modules and expert mentors help you build skills step by step.
Key Topics You’ll Learn in the Data Science Course at Advanto
Here’s a breakdown of the essential concepts covered in our program:
1. Python Programming
Python is the backbone of modern data science. You'll learn:
Variables, loops, and functions
Data structures like lists, tuples, and dictionaries
Libraries like NumPy, Pandas, and Matplotlib
By the end of this module, you'll be able to write scripts that automate data analysis tasks efficiently.
2. Statistics and Probability
Understanding the math behind data science is crucial. Our course simplifies:
Descriptive statistics
Inferential statistics
Probability distributions
Hypothesis testing
This foundation helps in making sense of real-world data and building predictive models.
3. Machine Learning
Here, you’ll explore how machines can learn from data using algorithms. The course covers:
Supervised and unsupervised learning
Algorithms like Linear Regression, Decision Trees, and K-Means
Model evaluation and tuning
You’ll build your first ML models and see them in action!
4. Data Visualization
Data is only as good as how you present it. Learn to use:
Matplotlib, Seaborn, and Plotly
Dashboards using Power BI or Tableau
Storytelling with data
You’ll turn complex datasets into simple, interactive visuals.
5. SQL and Databases
Learn how to work with structured data using:
SQL queries (SELECT, JOIN, GROUP BY, etc.)
Database design and normalization
Connecting SQL with Python
This is an essential skill for every data scientist.
6. Capstone Projects and Real-world Use Cases
At the end of your Data Science Course in Pune, you’ll work on real industry projects. Whether it’s predicting house prices, customer churn, or sales forecasting — your portfolio will show employers what you’re capable of.
Career Support and Certification
Our job-ready curriculum is backed by placement assistance, resume workshops, and mock interviews. Once you complete the course, you’ll receive a globally recognized certification that adds weight to your resume.
Who Can Enroll?
Our course is beginner-friendly, and anyone can join:
College students
Working professionals
Engineers and IT professionals
Career switchers
Whether you're in marketing, finance, or HR, learning data science opens new career opportunities.
Why Data Science is the Future
Data Science isn't just a trend — it’s the future. With industries increasingly relying on data, the demand for skilled professionals is skyrocketing. Pune, being a major tech hub, offers vast opportunities in this space. That’s why enrolling in a Data Science Course in Pune now can fast-track your career.
Conclusion: Take Your First Step with Advanto
The Data Science Course in Pune at Advanto Software is more than just a training program — it’s a career-launching experience. With expert faculty, hands-on projects, and strong placement support, Advanto is the right place to begin your journey into the exciting world of data science.
Visit Advanto Software to learn more and enroll today. Don’t just learn data science — live it with Advanto.
#best software testing institute in pune#classes for data science#software testing classes in pune#advanto software#data science and analytics courses#full stack web development#software testing courses in pune#software testing training in pune#software testing course with job guarantee#data science course in pune
1 note
·
View note
Text
Best Python Programming

However, your ideal Python version would depend on your specific needs and the libraries you use. Keeping these things in mind will help you to choose the best version and thus set yourself up for success with your machine learning projects. This means that data professionals can use it to complete essential daily tasks quickly. Python programming is also an essential skill that recruiters and employers look for in a true data scientist. It can enable data analysis, visualisation, automation, and machine learning tasks to be completed quickly and efficiently. Thus, Python is the best version that works on machine learning due to its modern features, active support, and compatibility with important libraries - python programming in Patel Nagar.
A supportive and engaging instructor can significantly improve your understanding and mastery of concepts. Python is also considered one of the easiest programming languages to learn, and there are Python short courses for beginners available through RMIT Online. Built-in functions do not need to be imported and can be utilised instantly as they are installed into Python by default. We have explored print and its complexities together in previous guide, along with a few other built-in functions, but here are all of them. This guide will provide a complete understanding of what operators within Python are - ai ml course in Patel Nagar.
Furthermore, it will elucidate every single operator type, summarise their purposes and demonstrate to you how they can be useful in your coding ventures. Operators are used to perform operations on variables and values. Depending on what they are they can be a method of comparison, give tools for calculations or even assign values to identifiers. We can be created using many different external packages in Python. Beginner-friendly syntax, it is unbelievably powerful. Whether you are working on a small personal project or want to go all cylinders and create a vast undertaking, Python has your back. The most complex technical concept used is tuple unpacking, but all the user needs to know here is that they’re getting multiple return values. Probably the single most important reason Python becomes so popular in the machine learning community is due to its diverse libraries. For more information, please visit our site https://eagletflysolutions.com/
0 notes
Text
Why Should You Learn Python Programming?

If you’re thinking about entering the tech world, learning Python programming is an excellent choice. It's one of the most popular and versatile programming languages, used across many industries including web development, data science, machine learning, and automation. But why is it so widely recommended, especially for beginners? Let’s explore.
What Will You Learn in a Python Programming Course?
A comprehensive Python course will introduce you to several key concepts:
Basic Syntax and Concepts: You’ll start with Python’s basic syntax, learning how to write simple programs and understanding core concepts like variables, loops, and conditionals.
Data Structures: Python makes it easy to work with data. You’ll learn to use lists, dictionaries, tuples, and sets to organize and manage information efficiently.
Functions and Loops: Functions allow you to reuse code, while loops help you automate repetitive tasks. Mastering these concepts is crucial for becoming an efficient programmer.
Object-Oriented Programming (OOP): This concept enables you to write scalable code by organizing it into reusable objects and classes—essential for building complex applications.
Libraries for Data Analysis: Python’s popularity in data science comes from its libraries, like Pandas, NumPy, and Matplotlib, that help you process, analyze, and visualize data with ease.
Why Choose an Online Python Programming Course?
Online Python courses offer great flexibility and benefits:
Self-paced Learning: You can study at your own pace, making it easier to balance your learning with other responsibilities.
Hands-on Projects: Many online courses feature real-world projects that allow you to apply what you've learned immediately.
Community Support: Online platforms often have active forums and communities where you can connect with peers and instructors for guidance.
Real-World Applications of Python
Learning Python opens the door to many exciting fields:
Web Development: Build dynamic websites and web applications using frameworks like Django or Flask.
Data Science: Use Python’s libraries to analyze large datasets and uncover insights.
Machine Learning: Python is a go-to language for building machine learning models with libraries such as Scikit-learn and TensorFlow.
Automation: Automate repetitive tasks and workflows using Python scripts, saving time and effort.
Example: Real-World Use of Python in Action
For example, Racila Softech, a tech company, utilizes Python in many of their projects, such as data analysis and web development. By using Python’s simplicity and powerful libraries, they can efficiently build robust systems and provide innovative solutions to their clients. This demonstrates how learning Python can lead to real-world applications that solve complex problems.
0 notes
Text
Learning Python Basics: A Step-by-Step Approach
Python is one of the easiest and most powerful programming languages for beginners to start with. Its clean syntax and readability make it a favorite among new learners, while its flexibility and vast community support make it useful across different fields like web development, data analysis, automation, and artificial intelligence. Considering the kind support of Python Course in Chennai Whatever your level of experience or reason for switching from another programming language, learning Python gets much more fun.
If you're new to Python, here's a step-by-step approach to help you get started with the basics.
Start with Understanding What Python Is Before diving into coding, it's helpful to know what Python is and why it's so popular. Python is a high-level, interpreted programming language known for its ease of use and versatility. It's used by companies, hobbyists, and professionals alike. Knowing its applications can give you extra motivation to learn.
Set Up Your Environment To start writing Python code, you need to set up your environment. Install Python on your computer and choose a simple text editor or an Integrated Development Environment (IDE) like IDLE or VS Code. Once you're set up, you can begin writing and running your Python scripts.
Learn the Fundamentals The core basics of Python include understanding variables, data types (strings, integers, floats, booleans), and how to perform simple operations like addition or string manipulation. Learn how to print messages, take user input, and understand basic syntax.
Explore Control Structures Control structures like conditional statements (if, else, elif) and loops (for, while) help control the flow of your program. Practice using them in simple examples like guessing games, calculators, or even creating basic menus.
With the aid of Best Online Training & Placement Programs, which offer comprehensive training and job placement support to anyone looking to develop their talents, it’s easier to learn this tool and advance your career.
Functions and Reusability Functions allow you to write reusable blocks of code. Learn how to define and call functions, pass parameters, and return results. This step is important in making your code more organized and scalable.
Lists, Tuples, and Dictionaries Data structures are essential in storing and manipulating data. Learn how to work with lists, tuples, and dictionaries, which are used frequently in Python programs. Practice accessing, updating, and looping through them to get comfortable.
Practice with Simple Projects Once you have the basics, start building small projects. These could be as simple as a to-do list, a temperature converter, or a number guessing game. These projects reinforce what you’ve learned and help you see how everything fits together.
Debug and Learn from Mistakes Errors are part of programming. Learn how to read error messages and understand what went wrong. Debugging your own code builds confidence and teaches you how to think like a developer.
Keep Practicing and Building The more you code, the more fluent you’ll become. Continue experimenting with new ideas and challenges. As you grow, explore more Python topics like file handling, classes and objects, and external libraries.
Learning Python step by step gives you a solid foundation not just in the language itself but in the broader world of programming. With patience and regular practice, you'll find yourself mastering the basics and ready to move on to more advanced projects.
#python course#python training#python#technology#python programming#python online training#python online course#python online classes#python certification#tech
0 notes
Text
Assignment-1 Solved
Q1. Create one variable containing following type of data: (i) string (ii) list (iii) float (iv) tuple Q2. Given are some following variables containing data: (i) var1 = ‘ ‘ (ii) var2 = ‘[ DS , ML , Python]’ (iii) var3 = [ ‘DS’ , ’ML’ , ‘Python’ ] (iv) var4 = 1. What will be the data type of the above given variable. Q3. Explain the use of the following operators using an example: (i) / (ii)…
0 notes
Text
Understanding Data Structures in Python
In the realm of programming, data structures are fundamental components that help in organizing and storing data efficiently. Python, being a versatile and high-level language, provides a rich set of built-in data structures that cater to various computational needs. Whether you are a beginner exploring Python or an experienced programmer, mastering data structures is crucial for optimizing performance and writing efficient code. If you are considering enrolling in a Python course, understanding these structures will undoubtedly enhance your learning experience.
What Are Data Structures?
Data structures refer to the ways in which data is organized, managed, and stored for efficient access and modification. They are essential in software development, playing a critical role in algorithms, databases, and system design. Python offers both built-in data structures and user-defined data structures, allowing developers to implement robust solutions with ease.
Built-in Data Structures in Python
1. Lists
Lists are one of the most commonly used data structures in Python. They are ordered, mutable, and can contain different data types. Lists allow for easy addition, removal, and modification of elements.
# Creating a list
my_list = [1, 2, 3, "Python", True]
# Accessing elements
print(my_list[0]) # Output: 1
# Modifying elements
my_list[1] = 20
# Adding elements
my_list.append(50)
# Removing elements
my_list.remove("Python")
2. Tuples
Tuples are similar to lists but are immutable, meaning their elements cannot be changed after creation. They are useful when you want to store data that should remain constant throughout the program.
# Creating a tuple
my_tuple = (10, 20, 30, "Python")
# Accessing elements
print(my_tuple[2]) # Output: 30
3. Sets
Sets are unordered collections of unique elements. They are useful when dealing with distinct values and performing operations like unions and intersections.
# Creating a set
my_set = {1, 2, 3, 4, 4, 5}
# Adding elements
my_set.add(6)
# Removing elements
my_set.discard(3)
4. Dictionaries
Dictionaries store data in key-value pairs, making them ideal for mapping relationships between elements.
# Creating a dictionary
my_dict = {"name": "Alice", "age": 25, "city": "New York"}
# Accessing values
print(my_dict["name"]) # Output: Alice
# Modifying values
my_dict["age"] = 26
User-Defined Data Structures
While Python’s built-in data structures are powerful, there are times when you need custom structures to handle complex problems. Some of the common user-defined data structures include:
1. Stack
A stack is a Last-In-First-Out (LIFO) data structure, meaning the last element added is the first one to be removed.
class Stack:
def __init__(self):
self.stack = []
def push(self, item):
self.stack.append(item)
def pop(self):
if not self.is_empty():
return self.stack.pop()
def is_empty(self):
return len(self.stack) == 0
my_stack = Stack()
my_stack.push(10)
my_stack.push(20)
print(my_stack.pop()) # Output: 20
2. Queue
A queue follows a First-In-First-Out (FIFO) order, where the first element added is the first to be removed.
from collections import deque
class Queue:
def __init__(self):
self.queue = deque()
def enqueue(self, item):
self.queue.append(item)
def dequeue(self):
if not self.is_empty():
return self.queue.popleft()
def is_empty(self):
return len(self.queue) == 0
my_queue = Queue()
my_queue.enqueue(10)
my_queue.enqueue(20)
print(my_queue.dequeue()) # Output: 10
3. Linked List
A linked list is a sequential data structure where each element (node) contains data and a reference to the next node.
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def insert(self, data):
new_node = Node(data)
new_node.next = self.head
self.head = new_node
def display(self):
current = self.head
while current:
print(current.data, end=" -> ")
current = current.next
print("None")
my_list = LinkedList()
my_list.insert(10)
my_list.insert(20)
my_list.display() # Output: 20 -> 10 -> None
Importance of Learning Data Structures
Understanding data structures is crucial for writing optimized and scalable code. Some benefits include:
Efficient Data Management – Organizing data properly enhances speed and efficiency.
Improved Algorithm Performance – The right data structure can significantly improve algorithm efficiency.
Better Problem Solving – Knowledge of data structures helps tackle complex problems effectively.
Essential for Technical Interviews – Many coding interviews focus on data structure problems.
Conclusion
Data structures form the backbone of programming and computational efficiency. Python offers a range of built-in and user-defined structures to handle diverse programming challenges. Whether it's lists, tuples, stacks, or linked lists, mastering data structures is essential for any Python programmer. By enrolling in a Python course, you can gain in-depth knowledge and practical experience to enhance your programming skills. Start your journey today and take a step closer to becoming a proficient Python developer.
0 notes
Text
Price: [price_with_discount] (as of [price_update_date] - Details) [ad_1] (2nd Edition: Covers Object Oriented Programming) Learn Python Fast and Learn It Well. Master Python Programming with a unique Hands-On Project. Have you always wanted to learn computer programming but are afraid it'll be too difficult for you? Or perhaps you know other programming languages but are interested in learning the Python language fast? This book is for you. You no longer have to waste your time and money learning Python from lengthy books, expensive online courses or complicated Python tutorials. What this book offers... Python for Beginners Complex concepts are broken down into simple steps to ensure that you can easily master the Python language even if you have never coded before. Carefully Chosen Python Examples Examples are carefully chosen to illustrate all concepts. In addition, the output for all examples are provided immediately so you do not have to wait till you have access to your computer to test the examples. Careful selection of topics Topics are carefully selected to give you a broad exposure to Python, while not overwhelming you with information overload. These topics include object-oriented programming concepts, error handling techniques, file handling techniques and more. Learn The Python Programming Language Fast Concepts are presented in a "to-the-point" style to cater to the busy individual. With this book, you can learn Python in just one day and start coding immediately. How is this book different... The best way to learn Python is by doing. This book includes a complete project at the end of the book that requires the application of all the concepts taught previously. Working through the project will not only give you an immense sense of achievement, it"ll also help you retain the knowledge and master the language. Are you ready to dip your toes into the exciting world of Python coding? This book is for you. With the first edition of this book being a #1 best-selling programming ebook on Amazon for more than a year, you can rest assured that this new and improved edition is the perfect book for you to learn the Python programming language fast. Click the BUY button and download it now. What you'll learn: - What is Python? - What software you need to code and run Python programs? - What are variables? - What are the common data types in Python? - What are Lists and Tuples? - How to format strings - How to accept user inputs and display outputs - How to control the flow of program with loops - How to handle errors and exceptions - What are functions and modules? - How to define your own functions and modules - How to work with external files - What are objects and classes - How to write your own class - What is inheritance - What are properties - What is name mangling .. and more... Finally, you'll be guided through a hands-on project that requires the application of all the topics covered. Click the BUY button and download the book now to start learning Python. Learn it fast and learn it well. ASIN : B071Z2Q6TQ Publisher : Learn Coding Fast; 2nd edition (10 May 2017) Language : English File size : 475 KB Text-to-Speech : Enabled Screen Reader
: Supported Enhanced typesetting : Enabled X-Ray : Enabled Word Wise : Not Enabled Print length : 175 pages [ad_2]
0 notes
Text
Master Your Python Interview with These Essential Q&A Tips Python continues to dominate the tech industry, powering applications in fields ranging from web development to machine learning. Its simplicity and versatility make it a favorite among developers and employers alike. For candidates preparing for Python interviews, understanding the commonly asked questions and how to answer them effectively can be the key to landing your dream job. This guide covers essential Python interview questions and answers, categorized for beginners, intermediates, and experts. Let’s dive in! 1. Basic Python Interview Questions Q1: What is Python, and what are its key features? Answer: Python is a high-level, interpreted programming language known for its readability and simplicity. Key features include: Easy syntax, similar to English. Dynamically typed (no need to declare variable types). Extensive standard libraries. Cross-platform compatibility. Supports multiple paradigms: object-oriented, procedural, and functional. Q2: What are Python’s data types? Answer: Python offers the following built-in data types: Numeric: int, float, complex Sequence: list, tuple, range Text: str Set: set, frozenset Mapping: dict Boolean: bool Binary: bytes, bytearray, memoryview Q3: Explain Python’s Global Interpreter Lock (GIL). Answer: The Global Interpreter Lock (GIL) is a mutex that protects access to Python objects, preventing multiple native threads from executing Python bytecode simultaneously. This ensures thread safety but can limit multithreading performance in CPU-bound tasks. Q4: What are Python’s popular frameworks? Answer: Some popular Python frameworks include: Web Development: Django, Flask, FastAPI Data Science: TensorFlow, PyTorch, Pandas Automation: Selenium, Robot Framework 2. Intermediate Python Interview Questions Q5: What is the difference between shallow and deep copying? Answer: Shallow Copy: Creates a new object but inserts references to the original objects within it. Use copy.copy(). Deep Copy: Creates a new object and recursively copies all objects within it. Use copy.deepcopy(). Q6: What are Python decorators? Answer: Decorators are functions that modify the behavior of another function or method. They are applied using the @decorator_name syntax and are commonly used for: Logging Authentication Performance measurement Access control Example: def decorator(func): def wrapper(): print("Before function execution") func() print("After function execution") return wrapper @decorator def say_hello(): print("Hello!") say_hello() Q7: How is memory managed in Python? Answer: Python uses automatic memory management through: Reference Counting: Tracks the number of references to an object. Garbage Collection: Reclaims memory when objects are no longer in use. Memory Pools: Allocates memory blocks to improve efficiency. 3. Advanced Python Interview Questions Q8: Explain Python’s metaclasses. Answer: Metaclasses define how classes behave. They control class creation and are specified using the metaclass keyword in class definitions. Metaclasses are commonly used to: Enforce coding standards. Add methods or attributes dynamically. Perform validation during class creation. Q9: What are Python’s comprehensions? Answer: Comprehensions provide a concise way to create sequences. Types include: List Comprehension: [x for x in range(10)] Set Comprehension: x for x in range(10) Dictionary Comprehension: x: x**2 for x in range(10) Generator Expression: (x for x in range(10)) Q10: How can you optimize Python code? Answer: Use built-in functions and libraries. Apply list comprehensions instead of loops. Use generators for large datasets. Leverage caching with functools.lru_cache. Profile code using cProfile and optimize hotspots.
Python Coding Challenges for Interviews Challenge 1: Reverse a String Write a function to reverse a string without using built-in functions. def reverse_string(s): result = "" for char in s: result = char + result return result Challenge 2: FizzBuzz Problem Print numbers from 1 to 100. For multiples of 3, print “Fizz”; for multiples of 5, print “Buzz”; for multiples of both, print “FizzBuzz”. for i in range(1, 101): if i % 3 == 0 and i % 5 == 0: print("FizzBuzz") elif i % 3 == 0: print("Fizz") elif i % 5 == 0: print("Buzz") else: print(i) Challenge 3: Find Duplicates in a List Write a function to find duplicate elements in a list. def find_duplicates(lst): seen = set() duplicates = set() for item in lst: if item in seen: duplicates.add(item) else: seen.add(item) return list(duplicates) Conclusion Preparing for a Python interview requires a mix of theoretical knowledge and hands-on practice. The questions above cover a wide range of topics, ensuring you are well-equipped for technical discussions. Remember, employers value problem-solving skills and clear communication as much as technical proficiency. Practice consistently, and you’ll be ready to ace that Python interview!
0 notes
Text
Best Online Python Programming Course by Pro Academys: Master Python with Us
If you're looking to jumpstart your programming career or enhance your coding skills, then Pro Academys is here to help you succeed with the Best Online Python Programming Course available. Python is a versatile and powerful language, widely used in fields like data science, artificial intelligence, web development, and machine learning. At Pro Academys, we offer the most comprehensive and practical Best Online Python Programming Course designed to help learners of all levels, from beginners to advanced coders, become proficient in Python.
Why Choose Pro Academys for Python Programming?
At Pro Academys, we understand that learning Python can open doors to various career opportunities, making it essential to receive high-quality training. Our Best Online Python Programming Course is structured to provide in-depth knowledge and hands-on experience, ensuring you not only understand the theory but can also apply what you’ve learned to real-world projects. Whether you're a student looking to build your portfolio or a professional seeking to enhance your skill set, Pro Academys offers the Best Online Python Programming Course tailored to meet your needs.
Course Structure and Content
The Best Online Python Programming Course by Pro Academys is meticulously designed, covering all fundamental and advanced Python topics. The course is split into several modules, each focusing on key areas such as:
Introduction to Python: Learn the basics of Python programming, including syntax, variables, data types, and basic operators.
Control Structures: Master control flow in Python through loops, conditional statements, and functions.
Data Structures: Gain expertise in working with lists, tuples, dictionaries, and sets to handle complex data efficiently.
Object-Oriented Programming (OOP): Understand the principles of OOP and how to implement classes, objects, inheritance, and polymorphism in Python.
File Handling: Learn how to read from and write to files in Python, a crucial skill for working with data.
Error Handling and Debugging: Understand how to handle exceptions and debug your code efficiently.
Modules and Libraries: Explore Python’s vast ecosystem of libraries such as NumPy, Pandas, Matplotlib, and more.
Web Development with Python: Get introduced to Python frameworks like Django and Flask for web development.
Python for Data Science: Dive deep into how Python is used in data analysis and visualization.
Throughout this Best Online Python Programming Course, Pro Academys ensures that learners are provided with interactive exercises, real-world projects, and assignments that challenge and enhance their coding abilities.
Benefits of the Best Online Python Programming Course by Pro Academys
Flexibility: Our online platform allows you to learn Python at your own pace, from anywhere, and at any time. Whether you're a student or a working professional, the flexibility of the Best Online Python Programming Course ensures that you can balance your learning with other commitments.
Industry-Relevant Projects: One of the key features of the Best Online Python Programming Course is the inclusion of hands-on projects that simulate real-world problems. These projects are designed to prepare you for a career in programming or data science by giving you practical experience in solving complex challenges using Python.
Experienced Instructors: At Pro Academys, we have a team of highly qualified instructors with years of experience in Python programming and software development. They provide guidance and mentorship throughout the Best Online Python Programming Course, helping you master both the theoretical and practical aspects of Python.
Certification: Upon successful completion of the Best Online Python Programming Course, you'll receive a certification from Pro Academys that demonstrates your proficiency in Python programming. This certificate is a great addition to your resume and will give you an edge in the job market.
Career Support: At Pro Academys, we don’t just offer the Best Online Python Programming Course; we also provide ongoing career support. Our team offers career advice, resume building, and interview preparation services to help you land a job in programming, data science, or AI after completing the course.
Who Should Enroll in Pro Academys Best Online Python Programming Course?
The Best Online Python Programming Course by Pro Academys is perfect for:
Beginners: If you're new to programming and want to start with a language that's easy to learn yet highly versatile, Python is the ideal choice. The Best Online Python Programming Course starts with the basics and gradually moves into more advanced topics, ensuring that beginners have a solid foundation.
Data Enthusiasts: Python is the language of choice for data scientists, and our Best Online Python Programming Course covers essential libraries such as Pandas and NumPy, making it a must for anyone interested in data analysis and machine learning.
Working Professionals: If you're a professional looking to add Python to your skillset, our Best Online Python Programming Course offers the flexibility to learn at your own pace without disrupting your work schedule.
Students: Students who want to enhance their resumes and improve their job prospects in fields like data science, software development, or AI will benefit immensely from our Best Online Python Programming Course.
What Sets Pro Academy Apart?
At Pro Academy, we’re committed to providing the Best Online Python Programming Course that not only teaches Python but prepares you for the practical challenges of a programming career. We focus on making learning accessible, affordable, and engaging. Our community of learners is constantly growing, and we strive to make every student's experience productive and rewarding. Here’s what makes our Best Online Python Programming Course unique:
Interactive Learning: We believe in learning by doing. That's why our Best Online Python Programming Course includes quizzes, assignments, and coding challenges that help you apply what you’ve learned.
Peer Interaction: Learning with Pro Academys means becoming part of a vibrant community. You’ll have opportunities to collaborate with fellow students, share insights, and work on projects together, making the learning experience more enriching.
Lifetime Access: When you enroll in our Best Online Python Programming Course, you gain lifetime access to all the course materials, updates, and future content additions.
Enroll in Pro Academys Best Online Python Programming Course Today!
Python is one of the most in-demand programming languages today, and mastering it can significantly boost your career prospects. At Pro Academys, we offer the Best Online Python Programming Course that is not only affordable but also tailored to fit your personal learning style. Whether you're aiming for a career in software development, data science, or AI, our Best Online Python Programming Course will equip you with the skills needed to succeed in today's competitive job market.
Don’t miss this opportunity to learn Python from the best! Enroll in Pro Academys Best Online Python Programming Course today and take the first step toward a successful programming career.
Follow Us:
website: https://www.proacademys.com/
Email Id: [email protected]
Contact No.: +91 6355016394
#PythonProgramming#LearnPython#PythonForBeginners#PythonCourse#OnlinePythonCourse#BestPythonCourse#ProAcademyPython#PythonTraining#PythonDevelopers#PythonLearning#PythonSkills#PythonCoding#PythonCertification#ProgrammingWithPython#PythonOnlineClass#PythonMastery#LearnPythonOnline#PythonForDataScience#PythonExperts#PythonEducation
0 notes
Text
What Is The Role of Python in Artificial Intelligence? - Arya College
Importance of Python in AI
Arya College of Engineering & I.T. has many courses for Python which has become the dominant programming language in the fields of Artificial Intelligence (AI), Machine Learning (ML), and Deep Learning (DL) due to several compelling factors:
1. Simplicity and Readability
Python's syntax is clear and intuitive, making it accessible for both beginners and experienced developers. This simplicity allows for rapid prototyping and experimentation, essential in AI development where iterative testing is common. The ease of learning Python enables new practitioners to focus on algorithms and data rather than getting bogged down by complex syntax.
2. Extensive Libraries and Frameworks
Python boasts a rich ecosystem of libraries specifically designed for AI and ML tasks. Libraries such as TensorFlow, Keras, PyTorch, sci-kit-learn, NumPy, and Pandas provide pre-built functions that facilitate complex computations, data manipulation, and model training. This extensive support reduces development time significantly, allowing developers to focus on building models rather than coding from scratch.
3. Strong Community Support
The active Python community contributes to its popularity by providing a wealth of resources, tutorials, and forums for troubleshooting. This collaborative environment fosters learning and problem-solving, which is particularly beneficial for newcomers to AI. Community support also means that developers can easily find help when encountering challenges during their projects.
4. Versatility Across Applications
Python is versatile enough to be used in various applications beyond AI, including web development, data analysis, automation, and more. This versatility makes it a valuable skill for developers who may want to branch into different areas of technology. In AI specifically, Python can handle tasks ranging from data preprocessing to deploying machine learning models.
5. Data Handling Capabilities
Python excels at data handling and processing, which are crucial in AI projects. Libraries like Pandas and NumPy allow efficient manipulation of large datasets, while tools like Matplotlib and Seaborn facilitate data visualization. The ability to preprocess data effectively ensures that models are trained on high-quality inputs, leading to better performance.
6. Integration with Other Technologies
Python integrates well with other languages and technologies, making it suitable for diverse workflows in AI projects. It can work alongside big data tools like Apache Spark or Hadoop, enhancing its capabilities in handling large-scale datasets. This interoperability is vital as AI applications often require the processing of vast amounts of data from various sources.
How to Learn Python for AI
Learning Python effectively requires a structured approach that focuses on both the language itself and its application in AI:
1. Start with the Basics
Begin by understanding Python's syntax and basic programming concepts:
Data types: Learn about strings, lists, tuples, dictionaries.
Control structures: Familiarize yourself with loops (for/while) and conditionals (if/else).
Functions: Understand how to define and call functions.
2. Explore Key Libraries
Once comfortable with the basics, delve into libraries essential for AI:
NumPy: For numerical computations.
Pandas: For data manipulation and analysis.
Matplotlib/Seaborn: For data visualization.
TensorFlow/Keras/PyTorch: For building machine learning models.
3. Practical Projects
Apply your knowledge through hands-on projects:
Start with simple projects like linear regression or classification tasks using datasets from platforms like Kaggle.
Gradually move to more complex projects involving neural networks or natural language processing.
4. Online Courses and Resources
Utilize online platforms that offer structured courses:
Websites like Coursera, edX, or Udacity provide courses specifically focused on Python for AI/ML.
YouTube channels dedicated to programming can also be valuable resources.
5. Engage with the Community
Join forums like Stack Overflow or Reddit communities focused on Python and AI:
Participate in discussions or seek help when needed.
Collaborate on open-source projects or contribute to GitHub repositories related to AI.
6. Continuous Learning
AI is a rapidly evolving field; therefore:
Stay updated with the latest trends by following relevant blogs or research papers.
Attend workshops or webinars focusing on advancements in AI technologies.
By following this structured approach, you can build a solid foundation in Python that will serve you well in your journey into artificial intelligence and machine learning.
0 notes
Text
What is the difference between list and tuple?
Lists and tuples are both used to store collections of items in Python, but they have key differences in terms of mutability, performance, and use cases.
1. Mutability
The primary difference is that lists are mutable, meaning their elements can be modified, added, or removed after creation. In contrast, tuples are immutable, meaning once they are created, their elements cannot be changed.# List example (mutable) my_list = [1, 2, 3] my_list.append(4) # Modifying the list print(my_list) # Output: [1, 2, 3, 4] # Tuple example (immutable) my_tuple = (1, 2, 3) # my_tuple[0] = 4 # This will raise a TypeError
2. Performance
Tuples are faster than lists because they have a fixed size, making them more memory-efficient. Lists, being dynamic, require additional memory and processing time when modified.
3. Usage
Lists are ideal for situations where the data needs to be changed frequently, such as dynamic collections.
Tuples are best for fixed data, ensuring data integrity, such as storing database records or configuration settings.
4. Syntax Difference
Lists are created using square brackets [ ].
Tuples are created using parentheses ( ).
my_list = [10, 20, 30] my_tuple = (10, 20, 30)
Conclusion
Choosing between lists and tuples depends on the specific use case. If data needs modification, use a list. If data should remain constant, a tuple is the better option.
To learn more about Python and its data structures, consider enrolling in a Python training in Noida for hands-on learning and expert guidance.
0 notes
Text
Mastering Python Programming at DICS Innovatives
Whether you’re a beginner looking to start your programming journey or an experienced developer aiming to expand your skill set, Python offers a rich ecosystem that can cater to various needs. In this blog, we'll explore the essentials of Python programming and highlight a reputable Python programming institute in Pitampura.
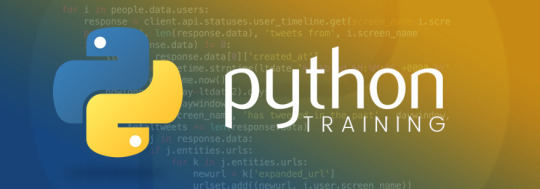
What is Python?
Python is an interpreted, high-level, and general-purpose programming language. It was created by Guido van Rossum and was first released in 1991. Known for its clean syntax and readability, Python enables developers to write code that is Python Training Institute in Pitampura easy to understand and maintain. It supports multiple programming paradigms, including procedural, object-oriented, and functional programming.
Why Learn Python?
High Demand: Python developers are in high demand across various industries, including tech, finance, healthcare, and education.
Career Opportunities: Proficiency in Python can open doors to roles such as data analyst, software developer, web developer, and machine learning engineer.
Versatile Applications: From building web applications to data science and automation, Python can be applied in numerous domains.
Strong Community: Learning Python means joining a supportive community that offers resources, tutorials, and forums for help.
Key Concepts in Python Programming
Variables and Data Types: Understanding how to define variables and the different data types (integers, floats, strings, lists, tuples, dictionaries) is fundamental in Python.
Control Structures: Learn how to use conditional statements (if, elif, else) and loops (for and while) to control the flow of your programs.
Functions: Functions are reusable blocks of code that perform specific tasks. Learning how to define and call functions is crucial.
Object-Oriented Programming (OOP): Python supports OOP principles such as classes, objects, inheritance, and polymorphism, which help in organizing code effectively.
File Handling: Learn how to read from and write to files, which is essential for data manipulation.
Error Handling: Understand how to handle exceptions and errors gracefully using try and except statements.
Libraries and Frameworks: Familiarize yourself with popular libraries like NumPy for numerical computations, Pandas for data manipulation, and Flask or Django for web development.
Python Programming Institute in Pitampura
For those looking to gain a solid foundation in Python, enrolling in a reputable Python programming institute in Pitampura can be highly beneficial. One such institute is Python Institute in Pitampura at DICS Innovatives, known for its comprehensive curriculum and experienced instructors. Here’s what you can expect:
Structured Curriculum: The course covers all essential topics, from basics to advanced concepts, ensuring a well-rounded understanding of Python.
Practical Projects: Engage in practical projects that allow you to apply your knowledge and build a portfolio.
Experienced Trainers: Learn from industry professionals with real-world experience who can provide insights and tips.
Flexible Learning Options: Choose from various formats, including classroom sessions, online courses, and weekend batches to accommodate your schedule.
Certification: Receive a certificate upon completion, enhancing your employability in the job market.
Conclusion
Python programming is not just a valuable skill; it is a gateway to numerous career opportunities in technology and beyond. By understanding its key concepts and enrolling in a respected Python programming institute in Pitampura, you can set yourself up for success in this dynamic field. Whether you're aiming to develop web applications, analyze data, or venture into artificial intelligence, Python has the tools you need to thrive.
Start your Python journey today and unlock a world of possibilities!
0 notes