#API Docs Tool
Explore tagged Tumblr posts
Text
API Documentation Tool | API Docs Tool
Let developers test your APIs directly in the documentation. They can send live requests, get real-time responses, and configure headers, parameters, and payloads easily. It’s a simple, hands-on way to explore and understand your API.
0 notes
Text
shitGPT
for uni im going to be coding with a chatGPT user, so i decided to see how good it is at coding (sure ive heard it can code, but theres a massive difference between being able to code and being able to code well).
i will complain about a specific project i asked it to make and improve on under the cut, but i will copy my conclusion from the bottom of the post and paste it up here.
-
conclusion: it (mostly) writes code that works, but isnt great. but this is actually a pretty big problem imo. as more and more people are using this to learn how to code, or getting examples of functions, theyre going to be learning from pretty bad code. and then theres what im going to be experiencing, coding with someone who uses this tool. theres going to be easily improvable code that the quote unquote writer wont fully understand going into a codebase with my name of it - a codebase which we will need present for our degree. even though the code is not the main part of this project (well, the quality of the code at least. you need it to be able to run and thats about it) its still a shitty feeling having my name attached to code of this quality.
and also it is possible to get it to write good (readable, idiomatic, efficient enough) code, but only if you can write this code yourself (and are willing to spend more time arguing with the AI than you would writing the code.) most of the things i pointed out to the AI was stuff that someone using this as a learning resource wont know about. if it never gives you static methods, class methods, ABCs, coroutines, type hints, multi-file programs, etc without you explicitly asking for them then its use is limited at best. and people who think that its a tool that can take all the info they need, and give it back to them in a concise, readable way (which is a surprising lot of people) will be missing out without even knowing about it.
i got it to write tic-tac-toe (the standard babee) in python (the lang i have to use for uni ;-; (held at gunpoint here)). my specific prompt was "write me a python program for tictactoe that is written in an object oriented way and allows for future expansion via multiple files"
it separated it into three files below (which i think would run, but i never actually ran any of this code. just reading and judging)
why does board use display instead of __str__ and __repr__?
why is the board stored as 1d instead of 2d? thats just confusing
why does it never early return aside from check_winner? (not a big issue here but kept on choosing to never early return when i asked it to add more methods)
why is there no handling of non-number user inputs?
why are non-int inputs truncated instead of telling the user that they should input ints only?
why is display implemented like that?
why are so many lines so bloody long (wide)?
why is there a redundant self.check_winner() after the while loop in TicTaacToe.play()? and if it wasnt redundant then you could finish the game without there being anything printed telling you that the game is finished?
why is the only comment useless? (this comment wouldnt be useless if it was a doc comment tho, but it aint a doc comment. speaking of, why is there no doc comments?)
these are the more immediate things i saw, but there are other things that are bad here.
whenever i write * this is where it updated the api without changing any usage of the api.
so i ask it to change board.display into __str__ and __repr__, it changes it to __str__*, it does not add a __repr__. asking it to add a __repr__ 1) removes the __str__ and 2) gives me this (the other methods are unchanged)
what. the. fuck. this would imply that board takes in an argument for the boardstate, but it clearly doesnt. after 4 more asks it finally has both __str__ and __repr__, without fixing the fact its implying board takes an optional arg, so i get it to add this arg. anything that needs to print the board still calls display btw.
the reasoning it gave for using display over the repr and str magics was this
While using __str__ and __repr__ is a more idiomatic approach in Python, choosing to have a separate display method can still be a valid choice, especially if the display logic is more complex or if you want to keep the __str__ method for a more concise or formal representation of the object.
which, erm what? why would __str__ be for a concise or formal repr when thats what __repr__ is for? who cares about how complex the logic is. youre calling this every time you print, so move the logic into __str__. it makes no difference for the performance of the program (if you had a very expensive func that prints smth, and you dont want it to run every time you try to print the obj then its understandable to implement that alongside str and repr)
it also said the difference between __str__ and __repr__ every damn time, which if youre asking it to implement these magics then surely you already know the difference?
but okay, one issue down and that took what? 5-10 minutes? and it wouldve taken 1 minute tops to do it yourself?
okay next implementing a tic-tac-toe board as a 1d array is fine, but kinda weird when 2d arrays exist. this one is just personal preference though so i got it to change it to a 2d list*. it changed the init method to this
tumblr wont let me add alt text to this image so:
[begin ID: Python code that generates a 2D array using nested list comprehensions. end ID]
which works, but just use [[" "] * 3 for _ in range(3)]. the only advantage listcomps have here over multiplying is that they create new lists, instead of copying the pointers. but if you update a cell it will change that pointer. you only need listcomps for the outermost level.
again, this is mainly personal preference, nothing major. but it does show that chatgpt gives u sloppy code
(also if you notice it got rid of the board argument lol)
now i had to explicitly get it to change is_full and make_move. methods in the same damn class that would be changed by changing to a 2d array. this sorta shit should be done automatically lol
it changed make_move by taking row and col args, which is a shitty decision coz it asks for a pos 1-9, so anything that calls make_move would have to change this to a row and col. so i got it to make a func thatll do this for the board class
what i was hoping for: a static method that is called inside make_move
what i got: a standalone function that is not inside any class that isnt early exited
the fuck is this supposed to do if its never called?
so i had to tell it to put it in the class as a static method, and get it to call it. i had to tell it to call this function holy hell
like what is this?
i cant believe it wrote this method without ever calling it!
and - AND - theres this code here that WILL run when this file is imported
which, errrr, this files entire point is being imported innit. if youre going to have example usage check if __name__ = "__main__" and dont store vars as globals
now i finally asked it to update the other classes not that the api has changed (hoping it would change the implementation of make_move to use the static method.) (it didnt.)
Player.make_move is now defined recursively in a way that doesnt work. yippe! why not propagate the error ill never know.
also why is there so much shit in the try block? its not clear which part needs to be error checked and it also makes the prints go offscreen.
after getting it to fix the static method not being called, and the try block being overcrowded (not getting it to propagate the error yet) i got it to add type hints (if u coding python, add type hints. please. itll make me happy)
now for the next 5 asks it changed 0 code. nothing at all. regardless of what i asked it to do. fucks sake.
also look at this type hint
what
the
hell
is
this
?
why is it Optional[str]???????? the hell??? at no point is it anything but a char. either write it as Optional[list[list[char]]] or Optional[list[list]], either works fine. just - dont bloody do this
also does anything look wrong with this type hint?
a bloody optional when its not optional
so i got it to remove this optional. it sure as hell got rid of optional
it sure as hell got rid of optional
now i was just trying to make board.py more readable. its been maybe half an hour at this point? i just want to move on.
it did not want to write PEP 8 code, but oh well. fuck it we ball, its not like it again decided to stop changing any code
(i lied)
but anyway one file down two to go, they were more of the same so i eventually gave up (i wont say each and every issue i had with the code. you get the gist. yes a lot of it didnt work)
conclusion: as you probably saw, it (mostly) writes code that works, but isnt great. but this is actually a pretty big problem imo. as more and more people are using this to learn how to code, or getting examples of functions, theyre going to be learning from pretty bad code. and then theres what im going to be experiencing, coding with someone who uses this tool. theres going to be easily improvable code that the quote unquote writer wont fully understand going into a codebase with my name of it - a codebase which we will need present for our degree. even though the code is not the main part of this project (well, the quality of the code at least. you need it to be able to run and thats about it) its still a shitty feeling having my name attached to code of this quality.
and also it is possible to get it to write good (readable, idiomatic, efficient enough) code, but only if you can write this code yourself (and are willing to spend more time arguing with the AI than you would writing the code.) most of the things i pointed out to the AI was stuff that someone using this as a learning resource wont know about. if it never gives you static methods, class methods, ABCs, coroutines, type hints, multi-file programs, etc without you explicitly asking for them then its use is limited at best. and people who think that its a tool that can take all the info they need, and give it back to them in a concise, readable way (which is a surprising lot of people) will be missing out without even knowing about it.
#i speak i ramble#effortpost#long post#progblr#codeblr#python#chatgpt#tried to add IDs in as many alts as possible. some didnt let me and also its hard to decide what to put in the IDs for code.#like sometimes you need implementation details but others just the broad overview is good enough yknow?#and i also tried to write in a way where you dont need the IDs to follow along. (but with something like this it is hard yknow?)#id in alt#aside from that one where i got cockblocked#codeblocked?#codeblocked.
40 notes
·
View notes
Text
Who Is a Technical Writer?
A technical writer is a professional who creates clear, concise documentation that explains complex information in a way that's easy to understand. They translate technical concepts into user-friendly content.
---
What Do They Write?
Technical writers produce a wide range of materials, including:
User manuals
Instruction guides
Product documentation
How-to articles
API documentation
Standard Operating Procedures (SOPs)
White papers
Training materials
Online help systems
Software release notes
---
Where Do They Work?
Industries that employ technical writers include:
Tech/software companies
Engineering firms
Medical and healthcare
Manufacturing
Finance
Government agencies
Telecommunications
---
Key Skills of a Technical Writer
1. Excellent writing and communication
2. Ability to understand complex technical information
3. Attention to detail
4. Research and interviewing skills
5. Organization and clarity
6. Collaboration with engineers, designers, developers, etc.
7. Basic design and formatting skills
---
Popular Tools Used
Microsoft Word / Google Docs
Markdown editors
Adobe FrameMaker / InDesign
MadCap Flare
Confluence / Jira
Snagit / Camtasia (for visuals and screen recordings)
Git / GitHub (for version control)
XML / HTML / CSS (basic web formatting)
---
Education & Background
A bachelor’s degree in English, Communications, Technical Writing, Engineering, or Computer Science is common.
Certifications can help (e.g., from the Society for Technical Communication (STC) or Coursera).
Some come from writing backgrounds; others transition from technical fields (like software development or engineering).
---
Career Path & Growth
Junior Technical Writer → Technical Writer → Senior Technical Writer
Specializations: API writer, UX writer, Information Architect, Content Strategist, etc.
Many go freelance or work as consultants.
Remote work is common in this field.
---
Why It's a Good Career
High demand, especially in tech
Remote flexibility
Well-paying (entry level: $50k–$70k; senior roles: $90k+)
Good for writers with an analytical mind
2 notes
·
View notes
Note
Maybe I’m just stupid but I downloaded Python, I downloaded the whole tumblr backup thing & extracted the files but when I opened the folder it wasn’t a system it was just a lot of other folders with like reblog on it? I tried to follow the instructions on the site but wtf does “pip-tumblr-download” mean? And then I gotta make a tumblr “app”? Sorry for bugging you w this
no worries! i've hit the same exact learning curve for this tool LMAO, so while my explanations may be more based on my own understanding of how function A leads to action B rather than real knowledge of how these things Work, I'll help where i can!
as far as i understand, pip is simply a way to install scripts through python rather than through manually downloading and installing something. it's done through the command line, so when it says "pip install tumblr-backup", that means to copy-paste that command into a command line window, press enter, and watch as python installs it directly from github. you shouldn't need to keep the file you downloaded; that's for manual installs.
HOWEVER! if you want to do things like saving audio/video, exif tagging, saving notes, filtering, or all of the above, you can look in the section about "optional dependencies" on the github. it lists the different pip install commands you can use for each of those, or an option to install all of them at once!
by doing it using pip, you don't have to manually tell the command line "hey, go to this folder where this script is. now run this script using these options. some of these require another script, and those are located in this other place." instead, it just goes "oh you're asking for the tumblr-backup script? i know where that is! i'll run it for you using the options you've requested! oh you're asking for this option that requires a separate script? i know where that is too!"
as for the app and oauth key, you can follow this tutorial in a doc posted on this post a while back! the actual contents of the application don't matter much; you just need the oauth consumer key provided once you've finished filling out the app information. you'll then go back to your command line and copy-paste in "tumblr-backup --set-api-key API_KEY" where API_KEY is that oauth key you got from the app page.
then you're ready to start backing up! your command line will be "tumblr-backup [options] blog-name", where blog-name is the name of the blog like it says on the tin, and the [options] are the ones listed on the github.
for example, the command i use for this blog is "tumblr-backup -i --tag-index --save-video --save-audio --skip-dns-check --no-reblog nocturne-of-illusions"... "-i" is incremental backups, the whole "i have 100 new posts, just add those to the old backup" function. "--tag-index" creates an index page with all of your tags, for easy sorting! "--save-video", "--save-audio", and "--no-reblog" are what they say they are.
⚠️ (possibly) important! there are two current main issues w backups, but the one that affected me (and therefore i know how to get around) is a dns issue. for any of multiple reasons, your backup might suddenly stall. it might not give a reason, or it might say your internet disconnected. if this happens, try adding "--skip-dns-check" to your options; if the dns check is your issue, this should theoretically solve it.
if you DO have an issue with a first backup, whether it's an error or it stalls, try closing the command window, reopening it, copy-pasting your backup command, and adding "--continue" to your list of options. it'll pick up where it left off. if it gives you any messages, follow the instructions; "--continue" doesn't work well with some commands, like "-i", so you'll want to just remove the offending option until that first backup is done. then you can remove "--continue" and add the other one back on!
there are many cool options to choose from (that i'm gonna go back through now that i have a better idea of what i'm doing ksjdkfjn), so be sure to go through to see if any of them seem useful to you!
#asks#lesbiandiegohargreeves#046txt#hope this is worded well ;; if you need clarification let me know!
2 notes
·
View notes
Text
youtube
People Think It’s Fake" | DeepSeek vs ChatGPT: The Ultimate 2024 Comparison (SEO-Optimized Guide)
The AI wars are heating up, and two giants—DeepSeek and ChatGPT—are battling for dominance. But why do so many users call DeepSeek "fake" while praising ChatGPT? Is it a myth, or is there truth to the claims? In this deep dive, we’ll uncover the facts, debunk myths, and reveal which AI truly reigns supreme. Plus, learn pro SEO tips to help this article outrank competitors on Google!
Chapters
00:00 Introduction - DeepSeek: China’s New AI Innovation
00:15 What is DeepSeek?
00:30 DeepSeek’s Impressive Statistics
00:50 Comparison: DeepSeek vs GPT-4
01:10 Technology Behind DeepSeek
01:30 Impact on AI, Finance, and Trading
01:50 DeepSeek’s Effect on Bitcoin & Trading
02:10 Future of AI with DeepSeek
02:25 Conclusion - The Future is Here!
Why Do People Call DeepSeek "Fake"? (The Truth Revealed)
The Language Barrier Myth
DeepSeek is trained primarily on Chinese-language data, leading to awkward English responses.
Example: A user asked, "Write a poem about New York," and DeepSeek referenced skyscrapers as "giant bamboo shoots."
SEO Keyword: "DeepSeek English accuracy."
Cultural Misunderstandings
DeepSeek’s humor, idioms, and examples cater to Chinese audiences. Global users find this confusing.
ChatGPT, trained on Western data, feels more "relatable" to English speakers.
Lack of Transparency
Unlike OpenAI’s detailed GPT-4 technical report, DeepSeek’s training data and ethics are shrouded in secrecy.
LSI Keyword: "DeepSeek data sources."
Viral "Fail" Videos
TikTok clips show DeepSeek claiming "The Earth is flat" or "Elon Musk invented Bitcoin." Most are outdated or edited—ChatGPT made similar errors in 2022!
DeepSeek vs ChatGPT: The Ultimate 2024 Comparison
1. Language & Creativity
ChatGPT: Wins for English content (blogs, scripts, code).
Strengths: Natural flow, humor, and cultural nuance.
Weakness: Overly cautious (e.g., refuses to write "controversial" topics).
DeepSeek: Best for Chinese markets (e.g., Baidu SEO, WeChat posts).
Strengths: Slang, idioms, and local trends.
Weakness: Struggles with Western metaphors.
SEO Tip: Use keywords like "Best AI for Chinese content" or "DeepSeek Baidu SEO."
2. Technical Abilities
Coding:
ChatGPT: Solves Python/JavaScript errors, writes clean code.
DeepSeek: Better at Alibaba Cloud APIs and Chinese frameworks.
Data Analysis:
Both handle spreadsheets, but DeepSeek integrates with Tencent Docs.
3. Pricing & Accessibility
FeatureDeepSeekChatGPTFree TierUnlimited basic queriesGPT-3.5 onlyPro Plan$10/month (advanced Chinese tools)$20/month (GPT-4 + plugins)APIsCheaper for bulk Chinese tasksGlobal enterprise support
SEO Keyword: "DeepSeek pricing 2024."
Debunking the "Fake AI" Myth: 3 Case Studies
Case Study 1: A Shanghai e-commerce firm used DeepSeek to automate customer service on Taobao, cutting response time by 50%.
Case Study 2: A U.S. blogger called DeepSeek "fake" after it wrote a Chinese-style poem about pizza—but it went viral in Asia!
Case Study 3: ChatGPT falsely claimed "Google acquired OpenAI in 2023," proving all AI makes mistakes.
How to Choose: DeepSeek or ChatGPT?
Pick ChatGPT if:
You need English content, coding help, or global trends.
You value brand recognition and transparency.
Pick DeepSeek if:
You target Chinese audiences or need cost-effective APIs.
You work with platforms like WeChat, Douyin, or Alibaba.
LSI Keyword: "DeepSeek for Chinese marketing."
SEO-Optimized FAQs (Voice Search Ready!)
"Is DeepSeek a scam?" No! It’s a legitimate AI optimized for Chinese-language tasks.
"Can DeepSeek replace ChatGPT?" For Chinese users, yes. For global content, stick with ChatGPT.
"Why does DeepSeek give weird answers?" Cultural gaps and training focus. Use it for specific niches, not general queries.
"Is DeepSeek safe to use?" Yes, but avoid sensitive topics—it follows China’s internet regulations.
Pro Tips to Boost Your Google Ranking
Sprinkle Keywords Naturally: Use "DeepSeek vs ChatGPT" 4–6 times.
Internal Linking: Link to related posts (e.g., "How to Use ChatGPT for SEO").
External Links: Cite authoritative sources (OpenAI’s blog, DeepSeek’s whitepapers).
Mobile Optimization: 60% of users read via phone—use short paragraphs.
Engagement Hooks: Ask readers to comment (e.g., "Which AI do you trust?").
Final Verdict: Why DeepSeek Isn’t Fake (But ChatGPT Isn’t Perfect)
The "fake" label stems from cultural bias and misinformation. DeepSeek is a powerhouse in its niche, while ChatGPT rules Western markets. For SEO success:
Target long-tail keywords like "Is DeepSeek good for Chinese SEO?"
Use schema markup for FAQs and comparisons.
Update content quarterly to stay ahead of AI updates.
🚀 Ready to Dominate Google? Share this article, leave a comment, and watch it climb to #1!
Follow for more AI vs AI battles—because in 2024, knowledge is power! 🔍
#ChatGPT alternatives#ChatGPT features#ChatGPT vs DeepSeek#DeepSeek AI review#DeepSeek vs OpenAI#Generative AI tools#chatbot performance#deepseek ai#future of nlp#deepseek vs chatgpt#deepseek#chatgpt#deepseek r1 vs chatgpt#chatgpt deepseek#deepseek r1#deepseek v3#deepseek china#deepseek r1 ai#deepseek ai model#china deepseek ai#deepseek vs o1#deepseek stock#deepseek r1 live#deepseek vs chatgpt hindi#what is deepseek#deepseek v2#deepseek kya hai#Youtube
2 notes
·
View notes
Text
This day in history
Tomorrow (November 29), I'm at NYC's Strand Books with my novel The Lost Cause, a solarpunk tale of hope and danger that Rebecca Solnit called "completely delightful."
#15yrsago Peak Population: when will population growth stop, why, and how? https://www.alexsteffen.com/peak_population_and_sustainability
#15yrsago James Boyle’s “The Public Domain” — a brilliant copyfighter’s latest book, from a law prof who writes like a comedian https://memex.craphound.com/2008/11/29/james-boyles-the-public-domain-a-brilliant-copyfighters-latest-book-from-a-law-prof-who-writes-like-a-comedian/
#10yrsago NSA and Canadian spooks illegally spied on diplomats at Toronto G20 summit https://www.cbc.ca/news/politics/new-snowden-docs-show-u-s-spied-during-g20-in-toronto-1.2442448
#10yrsago New CC licenses: tighter, shorter, more readable, more global https://creativecommons.org/Version4/
#10yrsago Berlusconi kicked out of Italian senate https://www.theguardian.com/world/2013/nov/27/silvio-berlusconi-ousted-italian-parliament-tax-fraud-conviction
#5yrsago Sennheiser’s headphone drivers covertly changed your computer’s root of trust, leaving you vulnerable to undetectable attacks https://www.bleepingcomputer.com/news/security/sennheiser-headset-software-could-allow-man-in-the-middle-ssl-attacks/
#5yrsago New York City’s municipal debt collectors have forged an unholy alliance with sleazy subprime lenders https://www.bloomberg.com/confessions-of-judgment
#5yrsago Here’s how the Pentagon swindled Congress with $21 trillion worth of undocumented, untraceable, unaccounted for expenditures https://www.thenation.com/article/archive/pentagon-audit-budget-fraud/
#5yrsago The prosecutor who helped Jeffrey Epstein escape justice is now a Trump Cabinet member https://www.miamiherald.com/news/local/article220097825.html
#5yrsago Reddit takes a stand against the EU’s plan to break the internet https://www.redditinc.com/blog/the-eu-copyright-directive-what-redditors-in-europe-need-to-know/
#5yrsago The secret history of science fiction’s women writers: The Future is Female! https://memex.craphound.com/2018/11/29/the-secret-history-of-science-fictions-women-writers-the-future-is-female/
#5yrsago Redaction ineptitude reveals names of Proud Boys’ self-styled new leaders https://splinternews.com/proud-boys-failed-to-redact-their-new-dumb-bylaws-and-a-1830700905
#5yrsago Redaction ineptitude reveals Facebook’s 2012 plan to sell Graph API access to user data for $250,000 https://arstechnica.com/tech-policy/2018/11/facebook-pondered-for-a-time-selling-access-to-user-data/
#5yrsago Google engineer calls for a walkout over China censorship and raises $200K strike fund in hours https://twitter.com/lizthegrey/status/1068208484053856256
#5yrsago Correlates of Trump voting: searches for erectile dysfunction, hair loss, how to get girls, penis enlargement, penis size, steroids, testosterone and Viagra https://www.washingtonpost.com/news/monkey-cage/wp/2018/11/29/how-donald-trump-appeals-to-men-secretly-insecure-about-their-manhood/
#5yrsago Google’s secret project to build a censored Chinese search engine bypassed the company’s own security and privacy teams https://theintercept.com/2018/11/29/google-china-censored-search/
#5yrsago Mozilla pulls a popular paywall circumvention tool from Firefox add-ons store https://web.archive.org/web/20181130141509/https://github.com/iamadamdev/bypass-paywalls-firefox/issues/82
#1yrago The Big Four accounting firms are one (more) scandal away from collapse https://pluralistic.net/2022/11/29/great-andersens-ghost/#mene-mene-bezzle
14 notes
·
View notes
Text
Notion is an all-in-one workspace designed to help individuals and teams organize their work and collaborate efficiently. It combines note-taking, project management, task management, and database capabilities into a single platform. Here is a detailed review of its features and functionalities:
Key Features
Workspace Customization:
Blocks and Pages: Notion’s modular approach allows users to create content using blocks, which can be text, images, tables, checklists, code snippets, and more. These blocks can be arranged on pages that act as the primary workspace.
Templates: Notion offers a variety of pre-built templates for different use cases such as meeting notes, project plans, to-do lists, and knowledge bases. Users can also create and share their own templates.
Note-Taking and Documentation:
Rich Text Editing: Notion supports rich text formatting, allowing users to create detailed and visually appealing documents.
Embedded Content: Users can embed various types of content, such as videos, audio files, and external web content, directly into their pages.
Database Integration: Notes and documents can be linked to databases, enabling dynamic content and relational data management.
Project and Task Management:
Kanban Boards: Notion offers Kanban-style boards for managing tasks and projects visually, providing an intuitive way to track progress.
Gantt Charts and Calendars: Users can create timelines and calendar views to manage deadlines and schedules.
Task Assignments and Reminders: Tasks can be assigned to team members, with due dates and reminders set to ensure timely completion.
Databases:
Relational Databases: Notion supports relational databases, allowing users to link different types of data and create complex workflows.
Views: Data can be viewed in multiple ways, including tables, lists, boards, calendars, and galleries, providing flexibility in how information is presented and accessed.
Filters and Sorting: Advanced filtering and sorting options help users manage and analyze data efficiently.
Collaboration:
Real-Time Collaboration: Multiple users can edit pages simultaneously, with changes reflected in real-time.
Comments and Mentions: Team members can leave comments, tag others, and start discussions directly within the content, facilitating communication.
Permissions and Sharing: Notion allows granular permission settings, enabling users to control access at the page, block, or workspace level.
Integration and API:
Third-Party Integrations: Notion integrates with various external tools such as Slack, Google Drive, and Trello, enhancing its functionality and connectivity.
API Access: The Notion API allows for custom integrations and automation, enabling users to extend the platform’s capabilities. Mobile and Desktop Apps:
Cross-Platform Access: Notion is available on iOS, Android, Windows, and macOS, ensuring users can access their work from any device.
Offline Access: The mobile and desktop apps support offline access, allowing users to work without an internet connection. Pros
Versatile and Flexible: Notion’s block-based system and customizable templates make it highly adaptable to various use cases, from simple note-taking to complex project management.
Unified Workspace: Combining notes, tasks, databases, and collaboration tools into one platform helps streamline workflows and reduce the need for multiple applications.
User-Friendly Interface: The intuitive and visually appealing interface makes it easy for users to navigate and create content.
Strong Collaboration Features: Real-time collaboration, comments, and mentions facilitate team communication and project coordination.
Cons Learning Curve: The extensive features and customization options may require time and effort for new users to fully grasp and utilize effectively.
Performance Issues: With large databases and extensive content, some users may experience performance slowdowns.
Limited Offline Functionality: While offline access is available, some features may be limited or not function as smoothly as they do online.
Complexity for Simple Tasks: For users with straightforward needs, the comprehensive feature set might feel overwhelming or unnecessarily complex.
Notion is a powerful and versatile tool that caters to a wide range of organizational and productivity needs. Its flexibility, comprehensive feature set, and strong collaboration capabilities make it a valuable resource for individuals and teams looking to streamline their workflows. However, the potential learning curve and performance considerations should be kept in mind. Overall, Notion provides significant value for those willing to invest the time to fully leverage its capabilities.
4 notes
·
View notes
Photo
There are so, so, so many reasons why this incredibly fake story is incredibly fake. But I just keep reading the API technobabble and I can't stop laughing.
Mf out here bragging about how not only is their code unreadable and unmaintainable, but also their documentation is unreadable and undiscoverable. The problem that this creative writer has is that they need to be indispensable in this story, but unfortunately an indispensable programmer is uniquely gifted at making themself superfluous as quickly and efficiently as possible in as many contexts as possible.
The "nobody is familiar with Python" part is probably my favorite. Python is one of the easiest programming languages to learn. It's so easy that it's the language that engineers (real engineers like chemical engineers, biomedical engineers, material engineers, not software engineers developers) use to help them automate things in their work. Anyone who is familiar with any other programming language can pick up Python in under an hour. You who are familiar with zero programming languages could probably pick up a decent amount of Python in a month. Try it! It is probably easier than you think.
More reasons why this fake story is fake:
This is posted on r/antiwork. It's one of the subreddits infamous for fake stories of bad bosses
It's a multipart series. One of the tropes of fake Reddit stories is the escalating updates. Creative writers hear an encore and they keep going back for more
It's a bit ambiguous how long this person has been in industry, but given the context of missing multiple children's school functions, I think 10+ years of experience is a cautious estimate. That is long enough in software for you to be a team lead, if not a people manager yourself. This person should be training junior devs. They explicitly say that they aren't
Software is incredibly collaborative. There's no way a manager would turn down an offer to train new devs on the existing tooling
Moreover there's no way the code got push to production without several eyes on it. Most companies do either code reviews or pair programming or both. It makes no sense that zero other people understand what's going on with this code. Unless it's really buggy
The fact that someone tried to use it and it corrupted a CSV file (??) shows that it's actually really buggy. If the software was so good, anyone would be able to run it
That goes double for the documentation being so bad that nobody knows how to read it. The entire purpose of documentation is to explain how code works. You failed at your one job.
If the only documentation is something that's hard to find, that looks bad on OOP for two reasons: 1) Documentation is normally put inline next to the code precisely for the reason that it would be easy to find. Don't want to see what a nightmare their code with no inline docs looks like. 2) Their programming practices are so bad that their other documentation is hard to find. The program should have a file called README that either has all the documentation or tells you where to find all the documentation.
This violates NDA so bad
"Out of compliance" for what? Which regulation? Why do they have a deadline to regain compliance? They should already be suffering whatever fines or consequences or whatever for already being out of compliance. It would make more sense if they were at risk of being out of compliance if they didn't implement XYZ by January
There's a lot of weird wording here that indicates a lack of familiarity with software: "complex API", "documentation library", "single threaded". That's not how we use those terms
If you're a software developer for a company the size of Disney (ABC's parent) then what OOP asked for is your starting salary straight out of undergrad. Def not a raise for a senior engineer who's been in industry 10+ years. Def not more than their manager is making.
At a company that size, your direct manager has no ability to decide what the terms of your hiring agreement would be. Def not over text. It would need to go through HR and probably legal as well
"Legal checked the contract and there's a clause stating" lmao get outta here!
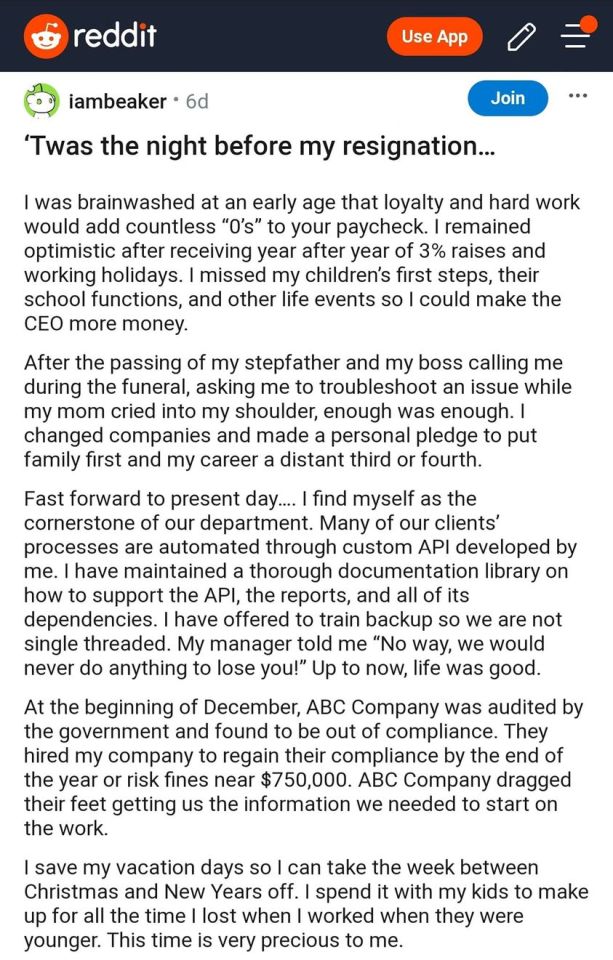
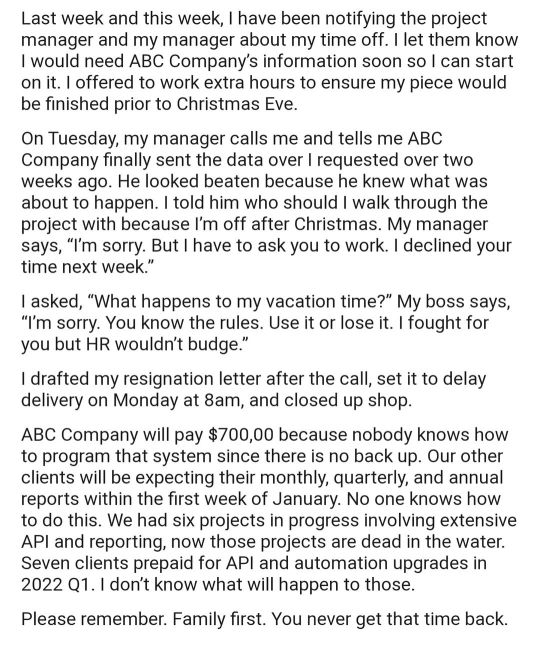
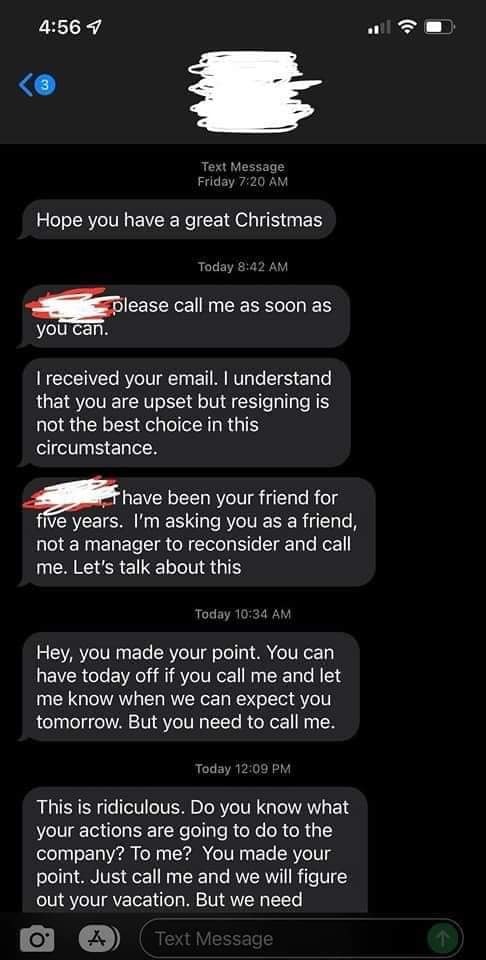
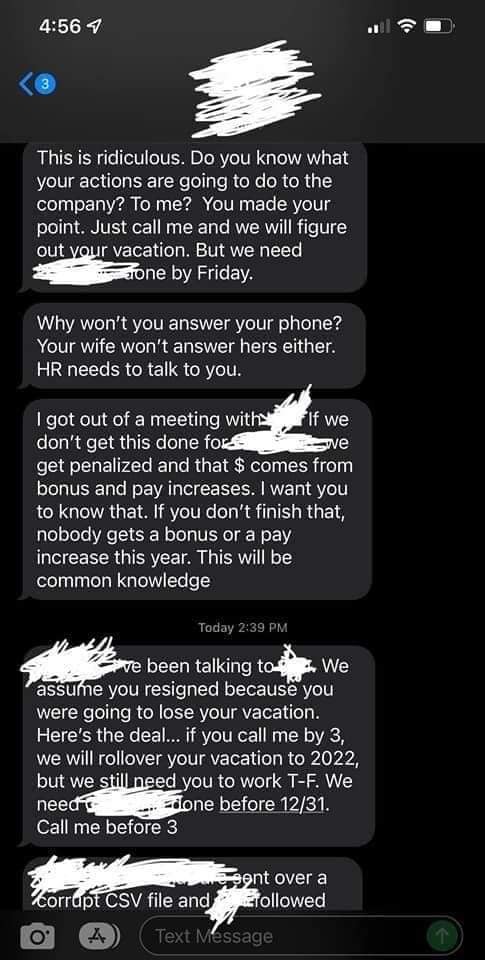
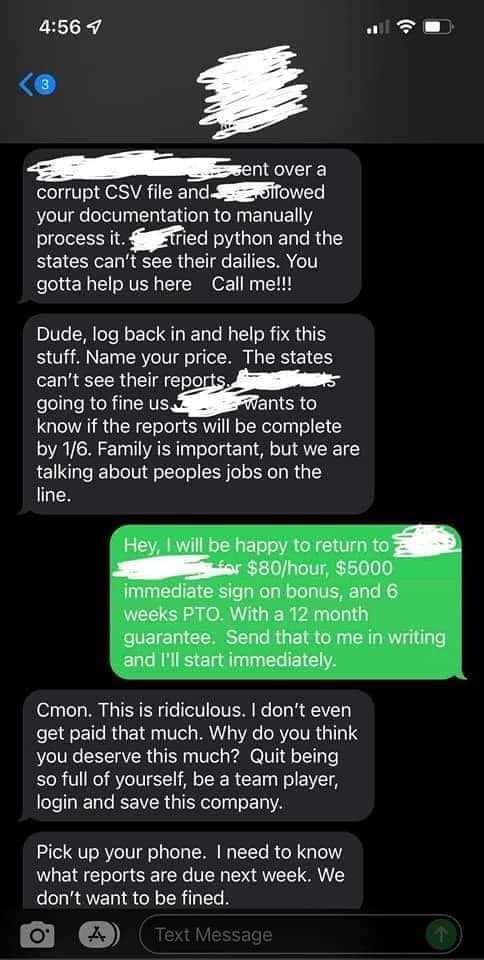
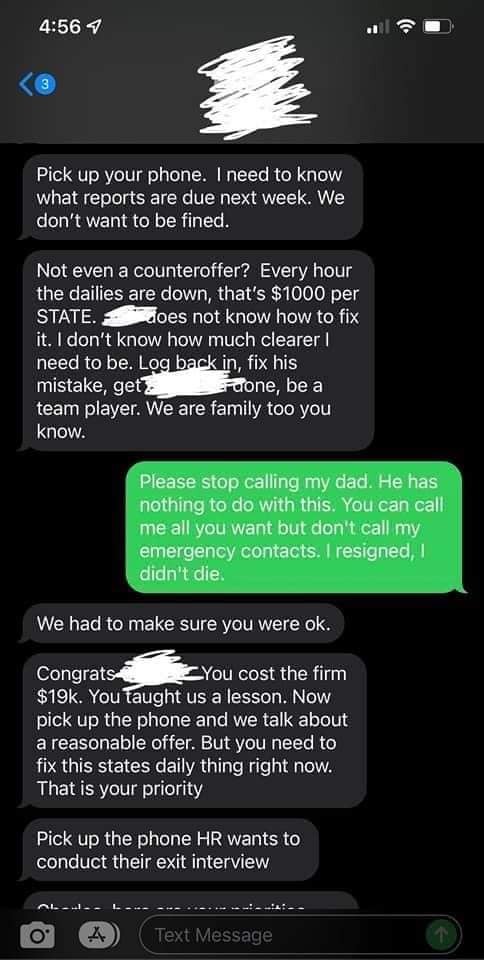
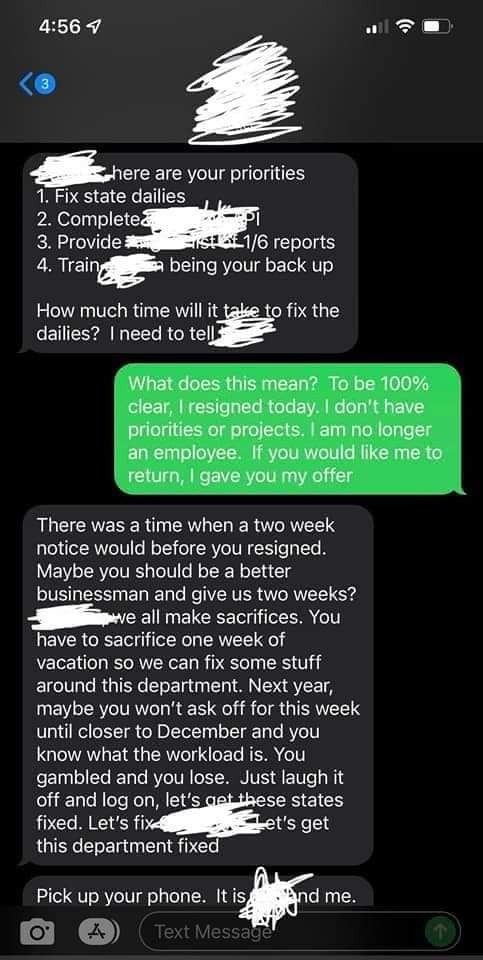
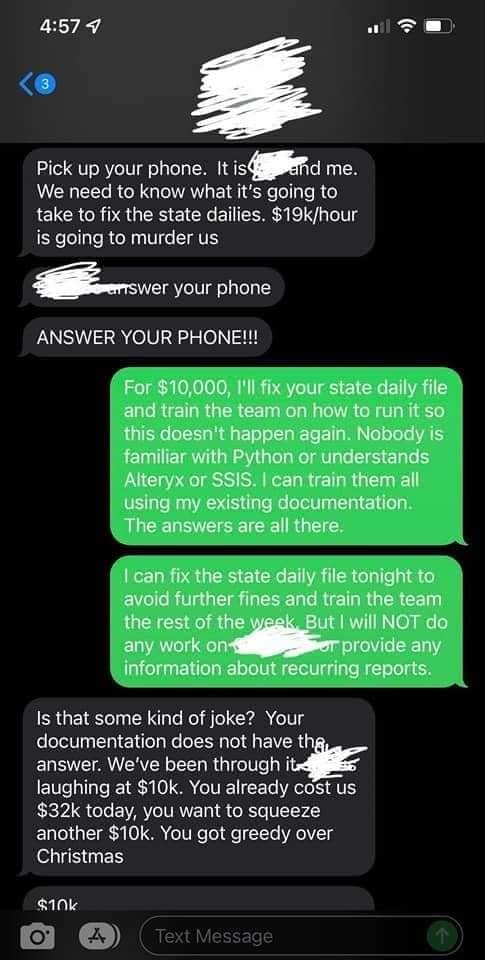
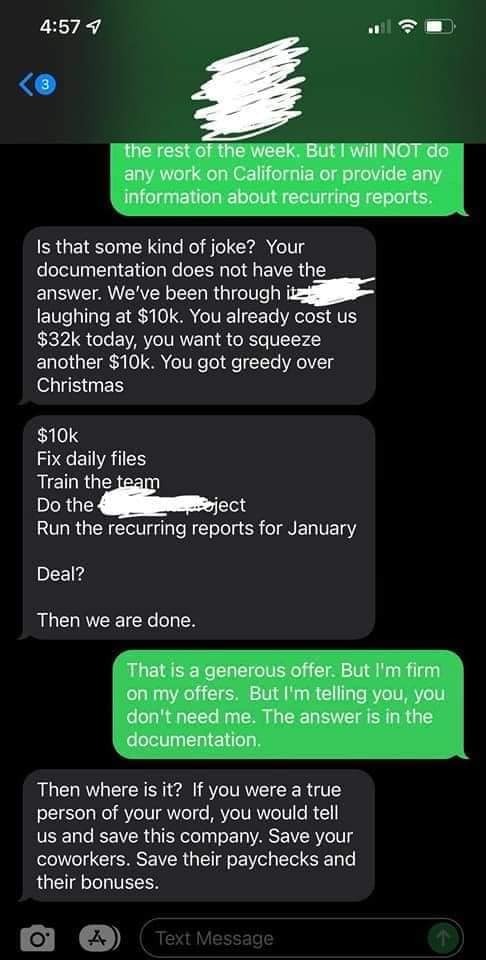
#Reddit creative writing exercise#codeblr#progblr#You can learn almost any programming language for free online if you first learn the essential software engineer skill of#googling what you need#Im hesitant to pick a specific python tutorial because I havent needed a python tutorial in over a decade#I dont know which of the modern ones are good#Freecodecamp seems to be fine for JavaScript#Similar but imo superior language to start with
247K notes
·
View notes
Text

The journey to mastering full-stack development often begins with choosing the right combination of technologies. For many developers, Python, Vue.js, and PostgreSQL form a powerful and versatile stack. This trio provides a robust backend, a dynamic front end, and a reliable database. Whether you’re a budding developer enrolled in an FSD Python course or a seasoned programmer exploring new frameworks, this guide will provide the foundational steps to set up a full-stack project.
Why Choose Python, Vue.js, and PostgreSQL?
Python: Known for its simplicity and versatility, Python is a favorite for backend development. Frameworks like Flask and Django make it easy to build scalable APIs and web applications.
Vue.js: Vue.js is a progressive JavaScript framework for building user interfaces. Its simplicity, flexibility, and reactive components make it ideal for crafting modern, dynamic frontends.
PostgreSQL: As one of the most advanced open-source relational database systems, PostgreSQL is renowned for its scalability, reliability, and extensive feature set.
Step 1: Plan Your Project Structure
In a standard full-stack project using Python, Vue.js, and PostgreSQL, the project structure is typically organized into several main directories. The backend directory contains the Python-based backend code, which could use frameworks like Django or Flask. The frontend directory holds the Vue.js frontend code and is responsible for the user interface. The database directory includes scripts and configuration files for setting up and managing the PostgreSQL database. The environment directory stores configuration files, such as environment variables and deployment settings. Finally, the docs directory is dedicated to documentation and notes related to the project. This structure ensures clarity and separation of concerns, making the project easier to manage and scale.
Step 2: Set Up the Backend with Python
Choose a Python Framework:
Flask: Lightweight and flexible for simple APIs.
Django: A full-fledged web framework with built-in features for authentication, ORM, and more.
Create the Virtual Environment:
Using virtual environments ensures project dependencies remain isolated, a key concept covered in any Python FSD curriculum.
Install Necessary Packages:
For Flask: Flask, Flask-Cors, and SQLAlchemy.
For Django, use core Django packages and DRF (Django Rest Framework) for API handling.
API Design:
Develop RESTful endpoints to handle CRUD operations.
Implement authentication mechanisms like JWT for user management.
Step 3: Develop the Frontend with Vue.js
Set Up the Vue.js Environment:
Use Vue CLI to scaffold your application. This creates a starter project with essential configurations.
Design Components:
Break down your application into modular components (e.g., header, footer, forms).
Use Vue’s data-binding and state management to handle user interactions.
API Integration:
Axios or Fetch can be used to connect the Vue.js frontend with your Python backend.
Styling:
Choose between frameworks like Vuetify, Tailwind CSS, or Bootstrap to style your application.
Step 4: Configure the PostgreSQL Database
Install PostgreSQL:
Ensure PostgreSQL is installed and running on your system. Tools like pgAdmin simplify database management.
Set Up the Database:
Define your schema and relationships. Django’s ORM or Flask’s SQLAlchemy can streamline this process.
Database Connection:
Configure your backend to connect to PostgreSQL using libraries like psycopg2.
Migrations:
Use migration tools provided by your framework (e.g., Django’s migrate command) to keep your database schema synchronized.
Step 5: Link the Frontend and Backend
Cross-Origin Resource Sharing (CORS):
Enable CORS in your backend to allow API requests from your frontend.
Environment Variables:
Use .env files to securely manage API keys, database credentials, and other sensitive information.
Serve the Frontend:
Deploy your Vue.js application and configure the backend to serve the frontend (or use a separate web server).
Step 6: Deployment
Containerization:
Use Docker to containerize your application for consistent deployment across environments.
Hosting Platforms:
For the backend, consider platforms like Heroku, AWS, or Azure.
For the front end, platforms like Vercel or Netlify are excellent choices.
Database Hosting:
Host PostgreSQL on cloud platforms like AWS RDS or DigitalOcean for better scalability.
Conclusion
Setting up a full-stack project with Python, Vue.js, and PostgreSQL may seem complex initially, but with proper guidance and practice, it becomes a manageable and rewarding process. Whether you’re enrolled in a Python Full Stack Development Course or self-learning, this stack equips you with the tools to build modern, scalable web applications.
Each step in this guide provides a foundation for exploring and implementing advanced features like authentication, real-time updates, or cloud deployment. With persistence and curiosity, you’ll be well on your way to mastering full-stack development.
Contact Us:
Phone
+917338811773
+918925903732
Email: [email protected]
0 notes
Text
🔍 1. It makes your code understandable (even to your future self)
You may think, "I’ll remember how this works." Spoiler alert: you won’t. Not after 6 months or even 2 weeks.
Clear documentation saves you from hours of code archaeology.
💡 Highlight: Well-documented code is self-explanatory and saves future you from pulling your hair out.
🧑🤝🧑 2. Enables Team Collaboration
Onboarding new devs? Good documentation = smoother, faster ramp-up.
If a teammate needs to work on your feature, they won’t need to Slack you 24/7.
💡 Highlight: Code is read more than it’s written — help others read it.
🔄 3. Supports Maintenance & Debugging
Bug fixing, upgrading dependencies, or adding features is way easier when you know what each part is doing and why.
Helps prevent the "WTF is this doing?" moments that cost time and energy.
💡 Highlight: Documentation acts as your map through complex logic.
🧪 4. Helps with Testing and QA
QA teams rely on docs to understand expected behaviors, edge cases, and how to reproduce bugs.
Testers can’t test what they don’t understand.
💡 Highlight: Documentation bridges the gap between dev and test.
📄 5. Boosts API and User Adoption
If you’re building an API, SDK, or tool for others: Good docs = people actually using it. Bad docs = abandoned GitHub repo.
💡 Highlight: Documentation is your best marketing tool for developers.
🧠 6. Documents the why, not just the what
Comments in code tell you what is happening. Documentation tells you why the decision was made.
That context is gold for future design decisions or debugging.
💡 Highlight: The “why” helps avoid repeating past mistakes.
✍️ What Should You Document?
High-level architecture
Setup and installation instructions
API references
Examples and use-cases
Change logs / version history
Known limitations or issues
Design decisions and trade-offs
🚀 In Short:
No documentation = Technical debt Good documentation = Dev superpower
"Code tells you how, documentation tells you why." – Someone who didn’t want to suffer in silence 😅
Want a quick template or checklist for good documentation?
🔍 1. It makes your code understandable (even to your future self)
You may think, "I’ll remember how this works." Spoiler alert: you won’t. Not after 6 months or even 2 weeks.
Clear documentation saves you from hours of code archaeology.
💡 Highlight: Well-documented code is self-explanatory and saves future you from pulling your hair out.
🧑🤝🧑 2. Enables Team Collaboration
Onboarding new devs? Good documentation = smoother, faster ramp-up.
If a teammate needs to work on your feature, they won’t need to Slack you 24/7.
💡 Highlight: Code is read more than it’s written — help others read it.
🔄 3. Supports Maintenance & Debugging
Bug fixing, upgrading dependencies, or adding features is way easier when you know what each part is doing and why.
Helps prevent the "WTF is this doing?" moments that cost time and energy.
💡 Highlight: Documentation acts as your map through complex logic.
🧪 4. Helps with Testing and QA
QA teams rely on docs to understand expected behaviors, edge cases, and how to reproduce bugs.
Testers can’t test what they don’t understand.
💡 Highlight: Documentation bridges the gap between dev and test.
📄 5. Boosts API and User Adoption
If you’re building an API, SDK, or tool for others: Good docs = people actually using it. Bad docs = abandoned GitHub repo.
💡 Highlight: Documentation is your best marketing tool for developers.
🧠 6. Documents the why, not just the what
Comments in code tell you what is happening. Documentation tells you why the decision was made.
That context is gold for future design decisions or debugging.
💡 Highlight: The “why” helps avoid repeating past mistakes.
✍️ What Should You Document?
High-level architecture
Setup and installation instructions
API references
Examples and use-cases
Change logs / version history
Known limitations or issues
Design decisions and trade-offs
🚀 In Short:
No documentation = Technical debt Good documentation = Dev superpower
"Code tells you how, documentation tells you why." – Someone who didn’t want to suffer in silence 😅
Want a quick template or checklist for good documentation?
0 notes
Text
The Essential Web Development Roadmap for 2025
In today’s digital world, web development is a highly sought-after skill. Whether you're aiming to build your own projects, land a tech job, or freelance for clients, understanding the web development roadmap is crucial for your success. A clear, step-by-step path not only makes learning more manageable but also ensures you build strong, competitive skills.
1. Start with the Basics
Every journey begins with mastering the fundamentals. The first step on any web development roadmap is learning HTML, CSS, and JavaScript. HTML structures your webpage, CSS styles it, and JavaScript makes it interactive. Without a solid understanding of these core technologies, progressing to more complex tasks will be difficult.
Online resources like freeCodeCamp, W3Schools, or MDN Web Docs are great starting points. Focus on creating simple projects like a personal portfolio or a basic landing page to apply what you learn.
2. Dive into Frontend Development
Once you are comfortable with the basics, it’s time to explore frontend frameworks and libraries. Popular choices include React.js, Vue.js, and Angular. React is particularly favored by employers and has a huge community, making it an excellent choice for beginners.
Along with frameworks, the web development roadmap suggests learning about version control systems like Git and GitHub. These tools allow you to collaborate with others and manage your code efficiently.
3. Understand Backend Development
To become a full-stack developer or simply to understand how web applications work behind the scenes, learning backend development is essential. Technologies like Node.js, Express.js, and Databases (like MongoDB or PostgreSQL) should be on your radar.
A typical web development roadmap will guide you through setting up servers, handling APIs, and managing databases. Backend skills enable you to create complete applications rather than just static pages.
4. Explore Advanced Topics
Once you are comfortable with frontend and backend basics, move on to advanced topics. Learn about authentication and authorization, RESTful APIs, GraphQL, WebSockets, and cloud platforms like AWS or Vercel. Modern web apps demand strong security and scalability, making these skills invaluable.
Also, understanding DevOps practices, CI/CD pipelines, and testing will give you a major advantage in the professional world.
5. Build, Build, Build
The best way to solidify your learning is by building real-world projects. A good web development roadmap always emphasizes project-based learning. Whether it’s an e-commerce site, a social media app, or a blog platform, practical experience showcases your skills and prepares you for job interviews.
A well-structured web development roadmap acts like a GPS for your tech career. Follow it step-by-step, stay consistent, and you’ll find yourself creating amazing digital experiences sooner than you think.
0 notes
Text
Global Sex Toys Market Size, Share, and Forecast: Vibrators, Dildos, and Emerging Segments
The global sex toys market was valued at USD 35.2 billion in 2023 and is projected to grow at a compound annual growth rate (CAGR) of 8.69% from 2024 to 2030. The market's growth is primarily driven by the increasing use of sex toy products and the growing awareness of sexual health among adolescents and young adults. As societal attitudes towards sex and intimacy continue to evolve, more individuals are exploring sexual wellness products as a means to enhance their sexual experiences.
A key factor contributing to this growth is the rising penetration of Artificial Intelligence (AI) within the industry. The introduction of AI-powered products such as sex robots, chatbots, and interactive dolls is transforming the landscape of sex toys. These innovations are helping to bridge the gap between traditional sex toys and SexTech products, which offer more personalized, interactive, and immersive experiences. AI-powered sex toys can now be controlled remotely and even sync with apps or audio erotica to tailor the user experience based on emotional and physical responses. For instance, in January 2023, Lovense integrated the ChatGPT API to launch the Lovense ChatGPT Pleasure Companion, a smart device that enhances intimacy by synchronizing its toys with an app, enabling a more responsive and customized experience.
The use of sex toys is also gaining momentum due to their medical benefits, as they are recognized for helping with neurological conditions such as lack of arousal, addressing menopausal symptoms, and improving sexual health. For men, these products offer solutions for conditions like premature ejaculation, low libido, and erectile dysfunction. The growing acceptance of sex toys as therapeutic tools is expected to further fuel the market’s expansion over the forecast period.
Regional Insights
North America held the largest revenue share of 33.24% in 2023, largely due to the presence of numerous manufacturers and retailers offering easy access to a wide range of sex toys. Companies like Doc Johnson have manufacturing facilities in the U.S., producing over 75% of their products domestically. These companies are also heavily involved in research and development (R&D), which drives innovation and expands product offerings in the market.
Canada Sex Toys Market Trends:
The Canada sex toys market is expected to see significant growth during the forecast period, driven by increasing consumer demand for adult toys. One of the major trends contributing to this growth is the rise of online retailers, which are popular in Canada due to the demand for discreet delivery and exclusive offers. Prominent online retailers such as PinkCherry, NaughtyNorth, and Fantasia have established a strong presence, while adult stores like Sex Toys Canada, Stag Shop, Hush Canada, Fantasy Factory Adult Store, and Aren’t We Naughty continue to serve the growing market.
Europe Sex Toys Market Trends:
In Europe, countries such as Germany, Italy, France, the UK, Denmark, and Belgium are leading contributors to the regional market. In these countries, traditional sex toys, such as vibrators and dildos, remain the most popular products. The presence of well-established brands like LELO, Lovehoney, Durex, and Fun Factory has positioned Europe as the second-largest market in the world by 2023. These companies have established a solid reputation in Europe by catering to the diverse needs and preferences of the European community, ensuring the continued growth and expansion of the market in the region.
Key Sex Toys Companies:
The following are the leading companies in the sex toys market. These companies collectively hold the largest market share and dictate industry trends.
Church & Dwight Co., Inc.
Reckitt Benckiser Group plc
LELO
LifeStyles Healthcare Pte Ltd
Doc Johnson Enterprises
Lovehoney Group Ltd
BMS Factory
PinkCherry
Tenga Co., Ltd.
Fun Factory
We-Vibe
Recent Developments
In May 2023, Doc Johnson Enterprises launched new signature Cocks dildos molded from performers Owen Grey and Small Hands.
In September 2023, LELO launched a new collection of next-generation stimulation massagers, ENIGMA Wave.
Order a free sample PDF of the Market Intelligence Study, published by Grand View Research.
0 notes
Text
API Documentation Made Simple with Ai
Simplify API documentation with AI-powered tools. Create clear, accurate, and developer-friendly docs faster than ever.
0 notes
Text
Effective API Documentation Strategies (With an API Documentation Example)
Effective API documentation is the backbone of a developer-friendly product. Whether you're offering a RESTful service, GraphQL endpoint, or SDK, your documentation must be clear, comprehensive, and easy to navigate. Poor documentation can discourage developers, increase support requests, and slow down adoption. Here are key strategies to ensure your API documentation is both useful and user-friendly.
1. Know Your Audience
Understanding who will use your API is the first step. Are they beginner developers or experienced backend engineers? Tailor your tone and examples accordingly. Use plain language for newer audiences and more technical depth for advanced users.
2. Organize Content Logically
Structure your documentation for ease of navigation. A typical layout might include:
Overview – What the API does
Authentication – How to get access
Endpoints – Core functionality
Examples – Real-world use cases
Errors – How to interpret error messages
SDKs & Tools – Any helper libraries available
Using a sidebar or table of contents can help users quickly jump to the relevant section.
3. Use Interactive Elements
Interactive documentation improves the developer experience. Tools like Swagger (OpenAPI), Postman, and Redoc allow developers to test endpoints directly in the browser. This can dramatically reduce the time it takes to understand and use the API.
4. Provide Clear Code Examples
Examples are often the most viewed part of API docs. Include request and response examples in multiple languages if possible (e.g., cURL, Python, JavaScript). Annotate them to explain each part of the request and what to expect from the response.
5. Be Honest and Upfront About Limitations
Transparency about rate limits, known issues, or incomplete features helps build trust. Include a changelog or release notes section so developers can track updates.
6. Keep It Updated
Outdated documentation is worse than no documentation. Set up version control and a documentation workflow that updates the docs with each API change. Use automation when possible.
✅ API Documentation Example (REST API – Notes Service)
Base URL: https://api.notesapp.io/v1
🔐 Authentication
All requests must include a bearer token:
http
CopyEdit
Authorization: Bearer YOUR_API_TOKEN
📄 GET /notes
Description: Retrieve a list of notes.
Request:
http
CopyEdit
GET /notes Host: api.notesapp.io Authorization: Bearer YOUR_API_TOKEN
Response:
json
CopyEdit
[ { "id": "note_123", "title": "Meeting Notes", "content": "Discuss Q2 goals...", "created_at": "2025-04-15T09:00:00Z" } ]
📝 POST /notes
Description: Create a new note.
Request:
http
CopyEdit
POST /notes Content-Type: application/json Authorization: Bearer YOUR_API_TOKEN { "title": "New Note", "content": "This is a test note" }
Response:
json
CopyEdit
{ "id": "note_456", "title": "New Note", "content": "This is a test note", "created_at": "2025-04-17T14:32:00Z" }
Conclusion
Great API documentation empowers developers, reduces friction, and enhances your product’s adoption. By combining clear structure, real-world examples, interactive elements, and ongoing maintenance, you create a reference that developers trust—and love to use.
0 notes
Text
Going to start developing this from Tommorow this will be my side project
**File Name**: CodeZap_Project_Specification.md
# CodeZap: A Collaborative Coding Ecosystem
**Tagline**: Code, Collaborate, Learn, and Share – All in One Place.
**Date**: April 15, 2025
## 1. Project Overview
**CodeZap** is a web and mobile platform designed to empower developers, students, educators, and teams to write, debug, review, store, and share code seamlessly. It integrates a powerful code editor with real-time collaboration tools (chat, video calls, live editing), gamified learning (quizzes, challenges), and a marketplace for code snippets, templates, and services. The platform aims to be a one-stop hub for coding, learning, and networking, catering to beginners, professionals, and enterprises.
**Vision**: To create an inclusive, engaging, and scalable ecosystem where users can grow their coding skills, collaborate globally, and monetize their expertise.
**Mission**: Simplify the coding experience by combining best-in-class tools, fostering community, and leveraging AI to enhance productivity and learning.
## 2. Core Features
### 2.1 Code Editor & Debugging
- **Real-Time Editor**: Browser-based IDE supporting 50+ languages (Python, JavaScript, C++, etc.) with syntax highlighting, auto-completion, and themes inspired by VS Code.
- **AI-Powered Debugging**: AI assistant suggests fixes, explains errors, and optimizes code in real time, reducing debugging time.
- **Version Control**: Built-in Git-like system with visual diffs, branching, and rollback for individual or team projects.
- **Environment Support**: Cloud-based execution with customizable environments (e.g., Node.js, Django, TensorFlow), eliminating local setup needs.
### 2.2 Collaboration Tools
- **Live Coding**: Multi-user editing with cursor tracking and role-based permissions (editor, viewer), akin to Google Docs for code.
- **Video & Voice Meet**: Integrated video calls and screen-sharing for pair programming or discussions, optimized for low latency.
- **Chat System**: Real-time chat with code snippet sharing, markdown support, and threaded replies. Includes project-specific or topic-based channels.
- **Whiteboard Integration**: Digital whiteboard for sketching algorithms, flowcharts, or architecture diagrams during brainstorming.
### 2.3 Code Review & Marking
- **Peer Review System**: Inline commenting and scoring for readability and efficiency. Gamified with badges for quality feedback.
- **Automated Linting**: Integration with tools like ESLint or Pylint to flag style issues or bugs before manual review.
- **Teacher Mode**: Educators can create assignments, automate grading, and annotate submissions directly in the editor.
### 2.4 Storage & Sharing
- **Cloud Storage**: Unlimited storage with tagging, search, and folder organization. Options for private, public, or team-shared repositories.
- **Shareable Links**: Generate links or QR codes for projects, snippets, or demos, with customizable access and expiration settings.
- **Portfolio Integration**: Curate public projects into a portfolio page, exportable as a website or PDF for job applications.
- **Fork & Remix**: Fork public projects, remix them, and share new versions to foster community-driven development.
### 2.5 Learning & Gamification
- **Quiz & Challenge Mode**: Interactive coding quizzes (“Fix this bug,” “Optimize this function”) with difficulty levels, timers, and leaderboards.
- **Learning Paths**: Curated tutorials (e.g., “Build a REST API in Flask”) with coding tasks, videos, and quizzes. Potential partnerships with freeCodeCamp.
- **Achievements & Rewards**: Badges for milestones (“100 Bugs Fixed,” “Top Reviewer”), unlockable themes, or premium features.
- **Hackathon Hub**: Host virtual coding competitions with real-time leaderboards, team formation, and prize pools.
### 2.6 Marketplace & Monetization
- **Code Store**: Marketplace for selling or sharing snippets, templates, or projects (e.g., React components, Python scripts). Includes ratings and previews.
- **Freelance Connect**: Hire or offer coding services (e.g., “Debug your app for $50”) with secure payment integration.
- **Premium Subscriptions**: Tiers for advanced debugging, private repos, or exclusive tutorials, following a freemium model.
- **Ad Space**: Non-intrusive ads for coding tools, courses, or conferences, targeting niche audiences.
### 2.7 Community & Networking
- **Forums & Groups**: Topic-based forums (e.g., “Web Dev,” “AI/ML”) for Q&A, showcases, or mentorship.
- **Events Calendar**: Promote coding meetups, webinars, or workshops with RSVP and virtual attendance options.
- **Profile System**: Rich profiles with skills, projects, badges, and GitHub/LinkedIn links. Follow/friend system for networking.
## 3. Enhanced Features for Scalability & Impact
- **Cross-Platform Sync**: Seamless experience across web, iOS, Android, and desktop apps. Offline mode with auto-sync.
- **Accessibility**: Screen reader support, keyboard navigation, and dyslexia-friendly fonts for inclusivity.
- **Enterprise Features**: Team management, SSO, analytics dashboards (e.g., productivity metrics), and compliance with SOC 2/GDPR.
- **Open Source Integration**: Import/export from GitHub, GitLab, or Bitbucket. Contribute to open-source projects directly.
- **AI Mentor**: AI chatbot guides beginners, suggests projects, or explains concepts beyond debugging.
- **Localization**: Multi-language UI and tutorials to reach global users, especially in non-English regions.
- **User Analytics**: Insights like “Most used languages,” “Debugging success rate,” or “Collaboration hours” for self-improvement.
## 4. Target Audience
- **Students & Beginners**: Learn coding through tutorials, quizzes, and peer support.
- **Professional Developers**: Collaborate, debug efficiently, and showcase portfolios.
- **Educators**: Create assignments, grade submissions, and host bootcamps.
- **Teams & Startups**: Manage projects, review code, and hire freelancers.
- **Hobbyists**: Share side projects, join hackathons, and engage with communities.
## 5. Unique Selling Points (USPs)
- **All-in-One**: Combines coding, debugging, collaboration, learning, and networking, reducing tool fatigue.
- **Gamified Experience**: Qu māizzes, badges, and leaderboards engage users of all levels.
- **Community-Driven**: Marketplace and forums foster learning, earning, and connection.
- **AI Edge**: Advanced AI for debugging and mentorship sets it apart from Replit or CodePen.
- **Scalability**: Tailored features for solo coders, teams, and classrooms.
## 6. Tech Stack
- **Frontend**: React.js (web), React Native (mobile) for responsive, cross-platform UI.
- **Backend**: Node.js with Express or Django; GraphQL for flexible APIs.
- **Database**: PostgreSQL (structured data); MongoDB (flexible code storage).
- **Real-Time Features**: WebSocket or Firebase for live editing, chat, and video.
- **Cloud Execution**: Docker + Kubernetes for sandboxed execution; AWS/GCP for hosting.
- **AI Integration**: xAI API (if available) or CodeLlama for debugging and suggestions.
- **Version Control**: Git-based system with Redis for caching diffs.
## 7. Monetization Strategy
- **Freemium Model**: Free access to basic editor, storage, and community; premium for advanced debugging, private repos, or marketplace access.
- **Marketplace Fees**: 10% commission on code or service sales.
- **Enterprise Plans**: Charge companies for team accounts with analytics and support.
- **Sponsorships**: Partner with bootcamps, tool providers (e.g., JetBrains), or cloud platforms for sponsored challenges or ads.
- **Certifications**: Paid certifications for completing learning paths, validated by industry partners.
For pricing inquiries:
- SuperGrok subscriptions: Redirect to https://x.ai/grok.
- x.com premium subscriptions: Redirect to https://help.x.com/en/using-x/x-premium.
- API services: Redirect to https://x.ai/api.
## 8. Potential Challenges & Solutions
- **Competition** (Replit, GitHub, LeetCode):
**Solution**: Differentiate with collaboration, gamification, and marketplace. Prioritize user experience and community.
- **Code Security** (e.g., malicious code):
**Solution**: Sandboxed environments, automated malware scanning, and strict moderation for public content.
- **Server Costs** (real-time features, cloud execution):
**Solution**: Serverless architecture and tiered pricing to offset costs.
- **User Retention**:
**Solution**: Rewards, certifications, and community features. Unique offerings like portfolio export or freelance opportunities.
## 9. Development Roadmap
### Phase 1: MVP (3-6 Months)
- Basic code editor with debugging and storage.
- Real-time collaboration (live editing, chat).
- Simple quiz feature and user profiles.
- Web platform launch with mobile responsiveness.
### Phase 2: Expansion (6-12 Months)
- Video calls, whiteboard, and code review tools.
- Marketplace and portfolio features.
- iOS/Android app launch.
- AI debugger and basic learning paths.
### Phase 3: Maturation (12-18 Months)
- Enterprise features and certifications.
- Expanded gamification (leaderboards, hackathons).
- Localization and accessibility support.
- Partnerships with coding schools or companies.
### Phase 4: Global Scale (18+ Months)
- Regional servers for low latency.
- Optional AR/VR coding environments.
- Integrations with IoT or blockchain (e.g., smart contract debugging).
## 10. Why CodeZap Matters
CodeZap addresses fragmented tools, uninspiring learning paths, and collaboration barriers. By blending IDEs (VS Code), collaboration platforms (Slack), and learning sites (LeetCode), it offers a seamless experience. It empowers users to code, grow, connect, and monetize skills in a dynamic ecosystem, making coding accessible and rewarding for all.
1 note
·
View note