#C Programming Language
Explore tagged Tumblr posts
Text
What is C? A Beginner’s Guide to C Language and C Programming
what C is, why it’s important, and how you can get started with C programming When it comes to programming languages, C holds a special place as one of the most popular and foundational languages in the software development world.
Whether you’re just starting your coding journey or want to build a strong base for advanced programming, understanding C is essential. Let’s dive into what C is, why…
#c programming#programming#c programming language#c programming for beginners#c (programming language)#c programming tutorial#programming in c#c programming course#programming language#c programming full course#programming for beginners#programming fundamentals#programming hero#programming language (software genre)#programming memes#c language programming#programiz c programming#c programming language tutorial#learn c programming#c programming basics
2 notes
·
View notes
Text
A recent paper for C2y has made it so that generic selection expressions can match on a type instead of an expression, and doing this will not perform lvalue conversions. This means that an array-length macro that performs typeċḣecking can replace the confusion it induces with nested generic selection expressions with an entirely new kind of confusion induced by nested typeofs.
#C#C2y#C programming#The C programming language#C programming language#Programming#Computers#Technology#I'm always tempted to pluralized “typeof” as “typesof” instead of “typeofs”.
2 notes
·
View notes
Text
C Tutorial
0 notes
Text
Java in Depth, become a complete Java Engineer

tart your journey into the world of Computer Science with a beginner-to-advanced course covering core concepts like Programming (C, C++, Python), Data Structures, Algorithms, Operating Systems, Database Management, Computer Networks, and emerging fields like Artificial Intelligence and Machine Learning.
This course is ideal for students aiming to build a solid foundation in software development, data analytics, or IT careers. With hands-on learning and expert guidance, it prepares you for real-world challenges in tech.
Students from Uttam Nagar and Yamuna Vihar looking to gain practical skills in computer science can benefit greatly from this industry-relevant training program. Join now to turn your tech passion into a profession!
Visit Attitude Academy
📚 Learn Computer Science: Attitude Academy
📍 Visit Us: Yamuna Vihar | Uttam Nagar
📞 Call: +91 9654382235
🌐 Website: www.attitudetallyacademy.com
📩 Email: [email protected]
📸 Follow us on: attitudeacademy4u
#computer science course in yamuna vihar#computer science course in uttam nagar#c programming language#C++ programmin course#python training in yamuna vihar#java script course in uttam nagar#web development course
0 notes
Text
C is a high level language and structured programming language that was originally developed by Dennis M. Ritchie to develop the UNIX operating system at Bell Labs. While C++ contains object oriented concepts which has so many advantages as compared to C lang.
#TCCI - Tririd Computer Coaching Institute#computer class in Ahmedabad#computer classes in bopal Ahmedabad#C programming language#C++ coaching bopal Ahmedabad
0 notes
Text
Learn Best C Programming Language Courses
C Language is one of the most basic or beginner C Programming Languages Course, C Language has had a direct bearing on most of the programming languages that have evolved out of it, and one must at least have an understanding of what is C Language in order to be able to boss any language around. As getting complete knowledge of programming languages is very crucial and essential to enter the world of development which is considered to be the most competitive ad prestigious profession and high paying job in today’s world. So to begin the journey of learning C, you can do so with some of the best courses.
Takeoff upskill today we are going to discuss the 10 Best C Programming Courses for Beginners: these are the best courses offering you good content for learning and at the meantime issued a certificate after completion of the course. To summarize, let’s consider each of them in detail, and perhaps you will decide which method is more suitable for you.
Takeoff upskill should first read some of the C programming language information before explaining the best courses to take for C programming.
Introduction to C Programming:
An overview of C language and where it fits.
Environmental planning (IDEs- for instance VS Code, Dev-C++, etc.).
The bare structure of a C program includes the following categories:
The first process that you need to go through when writing a “Hello World” program involves writing your first program and compiling it.
Variables and Data Types:
Knowledge regarding the different variable types that are available like integers, floating-point numbers, character, etc.
Declaring and initializing variables.
Basic arithmetic operations.
Control Flow:
Conditional statements (if-else, switch-case).
Control of experiments through looping structures such as for, while, do while.
Annotation of code using breaks and continues.
Functions:
Functions and their significance for calculating regularities.
Function declaration and definition.
Passing arguments to functions.
Returning values from functions.
Arrays and Strings:
Declaring and initializing arrays.
Accessing array elements.
Input-output (printf, scan, etc.), string manipulations (strcpy, strcat, strlen, etc.)
Multi-dimensional arrays.
Pointers:
What pointers are, why there are used, and how they and memory addresses?
Pointer arithmetic.
Pointers and arrays.
Malloc, calloc, realloc for dynamic memory allocation and free to free the memory space allocated dynamically.
Structures and Unions:
Defining and using structures.
Accessing structure members.
Nested structures.
Introduction to unions.
File Handling:
Reading and writing files from C (structuring, opening, accessing and closing).
Position(s) of the file (open, read-only, write-only or append)
Different methods, which should be implemented for error handling while processing the files.
Preprocessor Directives:
Significantly, one of the areas that most students face great trouble in is tackling pre-processor directives (#define, #include, #ifdef, etc.)
Taking advantage of macros throughout the program’s code to reduce code redundancy and increase signal-to-clutter ratio, thus improving code readability and maintainability.
Advanced Topics:
Recursion.
Enumerations.
Typedef.
Bitwise operations.
Command line arguments.
Best Practices and Tips:
Coding conventions and standards.
Debugging techniques.
Memory management practices.
Performance optimization tips.
Projects and Exercises:
Giving out a few Specific tasks and activities that come under the topic in question so as to ensure that the knowledge imparted is put into practice.
So if you’re looking for a project that will allow you to use C programming, the following are some suggestions to consider.
CONCLUSION:
All of these topics can be developed into full-scale articles, with various examples and subtopics further elaborated with actual code snippets and describes. To encourage the reader, they can also include quizzes or coding challenges at the end of each section for the reader to solve before moving to the next section. However, using the samples for download and the exercises which are usually included in the lessons make the lessons more effective.
#C Programming Language#C Programming course#Online & Offline course#IT & Software Course#Software course
0 notes
Text
Learn Best C Programming Language Courses
C Language is one of the simplest or entry-level C Programming Language Courses, C Language has caused a direct impact on most of the C Programming Languages that have been derived from it and it is a fact that if you have to at least have some idea of what is C Language in order to at least tell off any language.
Hence, it is a very important must for getting complete knowledge of programming languages so that it may easily enter the world of development which is one of the most competitive and prestigious professions and highly paid jobs of the modern world. Therefore, if you want to start the process and learn the basics of C, you are welcome to start with some of the courses listed above.

Takeoff upskill Today we are going to discuss the 10 Best C Programming Courses for Beginners: these are some of the best to choose from; they provide you with good content for learning and at the same time when you complete the course you are awarded with a certificate. In conclusion, we might want to expand on all of them and maybe you will be able to make your choice as to which of the methods is the most helpful to you.
Takeoff upskill is still new to C programming language information, then, before pointing beginners to the best courses to take for C programming language.
Conclusion:
All of these topics can be built up into a whole article on their own, where the various examples can be expanded upon with actual code snippets, and where they can describe in detail more subtopics. For motivation, the authors can also include questions and sometimes coding problems that the reader solves before advancing to the next section. However, employing the samples for download and the exercises which are often a part of the lessons help to make the lessons efficient. Takeoffupskill, a leader in technology and business education, exemplifies these principles in its courses.
#C Programming Language#C Programming#Programming Languages#C Programming Course#Programming Language
0 notes
Text
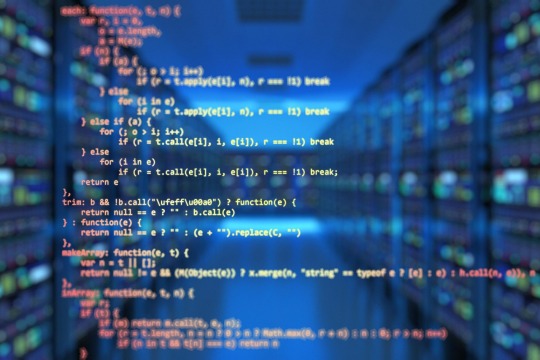
Are you interested to learn C language? Here are our free course you can check it out. On development for C programmers, specifically tailored for those interested in expanding their skills to include Raspberry Pi and sensor integration. In this course, you'll dive into coding with Raspberry Pi and Sense HAT, learning how to write code in C# that operates on a Raspberry Pi, handle joystick input, read data from motion sensors, collect meteorological data, and even flash LEDs and LED matrices.
Get your free course -
0 notes
Text
C Language
₹4,000.00
*COURSE-MERGE COMPUTER INSTITUTE OF TECHNOLOGIES* Hurry up! Must avail the opportunity of Learning a Computer Courses *COURSE-C Language* *COURSE DESCRIPTION* · Introduction to C · Operator & Expression · Managing Input/output · Conditions · Loops · Array · Character Array· Function · Structure · Pointer · Memory Allocation · Link List · File Management · The C Preprocessor *🕓 DURATION : 2 MONTHS* *📃CERTIFICATE : YES*
Merge Computer Institute Of Technologies
Call -097279 44368
A-411,412, 4th Floor, Pramukh Arcade, 2 opp nid, Reliance Cross Rd, Kudasan, Gandhinagar
Email Us: [email protected]
#c programming language#c language basics#c language#the c programming language#c programming for beginners
0 notes
Text
Xenia and Chujin are K&R friends
239 notes
·
View notes
Text
My program crashed for an hour or so and I tried to fix it
I finally fixed it
Twas a fucking compiler command gone bad
My code was good
FUCKKKKKKKKKKK
#cpp is a FUCKING bastard and I HATE it#programming#frc programming#code#coding#cpp#c++#cppprogramming#c++ language#c++ programming
77 notes
·
View notes
Text
#define A(...) for (int _a = (__VA_ARGS__), _0A #define _0A(...) _b = (__VA_ARGS__), _1A #define _1A(a, b) _c = 0; _c == 0; _c = !0) \ for (int a = _a; a <= _b; b a)
#C#The C programming language#C programming#C programming language#Computers#Programming#Technology#I wouldn't indent this with spaces if Tumblr understood how tabs work.#This code would not look like this in a normal program.
1 note
·
View note
Text

C Programming Language Tutorial: A Beginner’s Infographic Guide
This beginner-friendly infographic offers a clear and concise overview of the C programming language. Learn the basics of syntax, data types, loops, and functions—all visually explained for easy understanding. Ideal for students and aspiring developers, this guide simplifies C programming concepts to help you get started quickly and confidently. Perfect for quick reference and foundational learning in coding.
CONTACT INFORMATION
Email: [email protected]
Phone No. : +91-9599086977
Location: G-13, 2nd Floor, Sec-3, Noida, UP, 201301, India
Website: https://www.tpointtech.com/c-programming-language-tutorial
0 notes
Text
Look I don't hate C you can write good code in C if you try and I understand the reasons for its popularity but so many people who don't know shit about C give insane made up reasons for why C is good. C is like if after world war 2 everyone decided to pattern every single gun on the sten because it was really easy to make. And now people retroactively were like actually the sten is the original gun and it's good because it interacts very closely with the bullet and has fewer moving parts. This is nothing, the sten is like that because it's cheap as fuck and when it breaks you can fix it in a burned out French cottage with some sheet metal and a rock and a cigarette.
#computer stuff#saw someone talking about C performance again#it's not! it's not performant!#that's the incredibly fucking complex compiler you built it with you rube#programming languages
98 notes
·
View notes
Text
Learning C++ | Log #1
Friday 20th October 2023
I have been studying C++ for some time now, and I'm really happy with my progress. I really thought it was a super difficult programming language (I am jinxing it right now, aren't I?), but what I mean is that even the beginner stuff would be hard. But it's not!
Two days ago, I stumbled upon a website called Saymor Academy, buried seven pages deep in Google. I decided to check out the CS107: C++ Programming course, and I'm so glad I did! I've been learning so much, and it's been a blast, especially since I'm taking the course along with my friend over on Discord teaching me C++ too.
Learning can be challenging, but it's also incredibly rewarding. Once I master the basics, I'll be able to create all sorts of amazing things. I can't wait!
In the meantime, I'm just enjoying the journey~! So if you're ever thinking about learning C++, I highly recommend checking out Saymor Academy. It's a great resource, and it's a lot of fun!
☆ What I learnt today...
History of C++
Syntax
Comments
Data types
Variables
User Input
⤷ ♡ my shop �� my mini website ○ pinned ○ navigation ♡
#xc: studies#codeblr#coding#progblr#programming#studyblr#studying#computer science#tech#cplusplus#c++ studies#c++ programming#c++ language
153 notes
·
View notes
Text
I HAVE FINALLY SUCCEEDED
IT WORKS!!! IT WORKS!!!!!
[4, 5, 4.3] -> add BECOMES 13.3!!!!!!!!!!
YOU PEOPLE I HAVE DONE IT
source code will be coming soon (as soon as i get more helper functions working.
For now, PLEASE contribute if you can. Even as little as suggesting some helper functions could help me a ton.
Contribute below:
Open a PR, An issue, Anything, Just mention what the language lacks and you don't even need to implement it yourself, I'll add it to the To-Do list and get working on it ASAP.
Join the discord server, I will be posting updates and asking for suggestions and providing beta builds: https://discord.gg/JxnKn9jd
#code#codeblr#programming#compblr#programmer#progblr#developer#software engineering#c#programming languages#github#coding#coder#software engineer#technology#development#software development#software
108 notes
·
View notes