#LinkedHashMap
Explore tagged Tumblr posts
Text
📚 Comparing Java Collections: Which Data Structure Should You Use?
If you're diving into Core Java, one thing you'll definitely bump into is the Java Collections Framework. From storing a list of names to mapping users with IDs, collections are everywhere. But with all the options like List, Set, Map, and Queue—how do you know which one to pick? 🤯
Don’t worry, I’ve got you covered. Let’s break it down in simple terms, so you can make smart choices for your next Java project.
🔍 What Are Java Collections, Anyway?
The Java Collection Framework is like a big toolbox. Each tool (or data structure) helps you organize and manage your data in a specific way.
Here's the quick lowdown:
List – Ordered, allows duplicates
Set – Unordered, no duplicates
Map – Key-value pairs, keys are unique
Queue – First-In-First-Out (FIFO), or by priority
📌 When to Use What? Let’s Compare!
📝 List – Perfect for Ordered Data
Wanna keep things in order and allow duplicates? Go with a List.
Popular Types:
ArrayList – Fast for reading, not so much for deleting/inserting
LinkedList – Good for frequent insert/delete
Vector – Thread-safe but kinda slow
Stack – Classic LIFO (Last In, First Out)
Use it when:
You want to access elements by index
Duplicates are allowed
Order matters
Code Snippet:
java
🚫 Set – When You Want Only Unique Stuff
No duplicates allowed here! A Set is your go-to when you want clean, unique data.
Popular Types:
HashSet – Super fast, no order
LinkedHashSet – Keeps order
TreeSet – Sorted, but a bit slower
Use it when:
You care about uniqueness
You don’t mind the order (unless using LinkedHashSet)
You want to avoid duplication issues
Code Snippet:
java
🧭 Map – Key-Value Power Couple
Think of a Map like a dictionary. You look up values by their unique keys.
Popular Types:
HashMap – Fastest, not ordered
LinkedHashMap – Keeps insertion order
TreeMap – Sorted keys
ConcurrentHashMap – Thread-safe (great for multi-threaded apps)
Use it when:
You need to pair keys with values
You want fast data retrieval by key
Each key should be unique
Code Snippet:
java
⏳ Queue – For First-Come-First-Serve Vibes
Need to process tasks or requests in order? Use a Queue. It follows FIFO, unless you're working with priorities.
Popular Types:
LinkedList (as Queue) – Classic FIFO
PriorityQueue – Sorted based on priority
ArrayDeque – No capacity limit, faster than LinkedList
ConcurrentLinkedQueue – Thread-safe version
Use it when:
You’re dealing with task scheduling
You want elements processed in the order they come
You need to simulate real-life queues (like print jobs or tasks)
Code Snippet:
java
🧠 Cheat Sheet: Pick Your Collection Wisely
⚙️ Performance Talk: Behind the Scenes
💡 Real-Life Use Cases
Use ArrayList for menu options or dynamic lists.
Use HashSet for email lists to avoid duplicates.
Use HashMap for storing user profiles with IDs.
Use Queue for task managers or background jobs.
🚀 Final Thoughts: Choose Smart, Code Smarter
When you're working with Java Collections, there’s no one-size-fits-all. Pick your structure based on:
What kind of data you’re working with
Whether duplicates or order matter
Performance needs
The better you match the collection to your use case, the cleaner and faster your code will be. Simple as that. 💥
Got questions? Or maybe a favorite Java collection of your own? Drop a comment or reblog and let’s chat! ☕💻
If you'd like me to write a follow-up on concurrent collections, sorting algorithms, or Java 21 updates, just say the word!
✌️ Keep coding, keep learning! For More Info : Core Java Training in KPHB For UpComing Batches : https://linktr.ee/NIT_Training
#Java#CoreJava#JavaProgramming#JavaCollections#DataStructures#CodingTips#DeveloperLife#LearnJava#ProgrammingBlog#TechBlog#SoftwareEngineering#JavaTutorial#CodeNewbie#JavaList#JavaSet#JavaMap#JavaQueue#CleanCode#ObjectOrientedProgramming#BackendDevelopment#ProgrammingBasics
0 notes
Text
I have posted an article for Chinese.
0 notes
Text
Java Map: A Comprehensive Overview
The Java Map interface is a crucial part of the Java Collections Framework, providing a structure for storing key-value pairs. Among its popular implementations is the HashMap, which offers efficient performance for basic operations like insertion and retrieval by using a hash table.
For developers looking to dive deeper into this topic, resources such as JAVATPOINT offer comprehensive guides and tutorials on HashMap and other map implementations.
Understanding how to use HashMap and other Java Map types can significantly enhance your ability to manage and access data effectively in your Java applications.
Basic Concepts
A Map does not allow duplicate keys; each key can map to only one value. If you insert a new value for an existing key, the old value is overwritten. The primary operations that Map provides include adding entries, removing entries, and retrieving values based on keys. It also supports checking if a key or value is present and getting the size of the map.
Key Implementations
Several classes implement the Map interface, each with its own characteristics:
HashMap: This is the most commonly used Map implementation. It uses a hash table to store the key-value pairs, which provides average constant-time performance for basic operations such as get and put. However, HashMap does not guarantee the order of its entries. It is ideal for scenarios where performance is critical, and the order of elements is not important.
LinkedHashMap: This implementation extends HashMap and maintains a linked list of entries to preserve the insertion order. This means that when iterating over the entries of a LinkedHashMap, they will appear in the order they were inserted. This implementation is useful when you need predictable iteration order and can afford a slight performance trade-off compared to HashMap.
TreeMap: A TreeMap is a sorted map that uses a Red-Black tree to store its entries. It sorts the keys in their natural order or according to a specified comparator. This implementation is suitable when you need a map that maintains sorted order and supports range views. However, it has a higher overhead compared to HashMap and LinkedHashMap due to the tree-based structure.
Common Operations
The Map interface provides a variety of methods to interact with the data:
put(K key, V value): Adds a key-value pair to the map. If the key already exists, it updates the value.
get(Object key): Retrieves the value associated with the specified key. Returns null if the key does not exist.
remove(Object key): Removes the key-value pair associated with the specified key.
containsKey(Object key): Checks if the map contains the specified key.
containsValue(Object value): Checks if the map contains the specified value.
keySet(): Returns a set view of the keys contained in the map.
values(): Returns a collection view of the values contained in the map.
entrySet(): Returns a set view of the key-value pairs (entries) contained in the map.
Use Cases
Map implementations are versatile and can be used in a variety of scenarios:
Caching: Store frequently accessed data for quick retrieval.
Counting: Track the occurrence of elements or events.
Lookup Tables: Create efficient lookup tables for quick access to data.
Conclusion
Understanding the Map interface and its implementations is essential for effective Java programming. The HashMap is a popular choice for its efficiency in handling key-value pairs with average constant-time performance, making it ideal for various applications.
For those interested in delving deeper into data structures and their uses, resources like TpointTech offer valuable insights and tutorials on concepts such as the hashmap java.
Mastery of these tools allows developers to write more efficient and effective code, leveraging the strengths of each Map implementation to meet their specific needs.
0 notes
Text
Youtube Video Ideas
I want to post videos about the Java basics of: - Stack - ArrayDeque - HashMap - HashSet - LinkedHashMap - LinkedHashSet - TreeMap - TreeSet - IdentityHashMap
Do you have any ideas for more content? Any other Java class I should dive into?
My Channel: youtube.com/@DoSomeDev
0 notes
Text
Find the first non-repeated character in a given string input
Firstly, understand the problem, and write down any considerations & assumptions. Next, write pseudocode if that helps. Clarifications How should simple, capital, and camel case strings be considered? Throw error or return null when a given string is empty or null? Should the map maintain the order of character keys? if yes, use a LinkedHashMap Note: For this scenario case sensitivity is not…
View On WordPress
0 notes
Video
youtube
Java program to Find First Non Repeated Character in a String using LinkedHashMap #JavaInspires #CodingInJava #JavaCodingProblems https://youtu.be/bHe2i7O8pto
0 notes
Text
Коллекции?
Если коротко, то
Существует несколько типов коллекций (интерфейсов):
List - упорядоченный список. Элементы хранятся в порядке добавления, проиндексированы.
Queue - очередь. Первый пришёл - первый ушёл, то есть элементы добавляются в конец, а удаляются из начала.
Set - неупорядоченное множество. Элементы могут храниться в рандомном порядке.
Map - пара ключ-значение. Пары хранятся неупорядоченно.
У них есть реализации.
Реализации List
ArrayList - самый обычный массив. Расширяется автоматически. Может содержать элементы разных классов.
LinkedList - элементы имеют ссылки на рядом стоящие элементы. Быстрое удаление за счёт того, что элементы никуда не сдвигаются, заполняя образовавшийся пробел, как в ArrayList.
Реализации Queue
LinkedList - рассказано выше. Да, оно также реализует интерфейс Queue.
ArrayDeque - двунаправленная очередь, где элементы могут добавляться как в начало так и в конец. Также и с удалением.
PriorityQueue - элементы добавляются в сортированном порядке (по возрастанию или по алфавиту или так, как захочет разработчик)
Реализации Set
HashSet - элементы хранятся в хэш-таблице, в бакетах. Бакет каждого элемента определяется ��о его хэш-коду. Добавление, удаление и поиск происходят за фиксированное количество времени.
LinkedHashSet - как HashSet, но сохраняет порядок добавления элементов.
TreeSet - используется в том случает, когда элементы надо упорядочить. По умолчанию используется естественный порядок, организованный красно-чёрным деревом. (Нет, я не буду это здесь объяснять, не заставите)
LinkedList - рассказано выше. Да, оно также реализует интерфейс Queue.
ArrayDeque
Реализации Map
HashMap - то же самое что и HashSet. Использует хэш-таблицу для хранения.
TreeMap - тоже использует красно-чёрное дерево. Как и TreeSet, это достаточно медленно работающая структура для малого количества данных.
LinkedHashMap. Честно? Тяжеловато найти информацию про различия с LinkedHashSet, кроме того, что один - это Set, а второй - Map. Мда :/
А связи всего этого выглядят так
Овалы - интерфейсы; Прямоугольники - классы;
Здесь видно, что начало начал - интерфейс Iterable, ну а общий для всех интерфейс - Collection (Не путать с фреймворком Collections)
А где Map? а вот он
Map не наследуется от Collection - он сам по себе.
К этой теме также можно много чего добавить: устройство красно-черного дерева, работа HashSet'ов и HashMap'ов под капотом, сложность коллекций. Но это всё темы для следующих постов.
Ну, а пока надеюсь, что понятно.
#студент бормочет#List#Set#Queue#Map#ArrayList#LinkedList#ArrayDeque#PriorityOueue#HashMap#HashSet#LinkedHashMap#LinkedHashSet#TreeMap#TreeSet#как же много тегов#Коллекции
1 note
·
View note
Text
Video Tutorial: Java Hash and Maps
Video Tutorial: Java Hash and Maps
In this video, we get a closer glimpse at Map, HashMap, LinkedHashMap, TreeMap, and HashTable in Java with sample courses in Java Collections. Let’s get began! by Ram N · Sep. 29, 21 · Java Zone · Tutorial Supply link

View On WordPress
0 notes
Text
LinkedHashMap Example: LRU Cache Implementation
LinkedHashMap Example: LRU Cache Implementation
LRU cache can be implemented fairly easily using LinkedHashMap. In this article we examine what is LRU cache and how to implement it in Java.
What is LRU Cache?
LRU cache stands for least-recently-used cache. Before we examine the LRU policy, let’s take a moment to see what a cache is.
What is a cache?
In computer science a cache is a limited storage facility where items are stored for faster…
View On WordPress
0 notes
Text
maps in dart programming language
A collection of key/value pairs, from which you retrieve a value using its associated key. There is a finite number of keys in the map, and each key has exactly one value associated with it.
Maps, and their keys and values, can be iterated. The order of iteration is defined by the individual type of map. Examples:
· The plain HashMap is unordered (no order is guaranteed),
· the LinkedHashMap iterates in key insertion order,
· a sorted map like SplayTreeMap iterates the keys in sorted order.
Read more: https://dart.microcodes.in/index.php/collections/map/
0 notes
Text
[2022] Selected Java Interview Question For Experienced MNCs

If Your are struggling to find the most important and frequently asked face two face c interview questions, Then hold down and take a deep breath because you are at the right place. I know that It is very tough for fresher students to prepare for an interview as well as finding some great bunch of right questions that cover the most important topics. In this section, I have tried to cover all important and most asked c programming interview questions topics wise. Let us start Preparing.
Java interview questions for Experienced MNCs
Q1). Tell me something about HashMap in Java. Ans: HashMap is a Collection classes in java which implementation is based on the hash table data structure. HashMap class extends AbstractMap class and AbstractMap class implements the Map interface. Some Important point about Hashmap: - HashMap allows only one null key and multiple null value. - HashMap does not maintain the order of insertion into the map. - Initial size of hashmap bucket is 16 which grows to 32 when the map entries crosses 75%. - HashMap uses hashCode() and equals() methods on keys to perform put and get operations. It internally check the key is already exist or not? - Hashmap is not a thread safe. This is the reason it won't uses in multithreaded environment. Q2). What is load Factor, Threshold, Rehashing and collision in hashmap? Ans: Load factor is the measure to decide when to increase the size of the Map. By default, the load factor is 75% of the capacity. The threshold can be calculated by multiplying the current capacity and load factor. Rehashing means, re-calculating the hash code of already stored entries. When entries in the hash table exceed the threshold, the Map is rehashed and increases the size of buckets. Collision is a condition when a hash function returns the same bucket location for two different keys. For example: Suppose Our HashMap has an initial capacity is 16 and a load factor is 0.75. Threshold will be 16 * 0.75 = 12, It means after the 12th entry in the hashmap, the capacity of the hashmap will increase from 16 to 32. Q3). How HashMap Internally works in Java? Ans: Q4). Explain how hashcode will be calculated for String Key. Ans: Hashmap has hashCode() method. This method is used to calculate the hashcode value of the String as an Integer. Syntax of hascode: public int hashCode() Program import java.io.*; class Main { public static void main(String args) { String str = "Name"; System.out.println(str); int hashCode = str.hashCode(); System.out.println(hashCode); } } Output Name 2420395 Here we got Output 2420395 for String “Name”. Below is the way to calculate hashcode of string. How hashcode is calculated for string key? Below is the formula to calculate the hashcode value of a String: s*31^(n-1) + s*31^(n-2) + ... + s Here: - s is the ith character of the string - ^ is a the exponential operator - n shows the length of the string Q5). What is the Difference between HashMap and LinkedHashMap. Ans: - The difference between HashMap and LinkedHashMap is that LinkedHashMap maintains the insertion order of keys but HashMap doesn't maintain the insertion order of keys. - LinkedHashMap requires more memory than HashMap to keep the record of insertion order. Basically LinkedHashMap uses doubly LinkedList to keep track of the insertion order of keys. - LinkedHashMap extends HashMap and implements the Map interface and Hashmap extends AbstractMap class and implements the Map interface. - Hashmap were introduced in JDK 2.0 but LinkedHashMap introduced in JDK 4.0. For complete Post Visit our website post : https://quescol.com/interview-preparation/java-interview-question-experienced Read the full article
0 notes
Text
I have posted an article for Spanish.
0 notes
Text
Exploring the Benefits of Java Map

Exploring the Benefits of Java Map reveals its significant advantages, particularly with hashmap java. Java Map efficiently stores key-value pairs, ensuring fast data retrieval, unique keys, and easy integration into programs.
Implementations like `HashMap` offer constant-time performance for basic operations, enhancing application speed. Versatile and memory-efficient, Java Map simplifies data management in various real-world applications such as caching and configuration management.
For a detailed understanding of hashmap java, resources like TpointTech provide comprehensive tutorials and examples, making it easier for developers to leverage Java Map's full potential.
Efficient Data Retrieval
One of the primary benefits of using Java Map is its efficient data retrieval capability. Maps allow you to store data in key-value pairs, where each key is unique. This structure enables fast retrieval of values based on their keys, often in constant time, O(1), using hash-based implementations like HashMap. This efficiency is crucial for applications that require quick access to data, such as caching mechanisms and real-time data processing systems.
No Duplicate Keys
In Java Map, each key must be unique, which ensures that there are no duplicate keys in the collection. This feature is particularly useful when you need to maintain a unique set of identifiers and their associated values. For instance, in a database application, you can use a map to store user IDs and their corresponding information, ensuring that each user ID is unique.
Versatile Implementations
Java provides several implementations of the Map interface, each with its own strengths and use cases. The most commonly used implementations include:
HashMap: Offers constant-time performance for basic operations and is not synchronized, making it faster but not thread-safe.
LinkedHashMap: Maintains the insertion order of elements, making it useful for applications that require ordered iterations.
TreeMap: Implements the NavigableMap interface and ensures that the keys are sorted according to their natural order or a specified comparator, making it suitable for applications that require sorted data.
ConcurrentHashMap: Provides a thread-safe alternative to HashMap with improved concurrency, making it ideal for multithreaded applications.
This versatility allows developers to choose the most appropriate implementation based on their specific requirements.
Easy to Use
Java Maps are easy to use and integrate into your programs. The standard methods provided by the Map interface, such as put(), get(), remove(), and containsKey(), make it straightforward to perform common operations. Additionally, the enhanced for loop and the entrySet() method allow for easy iteration over the map's entries, simplifying the process of traversing and manipulating key-value pairs.
Memory Efficiency
Maps can be more memory-efficient compared to other data structures, especially when dealing with large datasets. By using keys to access values directly, you avoid the need for complex searching algorithms, which can save both time and memory. Implementations like HashMap use hash codes to distribute entries evenly across buckets, minimizing the likelihood of collisions and reducing the overhead associated with handling them.
Improved Code Readability
Using a Map can significantly improve the readability of your code. By clearly defining the relationship between keys and values, you make your data structures more intuitive and easier to understand. This clarity is particularly beneficial in large codebases, where maintaining readability and simplicity is crucial for effective collaboration and maintenance.
Real-World Applications
Java Maps are widely used in real-world applications across various domains. Some common use cases include:
Caching: Storing frequently accessed data for quick retrieval.
Database indexing: Mapping primary keys to database records.
Configuration management: Storing application settings and configurations.
Associative arrays: Implementing dictionaries or lookup tables.
Conclusion
Java Map, particularly the `HashMap`, offers an efficient and versatile way to manage key-value pairs, making it an essential tool for Java developers.
Its ability to provide fast data retrieval, ensure unique keys, and support various implementations makes it suitable for a wide range of applications, from caching to database indexing.
The ease of use and memory efficiency further enhance its appeal, contributing to improved code readability and maintainability. For a deeper understanding of `hashmap java`, exploring resources like TpointTech can provide valuable insights and practical examples.
0 notes
Text
Explain in detail how HashMap and LinkedHashMap handle collision in Java 8 and upwards versions? Are there any limitations and drawbacks?
In Java 8 and later versions, HashMap and LinkedHashMap handle collisions by using a combination of bucket-based hashing and linked lists (or trees in the case of HashMap) to store key-value pairs in the event of hash code collisions. Let’s delve into how collision handling works in both HashMap and LinkedHashMap: HashMap Collision Handling: Hashing: When a key-value pair is inserted into a…
View On WordPress
0 notes
Text
Complete Core Java Topics List | From Basic to Advanced Level [2021]
Attention Dear Reader, In this article, I will provide you a Complete Core Java Topics List & All concepts of java with step by step. How to learn java, when you need to start, what is the requirement to learn java, from where to start learning java,and Tools to Understand Java code. every basic thing I will include in this List of java.
core java topics list Every beginner is a little bit confused, at the starting point before learning any programming language. But you no need to worry about it. Our aim to teach you to become a good programmer. In below you can start with lesson 1 or find out the topics manually. it’s your choice.
Complete Core Java Topics List.
Chapter. 1Full Basic Introduction of java.1.1What is java?1.2Features if java?1.3What is Software?1.4What are Programs?1.5Need to know about Platforms.1.6Object Orientation.1.7What are objects in java?1.8Class in java.1.9Keywords or Reserved words of java.1.10Identifiers in java.1.11Naming convention / coding Standard.1.12Association (Has-A Relationship).1.13Aggregation and Composition in java.1.14Java Compilation process.1.15WORA Architecture of java.1.16What is JVM, JRE and JDK in java?Chapter. 2Classes and Objects in Detail2.1How to create Class?2.2How to Create Objects?2.3Different ways to create objects in class.2.4object Reference / object Address.2.5Object States and Behavior.2.6Object Creation & Direct Initialization.2.7Object initialization using Reference.Chapter. 3Data Types in Java.3.1Java Primitive Data Types.3.2Java Non-Primitive Data Types.Chapter. 4Instance Method.4.1Types of methods in java.4.2Rules of Methods.4.3How to use methods in a class.Chapter. 5Class Loading in Java?5.1What is Class Loading.5.2Class Loading Steps.5.3Program Flow explanation.5.4"this" Keyword in java?Chapter. 6Variables in java.6.1Types of Variables.6.2Scope of variables.6.3Variable initialization.Chapter. 7Variable Shadowing in Java?Chapter. 8 Constructors in java.8.1Rules for creating java Constructors.8.2Types of Construstors.8.3Difference between Constructor and method in java.Chapter. 9Categories of methods in java.9.1Abstract Method in java.9.2Concrete Method in java.Chapter. 10Overloading in java.10.1Types if Overloading.10.2Advantages and Disadvantages of overloading.10.3Can we overload main( ) method in java?10.4Constructor Overloading in java.Chapter. 11Inheritance in java?11.1Types of Inheritance.11.2Terms used in inheritance.11.3The syntax of java inheritance.11.4How to use Inheritance in java/Chapter. 12Overriding in java.12.1Usage of java method Overriding.12.2Rules of method Overriding.12.3Importance of access modifiers in overriding.12.4Real example of method Overriding.12.5Difference between method overriding and overloading java.12.6"Super" keyword in java.Chapter. 13Constructor chaining in java.13.1Generalization in java with Example.Chapter. 14Type-Casting in java.14.1Primitive and Non-Primitive Casting in java.14.2Data Widening and Data Narrowing in java.14.3Up-Casting in java.14.4Down-Casting in java.14.5Characteristics of Up-Casting.Chapter. 15Garbage Collection.15.1Advantages of Garbage Collection.15.2How can an object be unreferanced in java?15.3Some examples of Garbage Collection in java.Chapter. 16Wrapper Class in java.16.1Uses of wrapper class in java.16.2How to Convert Primitive form to object form.16.3How to Convert String form to Primitive form.16.4Learn public static xxx parsexxx(Strings srop).16.5Autoboxing in java.16.6Unboxing in java.Chapter. 17Polymorphism in java?17.1Compile time polymorphism.17.2Runtime Polymorphism.Chapter. 18Packages in java?18.1Advantages of Packages in java.18.2Standard Package structure if java Program.18.3How to access package from another package?18.4Inbuilt package in java?18.5What are Access Modifiers In java.18.6Types of Access Modifires.Chapter. 19Encapsulation in java.19.1Advantages of Encapsulation in java?19.2Java Bean Specification or Guidelines.Chapter. 20Abstraction in java.20.1What are abstract methods in java?20.2Abstract Class in java?20.3Difference between Abstract class and Concrete class.20.4Similarities between Abstract and Concrete class?20.5Interface in java?20.6Why use java interface?20.7How to declare an interface in java?20.8Types of Interface in java?20.9What are the uses of Abstraction?20.10Purpose or Advantages of Abstraction.Chapter. 21Collection Framework in java?21.1What is collection in java?21.2What is framework in java?21.3Advantages of collection over an array in java.21.4Collection Framework Hierarchy in java.21.5Generics in java?21.6List Interface in java.21.7Methods of List Interfae in java.21.8ArrayList in java?21.9Vector in java.21.10Difference between ArrayList and Vector.21.11LinkedList in Java.21.12Difference between ArrayList and LinkedList.21.13Iterating Data from List.21.14foreach Loop in java.21.15Difference between for loop and foreach loop.21.16Set Interface in java.21.17Has-based Collection in java.21.18What are HashSet in java?21.19What are LinkedHashSet in java?21.20What are TreeSet in Java?21.21Iterator interface in java.21.22Iterator Methods in java.21.23ListIterator interface in java.Chapter. 22What are Map in java?22.1Map interface method in java.22.2What are HashMap in java?22.3What are LinkedHashMap in java?22.4What are TreeMap in java?22.5HashTable in java?22.6ShortedMap in java?22.7Difference between HashMap and Hashtable in java?22.8Comparable and Comparator in java?22.9Where Comparable and Comparator is used in java?22.10What is Comparable and Comparator interface in java?Chapter. 23Exception Handling in Java.23.1Exception Hierarchy in java.23.2Checked and Unchecked Exception in java?23.3Java Try Block?23.4java catch Block?23.5Multi-catch block in java?23.6Sequence of catch Block in java?23.7Finally Block in java?23.8"throws" keyword in java?23.9"throw" keyword in java?23.10Difference between throw and throws in java.23.11Custom and user defined exception in java?23.12Difference between final, finally and finalize in java?Chapter. 24Other Advanced Topics24.1Advanced Programming Java Concepts"👆This is the Complete Core Java Topics List"
How to Learn Java Step by Step?
We know that java is the most popular programming language on the internet. and also know this is the most important language for Android Development. with the help of java, we can develop an Android application. All over the topics will helps you to understated the basic concepts to learn java step by step. which helps you to understand the structure of any application backend code for app development. we recommended you to do more practice of all concepts and topics list. which is given over to becoming a good java developer. Must Read: What is Java, Full Introduction of Java for Beginners with Examples?
What is a Programming Language?
In short, Any software language. which is used in order to build a program is called a programming language. Java is also an object-oriented programming language. using that we can develop any type of software or web application. Is it easy to learn Java?
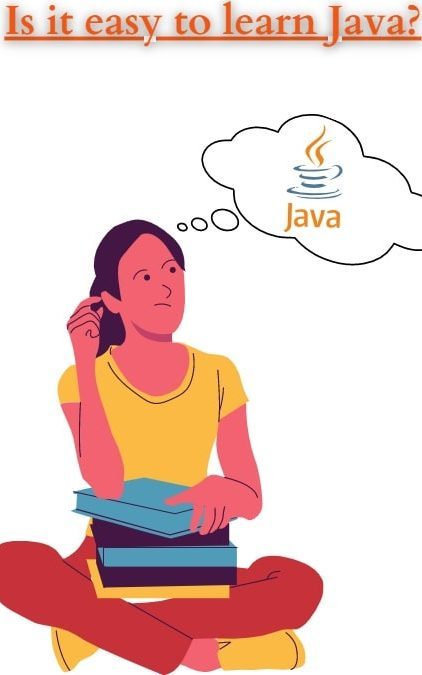
Is it easy to learn Java? My answer is "yes". Java is a beginner-friendly programming language. Because Every beginner easily learns java programming language. that means you don't need to give much effort to learn. you can start from a lower level to a higher level. Also, it provides you a user-friendly environment to develop software for clients. it has a garbage collector, which helps a lot to manage the memory space of your system for better performance. Tools to Understand Java Code. The market has lots of tools for programming. as a beginner, I will be recommending some Cool tools for Java Programming, which helps you to write and execute codes easily. 1. Eclipse: This is one of my favorite tools for practicing java codes. it will provide an integrated development environment for Java. Eclipse proved us so many interesting & modern features, modeling tools, testing tools. and frameworks development environment.
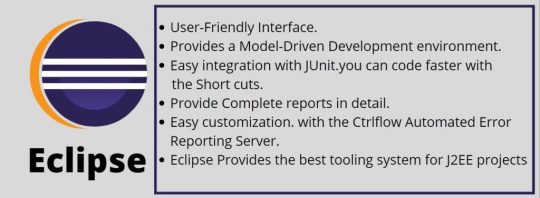
this Tools helps you to Understand Java Code. Here is Some Features of Eclipse: - User-Friendly Interface. - Provides a Model-Driven Development environment. - Easy integration with JUnit. - you can code faster with the Short-cuts. - Provide Complete reports in detail. - easy customization. with the Ctrlflow Automated Error Reporting Server. - Eclipse Provides the best tooling system for J2EE projects. - You can Download free. 2. JUnit: it is an open source unit for testing tool for Java programs. It is test-driven development and software deployment.

this Tools helps you to Understand Java Code. Here is Some Features of JUnit: - Prepare for input data and setup. - Some creation of fake objects. - Automatic Loading databases with a specific set of data - offers annotations, that test classes fixture, that's run before or after every test - support for write and execute tests on the platform. - Have some annotations to identify test methods - we can write codes faster, which increases the quality of codes and practices. - You can Download free Also Read: How to Download and Install Java JDK 15 in Windows 10 ? How longs to learn java? Every beginner student had pinged this question. How longs to learn java? now I will tell you according to the research. the speed of learning technologies and related subjects depends on the regularity of studies and the initial capacity of every student. I know you can do anything with your initial capacity level. but the regular study is a big responsibility of every student. I will recommend practicing daily to improve your programming skills and java concepts knowledge. and get stay motivated with your goals. definitely it will help you to get your first job. Note:- if any person has a little bit of programming knowledge, honestly it would take around 1 month to learn the basic concepts of java. if you are from a non-programming background but have a good knowledge of basic mathematics. definitely, you can also learn and understand fast all the concepts of java. it will all depend on your problem-solving skills and understanding concepts. Conclusions: At the starting of the article, I will provide you a complete core java topics list. it will help you to understand, where you need start, this is the basic step for a beginner. and also provides some tools for your practicing. I hope now you can start lean java with you motivation. Admin Word:- In this article, I provided A Complete Core Java Topics List. and also give your all pinged questions answers such as How to Learn Java Step by Step?, What is a Programming Language, Is it easy to learn Java, How longs to learn java. Also provides some free Tools to Understand Java Code which helps to Learn Java Step by Step. if you have any questions regarding this article please feel free to ask in the comment below of this article. Read the full article
0 notes
Text
map framework in Java
There are three primary ways of implementing the map framework in Java:
Hash Map: This JAVA Framework allows the implementation of hash table data structures. Elements in Hash Maps are stored in key/value pairs.
TreeMap: This JAVA framework allows the implementation of tree data structures. It provides accessibility to the Navigable Map interface.
LinkedHashMap: This framework allows the implementation of linked lists and hash tables. It extends to the HashMap class where its entries are stored in a hash table.
Stack, queueing and sorting using JFC: In Java Foundation Classes (JFC), stacks are abstract linear data types with predefined boundaries or capacities. They follow a certain order when adding or deleting elements. In linear data structures, components are organized in a straight line, meaning, if one removes or adds an element, the data structure will decrease or increase respectively. Stacks follow a LIFO (Last In First Out) or (FILO) First In Last Out technique of sorting elements, meaning, the last item is always the first one to be removed.
Graph structures and algorithms
Graphs are non-linear data structures comprising of nodes and edges. Sometimes, the nodes are also called vertices. Edges are simply the arcs or lines that connect nodes within the graph. In Java Assignment Help, graph algorithms are a given set of instructions that connect nodes together. Based on the type of data, an algorithm can be used to identify a specific node or even a path between two nodes. Graphs are important in modeling and solving real-world problems Linear and non-linear searching algorithms. Searching is a common action in business applications. It involves fetching the information stored in data structures like maps, lists, arrays, etc. to help the business in decision-making. In most cases, the search action determines how responsive a given application will be for the end-user. Programs written in Java use several types of search algorithms, the most common being linear and non-linear searching algorithms. The linear algorithm is used to identify elements in an array by looping over other elements of the array. The non-linear algorithm is mostly used with sorted arrays to identify various elements and usually returns the index of the found elements or a negative one if there is no index found.
RandomAccessFile
The Random Access File is a class in Java And Java Assignment Help used to write and read random access files. Basically, a random access file acts as a relatively large array of bytes. File pointer, a cursor applied to the array is used to perform the read-write operations and this is done by moving the cursor. If the program reaches the end-of-file and the required number of bytes have not been reached, then an EOFException is performed. In Java And JAVA Assignment Help, the Random Access File supports several access modes, which include, the read mode and the read and write mode. To read a byte, one must implement the read () method, and this will read a byte located in a position that is currently directed by the file pointer.
#JAVA Assignment Help#JAVA Assignment Homework Help#Best JAVA Assignment Help#Do My JAVA Assignment Help
0 notes