#java-best-practices
Explore tagged Tumblr posts
Text
Performance Best Practices Using Java and AWS Lambda: Combinations
Subscribe .tb0e30274-9552-4f02-8737-61d4b7a7ad49 { color: #fff; background: #222; border: 1px solid transparent; border-radius: undefinedpx; padding: 8px 21px; } .tb0e30274-9552-4f02-8737-61d4b7a7ad49.place-top { margin-top: -10px; } .tb0e30274-9552-4f02-8737-61d4b7a7ad49.place-top::before { content: “”; background-color: inherit; position: absolute; z-index: 2; width: 20px; height: 12px; }…
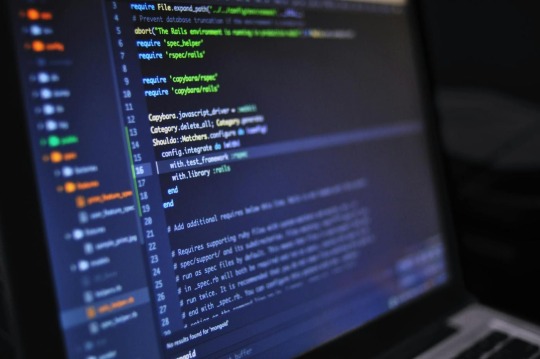
View On WordPress
#aws-lambda#aws-sdk#cloud-computing#java#java-best-practices#java-optimization#performance-testing#software-engineering
0 notes
Text
Explain about Collector.teeing feature in the Java Stream API in Java 12?
The Collector.teeing feature introduced in Java 12 is a powerful addition to the Collector interface in the Java Stream API. It allows you to combine the results of two downstream collectors into a single result using a provided BiFunction. Here’s a detailed explanation along with a sample code demonstrating its usage: Explanation: The Collector.teeing method takes three…
View On WordPress
#Best Practices#Coding Interview#Collector.teeing#interview#interview questions#Interview Success Tips#Interview Tips#Java#Java Stream API#programming#Senior Developer#Software Architects
0 notes
Text
Java is an established programming language and an ecosystem that has dominated the software business for many years. According to TIOBE index rankings, Java was the #1 popular programming language in 2020 and the fourth best currently for bespoke software development.
The key factor for its intensive popularity is its security, which is why it is extensively used in a broad range of disciplines such as Big data processing, AI application development, Android app development, Core Java software development, and many more. It provides a large set of tools and libraries, as well as cross-platform interoperability, allowing customers to build applications of their choice.
#java development#future of java#java trends#java developer for hire#Java programming language for cloud-native development#Java frameworks for microservices architecture#Java ecosystem tools for DevOps automation#Java web development trends in 2023#Agile Java development with DevOps best practices#software development company
0 notes
Text
GraphQL Resolver Explained with Examples for API Developers
Full Video Link - https://youtube.com/shorts/PlntZ5ekq0U Hi, a new #video on #graphql #resolver published on #codeonedigest #youtube channel. @java @awscloud @AWSCloudIndia @YouTube #youtube @codeonedigest #graphql #graphqlresolver #codeo
Resolver is a collection of functions that generate response for a GraphQL query. Actually, resolver acts as a GraphQL query handler. Every resolver function in a GraphQL schema accepts four positional arguments. Root – The object that contains the result returned from the resolver on the parent field. args – An object with the arguments passed into the field in the query. context – This is…

View On WordPress
#graphql#graphql api project#graphql example tutorial#graphql resolver arguments#graphql resolver async#graphql resolver best practices#graphql resolver chain#graphql resolver example#graphql resolver example java#graphql resolver field#graphql resolver functions#graphql resolver interface#graphql resolver java#graphql resolver mutation#graphql resolvers#graphql resolvers explained#graphql resolvers tutorial#graphql tutorial#what is graphql
0 notes
Text
Java Concurrency: Managing Threads and Synchronization
Is there anything more fascinating than how the applications you use for your everyday tasks can provide you with multiple tasks at once, seamlessly juggling between different actions? Java concurrency holds the answer.
Concurrency refers to the ability of a system or program to execute multiple tasks concurrently. In the context of Java programming, it involves managing threads and synchronization to ensure the efficient execution of concurrent tasks.
Concurrency in Java brings about its own set of challenges. Coordinating access to shared resources among threads can lead to issues like race conditions, deadlocks, and inconsistent data states. To tackle these challenges, developers need to understand how to manage threads and synchronize their actions effectively.
This article provide a comprehensive understanding of concurrent programming in Java. We will explore topics such as thread creation and management techniques, as well as synchronization mechanisms like locks, semaphores, monitors, etc.
Let's take a look at Java's threads and synchronization.
Understanding Threads in Java
Threads are a fundamental concept in Java that allows for concurrent execution of multiple tasks within a program. A thread can be considered an independent flow of control that operates within the context of a process. By utilizing threads, developers can achieve parallelism and improve the performance and responsiveness of their applications.
In Java, threads go through different states during their lifecycle:
New: When a thread is created but has not yet started.
Runnable: The thread is ready for execution and waiting for available CPU time.
Blocked: A blocked thread is temporarily paused due to synchronization or resource constraints.
Waiting: A thread enters the waiting state when it waits indefinitely until another notifies it.
Terminated: The final state of a terminated thread after it completes its execution or terminates prematurely.
In Java, there are two ways to create it:
By extending the Thread class: This approach involves creating a subclass of Thread and overriding its run() method.
By implementing the Runnable interface: This approach separates the code responsible for running tasks from the threading logic.
Each Java thread has an associated priority ranging from 1 (lowest) to 10 (highest). These priorities help determine how much CPU time each thread receives relative to others with different priorities. However, relying solely on priorities for precise control over scheduling is not recommended since it depends on platform-specific implementations.
Here is a list of the best Java development companies: Intelliware Development, Jelvix, Cleveroad, The Brihaspati Infotech, DevCom, BrainMobi, Zibtek, and Itransition. Each of these companies has extensive experience developing Java applications and is well-equipped to provide top-quality services.
Thread Synchronization
In concurrent programming, thread synchronization is essential to ensure the correct and predictable execution of multiple threads accessing shared resources. Without proper synchronization, race conditions and data inconsistencies can occur, leading to unexpected behavior.
Java provides synchronized blocks and methods as mechanisms for thread synchronization. By using the synchronized keyword, you can restrict access to critical sections of code, allowing only one thread at a time to execute them. This ensures that shared resources are accessed in a controlled manner.
In addition to synchronized blocks and methods, Java offers more explicit control over thread synchronization through locks. The ReentrantLock class provides advanced features such as fairness policies and condition variables that enable fine-grained control over locking mechanisms.
To achieve thread safety, it is crucial to design classes in a way that allows safe concurrent access by multiple threads without introducing race conditions or data corruption. One approach is creating immutable objects - objects whose state cannot be modified once created. Immutable objects eliminate the need for synchronization since they can be safely shared among threads without any risk of interference.
Java Concurrent Collections
Java provides a set of concurrent collections designed to be thread-safe and support efficient concurrent access. These collections ensure that multiple threads can safely access and modify the stored data without encountering issues like data corruption or race conditions.
ConcurrentHashMap is a high-performance concurrent implementation of the Map interface. It allows multiple threads to read from and write to the map concurrently, providing better scalability compared to traditional synchronized maps. It achieves this by dividing the underlying data structure into segments, allowing different threads to operate on different segments simultaneously.
The ConcurrentLinkedQueue class implements a thread-safe queue based on linked nodes. It supports concurrent insertion, removal, and retrieval operations without explicit synchronization. This makes it suitable for scenarios where multiple threads need to access a shared queue efficiently.
When iterating over a collection while other threads modify it concurrently, a ConcurrentModificationException is possible. To overcome this issue, Java provides fail-safe iterators in concurrent collections. Fail-safe iterators create copies of the original collection at the time of iteration, ensuring that modifications made during iteration do not affect its integrity.
Executor Framework
The Executor framework provides a higher-level abstraction for managing and executing tasks asynchronously. It simplifies the process of thread creation, management, and scheduling, allowing developers to focus on the logic of their tasks rather than dealing with low-level threading details.
ThreadPoolExecutor` is an implementation of the `ExecutorService` interface that manages a pool of worker threads. It allows efficient reuse of threads by maintaining a pool instead of creating new ones for each task. The ThreadPoolExecutor dynamically adjusts the number of threads based on workload and can handle large numbers of concurrent tasks efficiently.
The `Executors` class provides factory methods to create different types of executor services, such as fixed-size thread pools, cached thread pools, or scheduled thread pools. These pre-configured executor services simplify the setup process by providing convenient defaults for common use cases.
The `ExecutorService` interface represents an asynchronous execution service that extends the base `Executor`. It adds additional functionality like managing task submission, tracking progress using futures, and controlling termination.
The `Future` interface represents a result of an asynchronous computation. It provides methods to check if the computation has been completed, retrieve its result (blocking if necessary), or cancel it if desired.
The `Callable` interface is similar to a `Runnable`, but it can return a value upon completion. Callables are submitted to executor services using methods like `submit()` or `invokeAll()`, which return corresponding Future objects representing pending results.
Java Memory Model
The Java Memory Model (JMM) defines the rules and guarantees for how threads interact with memory in a multi-threaded environment. It specifies how changes made by one thread become visible to other threads.
The volatile keyword is used in Java to ensure that variables are read and written directly from/to the main memory, bypassing any local caching mechanisms. It guarantees visibility across multiple threads, ensuring that changes made by one thread are immediately visible to others.
The happens-before relationship is a key concept in JMM. It establishes order between actions performed by different threads. If an action A happens before another action B, then all changes made by A will be visible to B when it executes.
Memory consistency errors occur when multiple threads access shared data without proper synchronization, leading to unpredictable behavior or incorrect results. To avoid such errors, developers can use synchronization mechanisms like locks or synchronized blocks/methods to establish proper ordering of operations on shared data.
Additionally, using atomic classes from the java.util.concurrent package or concurrent collections ensures atomicity and thread safety without explicit locking.
Thread Communication
Interthread communication refers to the mechanisms used by threads to coordinate and exchange information with each other. It allows threads to synchronize their actions, share data, and work together towards a common goal.
The Object class provides methods like wait(), notify(), and notifyAll() for inter-thread communication. These methods are used in conjunction with synchronized blocks or methods to enable threads to wait for certain conditions and signal when those conditions are met.
The producer-consumer problem is a classic synchronization problem where one or more producer threads generate data items, while one or more consumer threads consume these items concurrently.
Proper thread communication techniques, such as using shared queues or buffers, can be employed to ensure that producers only produce when there is space available in the buffer, and consumers only consume when there are items present.
Java's BlockingQueue interface provides an implementation of a thread-safe queue that supports blocking operations such as put() (to add elements) and take() (to retrieve elements). Blocking queues facilitate efficient thread communication by allowing producers to block if the queue is full and consumers to block if it is empty.
Best Practices for Concurrency in Java
To avoid deadlocks, it is crucial to ensure that threads acquire locks in a consistent order and release them appropriately. Careful analysis of the locking hierarchy can prevent potential deadlocks. Additionally, using thread-safe data structures and synchronization mechanisms helps mitigate race conditions.
Minimizing shared mutable state reduces the complexity of concurrent programming. Immutable objects or thread-local variables eliminate the need for explicit synchronization altogether. When sharing mutable state is unavoidable, proper synchronization techniques like synchronized blocks or higher-level abstractions should be employed.
Thread pools provide efficient management of worker threads by reusing them instead of creating new ones for each task. However, it's important to choose an appropriate pool size based on available resources to avoid resource exhaustion or excessive context switching.
Properly shutting down threads is crucial to prevent resource leaks and ensure application stability. Using ExecutorService's shutdown() method allows pending tasks to complete while rejecting new tasks from being submitted. It's also important to handle interruptions correctly and gracefully terminate any long-running operations.
Conclusion
Understanding Java concurrency concepts and proper thread management is crucial for building successful and efficient applications. By leveraging the power of concurrency, developers can enhance application responsiveness and utilize system resources effectively. Properly managing threads ensures that tasks are executed concurrently without conflicts or resource contention.
Successful offshore development management also relies on a solid grasp of Java concurrency. Efficient use of concurrent programming allows distributed teams to collaborate seamlessly, maximizing productivity and minimizing communication overhead.
Incorporating concurrency in Java development enhances performance and scalability, a key to offshore success. Finoit, led by CEO Yogesh Choudhary, champions this approach for robust software solutions.
1 note
·
View note
Text
Java Performance Optimization: Techniques and Best Practices
Java is a powerful and versatile language, but it can also be slow. If you want to ensure that your Java applications are as fast as possible, you need to know how to optimize them.
https://medium.com/@rolandmack63/java-performance-optimization-techniques-and-best-practices-5b98979a2b6
1 note
·
View note
Text
how joe asked college!reader out
masterlist
Despite her 9 am Econ (thankfully) getting cancelled, y/n decided to keep her schedule consistent and go to Java Joe’s to get some work done before her afternoon classes. So, she woke herself up bright and early, threw on some sweatpants and made the trek downtown to her favorite coffee shop.
As soon as she entered, the intoxicating smell of coffee filled her lungs, immediately waking her up. Students scattered around the cafe, flaky pastries and steaming hot coffees sitting amongst laptops and homework. Over it all, some indie song played quietly in the background, giving the room a warmth that expanded further than just the temperature emanating from the espresso machine. Baristas milled about behind the bar, one of them coming up to greet y/n at the register before taking her order.
“Could I get a medium iced vanilla latte, please.” Y/n said, the barista nodding as she wrote her order down on one of the cups. Y/n dug through her backpack, looking for her wallet, when she was suddenly interrupted.
“I got it.” Someone said, stepping up to the counter next to her. “Just get me another of whatever she’s having.”
Y/n straightened, her brows furrowed as she turned to see Joe pulling his credit card out of his overstuffed wallet. His hair was damp, dripping onto his LSU football t-shirt, and a slight flush was in his cheeks as he looked over at her.
“Wait, no, I got it—” Y/n started, turning back to dig through her bag.
“I got it.” Joe said with a grin, inserting his card into the card reader. “It’s the least I can do after talking your ear off about Star Wars for the past month.”
“I…” Y/n sighed, the card reader buzzing that the transaction was complete. “Thanks.”
“No problem.” Joe shrugged, putting his wallet back into the pocket of his sweatpants. Y/n made her way to her usual spot in the corner, Joe awkwardly trailing behind as he looked around at the space.
“What are you doing up so early? You know class was cancelled right?” Y/n asked as she slid into the corner booth. Joe stood opposite her, his hand resting on the edge of the table as he rocked back and forth on his heels.
“Yeah, just had a morning practice.” Joe said. “Thought I’d see if I could finally catch you here one time.”
“You’ve been trying to catch me here?” Y/n quirked her brow, smiling up at Joe, who chuckled awkwardly.
“Well… yeah.” Joe ran a hand through his hair. “You seem to be the coffee professional so… thought I’d learn from the best.”
“You flatter me.” Y/n shook her head, looking away from Joe’s intense gaze as she felt her cheeks warm. “You can sit down… if you want.”
“Oh yeah, thanks.” Joe said, sliding into the booth. His long legs brushed against her under the table, sending goosebumps along her skin.
“So… how was practice?” Y/n asked, propping her elbow up on the table to rest her chin in. Joe adjusted in his seat, his forearms resting on the table just inches away from hers.
“Good,” Joe nodded. “Just usual stuff. Wouldn’t want to bore you with the details.”
“You’d much rather bore me with Star Wars?” Y/n smirked, causing Joe’s brows to furrow.
“You like Star Wars!” Joe scoffed, causing y/n to giggle.
“I know,” y/n said, reaching across the table and resting a hand on his forearm lightly, “I was just kidding.”
A cheesy grin stretched across Joe’s lips at the gesture, a bit of pink even making it into his cheeks as the contact. Noticing his sudden flustered expression, y/n quickly retracted her hand back to herself, her eyes avoiding his.
“Y/n, iced lattes.” The barista called. Y/n went to stand, but Joe beat her to it, climbing out of his seat and quickly grabbing their drinks from the pickup area.
“Thank you.” Y/n sang as she took her drink from him, excitedly opening the straw before stabbing it through the lid. She took a sip, closing her eyes as the sweet, coffee goodness spread across her tongue. With a small shimmy of her shoulders, she let out a hum before finally opening her eyes, to find Joe watching her as he opened his straw slowly.
“Wow.” Joe laughed as he pulled the straw out of the wrapper with his teeth. “Don’t think I’ve ever seen you so excited before.”
“Ew, that was so embarrassing.” Y/n groaned, running a hand down her face as Joe just laughed.
“No it was cute… you’re cute.” Joe said, biting on the inside of his cheek as he waited for y/n’s reaction at his risky move. She blinked quickly, looking down at her drink in front of her as she felt a small smile sneak across her lips. Sure the two of them had had witty banter that certainly towed the line between flirting and friendly, but this was something else. Now he was just outwardly flirting with her… and she liked it. A lot.
As she lifted her gaze, Joe’s eyes were still on her with the same smile on his face that always made her head spin.
“What?” Y/n asked, the smile on her face betraying her attempt at a serious tone.
“I’m just looking at you.” Joe shrugged, stirring his drink as he continued to look at her even as he took a drink.
“Well, what do you think?” Y/n asked as Joe savored the drink, smacking his lips dramatically before taking another sip.
“It’s alright.” He said with a shrug, curling his lip.
“Just ‘alright’?” Y/n scoffed, her mouth falling agape.
“Ok it’s pretty fucking good, is that what you wanted to hear?” Joe said, causing y/n to sink back into her seat, a smug grin on her face. Joe shook his head, laughing slightly to himself as he took another sip of his drink.
“So… what’re you doing tonight?” Joe asked, his calloused fingers absentmindedly tracing along the edge of his cup.
“Um, nothing really… probably just gonna watch a movie or something.” Y/n said with a shrug. Joe nodded, gnawing on his lips for a second before he would respond.
“Well, I was wondering— since you showed me your favorite spot— if you would maybe want to check out mine. For dinner.” Joe said, his eyes meeting her as he nervously let out a deep breath. Y/n’s lips parted slightly, her head still wrapping around Joe’s proposal.
“Or we could just watch a movie— or you could just do that by yourself if that’s what you want to do, either way that’s fine—” Joe rambled.
“Sure, let’s get dinner.” Y/n grinned. Joe’s nervous expression quickly dissipated, a sigh of relief escaping his lips before a smile spread across them.
“Ok, um, I’ll pick you up at… 6?” Joe said.
“Sounds good.” Y/n said, the two of them smiling giddily at each other as they took the next step after months of pining.
149 notes
·
View notes
Text
Enjolras (& Grantaire)
While not really a presence in what i'm usually writing about, I still wanted to design them. Enjolras certainly is more relevant than Grantaire when I'm writing about Marius, though. Sorry !
With a blond/..er, frosted tips? Version. Cause I know blondjolras is like canon and all but I'm not sure which I prefer.
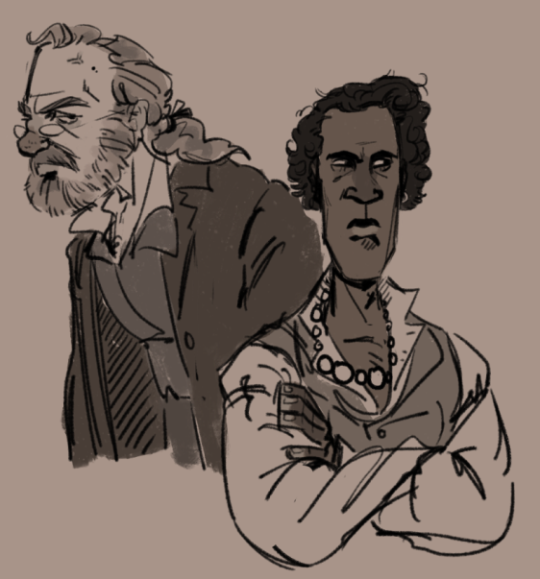
Grantaire's design is still very much in the early stages, and whether it will ever leave them still remains to be seen . I didn't draw him in colour, but he is indeed ginger and taller than enjolras, thanks Kyle Adams! (With credit to Tom Hext)
So! Design details!
My Enjolras is Javanese. Representing this (to the best of my very british ability) is his sarong (the garment around his waist). While not Javanese in essence, Enjolras is not currently living in Java/Indonesia to be able to receive that direct cultural influence. Some batik (the name of the textile pattern) would have had european influence. This can be seen in the design I have created in the branches, which I tried to base on the tree brances seen on the symbol of the French Revolution.
Also on the batik is a fleur-de-lis, and alongside the french flag stipes on the trim, connects the indonesian to the french. Admittedly the addition of the fleur-de-lis doesnt really make much sense considering it wasn't particularly used much after the Revolution, so that might end up getting changed.
The necklace he wears is also javanese in origin - admittedly I should really have looked at a reference as they are NOT made from beads, so that would be an amendment for later, but he wears that also to represent his heritage. It also somewhat symbolises his more fortunate background.
His earring is simply for flair, however since they weren't particularly common, I guess it's him straying from the status quo.
Same goes for the bleached hair - purely to stand out. But I'm not sure if that's a permanent addition to his design yet.
In my first design I had him wearing trousers and shoes, but I've changed it to a pair of boots, as they are more militaristic in nature and practical.
As for grantaire... not much to say. He looks older than his days, a bit bloated, certainly his swollen alcoholic's nose too. Hair's grown long as he's stopped caring about getting it cut and styled at the barber, but hates how cropped hair makes his head feel cold. I'd say that's the situation with his beard too - can't be arsed getting it shaved.
Anyway.... next post will probably be about the old men, don't worry. Just needed to get this out of my system.
Any requests or questions about them or the other amis are totally welcomed - but i can't guarantee i'll have a decent answer past these 2 or courfeyrac. SORRY
84 notes
·
View notes
Text
He doesn’t even know the other guy’s name. Eddie sits at a small, round table fit for two in the local coffee shop, idly sipping his java chip frappe as he waits for the latest in a long string of recent, terrible blind dates orchestrated by Chrissy. For someone who claims to be his best friend, Chrissy sure does seem to enjoy his constant suffering. She must— why else would she practically pry him out of his studio apartment this afternoon herself to make sure that he went on this date? A date for whom he doesn’t even have a fucking name. Chrissy had grinned like a Cheshire Cat when he’d asked, her reply ominous and terrifying: “You’ll know him when you see him. Trust me.” All he’d wanted was a quick coffee date: just something to appease his best friend that he can wrap up early enough to still have the rest of the day to himself. Call him a cynical fatalist, but the parade of absolute duds have left him feeling a bit more jaded lately. And the one person he is interested in is— Well, it doesn’t matter. All that matters right now is getting through another blind date, assuming that they show up. It's not looking great.
read the rest of cassette tapes and ticket stubs here on ao3! -> playlist!
a special surprise for @stevespookington! for all that you do and how amazing a friend you are! and thank you to @hexiewrites for the beta-read!
#steddie#steddie fic#steddie fanfic#steddie fanfiction#steve harrington x eddie munson#eddie munson x steve harrington#steve harrington#eddie munson#stranger things#stranger things fic#stranger things fanfic#stranger things fanfiction#myfic#back to back days of fics? who am i?
351 notes
·
View notes
Text
How can we handle deployment rollback challenges in microservice architecture?
Deployed microservices encounter critical issues, and rolling back to a previous version becomes complex or results in data inconsistencies. Solution: Utilize blue-green deployment or canary releases to minimize the impact of faulty deployments. Implement database schema versioning to handle changes in data structures during rollbacks. Terminology: Blue-Green Deployment: A deployment…
View On WordPress
#Best Practices#interview#interview questions#Interview Success Tips#Interview Tips#Java#Microservices#programming#Senior Developer#Software Architects
0 notes
Text
In a world where security is paramount, Java looks to be an unrivaled platform getting better with every new update. It is a premier programming language and an extraordinary ecosystem with the ability to deal with security concerns more effectively, thanks to its important tools and libraries.
Another aspect of Java’s unrivaled success is its “Write Once, Run Anywhere” principle. It doesn’t require recompilation when developing Java applications, which makes it the best choice for cross-platform software development.
Java is highly chosen for a wide range of projects, including AI/ML application development, Android app development, Bespoke Java software development, bespoke Blockchain development, and many more.
#java development#future of Java#java trends#java developer for hire#Java programming language for cloud-native development#Java frameworks for microservices architecture#Java ecosystem tools for DevOps automation#Java web development trends in 2023#Agile Java development with DevOps best practices#Java Development Unleashed#software development#angular development
0 notes
Note
LOL I can imagine for vampire au Lando starting to third wheel Carcar and so even though turning Franco is a complete accident he can’t feel too guilty bc he uses it as an excuse to hang around someone else, and somehow he learns more about vampirism lore through a human grad student than he’s learned in his whole life (he’s lived a long time, lots of info to absorb). Then also, if you don’t mind my ask, what do they all do for jobs/how do they get money and would Franco keep studying ?
HELP this is so cute. ok. norpinto-frando vampire au for those who aren't up to speed...
Lando starting to third wheel Carcar and so even though turning Franco is a complete accident, [Lando] can’t feel too guilty bc he uses it as an excuse to hang around someone else -> screaming cus, absolutely. random associated headcanons for this... i'll rewind a bit:
carlos is the oldest vampire, like, moorish/medieval era. he met lando while they were both at a masquerade ball in the early 1600s and smelled each other right away (carlos like wood and ink, lando like gas lamps and wet stone).
lando is an tudor era vampire. like he actually knew shakespeare and said he was one of the best viral marketers of the era
oscar was turned in the early days of the australian penal colony, he's like first or second generation white australian but he refuses to be called british. he moved in to the house because the rent was cheap and he doesn't feel the need to live extravagantly -- even though he, too, is $$ loaded $$
oscar didn't move in until about two decades ago - very short by vampire standards, to them it feels like yesterday - but carlos and oscar are basically They Were Roommates atp even though they squabble con-stant-ly
their neighbours think they are a new age-y polyam group but because the people who live opposite them are students, nobody ever hangs around longer than a year to remember them or dig deeper
so franco definitely brings a fun funky fresh dynamic
he learns more about vampirism lore through a human grad student than he’s learned in his whole life (he’s lived a long time, lots of info to absorb) -> things that baby vamp!franco teaches lando include
tiktok trends, like how to make ur teeth comically large in photos. lando finds this hilarious
how to use venmo
creating a roster on google docs for who needs to do what house chores
jailbreaking an apple watch so it doesn't read their pulses (they don't have any), but it will remind them of the moon phases and when they might be extra hungry to feed
at one point franco actually puts his academic skills to use and helps lando hunt down some of his family tree, because since lando was turned and it's been so long, he doesn't remember much about them : ( so one of franco's little gifts to lando is helping him trace his heritage
what do they all do for jobs/how do they get money and would Franco keep studying ? -> i love how practical-minded you are. um well let's say this fictional supernatural creatures' market mostly runs on barter trades and goodwill agreements. the entire house sometimes just gets lazy tbh so lando or carlos will just dig into one of the old chests of random shit and pull out an antique and go: "do we think this is worth anything?" then they take it to an antiques dealer who is also a mage (alex albon) and there is a 1 in 25 chance that the antique is actually is worth something, so that bankrolls them for another half a year or whatever.
carlos makes a lot of noise about being "an art dealer" just because he sold a goya painting to a museum once.
oscar is a man of industry, of the "newer" world (australia) etc etc so he spent the 80s and 90s learning C++ and Java and Python so he legit just codes for a living. or when he feels like it. oscar has helped launch at least a dozen startups under various pseudonyms and one of them is even a blue chip company by now. he doesn't do it for money tho. he just does it cus he likes a challenge, and otherwise fights with carlos too much. when he isn't coding he likes to tinker and fix things just for fun. like, he legit knows how to fix a boiler and stuff. his familiar is definitely a grumpy orange neighbourhood cat.
franco keeps studying!! he is such a nerd that he's like "i can totally learn everything about anything now, and i could in theory do like 20 masters degrees, and nobody can stop me"!! then lando is like, "well you might get bored of it after a while or burn out". but franco insists he will not. in fact with his enhanced neurological abilities he goes on an academic bender trying to fast forward through an entire harvard's undergrad degree's worth of material in a week, and he ends up faceplanting on his desk. and then poor lando has to go and find a fresh chicken or something to kill and revive franco 'cus franco wore himself out too fast being a bb vampire with accelerated mind powers.
franco promises never to do that again (but of course he will continue to do it once in a while, and everyone still looks after him in his lil study hangovers because he is so very nice. also he taught them how to use venmo.)
and. one time. franco is like. "i can't find this rare sonnet do you know what library i could maybe locate it in" and lando is like "wait i know that one" and pulls out an honest to god original copy that he at some point got laminated in the early 80s. and franco is like. "um i think this should be in a museum??" and lando is like "yeah but i gave them a copy of this, cus i spilled ink on the corner of this in 1603 after a really good night out" and franco is like "???? ok ????"
then lando swans off to moodily stare at the moon or some shit.
67 notes
·
View notes
Text
Helena leans over to change the radio station. "Honestly, I would have hoped that the glib pleastries of Masters of Ceremonies would have improved since my time, but it appears that this is not the case. I'm sure I heard half those inane blatherings at the Pavillion in the '90s and they were old hat then."
Myka checks her wing mirror. "I think you're being too hard on Rockin' Eddie and Rockin' KDBW, " she says. "He's trying his best to keep Muskogee 'boogeying' thru breakfast'. He's been very clear about this. And anyway, it's not as easy as it seems, being a radio announcer. Especially when you're on air alone, without someone else in the studio to talk to."
"Why, it seems perfectly simple to me," sniffs Helena, "You just open your mouth and say the first silly thing that comes to mind."
"No, there’s actually a lot of preparation and practice involved."
Helena gives Myka a shrewd glance. "You seem to know a lot about the subject. Is this another one of your abandoned careers?"
"I don't have 'abandoned careers'," says Myka, frowning, "Stop saying that. But yeah, I hosted a late night show on college radio for a couple years."
At this Helena sits up straight. "No! Really? Well!" She eyes her companion anew. "You are a dark horse Miss Bering. And so you too, I imagine, would utter those strange animal cries and then exalt your listeners to 'get down to the rhythm while they get up to the java'?"
"No, of course not," says Myka, "It was a late night show. People were going to bed."
Helena, who has been bored and irritable since they left the airport four hours ago — not that she would have admitted this, even to herself — finds that she is now quite cheered up. "And did you also play 'the greatest hits of the '70s, '80s, and beyond'?" She gasps. "Did you play 'Killing in the Name Of'?"
"And did you have a sobriquet? Like Rockin' Eddie?"
Myka shakes her head. "What is it with you and that song? And no, as I said it was a late night show, so it was relaxing music. And my show was mostly modern classical and jazz. Some baroque and early stuff. Some avant-garde." She changes lanes to let another car pass. "College radio is more, uh, eclectic than stations like KDBW Muskogee. People play all kinds of stuff."
Myka pauses. "Uh, yeah."
Helena waits.
Myka clears her throat. "I was, um... Velvet."
"Velvet."
"Yeah. The show was 'Vespers with Velvet'"
Helena considers this. "Surely it should have been 'Compline with Velvet'? If it was a late night show?"
"I know!" Myka grips the stearing wheel tighter. "I told them that! But they said they liked the alliteration. And so I had to introduce 'Vespers with Velvet' every Sunday night knowing it was wrong!" She shakes her head at the old frustration.
"But why 'Velvet' to begin with?" asks Helena. "Please don't misunderstand me, I think you have a lovely speaking voice. But I wouldn’t call it velvety."
"Oh, I didn’t speak like this," says Myka. And then her voice drops an octave. "I spoke like this."
Every hair on the back of Helena's neck stands up. A wave of anticipation passes down from her scalp, running through her body to settle heavily in her centre.
She leans back in her chair and ever-so casually crosses her legs.
"Forgive me," she says, "My mind was momentarily elsewhere. Could you repeat that, please?"
#bering & wells#myka bering#helena g wells#bering and wells#myka says that Kiling in the Name is a baby song for babies
20 notes
·
View notes
Text
How to Build Software Projects for Beginners
Building software projects is one of the best ways to learn programming and gain practical experience. Whether you want to enhance your resume or simply enjoy coding, starting your own project can be incredibly rewarding. Here’s a step-by-step guide to help you get started.
1. Choose Your Project Idea
Select a project that interests you and is appropriate for your skill level. Here are some ideas:
To-do list application
Personal blog or portfolio website
Weather app using a public API
Simple game (like Tic-Tac-Toe)
2. Define the Scope
Outline what features you want in your project. Start small and focus on the minimum viable product (MVP) — the simplest version of your idea that is still functional. You can always add more features later!
3. Choose the Right Tools and Technologies
Based on your project, choose the appropriate programming languages, frameworks, and tools:
Web Development: HTML, CSS, JavaScript, React, or Django
Mobile Development: Flutter, React Native, or native languages (Java/Kotlin for Android, Swift for iOS)
Game Development: Unity (C#), Godot (GDScript), or Pygame (Python)
4. Set Up Your Development Environment
Install the necessary software and tools:
Code editor (e.g., Visual Studio Code, Atom, or Sublime Text)
Version control (e.g., Git and GitHub for collaboration and backup)
Frameworks and libraries (install via package managers like npm, pip, or gems)
5. Break Down the Project into Tasks
Divide your project into smaller, manageable tasks. Create a to-do list or use project management tools like Trello or Asana to keep track of your progress.
6. Start Coding!
Begin with the core functionality of your project. Don’t worry about perfection at this stage. Focus on getting your code to work, and remember to:
Write clean, readable code
Test your code frequently
Commit your changes regularly using Git
7. Test and Debug
Once you have a working version, thoroughly test it. Look for bugs and fix any issues you encounter. Testing ensures your software functions correctly and provides a better user experience.
8. Seek Feedback
Share your project with friends, family, or online communities. Feedback can provide valuable insights and suggestions for improvement. Consider platforms like GitHub to showcase your work and get input from other developers.
9. Iterate and Improve
Based on feedback, make improvements and add new features. Software development is an iterative process, so don’t hesitate to refine your project continuously.
10. Document Your Work
Write documentation for your project. Include instructions on how to set it up, use it, and contribute. Good documentation helps others understand your project and can attract potential collaborators.
Conclusion
Building software projects is a fantastic way to learn and grow as a developer. Follow these steps, stay persistent, and enjoy the process. Remember, every project is a learning experience that will enhance your skills and confidence!
3 notes
·
View notes
Note
What advice could you give someone learning Java as a first language?
The best advice I can give to new programmers is:
Programming utilizes different problem solving skills that what you are likely used to. It will likely be overwhelming. This normal. It will get better as you grow more familiar with programming and learn new techniques, algorithms and best practices. Remember that this is Skill, and you improve your skill through practice.
DO NOT USE CHATGPT OR OTHER AI TOOLS TO START. If all you're doing is pasting what ChatGPT spits out then you are not really learning. It is more important to understand why you did something over getting a correct answer. You have to be able to defend your code and though process in a corporate setting if you are looking to pursue a career in software. (Think about this like showing your work in math class). When I took AP Comp Sci many moons ago, we used JGrasp a Java editor which is basically fancy notepad with a great debugger. No auto-complete, no code generation, not even spellchecking. When learning a new language you should learning the language, not the tools.
There are multiple correct answers any given problem. Programming is not like math where there is only 1 right answer for the problem. Solving the same problem in multiple ways is great way to expand your toolbox.
Some Java specific things:
If you're just starting, there is lots of AP Comp Sci course material out there. (books, quizzes, youtube, etc). You can surely find one that speaks to you.
If you have the language basics down make small graphical games like checkers, chess, etc in Swing. Its a great way to learn while doing something fun.
4 notes
·
View notes