#ASP.Net
Explore tagged Tumblr posts
Text
CORS in .NET
Setting up CORS in .NET
1. Install the necessary package
because we are displaying the raw identifiers to the user, especially sequential ones.
dotnet add package Microsoft.AspNetCore.Cors
2. Configure Services in Program.cs
Add CORS services to the DI container and set up a CORS policy.
builder.Services.AddCors(opt => { opt.AddPolicy("CorsPolicy", policy => { policy.AllowAnyMethod() .AllowAnyHeader() .WithOrigins("https://localhost:7235"); } });
3. Apply the CORS policy in the middleware pipeline:
Add CORS services to the DI container and set up a CORS policy.
app.UseCors("CorsPolicy");
Adjusting the CORS Policy
The policy we created is rather strict. You can adjust it as per your requirements. Some of the things you can configure are:
• Allowing multiple specific origins:
policy.WithOrigins("https://myapp.com", "https://anotherapp.com");
• Allowing all origins:
policy.AllowAnyOrigin();
• Allowing specific headers:
policy.WithHeaders("header1", "header2");
• Allowing specific HTTP methods:
policy.WithMethods("GET", "POST");
Note: Be cautious with allowing all origins (AllowAnyOrigin) as it can expose your API to potential security threats from malicious websites. Always ensure that you understand the security implications of the CORS settings you choose.
2 notes
·
View notes
Link
#ASP.NET#ASP.NETCore#ASP.NETCorerouting#HTTPRedirect#HttpRequest#HTTPResponse#MVC#RazorPages#Requestpipeline#Views#WorkInProgress
2 notes
·
View notes
Text
0 notes
Text
This is a huge gamble, but does anyone on here know how to work with passing JWTokens back and forth inside of HttpOnly cookies between a C# ASP.NET Web Api backend project and an ASP.NET Web MVC (Model-View-Controller) application frontend project (utilizing JavaScript and C#)???? I'm trying to work on a term project for an Advanced Web class, but I think I've blown it slightly out of proportion. I'm following tutorials, but having some difficulty wrapping my head around it.
#zer0pal's pen#student's gambit#hoping this pays off#csharp#asp.net#javascript#api#mvc application#model view controller#advanced web development#jwt
0 notes
Text
Learn Back-End Web Development
To practice and improve your web development abilities with ASP.NET Core, check out Microsoft's back-end web development tools, which include free tutorials, videos, and courses. This is a wonderful place to start if you work in the field or are interested in studying back-end web programming using ASP.NET, a well-liked free, open-source framework for creating web applications.
Since I work in the web industry myself, I wanted to share this because it's helping me understand how ASP.NET works and what I can use it for.
#coding#code#ASP#ASP.NET#web developer#educational#programming#program#open-source#web#tutorials#online courses#course work#web coding#web architect#web-coding
1 note
·
View note
Text

Partner with a leading ASP.NET Core development company. We deliver robust .NET Core solutions tailored to your business needs, ensuring efficiency and scalability.
0 notes
Text
Accelerate Your Learning: Master Angular 18 and ASP.NET 8.0

In the ever-evolving landscape of web development, two technologies continue to stand out: Angular and ASP NET. With Angular 18 offering robust front-end capabilities and ASP NET 8.0 providing a powerful back-end framework, mastering these technologies can accelerate your career and skill set. By combining these tools, developers can build dynamic, responsive, and scalable web applications that cater to modern requirements. In this article, we will explore how mastering Angular 18 and ASP NET 8.0 together can elevate your development capabilities.
Why Master Angular 18 and ASP NET 8.0 Together?
Angular and ASP NET have established themselves as cornerstones in web development. Angular is widely recognized for its component-based architecture, two-way data binding, and efficient single-page application (SPA) development. On the other hand, ASP NET 8.0 delivers high-performance server-side capabilities with support for REST APIs, MVC architecture, and Blazor for building web UIs with C#.
Combining these two technologies offers several advantages:
Seamless integration: Both Angular and ASP NET are highly compatible, allowing you to develop full-stack applications without friction.
Scalability: ASP NET 8.0’s scalability in the backend combined with Angular’s flexibility in the frontend ensures robust and scalable applications.
Efficiency in development: Using Angular for front-end development and ASP NET for backend enables faster, more streamlined workflows, reducing development time.
Understanding Angular 18: What’s New?
Component-Based Architecture
One of Angular’s strongest features is its component-based architecture, which enables developers to break down the UI into reusable components. This structure not only improves code maintainability but also fosters scalability and flexibility. Each component encapsulates its HTML, CSS, and TypeScript code, allowing for modular development.
Improved Performance
Angular 18 focuses heavily on performance improvements. With updates to its rendering engine, change detection has been optimized to reduce unnecessary re-renders, making applications faster and more responsive. Additionally, the Ivy compiler ensures smaller bundle sizes, resulting in faster load times.
Enhanced Forms and Validation
Forms are a crucial part of most web applications, and Angular 18 has introduced enhanced support for reactive forms and validation. Developers can now write cleaner, more efficient code to handle complex form scenarios such as nested forms, custom validators, and dynamic form controls.
CLI Improvements
The Angular CLI (Command Line Interface) has also been upgraded with new features to streamline the development process. You can now take advantage of faster build processes, enhanced test coverage reports, and better support for custom configurations.
Improved Testing and Debugging
Angular 18 includes enhancements for unit testing and end-to-end testing. The testing ecosystem now supports faster test execution and more reliable testing libraries. Additionally, Angular’s new debugging tools help developers quickly identify and resolve issues in complex applications.
Diving into ASP NET 8.0: Key Features
Blazor for WebAssembly
One of the standout features of ASP NET 8.0 is its deep integration with Blazor, enabling developers to create interactive web applications using C# instead of JavaScript. With Blazor WebAssembly, you can now build client-side applications that run directly in the browser, providing the speed and flexibility of SPA with the reliability of .NET.
Minimal APIs
ASP NET 8.0 introduces minimal APIs, which streamline the process of building lightweight APIs with fewer lines of code. Developers can now quickly set up RESTful services with simplified routing, error handling, and validation. This feature is particularly useful when building microservices or serverless applications.
Improved Performance
ASP NET has always been known for its high performance, and version 8.0 takes it even further. The Kestrel web server has received significant updates, making it faster and more efficient. These improvements include reduced memory usage, faster startup times, and enhanced performance when handling high-traffic applications.
Unified MVC and Razor Pages
ASP NET 8.0 merges MVC and Razor Pages into a unified programming model. This integration allows developers to switch seamlessly between building interactive UIs and web APIs, improving flexibility in application design and reducing code duplication.
Enhanced Authentication and Security
Security is a critical concern in web applications, and ASP NET 8.0 enhances authentication and authorization with better support for OAuth 2.0, OpenID Connect, and JWT tokens. Additionally, built-in protection against cross-site scripting (XSS), cross-site request forgery (CSRF), and SQL injection ensures your applications remain secure.
Building a Full-Stack Application with Angular 18 and ASP NET 8.0
Step 1: Setting Up the Environment
Before you start, ensure that you have the latest versions of Node.js, Angular CLI, and .NET SDK installed.
Install Angular CLI: bash Copy code npm install -g @angular/cli
Install .NET SDK: Download the latest version of the .NET SDK from the official Microsoft website.
Step 2: Creating an ASP NET 8.0 Backend
Start by creating a new ASP NET project:
bash
Copy code
dotnet new webapi -n MyAppBackend
Configure the backend to handle API requests, define controllers, and set up routes. Use Entity Framework to manage your database if necessary, and create minimal APIs for basic CRUD operations.
Step 3: Developing the Angular Frontend
Once the backend is ready, create a new Angular application:
bash
Copy code
ng new MyAppFrontend
You can start by building the core UI components, such as navigation, forms, and tables. Use Angular services to handle API requests and implement HTTP client modules to connect with the ASP NET backend.
typescript
Copy code
import { HttpClient } from '@angular/common/http';
@Injectable({
providedIn: 'root',
})
export class DataService {
constructor(private http: HttpClient) {}
getData() {
return this.http.get('https://localhost:5001/api/data');
}
}
Step 4: Integration and Deployment
Once both the front-end and back-end are complete, configure CORS (Cross-Origin Resource Sharing) in ASP NET to allow the Angular application to make API requests. Finally, deploy your application using Azure, AWS, or any other cloud provider.
Best Practices for Mastering Angular 18 and ASP NET 8.0
Follow Component and Service Best Practices: In Angular, always adhere to component-driven development and manage data flow using services. This ensures a cleaner, more maintainable codebase.
Use Lazy Loading for Angular Modules: Enhance the performance of your Angular application by implementing lazy loading, which loads modules only when they are needed.
Optimize ASP NET for Performance: Utilize caching, asynchronous programming, and compression to improve the performance of your ASP NET backend.
Test and Debug: Make use of unit testing frameworks like Jest for Angular and xUnit for ASP NET. Regular testing ensures fewer bugs and smooth deployment.
Conclusion
Mastering both Angular 18 and ASP NET 8.0 will give you a significant edge in building full-stack web applications. By combining Angular’s dynamic front-end capabilities with ASP NET’s robust server-side functionality, you can create scalable, high-performance applications that meet modern business needs. Whether you're building enterprise-level software or small web applications, these two technologies provide the tools you need to excel.
0 notes
Text
.NET 7 for ASP.NET Developers: Top Features You Need
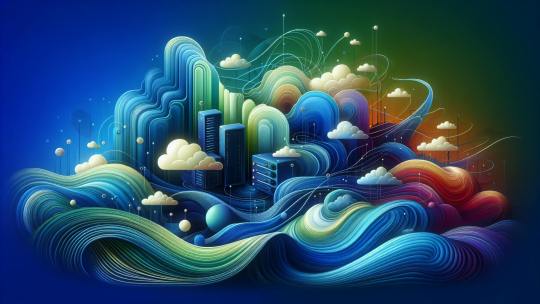
ASP.NET developers have a lot to look forward to in .NET 7, with enhancements designed to improve web development workflows. In this article, we cover the key updates that ASP.NET developers should be aware of, from minimal APIs to new cloud-native features. Understanding these changes will help you optimize your web applications for performance and scalability. Sign up for dotNetNews today, and stay updated on the latest ASP.NET, .NET 7, C#, and Azure developments.
0 notes
Text
software company in gorakhpur

0 notes
Text

Explore PHP and ASP.NET development services. Discover the strengths of each language and find out which is best for your web development project!
0 notes
Text
Kickstart Your Journey with Angular 18 and ASP.NET 8.0 Free Coupon
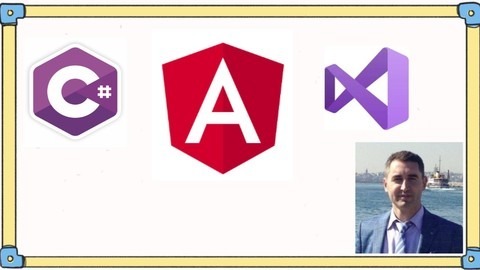
The ever-evolving world of web development demands continuous learning and adaptation. If you're looking to build modern, dynamic web applications, mastering a powerful front-end framework like Angular 18 in tandem with a robust back-end solution like ASP.NET 8.0 is a strategic move. This combination equips you with the tools to create seamless user experiences and high-performing applications.
Web Development Careers: Unveiling the Path to Success
This article serves as your comprehensive guide to kickstarting your journey with Angular 18 and ASP.NET 8.0. We'll delve into these technologies, explore their valuable features, and guide you through the learning process with a special bonus - a free coupon for a comprehensive Udemy course!
Why Angular 18 and ASP.NET 8.0?
Angular 18:
Modern Framework: Built with TypeScript for strong typing and improved developer experience.
Angular 18 New Features: A complete guide for developers - Kellton
Improved Performance: Ivy compiler optimizations for faster build times and smoother app performance.
Enhanced Forms Module: Streamlined form handling and validation for better user interaction.
Strict Mode by Default: Catches potential errors at compile time, promoting cleaner code.
Rich Ecosystem: Extensive library support and a vibrant developer community.
ASP.NET 8.0:
Cross-Platform Development: Build applications for Windows, Linux, and macOS with minimal code changes.
Improved Web API: Enhanced developer experience for creating RESTful APIs.
Enhanced Security: Robust built-in security features to protect your applications.
Cloud-Native Development: Seamless integration with cloud platforms like Azure.
Modern Development Tools: Visual Studio support provides a powerful IDE for development.
Together, Angular 18 and ASP.NET 8.0 offer a compelling combination for building full-fledged web applications.
Learning Path
1. Building a Strong Foundation:
HTML, CSS, and JavaScript: Mastering these fundamentals is crucial for understanding the building blocks of web applications.
TypeScript: Learn this superset of JavaScript for improved code type safety and maintainability.
2. Delving into Angular 18:
Understanding Components: Grasp the core building blocks of Angular applications.
Data Binding and Services: Utilize these techniques to manage data flow efficiently.
Routing and Navigation: Create seamless navigation experiences within your application.
Forms and Validation: Build user-friendly forms with robust validation.
Dependency Injection: Understand this design pattern for cleaner and more maintainable code.
3. Exploring ASP.NET 8.0:
Setting Up the Development Environment: Install the .NET SDK and learn to navigate Visual Studio.
Building Web APIs: Create RESTful APIs using ASP.NET Core MVC for communication between front-end and back-end.
Database Integration: Learn to connect your web APIs to databases for data persistence.
Security Best Practices: Implement authentication and authorization measures to secure your applications.
4. Building an Angular 18 and ASP.NET 8.0 Application:
Project Setup: Create separate projects for the Angular front-end and the ASP.NET back-end.
API Integration: Establish communication between the Angular app and the ASP.NET Web API.
Data Fetching and Display: Fetch data from the API endpoints and display it in the Angular application.
User Management: (Optional) Implement user login and registration functionalities through the API.
Resources and Learning Tools:
Udemy Courses (Free Coupon Included!): Explore a comprehensive Udemy course with video lectures, quizzes, and practical exercises. This article includes a special free coupon to unlock this valuable resource! (Details below)
Official Documentation: Both Angular and ASP.NET provide detailed documentation to guide your learning journey.
Online Tutorials and Blogs: Leverage the vast amount of online resources available for Angular and ASP.NET.
Community Forums: Engage with other developers on forums and communities to ask questions and share knowledge.
Free Udemy Course Coupon!
Get a head start on your Angular 18 and ASP.NET 8.0 journey with a free coupon for a Udemy course! This comprehensive course will equip you with the essential skills to build dynamic web applications.
0 notes
Video
youtube
The request was aborted: Could not create SSL/TLS secure channel - Asp.net
0 notes
Text
Net Development Services | Abella Systems
ASP.NET,.NET Core, Xamarin, SharePoint, and other Microsoft technologies are just a few of the industry-recognized platforms and frameworks that we offer as part of our dot net web development services at Abella Systems. We've assisted in the creation of software solutions with a range of complexity. Applications requiring numerous integrations, data-intensive apps, and more would be a few of them. Abella Systems team follows.NET requirements for code documentation and code portability and applies Microsoft's coding best practices.
0 notes