#Method overriding in Java
Explore tagged Tumblr posts
Text
Encapsulation in Java – A Complete Guide
Learn everything about Encapsulation in Java with Scientech Easy's beginner-friendly guide. Understand how encapsulation helps in data hiding, improves code maintainability, and secures your Java applications. This comprehensive article covers its real-world use, syntax, and practical examples to help you grasp the concept easily. Perfect for students and developers looking to strengthen their OOP fundamentals.Scientech Easy for clear explanations and step-by-step learning on Java programming topics. Start mastering encapsulation today!
#bca course subjects#python tuple#Interface in Java#Encapsulation in Java#Method overriding in Java#Polymorphism in Java#Constructor in java
0 notes
Text
Example of Java Method Overriding
Let us see the example of Java method overriding:
#java#programming#javaprogramming#code#coding#engineering#software#softwaredevelopment#education#technology#overriding#method#javamethod#rules#online
1 note
·
View note
Text
Understanding the Java toString() Method: Advantages and Disadvantages
Understanding the Java toString() Method In Java, the toString() method is an essential part of the Object class, the superclass of all Java classes. This method returns a string representation of the object, which can be useful for debugging, logging, and displaying object information in a human-readable format. In this blog post, we will explore the advantages and disadvantages of the Java toString() Method.
The Java toString() method is used to convert an object into a human-readable string representation.
Advantages:
✅ Easier Debugging – Helps print object details for debugging. ✅ Improves Readability – Provides meaningful object representation. ✅ Customizable – Can be overridden to display relevant object data.
Disadvantages:
❌ Default Output May Be Unreadable – If not overridden, it prints the object’s hashcode. ❌ Performance Overhead – Overriding toString() for complex objects may affect performance. ❌ Security Concerns – May expose sensitive data if not implemented carefully.
Conclusion: Overriding toString() makes debugging easier but should be used thoughtfully to avoid security risks.
#java tostring method#java tostring method override#java tostring() method#java tostring() method with example#method#object tostring() method#override tostring() method in java#the tostring() method#tostring method#tostring method in java#tostring method java#tostring()#tostring() method#tostring() method in java#tostring() method in java with an example#tostring() method in javascript#tostring() method java in tamil
0 notes
Text
Understanding Object-Oriented Programming and OOPs Concepts in Java
Object-oriented programming (OOP) is a paradigm that has revolutionized software development by organizing code around the concept of objects. Java, a widely used programming language, embraces the principles of OOP to provide a robust and flexible platform for developing scalable and maintainable applications. In this article, we will delve into the fundamental concepts of Object-Oriented Programming and explore how they are implemented in Java.
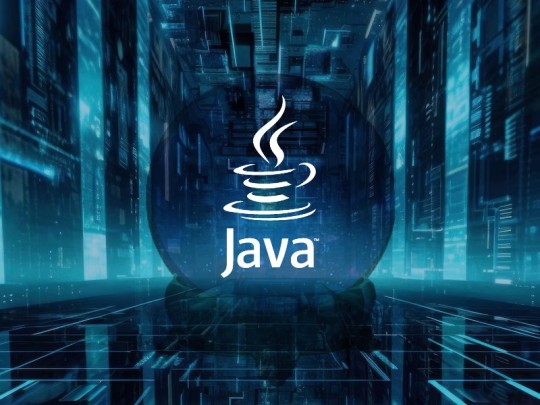
Object-Oriented Programming:
At its core, Object-Oriented Programming is centered on the idea of encapsulating data and behavior into objects. An object is a self-contained unit that represents a real-world entity, combining data and the operations that can be performed on that data. This approach enhances code modularity, and reusability, and makes it easier to understand and maintain.
Four Pillars of Object-Oriented Programming:
Encapsulation: Encapsulation involves bundling data (attributes) and methods (functions) that operate on the data within a single unit, i.e., an object. This encapsulation shields the internal implementation details from the outside world, promoting information hiding and reducing complexity.
Abstraction: Abstraction is the process of simplifying complex systems by modeling classes based on essential properties. In Java, abstraction is achieved through abstract classes and interfaces. Abstract classes define common characteristics for a group of related classes, while interfaces declare a set of methods that must be implemented by the classes that implement the interface.
Inheritance: Inheritance is a mechanism that allows a new class (subclass or derived class) to inherit properties and behaviors of an existing class (superclass or base class). This promotes code reuse and establishes a hierarchy, facilitating the creation of specialized classes while maintaining a common base.
Polymorphism: Polymorphism allows objects of different types to be treated as objects of a common type. This is achieved through method overloading and method overriding. Method overloading involves defining multiple methods with the same name but different parameters within a class, while method overriding allows a subclass to provide a specific implementation of a method that is already defined in its superclass.
Java Implementation of OOP Concepts:
Classes and Objects: In Java, a class is a blueprint for creating objects. It defines the attributes and methods that the objects of the class will have. Objects are instances of classes, and each object has its own set of attributes and methods. Classes in Java encapsulate data and behavior, fostering the principles of encapsulation and abstraction.
Abstraction in Java: Abstraction in Java is achieved through abstract classes and interfaces. Abstract classes can have abstract methods (methods without a body) that must be implemented by their subclasses. Interfaces declare a set of methods that must be implemented by any class that implements the interface, promoting a higher level of abstraction.
Inheritance in Java: Java supports single and multiple inheritances through classes and interfaces. Subclasses in Java can inherit attributes and methods from a superclass using the extends keyword for classes and the implements keyword for interfaces. Inheritance enhances code reuse and allows the creation of specialized classes while maintaining a common base.
Polymorphism in Java: Polymorphism in Java is manifested through method overloading and overriding. Method overloading allows a class to define multiple methods with the same name but different parameters. Method overriding occurs when a subclass provides a specific implementation for a method that is already defined in its superclass. This enables the use of a common interface for different types of objects.
Final Thoughts:
Object-oriented programming and its concepts form the foundation of modern software development. Java, with its robust support for OOP, empowers developers to create scalable, modular, and maintainable applications. Understanding the principles of encapsulation, abstraction, inheritance, and polymorphism is crucial for harnessing the full potential of OOPs concepts in Java. As you continue your journey in software development, a solid grasp of these concepts will be invaluable in designing efficient and effective solutions.
#javascript#javaprogramming#java online training#oops concepts in java#object oriented programming#education#technology#study blog#software#it#object oriented ontology#java course
3 notes
·
View notes
Text
How are hashCode and equals methods related?
In Java, the hashCode() and equals() methods play a critical role in determining object equality and behavior in hash-based collections like HashMap, HashSet, and Hashtable.
The equals() method is used to compare two objects for logical equality. By default, the equals() method in the Object class compares memory references. However, in most custom classes, this method is overridden to provide meaningful comparison logic—such as comparing object content (fields) rather than memory addresses.
The hashCode() method returns an integer representation of an object’s memory address by default. However, when overriding equals(), it is essential to also override hashCode() to maintain the general contract:
If two objects are equal according to the equals() method, then they must have the same hashCode() value.
Failing to do this can lead to unexpected behavior in collections. For instance, adding two logically equal objects (via equals()) to a HashSet may result in duplicates if hashCode() returns different values for them. This is because hash-based collections first use the hashCode() to find the correct bucket, and then use equals() to compare objects within the same bucket.
Example:
@Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null || getClass() != obj.getClass()) return false; MyClass other = (MyClass) obj; return this.id == other.id; } @Override public int hashCode() { return Objects.hash(id); }
In summary, always override both methods together to ensure correct object behavior in collections. A strong grasp of these concepts is crucial for building reliable applications and is a core topic in any full stack Java developer course.
0 notes
Text
Understanding Object-Oriented Programming (OOP) Concepts
Object-Oriented Programming (OOP) is a powerful programming paradigm used in many popular programming languages, including Java, Python, C++, and JavaScript. It helps developers organize code, promote reusability, and manage complexity more effectively. In this post, we'll cover the core concepts of OOP and why they matter.
What is Object-Oriented Programming?
OOP is a style of programming based on the concept of "objects", which are instances of classes. Objects can contain data (attributes) and functions (methods) that operate on the data.
1. Classes and Objects
Class: A blueprint or template for creating objects.
Object: An instance of a class with actual values.
Example in Python:class Car: def __init__(self, brand, model): self.brand = brand self.model = model def drive(self): print(f"The {self.brand} {self.model} is driving.") my_car = Car("Toyota", "Corolla") my_car.drive()
2. Encapsulation
Encapsulation is the bundling of data and methods that operate on that data within one unit (class), and restricting access to some of the object’s components.
Helps prevent external interference and misuse.
Achieved using private variables and getter/setter methods.
3. Inheritance
Inheritance allows one class (child/subclass) to inherit attributes and methods from another (parent/superclass). It promotes code reuse and logical hierarchy.class ElectricCar(Car): def charge(self): print(f"The {self.brand} {self.model} is charging.")
4. Polymorphism
Polymorphism means "many forms". It allows methods to do different things based on the object calling them. This can be achieved through method overriding or overloading.def start(vehicle): vehicle.drive() start(my_car) start(ElectricCar("Tesla", "Model 3"))
5. Abstraction
Abstraction means hiding complex implementation details and showing only essential features. It helps reduce complexity and increases efficiency for the user.
In Python, you can use abstract base classes with the abc module.
Why Use OOP?
Improves code organization and readability
Makes code easier to maintain and extend
Promotes reusability and scalability
Matches real-world modeling for easier design
Languages That Support OOP
Java
Python
C++
C#
JavaScript (with ES6 classes)
Conclusion
Object-Oriented Programming is a foundational concept for any serious programmer. By understanding and applying OOP principles, you can write cleaner, more efficient, and more scalable code. Start with small examples, and soon you'll be building full applications using OOP!
0 notes
Text
Java vs Kotlin in 2025: Is Java Still Worth Learning?
In a world charmed by shiny tools and swift transitions, dismissing what came before is easy. The tech landscape is always humming—louder than it ever did. And in that rush, Java, the stalwart, gets nudged into the wings as Kotlin walks center stage.
But in 2025, something quiet is happening—Java remains. And not just remains, but serves.
Let’s anchor this in something real: Core Java Training in KPHB is still in demand, still shaping the futures of developers, still grounding newcomers in the fundamentals of software craftsmanship. In this hub of learning, what’s being taught isn't obsolete—it's foundational.
Why Java Holds Ground
Java offers something that doesn't waver with trends: stability. It’s the kind of language that helps you understand the spine of programming logic. And in today’s ecosystem—where microservices, cloud, and data-heavy applications thrive—Java continues to power robust backends, massive systems, and enterprise solutions.
Kotlin, though elegant and concise, leans heavily on Java's shoulders. Without Java, Kotlin wouldn’t stand as tall. It complements, yes. But it does not replace.
The KPHB Perspective
In KPHB, a locality brimming with institutes, tech aspirations, and the rhythms of ambitious learners, Java is more than a language. It’s a rite of passage. Core Java Training in KPHB is not about resisting change—it’s about beginning at the root.
Think of it this way: a tree doesn’t shed its roots just because it’s grown new leaves.
Why Employers Still Respect Java
Look through the job boards in Hyderabad, and you'll see something telling: Java is still there. Required. Expected. Especially in companies where legacy systems coexist with modern frameworks. If you're job-ready in Java, you're already ahead in versatility.
Java is also the first language for many global certification paths. Oracle’s ecosystem, Spring Framework, Android—these remain deeply connected to Java.
What Learners Say
The learners walking through the doors of KPHB’s training centers aren’t chasing trends. They’re seeking direction. They often start with a question: "Should I learn Kotlin instead?" But by the end of their Core Java modules, they understand that this isn’t a choice between old and new—it’s about building a base before climbing.
Looking Ahead
The future doesn't cancel the past. It includes it. Kotlin will continue to rise, especially in Android development and cross-platform spaces. But Java’s rhythm won’t fade—it will deepen.
If you’re in KPHB, wondering where to begin—know this: Core Java Training in KPHB isn’t just a course. It’s a compass.
🧠 Previous Year Questions - Core Java
1. What is the difference between JDK, JRE, and JVM? Answer:
JDK (Java Development Kit) is a software development kit used to develop Java applications.
JRE (Java Runtime Environment) provides the environment to run Java applications.
JVM (Java Virtual Machine) executes Java bytecode and provides platform independence.
2. Explain the concept of inheritance in Java. Answer: Inheritance is an OOP concept where one class (child) acquires properties and behavior from another class (parent) using the extends keyword. It promotes code reusability.
3. What is the difference between method overloading and method overriding? Answer:
Overloading occurs within the same class with different parameter lists.
Overriding occurs in a subclass, redefining a superclass method with the same signature.
4. What are access specifiers in Java? Answer: Access specifiers define the scope of access:
private – within the class only
default – within the same package
protected – within the same package or subclasses
public – accessible from everywhere
5. Explain the use of the ‘final’ keyword. Answer: The final keyword is used to declare constants, prevent method overriding, and prevent inheritance of classes.
6. What is the difference between an abstract class and an interface? Answer:
Abstract class can have both abstract and non-abstract methods; supports constructors.
Interface contains only abstract methods (prior to Java 8) and multiple inheritance of type.
7. What is multithreading in Java? Answer: Multithreading allows concurrent execution of two or more parts of a program for maximum CPU utilization using the Thread class or Runnable interface.
8. What is garbage collection in Java? Answer: Garbage collection is the process of automatically reclaiming memory by destroying unused objects to free space.
9. How does exception handling work in Java? Answer: It uses five keywords: try, catch, throw, throws, and finally to handle run-time errors, improving program stability.
10. What is the use of the ‘this’ keyword? Answer: The this keyword refers to the current object of a class, often used to distinguish between instance and local variables.
#CoreJava#JavaTraining#JavaInterviewQuestions#JavaBasics#KPHBTraining#JavaForBeginners#JavaLearning#JavaDevelopment#JavaCertification#JavaConcepts#KPHBJava#JavaClassesHyderabad#SoftwareTraining#TechSkillsIndia#JavaPYQs
0 notes
Text
Master Polymorphism in Java – Scientech Easy
Dive into Polymorphism in Java with easy-to-follow guides at Scientech Easy. Learn how Java supports method overloading and overriding, enabling flexible and dynamic behavior in your programs. Perfect for enhancing your OOP skills!

#constructor in java#collection framework in java#Exception handling in Java#inheritance in java#Interface in Java#Python tuple#bca course subjects
0 notes
Text
Rules for Java Method Overriding
Let us see the rules for Java method overriding:

#java#programming#javaprogramming#code#coding#engineering#software#softwaredevelopment#education#technology#overriding#method#javamethod#rules#online
1 note
·
View note
Text
Java Mastery Challenge: Can You Crack These 10 Essential Coding Questions? Are you confident in your Java programming skills? Whether you're preparing for a technical interview or simply want to validate your expertise, these ten carefully curated Java questions will test your understanding of core concepts and common pitfalls. Let's dive into challenges that every serious Java developer should be able to tackle. 1. The Mysterious Output Consider this seemingly simple code snippet: javaCopypublic class StringTest public static void main(String[] args) String str1 = "Hello"; String str2 = "Hello"; String str3 = new String("Hello"); System.out.println(str1 == str2); System.out.println(str1 == str3); System.out.println(str1.equals(str3)); What's the output? This question tests your understanding of string pooling and object reference comparison in Java. The answer is true, false, true. The first comparison returns true because both str1 and str2 reference the same string literal from the string pool. The second comparison returns false because str3 creates a new object in heap memory. The third comparison returns true because equals() compares the actual string content. 2. Threading Troubles Here's a classic multithreading puzzle: javaCopypublic class Counter private int count = 0; public void increment() count++; public int getCount() return count; If multiple threads access this Counter class simultaneously, what potential issues might arise? This scenario highlights the importance of thread safety in Java applications. Without proper synchronization, the increment operation isn't atomic, potentially leading to race conditions. The solution involves either using synchronized methods, volatile variables, or atomic classes like AtomicInteger. 3. Collection Conundrum javaCopyList list = new ArrayList(); list.add("Java"); list.add("Python"); list.add("JavaScript"); for(String language : list) if(language.startsWith("J")) list.remove(language); What happens when you run this code? This question tests your knowledge of concurrent modification exceptions and proper collection iteration. The code will throw a ConcurrentModificationException because you're modifying the collection while iterating over it. Instead, you should use an Iterator or collect items to remove in a separate list. 4. Inheritance Insight javaCopyclass Parent public void display() System.out.println("Parent"); class Child extends Parent public void display() System.out.println("Child"); public class Main public static void main(String[] args) Parent p = new Child(); p.display(); What's the output? This tests your understanding of method overriding and runtime polymorphism. The answer is "Child" because Java uses dynamic method dispatch to determine which method to call at runtime based on the actual object type, not the reference type. 5. Exception Excellence javaCopypublic class ExceptionTest public static void main(String[] args) try throw new RuntimeException(); catch (Exception e) throw new RuntimeException(); finally System.out.println("Finally"); What gets printed before the program terminates? This tests your knowledge of exception handling and the finally block. "Finally" will be printed because the finally block always executes, even when exceptions are thrown in both try and catch blocks. 6. Interface Implementation javaCopyinterface Printable default void print() System.out.println("Printable"); interface Showable default void print() System.out.println("Showable"); class Display implements Printable, Showable // What needs to be added here? What must be
added to the Display class to make it compile? This tests your understanding of the diamond problem in Java 8+ with default methods. The class must override the print() method to resolve the ambiguity between the two default implementations. 7. Generics Genius javaCopypublic class Box private T value; public void setValue(T value) this.value = value; public T getValue() return value; Which of these statements will compile? javaCopyBox intBox = new Box(); Box strBox = new Box(); Box doubleBox = new Box(); This tests your understanding of bounded type parameters in generics. Only intBox and doubleBox will compile because T is bounded to Number and its subclasses. String isn't a subclass of Number, so strBox won't compile. 8. Memory Management javaCopyclass Resource public void process() System.out.println("Processing"); protected void finalize() System.out.println("Finalizing"); What's wrong with relying on finalize() for resource cleanup? This tests your knowledge of Java's memory management and best practices. The finalize() method is deprecated and unreliable for resource cleanup. Instead, use try-with-resources or implement AutoCloseable interface for proper resource management. 9. Lambda Logic javaCopyList numbers = Arrays.asList(1, 2, 3, 4, 5); numbers.stream() .filter(n -> n % 2 == 0) .map(n -> n * 2) .forEach(System.out::println); What's the output? This tests your understanding of Java streams and lambda expressions. The code filters even numbers, doubles them, and prints them. The output will be 4 and 8. 10. Serialization Scenarios javaCopyclass User implements Serializable private String username; private transient String password; // Constructor and getters/setters What happens to the password field during serialization and deserialization? This tests your knowledge of Java serialization. The password field, marked as transient, will not be serialized. After deserialization, it will be initialized to its default value (null for String). Conclusion How many questions did you get right? These problems cover fundamental Java concepts that every developer should understand. They highlight important aspects of the language, from basic string handling to advanced topics like threading and serialization. Remember, knowing these concepts isn't just about passing interviews – it's about writing better, more efficient code. Keep practicing and exploring Java's rich features to become a more proficient developer. Whether you're a beginner or an experienced developer, regular practice with such questions helps reinforce your understanding and keeps you sharp. Consider creating your own variations of these problems to deepen your knowledge even further. What's your next step? Try implementing these concepts in your projects, or create more complex scenarios to challenge yourself. The journey to Java mastery is ongoing, and every challenge you tackle makes you a better programmer.
0 notes
Text

What is Method overriding in Java?
Method overriding allows to make /take different parameters of the same method(name).In a situation you have to calculate x of different things with different number or types of values.(taking different parameters of data types)
Method overloading in Java is a feature that allows multiple methods in the same class to have the same name but different parameters (number, type, or both). It helps improve code readability and reusability.
Rules for Method Overloading:
Different Number of Parameters:
class MathUtils { int add(int a, int b) { return a + b; } int add(int a, int b, int c) { return a + b + c; } }
Different Parameter Types:
class MathUtils { int add(int a, int b) { return a + b; } double add(double a, double b) { return a + b; } }
Different Order of Parameters:
class MathUtils { void printInfo(String name, int age) { System.out.println(name + " is " + age + " years old."); } void printInfo(int age, String name) { System.out.println(name + " is " + age + " years old."); } }
Important Notes:
Method overloading is resolved at compile time (static binding).
The return type is not considered for overloading (i.e., methods cannot be overloaded by only changing the return type).
0 notes
Text
Share tips for improving code quality and maintainability.
1. Follow Java Naming Conventions
Classes: Use PascalCase for class names (e.g., EmployeeDetails).
Methods/Variables: Use camelCase for method and variable names (e.g., calculateSalary).
Constants: Use uppercase letters with underscores for constants (e.g., MAX_LENGTH).
2. Use Proper Object-Oriented Principles
Encapsulation: Make fields private and provide public getters and setters to access them.
Inheritance: Reuse code via inheritance but avoid deep inheritance hierarchies that can create tightly coupled systems.
Polymorphism: Use polymorphism to extend functionalities without changing existing code.
3. Write Clean and Readable Code
Keep Methods Small: Each method should do one thing and do it well. If a method is too long or does too much, break it down into smaller methods.
Avoid Nested Loops/Conditionals: Too many nested loops or conditionals can make code hard to read. Extract logic into separate methods or use design patterns like the Strategy or State pattern.
4. Use Design Patterns
Leverage proven design patterns like Singleton, Factory, Observer, and Strategy to solve common problems in a standardized, maintainable way.
Avoid overcomplicating things; use patterns only when they add clarity and solve a specific problem.
5. Implement Proper Error Handling
Use exceptions appropriately. Don’t overuse them, and catch only the exceptions you can handle.
Ensure that exceptions are logged for better debugging and auditing.
Use custom exceptions to represent domain-specific issues, so they are easier to debug.
6. Utilize Java’s Stream API
The Stream API (introduced in Java 8) helps reduce boilerplate code when performing collection operations like filtering, mapping, and reducing.
It makes code more concise and expressive, which helps with readability and maintainability.
7. Write Unit Tests
Use JUnit and Mockito for unit testing and mocking dependencies.
Write test cases for all critical methods and components to ensure the behavior is as expected.
Use Test-Driven Development (TDD) to ensure code correctness from the start.
8. Use Dependency Injection
Prefer Dependency Injection (DI) for managing object creation and dependencies. This decouples components and makes testing easier (using tools like Spring Framework or Guice).
DI helps to make your classes more modular and improves maintainability.
9. Avoid Code Duplication
Use methods or utility classes to avoid repeating code.
If the same logic is used in multiple places, refactor it into a single reusable method.
10. Use Annotations
Use Java annotations (like @Override, @NotNull, @Entity, etc.) to improve code clarity and reduce boilerplate code.
Annotations help to enforce business logic and constraints without having to manually check them.
11. Leverage IDE Features
Use tools like IntelliJ IDEA or Eclipse to automatically format code and identify potential issues.
Many IDEs have integrated tools for running tests, refactoring code, and applying coding standards, so make full use of these features.
12. Optimize for Performance Without Sacrificing Readability
Only optimize performance when necessary. Premature optimization can lead to complex code that’s difficult to maintain.
Profile your code to identify bottlenecks, but prioritize clarity and maintainability over micro-optimizations.
13. Implement Proper Logging
Use a logging framework like SLF4J with Logback or Log4j2 for logging. This provides a consistent logging mechanism across the application.
Ensure that logs are meaningful, providing information about the application’s state, errors, and flow, but avoid excessive logging that clutters output.
14. Document Your Code
Use JavaDocs to generate documentation for public methods and classes.
Document not just the what but also the why behind critical decisions in the codebase.
15. Keep Your Codebase Modular
Break your project into smaller, well-defined modules, and avoid large monolithic classes.
Use packages to group related classes, ensuring that each class or module has a single responsibility.
16. Use Static Analysis Tools
Integrate tools like Checkstyle, PMD, and SonarQube to enforce coding standards, detect bugs, and ensure code quality.
These tools help you identify code smells and areas where quality can be improved.
0 notes
Text
Finally, Finalize in Java is a keyword used to declare constants, prevent method overriding, and restrict inheritance. "Finally" is used in exception handling to execute code after try-catch blocks, ensuring resource cleanup. "Finalize" refers to a method in the Object class that can be overridden to define cleanup actions before garbage collection. Check here to learn more.
0 notes
Text
Top 60 most asked Java interview questions: crack like hack
Top 60 most asked Java interview questions It gives this guide easy navigation through every possible concept which could assist you either as a beginner entering into the tech world or an experienced developer wanting to progress and learn technical questions in java. Although, we have blogs related to becoming java developer full guide , how to become graphic designer in 2025 and Java full stack developer course free : A complete guide check it out.
Core Java Concepts: A refresher course on OOP principles, collections, and exceptions. Advanced Topics: Understanding multithreading, design patterns, Java 8 features including lambda expressions and streams with Java code interview questions. Practical Scenarios: Real-world examples that make you shine during the technical discussions. Interview-Ready: Java code interview questions Solaractical examples and explanations to build unfaltering confidence.
What we have for you ? core java interview question with answers
💡 You will now be brimming with strength, ready to tackle not just the routine questions but also the toughest questions if any arise, impress the hiring managers, and, of course, get through the offer!
👉 So begin your journey to becoming the Java professional everyone wants to have on board.
What is Java? Certainly, Java is a high-level, class-based object-oriented programming language, with minimal implementation dependency. It operates on the principle of “write once, run anywhere.” That is, compiled Java code can be run on all those platforms that support Java without recompilation.”
What are the unique features of Java? -Dynamic -High
Performance -Secure
Robust
Distributed
Multithreaded
Platform-independent
Object-oriented
3.Difference between JDK, JRE, and JVM. -JDK: Java Development Kit: It contains the Java Runtime Environment and an assortment of development tools used for Java application development. -JRE: Java Runtime Environment. This is a part of a computer and thus is not a tool given for Java. It provides the set of libraries and JVM needed to run Java applications. -JVM: Java Virtual Machine. An abstraction of a computer that allows a computer to execute Java programs by converting bytecode into machine-specific code.
4,What do you understand by ClassLoader in Java? ClassLoader in Java is a component of the Java Runtime Environment that is responsible for dynamically loading Java classes into the Java Virtual Machine. A ClassLoader finds and loads the class files at runtime.
5.Explain Object-Oriented Programming (OOP)
OOP is the programming paradigm based on the idea of “objects,” containing both data and code to manipulate that data. Four key important principles:
Encapsulation -Inheritance
Polymorphism
Abstraction
6.What is inheritance in Java?
Inheritance is the process of taking attributes and behaviors from one class to another, It is the mechanism through which a new class (subclass) inherits from an existing one (superclass). Inheritance supports code reusability and creates a relationship between classes, i.e., a superclass-subclass relationship. Top 60 most asked Java interview questions is one of important question
7.Polymorphism in Java?
More simply, polymorphic methods work differently depending on the object invoking them. Thus, polymorphism is of two types: name polymorphism and method overriding.
Compile-time polymorphism (Method Overloading)
Runtime polymorphism (Method Overriding)
8. What is encapsulation in Java?
Certainly, Encapsulation wraps the data (variables) and the code (methods) together as a single unit. Also, Developers achieve this by making the variables private and providing public getter and setter methods. But restricts direct access to some of the object’s components, which can prevent an accident song of data.
9. What is abstraction in Java?
Abstraction refers to the preventing the viewing of the complex implementation details while showing only the essential features of an object. It can be implemented. through abstract classes and interfaces.
10. What is the difference between an abstract class and an interface?
An abstract class is the one with both abstract and concrete methods and can maintain state via instance variables. A class can inherit an abstract class. An interface only has abstract methods (until Java 8, which introduced default and static methods) and cannot maintain state. A class can implement multiple interfaces.
11. What is a constructor in Java?
A constructor is a special method. which is called when an object is created. It has the same name as the class and no return type. It can also be overloaded, meaning that one class can have multiple constructors that can accept different numbers of parameters.
12. What is the difference between method overloading Certainly, Method overloading introduces the same names to multiple methods in the same class. But Method overriding means that a subclass provides a specific implementation. for a method that was already defined in the superclass.
13. What is the ‘this’ keyword in Java?
Although, The ‘this’ keyword refers to the current instance of class. Also It is used to indicate access to class variables and methods, and it helps in distinguishing the class attributes and parameters with identical names. and Top 60 most asked Java interview questions is one of important question
14. What is the ‘super’ keyword in Java?
The ‘super’ keyword refers to the immediate parent class object and can be used to access superclass methods and constructors.
15. A different comparison is the ‘== operator’ and the ‘equals()’ method in Java.
‘== operator’: Reference Comparison. It checks whether both references point to the same object or not.
‘equals()’ method: Compares for equality of the actual contents of the objects.
16. What is a static variable and a static method?
Static Variable: The variable that is shared across all instances of a class. It relates more to the class than to any instance of it.
Static Method: Refers to methods that belong to a class rather than the instance of an object. They do not require an instance of a class to be called.
17. What are Java Collections? Framework is a name given to the entire collection of classes and interfaces forming commonly reusable collection data structures such as lists, sets, queues, and Maps.
18. What is the difference between an ArrayList and a LinkedList in Java?
ArrayList: Certainly, Use dynamic arrays to store elements; it will provide fast random access but will be slow on insertions and deletions, especially in the middle.
LinkedList: Use doubly linked lists to store elements. It provides for faster insertions and deletions, but slower random access.
19. What is a Map in Java? Although, A map is an object that maps keys to values. It does not permit duplicate keys, and each key can map to at most one value worldwide.
20.What is the difference between HashMap and TreeMap in Java?
HashMap: Implements the Map interface using
21.What is the difference between HashSet and TreeSet?
HashSet: Uses a hash table for storage; does not maintain any order of elements.
TreeSet: Implements the NavigableSet interface and uses a red-black tree to maintain elements in sorted order.
22. Explain the differences between List and Set in Java.
List: It allows duplicate elements and maintains insertion order. Set: Does not allow duplicate elements and does not guarantee any particular order.
23. Explain the differences between an array and an ArrayList.
Array: Fixed-size, which can store both primitives and objects. ArrayList: Resizable, storing only objects.
24. What does the final keyword do in Java?
Final Variable: Cannot change its value. Final Method: This Cannot be overridden. Final Class: Cannot be subclassed.
25. What is the difference between String, StringBuilder, and StringBuffer?
String: Immutable, thread-safe. StringBuilder: Mutable, not synchronized, faster than StringBuffer. StringBuffer: Mutable, thread-safe.
26. What is the purpose of the transient keyword in Java?
The transient keyword is used to indicate that a field should not be serialized.
27. What is a volatile keyword in Java?
It ensures all the changes to a variable are visible to all threads. Top 60 most asked Java interview questions is one of important question.
28. What are the differences between synchronized and lock in Java?
Synchronized: Implicit locking mechanism. Lock: Offers more control and flexibility in locking.
29. What is multithreading in Java? Multithreading allows concurrent execution of two or more threads for maximum utilization of CPU.
30. What are the states of a thread in Java?
New
Runnable
Blocked
Waiting
Timed Waiting
Terminated
31. What is the difference between wait(), notify(), and notifyAll()?
wait(): Pauses the thread and releases the lock.
notify(): Wakes up a single thread waiting on the object’s monitor.
notifyAll(): Wakes up all threads waiting on the object’s monitor.
32. What is garbage collection in Java? Garbage collection is the process of automatically reclaiming memory by removing unused objects.
33. What are the types of memory areas allocated by JVM?
Heap
Stack
Method Area
Program Counter Register
Native Method Stack
34. What are the differences between throw and throws?
throw: Used to explicitly throw an exception.
throws: Declares exceptions in the method signature.
35. What is the difference between checked and unchecked exceptions?
Checked Exceptions: Must be handled or declared in the method signature.
Unchecked Exceptions: Runtime exceptions that do not need to be explicitly handled.
36. What is an enum in Java?
Enums are special data types that define the list of constants.
37. What is reflection in Java?
Reflection is the ability to dynamically inspect and modify a class’s behavior during runtime.
38. What is the difference between shallow cloning and deep cloning?
Certainly, Shallow cloning, like cloning, copies the values of all fields without taking into account the objects referred to. But , Deep cloning is like for all fields, a brand new class instance is created at those places. Top 60 most asked Java interview questions is one of important question
39. What are the types of design patterns in Java?
Creational (e.g., Singleton, Factory) Structural (e.g., Adapter, Proxy) Behavioral (e.g., Observer, Strategy)
40. What is the Singleton design pattern?
A design pattern that restricts the instantiation of a class to just one object and provides a global point of access to it.
To know more click here
0 notes
Text
Key Concepts to Review Before Your Java Interview
youtube
Java interviews can be both challenging and rewarding, often acting as a gateway to exciting roles in software development. Whether you're applying for an entry-level position or an advanced role, being well-prepared with core concepts is essential. In this guide, we’ll cover key topics to review before your Java interview, ensuring you're confident and ready to impress. Additionally, don't forget to check out this detailed video guide to strengthen your preparation with visual explanations and code demonstrations.
1. Object-Oriented Programming (OOP) Concepts
Java is known for its robust implementation of OOP principles. Before your interview, make sure to have a firm grasp on:
Classes and Objects: Understand how to create and use objects.
Inheritance: Review how subclasses inherit from superclasses, and when to use inheritance.
Polymorphism: Know the difference between compile-time (method overloading) and runtime polymorphism (method overriding).
Abstraction and Encapsulation: Be prepared to explain how and why they are used in Java.
Interview Tip: Be ready to provide examples of how you’ve used these concepts in your projects or coding exercises.
2. Core Java Concepts
In addition to OOP, there are foundational Java topics you need to master:
Data Types and Variables: Understand primitive types (int, double, char, etc.) and how they differ from non-primitive types.
Control Structures: Revise loops (for, while, do-while), conditional statements (if-else, switch-case), and how they control program flow.
Exception Handling: Know how try, catch, finally, and custom exceptions are used to manage errors in Java.
Collections Framework: Familiarize yourself with classes such as ArrayList, HashSet, HashMap, and their interfaces (List, Set, Map).
Interview Tip: Be prepared to discuss the time and space complexities of different collection types.
3. Java Memory Management
Understanding how Java manages memory can set you apart from other candidates:
Heap vs. Stack Memory: Explain the difference and how Java allocates memory.
Garbage Collection: Understand how it works and how to manage memory leaks.
Memory Leaks: Be prepared to discuss common scenarios where memory leaks may occur and how to avoid them.
Interview Tip: You may be asked how to optimize code for better memory management or to explain how Java’s finalize() method works.
4. Multithreading and Concurrency
With modern applications requiring multi-threading for efficient performance, expect questions on:
Threads and the Runnable Interface: Know how to create and run threads.
Thread Lifecycle: Be aware of thread states and what happens during transitions (e.g., from NEW to RUNNABLE).
Synchronization and Deadlocks: Understand how to use synchronized methods and blocks to manage concurrent access, and how deadlocks occur.
Concurrency Utilities: Review tools like ExecutorService, CountDownLatch, and Semaphore.
Interview Tip: Practice writing simple programs demonstrating thread synchronization and handling race conditions.
5. Java 8 Features and Beyond
Many companies expect candidates to be familiar with Java’s evolution, especially from Java 8 onward:
Lambda Expressions: Know how to write concise code with functional programming.
Streams API: Understand how to use streams for data manipulation and processing.
Optional Class: Learn to use Optional for handling null checks effectively.
Date and Time API: Review java.time package for managing date and time operations.
Interview Tip: Be prepared to solve coding problems using Java 8 features to show you’re up-to-date with recent enhancements.
6. Design Patterns
Java interviews often include questions on how to write clean, efficient, and scalable code:
Singleton Pattern: Know how to implement and when to use it.
Factory Pattern: Understand the basics of creating objects without specifying their exact class.
Observer Pattern: Be familiar with the publish-subscribe mechanism.
Decorator and Strategy Patterns: Understand their practical uses.
Interview Tip: Have examples ready that demonstrate how you’ve used these patterns in your projects.
7. Commonly Asked Coding Problems
Prepare by solving coding problems related to:
String Manipulations: Reverse a string, find duplicates, and check for anagrams.
Array Operations: Find the largest/smallest element, rotate arrays, or merge two sorted arrays.
Linked List Questions: Implement basic operations such as reversal, detecting cycles, and finding the middle element.
Sorting and Searching Algorithms: Review quicksort, mergesort, and binary search implementations.
Interview Tip: Practice on platforms like LeetCode or HackerRank to improve your problem-solving skills under time constraints.
Final Preparation Tips
Mock Interviews: Conduct practice interviews with peers or mentors.
Review Your Code: Ensure your past projects and code snippets are polished and ready to discuss.
Brush Up on Basics: Don’t forget to revise simple concepts, as interviews can include questions on any level of difficulty.
For more in-depth preparation, watch this helpful video that provides practical examples and coding tips to boost your confidence.
With these concepts in mind, you'll be well-equipped to handle any Java interview with poise. Good luck!
0 notes
Text
java q
try-catch block with a return statement typically looks like this there’s a return statement in the finally block, it will override the return in both try and catch, as finally always executes last:
…
to access a non-static (instance) variable or method from a static context (like a static method), you need an instance of the class.
Hprof file (Heap Profiler) is a binary file generated by Java applications to capture memory snapshots or heap dumps.
ThreadLocal in Java is a class that provides thread-local variables. Each thread accessing a ThreadLocal variable has its own, independent copy of the variable, ensuring that it’s not shared across threads. This is particularly useful when you want to prevent concurrent access issues or ensure that certain data is not shared across threads, for example, in scenarios involving user sessions, transaction contexts, or object caching within a thread.
top command Linux top command is a popular utility in Unix and Linux systems used to monitor system performance and resource usage in real-time.
public String reverseString(String s) { char[] chars = s.toCharArray(); int left = 0; int right = chars.length - 1;while (left < right) { // Swap characters at left and right char temp = chars[left]; chars[left] = chars[right]; chars[right] = temp;
O(n), where n is the length of the string.
Bean A bean is a generic term in Spring for any object managed by the Spring container. A bean is an instance of a class that is created, Component--Components are classes annotated with @Component A component is a specific type of bean, marked with a Spring stereotype annotation, such as @Component,
0 notes