#Python Wheel Libraries
Explore tagged Tumblr posts
Text
Spin the Wheel: Python Packages Meet Databricks
In this comprehensive guide, we will walk you through the entire process of creating a Python Wheel file (Python Packages) using PyCharm. But we won't stop there; we'll also show you how to deploy this Wheel file to a Databricks Cluster Library and use it
In today’s fast-paced development environment, sharing and distributing Python code across teams and within organizations can be a daunting task. While there are various methods to package Python code, one of the most efficient ways is to use Python Wheel files. These .whl files offer a plethora of advantages, including smaller file sizes for quicker network transfers and the elimination of the…

View On WordPress
#Azure Databricks#Code reuse#Databricks#PyCharm Package#Python Library#Python Wheel Libraries#Python wheels
0 notes
Text
Learning About Different Types of Functions in R Programming
Summary: Learn about the different types of functions in R programming, including built-in, user-defined, anonymous, recursive, S3, S4 methods, and higher-order functions. Understand their roles and best practices for efficient coding.
Introduction
Functions in R programming are fundamental building blocks that streamline code and enhance efficiency. They allow you to encapsulate code into reusable chunks, making your scripts more organised and manageable.
Understanding the various types of functions in R programming is crucial for leveraging their full potential, whether you're using built-in, user-defined, or advanced methods like recursive or higher-order functions.
This article aims to provide a comprehensive overview of these different types, their uses, and best practices for implementing them effectively. By the end, you'll have a solid grasp of how to utilise these functions to optimise your R programming projects.
What is a Function in R?
In R programming, a function is a reusable block of code designed to perform a specific task. Functions help organise and modularise code, making it more efficient and easier to manage.
By encapsulating a sequence of operations into a function, you can avoid redundancy, improve readability, and facilitate code maintenance. Functions take inputs, process them, and return outputs, allowing for complex operations to be performed with a simple call.
Basic Structure of a Function in R
The basic structure of a function in R includes several key components:
Function Name: A unique identifier for the function.
Parameters: Variables listed in the function definition that act as placeholders for the values (arguments) the function will receive.
Body: The block of code that executes when the function is called. It contains the operations and logic to process the inputs.
Return Statement: Specifies the output value of the function. If omitted, R returns the result of the last evaluated expression by default.
Here's the general syntax for defining a function in R:
Syntax and Example of a Simple Function
Consider a simple function that calculates the square of a number. This function takes one argument, processes it, and returns the squared value.
In this example:
square_number is the function name.
x is the parameter, representing the input value.
The body of the function calculates x^2 and stores it in the variable result.
The return(result) statement provides the output of the function.
You can call this function with an argument, like so:
This function is a simple yet effective example of how you can leverage functions in R to perform specific tasks efficiently.
Must Read: R Programming vs. Python: A Comparison for Data Science.
Types of Functions in R
In R programming, functions are essential building blocks that allow users to perform operations efficiently and effectively. Understanding the various types of functions available in R helps in leveraging the full power of the language.
This section explores different types of functions in R, including built-in functions, user-defined functions, anonymous functions, recursive functions, S3 and S4 methods, and higher-order functions.
Built-in Functions
R provides a rich set of built-in functions that cater to a wide range of tasks. These functions are pre-defined and come with R, eliminating the need for users to write code for common operations.
Examples include mathematical functions like mean(), median(), and sum(), which perform statistical calculations. For instance, mean(x) calculates the average of numeric values in vector x, while sum(x) returns the total sum of the elements in x.
These functions are highly optimised and offer a quick way to perform standard operations. Users can rely on built-in functions for tasks such as data manipulation, statistical analysis, and basic operations without having to reinvent the wheel. The extensive library of built-in functions streamlines coding and enhances productivity.
User-Defined Functions
User-defined functions are custom functions created by users to address specific needs that built-in functions may not cover. Creating user-defined functions allows for flexibility and reusability in code. To define a function, use the function() keyword. The syntax for creating a user-defined function is as follows:
In this example, my_function takes two arguments, arg1 and arg2, adds them, and returns the result. User-defined functions are particularly useful for encapsulating repetitive tasks or complex operations that require custom logic. They help in making code modular, easier to maintain, and more readable.
Anonymous Functions
Anonymous functions, also known as lambda functions, are functions without a name. They are often used for short, throwaway tasks where defining a full function might be unnecessary. In R, anonymous functions are created using the function() keyword without assigning them to a variable. Here is an example:
In this example, sapply() applies the anonymous function function(x) x^2 to each element in the vector 1:5. The result is a vector containing the squares of the numbers from 1 to 5.
Anonymous functions are useful for concise operations and can be utilised in functions like apply(), lapply(), and sapply() where temporary, one-off computations are needed.
Recursive Functions
Recursive functions are functions that call themselves in order to solve a problem. They are particularly useful for tasks that can be divided into smaller, similar sub-tasks. For example, calculating the factorial of a number can be accomplished using recursion. The following code demonstrates a recursive function for computing factorial:
Here, the factorial() function calls itself with n - 1 until it reaches the base case where n equals 1. Recursive functions can simplify complex problems but may also lead to performance issues if not implemented carefully. They require a clear base case to prevent infinite recursion and potential stack overflow errors.
S3 and S4 Methods
R supports object-oriented programming through the S3 and S4 systems, each offering different approaches to object-oriented design.
S3 Methods: S3 is a more informal and flexible system. Functions in S3 are used to define methods for different classes of objects. For instance:
In this example, print.my_class is a method that prints a custom message for objects of class my_class. S3 methods provide a simple way to extend functionality for different object types.
S4 Methods: S4 is a more formal and rigorous system with strict class definitions and method dispatch. It allows for detailed control over method behaviors. For example:
Here, setClass() defines a class with a numeric slot, and setMethod() defines a method for displaying objects of this class. S4 methods offer enhanced functionality and robustness, making them suitable for complex applications requiring precise object-oriented programming.
Higher-Order Functions
Higher-order functions are functions that take other functions as arguments or return functions as results. These functions enable functional programming techniques and can lead to concise and expressive code. Examples include apply(), lapply(), and sapply().
apply(): Used to apply a function to the rows or columns of a matrix.
lapply(): Applies a function to each element of a list and returns a list.
sapply(): Similar to lapply(), but returns a simplified result.
Higher-order functions enhance code readability and efficiency by abstracting repetitive tasks and leveraging functional programming paradigms.
Best Practices for Writing Functions in R
Writing efficient and readable functions in R is crucial for maintaining clean and effective code. By following best practices, you can ensure that your functions are not only functional but also easy to understand and maintain. Here are some key tips and common pitfalls to avoid.
Tips for Writing Efficient and Readable Functions
Keep Functions Focused: Design functions to perform a single task or operation. This makes your code more modular and easier to test. For example, instead of creating a function that processes data and generates a report, split it into separate functions for processing and reporting.
Use Descriptive Names: Choose function names that clearly indicate their purpose. For instance, use calculate_mean() rather than calc() to convey the function’s role more explicitly.
Avoid Hardcoding Values: Use parameters instead of hardcoded values within functions. This makes your functions more flexible and reusable. For example, instead of using a fixed threshold value within a function, pass it as a parameter.
Common Mistakes to Avoid
Overcomplicating Functions: Avoid writing overly complex functions. If a function becomes too long or convoluted, break it down into smaller, more manageable pieces. Complex functions can be harder to debug and understand.
Neglecting Error Handling: Failing to include error handling can lead to unexpected issues during function execution. Implement checks to handle invalid inputs or edge cases gracefully.
Ignoring Code Consistency: Consistency in coding style helps maintain readability. Follow a consistent format for indentation, naming conventions, and comment style.
Best Practices for Function Documentation
Document Function Purpose: Clearly describe what each function does, its parameters, and its return values. Use comments and documentation strings to provide context and usage examples.
Specify Parameter Types: Indicate the expected data types for each parameter. This helps users understand how to call the function correctly and prevents type-related errors.
Update Documentation Regularly: Keep function documentation up-to-date with any changes made to the function’s logic or parameters. Accurate documentation enhances the usability of your code.
By adhering to these practices, you’ll improve the quality and usability of your R functions, making your codebase more reliable and easier to maintain.
Read Blogs:
Pattern Programming in Python: A Beginner’s Guide.
Understanding the Functional Programming Paradigm.
Frequently Asked Questions
What are the main types of functions in R programming?
In R programming, the main types of functions include built-in functions, user-defined functions, anonymous functions, recursive functions, S3 methods, S4 methods, and higher-order functions. Each serves a specific purpose, from performing basic tasks to handling complex operations.
How do user-defined functions differ from built-in functions in R?
User-defined functions are custom functions created by users to address specific needs, whereas built-in functions come pre-defined with R and handle common tasks. User-defined functions offer flexibility, while built-in functions provide efficiency and convenience for standard operations.
What is a recursive function in R programming?
A recursive function in R calls itself to solve a problem by breaking it down into smaller, similar sub-tasks. It's useful for problems like calculating factorials but requires careful implementation to avoid infinite recursion and performance issues.
Conclusion
Understanding the types of functions in R programming is crucial for optimising your code. From built-in functions that simplify tasks to user-defined functions that offer customisation, each type plays a unique role.
Mastering recursive, anonymous, and higher-order functions further enhances your programming capabilities. Implementing best practices ensures efficient and maintainable code, leveraging R’s full potential for data analysis and complex problem-solving.
#Different Types of Functions in R Programming#Types of Functions in R Programming#r programming#data science
4 notes
·
View notes
Text
Dean Winchester- Hunter, Brother...Grandfather?
Word count: 861
Read on AO3
Part 5 of Just a Glimpse
Mary watched you, your hands gripping the arms of the chair. “She’s not moving from this chair, Dean.”
“She’s going to have to!”
“Why?” As if on cue, Sammy’s fussing came through the monitor. “…There’s a baby in the bunker?”
You clenched your jaw. “I told you! My son.” Moving to get up, she moved forward with the gun. “I’m going to my son.”
“Mom! Let her get to him! We’re heading to the car now.” Dean told her, rushing.
Dean had the phone between his shoulder and ear as he started the car. Hearing a gunshot, he froze.
His heart was racing. Did he get his daughter killed by not sending his mother a text? Would he go back to a dead teenager on his floor? Sam had never seen that look on Dean’s face. He knew that he was scared, he was, too. This was something beyond fear, that not even he could place. He sped the whole way home, his knuckles white from gripping the steering wheel.
Baby was barely in park and he was running towards the bunker door. “Mom?!” He called out, rushing around. “Y/N?” His voice was dripping with panic and worry.
“In here, Dean.” Mary called out from the library.
Both Dean and Sam ran through the bunker, skidding to a stop in the doorway. You were laying on the ground, holding your side. Dean’s breathing picked up, and for the first time he felt like he might have a panic attack. “CAS!” He called out, his voice cracking. “Come on, Cas!” Dean moved forward, feeling like his knees would give out at any moment. Sam was frozen in place, staring. “Where the hell is he?!” Dean breathed as he dropped to his knees next to you. He pulled your head into his lap.
“Mom! What did you do?” Sam finally asked, Sammy’s crying breaking him from his trance. His voice was firm, and angry. Walking through, he rushed to get to Sammy.
Mary looked scared as she watched Dean cry over you, brushing your hair back from your face. “Come on, sweetheart.” He begged.
You gave him a weak smile. “Tis but a flesh wound.”
He let out a chuckle mid sob. “Quoting Monty Python? Now?”
“Someone needs to be calm.” You breathed.
Dean looked to where your hand was and pulled off his jacket, tossing it to the side. Pulling off his flannel, he pushed it down on your hands. “CASTIEL!” He hollered, looking up.
There was a fluttering of wings behind him. “Dean?” He asked, moving forward, his brows furrowed.
“Heal her.” He said, looking up, his green eyes full of tears. “Please.” Of course he didn’t need to say that, but he’d beg if it meant saving her.
“Of course.” Cas nodded, kneeling down just as your eyes were starting to grow heavy. He touched your forehead gently, healing you. Your eyes remained closed, but your breathing improved. “She will need to rest. What happened?”
Dean moved to lift you off the floor. “I’ll explain in a minute. And we’re going to have a talk.” He glared at Mary, who was silently crying on the floor.
After Dean had put you in your room, he stormed back towards the library. Sam was sitting in a chair feeding Sammy, Cas was standing around, and Mary was pacing. They all looked over and it was clear that the fear and panic turned into pure rage. Mary moved forward. “I’m so–”
His clenching jaw cut her off. “I TOLD YOU!” He yelled, making her jump. “I told you that we knew she was here. I told you to LET HER GO.” Dean’s chest was heaving. “And you shot her. You shot MY DAUGHTER.” Sammy started crying at that. He took a breath, calming himself. “If Cas hadn’t shown up, my grandson would be an orphan. Now, I know you didn’t know wh–”
“She told me.” Mary whispered.
“She…told you?” He asked. She nodded. “And then I tell you I know she’s here. To let her go. You didn’t add it up?”
Mary shook her head, Sam could tell she felt guilty, but Dean had to get there. “You’d never mentioned her. Or him.” She looked to Sam.
Dean ran a hand through his hair. “Look at our life, mom. Our life is harsh. I should have called you or texted you when she showed up. But you? You shot her.” His voice was quiet, and it broke. His eyes locked on hers. “I nearly lost a daughter I barely know.”
“She’s from the future, mom.” Sam spoke up. “She was in danger, so Dean had Cas bring the two most important people in his life here. For who knows how long.” He explained.
“I’m so sorry, I didn’t mean to.” She told them. “I got jumpy, worried about you two.” Mary explained. Her eyes went back to Dean. “So, you’re a grandfather?” She smiled softly.
He nodded. “Yeah. I am. Or…will be.” The whole time line thing screwed with him.
She moved over to where Sam was. “He’s gorgeous.” She ran her thumb over his head. “You can tell he’s a Winchester.”
4 notes
·
View notes
Text
Exploring Python - A Perfect Easy Language Choice for Beginners
Python is a popular programming language, and it's particularly known for being easy to grasp. But why is Python a common recommendation, especially for people who are new to programming?
1. Language That Reads Like English:
Python stands out because when you read Python code, it looks a lot like the English language. It doesn't use complex symbols or strange commands. Instead, Python uses words and phrases that you're already familiar with.
2. Emphasis on Easy Reading:
Python places a strong emphasis on making code easy to read. This means that not only is your code easy for you to understand, but it's also simple for others who might work on your code. This focus on readability is like having a friendly guide that helps everyone who deals with the code.
3. Plenty of Learning Help:
Starting something new can often be intimidating, but Python makes the learning process more accessible. There's a wealth of resources available to help you learn Python. These resources include official documentation, tutorials, online courses, and forums. It's like having guidebooks, teachers, and fellow learners who are ready to assist you. Python's vast learning support network ensures you're never alone on your learning journey.
4. Super Flexible:
Python is an incredibly versatile programming language used in various domains. It's employed in web development, data analysis, artificial intelligence, and more. Learning Python opens doors to a wide range of career opportunities, just like a master key unlocks many doors.
5. A Library of Shortcuts:
Python boasts an extensive standard library that includes pre-written code for various common tasks. This is like having a collection of shortcuts to simplify everyday coding tasks. These pre-written pieces of code can save you a great deal of time and effort when you're building applications.
6. A Crowd of Friends:
Python has a vast and active community of developers. These Python enthusiasts hang out online, answering questions, sharing tips, and making sure you're never stranded for too long when you encounter programming challenges. It's like being part of a giant, friendly classroom where help is always at hand.
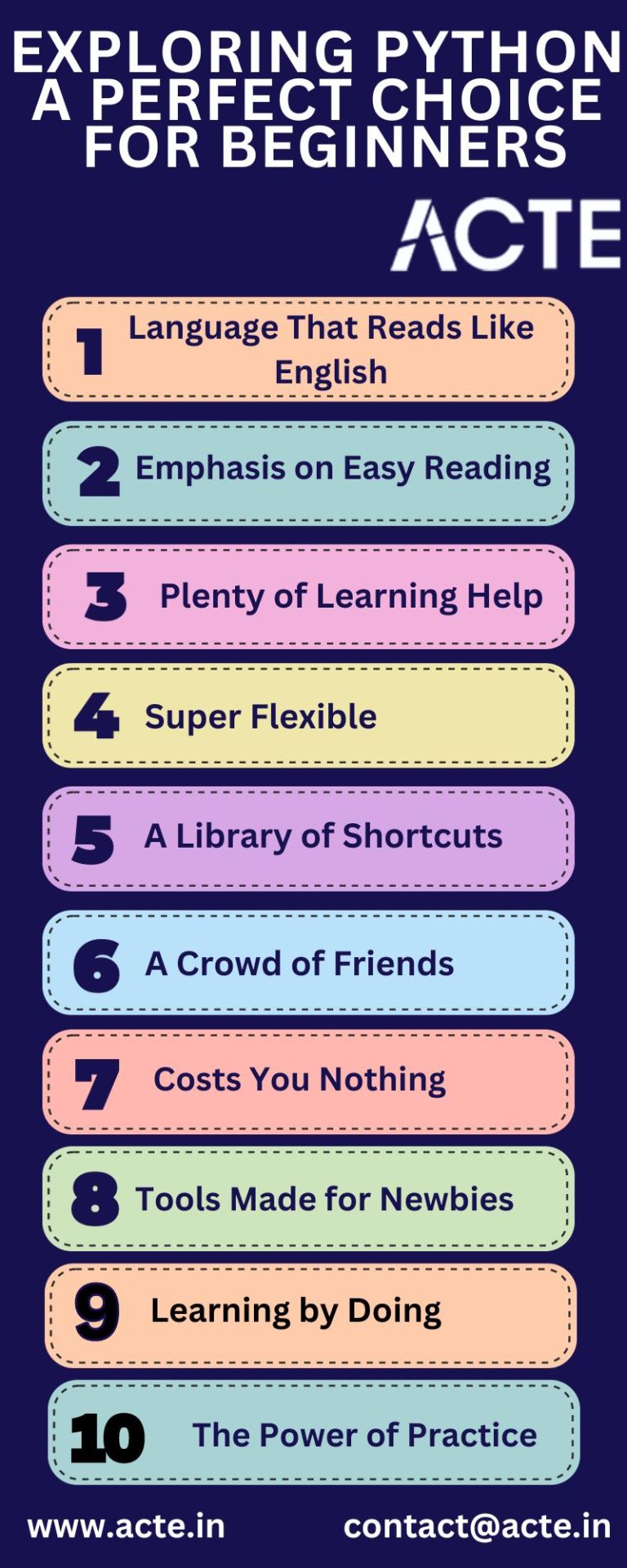
7. Costs You Nothing:
Learning Python doesn't come with a price tag. Python is open-source, which means it's entirely free to use. You can download and install Python on your computer without spending any money, similar to finding a cool toy without having to make a purchase.
8. Tools Made for Newbies:
Python also offers beginner-friendly integrated development environments (IDEs) like IDLE and user-friendly code editors like Visual Studio Code. These tools are designed to make the coding process simpler and less intimidating for beginners, much like having training wheels on your first bicycle.
9. Learning by Doing:
Python strongly encourages hands-on learning. It's similar to learning to cook by actually cooking. With Python, you can start writing and executing code right away, gaining practical experience that accelerates your progress.
10. The Power of Practice:
While Python's simplicity is a significant advantage for beginners, mastering any programming language still requires practice. It's comparable to learning to play a musical instrument. You might hit a few wrong notes at first, but with practice, you'll play beautiful music.
In summary, Python is not tough to learn, especially for beginners. Its user-friendly syntax, readability, extensive resources, and supportive community make it an excellent choice for those starting their programming journey. Remember, with dedication and practice, you can master Python and open doors to a world of coding possibilities.
If you're eager to start your Python learning journey, consider exploring the Python courses and certifications offered by ACTE Technologies. Their expert instructors and comprehensive curriculum can provide you with the guidance and knowledge you need to become proficient in Python.
2 notes
·
View notes
Text
Unleash Your Web Testing Potential with Selenium Training: Master the Art of Automation!
Education: How do I learn Selenium on my own?
Are you a web developer or a software testing enthusiast looking to upgrade your skills in web testing? Look no further than Selenium! Selenium is a powerful open-source tool that allows you to automate your web testing and maximize your efficiency. In this article, we will guide you through the process of learning Selenium on your own, so you can unleash your web testing potential and become a master of automation.
So, how can you get started? Here are some key points to consider on your self-learning journey:
1. Understand the Basics of Selenium
Before diving into Selenium, it's crucial to familiarize yourself with its fundamentals. Start by understanding what Selenium is and how it works. Selenium is a suite of tools used for automating web browsers, and it supports various programming languages such as Java, Python, C#, Ruby, and more. Knowing the core concepts and the underlying architecture of Selenium will provide you with a solid foundation for your learning journey.
2. Choose a Programming Language
Once you have a grasp of the basics, it's time to choose a programming language to work with Selenium. Java is a popular choice due to its simplicity and robustness, but you can also opt for Python, C#, or any other language that you are comfortable with. Selecting a programming language that aligns with your goals and prior experience can smoothen your learning curve and enhance your understanding of Selenium capabilities.
3. Set Up Your Development Environment
To start practicing Selenium, you need to set up your development environment. Install the necessary software, such as the chosen web browser (e.g., Chrome or Firefox), Integrated Development Environment (IDE) like Eclipse or Visual Studio Code, and the Selenium WebDriver library for your preferred programming language. Ensure that you have all the dependencies and configurations in place to prevent any obstacles during your learning process.
4. Explore Online Resources and Documentation
Learning Selenium on your own doesn't mean you have to reinvent the wheel. Utilize the vast array of online resources available to enhance your learning experience. Visit the official Selenium website, read through the documentation, and explore the comprehensive guides and tutorials provided. Online forums and communities like Stack Overflow can also be valuable sources to seek answers to your queries and learn from the experiences of other Selenium enthusiasts.
5. Hands-on Practice and Project-based Learning
Theory alone won't make you a Selenium expert. It's crucial to reinforce your knowledge through hands-on practice. Start by writing simple test scripts to automate basic web interactions, such as clicking buttons, filling forms, and navigating between pages. As you gain more confidence, challenge yourself with more complex scenarios and real-life web testing projects. Building a portfolio of projects will not only solidify your skills but also showcase your expertise to potential employers.
6. Join Selenium Communities and Network
Learning doesn't have to be a solitary journey. Engaging with fellow Selenium enthusiasts and professionals can greatly accelerate your learning process. Join Selenium communities and forums, participate in discussions, and network with like-minded individuals. Collaborating with others will expose you to diverse perspectives, practical insights, and valuable tips and tricks that can take your Selenium expertise to new heights.
7. Stay Updated with the Latest Trends
The field of web testing is constantly evolving, and staying updated with the latest trends and advancements is essential. Follow authoritative blogs, subscribe to relevant newsletters, and join webinars and conferences to keep yourself abreast of the latest happenings in the Selenium ecosystem. Knowing the current best practices and emerging technologies will enable you to adapt to changing industry demands and further refine your automation skills.
With these key steps and a determined mindset, you can embark on your Selenium learning journey with confidence. Mastering the art of automation through Selenium training at ACTE institute will not only enhance your web testing skills but also unlock a plethora of opportunities in the world of software development and testing. So don't wait any longer, unleash your web testing potential with Selenium, and pave your way to a successful and fulfilling career in automation!
3 notes
·
View notes
Text
The Best Python Libraries for Machine Learning in 2025 – What You Should Know
Python is everywhere in the world of tech—and for good reason. If you're exploring machine learning (ML) in 2025, one thing is clear: Python and its libraries are your best allies.
Whether you're a student, a self-learner, or someone looking to switch careers into tech, understanding the most effective tools in ML will give you a head start. This blog breaks down the top Python libraries used by professionals across India, especially in growing tech hubs like Hyderabad.
Why Do Python Libraries Matter in ML?
When building machine learning models, you don’t want to reinvent the wheel. Python libraries are collections of functions and tools designed to make your work easier.
They help you:
Clean and organize data
Train machine learning models
Visualize results
Make accurate predictions faster
Think of them like essential tools in a workshop. Instead of building everything from scratch, you pick up the tool that does the job best—and get to work.
Why Indian Professionals Should Care
India’s tech industry has embraced machine learning in a big way. From healthcare startups to global IT firms, organizations are using ML to automate tasks, make predictions, and personalize services.
In cities like Hyderabad, there’s growing demand for professionals with Python ML skills. Roles like Data Analyst, ML Engineer, and AI Developer now require hands-on knowledge of popular Python libraries. Knowing the right tools can set you apart in a competitive job market.
The Top 10 Python Libraries for ML in 2025
Here’s a list of libraries that are shaping the ML landscape this year:
1. Scikit-learn
A great starting point. This library simplifies common ML tasks like classification, regression, and clustering. It’s lightweight, reliable, and perfect for beginners.
2. TensorFlow
Developed by Google, TensorFlow is ideal for deep learning tasks. If you're working on image recognition, natural language processing, or neural networks, this is your go-to.
3. PyTorch
Favored by researchers and startups, PyTorch is known for its flexibility. It’s widely used in academic research and increasingly in production environments.
4. Pandas
If you’re working with spreadsheets or structured datasets, Pandas helps you manipulate and clean that data effortlessly.
5. NumPy
The foundation of scientific computing in Python. Most ML libraries depend on NumPy for numerical operations and matrix handling.
6. Matplotlib
Used to create basic plots and charts. It helps in visually understanding the performance of your models.
7. Seaborn
Built on Matplotlib, Seaborn allows for more attractive and informative statistical graphics.
8. XGBoost
A high-performance gradient boosting library. It’s used in many real-world systems for tasks like fraud detection and recommendation engines.
9. LightGBM
Faster and more memory-efficient than XGBoost. Especially useful for large datasets and real-time predictions.
10. OpenCV
Focused on computer vision. Great for image processing tasks like face detection, motion tracking, and object recognition.
Real-World Use Cases in India
These libraries are more than just academic. They’re being used every day in industries such as:
Retail – To personalize shopping experiences
Finance – For credit scoring and fraud prevention
Healthcare – In patient data analysis and disease prediction
EdTech – To deliver adaptive learning platforms
Government – For data-backed policy-making and smart city management
Companies in Hyderabad like Innominds, Darwinbox, and Novartis actively hire ML professionals skilled in these tools.
Where Should You Start?
If you’re new to machine learning, here’s a basic learning path:
Begin with NumPy and Pandas to understand data manipulation.
Learn Matplotlib and Seaborn for data visualization.
Dive into Scikit-learn to learn standard ML algorithms.
Once you’re confident, move on to TensorFlow, PyTorch, and XGBoost.
Starting with foundational tools makes it easier to understand complex ones later.
Tips to Learn These Tools Effectively
Here are a few things that helped many learners master these libraries:
Start with small projects like predicting house prices or student grades
Use publicly available datasets from Indian sources like data.gov.in
Practice regularly—30 minutes a day is better than none
Read documentation but also apply what you learn immediately
Watch tutorial videos to see how others solve ML problems step-by-step
Avoid the mistake of rushing into deep learning before understanding basic concepts.
How to Learn These Libraries Online
Online training is the best option if you want flexibility and practical learning. At Varniktech, you can access:
Instructor-led live sessions focused on real-world problems
Projects based on Indian industry use cases
Job preparation support, including mock interviews and resume building
Flexible batch timings for working professionals and students
Whether you're in Hyderabad or learning from another city, you can access everything online and complete your training from home.
Final Thoughts
Mastering the right Python libraries for machine learning can boost your career, help you build better projects, and make you stand out in job applications. With the tech industry growing rapidly in India, especially in cities like Hyderabad, there’s never been a better time to learn these tools.
The key is to start small, be consistent, and focus on building real projects. Once you’re confident with the basics, you can take on more advanced challenges and explore deep learning.
Want to dive deeper into machine learning with Python?
Visit varniktech.com to access structured courses, download free resources, and join our upcoming batch focused on Python for Machine Learning.
0 notes
Text
Finally got some time earlier this morning to work on my language project, which helped with some of the stress I was feeling. And that's funny to say, because holy hell was this morning's work some of the deepest-cutting agony I've gone through so far.
I finally have a CPython native module compiling from C, which can start to replace my misuse of stdlib ctypes. Source wise, it's going to be a big improvement! I finally have a platform where I can do stuff correctly and coherently - I have my foot in the door. Build system wise, it's jank as fuck and very not-good. I had to temporarily give up on making setuptools work, so I'm building the C modules with Make, so there's no way I could distribute as a wheel without fixing that. UV and Nix fought a lot about how to build and link things. I'm essentially working around every form of proper packaging right now with a promise to myself to figure it out later.
But... it works. I have a Python module written in pure C, which I can import and poke at with pytest, and use as an internal implementation library from the pure Python frontend. Which means I finally have a clean and acceptable boundary between memory management models, instead of the cursed hybrid approach I was using before. Truly a wild amount of effort to represent ASTs with native types, but that effort will immediately pay off with being able to manage the union logic natively. The cool thing about all this, aside from the immediate doors being unlocked with the storage model, is that now there's an inroad for prototyping a bunch of logic for things like the "simplify" function in frontend Python, and seamlessly port them to backend C without changing the test suite. It's also going to make transpilation... well, possible, frankly.
1 note
·
View note
Text
The Evolution of Programming: A Look at Current Trends
Programming has undergone an incredible transformation over the years. From the early days of basic machine language and assembly code to the sophisticated, high-level languages we use today, the world of programming has expanded in both scope and complexity. Modern programming isn’t just about writing lines of code; it’s about creating solutions to solve real-world problems, improving efficiency, and enabling innovation across industries.
One of the most notable shifts in the programming landscape has been the rise of high-level languages like Python, JavaScript, and Rust, which allow developers to write more efficient and readable code. Python, for instance, is widely praised for its simplicity, making it a favorite among beginners and professionals alike. Its extensive libraries and frameworks, such as Django for web development and TensorFlow for machine learning, enable developers to rapidly build applications without reinventing the wheel. Similarly, JavaScript remains the backbone of web development, powering everything from interactive websites to mobile applications through frameworks like React and Angular.
Another key trend in programming is the growing emphasis on automation and DevOps practices. Automation tools like Docker and Kubernetes have revolutionized how applications are built, deployed, and maintained. These tools allow developers to streamline workflows, reduce errors, and enhance collaboration between teams. DevOps, a culture that bridges the gap between development and operations teams, emphasizes continuous integration and continuous delivery (CI/CD). This ensures faster and more reliable software releases, contributing to more agile development cycles.
The increasing use of artificial intelligence (AI) and machine learning (ML) is also reshaping the programming world. In the past, building AI-powered applications required specialized knowledge, but with advancements in libraries like TensorFlow, Keras, and PyTorch, developers can now integrate machine learning models into their applications with ease. These technologies have wide applications, from natural language processing (NLP) in chatbots to predictive analytics in business solutions.
As more companies shift towards cloud computing, serverless architecture has emerged as a game-changer. Serverless computing enables developers to focus solely on writing code, without worrying about managing the underlying infrastructure. This is especially beneficial for building scalable and cost-efficient applications, making it easier for startups and established companies to scale rapidly.
Furthermore, security has become a critical concern in programming, particularly with the rise of cyber threats. Secure coding practices, such as input validation and encryption, are now part of the standard toolkit for developers. Technologies like blockchain have also gained popularity, offering a decentralized approach to data security and integrity.
In conclusion, programming is no longer just about writing code—it’s about building scalable, secure, and innovative solutions that address modern challenges. As the field continues to evolve, it promises even more exciting opportunities for developers and businesses alike.
0 notes
Text
Quantum [Assembly Language]
There's a lot of confusion as to what people are talking about about when it comes to programming with qBits; and a lot of [verbage used by professionals] that seems very *rough* transitioning from Digital space to Quantum space.
There are two ways to approach the qAssembly; sometimes directly through Qiskit, Circle, and Q#, and other ways through standard high-level Binary Languages like Python.
Python has a library which allows access to the Quantum Assembly layer, and developers may have a preference with this kind of interface until qAssembly is more understood.
Now; Unlike Binary-Languages, which at their rudimentary level is all about pushing 1s and 0s in interesting ways; and has been built on top of with high-level languages (which don't particularly care that typical computer systems are in Binary) makes a bunch of different ways for developers to interact with computers these days.
Right now, qAssembly is only at the level of trying to figure out how to control and use logic gates. Again; they're not binary logic gates which are more readily understood these days; they're quantum logic gates.
And most of our understanding is through binary systems simulating a quantum environment.
And the way that we understand *how they work* is through vectors, angles, and rotational matrices.
I heavily suspect this complexity can be broken down into simpler concepts, especially as we get more used to developing with quantum systems.
For *most* developers; they won't really swap to quantum systems until higher-level languages are developed for the quantum computing landscape.
For cutting-edge developers; we're literally reinventing the wheel. We're developing Quantum Systems and therefore; Quantum Languages, from the ground up.
So a lot of these explanations are very rough shod if you're already a software development expert. And aren't familiar with how Assembly and Binary Systems were developed originally.
However; if you've a basic understanding of the history, were looking much at the same paths that binary computers have taken to get here.
We have Quantum Systems (Very Large due to need for Super Cooled environments) and aren't readily accessible by the laymen (hence the simulated languages on binary systems).
The current way qAssembly works is by literally following a qBit through the various logic gates and seeing what they do.
And for many applications; if you actually understand the logic; it can have exponential speed gains in many applications in comparison to binary systems.
Yet; that's due to the qBit being a fundamentally different [object] than the Binary Bit. Binary bits can be 0 or 1 (low or High) yet a qBit is simply... Well ... It can be any sided Dice you want, as long as you label your states correctly.
This was a way to explain *where* our understanding of quantum computers are at currently.
0 notes
Text
An INSECURE Python Library That Makes Bitcoin Safer
Until now, every Bitcoin Improvement Proposal (BIP) that needed cryptographic primitives had to reinvent the wheel. Each one came bundled with its own custom Python implementation of the secp256k1 elliptic curve and related algorithms, each subtly different from one another. These inconsistencies introduced quiet liabilities and made reviewing BIPs unnecessarily complicated. This problem was…
0 notes
Text
A Practical Guide to Porting and Interacting with AI Large Language Models from DeepSeek to Qwen
In a previously published Technical Practice | A Complete Guide to Deploying the DeepSeek-R1 Large Language Model on the OK3588-C Development Board article, the porting and deployment of DeepSeek-R1 on Forlinx Embedded's OK3588-C development board were introduced, its effects were demonstrated, and performance evaluations were conducted. In this article, in-depth knowledge about DeepSeek-R1 will not only be continued to be shared but also porting methods on various platforms will be explored and more diverse interaction methods will be introduced to help better utilize large language models.
1. Porting Process
1.1 Deploying to the NPU Using RKLLM-Toolkit
RKLLM-Toolkit is a conversion and quantization tool specially developed by Rockchip for large language models (LLMs). It can convert trained models into the RKLLM format suitable for Rockchip platforms. This tool is optimized for large language models, enabling them to run efficiently on Rockchip's NPU (Neural Processing Unit). The deployment method mentioned in the previous article was NPU deployment through RKLLM-Toolkit. The specific method of operation is as follows:
(1) Download the RKLLM SDK:
First, download the RKLLM SDK package from GitHub and upload it to the virtual machine. SDK download link:
[GitHub-airockchip/rknn-llm]( https://github.com/airrockchip/rknn-llm)。
(2) Check the Python version:
Ensure that the installed SDK version is compatible with the target environment (currently only Python 3.8 or Python 3.10 is supported).
(3) Prepare the virtual machine environment:
Install therkllm-toolkitwheel in the virtual machine. The wheel package path is (rknn-llm-main\rkllm-toolkit).pip install rkllm_toolkit-1.1.4-cp38-cp38-linux_x86_64.whl
(4) Download the model:
Select the DeepSeek-R1 model to be deployed.git clone https://huggingface.co/deepseek-ai/DeepSeek-R1-Distill-Qwen-1.5B
(5) Use the sample code for model conversion:
In the path rknn-llm-main\examples\DeepSeek-R1-Distill-Qwen-1.5B_Demo, use the sample code provided by RKLLM-Toolkit for model format conversion.python generate_data_quant.py -m /path/to/DeepSeek-R1-Distill-Qwen-1.5B python export_rkllm.py
(6) Compile the executable program:
Compile the sample code directly by running the build-linux.sh script under the deploy directory (replace the cross-compiler path with the actual path). This will generate a folder in the directory, which contains the executable file and other folders. Perform cross-compilation to generate the executable file.
Cross-compile to generate an executable file../build-linux.sh
(7) Deploy the model:
Copy the compiled _W8A8_ RK3588.rkllm file and the librkllmrt.so dynamic library file (path: rknn-llm-main\rkllm-runtime\Linux\librkllm_api\aarch64) together into the build_linux_aarch64_Release folder generated after compilation, and then upload this folder to the target board.
Next, add execution permissions to the llm_demofile in the build_linux_aarch64_Release folder on the target board and execute it.chmod +x llm_demo ./llm_demo _W8A8_RK3588.rkllm 10000 10000
Demo 1
Advantages and Disadvantages:
Advantages: After being deployed to the NPU, the large language model can run efficiently, with excellent performance and less CPU resource consumption.
Disadvantages: Compared with other methods, the deployment process is a bit more complex and requires a strong technical background and experience.
1.2 One-Click Deployment to the CPU Using Ollama
Ollama is an open-source local large language model (LLM) running framework that supports running various open-source LLM models (such as LLaMA, Falcon, etc.) in a local environment and provides cross-platform support (macOS, Windows, Linux).
With Ollama, users can easily deploy and run various large language models without relying on cloud services. Although Ollama supports rapid deployment, since DeepSeek-R1 has not been optimized for the RK3588 chip, it can only run on the CPU, which may consume a relatively high amount of CPU resources. The specific method of operation is as follows:
(1) DownloadOllama:
Download and install Ollama as needed.Ollama,curl -fsSL https://ollama.com/install.sh | sh
If the download speed is slow, you can refer to the following mirror method for acceleration.curl -fsSL https://ollama.com/install.sh -o ollama_install.sh chmod +x ollama_install.sh sed -i 's|https://ollama.com/download/|https://github.com/ollama/ ollama/releases/download/v0.5.7/|' ollama_install.sh sh ollama_install.sh
(2) Check the Ollama results:
Confirm that Ollama is installed correctly and run relevant commands to view the deployment results.Ollama --help
(3) Download DeepSeek-R1:
Get the instructions for downloading the DeepSeek-R1 model from the officialOllama website.
(4) Run DeepSeek-R1:
Start the DeepSeek-R1 model through the Ollama command-line interface.ollama run deepseek-r1:1.5b
Demo 2
Advantages and Disadvantages:
Advantages: The deployment process is simple and fast, suitable for rapid testing and application.
Disadvantages: Since the model is not optimized for RK3588, running it on the CPU may lead to high CPU usage and affect performance.
Deploying Other Large Models on the FCU3001 Platform
Apart from DeepSeek-R1, Ollama also supports the deployment of other large language models, such as Qwen. This demonstrates the wide applicability of Ollama. Next, taking Qwen as an example, a large language model will be deployed on the FCU3001, an AI edge computing terminal launched by Forlinx Embedded, which is equipped with an NVIDIA processor (based on the NVIDIA Jetson Xavier NX processor).
Through its powerful computing power and optimized software support, FCU3001 can efficiently run the large language models supported by Ollama, such as Qwen. During the deployment process, the flexibility and ease-of-use provided by Ollama can be fully leveraged to ensure the stable and smooth operation of the large language model on the FCU3001. The steps are as follows:
(1) CUDA Installation:
The GPU of the NVIDIA Jetson Xavier NX can be used to run the model. Refer to the above-mentioned method for installing Ollama.sudo apt update sudo apt upgrade sudo apt install nvidia-jetpack -y
(2) Visit the Ollama official website:
Browse other models supported by Ollama.
(3) Select a version:
Choose the Qwen 1.8B version from the list of models supported by Ollama.
(4) Run the model:
In the Ollama environment, use the command ollama run qwen:1.8b to start the model.ollama run qwen:1.8b
Demo 3
3.Interaction Methods
In the previously mentioned deployment methods, the interaction is mainly based on serial port debugging. There is a lack of a graphical interface, so elements such as pictures and forms cannot be displayed, and historical conversations cannot be presented. To enhance the user experience, a more diverse interaction experience can be provided by integrating Chatbox UI or Web UI.
3.1 Chatbox UI
Chatbox is an AI assistant tool that integrates multiple language models and supports various models such as ChatGPT and Claude. It not only has the functions of local data storage and multilingual switching but also supports image generation and formats like Markdown and LaTeX, providing a user-friendly interface and team collaboration features. Chatbox supports Windows, macOS, and Linux systems. Users can quickly interact with large language models locally. The steps are as follows:
(1) Download Chatbox:
Download the appropriate installation package from the official Chatbox website (https://chatboxai.app/zh).
(2) Install and configure:
After the download is complete, get a file named Chatbox-1.10.4-arm64.AppImage, which is actually an executable file. You can run it after adding permissions, and then you can configure the LLM model under the local ollama API.chmod +x Chatbox-1.10.4-arm64.AppImage ./Chatbox-1.10.4-arm64.AppImage
(3) Q&A dialogue:
Users can communicate with the model through an intuitive graphical interface and experience a more convenient and smooth interaction.
Demo 4
3.2 Web UI
Web UI provides a graphical user interface through web pages or web applications, enabling users to easily interact with large language models via a browser. Users only need to access the corresponding IP address and port number in the browser to ask real-time questions. The steps are as follows:
(1) Set up the Web UI environment:
Configure the environment required for Web UI. It is recommended to use Python 3.11 for Web UI. So, use Miniconda to create a virtual environment with Python == 3.11.
Install Minicondawget https://repo.anaconda.com/miniconda/Miniconda3-latest-Linux-aarch64.sh chmod +x Miniconda3-latest-Linux-aarch64.sh ./Miniconda3-lates
Set up theWeb UIenvironment.conda create --name Web-Ui python=3.11 conda activate Web-Ui pip install open-webui -i https://pypi.tuna.tsinghua.edu.cn/simple
(2) Start the Web UI:
Use ''open-webui serve'' to start the Web UI application. The IP address and port number of the server are 0.0.0.0:8080.open-webui serve
If the information in the following red box appears, it means the startup is successful.
(3) Access the Web UI:
Enter the IP address and port number in the browser to open the Web UI interface and start interacting with the large language model.
Register an account
Demo 5
4. Summary
This article comprehensively demonstrates various transplantation methods of large language models on the OK3588-C development board and the FCU3001 edge AI gateway, and introduces how to enhance the user experience through multiple interaction methods such as Chatbox UI and Web UI.
Forlinx Embedded has launched multiple embedded AI products, such as development boards like OK3588-C, OK3576-C, OK-MX9352-C, OK536-C, and the AI edge computing terminal FCU3001. The computing power ranges from 0.5 TOPS to 21 TOPS, which can meet the AI development needs of different customers. If you are interested in these products, please feel free to contact us. Forlinx Embedded will provide you with detailed technical support and guidance.
Dear friends, we have created an exclusive embedded technical exchange group on Facebook, where our experts share the latest technological trends and practical skills. Join us and grow together!
0 notes
Text
What is a Backend Framework? A Complete Guide
Websites and applications need robust infrastructures to perform optimally. A backend framework plays a crucial role in this realm, handling server-side operations that ensure data integrity, security, and seamless communication with front-end interfaces. This guide explores what a backend framework is, why it matters, and how to choose the best one for your project.
Understanding Backend Frameworks
A backend framework is a set of tools, libraries, and conventions designed to streamline server-side development. It provides a structured environment where developers can write and maintain code more efficiently. These frameworks handle databases, user authentication, security, and other intricate tasks required to power modern websites and applications.
Because back-end code often deals with complex logic, frameworks exist to reduce repetitive work. They offer built-in modules for common functions, such as routing requests or managing sessions. This organization allows developers to focus on unique features rather than reinventing the wheel every time they build a new application.
Why Backend Frameworks Are Important?
Efficiency
Backend frameworks come with pre-coded components and libraries. These resources save time by preventing developers from coding everything from scratch, speeding up the overall development process.
2. Security
Server-side code often handles sensitive information, like passwords and payment details. Well-maintained frameworks incorporate security best practices and updates, offering built-in protection against common threats such as SQL injection or cross-site scripting.
3. Scalability
As your application grows, so do its requirements. A robust backend framework accommodates increasing traffic, data, and user demands without compromising performance.
4. Consistency
Frameworks encourage a uniform coding style across development teams. This consistency reduces confusion, simplifies debugging, and helps new developers get up to speed quickly.
Popular Backend Frameworks
Many programming languages have their own backend frameworks. Here are a few well-known examples:
Node.js (Express, NestJS)
Node.js uses JavaScript, making it popular among developers familiar with front-end technologies. Express is a minimalist framework, while NestJS offers a more opinionated, structured approach.
Python (Django, Flask)
Python’s readability and versatility make it a favorite in web development. Django is a “batteries-included” framework with built-in tools for most tasks. Flask is more lightweight, allowing developers to pick and choose additional libraries based on project needs.
Ruby (Ruby on Rails)
Rails emphasizes convention over configuration. It’s known for its ability to bootstrap projects quickly, offering a clean structure and a host of built-in features that streamline development.
PHP (Laravel, Symfony)
PHP remains a popular choice for web projects due to its widespread hosting support. Laravel provides an elegant syntax and comprehensive toolset, while Symfony offers flexibility and modularity.
The Role of Development Teams
Sometimes, building a powerful, reliable system requires specialized knowledge. Whether you’re a startup or an established enterprise, you may benefit from professional back-end expertise. If you are seeking partners in Backend web development ottawa, you want to ensure they have a solid track record, updated skill sets, and a deep understanding of your chosen framework’s best practices.
Similarly, teams that excel in Backend web development ontario often combine expertise in front-end technologies, cloud deployment, and DevOps methodologies. Their multifaceted knowledge helps ensure a smooth development cycle and maintainable, long-term solutions.
Best Practices for Using Backend Frameworks
Keep Dependencies Updated
Regularly update libraries and plugins to guard against security vulnerabilities. Use dependency management tools to track new releases and updates.
Use Version Control
Systems like Git are essential for modern development. They help teams collaborate, track changes, and revert to earlier versions if something goes wrong.
Adopt Testing and CI/CD
Automated testing ensures code quality, while Continuous Integration and Continuous Deployment pipelines streamline updates. This combination greatly reduces bugs and improves reliability.
Document Your Code
Good documentation fosters better collaboration. Future developers — or even your future self — will thank you when they need to revisit or maintain the code.
Backend frameworks are the backbone of modern web applications, providing the tools and structure necessary for efficient, secure, and scalable development. By choosing a framework that aligns with your team’s skills, project requirements, and performance needs, you can significantly reduce development time and bolster the stability of your application.
0 notes
Text
What is python and its importance? Before that I will tell you the best institute for python course in Chandigarh

What is python?
Python is a high-level, interpreted programming language known for its simplicity and readability and versatility . It was created by Guido van Rossum and first released in 1991. Python's design philosophy emphasizes code readability and simplicity, making it a popular choice for both beginners and experienced developers .
Importance of python
Ease of Learning and Use: Python's syntax is clean and easy to understand, making it a great language for beginners. It allows new programmers to quickly pick it up and start coding without getting bogged down by complex syntax rules.
Versatility: Python is a general-purpose language, meaning it can be used in various applications, from web development to data analysis, machine learning, automation, and scientific computing.
Large Ecosystem and Libraries: Python has a rich set of libraries and frameworks like Django (for web development), NumPy and Pandas (for data science), TensorFlow and PyTorch (for machine learning), and Matplotlib (for data visualization). This allows developers to quickly solve problems without reinventing the wheel.
Data Science and Machine Learning: Python has become the go-to language for data science, machine learning, and artificial intelligence due to libraries like Pandas, NumPy, Scikit-learn, TensorFlow, and others. It simplifies complex tasks, enabling rapid prototyping and research.
Community and Support: Python has one of the largest programming communities, meaning it’s easy to find resources, tutorials, and help when you need it. The community-driven development ensures that the language keeps evolving with new features and improvements.
Cross-Platform: Python is cross-platform, meaning code written on one operating system (e.g., Windows) can be run on another (e.g., Linux, macOS) with minimal changes

Now I will tell you the best institute for python course in Chandigarh

Excellence Technology is a leading EdTech (Educational Technology) company dedicated to empowering individuals with cutting-edge IT skills and bridging the gap between education and industry demands. Specializing in IT training, career development, and placement assistance, the company equips learners with the technical expertise and practical experience needed to thrive in today’s competitive tech landscape.
We provide IT courses like Python , Full Stack Development, Web Design, Graphic Design and Digital Marketing
Contact Us for more details: 93177-88822
Extech Digital is a leading software development company dedicated to empowering individuals with cutting-edge IT skills and bridging the gap between education and industry demands. Specializing in IT training, career development, and placement assistance, the company equips learners with the technical expertise and practical experience needed to thrive in today’s competitive tech landscape.
We provide IT courses like Python , Full Stack Development, Web Design, Graphic Design and Digital MarketingContact Us for more details: 93177-88822

Excellence Academy is a leading software development company dedicated to empowering individuals with cutting-edge IT skills and bridging the gap between education and industry demands. Specializing in IT training, career development, and placement assistance, the company equips learners with the technical expertise and practical experience needed to thrive in today’s competitive tech landscape.
We provide IT courses like Python , Full Stack Development, Web Design, Graphic Design and Digital Marketing
About Author
Saksham
Python AI Developer | 2+ Years of Experience | Excellence Technology
Professional Summary
Saksham is a passionate Python AI Developer with 2+ years of hands-on experience in designing, developing, and deploying intelligent solutions at Excellence Technology, a leading EdTech company specializing in IT training and career development. Proficient in leveraging Python, machine learning (ML), and artificial intelligence (AI) frameworks, Saksham combines technical expertise with a problem-solving mindset to deliver innovative, scalable, and industry-aligned AI applications.
0 notes
Text
Building a Full-Stack Web Application with Django and React
The process of developing web applications has dramatically evolved over the past few years, with developers now opting for technologies that allow them to create powerful, scalable, and maintainable applications. A popular choice in the web development community is combining Django for the backend and React for the frontend to build full-stack web applications. This combination of tools provides an efficient approach to create modern, data-driven web applications that meet both user experience and performance demands.
In this article, we will explore why Django and React are an excellent pair for full-stack web development and walk through the steps involved in integrating these two technologies. We will also discuss the advantages of using Django as the backend and React for the frontend, how they interact, and how developers can benefit from this powerful stack.
Why Choose Django and React for Full-Stack Development?
Django, a high-level Python web framework, and React, a JavaScript library for building user interfaces, are both incredibly powerful tools when used in tandem. Each technology brings unique strengths to the table:
Django: A Robust Backend Framework
Django is a well-established, high-level Python framework used to build secure and scalable web applications. It follows the "batteries-included" philosophy, meaning it comes with many built-in features that allow developers to focus on business logic instead of reinventing the wheel. Some of the key benefits of using Django for backend development are:
Rapid Development: Django includes a vast number of pre-built components, including authentication, form handling, database migrations, and an admin panel. This means developers can build applications more quickly and efficiently.
Security: Django has built-in features like protection against common security vulnerabilities such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF), which makes it a secure choice for backend development.
Scalability: Django’s architecture is designed to scale. As your web application grows in size and complexity, Django can handle large amounts of data and traffic, making it ideal for large-scale web applications.
Built-in ORM (Object-Relational Mapping): Django simplifies database interactions by providing an ORM that maps Python objects to database tables, making it easier for developers to interact with databases without needing to write raw SQL queries.
React: A Dynamic Frontend Library
React is a declarative, component-based JavaScript library created by Facebook that is widely used for building interactive user interfaces. React allows developers to build reusable UI components and manage the application’s state efficiently. Some of the reasons why React is ideal for the frontend are:
Component-Based Architecture: React’s component-based structure enables developers to build modular and reusable UI elements, making the code easier to maintain and scale.
Efficient Rendering: React uses a virtual DOM (Document Object Model) to optimize rendering. When a component’s state changes, React updates only the necessary parts of the UI, resulting in faster rendering and improved performance.
Declarative Syntax: React allows developers to describe what the UI should look like for a given state, simplifying the process of building dynamic interfaces.
Ecosystem: React has a rich ecosystem of libraries, tools, and extensions that help with routing (React Router), state management (Redux, Context API), and even testing (Jest).
How Django and React Work Together
When combining Django with React, the architecture typically follows a client-server model where:
Django acts as the backend server, handling requests, business logic, authentication, data processing, and interacting with the database.
React functions as the frontend, responsible for rendering the user interface and making API calls to Django to fetch and display data.
The communication between the frontend and backend happens through APIs. Django provides the backend API, often built using Django REST Framework (DRF), to serve data in JSON format. React, on the other hand, makes HTTP requests (usually via the fetch API or Axios) to the Django backend to retrieve this data and update the UI accordingly.
For example, if you’re building a blog application, Django will handle the creation, storage, and retrieval of blog posts from the database, while React will handle rendering the list of blog posts and managing user interactions, such as submitting new posts or commenting on existing ones.
Integration Process
The integration of Django and React typically follows these steps:
Backend Setup with Django: You first build the backend logic, including models, views, and database interactions. Using Django REST Framework, you create API endpoints that expose the necessary data to the frontend. For instance, if you are building a blog, you would create API endpoints that allow React to fetch blog posts, submit new posts, and update existing posts.
Frontend Setup with React: In parallel, you develop the user interface using React. You create components that will display data from the Django API. These components might include things like a list of blog posts, a form for creating new posts, and buttons for user interactions.
Connecting Frontend and Backend: To connect React to Django, you set up HTTP requests in React to fetch data from the Django API. This can be done using the fetch API or a library like Axios. When React receives the data, it updates the state and re-renders the UI to reflect the new information.
Running Both Servers: Typically, Django runs on one port (e.g., localhost:8000), and React runs on another (e.g., localhost:3000). While you’re developing the application, you’ll run both servers simultaneously. For production, you would typically use Django to serve the React app by using tools like webpack or by building the React app into static files that Django can serve.
Advantages of Using Django and React Together
The combination of Django and React offers several benefits:
Separation of Concerns: By using Django for the backend and React for the frontend, you can separate business logic from presentation. This makes the codebase more modular and easier to maintain.
Improved User Experience: React’s efficient rendering and component-based architecture allow you to build highly interactive, fast, and smooth user interfaces. This results in a better user experience compared to traditional server-side rendered pages.
Faster Development: Django’s built-in features, along with React’s reusable components, enable faster development of both the backend and frontend. Django handles the server-side logic, while React focuses on delivering a dynamic and responsive UI.
Scalability: Django and React both offer scalability in different ways. Django’s architecture supports large-scale applications, while React’s virtual DOM ensures fast updates to the UI as the application grows in complexity.
API-Driven Development: The API-driven approach used by Django and React enables you to decouple the frontend from the backend. This makes it easier to scale the application or switch out one part of the stack without affecting the other.
Key Considerations for Full-Stack Development with Django and React
While the combination of Django and React is powerful, there are some considerations to keep in mind:
Cross-Origin Resource Sharing (CORS): During development, Django and React will run on different ports, so you'll need to configure CORS settings to allow the frontend (React) to make requests to the backend (Django).
Authentication: Handling authentication and authorization across the backend and frontend requires careful consideration. Django can handle user authentication on the server side, but you’ll need to implement token-based authentication (such as JWT) to authenticate API requests from the React frontend.
Deployment: When you’re ready to deploy the application, you need to think about how to serve both the Django backend and React frontend. Tools like Docker, Nginx, and cloud platforms like Heroku, AWS, or DigitalOcean can be used to deploy full-stack applications.
Conclusion
Building a full-stack web application with Django and React allows developers to harness the strengths of both technologies. Django provides a solid and secure backend, while React delivers an interactive, dynamic frontend. Together, they offer an efficient development process, scalability, and a great user experience. Whether you are developing a simple web app or a complex, data-driven platform, this combination is an excellent choice.
If you're looking to further enhance your skills in full-stack development and want hands-on guidance with Django, React, and other industry-standard technologies, consider enrolling in Pune’s Best IT Training Institute. They offer comprehensive courses on full-stack development that will help you build real-world applications, work with databases, and gain experience with modern web development techniques.
With the right training, you can unlock your potential as a skilled full-stack developer and be prepared to tackle the challenges of building robust, scalable web applications using Django and React.
0 notes
Text
To Be or Not to Be: Today the Skull Used in the Monologue Hamlet Has Been Replaced by a Gadget
"Ai technology mimics human beings, trying to reproduce emotions, feelings and reflections in search of answers."
I don't think many people have noticed the change from skull to gadget in this AI revolution. In this simple fact, humanity will be able to accept this dramatic change, which has equal or more importance than the discovery of fire, the invention of the wheel, and electricity. Society must be aware of the changes and its individual rights in the face of AI in society.
In the 21st century, Shakespeare's iconic monologue "To be or not to be" has found a new stage—a gadget that fits in the palm of our hands. As we delve into the realm of Artificial Intelligence (AI), it becomes evident that while AI can mimic humans to a certain extent, it still has a long way to go before it can truly express the depth of human feelings and emotions exhibited by the genius William Shakespeare.
Evolution of AI Resources for Men's Mimicry:
To achieve the level of mimicry seen in Shakespeare's monologue, AI relies on a variety of resources and techniques. Let's explore some of the key components driving the development of men's mimicry and the journey towards achieving Artificial General Intelligence (AGI).
Discover how AI mimics human emotions in the 21st century and the resources and techniques driving men's mimicry development. Unleash the power of NLP, LLM, neural networks, deep learning, and more.
1. Natural Language Processing (NLP):
NLP enables machines to understand and process human language, allowing AI to analyze and respond to text or speech inputs. By leveraging NLP, AI systems can mimic human conversation, but true emotional depth remains a challenge.
2. Language Models (LLM):
LLMs play a vital role in AI's mimicry journey by training models to generate human-like text. These models learn from vast amounts of data, including literature, to produce coherent and contextually relevant responses. However, capturing the intricate nuances of human emotions still poses a significant hurdle.
3. Neural Networks:
Neural networks form the backbone of AI's ability to mimic human behavior. Through layers of interconnected nodes, neural networks can learn patterns, recognize emotions from facial expressions, and even generate human-like speech. Yet, the complexity of human emotions continues to elude complete replication.
4. Deep Learning:
Deep learning algorithms empower AI systems to analyze vast amounts of data, extract meaningful patterns, and make predictions. By mimicking human cognitive processes, deep learning algorithms contribute to the development of more sophisticated AI systems. However, the inner workings of human emotions remain a mystery yet to be fully understood.
5. Machine Learning (ML):
ML algorithms enable AI systems to improve their performance over time by learning from data and experiences. Through ML, AI can refine its mimicry capabilities, but bridging the gap between reproducing human emotions and truly experiencing them remains a formidable challenge.
6. Libraries, Algorithms, and More:
A plethora of libraries, algorithms, and tools exist to support AI development. From TensorFlow and PyTorch to advanced algorithms like GANs (Generative Adversarial Networks), researchers and developers have at their disposal an extensive toolkit to advance the field of AI and its mimicry capabilities.
My Prompts Showcase (Python Snippet):
```python
import openai
prompt = '''
To be, or not to be: that is the question:
Whether 'tis nobler in the mind to suffer
The slings and arrows of outrageous fortune,
Or to take arms against a sea of troubles,
And by opposing end them? To die: to sleep;
No more; and by a sleep to say we end
The heart-ache and the thousand natural shocks
That flesh is heir to, 'tis a consummation
Devoutly to be wish'd. To die, to sleep;
To sleep: perchance to dream: ay, there's the rub;
AI Prompt Generation Showcase:
prompt = "To be, or not to be: that is the question:"
response = openai.Completion.create(
engine="davinci-codex",
prompt=prompt,
max_tokens=50,
temperature=0.7,
n = 3,
stop=None,
temperature=0.7
)
for choice in response['choices']:
print(choice['text'])
```
AI in the 21st century has made strides in mimicking human emotions, yet AGI has a long way to go to match the depth of a Shakespearean monologue. Through resources like NLP, LLM, neural networks, deep learning, ML, libraries, and algorithms, AI continues to evolve, inching closer to unraveling the enigma of human emotions. Let's appreciate the genius of William Shakespeare and the unique capabilities that make us human. Together, let's push the boundaries of AI, unlocking new possibilities in the realm of human mimicry.
Join the conversation by sharing your thoughts and insights in the comments below. And remember, while AI can mimic, it is our humanity that truly sets us apart.
RDIDINI PROMPT ENGINEER
0 notes
Text
Why Python Reigns Supreme: A Comprehensive Analysis
Python has grown from a user-friendly scripting language into one of the most influential and widely adopted languages in the tech industry. With applications spanning from simple automation scripts to complex machine learning models, Python’s versatility, readability, and power have made it the reigning champion of programming languages. Here’s a comprehensive look at why Python has reached this esteemed position and why it continues to dominate.
Considering the kind support of Learn Python Course in Hyderabad Whatever your level of experience or reason for switching from another programming language, learning Python gets much more fun.
1. Readable Syntax, Rapid Learning Curve
Python is celebrated for its straightforward, readable syntax, which resembles English and minimizes the use of complex symbols and structures. Unlike languages that can be intimidating to beginners, Python's design philosophy prioritizes readability and simplicity, making it approachable for people new to programming. This ease of understanding is crucial in educational settings, where Python has become a top choice for teaching programming fundamentals.
Python’s gentle learning curve allows beginners to focus on mastering logic and problem-solving rather than wrestling with complicated syntax, making it a universal favorite.
2. A Thriving Ecosystem of Libraries and Tools
Python boasts a rich ecosystem of libraries and tools, which provide ready-made functionalities that allow developers to get straight to work without reinventing the wheel. For instance:
Data Science and Machine Learning: Libraries like pandas, NumPy, scikit-learn, and TensorFlow offer robust tools for data manipulation, statistical analysis, and machine learning.
Web Development: Frameworks like Django and Flask make it simple to build and deploy scalable web applications.
Automation: Tools like Selenium and BeautifulSoup enable developers to automate tasks, scrape websites, and handle repetitive processes efficiently.
Visualization: Libraries such as Matplotlib and Seaborn offer powerful ways to create data visualizations, a crucial feature for data analysts and scientists.
These libraries, created and maintained by a vast community, save developers time and effort, allowing them to focus on innovation rather than the details of implementation.
3. Multi-Paradigm Language with Versatile Use Cases
Python is inherently a multi-paradigm language, which means it supports procedural, object-oriented, and functional programming. This flexibility allows developers to choose the best approach for their projects and adapt their programming style as necessary. Python is ideal for a wide range of applications, including:
Web and App Development: Python’s frameworks, such as Django and Flask, are widely used for building web applications and APIs.
Data Science and Analytics: With its powerful libraries, Python is the language of choice for data analysis, manipulation, and visualization.
Machine Learning and Artificial Intelligence: Python has become the industry standard for machine learning, AI, and deep learning, with major frameworks optimized for Python.
Automation and Scripting: Python excels in automating repetitive tasks, from data scraping to system administration.
This adaptability means that developers can work across projects in different domains, without needing to switch languages, increasing productivity and consistency.
. Enrolling in the Best Python Certification Online can help people realise Python's full potential and gain a deeper understanding of its complexities.
4. The Power of the Community
Python’s growth and success are deeply rooted in its active, vibrant community. This worldwide community contributes to Python’s extensive documentation, support forums, and open-source projects, ensuring that resources are available for developers at all levels. Additionally, Python’s open-source nature allows anyone to contribute to its libraries, frameworks, and core features. This constant improvement means Python stays relevant, innovative, and well-supported.
The Python community is also highly collaborative, hosting events, tutorials, and conferences like PyCon, which facilitate learning and networking. With a community of developers continuously advancing the language and sharing their knowledge, Python remains a constantly evolving, resilient language.
5. Dominance in Data Science, Machine Learning, and AI
Python’s popularity surged with the rise of data science, machine learning, and AI. As industries recognized the value of data-driven decision-making, demand for data analytics and predictive modeling grew rapidly. Python, with its well-developed libraries like pandas, scikit-learn, TensorFlow, and Keras, became the preferred language in these fields. These libraries allow developers and researchers to experiment, build, and scale machine learning models with ease, fostering innovation in AI-driven industries.
The popularity of Jupyter Notebooks also propelled Python’s use in data science. This interactive development environment allows users to combine code, visualizations, and explanations in a single document, making data exploration and sharing more efficient. As a result, Python has become a staple in data science courses, research, and industry applications.
6. Cross-Platform Compatibility and Open Source Advantage
Python’s cross-platform compatibility allows it to run on various operating systems like Windows, macOS, and Linux, making it ideal for development in diverse environments. Python code is generally portable, which means that scripts written on one platform can often run on another with little to no modification, providing developers with added flexibility and ease of deployment.
As an open-source language, Python is free to use and backed by a vast global community. The lack of licensing costs makes Python an accessible choice for startups, educational institutions, and corporations alike, contributing to its widespread adoption. Python’s open-source nature also allows the language to evolve rapidly, with new features and libraries continuously added by its active user base.
Python’s Future as a Leading Language
Python’s blend of readability, flexibility, and community support has catapulted it to the top of the programming world. Its stronghold in data science, AI, web development, and automation ensures it will remain relevant across a broad spectrum of industries. With its rapid development, Python is poised to tackle emerging challenges, from ethical AI to quantum computing. As more people turn to Python to solve complex problems, it will continue to be a language that’s easy to learn, powerful to use, and versatile enough to stay at the cutting edge of technology.
Whether you’re a newcomer or a seasoned developer, Python is a language that opens doors to endless possibilities in the world of programming.
0 notes