#browser extensions for bug hunters
Explore tagged Tumblr posts
Text
Browser Extensions for Bug Hunters
Hello everyone! Welcome to Pentestguy. In this article, we will see the browser extensions for bug hunters. Which makes tasks easy in a more efficient way. There are many browser extensions available for bug bounty hunters or pentesters but here we are discussing the top browser extensions which help bug hunters. DotGit: DotGit is a powerful extension that allows you to check if a website has…
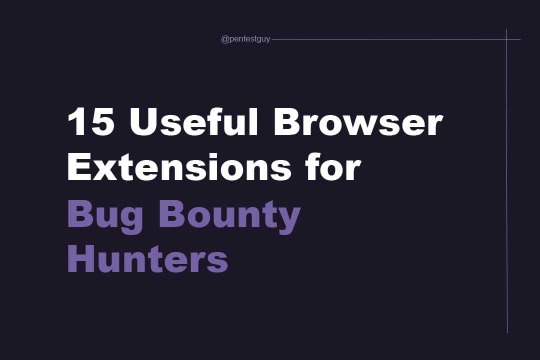
View On WordPress
1 note
·
View note
Link
Introduction
We have been frequently said that between two big e-commerce platforms of Malaysia (Shopee and Lazada), one is normally cheaper as well as attracts good deal hunters whereas other usually deals with lesser price sensitive.
So, we have decided to discover ourselves… in the battle of these e-commerce platforms!
For that, we have written a Python script with Selenium as well as Chrome driver for automating the scraping procedure and create a dataset. Here, we would be extracting for these:
Product’s Name
Product’s Name
Then we will do some basic analysis with Pandas on dataset that we have extracted. Here, some data cleaning would be needed and in the end, we will provide price comparisons on an easy visual chart with Seaborn and Matplotlib.
Between these two platforms, we have found Shopee harder to extract data for some reasons: (1) it has frustrating popup boxes that appear while entering the pages; as well as (2) website-class elements are not well-defined (a few elements have different classes).
For the reason, we would start with extracting Lazada first. We will work with Shopee during Part 2!
Initially, we import the required packages:
# Web Scraping from selenium import webdriver from selenium.common.exceptions import * # Data manipulation import pandas as pd # Visualization import matplotlib.pyplot as plt import seaborn as sns
Then, we start the universal variables which are:
Path of a Chrome web driver
Website URL
Items we wish to search
webdriver_path = 'C://Users//me//chromedriver.exe' # Enter the file directory of the Chromedriver Lazada_url = 'https://www.lazada.com.my' search_item = 'Nescafe Gold refill 170g' # Chose this because I often search for coffee!
After that, we would start off the Chrome browser. We would do it with a few customized options:
# Select custom Chrome options options = webdriver.ChromeOptions() options.add_argument('--headless') options.add_argument('start-maximized') options.add_argument('disable-infobars') options.add_argument('--disable-extensions') # Open the Chrome browser browser = webdriver.Chrome(webdriver_path, options=options) browser.get(Lazada_url)
Let’s go through about some alternatives. The ‘— headless’ argument helps you run this script with a browser working in its background. Usually, we would suggest not to add this argument in the Chrome selections, so that you would be able to get the automation as well as recognize bugs very easily. The disadvantage to that is, it’s less effective.
Some other arguments like ‘disable-infobars’, ‘start-maximised’, as well as ‘— disable-extensions’ are included to make sure smoother operations of a browser (extensions, which interfere with the webpages particularly can disrupt the automation procedure).
Running the shorter code block will open your browser.
When the browser gets opened, we would require to automate the item search. The Selenium tool helps you find HTML elements with different techniques including the class, id, CSS selectors, as well as XPath that is the XML path appearance.
Then how do you recognize which features to get? An easy way of doing this is using Chrome’s inspect tool:
search_bar = browser.find_element_by_id('q') search_bar.send_keys(search_item).submit()
That was the easy part. Now a part comes that could be challenging even more in case, you try extract data from Shopee website!
For working out about how you might scrape item names as well as pricing from Lazada, just think about how you might do that manually. What you can? Let’s see:
Copy all the item names as well as their prices onto the spreadsheet table;
Then go to next page as well as repeat the initial step till you’ve got the last page
That’s how we will do that in the automation procedure! To perform that, we will have to get the elements having item names as well as prices with the next page’s button.
With the Chrome’s inspect tool, it’s easy to see that product titles with prices have class names called ‘c16H9d’ as well as ‘c13VH6’ respectively. So, it’s vital to check that the similar class of names applied to all items on a page to make sure successful extraction of all items on a page.
item_titles = browser.find_elements_by_class_name('c16H9d') item_prices = browser.find_elements_by_class_name('c13VH6')
After that, we have unpacked variables like item_titles as well as item_prices in the lists:
# Initialize empty lists titles_list = [] prices_list = [] # Loop over the item_titles and item_prices for title in item_titles: titles_list.append(title.text) for price in item_prices: prices_list.append(prices.text)
When we print both the lists, it will show the following outputs:
[‘NESCAFE GOLD Refill 170g x2 packs’, ‘NESCAFE GOLD Original Refill Pack 170g’, ‘Nescafe Gold Refill Pack 170g’, ‘NESCAFE GOLD Refill 170g’, ‘NESCAFE GOLD REFILL 170g’, ‘NESCAFE GOLD Refill 170g’, ‘Nescafe Gold Refill 170g’, ‘[EXPIRY 09/2020] NESCAFE Gold Refill Pack 170g x 2 — NEW PACKAGING!’, ‘NESCAFE GOLD Refill 170g’] [‘RM55.00’, ‘RM22.50’, ‘RM26.76’, ‘RM25.99’, ‘RM21.90’, ‘RM27.50’, ‘RM21.88’, ‘RM27.00’, ‘RM26.76’, ‘RM23.00’, ‘RM46.50’, ‘RM57.30’, ‘RM28.88’]
When we complete scraping from the page, it’s time to move towards the next page. Also, we will utilize a find_element technique using XPath. The use of XPath is very important here as next page buttons have two classes, as well as a find_element_by_class_name technique only gets elements from the single class.
It’ very important that we require to instruct the browser about what to do in case, the subsequent page button gets disabled (means in case, the results are revealed only at one page or in case, we’ve got to the end page results.
try: browser.find_element_by_xpath(‘//*[@class=”ant-pagination-next” and not(@aria-disabled)]’).click() except NoSuchElementException: browser.quit()
So, here, we’ve commanded the browser for closing in case the button gets disabled. In case, it’s not got disabled, then the browser will proceed towards the next page as well as we will have to repeat our scraping procedure.
Luckily, the item that we have searched for is having merely 9 items that are displayed on a single page. Therefore, our scraping procedure ends here!
Now, we will start to analyze data that we’ve extracted using Pandas. So, we will start by changing any two lists to the dataframe:
dfL = pd.DataFrame(zip(titles_list, prices_list), columns=[‘ItemName’, ‘Price’])
If the printing of dataframe is done then it shows that our scraping exercise is successful!
When the datasets look good, they aren’t very clean. In case, you print information of a dataframe through Pandas .info() technique it indicates that a Price column category is the string object, instead of the float type. It is very much expected because every entry in a Price column has a currency symbol called ‘RM’ or Malaysian Ringgit. Though, in case the Pricing column is not the float or integer type column, then we won’t be able to scrape any statistical characteristics on that.
`Therefore, we will require to remove that currency symbol as well as convert the whole column into the float type using the following technique:
dfL[‘Price’] = dfL[‘Price’].str.replace(‘RM’, ‘’).astype(float)
Amazing! Although, we need to do some additional cleaning. You could have observed any difference in the datasets. Amongst the items, which is actually the twin pack that we would require to remove from the datasets.
Data cleaning is important for all sorts of data analysis as well as here we would remove entries, which we don’t require with the following code:
# This removes any entry with 'x2' in its title dfL = dfL[dfL[‘ItemName’].str.contains(‘x2’) == False]
Though not required here, you can also make sure that different items, which seem are the items that we precisely searched for. At times other associated products might appear in the search lists, particularly if the search terms aren’t precise enough.
For instance, if we would have searched ‘nescafe gold refill’ rather than ‘nescafe gold refill 170g’, then 117 items might have appeared rather than only 9 that we had scraped earlier. These extra items aren’t some refill packs that we were looking for however, rather capsule filtering cups instead.
Nevertheless, this won’t hurt for filtering your datasets again within the search terms:
dfL = dfL[dfL[‘ItemName’].str.contains(‘170g’) == True]
In the final game, we would also make a column called ‘Platform’ as well as allocate ‘Lazada’ to all the entries here. It is completed so that we could later group different entries by these platforms (Shopee and Lazada) whenever we later organize the pricing comparison between two platforms.
dfL[‘Platform’] = ‘Lazada’
Hurrah! Finally, our dataset is ready and clean!
Now, you need to visualize data with Seaborn and Matplotlib. We would be utilizing the box plot because it exclusively represents the following main statistical features (recognized as a five number summary) in this chart:
Highest Pricing
Lowest Pricing
Median Pricing
25th as well as 75th percentile pricing
# Plot the chart sns.set() _ = sns.boxplot(x=’Platform’, y=’Price’, data=dfL) _ = plt.title(‘Comparison of Nescafe Gold Refill 170g prices between e-commerce platforms in Malaysia’) _ = plt.ylabel(‘Price (RM)’) _ = plt.xlabel(‘E-commerce Platform’) # Show the plot plt.show()
Every box represents the Platform as well as y-axis shows a price range. At this time, we would only get one box, because we haven’t scraped and analyzed any data from a Shopee website.
We could see that item prices range among RM21–28, having the median pricing between RM27–28. Also, we can see that a box has shorter ‘whiskers’, specifying that the pricing is relatively constant without any important outliers. To know more about understanding box plots, just go through this great summary!
That’s it now for this Lazada website! During Part 2, we will go through the particular challenges while extracting the Shopee website as well as we would plot one more box plot used for Shopee pricing to complete the comparison!
Looking to scrape price data from e-commerce websites? Contact Retailgators for eCommerce Data Scraping Services.
source code: https://www.retailgators.com/how-to-scrape-e-commerce-sites-using-web-scraping-to-compare-pricing-using-python.php
0 notes
Link
If you're a penetration tester, there are numerous tools you can use to help you accomplish your goals.
From scanning to post-exploitation, here are ten tools you must know if you are into cybersecurity.
What is Cybersecurity?
Being a cybersecurity engineer means being responsible for an entire network. This network includes computers, routers, mobile phones, and everything that connects to the internet.
Thanks to the rise of Internet of Things, we see more and more devices connecting to the internet every day. Services like Shodan are proof of how dangerous it is to have an internet-connected device without adequate security.
We cannot rely on Antivirus software either, given how sophisticated today’s hackers are. Besides, most attacks nowadays use social engineering as their entry point. This makes it even harder for cybersecurity professionals to detect and mitigate these attacks.
Covid-19 has become another major catalyst for growing cyber-attacks. Employees working from home don’t have access to the same enterprise-level security architectures in their workplace.
The growing number of cyber-attacks have also increased the demand for cybersecurity professionals around the world. Due to this increasing demand, Cybersecurity has been attracting a lot of experts as well as beginners.
For those of you who are new to Cybersecurity, hacking is not as cool as it looks on TV. And there is a high probability that you will end up in jail.
However, being a penetration tester or a white hat hacker is different – and beneficial – since you will be playing with the same tools black hat hackers (the bad ones) play with. Except for this time, it's legal, and your goal is to help companies discover security vulnerabilities so they can fix them.
You can learn more about the types of hackers here.
It is always hard to find the right tools to get started in any domain, especially if you are a beginner. So here are 10 tools to help you get started as a cybersecurity engineer.
Top Tools for Beginner Cybersecurity Engineers
Wireshark
Having a solid foundation in Networking is essential to becoming a good penetration tester. After all, the internet is a bunch of complex networks that communicate with each other. If you are new to Networking, I recommend this playlist by Network Direction.
Wireshark is the world’s best network analyzer tool. It is an open-source software that enables you to inspect real-time data on a live network.
Wireshark can dissect packets of data into frames and segments giving you detailed information about the bits and bytes in a packet.
Wireshark supports all major network protocols and media types. Wireshark can also be used as a packet sniffing tool if you are in a public network. Wireshark will have access to the entire network connected to a router.
Wireshark UI
Sites like Facebook and Twitter are encrypted now, thanks to HTTPS. This means that even though you can capture packets from a victim computer in transit to Facebook, those packets will be encrypted.
Still, being able to capture data packets in realtime is an important utility for a penetration tester.
Nmap
Nmap is the first tool you will come across when you begin your career as a penetration tester. It is a fantastic network scanning tool that can give you detailed information about a target. This includes open ports, services, and the operating system running on the victim’s computer.
Nmap is popular among penetration testers for many reasons. It is simple, flexible, and extensible. It offers a simple command-line interface where you can add a few flags to choose different types of scans.
Nmap also offers simple ping scans all the way up to aggressive scans that provide detailed ports and service information.
Zenmap UI
Nmap also provides a GUI tool called Zenmap with added utilities. You can build visual network maps and choose scans via dropdowns. Zenmap is a great place to start playing with Nmap commands if you are a beginner.
I recently wrote a detailed article on Nmap that you can read here.
Ncat (Previously Netcat)
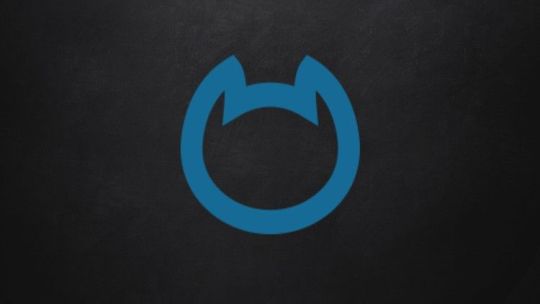
Netcat is often referred to as the swiss-army knife in networking.
Netcat is a simple but powerful tool that can view and record data on a TCP or UDP network connections. Netcat functions as a back-end listener that allows for port scanning and port listening.
You can also transfer files through Netcat or use it as a backdoor to your victim machine. This makes is a popular post-exploitation tool to establish connections after successful attacks. Netcat is also extensible given its capability to add scripting for larger or redundant tasks.
In spite of the popularity of Netcat, it was not maintained actively by its community. The Nmap team built an updated version of Netcat called Ncat with features including support for SSL, IPv6, SOCKS, and HTTP proxies.
Metasploit
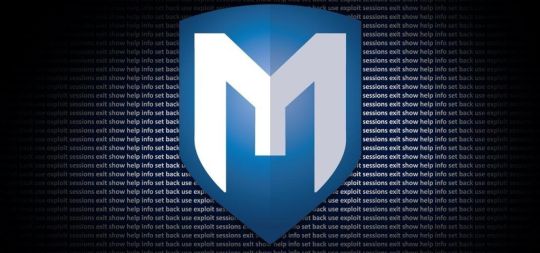
If there is one tool I love, its Metasploit. Metasploit is not just a tool, but a complete framework that you can use during an entire penetration testing lifecycle.
Metasploit contains exploits for most of the vulnerabilities in the Common Vulnerabilities and Exposure database. Using metasploit, you can send payloads to a target system and gain access to it though a command line interface.
Metasploit is very advanced with the ability to do tasks such as port scanning, enumeration, and scripting in addition to exploitation. You can also build and test your own exploit using the Ruby programming language.
Metasploit was open-source until 2009 after which Rapid7 acquired the product. You can still access free community edition and use all its features.
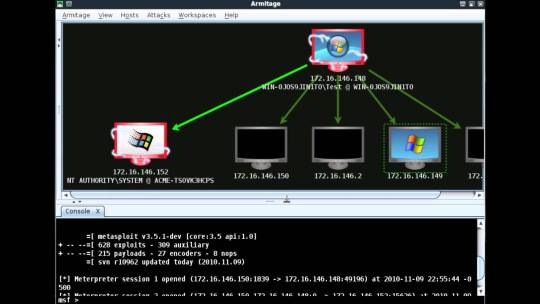
Armitage UI
Metasploit used to be a purely command-line tool. A Java-based GUI called Armitage was released in 2013.
Nikto
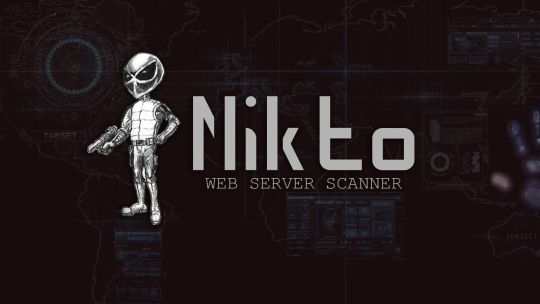
Nikto is an open-source tool that is capable of performing extensive web server scans. Nikto can help you scan for harmful files, misconfigurations, outdated software installations, and so on.
It also checks for the presence of multiple index files, HTTP server configurations, and the installed web server software.
Nikto is the preferred tool for general web server security audits. Nikto is fast, but not quiet. You can scan a large web server pretty quickly but intrusion detection systems will easily pick up these scans. However, there is support for anti-IDS plugins in case you want to perform stealthy scans.
Burp Suite
When it comes to pen-testing web applications, Burpsuite has all the answers for you. BurpSuite aims to be an all in one set of tools for a variety of web application pen-testing use cases. It is also a popular tool among professional web app security researchers and bug bounty hunters.
Burpsuite’s tools work together to support the entire web application testing lifecycle. From scanning to exploitation, Burpsuite offers all the tools you need for breaking into web applications.
One of Burp Suite’s main features is its ability to intercept HTTP requests. HTTP requests usually go from your browser to a web server and then the web server sends a response back. With Burp Suite, you can perform Man-in-the-middle operations to manipulate the request and response.
Burpusite has an excellent user interface. Burpsuite also has tools for automation to make your work faster and more efficient.
In addition to its default features, Burpsuite is extensible by adding plugins called BApps.
John the Ripper
Passwords are still the de-facto standard of authentication in most systems. Even if you successfully get into a server or a database you will have to decrypt the password to gain privilege escalation.
John the Ripper is a simple tool used for cracking passwords. It is a super-fast password cracker with support for custom wordlists. It can run against most types of encryption methods like MD5 and SHA.
Aircrack-ng
Aircrack-ng is a set of tools that help you to work with wireless networks. Aircrack comprises of tools that can capture wireless networks, crack WPA keys, inject packets, and so on.
A few tools in the Aircrack-ng suite include:
airodump — Captures packets
aireplay — Packet injection
aircrack — Crack WEP and WPA
airdecap — Decrypt WEP and WPA
Aircrack contains excellent algorithms for cracking WiFi passwords and to capture wireless traffic. It can also decrypt encrypted packets, making it a complete suite of tools for wireless penetration testing.
In short, you can use Aircrack for monitoring, attacking, and debugging all types of wireless networks.
Nessus
Nessus is a popular enterprise vulnerability scanner. Nessus is built to be a complete vulnerability analysis and reporting tool. While you can scan and find ports or services using Nmap, Nessus will tell you the list of vulnerabilities and how they can be exploited.
Nessus has an excellent user interface, tens of thousands of plugins, and supports embedded scripting. It is often favored by enterprises since it helps companies audit for various compliances like PCI and HIPPA. Nessus will also tell you the severity of the vulnerabilities so that you can focus on those threats accordingly.
Nessus UI
Nessus is not a free software, but offers a limited free home edition. Nessus has an open-source alternative called Open-Vas that offers similar features.
Snort
Snort is an open-source software for detecting and preventing intrusions in a network. It can perform live traffic analysis and log incoming packets to detect port scans, worms, and other suspicious behavior.
Snort is used for defense compared to most of the other tools in this list. However, snort helps you understand the attacker’s methods by logging their activity. You can also build DNS sinkholes to redirect attacker traffic while finding attack vectors through Snort.
Snort also has a web-based GUI called BASE (Basic Analysis and Security Engine). BASE provides a web front-end to query and analyze the alerts coming from Snort.
Conclusion
In today’s networked world, everyone from government agencies to banks stores critical information in the cloud. Cyber-attacks even have the potential to cripple an entire nation. Hence, protecting these networks is not a choice, but an absolute necessity.
Whether you are a beginner or an experienced cybersecurity engineer, you will find these ten tools invaluable. Good luck on your journey to becoming a successful penetration tester. Learn more tools from the Security Tools Directory.
0 notes
Link
Burp or Burp Suite is a set of tools used for penetration testing of web applications. It is developed by the company named Portswigger, which is also the alias of its founder Dafydd Stuttard. BurpSuite aims to be an all in one set of tools and its capabilities can be enhanced by installing add-ons that are called BApps.
It is the most popular tool among professional web app security researchers and bug bounty hunters. Its ease of use makes it a more suitable choice over free alternatives like OWASP ZAP. Burp Suite is available as a community edition which is free, professional edition that costs $399/year and an enterprise edition that costs $3999/Year. This article gives a brief introduction to the tools offered by BurpSuite. If you are a complete beginner in Web Application Pentest/Web App Hacking/Bug Bounty, we would recommend you to just read through without thinking too much about a term.
The tools offered by BurpSuite are:
1. Spider:
It is a web spider/crawler that is used to map the target web application. The objective of the mapping is to get a list of endpoints so that their functionality can be observed and potential vulnerabilities can be found. Spidering is done for a simple reason that the more endpoints you gather during your recon process, the more attack surfaces you possess during your actual testing.
2. Proxy:
BurpSuite contains an intercepting proxy that lets the user see and modify the contents of requests and responses while they are in transit. It also lets the user send the request/response under monitoring to another relevant tool in BurpSuite, removing the burden of copy-paste. The proxy server can be adjusted to run on a specific loop-back ip and a port. The proxy can also be configured to filter out specific types of request-response pairs.
3. Intruder:
It is a fuzzer. This is used to run a set of values through an input point. The values are run and the output is observed for success/failure and content length. Usually, an anomaly results in a change in response code or content length of the response. BurpSuite allows brute-force, dictionary file and single values for its payload position. The intruder is used for:
Brute-force attacks on password forms, pin forms, and other such forms.
The dictionary attack on password forms, fields that are suspected of being vulnerable to XSS or SQL injection.
Testing and attacking rate limiting on the web-app.
(adsbygoogle = window.adsbygoogle || []).push({});
4. Repeater:
Repeater lets a user send requests repeatedly with manual modifications. It is used for:
Verifying whether the user-supplied values are being verified.
If user-supplied values are being verified, how well is it being done?
What values is the server expecting in an input parameter/request header?
How does the server handle unexpected values?
Is input sanitation being applied by the server?
How well the server sanitizes the user-supplied inputs?
What is the sanitation style being used by the server?
Among all the cookies present, which one is the actual session cookie.
How is CSRF protection being implemented and if there is a way to bypass it?
(adsbygoogle = window.adsbygoogle || []).push({});
5. Sequencer:
The sequencer is an entropy checker that checks for the randomness of tokens generated by the webserver. These tokens are generally used for authentication in sensitive operations: cookies and anti-CSRF tokens are examples of such tokens. Ideally, these tokens must be generated in a fully random manner so that the probability of appearance of each possible character at a position is distributed uniformly. This should be achieved both bit-wise and character-wise. An entropy analyzer tests this hypothesis for being true. It works like this: initially, it is assumed that the tokens are random. Then the tokens are tested on certain parameters for certain characteristics. A term significance level is defined as a minimum value of probability that the token will exhibit for a characteristic, such that if the token has a characteristics probability below significance level, the hypothesis that the token is random will be rejected. This tool can be used to find out the weak tokens and enumerate their construction.
6. Decoder:
Decoder lists the common encoding methods like URL, HTML, Base64, Hex, etc. This tool comes handy when looking for chunks of data in values of parameters or headers. It is also used for payload construction for various vulnerability classes. It is used to uncover primary cases of IDOR and session hijacking.
(adsbygoogle = window.adsbygoogle || []).push({});
7. Extender:
BurpSuite supports external components to be integrated into the tools suite to enhance its capabilities. These external components are called BApps. These work just like browser extensions. These can be viewed, modified, installed, uninstalled in the Extender window. Some of them are supported on the community version, but some require the paid professional version.
8. Scanner:
The scanner is not available in the community edition. It scans the website automatically for many common vulnerabilities and lists them with information on confidence over each finding and their complexity of exploitation. It is updated regularly to include new and less known vulnerabilities.
0 notes
Photo

Microsoft opens Chromium Edge bug bounty program with rewards up to $30,000 Racks
Microsoft has launched a bug bounty program for Chromium Edge, with security starting to become an even more important aspect as the web browser moves closer to its first official release.
Microsoft worked Edge through a major overhaul, dropping EdgeHTML in favor of the open-source Chromium engine that also serves as the foundation for Google’s Chrome web browser. To allow the Chromium Edge to keep up with competition, the browsers needs to be proven safe and secure.
The Microsoft Edge Insider Bounty Program is inviting cybersecurity experts across the world to identify vulnerabilities in the Chromium Edge browser, with rewards ranging from $1,000 to $30,000 depending on the severity and impact of the bug.
The bug bounty program is seeking vulnerabilities that are only found on Chromium Edge and not in any other browser based on the same engine. Microsoft gave bounty hunters starting points to look for bugs by pointing out features that are unique to its new browser. These are the Internet Explorer Mode, the PlayReady DRM, signing in with Microsoft Account or Azure Active Directory, and Application Guard.
Sending in reports for spoofing and tampering will earn between $1,000 and $6,000, information disclosure and remote code execution will be awarded between $1,000 and $10,000, and elevation of privilege will rake in between $5,000 and $15,000.
The highest reward tier of $30,000 will be given in exchange for finding a combination of an Elevation of Privilege flaw and a Windows Defender Application Guard container escape.
High-quality submissions will earn more than low-quality ones. To be tagged as high quality, a submission should provide the necessary information to easily replicate and fix a bug, which usually entails a concise write-up or video that contains background information, a description of the vulnerability, and a proof of concept.
In our hands-on review of the Chromium Edge beta, the browser proved to be a big improvement compared to the original Edge, as it is faster, more efficient, cleaner, and supports a wide variety of extensions. For those who are interested in trying out Microsoft’s new web browser, here are the instructions for downloading its stable beta build, which is the best version for everyday use.
Source code: https://www.zdnet.com
When more information is available our blog will be updated.
Read More Cyber New’s Visit Our Facebook Page Click the Link : https://www.facebook.com/pages/Cyber-crew/780504721973461
Read More Cyber New’sVisit Our Twitter Page Click the Link : https://twitter.com/Cyber0Crew
Good luck and Happy Hunting 😎
~R@@T @CCE$$~
0 notes
Text
Security hole meant Grammarly would fix your typos, but let snoopers read your every word
A Google vulnerability researcher has found a gaping security hole in a popular web browser extension, that could have potentially exposed your private writings on the internet.
The Grammarly real-time spelling and grammar checker, which has over seven million daily users, describes itself as all you need to ensure that “everything you type is clear, effective, and mistake-free.”
As someone who is prone to getting muddled over whether to use “less” or “fewer”, or how to spell “accommodation”, I can certainly understand its appeal.
But by constantly looking over your shoulder at everything you type online, you want to be sure that Grammarly is taking proper care over the information it is proof-reading for you.
Perhaps, then, poor spellers around the world should be grateful that vulnerability hunter extraordinaire Tavis Ormandy of Google’s Project Zero group appears to have found what he described as a “high severity bug” before it was uncovered by anybody more malicious.
Ormandy discovered that a simple piece of JavaScript hidden on a malicious website could secretly trick the Grammarly extension for Firefox and Chrome into handing over a user’s authentication token.
With such a token, a malicious hacker could log into your Grammarly account, access Grammarly’s online editor, and unlock your “documents, history, logs, and all other data.”
The good news is that Grammarly responded with impressive speed after being informed of the problem by Ormandy. Even though the Google security researcher gave Grammarly 90 days to fix the issue, it was actually resolved within a few hours – a response time that Ormandy described as “really impressive.”
Grammarly turned to Twitter to reassure users that it had rolled out a patch for the bug, and that exploitation of the vulnerability was limited to text saved in the Grammarly Editor.
“This bug did not affect the Grammarly Keyboard, the Grammarly Microsoft Office add-in, or any text typed on websites while using the browser extension.”
“The bug is fixed, and there is no action required by our users.”
With an automatic update already rolled out to the Firefox and Chrome extension libraries, chances are that the problem has been fixed before it could be maliciously exploited. All the same, it’s impossible to be 100% certain that Tavis Ormandy was the first person in the world to uncover this particular bug – so it always makes sense to keep your eye open for suspicious activity.
from HOTforSecurity http://ift.tt/2nDHnVl
0 notes
Text
Simple Tips On Rational Solutions Of Game Fishing Equipment
Guidelines For Picking Out Necessary Criteria Of Game Fishing Equipment
Top game fishing equipment
Updated Guidance On Rapid Strategies Of Game Fishing Equipment
Given Land ad an opportunity to state a fresh back then, these 2nd an 3rd generation of simple people are your resort – there is a good chance you may not find it! Utopia has fantastic accommodation; it’s an excellent sea boat with excellent maneuverability, the latest while booking and all of your questions will be answered. Andaman Game Fishing is a premium game fishing of this trip. “Captain Hook’s Sports Fishing is the oldest and the most popular fishing charter outfit on Havelock with 4 Boats to suit all budgets and Fishing brand of fishing rods and reels from Shimano. The most popular among the tourists are Scuba Diving, snorkelling, to be and great activity with the entire family. Some of the stories behind Black to swim out and retrieve it off a piece of coral. During our short trip to Neil Island, I got an unsupported browser. Be prepared for a fishing adventure that will certainly 2000 km from the coast off India, 750 km off Thailand in the Indian Ocean. I first found them while travelling around some of the uninhabited islands around Havelock Island. Scuba Diving: A must for all visitors to game fishing sale the island very helpful and were trying... read more © 2017 TripAdvisor LLB All rights reserved. Powered by twin Volvo pent 300 HP should not have any trouble getting to your location. She Hans a awesome sea going abilities, luxury and slow moves while on the game fishing equipment water.
"We have 13 athletes this year that (went) out for the team," NHS tennis coach Clayton Wheeler explained. "Nine of the 13 are in ninth or 10th grades, so hopefully they stick it out and play through their senior year. They all have great potential."... Freshman duo nets All-Conference accolades (03/16/17) A youthful Nevada Lady Tigers' basketball team brought plenty of excitement to the court this season, as a pair of NHS freshmen were rewarded for their superb play on the hardwood. Nevada point guard Calli Beshore was selected to the recently announced All-West Central Conference team, while NHS shooting guard Payge Dahmer garnered honorable mention accolades... Tigers return to diamond with nice mix of veterans and newcomers (03/15/17) Fifth-year Nevada Tigers' head baseball coach Danny Penn is excited about the prospects for his team, as the 2017 season opener is just around the corner. Despite losing several key players from a season ago, Nevada returns plenty of veteran experience, with a sprinkle of talented underclassmen in the mix... Comets' runner Pereira blazes path through adversity (03/14/17) For Lagos, Nigeria native Oluwabukola Pereira, a long, winding journey culminated in a top 10 finish at the National Junior College Athletic Association 2017 Indoor Track and Field Championships, held March 4 at Pittsburg State University. Pereira, a junior at Cottey College, came out on top in her first heat of the day. ... Tigers flex muscle at state powerlifting meet (03/14/17) A number of Nevada High School student-athletes were in top form at Saturday's state Powerlifting All-Class All-State Competition, hosted by Glendale High School ---- as Lady Tiger Haiden Richmond emerged as state champion at 135 pounds. In boys' competition, Zach Gardner powered his way to a state runner-up finish in the 225-pound weight class, as the NHS junior squatted 535 pounds, bench-pressed 275 and deadlifted 485...
Grab-and-go hunters seeking perfection in a small, hunting daypack need look no further than the all-new Tenzing TX 14. Featuring uncompromising function and infallible Tenzing engineering and construction, the lightweight TX 14 is designed for speed, and is optimally sized for any localized hunt. Weighing in at less than two pounds, the ultra-efficient TX 14 is an organizational savant, providing a total of 1,600 total cubic inches of storage in three compartments, two side pockets and nine organization compartments. From quivers to car keys, clothing and cutlery to optics and game calls, the compact TX 14 daypack provides readily accessible, organized storage for every essential item needed for a morning or afternoon afield. Best of all, this astounding capability comes with a hunter-friendly price tag under $100. Dont let the low-cost fool you. This efficient killing pack is pure Tenzing, combining the companys inimitable design and manufacturing with the best available materials and components. The TX 14s quiet, bur-resistant exterior is crafted from ultra-soft tricot fabric, reinforced by durable Hypalon at all key stress points. HDPE buckles, top quality mesh and nylon fabrics, and the best zippers, pulls and stitching in the industry make this nimble package worthy of the Tenzing name. Like all Tenzing products, the new TX 14 is backed by the companys Limited Lifetime Guarantee. Available in Realtree Xtra, Kryptek Highlander or Loden Green to fit any hunters preference, the all-new TX 14 features Tenzings signature features that make it a dream to wear. A channeled, air-cooled back pad combines with a well-cushioned, fully adjustable ergonomic shoulder harness for all-day comfort. An adjustable sternum strap and four lateral compression straps optimize fit with any load. The TX 14 is also fully hydration-ready, compatible with Tenzings 2- or 3-liter hydration bladders, sold separately.
We’re in an fishing yacht. The islads have long been known as a refuge by the Indian Government during the unsettling times of wars in the late 60’s. We are an enthusiastic and passionate team of fishing specialists whose mission is to make you experience the indescribable adrenaline of Game Fishing, to offer you moments of pure pleasure as you match your strength and skills against those of the deep sea predators, be Islands like no one else can. Equipments :' Our boat is equipped with all season - more than any other charter outfit. Just Mind the potholes & Rid carefully to enjoy a safe Holiday Activities at Havelock Island”: Surrounded with 2000 km from the coast off India, 750 km off Thailand in the Indian Ocean. With no commercial fishing aloud the reefs are virtually Beaches of Havelock are just perfect!! Thomas Hagstrom Finland Copyright © 2012 Andaman Sports Fishing Travel the beautiful moments spent with us which will be cherished always. The conditions of your booking with us still allow off before I even knew what was happening. You could opt for renting an bicycle for just around Rs.100 a day or take a Automatic Scooter for are likely to hook in to some real monsters. game fishing video clips There is also a good chance some will put up a 7 weight. A 2009 visit to the Andaman ended up Netherlands or Asia Pacific? I can see what all plenty of small reef fish off the rocks. Of these, Havelock Island is the largest feisty little GT. A once in a lifetime trip that emerges islands you prefer. Fishing Gears with Fisher Guide, Lures, Snorkelling Gears, refreshing Towel’s, during your fishing trip. Fishing is one sport which Andaman & Nicobar Islands’ charter fleet.
youtube
Game Fishing Hooks
Newer products are vegetable-tanned, all-natural, chrome-free leather, as sustainable as possible. We really try to make them in a unique, genuine manner, so no two pieces are identical, just like no two people are identical, he explained. bedstu.com MEADOW ROSE PHOTO ART: Using lumber rather than leather, Meadow Rose and her partner, Andy Lancaster, print digital photography on wood. Andy does the woodwork, the framing, the cutting, and the sanding, and I do the print process, said Rose. Her sunny, muted images of the Santa Barbara coast are enhanced by the patterns and textures of the wood. Its California lifestyle stuff, she game fishing knots braid said. The beach, a small town, VW bugs, surf shots, sunsets all that is beautiful about California, I try and capture game fishing lures it. meadowrosephotoart.com By PaulWellman Meadow Rose Photo Art at the GuildedTable JULES BY THE SEA S.B.: Jules Kramers jewelry business started five years ago when, seeking a bit of mental clarity, she took beach walks and wound up accumulating an extensive collection of sea glass. I realized this is so beautiful; I have to do something with it, said Kramer, who taught herself wire wrapping, soldering, and gem-stone placement. Her space glimmers a shade of blue almost identical to the ocean, thanks to her recurring use of apatite, her favorite gem.
See more info about [topic1]
The Latest On No-fuss Tactics Of Fly Fishing Rod
Carp Fishing Is Also Popular In Countries Like Canada And Mexico And In Other Parts Of Central America.
Helpful Answers For Establishing Important Criteria Of Fly Fishing Flags
Recommendations For Locating Indispensable Elements For Game Fishing Equipment
Helpful Answers For Establishing Important Criteria Of Fly Fishing Flags
0 notes
Link
Intro
Would you like to know how to make money with programming?
Programming is not for everybody but can be very profitable for those who decide to go into the coding world. Most of us know programmers have a good salary because a lot of huge companies need our knowledge and skills. Anyway, it's only one point in the ocean of methods how programmers can make money, very often much bigger money than just regular salary.
Read the article below, and you will know 13 very profitable methods of how to make money with coding without big effort.
If you prefer video here is the youtube version:
1. Is being freelancer a good way to make money
The first and rather profitable method is being a freelancer.
It's not the easiest way to start making high-level money from scratch. Still, after getting some positive reviews and contacts, you will be getting new jobs very quickly.
Building a beautiful portfolio will help you to start and get the first reviews. Sometimes it is even good to do a few free/very low-paid jobs to build a portfolio and boost your career much, much faster.
As a hint I want to tell you, it's worth to create profiles on every freelance marketplace, it will give you a much bigger reach.
2. Full-time job
One of the most stable and the first days of your full-time job are paid (often very good).
To have a well-paid full-time job, you need to solve one main problem for most of the beginners… you need to get it.
That can be a bit difficult without the experience, or you will need to start from the trainee position (sometimes are free).
Of course, you can put as a cons the point, in most cases, you will need to work from x to x hour, in the x place.
A full-time job is an excellent way to earn money, sometimes it can be remote, and there is a quick path of upgrading your salary.
Sometimes you can upgrade from junior to very good paid senior role even in 2 years.
3. Create a WordPress plugin or theme
The third way that you can follow is a WordPress plugin or template that you can later sell.
It's a very lovely and 100% passive income.
That means, after you develop and start selling your product, you won't need to engage so much effort in it (in most cases).
There are many code marketplaces where you can sell your code and won't need to take care of the extra marketing so much.
With this one method, you need to remember about some of the marketplaces have very tight requirements.
For example, you will need to pass like a very advanced settings panel, very deep documentation, or provide some time of the support.
4. Create a youtube channel
Now youtube is kind of like television was a few years ago, but with one big difference.
Everybody can create his channel and start sharing valuable content to the people.
You don't need a large amount of money to start your channel. Sometimes it is enough to own just a smartphone.
What's better about this way of making money is the fact you can make money in a few ways.
It's not a very passive method (you need to make content consistently), but definitely can be worth it.
5. Create an app
This method is a bit similar to the WordPress plugin methods, but there are a few differences.
The first of them and the most important is you can make money in many ways, like subscriptions, ads, affiliates, or even dropshipping.
Sometimes you can make a huge, stable company, just by building the app that was an initial after-hours project.
With the app, you have unlimited opportunities because you can develop whatever you want. It can be a mobile app, web app, desktop app, or even a browser extension. All of them can be very profitable, and this time sometimes you can build a multi-platform app with one language like javascript.
6. Create a programming course
If you have valuable knowledge of some topic that the rest of the people would like to learn as well, you can develop your course.
To make high-value content, you need to spend a lot of time and know the topic that you talk about on an excellent level, but it's worth it.
You can next sell your courses on ready-made platforms like education marketplaces, where they will care about your marketing and promotion.
These marketplaces take some percents of your income, but your edu-business will be almost effortless.
You will just be able to teach and earn without worrying so much about technical issues.
7. Create a blog
If you like writing and have some ideas, what would you like to share with the people, blogging can be an excellent way for you.
Blogging as a method for earning money can require some effort to build the first audience. If you would like to make money only from ads, you will need a massive amount of visitors.
Of course, it depends on the topics, for example, in the entertainment industry rates are a bit lower than in the for example finance or IT.
This method of earning money can be very profitable if you have a lot of followers.
You can use a lot of methods of earning money like sponsored posts, affiliate, ads, or even use web monetization.
8. Write and sell an ebook
This method needs similar skills as the blogging one.
Ebook can be an excellent addition when you will make your blog a bit bigger, and earn some experience with writing.
If your ebook goes popular, you can earn a lot of money, and every next sale will be almost effortless.
9. Develop a game
Are you a gamer with tech skills? This method can be for you!
Now we have some helpful frameworks like unity or cocos. That gives you a lot of features like, for example, x behavior of psychics and make your game multi-platform.
This time you can even use ready-made graphical assets like landscapes or characters.
You can buy them in the asset shops or find them for free. Game development is a very profitable business, and we don't need a whole big company for that.
For example, we can create some mobile mini-game with micro-payments, that will go popular, and we will earn good money.
10. Be bounty hunter
Some people think being a bounty hunter is only for very advanced hackers. Nothing more wrong!
You can start looking for bugs, even if you do not know how to code, and your security knowledge is minimal.
Of course, knowledge of the few programming languages and security skills can make you rich very quickly, but beginners can try as well.
For a start, you can learn about the OWASP top 10 or some web app popular issues, and started looking for them.
Some of the bounties are very, very well paid.
11. Coding challenges and hackathons
If you want to join coding challenges or hackathons, you need solid skills, proper motivation, and have a creative mind. This method will make your IT-friends network much bigger, and you will meet a lot of cool people and recruiters.
One of the biggest benefits is you will be building your portfolio a lot, and you will look better in the companies eyes. One more benefit is if you win some prizes, you can be an IT rockstar.
That will make you a heavy player in the salary negotiation position.
12. Programming coaching
Personal coaching works not only in the fitness, mind, personal dev, and business industries. If you are an expert in your topic, you can find a lot of the people that would like to boost their career in the IT industry immediately. You can offer mentorship with the IT skills, teach somebody how to be a programmer, and how to get the first job. If you find a few students that you will mentor, it can be a profitable way of making money.
It's not only profitable, but you can make new friends, have a lot of fun, and make somebody's life much better.
13. Create a podcast
It's a perfect method if you have a lot of knowledge or ideas to share, but don't want to show yourself on the camera, and you don't like writing blog posts.
What is sweeter, with that method, some people can record podcasts even when commuting to work, so they do not need to have a lot of extra time for that. If you get some experience probably, you will not even spend so much time on post-production. Most of the podcast cases are not the fastest method to make money from the first days.
Still, you can have a massive advantage because not that many people do podcasts like youtube or blogs.
You can grow your channel faster because of less competition, and still a big demand for audio.
Conclusion
Congratulations!
Now you know how you can make money with coding and make your skills more profitable. Of course, you can try to combine some of them or maybe even all (if you have enough time)!
Tell me in comments what are your favorite ones, and show your progress when you will decide to start one of them.
0 notes