#data management and visualization
Explore tagged Tumblr posts
Text
Assignment 4: Creating Graphs for Your Data
PREVIOUS CONTENT
Assignment 1.
Assignment 2.
Assignment 3.
Link to download the dataset here.
Link to download the codebook here.
WHAT TO SUBMIT:
Once you have written a successful program that creates univariate and bivariate graphs, create a blog entry where you post your program and the graphs that you have created. Write a few sentences describing what your graphs reveal in terms of your individual variables and the relationship between them.
Download the graph program here.
In the last assignment (3), I had made the data management that I thought necessary. Now is time to create the graphics that represent this data.
I did that in two ways, in the first one I made the Quantitative->Quantitave method generating a scatterplot and the second one was a Qualitative->Quantitative method that creates a bar graph. Before I present the result of the relationship between the two variables in the graph, let’s see the histogram and the metrics extracted in each attribute separated.
Univariate graphs
Incidence of breast cancer
The first attribute was the incidence of breast cancer in 100,000 female residents during the 2002 year. As it is a quantitative attribute, was generated the histogram of the data. #Univariate histogram of the incidence of breast cancer in 100,000 female residents during the 2002 year.seaborn.distplot(sub1["breastCancer100th"].dropna(), kde=False);plt.xlabel('Incidence of breast cancer in 100,000 female residents during the 2002 year.')plt.ylabel('Number of counties.')plt.title('Histogram of the Incidence of Breast Cancer.')plt.show()
We can observe in the histogram that most of the countries have an incidence of cancer around 30 and 40 cases per 100,000 females. The extracted metrics of this attribute were: desc1 = sub1["breastCancer100th"].describe()print(desc1) count 129.000000mean 37.987597std 24.323873min 3.90000025% 20.60000050% 29.70000075% 50.300000max 101.100000Name: breastCancer100th, dtype: float64
With this, we can see that 75% of the countries have an incidence of breast cancer under 50.30 per 100,000 females.
Sugar consumption
The second attribute is the sugar consumption. For this attribute, I have made two graphs: one that shows the histogram of the original data and the other one that shows the bar graph of this attribute relocated into categories.
Histogram
#Univariate histogram of the Mean of the sugar consumption (grams per person and day) between 1961 and 2002.seaborn.distplot(sub1["meanSugarPerson"].dropna(), kde=False);plt.xlabel('Mean of the sugar consumption (grams per person and day) between 1961 and 2002.')plt.ylabel('Number of counties.')plt.title('Histogram of the Sugar Consumption.')plt.show()
This histogram is almost evenly distributed, we can see that the countries that have the most sugar consumption are in the 20 and the 110 grams per person. desc2 = sub1["meanSugarPerson"].describe()print(desc2) count 129.000000mean 76.238394std 42.488004min 6.13238125% 42.20642950% 79.71452475% 110.307619max 163.861429Name: meanSugarPerson, dtype: float64
The mean of sugar consumption is 76.24 and we can see that 75% of the countries have a consumption of sugar under 110.31 grams per day.
Bar graph
#Univariate bar graph of the Mean of the sugar consumption (grams per person and day) between 1961 and 2002.seaborn.countplot(x="sugar_consumption", data=sub1)plt.xlabel('Mean of the sugar consumption (grams per person and day) between 1961 and 2002.')plt.ylabel('Number of counties.')plt.title('Histogram of the Sugar Consumption.')plt.show()
Where the consumption is:
(0) Desirable between 0 and 30 g.
(1) Raised between 30 and 60 g.
(2) Borderline high between 60 and 90 g.
(3) High between 90 and 120 g.
(4) Very high under 120g.
The bar graph behaved very similarly to the histogram.
Bivariate graphs
The two bivariate graphics are presented below: #Bivariate Scatterplot Q->Q - Incidence of breast cancer versus sugar consumptionscat1 = seaborn.regplot(x="meanSugarPerson", y="breastCancer100th", fit_reg=True, data=sub1)plt.xlabel('Mean of the sugar consumption between 1961 and 2002.')plt.ylabel('Incidence of breast cancer in 100,000 female residents during the 2002 year.')plt.title('Scatterplot for the association between the incidence of breast cancer and the sugar consumption.')plt.show() #Bivariate bar graph C->Q - Incidence of breast cancer versus sugar consumptionseaborn.factorplot(x='sugar_consumption', y='breastCancer100th', data=sub1, kind="bar", ci=None)plt.xlabel('Mean of the sugar consumption between 1961 and 2002.')plt.ylabel('Incidence of breast cancer in 100,000 female residents during the 2002 year.')plt.title('Bar graph for the Association between the incidence of breast cancer and the sugar consumption.')plt.show()
In both graphics, we can see that there is a relation with the incidence of breast cancer and the consumption of sugar. While sugar consumption is increased the incidence of new breast cancer cases is increased too.
Review criteria
Your assessment will be based on the evidence you provide that you have completed all the steps. When relevant, gradients in the scoring will be available to reward clarity (for example, you will get one point for submitting graphs that do not accurately represent your data, but two points if the data is accurately represented). In all cases, consider that the peer assessing your work is likely not an expert in the field you are analyzing. You will be assessed equally in your description of your frequency distributions.
Specific rubric items, and their point values, are as follows:
Was a univariate graph created for each of the selected variables? (2 points)
Was a bivariate graph created for the selected variables? (2 points)
Did the summary describe what the graphs revealed in terms of the individual variables and the relationship between them? (2 points)
2 notes
·
View notes
Text
Week 4
Creating graphs for your data code
import pandas import numpy import pandas as pd import seaborn import matplotlib.pyplot as plt
data = pd.read_csv('gapminder_pds.csv', low_memory=False)
bindata = data.copy()
convert variables to numeric format using convert_objects function
data['internetuserate'] = pd.to_numeric(data['internetuserate'], errors='coerce') bindata['internetuserate'] = pd.cut(data.internetuserate, 10)
data['incomeperperson'] = pd.to_numeric(data['incomeperperson'], errors='coerce') bindata['incomeperperson'] = pd.cut(data.incomeperperson, 10)
data['employrate'] = pd.to_numeric(data['employrate'], errors='coerce') bindata['employrate'] = pd.cut(data.employrate, 10)
data['femaleemployrate'] = pd.to_numeric(data['femaleemployrate'], errors='coerce') bindata['femaleemployrate'] = pd.cut(data.femaleemployrate, 10)
data['polityscore'] = pd.to_numeric(data['polityscore'], errors='coerce') bindata['polityscore'] = data['polityscore'] sub2 = bindata.copy()
Scatterplot for the Association Between Employment rate and lifeexpectancy
scat1 = seaborn.regplot(x="internetuserate", y="incomeperperson", fit_reg=False, data=data) plt.xlabel('Internet use rate') plt.ylabel('Income per person') plt.title('Scatterplot for the Association Between Internet use rate and Income per person')
This scatterplot show the relationship and seems to be exponential.
Univariate histogram for quantitative variable:
seaborn.distplot(data["incomeperperson"].dropna(), kde=False); plt.xlabel('Income per person')
The graph is highly right skewed. Incomes are small for most of the world and the wealthy tail is quite long.
Univariate histogram for quantitative variable:
seaborn.distplot(data["employrate"].dropna(), kde=False); plt.xlabel('Employ rate')
Summary
It looks like there are associations between Internet use rate and income per person going up with internet use rate and going up an an accelerating rate.
0 notes
Note
HAPPY BIRTHDAY OMG!!! HAVE THE LOVELIEST DAY EVER ‼️‼️‼️ and yummy cake!! and lots of presents!!
Thank you bby love 🫶🫶🫶🫶 I've been making charts all day lol 😅😅😅 decently fun, I suppose ❤️
Big forehead kisses 4 u ❤️ (ɔˆ ³(ˆ⌣ˆc)
#stay babbling#babs answers#the charts are a visual representationnof my work schedule for anyone wondering#bc my managers are terrible at their jobs 🥰🫶#ive even included a way to automatically track hours#yknow#for overtime prevention#bc they suck at it#is this the most passive agressive thing ive ever done?#not even close#but its like#top 5 for sure#Today on “Babs is pissed”: theyre making a chart abt it 😱#its when i start pulling out the data that u kno you done fucked up
2 notes
·
View notes
Text
#Business Analytics#Colleges in India#Data Analytics#Top Colleges in India#Business Analytics Courses#Management#Colleges for Business Analytics#Big Data Analytics#Management Programs in India#Data Visualization
2 notes
·
View notes
Text
Make Smarter Moves, Not Just Faster Ones: The AI Decision Matrix You Didn’t Know You Needed
Make Smarter Moves, Not Just Faster Ones The AI Decision Matrix You Didn’t Know You Needed Ever felt like you were making business decisions with one eye closed, spinning the Wheel of Fortune, and hoping for the best? Yeah, me too. Let’s be honest: most entrepreneurs spend more time guessing than assessing. But here’s the plot twist, guesswork doesn’t scale. That’s where the AI-powered…
#AI decision matrix#AI predictive metrics#AI strategy for business growth#Business consulting#Business Growth#Business Strategy#data-driven business planning#Entrepreneur#Entrepreneurship#goal-based business dashboards#how to make smarter business decisions with AI#Leadership#Lori Brooks#Motivation#NLP-based decision making#Personal branding#Personal Development#predictive dashboard tools#Productivity#strategic clarity with AI#Technology Equality#Time Management#visual decision-making for entrepreneurs
1 note
·
View note
Text
Explore IGMPI’s Big Data Analytics program, designed for professionals seeking expertise in data-driven decision-making. Learn advanced analytics techniques, data mining, machine learning, and business intelligence tools to excel in the fast-evolving world of big data.
#Big Data Analytics#Data Science#Machine Learning#Predictive Analytics#Business Intelligence#Data Visualization#Data Mining#AI in Analytics#Big Data Tools#Data Engineering#IGMPI#Online Analytics Course#Data Management#Hadoop#Python for Data Science
0 notes
Text
Retailers face inventory mismanagement, demand fluctuations, and poor customer insights, impacting sales and profitability. Infoveave Pvt. Ltd. delivers retail analytics solutions, utilizing AI to track consumer behavior, forecast demand, and optimize pricing. With real-time data, retailers enhance supply chain efficiency, boost customer engagement, and maximize revenue through data-driven strategies.
#tools for data visualization#data visualization tools#unified data analytics platform#data visualization softwares#unified data management platform#data analytics tools#robotic process automation software
0 notes
Text
Human Factors: Decision making in the real world

It’s not enough for one person to know what happened yesterday—teams need to spot long-term trends to predict anomalies. Data is streaming, so static analysis doesn’t cut it. Unparsed data dumps don’t help. Being able to visualize data through dashboards or graphs helps to make sense of patterns. It is not about becoming data scientists. You do not need a degree in mechanics to drive a car. Your driving instructor tells you in simple terms how an engine works, where the oil goes, how to turn the steering wheel and which pedal to press.
Data should not be siloed. Maintenance, logistics, management, production teams — everyone needs to know some basics to make cohesive decisions. Cross-functional training is a key element to a deployment. There is a natural reluctance to embrace new things. Knowledge empowers and concurrently dispels fear of change. In hierarchical organizations there can be an aversion to the wider distribution of real-time information outside the management cadre. Inertia comes bottom up or top down, usually it is both at the same time.
#Change Management#Charts#Dashboards#Data#Data Modelling#Education#Ergonomics#Information Flow#Learning#Statistics#Tools#Training#visualization#Visuals
0 notes
Text
SQL & Power BI Certification | MITSDE
Enhance your career with MITSDE’s Online Power BI Certification Course! This comprehensive program equips you with essential skills in data visualization, analytics, and business intelligence, helping you make data-driven decisions. Learn to create interactive dashboards, generate insightful reports, and analyze business trends effectively. Designed for professionals and beginners alike, this course offers hands-on training and expert guidance to boost your expertise. Stay ahead in the competitive job market—enroll today and transform your data analysis skills with Power BI!
#SQL & Power BI Certification Program#Power BI Certification#powerbi course#MITSDE#Data management#Data visualization#Data Specialist#Data manipulation#Data analytics#Business intelligence#Power BI Course
0 notes
Text
Exploring the Drupal Views module
Sure you’ve worked with the Views module in Drupal but have you made the most of it? Get the full breakdown of its features and learn how to create dynamic displays in this article.

0 notes
Text
Assignment 3: Making Data Management Decisions
WHAT TO SUBMIT:
Once you have written a successful program that manages your data, create a blog entry where you post your program and the results/output that displays at least 3 of your data managed variables as frequency distributions. Write a few sentences describing these frequency distributions in terms of the values the variables take, how often they take them, the presence of missing data, etc.
Download the program here and the dataset here;
In the last assignment, I had already made the data management that I thought necessary, but I made it in the excel with formulas.
Now, I remade the data management directly in python, and the program output can be seen down in the post.
The results were still the same. The sample used was the incidence of new breast cancer cases in 129 different countries. After running the program, it was possible to observe that the consumption of sugar is considered desirable only in 20.9% of the countries of the dataset. Taking into account that this metric is based on the average of the desirable sugar ingest in grams per day of the woman (25g) and the man (36g) [1] and [2].
To the food consumption data, I made the average of all countries consumption and compared each country consumption to this mean. 55% of the countries stay under the average.
At last, to range the total cholesterol in the blood of the countries I used as a base the metric of Mayo Clinic [3]. In the dataset, none of the values exceeded to a high level of total cholesterol and almost 73% of the countries presented to be in the desirable level.
Reference
[1] Life by Daily Burn Are You Exceeding Your Daily Sugar Intake in Just One Meal INFOGRAPHIC. Visited 05 Jul 2016. URL: http://dailyburn.com/life/health/daily-sugar-intake-infographic/.
[2] MD-Health How Many Grams of Sugar Per Day. Visited 06/07/2016. URL: http://www.md-health.com/How-Many-Grams-Of-Sugar-Per-Day.html.
[3] Cholesterol Test - Procedure details. Visited 05 Jul 2016. URL: http://www.mayoclinic.org/tests-procedures/cholesterol-test/details/results/rsc-20169555.
Output of the program: Importing the packages and the data set (csv)
import pandasimport numpyimport statistics # Import the data set to memorydata = pandas.read_csv("separatedData.csv", low_memory = False) # Change data type among variables to numericdata["breastCancer100th"] = data["breastCancer100th"].convert_objects(convert_numeric=True)data["meanSugarPerson"] = data["meanSugarPerson"].convert_objects(convert_numeric=True)data["meanFoodPerson"] = data["meanFoodPerson"].convert_objects(convert_numeric=True)data["meanCholesterol"] = data["meanCholesterol"].convert_objects(convert_numeric=True) # Create a subData with only the variables breastCancer100th, meanSugarPerson, meanFoodPerson, meanCholesterolsub1=data[['breastCancer100th','meanSugarPerson', 'meanFoodPerson', 'meanCholesterol']]
Making the new variable sugar_consumption
# Create the conditions to a new variable named sugar_consumption that will categorize the meanSugarPerson answersdef sugar_consumption (row): if 0 < row['meanSugarPerson'] <= 30 : return 0 # Desirable between 0 and 30 g. if 30 < row['meanSugarPerson'] <= 60 : return 1 # Raised between 30 and 60 g. if 60 < row['meanSugarPerson'] <= 90 : return 2 # Borderline high between 60 and 90 g. if 90 < row['meanSugarPerson'] <= 120 : return 3 # High between 90 and 120 g. if row['meanSugarPerson'] > 120 : return 4 # Very high under 120g. # Add the new variable sugar_consumption to subDatasub1['sugar_consumption'] = sub1.apply (lambda row: sugar_consumption (row),axis=1) # Count of sugar_consumptionprint("Count of sugar_consumption - Range of sugar consumption based on the mean of the quantity (grams per person and day) of sugar and sweeters between 1961 and 2002")c1 = sub1["sugar_consumption"].value_counts(sort=False)print(c1) # Percentage of sugar_consumptionprint("Percentage of sugar_consumption - Range of sugar consumption based on the mean of the quantity (grams per person and day) of sugar and sweeters between 1961 and 2002")p1 = sub1["sugar_consumption"].value_counts(sort=False,normalize=True)print(p1)
1.1.1.2 Count and Percentage of the new variable sugar_consumption
Count of sugar_consumption - Range of sugar consumption based on the mean of the quantity (grams per person and day) of sugar and sweeters between 1961 and 20020 271 192 313 314 21Name: sugar_consumption, dtype: int64 Percentage of sugar_consumption - Range of sugar consumption based on the mean of the quantity (grams per person and day) of sugar and sweeters between 1961 and 20020 0.2093021 0.1472872 0.2403103 0.2403104 0.162791Name: sugar_consumption, dtype: float64
Making the new variable food_consumption
#Make the average of meanFoodPerson values.food_mean = statistics.mean(data["meanFoodPerson"]) # Create the conditions to a new variable named food_consumption that will categorize the meanFoodPerson answersdef food_consumption (row): if row['meanFoodPerson'] <= food_mean : return 0 # Food consumption below the world average. if row['meanFoodPerson'] > food_mean : return 1 # Food consumption under the world average. # Add the new variable food_consumption to subDatasub1['food_consumption'] = sub1.apply (lambda row: food_consumption (row),axis=1) # Count of food_consumptionprint("Count of food_consumption - Mean of the food consumption of countries based on the mean of the total supply of food (kilocalories / person & day) between 1961 and 2002")c2 = sub1["food_consumption"].value_counts(sort=False)print(c2) # Percentage of food_consumptionprint("Percentage of food_consumption - Mean of the food consumption of countries based on the mean of the total supply of food (kilocalories / person & day) between 1961 and 2002")p2 = sub1["food_consumption"].value_counts(sort=False, normalize=True)print(p2)
Count and Percentage of the new variable food_consumption
Count of food_consumption - Mean of the food consumption of countries based on the mean of the total supply of food (kilocalories / person & day) between 1961 and 20020 711 58Name: food_consumption, dtype: int64 Percentage of food_consumption - Mean of the food consumption of countries based on the mean of the total supply of food (kilocalories / person & day) between 1961 and 20020 0.5503881 0.449612Name: food_consumption, dtype: float64
Making the new variable cholesterol_blood
# Create the conditions to a new variable named cholesterol_blood that will categorize the meanCholesterol answersdef cholesterol_blood (row): if row['meanCholesterol'] <= 5.2 : return 0 # Desirable below 5.2 mmol/L if 5.2 < row['meanCholesterol'] <= 6.2 : return 1 # Borerline high between 5.2 and 6.2 mmol/L if row['meanCholesterol'] > 6.2 : return 2 # High above 6.2 mmol/L # Add the new variable cholesterol_blood to subDatasub1['cholesterol_blood'] = sub1.apply (lambda row: cholesterol_blood (row),axis=1) # Count of cholesterol_bloodprint("Count of cholesterol_blood - Range of the average of the mean TC (Total Cholesterol) of the female population counted in mmol per L between 1980 and 2002")c3 = sub1["cholesterol_blood"].value_counts(sort=False)print(c3) # Percentage of cholesterol_bloodprint("Percentage of cholesterol_blood - Range of the average of the mean TC (Total Cholesterol) of the female population counted in mmol per L between 1980 and 2002")p3 = sub1["cholesterol_blood"].value_counts(sort=False, normalize=True)print(p3)
Count and Percentage of the new variable cholesterol_blood
Count of cholesterol_blood - Range of the average of the mean TC (Total Cholesterol) of the female population counted in mmol per L between 1980 and 20020 941 35Name: cholesterol_blood, dtype: int64 Percentage of cholesterol_blood - Range of the average of the mean TC (Total Cholesterol) of the female population counted in mmol per L between 1980 and 20020 0.7286821 0.271318Name: cholesterol_blood, dtype: float64
Review criteria
Your assessment will be based on the evidence you provide that you have completed all the steps. When relevant, gradients in the scoring will be available to reward clarity (for example, you will get one point for submitting output that is not understandable, but two points if it is understandable). In all cases, consider that the peer assessing your work is likely not an expert in the field you are analyzing. You will be assessed equally in your description of your frequency distributions.
Specific rubric items, and their point values, are as follows:
Was the program output interpretable (i.e., organized and labelled)? (1 point)
Does the program output display three data managed variables as frequency tables? (1 point)
Did the summary describe the frequency distributions in terms of the values the variables take, how often they take them, the presence of missing data, etc.? (2 points)
0 notes
Text

VADY – Transforming Raw Data into Strategic Masterpieces
VADY excels at transforming raw, unprocessed data into valuable business assets. Using AI, we refine complex data sets and convert them into actionable insights that drive strategic decision-making. Whether it's improving operational efficiency, enhancing customer experiences, or identifying new market opportunities, VADY ensures that data is a driving force behind your business’s growth. We offer businesses the tools to unlock their data’s potential, turning it into a strategic masterpiece. With VADY, companies gain a clearer understanding of their landscape, enabling them to make informed decisions that enhance performance, innovation, and profitability for the long run.
#vady#newfangled#big data#data analytics#data democratization#ai to generate dashboard#nlp#etl#machine learning#data at fingertip#ai enabled dashboard#generativebi#generativeai#artificial intelligence#data visualization#data analysis#data management#data privacy#data#datadrivendecisions
0 notes
Text
How to use COPILOT in Microsoft Word | Tutorial
This page contains a video tutorial by Reza Dorrani on how to use Microsoft 365 Copilot in Microsoft Word. The video covers: Starting a draft with Copilot in Word. Adding content to an existing document using Copilot. Rewriting text with Copilot. Generating summaries with Copilot. Overall, using Copilot as a dynamic writing companion to enhance productivity in Word. Is there something…
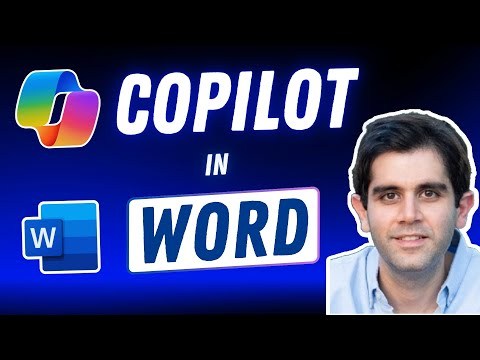
View On WordPress
#Advanced Excel#Automation tools#Collaboration#copilot#Data analysis#Data management#Data visualization#Excel#Excel formulas#Excel functions#Excel skills#Excel tips#Excel tutorials#MIcrosoft Copilot#Microsoft Excel#Microsoft Office#Microsoft Word#Office 365#Power BI#productivity#Task automation
1 note
·
View note
Text
Integrating Data Science Management with Business Strategy: Aligning Goals and Objectives

#Data Science Management#PG Diploma in Data Science#PGDM in Data Science#Data Visualization#Artificial Intelligenc#Data-Driven Culture#Business Strategy in Data Science#Professional Development
1 note
·
View note
Text
How to Optimize Data Management for a Seamless Digital Transformation and ERP Implementation?
Digital transformation and ERP (Enterprise Resource Planning) strategies have become pivotal for businesses aiming to stay competitive in today’s fast-evolving landscape. At the core of these strategies lies data management — a critical aspect that ensures accurate, reliable, and actionable insights for decision-making.
Watch this comprehensive video on starting data management in your ERP strategy for an in-depth understanding.
youtube
If you’re wondering how to start data management in your digital transformation and ERP strategy, this guide provides actionable steps to help you begin your journey effectively.
Why Data Management is Crucial in Digital Transformation
Before diving into the “how,” it’s essential to understand the “why.” Here’s why data management is indispensable:
Data as the Backbone of ERP Systems: ERP systems thrive on clean, structured, and well-organized data. Without proper data management, the efficiency of ERP systems diminishes.
Informed Decision-Making: Reliable data leads to better analytics, which fuels strategic decisions.
Cost Optimization: Effective data management reduces redundancies, eliminates errors, and cuts costs in operations.
5 Steps to Start Data Management in Your Digital Transformation Journey
1. Assess Your Current Data Landscape
Before implementing any strategy, audit your current data repositories. Identify duplicate, incomplete, or irrelevant data that might be affecting your systems.
2. Define Your Data Governance Framework
A strong governance framework ensures consistency and accountability. This includes setting rules for data collection, usage, storage, and security.
3. Choose the Right Tools and Technologies
Invest in master data management (MDM) tools that integrate seamlessly with your ERP system. These tools enable:
Centralized data storage
Automated data cleansing
Real-time data updates
4. Involve Key Stakeholders
Data management isn’t an IT-only responsibility. Include leaders from finance, operations, and supply chain departments to ensure holistic alignment.
5. Provide Continuous Training
Educate your employees about the importance of clean data and how their inputs impact larger systems like ERP. A data-driven culture begins with informed employees.
How ERP Systems and Data Management Work Hand-in-Hand
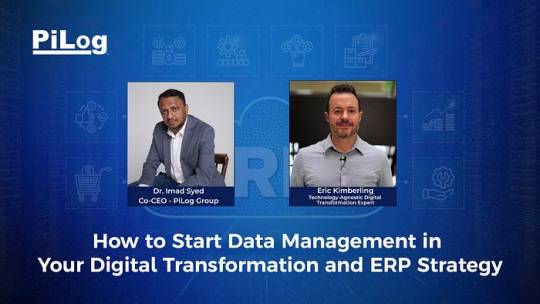
High-Impact Benefits of Starting Data Management Now
Enhanced Business Agility: With structured data, businesses can respond quickly to changes.
Improved Compliance: Regulatory requirements like GDPR and CCPA demand clean and transparent data practices.
Better Customer Experiences: When your systems are fueled by high-quality data, you deliver superior services.
Conclusion
Starting your data management journey may seem daunting, but with the right approach and resources, it can transform your digital transformation and ERP strategy into a powerful business enabler.
To gain deeper insights and actionable advice on this topic, make sure to watch this detailed video here.
Take the first step today — because the future of your business depends on how well you manage your data today!
#digital transformation#data management in erp#erp services#piloggroup#data analytics#data governance#erp solutions provider#data visualization#data visualisation#youtube#data scientist#data management solutions#datadriven#Youtube
0 notes
Photo
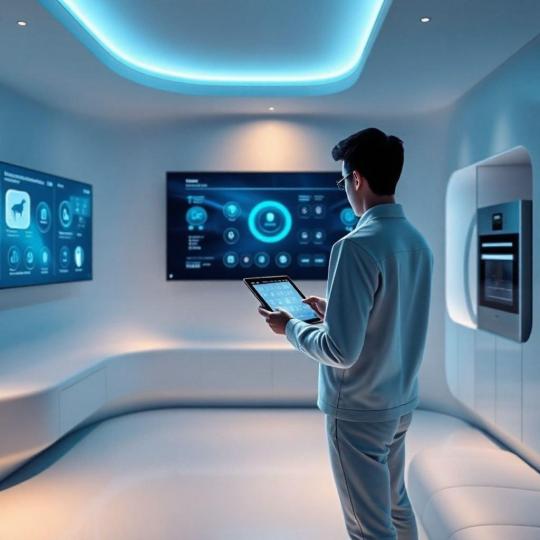
Dive into the Future with Our Modern Technology Control Room Wallpaper! 🚀
Hey there, tech enthusiasts! Are you ready to step into a world where technology reigns supreme? Our latest creation, the Modern Technology Control Room wallpaper, is here to take you on a futuristic journey!
Imagine a sleek control room filled with vibrant digital interfaces, where a savvy individual expertly navigates through a sea of data with just a swipe on their tablet. This wallpaper captures that exhilarating moment, blending innovation and style in one stunning visual.
Whether you're a fan of cutting-edge gadgets or simply love the aesthetic of modern tech environments, this wallpaper is perfect for your desktop or mobile device. It brings a touch of the future right to your screen, inspiring creativity and productivity every time you glance at it!
Ready to transform your digital space? Check out the wallpaper here: Explore the Control Room and give your devices a fresh new look!
Let us know what you think! We love hearing your feedback and seeing how you use our wallpapers. Happy downloading! 😄
#technology#control room#futuristic#digital interfaces#tablet#modern design#innovation#tech aesthetics#desktop wallpaper#mobile wallpaper#digital art#futurism#data management#gadgets#high-tech#visual inspiration#creative workspace#tech lovers#sci-fi#wallpaper design
1 note
·
View note