#laravel apis
Explore tagged Tumblr posts
Text
Protect Your Laravel APIs: Common Vulnerabilities and Fixes
API Vulnerabilities in Laravel: What You Need to Know
As web applications evolve, securing APIs becomes a critical aspect of overall cybersecurity. Laravel, being one of the most popular PHP frameworks, provides many features to help developers create robust APIs. However, like any software, APIs in Laravel are susceptible to certain vulnerabilities that can leave your system open to attack.
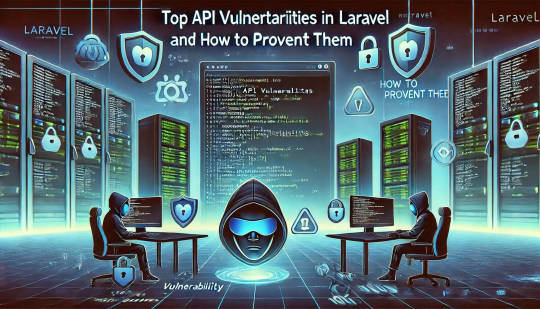
In this blog post, we’ll explore common API vulnerabilities in Laravel and how you can address them, using practical coding examples. Additionally, we’ll introduce our free Website Security Scanner tool, which can help you assess and protect your web applications.
Common API Vulnerabilities in Laravel
Laravel APIs, like any other API, can suffer from common security vulnerabilities if not properly secured. Some of these vulnerabilities include:
>> SQL Injection SQL injection attacks occur when an attacker is able to manipulate an SQL query to execute arbitrary code. If a Laravel API fails to properly sanitize user inputs, this type of vulnerability can be exploited.
Example Vulnerability:
$user = DB::select("SELECT * FROM users WHERE username = '" . $request->input('username') . "'");
Solution: Laravel’s query builder automatically escapes parameters, preventing SQL injection. Use the query builder or Eloquent ORM like this:
$user = DB::table('users')->where('username', $request->input('username'))->first();
>> Cross-Site Scripting (XSS) XSS attacks happen when an attacker injects malicious scripts into web pages, which can then be executed in the browser of a user who views the page.
Example Vulnerability:
return response()->json(['message' => $request->input('message')]);
Solution: Always sanitize user input and escape any dynamic content. Laravel provides built-in XSS protection by escaping data before rendering it in views:
return response()->json(['message' => e($request->input('message'))]);
>> Improper Authentication and Authorization Without proper authentication, unauthorized users may gain access to sensitive data. Similarly, improper authorization can allow unauthorized users to perform actions they shouldn't be able to.
Example Vulnerability:
Route::post('update-profile', 'UserController@updateProfile');
Solution: Always use Laravel’s built-in authentication middleware to protect sensitive routes:
Route::middleware('auth:api')->post('update-profile', 'UserController@updateProfile');
>> Insecure API Endpoints Exposing too many endpoints or sensitive data can create a security risk. It’s important to limit access to API routes and use proper HTTP methods for each action.
Example Vulnerability:
Route::get('user-details', 'UserController@getUserDetails');
Solution: Restrict sensitive routes to authenticated users and use proper HTTP methods like GET, POST, PUT, and DELETE:
Route::middleware('auth:api')->get('user-details', 'UserController@getUserDetails');
How to Use Our Free Website Security Checker Tool
If you're unsure about the security posture of your Laravel API or any other web application, we offer a free Website Security Checker tool. This tool allows you to perform an automatic security scan on your website to detect vulnerabilities, including API security flaws.
Step 1: Visit our free Website Security Checker at https://free.pentesttesting.com. Step 2: Enter your website URL and click "Start Test". Step 3: Review the comprehensive vulnerability assessment report to identify areas that need attention.
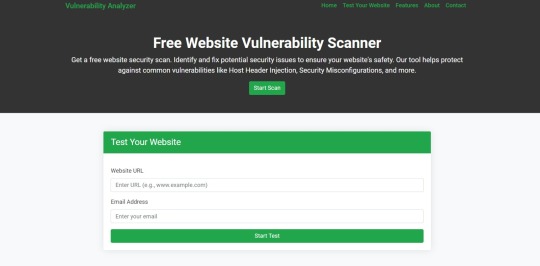
Screenshot of the free tools webpage where you can access security assessment tools.
Example Report: Vulnerability Assessment
Once the scan is completed, you'll receive a detailed report that highlights any vulnerabilities, such as SQL injection risks, XSS vulnerabilities, and issues with authentication. This will help you take immediate action to secure your API endpoints.
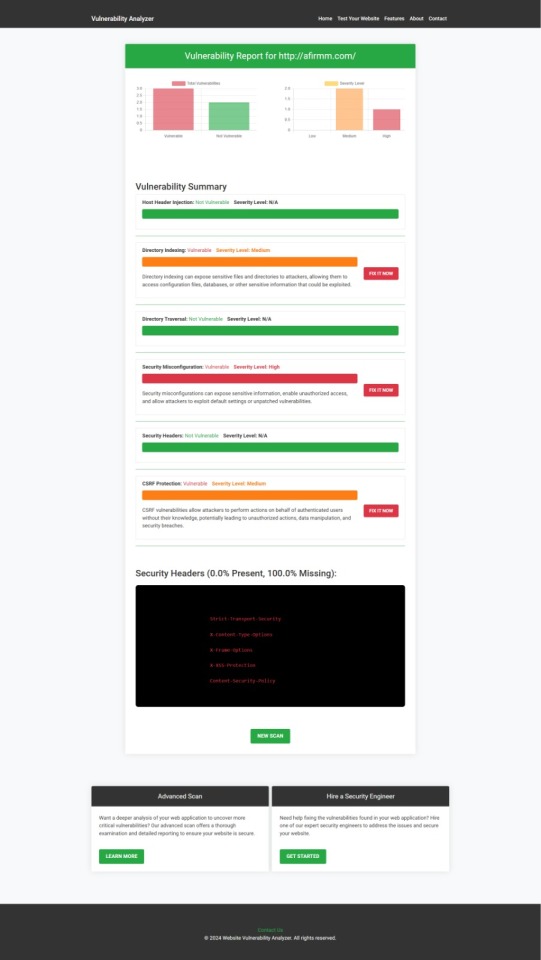
An example of a vulnerability assessment report generated with our free tool provides insights into possible vulnerabilities.
Conclusion: Strengthen Your API Security Today
API vulnerabilities in Laravel are common, but with the right precautions and coding practices, you can protect your web application. Make sure to always sanitize user input, implement strong authentication mechanisms, and use proper route protection. Additionally, take advantage of our tool to check Website vulnerability to ensure your Laravel APIs remain secure.
For more information on securing your Laravel applications try our Website Security Checker.
#cyber security#cybersecurity#data security#pentesting#security#the security breach show#laravel#php#api
2 notes
·
View notes
Text
Need to update the title.
Id eius voluptatum v #laravel#tumblr#api
0 notes
Text
APIs (Application Programming Interfaces) allow applications to communicate with each other. Laravel provides multiple ways to build APIs, and Sanctum is the most efficient method for token-based authentication. In this tutorial, we’ll build a secure REST API in Laravel 12 using Sanctum with authentication, CRUD operations, and middleware protection.
#Laravel12#RESTAPI#Sanctum#Laravel#APIIntegration#LaravelSanctum#WebDevelopment#APIAuthentication#LaravelAPI#BackendDevelopment#LaravelApp#LaravelTutorial#PHP#LaravelSecurity#LaravelDevelopment#TokenAuthentication#API#RESTfulAPI#LaravelProjects#Authentication#APIRequests#SanctumAuthentication#WebAppDevelopment#LaravelBestPractices#LaravelAuth#APIFeatures#FullStackDevelopment
0 notes
Text
Why Headless Laravel CMS is Taking Over Traditional Web Development
Hey folks! 🚀 If you’ve been keeping up with web development trends, you’ve probably heard the buzz about headless Laravel CMS. It’s revolutionizing how we build and manage websites, leaving traditional CMS platforms like WordPress and Drupal in the dust. But why? Let’s dive in and explore why businesses and developers are making the switch—spoiler alert: it’s all about flexibility, speed, and scalability!
Understanding Headless Laravel CMS and Its Growing Popularity
A headless CMS isn’t some futuristic tech—it’s a smarter way to manage content. Unlike traditional CMS platforms that bundle the frontend and backend together, a headless CMS decouples them, giving developers the freedom to use any frontend framework while Laravel handles the backend like a pro.
What is a Headless CMS and How Does It Work?
Imagine a restaurant where the kitchen (backend) and dining area (frontend) operate independently. 🍽️ The kitchen prepares the food (content), and the waitstaff (APIs) deliver it to any dining setup—be it a food truck, rooftop café, or home delivery. That’s how a headless CMS works! It stores and manages content, then delivers it via APIs to any device or platform—websites, mobile apps, smartwatches, you name it.
Why Laravel is Perfect for a Headless CMS
Laravel isn’t just another PHP framework—it’s a powerhouse for API-driven development. With built-in support for RESTful and GraphQL APIs, Eloquent ORM for smooth database interactions, and a robust ecosystem, it’s no wonder Laravel is the top pick for headless CMS setups.
Headless Laravel CMS vs. Traditional CMS Solutions
Traditional CMS platforms like WordPress are great for simple websites, but they struggle with scalability and multi-channel content delivery. A headless Laravel CMS, on the other hand, offers:
No frontend restrictions (use React, Vue.js, or even a mobile app).
Better performance (no bloated themes or plugins slowing things down).
Future-proof flexibility (adapt to new tech without overhauling your backend).
Benefits of Using a Headless CMS with Laravel
Enhanced Performance and Scalability
Did you know? Websites using headless CMS architectures load up to 50% faster than traditional setups. 🏎️ By separating the frontend and backend, Laravel ensures your content is delivered lightning-fast, whether you’re serving 100 or 100,000 users.
Multi-Platform Content Delivery
With a headless Laravel CMS, your content isn’t tied to a single website. Publish once, and distribute everywhere—web, mobile apps, IoT devices, even digital billboards! Companies like Netflix and Spotify use headless CMS to deliver seamless experiences across platforms.
Improved Security and Backend Control
Traditional CMS platforms are hacker magnets (looking at you, WordPress plugins!). A headless Laravel CMS reduces vulnerabilities by:
Limiting exposure (no public-facing admin panel).
Using Laravel’s built-in security (CSRF protection, encryption).
Offering granular API access control.
Key Technologies Powering Headless Laravel CMS
RESTful and GraphQL APIs in Laravel CMS
Laravel makes API development a breeze. Whether you prefer REST (simple and structured) or GraphQL (flexible and efficient), Laravel’s got you covered. Fun fact: GraphQL can reduce API payloads by up to 70%, making your apps faster and more efficient.
Integrating Laravel CMS with JavaScript Frontend Frameworks
Pairing Laravel with React, Vue.js, or Next.js is like peanut butter and jelly—perfect together! 🥪 Frontend frameworks handle the UI, while Laravel manages data securely in the background. Many Laravel web development companies leverage this combo for high-performance apps.
Database and Storage Options for Headless Laravel CMS
Laravel plays nice with MySQL, PostgreSQL, MongoDB, and even cloud storage like AWS S3. Need to scale? No problem. Laravel’s database abstraction ensures smooth performance, whether you’re running a blog or a global e-commerce site.
Use Cases and Real-World Applications of Headless Laravel CMS
E-Commerce and Headless Laravel CMS
E-commerce giants love headless CMS for its agility. Imagine updating product listings once and seeing changes reflected instantly on your website, mobile app, and marketplace integrations. Companies like Nike and Adidas use headless setups for seamless shopping experiences.
Content-Heavy Websites and Laravel Headless CMS
News portals and media sites thrive with headless Laravel CMS. Why? Because journalists can publish content via a streamlined backend, while developers use modern frameworks to create dynamic, fast-loading frontends.
API-Driven Web and Mobile Applications
From fitness apps to banking platforms, headless Laravel CMS ensures real-time data sync across devices. No more clunky updates—just smooth, consistent user experiences.
Challenges and Best Practices for Headless Laravel CMS
Managing API Requests Efficiently
Too many API calls can slow things down. Solution? Caching and webhooks. Laravel’s caching mechanisms (Redis, Memcached) and event-driven webhooks keep performance snappy.
Handling SEO in a Headless Laravel CMS Setup
SEO isn’t dead—it’s just different! Use server-side rendering (SSR) with Next.js or Nuxt.js, and leverage Laravel’s meta-tag management tools to keep search engines happy.
Ensuring Smooth Frontend and Backend Communication
Clear API documentation and webhook integrations are key. A well-structured Laravel backend paired with a modular frontend ensures seamless updates and maintenance.
Final Thoughts
Headless Laravel CMS isn’t just a trend—it’s the future. With better performance, unmatched flexibility, and ironclad security, it’s no surprise that Laravel development companies are leading the charge. Whether you’re building an e-commerce platform, a content hub, or a multi-platform app, going headless with Laravel is a game-changer.
Key Takeaways
Headless Laravel CMS = Speed + Flexibility 🚀
API-first architecture = Content everywhere 📱💻
Security and scalability built-in 🔒
Frequently Asked Questions (FAQs)
1. What is the difference between a traditional CMS and a headless CMS?
A traditional CMS (like WordPress) combines the backend (content management) and frontend (display) in one system. A headless CMS decouples them, allowing content to be delivered via APIs to any frontend—websites, apps, or even smart devices. This offers greater flexibility and performance.
2. Why should I use Laravel for a headless CMS?
Laravel’s robust API support, security features, and scalability make it ideal for headless CMS setups. Its ecosystem (including tools like Laravel Sanctum for API auth) simplifies development, making it a top choice for Laravel web development services.
3. Can I integrate Laravel’s headless CMS with React or Vue.js?
Absolutely! Laravel works seamlessly with JavaScript frameworks like React, Vue.js, and Next.js. The backend serves content via APIs, while the frontend framework handles the UI, creating a fast, dynamic user experience.
4. How does a headless CMS improve website performance?
By separating the frontend and backend, a headless CMS reduces server load and eliminates bloated themes/plugins. Content is delivered via optimized APIs, resulting in faster load times and better scalability.
5. Is SEO more challenging in a headless CMS setup?
Not if you do it right! Use server-side rendering (SSR) with frameworks like Next.js, implement proper meta tags, and leverage Laravel’s SEO tools. Many headless CMS sites rank just as well—or better—than traditional ones.
There you have it, folks! 🎉 Headless Laravel CMS is reshaping web development, and now you know why. Ready to make the switch?
#headless Laravel CMS#headless CMS Laravel#Laravel API backend#Laravel content management#Laravel for headless websites#Laravel vs traditional CMS#modern web development Laravel#Laravel frontend frameworks#Laravel headless architecture#decoupled Laravel CMS
0 notes
Text
0 notes
Text
Why Laravel’s Features Make It Ideal for Startups
🚀 Startups, Laravel is your go-to web framework! With MVC architecture, built-in authentication, and seamless APIs, it powers rapid development and scalability. ✅ Build your MVP fast and scale with confidence!
Read more: https://nectarbits.ca/blog/key-features-of-laravel-that-benefit-startups/
1 note
·
View note
Text
Weak API Authentication in Laravel: How to Secure It
Introduction
API authentication is a critical aspect of securing web applications. In Laravel, APIs allow developers to connect with the backend while keeping things modular and efficient. However, when API authentication is weak or poorly implemented, it leaves the door open for attackers to exploit sensitive data.

In this post, we’ll explore the risks of weak API authentication in Laravel, how to identify vulnerabilities, and ways to secure your API endpoints. We’ll also guide you through a coding example and introduce a free tool for a website security test to help you identify API vulnerabilities on your site.
Why Weak API Authentication Is Dangerous
APIs are a primary target for cybercriminals due to the sensitive data they expose. When authentication methods are weak, such as using insecure or predictable tokens, attackers can easily bypass security mechanisms and gain unauthorized access to your backend systems.
Here’s why weak API authentication is dangerous:
Data Breaches: Hackers can access user data, financial information, or any sensitive data stored in your database.
Unauthorized API Calls: Without proper authentication, malicious users can make API requests on behalf of authenticated users.
Denial of Service Attacks: Exploiting weak authentication can allow attackers to overload your systems or take them down entirely.
Common Causes of Weak API Authentication
Some common causes of weak API authentication include:
Using Default Tokens: Laravel provides several ways to authenticate APIs, but many developers still use the default tokens or insecure methods.
No Token Expiration: Not setting an expiration time for API tokens can lead to long-term vulnerabilities.
Insecure Password Storage: If you store passwords in plain text or use weak hashing algorithms, hackers can easily retrieve them.
Improper Rate Limiting: Failing to limit the number of API requests from a user can lead to brute-force attacks.
How to Secure API Authentication in Laravel
Here, we’ll walk you through securing your API authentication in Laravel, step by step, using modern techniques.
1. Use Laravel Passport for OAuth Authentication
Laravel Passport provides a complete OAuth2 server implementation for your Laravel application. It is the most secure and robust way to handle API authentication.
To install Laravel Passport, follow these steps:
composer require laravel/passport php artisan migrate php artisan passport:install
After installation, you need to configure the AuthServiceProvider to use Passport:
use Laravel\Passport\Passport; public function boot() { Passport::routes(); }
Then, update your api guard in config/auth.php to use Passport:
'guards' => [ 'api' => [ 'driver' => 'passport', 'provider' => 'users', ], ],
Now, you can authenticate users using OAuth tokens, which provides a much higher level of security.
2. Enable Token Expiration
Another important step is ensuring that API tokens expire after a certain period. By default, tokens generated by Passport are long-lived, but you can customize their expiration time.
To set token expiration, update the config/passport.php file:
'personal_access_tokens_expire_in' => now()->addDays(7),
This will ensure that tokens expire after 7 days, requiring users to re-authenticate.
3. Use Strong Hashing for Passwords
Make sure that you store passwords securely using bcrypt or Argon2 hashing. Laravel automatically hashes passwords using bcrypt, but you can configure it to use Argon2 as well in config/hashing.php:
'driver' => 'argon2i',
4. Implement Rate Limiting
To prevent brute-force attacks, you should implement rate limiting for your API. Laravel has a built-in rate limiting feature that you can enable easily in routes/api.php:
Route::middleware('throttle:60,1')->get('/user', function (Request $request) { return $request->user(); });
This will limit the API requests to 60 per minute, helping to prevent excessive login attempts.
Testing Your API Security
After implementing the above security measures, it's important to test your API for vulnerabilities. Use our Website Vulnerability Scanner to check your website’s API security and identify any weaknesses in your authentication methods.

Screenshot of the free tools webpage where you can access security assessment tools.
Conclusion
Securing API authentication in Laravel is crucial for preventing unauthorized access and protecting sensitive user data. By using OAuth tokens, setting expiration times, applying strong password hashing, and implementing rate limiting, you can significantly enhance your API security.
If you’re unsure about the security of your website or API, try out our Free Website Security Scanner tool to perform a vulnerability assessment.

An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities.
For more security tips and updates, visit our blog at Pentest Testing Corp.
0 notes
Text
Learn how Laravel makes API-first development easier! This blog talks about its strong features, simple integration, and tips for building APIs that can grow with your needs. It's great for developers who want to create reliable and future-ready solutions. Get insights into API-based app development with Laravel!
#benefits of laravel in api-first development#laravel api development#laravel development company in india
1 note
·
View note
Text
Best Practices for Laravel API Documentation Using Swagger
Creating clear and professional API documentation in Laravel is essential for seamless development and integration. Whether you're a solo developer or part of a Laravel web development company, proper API documentation ensures smooth collaboration, easier debugging, and a better developer experience.
Why API Documentation Matters in Laravel
API documentation is like a roadmap for developers—it tells them how to interact with your system without unnecessary guesswork. Well-structured documentation saves time, prevents errors, and improves overall productivity.
According to research, developers spend around 60% of their time understanding existing code rather than writing new code. Proper documentation significantly reduces this inefficiency.
Moreover, for businesses offering Laravel development services, detailed API documentation enhances reliability, making integrations seamless for clients and third-party developers.
Introduction to Swagger for Laravel APIs
Swagger is an industry-standard tool for API documentation that helps developers visualize, interact with, and test API endpoints in real time. It provides a user-friendly interface to explore available endpoints, request parameters, and response structures.
By integrating Swagger with Laravel, you create a self-explanatory API that minimizes back-and-forth communication between teams. It also supports OpenAPI Specification (OAS), making it compatible with various tools and platforms for automated API validation and compliance checks.
Installing Swagger in a Laravel Project
Getting Swagger up and running in Laravel is straightforward. First, you need to install the necessary package, configure it, and then generate the documentation. The typical installation process involves:
Installing a Swagger-compatible package such as darkaonline/l5-swagger using Composer.
Configuring Swagger settings in config/l5-swagger.php to align with your API structure.
Running a command to generate and serve the documentation.
Once set up, your API documentation becomes accessible through a browser-friendly interface, making it easy for developers to explore and test endpoints.
Structuring API Documentation with Swagger in Laravel
Good API documentation isn't just about listing endpoints; it's about making the information structured, readable, and usable. A well-organized Swagger documentation typically includes:
API Overview – A brief explanation of the API's purpose.
Endpoint Details – Each API route is documented with parameters, request types, and response structures.
Authentication Requirements – Specifies how users should authenticate requests.
Response Codes – Indicates expected success and error responses for clarity.
Annotating Laravel Controllers with Swagger
Swagger annotations make your API documentation dynamic and up-to-date by directly embedding documentation into Laravel controllers. Annotations define:
Endpoints – What routes exist and their HTTP methods.
Parameters – What request data is needed.
Responses – Expected data structures for various scenarios.
By embedding these annotations within controller methods, you ensure that documentation updates automatically when changes are made, reducing the risk of outdated documentation.
Defining API Models and Response Structures
To make API documentation crystal clear, defining request and response models is crucial. Models specify the expected input and output formats, helping developers understand how to interact with the API effectively.
For example, if an API returns a User object, defining it in Swagger ensures that developers know what attributes to expect, such as id, name, and email. This reduces confusion and eliminates guesswork when integrating the API into applications.
Generating and Accessing the API Documentation
Once annotations and models are in place, generating API documentation with Swagger is as simple as running a command. Laravel provides easy ways to access this documentation through the browser, usually at a predefined URL like /api/documentation.
With this setup, developers can test endpoints, send requests, and view responses in real time, ensuring accurate API interaction. This interactivity speeds up development and debugging, making it a must-have for Laravel projects.
Optimizing Laravel API Documentation for Better Usability
Great API documentation isn't just about completeness; it's about usability. Developers should be able to find information quickly, understand it easily, and apply it without hassle. To achieve this, best practices include:
Using clear, concise descriptions for endpoints and parameters.
Organizing endpoints logically by grouping related functionality.
Providing sample requests and responses to illustrate API behavior.
Ensuring documentation is mobile-friendly, as many developers refer to API docs on the go.
Keeping Documentation Up-to-Date
Outdated documentation is worse than no documentation. When APIs evolve, documentation must be updated accordingly. Failing to do so leads to frustration and miscommunication. Automated documentation generation using Swagger reduces this risk by ensuring real-time updates whenever API changes occur.
Many Laravel development companies enforce strict versioning policies, ensuring that updates to APIs come with corresponding documentation updates, keeping everything aligned for seamless development.
Making API Documentation Interactive with Swagger UI
One of Swagger’s standout features is Swagger UI, which transforms static API documentation into an interactive, web-based interface. Instead of manually testing API calls via command-line tools like cURL, developers can test them directly from the documentation interface.
With just a click, they can send requests, tweak parameters, and immediately see responses. This speeds up debugging, making it easier to spot errors and verify expected behaviors.
Laravel Development Services and API Documentation
For any professional Laravel development company, well-structured API documentation is a non-negotiable asset. It ensures smoother project handovers, third-party integrations, and overall application maintainability. Without clear API documentation, onboarding new developers takes longer, and troubleshooting becomes a nightmare.
How Laravel Development Companies Benefit from Swagger
Laravel development services rely on Swagger to:
Streamline communication between backend and frontend teams.
Ensure faster debugging and testing with an interactive UI.
Improve security by documenting authentication and authorization mechanisms.
Enhance scalability, as well-documented APIs make it easier to add new features without breaking existing ones.
Using Swagger ensures a professional and systematic approach to API management, making Laravel applications more reliable and scalable.
Ensuring API Consistency and Compliance
API consistency is critical for maintainability. Laravel developers follow strict API standards to ensure that endpoints, data formats, and error handling remain predictable.
Moreover, many industries require compliance with standards like RESTful API best practices and OpenAPI specifications. By leveraging Swagger, Laravel developers can maintain compliance effortlessly, ensuring APIs meet industry standards without additional effort.
Final Thoughts
Swagger has revolutionized API documentation, making it simpler, more interactive, and more accessible. Whether you’re a solo developer or a Laravel web development company, investing time in proper API documentation saves hours of troubleshooting in the long run.
Key Takeaways
Well-documented APIs enhance developer productivity.
Swagger provides an easy-to-use interface for API documentation.
Annotations help structure API descriptions effectively.
Laravel development services rely on clear documentation for smooth integration.
Regular updates keep API documentation useful and accurate.
Frequently Asked Questions (FAQs)
1. What is Swagger, and why should I use it for Laravel APIs?
Swagger is a powerful tool for API documentation that helps developers visualize, test, and interact with APIs. Using Swagger for Laravel APIs makes it easier to maintain documentation, ensure accuracy, and provide an interactive UI for developers to explore endpoints.
2. How do I install Swagger in a Laravel project?
Installing Swagger in Laravel involves adding a package like darkaonline/l5-swagger via Composer, configuring it in Laravel settings, and generating documentation using predefined commands. Once installed, API documentation becomes available through a web-based interface for easy access.
3. Can Swagger generate API documentation automatically?
Yes, Swagger can generate API documentation automatically by reading annotations embedded within Laravel controllers and models. This ensures that documentation remains up-to-date with minimal manual effort, reducing inconsistencies.
4. What are the benefits of using interactive API documentation?
Interactive API documentation allows developers to test endpoints directly from the documentation interface, eliminating the need for external tools. It speeds up development, simplifies debugging, and provides a better understanding of API behavior in real time.
5. How do Laravel development companies ensure API documentation quality?
Laravel development companies maintain API documentation quality by following structured guidelines, using tools like Swagger for automation, and ensuring that documentation updates align with API changes. Regular reviews and versioning policies further enhance clarity and usability.
#Laravel#Laravel Development#Laravel Framework#PHP Development#Web Development#Laravel Tips#Coding#Backend Development#Laravel Community#Software Development#Laravel API Documentation
0 notes
Text
Top Tools for Web Development in 2025
Web development is an ever-evolving field, requiring developers to stay updated with the latest tools, frameworks, and software. These tools not only enhance productivity but also simplify complex development processes. Whether you’re building a small business website or a complex web application, having the right tools in your toolkit can make all the difference. Here’s a rundown of the top…
View On WordPress
#Angular Framework#API Development Tools#Back-End Development Tools#Best Tools for Web Development 2024#Bootstrap for Responsive Design#Django Python Framework#Docker for Deployment#Front-End Development Tools#GitHub for Developers#Laravel PHP Framework#Modern Web Development Tools#Node.js Back-End Framework#Popular Web Development Software#React Development#Tailwind CSS#Testing and Debugging Tools#Vue.js for Web Development#Web Development Frameworks
0 notes
Text
This blog post explores how feature flags can be effectively implemented in #PHP and #Laravel projects to manage feature releases, perform A/B testing, and ensure safe, controlled deployments in a CI/CD environment.
1 note
·
View note
Text
The digital landscape thrives on seamless communication and data exchange. This is where APIs (Application Programming Interfaces) come into play, acting as the bridge between different applications. For developers seeking a powerful and efficient framework to build APIs, LaravelAPIs, Laravel shines brightly.
This comprehensive guide, brought to you by Sohojware, a leading US-based software development company, dives deep into the world of building RESTful APIs with Laravel. We'll explore the core aspects, equipping you with the knowledge to craft powerful APIs that fuel your applications.
What are RESTful APIs with Laravel?
APIs with Laravel, specifically RESTful APIs, adhere to the principles of Representational State Transfer (REST) architecture. REST dictates a set of guidelines for designing APIs that promote scalability, interoperability, and ease of use. Laravel, a popular PHP framework, offers exceptional support for building these RESTful APIs. Its built-in features and structured approach streamline development, making it a favorite among developers.
Why Build APIs with Laravel?
There are numerous reasons why Laravel stands out for crafting APIs. Here are just a few:
Rapid Development: Laravel's elegant syntax and pre-built functionalities accelerate the development process. You can focus on core functionalities rather than reinventing the wheel.
Robust Security: Laravel prioritizes security with features like built-in authentication, authorization, and CSRF protection. This ensures your APIs are well-guarded against vulnerabilities.
Scalability: APIs with Laravel are built to scale. The framework can handle increasing traffic and data demands efficiently, future-proofing your applications.
Elegant Documentation: Laravel encourages the creation of well-documented APIs. This makes it easier for other developers to understand and integrate with your creation.
Large Community: Laravel boasts a vast and active community. This provides you with ample resources, tutorials, and support when needed.
Building Blocks of a RESTful API with Laravel
Now, let's delve into the core components involved in building APIs with Laravel:
Setting Up Your Laravel Project:
Defining Data Models and Migrations:
Building Controllers:
Defining API Routes:
Handling Authentication and Authorization:
Testing Your API:
Beyond the Basics: Advanced Considerations for APIs with Laravel
While the above steps form the foundation, building production-grade APIs with Laravel involves additional considerations:
Error Handling: Implement comprehensive error handling mechanisms to provide informative messages in case of issues.
Data Validation: Enforce data validation rules to ensure data integrity and prevent unexpected behavior in your API.
Versioning: As your API evolves, implement versioning to manage changes and maintain compatibility with existing integrations.
Rate Limiting: Prevent abuse by implementing rate-limiting mechanisms to control the number of requests an API can receive.
FAQs about Building APIs with Laravel
Is Sohojware experienced in building APIs with Laravel?
Absolutely! Sohojware's expert developers have extensive experience crafting powerful and scalable APIs using Laravel. We can help you design, develop, and deploy APIs that align perfectly with your needs.
What are the benefits of using Sohojware for building my API?
Sohojware offers a comprehensive approach to API development. We not only focus on technical excellence but also prioritize security, scalability, and ongoing maintenance. We work closely with you to understand your requirements and deliver a high-performing API that fuels your application's success.
Does Sohojware provide post-development support for APIs with Laravel?
Sohojware understands that API maintenance is critical. We offer ongoing support plans to ensure your API remains secure, and up-to-date, and continues to meet your evolving needs.
How can I get started with building APIs with Laravel?
If you're new to Laravel or API development, don't worry! Sohojware can be your trusted partner in this journey. We offer comprehensive API development services, including:
API Consultation: Our experts can assess your project requirements and provide tailored recommendations.
API Design and Development: We'll craft a well-structured and efficient API that meets your specific needs.
API Testing and Optimization: Rigorous testing ensures your API performs optimally and delivers accurate results.
API Deployment and Maintenance: We handle the deployment process and provide ongoing support to keep your API running smoothly.
Sohojware's commitment to excellence ensures that your API project is in capable hands.
Conclusion
Building APIs with Laravel empowers you to create powerful and scalable applications. By understanding the fundamentals of RESTful architecture and leveraging Laravel's powerful features, you can develop APIs that drive innovation.
Sohojware, as a leading API development company in the US, is dedicated to helping you harness the full potential of Laravel for your API projects. Contact us today to discuss your API needs and let us guide you towards success.
Remember: A well-crafted API is the cornerstone of modern applications. Choose Laravel and Sohojware for a winning combination.
1 note
·
View note
Text
Looking to master API development? Check out our latest blog, "Building RESTful APIs with Laravel: A Complete Guide," for step-by-step instructions, best practices, and expert tips to enhance your Laravel projects. 🚀 Read More: https://greyspacecomputing.com/building-restful-apis-with-laravel/
0 notes