#laravel design patterns
Explore tagged Tumblr posts
Text
Crafting Clean and Maintainable Code with Laravel's Design Patterns
In today's fast-paced world, building robust and scalable web applications is crucial for businesses of all sizes. Laravel, a popular PHP framework, empowers developers to achieve this goal by providing a well-structured foundation and a rich ecosystem of tools. However, crafting clean and maintainable code remains paramount for long-term success. One powerful approach to achieve this is by leveraging Laravel's built-in design patterns.
What are Design Patterns?
Design patterns are well-defined, reusable solutions to recurring software development problems. They provide a proven approach to structuring code, enhancing its readability, maintainability, and flexibility. By adopting these patterns, developers can avoid reinventing the wheel and focus on the unique aspects of their application.
Laravel's Design Patterns:
Laravel incorporates several design patterns that simplify common development tasks. Here are some notable examples:
Repository Pattern: This pattern separates data access logic from the business logic, promoting loose coupling and easier testing. Laravel's Eloquent ORM is a practical implementation of this pattern.
Facade Pattern: This pattern provides a simplified interface to complex functionalities. Laravel facades, like Auth and Cache, offer an easy-to-use entry point for various application functionalities.
Service Pattern: This pattern encapsulates business logic within distinct classes, promoting modularity and reusability. Services can be easily replaced with alternative implementations, enhancing flexibility.
Observer Pattern: This pattern enables loosely coupled communication between objects. Laravel's events and listeners implement this pattern, allowing components to react to specific events without tight dependencies.
Benefits of Using Design Patterns:
Improved Code Readability: Consistent use of design patterns leads to cleaner and more predictable code structure, making it easier for any developer to understand and modify the codebase.
Enhanced Maintainability: By separating concerns and promoting modularity, design patterns make code easier to maintain and update over time. New features can be added or bugs fixed without impacting other parts of the application.
Increased Reusability: Design patterns offer pre-defined solutions that can be reused across different components, saving development time and effort.
Reduced Complexity: By providing structured approaches to common problems, design patterns help developers manage complexity and write more efficient code.
Implementing Design Patterns with Laravel:
Laravel doesn't enforce the use of specific design patterns, but it empowers developers to leverage them effectively. The framework's built-in libraries and functionalities often serve as implementations of these patterns, making adoption seamless. Additionally, the vast Laravel community provides numerous resources and examples to guide developers in using design patterns effectively within their projects.
Conclusion:
By understanding and applying Laravel's design patterns, developers can significantly improve the quality, maintainability, and scalability of their web applications. Clean and well-structured code not only benefits the development team but also creates a valuable asset for future maintenance and potential growth. If you're looking for expert guidance in leveraging Laravel's capabilities to build best-in-class applications, consider hiring a Laravel developer.
These professionals possess the necessary expertise and experience to implement design patterns effectively, ensuring your application is built with long-term success in mind.
FAQs
1. What are the different types of design patterns available in Laravel?
Laravel doesn't explicitly enforce specific design patterns, but it provides functionalities that serve as implementations of common patterns like Repository, Facade, Service, and Observer. Additionally, the framework's structure and libraries encourage the use of various other patterns like Strategy, Singleton, and Factory.
2. When should I use design patterns in my Laravel project?
Design patterns are particularly beneficial when your application is complex, involves multiple developers, or is expected to grow significantly in the future. By adopting patterns early on, you can establish a well-structured and maintainable codebase from the outset.
3. Are design patterns difficult to learn and implement?
Understanding the core concepts of design patterns is essential, but Laravel simplifies their implementation. The framework's built-in libraries and functionalities often serve as practical examples of these patterns, making it easier to integrate them into your project.
4. Where can I find more resources to learn about design patterns in Laravel?
The official Laravel documentation provides a good starting point https://codesource.io/brief-overview-of-design-pattern-used-in-laravel/. Additionally, the vast Laravel community offers numerous online resources, tutorials, and code examples that delve deeper into specific design patterns and their implementation within the framework.
5. Should I hire a Laravel developer to leverage design patterns effectively?
Hiring a Laravel developer or collaborating with a laravel development company can be advantageous if you lack the in-house expertise or require assistance in architecting a complex application. Experienced developers can guide you in selecting appropriate design patterns, ensure their proper implementation, and contribute to building a robust and maintainable codebase.
0 notes
Text
Efficient Web Application Management with Modular Designs
When designing with modularity in web application development the sole main focus is enhancing efficiency, scalability, and maintainability . This actually possible by breaking down an application into independent, reusable modules. In contrast to a monolithic approach, where all components are tightly coupled, modular architectural design structures the application into separate, self-contained units. In such case, you can modularize the account verification, product management, and payment processing.
This separation allows web developers to work on individual modules without disrupting the entire system, making updates, debugging, and feature additions more manageable. Just like by following Laravel’s modular principles using Service Providers, Repositories, and Packages, teams can develop cleaner, more structured codebases that are easier to scale and maintain.
Support Parallel Development and Flexibility
Modular architecture enables software development teams to build, test, and deploy individual features independently. If one module requires changes or optimizations, it can be modified without affecting the rest of the application, reducing downtime and improving development speed. So, the modular architecture is particularly beneficial for large-scale applications like an office furniture online system, where different teams may handle inventory, customer management, and order processing as separate modules. Try implementing modularization with Laravel, you'll achieve a robust, high-performing, and future-proof web application that efficiently adapts to growing demands.
#modular#architecture#modular architecture#laravel modular architecture#modular design#office furniture#online office furniture system#high performance#laravel modules#laravel framework#PHP framework#web application design#monolithic#parallel development#large scale applications#service providers#flexibility#maintainable application design#scalable application design#coupling#cohesion#reusable patterns#software patterns#software testing#web deevlopment
0 notes
Text
#coding#programming#laravel#laravel development company#laravel development services#laravel development agency#laravel development team#database#design patterns
1 note
·
View note
Text
Top 5 Common Database Design patterns in Laravel
In the world of Laravel development, a well-structured database is the bedrock of a robust and scalable application. While Laravel's Eloquent ORM provides a powerful abstraction layer for interacting with your data, understanding common database design patterns can significantly enhance your development process.
These patterns not only promote code organization and maintainability but also enable you to adapt your database structure to the unique needs of your application. By mastering these patterns, you can build efficient, reliable, and easily maintainable Laravel applications that can handle diverse data requirements.
1. Active Record Pattern:
This is the most common pattern used by Eloquent ORM in Laravel. It encapsulates database logic within model classes, allowing you to interact with the database using object-oriented methods.
Application
This pattern is well-suited for projects of any size and complexity. It simplifies database operations, making them easier to understand and maintain.
Example:
Advantages:
Simplicity: Easy to understand and implement.
Code Reusability: Model methods can be reused throughout your application.
Relationship Management: Built-in support for relationships between models.
Disadvantages:
Tight Coupling: Model logic is tightly coupled to the database, making it harder to test independently.
Complexity: Can become complex for large applications with complex data structures.
2. Data Mapper Pattern:
This pattern separates data access logic from domain logic. It uses a dedicated "mapper" class to translate between domain objects and database records.
Application
This pattern is useful for large-scale applications with complex domain models, as it allows for greater flexibility and modularity. It is particularly useful when working with multiple data sources or when you need to optimize for performance.
Example:
Advantages:
Flexibility: Easily change the database implementation without affecting business logic.
Testability: Easy to test independently from the database.
Modularity: Promotes a modular structure, separating concerns.
Disadvantages:
Increased Complexity: Requires more code and might be overkill for simple applications.
3. Repository Pattern:
This pattern provides an abstraction layer over the data access mechanism, offering a consistent interface for interacting with the database.
Application
This pattern promotes loose coupling and simplifies testing, as you can easily mock the repository and control the data returned. It is often used in conjunction with the Data Mapper pattern.
Example:
Advantages:
Loose Coupling: Decouples business logic from specific data access implementation.
Testability: Easy to mock repositories for testing.
Reusability: Reusable interface for accessing different data sources.
Disadvantages:
Initial Setup: Can require more setup compared to Active Record.
4. Table Inheritance Pattern:
This pattern allows you to create a hierarchical relationship between tables, where child tables inherit properties from a parent table.
Application
This pattern is useful for creating polymorphic relationships and managing data for different types of entities. For example, you could have a User table and separate tables for AdminUser and CustomerUser that inherit from the parent table.
Example:
Advantages:
Polymorphism: Enables handling different types of entities using a common interface.
Code Reusability: Reuses properties and methods from the parent table.
Data Organization: Provides a structured way to organize data for different types of users.
Disadvantages:
Increased Database Complexity: Can lead to a more complex database structure.
5. Schema-less Database Pattern:
This pattern avoids the use of a predefined schema and allows for dynamic data structures. This is commonly used with NoSQL databases like MongoDB.
Application
This pattern is suitable for projects that require highly flexible data structures, such as social media platforms or analytics systems.
Example:
Advantages:
Flexibility: Easily adapt to changing data structures.
Scalability: Suitable for high-volume, rapidly changing data.
High Performance: Efficient for specific use cases like real-time analytics.
Disadvantages:
Increased Complexity: Requires a different approach to querying and data manipulation.
Data Consistency: Can be challenging to maintain data consistency without a schema.
Choosing the Right Pattern:
The best pattern for your project depends on factors like project size, complexity, performance requirements, and your team's experience. It is important to choose patterns that align with the specific needs of your application and ensure long-term maintainability and scalability.
Conclusion:
This exploration of common database design patterns used in Laravel has shed light on the importance of strategic database structuring for building robust and scalable applications. From the simplicity of the Active Record pattern to the sophisticated capabilities of the Data Mapper and Repository patterns, each pattern offers distinct benefits that cater to specific project needs.
By understanding the strengths and applications of these patterns, Laravel developers can choose the optimal approach for their projects, ensuring a well-organized, efficient, and maintainable database architecture. Ultimately, mastering these patterns empowers you to create Laravel applications that are not only functional but also adaptable to evolving data requirements and future growth.
#laravel#laravel development company#laravel framework#laravel developers#database#design#coding#programming
4 notes
·
View notes
Text
Comparing Laravel And WordPress: Which Platform Reigns Supreme For Your Projects? - Sohojware
Choosing the right platform for your web project can be a daunting task. Two popular options, Laravel and WordPress, cater to distinct needs and offer unique advantages. This in-depth comparison by Sohojware, a leading web development company, will help you decipher which platform reigns supreme for your specific project requirements.
Understanding Laravel
Laravel is a powerful, open-source PHP web framework designed for the rapid development of complex web applications. It enforces a clean and modular architecture, promoting code reusability and maintainability. Laravel offers a rich ecosystem of pre-built functionalities and tools, enabling developers to streamline the development process.
Here's what makes Laravel stand out:
MVC Architecture: Laravel adheres to the Model-View-Controller (MVC) architectural pattern, fostering a well-organized and scalable project structure.
Object-Oriented Programming: By leveraging object-oriented programming (OOP) principles, Laravel promotes code clarity and maintainability.
Built-in Features: Laravel boasts a plethora of built-in features like authentication, authorization, caching, routing, and more, expediting the development process.
Artisan CLI: Artisan, Laravel's powerful command-line interface (CLI), streamlines repetitive tasks like code generation, database migrations, and unit testing.
Security: Laravel prioritizes security by incorporating features like CSRF protection and secure password hashing, safeguarding your web applications.
However, Laravel's complexity might pose a challenge for beginners due to its steeper learning curve compared to WordPress.
Understanding WordPress
WordPress is a free and open-source content management system (CMS) dominating the web. It empowers users with a user-friendly interface and a vast library of plugins and themes, making it ideal for creating websites and blogs without extensive coding knowledge.
Here's why WordPress is a popular choice:
Ease of Use: WordPress boasts an intuitive interface, allowing users to create and manage content effortlessly, even with minimal technical expertise.
Flexibility: A vast repository of themes and plugins extends WordPress's functionality, enabling customization to suit diverse website needs.
SEO Friendliness: WordPress is inherently SEO-friendly, incorporating features that enhance your website's ranking.
Large Community: WordPress enjoys a massive and active community, providing abundant resources, tutorials, and support.
While user-friendly, WordPress might struggle to handle complex functionalities or highly customized web applications.
Choosing Between Laravel and WordPress
The optimal platform hinges on your project's specific requirements. Here's a breakdown to guide your decision:
Laravel is Ideal For:
Complex web applications require a high degree of customization.
Projects demanding powerful security features.
Applications with a large user base or intricate data structures.
Websites require a high level of performance and scalability.
WordPress is Ideal For:
Simple websites and blogs.
Projects with a primary focus on content management.
E-commerce stores with basic product management needs (using WooCommerce plugin).
Websites requiring frequent content updates by non-technical users.
Sohojware, a well-versed web development company in the USA, can assist you in making an informed decision. Our team of Laravel and WordPress experts will assess your project's needs and recommend the most suitable platform to ensure your web project's success.
In conclusion, both Laravel and WordPress are powerful platforms, each catering to distinct project needs. By understanding their strengths and limitations, you can make an informed decision that empowers your web project's success. Sohojware, a leading web development company in the USA, possesses the expertise to guide you through the selection process and deliver exceptional results, regardless of the platform you choose. Let's leverage our experience to bring your web vision to life.
FAQs about Laravel and WordPress Development by Sohojware
1. Which platform is more cost-effective, Laravel or WordPress?
While WordPress itself is free, ongoing maintenance and customization might require development expertise. Laravel projects typically involve developer costs, but these can be offset by the long-term benefits of a custom-built, scalable application. Sohojware can provide cost-effective solutions for both Laravel and WordPress development.
2. Does Sohojware offer support after project completion?
Sohojware offers comprehensive post-development support for both Laravel and WordPress projects. Our maintenance and support plans ensure your website's continued functionality, security, and performance.
3. Can I migrate my existing website from one platform to another?
Website migration is feasible, but the complexity depends on the website's size and architecture. Sohojware's experienced developers can assess the migration feasibility and execute the process seamlessly.
4. How can Sohojware help me with Laravel or WordPress development?
Sohojware offers a comprehensive range of Laravel and WordPress development services, encompassing custom development, theme and plugin creation, integration with third-party applications, and ongoing maintenance.
5. Where can I find more information about Sohojware's Laravel and WordPress development services?
You can find more information about Sohojware's Laravel and WordPress development services by visiting our website at https://sohojware.com/ or contacting our sales team directly. We'd happily discuss your project requirements and recommend the most suitable platform to achieve your goals.
3 notes
·
View notes
Text
Top 10 Laravel Development Companies in the USA in 2024
Laravel is a widely-used open-source PHP web framework designed for creating web applications using the model-view-controller (MVC) architectural pattern. It offers developers a structured and expressive syntax, as well as a variety of built-in features and tools to enhance the efficiency and enjoyment of the development process.
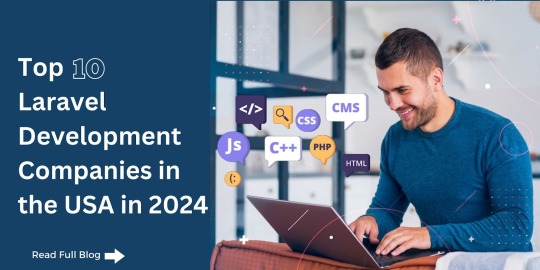
Key components of Laravel include:
1. Eloquent ORM (Object-Relational Mapping): Laravel simplifies database interactions by enabling developers to work with database records as objects through a powerful ORM.
2. Routing: Laravel provides a straightforward and expressive method for defining application routes, simplifying the handling of incoming HTTP requests.
3. Middleware: This feature allows for the filtering of HTTP requests entering the application, making it useful for tasks like authentication, logging, and CSRF protection.
4. Artisan CLI (Command Line Interface): Laravel comes with Artisan, a robust command-line tool that offers commands for tasks such as database migrations, seeding, and generating boilerplate code.
5. Database Migrations and Seeding: Laravel's migration system enables version control of the database schema and easy sharing of changes across the team. Seeding allows for populating the database with test data.
6. Queue Management: Laravel's queue system permits deferred or background processing of tasks, which can enhance application performance and responsiveness.
7. Task Scheduling: Laravel provides a convenient way to define scheduled tasks within the application.
What are the reasons to opt for Laravel Web Development?
Laravel makes web development easier, developers more productive, and web applications more secure and scalable, making it one of the most important frameworks in web development.
There are multiple compelling reasons to choose Laravel for web development:
1. Clean and Organized Code: Laravel provides a sleek and expressive syntax, making writing and maintaining code simple. Its well-structured architecture follows the MVC pattern, enhancing code readability and maintainability.
2. Extensive Feature Set: Laravel comes with a wide range of built-in features and tools, including authentication, routing, caching, and session management.
3. Rapid Development: With built-in templates, ORM (Object-Relational Mapping), and powerful CLI (Command Line Interface) tools, Laravel empowers developers to build web applications quickly and efficiently.
4. Robust Security Measures: Laravel incorporates various security features such as encryption, CSRF (Cross-Site Request Forgery) protection, authentication, and authorization mechanisms.
5. Thriving Community and Ecosystem: Laravel boasts a large and active community of developers who provide extensive documentation, tutorials, and forums for support.
6. Database Management: Laravel's migration system allows developers to manage database schemas effortlessly, enabling version control and easy sharing of database changes across teams. Seeders facilitate the seeding of databases with test data, streamlining the testing and development process.
7. Comprehensive Testing Support: Laravel offers robust testing support, including integration with PHPUnit for writing unit and feature tests. It ensures that applications are thoroughly tested and reliable, reducing the risk of bugs and issues in production.
8. Scalability and Performance: Laravel provides scalability options such as database sharding, queue management, and caching mechanisms. These features enable applications to handle increased traffic and scale effectively.
Top 10 Laravel Development Companies in the USA in 2024
The Laravel framework is widely utilised by top Laravel development companies. It stands out among other web application development frameworks due to its advanced features and development tools that expedite web development. Therefore, this article aims to provide a list of the top 10 Laravel Development Companies in 2024, assisting you in selecting a suitable Laravel development company in the USA for your project.
IBR Infotech
IBR Infotech excels in providing high-quality Laravel web development services through its team of skilled Laravel developers. Enhance your online visibility with their committed Laravel development team, which is prepared to turn your ideas into reality accurately and effectively. Count on their top-notch services to receive the best as they customise solutions to your business requirements. Being a well-known Laravel Web Development Company IBR infotech is offering the We provide bespoke Laravel solutions to our worldwide customer base in the United States, United Kingdom, Europe, and Australia, ensuring prompt delivery and competitive pricing.
Additional Information-
GoodFirms : 5.0
Avg. hourly rate: $25 — $49 / hr
No. Employee: 10–49
Founded Year : 2014
Verve Systems
Elevate your enterprise with Verve Systems' Laravel development expertise. They craft scalable, user-centric web applications using the powerful Laravel framework. Their solutions enhance consumer experience through intuitive interfaces and ensure security and performance for your business.
Additional Information-
GoodFirms : 5.0
Avg. hourly rate: $25
No. Employee: 50–249
Founded Year : 2009
KrishaWeb
KrishaWeb is a world-class Laravel Development company that offers tailor-made web solutions to our clients. Whether you are stuck up with a website concept or want an AI-integrated application or a fully-fledged enterprise Laravel application, they can help you.
Additional Information-
GoodFirms : 5.0
Avg. hourly rate: $50 - $99/hr
No. Employee: 50 - 249
Founded Year : 2008
Bacancy
Bacancy is a top-rated Laravel Development Company in India, USA, Canada, and Australia. They follow Agile SDLC methodology to build enterprise-grade solutions using the Laravel framework. They use Ajax-enabled widgets, model view controller patterns, and built-in tools to create robust, reliable, and scalable web solutions
Additional Information-
GoodFirms : 4.8
Avg. hourly rate: $25 - $49/hr
No. Employee: 250 - 999
Founded Year : 2011
Elsner
Elsner Technologies is a Laravel development company that has gained a high level of expertise in Laravel, one of the most popular PHP-based frameworks available in the market today. With the help of their Laravel Web Development services, you can expect both professional and highly imaginative web and mobile applications.
Additional Information-
GoodFirms : 5
Avg. hourly rate: < $25/hr
No. Employee: 250 - 999
Founded Year : 2006
Logicspice
Logicspice stands as an expert and professional Laravel web development service provider, catering to enterprises of diverse scales and industries. Leveraging the prowess of Laravel, an open-source PHP framework renowned for its ability to expedite the creation of secure, scalable, and feature-rich web applications.
Additional Information-
GoodFirms : 5
Avg. hourly rate: < $25/hr
No. Employee: 50 - 249
Founded Year : 2006
Sapphire Software Solutions
Sapphire Software Solutions, a leading Laravel development company in the USA, specialises in customised Laravel development, enterprise solutions,.With a reputation for excellence, they deliver top-notch services tailored to meet your unique business needs.
Additional Information-
GoodFirms : 5
Avg. hourly rate: NA
No. Employee: 50 - 249
Founded Year : 2002
iGex Solutions
iGex Solutions offers the World’s Best Laravel Development Services with 14+ years of Industry Experience. They have 10+ Laravel Developer Experts. 100+ Elite Happy Clients from there Services. 100% Client Satisfaction Services with Affordable Laravel Development Cost.
Additional Information-
GoodFirms : 4.7
Avg. hourly rate: < $25/hr
No. Employee: 10 - 49
Founded Year : 2009
Hidden Brains
Hidden Brains is a leading Laravel web development company, building high-performance Laravel applications using the advantage of Laravel's framework features. As a reputed Laravel application development company, they believe your web application should accomplish the goals and can stay ahead of the rest.
Additional Information-
GoodFirms : 4.9
Avg. hourly rate: < $25/hr
No. Employee: 250 - 999
Founded Year : 2003
Matellio
At Matellio, They offer a wide range of custom Laravel web development services to meet the unique needs of their global clientele. There expert Laravel developers have extensive experience creating robust, reliable, and feature-rich applications
Additional Information-
GoodFirms : 4.8
Avg. hourly rate: $50 - $99/hr
No. Employee: 50 - 249
Founded Year : 2014
What advantages does Laravel offer for your web application development?
Laravel, a popular PHP framework, offers several advantages for web application development:
Elegant Syntax
Modular Packaging
MVC Architecture Support
Database Migration System
Blade Templating Engine
Authentication and Authorization
Artisan Console
Testing Support
Community and Documentation
Conclusion:
I hope you found the information provided in the article to be enlightening and that it offered valuable insights into the top Laravel development companies.
These reputable Laravel development companies have a proven track record of creating customised solutions for various sectors, meeting client requirements with precision.
Over time, these highlighted Laravel developers for hire have completed numerous projects with success and are well-equipped to help advance your business.
Before finalising your choice of a Laravel web development partner, it is essential to request a detailed cost estimate and carefully examine their portfolio of past work.
#Laravel Development Companies#Laravel Development Companies in USA#Laravel Development Company#Laravel Web Development Companies#Laravel Web Development Services
2 notes
·
View notes
Text

What is MVC Architecture?
MVC stands for Model-View-Controller. It is a design pattern that divides an application into three key components:
Model – Handles data and business logic.
View – Manages the user interface and presentation layer.
Controller – Acts as a bridge between the Model and the View, managing user requests and responses.
This separation ensures cleaner code, better maintainability, and faster development.
The Model-View-Controller (MVC) framework is an architectural/design pattern that separates an application into three main logical components Model, View, and Controller. Each architectural component is built to handle specific development aspects of an application.
0 notes
Text
Detect SSRF in Symfony Apps – Free Security Checker
Server-Side Request Forgery (SSRF) is a critical web application vulnerability that occurs when an attacker is able to make the server perform unintended requests to internal or external systems. Symfony, while secure by design, can still be vulnerable to SSRF when insecure coding patterns are used.

In this post, we'll break down how SSRF works in Symfony, show coding examples, and share how you can detect it using our website vulnerability scanner online free.
🚨 What is SSRF and Why is it Dangerous?
SSRF occurs when a web server is tricked into sending a request to an unintended destination, including internal services like localhost, cloud metadata endpoints, or other restricted resources.
Impact of SSRF:
Internal network scanning
Accessing cloud instance metadata (AWS/GCP/Azure)
Bypassing IP-based authentication
🧑💻 SSRF Vulnerability in Symfony: Example
Here’s a simplified example in a Symfony controller where SSRF could be introduced:
// src/Controller/RequestController.php namespace App\Controller; use Symfony\Component\HttpFoundation\Request; use Symfony\Component\HttpFoundation\Response; use Symfony\Bundle\FrameworkBundle\Controller\AbstractController; class RequestController extends AbstractController { public function fetch(Request $request): Response { $url = $request->query->get('url'); // Unvalidated user input! // SSRF vulnerability: external requests with unsanitized user input $content = file_get_contents($url); return new Response($content); } }
⚠️ What’s wrong here?
The $url parameter comes directly from user input and is passed to file_get_contents() with no validation. This allows attackers to make arbitrary requests through your server.
✅ How to Fix It
Use a whitelist approach:
$allowedHosts = ['example.com', 'api.example.com']; $parsedUrl = parse_url($url); if (!in_array($parsedUrl['host'], $allowedHosts)) { throw new \Exception("Disallowed URL"); }
Better yet, avoid allowing user-defined URLs entirely unless absolutely necessary. Always sanitize and validate any user input that affects backend requests.
🧪 Test Your Site for SSRF and Other Vulnerabilities
Our free website vulnerability scanner helps you find SSRF and many other issues instantly. No sign-up required.
📷 Screenshot of the tool homepage

Screenshot of the free tools webpage where you can access security assessment tools.
Just input your domain and get a detailed vulnerability assessment to check Website Vulnerability in seconds.
📷 Screenshot of a generated vulnerability report

An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities.
🔗 Try Our Free Website Security Checker
Go to https://free.pentesttesting.com and check your site for SSRF and dozens of other vulnerabilities.
💡 Real-World SSRF Exploitation: Metadata Services
In many cloud setups (like AWS), SSRF is used to access instance metadata services, such as:
curl http://169.254.169.254/latest/meta-data/
If the Symfony app allows attackers to proxy requests through the server, they can potentially leak AWS credentials!
🔍 Symfony + SSRF in HTTP Client
If you're using the HttpClient component:
use Symfony\Contracts\HttpClient\HttpClientInterface; public function __construct(HttpClientInterface $client) { $this->client = $client; } public function fetchUrl(string $url) { $response = $this->client->request('GET', $url); return $response->getContent(); }
Danger: Still vulnerable if $url is user-controlled.
Mitigation:
Validate and sanitize the input
Use allowlists
Block private IPs like 127.0.0.1, 169.254.169.254, etc.
📘 Learn More from Our Cybersecurity Blog
Visit the Pentest Testing Blog for in-depth guides on web application security, including Symfony, Laravel, and Node.js vulnerabilities.
🚀 New: Web App Penetration Testing Services
Want professional help?
Check out our Web Application Penetration Testing Services for in-depth manual testing, compliance audits, and security consulting by certified experts.
📣 Conclusion
SSRF is a silent killer when left unchecked. Symfony developers must avoid directly using unvalidated input for server-side HTTP requests. You can:
Use input validation
Use whitelists
Block internal IP access
Test your applications regularly
👉 Scan your site now for Website Security check with our free security tool.
Stay secure. Stay informed. Follow us at https://www.pentesttesting.com/blog/ for more tips!
#cyber security#cybersecurity#data security#pentesting#security#php#the security breach show#coding#symfony
1 note
·
View note
Text
Sky Appz Academy: Best Full Stack Development Training in Coimbatore
Revolutionize Your Career with Top-Class Full Stack Training
With today's digital-first economy, Full Stack developers have emerged as the pillars of the technology sector. Sky Appz Academy in Coimbatore is at the cutting edge of technology training with a full-scale Full Stack Development course that makes beginners job-ready professionals. Our 1000+ hour program is a synergy of theoretical training and hands-on practice, providing students with employers' sought skills upon graduation.
Why Full Stack Development Should be Your Career?
The technological world is transforming at a hitherto unknown speed, and Full Stack developers are the most skilled and desired experts in the job market today. As per recent NASSCOM reports:
High Demand: There is a 35% year-over-year rise in Full Stack developer employment opportunities
Lucrative Salaries: Salary ranges for junior jobs begin from ₹5-8 LPA, while mature developers get ₹15-25 LPA
Career Flexibility: Roles across startups, businesses, and freelance initiatives
Future-Proof Skills: Full Stack skills stay up-to-date through technology changes
At Sky Appz Academy, we've structured our course work to not only provide coding instructions, but also to develop problem-solving skills and engineering thinking necessary for long-term professional success.
In-Depth Full Stack Course
Our carefully structured program encompasses all areas of contemporary web development:
Frontend Development (300+ hours)
•Core Foundations: HTML5, CSS3, JavaScript (ES6+)
•Advanced Frameworks: React.js with Redux, Angular
•Responsive Design: Bootstrap 5, Material UI, Flexbox/Grid
•State Management: Context API, Redux Toolkit
•Progressive Web Apps: Service workers, offline capabilities
Backend Development (350+ hours)
•Node.js Ecosystem: Express.js, NestJS
•Python Stack: Django REST framework, Flask
•PHP Development: Laravel, CodeIgniter
•API Development: RESTful services, GraphQL
•Authentication: JWT, OAuth, Session management
Database Systems (150+ hours)
•SQL Databases: MySQL, PostgreSQL
•NoSQL Solutions: MongoDB, Firebase
•ORM Tools: Mongoose, Sequelize
•Database Design: Normalization, Indexing
•Performance Optimization: Query tuning, caching
DevOps & Deployment (100+ hours)
•Cloud Platforms: AWS, Azure fundamentals
•Containerization: Docker, Kubernetes basics
•CI/CD Pipelines: GitHub Actions, Jenkins
• Performance Monitoring: New Relic, Sentry
• Security Best Practices: OWASP top 10
What Sets Sky Appz Academy Apart?
1)Industry-Experienced Instructors
• Our faculty includes senior developers with 8+ years of experience
• Regular guest lectures from CTOs and tech leads
• 1:1 mentorship sessions for personalized guidance
Project-Based Learning Approach
• 15+ mini-projects throughout the course
• 3 major capstone projects
• Real-world client projects for select students
• Hackathons and coding competitions
State-of-the-Art Infrastructure
• Dedicated coding labs with high-end systems
• 24/7 access to learning resources
• Virtual machines for cloud practice
•\tNew software and tools
Comprehensive Career Support
•Resume and LinkedIn profile workshops
•Practice technical interviews (100+ held every month)
•Portfolio development support
•Private placement drives with 150+ recruiters
•Access to alumni network
Detailed Course Structure
•Month 1-2: Building Foundations
•Web development basics
•JavaScript programming logic
•Version control using Git/GitHub
•Algorithms and data structures basics
Month 3-4: Core Development Skills
•Frontend frameworks in-depth
•Backend architecture patterns
•Database design and implementation
•API development and integration
Month 5-6: Advanced Concepts & Projects
•Microservices architecture
•Performance optimization
•Security implementation
•Deployment strategies
•Capstone project development
Career Outcomes and Placement Support
•Our graduates have been placed successfully in positions such as:
•Full Stack Developer
•Frontend Engineer
•Backend Specialist
•Web Application Developer
•UI/UX Engineer
•Software Developer
Placement Statistics (2024 Batch):
•94% placement rate within 3 months
•Average starting salary: ₹6.8 LPA
•Highest package: ₹14.5 LPA
•150+ hiring partners including startups and MNCs
Our placement cell, dedicated to serving our students, offers:
•Regular recruitment drives
•Profile matching with company needs
•Salary negotiation support
•Continuous upskilling opportunities
Flexible Learning Options
•Understanding the varied needs of our students, we provide:
•Weekday Batch: Monday-Friday (4 hours/day)
• Weekend Batch: Sat-Sun (8 hours/day)
• Hybrid Model: Blend online and offline learning
• Self-Paced Option: For working professionals
Who Should Enroll?
Our course is perfect for:
• Fresh graduates interested in tech careers
• Working professionals who wish to upskillCareer changers joining IT field
• Entrepreneurs to create their own products
• Freelancers who wish to increase service offerings
Admission Process
Application: Fill online application
Counseling: Career counseling session
Assessment: Simple aptitude test
Enrollment: Payment of fees and onboarding
EMI options available
Scholarships for deserving students
Group discounts applicable
Why Coimbatore for Tech Education?
•Coimbatore has become South India's budding tech hub with:
•300+ IT organizations and startups
•Lower cost of living than metros
•Vibrant developer community
•Very good quality of life
Take the First Step Toward Your Dream Career
Sky Appz Academy's Full Stack Development course is not just a course - it is a career change experience. With our industry-relevant course material, experienced mentors, and robust placement assistance, we bring you all it takes to shine in the modern-day competitive tech industry.
Limited Seats Left! Come over to our campus at Gandhipuram or speak with one of our counselors today to plan a demo class and see how we can guide you to become successful in technology.
Contact Information:
Sky Appz Academy
123 Tech Park Road, Gandhipuram
Coimbatore - 641012
Website: www.skyappzacademy.com
Frequently Asked Questions
Q: Do we need programming background?
A: No, but basic computer know-how is required.
Q: What is the class size?
A: We maintain 15:1 student-teacher ratio for personalized attention.
Q: Do you provide certification?
A: Yes, course completion certificate with project portfolio.
Q: Are there installment options?
A: Yes, we offer convenient EMI plans.
Q: What if I miss classes?
A: Recorded sessions and catch-up classes are available.
Enroll Now!
By
Skyappzacademy
0 notes
Text
PHP Development: A Timeless Technology Powering the Web in 2025
PHP development remains one of the most trusted and widely used approaches for building dynamic websites and web applications. Despite the rise of new programming languages and frameworks, PHP continues to evolve and maintain its stronghold in the web development ecosystem. In fact, popular platforms like WordPress, Facebook, and Wikipedia still rely on PHP as a key part of their technology stack. In this blog, we’ll explore what PHP development is, its advantages, common use cases, modern tools, and why it continues to be a smart choice in 2025. What is PHP Development? PHP (Hypertext Preprocessor) is a server-side scripting language specifically designed for web development. PHP development refers to the process of using PHP to create interactive and dynamic web pages, manage backend functionality, and build full-stack applications. From simple landing pages to complex enterprise systems, PHP allows developers to create secure, scalable, and performance-driven websites efficiently. Why Choose PHP for Web Development? Even in 2025, PHP offers several compelling reasons to be your go-to web development language: 1. Open-Source and Cost-Effective PHP is free to use, reducing development costs for individuals and businesses alike. With a wide range of open-source tools and libraries, developers can build robust applications without high overhead. 2. Cross-Platform Compatibility PHP runs on all major operating systems, including Windows, Linux, and macOS. It's compatible with nearly all servers and easily integrates with MySQL, PostgreSQL, and other popular databases. 3. Massive Community Support One of PHP’s greatest strengths is its global community. Thousands of developers contribute to its ecosystem, creating libraries, plugins, and frameworks that enhance productivity and solve real-world challenges. 4. Frameworks That Speed Up Development Modern PHP frameworks like Laravel, Symfony, and CodeIgniter simplify development with built-in tools, reusable components, and architectural patterns like MVC (Model-View-Controller). 5. High Performance with PHP 8+ The release of PHP 8 and above has brought significant performance improvements, thanks to features like JIT (Just-In-Time) compilation. PHP applications now load faster and use fewer server resources. Popular Use Cases of PHP Development PHP is highly versatile and used in a variety of web development scenarios: Content Management Systems (CMS): WordPress, Joomla, Drupal eCommerce Platforms: Magento, WooCommerce, OpenCart Custom Web Applications: CRMs, ERPs, dashboards API Development: RESTful APIs for mobile and web apps Social Networks & Forums: Community platforms, blogs, discussion boards Essential Tools for PHP Developers To build and manage PHP applications efficiently, developers often use the following tools: Laravel – A modern PHP framework for clean, elegant code. Composer – Dependency manager for PHP. PHPStorm – Feature-rich IDE tailored for PHP. XAMPP/WAMP – Local development environments. Postman – Testing APIs built with PHP. PHP Development Best Practices To get the most out of PHP development, follow these proven practices: Use a framework to structure your application. Sanitize and validate all user inputs to prevent security threats. Write modular, reusable code using object-oriented programming. Leverage Composer for package management.
0 notes
Text
Affordable PPC advertising services for small businesses
Laravel Customized Portal Development Services – Config Infotech
Introduction to Laravel Customized Portal Development
In today's digital world, companies need scalable, secure, and strong web solutions to address their business requirements. Laravel, a highly powerful PHP framework, is a preferred choice for building customized portals that improve business efficiency and user experience. Config Infotech offers Laravel customized portal development services, providing customized solutions that meet the specific needs of businesses from various industries.
Our skilled Laravel programmers create high-performance, feature-rich portals that enable enterprises to automate operations, streamline workflow, and boost customer interaction. Whether you are looking to create a business management portal, eCommerce portal, customer relationship management (CRM) system, or employee management portal, our developers facilitate smooth development and deployment.
Why Laravel is Best Suited for Custom Portal Development?
Laravel is an extremely flexible, open-source PHP framework that boasts security, scalability, and features that are very developer-friendly. The following are some of the reasons why Laravel is perfect for creating customized portals:
MVC Architecture – Laravel uses the Model-View-Controller (MVC) architecture pattern, ensuring clean code structure and maintenance is easier.
Built-in Authentication & Security – Laravel has strong security features that defend against SQL injection, cross-site request forgery, and other security attacks.
Blade Templating Engine – Facilitates UI development with reusable templates.
Eloquent ORM – Simplifies database interactions through an object-relational mapping (ORM) system.
Seamless Third-Party Integration – Laravel makes it easy to integrate APIs, payment gateways, and external applications.
Scalability & Performance Optimization – Guarantees that your portal remains responsive and efficient even as your business expands.
Our Laravel Customized Portal Development Services
We, at Config Infotech, offer full-cycle Laravel portal development solutions to ensure your business receives a rich-featured, tailored solution. Our solutions are:
Business Process Automation Portals
We create automated business management portals that assist businesses in workflow automation, increased productivity, and collaboration between teams. These portals may incorporate modules such as project management, task assignment, reporting, and communication.
Custom eCommerce Portals
Our team is eCommerce portal development experts on Laravel. We implement secure payment gateways, product filtering with advanced features, inventory management, and easy-to-use dashboards to improve shopping.
Customer Relationship Management (CRM) Portals
Config Infotech develops custom CRM solutions that assist companies in handling customer interactions, lead tracking, and streamlining sales processes. Customer segmentation, automated follow-up, analytics, and customized marketing tools are among the features.
Employee & HR Management Portals
We create employee and HR management portals with features such as employee attendance tracking, payroll management, performance evaluation, and internal communication tools for streamlined workforce management.
Healthcare & Telemedicine Portals
We build HIPAA-compliant Laravel-based healthcare portals for healthcare companies that have patient management systems, appointment scheduling, telemedicine consultation, and electronic health records (EHR) management.
Learning Management Systems
Config Infotech designs scalable Learning Management Systems (LMS) that enable online education and corporate training. Our LMS offerings are inclusive of course management, student tracking, assessment tools, and real-time analytics.
Real Estate & Property Management Portals
We provide bespoke real estate portals enabling property listings, virtual tours, tenant management, and payment processing for real estate companies and property managers.
Job Portals & Recruitment Platforms
Our employment portals built in Laravel feature functionalities such as posting jobs, profiles of candidates, parsing resumes, matchmaking based on AI, and employer dashboards.
Finance & Accounting Portals
We develop reliable financial portals for automatic invoicing, tax deduction, financial report generation, and multiple currency processing for companies undertaking global operations.
Most Important Features of Our Laravel Portals
Easy-to-Use Dashboard – User-friendly dashboard for simple accessibility and management.
Multi-User Roles & Access Control – Allow the definition of user roles and access permissions to secure access.
API Integration – Smooth integration with third-party services such as payment gateways, SMS, and email notifications.
SEO-Friendly URLs & Performance Optimization – Improves visibility and enhances speed.
Real-time Data Processing & Analytics – Facilitates data-driven business decisions.
Responsive & Mobile-Friendly Design – Designed for desktop and mobile devices.
Multi-Language & Multi-Currency Support – Suitable for international businesses.
Regular Maintenance & Support – Regular technical support for hassle-free operations.
Our Laravel Development Process
At Config Infotech, we implement a systematic development process to ensure quality and on-time delivery:
Requirement Analysis – Getting to know your business objectives, target segment, and requirements.
Planning & Wireframing – Developing a roadmap, defining the architecture, and creating wireframes.
Development & Integration – Writing clean code, making it scalable, and integrating required features.
Testing & Quality Assurance – Performing thorough testing for performance, security, and usability.
Deployment & Training – Deployment of the portal and training for its effective usage.
Maintenance & Upgrades – Providing post-launch support, routine updates, and feature additions.
Why Opt for Config Infotech for Laravel Customized Portal Development?
Experienced Laravel Developers – Our developers possess years of experience in Laravel development.
Custom Solutions for Every Business – We provide customized solutions as per your individual business requirements.
End-to-End Development – From ideation to deployment and maintenance, we do it all.
Affordable Pricing – Solutions at affordable costs without any compromise on quality.
100% Client Satisfaction – We provide the best service and customer satisfaction.
0 notes
Text
Tips for Hiring the Best Python Developers
Hiring expert Python developers is essential to build scalable, efficient business applications. The process of hiring Python developers involves understanding the project requirements. Python developers need to have a comprehensive understanding of the language’s ecosystem, including its libraries and frameworks, deep knowledge of Python core concepts, tools like MongoDB for database management, and automation to streamline development processes. Evaluating developers based on their technical skills and experience contributes to team dynamics and project goals needed to deliver high-quality, efficient solutions that meet project requirements.
If you want to hire the best Python developers, here are the key elements that can help you:
Explain the requirements in detail:
Define your project, outlining deliverables, goals, and technical specifications.
Core Python Proficiency:
This includes a strong grasp of object-relational mapping, data structures, and the nuances of software development and design.
Solve complex programming challenges:
Proficient Python developers are adept at optimizing code for performance and scalability. They implement efficient algorithms with clean, maintainable code that enhance the functionality and user experience of the applications they develop.
Python frameworks:
Frameworks like Django facilitate rapid Python development by providing reusable modules and patterns, significantly reducing development time and increasing project efficiency.
Python Libraries:
Python's extensive libraries emphasize a developer's expertise in manipulating data and building machine learning models.
Dataset Management:
Hire Python developers proficient in creating a business application that can process and analyze large datasets, providing valuable insights and driving innovation.
Technical Skills:
Evaluate the developers' skills in core Python, frameworks, libraries, front-end development, and version control.
Experience:
Discuss targeted questions to assess technical knowledge, soft skills, and experience in handling complex projects.
Tech Prastish is a Python development service company offering end-to-end Python development services, including designing web crawlers for collecting information, developing feature-rich web apps, building data analytic tools, and much more.
Python’s versatility and broad range of applications make it a top choice for businesses looking to innovate. If you’re ready to find the perfect Python developer, look no further than Tech Prastish. With access to a top-tier talent pool, you’ll be well on your way to success. We can help you connect with developers who fit your specific needs, ensuring your project is in expert hands. Get started today with Tech Prastish and take your development to the next level.
Also Read:
How To Create Custom Sections In Shopify
How to use Laravel Tinker
‘PixelPhoto’: Setup Your Social Platform
Custom Post Type in WordPress Without a Plugin
0 notes
Text
Designing and Implementing Microservices in PHP 8 with Symfony: A Comprehensive Guide
- An introduction to microservices and their advantages in PHP environments. - Core microservices design patterns like API Gateway, Circuit Breaker, and Event Sourcing. - Service discovery techniques in Symfony. - Communication patterns, including synchronous and asynchronous messaging. - Deployment best practices using Docker, Kubernetes, and CI/CD pipelines. - Code snippets and practical examples to illustrate key concepts.
Introduction to Microservices
Microservices are an architectural approach to building software as a collection of small, independent services that communicate over a network (What are Microservices? - GeeksforGeeks). Each service is focused on a specific business capability, unlike monolithic architectures where all functionality resides in one tightly integrated codebase. This separation yields multiple advantages: microservices can be developed, deployed, and scaled independently, improving overall scalability and resilience (Creating Microservices with Symfony: A Guide for Businesses and Professionals - PHP Developer | Symfony | Laravel | Prestashop | Wordpress | ShopWare | Magento | Sylius | Drupal). For example, if one service becomes a bottleneck, you can scale only that service rather than the entire application. Maintenance is also easier since each service has a narrower scope (fewer intertwined dependencies) and teams can update one service without affecting others (Creating Microservices with Symfony: A Guide for Businesses and Professionals - PHP Developer | Symfony | Laravel | Prestashop | Wordpress | ShopWare | Magento | Sylius | Drupal). These benefits have led companies like Amazon, Uber, and Netflix to adopt microservices for faster development and more robust systems (Symfony in microservice architecture - Episode I : Symfony and Golang communication through gRPC - DEV Community). Why PHP and Symfony? PHP, especially with version 8, offers significant performance improvements and strong typing features that make it a viable choice for modern microservices. Symfony, one of the most widely used PHP frameworks, is well-suited for microservice architectures due to its modular design and rich ecosystem (PHP And Microservices: Guide For Advanced Web Architecture). Symfony’s component-based architecture (the “Swiss Army knife” of frameworks) lets you use only what you need for each microservice, avoiding bloat while still providing tools for common needs like routing, dependency injection, and caching (PHP And Microservices: Guide For Advanced Web Architecture). It integrates seamlessly with technologies often used in microservice environments (e.g. Docker, Redis, RabbitMQ), and its API Platform facilitates quickly building RESTful or GraphQL APIs (Creating Microservices with Symfony: A Guide for Businesses and Professionals - PHP Developer | Symfony | Laravel | Prestashop | Wordpress | ShopWare | Magento | Sylius | Drupal). In short, Symfony provides a robust foundation for building small, self-contained services with PHP, allowing teams to leverage their PHP expertise to build scalable microservices without reinventing the wheel.
Core Design Patterns for Microservices in Symfony
Designing microservices involves certain key patterns to manage the complexity of distributed systems. In this section, we discuss a few core design patterns – API Gateway, Circuit Breaker, and Event Sourcing – and how to implement or leverage them in a Symfony (PHP 8) context. API Gateway An API Gateway is a common pattern in microservices architectures where a single entry point handles all client interactions with the backend services (Symfony Microservices: A Comprehensive Guide to Implementation - Web App Development, Mobile Apps, MVPs for Startups - Digers). Instead of having clients call dozens of services directly (which would require handling multiple URLs, authentication with each service, etc.), the gateway provides one unified API. It can route requests to the appropriate microservice, aggregate responses from multiple services, and enforce cross-cutting concerns like authentication, rate limiting, and caching in one place (Symfony Microservices: A Comprehensive Guide to Implementation - Web App Development, Mobile Apps, MVPs for Startups - Digers) (Symfony Microservices: A Comprehensive Guide to Implementation - Web App Development, Mobile Apps, MVPs for Startups - Digers). This simplifies client interactions and keeps the internal architecture flexible (services can change or be added without impacting external clients, as long as the gateway API remains consistent).
(Pattern: API Gateway / Backends for Frontends) Diagram: Using an API Gateway as a single entry point to route requests (REST calls in this example) to multiple backend microservices. The gateway can also provide client-specific APIs and handle protocol translation. In a Symfony project, you can implement an API Gateway as a dedicated Symfony application that proxies or orchestrates calls to the microservices. For instance, you might create a “Gateway” Symfony service that exposes REST endpoints to clients and internally uses Symfony’s HTTP client to call other microservices’ APIs. Symfony’s HttpClient component (or Guzzle) is useful for making these internal calls. The gateway can combine data from multiple services (for example, a product service and a review service) into one response before returning it to the client. Additionally, you could utilize Symfony’s security features at the gateway to authenticate incoming requests (e.g., validate a JSON Web Token) and only forward authorized requests to the downstream services (Symfony Microservices: A Comprehensive Guide to Implementation - Web App Development, Mobile Apps, MVPs for Startups - Digers). Tip: In many cases, teams use off-the-shelf API gateway solutions (like Kong, Traefik, or NGINX) in front of microservices. These are highly optimized for routing and policy enforcement. However, implementing a simple gateway in Symfony can make sense if you need custom aggregation logic or want to keep everything in PHP. Ensure that the gateway itself is stateless and scalable, as it can become a critical component. Circuit Breaker In a distributed system, failures are inevitable. The Circuit Breaker pattern is a design pattern for building fault-tolerant microservices that prevents cascading failures when a service is unresponsive or slow (What is Circuit Breaker Pattern in Microservices? - GeeksforGeeks). It works analogous to an electrical circuit breaker: if a service call fails repeatedly (e.g., due to the downstream service being down), the circuit breaker “trips” and subsequent calls to that service are short-circuited (i.e., fail immediately or return a fallback response) for a certain cooldown period (Pattern: Circuit Breaker) (What is Circuit Breaker Pattern in Microservices? - GeeksforGeeks). This stops wasting resources waiting on a dead service and gives the failing service time to recover. After the timeout, a few trial requests are allowed (“half-open” state); if they succeed, the circuit closes again, resuming normal operation (What is Circuit Breaker Pattern in Microservices? - GeeksforGeeks).
(What is Circuit Breaker Pattern in Microservices? - GeeksforGeeks) Circuit Breaker states and transitions: when a service call fails beyond a threshold, the breaker goes from Closed (normal operation) to Open (stop calls). After a delay, it enters Half-Open to test the service. Success closes the circuit (resuming calls); failure re-opens it. This pattern prevents one service’s failure from crashing others. In practice, implementing a circuit breaker in PHP/Symfony involves wrapping remote service calls (HTTP requests, database calls, etc.) with logic to monitor failures. For example, if a Symfony service calls another service via an HTTP client, you might use a counter (in memory or in a shared cache like Redis) to track consecutive failures. Once a threshold is exceeded, the client could immediately return an error (or a default fallback response) without attempting the remote call. After a set delay, it can try calling the service again to see if it’s back up. Libraries exist to assist with this in PHP – for instance, there are Symfony bundles and packages that provide circuit breaker functionality out-of-the-box (some use Redis or APCu to track state across instances). Using such a library or bundle can abstract away the boilerplate. If you prefer a custom solution, you can integrate it with Symfony’s event system or middleware. For example, you might create an HttpClient decorator that intercepts requests to certain hostnames and applies circuit-breaking logic. The key is to ensure that when the circuit is open, your code returns promptly, and that you log or monitor these events (so you’re aware of outages). By incorporating a circuit breaker, your Symfony microservice system becomes more resilient – a downstream failure in, say, the “Payment Service” will trigger quick failure responses in the “Order Service” instead of hanging threads and resource exhaustion (Pattern: Circuit Breaker). This keeps the overall system responsive and prevents a chain reaction of failures. Event Sourcing Event Sourcing is a design pattern that persists the state changes of an application as a sequence of events, rather than storing just the latest state (Event Sourcing). In an event-sourced system, every change (e.g., a user placed an order, an order was shipped) is recorded as an immutable event in an event log. The current state of an entity can always be derived by replaying the sequence of events from the beginning up to the present (Event Sourcing). This approach provides a complete audit trail of how the system reached its current state and enables powerful capabilities like time-travel (reconstructing past states) and event-driven integrations. In a Symfony microservices architecture, leveraging event sourcing can ensure data consistency across services and improve traceability (Symfony Microservices: A Comprehensive Guide to Implementation - Web App Development, Mobile Apps, MVPs for Startups - Digers). For example, instead of a traditional update that directly writes to a database, a microservice would emit an event like OrderPlaced or InventoryAdjusted. These events are stored (in a log or message broker), and the service’s own state (and other interested services’ states) are updated by consuming those events. By storing every event, you can rebuild the state of a service at any point in time by replaying the events in order (Symfony Microservices: A Comprehensive Guide to Implementation - Web App Development, Mobile Apps, MVPs for Startups - Digers). This is particularly useful in scenarios that require audit logs or retroactive computations (e.g., if a bug in logic is found, you can fix the code and replay events to correct the state). Symfony doesn’t have event sourcing built into its core, but you can implement it using libraries like Broadway or Prooph (PHP libraries specifically for event sourcing and CQRS) (CQRS and Event Sourcing implementation in PHP | TSH.io). These libraries integrate with Symfony and provide tools to define events, event stores (e.g., storing events in a database or event stream), and projectors (to build read models from events). The Symfony Messenger component can also play a role here by dispatching events to message handlers, which could persist them or propagate them to other services. Additionally, Symfony’s Event Dispatcher component is useful for decoupling internal logic via events – for instance, within a single microservice, domain events (like UserRegistered) can be dispatched and multiple listeners can react to update different parts of the state or send notifications (Symfony Microservices: A Comprehensive Guide to Implementation - Web App Development, Mobile Apps, MVPs for Startups - Digers). Implementing event sourcing requires careful planning of your event schema and handling eventual consistency (since state changes are not immediate but via events). For data that truly benefits from an audit log and history (like financial transactions or orders), event sourcing can greatly enhance consistency and auditability (Symfony Microservices: A Comprehensive Guide to Implementation - Web App Development, Mobile Apps, MVPs for Startups - Digers). However, it adds complexity, so it might not be necessary for every service. In Symfony, start by defining clear event classes and an event store. Ensure each service only acts on events relevant to it. Over time, you'll find you can evolve services by adding new event handlers or new event types without breaking existing ones – a key to maintainable, extensible microservices.
Service Discovery in Symfony
In a microservices architecture with many services running across different hosts or containers, service discovery is how services find each other’s locations (IP addresses/ports) dynamically. Unlike a monolith, where internal calls are just function calls, microservices need to know where to send requests for a given service. The set of active service instances is often changing – instances scale up or down, move, or restart – so hard-coding addresses is not feasible (Service Discovery Explained | Consul | HashiCorp Developer). Service discovery systems address this by keeping a registry of available service instances and allowing lookups by service name. There are two main approaches to service discovery: client-side and server-side. In client-side discovery, each microservice is responsible for querying a service registry (or using DNS) to find the endpoint of another service before calling it. Tools like Consul, etcd, or Eureka maintain a catalog of services that clients can query. In server-side discovery, a load balancer or gateway sits in front of services and routes requests to an available instance – here the clients just call the gateway with a logical name and the gateway/loader does the lookup. In Symfony-based microservices, you can implement service discovery in several ways: - Using Containers & DNS: If you deploy your Symfony services in Docker containers using orchestration tools (like Kubernetes or Docker Compose), you often get basic service discovery via DNS naming. For example, in Docker Compose, each service can be reached by its name as a hostname. In Kubernetes, every service gets a DNS name (e.g., http://product-service.default.svc.cluster.local) that resolves to the service’s IP (Symfony Microservices: A Comprehensive Guide to Implementation - Web App Development, Mobile Apps, MVPs for Startups - Digers). Read the full article
0 notes
Text
Hire Laravel Developers: Your Gateway to Seamless Web Development
In today’s fast-paced digital landscape, businesses across the globe are focusing on developing robust, scalable, and dynamic web applications to maintain a competitive edge. Laravel, a PHP framework celebrated for its elegance and simplicity, has emerged as a top choice among developers and businesses. Whether you’re a startup, SME, or retail giant, partnering with a Laravel development company and hiring a dedicated Laravel developer has become essential for creating tailored solutions that meet unique business needs.
This article delves into why Laravel is the go-to framework for web development and highlights the advantages of collaborating with an Expert Laravel developer to achieve your business objectives effectively.
Why Choose Laravel for Your Web Development Projects?
Laravel stands out as a versatile and user-friendly framework that empowers developers to create innovative and high-performing web applications. Here are some key reasons to opt for Laravel:
1. MVC Architecture Laravel’s Model-View-Controller (MVC) architecture provides a structured approach to application development, separating the business logic, user interface, and data interaction layers. This separation ensures better organization, making the code easier to manage, debug, and scale. Developers can work on individual components independently without affecting the overall application. By improving clarity and maintaining consistency, the MVC pattern allows for efficient team collaboration and streamlined application development, ensuring long-term maintainability.
2. Rich Ecosystem Laravel offers a well-rounded ecosystem of tools that simplify and enhance development workflows. The Eloquent ORM enables smooth and intuitive database interactions, eliminating complex SQL queries. The Blade templating engine allows developers to create dynamic and reusable views efficiently, while Laravel Mix handles asset compilation for CSS, JavaScript, and other front-end needs. This rich toolkit reduces development time and provides a comprehensive foundation for building feature-rich applications.
3. Advanced Security Security is at the forefront of Laravel’s design, making it a reliable choice for safeguarding applications. The framework includes built-in features such as hashed passwords, preventing sensitive data from being stored in plain text. It also protects against common vulnerabilities like SQL injection, Cross-Site Scripting (XSS), and Cross-Site Request Forgery (CSRF). These integrated security measures allow developers to focus on building applications while ensuring robust protection for users and their data.
4. Scalability Laravel’s architecture is designed to handle growing business needs, making it an ideal choice for scalable web applications. Its modular design and support for distributed systems allow developers to integrate caching, load balancing, and database sharding to accommodate increased user traffic. Laravel’s flexibility ensures that as your business expands, the application can seamlessly scale to meet new demands, whether it’s supporting higher traffic loads or adding new functionalities.

Benefits of Hiring a Laravel Developer
To harness the full potential of Laravel, it’s crucial to hire a Laravel developer who can transform your vision into reality. Here’s why partnering with an expert makes a difference:
1. Customized Solutions Hiring a dedicated Laravel developer ensures that your project is tailored to meet your specific business needs. They take the time to understand your goals, industry requirements, and target audience, delivering solutions that align with your vision. This personalized approach ensures that your web application stands out, offering functionality and user experiences designed exclusively for your business success.
2. Cost-Effectiveness Outsourcing Laravel development can be a smart financial decision. It eliminates the need for investing in infrastructure, software tools, or training an in-house team. Instead, you gain access to experienced professionals at a fraction of the cost, helping you allocate resources more efficiently. This cost-effective approach allows businesses to achieve high-quality results without overshooting their budget.
3. Faster Time-to-Market With their expertise and experience, Laravel developers can complete your project more efficiently, meeting tight deadlines without compromising quality. They are familiar with the framework’s tools and best practices, which accelerates the development process. By reducing the time it takes to launch your application, you can stay ahead of competitors and start serving your audience sooner.
4. Maintenance and Support A skilled Laravel developer doesn’t just build your application — they ensure it remains functional and up-to-date over time. From fixing bugs to implementing updates, their ongoing maintenance and support services ensure your application operates smoothly. This long-term commitment ensures stability, reliability, and the ability to adapt to evolving market demands or user feedback.
5. Focus on Core Business By outsourcing Laravel development, you can delegate the technical complexities to experts while focusing on your core business operations. This allows you to channel your energy into areas like strategy, sales, and customer engagement. With the technical side handled professionally, you can drive growth, foster innovation, and enhance your market presence without distractions.
Why Xcrino Business Solutions is the Best Choice
At Xcrino Business Solutions, we take pride in being the best Laravel development company that delivers end-to-end solutions tailored to your needs. Here’s what sets us apart:
1. Experienced Laravel Developers Our team consists of highly skilled and certified Laravel developers with extensive experience in building web applications. They specialize in creating solutions that are not only feature-rich but also scalable and secure. Their deep knowledge of Laravel’s tools and best practices ensures that every project meets the highest standards of quality and performance.
2. Comprehensive Services We offer a broad spectrum of Laravel development services to meet diverse business needs. Whether it’s custom web application development, seamless API integration, or building robust e-commerce platforms, our expertise covers it all. Our end-to-end services ensure that your project is handled efficiently, delivering tailored solutions that align perfectly with your goals.
3. Global Clientele We have had the privilege of working with clients from various industries and regions, including startups, SMBs, and large enterprises. This diverse experience allows us to understand unique business challenges and deliver exceptional results tailored to each client’s specific requirements. Our global reach reflects the trust and satisfaction of businesses worldwide.
4. Agile Development Approach Our development process is rooted in agile methodology, promoting flexibility, transparency, and continuous collaboration. This approach allows us to adapt quickly to changing requirements while maintaining clear communication with our clients. By prioritizing incremental development, we ensure that your application evolves seamlessly to meet your business needs at every stage.

Key Steps to Hiring Laravel Developers
Finding the right Laravel developer can be a game-changer for your business. Here’s how you can ensure a successful hiring process:
1. Define Your Project Requirements Start by outlining your project’s scope, objectives, and technical needs in detail. This clarity ensures you know exactly what you’re looking for in a developer. A well-defined plan helps filter candidates with the right expertise and aligns your goals with their skills, setting the foundation for a successful collaboration.
2. Assess Technical Expertise It’s crucial to evaluate a developer’s technical skills in Laravel and related technologies. Check their proficiency in PHP, MySQL, RESTful APIs, and front-end languages like HTML, CSS, and JavaScript. Their expertise in these areas ensures they can handle your project’s requirements effectively and deliver a high-performing application.
3. Check Portfolio and References Reviewing a developer’s past projects gives insight into their experience and capabilities. A strong portfolio showcases their ability to handle projects similar to yours, while client testimonials and references provide reassurance about their reliability, professionalism, and track record of delivering quality work.
4. Conduct Interviews Interviews are a key step to understanding a developer’s problem-solving approach, communication skills, and ability to work well within your team. Ask scenario-based questions to evaluate how they tackle challenges and determine if their work style aligns with your company culture and project needs.
5. Choose the Right Engagement Model Decide whether you need a dedicated developer for long-term projects or a project-based engagement for shorter tasks. This decision depends on the complexity and timeline of your project. The right engagement model ensures efficient resource allocation and a smoother development process, tailored to your specific needs.
Laravel Use Cases Across Industries
Laravel’s flexibility and robustness make it suitable for diverse industries. Here are some examples:
1. E-Commerce Laravel is a powerful framework for building feature-rich e-commerce platforms. It enables seamless integration of essential functionalities such as inventory management, secure payment gateways, and user-friendly interfaces. With its scalability and flexibility, Laravel allows developers to create platforms that handle high traffic volumes while ensuring a smooth shopping experience for customers, making it ideal for online businesses.
2. Healthcare In the healthcare industry, Laravel provides a reliable foundation for developing HIPAA-compliant applications. It supports features like patient management systems, telemedicine platforms, and secure handling of sensitive medical data. Laravel’s robust security measures and customizable architecture ensure that healthcare applications are not only functional but also safe, meeting the rigorous demands of this critical sector.
3. FinTech Laravel is a trusted choice for building secure and scalable solutions in the FinTech industry. It powers applications for banking, investment platforms, and financial planning tools. With its advanced security features and ability to handle complex processes, Laravel ensures that financial transactions are conducted safely while delivering a seamless user experience for customers.
4. Education Laravel is widely used in the education sector for creating e-learning platforms, virtual classrooms, and content management systems. Its flexibility allows developers to build interactive features like quizzes, progress tracking, and real-time collaboration tools. By enabling innovative and engaging learning experiences, Laravel helps educational institutions and businesses reach a wider audience effectively.
5. Real Estate Laravel is well-suited for real estate businesses, supporting the development of property listing platforms, customer relationship management (CRM) systems, and advanced search functionalities. Its ability to handle dynamic data and integrate with third-party APIs ensures that real estate platforms are both user-friendly and efficient, making it easier for users to find and manage property information.
Why Laravel is Ideal for Startups and SMBs
For startups and SMBs, choosing Laravel can be a strategic decision. Here’s why:
1. Cost-Effective Development Laravel’s open-source framework eliminates licensing fees, making it an economical choice for startups and small businesses. It allows access to a rich ecosystem of tools and resources without additional costs. This affordability ensures you can allocate your budget to other critical areas while still building a high-quality web application.
2. Rapid Development Laravel comes equipped with pre-built modules, libraries, and a streamlined workflow that significantly accelerates development. Startups benefit from faster product launches, which is crucial for gaining a competitive edge. This rapid development capability allows businesses to test ideas, gather user feedback, and iterate quickly, ensuring they stay ahead in dynamic markets.
3. Scalable Infrastructure As your business grows, your application needs to scale alongside it. Laravel’s architecture is designed to handle increasing traffic and complex functionalities with ease. Whether you’re adding new features or expanding your user base, Laravel ensures your web application remains efficient and reliable, making it a future-ready choice for SMBs.
4. Enhanced User Experience Delivering a seamless and engaging user experience is essential for customer retention. Laravel provides developers with tools to create intuitive, user-friendly interfaces that align with your brand’s goals. Its robust architecture ensures fast load times and smooth navigation, creating a positive impression that keeps customers coming back.
Partner with Xcrino Business Solutions for Your Laravel Needs
If you’re ready to take your web development projects to the next level, Xcrino Business Solutions is here to help. Whether you want to hire a Laravel developer or collaborate with a Laravel development company, our team ensures:
Transparent communication
Timely project delivery
Competitive Pricing
Tailored solutions
The decision to hire Laravel developers can redefine your business’s digital presence. Laravel’s unmatched features, combined with the expertise of a dedicated Laravel developer, provide the foundation for innovative and scalable web applications.
Xcrino Business Solutions stands out as a trusted partner for businesses worldwide, offering comprehensive Laravel development services tailored to diverse industries and business needs. Whether you’re a startup, an SME, or a global enterprise, our team of experts is here to bring your vision to life.
Ready to get started? Contact Xcrino Business Solutions today and let us help you achieve your business goals with Laravel.
0 notes
Text
Design Patterns in Laravel
If you want to code like a pro in Laravel, understanding design patterns is a game-changer. Laravel itself is built on various design patterns, and using them correctly can make your applications more scalable, maintainable, and efficient. Here are some essential Laravel design patterns you need to know: Singleton Pattern 📌 Ensures a class has only one instance and provides a global point of…
0 notes
Text
what is laravel

Here are some briefely of What is laravel? Laravel is a free, open-source PHP web framework created by Taylor Otwell in 2011. It is designed to make web development easier by providing elegant syntax, robust tools, and a well-structured framework. Laravel follows the Model-View-Controller (MVC) architectural pattern and is widely used for building modern, scalable, and secure web applications.
Over the years, Laravel has gained immense popularity among developers due to its simplicity, flexibility, and powerful features. It offers built-in authentication, routing, caching, and database management, making it a preferred choice for developers worldwide.
0 notes