#laravel testing tools
Explore tagged Tumblr posts
Text
Unraveling Laravel Test: Best Practices for Efficient Coding
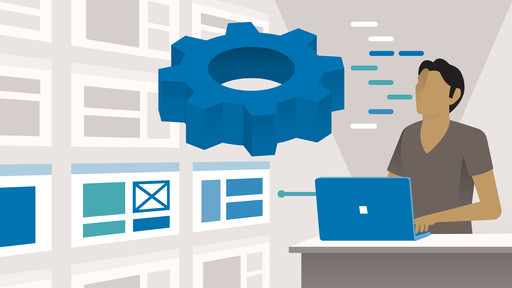
Laravel, a powerful PHP web framework, has gained immense popularity for its elegant syntax, robust features, and developer-friendly environment. One of its key strengths lies in its testing capabilities, allowing developers to ensure the reliability and stability of their applications through automated testing. In this article, we will delve into the world of Laravel testing and explore the best practices for efficient coding.
Understanding Laravel Testing
Laravel provides a comprehensive testing suite that supports both unit and feature testing. Unit tests focus on individual components, ensuring they function correctly in isolation, while feature tests assess the behavior of multiple components working together to achieve a specific functionality.
Testing in Laravel is built on the PHPUnit framework, offering a familiar environment for developers with experience in PHPUnit. Laravel's testing tools extend PHPUnit and provide additional functionalities tailored to the Laravel ecosystem.
Best Practices for Laravel Testing
1. Isolation and Dependency Management:
When writing unit tests, it's crucial to isolate the code under test from external dependencies. Laravel's dependency injection system facilitates the use of interfaces and dependency injection, allowing you to mock external services or dependencies. This promotes testing the specific behavior of the code without relying on external factors.
phpCopy code
// Example of dependency injection in Laravel public function __construct(ExternalServiceInterface $service) { $this->service = $service; }
2. Use Factories for Data Setup:
Laravel provides a convenient way to create model instances for testing purposes using factories. Factories allow you to generate realistic data for your tests, making it easier to simulate various scenarios and edge cases.
phpCopy code
// Example of using a factory in Laravel factory(User::class)->create();
3. Database Transactions for Speed:
Wrapping your tests in a database transaction can significantly improve test speed. Laravel automatically rolls back transactions after each test, ensuring that the database remains in a consistent state. This approach reduces the overhead of migrating and seeding the database for every test.
phpCopy code
// Example of using a database transaction in Laravel use Illuminate\Foundation\Testing\DatabaseTransactions; class ExampleTest extends TestCase { use DatabaseTransactions; }
4. Test Only What Matters:
Focus your tests on critical parts of your application. Prioritize testing business logic, validation, and key functionalities. Avoid testing Laravel's built-in features, as they are already covered by the framework's own tests.
5. Organize Tests Effectively:
Keep your test suite organized by following Laravel's naming conventions. Place your test files in the tests directory and ensure that the test file names correspond to the classes they are testing. Laravel's artisan command can generate test files with the make:test command.
bashCopy code
php artisan make:test ExampleTest
6. Continuous Integration (CI):
Integrate your Laravel tests into a continuous integration system to automate the testing process. This ensures that tests are run consistently on every code change, helping to catch issues early in the development cycle.
7. Documentation and Comments:
Write clear and concise documentation for your tests, explaining the purpose of each test and any specific conditions it addresses. Additionally, use comments within your test code to provide context for future developers.
Conclusion
Laravel's testing capabilities empower developers to build robust and reliable applications. By adhering to best practices such as isolation, effective data setup, database transactions, targeted testing, organization, CI integration, and thorough documentation, developers can ensure the efficiency and effectiveness of their test suites. As you unravel the world of Laravel testing, these best practices will serve as a guide to elevate your coding standards and contribute to the overall success of your Laravel projects.
#laravel test#laravel testing#laravel testing tools#laravel applications#laravel development company#laravel
0 notes
Text
Top Tools for Web Development in 2025
Web development is an ever-evolving field, requiring developers to stay updated with the latest tools, frameworks, and software. These tools not only enhance productivity but also simplify complex development processes. Whether you’re building a small business website or a complex web application, having the right tools in your toolkit can make all the difference. Here’s a rundown of the top…
View On WordPress
#Angular Framework#API Development Tools#Back-End Development Tools#Best Tools for Web Development 2024#Bootstrap for Responsive Design#Django Python Framework#Docker for Deployment#Front-End Development Tools#GitHub for Developers#Laravel PHP Framework#Modern Web Development Tools#Node.js Back-End Framework#Popular Web Development Software#React Development#Tailwind CSS#Testing and Debugging Tools#Vue.js for Web Development#Web Development Frameworks
0 notes
Text
Symfony Clickjacking Prevention Guide
Clickjacking is a deceptive technique where attackers trick users into clicking on hidden elements, potentially leading to unauthorized actions. As a Symfony developer, it's crucial to implement measures to prevent such vulnerabilities.

🔍 Understanding Clickjacking
Clickjacking involves embedding a transparent iframe over a legitimate webpage, deceiving users into interacting with hidden content. This can lead to unauthorized actions, such as changing account settings or initiating transactions.
🛠️ Implementing X-Frame-Options in Symfony
The X-Frame-Options HTTP header is a primary defense against clickjacking. It controls whether a browser should be allowed to render a page in a <frame>, <iframe>, <embed>, or <object> tag.
Method 1: Using an Event Subscriber
Create an event subscriber to add the X-Frame-Options header to all responses:
// src/EventSubscriber/ClickjackingProtectionSubscriber.php namespace App\EventSubscriber; use Symfony\Component\EventDispatcher\EventSubscriberInterface; use Symfony\Component\HttpKernel\Event\ResponseEvent; use Symfony\Component\HttpKernel\KernelEvents; class ClickjackingProtectionSubscriber implements EventSubscriberInterface { public static function getSubscribedEvents() { return [ KernelEvents::RESPONSE => 'onKernelResponse', ]; } public function onKernelResponse(ResponseEvent $event) { $response = $event->getResponse(); $response->headers->set('X-Frame-Options', 'DENY'); } }
This approach ensures that all responses include the X-Frame-Options header, preventing the page from being embedded in frames or iframes.
Method 2: Using NelmioSecurityBundle
The NelmioSecurityBundle provides additional security features for Symfony applications, including clickjacking protection.
Install the bundle:
composer require nelmio/security-bundle
Configure the bundle in config/packages/nelmio_security.yaml:
nelmio_security: clickjacking: paths: '^/.*': DENY
This configuration adds the X-Frame-Options: DENY header to all responses, preventing the site from being embedded in frames or iframes.
🧪 Testing Your Application
To ensure your application is protected against clickjacking, use our Website Vulnerability Scanner. This tool scans your website for common vulnerabilities, including missing or misconfigured X-Frame-Options headers.

Screenshot of the free tools webpage where you can access security assessment tools.
After scanning for a Website Security check, you'll receive a detailed report highlighting any security issues:

An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities.
🔒 Enhancing Security with Content Security Policy (CSP)
While X-Frame-Options is effective, modern browsers support the more flexible Content-Security-Policy (CSP) header, which provides granular control over framing.
Add the following header to your responses:
$response->headers->set('Content-Security-Policy', "frame-ancestors 'none';");
This directive prevents any domain from embedding your content, offering robust protection against clickjacking.
🧰 Additional Security Measures
CSRF Protection: Ensure that all forms include CSRF tokens to prevent cross-site request forgery attacks.
Regular Updates: Keep Symfony and all dependencies up to date to patch known vulnerabilities.
Security Audits: Conduct regular security audits to identify and address potential vulnerabilities.
📢 Explore More on Our Blog
For more insights into securing your Symfony applications, visit our Pentest Testing Blog. We cover a range of topics, including:
Preventing clickjacking in Laravel
Securing API endpoints
Mitigating SQL injection attacks
🛡️ Our Web Application Penetration Testing Services
Looking for a comprehensive security assessment? Our Web Application Penetration Testing Services offer:
Manual Testing: In-depth analysis by security experts.
Affordable Pricing: Services starting at $25/hr.
Detailed Reports: Actionable insights with remediation steps.
Contact us today for a free consultation and enhance your application's security posture.
3 notes
·
View notes
Text
Transforming Businesses with DI Solutions: Innovative IT Expertise
Transform your business with DI Solutions
In the ever-evolving digital landscape, businesses must harness cutting-edge technology to remain competitive. At DI Solutions, we specialize in driving business transformation through advanced IT solutions and expert services. Our dedication to innovation and excellence has empowered numerous clients to achieve their goals and excel in their industries.
Innovative IT Solutions DI Solutions excels in providing tailored IT solutions that meet each client's unique needs. Our services include custom software development, mobile app creation, web development, and UI/UX design. By leveraging the latest technologies, we deliver state-of-the-art solutions that enhance growth and efficiency.
Expert Team of Professionals Our team consists of highly skilled professionals—creative designers, experienced developers, and strategic problem-solvers. We emphasize continuous learning to stay at the forefront of industry trends and technological advancements, ensuring that our clients receive the most effective and innovative solutions.
Global Reach and Impact
With over a decade of experience, DI Solutions has made a significant impact globally, partnering with more than 120 clients across North America, Europe, Asia, and Australia. Our extensive global presence demonstrates our capability to provide exceptional IT services that address diverse business needs.
Client-Centric Approach
At DI Solutions, clients are central to our mission. We take the time to understand their business objectives, challenges, and requirements, enabling us to deliver customized solutions that surpass expectations. Our client-centric approach ensures we provide not just what is needed but what drives success and growth.
Comprehensive IT Services
Our service offerings include:
Custom Software Development: Tailored software solutions for optimal efficiency and performance.
Mobile App Development: Innovative mobile applications for Android and iOS platforms.
Web Development: Expert web development to create responsive and user-friendly websites.
UI/UX Design: Engaging user interfaces that enhance the overall user experience.
Quality Assurance: Rigorous testing to ensure the highest quality standards.
DevOps Services: Streamlined operations through integrated cultural philosophies, practices, and tools.
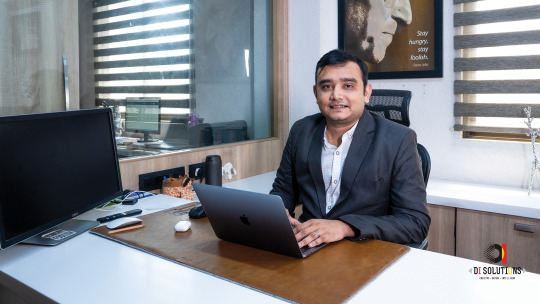
Join Hands with DI Solutions
Partner with DI Solutions to harness the power of innovative IT expertise. Whether you’re a startup aiming to establish a presence or an established business seeking new heights, we have the solutions and expertise to propel you forward.
For more information, visit our website or contact us directly. Let’s embark on a journey of transformation and growth together.
Transform your business with DI Solutions – where innovation meets excellence.
Contact Us Website: https://disolutions.net/ Email: [email protected] , Call: 91-9904566590 , B-301, 307, 406 Apex Commercial Center, Varachha Road, Nr. Yash Plaza, Surat, Gujarat,India-395006.
youtube
#disolutions #DI Solutions #Hire Angular.js Developers #Hire React.js Developers #Hire Vue.js Developers #Hire UI/UX Developers #Hire .NET Developers #Hire Node.js Developers #Hire Laravel/PHP Developers #Hire Android Developers #Hire IOS Developers #Hire Ionic Developers #Hire React Native Developers #Hire Full Stack Developers #Hire MERN Stack Developers #Hire MEAN Stack Developers #Mobile App Development #Web Development #UI/UX Design #Quality Assurance #DevOps Services
2 notes
·
View notes
Text
Comparing Laravel And WordPress: Which Platform Reigns Supreme For Your Projects? - Sohojware
Choosing the right platform for your web project can be a daunting task. Two popular options, Laravel and WordPress, cater to distinct needs and offer unique advantages. This in-depth comparison by Sohojware, a leading web development company, will help you decipher which platform reigns supreme for your specific project requirements.
Understanding Laravel
Laravel is a powerful, open-source PHP web framework designed for the rapid development of complex web applications. It enforces a clean and modular architecture, promoting code reusability and maintainability. Laravel offers a rich ecosystem of pre-built functionalities and tools, enabling developers to streamline the development process.
Here's what makes Laravel stand out:
MVC Architecture: Laravel adheres to the Model-View-Controller (MVC) architectural pattern, fostering a well-organized and scalable project structure.
Object-Oriented Programming: By leveraging object-oriented programming (OOP) principles, Laravel promotes code clarity and maintainability.
Built-in Features: Laravel boasts a plethora of built-in features like authentication, authorization, caching, routing, and more, expediting the development process.
Artisan CLI: Artisan, Laravel's powerful command-line interface (CLI), streamlines repetitive tasks like code generation, database migrations, and unit testing.
Security: Laravel prioritizes security by incorporating features like CSRF protection and secure password hashing, safeguarding your web applications.
However, Laravel's complexity might pose a challenge for beginners due to its steeper learning curve compared to WordPress.
Understanding WordPress
WordPress is a free and open-source content management system (CMS) dominating the web. It empowers users with a user-friendly interface and a vast library of plugins and themes, making it ideal for creating websites and blogs without extensive coding knowledge.
Here's why WordPress is a popular choice:
Ease of Use: WordPress boasts an intuitive interface, allowing users to create and manage content effortlessly, even with minimal technical expertise.
Flexibility: A vast repository of themes and plugins extends WordPress's functionality, enabling customization to suit diverse website needs.
SEO Friendliness: WordPress is inherently SEO-friendly, incorporating features that enhance your website's ranking.
Large Community: WordPress enjoys a massive and active community, providing abundant resources, tutorials, and support.
While user-friendly, WordPress might struggle to handle complex functionalities or highly customized web applications.
Choosing Between Laravel and WordPress
The optimal platform hinges on your project's specific requirements. Here's a breakdown to guide your decision:
Laravel is Ideal For:
Complex web applications require a high degree of customization.
Projects demanding powerful security features.
Applications with a large user base or intricate data structures.
Websites require a high level of performance and scalability.
WordPress is Ideal For:
Simple websites and blogs.
Projects with a primary focus on content management.
E-commerce stores with basic product management needs (using WooCommerce plugin).
Websites requiring frequent content updates by non-technical users.
Sohojware, a well-versed web development company in the USA, can assist you in making an informed decision. Our team of Laravel and WordPress experts will assess your project's needs and recommend the most suitable platform to ensure your web project's success.
In conclusion, both Laravel and WordPress are powerful platforms, each catering to distinct project needs. By understanding their strengths and limitations, you can make an informed decision that empowers your web project's success. Sohojware, a leading web development company in the USA, possesses the expertise to guide you through the selection process and deliver exceptional results, regardless of the platform you choose. Let's leverage our experience to bring your web vision to life.
FAQs about Laravel and WordPress Development by Sohojware
1. Which platform is more cost-effective, Laravel or WordPress?
While WordPress itself is free, ongoing maintenance and customization might require development expertise. Laravel projects typically involve developer costs, but these can be offset by the long-term benefits of a custom-built, scalable application. Sohojware can provide cost-effective solutions for both Laravel and WordPress development.
2. Does Sohojware offer support after project completion?
Sohojware offers comprehensive post-development support for both Laravel and WordPress projects. Our maintenance and support plans ensure your website's continued functionality, security, and performance.
3. Can I migrate my existing website from one platform to another?
Website migration is feasible, but the complexity depends on the website's size and architecture. Sohojware's experienced developers can assess the migration feasibility and execute the process seamlessly.
4. How can Sohojware help me with Laravel or WordPress development?
Sohojware offers a comprehensive range of Laravel and WordPress development services, encompassing custom development, theme and plugin creation, integration with third-party applications, and ongoing maintenance.
5. Where can I find more information about Sohojware's Laravel and WordPress development services?
You can find more information about Sohojware's Laravel and WordPress development services by visiting our website at https://sohojware.com/ or contacting our sales team directly. We'd happily discuss your project requirements and recommend the most suitable platform to achieve your goals.
3 notes
·
View notes
Text
Exploring Essential Laravel Development Tools for Building Powerful Web Applications
Laravel has emerged as one of the most popular PHP frameworks, providing builders a sturdy and green platform for building net packages. Central to the fulfillment of Laravel tasks are the development tools that streamline the improvement process, decorate productiveness, and make certain code quality. In this article, we will delve into the best Laravel development tools that each developer should be acquainted with.
1 Composer: Composer is a dependency manager for PHP that allows you to declare the libraries your project relies upon on and manages them for you. Laravel itself relies closely on Composer for package deal management, making it an essential device for Laravel builders. With Composer, you may without problems upload, eliminate, or update applications, making sure that your Laravel project stays up-to-date with the present day dependencies.
2 Artisan: Artisan is the command-line interface blanketed with Laravel, presenting various helpful instructions for scaffolding, handling migrations, producing controllers, models, and plenty extra. Laravel builders leverage Artisan to automate repetitive tasks and streamline improvement workflows, thereby growing efficiency and productiveness.
3 Laravel Debugbar: Debugging is an crucial component of software program development, and Laravel Debugbar simplifies the debugging procedure by using supplying exact insights into the application's overall performance, queries, views, and greater. It's a accessible device for identifying and resolving problems all through improvement, making sure the clean functioning of your Laravel application.
4 Laravel Telescope: Similar to Laravel Debugbar, Laravel Telescope is a debugging assistant for Laravel programs, presenting actual-time insights into requests, exceptions, database queries, and greater. With its intuitive dashboard, developers can monitor the software's behavior, pick out performance bottlenecks, and optimize hence.
5 Laravel Mix: Laravel Mix offers a fluent API for outlining webpack build steps on your Laravel application. It simplifies asset compilation and preprocessing duties together with compiling SASS or LESS documents, concatenating and minifying JavaScript documents, and dealing with versioning. Laravel Mix significantly streamlines the frontend improvement procedure, permitting builders to attention on building notable consumer reviews.
6 Laravel Horizon: Laravel Horizon is a dashboard and configuration system for Laravel's Redis queue, imparting insights into process throughput, runtime metrics, and more. It enables builders to monitor and control queued jobs efficiently, ensuring most beneficial performance and scalability for Laravel programs that leverage history processing.
7 Laravel Envoyer: Laravel Envoyer is a deployment tool designed specifically for Laravel packages, facilitating seamless deployment workflows with 0 downtime. It automates the deployment process, from pushing code adjustments to more than one servers to executing deployment scripts, thereby minimizing the chance of errors and ensuring smooth deployments.
8 Laravel Dusk: Laravel Dusk is an cease-to-give up browser testing tool for Laravel applications, built on pinnacle of the ChromeDriver and WebDriverIO. It lets in builders to put in writing expressive and dependable browser assessments, making sure that critical user interactions and workflows function as expected across exceptional browsers and environments.
9 Laravel Valet: Laravel Valet gives a light-weight improvement surroundings for Laravel applications on macOS, offering seamless integration with equipment like MySQL, NGINX, and PHP. It simplifies the setup process, permitting developers to consciousness on writing code instead of configuring their development environment.
In end, mastering the vital Laravel development tools noted above is important for building robust, green, and scalable internet packages with Laravel. Whether it's handling dependencies, debugging troubles, optimizing overall performance, or streamlining deployment workflows, those equipment empower Laravel developers to supply outstanding answers that meet the demands of current internet development. Embracing these gear will certainly increase your Laravel improvement enjoy and accelerate your journey toward turning into a talented Laravel developer.
3 notes
·
View notes
Text
Top 10 Laravel Development Companies in the USA in 2024
Laravel is a widely-used open-source PHP web framework designed for creating web applications using the model-view-controller (MVC) architectural pattern. It offers developers a structured and expressive syntax, as well as a variety of built-in features and tools to enhance the efficiency and enjoyment of the development process.
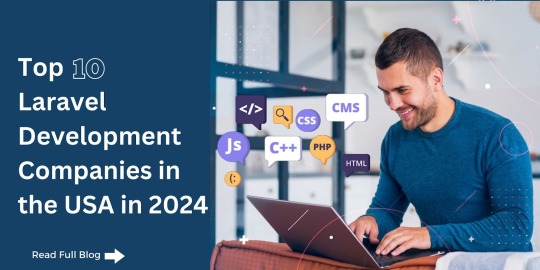
Key components of Laravel include:
1. Eloquent ORM (Object-Relational Mapping): Laravel simplifies database interactions by enabling developers to work with database records as objects through a powerful ORM.
2. Routing: Laravel provides a straightforward and expressive method for defining application routes, simplifying the handling of incoming HTTP requests.
3. Middleware: This feature allows for the filtering of HTTP requests entering the application, making it useful for tasks like authentication, logging, and CSRF protection.
4. Artisan CLI (Command Line Interface): Laravel comes with Artisan, a robust command-line tool that offers commands for tasks such as database migrations, seeding, and generating boilerplate code.
5. Database Migrations and Seeding: Laravel's migration system enables version control of the database schema and easy sharing of changes across the team. Seeding allows for populating the database with test data.
6. Queue Management: Laravel's queue system permits deferred or background processing of tasks, which can enhance application performance and responsiveness.
7. Task Scheduling: Laravel provides a convenient way to define scheduled tasks within the application.
What are the reasons to opt for Laravel Web Development?
Laravel makes web development easier, developers more productive, and web applications more secure and scalable, making it one of the most important frameworks in web development.
There are multiple compelling reasons to choose Laravel for web development:
1. Clean and Organized Code: Laravel provides a sleek and expressive syntax, making writing and maintaining code simple. Its well-structured architecture follows the MVC pattern, enhancing code readability and maintainability.
2. Extensive Feature Set: Laravel comes with a wide range of built-in features and tools, including authentication, routing, caching, and session management.
3. Rapid Development: With built-in templates, ORM (Object-Relational Mapping), and powerful CLI (Command Line Interface) tools, Laravel empowers developers to build web applications quickly and efficiently.
4. Robust Security Measures: Laravel incorporates various security features such as encryption, CSRF (Cross-Site Request Forgery) protection, authentication, and authorization mechanisms.
5. Thriving Community and Ecosystem: Laravel boasts a large and active community of developers who provide extensive documentation, tutorials, and forums for support.
6. Database Management: Laravel's migration system allows developers to manage database schemas effortlessly, enabling version control and easy sharing of database changes across teams. Seeders facilitate the seeding of databases with test data, streamlining the testing and development process.
7. Comprehensive Testing Support: Laravel offers robust testing support, including integration with PHPUnit for writing unit and feature tests. It ensures that applications are thoroughly tested and reliable, reducing the risk of bugs and issues in production.
8. Scalability and Performance: Laravel provides scalability options such as database sharding, queue management, and caching mechanisms. These features enable applications to handle increased traffic and scale effectively.
Top 10 Laravel Development Companies in the USA in 2024
The Laravel framework is widely utilised by top Laravel development companies. It stands out among other web application development frameworks due to its advanced features and development tools that expedite web development. Therefore, this article aims to provide a list of the top 10 Laravel Development Companies in 2024, assisting you in selecting a suitable Laravel development company in the USA for your project.
IBR Infotech
IBR Infotech excels in providing high-quality Laravel web development services through its team of skilled Laravel developers. Enhance your online visibility with their committed Laravel development team, which is prepared to turn your ideas into reality accurately and effectively. Count on their top-notch services to receive the best as they customise solutions to your business requirements. Being a well-known Laravel Web Development Company IBR infotech is offering the We provide bespoke Laravel solutions to our worldwide customer base in the United States, United Kingdom, Europe, and Australia, ensuring prompt delivery and competitive pricing.
Additional Information-
GoodFirms : 5.0
Avg. hourly rate: $25 — $49 / hr
No. Employee: 10–49
Founded Year : 2014
Verve Systems
Elevate your enterprise with Verve Systems' Laravel development expertise. They craft scalable, user-centric web applications using the powerful Laravel framework. Their solutions enhance consumer experience through intuitive interfaces and ensure security and performance for your business.
Additional Information-
GoodFirms : 5.0
Avg. hourly rate: $25
No. Employee: 50–249
Founded Year : 2009
KrishaWeb
KrishaWeb is a world-class Laravel Development company that offers tailor-made web solutions to our clients. Whether you are stuck up with a website concept or want an AI-integrated application or a fully-fledged enterprise Laravel application, they can help you.
Additional Information-
GoodFirms : 5.0
Avg. hourly rate: $50 - $99/hr
No. Employee: 50 - 249
Founded Year : 2008
Bacancy
Bacancy is a top-rated Laravel Development Company in India, USA, Canada, and Australia. They follow Agile SDLC methodology to build enterprise-grade solutions using the Laravel framework. They use Ajax-enabled widgets, model view controller patterns, and built-in tools to create robust, reliable, and scalable web solutions
Additional Information-
GoodFirms : 4.8
Avg. hourly rate: $25 - $49/hr
No. Employee: 250 - 999
Founded Year : 2011
Elsner
Elsner Technologies is a Laravel development company that has gained a high level of expertise in Laravel, one of the most popular PHP-based frameworks available in the market today. With the help of their Laravel Web Development services, you can expect both professional and highly imaginative web and mobile applications.
Additional Information-
GoodFirms : 5
Avg. hourly rate: < $25/hr
No. Employee: 250 - 999
Founded Year : 2006
Logicspice
Logicspice stands as an expert and professional Laravel web development service provider, catering to enterprises of diverse scales and industries. Leveraging the prowess of Laravel, an open-source PHP framework renowned for its ability to expedite the creation of secure, scalable, and feature-rich web applications.
Additional Information-
GoodFirms : 5
Avg. hourly rate: < $25/hr
No. Employee: 50 - 249
Founded Year : 2006
Sapphire Software Solutions
Sapphire Software Solutions, a leading Laravel development company in the USA, specialises in customised Laravel development, enterprise solutions,.With a reputation for excellence, they deliver top-notch services tailored to meet your unique business needs.
Additional Information-
GoodFirms : 5
Avg. hourly rate: NA
No. Employee: 50 - 249
Founded Year : 2002
iGex Solutions
iGex Solutions offers the World’s Best Laravel Development Services with 14+ years of Industry Experience. They have 10+ Laravel Developer Experts. 100+ Elite Happy Clients from there Services. 100% Client Satisfaction Services with Affordable Laravel Development Cost.
Additional Information-
GoodFirms : 4.7
Avg. hourly rate: < $25/hr
No. Employee: 10 - 49
Founded Year : 2009
Hidden Brains
Hidden Brains is a leading Laravel web development company, building high-performance Laravel applications using the advantage of Laravel's framework features. As a reputed Laravel application development company, they believe your web application should accomplish the goals and can stay ahead of the rest.
Additional Information-
GoodFirms : 4.9
Avg. hourly rate: < $25/hr
No. Employee: 250 - 999
Founded Year : 2003
Matellio
At Matellio, They offer a wide range of custom Laravel web development services to meet the unique needs of their global clientele. There expert Laravel developers have extensive experience creating robust, reliable, and feature-rich applications
Additional Information-
GoodFirms : 4.8
Avg. hourly rate: $50 - $99/hr
No. Employee: 50 - 249
Founded Year : 2014
What advantages does Laravel offer for your web application development?
Laravel, a popular PHP framework, offers several advantages for web application development:
Elegant Syntax
Modular Packaging
MVC Architecture Support
Database Migration System
Blade Templating Engine
Authentication and Authorization
Artisan Console
Testing Support
Community and Documentation
Conclusion:
I hope you found the information provided in the article to be enlightening and that it offered valuable insights into the top Laravel development companies.
These reputable Laravel development companies have a proven track record of creating customised solutions for various sectors, meeting client requirements with precision.
Over time, these highlighted Laravel developers for hire have completed numerous projects with success and are well-equipped to help advance your business.
Before finalising your choice of a Laravel web development partner, it is essential to request a detailed cost estimate and carefully examine their portfolio of past work.
#Laravel Development Companies#Laravel Development Companies in USA#Laravel Development Company#Laravel Web Development Companies#Laravel Web Development Services
2 notes
·
View notes
Text
Laravel Programming: A Comprehensive Guide
Table of Contents
Introduction to Laravel
Why Use Laravel?
Installing Laravel
Laravel Folder Structure
Routing and Controllers
Blade Templating Engine
Laravel Models and Eloquent ORM
Migrations and Database Seeding
Request Lifecycle and Middleware
Form Handling and Validation
Authentication and Authorization
Laravel Artisan CLI
RESTful API Development in Laravel
Testing in Laravel
Deployment and Performance Optimization
Laravel Ecosystem and Tools
Conclusion
1. Introduction to Laravel
Laravel is an open-source PHP framework built by Taylor Otwell. It follows the MVC (Model-View-Controller) architectural pattern, which promotes a clean separation between business logic, UI, and data.
Laravel aims to make development faster and easier by providing powerful tools such as:
Routing
Middleware
Authentication
Blade templating
ORM (Eloquent)
Queues
Artisan command-line tool
Laravel is currently one of the most popular PHP frameworks and powers thousands of web applications globally.
2. Why Use Laravel?
Benefits of Laravel:
Clean and Elegant Syntax: Laravel simplifies complex tasks.
MVC Architecture: Ensures separation of concerns.
Eloquent ORM: Elegant database abstraction layer.
Blade Templating: Lightweight yet powerful templating engine.
Built-in Authentication & Authorization: Secure and easy to implement.
Community and Ecosystem: Laravel has a rich ecosystem like Nova, Horizon, Forge, Envoyer, etc.
Testing Ready: PHPUnit integration for test-driven development (TDD).
0 notes
Text
How to Protect Your Laravel App from JWT Attacks: A Complete Guide
Introduction: Understanding JWT Attacks in Laravel
JSON Web Tokens (JWT) have become a popular method for securely transmitting information between parties. However, like any other security feature, they are vulnerable to specific attacks if not properly implemented. Laravel, a powerful PHP framework, is widely used for building secure applications, but developers must ensure their JWT implementation is robust to avoid security breaches.

In this blog post, we will explore common JWT attacks in Laravel and how to protect your application from these vulnerabilities. We'll also demonstrate how you can use our Website Vulnerability Scanner to assess your application for potential vulnerabilities.
Common JWT Attacks in Laravel
JWT is widely used for authentication purposes, but several attacks can compromise its integrity. Some of the most common JWT attacks include:
JWT Signature Forgery: Attackers can forge JWT tokens by modifying the payload and signing them with weak or compromised secret keys.
JWT Token Brute-Force: Attackers can attempt to brute-force the secret key used to sign the JWT tokens.
JWT Token Replay: Attackers can capture and replay JWT tokens to gain unauthorized access to protected resources.
JWT Weak Algorithms: Using weak signing algorithms, such as HS256, can make it easier for attackers to manipulate the tokens.
Mitigating JWT Attacks in Laravel
1. Use Strong Signing Algorithms
Ensure that you use strong signing algorithms like RS256 or ES256 instead of weak algorithms like HS256. Laravel's jwt-auth package allows you to configure the algorithm used to sign JWT tokens.
Example:
// config/jwt.php 'algorithms' => [ 'RS256' => \Tymon\JWTAuth\Providers\JWT\Provider::class, ],
This configuration will ensure that the JWT is signed using the RSA algorithm, which is more secure than the default HS256 algorithm.
2. Implement Token Expiry and Refresh
A common issue with JWT tokens is that they often lack expiration. Ensure that your JWT tokens have an expiry time to reduce the impact of token theft.
Example:
// config/jwt.php 'ttl' => 3600, // Set token expiry time to 1 hour
In addition to setting expiry times, implement a refresh token mechanism to allow users to obtain a new JWT when their current token expires.
3. Validate Tokens Properly
Proper token validation is essential to ensure that JWT tokens are authentic and have not been tampered with. Use Laravel’s built-in functions to validate the JWT and ensure it is not expired.
Example:
use Tymon\JWTAuth\Facades\JWTAuth; public function authenticate(Request $request) { try { // Validate JWT token JWTAuth::parseToken()->authenticate(); } catch (\Tymon\JWTAuth\Exceptions\JWTException $e) { return response()->json(['error' => 'Token is invalid or expired'], 401); } }
This code will catch any JWT exceptions and return an appropriate error message to the user if the token is invalid or expired.
4. Secure JWT Storage
Always store JWT tokens in secure locations, such as in HTTP-only cookies or secure local storage. This minimizes the risk of token theft via XSS attacks.
Example (using HTTP-only cookies):
// Setting JWT token in HTTP-only cookie $response->cookie('token', $token, $expirationTime, '/', null, true, true);
Testing Your JWT Security with Our Free Website Security Checker
Ensuring that your Laravel application is free from vulnerabilities requires ongoing testing. Our free Website Security Scanner helps identify common vulnerabilities, including JWT-related issues, in your website or application.
To check your site for JWT-related vulnerabilities, simply visit our tool and input your URL. The tool will scan for issues like weak algorithms, insecure token storage, and expired tokens.

Screenshot of the free tools webpage where you can access security assessment tools.
Example of a Vulnerability Assessment Report
Once the scan is completed, you will receive a detailed vulnerability assessment report to check Website Vulnerability. Here's an example of what the report might look like after checking for JWT security vulnerabilities.

An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities.
By addressing these vulnerabilities, you can significantly reduce the risk of JWT-related attacks in your Laravel application.
Conclusion: Securing Your Laravel Application from JWT Attacks
Securing JWT tokens in your Laravel application is essential to protect user data and maintain the integrity of your authentication system. By following the steps outlined in this post, including using strong algorithms, implementing token expiry, and validating tokens properly, you can safeguard your app from common JWT attacks.
Additionally, make sure to regularly test your application for vulnerabilities using tools like our Website Security Checker. It’s a proactive approach that ensures your Laravel application remains secure against JWT attacks.
For more security tips and detailed guides, visit our Pentest Testing Corp.
2 notes
·
View notes
Text
Custom Web Development vs. Website Builders: What’s Right for Your Business?
In a digital-first world, your website is the face of your business. It’s where your brand makes its first impression, engages visitors, and converts them into customers. When building or upgrading a website, businesses often find themselves at a crossroads: Should you choose a website builder for a quick launch or invest in custom web development for long-term growth? Understanding the difference is crucial—and consulting a trusted Web Development Company can help you decide what’s truly right for your business.
Let’s explore both options in detail—along with their pros, cons, and ideal use cases.
Understanding Website Builders
Website builders like Wix, Squarespace, and Shopify offer a do-it-yourself approach. They provide templates, drag-and-drop features, and built-in hosting—all designed to make website creation easy, especially for non-tech-savvy users. These platforms are ideal for individuals or small businesses looking to establish an online presence quickly and affordably.
The biggest advantages of website builders are their speed and simplicity. You don’t need coding knowledge, and most platforms offer ready-to-use templates and essential tools. For those on a tight budget or short timeline, they’re a practical starting point.
However, website builders come with limitations. Customization options are often restricted, meaning you might not be able to create a unique design or integrate complex features. Additionally, scalability is an issue—most website builders aren’t built to support large, growing businesses with high traffic or advanced functionality needs. SEO control is also basic, which can hurt your visibility in search engine rankings over time.
Exploring Custom Web Development
Custom web development involves building your site from scratch or with flexible frameworks like WordPress, React, or Laravel. It is executed by skilled developers who tailor every element—from the design to the backend functionality—to suit your brand, goals, and customer needs.
The biggest benefit of custom development is full control. Your website can look and function exactly the way you want, with no compromises on design, features, or integrations. Need a multi-language portal, real-time inventory management, or custom payment flow? Custom development makes it possible.
Another major advantage is scalability. As your business grows, your website can evolve with it—handling increased traffic, advanced features, and third-party integrations. Security is also stronger, as custom sites allow for robust protection against common threats. Additionally, SEO capabilities are far superior, giving you full control over metadata, site speed, mobile responsiveness, and content structure—all critical for ranking on Google.
The downsides? Custom web development takes longer and costs more upfront. It also requires ongoing technical support for maintenance, updates, and security patches. However, for businesses serious about growth and digital presence, the long-term returns often outweigh the initial investment.
When to Use a Website Builder
Website builders are ideal for small-scale projects. If you’re launching a personal blog, a basic business website, or an online portfolio, a builder offers everything you need. They’re also a good choice for testing an idea or creating a temporary promotional site with limited functionality.
Startups with very tight budgets and short timelines may also benefit from a builder in the early stages. But as the business grows, most brands eventually outgrow these platforms and shift to custom-built solutions for greater flexibility and performance.
When to Choose Custom Development
If your business depends on a strong digital presence, a custom-built website is the better long-term choice. Custom development is particularly recommended for eCommerce businesses, SaaS platforms, large corporations, or any company that requires personalized user experiences and backend integrations.
It’s also the right option if you need your website to reflect your brand identity with precision. Templates can only go so far—custom design ensures that your visuals, functionality, and user flow are aligned with your brand strategy.
Moreover, if you need advanced features like user accounts, databases, API integrations, or dynamic content generation, a website builder will fall short. Custom development provides the technical flexibility required to implement such features efficiently.
Final Thoughts
Choosing between a website builder and custom development isn’t just about budget—it’s about vision, goals, and how much control you want over your digital presence. Website builders are great for quick and simple projects, but if you’re looking to build a robust, scalable, and high-performing site that grows with your business, custom development is the way forward.
To make the right decision for your business, consider consulting a professional Web Development Company. They can assess your needs, recommend the best approach, and create a digital solution that supports your growth—whether you’re just getting started or ready to scale. In the end, your website should work as hard as you do—and with the right development path, it can become your most valuable digital asset.
0 notes
Text
What Is Custom Software Development?
Custom software development involves designing and building software applications specifically tailored to a business’s unique processes, workflows, or goals. Unlike off-the-shelf software, custom-built solutions are flexible, scalable, and optimized for exactly what your company needs.
We specialize in building customized software tailored to your unique business requirements. Whether you need a custom web application, CRM system, or backend API, our development team uses modern technologies like PHP, Laravel, React.js, and MySQL to deliver scalable, secure, and efficient solutions. From UI/UX design to database architecture, every feature is designed with your workflows in mind. We also follow best practices in version control (Git), automated testing, and CI/CD pipelines, ensuring high-quality and maintainable code.
✅ Why Choose Custom Software?
Perfect Fit – It does exactly what your business needs, nothing more or less
Flexible – You can add or change features as your business grows
Works with Other Tools – Can connect to your website, payment system, or any third-party app using APIs
Saves Time – You can automate tasks like sending emails, creating reports, or tracking sales

#CustomSoftware#SoftwareSolutions#BusinessSoftware#SmallBusinessTech#CustomApp#TechForBusiness#BusinessGrowth
0 notes
Text
What Makes a PHP Development Company Stand Out in a Competitive Market?

In today’s rapidly evolving tech landscape, selecting the right PHP development company can make or break your web project. With businesses moving online at lightning speed, the demand for robust, scalable, and cost-effective web solutions is higher than ever. PHP, being one of the oldest and most widely used server-side scripting languages, continues to dominate the web development space.
But not all development firms are created equal. What really makes a PHP development company stand out in this competitive and saturated market?
Experience Matters in a PHP-Driven World
The first distinguishing factor is experience. A seasoned PHP development company will have in-depth expertise in building websites and applications across a wide variety of domains—from eCommerce to healthcare, and from finance to social media.
Such companies have seen PHP evolve and know how to use its full potential. They can create scalable solutions, write clean and optimized code, and integrate third-party APIs efficiently. Their developers are also well-versed in Why PHP Development: Is Still Worth it in 2025?, understanding how PHP continues to adapt to the needs of modern businesses.
Proven Portfolio and Industry Recognition
A trustworthy PHP development company showcases its capabilities through a solid portfolio. Whether it’s custom content management systems (CMS), enterprise-level web apps, or dynamic eCommerce platforms, you should expect to see real-world applications that reflect innovation and functionality.
It also helps to see if they’re featured in industry listings or review platforms as one of the Top PHP Web Development Companies. Recognition by platforms like Clutch, GoodFirms, or TechReviewer adds a layer of credibility and assures quality service.
Leveraging the Latest PHP Tools and Frameworks
Modern PHP is not just about procedural scripting. The best PHP development company uses powerful tools and frameworks like Laravel, Symfony, Zend, and CodeIgniter to accelerate development, improve maintainability, and ensure security.
They also work with modern version control systems like Git, CI/CD pipelines for automated testing, and use Docker or Kubernetes for deployment. Keeping up with the most Powerful PHP Development Tools ensures your app is built on a solid and future-ready tech foundation.
Moreover, a proficient company always ensures backward compatibility with older PHP versions while fully embracing the latest upgrades.
Technical Versatility and Language Comparison
In a world of multiple tech stacks, a standout PHP development company doesn’t just know PHP—they understand when and why to use it. Often, clients are confused about whether to go with PHP or an alternative like Python, Node.js, or Ruby on Rails.
This is where expertise in PHP vs Python: Which is Best comes into play. A top-tier company will guide you on the pros and cons of each based on your specific business goals, application complexity, scalability requirements, and future growth potential.
They won’t sell you PHP as the only solution—they’ll position it where it fits best.
Agile Methodology and Transparent Communication
Top PHP development companies follow agile development practices, allowing for continuous integration and feedback throughout the project. Clients are involved at every stage—from requirement gathering to prototyping, development, testing, and deployment.
Weekly sprints, task boards, progress trackers, and sprint reviews ensure that clients are always in the loop. Agile also allows for flexibility in scope and timely adjustments—critical for evolving digital needs.
They also provide clients with a Guide to Web Application Development so that even non-technical stakeholders can stay informed and make strategic decisions confidently.
Strong Support and Post-Launch Maintenance
One of the most overlooked yet crucial qualities of a leading PHP development company is its dedication to post-launch support and maintenance. Technology is dynamic—updates, patches, and new feature rollouts are inevitable.
Reliable companies offer continuous monitoring, performance optimization, bug fixing, and security updates. They don't disappear after project delivery. Instead, they stay engaged to help your application adapt and scale with changing user behavior and market trends.
Competitive Pricing Without Compromising Quality
While cost is often a key concern, a successful PHP development company strikes the right balance between affordability and value. Their pricing model is transparent, and they provide clear deliverables at each milestone.
These companies may offer multiple engagement models—fixed-cost, hourly, or dedicated resource hiring—based on project scope and client preference. They offer clear ROI through productivity, reliability, and rapid time-to-market.
When you pay for seasoned expertise, optimized development workflows, and comprehensive QA testing, the long-term savings far outweigh the initial investment.
Final Thoughts: What Really Sets a PHP Development Company Apart?
To sum it up, a great PHP development company is defined by its experience, technical capability, transparency, client-first approach, and adaptability. In 2025, with tech trends changing faster than ever, businesses need partners that don’t just write code—but craft complete digital experiences.
Whether you’re building a small business website or a scalable enterprise web application, choosing the right partner ensures stability, speed, and success. With their mastery of Powerful PHP Development Tools, understanding of modern tech comparisons like PHP vs Python, and guidance through the Web Application Development lifecycle, these companies help you unlock the full power of PHP.
So, as the digital race accelerates, partnering with a forward-thinking PHP development company could be the smartest move for your business this year.
0 notes
Text
From Concept to Conversion: How a Web Development Company in Bangalore Delivers Business Impact
The digital world is evolving rapidly. Businesses today must have a strong online presence to stay relevant, reach new customers, and drive growth. At the center of this transformation is web development—a key component that turns digital ideas into practical, impactful solutions.
For businesses in India and across the globe, Bangalore has emerged as a leading destination for web development services. Among the top names in the region is WebSenor, a professional web development agency in Bangalore known for delivering real business outcomes through smart, scalable, and customized solutions.
The Bangalore Advantage
Why Bangalore is a Hub for Digital Innovation
Bangalore, often referred to as the “Silicon Valley of India,” offers a unique ecosystem of tech talent, startups, and global IT companies. The city is home to some of the most top web development companies in Bangalore, offering both innovation and reliability.
Whether it's custom web development in Bangalore or enterprise-level software solutions, the local talent pool excels in front-end and back-end development, mobile optimization, and UX design. This ensures high-quality work at a competitive cost.
Local Insight Meets Global Standards
What sets Bangalore apart is its ability to blend local business knowledge with global technical standards. Companies like WebSenor bring this advantage to clients across industries by understanding market-specific challenges and developing tailored digital strategies.
Case in point: A Bangalore-based retailer partnered with WebSenor for a responsive, eCommerce website. The result? A 4x increase in sales within six months—proving how eCommerce website development in Bangalore can truly scale a business.
WebSenor’s Strategic Web Development Approach
At WebSenor, the focus is not just on building websites but on driving business success. Here’s how the process unfolds:
Step 1 – Discovery & Conceptualization
Every successful project begins with deep understanding. WebSenor starts by exploring the client’s goals, audience, and competition.
Stakeholder interviews to gather insights.
Competitor analysis to identify opportunities.
Initial project roadmap and timeline.
This phase sets the foundation for a result-driven approach.
Step 2 – UX/UI Strategy & Wireframing
User experience is critical. WebSenor uses tools like Figma and Adobe XD to create intuitive, attractive interfaces.
Wireframes and mockups based on user behavior.
User personas to guide design decisions.
Focus on custom website design for unique brand identity.
Whether it’s mobile-friendly website development or a complex dashboard, the team ensures every click serves a purpose.
Step 3 – Agile Development Process
WebSenor follows agile methodology to allow flexibility and speed.
Use of modern technologies like React, Angular, Node.js, Laravel.
Regular sprint reviews and updates.
Backend integration with databases and APIs.
This makes them a full-stack web development company in Bangalore capable of handling end-to-end solutions.
Step 4 – SEO, Performance & Security Optimization
Your website isn’t effective if it isn’t visible. WebSenor ensures every site is:
Optimized for search engines (on-page SEO, metadata, schema markup).
Fast-loading and responsive across devices.
Secure, with SSL implementation and data protection practices.
Their team understands the importance of responsive web design in Bangalore’s mobile-first digital landscape.
Step 5 – QA Testing & Iteration
Before going live, every project goes through rigorous testing:
Browser and device compatibility.
Load testing for high traffic.
Bug tracking and resolution.
This guarantees a seamless user experience from day one.
Step 6 – Launch, Training & Maintenance
WebSenor doesn’t stop at delivery. Post-launch support includes:
Deployment on live servers or cloud platforms.
Client training for CMS and content updates.
Ongoing maintenance and performance tracking.
It’s a complete package for businesses that want reliable web development services in Bangalore.
Converting Traffic into Business Impact
Beyond Aesthetics – WebSenor’s Focus on Conversion Rate Optimization (CRO)
A beautiful site is great, but one that converts is even better. WebSenor incorporates CRO best practices from the start:
Strong CTAs placed strategically.
Landing page optimization.
Integration with CRM tools and Google Analytics.
By focusing on conversions, they help businesses increase revenue, not just traffic.
Success Metrics That Matter
WebSenor tracks key performance indicators to measure results:
Bounce rate reduction.
Increased session duration.
Growth in qualified leads.
Sales and customer acquisition.
Real-time dashboards and ROI reports help clients see the tangible benefits of their investment.
Case Studies: Real Business Impact with WebSenor
Case Study 1 – E-Commerce Brand Boost
A mid-sized fashion brand needed a makeover. Their old website was slow, unresponsive, and didn’t convert.
Solution: WebSenor built a custom Shopify-like platform with integrated payment gateways and product filters.
Result: Site speed improved by 60%, and monthly revenue tripled within 6 months.
Case Study 2 – SaaS Platform Transformation
A SaaS startup required scalable infrastructure and improved UI.
Solution: WebSenor delivered a modern web app using Node.js and React, built for scalability.
Result: Customer retention improved by 40%, and the company secured a funding round soon after.
Client Testimonials & Industry Recognition
Clients consistently praise WebSenor’s professionalism and results. With Clutch ratings, verified Google reviews, and a portfolio of over 200 projects, WebSenor has established itself as a professional web development agency in Bangalore.
The WebSenor Edge: Experience, Expertise & Trust
Our Team of Experts
WebSenor’s team includes senior developers, UI/UX designers, digital strategists, and QA specialists. Their combined experience in web development for startups, enterprises, and NGOs helps tailor solutions for every client.
Certifications, Partnerships, and Methodologies
WebSenor is recognized as a:
Google Partner
AWS Cloud Partner
ISO-Certified Company
Their adoption of DevOps practices, CI/CD pipelines, and agile tools enhances reliability and speed.
Transparent Communication & Long-Term Partnerships
Clients enjoy:
Regular updates and open communication.
Dedicated account managers.
Ongoing support and service-level agreements.
This client-first approach is why WebSenor is often regarded as the best web development company in Bangalore.
Why Choose WebSenor as Your Web Development Partner
Here’s what sets WebSenor apart from other affordable web development companies in Bangalore:
Custom development tailored to business needs.
Affordable pricing without compromising quality.
Full-stack expertise across front-end and back-end.
Reliable support and proactive communication.
Whether you want to hire web developers in Bangalore for a short-term project or need a long-term digital partner, WebSenor is ready to help.
Explore our web development services in Bangalore or check out our portfolio to see what we’ve built.
Conclusion
Web development isn’t just about putting up a website—it’s about creating a tool that drives growth, improves customer experience, and delivers measurable results.
WebSenor, a leading web development company in Bangalore, offers a complete journey from concept to conversion. With its skilled team, robust process, and customer-first approach, businesses can confidently build their digital presence and achieve lasting success. If you're ready to take your digital strategy to the next level, contact WebSenor today and see how we can turn your vision into impact.
#WebSenor#WebDevelopmentCompany#WebDevelopmentBangalore#BangaloreTech#WebsiteDesign#CustomWebDevelopment#ResponsiveDesign#EcommerceDevelopment#FullStackDevelopment#TechInBangalore
0 notes
Text
The Role of Professional Web Development in Business Growth – A 2025 Perspective

With the rapidly changing digital landscape, a company without a website is similar to a store without a signboard. Far from being an electronic placeholder, a website serves as your brand's digital identity, marketing tool, and communication channel all in one. With hundreds of millions of users visiting the web on a daily basis, companies need websites that are not only good to look at but also fast, functional, secure, and search engine optimized. This is where expert web development comes in. Here at Instant Website Development, we excel at building websites that are technologically robust, strategically designed, and optimized to convert visitors into long-term customers.
Expert web development is more than coding or loading content onto a domain. It's a holistic process that combines design aesthetics, user experience (UX), backend functionality, and performance optimization. The web user of today wants a frictionless experience, whether on a desktop or on a mobile. Research indicates that a visitor decides about a site in 0.05 seconds, and more than 75% of the consumers judge the credibility of a company based on its website design. This renders it essential for companies to invest in custom-developed, responsive, and easy-to-use websites that address the needs of their audience and remain in line with their company values.
Another important component of web development nowadays is mobile responsiveness. With over 60% of web traffic around the world originating from mobile devices, it is no longer optional that websites be mobile-friendly. A responsive design makes it so that the website adapts and performs flawlessly on every screen size, providing an identical and friendly experience. Additionally, Google deems mobile-friendliness a ranking signal, and websites that rank poorly on mobile can find difficulty surfacing in results. Our development team takes the initiative to test every website across devices, screen widths, and browsers to ensure performance and accessibility.
Apart from design and responsiveness, functionality is the core of web development. No matter if you are developing a simple business website, an eCommerce site, or a sophisticated web application, your platform should work flawlessly and should load in a reasonable time. At Instant Website Development, we utilize the most recent technologies, such as HTML5, CSS3, JavaScript, PHP, Laravel, React, Node.js, and CMS platforms like WordPress and Shopify, to provide feature-rich, scalable, and fast-loading websites. This guarantees that your website not just appears wonderful but also holds up to pressure, either it is receiving a traffic overflow or supporting real-time interactions.
Security is yet another pillar of successful web development. With an increase in cyber attacks, companies cannot take data protection lightly. A professionally designed website includes stringent security measures, such as SSL integration, firewall configuration, data encryption, and periodic software updates. Our staff values secure coding standards and runs vulnerability scans to reduce vulnerabilities and guard business as well as customer information. This is especially important for eCommerce websites, where buyer trust relies on secure payment processing and data management.
Search engine optimization (SEO) is more than content and keywords; it starts with your site's architecture. Clean coding, quick loading time, image optimization, good use of tags, and schema markup all work towards improved search visibility. When we are building a website, we implement technical SEO best practices from the very beginning. These include mobile friendliness, secure HTTPS protocol, crawlable site structure, and structured data enabling search engines to better comprehend your content. An optimization website not only ranks higher but also provides a better overall user experience.
Another essential advantage of hiring professional web developers is customization. Pre-made templates might be easy to use, yet they cannot be flexible and scalable. Instant Website Development offers personalized solutions tailored to your brand and demographic. Regardless of whether you require a corporate website, service landing page, or interactive portfolio, our developers create everything from scratch or redesign existing platforms to provide the exact solution your business requires. Such customization enhances not just looks but also functionality and conversion rate.
A website is not a single project but an ongoing investment. This is why we don't just offer initial development. We have regular maintenance, updates, performance tracking, and scalability solutions to ensure your online presence grows with your business. As technology and consumer patterns change, your website needs to keep up pace to stay competitive. Our strategy makes your website remain relevant, responsive, and result-driven even after years of its launch.
The selection of the right web development partner can make or break your business's online success. Instant Website Development, with a group of experienced developers, innovative designers, and SEO experts, has assisted many startups, SMEs, and businesses in creating digital platforms that exceed their expectations. Our transparent development process is agile and customer-centric, with a commitment to delivering on time and on budget without ever compromising quality.
In summary, professional web development is not a luxury anymore—it's a 2025 digital-first economy necessity. A well-designed, secure, and optimized website is the foundation of your online marketing plan and is essential to establishing brand authority, customer engagement, and conversion. As a reputable web development firm, Instant Website Development is dedicated to building high-performance websites that enable businesses to grow, evolve, and succeed in the digital age.
Visit now: https://instantwebsitedevelopment.in/
0 notes
Text
Top Programming Languages to Learn for Freelancing in India

The gig economy in India is blazing a trail and so is the demand for skilled programmers and developers. Among the biggest plus points for freelancing work is huge flexibility, independence, and money-making potential, which makes many techies go for it as a career option. However, with the endless list of languages available to choose from, which ones should you master to thrive as a freelance developer in India?
Deciding on the language is of paramount importance because at the end of the day, it needs to get you clients, lucrative projects that pay well, and the foundation for your complete freelance career. Here is a list of some of the top programming languages to learn for freelancing in India along with their market demand, types of projects, and earning potential.
Why Freelance Programming is a Smart Career Choice
Let's lay out really fast the benefits of freelance programmer in India before the languages:
Flexibility: Work from any place, on the hours you choose, and with the workload of your preference.
Diverse Projects: Different industries and technologies put your skills to test.
Increased Earning Potential: When most people make the shift toward freelancing, they rapidly find that the rates offered often surpass customary salaries-with growing experience.
Skill Growth: New learning keeps on taking place in terms of new technology and problem-solving.
Autonomy: Your own person and the evolution of your brand.
Top Programming Languages for Freelancing in India:
Python:
Why it's great for freelancing: Python's versatility is its superpower. It's used for web development (Django, Flask), data science, machine learning, AI, scripting, automation, and even basic game development. This wide range of applications means a vast pool of freelance projects. Clients often seek Python developers for data analysis, building custom scripts, or developing backend APIs.
Freelance Project Examples: Data cleaning scripts, AI model integration, web scraping, custom automation tools, backend for web/mobile apps.
JavaScript (with Frameworks like React, Angular, Node.js):
Why it's great for freelancing: JavaScript is indispensable for web development. As the language of the internet, it allows you to build interactive front-end interfaces (React, Angular, Vue.js) and powerful back-end servers (Node.js). Full-stack JavaScript developers are in exceptionally high demand.
Freelance Project Examples: Interactive websites, single-page applications (SPAs), e-commerce platforms, custom web tools, APIs.
PHP (with Frameworks like Laravel, WordPress):
Why it's great for freelancing: While newer languages emerge, PHP continues to power a significant portion of the web, including WordPress – which dominates the CMS market. Knowledge of PHP, especially with frameworks like Laravel or Symfony, opens up a massive market for website development, customization, and maintenance.
Freelance Project Examples: WordPress theme/plugin development, custom CMS solutions, e-commerce site development, existing website maintenance.
Java:
Why it's great for freelancing: Java is a powerhouse for enterprise-level applications, Android mobile app development, and large-scale backend systems. Many established businesses and startups require Java expertise for robust, scalable solutions.
Freelance Project Examples: Android app development, enterprise software development, backend API development, migration projects.
SQL (Structured Query Language):
Why it's great for freelancing: While not a full-fledged programming language for building applications, SQL is the language of databases, and almost every application relies on one. Freelancers proficient in SQL can offer services in database design, optimization, data extraction, and reporting. It often complements other languages.
Freelance Project Examples: Database design and optimization, custom report generation, data migration, data cleaning for analytics projects.
Swift/Kotlin (for Mobile Development):
Why it's great for freelancing: With the explosive growth of smartphone usage, mobile app development remains a goldmine for freelancers. Swift is for iOS (Apple) apps, and Kotlin is primarily for Android. Specializing in one or both can carve out a lucrative niche.
Freelance Project Examples: Custom mobile applications for businesses, utility apps, game development, app maintenance and updates.
How to Choose Your First Freelance Language:
Consider Your Interests: What kind of projects excite you? Web, mobile, data, or something else?
Research Market Demand: Look at popular freelance platforms (Upwork, Fiverr, Freelancer.in) for the types of projects most requested in India.
Start with a Beginner-Friendly Language: Python or JavaScript is an excellent start due to their immense resources and helpful communities.
Focus on a Niche: Instead of trying to learn everything, go extremely deep on one or two languages within a domain (e.g., Python for data science, JavaScript for MERN stack development).
To be a successful freelance programmer in India, technical skills have to be combined with powerful communication, project management, and self-discipline. By mastering either one or all of these top programming languages, you will be set to seize exciting opportunities and project yourself as an independent professional in the ever-evolving digital domain.
Contact us
Location: Bopal & Iskcon-Ambli in Ahmedabad, Gujarat
Call now on +91 9825618292
Visit Our Website: http://tccicomputercoaching.com/
#Freelance Programming#Freelance India#Programming Languages#Coding for Freelancers#Learn to Code#Python#JavaScript#Java#PHP#SQL#Mobile Development#Freelance Developer#TCCI Computer Coaching
0 notes
Text
FoodTiger Nulled Script 3.6.0

Unlock the Power of FoodTiger Nulled Script for Your Food Delivery Business Are you ready to revolutionize your food delivery startup with a robust and feature-rich platform? Look no further than the FoodTiger Nulled Script—a powerful solution designed to streamline operations, support multiple restaurants, and deliver exceptional user experience. This script is perfect for entrepreneurs and developers looking for a fast, reliable, and customizable tool to kick-start their food ordering service without the hefty price tag. What is FoodTiger Nulled Script? The FoodTiger Nulled Script is a premium food delivery software that has been unlocked for free access. Originally developed as a high-end solution for managing food delivery businesses, this nulled version offers you all the premium features—without any restrictions. With its intuitive interface and powerful admin dashboard, you can manage restaurants, orders, and customers with ease. It’s an excellent alternative for startups looking to save money while still accessing top-tier software. Technical Specifications Platform: Laravel PHP Framework Database: MySQL Languages: Fully multilingual with built-in translation support Responsive: 100% mobile-friendly layout Third-Party Integration: Supports Stripe, PayPal, and more Outstanding Features & Benefits Multi-Restaurant System: Perfect for marketplaces—host multiple restaurants under one roof. Real-Time Order Tracking: Let your users track their orders from kitchen to doorstep. Advanced Admin Panel: Manage restaurants, menus, discounts, and delivery zones effortlessly. Modern UI/UX: Sleek and intuitive design that enhances customer satisfaction. Contactless Delivery: Built-in support for safe, contactless food delivery methods. Why Choose FoodTiger Nulled Script? Choosing the FoodTiger gives you access to premium tools without licensing fees. You get the flexibility to customize every part of the system, from UI layouts to backend logic. It’s ideal for freelancers, startups, and developers who want full control over their food delivery platforms. Say goodbye to limitations and hello to a world of possibilities—all without spending a dime. Common Use Cases Launching a multi-vendor food delivery app in your local area Building a white-label solution for restaurant chains Starting a commission-based food ordering marketplace Testing new delivery models and logistics solutions with zero upfront cost How to Install FoodTiger Nulled Script Download the FoodTiger Nulled Script from our website. Upload the files to your server using FTP or cPanel. Set file permissions and create a new MySQL database. Run the installer by accessing your domain in a web browser. Follow the setup wizard and enter your database credentials. Login to the admin panel and start configuring your platform. Frequently Asked Questions (FAQs) Is it legal to use the FoodTiger Nulled Script? While using nulled scripts may violate the original developer’s terms, many users opt for them to test or build proof-of-concept platforms. We encourage using it responsibly and considering licensing options if you plan to scale. Can I customize the FoodTiger Nulled Script? Absolutely. The script is fully open-source and built on Laravel, allowing deep customization of features, design, and functionalities. Is this version secure for live deployments? The FoodTiger Nulled Script includes the same core security features as the licensed version. However, always perform your own audits and use secure hosting practices for best results. Where can I download the FoodTiger Nulled Script? You can download the latest version directly from our website and get started today with no hidden costs or restrictions. Start Building Your Delivery Empire Today Whether you’re a developer building a food delivery app or an entrepreneur aiming to create the next big thing in local delivery services, the FoodTiger is your gateway to fast, affordable, and scalable success. Don’t miss the opportunity to harness this powerful tool for free.
Check out our other valuable downloads like betheme nulled and explore even more resources to enhance your web projects. Need additional themes and scripts? Visit our trusted partner for more tools like betheme nulled to power up your development journey.
0 notes