#python importing modules
Explore tagged Tumblr posts
Text
op you speak to my soul
bitch java is so fucking annoying why does the S in String need to be capitalized but the i in int has to be in lowercase??? why do you need to import a whole ass module just to take an input from the user??? why are all the commands so fucking long and hard to remember??? JUST DIE
#i can never frickin remember what the heck to do in my java code#the S in String still pisses me off#and exactly why do i need to import a whole module#mean python: a=input()#now that's what i call short and sweet ^^#im forever going go have a love hate relationship with java#java#codeblr#coding#computer science#java code#java developer#programming languages#programming#code#comp sci#app dev
137 notes
·
View notes
Note
Any good python modules I can learn now that I'm familiar with the basics?
Hiya 💗
Yep, here's a bunch you can import them into your program to play around with!
math: Provides mathematical functions and constants.
random: Enables generation of random numbers, choices, and shuffling.
datetime: Offers classes for working with dates and times.
os: Allows interaction with the operating system, such as file and directory manipulation.
sys: Provides access to system-specific parameters and functions.
json: Enables working with JSON (JavaScript Object Notation) data.
csv: Simplifies reading and writing CSV (Comma-Separated Values) files.
re: Provides regular expression matching operations.
requests: Allows making HTTP requests to interact with web servers.
matplotlib: A popular plotting library for creating visualizations.
numpy: Enables numerical computations and working with arrays.
pandas: Provides data structures and analysis tools for data manipulation.
turtle: Allows creating graphics and simple games using turtle graphics.
time: Offers functions for time-related operations.
argparse: Simplifies creating command-line interfaces with argument parsing.
How to actually import to your program?
Just in case you don't know, or those reading who don't know:
Use the 'import' keyword, preferably at the top of the page, and the name of the module you want to import. OPTIONAL: you could add 'as [shortname you want to name it in your program]' at the end to use the shortname instead of the whole module name
Hope this helps, good luck with your Python programming! 🙌🏾
60 notes
·
View notes
Text
Object-Oriented Programming (OOP) Explaine
Object-Oriented Programming (OOP) is a programming paradigm based on the concept of "objects," which represent real-world entities. Objects combine data (attributes) and functions (methods) into a single unit. OOP promotes code reusability, modularity, and scalability, making it a popular approach in modern software development.
Core Concepts of Object-Oriented Programming
Classes and Objects
Class: A blueprint or template for creating objects. It defines properties (attributes) and behaviors (methods).
Object: An instance of a class. Each object has unique data but follows the structure defined by its
Encapsulations
Encapsulation means bundling data (attributes) and methods that operate on that data within a class. It protects object properties by restricting direct access.
Access to attributes is controlled through getter and setter methods.Example: pythonCopyEditclass Person: def __init__(self, name): self.__name = name # Private attribute def get_name(self): return self.__name person = Person("Alice") print(person.get_name()) # Output: Alice
Inheritance
Inheritance allows a class (child) to inherit properties and methods from another class (parent). It promotes code reuse and hierarchical relationships.Example: pythonCopyEditclass Animal: def speak(self): print("Animal speaks") class Dog(Animal): def speak(self): print("Dog barks") dog = Dog() dog.speak() # Output: Dog barks
Polymorphism
Polymorphism allows methods to have multiple forms. It enables the same function to work with different object types.
Two common types:
Method Overriding (child class redefines parent method).
Method Overloading (same method name, different parameters – not natively supported in Python).Example: pythonCopyEditclass Bird: def sound(self): print("Bird chirps") class Cat: def sound(self): print("Cat meows") def make_sound(animal): animal.sound() make_sound(Bird()) # Output: Bird chirps make_sound(Cat()) # Output: Cat meows
Abstraction
Abstraction hides complex implementation details and shows only the essential features.
In Python, this is achieved using abstract classes and methods (via the abc module).Example: pythonCopyEditfrom abc import ABC, abstractmethod class Shape(ABC): @abstractmethod def area(self): pass class Circle(Shape): def __init__(self, radius): self.radius = radius def area(self): return 3.14 * self.radius * self.radius circle = Circle(5) print(circle.area()) # Output: 78.5
Advantages of Object-Oriented Programming
Code Reusability: Use inheritance to reduce code duplication.
Modularity: Organize code into separate classes, improving readability and maintenance.
Scalability: Easily extend and modify programs as they grow.
Data Security: Protect sensitive data using encapsulation.
Flexibility: Use polymorphism for adaptable and reusable methods.
Real-World Applications of OOP
Software Development: Used in large-scale applications like operating systems, web frameworks, and databases.
Game Development: Objects represent game entities like characters and environments.
Banking Systems: Manage customer accounts, transactions, and security.
E-commerce Platforms: Handle products, users, and payment processing.
Machine Learning: Implement models as objects for efficient training and prediction.
Conclusion
Object-Oriented Programming is a powerful paradigm that enhances software design by using objects, encapsulation, inheritance, polymorphism, and abstraction. It is widely used in various industries to build scalable, maintainable, and efficient applications. Understanding and applying OOP principles is essential for modern software development.
: pythonCopyEdit
class Car: def __init__(self, brand, model): self.brand = brand self.model = model def display_info(self): print(f"Car: {self.brand} {self.model}") my_car = Car("Toyota", "Camry") my_car.display_info() # Output: Car: Toyota Camry
Encapsulation
2 notes
·
View notes
Text
how to download (and mirror and transcribe) youtube videos
so the news that google is deleting inactive youtube channels was a miscommunication -- "Additionally, we do not have plans to delete accounts with YouTube videos at this time" (source, emphasis mine). but i hope this was a wake up call that archiving videos (and other content) you care about is really important. buy hard drives, save, reshare. videos dont stay up forever. youtube isnt forever.
i know how difficult it is to get into downloading videos, with how all youtube to mp4 websites seem to be broken. this post compiles general guides on how to manually download youtube videos (among other actions) through python programs. it's simple if you just follow the steps and constantly search the errors you encounter. i will also detail how i personally do it with my windows 10 pc, in case you use the same tools.
remember: your search engine, reddit, github, and help commands are your best friends.
* downloading youtube videos
reddit yt-dlp guide
original yt-dlp guide
how to download the best quality mp4
how to download videos from a search result
how to use command prompt
what is command prompt? this is a windows application where you navigate folders and run programs. you just type a command and hit enter. ctrl+c ends a command/program, ctrl+s pauses it (pressing any key unpauses)
how do i navigate folders? the basic commands are so: a) cd "[path]" to change directory (always put path and link names in double quotes so they are processed properly), b) cd .\.. takes you to the previous folder (ex: if you're in C:\folder A\folder B and run cd .\.. you go to C:\folder A), c) you can go to other drives by typing the letter and colon (ex: if you are in C:, typing D: then entering takes you to your D drive). this is important because where your python programs are stored is where you have to run them.
how to run python programs through cmd prompt? a) download the latest version of python. b) use pip to install programs. c) make sure you have also downloaded a program's dependencies (analogous to "pre-requisites"). d) type the program name then the command.
make sure to always update python and pip.
how to use yt-dlp to download youtube videos
how to get download yt-dlp? this guide worked perfectly for me. make sure to download all python programs in the same folder.
navigate to the folder you installed yt-dlp
the following are examples of commands you can use:
yt-dlp -h -- get a list of all commands
yt-dlp "[link]" -- download video as is (often in webm format)
yt-dlp "[link]" -f "bestvideo[ext=mp4]+bestaudio[ext=m4a]/best[ext=mp4]/best" -- download the highest quality mp4 video (highest possible in mp4 is 1080p)
yt-dlp -x --audio-format mp3 "[link]" -- download audio only as mp3
yt-dlp -i "[playlist link]" -- download a full playlist (you may also use the best quality command here)
yt-dlp -i "[playlist link]" --playlist-items [range] -- download range of playlist items
look at the guides at the top of this section for my ideas of what you can do with yt-dlp. you can even use yt-dlp to download from other websites
note: if you want to download instagram reels, you must include: --cookies-from-browser [firefox / chrome / etc] -- choose your browser
** mirroring youtube videos to archive.org
github tubeup guide: "tubeup uses yt-dlp to download a Youtube video (or any other provider supported by yt-dlp), and then uploads it with all metadata to the Internet Archive using the python module internetarchive."
this guide shows you how to install and use the program. this is an easy way to archive videos with the proper metadata -- do not archive videos en masse
the mirrortube archive.org community
*** transcribing videos
transcribing youtube videos w/o downloading: application
transcribing any downloaded video: openai guide
extra1: searching videos
ive seen confusion on how to naviagte youtube search these days. i know!!!! here are some tips:
changing search options to search by upload date shows *ACTUAL* results, rather than suggestions.
the same google tricks work on youtube: google tricks guide
using yt-dlp to search can be helpful to search youtube more precisely
extra2: downloading twitter videos online
i use this regularly, so i thought id also share.
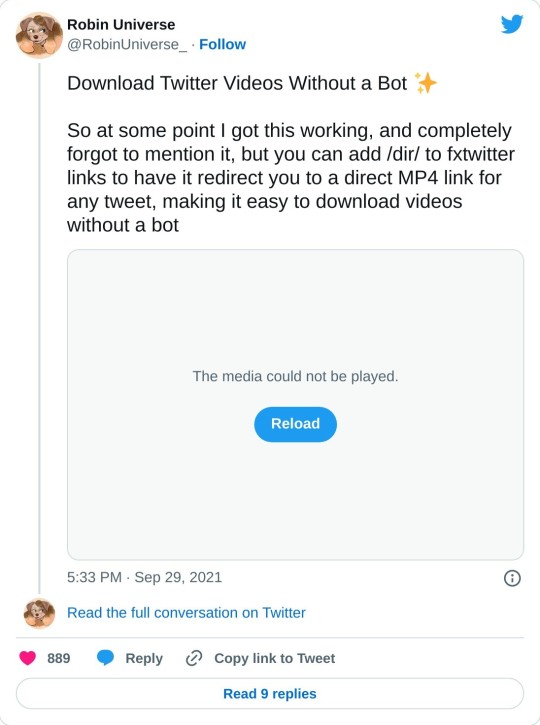
76 notes
·
View notes
Text
Project Euler #2
Welcome back to my series on Project Euler problems. HackerRank Lets get into it.
Links:
Project Euler: https://projecteuler.net/problem=2 HackerRank: https://www.hackerrank.com/contests/projecteuler/challenges/euler002
Each new term in the Fibonacci sequence is generated by adding the previous two terms. By starting with 1 and 2, the first 10 terms will be: 1,2,3,5,8,13,21,34,55,89,… By considering the terms in the Fibonacci sequence whose values do not exceed N, find the sum of the even-valued terms.
So first thing to note is just how odds and evens work. This starts with 1 and 2 so Odd + Even = Odd, then the next term would just be Even + Odd = Odd. It isn't until the 3rd addition that we get Odd + Odd = Even. After that this cycle will repeat.
So really what this is asking for is, starting at index 2, what is the sum of every 3rd term less than N.
F(2) + F(5) + ... + F(3k + 2)
(Where F(N) is the Nth Fibonacci number)
Now, computing Fibonacci numbers notoriously sucks to do, with the naive way of doing it causing you to compute from just F(20) would require computing F(3) like hundreds if not thousands of times over. So the best way to do it is to create either a hash or a cache to store it. I'm going to be utilizing something built into Python, "lru_cache" from the "functools" module. It'll just store the answers for me so I don't have to recompute what F(10) is thousands of times.
Here's my HackerRank code:
import sys from functools import lru_cache @lru_cache def fibonacci(N: int) -> int: if N < 0: return 0 if N <= 1: return 1 return fibonacci(N-1) + fibonacci(N-2) t = int(input().strip()) for a0 in range(t): n = int(input().strip()) x = 2 f = fibonacci(x) total = 0 while f <= n: total += f x += 3 f = fibonacci(x) print(total)
So as you can see, I just start at 2 and keep incrementing by fibonacci number by 3 each time. Then the loop will stop when my fibonacci number exceeds N.
And that's all tests passed! Onto the next one!
2 notes
·
View notes
Text
"DCA"(DIPLOMA IN COMPUTER APPLICATION)
The best career beginning course....
Golden institute is ISO 9001-2015 certified institute. Here you can get all types of computer courses such as DCA, CFA , Python, Digital marketing, and Tally prime . Diploma in Computer Applications (DCA) is a 1 year "Diploma Course" in the field of Computer Applications which provides specialization in various fields such as Fundamentals & Office Productivity tools, Graphic Design & Multimedia, Programming and Functional application Software.
A few of the popular DCA study subjects are listed below
Basic internet concepts Computer Fundamentals Introduction to programming Programming in C RDBMS & Data Management Multimedia Corel draw Tally ERP 9.0 Photoshop
Benefits of Diploma in Computer Application (DCA)
After completion of the DCA course student will able to join any computer jobs with private and government sectors. The certification of this course is fully valid for any government and private deportment worldwide. DCA is the only best option for the student to learn computer skills with affordable fees.
DCA Computer course : Eligibilities are here... Students aspiring to pursue Diploma in Computer Applications (DCA) course must have completed their higher school/ 10 + 2 from a recognized board. Choosing Computers as their main or optional subject after class 10 will give students an additional edge over others. Apart from this no other eligibility criteria is set for aspirants. No minimum cutoff is required.
"TALLY"
A Tally is accounting software. To pursue Tally Course (Certificate and Diploma) you must have certain educational qualifications to thrive and prosper. The eligibility criteria for the tally course is given below along with all significant details on how to approach learning Tally, and how you can successfully complete the course. Generally, the duration of a Tally course is 6 month to 1 year ,but it varies depending on the tally institution you want to join. Likewise, tally course fees are Rs. 10000-20000 on average but it also varies depending on what type of tally course or college you opt for. accounting – Accounting plays a pivotal role in Tally
Key Benefits of the Course:
Effective lessons (topics are explained through a step-by-step process in a very simple language) The course offers videos and e-books (we have two options Video tutorials in Hindi2. e-book course material in English) It offers a planned curriculum (the entire tally online course is designed to meet the requirements of the industry.) After the completion of the course, they offer certificates to the learners.
Tally Course Syllabus – Subjects To Learn Accounting Payroll Taxation Billing Banking Inventory
Tally Course
Eligibility criteria: 10+2 in commerce stream Educational level: Certificate or Diploma Course fee: INR 2200-5000 Skills required: Accounting, Finance, Taxation, Interpersonal Skills Scope after the course: Accountant, Finance Manager, Chartered Accountant, Executive Assistant, Operations Manager Average salary: INR 5,00,000 – 10,00,000
"In this Python course"
Rapidly develop feature-rich applications using Python's built-in statements, functions, and collection types. Structure code with classes, modules, and packages that leverage object-oriented features. Create multiple data accessors to manage various data storage formats. Access additional features with library modules and packages.
Python for Web Development – Flask Flask is a popular Python API that allows experts to build web applications. Python 2.6 and higher variants must install Flask, and you can import Flask on any Python IDE from the Flask package. This section of the course will help you install Flask and learn how to use the Python Flask Framework.
Subjects covered in Python for Web development using Flask:
Introduction to Python Web Framework Flask Installing Flask Working on GET, POST, PUT, METHODS using the Python Flask Framework Working on Templates, render template function
Python course fees and duration
A Python course costs around ₹2200-5000.This course fees can vary depending on multiple factors. For example, a self-paced online course will cost you less than a live interactive online classroom session, and offline training sessions are usually expensive ones. This is mainly because of the trainers’ costs, lab assistance, and other facilities.
Some other factors that affect the cost of a Python course are its duration, course syllabus, number of practical sessions, institute reputation and location, trainers’ expertise, etc. What is the duration of a Python course? The duration of a basic Python course is generally between 3 month to 6 months, and advanced courses can be 1 year . However, some courses extend up to 1 year and more when they combine multiple other courses or include internship programs.
Advantages of Python Python is easy to learn and put into practice. … Functions are defined. … Python allows for quick coding. … Python is versatile. … Python understands compound data types. … Libraries in data science have Python interfaces. … Python is widely supported.
"GRAPHIC DESIGN"
Graphic design, in simple words, is a means that professional individuals use to communicate their ideas and messages. They make this communication possible through the means of visual media.
A graphic designing course helps aspiring individuals to become professional designers and create visual content for top institutions around the world. These courses are specialized to accommodate the needs and requirements of different people. The course is so popular that one does not even need to do a lot of research to choose their preferred colleges, institutes, or academies for their degrees, as they are almost mainstream now.
A graphic design course have objectives:
To train aspirants to become more creative with their visual approach. To train aspirants to be more efficient with the technical aspects of graphics-related tasks and also to acquaint them with relevant aspects of a computer. To train individuals about the various aspects of 2-D and 3-D graphics. To prepare aspirants to become fit for a professional graphic designing profession.
Which course is best for graphic design? Best graphic design courses after 12th - Graphic … Certificate Courses in Graphic Design: Adobe Photoshop. CorelDraw. InDesign. Illustrator. Sketchbook. Figma, etc.
It is possible to become an amateur Graphic Designer who is well on the road to becoming a professional Graphic Designer in about three months. In short, three months is what it will take to receive the professional training required to start building a set of competitive professional job materials.
THE BEST COMPUTER INSTITUTE GOLDEN EDUCATION,ROPNAGAR "PUNJAB"
The best mega DISCOUNT here for your best course in golden education institute in this year.
HURRY UP! GUYS TO JOIN US...
Don't miss the chance
You should go to our institute website
WWW.GOLDEN EDUCATION
CONTACT US: 98151-63600
VISIT IT:
#GOLDEN EDUCATION#INSTITUTE#COURSE#career#best courses#tallyprime#DCA#GRAPHICAL#python#ALL COURSE#ROOPAR
2 notes
·
View notes
Text
How do I learn Python in depth?
Improving Your Python Skills
Writing Python Programs Basics: Practice the basics solidly.
Syntax and Semantics: Make sure you are very strong in variables, data types, control flow, functions, and object-oriented programming.
Data Structures: Be able to work with lists, tuples, dictionaries, and sets, and know when to use which.
Modules and Packages: Study how to import and use built-in and third-party modules.
Advanced Concepts
Generators and Iterators: Know how to develop efficient iterators and generators for memory-efficient code.
Decorators: Learn how to dynamically alter functions using decorators.
Metaclasses: Understand how classes are created and can be customized.
Context Managers: Understand how contexts work with statements.
Project Practice
Personal Projects: You will work on projects that you want to, whether building a web application, data analysis tool, or a game.
Contributing to Open Source: Contribute to open-source projects in order to learn from senior developers. Get exposed to real-life code.
Online Challenges: Take part in coding challenges on HackerRank, LeetCode, or Project Euler.
Learn Various Libraries and Frameworks
Scientific Computing: NumPy, SciPy, Pandas
Data Visualization: Matplotlib, Seaborn
Machine Learning: Scikit-learn, TensorFlow, PyTorch
Web Development: Django, Flask
Data Analysis: Dask, Airflow
Read Pythonic Code
Open Source Projects: Study the source code of a few popular Python projects. Go through their best practices and idiomatic Python.
Books and Tutorials: Read all the code examples in books and tutorials on Python.
Conferences and Workshops
Attend conferences and workshops that will help you further your skills in Python. PyCon is an annual Python conference that includes talks, workshops, and even networking opportunities. Local meetups will let you connect with other Python developers in your area.
Learn Continuously
Follow Blogs and Podcasts: Keep reading blogs and listening to podcasts that will keep you updated with the latest trends and developments taking place within the Python community.
Online Courses: Advanced understanding in Python can be acquired by taking online courses on the subject.
Try It Yourself: Trying new techniques and libraries expands one's knowledge.
Other Recommendations
Readable-Clean Code: For code writing, it's essential to follow the style guide in Python, PEP
Naming your variables and functions as close to their utilization as possible is also recommended.
Test Your Code: Unit tests will help in establishing the correctness of your code.
Coding with Others: Doing pair programming and code reviews would provide you with experience from other coders.
You are not Afraid to Ask for Help: Never hesitate to ask for help when things are beyond your hand-on areas, be it online communities or mentors.
These steps, along with consistent practice, will help you become proficient in Python development and open a wide range of possibilities in your career.
2 notes
·
View notes
Text
why the hell is python basically "the beginner language" its so fucking annoying i wanted to try to do something relatively simple (click when the pixel under my cursor changes color) but i had to spend like 45 minutes getting it to be able to control my mouse and do things such as click because i found the module i need for it and tried to install that only for pip to just not work because apparently you need to either be in regular command prompt and type "py -m" before it or you need this longass command in order to do it through the python console and it took way too long to find someone who mentioned that information necessary to install modules but then it wasnt working in pycharm because apparently the installations are specific to the venv rather than just python and so i had to figure out hwo to open up the console in pycharm and use the longass command to install it there BUT THEN that dindt work because the venv didnt allow installing other software or whatever so i had to go to the cfg file for it and FINALLY was able to install it and use it in pycharm. and then i learned that i have to install another module in order to use the sleep command. sleep. why does this beginner language not have a sleep command by default thats such a basic common mechanic why to i need to install and import time anyway if you actually read through all of my rambling and complaining thank you for that and FUCK python. i am however going to continue using it for this little project (building off of the original purpose) because once the modules are installed it is a very simple language
#i vaguely remember pycharm having some way of installing modules built into it but i thought it would be quicker to just use the pip command#it was not#also i did learn python a long time ago but have completely forgotten all of it#196#rule#python
3 notes
·
View notes
Text
The Ultimate Guide to Mastering Power BI: A Comprehensive Course by Zabeel Institute
In today's data-driven world, businesses are constantly seeking ways to leverage data for better decision-making. One of the most powerful tools to achieve this is Microsoft Power BI, a business analytics solution that empowers organizations to visualize their data, share insights, and make data-driven decisions in real time. If you're looking to gain expertise in this powerful tool, Zabeel Institute's Power BI course in Dubai is the perfect starting point.
What is Power BI?
Power BI is a suite of business analytics tools that allows users to analyze data and share insights. With its user-friendly interface and robust capabilities, Power BI enables both beginners and seasoned professionals to create interactive dashboards and reports. Whether you're dealing with simple data sets or complex analytics, Power BI makes data visualization intuitive and accessible.
Why Learn Power BI?
Learning Power BI opens up a world of opportunities. As businesses increasingly rely on data to drive their decisions, professionals skilled in Power BI are in high demand. Here are some compelling reasons why you should consider enrolling in a Power BI course:
High Demand for Power BI Skills: With the rise of data-driven decision-making, there is a growing demand for professionals who can interpret and visualize data effectively.
Career Advancement: Mastering Power BI can significantly enhance your career prospects, especially in fields such as data analysis, business intelligence, and management.
Versatility: Power BI is versatile and can be applied across various industries, including finance, healthcare, marketing, and more.
Improved Decision-Making: By learning how to create detailed and interactive reports, you can help your organization make informed decisions based on real-time data.
Course Overview: Analyzing Data with Microsoft Power BI
At Zabeel Institute, the Analyzing Data with Microsoft Power BI course is designed to equip you with the skills needed to harness the full potential of Power BI. This comprehensive course covers everything from the basics to advanced data visualization techniques.
1. Introduction to Power BI
The course begins with an introduction to the Power BI environment. You'll learn about the Power BI service, Power BI Desktop, and how to navigate through these tools efficiently. Understanding the interface is crucial for leveraging the full capabilities of Power BI.
2. Connecting to Data Sources
Power BI allows you to connect to a wide range of data sources, including Excel, SQL Server, Azure, and many more. In this module, you'll learn how to import data from various sources and prepare it for analysis.
3. Data Transformation and Cleaning
Before you can visualize your data, it often needs to be cleaned and transformed. This section of the course will teach you how to use Power Query to shape and clean your data, ensuring it's ready for analysis.
4. Creating Data Models
Data modeling is a crucial step in the data analysis process. In this module, you'll learn how to create relationships between different data sets and build a robust data model that supports your analysis.
5. Building Interactive Dashboards
One of Power BI's strengths is its ability to create interactive dashboards. You'll learn how to design visually appealing dashboards that provide meaningful insights at a glance.
6. Advanced Data Visualizations
Once you're comfortable with the basics, the course delves into more advanced visualizations. You'll explore custom visuals, R and Python integration, and how to create sophisticated reports that stand out.
7. DAX (Data Analysis Expressions)
DAX is a powerful formula language in Power BI. This section covers the fundamentals of DAX, enabling you to perform complex calculations and create dynamic reports.
8. Power BI Service and Collaboration
Power BI is not just about creating reports—it's also about sharing and collaborating on those reports. You'll learn how to publish your reports to the Power BI service, set up security, and collaborate with your team.
9. Power BI Mobile App
In today's mobile world, being able to access your reports on the go is essential. The course will show you how to use the Power BI Mobile App to view and interact with your dashboards from anywhere.
10. Best Practices for Power BI
To ensure you're getting the most out of Power BI, the course concludes with a module on best practices. This includes tips on performance optimization, report design, and maintaining data security.
Why Choose Zabeel Institute?
When it comes to learning Power BI, choosing the right institute is crucial. Zabeel Institute stands out for several reasons:
Experienced Instructors: Zabeel Institute's instructors are industry experts with years of experience in data analysis and business intelligence.
Hands-On Training: The course is designed to be highly practical, with plenty of hands-on exercises to reinforce your learning.
Industry-Recognized Certification: Upon completion, you'll receive a certification that is recognized by employers globally, giving you an edge in the job market.
Flexible Learning Options: Whether you prefer in-person classes or online learning, Zabeel Institute offers flexible options to suit your schedule.
Real-World Applications of Power BI
Understanding Power BI is one thing, but knowing how to apply it in the real world is what truly matters. Here are some examples of how Power BI can be used across various industries:
Finance: Create detailed financial reports and dashboards that track key metrics such as revenue, expenses, and profitability.
Healthcare: Analyze patient data to improve healthcare delivery and outcomes.
Retail: Track sales data, customer trends, and inventory levels in real time.
Marketing: Measure the effectiveness of marketing campaigns by analyzing data from multiple channels.
Human Resources: Monitor employee performance, track recruitment metrics, and analyze workforce trends.
Success Stories: How Power BI Transformed Businesses
To illustrate the impact of Power BI, let's look at a few success stories:
Company A: This retail giant used Power BI to analyze customer purchasing behavior, resulting in a 15% increase in sales.
Company B: A financial services firm leveraged Power BI to streamline its reporting process, reducing the time spent on report generation by 50%.
Company C: A healthcare provider used Power BI to track patient outcomes, leading to improved patient care and reduced readmission rates.
Mastering Power BI is not just about learning a tool—it's about acquiring a skill that can transform the way you work with data. Whether you're looking to advance your career, enhance your business's decision-making capabilities, or simply stay ahead in today's data-driven world, Zabeel Institute's Power BI course is the perfect choice.
Don't miss out on the opportunity to learn from the best. Enroll in Zabeel Institute's Power BI course today and take the first step towards becoming a Power BI expert.
Ready to transform your career with Power BI? Enroll in Zabeel Institute's Power BI course now and start your journey towards mastering data analysis and visualization. Visit Zabeel Institut for more information.
2 notes
·
View notes
Text
Python Day 2
Today I am starting off with exercise 13. Exercise 13 introduces the concepts of variables, modules, and argv.
[ID: Exercise 13 code. It imports the argv module from sys, then uses argv to create 4 variables, script, first, second, and third. Next print() is used to print out the different variables /ID]
When calling the program I was confused as to why I got the error of too many variables. Looking into this I found that the first variable of 'argv' is always going to be the script. I then fixed that and added in script as the first variable.
Next for the study drill I wrote a new variable and updated the code to print the retrieved information.
Alrighty then - onto exercise 14. Exercise 14 is about practicing prompts and variables.
In the study drills I updated the script with a new prompt and print statement.
Exercise 15 is a simple program that prints out the contents of a file. An important thing to note is to always close the file when doing things like this!
Exercise 16 practices making a copy of a file and then updating it with 3 lines from user input.
I ended up running into the issue where it was saying that it couldn't read the file. I ended up finding out that .read() starts from the cursor position - and if the cursor is at the end of the file from writing it you will not have your file printed.
Exercise 17 is practicing copying files over and was relatively simple.
#learn python with F.M.#learn to code#learn python#coding resources#python#coding#lpthw#transgender programmer#codeblr#code#image undescribed#Sorry for not adding ID folks - my spoons are too low
4 notes
·
View notes
Text
Exploring Python: Features and Where It's Used
Python is a versatile programming language that has gained significant popularity in recent times. It's known for its ease of use, readability, and adaptability, making it an excellent choice for both newcomers and experienced programmers. In this article, we'll delve into the specifics of what Python is and explore its various applications.
What is Python?
Python is an interpreted programming language that is high-level and serves multiple purposes. Created by Guido van Rossum and released in 1991, Python is designed to prioritize code readability and simplicity, with a clean and minimalistic syntax. It places emphasis on using proper indentation and whitespace, making it more convenient for programmers to write and comprehend code.
Key Traits of Python :
Simplicity and Readability: Python code is structured in a way that's easy to read and understand. This reduces the time and effort required for both creating and maintaining software.
Python code example: print("Hello, World!")
Versatility: Python is applicable across various domains, from web development and scientific computing to data analysis, artificial intelligence, and more.
Python code example: import numpy as np
Extensive Standard Library: Python offers an extensive collection of pre-built libraries and modules. These resources provide developers with ready-made tools and functions to tackle complex tasks efficiently.
Python code example: import matplotlib.pyplot as plt
Compatibility Across Platforms: Python is available on multiple operating systems, including Windows, macOS, and Linux. This allows programmers to create and run code seamlessly across different platforms.
Strong Community Support: Python boasts an active community of developers who contribute to its growth and provide support through online forums, documentation, and open-source contributions. This community support makes Python an excellent choice for developers seeking assistance or collaboration.
Where is Python Utilized?
Due to its versatility, Python is utilized in various domains and industries. Some key areas where Python is widely applied include:
Web Development: Python is highly suitable for web development tasks. It offers powerful frameworks like Django and Flask, simplifying the process of building robust web applications. The simplicity and readability of Python code enable developers to create clean and maintainable web applications efficiently.
Data Science and Machine Learning: Python has become the go-to language for data scientists and machine learning practitioners. Its extensive libraries such as NumPy, Pandas, and SciPy, along with specialized libraries like TensorFlow and PyTorch, facilitate a seamless workflow for data analysis, modeling, and implementing machine learning algorithms.
Scientific Computing: Python is extensively used in scientific computing and research due to its rich scientific libraries and tools. Libraries like SciPy, Matplotlib, and NumPy enable efficient handling of scientific data, visualization, and numerical computations, making Python indispensable for scientists and researchers.
Automation and Scripting: Python's simplicity and versatility make it a preferred language for automating repetitive tasks and writing scripts. Its comprehensive standard library empowers developers to automate various processes within the operating system, network operations, and file manipulation, making it popular among system administrators and DevOps professionals.
Game Development: Python's ease of use and availability of libraries like Pygame make it an excellent choice for game development. Developers can create interactive and engaging games efficiently, and the language's simplicity allows for quick prototyping and development cycles.
Internet of Things (IoT): Python's lightweight nature and compatibility with microcontrollers make it suitable for developing applications for the Internet of Things. Libraries like Circuit Python enable developers to work with sensors, create interactive hardware projects, and connect devices to the internet.
Python's versatility and simplicity have made it one of the most widely used programming languages across diverse domains. Its clean syntax, extensive libraries, and cross-platform compatibility make it a powerful tool for developers. Whether for web development, data science, automation, or game development, Python proves to be an excellent choice for programmers seeking efficiency and user-friendliness. If you're considering learning a programming language or expanding your skills, Python is undoubtedly worth exploring.
9 notes
·
View notes
Text
Good afternoon TUMBLR - March 23th - 2024
''Mr. Plant has owed me a shoe since July 5, 1971."
January 1998 - October 1999 - NLNG - TSKJ Port Harcourt Project (Nigeria).
Part 3.
SWIMMING SUIT I hadn't brought a swimsuit from Italy, and since we spent Sunday in one of the hotel pools in the area, I decided to buy one. I told the driver to take me to a location where I could find one, he stopped the Land Rover in front of a small shop along Aba Road. There were different types and colors of swimsuits inside, in the end I opted for one in red and blue. Before the shopkeeper placed it in the usual supermarket plastic bag, I wanted to take one last look - and here's the ''surprise'': IT WAS 2nd HAND!! But the most incredible thing was that they had put it up for sale with ''clear signals'' of those who had used it before!!! I told the sales man: at least wash it? Naaa…… I left with a mixture of fury and disbelief: I couldn't belive to what my eyes have seen.
SOKU PROJECT SITE
Niger river - On the way to Soku.
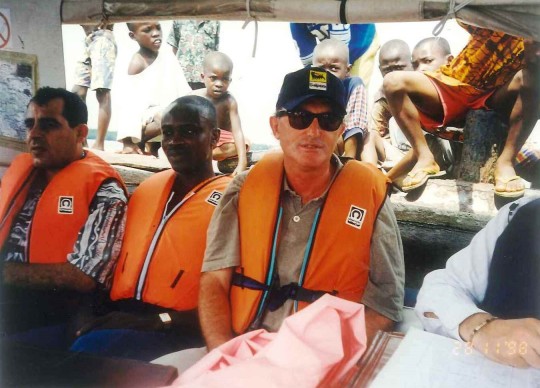
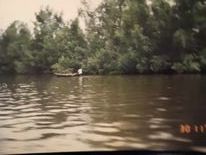
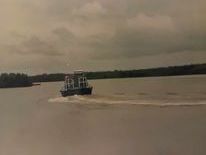
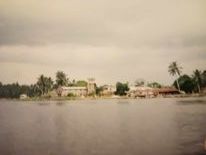
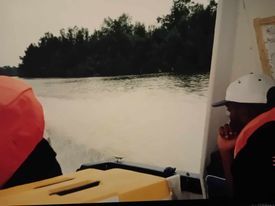
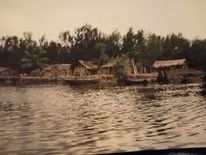
As mentioned elsewhere, the Soku Gas Plant was a project that SAIPEM Nigeria was executing in parallel to our TSKJ project. The site was located about 2 hours by speed boat from Port Harcourt, in the middle of the Niger River Delta. All around there was a dense rainforest, so to avoid cutting down thousands of trees it was decided that the plant would be built on an artificial island in the middle of the river. A Spanish company specialized in dredging and construction of artificial islands (called reclaimed land) was commissioned and within 6 months a 1.5 km by 800 meter platform was ready to begin work. A camp was built by importing portacabins from SAIPEM NORWAY (yes, you read that right). The reasons behind SAIPEM Procurement was:
''If they are good for the cold of Norway, they will also be good for the tropical forest of Nigeria''. Carlo Sgorbani arrival to Port Harcourt. Sgorbani was also an ''old'' SAIPEM guy. I met him during the Bir Rebaa project in Algeria. I then found it again in Abu Dhabi for the Taweela aWater Project, where during the last part of the project he assumed the position of Site Manager (And immediately left our compound to rent a villa at Hilton hotel). An early one morning I went to Branch Office and met Sgorbani who was still under shock due the night flight from Paris.
Ohh...I cant believe to my eyes! How come you're here Carlo?
And he, with the perpetual cigarette in his hand: well… I should be the Site Manager of the Soku Gas Plant project…
Soku Gas Plant?? But… are you sure of what you say? I'm quite sure there are any Hilton villas in Soku….... well, come on… you can't always go for luxury… and then you'll see that the ex-Norway containers won't be so uncomfortable!
He didn't reply.
SAIPEM Club SAIPEM has always cared about the ''welfare'' of its employees located in the most sh****hole remote and difficult areas of the planet. Therefore the Management decided to install a clubhouse inside the Soku compound. As soon as the Worry project structure was made available, it was transported by barge to Soku. It consisted of different container modules, and inside it had a billiard room, ping pong, TV room, bar with tables where can play cards in the evening. Behind the bar counter, above the shelves that held the bottles of liquor, a long python skin had been fixed. And this will cause several problems.
PYTHON STRIKE The morning after the club inauguration, Sgorbani and all the other colleagues were woken up by strange moans and screams coming from inside the camp. They immediately rushed to the front of the club and found themselves in front of dozens of people lying on the ground crying, wriggling and sprinkling sand on their heads. Local people were screaming like:
Our Lord is dead…you killed our Lord our Lord is dead…you have killed our God....... All the expatriates present could not understand what was going on before their eyes. Sgorbani called the local PRO, who revealed the burden:
''Sir – he said – these people are telling that you from SAIPEM captured and killed their Lord, and then after having slaughtered him you hung his skin in your club, offending his memory even more – now they expect years of disgrace if nothing is done to remedy this tragedy."
But…but…All of this is inconceivable – replied Sgorbani – the python skin was hung on the wall of the bar when the structure was in Warry, months ago, not here……….
I know, Sir – said the PRO – but these people say that this was why they hadn't seen their Lord for months: he had migrated to Warry, someone from SAIPEM captured him and made him a trophy.
Sgorbani and all other expats know whether to laugh or get angry at what they had heard and what was happening in front of them - however the Site Manager understood that laughing at the matter could generate further and serious problems - so he asked the PRO:
Ok… okay… so what should we do?
Sir – said the PRO – I spoke with their Chief and he says that the only solution to this serious matter is to call a Great Medicine Man who lives in Calabar (about 200 km from Soku) Only he will be able to exorcise the bad influences caused to what SAIPEM people did. But first of all we need to remove the python skin off the bar wall, Sir.
Ok – replied Sgorbani – we'll do it right away, but in the meantime we have to get back to work!
No Sir, until everything is resolved, work cannot resume.
Sgorbani was about to fly into a rage, but was able to contain himself - he ordered the PRO to proceed as quickly as possible with the call of this ''medicine man'' - that he should come immediately, SAIPEM would spare no expense, and would do everything needed made available, starting from the speed boat. Two days of great tension passed in the camp and on the artificial island. Time seemed suspended, waiting for the arrival of the sorcerer who would resolve the drama. An eerie silence loomed over everything and everyone, when construction activities usually filled the place with noise. In just two days (a great achievement considering that it was happening in Nigeria) the ''Great Medicine Man of Calabar'' arrived in great pomp, dressed in traditional clothes, covered by a bright apple-green cloak, with a headdress of peacock feathers and studded with precious stones (nobody knew if true or fake…).
Between two wings of the crowd who knelt as he passed on the way from the docking pier to the club, the sorcerer distributed blessings with his inlaid staff. No one, except the faithful, was allowed inside the club, where, after a brief ceremony, the python skin was peeled off the wall and carefully carried outside by at least six weeping people. The skin was carried as if in procession to the pier, where a previously prepared canoe transported it across the river to the vicinity of the village where the local tribe lived. A pit had been dug there which, covered with a white sheet, welcomed the python's skin. Once the skin was wrapped, the burial proceeded, accompanied by songs, blessings and mysterious formulas recited by the Great Medicine Man, who every now and then drew water from a drum with a palm leaf and sprinkled the grave. Once filled with earth, flowers and tree branches were planted on the tomb, and the area was marked off with stones. The funeral was over, and the sorcerer moved on to collect the money from SAIPEM PRO - the cost of the entire "ceremony was over 20,000 dollars. The next day, work at site could finally resume.
ATTACK on SOKU COMPOUND Perhaps the python affair had been a sort of ''dress rehearsal'' to see the type of response that SAIPEM would have given to an emergency. The fact is that a few weeks passed, and a new big problem arose for Sgorbani and the SAIPEM expatriates on Soku project. It was an August hot morning, when they woke up with a noise caused by rifle and stick blows that an handful of ''rebels'' were giving to their dormitory containers who had come from Norway. After a few minutes, all the expatriates were gathered in front of the offices, and when asked by the one who seemed to be in charge "where the Chief was", the answer was that he was in his office. (Sgorbani used to get up very early). Some rebels entered the offices, and to make it clear that they had serious intentions, they fired a couple of rifle shots which fortunately did not hit Sgorbani, but lodged in the wall behind his head. He too was pushed into the square in front of the offices, and made to lie on his stomach like everyone else. The rebels claimed to have taken possession of the artificial island of SOKU and the site, to put an end to the indiscriminate exploitation of the River State's resources. They wanted money, hiring of local staff, salary increases for those already working with SAIPEM, construction of a school and a hospital in the adjacent community. These intentions would be followed by an ultimatum, after which if the requests were not accepted by the local governments and the top management of SAIPEM, they would begin to eliminate a hostage every 2 hours. While all this was happening, the Nigerian Army Security forces had surrounded the artificial island. A long-distance dialogue then began between the Major who led the military and the guy who professed to be the leader of the rebels. The military's strategy immediately appeared clear:
''First free the hostages and then we will sit down for negotiation'' - this was the message delivered to the attackers. Who naturally refused, demanding at least the delivery of a large sum of cash, the arrival of the local Governor, who should have handed himself over to them, to unblock the situation. In the meantime, time passed, and the time set for the expiry of the ultimatum approached, and the hostages were still on the ground with their hands behind their heads. The tension mounted as the hours passed, and when there were only a few minutes left before the ultimatum expired, suddenly the Security forces attacked the Rebels! Taken by surprise, many of them tried to escape, others took some of the hostages by force to shield themselves from the military fire. For a few minutes there was total chaos: shots, shouts, the rebels trying to reach some canoes hidden among the branches on the river bank, to escape capture. The clashes lasted a few minutes, and soon everything was silent, only a few sporadic bursts could be heard from the automatic weapons with which the Task Force eliminated the remaining resistance. Some rebels were captured and immediately knocked to the ground with rifle butts. Most of the hostages had fled to the dormitory containers and locked themselves inside. Once order was restored, the Major asked to speak to Sgorbani.
Do you have a couple of empty containers?
Yes, of course – replied the Site Manager
Have them taken to an open, sunny area. Zaccagnini had the order carried out, and after an hour the SAIPEM crane deposited two empty containers in an open area on the island. The Major then ordered his men to let the captured rebels enter the containers. When the operation was concluded, the containers were closed with heavy padlocks, and the Major set up guards. Then he said aloud:
''No one must go near the containers, this scum must remain awaiting the decisions of the Military Tribunal of Port Harcourt''.
6 notes
·
View notes
Text
More Notes on the Computer Music Playpen
I have finished maintenance on the VST3 plugin opcodes for Csound, Csound for Android, and some other things, and am re-focusing in composition.
One thing that happened as I was cleaning up the VST3 opcodes is that I discovered a very important thing. There are computer music programs that function as VST3 plugins and that significantly exceed the quality or power what Csound has so far done on it own, just for examples that I am using or plan to use:
The Valhalla reverbs by Sean Costello -- I think these actually derive from a reverb design that Sean did in the 1990s when he and I both were attending the Woof meetings at Columbia University. Sean's reverb design was ported first to Csound orchestra code, and then to C as a built-in opcode. It's the best and most widely used reverb in Csound, but it's not as good as the Valhalla reverbs, partly because the Valhalla reverbs can do a good job of preserving stereo.
Cardinal -- This is a fairly complete port of the VCV Rack "virtual Eurorack" patchable modular synthesiser not only to a VST3 plugin, but also to a WebAssembly module. This is exactly like sticking a very good Eurorack synthesizer right into Csound.
plugdata -- This is most of Pure Data, but with a slightly different and somewhat smoother user interface, as a VST3 plugin.
I also discovered that some popular digital audio workstations (DAWs), the workhorses of the popular music production industry, can embed algorithmic composition code written in a scripting language. For example, Reaper can host scripts written in Lua or Python, both of which are entirely capable of sophisticated algorithmic composition, and both of which have Csound APIs. And of course any of these DAWs can host Csound in the form of a Cabbage plugin.
All of this raises for me the question: What's the playpen? What's the most productive environment for me to compose in? Is it a DAW that now embeds my algorithms and my Csound instruments, or is it just code?
Right now the answer is not simply code, but specifically HTML5 code. And here is my experience and my reasons for not jumping to a DAW.
I don't want my pieces to break. I want my pieces to run in 20 years (assuming I am still around) just as they run today. Both HTML5 and Csound are "versionless" in the sense that they intend, and mostly succeed, in preserving complete backwards compatibility. There are Csound pieces from before 1990 that run just fine today -- that's over 33 years. But DAWs, so far, don't have such a good record in this department. I think many people find they have to keep porting older pieces to keep then running in newer software.
I'm always using a lot of resources, a lot of software, a lot of libraries. The HTML5 environment just makes this a great deal easier. Any piece of software that either is written in JavaScript or WebAssembly, or provides a JavaScript interface, can be used in a piece with a little but of JavaScript glue code. That includes Csound itself, my algorithmic composition software CsoundAC, the live coding system Strudel, and now Cardinal.
The Web browser itself contains a fantastic panoply of software, notably WebGL and WebAudio, so it's very easy to do visual music in the HTML5 environment.
2 notes
·
View notes
Note
do you have rofi config recommendations for the coding-illiterate? :3
if you're looking for a nice theme i would recommend something like this catppuccin one (one of my fave colourschemes) :3 i think other popular colourschemes have rofi themes too !
the instructions are straightforward and at most require changing two config options as explained on the page.
if you're interested in using rofi for more advanced stuff there's a "not really programming" way and a "basic programming" way.
there's plenty of custom scripts by other people that use rofi's dmenu mode (the rough format is input_command | rofi -dmenu --other-options | output_command) and all you have to do is run the script/bind it to a shortcut.
the method i use does involve coding but nothing advanced - in fact the github page for it has some good examples ! this is a python module that lets you write a python script to interact with rofi. you can install it from the github or via pip (python's package manager).
a simple script to pick favourite programs from a list might something look like this:
#importing the modules for running commands and using rofi import os import sys from rofi import Rofi
r = Rofi() #setting our label string and our lists for options to select from and commands to run after selecting label = "Pinned Programs:" options = ['browser', 'discord', 'files', 'editor'] commands = ['firefox', 'flatpak run com.discordapp.Discord', 'nemo', 'gedit'] #the magic rofi command ! ^w^ opens a rofi window in selection mode, showing the label and options we set earlier, and returns us an index (from 0) for which selection was chosen index, key = r.select(label,options) #runs the command located in our commands list, using the index from our rofi command os.system(commands[index])
my personal script does a more complex version of this (and needs tidying bc it's hella messy lmao) but i hope some of this is useful ? and if not then lmk what else i can do to help :3
3 notes
·
View notes
Text
Learn Django the Right Way: A Complete Guide for Beginners
Django, a powerful and high-level web framework for Python, has become one of the most popular tools for building web applications. Known for its simplicity, flexibility, and scalability, Django allows developers to build sophisticated and secure websites in less time. If you're a beginner looking to dive into web development or you're familiar with Python but new to Django, this guide will walk you through the essential steps to learn Django Tutorial the right way.

Whether you're looking to build simple websites or complex web applications, Django is a great choice due to its comprehensive feature set. But before you jump into Django, it’s important to lay a solid foundation and understand the core concepts of the framework. In this guide, we’ll cover the basic steps for getting started with Django, including setting up your development environment, understanding key concepts, and building your first Django app.
Step 1: Understanding What Django Is
Django is an open-source web framework written in Python that follows the Model-View-Template (MVT) architectural pattern. It simplifies web development by providing developers with the tools to handle common tasks, such as database management, user authentication, and URL routing. By using Django, you can focus on building the unique features of your application while the framework takes care of many routine tasks.
The key benefits of Django include:
Rapid Development: Django’s "batteries-included" philosophy means that most of the components you need are already included, making it faster to build applications.
Security: Django helps protect your site by automatically managing security measures such as user authentication, password management, and protection against SQL injection.
Scalability: Django can scale to support high-traffic sites. It's been used by companies like Instagram, Pinterest, and Disqus, proving its ability to handle large-scale applications.
By mastering Django, you'll be able to create dynamic websites and web apps that are both powerful and efficient.
Step 2: Setting Up Your Development Environment
Before you can start using Django, you'll need to set up a Python development environment. This involves installing Python, Django, and a few additional tools to make development easier. While the installation process can vary depending on your operating system, Django’s official documentation provides clear instructions for Windows, macOS, and Linux users.
Once you have Python installed, the next step is to install Django. Using Python’s package manager, pip, you can easily install Django and get started with your first project. It's also recommended to use a virtual environment to isolate your project dependencies. This ensures that your project’s libraries and dependencies do not interfere with other Python projects on your machine.
After the installation is complete, you can verify that Django is correctly installed by running a simple command in your terminal. This will allow you to see the installed version of Django, confirming everything is set up correctly.
Step 3: Learning Key Concepts in Django
Before building any web application, it’s important to understand the core components of Django. These key concepts are fundamental to how Django operates, and understanding them will make the development process much smoother.
Project vs. App: In Django, a project is a collection of settings and configurations for a web application, while an app is a specific component or module within that project. For example, you could have a "Blog" app within your project, and it would handle all things related to blog posts.
Models: Models are Python classes that define the structure of your database. They represent the data of your application, such as users, blog posts, or products. Django uses models to automatically generate database tables and allows you to easily interact with the database through Python code.
Views: Views are functions that handle HTTP requests and return HTTP responses. They serve as the link between the data in your database and what users see in their web browser. Views control what information is displayed to users and can render templates to display HTML content.
Templates: Templates in Django are HTML files that allow you to separate the presentation of data from the underlying business logic. With Django’s templating engine, you can dynamically generate HTML content and insert data from your views and models.
URLs and Routing: Django uses a flexible URL routing system to map URLs to views. This allows you to define specific routes for different parts of your site and decide what content to display when users visit a particular URL.
Admin Interface: One of Django’s standout features is its built-in admin interface. This allows you to easily manage the data in your application through a user-friendly web interface, without having to manually interact with the database.
Step 4: Building Your First Django App
Once you understand the key concepts, you’re ready to build your first Django app. While the process might seem daunting at first, Django makes it easy for beginners to get started. The first step is creating a new Django project, which you can do by running a simple command in the terminal.
From there, you’ll need to create an app within your project. Each app can handle a specific piece of functionality for your site, such as user authentication or blog post management. You can define models for your app, create views to handle the data, and then use templates to display that data on a webpage.
One of the most exciting things about Django is that it takes care of many common tasks, such as form handling, database queries, and URL routing. This allows you to focus on developing the core functionality of your app rather than worrying about repetitive tasks.
As you build your app, you’ll learn how to manage data with Django’s powerful ORM (Object-Relational Mapping), create forms to accept user input, and set up URL patterns to control how users navigate your site.
Step 5: Going Further with Django
Once you’ve built your first Django app, there are several advanced topics you can explore to take your skills to the next level. Some of the most important topics to dive into include:
User Authentication and Permissions: Django comes with built-in support for user authentication, allowing you to easily implement features like login, registration, and password management.
Working with Databases: Django’s ORM provides a powerful way to interact with databases without writing raw SQL. You can create, read, update, and delete records through Python code.
Building APIs with Django Rest Framework: If you’re interested in building APIs, Django Rest Framework (DRF) is a great tool to help you create RESTful APIs for your Django applications.
Testing: Django makes it easy to write unit tests for your application to ensure that everything is working as expected. Testing is an essential part of the development process and helps catch bugs early.
Deployment: Once you’ve finished building your app, the final step is deploying it to a live server. Django supports deployment to various platforms, including cloud services like Heroku and AWS.
Step 6: Keep Practicing and Building Projects
The best way to master Django is through practice. Building real-world projects is the fastest way to improve your skills and gain confidence. Whether it's a personal blog, an e-commerce site, or a social media platform, the more you build, the more you'll learn.
By working on different projects, you’ll also encounter challenges and problems that will help you grow as a developer. Don’t be afraid to experiment, seek out tutorials, and learn from the Django community.
Conclusion
Learning Django is an exciting and rewarding journey. With its comprehensive features and ease of use, Django Tutorial allows you to create powerful web applications quickly and efficiently. By following this guide and building real projects, you’ll be on your way to becoming a proficient Django developer. Remember, practice is key, so keep coding, keep building, and enjoy the process of learning.
0 notes
Text
What You’ll Learn in a Data Analyst Course in Noida: A Complete Syllabus Breakdown

If you are thinking about starting a career in data analytics, you’re making a great decision. Companies today use data to make better decisions, improve services, and grow their businesses. That’s why the demand for data analysts is growing quickly. But to become a successful data analyst, you need the right training.
In this article, we will give you a complete breakdown of what you’ll learn in a Data Analyst Course in Noida, especially if you choose to study at Uncodemy, one of the top training institutes in India.
Let’s dive in and explore everything step-by-step.
Why Choose a Data Analyst Course in Noida?
Noida has become a tech hub in India, with many IT companies, startups, and MNCs. As a result, it offers great job opportunities for data analysts. Whether you are a fresher or a working professional looking to switch careers, Noida is the right place to start your journey.
Uncodemy, located in Noida, provides industry-level training that helps you learn not just theory but also practical skills. Their course is designed by experts and is updated regularly to match real-world demands.
Overview of Uncodemy’s Data Analyst Course
The Data Analyst course at Uncodemy is beginner-friendly. You don’t need to be a coder or tech expert to start. The course starts from the basics and goes step-by-step to advanced topics. It includes live projects, assignments, mock interviews, and job placement support.
Here’s a detailed syllabus breakdown to help you understand what you will learn.
1. Introduction to Data Analytics
In this first module, you will learn:
What is data analytics?
Why is it important?
Different types of analytics (Descriptive, Diagnostic, Predictive, Prescriptive)
Real-world applications of data analytics
Role of a data analyst in a company
This module sets the foundation and gives you a clear idea of what the field is about.
2. Excel for Data Analysis
Microsoft Excel is one of the most used tools for data analysis. In this module, you’ll learn:
Basics of Excel (formulas, formatting, functions)
Data cleaning and preparation
Creating charts and graphs
Using pivot tables and pivot charts
Lookup functions (VLOOKUP, HLOOKUP, INDEX, MATCH)
Conditional formatting
Data validation
After this module, you will be able to handle small and medium data sets easily using Excel.
3. Statistics and Probability Basics
Statistics is the heart of data analysis. At Uncodemy, you’ll learn:
What is statistics?
Mean, median, mode
Standard deviation and variance
Probability theory
Distribution types (normal, binomial, Poisson)
Correlation and regression
Hypothesis testing
You will learn how to understand data patterns and make conclusions from data.
4. SQL for Data Analytics
SQL (Structured Query Language) is used to work with databases. You’ll learn:
What is a database?
Introduction to SQL
Writing basic SQL queries
Filtering and sorting data
Joins (INNER, LEFT, RIGHT, FULL)
Group By and aggregate functions
Subqueries and nested queries
Creating and updating tables
With these skills, you will be able to extract and analyze data from large databases.
5. Data Visualization with Power BI and Tableau
Data visualization is all about making data easy to understand using charts and dashboards. You’ll learn:
Power BI:
What is Power BI?
Connecting Power BI to Excel or SQL
Creating dashboards and reports
Using DAX functions
Sharing reports
Tableau:
Basics of Tableau interface
Connecting to data sources
Creating interactive dashboards
Filters, parameters, and calculated fields
Both tools are in high demand, and Uncodemy covers them in depth.
6. Python for Data Analysis
Python is a powerful programming language used in data analytics. In this module, you’ll learn:
Installing Python and using Jupyter Notebook
Python basics (variables, loops, functions, conditionals)
Working with data using Pandas
Data cleaning and transformation
Visualizing data using Matplotlib and Seaborn
Introduction to NumPy for numerical operations
Uncodemy makes coding easy to understand, even for beginners.
7. Exploratory Data Analysis (EDA)
Exploratory Data Analysis helps you find patterns, trends, and outliers in data. You’ll learn:
What is EDA?
Using Pandas and Seaborn for EDA
Handling missing and duplicate data
Outlier detection
Data transformation techniques
Feature engineering
This step is very important before building any model.
8. Introduction to Machine Learning (Optional but Included)
Even though it’s not required for every data analyst, Uncodemy gives you an introduction to machine learning:
What is machine learning?
Types of machine learning (Supervised, Unsupervised)
Algorithms like Linear Regression, K-Means Clustering
Using Scikit-learn for simple models
Evaluating model performance
This module helps you understand how data analysts work with data scientists.
9. Projects and Assignments
Real-world practice is key to becoming job-ready. Uncodemy provides:
Mini projects after each module
A final capstone project using real data
Assignments with detailed feedback
Projects based on industries like banking, e-commerce, healthcare, and retail
Working on projects helps you build confidence and create a strong portfolio.
10. Soft Skills and Resume Building
Along with technical skills, soft skills are also important. Uncodemy helps you with:
Communication skills
Resume writing
How to answer interview questions
LinkedIn profile optimization
Group discussions and presentation practice
These sessions prepare you to face real job interviews confidently.
11. Mock Interviews and Job Placement Assistance
Once you complete the course, Uncodemy offers:
Multiple mock interviews
Feedback from industry experts
Job referrals and placement support
Internship opportunities
Interview scheduling with top companies
Many Uncodemy students have landed jobs in top IT firms, MNCs, and startups.
Tools You’ll Learn in the Uncodemy Course
Throughout the course, you will gain hands-on experience in tools like:
Microsoft Excel
Power BI
Tableau
Python
Pandas, NumPy, Seaborn
SQL (MySQL, PostgreSQL)
Jupyter Notebook
Google Sheets
Scikit-learn (Basic ML)
All these tools are in high demand in the job market.
Who Can Join This Course?
The best part about the Data Analyst Course at Uncodemy is that anyone can join:
Students (B.Tech, B.Sc, B.Com, BBA, etc.)
Fresh graduates
Working professionals looking to switch careers
Business owners who want to understand data
Freelancers
You don’t need any prior experience in coding or data.
Course Duration and Flexibility
Course duration: 3 to 5 months
Modes: Online and offline
Class timings: Weekdays or weekends (flexible batches)
Support: 24/7 doubt support and live mentoring
Whether you’re a student or a working professional, Uncodemy provides flexible learning options.
Certifications You’ll Get
After completing the course, you will receive:
Data Analyst Course Completion Certificate
SQL Certificate
Python for Data Analysis Certificate
Power BI & Tableau Certification
Internship Letter (if applicable)
These certificates add great value to your resume and LinkedIn profile.
Final Thoughts
The job market for data analysts is booming, and now is the perfect time to start learning. If you’re in or near Noida, choosing the Data Analyst Course at Uncodemy can be your best career move.
You’ll learn everything from basics to advanced skills, work on live projects, and get support with job placement. The trainers are experienced, the syllabus is job-focused, and the learning environment is friendly and supportive.
Whether you’re just starting or planning a career switch, Uncodemy has the tools, training, and team to help you succeed.
Ready to start your journey as a data analyst? Join Uncodemy’s Data analyst course in Noida and unlock your future today!
0 notes