#React Native course
Explore tagged Tumblr posts
Text
React JS form validation
Introduction:
Form validation in React JS & Native Course ensures that the user inputs correct and complete information in your forms before submitting them. Here’s a simple guide to help you understand how to add validation to your forms in React.
Why Validate Forms?
Validating forms helps you:
Ensure the user inputs the required information.
Prevent incorrect or incomplete data submission.
Improve the user experience by guiding users on how to fill out the form correctly.
Setting Up Your Form
Let's create a basic form with fields for name and email. We will also add simple validation to check if these fields are filled out correctly.
Create the Form Component
First, create a new component for your form:
// Form.js import React, { useState } from 'react';
function Form() { const [name, setName] = useState(''); const [email, setEmail] = useState(''); const [errors, setErrors] = useState({});
const validate = () => { let formErrors = {}; if (!name) formErrors.name = "Name is required"; if (!email) formErrors.email = "Email is required"; else if (!/\S+@\S+.\S+/.test(email)) formErrors.email = "Email is invalid"; return formErrors; };
const handleSubmit = (e) => { e.preventDefault(); const formErrors = validate(); if (Object.keys(formErrors).length === 0) { console.log("Form submitted successfully!"); // Process form data here } else { setErrors(formErrors); } };
return ( Name:
setName(e.target.value)} /> {errors.name &&
{errors.name}} Email:
setEmail(e.target.value)} /> {errors.email &&
{errors.email}} Submit ); }
export default Form;
How It Works
State Management: We use useState to manage the state of the form inputs (name and email) and any validation errors.
Validation Function: The validate function checks if the fields are filled out and if the email is in the correct format.
Handle Submit: The handleSubmit function is called when the form is submitted. It prevents the default form submission, validates the inputs, and sets errors if there are any.
Displaying Errors: If there are validation errors, they are displayed below the respective input fields.
Adding More Validations:
You can add more validation rules as needed. For example, to check if the name is at least 3 characters long:
if (name.length < 3) formErrors.name = "Name must be at least 3 characters long";
To check if the email is in the correct format:
if (!/\S+@\S+.\S+/.test(email)) formErrors.email = "Email is invalid";
Summary:
Form validation in React JS helps ensure users fill out forms correctly before submitting. By using state to manage input values and validation errors, you can guide users to enter the right information. This simple setup can be expanded with more complex validation rules as needed.
Start adding validation to your React forms to improve data accuracy and user experience!
#react course#react js online training#React Native course#React js online Training#react native#react developer#teacher#reactjs#developer#javascript#web developers#web designers#web developemnt#React js training in Hyderabad#React Online React Training#placement oppurtunites#job support#Form validation#React JS Forms
0 notes
Text
React Native Certification
Validate expertise in cross-platform mobile app development with React Native. Essential for mobile developers seeking proficiency in building robust applications for both iOS and Android platforms.
0 notes
Text
Top 13 Best React Native Courses for Beginners to Learn online in 2024
As technology continues to advance, mobile app development remains a thriving field, with React Native standing out as a popular framework for building cross-platform applications. For beginners eager to delve into React Native development, choosing the right course is crucial. In this blog post, we’ll explore the top 13 React Native courses for beginners available online in 2024.
#react native course#react native course for beginners#react native courses 2024#best react native courses
0 notes
Text
Learn React Native with Appwars Technologies – Your Gateway to Mobile App Development
In today's fast-paced digital era, mobile app development is a skill that opens doors to countless opportunities. One of the key technologies driving this field is React Native. This powerful framework allows developers to build cross-platform mobile applications with ease, combining the efficiency of web development with the performance of native apps.
Why Choose React Native Training?
React Native offers a unique advantage by enabling developers to write code once and deploy it on both iOS and Android platforms. This not only saves time and effort but also ensures a consistent user experience across different devices. Learning React Native provides a solid foundation for anyone aspiring to be a proficient mobile app developer.
What You Will Learn:
Our React Native training program at Appwars Technologies is designed for beginners and intermediate developers alike. You will start with the fundamentals of React Native, understanding its core concepts and components. As you progress, you'll delve into advanced topics, including navigation, state management, and integrating third-party libraries.
Hands-On Experience:
At Appwars Technologies, we believe in a hands-on learning approach. Our training includes practical exercises and real-world projects, allowing you to apply your knowledge and build a portfolio of impressive mobile applications. You'll receive guidance from experienced instructors who are passionate about helping you succeed in your mobile development journey.
Why Appwars Technologies?
Choosing the right institute for your React Native training is crucial, and Appwars Technologies stands out for several reasons. Our courses are designed to be beginner-friendly, ensuring that even those with no prior coding experience can grasp the concepts easily. We focus on providing a supportive learning environment, fostering a community of aspiring developers.
Conclusion:
Embark on your React Native journey with confidence by choosing Appwars Technologies. Whether you dream of creating innovative mobile apps or enhancing your skills as a developer, our comprehensive training program is your stepping stone to success. Join us and unlock the doors to a world of possibilities in mobile app development.
0 notes
Text
React Native Course
If you’re curious about mobile app development, consider enrolling in a React Native Course to understand how to make effective applications for both Android and iOS platforms employing a single codebase.
0 notes
Text
ReactJS and the Technology World - A Perfect Fit
When you are on the lookout of a programming tool for the purpose of development of the frontend portion of a web application, then ReactJS should be the perfect fit for your purpose. ReactJS has been used for the development purpose of millions of websites worldwide. Thousands of businesses have used this technology successfully.
Use of JavaScript - An Added Advantage
There are several benefits associated with the use of ReactJS and one of the most important of them happens to be the language it uses which is JavaScript and JavaScript is the most popular programming language that is in use in the world. This makes ReactJS an easy to learn as well as easy to use technology and it also ensures that the performance of the web applications is improved to a great extent. It also brings about improvements associated with interoperability.
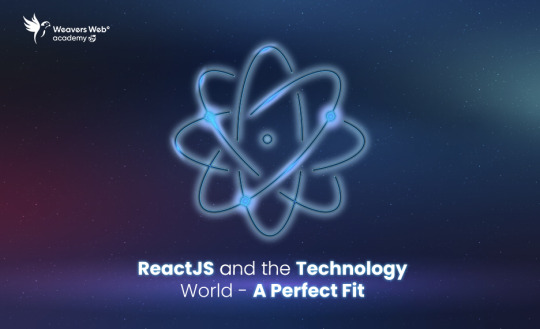
The Creation
Jordan Walke, a Facebook engineer created ReactJS in the year 2011. The main purpose behind the creation was the development of an open-source library based on JavaScript that would facilitate the development of the front-end part of the web application. At that point of time, the engineers at Facebook needed a library that would be enabled by JavaScript and would allow them to run at once several user interfaces for instance updates associated with chat as well as news feed.
The library was a major success and it happens to be a technology that is used more often today for the purpose of website development. ReactJS receives strong support from Facebook and also from the big community of users. The programming world today recognizes the worth of ReactJS.
The Need of the Hour
It is just the right time to enrol in the best ReactJS training institute and acquire the skills and knowledge of this technology by availing the ReactJS course. This is a much coveted course today and skilled ReactJS developers are in high demand in the IT industry.
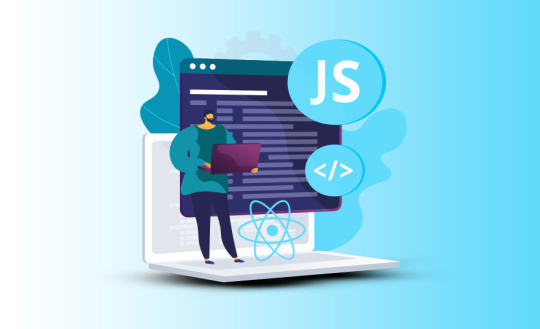
Some Great Advantages
ReactJS helps to foster the process of UI development and it makes use of shortcut ways and UI components that are reusable. ReactJS is capable of managing the view layer of the frontend applications of the web as well as mobile applications.
ReactJS helps in the development of applications that never compromise on quality and consume less time. Hence it is advantageous for both developers and customers.
Getting a strong grasp
It would be even more advantageous if you go in for the ReactJS certification training course to get a grasp over the technology working behind it.
Benefiting the developers in a number of ways
No extra efforts are required during the process of updating the user interface. ReactJS renders components that are reusable and which developers can reuse for the creation of a new application. This leads to reduction in the effort involved as the components used for some other application, having similar functionality can be reused.
The fine blending of JavaScript as well as HTML syntax leads to the simplification of the whole process associated with writing of codes for the project that is concerned.
Meta stays behind the back to extend support and also the community of developers all over the world keeps providing the much needed support whenever the need arises. This happens to be a great advantage for anybody or business using ReactJS in their project.
ReactJS plays an important part in reducing the loading times. Thus its role in making the application absolutely SEO friendly cannot be overlooked.
Conclusion
Having said that, we see how ReactJS is helping the programmers out immensely by solving critical problems and giving perfect solutions. The technology world has been benefited to a great extent and it can be considered to be nothing but a boon given by Meta to the developers all around the world.
0 notes
Text
lmfao
#“hes part native” blah blah blah its still very shaky evidence whether hes actually even a little bit native or not#either way sokka is supposed to be dark skin and visibly native which wouldve avoided this issue in the first place#its funny tho casting directors will hire a kid whos part white part poc but u think theyd even considered someone. like. black and native?#or a blasian? no. absolutely not of course.#this whole sokka sexism thing is so funny#im glad majority of the ppl are reacting negatively as they should#atla live action#atla#twitter#queue#natla
41 notes
·
View notes
Text
Professional React Native App Development – Reptile IT Service
React Native, a popular framework developed by Facebook, allows developers to build native-like mobile apps using a single codebase for both iOS and Android platforms. Reptile IT Service offers professional React Native App Development services, delivering fast, responsive, and feature-rich mobile applications that enhance user experience and drive business growth.
Whether you need a new app, a redesign, or an upgrade, their experienced developers are ready to create a solution that meets your needs. Contact Reptile IT Service today to get started!
0 notes
Text
What Is React Native? A Beginner’s Guide
In case you have ever puzzled how your favorite apps run nicely on both iOS and Android it is likely that React Native had a role. You will hear the term "React Native" a lot in IT circles, but exactly what is React Native and why is it so important? This article will explain everything in plain language, regardless of if you are a beginner developer or someone who loves technology.
What Is React Native?
React Native is an open framework developed by Facebook that lets developers create mobile apps using JavaScript. But here is the trick: By choice of building separate apps for iOS and Android React Native enables developers to write the code once and use it across both platforms. Think of it as the Swiss Army knife for app development efficient versatile and saves a ton of effort.
It launched in 2015 React Native fastly gained popularity because it bridges the gap in native app development or web development. It is based on React a JavaScript library used for building user interfaces but customized for mobile platforms.
How Does React Native Work?
Here is where the magic happens. React Native does not exactly create a single app that runs on both iOS and Android; instead it uses a combination of JavaScript and native code (the stuff specific to each platform) to create apps that feel native.
When you use React Native you are essentially writing JavaScript code that interacts with native APIs. For example if you want to access a phone’s camera or GPS React Native taps into the device’s native capabilities. Behind the scenes it uses a “bridge” to communicate between the JavaScript and native code. This approach allows for high performance and a user experience that feels truly native.
Why Do Developers Love React Native?
Now that we have got the technical jargon out of the way let’s talk about why developers and businesses are head over heels for React Native.
Cross Platform Development:- This is the big one. With React Native developers can write code once and use it for both iOS and Android apps. It is a big time saver and cuts down on the costs of maintaining separate codebases.
Hot Reloading:- Imagine tweaking your app’s design and seeing the changes instantly no need to recompile the entire app. React Native’s hot reloading feature makes this possible which speeds up development and keeps the creative juices flowing.
Rich Ecosystem and Community:- React Native has a massive community of developers who contribute libraries tools and tutorials. Whether you are stuck on a problem or looking for a plugin to add a feature chances are someone has already built a solution.
Native Like Performance:- While it is not 100% the same as building a fully native app React Native gets pretty darn close. The apps feel smooth responsive and “native” enough that most users would not notice the difference.
Backed by Big Names:- React Native is used by tech experts like Facebook, Instagram Airbnb (before they moved away) and even Tesla. If it is good enough for them it is probably good enough for your project too!
What Are the Downsides?
Of course React Native is not all sunshine and rainbows. Any technology it has its quirks and limitations:-
Performance Limitations:- For apps that require heavy animations or complex functionalities fully native development might still be a better option.
Learning Curve:- If you are coming from a purely web development background understanding native modules and platform specific nuances can take some time.
Dependency Management:- Relying on third party libraries can sometimes lead to headaches specially if those libraries are not actively maintained.
Who Should Use React Native?
React Native is perfect for:-
Startups:- With limited resources startups can save time and money by developing apps for both platforms simultaneously.
Web Developers Transitioning to Mobile:- If you already know JavaScript and React moving into React Native is a logical next step.
Companies Needing Faster Development:- Businesses that want to roll out updates or test features quickly can benefit from React Native’s speed and flexibility.
However if you are building a highly specialized app (like a high performance game or one with intricate animations) native development might still be the way to go.
Getting Started with React Native
Ready to dip your toes into the React Native pool? Here is a quick roadmap to get started:-
Set Up Your Environment:- Install Node.js a code editor like VS Code and the React Native CLI.
Create Your First App:- Use npx react native init YourAppName to generate a basic project structure.
Test on Simulators:- Run your app on an iOS or Android emulator to see it in action.
Start Building:- Explore React Native components like and to build your UI.
Dive Deeper:- Learn how to handle navigation manage state with libraries like Redux and integrate native modules through React Native Online Training.
Final Thoughts
When it comes to app development React Native is unique. It integrates the best of both web and mobile making it a excellent choice for designing robust cross platform apps. If there are certain difficulties or the advantages continuously increase the disadvantages and the particularly for small teams and businesses trying to save costs.
So if you are someone who’s curious about app development but feels intimidated by the idea of mastering both iOS and Android platforms React Native might just be your new best friend. Grab your laptop install React Native and start building you might be surprised at how quickly you can create something amazing!
You can also read: React Native Interview Questions
0 notes
Text
Software Development Training Institute In Bangalore
Techaedu best software training institute in Bangalore offering online & classroom training courses on software development, Python, ML, AI, Digital Marketing, Full Stack Developer, React Native etc. Visite Websitehttps://www.techaedu.com/ Mobile number: 8867 746 186
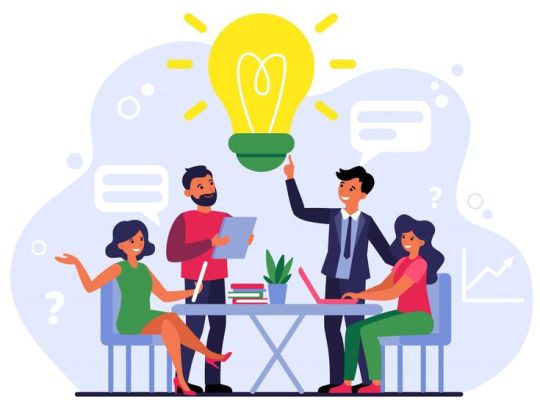
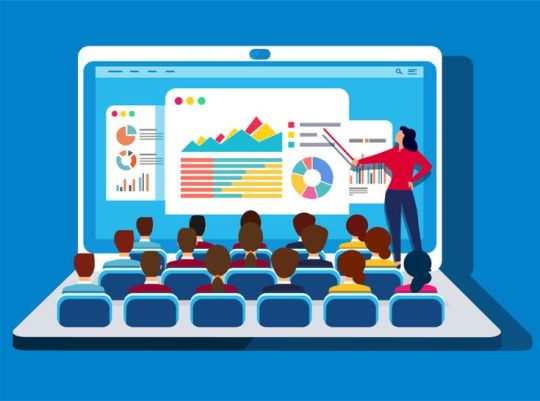
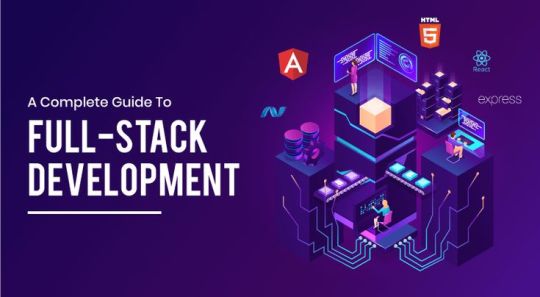
#Software development training#Full stack development course#UI/UX design training#React Native development#Digital marketing certification
0 notes
Text
React Native Reanimated Installation
React Native Reanimated is a powerful library that creates smooth, complex animations in React Native applications.
This article walks you through its installation process while covering essential topics related to React Native development.
What is React Native?
React Native is a popular open-source framework created by Meta (formerly Facebook) that allows developers to build mobile applications for iOS and Android using JavaScript and React.
With React Native, you can create cross-platform apps with a single codebase, reducing development time and cost.
Why is React Native Good?
Cross-Platform Development: Write once, run on both iOS and Android.
Large Community Support: React Native has a vast and active developer community for support.
Reusable Components: React Native allows code reuse, making development efficient.
Performance: By utilizing native components, React Native ensures near-native performance.
Integration with Native Code: You can integrate React Native with existing native apps and third-party libraries.
What is React Native Gesture Handler?
React Native Gesture Handler is an essential library for handling touch gestures in React Native applications. It provides advanced gesture recognition and makes animations and interactions smoother and more intuitive.
Why Use React Native Gesture Handler?
It reduces the complexity of handling gestures in mobile apps.
Works seamlessly with libraries like React Native Reanimated to create fluid animations.
Offers better performance compared to React Native’s built-in gesture system.
Using React Native CLI for Development
React Native CLI is the official tool for creating and managing React Native projects. It provides full control over the development process.
How to Install React Native Using CLI
Install Node.js: Download and install Node.js from the official website.
Install React Native CLI:bashCopyEdit
npm install -g react-native-cli
3. Create a New Project :
react-native init MyProject
4. Start the Development Server:
cd MyProject npx react-native run-android
React Native Reanimated Installation
React Native Reanimated is a library designed to handle complex animations with smooth performance. Follow these steps for installation:
Step 1: Install Dependencies
Ensure you have React Native and React Native Gesture Handler installed:
npm install react-native-gesture-handler react-native-reanimated
Step 2: Configure Babel Plugin
Add the react-native-reanimated/plugin to your babel.config.js file:
module.exports = { presets: ['module:metro-react-native-babel-preset'], plugins: ['react-native-reanimated/plugin'], };
Step 3: Link Native Modules
For React Native versions below 0.60, use:
react-native link react-native-reanimated
Step 4: Rebuild the Project
Run your project after installation:
npx react-native run-android npx react-native run-ios
Common Issues During Installation
Babel Plugin Errors: Ensure the plugin is correctly added to babel.config.js.
Build Failures: Clear the cache using:
npm start --reset-cache
Dependency Mismatch: Verify compatibility between React Native, Reanimated, and Gesture Handler versions.
Final Thoughts
React Native Reanimated is a must-have library for developers aiming to create visually appealing and interactive mobile applications.
When paired with React Native Gesture Handler, it elevates the user experience with smooth animations and responsive gestures.
Start exploring React Native Reanimated today, and bring your app’s animations to life!
#reactjs#react js online training#reactjscourse#reactnative#react course#react native#web design#web development#reactnativecourse
1 note
·
View note
Text
Hybrid Mobile Application Development Online Course In React Native
Unlock the world of mobile app creation with our Hybrid Mobile Application Development online course in React Native. Master the art of building cross-platform apps seamlessly, blending the power of React and native capabilities. Elevate your skills, design sleek interfaces, and embark on a dynamic journey in app development. Enroll now for hands-on learning and pave the way to a future in cutting-edge technology.
#Hybrid Mobile Application Development Online Course In React Native#React Native Mobile App Development Course#Hybrid Mobile Application Development Online Course#mobile application development react native online course#Hybrid Mobile Application Development Course In React Native#Hybrid Mobile Application Development Online Course In React Native In India#Hybrid Mobile Application Development Course with React Native
0 notes
Text
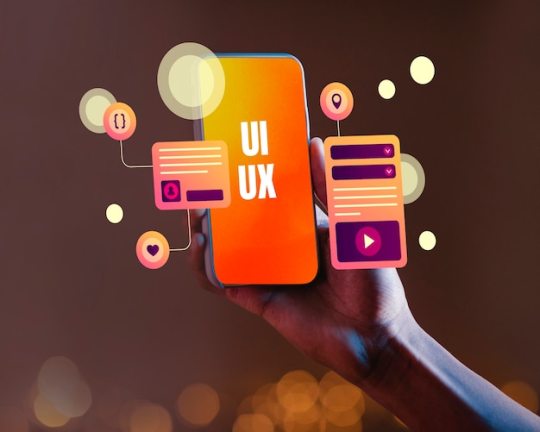
Navigating your way to the Top UI/UX Design Institute in Delhi
Discovering the best in the city is both challenging and rewarding in the digital era. Amidst the options, Dice Academy shines as an excellent choice for those aspiring to excel in UI/UX design. Uncover valuable insights by reading the article and navigate your way to excellence in UI/UX design courses. Choose Dice Academy for a journey where innovation meets expertise.
#ui ux design courses#web designing course in delhi#python full stack developer course#react native full course
0 notes
Text
this is a certified
Ñ
post
#as a native spanish speaker i want to point out the fact that they added an unnecessary “ah” sound at the end of their pronounciation#it's actually just pronounced “nyuh” by itself. of course if it has an a after it then yes it would be “nya”#but if it has an e then it's “nyeh” and if it has an i it's “nyee” (i is pronounced like “ee” in spanish)#and if it has an o it's “nyoh” and with a u it's “nyoo” (u is pronounced like “oo” in spanish)#(the long one like in food. not the short one like in book)#although i also wanna point out that the long “ah” sound doesn't exist in spanish. our “a” is pronounced more open and short#so you're not gonna hear a spanish guy say “nyah :3” when speaking#like you know those “a-” noises you put in front of words when you do an italian accent?#yeah that's how the letter a sounds in spanish#anyways it's really funny seeing people react to letters that aren't in there language like this lmao#shitpost#shitposting
939 notes
·
View notes
Text

Do you want to make a successful career in React Native? Shiv Tech Institute offers best React Native training in Ahmedabad. Enroll in our premier course today and become a certified developer.
React Native Fundamentals
Environment Setup
Application Design
Live Project Training If you join the course now, you get up to 25%* instant discount. Offer is valid for limited period only!
0 notes