#React Online React Training
Explore tagged Tumblr posts
Text
React JS form validation
Introduction:
Form validation in React JS & Native Course ensures that the user inputs correct and complete information in your forms before submitting them. Here’s a simple guide to help you understand how to add validation to your forms in React.
Why Validate Forms?
Validating forms helps you:
Ensure the user inputs the required information.
Prevent incorrect or incomplete data submission.
Improve the user experience by guiding users on how to fill out the form correctly.
Setting Up Your Form
Let's create a basic form with fields for name and email. We will also add simple validation to check if these fields are filled out correctly.
Create the Form Component
First, create a new component for your form:
// Form.js import React, { useState } from 'react';
function Form() { const [name, setName] = useState(''); const [email, setEmail] = useState(''); const [errors, setErrors] = useState({});
const validate = () => { let formErrors = {}; if (!name) formErrors.name = "Name is required"; if (!email) formErrors.email = "Email is required"; else if (!/\S+@\S+.\S+/.test(email)) formErrors.email = "Email is invalid"; return formErrors; };
const handleSubmit = (e) => { e.preventDefault(); const formErrors = validate(); if (Object.keys(formErrors).length === 0) { console.log("Form submitted successfully!"); // Process form data here } else { setErrors(formErrors); } };
return ( Name:
setName(e.target.value)} /> {errors.name &&
{errors.name}} Email:
setEmail(e.target.value)} /> {errors.email &&
{errors.email}} Submit ); }
export default Form;
How It Works
State Management: We use useState to manage the state of the form inputs (name and email) and any validation errors.
Validation Function: The validate function checks if the fields are filled out and if the email is in the correct format.
Handle Submit: The handleSubmit function is called when the form is submitted. It prevents the default form submission, validates the inputs, and sets errors if there are any.
Displaying Errors: If there are validation errors, they are displayed below the respective input fields.
Adding More Validations:
You can add more validation rules as needed. For example, to check if the name is at least 3 characters long:
if (name.length < 3) formErrors.name = "Name must be at least 3 characters long";
To check if the email is in the correct format:
if (!/\S+@\S+.\S+/.test(email)) formErrors.email = "Email is invalid";
Summary:
Form validation in React JS helps ensure users fill out forms correctly before submitting. By using state to manage input values and validation errors, you can guide users to enter the right information. This simple setup can be expanded with more complex validation rules as needed.
Start adding validation to your React forms to improve data accuracy and user experience!
#react course#react js online training#React Native course#React js online Training#react native#react developer#teacher#reactjs#developer#javascript#web developers#web designers#web developemnt#React js training in Hyderabad#React Online React Training#placement oppurtunites#job support#Form validation#React JS Forms
0 notes
Text
coworker called the trans flag the trans girl flag and kept insisting on it and i like. Ah little buddy you are so naive.
I clarified twice that just because your friend who is a trans woman has it everywhere does not mean its only transfem and upon bringing up the many MANY labels that exist and the many different flags ( vague mention ) the duty manager brought up how she found ‘top/bottom’ confusing and how it shouldnt be a label and.
Man. The shit i know. I am cursed (neutral) with knowledge of ithrrkin and micro identities and labels and these guys are at base 1 LMAO
#train rambles#i found that thing im insane about i guess#The whole talk for 30m straight? yea labels and identities is it LOL#makes me wonder how they would react to otherkin and such but i feel they’ll probably just not understand or think it wierd and move on#< not a bad thing#Its just i know coworker one is online so surely he’s heard of some of these labels right?#But confusing the trans flag with the transfemme flag.
2 notes
·
View notes
Text
Beatrix's 22M goes under Vane's 22U this game sucks
#her 22l and 22h don't go under it but 22u does because of course it does#but 22m what the hell lol#he can 2l or sweep to stop her but it's not like the shield is hard to react to#and it feels like i have to delay her 22m a lot to get hit#it's probably easier in training mode than it would be online idk how often this will happen#but uh yeah beatrix is really strong lol#sage speaks
1 note
·
View note
Text
Mastering React: A Comprehensive Guide to Building Dynamic Web Applications
In this in-depth React course, you'll embark on a journey to master one of the most popular JavaScript libraries for building user interfaces. Whether you're a beginner eager to dive into the world of front-end development or an experienced developer looking to enhance your skills, this course offers something for everyone.
Throughout the course, you'll learn the fundamentals of React, including JSX syntax, component-based architecture, state management, and handling user input. You'll explore advanced topics such as routing, hooks, context API, and working with external APIs. By the end, you'll have the skills and confidence to build dynamic, interactive web applications with React.
Key topics covered:
Understanding the Basics: Get started with React by learning about JSX, components, props, and state.
Building Reusable Components: Explore the power of component-based architecture and learn how to create reusable UI elements.
Managing State: Master the various techniques for managing state in React applications, including useState and useContext hooks.
Handling User Input: Learn how to handle user input through forms, controlled components, and form validation.
Routing with React Router: Dive into client-side routing with React Router and create single-page applications with multiple views.
Working with External Data: Connect your React applications to external APIs and learn best practices for fetching and displaying data.
Styling in React: Explore different approaches for styling React components, including CSS modules, styled-components, and inline styles.
Optimizing Performance: Discover techniques for optimizing the performance of your React applications, including code splitting and memoization.
Testing React Applications: Learn the basics of testing React components using popular testing libraries like Jest and React Testing Library.
Deployment and Beyond: Deploy your React applications to hosting platforms like Netlify or Vercel and explore additional resources for further learning.
By the end of this course, you'll have a solid understanding of React and be equipped with the skills to build modern, responsive web applications that delight users and impress employers. Whether you're pursuing a career in web development or working on personal projects, mastering React is sure to open doors and elevate your programming skills.
0 notes
Text
Functional Components and Hooks: The New Era of React
The world of web development continuously evolves, bringing forth new paradigms and methodologies that revolutionize how we build and interact with technology. Among these, React, a JavaScript library for building user interfaces, has been at the forefront of this evolution. At Softs Solution Service, a leading IT Training Institute in Ahmedabad, we're witnessing an exciting shift towards functional components and hooks, a change that's defining a new era of React development.
Understanding Functional Components and Hooks:
Gone are the days when Reacts class components were the go-to solution for stateful components. Functional components, initially introduced for stateless presentation purposes, have now taken center stage with the introduction of hooks in React 16.8. This update has significantly streamlined the process of writing components, making it more intuitive and efficient.
Functional components are JavaScript functions that return React elements. They’re simpler, easier to test and maintain, and reduce the amount of boilerplate code developers have to write. With hooks, these functional components can now manage state, side effects, context, and more, which were once only possible with class components.
The Benefits of Functional Components and Hooks:
Simplicity and Readability: One of the core advantages of using functional components is their simplicity. They encourage writing smaller, reusable components that are easier to test and reason about. With hooks, we can use plain JavaScript functions instead of having to understand the 'this' keyword or bind event handlers in class components.
Performance Gains: Functional components can lead to performance improvements. Since they're just functions, they avoid some of the overhead that class components have. Hooks also help avoid nesting higher-order components or render props patterns, which can lead to a cleaner component tree and faster re-renders.
Sharing Non-UI Logic: Hooks make it simpler to share logic with state between components without changing your component hierarchy. Custom hooks can encapsulate non-UI logic, which can be reused across different components, enhancing code reusability and abstraction.
Streamlined Life-Cycle Management: With the introduction of the use Effect hook, it's now possible to handle side effects in functional components. This hook replaces several life-cycle methods from class components, making it easier to organize and manage side effects in one place.
Embracing the New Era with Training and Development
At Softs Solution Service, we understand the importance of keeping abreast with the latest developments in technology. That's why our React JS Development Course includes comprehensive training on functional components and hooks. We prepare developers to harness the full potential of React's modern features through our React JS Development Training, both in-person in Ahmedabad and through our Online React JS Development Course.
The React JS Development Course in Ahmedabad at Softs Solution Service is meticulously designed to guide you through the foundational concepts to advanced applications of React's latest features. Our curriculum is practical, industry-relevant, and tailored to meet the demands of today’s web development landscape.
Conclusion:
The shift to functional components and hooks in React signifies more than just a technical update; it represents a broader move towards a more efficient, intuitive, and scalable approach to building web applications. It's a testament to the adaptability and forward-thinking ethos that underpins the React community.
As developers, embracing these changes is not optional but essential for staying relevant in the fast-paced tech industry. At Softs Solution Service, we're committed to facilitating this transition, empowering our students with the skills and knowledge to succeed in this new era of React development.
Whether you are new to the field or looking to update your skills, now is the perfect time to dive into our React JS Development Course and master the art of building modern, reactive user interfaces with React. Join us to unlock your potential and carve your niche in the ever-evolving world of web development.
#it training institute#it training institute in Ahmedabad#react js development course#softs solution service#online react js development course
0 notes
Text
Unveiling the Marvels of React Courses: A Journey into Web Development Excellence
Embarking on a React Adventure: A Glimpse into the World of Web Development
The realm of web development is a fascinating journey, and at its heart lies the omnipresent React. Whether you're a novice exploring the vast universe of coding or a seasoned developer seeking to level up your skills, a best React course is the compass guiding you through this intricate landscape.
1. The Genesis of React: A Prelude to Mastery
The Birth of React: Where it All Began
In the mid-2000s, Facebook's need for a dynamic and responsive user interface birthed React. Developed by the social media giant, React quickly evolved into an open-source library transforming the way developers approach front-end development.
Why React? Understanding the Need for Specialized Courses
React courses have surged in popularity, providing a structured path for learners to grasp the intricacies of this JavaScript library. The question arises: Why React? The answer lies in its component-based architecture, making UI development efficient and modular.
2. Navigating the React Course Landscape: A Map for Success
Choosing the Right React Course: A Critical Decision
Selecting the ideal React course can be overwhelming with the myriad of options available. From online platforms to in-person workshops, each avenue presents a unique learning experience. The key lies in understanding your learning style and choosing a course that aligns with your goals.
Diving into the Fundamentals: What to Expect in a React Course
In the initial modules of a React course, learners delve into the fundamentals. Understanding JSX, components, and state management forms the bedrock of React proficiency. It's the phase where the abstract becomes tangible, laying a robust foundation for advanced concepts.
3. Mastering React: Beyond the Basics
State Management Mastery: Elevating Your React Prowess
Within a React course, mastering state management is a pivotal milestone. Grasping concepts like props, state, and the component lifecycle allows developers to create dynamic, data-driven applications.
Hooks: Unleashing the Power of React's Functional Components
In the middle segment of a React course, the spotlight often shifts to hooks. Introducing developers to useEffect, useState, and useContext, these powerful tools unlock the potential of functional components, amplifying code readability and maintainability.
4. Crafting Dynamic User Interfaces: Advanced React Techniques
Dynamic Routing: Navigating Through React Applications
Advanced React courses delve into dynamic routing, allowing developers to create seamless navigation experiences. Understanding libraries like React Router becomes essential in building applications with multiple views.
Stateful vs Stateless Components: Striking the Right Balance
An intricate dance unfolds between stateful and stateless components. A well-crafted React course elucidates when and how to deploy each, ensuring an optimal balance between dynamic functionality and efficient rendering.
5. Real-world Applications: Transcending Theoretical Knowledge
Project-Based Learning: Breathing Life into Concepts
The pinnacle of a React course often lies in project-based learning. Building real-world applications propels learners from theoretical understanding to practical mastery. This hands-on approach fosters creativity and problem-solving skills.
Collaborative Coding: The Social Dimension of React Courses
React courses, especially those offered online, often emphasize collaborative coding. Platforms like GitHub provide an arena for developers to engage, share insights, and collectively overcome coding challenges. This collaborative aspect mirrors the real-world dynamics of modern development teams.
6. Beyond the Course: Continuous Learning and Industry Integration
Staying Current: The Ever-evolving React Ecosystem
Completing a React course is not the end but the beginning of a perpetual learning journey. React's ecosystem evolves rapidly, with regular updates and the emergence of new libraries. Developers must embrace continuous learning to stay at the forefront of web development.
Industry Integration: Bridging the Gap Between Learning and Application
The ultimate litmus test of a React course lies in its ability to bridge the gap between theoretical knowledge and real-world application. Integrating industry best practices, understanding deployment strategies, and exploring performance optimization are crucial components of a well-rounded React education.
7. The Grand Finale: Flourishing in the Reactverse
Unleashing Your Potential: A Flourishing Future in React Development
As we near the end of our journey through the enchanting world of React courses, envision the limitless possibilities that unfold. Armed with a robust skill set, you're poised to embark on a flourishing career in React development, contributing to the ever-evolving landscape of web technologies.
In Conclusion: A Symphony of Learning and Development
In conclusion, a React course is not merely a learning endeavor; it's a transformative experience. From understanding the library's inception to crafting intricate user interfaces, each phase contributes to the symphony of web development mastery. As you traverse this path, remember: the journey is as valuable as the destination. Embrace every challenge, celebrate each milestone, and let the magic of React courses propel you towards boundless possibilities in the digital realm.
0 notes
Text
Learn React Interview Questions and answers through the blog
0 notes
Text
#react native courses#react js course in Kolkata#react native courses in Kolkata#react js course online#react js training
0 notes
Text
satoru who reads one online article on how to make his crush fall in love with him and is convinced that the best way to do so is to watch horror movies with you. he plans it all out in his head: you'll get scared and he'll wrap an arm around you and tell you that there's no reason for you to be afraid since he's with you and he'd never let anything bad happen to you. and afterwards, you'll fall asleep with your head on his shoulder or in his lap and realize you're so in love with him you never want to leave him.
only problem? he doesn't account for the fact that you may not get scared.
so when the time comes around and he's set everything up and presses play on the movie, he's so excited he can barely contain himself. he doesn't even watch the movie, his eyes are trained on you, waiting for the perfect moment when you cover your face with your hands and only peek at the screen.
only… that moment never comes. he sits through all of the one hour and fifty minutes that the movie runs and you don't get scared. you don't hide behind him. you don't even flinch at the loud noises and the ugly monster. but now the movie's over and realistically, he has no reason to keep you there.
“i think i'll have to leave now, satoru,” you say after a while, looking down at your phone. “it's getting late.”
he gives an awkward laugh. “hey, c'mon. it's not that late yet. let's watch another one!”
he bothers you until you sigh and agree because it's satoru and he'd never let you go otherwise. he bribes you by telling you that you can pick the movie this time around.
another thing he didn't account for in his plan is that he may end up being the one getting scared.
whatever hellish movie it is you put on, it is not up satoru's alley. when there's a particular bad jumpscare—which you don't react to either—he actually flinches hard, his arm wrapping around you shoulder. he buries his face against your neck with a pout on his lips.
this isn't how it was supposed to go! the stupid article didn't say anything about this!
but once you place your hand on his head and run your fingers through his hair, he realizes that this isn't so bad either. he spends the rest of the movie flinching and whining at every little thing just so he has an excuse to hang on tighter.
#◟ヾ drabble#jjk x gender neutral reader#jjk x male reader#jjk x y/n#jjk x you#jjk x reader#gojo x reader#gojo x you#gojo x y/n#gojo satoru x reader#gojo satoru x you#gojo satoru x y/n
2K notes
·
View notes
Text
I’m listening to you

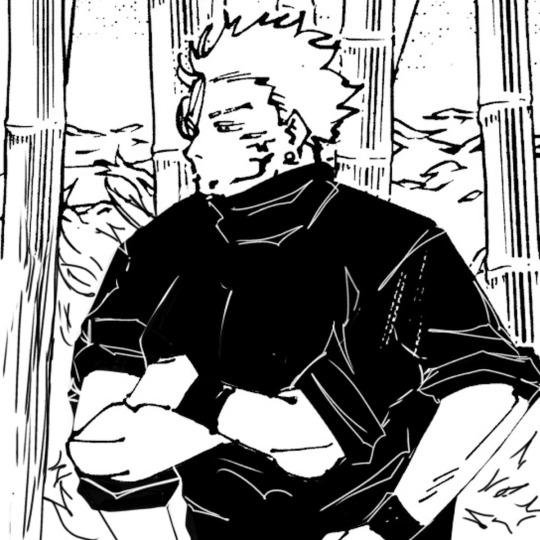

Including: Gojo, Nanami, Choso, Sukuna, Toji, Yuuji, and Megumi
Synopsis: You stop talking because you assume they aren’t listening… how silly of you, but how do they react?
my masterlist
〰・♡・〰〰・♡・〰〰・♡・〰〰・♡・〰〰・♡・〰
Satoru

It would probably be like any other day, he had probably just come back from a mission, laying across the couch and you would be in the kitchen making a snack board as you talked about numerous different things.
The conversation would shift through a multitude of topics as one thing would then reminded you of the next.
As you were getting the chips, you would explain the drama at work after that you would go and get some hummus while you explained the love life of one of your coworkers thereafter you would be reminded of a friend of yours from high school while piling up some cheese cubes.
Throughout all of this, there were very few breaks in your speech. When you finally came to the living room, Satoru would be facing towards you, with his head slightly tilted back on the armrest and his feet dangling off the other edge.
You could see him, just not very well. His blindfold would also be on, not an uncommon occurrence, but it would still be hard for you to see the slight changes in his facial features that typically depicted his emotions to you.
After awhile, you might start to wonder if he was feeling tired from the mission or if he just wasn’t paying attention. It’s okay, getting distracted happens to everyone sometimes.
Your words would taper off.
Believe me when I say it would not take this man any longer than say…five seconds for his head to raise and voice to pipe up,
“Well what happened next?”
And with a grabby hand he would add,
“Aren’t you gonna share those?”
Turning to point at the snacks in your lap.
For such a chatty guy, you would be surprised by how much he enjoyed just listening to others. Especially if it meant listening to you.
Nanami

Let’s be realistic here, this man would never take his undivided attention away from you.
That being said, the situation would be a little bit different with him. You guys would probably be out for coffee. Maybe the both of you had been busy for a while and felt the need to make a day out of catching up.
You know that embarrassing feeling when you get the notion you’ve been talking too much? It would be something like that.
You would have been gesturing and looking around the room while you talked about this and that. Unbeknownst to you, he would have hearts in his eyes while he listened to your ramblings.
That sudden itch would get to you though, the notion that, maaaaaaaaybe you have been talking about yourself too long.
One quick look at your man caused a shiver to run up your back at the eye contact.
There would be a pause in your speech, and he would nod at you to encourage more words to spill fourth, but now you were all flustered.
You would trip over your words, stuttering every so often before eventually apologizing.
“What is it, dear?”
He would ask, after a moment of you trying to collect yourself, he would assume that you had simply lost your train of thought and remind you of the topics you had been previously sharing.
You would thank him even though that wouldn’t have been the issue at all.
How could you not get shy when he was looking at you like that?
Choso

This would have to occur after a long day. Either during nap time or before you both went to bed.
He would be laying his head on your chest as you played with his freed hair. Talking about stuff you had seen online, talking about your plans for the next day, talking about what you were planning to eat for lunch tomorrow, the list could go on.
Every so often he would hum or nod his head. But after a while, you would look down and see that his eyes were closed.
It wasn’t hurtful, or embarrassing. It has been a long day for the both of you, and he was probably just tired. He often fell asleep easily when you combed through his hair with your nails. So you wouldn’t be offended as you go silent.
After you stop talking, though, he would grab your free hand and fiddle with your fingers, saying,
“I like those tacos too… we should get lunch together!”
You would giggle and tug on a lock of his hair, “I thought you were asleep.”
He would spin around onto his chest, chin pressed slightly into you while he looked up at your eyes through those big, long, lashes of his, “But you were talking to me?”
Sukuna
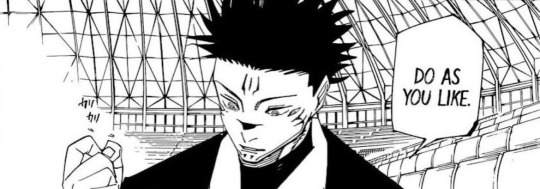
This man :| he would get offended if you insinuated that you could possibly be boring him.
Of course, it would be his fault, too. He would complain and whine all the time about how uninterested he was in your life. Griping that he had better things to do than just sit around and listen to you talk.
But don’t misunderstand, there was nothing he desired more than to watchyou speak about things that impassioned you.
But perhaps one of those instances you would take to heart, he was a busy man after all. It’s not like he had all the time in the world to listen to you yap about nonsense.
So after a long while in his chambers, you would just kind of go silent. ‘Allowing’ him a little time to himself.
Just earlier that day, you would have been telling him a story about one of your fellow workers at the estate. Sukuna had rolled his eyes at the incompetence of his staff as you giggled on about how a man got his hand stuck in a jar full of fertilizer.
You would take his disinterested countenance to assume that he was bored with you. For someone so ancient, he did have an attention span fitting to the times.
He always told you not to make assumptions about him, but after him asking you on several occasions, “and why are you telling me this?” You couldn’t help or conclude that he wasn’t all that interested.
I’ll tell you now. As much as he would like to pretend that was the case, it is far from the truth.
He would frown while laying on his bed. “Why did you stop?”
You would spin around, slightly surprised that he had even been paying attention. “Oh I just thought maybe I was boring you…”
That would cause him to sit up, scowling in your direction, “Did I say that?”
“Well…”
“Did. I. Say. That.”
“…no?”
Undeniably, sassy, he would splay out his arms in a “see??” type of motion. Waiting rather impatiently for you to continue on so he could relax to the sound of his lovers soothing voice while pretending to be impartial to it all.
Toji

This prick.
He wouldn’t do a thing lol
This man would let you assume he isn’t listening, let you think he’s off somewhere else, let you think he’s checked out.
But that could not be more wrong.
Maybe he’s eating his lunch, or watching tv, or texting someone. Whatever the case, there’s nothing he’s more locked into than your words.
In fact, it would take you a while into your relationship for you to realize this, but sometimes you would have to be careful around Toji because once you said something, this man would remember it forever.
You probably wouldn’t even think about it when you stopped talking, deciding to put your efforts into something else. But Toji would notice.
He wouldn’t bring it up though, not for days. But eventually he would crack the conversation back again, flipping the newspaper over and avoiding your eyes.
You would spin around on him, wondering how he even knew the things he was talking about. Then it would hit you.
“Wait… you were listening?”
He would scoff, elbows on the table, finally looking at you over his reading glasses. “Excuse me?” He would point an accusatory finger at you and set down the paper. “Was I listening?”
You would gape at him open mouthed, “Well… how was I supposed to know?”
He would roll his eyes in the most dramatic of fashions, getting up from the kitchen table to stroll over to you. “Please,” he would groan, grazing a knuckle over your neck,
“You’ve always got my attention.”
Yuuji

One might think this would happen early on in the relationship but I would argue this situation would happen after years together.
Why? Well, Yuuji is a super excitable guy, he also just loves talking to you. You both make a great pair because the two of you always converse in a way suitable to each other.
For example, sometimes you’d interrupt one another, never in a rude way, but in a way that shows passion about the topic at hand. That would bring on a whole new discussion and keep the conversation flowing.
Yuuji was a great listener when he needed to be but mainly he was a great conversationalist. Neither of you needed to do heavy lifting when you talked. It was great!
After awhile though, the two of you would become more and more comfortable around one another and more accustomed to the way you spoke.
That’s why it was so weird to see him less active in a conversation. He wasn’t interjecting with his little agreements or experiences. He would still be looking at you, but it was different.
It wasn’t out of the realm of possibility that he might just be uninterested in the topic so you would get all quiet, focusing on something else until he spoke up.
“No, keep talking.”
Grinning at you, he looked just like he always did.
“You’re not bored?”
He would squint, confused at the notion, “Why would you think that?”
“I don’t know, you just seem a little quiet I guess.”
But he would just smile at you, “I don’t know what it is… but recently I’ve been liking just admiring you.”
He would say things like that out of the blue all the time by the way.
“Yuuuuujiiiii” you would groan.
“What? Doesn’t mean I’m not listening!”
Megumi

Inversely to Yuuji, this would happen with Megumi early on in y’all’s relationship, likely pre-relationship when the two of you were just getting to know one another.
Megumi wasn’t really shy at all, he was more reserved, even though you weren’t all that talkative, he could still unintentionally make you feel like a blabbermouth at times.
The two of you would probably have been out on a walk together, or maybe in the cafeteria getting lunch, wherever, you would have been sharing some piece of yourself with him.
It would also probably have been a long time since he had spoke up. Sometimes he wouldn’t even give listening cues so it’s not too unusual to suppose that he was checked out.
Megumi was NOT checked out though. He was filing every little word you said away into his brain, and thinking of the best way to respond to you.
You might not know this though, so after awhile you might get all shy, suddenly looking off, fiddling with the hem of your shirt. Embarrassed that he hadn’t heard a word, that’s when he would turn to you,
“I’m listening.”
Simple as that.
Reassuring but not overly affectionate.
He would do it in public and in private. If you were in a group and he could tell your confidence was slipping he would jump in to let you know that he cared about your thoughts and opinions.
And like in this instance when it was just the two of you, he might reach over to grab your hand, letting you know,
“I’m still here.”
〰・♡・〰〰・♡・〰〰・♡・〰〰・♡・〰〰・♡・〰
#jjk hcs#jjk headcanons#jjk fluff#jjk angst#jjk comfort#gojo comfort#gojo headcanons#gojo imagine#nanami imagine#nanami comfort#nanami fluff#choso fluff#choso x you#choso imagine#jujutsu kaisen x reader#sukuna headcanons#sukuna x reader fluff#sukuna x you#toji x reader#toji imagine#toji fluff#toji x reader angst#sukuna x reader#yuuji x reader#yuuji fluff#megumi x reader#megumi fluff#jujutsu kaisen#gojo x reader angst#nanami x reader fluff
3K notes
·
View notes
Text
React Training
In a way, react is one of the most likable frameworks for web development and application-building purposes. If you also have an interest in knowing deep details about this advancement, you must take up the React Training and know its functioning.

0 notes
Text
#software training institute#python coding#it courses#certification#courses#online courses#phpcourse#mysql#artificial intelligence#flutter mobile app development company#ui/ux design#website design#digital marketing#react native#django#live projects#industry standards#affordable fees#startup#coaching
0 notes
Text
Best React JS Developer Online Course | Technovids
React JS Developer Online Course React JS Developer Online Course covers all the essential topics of React JS.
#corporate training#educational course#online courses#react js development company#react js developers
0 notes
Text
Happy International Yoga Day from Solitaire Infosys.
Make sure this summer you learn from the best. Solitaire Infosys is best coding and non-coding technologies internship provider in Mohali.
#python#6 month industrial training#digital marketing#industrial training in mohali#itcompany#training#coding#industrial training#internship#indstrial training#6 weeks industrial training in mohali#6 weeks industrial training in chandigarh#6 month industrial training in mohali#6 month industrial training in chandigarh#React js training in Chandigarh#web designing course in Chandigarh#Python training in Mohali#Python training institutes in Mohali#affiliate program management Services#online reputation management services#6 week internship in mohali#Best IT company in chandigrah#development course in mohali#Best IT companies in Mohali#App development in Mohali#Best IT Company in Mohali#enterprise resource planning maintenance#ionic app development company#wordpress theme development#website Development internship in Mohali
0 notes
Text
chaotic trio- k.antonelli, o.bearman, p.aron



�� ☆summary: how your best mates react when they see you're getting hate online
★ ☆pairing: reader x ollie bearman x kimi antonelli (kinda)x paul aron (all platonic)
skysportsf1


liked by haasf1team, kimiantonelli, and 778,249 others
skysportsf1 Triple Trouble back at it again! We're only a few races in and your favourite chaotic trio are causing mayhem on track @.yourusername @.kimiantonelli @.olliebearman
comments
user729 Y/N'S RADIOS WERE GOLDEN -> user264 'why's ollie not even racing me? is his car that bad?' -> user67 kimi: 'WHAT THE FUCK MATE WHY IS Y/N UP MY ASS?! HOW DID WE LET THIS HAPPEN?!' -> user720 y/n gets past him: 'i think i could hear him screaming there'
user60 her overtake on kimi was chef's kiss -> user764 On god it was so fire ⁉️
user6238 ollie racing esteban and having esteban make a mistake? SLAYYY KING GET HIM BACK!!! -> user8834 AVENGE ALONSO
user899 She even surprised lewis like damn -> user92 lewis: 'oh shit she's up here???'
yourusername I wish to not be grouped with these hooligans -> kimiantonelli We were literally on the podium together what do you mean? -> yourusername were you top step? -> olliebearman Were you? -> paularon clock it! 💯💯💯
premaracing I'm so glad other people have to deal with them now :) -> user35 ik whoever babysat them was TIRED
user009 y/ns overtakes were actual cinema where has she been for the past few years wtf.
user233 babes why are they my favourite rookies of all time. -> alexalbon same tbh
user98 I think we need to apologise to each of their teams because they must be so much to deal with -> redbullf1team we're so tired... -> hassf1team exhausted even... -> mercedesf1team kimi is perfect and does no wrong :) -> yourusername ho is you toto?
kimiantonelli Someone needs to cut her brakes fuck's sake -> yourusername don't know if you noticed but my car is already a shitbox and i had no brakes by the end of that fucking race, so please stop praying on my downfall and focus on your maths homework you fucking nerd 😜 -> redbullf1team Y/n. More media training. ->yourusername FUCKS SAKE KIMI THIS IS YOUR FAULT.
---------------
yourusername


liked by kimiantonelli, maxverstappen, and 1,345,354 others
yourusername stunted on those hoes. hashtag slay (kimi looks ugly on a podium but felt the need to post my best mate of 10 years when he finally makes it in f1, even if he's copying me)
comments
olliebearman hater ->yourusername alright mr.haas calm tf down i'll give you ur boyfriend back
kimiantonelli so sweet (not) -> yourusername this is as sweet as it gets girl enjoy it while it lasts 🕺
user892 they're so pookie
user233 FIRST MODERN FEMALE PODIUM IN F1 THANK YOU Y/N Y/L/N!!!!!!!!! -> liked by lewishamilton, maxverstappen, kimiantonelli, olliebearman, isackhadjar, liamlawson, jackdoohan, yukistunoda, logansargeant, danielriccardo, gabrielborteleto, paularon, lukebrowning, alexdunne and 692,424 others
oscarpiastri You really did stunt on those hoes (did I say that right?) -> yourusername i genuinely love u sm osc
landonorris HASHTAG SLAY
redbullf1team we will take ur account away girl. -> yourusername bold of you to assume I don't have a backup.
user889 DID YALL SEE THE SMILE ON OLLIE'S FACE?????
user8844 LEWIS'S INTERVIEW WAS SO CUTE ->user22 ??? -> user8844 he was just super proud of both kimi and y/n and it was adorable
user709 i'm actually sobbing i never thought i'd get to see a woman on the podium i'm so emotional and she's just posted the most nonchalant caption what the fuck
user0029 she's actually so goated did we see her overtake on alonso??? even he was impressed.
---------------
yourusername


liked by maxverstappen, oscarpiastri, kimiantonelli and 2,729,423 others
yourusername me and max when we realise we have to get back into the car for another fucking weekend with no updates still...
comments
maxverstappen 😹😹😹 ->user444 yes king laugh through the pain -> user234 fucking hell he's actually loosing it lol
olliebearman please stop with the complaints ms. podium ->yourusername ur just mad because kim hugged me first stfu🤷♀️ -> kimiantonelli I actually hate both of you so 😼
redbullf1team I'm TIRED y/n. you're explaining this to christian.
user66 god she's so annoying can she take anything seriously?
user673 women shouldn't be driving in f1 they should be getting coffee
user7724 she was so shit how did she even get a podium?
user777 this sport has gone to shit
user999 she's so fucking stupid
user7 i'm so hyped for race weekend just to watch her crash lol
user667 get her out of that seat i could probably drive better ffs
user72442 girls don't belong in motorsport
user63624 get this cunt out
user343 paddock bunny
user2213 probably fucked half the grid to get there lol -> user7889 she's barely legal what?? -> user2213 stfu
user7555 she's such an obvious marketing ploy
user8724 she's got no talent
user3123 slut
---------------
olliebearman


liked by yourusername, kimiantonelli, paularon and 824,243 others
olliebearman Some sun, some fun, it's all inside this happy meal :) @.kimiantonelli @.yourusername @.paularon @.arthurleclerc
comments
user244 why do they even invite her anymore?? ->user67 bc they're mates???????
user789 wow they've all had a turn lol -> user7920 you're such a pig
charlesleclerc Be safe kids 🫡 -> user729 i love how they're still keeping the adoption jokes going
user2345 him and kimi were soooo drunk
user576 i actually adore this group so much
paularon forgot to add the photo of my gorgeous biceps... -> olliebearman sorry i just made me vomit so i thought better not to
user638 she's so random in that group ewww
user8899 does she have any other friends she can leach off of???
user8978 she's a slut
yourusername take this shit down buddy 👎 -> kimiantonelli just because he posted me and him doesn't mean he doesn't love you -> user57588 why's she such a bitch????
user399 proud y/n hater ->user35 fr she's such a bop
user423 ran through ->user3445 you're disgusting
---------------
kimiantonelli's story

[caption: congrats to the winner ig... @.yourusername]
dms
user553 she's such a slut leave her die
user939459 she's run through
user42 send me her nudes
user244 she's such a bitch
user523 paddock bunny
yourusername please take this down kim kimiantonelli why? yourusername please just take it down kimiantonelli alright, sorry :( yourusername thank you, sorry.
---------------
paularon



liked by olliebearman, kimiantonelli, and 983,234 others
paularon Out and about recently :) @.yourusername @.kimiantonelli @.olliebearman
comments
kimiantonelli ngl those smoothies were mega
olliebearman dinner was so good
user35 why do they hang with her???
user89 free them from her grasp
user989 biggest slut in the paddock and she's up against charles leclerc
user139 shittest driver since mazepin
user766 blonde bimbo
user345 i'm so tired of pretending to like her GOD ->user399 why don't u like her? -> user345 women shouldn't be in motorsport. end of conversation.
user3554 she's so annoying just leave kimi and ollie alone! -> user89 fr she's probably fucking both tho lmao
user6789 every guy in the paddock has fucked her haven't they lmao
user573 christian horny's pet probably
user233 she's my fav driver this is my fav group -> user689 ur stupid if she's ur fav
user7832 can't wait to see her crash
user7909 why is everyone hating???? -> user65 bc men hate to see a woman doing well!
user4333 exhausted by all of these insecure men
user34 did yall see kimi's deleted story too? i fear a friendship break-up might be going on behind the scenes ->user8999 PLEASE NO🙏🙏🙏 ---------------
redbullf1team



likedby yourusername, maxverstappen, kimiantonelli, olliebearman, and 2,234,423 others
redbullf1team Top step for our no.19 :)
comments are limited
maxverstappen Wonderful drive.
lewishamilton SO proud.
olliebearman No, I wasn't crying. There was something in my eye. -> kimiantonelli fr. there was something in his eye.
fernandoalonso Vamos!
charlesleclerc It was an honour to be on the podium with her :)
georgerussell Blimey what a race! Incredible drive from herself :)
---------------
kimiantonelli



liked by olliebearman, maxverstappen, landonorris and 824,243 others
kimiantonelli our best mate just won a race!?
comments
user8923 slut
paularon SLAYYYED
landonorris i thought she was my best mate?
user80 i hope she crashes and burns
user43 no women in motorsport
user23 she's so inspirational it's insane
user11 die
user32 they're both playing her i bet
user90 she's so bad why are we glazing her
user87 CHEATER
user789 omg if they wanted to they would this is so cute
---------------
messages
yourusername Hey guys, I'm deleting insta and I probs won't be super in touch just because everything is going mad in redbull so I won't see much of yall at races. sorry, love ya xxx
yourusername has left this group!
paularon wtf is going on???
olliebearman did we upset her?
paularon why did she leave???
kimiantonelli Mate... look under any post of hers or yours with her in it. It's brutal. She's getting so much hate.
paularon holy shit i've just seen it now
olliebearman i actually feel sick wtf is this shit.
kimiantonelli we should say something
paularon good idea
olliebearman let's ask her first, yes?
kimiantonelli and paul aron yes
---------------
messages
yourusername was added to 'the trip' groupchat!
yourusername hello?
kimiantonelli SUMMER TRIP HELLO? we know you've been busy but it's tradition
olliebearman yeah fr we miss you
paularon I'm going to suggest Italy???
yourusername omg i'm so sorry guys i promised my home friends i'd spend my break with them. we're going to greece but you're more than welcome to join us :)
olliebearman sounds like a plan!
kimiantonelli works for me!
paularon YAY!
yourusername one rule tho- DONT flirt with my mates
---------------
messages
paularon was added to the 'boyos' groupchat
olliebearman why is she being so weird in public?
kimiantonelli she's scared of being seen with us duh
olliebearman why?????? we're her best mates????
paularon bc she'll get murder online, duh!
kimiantonelli this is so annoyinggggggg
olliebearman fr i just want regular y/n back
paularon it's not her fault
kimiantonelli come on we'll talk to her about it another time
---------------
yourusername



liked by kimiantonelli, paularon, olliebearman and 2,394,094 others
yourusername summer breakin' :)
comments
paularon forgetting the photos of me????
user54 anyone else catch paul's comment? ->user23 omg PLEASE tell me the pookies are back
user3423 yall why is paul lowkey messy 😏
usser8390 she hasn't posted in so long, we were missing u queen!
user444 goated driver
user8990 ugly bitch
user22 shit driver
user23 omg she's so gorg
user3423 guys, anyone else noticed how paul, ollie, and kimi have been hanging out but y/n hasn't? -> user99 ikr i miss them so much 🫶 -> user12 she's just too stuck up for them now -> user23 or maybe she drives for a team that is literally falling apart???😸🔫 ->user344 it's deffo the hate
olliebearman damn, forgetting all about us
user99 YO OLLIE'S COMMENT
user4558 she's delteing their comments i swear to god
user90 y/n's such a gold digger
user3434 bitch
user221 crash next race
---------------
messages
olliebearman why did you delete our comments?
yourusername i can't get into it
paularon we deserve an answer y/n. you won't hang out with us anymore, you won't be seen talking to us, what gives???
yourusername i just don't want to get more hate, alright? it's hard enough as it is, i don't need people thinking i'm sleeping with all three of you just because we're friends and then flooding my comments with the most vile shit they can think of. I am your mate, don't worry, it's just i don't want to drag any of you into this.
kimiantonelli we're here for you y/n. this is shit and we're sorry, and we don't want you to feel like you have to hide being our friend. we want to support you and make a post about it. a bunch of the other drivers are in on it as well
yourusername you don't have to do that
paularon we want to
olliebearman if it means we can hang out again, of course we'll do it
yourusername you guys are the best :)
---------------
kimiantonelli, olliebearman, paularon, lewishamilton, charlesleclerc, landonorris, oscarpiastri, alexalbon, carlossainz, lancestroll, fernandoalonso, maxverstappen, yukitsunoda, liamlawson, esteban ocon, jackdoohan, pierregasly, georgerussell, gabrielborteleto, nicohulkenberg, and isackhadjar



liked by mercedsf1team, redbullf1team, jackdoohan and 10,323,433 others
Hey all, we just wanted to come on here and say that we don't support bullying, but some of yall need a fucking reality check. Y/n Y/l/n is in the sport and she will continue to be. She is a talented, incredible driver who will continue to inspire thousands, if not millions. The world is changing, and while that can be scary, but having a woman in F1 should not be a shocking thing. We have all said it's only a matter of time, and the time came when Y/n got her seat. A seat she deserved, and continues to deserve. Women deserve a place in motorsports, and if you can't see that, shame on you.
limited comments
mercedesf1team no.1 Y/n Y/l/n fan over here
reddbullf1team class act, thank you boys
ferrarif1team wonderful message, forza y/n!
alpinef1team Behind you all the way :)
astonmartinf1team slaying the house boots down!
sauberf1team Wonderful woman, wonderful driver, wonderful words. Thank you for speaking out!
mclarenf1team Thank you for speaking up about this!
haasf1team The trio shall continue forevermore
vcarbf1team Stunted on those hoes :) ->liked by yourusername
williamsf1team This is such a wonderful message, thank you for standing up against the bullies :)
navigation for my blog :)
mercedes & williams masterlist
alpine masterlist
#ollie bearman x reader#ollie bearman#ollie bearman x you#ollie bearman x female reader#ollie bearman x y/n#ollie bearman fluff#ollie bearman imagine#ollie bearman instagram au#prema racing#formula 1#formula 2#f1#f2#f1 x reader#f2 x reader#f1 imagine#formula one x reader#f1 fluff#formula one imagine#formula 1 x you#formula one#haas f1 team#f1 fanfic#formula 2 imagine#f1 imagines#formula 1 imagine#formula 1 smau#formula 1 imagines#f2 smau#formula 2 smau
993 notes
·
View notes