#Python variable naming rules
Explore tagged Tumblr posts
Text
Day-1: Demystifying Python Variables: A Comprehensive Guide for Data Management
Python Boot Camp Series 2023.
Python is a powerful and versatile programming language used for a wide range of applications. One of the fundamental concepts in Python, and in programming in general, is working with variables. In this article, we will explore what variables are, how to use them effectively to manage data, and some best practices for their usage. What are Variables in Python? Definition of Variables In…
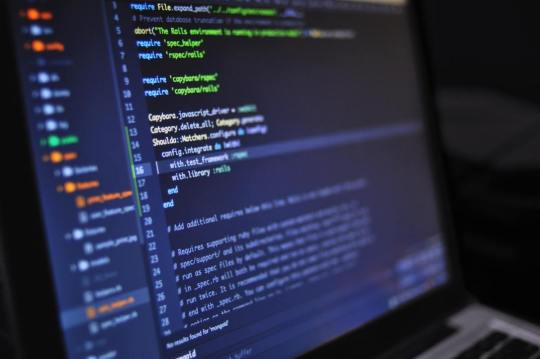
View On WordPress
#best practices for variables#data management in Python#dynamic typing#Python beginners guide#Python coding tips#Python data manipulation#Python data types#Python programming#Python programming concepts#Python tutorials#Python variable naming rules#Python variables#variable scope#working with variables
0 notes
Text
Key Features to Look for in Direct Mail Automation Software for 2025
In an era of increasing digital noise, direct mail automation software stands as a powerful tool for businesses aiming to deliver personalized, tangible marketing experiences. As we step into 2025, choosing the right software means evaluating features that align with modern marketing needs—API integration, personalization, multichannel support, and analytics. This guide breaks down the most crucial features to consider when selecting direct mail automation tools for maximum ROI and customer engagement.
1. Seamless CRM Integration
One of the most vital features to consider is CRM integration. Whether you're using Salesforce, HubSpot, Zoho, or a custom solution, your direct mail software should easily sync with your CRM platform. This integration enables:
Automated trigger-based mail campaigns
Access to customer behavior and segmentation
Real-time updates on campaign performance
Why it matters in 2025: Personalized marketing driven by real-time customer data enhances engagement rates and streamlines campaign delivery.
2. API Access for Custom Workflows
A robust Direct Mail API allows developers and marketers to build custom workflows, trigger print-mail jobs based on events, and integrate with internal systems. Look for:
RESTful API with extensive documentation
Webhook support for real-time updates
Batch processing capabilities
SDKs in popular languages (Python, JavaScript, Ruby)
Benefits: APIs enable full automation and scalability, making it ideal for enterprise-level or high-volume businesses.
3. Variable Data Printing (VDP) Support
VDP lets you personalize every piece of mail—from names and offers to images and QR codes. The best software platforms will include:
Easy-to-use VDP templates
Integration with dynamic content from your CRM
Automated personalization for large campaigns
2025 Trend: Consumers expect personalized experiences; generic mailers are far less effective.
4. Omnichannel Campaign Support
Today’s marketing isn't just physical or digital—it’s both. Look for software that integrates with:
Email
SMS
Social retargeting
Retention platforms
Bonus: Omnichannel sequencing allows you to create smart workflows like sending a postcard if a user doesn’t open your email within 3 days.
5. Campaign Performance Analytics
Your software should offer deep insights into campaign results. Key metrics to look for include:
Delivery status
Response and conversion rates
Print volume tracking
QR code scans and redemption data
Advanced analytics in 2025 should include AI-driven performance predictions and suggestions for campaign improvements.
6. Address Verification & Data Hygiene Tools
Bad addresses cost money. Your software should offer built-in address verification, using tools like:
CASS Certification
NCOA (National Change of Address) updates
International address formatting
Postal barcode generation
2025 Consideration: With global shipping more common, international address validation is a must-have.
7. Pre-Built Templates and Design Tools
Not every marketer is a designer. High-quality platforms provide:
Drag-and-drop editors
Pre-designed templates for postcards, letters, flyers, catalogs
Brand asset management tools
These reduce campaign creation time and ensure brand consistency across every print asset.
8. Automation Triggers and Rules
Software should support rule-based automations, such as:
“Send a thank-you postcard 7 days after purchase”
“Trigger a re-engagement letter if a customer hasn’t interacted in 60 days”
“Send a discount coupon after a cart abandonment”
Smart triggers improve relevance and timing, critical for campaign success.
9. Cost Estimator and Budget Control
In 2025, transparency is key. The best platforms provide real-time:
Printing and postage cost estimations
Budget tracking dashboards
ROI calculators
Spend caps and approval flows
Marketing teams can stay within budget while ensuring campaign effectiveness.
10. Security and Compliance Features
Data privacy is non-negotiable. Your software should support:
GDPR, HIPAA, and CCPA compliance
Data encryption at rest and in transit
Role-based access control
Audit trails and logs
2025 Focus: With AI data processing and automation increasing, choosing a secure platform is mission-critical.
11. Print & Mail Network Integration
Top-tier software connects with certified printers and mail houses globally, allowing for:
Localized printing to reduce shipping time/costs
International delivery tracking
SLA-based delivery guarantees
Distributed networks enhance scalability and ensure timely delivery.
12. Scalability for Enterprise Growth
As your marketing grows, your platform should grow with you. Key considerations:
Support for millions of monthly mail pieces
User management for teams
Advanced scheduling
SLAs for uptime and performance
Conclusion
Direct mail is no longer old-fashioned—it’s a data-driven, automated marketing channel. When choosing direct mail automation software in 2025, prioritize platforms that offer integration, personalization, scalability, security, and advanced analytics. Investing in the right tool ensures your campaigns are cost-effective, personalized, and impactful across every customer touchpoint.
youtube
SITES WE SUPPORT
Automated Mailing API – Wix
1 note
·
View note
Text
What Are Python Variables and Data Types?

Python is one of the most beginner-friendly and widely used programming languages today. Understanding Python Variables and Data Types is essential for anyone starting out with coding. Whether you're learning it for data science, web development, or automation, this foundational concept helps you write clear and efficient code.
What Is a Variable in Python?
The variable is similar to a closet where we store information. You can give it a name, and an object of any data type can be assigned to it.
Example:
python
name = "Zen"
age = 25
Here, the variable name stores a string, and the variable age stores a number (integer). Contrary to many other languages, in Python, you do not need to specify the type-since Python figures forever variable.
Learn more: Python Programming Course
Python Variables Rules
Any capitalization difference matters (age ≠ Age)
Can include letters, numbers, and underscores (e.g., student_name)
Cannot start with a number (for example, 1value is invalid)
Avoid using Python reserved keywords (for instance, for, class, if)
Common Data Types in Python
Python supports a common set of built-in data types. The ones you use most often are:
String (str): text data like "Zen"
Integer (int): whole numbers like 25
Float (float): decimal numbers like 3.14
Boolean (bool): logical values- True or False
List: collection of objects, e.g., [1, 2, 3]
Tuple: like a list but cannot be changed, e.g., (1, 2, 3)
Dictionary: key-value pairings, e.g., {"name": "Zen"}
NoneType: denotes lack of value- None
Watch Now: Different data types in Python
Why Are Data Types Considered AGS?
Well, each data type has its operational nuances. If you understand how these work, you are less likely to:
Fall into certain cliché errors in programming
Use the wrong operations (e.g., adding or concatenating a string to an integer is forbidden)
Write inefficient and less readable programs
Use the type() function to discover the data type:
python
x = 10
print(type(x)) # Output: <class 'int'>
Conclusion
Learning variables and data types is the stepping stone toward writing meaningful Python programs. Whether one is a student or pursues Python differently from some other field, this grounding will allow confidence to grow with more Python looks.
Learn more?
Learn Python programming with TCCI – Tririd Computer Coaching Institute, Ahmedabad.
Call now on +91 9825618292
Visit Our Website: http://tccicomputercoaching.com/
#Best Programming Classes near Bopal Ahmedabad#Learn Python at TCCI Ahmedabad#Python Programming Course Iskcon-Ambli Road#Software Training Institute Iskcon-Ambli Road#TCCI - Tririd Computer Coaching Institute
0 notes
Text
Errors in Python Code: How to Debug Them
Python is known for its simplicity and readability, but even the most experienced developers encounter bugs. Whether you're just starting or enrolled in the best Python training in Hyderabad, understanding how to debug common errors is crucial to mastering the language. Debugging not only sharpens your problem-solving skills but also helps in writing efficient, error-free code.
1. Syntax Errors
One of the most common issues beginners face is the SyntaxError—usually caused by missing colons, unmatched brackets, or incorrect indentation. These are easily fixed by double-checking your code structure and following Python’s formatting rules.
2. Name Errors
When a variable or function is used before it has been defined, a NameError occurs. This can be avoided by ensuring all variables are initialized properly. Using print statements or integrated development environment (IDE) suggestions can help identify these issues.
3. Type and Value Errors
An operation that is applied to an inappropriate type, like adding a string to a number, will result in a TypeError.Variables and functions that have not been defined will raise a NameError. Understanding Python's error messages can guide you to quick fixes.
4. Logical Errors
There are several types of logical errors, but the most difficult is when the code fails to produce the expected result but doesn't crash. These require careful review of your logic and expected results. Tools like breakpoints, assertions, and logging are helpful in spotting such mistakes.
Conclusion
While errors are a natural part of coding, developing strong debugging skills will greatly improve your programming efficiency. For structured learning, expert guidance, and real-time debugging practice, we recommend SSSIT Computer Education, a trusted name in Python and software training in Hyderabad.
0 notes
Text
Cracking Wordle (kinda) with Monte Carlo Simulations: A Statistical Approach to Predicting the Best Guesses
Wordle, the viral word puzzle game, has captivated millions worldwide with its simple yet challenging gameplay. The thrill of uncovering the five-letter mystery word within six attempts has led to a surge in interest in word strategies and algorithms. In this blog post, we delve into the application of the Monte Carlo method—a powerful statistical technique—to predict the most likely words in Wordle. We will explore what the Monte Carlo method entails, its real-world applications, and a step-by-step explanation of a Python script that harnesses this method to identify the best guesses using a comprehensive list of acceptable Wordle words from GitHub.
Understanding the Monte Carlo Method
What is the Monte Carlo Method?
The Monte Carlo method is a statistical technique that employs random sampling and statistical modeling to solve complex problems and make predictions. Named after the famous Monte Carlo Casino in Monaco, this method relies on repeated random sampling to obtain numerical results, often used when deterministic solutions are difficult or impossible to calculate.
How Does It Work?
At its core, the Monte Carlo method involves running simulations with random variables to approximate the probability of various outcomes. The process typically involves:
Defining a Model: Establishing the mathematical or logical framework of the problem.
Generating Random Inputs: Using random sampling to create multiple scenarios.
Running Simulations: Executing the model with the random inputs to observe outcomes.
Analyzing Results: Aggregating and analyzing the simulation outcomes to draw conclusions or make predictions.
Real-World Applications
The Monte Carlo method is widely used in various fields, including:
Finance: To evaluate risk and uncertainty in stock prices, investment portfolios, and financial derivatives.
Engineering: For reliability analysis, quality control, and optimization of complex systems.
Physics: In particle simulations, quantum mechanics, and statistical mechanics.
Medicine: For modeling the spread of diseases, treatment outcomes, and medical decision-making.
Climate Science: To predict weather patterns, climate change impacts, and environmental risks.
Applying Monte Carlo to Wordle
Objective
In the context of Wordle, our objective is to use the Monte Carlo method to predict the most likely five-letter words that can be the solution to the puzzle. We will simulate multiple guessing scenarios and evaluate the success rates of different words.
Python Code Explanation
Let's walk through the Python script that accomplishes this task.
1. Loading the Word List
First, we need a comprehensive list of acceptable five-letter words used in Wordle. We can obtain the list of all 2315 words that will become the official wordle at some point. The script reads the words from a line-delimited text file and filters them to ensure they are valid.
2. Generating Feedback
To simulate Wordle guesses, we need a function to generate feedback based on the game's rules. This function compares the guessed word to the answer and provides feedback on the correctness of each letter.
3. Simulating Wordle Games
The simulate_wordle function performs the Monte Carlo simulations. For each word in the list, it simulates multiple guessing rounds, keeping track of successful guesses within six attempts.
4. Aggregating Results
The monte_carlo_wordle function aggregates the results from all simulations to determine the most likely words. It also includes progress updates to monitor the percentage of words completed.
5. Running the Simulation
Finally, we load the word list from the text file and run the Monte Carlo simulations. The script prints the top 10 most likely words based on the simulation results.
The Top 50 Words (Based on this approach)
For this article I amended the code so that each simulation runs 1000 times instead of 100 to increase accuracy. I have also amended the script to return the top 50 words. Without further ado, here is the list of words most likely to succeed based on this Monte Carlo method:
trope: 10 successes
dopey: 9 successes
azure: 9 successes
theme: 9 successes
beast: 8 successes
prism: 8 successes
quest: 8 successes
brook: 8 successes
chick: 8 successes
batch: 7 successes
twist: 7 successes
twang: 7 successes
tweet: 7 successes
cover: 7 successes
decry: 7 successes
tatty: 7 successes
glass: 7 successes
gamer: 7 successes
rouge: 7 successes
jumpy: 7 successes
moldy: 7 successes
novel: 7 successes
debar: 7 successes
stave: 7 successes
annex: 7 successes
unify: 7 successes
email: 7 successes
kiosk: 7 successes
tense: 7 successes
trend: 7 successes
stein: 6 successes
islet: 6 successes
queen: 6 successes
fjord: 6 successes
sloth: 6 successes
ripen: 6 successes
hutch: 6 successes
waver: 6 successes
geese: 6 successes
crept: 6 successes
bring: 6 successes
ascot: 6 successes
lumpy: 6 successes
amply: 6 successes
eerie: 6 successes
young: 6 successes
glyph: 6 successes
curio: 6 successes
merry: 6 successes
atone: 6 successes
Edit: I ran the same code again, this time running each simulation 10,000 times for each word. You can find the results below:
bluer: 44 successes
grown: 41 successes
motel: 41 successes
stole: 41 successes
abbot: 40 successes
lager: 40 successes
scout: 40 successes
smear: 40 successes
cobra: 40 successes
realm: 40 successes
queer: 39 successes
plaza: 39 successes
naval: 39 successes
tulle: 39 successes
stiff: 39 successes
hussy: 39 successes
ghoul: 39 successes
lumen: 38 successes
inter: 38 successes
party: 38 successes
purer: 38 successes
ethos: 38 successes
abort: 38 successes
drone: 38 successes
eject: 38 successes
wrath: 38 successes
chaos: 38 successes
posse: 38 successes
pudgy: 38 successes
widow: 38 successes
email: 38 successes
dimly: 38 successes
rebel: 37 successes
melee: 37 successes
pizza: 37 successes
heist: 37 successes
avail: 37 successes
nomad: 37 successes
sperm: 37 successes
raise: 37 successes
cruel: 37 successes
prude: 37 successes
latch: 37 successes
ninja: 37 successes
truth: 37 successes
pithy: 37 successes
spiky: 37 successes
tarot: 36 successes
ashen: 36 successes
trail: 36 successes
Conclusion
The Monte Carlo method provides a powerful and flexible approach to solving complex problems, making it an ideal tool for predicting the best Wordle guesses. By simulating multiple guessing scenarios and analyzing the outcomes, we can identify the words with the highest likelihood of being the solution. The Python script presented here leverages the comprehensive list of acceptable Wordle words from GitHub, demonstrating how statistical techniques can enhance our strategies in the game.
Of course, by looking at the list itself it very rarely would allow a player to input the top 6 words in this list and get it right. It's probalistic nature means that although it is more probable that these words are correct, it is not learning as it goes along and therefore would be considered "dumb".
Benefits of the Monte Carlo Approach
Data-Driven Predictions: The Monte Carlo method leverages extensive data to make informed predictions. By simulating numerous scenarios, it identifies patterns and trends that may not be apparent through simple observation or random guessing.
Handling Uncertainty: Wordle involves a significant degree of uncertainty, as the correct word is unknown and guesses are constrained by limited attempts. The Monte Carlo approach effectively manages this uncertainty by exploring a wide range of possibilities.
Scalability: The method can handle large datasets, such as the full list of acceptable Wordle words from GitHub. This scalability ensures that the predictions are based on a comprehensive dataset, enhancing their accuracy.
Optimization: By identifying the top 50 words with the highest success rates, the Monte Carlo method provides a focused list of guesses, optimizing the strategy for solving Wordle puzzles.
Practical Implications
The application of the Monte Carlo approach to Wordle demonstrates its practical value in real-world scenarios. The method can be implemented using Python, with scripts that read word lists, simulate guessing scenarios, and aggregate results. This practical implementation highlights several key aspects:
Efficiency: The Monte Carlo method streamlines the guessing process by focusing on the most promising words, reducing the number of attempts needed to solve the puzzle.
User-Friendly: The approach can be easily adapted to provide real-time feedback and progress updates, making it accessible and user-friendly for Wordle enthusiasts.
Versatility: While this essay focuses on Wordle, the Monte Carlo method’s principles can be applied to other word games and puzzles, showcasing its versatility.
Specific Weaknesses in the Context of Wordle
Non-Deterministic Nature: The Monte Carlo method provides probabilistic predictions rather than deterministic solutions. This means that it cannot guarantee the correct Wordle word but rather offers statistically informed guesses. There is always an element of uncertainty.
2. Dependence on Word List Quality: The accuracy of predictions depends on the comprehensiveness and accuracy of the word list used. If the word list is incomplete or contains errors, the predictions will be less reliable.
3. Time Consumption: Running simulations for a large word list (e.g., thousands of words) can be time-consuming, especially on average computing hardware. This can limit its practicality for users who need quick results.
4. Simplified Feedback Model: The method uses a simplified model to simulate Wordle feedback, which may not capture all nuances of human guessing strategies or advanced linguistic patterns. This can affect the accuracy of the predictions.
The House always wins with Monte Carlo! Is there a better way?
There are several alternative approaches and techniques to improve the Wordle guessing strategy beyond the Monte Carlo method. Each has its own strengths and can be tailored to provide effective results. Here are a few that might offer better or complementary strategies:
1. Machine Learning Models
Using machine learning models can provide a sophisticated approach to predicting Wordle answers:
Neural Networks: Train a neural network on past Wordle answers and feedback. This approach can learn complex patterns and relationships in the data, potentially providing highly accurate predictions.
Support Vector Machines (SVMs): Use SVMs for classification tasks based on features extracted from previous answers. This method can effectively distinguish between likely and unlikely words.
2. Heuristic Algorithms
Heuristic approaches can provide quick and effective solutions:
Greedy Algorithm: This method chooses the best option at each step based on a heuristic, such as maximizing the number of correct letters or minimizing uncertainty. It's simple and fast but may not always find the optimal solution.
Simulated Annealing: This probabilistic technique searches for a global optimum by exploring different solutions and occasionally accepting worse solutions to escape local optima. It can be more effective than a greedy algorithm in finding better solutions.
3. Bayesian Inference
Bayesian inference provides a probabilistic approach to updating beliefs based on new information:
Bayesian Models: Use Bayes’ theorem to update the probability of each word being correct based on feedback from previous guesses. This approach combines prior knowledge with new evidence to make informed guesses.
Hidden Markov Models (HMMs): HMMs can model sequences and dependencies in data, useful for predicting the next word based on previous feedback.
4. Rule-Based Systems
Using a set of predefined rules and constraints can systematically narrow down the list of possible words:
Constraint Satisfaction: This approach systematically applies rules based on Wordle feedback (correct letter and position, correct letter but wrong position, incorrect letter) to filter out unlikely words.
Decision Trees: Construct a decision tree based on the feedback received to explore different guessing strategies. Each node represents a guess, and branches represent the feedback received.
5. Information Theory
Using concepts from information theory can help to reduce uncertainty and optimize guesses:
Entropy-Based Methods: Measure the uncertainty of a system using information entropy and make guesses that maximize the information gained. By choosing words that provide the most informative feedback, these methods can quickly narrow down the possibilities.
Whether you're a Wordle enthusiast or a data science aficionado, the Monte Carlo method offers a fascinating glimpse into the intersection of statistics and gaming. Happy Wordle solving!
1 note
·
View note
Text
Python Prioritize Courses for Kids: Fitting the Innovators of Later accompanying Intelligent n Bright
As we move further into the 21st centennial, the strength to appreciate and utilize science is not any more just an benefit—it’s a necessity. Systematize, frequently named as the “language of the future,” is a important ability that opens doors to perpetual potential. For young learners, Python is an ideal beginning due to allure directness and effective applications. At Intelligent n Sunny, we offer Python Register Courses for Kids designed to stimulate, experience, and enable children to guide along route, often over water the mathematical countryside confidently.
Reason Python is the Ideal Native language for Kids When presenting children to systematize, the aim search out create an knowledge that is to say two together accessible and charming. Python surpasses in two together areas, making it a top choice for young learners.
Handy Arrangement: Unlike added the study of computers that demand complex coding makeups, Python is legible and natural. Its simple arrangement form it possible for juveniles to rewrite clean law without assimilating stuck by difficult rules.
Versatility Across Energies: From netting incident to artificial intelligence and controlled estimating, Python is secondhand in virtually each important manufacturing. This means that kids the one gain Python are gaining abilities bound for appropriate in a wide sort of future courses.
Perpetual Creativity: Python allows teenagers to convert their imaginative plans into concrete projects, either it's creating trick, construction apps, or crafty interactive websites. The imaginative immunity Python offers form learning fun and beneficial.
Construction a Stable Foundation for Different Styles: Already children master Python, they can surely change to more intricate programming languages like Hot beverage made from beans of a tree, C++, or JavaScript. Python serves as a entrance language, making it smooth for kids to gain supplementary coding styles later.
The Lasting Benefits of Learning Python at a Young Age Python is in addition just a mechanics ability. By learning to law, offsprings expand crucial growth abilities that will benefit bureaucracy academically and personally for age at hand.
Embellished Problem-Solving Talents: Systematize in Python educates kids how to approach questions accurately. They determine to break down complex issues, consider precariously, and conceive logical resolutions. These abilities can be used not just in systematize but in added subjects like arithmetic, erudition, and even ordinary challenges.
Strengthening Capacity and Elasticity: Systematize often includes experimental approach, that encourages offsprings to endure when covering obstacles. Troubleshooting, while thwarting now and then, helps kids build resilience and evolve a progress psychology—understanding that mistakes are part of the knowledge process.
Revised Academic Efficiency: Studies have shown that infants the one discover coding inappropriate act better in math and skill cases. The reasonable thinking and analytical abilities win from register have a positive affect their overall academic depiction.
Pushed Confidence and Freedom: As kids complete systematize projects and see the results of their work revive, their self-assurance evolves. They feel empowered, experienced they can establish entity impactful utilizing their own capabilities. This sense of attainment fosters liberty and a full of enthusiasm approach to education.
What Kids Will Learn in Intelligent n Sunny’s Python Prioritize Courses At Brainy n Sunny, we make that each child’s knowledge style and pace are singular. Our Python Register Courses for Kids are tailored to specify a inclusive knowledge experience that focuses on two together hypothesis and useful application.
Debut to Python Essentials: Kids will start accompanying the basics, education about variables, loops, functions, and dossier types. Our communication are designed to guarantee that even novices can surely grasp these concepts and ask bureaucracy in experiential projects.
Fun, Interactive Projects: Education is more pleasing when it’s fun! We mix project-based education into our Python courses, admitting children to generate plot, design websites, or even build plain AI models. These projects help reinforce the ideas they determine and present them a sense of property over their work.
Logical Challenges: As pupils progress through the course, they’ll be given progressively complex systematize challenges that excite critical thinking and logical. Our instructors reassure pupils to explore various resolutions, promoting a mindset of artistry and novelty.
Certain-World Uses: We present undergraduates to Python’s real-planet uses, from computerization to AI and data reasoning. This helps ruling class understand the more extensive impact of systematize and by virtue of what Python is used to solve corporal questions.
State-of-the-art Concepts for Enthusiastic Learners: For kids the one are ready to take their abilities to the next level, we offer advanced materials in the way that thinking mainly about physical things programming (OOP), atheneums like NumPy and Pandas for dossier guidance, and even machine learning fundamentals.
Reason Select Brainy n Brilliant for Your Youth’s Systematize Journey? At Brainy n Brilliant, we are hard-working to providing a education environment that nurtures interest, artistry, and fault-finding thinking. Our Python Programming Courses for Kids prominent by way of our unique approach to instruction.
Expert Instructors: Our crew of instructors exists of professionals accompanying far-reaching occurrence in coding and education. They are desirous about making compute accessible to kids and are skillful at clarifying complex ideas in a way namely fun and easy to use.
Limited Class Sizes for Personalized Consideration: We trust that each toddler’s learning journey is singular. Our narrow class sizes admit our instructors to provide distinguished consideration, guaranteeing that every scholar is financed and heartened to achieve their thorough potential.
Bendable Learning Manners: We offer connected to the internet, onsite, and mixture learning alternatives to sustain various learning predilections and schedules. Either your baby prefers the interaction of in-man classes or the availability of connected to the internet learning, Intelligent n Sunny has a program to suit their needs.
Shared and Engaging Educational program: Our educational program is created to be charming, common, and disputing enough to keep adolescents instigated. We blend systematize theory accompanying realistic, experiential experience to guarantee scholars not only understand the "by means of what" but further the "reason" behind the code.
By virtue of what Python Prepares Kids for a Type of educational institution-Compelled Future The digital countryside is developing at an original pace, and those who are outfitted accompanying systematize skills will have a meaningful benefit. Here’s in what way or manner Python programming can set your infant brave future accomplishment:
Future-Proof Course Abilities: By knowledge Python, children are win individual of ultimate sought-after abilities in the task market. Python is established in fields like netting happening, artificial intelligence, machine intelligence, and dossier learning—all industries that be necessary to evolve exponentially in the coming age.
Opportunities for All-encompassing Cooperation: Systematize is a universal language that surpasses borders. Kids the one discover Python can participate in worldwide systematize competitions, hackathons, and cooperations with peers from about the realm. They’ll gain worldwide exposure and gain to work as constituent a best, connected society.
Guidance in Novelty: Python gives kids the tools expected gods, not just consumers. Either they be going to cultivate their own apps, create mathematical answers, or even start their own type of educational institution company, Python supports a dependable establishment for innovation and venture capital.
Ready to Excite? Enter Today! Immediately is the perfect opportunity to present your child to the exhilarating globe of systematize with Python Programming Courses for Kids at Intelligent n Sunny. Whether your youngster dreams of construction their own television games, crafty apps, or diving into the realm of AI, Python offers the perfect program to launch their journey.
At Intelligent n Sunny, we are committed to providing the highest quality systematize instruction in a supportive and charming surroundings. Don’t wait—present your child the freedom to enhance a certain coder and prepare ruling class for a future entire of potential.
Enroll contemporary and take the beginning towards enabling your child accompanying the abilities they need to blossom in the digital age!
1 note
·
View note
Text
Breaking Down Python: An Easy Way to Understand the Basics
Python is one of the most beginner-friendly programming languages, often celebrated for its readability and versatility. Whether you're new to coding or exploring a new hobby, understanding Python basics can open the door to an exciting world of possibilities. For individuals who want to work in the sector, a respectable python training in pune can give them the skills and information they need to succeed in this fast-paced atmosphere. This blog will break down Python into easy-to-understand parts, making it accessible and enjoyable to learn.
1. What is Python?
Python is a high-level, interpreted programming language known for its simplicity and readability. Created by Guido van Rossum and first released in 1991, Python has grown into one of the most popular languages in the tech industry. It’s often used for web development, data analysis, automation, and much more. Its ease of use makes it an ideal choice for beginners.
2. Why Learn Python?
Python is great for beginners for several reasons:
Simple Syntax: Python’s syntax is clean and easy to understand, which allows new learners to focus on learning programming concepts rather than complex language rules.
Versatile Use Cases: From creating websites to analyzing data or building simple games, Python's wide range of applications keeps learning fun and rewarding.
Large Community: With Python being so popular, there’s a massive community of developers who are always ready to help. Numerous resources, tutorials, and forums are available online.
3. Key Concepts to Get Started
Let’s dive into some of the core concepts that form the foundation of Python:
Variables and Data Types
Variables are used to store information that can be manipulated by your program. In Python, assigning a value to a variable is simple:
python
Copy code
name = "Alice" # This is a string variable age = 25 # This is an integer variable height = 5.6 # This is a float variable
Python supports several data types, such as integers (int), floating-point numbers (float), strings (str), and booleans (bool).
Basic Syntax and Indentation
Unlike many programming languages, Python uses indentation to define code blocks rather than braces {}. Proper indentation is essential for your Python code to work:
python
Copy code
if age > 18: print("You are an adult.")
This indentation makes Python code easier to read and understand, even for someone who isn't a programmer. Enrolling in python online training can enable individuals to unlock full potential and develop a deeper understanding of its complexities.
Functions
Functions in Python are reusable pieces of code that perform specific tasks. Defining a function is simple:
python
Copy code
def greet(): print("Hello, world!") greet() # This will output: Hello, world!
Functions can also take arguments to perform actions based on the inputs:
python
Copy code
def greet_user(name): print(f"Hello, {name}!") greet_user("Alice") # This will output: Hello, Alice!
Lists and Loops
A list is a collection of items that can be of any data type, such as integers, strings, or even other lists. Loops, such as for and while, can be used to iterate over these collections:
python
Copy code
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(fruit)
This code will output each fruit in the list, one at a time.
Conditionals
Conditionals are used to execute certain pieces of code based on a condition. Python provides the if, elif, and else statements for this purpose:
python
Copy code
number = 10 if number > 0: print("The number is positive.") elif number == 0: print("The number is zero.") else: print("The number is negative.")
4. Writing Your First Python Program
Let’s write a simple program that asks for your name and greets you:
python
Copy code
name = input("Enter your name: ") print(f"Hello, {name}! Welcome to Python.")
This program takes user input and prints a customized greeting message. Try running it and see how it works!
5. Practice, Practice, Practice!
The best way to understand Python is to practice. Here are a few fun exercises to get you started:
Create a program that calculates the area of a rectangle.
Write a function that takes two numbers as input and returns their sum.
Build a simple text-based game, such as a guessing game where the computer picks a random number, and the player has to guess it.
6. Resources to Help You Learn
To make your Python learning journey more enjoyable, here are some great resources:
Codecademy and freeCodeCamp: Both platforms offer interactive Python tutorials.
Books: "Automate the Boring Stuff with Python" by Al Sweigart is a great book for beginners.
Online Communities: Websites like Stack Overflow and Reddit have active Python communities where you can ask questions and share your progress.
Conclusion
Python is a fantastic language for beginners because of its simplicity and the wide range of fun projects you can create. By understanding its core concepts—such as variables, functions, lists, loops, and conditionals—you can start building your own programs and gain the confidence to explore more complex programming challenges. Dive in, experiment, and enjoy the process of learning Python!
0 notes
Text
Code Embedding: A Comprehensive Guide
New Post has been published on https://thedigitalinsider.com/code-embedding-a-comprehensive-guide/
Code Embedding: A Comprehensive Guide
Code embeddings are a transformative way to represent code snippets as dense vectors in a continuous space. These embeddings capture the semantic and functional relationships between code snippets, enabling powerful applications in AI-assisted programming. Similar to word embeddings in natural language processing (NLP), code embeddings position similar code snippets close together in the vector space, allowing machines to understand and manipulate code more effectively.
What are Code Embeddings?
Code embeddings convert complex code structures into numerical vectors that capture the meaning and functionality of the code. Unlike traditional methods that treat code as sequences of characters, embeddings capture the semantic relationships between parts of the code. This is crucial for various AI-driven software engineering tasks, such as code search, completion, bug detection, and more.
For example, consider these two Python functions:
def add_numbers(a, b): return a + b
def sum_two_values(x, y): result = x + y return result
While these functions look different syntactically, they perform the same operation. A good code embedding would represent these two functions with similar vectors, capturing their functional similarity despite their textual differences.
Vector Embedding
How are Code Embeddings Created?
There are different techniques for creating code embeddings. One common approach involves using neural networks to learn these representations from a large dataset of code. The network analyzes the code structure, including tokens (keywords, identifiers), syntax (how the code is structured), and potentially comments to learn the relationships between different code snippets.
Let’s break down the process:
Code as a Sequence: First, code snippets are treated as sequences of tokens (variables, keywords, operators).
Neural Network Training: A neural network processes these sequences and learns to map them to fixed-size vector representations. The network considers factors like syntax, semantics, and relationships between code elements.
Capturing Similarities: The training aims to position similar code snippets (with similar functionality) close together in the vector space. This allows for tasks like finding similar code or comparing functionality.
Here’s a simplified Python example of how you might preprocess code for embedding:
import ast def tokenize_code(code_string): tree = ast.parse(code_string) tokens = [] for node in ast.walk(tree): if isinstance(node, ast.Name): tokens.append(node.id) elif isinstance(node, ast.Str): tokens.append('STRING') elif isinstance(node, ast.Num): tokens.append('NUMBER') # Add more node types as needed return tokens # Example usage code = """ def greet(name): print("Hello, " + name + "!") """ tokens = tokenize_code(code) print(tokens) # Output: ['def', 'greet', 'name', 'print', 'STRING', 'name', 'STRING']
This tokenized representation can then be fed into a neural network for embedding.
Existing Approaches to Code Embedding
Existing methods for code embedding can be classified into three main categories:
Token-Based Methods
Token-based methods treat code as a sequence of lexical tokens. Techniques like Term Frequency-Inverse Document Frequency (TF-IDF) and deep learning models like CodeBERT fall into this category.
Tree-Based Methods
Tree-based methods parse code into abstract syntax trees (ASTs) or other tree structures, capturing the syntactic and semantic rules of the code. Examples include tree-based neural networks and models like code2vec and ASTNN.
Graph-Based Methods
Graph-based methods construct graphs from code, such as control flow graphs (CFGs) and data flow graphs (DFGs), to represent the dynamic behavior and dependencies of the code. GraphCodeBERT is a notable example.
TransformCode: A Framework for Code Embedding
TransformCode: Unsupervised learning of code embedding
TransformCode is a framework that addresses the limitations of existing methods by learning code embeddings in a contrastive learning manner. It is encoder-agnostic and language-agnostic, meaning it can leverage any encoder model and handle any programming language.
The diagram above illustrates the framework of TransformCode for unsupervised learning of code embedding using contrastive learning. It consists of two main phases: Before Training and Contrastive Learning for Training. Here’s a detailed explanation of each component:
Before Training
1. Data Preprocessing:
Dataset: The initial input is a dataset containing code snippets.
Normalized Code: The code snippets undergo normalization to remove comments and rename variables to a standard format. This helps in reducing the influence of variable naming on the learning process and improves the generalizability of the model.
Code Transformation: The normalized code is then transformed using various syntactic and semantic transformations to generate positive samples. These transformations ensure that the semantic meaning of the code remains unchanged, providing diverse and robust samples for contrastive learning.
2. Tokenization:
Train Tokenizer: A tokenizer is trained on the code dataset to convert code text into embeddings. This involves breaking down the code into smaller units, such as tokens, that can be processed by the model.
Embedding Dataset: The trained tokenizer is used to convert the entire code dataset into embeddings, which serve as the input for the contrastive learning phase.
Contrastive Learning for Training
3. Training Process:
Train Sample: A sample from the training dataset is selected as the query code representation.
Positive Sample: The corresponding positive sample is the transformed version of the query code, obtained during the data preprocessing phase.
Negative Samples in Batch: Negative samples are all other code samples in the current mini-batch that are different from the positive sample.
4. Encoder and Momentum Encoder:
Transformer Encoder with Relative Position and MLP Projection Head: Both the query and positive samples are fed into a Transformer encoder. The encoder incorporates relative position encoding to capture the syntactic structure and relationships between tokens in the code. An MLP (Multi-Layer Perceptron) projection head is used to map the encoded representations to a lower-dimensional space where the contrastive learning objective is applied.
Momentum Encoder: A momentum encoder is also used, which is updated by a moving average of the query encoder’s parameters. This helps maintain the consistency and diversity of the representations, preventing the collapse of the contrastive loss. The negative samples are encoded using this momentum encoder and enqueued for the contrastive learning process.
5. Contrastive Learning Objective:
Compute InfoNCE Loss (Similarity): The InfoNCE (Noise Contrastive Estimation) loss is computed to maximize the similarity between the query and positive samples while minimizing the similarity between the query and negative samples. This objective ensures that the learned embeddings are discriminative and robust, capturing the semantic similarity of the code snippets.
The entire framework leverages the strengths of contrastive learning to learn meaningful and robust code embeddings from unlabeled data. The use of AST transformations and a momentum encoder further enhances the quality and efficiency of the learned representations, making TransformCode a powerful tool for various software engineering tasks.
Key Features of TransformCode
Flexibility and Adaptability: Can be extended to various downstream tasks requiring code representation.
Efficiency and Scalability: Does not require a large model or extensive training data, supporting any programming language.
Unsupervised and Supervised Learning: Can be applied to both learning scenarios by incorporating task-specific labels or objectives.
Adjustable Parameters: The number of encoder parameters can be adjusted based on available computing resources.
TransformCode introduces A data-augmentation technique called AST transformation, applying syntactic and semantic transformations to the original code snippets. This generates diverse and robust samples for contrastive learning.
Applications of Code Embeddings
Code embeddings have revolutionized various aspects of software engineering by transforming code from a textual format to a numerical representation usable by machine learning models. Here are some key applications:
Improved Code Search
Traditionally, code search relied on keyword matching, which often led to irrelevant results. Code embeddings enable semantic search, where code snippets are ranked based on their similarity in functionality, even if they use different keywords. This significantly improves the accuracy and efficiency of finding relevant code within large codebases.
Smarter Code Completion
Code completion tools suggest relevant code snippets based on the current context. By leveraging code embeddings, these tools can provide more accurate and helpful suggestions by understanding the semantic meaning of the code being written. This translates to faster and more productive coding experiences.
Automated Code Correction and Bug Detection
Code embeddings can be used to identify patterns that often indicate bugs or inefficiencies in code. By analyzing the similarity between code snippets and known bug patterns, these systems can automatically suggest fixes or highlight areas that might require further inspection.
Enhanced Code Summarization and Documentation Generation
Large codebases often lack proper documentation, making it difficult for new developers to understand their workings. Code embeddings can create concise summaries that capture the essence of the code’s functionality. This not only improves code maintainability but also facilitates knowledge transfer within development teams.
Improved Code Reviews
Code reviews are crucial for maintaining code quality. Code embeddings can assist reviewers by highlighting potential issues and suggesting improvements. Additionally, they can facilitate comparisons between different code versions, making the review process more efficient.
Cross-Lingual Code Processing
The world of software development is not limited to a single programming language. Code embeddings hold promise for facilitating cross-lingual code processing tasks. By capturing the semantic relationships between code written in different languages, these techniques could enable tasks like code search and analysis across programming languages.
Choosing the Right Code Embedding Model
There’s no one-size-fits-all solution for choosing a code embedding model. The best model depends on various factors, including the specific objective, the programming language, and available resources.
Key Considerations:
Specific Objective: For code completion, a model adept at local semantics (like word2vec-based) might be sufficient. For code search requiring understanding broader context, graph-based models might be better.
Programming Language: Some models are tailored for specific languages (e.g., Java, Python), while others are more general-purpose.
Available Resources: Consider the computational power required to train and use the model. Complex models might not be feasible for resource-constrained environments.
Additional Tips:
Experimentation is Key: Don’t be afraid to experiment with a few different models to see which one performs best for your specific dataset and use case.
Stay Updated: The field of code embeddings is constantly evolving. Keep an eye on new models and research to ensure you’re using the latest advancements.
Community Resources: Utilize online communities and forums dedicated to code embeddings. These can be valuable sources of information and insights from other developers.
The Future of Code Embeddings
As research in this area continues, code embeddings are poised to play an increasingly central role in software engineering. By enabling machines to understand code on a deeper level, they can revolutionize the way we develop, maintain, and interact with software.
References and Further Reading
CodeBERT: A Pre-Trained Model for Programming and Natural Languages
GraphCodeBERT: Pre-trained Code Representation Learning with Data Flow
InferCode: Self-Supervised Learning of Code Representations by Predicting Subtrees
Transformers: Attention Is All You Need
Contrastive Learning for Unsupervised Code Embedding
#Abstract Syntax Tree#ai#Analysis#applications#approach#Artificial Intelligence#attention#Behavior#bug#bugs#Capture#code#Code Embedding#Code embeddings#Code Review#Code Search#Code2vec#CodeBERT#coding#Community#comprehensive#computing#continuous#contrastive learning#data#Deep Learning#detection#developers#development#diversity
0 notes
Text
How Non-Technical People Can Learn to Code: A Step-by-Step Guide

Coding Classes For Beginners In Bhopal
Software development is a highly intriguing and rewarding field, promising a bright future with the rapid growth of the computer science industry. As demand for skilled coders continues to rise, opportunities abound for students, working professionals, and even those from non-technical backgrounds such as biology, finance, literature, or mechanical engineering. If you're new to coding and want to start your journey, this guide will provide a step-by-step process to help you learn to code effectively full stack development institute in Bhopal.
Prerequisites
You might be wondering if coding is suitable for you, especially if you come from a non-technical background. Contrary to what some might think, coding does not require any specific prerequisites. Whether you are a science student, commerce student, arts student, or from any other field, anyone with a laptop and internet connection can learn to code.
What is Coding?
Coding is the process of giving instructions to a computer to perform specific tasks. Just like how humans communicate with each other using languages such as English, Hindi, or French, computers understand instructions in the form of code. These instructions are written in programming languages like C++, Java, Python, and Coding classes for beginners in Bhopal, which translate human commands into machine-level language (binary code). For instance, while writing “65” in binary would be “01000001”, using programming languages simplifies this process, making it more accessible for humans.
Why Learn to Code?
Before starting your coding journey, it's crucial to ask yourself why you want to learn to code. Are you driven by a passion for technology, inspired by friends, or motivated by the potential for high-paying jobs? Understanding your motivation will help you stay committed, Programming or Language classes in Bhopal especially during the challenging early stages of learning. Knowing your “why” will provide the resilience needed to overcome initial obstacles.
What to Learn
Determining your goal is essential before diving into a specific programming language. Do you aspire to become a web developer, Android developer, data scientist, AI specialist, or blockchain developer? Your career goal will guide you in choosing the right language to learn. For example, aspiring web developers might start with HTML, CSS, and JavaScript, while those interested in data science might begin with Python Robotics Programming in Bhopal.
Many beginners are confused about which language to start with: C++, Java, or Python. Each has its advantages. Java is a balanced choice, not as simple as Python but easier than C++, and it has great demand in the industry. If your goal doesn't require in-depth data structures and algorithms knowledge, starting with simpler languages like HTML or CSS can be beneficial to avoid getting overwhelmed.
Learning the Basics
Once you have chosen a language, it's time to learn its basics. Key concepts to understand include:
Variables: Containers that store information in a program.
Data Structures: Named locations used to store and organize data.
Syntax: The set of rules defining the combinations of symbols that are considered valid code.
Data Structures and Algorithms
Mastering data structures and algorithms is crucial for job opportunities, especially with major tech companies like Google, Microsoft, and Meta Java coding Classes in Bhopal. Data structures are ways to store and organize data efficiently, while algorithms are steps to solve specific problems. These skills are essential for coding interviews and solving complex problems.
Programming Or Language Classes In Bhopal
Join Coding Communities
The technology landscape is continuously evolving, making it essential to have mentors or guides. Coding communities connect you with like-minded individuals, providing support, mentorship, code reviews, and much more. Communities like GitHub, DevRel Collective, Codedamn, and Stack Overflow offer platforms where professionals share experiences, network, and help each other grow.
“If you want to go quickly, go alone. If you want to go far, go together.” – African Proverb
Build Projects
Building projects is an excellent way to apply theoretical knowledge practically. Projects demonstrate your skills to potential employers and provide practical experience. Start with simple projects like a basic website, a coin flip app, or a calculator. These projects will help you solidify your learning and build a portfolio showcasing your capabilities Learn java Programming in Bhopal.
Conclusion
No one is born a technical expert; everyone starts as a non-technical person when learning to code. Regardless of your background—whether you are a biology student, an electrical engineer, or a 12th pass out—learning to code is within your reach. The beginning may be frustrating, but persistence will pay off as the process becomes easier over time. The effort you put into writing your first ""Hello World"" code will yield compounding returns, opening up opportunities in the tech industry.
Remember, Java coaching in Bhopal, coding is about instructing a computer on what to do and how to do it using a programming language. By following the steps outlined in this guide, you can embark on a successful coding journey, gain valuable skills, and achieve your career goals in the dynamic world of software development.
The technology landscape is continuously evolving, making it essential to have mentors or guides. Coding communities connect you with like-minded individuals, providing support, mentorship, code reviews, and much more. Communities like GitHub, DevRel Collective, Code damn, and Stack Overflow offer platforms where professionals share experiences, network, and help each other grow. Mastering data structures and algorithms is crucial for job opportunities, especially with major tech companies like Google, Microsoft, and Meta. Data structures are ways to store and organize data efficiently, while algorithms are steps to solve specific problems. These skills are essential for coding interviews and solving complex problems.
#Java coaching in Bhopal#Learn java Programming in Bhopal#Java coding Classes in Bhopal#Robotics Programming in Bhopal#Programming or Language classes in Bhopal#Coding classes for beginners in Bhopal#full stack development institute in Bhopal
0 notes
Text
Python Basics: Variables, Loops & Functions Explained Simply

🔹 Introduction to Python Programming
Python is like the cool friend who can suddenly make everything easier. Whether one is creating a calculator, automating mundane gestures, or simply dreaming of developing their own game, Python is often the first language anyone can turn to. But why?
👉 Join our full Python course to start learning step by step.
Being Python-Friendly for a Beginner
Because it has a clean readable syntax without a lot of techno babble. Python feels like English. You do not have to memorize some wild symbols or worry about setup-heavy jargon. It is just simple and direct, yet powerful.
What Can You Build with Python?
Python builds web apps, machine learning models, multimedia dashboards, even robots. Instagram, Dropbox, and Netflix all `speak` Python. Now, that is a cool thing!
🔹 Getting Started with Python
How to Install Python
To install Python and start coding, go to python.org. Click on download and install it just as you would install any application. Make sure you check the box that says "Add Python to PATH!"
Writing Your First Python Program
Open the terminal or IDLE, and type now:
python
print("Hello, world!")
Hit Enter. Boom! You just wrote your first Python program!
🔹 Understanding Variables in Python.
What Are Variables?
Think of variables as containers; they contain data that you may want to access later. Think of it like a jar with a label- the variable stands for something we can retrieve and reuse.
python
name = "Alice"
age = 25
Here, name holds "Alice," and age holds 25.
Python Variable Naming Rules
Start with a letter or underscore (_)
Cannot begin with a number
Use snake_case for readability
Be descriptive: user_age is better than x
Different Data Types in Python
Python variables can store:
Integers: 5
Floats: 3.14
Strings: "hello"
Booleans: True, False
Lists: [1, 2, 3]
Dictionaries: {"name": "Alice", "age": 25}
🔹 Introduction to Loops in Python
What Are Loops?
They let you execute the same set of commands repeatedly without having to copy the code a hundred times.
For Loop in Python
Perfect when you know exactly how many times to repeat something.
python
for i in range(5):
print("Loop number",i)
While Loop in Python
Perfect for when you don’t really know quite how many times you’re going to do something — you simply go on until a state-of- affairs has been met.
python
x = 0
while x < 5:
print(x)
x += 1
Loop Control Statements
break: terminates loop execution prematurely
continue: skips all statements in the current iteration and jumps to the next iteration of the loop
pass: does nothing; it is used as a placeholder
Also Read: Why Is Python A Good Programming Language For Beginners
🔹 Python Functions Made Easy
What Is a Function?
A function is a reusable block of code. It's like a recipe, one written once and used whenever a situation arises.
def greet():
print("Hello!")
Built-in Vs User-Defined Functions
Built-in: Already present, like print(), len(), and type()
User Defined: Created by you via def.
How to Define a Function
python
def say_hello(name):
print("Hello", name)
Call it using say_hello("Alice")
Function Parameters and Return Values
You can pass information into a function and possibly get a result back:
python
def add(a, b):
return a + b
🔹 Practical Examples of Variables, Loops & Functions
Simple Calculator Using Functions
python
def add(a, b):
return a + b
print(add(10, 5))
Looping through a List of Names
python
names= ["Alice", "Bob", "Charlie"]
for name in names:
print("Hello", name)
Using Variables to Keep Score
python
score = 0
score += 10
print("Your score is", score)
🔹 Common Mistakes Beginners Make
Variable Name Confusion
name and Name can be entirely different variables; Python is case-sensitive.
Infinite Loops
If you forget to update a variable used in a while loop, the program might never terminate.
Forget Return Statements
If a function is not specified with return, no value is returned; it simply performs an action.
🔹 Tips to Improve Your Python Skills
Practice Small Projects
Start with building a calculator, to-do app, or number guessing game.
Read Others' Code
Check out GitHub, follow tutorials, and watch how others approach a problem.
Use Online Platforms for Coding Practice
Try HackerRank, LeetCode, or Codecademy to get your practice and challenges.
Conclusion
Python is a beginner's best friend, especially once you understand the concepts of variables, loops, and functions. These concepts form the basis of almost every program. You will use these tools at every stage, from printing 'Hello World' to building a weather app.
So go ahead, code, break things, fix things, and most importantly, have fun with it. Python is always there for you!
🎓 Want to go beyond Python? Check out our full programming course library and take the next step in your coding journey.
At TCCI, we don't just teach computers — we build careers. Join us and take the first step toward a brighter future.
Location: Bopal & Iskcon-Ambli in Ahmedabad, Gujarat
Call now on +91 9825618292
Visit Our Website: http://tccicomputercoaching.com/
Note: This Post was originally published on https://tccicomputercoaching.wordpress.com/2025/05/07/python-basics-variables-loops-functions-explained-simply/ and is shared here for educational purposes.
0 notes
Text
Demystifying Python Keywords and Identifiers: A Beginner's Guide with Examples
Unravel the core concepts of Python keywords and identifiers in this beginner-friendly tutorial. Learn what keywords are and how they are reserved for specific functionalities within Python. Explore the rules and conventions for naming identifiers, including variables, functions, classes, and modules, and discover best practices for creating meaningful and descriptive identifiers. With illustrative examples and clear explanations, this guide will equip you with the knowledge to navigate Python's syntax and structure confidently, laying a solid foundation for your journey into Python programming.
0 notes
Text
A Guide to Avoiding Common Programming Errors in Python
Python, a widely-used programming language known for its simplicity and readability, is not immune to errors. In this guide, we'll explore some of the most prevalent programming errors in Python and provide practical tips on how to steer clear of them.
In order to gain the necessary skills and information for successfully navigating this ever-changing landscape, anyone seeking to master the art of digital marketing should register in Best Python Training in Pune .
Syntax Errors: Syntax errors arise when code violates Python's syntax rules, often due to missing colons, parentheses, or incorrect indentation. To prevent syntax errors, it's crucial to carefully review code for missing or misplaced symbols and ensure consistent and correct indentation.
Name Errors: Name errors occur when attempting to use an undefined or out-of-scope variable or function. To mitigate name errors, it is essential to define variables and functions before using them, be mindful of typos, and ensure that variables are accessible within the appropriate scope.
Type Errors: Type errors occur when operations are performed on incompatible object types. For instance, trying to concatenate a string and an integer will result in a type error. To avoid such errors, it is important to pay attention to object types and ensure compatibility before performing operations. Utilizing type-checking functions or libraries can also help identify potential type errors.
Index Errors: Index errors occur when attempting to access elements at invalid indices within sequences like lists or strings. These errors typically result from exceeding the bounds of a sequence. To mitigate index errors, it is crucial to use valid indices within the range of the sequence and exercise caution when iterating over sequences to avoid going out of bounds.
Additionally, choosing the right strategies and techniques is crucial, and obtaining the right skills is equally important. This is where enrolling in the Best Python Online Training can make a significant difference.
Attribute Errors: Attribute errors occur when trying to access non-existent attributes or methods of an object. Mistyping the attribute name or attempting to access an attribute that the object does not possess can lead to attribute errors. To prevent such errors, carefully verify the attributes and methods available for an object or class before accessing them.
Indentation Errors: Python employs indentation to define code blocks instead of braces or keywords. Inconsistent or incorrect indentation can result in indentation errors. To prevent these errors, it is important to adhere to consistent indentation practices throughout the code. Modern text editors and integrated development environments (IDEs) often assist in ensuring proper indentation.
Key Errors: Key errors occur when attempting to access a non-existent key in a dictionary. To avoid key errors, it is advisable to verify the presence of the key in the dictionary using the in keyword or the get() method before accessing it.
While Python's simplicity is one of its defining features, it is not exempt from common programming errors. By being aware of these errors and following best practices, such as reviewing syntax, properly defining variables and functions, validating types and indices, and maintaining consistent indentation, developers can significantly reduce the occurrence of errors and write more robust Python code. Embrace Python's helpful error messages as valuable guides in troubleshooting and resolving these issues. Happy coding!
0 notes
Text
Python Essentials: A Beginner's Manual for Aspiring Coders
Learning Python for Beginners: Getting Started
Welcome to the world of coding! If you're just starting, Python is a fantastic language to begin your coding journey. In this beginner's manual, we'll explore the basics of Python and help you take your first steps into the exciting realm of programming.
Why Python?
Python is like the friendly neighbourhood of programming languages. It's easy to read, write, and understand. Plus, it's versatile – used for web development, data analysis, artificial intelligence, and more! Learning Python can open doors to countless opportunities.
Setting Up Your Python Environment
Before diving into coding, you need to set up your Python programming environment. Don't worry; it's not as complicated as it sounds. Follow these simple steps to install Python on your computer and get ready to code!
Understanding Variables and Data Types
In Python, you work with variables to store and manage data. Think of a variable as a container holding information. We'll cover different data types like numbers, strings, and lists. Understanding these basics will give you the foundation to build more complex programs.
Mastering Python Syntax
Every language has its own set of rules, and Python is no exception. We'll break down the syntax – the way you write code in Python. Learning the correct syntax is crucial because it ensures that the computer understands and executes your instructions correctly.
Making Decisions with Conditional Statements
Coding is like giving instructions to a computer. Sometimes you want it to make decisions based on certain conditions. We'll introduce you to if statements, helping your program make choices and perform different actions depending on the situation.
Repeating Actions with Loops
Imagine doing a task over and over – it gets boring, right? Computers don't get bored, but they do use loops to repeat actions. We'll explore different types of loops, such as for and while loops, making your programs efficient and dynamic.
Functions: Building Blocks of Code
Functions are like mini-programs within your program. They make your code organized and reusable. We'll guide you through creating your functions and show you how they make coding more manageable and enjoyable.
Working with Lists and Dictionaries
Lists and dictionaries are Python's way of organizing data. We'll show you how to create, modify, and use these structures to store information efficiently. Whether you're handling a list of names or a dictionary of contacts, Python's got you covered.
File Handling in Python
As you advance, you'll often need to work with files – reading data from them or writing new information. We'll teach you how to handle files in Python, an essential skill for many real-world applications.
Introduction to Object-Oriented Programming (OOP)
Python supports OOP, a powerful programming concept. We'll provide a gentle introduction to OOP, explaining classes and objects. This knowledge will open doors to more advanced Python programming and other languages that follow OOP principles.
Debugging: Finding and Fixing Mistakes
Mistakes happen, even to the best of coders. We'll introduce you to debugging – the process of finding and fixing errors in your code. Debugging is a crucial skill that will help you become a more confident and effective programmer.
Conclusion: Your Coding Adventure Begins!
Congratulations! You've completed our beginner's manual on learning Python. Remember, coding is a journey, not a destination. Practice regularly, challenge yourself with new projects, and don't be afraid to make mistakes – that's how you learn and grow as a coder.
0 notes
Text
8 Data Science Techniques for Actionable Business Insights
In today's rapidly evolving environment, there is a huge demand for people who can translate data for the business, evaluate data, and make recommendations for the company to follow up on. There is data everywhere. Many businesses have adopted data science, and the position of data scientist is swiftly rising to the top of the list of positions sought by data-centric businesses. The company wants to use the data to make better decisions, be flexible, and compete in the market. Leveraging data science may produce meaningful insights that lead to business success, regardless of whether you run a start up, an established business, or something in between. This post explains about Data Science techniques for actionable business insights
Classification
Classification is finding a function that categorizes a dataset into groups depending on several factors. The training dataset is used to train a computer algorithm, which is subsequently used to classify the data into several groups. The classification algorithm aims to discover a mapping function that transforms a discrete input into a discrete output. If you are seeking the right institution to learn Python for Data Science, choosing H2k Infosys will be the better option.
Regression analysis
Regression analysis is used to make decisions. That is the degree to which two closely linked independent data variables rely on one another. In terms of independent variables that differ from other fixed data. This method aims to create models using datasets to calculate the values of the dependent variables. Learning Python programming for Data Science from a reputed institution can help you to get placement quickly.
Predictive analytics
Predictive analytics uses statistical algorithms and historical data to predict what will happen in the future. This strategy can be a game-changer for companies seeking to predict client requirements, optimize resource allocation, and reduce risks. Predictive analytics can create strategies for customer retention, fraud detection, and demand forecasting in various industries, from finance to healthcare.
Machine Learning
Creating models that can make predictions and judgments based on data is the main goal of the artificial intelligence subfield of machine learning. Businesses can develop predictive models for customer churn prediction, sentiment analysis, and image identification by training algorithms on historical data. Different business processes can use machine learning models, automating decision-making and increasing effectiveness.
Jack knife Regression
This is a time-tested resampling method first described by Quenouille and afterwards given the name Tukey. Due to its strength and lack of parameters, it can be utilized as a black box. Furthermore, non-statisticians who predict the variance and bias of a large population can easily break this rule.

Lift analysis
Assume your boss has requested that you match a model to some data and send a report to him. Based on a model you had fitted and drawn specific conclusions. You now discover a group of individuals at your employment who have all included various models and arrived at various conclusions. You need evidence to back up your findings when your boss loses his head and fires you all.
Time series analysis
Time series analysis focuses on looking at data points gathered over time. This technique is essential for sectors like banking, industry, and healthcare, where historical data might offer insightful information. Businesses can make well-informed choices about inventory management, financial forecasting, and operational optimization by looking at past trends and patterns.
Decision tree
A decision tree is a diagram with a structure similar to a flowchart, where each node represents a test on an attribute and each branch a grade. The routes from the root to the leaf define the categorization rules. The predicted values of difficult options are measured using a decision tree and the closely related impact diagram as an analytical and visual decision support approach in decision analysis.
youtube
Bottom line
Finally, those mentioned above are about the Data Science techniques for actionable business insights. Data science approaches are effective tools for drawing useful insights from the enormous amounts of data currently available to enterprises. You may fully realize the potential of data science for useful business insights if you take the right approach and adopt data-driven insights.
#PythonDataScience#DataScienceInPython#DataScienceCertification#DataScienceCommunity#BigDataPython#Youtube
0 notes
Text
Don't Know About Python Variable Scope? Learn with MarsDevs
Do you have any experience with Python or another programming language? Then, you'll know that variables must be specified before they can be used in your program. The idea of scope governs how variables and names are searched in your code. It controls the visibility of a variable within the code. The scope of a name or variable is determined by where it is defined in your code. The LEGB rule is commonly used to explain the Python scope idea.
Click here to know more: https://www.linkedin.com/posts/marsdevs_dont-know-about-python-variable-scope-activity-7125367421200195584-9tUB
0 notes
Text
How To Scrape Data From Zomato Food Delivery Website?
Introduction

If you want to get your hands on some of the most essential pieces of info from Zomato's app, you can hop on web scraping. Zomato has numerous lumps of data about restaurants, menus, and reviews. Web scraping comes to your resume for collecting this info for various reasons like studying the market, checking competitors, or making your apps.
But before you step into the market, remember that Zomato restaurant data scraping should be fair and follow the rules. Keep an eye on Zomato's terms and rules before you begin your web scraping journey. You can easily use various coding languages like Python and tools to scrape better. You can seamlessly make a scraping plan once you are well-versed in how a website is built and its parts work in HTML. But know that websites change, so your plan might also require updates.
In this blog, we will examine how you can scrape data from the Zomato food delivery website. So, without any further adieu, let's dig deep!
Which Data To Scrape From Zomato Food Delivery Website?
When it comes down to scraping data from various food delivery websites, the list can be a long one. Some of the most common pieces of information that web scrapers consider include:
Restaurant's ID
Restaurant's Name
Address
State
City
Country Code
Postal Code
Cost
Aggregate Ratings
Highlights
Email Id
Cuisines
Latitude
Longitude
Opening Hours
Once this information is gathered, it can be processed and organized in a structured format.
Why Scrape Data From Food Delivering Websites?
Web scraping, a powerful method of collecting information from websites, is critical for gaining valuable insights. While your initial points highlighted the significance of web scraping services in the food delivery sector, there are additional dimensions to explore, each offering unique benefits to businesses.
Enhanced Understanding of the Market
Web scraping is used for more than just pricing information. It serves as a portal for conducting in-depth market research. Businesses can learn a lot about their competitors' pricing strategies, as well as their menu offerings, promotional activities, and delivery options.
Adaptive Pricing Strategies
Real-time monitoring of price changes made by competitors is possible with web scraping. Companies can improve their pricing strategies by gathering information on how competitors adjust their prices in response to variables like shifting demand or seasonal trends. By doing this, they can maintain their profitability while remaining competitive.
Tailored Local Advantage
Web scraping helps businesses understand their competitors' local performance in a world where local preferences matter. Insights are gained by extracting data on specific delivery areas, customer preferences, and regional menu variations, which fuel more precise and impactful marketing campaigns.
Streamlined Operations
Through efficient data scraping services, businesses can extensively improve their operations by gathering competitor information such as contact information, operating hours, and delivery routes. Reduced delivery times and higher customer satisfaction may result from this optimization, based on rival companies' data.
Harvesting Customer Sentiments
Customer reviews are critical in the digital age. Web scraping enables businesses to collect and analyze customer feedback from various platforms.
This aggregate sentiment data can be subjected to sentiment analysis, revealing information about customer preferences, pain points, and trends. With this information, businesses can improve their offerings to meet the needs of their customers better.
Tailored Marketing Approaches
Web scraping expands beyond competitor insights. Businesses can decode individual customer behavior and preferences by aggregating data from food delivery platforms. This data can create personalized marketing campaigns, suggest menu items based on past orders, and foster stronger customer-brand connections.
Forging Strategic Alliances
Extraction of food delivery data also opens the door to future collaborations. Businesses may identify popular restaurants, understand their operational capabilities, and form partnerships. This symbiotic strategy can benefit both parties and result in mutual growth.
Web scraping services as a driver for well-informed decision-making, innovation, and operational excellence for food delivery data and goes beyond simple data collection.
The benefits of online scraping are wide-ranging and include improving pricing tactics, comprehending client sentiment, and streamlining processes. Those who harness the potential of web scraping as the food delivery sector develops will survive and prosper in this cutthroat environment.
Zomato Restaurant Data Scraping - A Brief Overview
Using specific techniques to simplify web content retrieval, particularly from sites like Zomato, is required. The Python' queries' package comes in handy here, removing the need for manual URL manipulation and streamlining HTTP/1.1 queries. It supports the addition of data such as form data and headers. 'BeautifulSoup' is another Python package for parsing complicated HTML and XML and facilitating data extraction.
The target URL must be specified when requesting Zomato's data, primarily for restaurant listings. A loop locates particular HTML div tags with the needed information ('col-s-8 col-l-1by3' class). Iteration extracts data from each restaurant separately, creating an exhaustive list.
The script stores various restaurant data in a 't' variable, including addresses, names, cuisines, pricing, and reviews. HTML 'tr' tags enclose these attributes. Accessing Zomato content is more accessible by leveraging tools such as Requests and BeautifulSoup. This automated method speeds up restaurant data extraction by eliminating the need for manual URL manipulation.
What To Do With The Extracted Food Delivery Data?
A wide range of stakeholders in the food industry and beyond can benefit from the knowledge and opportunities gleaned from mined food delivery data. Here are some ways how you can use the fetched food delivery data from Zomato's website:
Restaurant Information
You can discover new nearby eateries and monitor their popularity by examining details like restaurant names, categories, menus, and images.
Pricing and Discounts Insights
By analyzing data related to deals and discounts, you can undercut the prices of your rivals. After that, you may focus on your pricing strategy to ensure that each offer is fair.
Evaluating Ratings & Reviews
Every multi-location firm may quickly assess the service quality gaps in each location and choose your branding strategy thanks to data connected to ratings and reviews.
Understanding Opening Hours
Determine whether chains and services offer early breakfast or late-night delivery options by studying places where competition has limited operating hours and taking advantage of the market.
Enhanced Marketing Approaches
Utilizing data insights about reasonable pricing and delivery charges, you can collaborate with micro-influencers to optimize your marketing campaigns.
Wrapping Up
Making websites and apps has gotten way better. There are no fixed rules for how today's apps or websites should be. Every business has its reasons for getting info from the web. So, there's no one-size-fits-all way to pick a web scraping solution.
Foodspark is an excellent option to hop on if you opt for Zomato restaurant data scraping. It's one of the top services for web scraping that help students, small businesses, and analysts get essential information from popular websites without making a hole in your pocket.
Stay in contact!
#restaurantdataextraction#grocerydatascraping#zomato api#food data scraping#food data scraping services#restaurant data scraping#web scraping services
0 notes