#React JS coding test questions
Explore tagged Tumblr posts
Text
Backend update
Had the most horrible time working with Sequelize today! As I usually do whenever I work with Sequelize! Sequelize is an SQL ORM - instead of writing raw SQL, ORM gives you an option to code it in a way that looks much more like an OOP, which is arguably simpler if you are used to programming that way. So to explain my project a little bit, it's a full stack web app - an online photo editor for dragging and dropping stickers onto canvas/picture. Here is the diagram.
I'm doing it with Next which I've never used before, I only did vanilla js, React and a lil bit of Angular before. The architecture of a next project immediately messed me up so much, it's way different from the ones I've used before and I often got lost in the folders and where to put stuff properly (this is a huge thing to me because I always want it to be organized by the industry standard and I had no reference Next projects from any previous jobs/college so it got really overwhelming really soon :/) . The next problem was setting up my MySQL database with Sequelize because I know from my past experience that Sequelize is very sensitive to where you position certain files/functions and in which order are they. I made all the models (Sequelize equivalent of tables) and when it was time to sync, it would sync only two models out of nine. I figured it was because the other ones weren't called anywhere. Btw a fun fact
So I imported them to my index.js file I made in my database folder. It was reporting an db.define() is not a function error now. That was weird because it didn't report that for the first two tables that went through. To make a really long story short - because I was used to an server/client architecture, I didn't properly run the index.js file, but just did an "npm run dev" and was counting on all of the files to run in an order I am used to, that was not the case tho. After about an hour, I figured I just needed to run index.js solo first. The only reasons those first two tables went through in the beginning is because of the test api calls I made to them in a separate file :I I cannot wait to finish this project, it is for my bachelors thesis or whatever it's called...wish me luck to finish this by 1.9. XD
Also if you have any questions about any of the technologies I used here, feel free to message me c: <3 Bye!
#codeblr#code#programming#webdevelopment#mysql#nextjs#sequelize#full stack web development#fullstackdeveloper#student#computer science#women in stem#backend#studyblr
15 notes
·
View notes
Text
CodeBattle: The Ultimate 1v1 Real-Time Coding Battle Platform
Introduction
Hello coder! How are you , In the world of competitive programming and coding challenges, real-time battles are becoming increasingly popular. CodeBattle is a cutting-edge 1v1 real-time coding battle platform designed to test programmers’ skills in a fast-paced MCQ-based format. Whether you’re a beginner or an experienced coder, CodeBattle offers an exciting and challenging way to improve your coding knowledge.
In this article, we will dive deep into the development of CodeBattle, covering project structure, technology stack, real-time matchmaking, styling tips, and live demo setup. Additionally, we will provide code snippets to help you understand the implementation better.
Features of CodeBattle
Real-time 1v1 Coding Battles
MCQ-based Questions with a 20-second timer
Live Scoreboard
Leaderboard System (Daily, Weekly, and All-time Rankings)
Secure Authentication (Google/Firebase Login)
Admin Panel to manage questions & users
Fully Responsive UI
Tech Stack: React (Next.js), Node.js, Express.js, MongoDB, and Socket.io
Project Structure
CodeBattel/ ├── frontend/ # React (Next.js) UI │ ├── components/ # Reusable Components │ ├── pages/ # Next.js Pages (Home, Play, Leaderboard, etc.) │ ├── styles/ # CSS Modules / Tailwind CSS │ └── utils/ # Helper Functions │ ├── backend/ # Node.js Backend │ ├── models/ # MongoDB Models │ ├── routes/ # Express Routes (API Endpoints) │ ├── controllers/ # Business Logic │ ├── config/ # Configuration Files │ ├── socket/ # Real-time Matchmaking with Socket.io │ └── index.js # Main Server Entry Point │ └── README.md # Project Documentation
Building the Frontend with React (Next.js)
1. Installing Dependencies
npx create-next-app@latest codebattel cd codebattel npm install socket.io-client axios tailwindcss npm install --save firebase
2. Setting up Tailwind CSS
npx tailwindcss init -p
Edit tailwind.config.js:module.exports = { content: ["./pages/**/*.{js,ts,jsx,tsx}", "./components/**/*.{js,ts,jsx,tsx}"], theme: { extend: {}, }, plugins: [], };
Developing the 1v1 Battle System
1. Setting Up Real-Time Matchmaking
import { io } from "socket.io-client"; import { useEffect, useState } from "react";const socket = io("http://localhost:5000");export default function BattleRoom() { const [question, setQuestion] = useState(null); const [timer, setTimer] = useState(20); useEffect(() => { socket.emit("joinBattle"); socket.on("newQuestion", (data) => { setQuestion(data); setTimer(20); }); }, []); return ( <div> <h1>CodeBattel</h1> {question && ( <div> <h2>{question.text}</h2> <ul> {question.options.map((opt, index) => ( <li key={index}>{opt}</li> ))} </ul> <p>Time Left: {timer} sec</p> </div> )} </div> ); }
Building the Backend with Node.js & Socket.io
1. Installing Dependencies
npm init -y npm install express socket.io mongoose cors dotenv
2. Creating the Server
const express = require("express"); const http = require("http"); const { Server } = require("socket.io"); const app = express(); const server = http.createServer(app); const io = new Server(server, { cors: { origin: "*" } });let rooms = []; io.on("connection", (socket) => { socket.on("joinBattle", () => { if (rooms.length > 0) { let room = rooms.pop(); socket.join(room); io.to(room).emit("newQuestion", { text: "What is React?", options: ["Library", "Framework", "Language", "None"] }); } else { let newRoom = "room-" + socket.id; rooms.push(newRoom); socket.join(newRoom); } }); });server.listen(5000, () => console.log("Server running on port 5000"));
Live Demo Setup
Clone the repo:
git clone https://github.com/ashutoshmishra52/codebattel.git cd codebattel
Install dependencies:
npm install && cd backend && npm install
Run the project:
npm run dev
Open http://localhost:3000 in your browser.
FAQ
Q1: What is CodeBattle?
CodeBattle is a 1v1 real-time coding battle platform where players answer multiple-choice questions under a 20-second timer.
Q2: How does matchmaking work?
Players are randomly paired in real time using Socket.io.
Q3: Can I add my own questions?
Yes! The Admin Panel allows you to add/edit/delete MCQs.
Q4: How do I contribute?
Check out the GitHub repository and submit a pull request.
About the Author
Ashutosh Mishra is a full-stack developer, AI researcher, and content writer with 4+ years of experience. He is the founder of CodeWithAshutosh, a platform dedicated to teaching web development, AI, and competitive coding.
For more coding tutorials and projects, follow Ashutosh Mishra.
Conclusion
CodeBattle is an innovative way to enhance your coding skills in a competitive environment. With real-time battles, an engaging UI, and a powerful backend, it stands out as a top-tier coding battle platform. Start coding, challenge your friends, and rise up the leaderboard!
0 notes
Text
Web App Development Technologies in 2024

The Right Front End Stack for the Right Project
The landscape of web development is brimming with powerful technologies, each with its own strengths and ideal use cases. There’s no single ‘one size fits all’ answer to the question of the most in-demand stack.
Full-stack developers offer the flexibility to handle both front end and back end development, making them a valuable asset for some projects. However, many organizations choose to hire full stack developers based on specific needs.
This allows them to leverage specialists who are experts in particular technologies within a stack (e.g., front end frameworks like React or back end languages like Python).
Top Front End Technologies for Web App Development in 2024!
React Web Development
React, often referred to as React.js, is more than just a framework — it’s a powerful JavaScript library that has revolutionized front end development. Unlike traditional frameworks with rigid structures, React offers a flexible and component-based approach. This allows developers to create dynamic and interactive user interfaces (UIs) with ease.
React’s popularity stems from its efficiency, performance, and ability to adapt to changing requirements. It empowers developers to build UIs that are:
Component-Based: Complex UIs are broken down into reusable components, promoting code organization and maintainability.
Efficient Rendering: React utilizes a virtual DOM (Document Object Model) to optimize UI updates, ensuring smooth performance.
Flexible & Adaptable: React readily integrates with other libraries and frameworks, allowing for customization to fit specific project needs.
Advantages:
– Simplified Learning Curve: React’s clean syntax and component-based approach make it easier to grasp, even for beginners.
– Extensive Library Support: Leverage a vast ecosystem of pre-built JavaScript libraries to accelerate development and access ready-made functionalities.
– Reusable Components: Build modular and reusable components to streamline development, improve code maintainability, and ensure consistency throughout your application.
– Performance Optimization: React’s virtual DOM enables efficient rendering and updates, resulting in a smoother user experience.
– SEO Friendliness: React applications can be SEO-friendly with proper coding practices and, if needed, server-side rendering techniques.
– Handy Development Tools: Utilize a rich set of tools like Redux, DevTools, and testing frameworks to simplify development, debugging, and code management.
– Dynamic Web Application Development: Build interactive and dynamic web applications that adapt to user behavior with ease.
– Comprehensive Testing Capabilities: The component-based architecture facilitates unit testing, promoting overall code quality.
Disadvantages:
– Limited Official Documentation: While extensive community resources exist, official React documentation can be lacking for specific functionalities, requiring additional research.
– Potential SEO Challenges: Client-side rendering can impact SEO in some scenarios. Careful planning and optimization techniques might be necessary.
– JSX Learning Curve: JSX, a syntax extension for JavaScript, can add an initial learning hurdle for developers unfamiliar with it.
– Rapidly Evolving Ecosystem: The fast pace of React development and its ecosystem necessitates ongoing learning to stay up-to-date with the latest advancements.
Angular Web Development
Launched in 2010 by Google, Angular has become a popular framework for front-end development. It leverages TypeScript, a superset of JavaScript, for enhanced code maintainability and type safety. While AngularJS, an earlier version, laid the groundwork, Angular (without the JS suffix) has emerged as the preferred choice for modern development.
Why Angular for Enterprise Applications?
Angular’s strengths make it particularly well-suited for building enterprise-grade web applications:
Scalability: Angular applications are designed to handle the complexity and growing data demands of large-scale enterprise applications.
Structure and Maintainability: The use of TypeScript and a component-based architecture promote clean code, easier maintenance, and reduced development overhead for large projects.
Rich Ecosystem and Support: Angular benefits from a vast ecosystem of libraries, tools, and a large developer community, providing extensive support and resources.
Angular 13: The Latest and Greatest?
Angular releases regular updates, and Angular 13 is the latest iteration. While not necessarily the absolute “future” of web development, it offers several advancements over previous versions, including:
– Improved Performance: Optimizations in Angular 13 ensure smoother user experiences for even demanding applications.
– Enhanced Developer Experience: New features and tooling improvements streamline development workflows.
– Staying Up-to-Date: Upgrading to the latest version ensures access to the newest features and security patches. Angular Development Advantages:
Trusted Foundation: Developed and maintained by Google, Angular offers stability and ongoing support.
Extensive Community: Benefit from a large and active developer community for knowledge sharing, troubleshooting, and finding pre-built components.
Streamlined Development: Two-way data binding simplifies data flow between your application’s model and view, reducing development complexity.
Rapid Prototyping: Angular’s features facilitate iterative development, enabling faster creation and testing of prototypes.
Familiar Templates: Utilize familiar HTML templates for building user interfaces, making it easier for developers with HTML experience to pick up.
Enhanced Code Quality: TypeScript, used with Angular, enforces type safety and promotes cleaner, more maintainable code.
Modular Design: The component-based architecture promotes code reusability and simplifies development of complex applications.
Rich Ecosystem: Angular leverages a vast ecosystem of libraries, tools, and frameworks to extend functionalities and streamline development.
Angular Development Disadvantages:
Steeper Learning Curve: While conceptually similar to HTML, Angular introduces new concepts and patterns that might require more effort to learn for beginners.
Potential for Complexity: Angular’s structure and features can lead to complex codebases for smaller projects, potentially requiring more development effort.
Memory Consumption: In some cases, Angular applications can have a higher memory footprint compared to simpler frameworks.
Enforced Structure: The hierarchical tree-like structure can be less flexible for specific use cases compared to some other frameworks.
Migration Challenges:: Upgrading between major Angular versions can involve significant code changes, requiring careful planning and effort. VueJS Web Developement
VueJS Web Developement
Vue.js has emerged as a compelling contender in the front-end development landscape. This open-source JavaScript framework empowers sweb app developers to create user interfaces and single-page applications (SPAs) with ease.
Key Advantages of Vue.js:
Lightweight and approachable: Compared to some frameworks, Vue.js boasts a gentler learning curve for beginners due to its intuitive nature and focus on core web development concepts.
MVVM Architecture: Vue.js leverages the Model-View-ViewModel (MVVM) architectural pattern, promoting clean separation of concerns and simplified code management.
Flexibility for Migration: Migrating existing web applications can be smoother with Vue.js, thanks to its compatibility with existing HTML structures and the potential for incremental adoption.
Rich HTML Functionality: Vue.js seamlessly integrates with HTML, allowing developers to leverage their existing web development knowledge and avoid complex syntax.
Modern Tooling: Vue utilizes Vite.js, a next-generation bundler, for efficient development workflows and faster build times.
While Vue.js offers many advantages, it’s important to consider some potential drawbacks:
Managing Reactivity: Vue.js’s reactive system can be complex for large-scale applications, requiring careful planning and attention to potential performance bottlenecks.
Balancing Flexibility: The framework’s flexibility can be a double-edged sword. While it allows for customization, it can also lead to inconsistent code structures in large projects if not managed effectively.
Memory Usage Considerations: In some cases, Vue.js applications might have a slightly higher memory footprint compared to certain minimal frameworks. Optimization strategies might be required for memory-constrained environments.
Developer Availability: While the developer community is growing, it might still be smaller compared to some other established frameworks. Finding highly experienced Vue.js developers for large projects could require a wider search.
Resource Ecosystem: The ecosystem of third-party libraries and plugins for Vue.js is still expanding. While extensive resources are available, it might not be as comprehensive as those for more mature frameworks.
ASP .NET Web Development
ASP.NET Core, the open-source and powerful web framework for building modern web applications, excels in backend development. While primarily focused on web application development, ASP.NET Core can be leveraged with other .NET technologies to create various applications.
.NET leverages the Common Language Runtime (CLR) to achieve platform independence. This, along with its strong focus on best practices, empowers developers to build robust applications using their preferred languages. The open-source nature of .NET adds another advantage — you can join a vast and active developer community constantly improving the framework.
Benefits of ASP.NET Core:
Clean Code Structure Encourages separation of concerns, keeping your code organized and maintainable.
Faster Development Provides pre-built features to jumpstart development, saving you coding time.
Flexible and Adaptable Offers extensive customization options and extensibility for unique project needs.
Enhanced Security Built-in security features help protect your web applications from vulnerabilities.
Simplified Management Streamlines monitoring and management processes for your applications.
Cross-Platform Freedom Develop and deploy applications seamlessly across Windows, macOS, and Linux environments.
Considerations for ASP.NET Core:
Learning Curve ASP.NET Core, while well-documented, might require additional learning compared to some very established frameworks due to its evolving nature.
Migration Costs Migrating existing projects from a different framework to ASP.NET Core can involve some investment in time and resources.
Node.js Web Development
Node.js is a highly popular open-source runtime environment built on Chrome’s JavaScript engine (V8). This empowers web development teams to create fast, scalable web applications using JavaScript on both the front-end and back-end.
Node.js paves the way for innovative approaches to server-side development with its unique event-driven architecture.
While Node.js wasn’t necessarily the most used programming language in 2020 (reports may vary on usage statistics), it has gained significant traction among developers for its unique strengths.
Benefits of Node.js:
Effortless Scalability Node.js excels at handling growing applications. Its architecture allows for easy scaling to meet increasing user demands.
Quick Start for Developers JavaScript proficiency translates well to Node.js, making it a breeze for developers to learn and become productive quickly.
Full-Stack Flexibility Embrace JavaScript for both front-end and back-end development, streamlining your workflow and reducing the need for multiple languages.
Thriving Community Benefit from a vast and active community of Node.js developers who contribute libraries, tools, and support.
Real-Time Advantage Node.js excels at handling concurrent requests efficiently, making it ideal for real-time applications like chat or collaborative tools.
Speedy Performance Node’s event-driven architecture minimizes waiting time, leading to faster application performance and improved user experience.
Intelligent Caching Node.js allows for efficient caching mechanisms, further reducing load times and enhancing application responsiveness.
Considerations for Node.js:
Evolving API While uncommon, breaking changes can occur in the Node.js API, requiring developers to adapt their code. Staying updated with the latest releases helps mitigate this.
Asynchronous Mindset Shift Node.js utilizes an asynchronous programming model, which can require a different approach compared to traditional synchronous coding. While powerful, it might have a learning curve for some developers.
CPU-Intensive Tasks Node.js performs best with I/O bound tasks (tasks involving waiting for data). For computationally heavy operations, other technologies might be better suited.
Performance in Specific Scenarios While generally performant, Node.js might not always be the optimal choice for highly complex calculations. Consider the specific needs of your application.
Maturity of Libraries While the Node.js ecosystem is vast, some specialized libraries might not be as mature or widely adopted compared to older, more established languages.
Python Web Development
Both Node.js and Python are popular back end development languages, each with their strengths and weaknesses. The best choice depends on your project’s specific requirements.
1. Web Application Development Python excels in building feature-rich websites and web applications, thanks to its robust frameworks like Django and Flask.
2. Machine Learning and Data Science Python reigns supreme in these fields due to its extensive libraries like NumPy, pandas, and scikit-learn. These tools make data manipulation, analysis, and machine learning model creation a breeze.
3. Task Automation Python’s ability to automate repetitive tasks makes it a valuable asset for streamlining workflows across various domains.
4. Software Testing Python’s readability and vast testing frameworks like Pytest empower developers to write efficient and maintainable test suites.
Beyond traditional programming, Python’s easy-to-learn nature makes it accessible to non-programmers as well. Scientists, accountants, and other professionals can leverage Python for tasks like:
– Data Analysis: Python simplifies data cleaning, exploration, and visualization, making it easier to extract insights from data.
– Financial Modeling: Python’s libraries enable the creation of complex financial models for better decision-making.
With its versatility and ease of use, Python remains a top choice for programmers and non-programmers alike.
Benefits of Python for Developers:
Boost Productivity Python’s clean syntax and focus on readability allow web app developers to write code quickly and maintain it easily. This translates to faster development cycles and more efficient use of time.
Rich Ecosystem of Libraries The vast Python Package Index (PyPI) offers a library for nearly any task imaginable. From data science (NumPy, Pandas) to web development (Django, Flask) and machine learning (scikit-learn), you’ll find powerful tools to streamline your development process.
Gentle Learning Curve Python’s clear and concise structure makes it one of the easiest programming languages to learn. This low barrier to entry makes it ideal for beginners and allows experienced developers to pick it up quickly for new projects.
Integration Powerhouse Python integrates seamlessly with various languages and frameworks, enabling developers to leverage existing components and avoid reinventing the wheel. Additionally, built-in features like modules and packages promote code organization and reusability.
Fine-Grained Control Python offers a good balance between high-level abstraction and low-level control. Developers can work at a level of detail appropriate for the task, making it suitable for various development needs.
Object-Oriented Approach Python supports object-oriented programming principles, allowing developers to create well-structured, maintainable, and reusable code. This promotes modularity and simplifies complex project management.
Web Development Friendly Python’s robust web frameworks like Django and Flask provide powerful tools for building modern and scalable web applications. With these frameworks, developers can focus on core functionalities without getting bogged down in low-level details.
Considerations for Python Development:
Potential Integration Challenges While Python integrates well with many languages, complex integrations with some non-Python systems might require additional effort.
Performance Considerations Compared to compiled languages like C++, Python can be slower for computationally intensive tasks. However, for many applications, Python’s speed is sufficient.
Database Access Options Python has database access layers, but they might not be as mature or optimized as those in languages specifically designed for database interaction. Consider the project’s database needs when choosing a language.
Runtime Errors Python’s dynamic typing can lead to runtime errors if data types aren’t handled carefully during development. Implementing robust testing practices helps mitigate this risk.
Mobile App Development While Python can be used for mobile app development with frameworks like Kivy, it’s not the most common choice for native mobile app development. Consider the target platforms and desired user experience when selecting a language.
Memory Management Python’s automatic memory management can lead to higher memory usage compared to some languages. However, for most web development scenarios, this isn’t a major concern. If memory optimization is critical, explore alternative approaches within Python or consider other languages.
Top Databases in 2024!
AWS (Amazon Web Services)
AWS is a powerful and ever-expanding cloud computing platform from Amazon. It provides a one-stop shop for businesses and individuals to leverage a variety of services to build, deploy, and manage applications in the cloud.
Launched in 2006 by Amazon, AWS has become a dominant force in cloud computing.
Amazon Web Services (AWS) is a powerful cloud computing platform, not just a database. It provides a secure and scalable foundation for organizations to build, deploy, and manage a wide range of applications, regardless of their size or industry-
Scalability and Cost-Effectiveness AWS allows you to scale your computing resources (like storage and processing power) up or down as needed. This flexibility ensures you only pay for what you use, leading to significant cost savings compared to traditional on-premises infrastructure.
Robust Security AWS prioritizes security with a layered approach that includes encryption, access controls, and threat detection. This empowers organizations to safeguard their sensitive data and applications.
Broad Applicability AWS caters to diverse industries and application types. From building web applications and mobile backends to running complex data analytics or machine learning workloads, AWS offers the tools and services needed.
Simplified Management AWS offers a user-friendly interface and well-documented services, making it easy for businesses to get started and manage their cloud resources without needing extensive cloud expertise.
Enhanced Speed and Agility AWS empowers businesses to deploy applications and services faster. The scalable nature of AWS cloud resources allows businesses to react quickly to changing market conditions and innovate at an accelerated pace.
Considerations for Using AWS:
Security is a Shared Responsibility While AWS offers robust security features, it’s important to remember that security is a shared responsibility. Businesses must configure and manage their cloud resources securely to maximize protection.
Potential Support Costs While AWS provides basic customer support, in-depth technical assistance might require additional fees. Carefully evaluate your support needs when budgeting for AWS.
Amazon EC2 Resource Constraints AWS imposes limitations on resources allocated to a single EC2 instance (virtual server) in certain regions. However, requesting higher limits is usually an option. For very demanding workloads, alternative AWS services or configurations might be more suitable.
General Cloud Considerations Cloud computing introduces inherent considerations like potential vendor lock-in, reliance on internet connectivity, and managing costs associated with data egress (transferring data out of AWS). Evaluate your project needs and risk tolerance when deciding if cloud computing is the right fit.
Firebase
In today’s mobile development landscape, selecting a suitable backend solution is crucial for your app’s success. Two popular options are Firebase and AWS. Both offer a comprehensive suite of services but cater to different needs.
This comparison will explore the key features of each platform to help you make an informed decision.
Developed by Google, Firebase is a Backend-as-a-Service (BaaS) platform designed to simplify mobile and web app development. It offers a variety of tools and services to streamline various aspects of the development process, potentially helping you build high-quality applications and expand your user base.
Robust Database Solutions Firebase offers flexible NoSQL databases that store and manage data in JSON-like documents. This structure is ideal for frequently changing or complex data, often encountered in modern applications.
Cost-Effective Development Firebase provides a generous free tier for many of its services, making it a cost-effective option for startups and businesses of all sizes. This allows for building core functionalities without upfront investment.
Effortless Static Hosting Firebase simplifies web content hosting with its built-in static hosting capabilities. This eliminates the need to manage separate web servers, saving development time and resources.
Clear and Comprehensive Documentation Firebase boasts excellent documentation with tutorials, code samples, and clear explanations. This comprehensive library of resources empowers developers to learn and leverage Firebase features effectively.
Intuitive User Interface Firebase’s user interface is well-designed and easy to navigate. This allows developers to quickly access and manage their projects and cloud resources without a steep learning curve.
Seamless Integration Firebase integrates smoothly with various Google products and development tools, streamlining workflows and simplifying development processes.
Actionable Insights with Analytics Firebase Analytics provides valuable insights into user behavior and app performance. This data empowers developers to make data-driven decisions and optimize their applications for better user engagement.
Enhanced App Quality with Testing Tools Firebase offers robust testing services that help developers identify and fix bugs before release. This ensures high-quality applications that deliver a positive user experience.
Serverless Functions for Scalability Firebase’s serverless functions enable developers to build scalable back-end logic without managing servers. This simplifies development and reduces infrastructure overhead.
Integration of Machine Learning Firebase allows integration with Google’s machine learning capabilities, enabling developers to add intelligent features to their applications without extensive machine learning expertise.
MongoDB
MongoDB is a popular NoSQL database known for its scalability and flexibility. Unlike traditional relational databases, MongoDB stores data in JSON-like documents, offering several advantages:
– Schema Flexibility: Documents can have different structures, allowing you to store data with varying fields without rigid table definitions. This makes MongoDB a good fit for evolving data models and complex data structures.
– High Performance: MongoDB excels at handling large datasets and offers efficient querying capabilities. This makes it suitable for applications that require frequent data updates and retrieval.
– Horizontal Scalability: MongoDB scales horizontally by adding more servers, making it easy to accommodate growing data volumes.
However, it’s important to consider these factors when evaluating MongoDB for your project:
1. Data Relationships
Modeling complex relationships between data points might require additional design considerations in MongoDB compared to relational databases.
2. Schema Enforcement
Without a predefined schema, data consistency might require more development effort compared to strictly typed databases.
Who Uses MongoDB?
While not a one-size-fits-all solution, MongoDB is a popular choice for various applications and industries due to its flexibility and performance:
Real-time Applications: MongoDB’s responsiveness makes it suitable for applications requiring real-time data updates and retrieval.
Content Management Systems (CMS): The schema flexibility allows for storing diverse content types efficiently.
E-commerce Platforms: MongoDB can handle the high volume and variety of data associated with e-commerce transactions.
MongoDB offers a robust query language that allows developers to retrieve specific data efficiently.
Here are some key features:
Precise Field Selection Queries can target specific fields within documents, returning only the data you need. This improves performance and reduces the amount of data transferred.
Range-Based Queries MongoDB allows querying documents based on a range of values for a particular field. This is useful for filtering data within specific boundaries, like dates or product prices.
Regular Expression Support MongoDB supports regular expressions for pattern matching within fields. This enables powerful searches for text data that might have variations or specific patterns.
Flexible Return Options Queries can return entire documents, specific fields, or even random samples of data depending on your needs. This level of control allows developers to optimize their queries for different use cases.
By leveraging these capabilities, developers can write efficient queries that retrieve the exact data they need, optimizing performance and reducing unnecessary data transfer.
Selecting the optimal technology stack is a critical decision that lays the groundwork for any web application development project. To make an informed choice, consider these key factors:
Project Requirements: Clearly define your project’s goals, functionalities, and target audience. The tech stack should align with the specific needs of your application.
Team Expertise: Evaluate your development team’s skills and experience. Choosing a stack that leverages their existing knowledge can streamline development and reduce the learning curve.
Market Landscape: Consider current technology trends and the availability of resources like libraries and frameworks for your chosen stack. A mature stack with a large community often offers more extensive support.
Scalability and Maintainability: Think about the long-term vision for your application. The tech stack should be able to accommodate future growth and simplify ongoing maintenance efforts.
Security Considerations: Prioritize security throughout the development process. Choose technologies with a strong track record of security and ensure your team follows security best practices.
By carefully evaluating these factors, you can make a well-informed decision about the tech stack that will empower your team to build a successful and sustainable web application.
Tech Stack Decisions: Driven by Project Needs
The functionalities and features you envision for your project significantly influence the technology choices you make. For instance, building a real-time chat application necessitates technologies like WebSockets to ensure seamless communication.
When it comes to performance and scalability, some programming languages and frameworks excel. Microservices architecture, containerization (like Docker), and cloud services work together to handle high workloads and enable horizontal scaling for applications that require it.
On the other hand, if your project is a smaller, simpler application with minimal anticipated changes, a monolithic architecture and a straightforward language like PHP can be a cost-effective option for both development and future maintenance.
In essence, the ideal tech stack aligns with your project’s specific requirements, striking a balance between functionality, performance, and long-term maintainability.
Building on Your Team’s Strengths:
Leveraging your development team’s existing knowledge is a strategic move. When developers work with familiar technologies, they experience:
Enhanced Productivity: Reduced learning curves lead to faster development cycles and quicker time-to-market.
Efficient Coding: Existing knowledge translates to cleaner, more maintainable code.
Stronger Decision-Making: Experience with the technology allows for better-informed technical choices.
However, new project requirements or evolving trends might necessitate the introduction of new technologies. Here are two approaches to consider:
Hybrid Team Approach: Engage external specialists to collaborate with your core team. This allows for knowledge transfer and skill development within your team while leveraging the specialist’s expertise in the new technology.
Strategic Outsourcing: Partner with a reputable IT staff augmentation company specializing in projects like yours. This can be ideal for complex projects or situations where in-house expertise is limited.
The key takeaway?
Align the chosen approach with your team’s existing skillset and project complexity. This minimizes the learning curve, maximizes efficiency, and keeps your team motivated throughout the development process.
The Power of Market Trends
Staying attuned to current market trends can give your project a significant edge. Here’s how:
Competitive Advantage: Leveraging trending technologies can translate into innovative features, a more engaging user experience, or enhanced security, differentiating your project from competitors.
Talent Pool: A tech stack in high demand means a larger pool of skilled developers familiar with the chosen technologies. This simplifies the recruitment process and ensures access to qualified personnel for ongoing maintenance or future development.
Streamlined Development: Popular tech stacks often benefit from a wider range of readily available third-party integrations and libraries. These tools can streamline development workflows and accelerate project completion.
However, it’s important to strike a balance. While staying current is valuable, don’t chase fleeting trends. Focus on technologies that demonstrably align with your project’s goals and offer long-term value.
How the Right
Web Application Development Technology
Drives Success
Building a Unified Team Through Technology
A standardized tech stack, with the same programming languages, frameworks, and tools across the team, offers a powerful advantage: enhanced collaboration. Here’s how:
Shared Knowledge and Support: Developers can readily understand and contribute to each other’s code, fostering knowledge sharing and mutual support. This streamlines development processes and reduces roadblocks.
Streamlined Communication: A common language of technology eliminates confusion and allows for clearer communication within the team. Discussions about code become more efficient and productive.
Consistent Best Practices: Standardized tools and frameworks often lead to well-documented best practices and coding guidelines. These serve as a shared reference point, ensuring code consistency and simplifying onboarding for new team members.
By leveraging a unified tech stack, your team can work together more effectively, achieve better results, and lay the foundation for long-term project success.
Performance Gains with the Right Tech Stack
A well-considered tech stack goes beyond just functionality. It lays the groundwork for a high-performing application:
Scalability and Efficiency: The chosen technologies should be able to handle a growing user base and increasing data demands without compromising performance. This ensures your application can adapt and thrive as it scales.
Performance Optimization: The tech stack should include tools and technologies that are known for their efficiency and speed. This translates to a smoother user experience for your application’s end users.
Ongoing Improvement: Selecting technologies with a strong track record of regular updates ensures access to performance enhancements and security patches for a future-proof application.
By prioritizing performance through your tech stack choices, you can create a product that delivers a consistently smooth and responsive user experience, even as your user base grows.
Optimizing Development Costs: The Benefits of a Standardized Tech Stack
A standardized tech stack delivers benefits beyond streamlined development. It translates to significant cost savings in several ways:
Reduced Development Time: Familiarity with the chosen technologies allows your team to work efficiently and develop code faster. This translates directly to lower labor costs associated with development.
Minimized Troubleshooting: Popular tech stacks often benefit from active developer communities and robust support systems. When issues arise, developers can quickly find solutions, reducing troubleshooting time and associated expenses.
Automation Advantages: Modern tech stacks frequently include built-in automation tools for deployment, testing, and monitoring. This frees up valuable developer time, minimizes manual effort, and ultimately leads to cost savings.
By leveraging a standardized tech stack, you can optimize your development process, reduce overall project costs, and free up your team to focus on innovation.
How Tech Stack Decisions Shape Industry Leaders
Choosing the right tech stack is fundamental to creating a sustainable and scalable product. Many of today’s tech giants credit their success, in part, to well-informed tech stack decisions.
Here are some real-world examples:
Netflix
This streaming giant leverages a powerful combination of technologies:
Frontend: React (user interfaces) and Redux (state management) for a dynamic and responsive user experience.
Backend: A mix of Java, Spring Boot, and Node.js allows for efficient web application development and global content delivery.
Databases: Netflix utilizes various databases like Cassandra, MySQL, and Elasticsearch to cater to different data storage requirements.
This strategic tech stack empowers Netflix to:
– Manage a Large User Base: The microservices architecture facilitates easier maintenance and scaling as the user base grows.
Deliver Global Streaming: The backend combination enables seamless streaming experiences worldwide.
Create an Engaging User Interface: React and Redux contribute to a rich and interactive experience that keeps users engaged.
Handle Diverse Data: Different databases effectively manage various data types, from user profiles to content recommendations.
Airbnb: A Tech Stack Tailored for User Experience and Growth
Similar to Netflix, Airbnb has achieved success in part due to its strategic tech stack choices. Here’s a breakdown of their key technologies:
Frontend: Airbnb prioritizes a user-friendly interface with a combination of JavaScript, React, and Redux. This trio enables a dynamic and responsive user experience that keeps users engaged.
Backend: Airbnb leverages a diverse backend stack:
Ruby on Rails: This framework powers the main website and services, offering a developer-friendly environment for rapid feature updates.
Python: Airbnb utilizes Python for machine learning capabilities, enabling data-driven insights and personalization.
Node.js: This JavaScript runtime environment handles specific microservices within the application.
Databases: Airbnb utilizes a multi-database approach, including MySQL, PostgreSQL, and Redshift. Each database caters to specific data needs, allowing Airbnb to efficiently collect, analyze, and gain valuable insights from vast amounts of user data.
This combination empowers Airbnb to:
– Prioritize User Experience: The front end tech stack ensures a dynamic and user-friendly interface.
– Maintain Development Agility: Ruby on Rails and Node.js facilitate rapid feature updates and innovation.
– Unlock Data-Driven Decisions: The use of Python for machine learning and diverse databases allows Airbnb to leverage data for informed decision-making.
By carefully selecting each technology to address specific needs, Airbnb has built a tech stack that supports their user experience, growth, and data-driven approach.
IProgrammer as Your Web Application Development Company
Here’s why partnering with us for your web application tech stack selection is the secret sauce to your web app’s success:
Seasoned Expertise: We don’t just follow trends; we understand the nuances of each technology and its impact on your specific project.
Future-Proof Foundations: We build with scalability and long-term maintainability in mind, ensuring your web app can adapt and thrive as your business grows.
Performance Optimization: Our team prioritizes speed, security, and a seamless user experience, delivering web applications that delight your audience.
Ready to cook up something extraordinary with iProgrammer’s Web Application Development Services?
Contact our experts today and craft the perfect tech stack recipe for your next web application development masterpiece.
Blog Reference Link — https://www.iprogrammer.com/web-app-development-technologies-in-2024/
#iprogrammer#webappdevelopment#webapplication#webapplicationdevelopment#webapplicationdevelopmenttechnologies#webappdevelopmenttechnologies#webapplicationdevelopmentservices#webapplicationdevelopmentcompany
0 notes
Text
WHAT ARE THE FEATURES OF REACT JS COURSE?
Features of React JS Course: Unlocking the Power of React
Introduction:
Welcome to the world of React JS, where dynamic web applications come to life with ease. If you're considering delving into the Features of React JS Course, you're in the right place. In this comprehensive guide, we will explore the ins and outs of this course, shedding light on its unique features and how it can benefit you.
Why Choose React JS?
The decision to learn React JS is a smart one. React JS, a JavaScript library developed by Facebook, is renowned for its ability to create user-friendly, efficient, and interactive web applications. Let's explore some compelling reasons to choose this course:
User Interface (UI) Excellence: React JS excels in creating highly responsive and visually stunning user interfaces. It ensures a seamless and enjoyable user experience.
Component-Based Architecture: React's component-based approach makes code reusable and maintainable, saving you time and effort.
Vibrant Community: The React JS community is extensive and supportive, providing a plethora of resources, plugins, and components.
Efficiency and Performance: React optimizes performance, making your web applications faster and more efficient.
SEO-Friendly: React can be easily integrated with SEO strategies, enhancing the visibility of your web apps.
Career Opportunities: Learning React JS opens doors to a wide range of job opportunities, as it is in high demand.
Versatile: React can be used for various types of applications, from e-commerce sites to social media platforms.
Course Structure
Now that you're convinced of the value of React JS, let's delve into the structure of the Features of React JS Course:
Fundamentals of React: Lay a strong foundation by understanding the basics of React and its core concepts.
Component Development: Learn to create and manage components efficiently for a dynamic UI.
State and Props: Master the handling of state and props to build interactive applications.
Routing: Explore React Router for creating seamless navigation in single-page applications.
Hooks: Dive into React Hooks, making your code cleaner and more maintainable.
Context API: Understand how to manage the state of your application effectively.
Building a Complete Project: Apply your knowledge by building a complete React application from scratch.
Testing and Debugging: Discover best practices for testing and debugging your React applications.
FAQs
How long does it take to complete the Features of React JS Course?
The duration can vary depending on your prior experience with JavaScript and web development. On average, it takes approximately 2-3 months to complete the course.
Is prior coding experience necessary?
While prior coding experience is not mandatory, having a basic understanding of HTML, CSS, and JavaScript will be beneficial.
What kind of projects can I build after completing the course?
You can develop a wide range of projects, from personal blogs and e-commerce websites to interactive web applications.
Are there any prerequisites for the course?
No, there are no specific prerequisites, but a passion for web development and a desire to learn are essential.
Can I get help and support while learning?
Absolutely! The React JS community is known for its support and resources. You can find answers to your questions and join discussions on various forums.
Is this course suitable for beginners?
Yes, the course is designed to cater to beginners and those with some coding experience.
Conclusion
The Features of React JS Course is your gateway to becoming a proficient React developer. With a solid foundation in React's core concepts, you'll be equipped to create dynamic and interactive web applications that stand out. Embrace the world of React and unlock a world of possibilities in web development.
1 note
·
View note
Text
React Developer Technical Assessment Test for Hiring: Best Practices
In the competitive world of web development, hiring the right React developer can be a game-changer for your projects. ReactJS, with its component-based architecture and virtual DOM, has become one of the most popular libraries for building modern web applications. To ensure you find the best fit for your team, conducting a well-structured react technical test is essential. In this article, we will explore the best practices for designing a React Developer Technical Assessment Test that effectively evaluates a candidate's React skills, problem-solving abilities, and coding proficiency. By following these practices, you can identify top-notch React developers who will contribute to the success of your projects.
Where to Find Qualified React JS Developers?
Online Job Boards and Platforms: Start your search by posting job listings on popular platforms such as LinkedIn, Stack Overflow, and Indeed. These platforms have a vast pool of qualified ReactJS developers actively seeking opportunities.
Freelance Platforms: If you require freelance or contract ReactJS developers, platforms like Upwork, Toptal, and Freelancer offer a wide range of skilled freelancers.
Dedicated Developer Platforms: Consider using platforms like Hired, Gigster, or Gun.io, which specifically connect businesses with top-tier developers after a rigorous vetting process.
Tech Meetups and Hackathons: Offline events like tech meetups and hackathons can be great opportunities to network and connect with passionate and proactive ReactJS developers in your local community.
How to Hire a ReactJS Developer?
Determine Your Project Requirements: Clearly define your project's scope, technology stack, and specific ReactJS skills required to find the right fit for your project.
Conduct a React Assessment Test: Use a well-designed React assessment test to evaluate candidates' technical prowess, problem-solving abilities, and practical application skills. Include scenarios relevant to your project to assess real-world capabilities.
Conduct a Technical Interview: Follow up the assessment test with a technical interview to delve deeper into candidates' problem-solving approach, communication skills, and project experiences.
Review Past Projects and Experience: Examine candidates' portfolios and past projects to assess their coding quality, problem-solving capabilities, and domain expertise.
Finalize the Contract: After shortlisting promising candidates, finalize the contract with clearly defined terms, project scope, and compensation details.
Top ReactJS Interview Questions
Basic Understanding and Knowledge Questions:
What is JSX, and how is it different from standard JavaScript?
Explain the differences between functional and class components in React.
Elaborate on the purpose and advantages of React Hooks.
Practical Application and Problem-Solving Questions:
Develop a React component that fetches and displays data from an API.
Describe your approach to managing state in a complex React application.
Discuss the benefits and drawbacks of using Redux for state management.
Questions Specific to Your Project:
How would you implement [specific feature relevant to your project] using React?
Have you worked with [specific technology or library used in your project] in your past projects?
React Assessment Test-Based Discussion
Defining the Test Objectives:
Clearly outline the objectives of the React assessment test and the specific skills you aim to evaluate.
Aligning Test Objectives with Job Requirements:
Ensure that the test questions align closely with the ReactJS role's necessary skills.
Assessing Problem-Solving Skills and Knowledge:
Include coding challenges and real-world scenarios to assess candidates' ability to handle practical problems.
Emphasizing Practical Coding Tasks:
Prioritize hands-on coding tasks to evaluate candidates' practical skills.
Designing the Test
Choosing Problems and Setting Time Limits:
Select problems that challenge candidates within a reasonable time frame.
Balancing Theoretical Questions and Practical Coding Tasks:
Achieve a balance between theoretical questions and coding challenges to assess both knowledge and application.
Including Real-World Problems:
Integrate real-world scenarios to evaluate candidates' approach to authentic challenges.
Test Content
Focus on Core Concepts:
Assess candidates' understanding of fundamental ReactJS concepts, such as JSX, components, and props.
Incorporate Real-World Scenarios:
Present problems that reflect real-life challenges faced by developers.
Assess Coding Skills:
Include coding exercises to test a candidate's ability to write clean, efficient, and maintainable code.
Implement Time Constraints:
Set appropriate time limits to evaluate candidates' time management skills.
Include Domain-Specific Challenges:
Incorporate relevant challenges to assess candidates' domain-specific knowledge, if applicable.
Managing the Process
Choose the Right Platform:
Select a suitable platform or testing environment to administer the React assessment test.
Set a Regular Review for Biased or Discriminatory Questions:
Ensure the test questions are unbiased and free from discriminatory elements.
Pilot Testing:
Conduct a pilot test with your team to identify and rectify any issues with the test before candidate evaluations.
Conducting the Test
Providing Clear Instructions:
Furnish candidates with clear instructions for taking the React assessment test, including time limits and specific requirements.
Ensuring a Fair and Consistent Testing Environment:
Guarantee that all candidates have an equal opportunity to take the test under fair conditions.
Monitoring the Test:
Monitor the assessment process to address any technical issues that may arise promptly.
Evaluating the Test
Grading Rubric:
Create a grading rubric to evaluate candidates consistently and fairly.
Assessing Clean and Efficient Code:
Evaluate candidates based on their ability to produce clean, well-organized, and efficient code.
Understanding of React Principles:
Gauge candidates' understanding of React principles and best practices.
Problem-Solving Skills:
Assess candidates' problem-solving skills based on their approach to and resolution of given challenges.
Providing Constructive Feedback:
Offer constructive feedback to candidates, regardless of the outcome, to assist them in improving their skills.
Conclusion
Designing an effective Technical Assessment Build with React is crucial for making informed hiring decisions and building a skilled development team. By aligning the test objectives with job requirements, including practical coding tasks and real-world scenarios, and providing constructive feedback to candidates, you can ensure a fair and thorough evaluation process. Remember that an ideal React developer should not only possess strong React skills but also demonstrate problem-solving abilities, clean coding practices, and a deep understanding of React principles. Implementing these best practices will help you attract and hire talented React developers who will drive innovation and excellence in your web development endeavors
.
#assessment built with react#react technical test#react assessment test#react developer test#react assessment#react coding test#react skills test#react skill test#Technical Assessment Build with React
0 notes
Text
How to Find a React Developer with UI/UX Skills?
React has become one of the most popular JavaScript libraries for building dynamic and interactive user interfaces. As web applications become increasingly sophisticated, the demand for skilled React developers who possess UI/UX skills is on the rise. Finding the right React developer who can not only write robust code but also design intuitive and visually appealing interfaces can be a challenging task. In this comprehensive guide, we will explore various strategies and best practices to help you find a React developer with UI/UX skills who will elevate your web application to new heights.
1. Define Your Requirements
Before starting your search for a React developer with UI/UX skills, it is crucial to define your project's requirements clearly. Outline the scope of the project, desired features, and the level of expertise needed in React and UI/UX design. Additionally, consider factors like project duration, team collaboration, and budget. Having a clear understanding of your requirements will enable you to target the right candidates.
2. Job Descriptions and Postings
Crafting a compelling and detailed job description is essential for attracting the right candidates. The job description should highlight the core skills required, such as React proficiency, knowledge of front-end technologies, and UI/UX design experience. Clearly state the level of expertise you expect and any specific projects the candidate will be involved in.
Ensure that the job posting is well-distributed across relevant platforms, such as job boards, developer communities, social media, and tech forums. Additionally, consider leveraging industry-specific platforms or attending tech conferences to reach out to potential candidates directly.
3. Review Portfolios and Previous Projects
One of the most effective ways to assess a React developer's UI/UX skills is by reviewing their portfolios and previous projects. Look for examples of web applications they have built using React, and assess the quality of the user interfaces they have designed. Analyze how they handle responsiveness, interactivity, and overall user experience.
When reviewing portfolios, pay attention to the diversity of projects they have worked on. A developer with experience in various industries and application types will likely bring a broader perspective and versatility to your project.
4. Conduct Technical Assessments
To evaluate a React developer's coding proficiency, consider conducting technical assessments. These assessments can range from simple coding challenges to more comprehensive take-home projects. You can find ready-made coding challenges or create one tailored to your specific project requirements.
Additionally, consider conducting code reviews of the candidate's previous projects to gain insight into their coding practices, code structure, and adherence to best practices. Ensure that the technical assessments are relevant to your project and focus on React-related tasks.
5. Evaluate UI/UX Design Skills
Assessing a React developer's UI/UX skills requires a different approach from technical assessments. You can request candidates to present their design process, wireframes, and mockups for past projects. Evaluate their ability to create visually appealing and user-friendly interfaces that align with modern design principles.
Ask candidates about their understanding of design systems, user research, and usability testing. A developer who can incorporate UI/UX principles into their React development process is more likely to create intuitive and engaging user experiences.
6. Behavioral Interviews
Apart from technical skills, a React js developer with UI/UX skills should be an effective communicator and a collaborative team player. Conduct behavioral interviews to gauge their problem-solving abilities, communication skills, and adaptability.
Ask questions about how they handle project challenges, their experience working in teams, and their approach to learning new technologies and design trends. Assess their ability to understand user requirements and how they translate those into effective UI/UX implementations.
7. Test Soft Skills and Cultural Fit
In addition to technical and design skills, consider the soft skills and cultural fit of potential candidates. A React developer with UI/UX design skills should be able to work effectively with other team members, understand and align with your company's values and work culture, and be receptive to feedback and constructive criticism.
Conduct informal conversations or social events to interact with potential candidates in a more relaxed setting. This can help you get a better sense of their personality and how well they would integrate into your existing development team.
8. Engage in Remote Hiring
In a globalized workforce, you are not restricted to hiring locally. Embrace the possibility of remote hiring to access a broader talent pool of React developers with UI/UX skills. Remote developers can bring fresh perspectives and diverse experiences to your team while reducing the limitations imposed by geographical boundaries.
Use video interviews and collaboration tools to effectively communicate and assess remote candidates. Additionally, consider offering a trial period to evaluate their work compatibility with your team and project.
Conclusion
Finding a skilled React developer with UI/UX skills requires a thoughtful and well-structured hiring process. By defining your project requirements, crafting compelling job descriptions, reviewing portfolios, conducting technical and design assessments, and evaluating soft skills, you can identify the ideal candidate who will bring value to your development team and elevate your web application's user experience. Embrace remote hiring to access a broader talent pool and remember to consider not only technical proficiency but also the candidate's ability to collaborate and fit into your team's culture. With a robust hiring process in place, you can find the perfect React developer with UI/UX skills to bring your web application to life.
0 notes
Text
React.js Skills Assessment Online Test
It is a front-end, open source JavaScript library released for creating user interface components. This library allows developers to build optimistically performing web applications without reloading all the data for a particular web page. Furthermore, React.js allows you to handle the display layer of web and mobile applications.
Quizack React.js tutorial will assess skills based on ongoing trends
Quizack React.js Your skills assessment will be really effective if you are given the latest and authentic information and this is not possible if you are consulting random online resources. It will only be possible if you have subject matter experts with years of practical knowledge. So we follow this approach and have the most relevant industry experts.
Your skills and abilities will be assessed and assessed through the latest topics, 100% verified answers, typical scenarios, and interactive online sessions. You can quickly prepare in minutes for upcoming online assessments, IT exams, freelance skills tests, and job interviews.
Experts have designed the Quizack React JS Skill Test to cover topics
Event handling
Rendering functions
React rules and facts
React multiple choice quiz
Mounting method
Component optimization
React JS Coding Test Questions
Quickly diagnose scenarios through React JS interactive online practice
For the sake of your best online practice, we have made the React.js Q&A practice session available. This online exercise has no limitations. You can browse the questions of your choice and see their answers on the spot. To further encourage you, we have presented questions in the same format as in the actual exams. Consequently, our online practice will provide you with an understanding of how to tackle scenarios in real time and what the possible solutions would be.
React.js Online Test is an evaluation tool. Yes, you can also call it a virtual session because it has been designed to pick randomly selected questions one by one. Here, you will be asked to submit responses within the set time limit. At the end, you will see a list of correct answers for cross-checking purposes.
Who can join us?
You want to be a React.js consultant
Looking for the React.js interview questions and answers
Aspiring to become a React.js developer
I want to become a React.js front-end developer
Need to take IT exam, online assessment, independent skills test
#react.js#react#React.js skill Assessment#React JS skill test#React JS coding test questions#React JS online practice#React.js Q&A#React.js Online Test#React.js interview questions and answers#React.js Developer#React.js Front End Developer#React.js online Assessment#react.js interview questions#react js skill assessment online test#react js coding test questions and answers#react js test online#interview Question And Answers#interview online test#interview online MCQs#interview test MCQs
0 notes
Text
Comparative Study on Flutter State Management
Background
I am going to build a new flutter app. The app is aimed to be quite big. I'm going to need a state management tools. So, I think it’s a good idea to spent some time considering the options. First of all, i do have a preference on flutter’s state management. Itu could affect my final verdict. But, I want to make a data based decision on it. So, let’s start..
Current State of the Art
Flutter official website has a listing of all current available state management options. As on 1 Aug 2021, the following list are those that listed on the website.
I marked GetIt since it’s actually not a state management by it’s own. It’s a dependency injection library, but the community behind it develop a set of tools to make it a state management (get_it_mixin (45,130,81%) and get_it_hooks (6,100,33%)). There’s also two additional lib that not mentioned on the official page (Stacked and flutter_hooks). Those two are relatively new compared to others (since pretty much everything about flutter is new) but has high popularity.
What is Pub Point
Pub point is a curation point given by flutter package manager (pub.dev). Basically this point indicate how far a given library adhere to dart/flutter best practices.
Provider Package Meta Scores
Selection Criteria
I concluded several criteria that need to be fulfilled by a good state management library.
Well Known (Popular)
There's should be a lot of people using it.
Mature
Has rich ecosystem, which mean, resources about the library should be easily available. Resources are, but not limited to, documentation, best practices and common issue/problem solutions.
Rigid
Allow for engineers to write consistent code, so an engineer can come and easily maintain other's codebase.
Easy to Use
Easy in inter-component communication. In a complex app, a component tend to need to talk to other component. So it's very useful if the state manager give an easy way to do it.
Easy to test. A component that implement the state management need to have a good separation of concern.
Easy to learn. Has leaner learning curve.
Well Maintained:
High test coverage rate and actively developed.
First Filter: Popularity
This first filter can give us a quick glance over the easiness of usage and the availability of resources. Since both are our criteria in choosing, the first filter is a no brainer to use. Furthermore when it’s not popular, there’s a high chance that new engineers need more time to learn it.
Luckily, we have a definitive data to rank our list. Pub.dev give us popularity score and number of likes. So, let’s drop those that has less than 90% popularity and has less than 100 likes.
As you can see, we drop 6 package from the list. We also drop setState and InheritedWidget from the list, since it’s the default implementation of state management in flutter. It’s very simple but easily increase complexity in building a bigger app. Most of the other packages try to fix the problem and build on top of it.
Now we have 9 left to go.
Second Filter: Maturity
The second filter is a bit hard to implement. After all, parameter to define “maturity” is kinda vague. But let’s make our own threshold of matureness to use as filter.
Pub point should be higher than 100
Version number should be on major version at least “1.0.0”
Github’s Closed Issue should be more than 100
Stackoverflow questions should be more than 100
Total resource (Github + Stackoverflow) should be more than 500
The current list doesn’t have 2 parameter defined above, so we need to find it out.
So let’s see which state management fulfill our threshold.
As you can see, “flutter_redux” is dropped. It’s not satisfied the criteria of “major version”. Not on major version can be inferred as, the creator of the package marked it is as not stable. There could be potentially breaking API changes in near future or an implementation change. When it happens we got no option but to refactor our code base, which lead to unnecessary work load.
But, it’s actually seems unfair. Since flutter_redux is only a set of tool on top redux . The base package is actually satisfy our threshold so far. It’s on v5.0.0, has pub point ≥ 100, has likes ≥ 100 and has popularity ≥ 90%.
So, if we use the base package it should be safe. But, let’s go a little deeper. The base package is a Dart package, so it means this lib can be used outside flutter (which is a plus). Redux package also claims it’s a rich ecosystem, in which it has several child packages:
As i inspect each of those packages, i found none of them are stables. In fact, none of them are even popular. Which i can assume it’s pretty hard to find “best practices” around it. Redux might be super popular on Javascript community. We could easily find help about redux for web development issue, but i don’t think it stays true for flutter’s issue (you can see the total resource count, it barely pass 500, it’s 517).
Redux package promises big things, but as a saying goes “a chain is as strong as its weakest link”. It’s hard for me to let this package claim “maturity”.
Fun fact: On JS community, specifically React community, redux is also losing popularity due to easier or simpler API from React.Context or Mobx.
But, Just in case, let’s keep Redux in mind, let’s say it’s a seed selection. Since we might go away with only using the base package. Also, it’s might be significantly excel on another filter. So, currently we have 4+1 options left.
Third Filter: Rigid
Our code should be very consistent across all code base. Again, this is very vague. What is the parameters to say a code is consistent, and how consistent we want it to be. Honestly, i can’t find a measurable metric for it. The consistency of a code is all based on a person valuation. In my opinion every and each public available solutions should be custom tailored to our needs. So to make a codebase consistent we should define our own conventions and stick on it during code reviews.
So, sadly on this filter none of the options are dropped. It stays 4+1 options.
Fourth Filter: Easy to Use
We had already define, when is a state management can be called as easy to use in the previous section. Those criteria are:
Each components can talk to each other easily.
Each components should be easy to test. It can be achieved when it separates business logic from views. Also separate big business logic to smaller ones.
We spent little time in learning it.
Since the fourth filter is span across multiple complex criteria, I think to objectively measure it, we need to use a ranking system. A winner on a criteria will get 2 point, second place will get 1, and the rest get 0 point. So, Let’s start visiting those criteria one by one.
Inter Component Communication
Let’s say we have component tree like the following diagram,
In basic composition pattern, when component A needs something from component D it needs to follow a chain of command through E→G→F→D
This approach is easily get very complex when we scale up the system, like a tree with 10 layers deep. So, to solve this problem, state management’s tools introduce a separate class that hold an object which exposed to all components.
Basically, all state management listed above allows this to happen. The differences located on how big is the “root state” allowed and how to reduce unnecessary “render”.
Provider and BLoC is very similar, their pattern best practices only allow state to be stored as high as they needed. In example, on previous graph, states that used by A and B is stored in E but states that used by A and D is stored in root (G). This ensure the render only happen on those component that needed it. But, the problem arise when D suddenly need state that stored in E. We will need a refactor to move it to G.
Redux and Mobx is very similar, it allows all state to be stored in a single state Store that located at root. Each state in store is implemented as an observable and only listened by component that needs it. By doing it that way, it can reduce the unnecessary render occurred. But, this approach easily bloated the root state since it stores everything. You can implement a sub store, like a store located in E to be used by A and B, but then they will lose their advantages over Provider and BLoC. So, sub store is basically discouraged, you can see both redux and mobx has no implementation for MultiStore component like MultiProvider in provider and MultiBlocProvider in BLoC.
A bloated root state is bad due to, not only the file become very big very fast but also the store hogs a lot of memory even when the state is not actively used. Also, as far as i read, i can’t find any solution to remove states that being unused in either Redux and Mobx. It’s something that won’t happen in Provider, since when a branch is disposes it will remove all state included. So, basically choosing either Provider or Redux is down to personal preferences. Wether you prefer simplicity in Redux or a bit more complex but has better memory allocation in Provider.
Meanwhile, Getx has different implementation altogether. It tries to combine provider style and redux style. It has a singleton object to store all active states, but that singleton is managed by a dependency injector. That dependency injector will create and store a state when it’s needed and remove it when it’s not needed anymore. Theres a writer comment in flutter_redux readme, it says
Singletons can be problematic for testing, and Flutter doesn’t have a great Dependency Injection library (such as Dagger2) just yet, so I’d prefer to avoid those. … Therefore, redux & redux_flutter was born for more complex stories like this one.
I can infer, if there is a great dependency injection, the creator of flutter redux won’t create it. So, for the first criteria in easiness of usage, i think, won by Getx (+2 point).
There is a state management that also build on top dependency injection GetIt. But, it got removed in the first round due to very low popularity. Personally, i think it got potential.
Business logic separation
Just like in the previous criteria, all state management also has their own level of separation. They differ in their way in defining unidirectional data flow. You can try to map each of them based on similarity to a more common design pattern like MVVM or MVI.
Provider, Mobx and Getx are similar to MVVM. BLoC and Redux are similar to MVI.
In this criteria, i think there’s no winner since it boils down to preference again.
Easy to learn
Finally, the easiest criteria in easiness of usage, easy to learn. I think there’s only one parameter for it. To be easy to learn, it have to introduced least new things. Both, MVVM and MVI is already pretty common but the latter is a bit new. MVI’s style packages like redux and bloc, introduce new concepts like an action and reducer. Even though Mobx also has actions but it already simplified by using code generator so it looks like any other view model.
So, for this criteria, i think the winner are those with MVVM’s style (+2 Point), Provider, Mobx and Getx. Actually, google themself also promote Provider (Google I/O 2019) over BLoC (Google I/O 2018) because of the simplicity, you can watch the show here.
The fourth filter result
We have inspect all criteria in the fourth filter. The result are as the following:
Getx won twice (4 point),
Provider and Mobx won once (2 point) and
BLoC and Redux never won (0 point).
I guess it’s very clear that we will drop BLoC and Redux. But, i think we need to add one more important criteria.
Which has bigger ecosystem
Big ecosystem means that a given package has many default tools baked or integrated in. A big ecosystem can help us to reduce the time needed to mix and match tools. We don’t need to reinvent the wheel and focused on delivering products. So, let’s see which one of them has the biggest ecosystem. The answer is Getx, but also unsurprisingly Redux. Getx shipped with Dependency Injection, Automated Logging, Http Client, Route Management, and more. The same thing with Redux, as mentioned before, Redux has multiple sub packages, even though none of it is popular. The second place goes to provider and BLoC since it gives us more option in implementation compared to one on the last place. Finally, on the last place Mobx, it offers only state management and gives no additional tools.
So, these are the final verdict
Suddenly, Redux has comeback to the race.
Fifth Filter: Well maintained
No matter how good a package currently is, we can’t use something that got no further maintenance. We need a well maintained package. So, as always let’s define the criteria of well maintained.
Code Coverage
Last time a commit merged to master
Open Pull Request count
Just like previous filter, we will implement ranking system. A winner on a criteria will get 2 point, second place will get 1, and the rest get 0 point.
So, with above data, here are the verdicts
Getx 4 point (2+2+0)
BLoC 4 point (1+1+2)
MobX 0 point (0+0+0)
Provider 1 point (0+0+1)
Redux 0 point (0+0+0)
Lets add with the previous filter point,
Getx 10 point (4+6)
BLoC 5 point (4+1)
MobX 2 point (0+2)
Provider 4 point (1+3)
Redux 2 point (0+2)
By now we can see that the winner state management, that allowed to claim the best possible right now, is Getx. But, it’s a bit concerning when I look at the code coverage, it’s the lowest by far from the others. It makes me wonder, what happen to Getx. So i tried to see the result more closely.
After seeing the image above, i can see that the problem is the get_connect module has 0 coverage also several other modules has low coverage. But, let’s pick the coverage of the core modules like, get_core (100%), get_instance(77%), get_state_manager(51%,33%). The coverage get over 50%, not too bad.
Basically, this result means we need to cancel a winner status from Getx. It’s the win on big ecosystem criteria. So, lets subtract 2 point from the end result (10-2). It got 8 points left, it still won the race. We can safely say it has more pros than cons.
Conclusions
The final result, current best state management is Getx 🎉🎉🎉. Sure, it is not perfect and could be beaten by other state management in the future, but currently it’s the best.
So, my decision is "I should use GetX"
1 note
·
View note
Text
From Meh To Great: My Experience with NodeJS Browser Testing since 2015
A lot can change in a few years. Just look at the state of affairs in the JavaScript (JS) test automation space.
In 2015, if you asked me my opinion on JS and browser testing, I would've said "Avoid using JS altogether as much as possible, but if you need to use JS use Protractor."
Now, my position has completely flipped: "WebdriverIO is the best choice overall for browser (and mobile app) UI test automation, and I would definitely encourage JS-based tools over Java-based tools."
(If you're thinking "Where do you think JS tools for test automation be in 2026?" my answer is "No friggin idea".)
I think it might be helpful to think through why this position has flipped, since it might be helpful to understand the current landscape of JS-based testing tools but also for browser test automation tools more generally. Not just that, but JS-based tools are now applicable beyond purely JS projects and organizations, with Playwright going cross-language after beginning in JS. Test tools with JS aren't just surviving, but also thriving.
First, back in '15, Angular was de rigeur in the front-end JS framework world. React was only coming out, and tools like VueJS and Svelte were still many years away. Angular had a lot of mindshare and a lot of usage, so naturally a test framework that came along with it would get a lot of attention as well. And the Angular project (correctly) treated browser testing as a first-class citizen in its ecosystem, putting appropriate resources behind getting Protractor right. Protractor itself had at least one full-time developer at Google dedicated to it and a lively GitHub with issues and bugs being opened a closed.
As well, JS was a dramatically different language then. Promises were being incorporated into NodeJS itself, meaning asynchronicity had a better API to work with than function callbacks. ES6 classes were a few years away, and even npm scripts were not widely used in 2015. All of these features of NodeJS (or lack thereof) also helped buoy Protractor's interest from the development and testing communities. Since Protractor used WebdriverJS, the JS language bindings provided by the Selenium project, and WebdriverJS made use of promises, Protractor code was as idiomatic and "standard" as possible for NodeJS around 2015.
Still, despite all of the above, JS was an asynchronous language. Since virtually all browser-based automated tests were designed to be run synchronously, learning promise syntax and semantics were an extra and nontrivial step in learning to write good Protractor tests. As well, writing page objects was, well, tricky in a language that didn't have the concept of class-based objects. There was a lot of overhead in writing good tests in Protractor compared to Java or Python. Similar projects for writing browser-tests in NodeJS ranged from not quite mature enough (WebdriverIO) to almost unusable (NightwatchJS) mostly because these projects were also relatively young. Add in the fact that Protractor was Angular-specific and Node tooling wasn't as fully developed as compared to Java (remember Grunt for running things?), choosing Java over Protractor (for example) was a somewhat easy choice.
Let's roll forward to 2021.
The world has changed, and with it so has server-side JS. Now class-based object-oriented programming is possible in JS, and defining and running scripts from a package.json is standard fare. Server-side JavaScript, both the language and tooling around it, has matured quite quickly. Now writing scalable codebases of mostly synchronous code (including test projects) that are maintainable is completely possible in NodeJS. Along with this, the maturity of tools such as WebdriverIO and NightwatchJS has grown too. There is even a new category of tools that do no use Selenium in any way (using the client-side JS bindings or the W3C protocol) including Cypress, Playwright and TestCafe. There is a complete buffet of choices for anyone who wants to write browser-based test automation in JS these days.
And in my opinion, the best of these tools is WebdriverIO. The main reasons for choosing this are
its has a robust service/plugin ecosystem, which allows teams to pick and choose what functionality they need,
its active community, where questions can be answers, features discussed and general help with the tool is encouraged, and
its well-thought-out API and tooling design allows for different tests and structures to reuse the same code.
Overall WebdriverIO isn't just the best tool (in my opinion) in the NodeJS world, it might be the best choice overall for teams starting with browser (or mobile!) test automation.
Meanwhile, Protractor is reaching the end of its life. For some, this comes as no surprise. Protractor bugs and issues keep steadily increasing for a few years, while contributors and dedicated engineers from Google kept decreasing. Using WebdriverJS and not encapsulating asynchronicity from end users turned out to be a mistake, while Angular-specific features eventually were removed or lost value for test developers. Finally, the Angular project announced that Protractor will not be maintained after the release of Angular 15. The go-to JS tool of my halcyon days of test development will be no more.
I'm reminded of the quote that "The only constant is change". It looks like this definitely applies to the NodeJS test tool landscape.
1 note
·
View note
Text
JavaScript Usage by Industry
We’re continuing our analysis of the results of last winter’s JavaScript Ecosystem Survey, a survey of over 16,000 developers conducted by npm in collaboration with the Node.JS Foundation and the JS Foundation. Our second topic is How JavaScript is used across industries — and, more specifically, how different industries use certain JavaScript tools, frameworks, and practices. To read more about the methodology behind this survey, click here.
We asked about what industry our respondents worked in. 45% of users answered “tech”, revealing an underlying ambiguity in our question. For instance, if you work at Google, do you work at a tech company or an advertising company? What about at Microsoft, which many consider a tech company, but also has advertising and even hardware manufacturing arms? Next time, we’ll ask for more detailed information about industry concentrations.
“We asked about what industry our respondents worked in. The most common answer was “tech” at 45%”
Despite this, we still gathered some good data about how use of JavaScript varies by industry. The top industries were:
finance: 7%
advertising and marketing: 5%
education: 5%
entertainment: 5%
business support and logistics: 4%
healthcare: 4%
retail: 3%
government: 2%
manufacturing: 2%
There were meaningful differences across industries in how and why people use JavaScript. There were also some clear commonalities, not all of which we’re going to mention. But from a statistician’s point of view, the questions where all the industries answered very similarly are useful because it indicates the differences in other questions are “real” and not just random variation.
With 16,000 responses, even the single-digit groups per industry constituted enough data to make meaningful conclusions. We discarded answers from industries with less than 2% responses (i.e. less than 300 individual responses).
JavaScript tools
First, we asked about common tools: bundlers, linters, testing frameworks, transpilers, CSS preprocessors, and more.
Manufacturing across the board uses less of everything — only 51% of manufacturing respondents say they use a testing framework, compared to 75% in finance.
The explanation for this may lie in the answer to another question; “Where does the JavaScript you write run?” 15% of manufacturing developers say their code runs on embedded devices which is twice as much as any other industry. Embedded platforms often have intrinsic transpilers for JS, so you do not need to use your own and have no need for browser-specific optimizations that most of these tools provide.
“Manufacturing across the board uses less of everything — only 51% of manufacturing respondents say they use a testing framework, compared to 75% in finance.”
Put another way: hardware isn’t a browser. This view of manufacturing respondents is backed up by another question, in which 31% of manufacturing respondents say their code is put to use in IoT (Internet of Things). No other industry gets above double digits for that answer. This makes manufacturing an interesting set of answers across the board, as we’ll see.
Finance, on the other hand, uses everything the most. They are the most likely to use a bundler, second-most likely to use a linter (after healthcare), most likely to test, second-most likely to use a web framework (after retail), most likely to use a transpiler, and second-most likely to use a CSS preprocessor (after advertising). Finance just does all the things.
JavaScript frameworks
We’ve examined the popularity of JavaScript frameworks in the past. Our survey data provides another view into these preferences, and the results are enlightening.
Angular was a fairly popular choice across all industries, but strongest in finance. Developers in entertainment were the least likely to use Angular, their strongest preference being for React (65%).
React was the most popular framework in the survey overall, though with strong variations by industry. As mentioned, 65% of developers in entertainment chose it, but that fell to 46% in government and 38% in manufacturing. Manufacturing’s strongest choice for a framework was jQuery (52%), suggesting the industry is a late adopter. Government also had jQuery as its top pick at 52%.
Around 20% of developers in most industries reported using Vue, though it was notably more popular in advertising, with 34% of developers reporting it there.
“We also asked developers how they made decisions about choosing frameworks. Big majorities (60-90%) in every industry reported things like support, features, and stability being important, with little variation.”
Ember did not get a lot of mention from our respondents, with an average of 4% of developers reporting that they used it. Also in this range were Preact (5%), Hapi (5%), Next.js (5%), and Meteor (5%).
A surprisingly popular choice was Electron, which is obviously not a web framework at all but we included in our options. More than 20% of developers in every industry reported using Electron for some projects.
We also asked developers how they made decisions about choosing frameworks. Big majorities (60-90%) in every industry reported things like support, features, and stability being important, with little variation. Security was the lowest ranked concern for frameworks, averaging only 25%, though finance was most concerned at 30%. We’ll go more into attitudes to security later in this post.
Language choices
Obviously everybody in the survey uses JavaScript, but lots of respondents use another primary language as their back-end choice and there were noticeable variations by category.
Python was the choice of the unemployed. This sounds unflattering, but thanks to a poorly-phrased question on our part, the “unemployed” category includes people in full time education. Their questions (such as what level of schooling the respondent had completed) lend weight to the idea that the unemployed category was full of people who are still in school, so we believe Python is popular in education.
Java was the choice of the finance industry by quite a margin—41% versus 27% for the second most popular language in finance, .NET.
PHP was the choice of the advertising industry, again by a huge margin — 49% to 26% for Python, the second most popular. Why advertising companies choose PHP is unclear to us, but PHP is popular with advertising agencies putting together micro-sites. Furthermore, a question about company size showed that over 50% of advertising respondents worked at small companies (less than 50 people), so this category is probably dominated by small shops putting together these sorts of sites.
Our poorly-chosen “.NET” category (not really a language) was no industry’s first choice. Its strongest showing was in the manufacturing category at 33%, a very close second to Python at 34%. However, because we didn’t pick out C# and F# separately, it’s possible these results are skewed by people who use those languages, but don’t use .NET.
Ruby is a relatively unpopular option across all our respondents, chosen by less than 13% in all cases. Its weakest showing was in manufacturing, where only 3% chose it, and its strongest in education, where 13% did.
C and C++ were unsurprisingly most popular in the hardware-dominated manufacturing industry, at 9% and 18% of respondents respectively. C++ was more popular than C across the board. The retail industry is the least fond of C++, with only 4% of respondents reporting using it.
We also polled on Go, Swift, and Rust. Go was about 10% usage across the board, Swift was 3-5%, and Rust about 3% except in manufacturing, where it hit 7%.
Why do you choose JavaScript?
In general, people are pretty clear why they choose JavaScript: the huge ecosystem of libraries. An academic study of the same topic in 2013, An Empirical Analysis of Programming Language Choices (Meyerovich and Rabkin, 2013) exhaustively researched what makes developers choose programming languages and it reached the same conclusion. It’s not controversial to conclude that the modules in the npm registry are a major reason people choose JavaScript. However, people cited a number of other reasons and there were notable variations by industry.
Respondents who say they work in government were the least likely to report that they chose JavaScript for productivity gains, with only 51% saying so versus 60% in the finance industry, where this belief is strongest. Instead, government was most likely to believe that using JavaScript gave them performance gains: 31% of government respondents cite this, while in most other industries only 20-21% said this.
The advertising industry is the one most likely to say that developer satisfaction is a reason they choose to use JavaScript, with 50% of respondents saying so. This is notable since the advertising industry has a lot of PHP developers, and as we’ll see in a future analysis based on programming language choices, high satisfaction with JavaScript is a characteristic shared by most PHP developers.
“Across every industry, solid majorities (more than 90% in every case) expected to use JavaScript more or about the same amount as they had previously in the next 12 months.”
Cost savings as a reason for choosing JavaScript were most cited by respondents who work in finance (41%). This seems pretty logical, as finance is an industry that presumably can be relied upon to think of the costs of things.
The entertainment industry was the most likely to cite the size of the developer pool (41%) while the retail industry was most likely to say the ease of on-boarding new developers (40%) was their reason for choosing JavaScript. While JavaScript has a big pool of developers and we think on-boarding developers in JavaScript is pretty easy, it’s unclear why these industries in particular would note those advantages.
Finally, some people don’t get to choose what programming language they work in. This number was highest in government, at 21%.
Across every industry, solid majorities (more than 90% in every case) expected to use JavaScript more or about the same amount as they had previously in the next 12 months.
Attitudes to security
npm is making a big push to improve the security of the modules in the registry in 2018, so we asked our users about their attitudes toward security. You can see our previous post for a deeper analysis of attitudes to security, but there are some interesting variations by industry.
Firstly, everyone is concerned about the security of the code they write (87-90%) and of the open source code they use (73-79%). Developers who work in tech were the most likely to be concerned, but there wasn’t a lot of variation.
Majorities in every industry reported that they were dissatisfied with current methods of evaluating the security of code (51-60%). Advertising and entertainment were the most likely to say they were dissatisfied with available methods.
“Firstly, everyone is concerned about the security of the code they write (87-90%) and of the open source code they use (73-79%). Developers who work in tech were the most likely to be concerned, but there wasn’t a lot of variation.”
A whopping 90% of people working in education (non-students) were likely to be concerned about the quality of the code they wrote themselves. This was an interesting result as they were also the industry most likely to say they were doing nothing at all to assess security (33%):
The industry most likely to be actively doing something about security is the finance industry. They were the group most likely to participate in code reviews (81%), most likely to have external audits of their code (30%), and the most likely to be using automated scans of their code (52%). The tech industry was a close second to finance in all of these answers.
Private code
We explored the ways developers use and store private code.
Everybody reports having private code and GitHub is an extremely popular place to store it — 93% of the advertising industry report using it, with most industries in the high 80’s. Manufacturing was an outlier here, with only 75% of developers saying they used GitHub. We thought this was a strange result, but it was consistent; when asked how they discovered packages, developers in manufacturing were also the least likely to report using GitHub (14% versus 20% for other groups).
Developers also store private npm packages. Across all industries, about a third of developers said they had created private npm packages. However, their reasons for doing so varied.
“However, by far the most popular reason for everyone using private packages was the most obvious: the packages contain private IP. Majorities from 65% in education to 91% in manufacturing reported this as a reason for creating private npm packages.”
Developers sometimes use private modules to hold test projects which aren’t ready for release. This practice varies widely across industries, with 23% of developers in education saying they do this but only 8% in retail.
More common was keeping code private for release as open source later. Education was likely to do this, with 27% saying so, and government employees following close behind at 25%. Healthcare developers, who reported less engagement with open source, were the least likely to report this as a reason, at 9%.
A big reason for everyone to keep private packages was re-use by their co-workers — including majorities from 53% in finance and 67% in retail.
However, by far the biggest reason for everyone using private packages was the most obvious: the packages contain private IP. Majorities from 65% in education to 91% in manufacturing reported this as a reason for creating private npm packages.
Testing
Finally, we explored attitudes toward testing across the industries.
In keeping with its answers in every other category, finance was the most likely to report that they use a testing framework (88%). Government and manufacturing developers on the other hand were the least likely to use a testing framework, with only 68% of developers in both industries saying so.
Across every industry, the most popular testing framework choice was Mocha (50%), followed by Jasmine (33%) and Jest (19%). Unlike web framework choices, there was less variation between the popularity of testing frameworks across industries.
Conclusions
There were some fascinating differences across the industries. The advertising and entertainment industries often found themselves paired together in terms of practices. Finance was cautious and security-focused. Government and manufacturing were mostly on the opposite end of that scale, with lower reported use of best practices and modern tooling. Whether you’re in these industries or building products for developers in these industries, we hope these results help you get a better sense of the broader universe of JavaScript developers.
Thanks to everyone who took the survey. We’ll be providing more analysis in the near future, so stay tuned!
190 notes
·
View notes
Text
How to Build a Career in React: A Beginner’s Guide
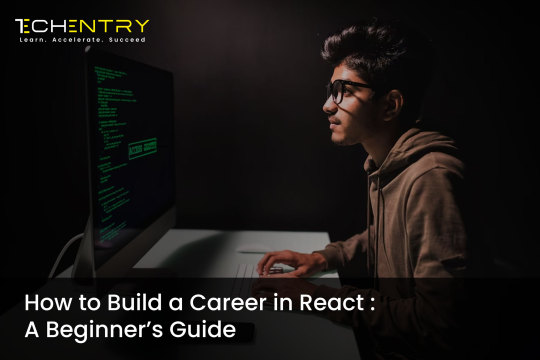
If you are planning to start your career in web development, you must be familiar with Javascript as a programming language. As a developer, you will need to choose a framework to create interactive web pages. React JS (or React) is one of the JavaScript frameworks you'll come across when choosing one to build your first web page.
Developers need a framework that lets them break down complex components and reuse the codes to fulfil projects at a faster pace. React is one of the most well-liked and rapidly expanding Javascript libraries for creating user interfaces. Businesses are switching to React JS, and most front-end developer jobs ask for experience with React JS, which is why both new and seasoned developers are increasing their skill sets by learning this library.
For beginners, not knowing how to learn or what career opportunities are available after learning can be overwhelming. This blog is for you if you have the same questions in mind.
What is React JS?
React, or React JS, is a Javascript library that is based on components. Breaking down complex code into individual pieces helps developers build fast and interactive user interfaces for web and mobile applications. React is quick, flexible, and the most popular front-end JavaScript library in the field of web development. It is best for building web apps, dynamic libraries, and UIs that render responsive web pages.
What does a React JS developer do?
React JS developers create and implement user interface (UI) elements for JavaScript-based web and mobile apps. Developers working with React JS are involved in every stage of UI component design, from the start of the project to final testing.
Why is React JS a good career choice?
Front-end developers can create user interfaces (UIs) more quickly, effectively, and efficiently with the help of React JS. React JS is the ideal front-end framework to start with, even though there are many others that are quite popular, such as Angular and Vue.js.
It's the ideal time to learn React JS since so many industry leaders use it, including Uber, Airbnb, Facebook, and Udemy. A lot of businesses have made significant investments in the React ecosystem to provide new tools and libraries to simplify React development.
• Create front-end components such as icons, images, and gesture-based functionality such as swiping, long-pressing, and drag-and-drop.
• They also work with CSS selectors and HTML tags and translate wireframes into code.
• They design and create new features and are responsible for optimizing the app's functionality to ensure that it runs efficiently on different browsers and devices.
• React developers must test the applications they produce because they are involved at every level of the interface.
How to build your career in React JS
The majority of React developers concentrate on front-end development. Content structuring, visuals, and interactive user experiences are part of front-end development. A front-end development professional certificate is a great way to acquire the knowledge required to equip yourself for an entry-level position. Full-stack engineers work with server-side frameworks and languages like Node.js, Django, and Python.
Obtain a relevant professional certificate through a training program if you're interested in learning more about full-stack development using React.
Some core responsibilities of React developers are:
• Learn the basics of JavaScript: React is a JavaScript library, so it's essential that you have a solid understanding of the language. Start by learning the basics of JavaScript syntax: variables, functions, arrays, and objects.
• Familiarize yourself with React tools: There are a number of tools that are commonly used in React development, including React Developer Tools, Create React App, and Redux.
• Get used to Git. Git is a version control system that's commonly used in React development. It's important that you understand how to use Git to manage your code.
• As you build React applications, be sure to keep track of your work and build a portfolio that showcases your skills.
• Join online communities and attend React meetups to network with other React developers and learn from their experiences.
• React is a constantly evolving technology, so it's crucial that you stay up-to-date with the latest developments and continue to improve your skills.
Conclusion
There are numerous career opportunities for React JS developers, as they are needed in all regions and business sectors. However, to stand out in the hiring and selection process, you must present your portfolio. The ideal approach to demonstrating your skills is to work on real projects and develop applications that are used in the real world.
It’s a daunting process to learn this online by watching some tutorials, and you might feel overwhelmed not knowing where to start. Techentry is a platform that allows both freshmen and working professionals to hone their skills and learn software development in a working environment. With a large team of experienced industry professionals to mentor candidates, Techentry enables real-time learning with practical projects and client management in a physical work setting. Trainees can be a part of project management and track their learning through doubt-solving sessions.
0 notes
Text
10 Must-Have VSCode Extensions for Web Developers to Boost Productivity in 2022
Visual Studio Code is a free and open-source editor for building modern web applications
It helps us with a wide range of extensions that you can use for web development. In this blog, we are going to talk about the 10 most useful extensions which can make your development process easier, efficient and enhance your contribution to teammates.
To install these extensions simply press SHIFT+COMMAND +X or just click on the extension icon of visual studio code then search for the extension and press install.
JavaScript (ES6) Code Snippets
this extension helps you by not writing that repetitive code again and again. I think this is a must-have extension because it provides JavaScript, TypeScript, Vue, React, and HTML code snippets.
2. REST Client
If I ask you how you test your APIs?
Most probably you’ll say, Postman. But with this extension, we can test our APIs and observe their response directly in vs code.
Testing API and integration to the UI is going to be more fun
3. Auto Close Tag
This extension automatically close, any opening tag in HTML and XML. This saves your time by reducing the bug of not closing ending tags. It also works with other Frameworks too.
4.Quokka.js
Wanna see the runtime values of your js or ts code without console.log()?
Here is the solution, with the help of this extension you can see and update your runtime values in your vs code without going to rum in the browser without using console.log() in a browser.
5. Path intelligence
You type the name of the file in statements and it will search and give you suggestions for the file paths of a project. This extension will help you a lot while you’re working on a project that has a large number of files in it. You won’t have to type out all of your filenames.
6. Better Comments
Commenting helps us to understand our or others’ code easily. This extension helps to visually organize your comments by categorizing them into highlighting text, questions, todo, errors/ warnings & strikethroughs so that they’re easy for reading when looking through the project later on which is more human-friendly, readable comments
7: Settings Sync
If you’re someone who works on different machines and when you don’t want to reconfigure the editor everything then this extension will help you a lot.
It creates and stores your configuration in Github gist and synchronizes wherever you want
8. Browser Preview
Let’s imagine you’re developing a site and you change something, not you want to see the changes in your site. So what you are going to do? Go to the browser and look at it. Right?
But no longer you need to do it, Now you simply open a real browser inside the vs code editor to debug and check the changes that you have made.
9. Live Share
When I started my journey in web development, Sometimes I find some bugs hard to solve the search online, and if the online solution does not work I go ask my friend to help me.
And we go to google meet and try to solve that.
But now there is something that can handle this task very easily, Live share extension helps you to share and collaborate your code among your friends or team in real-time at the same time you can chat with them and they can edit in real-time
10. Github Copilot
GitHub Copilot generates code and function suggestions directly on your code editor using natural language cues like comments and method names after being trained on billions of lines of public code.
Conclusion
These extensions really help me to boost my productivity. Are there any others that you think like? If so, please let us know in the comments!
If this article seems useful to you please follow and share to let your friends know about these cool extensions.
1 note
·
View note
Text
Vector icons expo

Vector icons expo how to#
Vector icons expo update#
Vector icons expo android#
Vector icons expo code#
Vector icons expo download#
This is expected to take about five minutes, and you may need to adapt it. Once installation is complete, apply the changes from the following diffs to configure Expo modules in your project. Job search 20 free icons (svg, eps, psd, png files). The following instructions apply to installing the latest version of Expo modules in React Native 0.68. Wildrose technical tournoi belote pascack clipground oradell acle brundall
Vector icons expo download#
Monitoring remote icons Summer Camp - Vector Illustration | Pre-Designed Illustrator Graphics Ĭamp summer illustration vector graphics creativemarket graphic Conalep Logo Download Vector conalep educación vector colegio profesional nacional técnica Expo Vector Icons At | Collection Of Expo Vector Icons īleachers utrustning expon bås festivalen händelse symbolerna linje inkluderade symbolsuppsättning 设备 节日 vectorified attrezzature isolati veicoli speciali aeroporti 集合 作为 Lions Club Logo Clip Art 10 Free Cliparts | Download Images On Vector elements web variety eps Icons_Remote Monitoring - Equinox MHE Format Įxpo vector eps svg Free Technology Vectors Vector Art & Graphics | freevector Radio Show Icons Vector Pack - freeload Vector Art, Stock Graphics A Variety Of Web Design Elements (119643) Free EPS Download / 4 Vector Job Search 20 Free Icons (SVG, EPS, PSD, PNG Files) job icon seeker vector acknowledge pluspng icons psd 1200 gesturing library royalty transparent Expo (70238) Free EPS, SVG Download / 4 Vector Įxpo vector svg eps 65kb Expo Free Vector Download (56 Free Vector) For Commercial Use. format and also Expo (70238) Free EPS, SVG Download / 4 Vector. 11 Images about Job search 20 free icons (SVG, EPS, PSD, PNG files) : Expo Vector Icons at | Collection of Expo Vector Icons, Expo free vector download (56 Free vector) for commercial use. There are 5 other projects in the npm registry using react-web-vector-icons. Start using react-web-vector-icons in your project by running npm i react-web-vector-icons. Latest version: 1.0.2, last published: 3 years ago. The website will be deployed when you merge to master.Job search 20 free icons (SVG, EPS, PSD, PNG files). An adaptation of react-native-vector-icons for react web.
Vector icons expo update#
If it's good to go, publish the final version, update the website version again, then merge.
Open a PR, have someone else like look at it.
Publish an alpha release, switch back the version in the website to that version.
While you're here, it would be kind of you to update the Expo SDK version to latest.
If new icons were added, ensure that they work here.
Go to the website directory, test it out by changing the version to "./" (TODO: investigate this quirk!).
Run yarn when you're done and it'll copy vendor files over to build.
TypeScript/Flow types for Icon/Icon.Button components may need to be updated.
Were any dependencies added? Check imports against those in the current package.json, see why they were added - maybe they support the bin scripts, in which case we need them.
The main thing to look out for are user-facing API changes, the internals are different enough that you don't need to worry about it.
Probably there won't be anything important.
ToolBarAndroid and TabBarIOS are not included in Neither are the native vendor font loading or image source related methods.
Vector icons expo code#
Run git diff **/*.js - do any of the changes look like they should be synced over to the equivalent. Are you looking for a code example or an answer to a question «puncture icons expo vector icons» Examples from various sources (github,stackoverflow, and others).
Things to look out for are new icon fonts or new create-* files. Remove anything that doesn't seem needed. Flaticon, the largest database of free icons.
Run git status and look at the untracked files. Vector icons in SVG, PSD, PNG, EPS and ICON FONT Download over 14,273 icons of conference in SVG, PSD, PNG, EPS format or as webfonts.
Copy files from the cloned directory into src/vendor/react-native-vector-icons, except the dotfiles.
custom colors and size can be added using color and size props. Name the new set and save Create New Set. Another option is to drag your icons over the app (keep in mind you can only import SVG files, all other formats will be ignored). v3 uses a third-party icon library ( such as expo/vector-icons ), with as prop. To create a new set, click the ‘import icons’ button in the top left, or right-click on the left panel > New Set. default Icon type i.e Ionicons has been removed, now v3 does not uses any.
Vector icons expo android#
You should set aside about an hour to do this. Migrating Icon components can broadly described in these points: ios, android and type props have been deprecated. I'll be honest with you, it's not straightforward.
Vector icons expo how to#
Import React from 'react' import įor more usage see Expo icons documentation Maintainers How to upgrade the react-native-vector-icons version

0 notes
Text
The Meta’s Coding Invention: REACT
If you are a coder or programmer, you are aware of the fact that React or React.js is one of the most useful coding languages. However, if you are a newly introduced coder, then there must be a flood of questions in your mind related to “REACT”, which are going to be answered in this blog.
If you wish to become one of the top React developers, read this blog till the end:
What is React?
As mentioned above, React is a coding language for developers. One highlighted fact about React is that React is a coding language divided into two parts. The first is Ract.js or also referred to as React.JS and React Native.
Let’s know the difference between the two in the following words.
What is React JS or React.js?
React JS or React.js is a free and open-source front-end framework for the JavaScript library, which helps in building User Interfaces websites, based on UI components.
React was invented by Meta, formerly known as Facebook back in the year 2011.
Facebook was one of the highly utilized social media networking websites back in those days. So, Facebook invented React JS or React.js as an opened-source coding language.
In 2012, Instagram, which is Facebook’s substitute, also started using React JS.
In the initial days of 2013, React.js wasn’t well accepted by the developers. The reason behind this was, React.js used both Markup and JavaScript language in the same file.
React is currently the most used and popular JavaScript front-end library which has a strong foundation and a large community supporting and using it regularly.
React is popular because it allows coders to edit and add changes as many times as there is any need. If there are any changes required the changes can happen in small parts, the whole coding will remain hinged.
Prerequisites For React JS
Before you start working as a react developer, you have to be known with the below-mentioned coding languages:
HTML
CSS
DOM
Node.js
NPM
ES6
What Is React Native?
React JS was one part, the next is React Native. It is also an open-source User Interface software framework invented and designed by Meta. React Native is merely used to build and create Hybrid Mobile Applications.
React Native can be used to build and create iOS, Android, Android TV, macOS, tvOS, Web, Windows, and UWP.
React Native was launched in the year 2015 by Facebook, currently known as Meta.
React Native allows the top react native developers to build cross-compatible mobile applications. With React Native, the React developers don’t have to worry about learning platform-specific application development languages.
Prerequisites For React Native
React Native developers should be familiar with concepts like functions, objects, arrays, and classes
React Native developers should know HTML, CSS, and JavaScript
Lastly, React Native developers should be known with React JS.
Advantages And Disadvantages Of React JS
Advantages:
Easy to Learn and Use
Reusable Components
SEO friendly
Flexible
Easy to test
Disadvantages:
View Part
JSX as a barrier
The high pace of development
IN A CRUX: React JS was not used as a very much helpful coding language for coders and developers because it was made up of two different coding languages. However, now, as time has passed, the top react developers find “REACT” helpful.
As React JS and React Natives work on front-end UI, the changes are seen clearly at the user’s end. Developers are creating codes every day, and the React variants have just helped enhance the coding experience.
1 note
·
View note
Text
9 Ways Python Development can benefit your business.
Presently as you realise that it is a modern programming language with versatile highlights, let us focus on the elements that will empower you to choose how Python development can profit your business.
User-accommodating
Python includes implicit word article knowledge structures that can be utilized to build quick runtime information structures in Python development. It likewise gives the chance to abnormal state dynamic information including that abatements the length of support code that is required.
Highly Productive Python development
With regards to python development, it gives a question-designed plan, upgraded process control capacities, a solid combination with content preparation techniques, and unit testing structure. All these add to expanding its profitability and also speed. Python development is a decent option if you need to create complex multi-convention organised applications.
Python shop
Python is considered by Google as a matter of first value choice. This is because the most extreme of Google’s devices and present is produced utilising Python development. Truth be told, it is the most secure alternative for the ideal mix abilities for Google’s cloud services and Google Apps.
Free accessibility
Python is free and stays available at no expense in the future. When we say it is free of cost, it means its development set of supporting devices, libraries, and modules is free. Huge numbers of its popular IDEs incorporated development conditions, for example, Pydev with Eclipse, PTVS, and Spyder Python are accessible for free download.
A large network of supporters in Python development
In this cutting-edge period, few open-source networks are great and also applied as Python. You will get full support for a many-sided quality that is found in Python development. There are a huge number of programmers and supporters who deal with the routine keeping in mind the end goal to enhance the language’s centre highlights and functionalities.
Third-Party Modules
Because of the nearness of PyPI(Python bundle), Python associates with different languages and stages effectively. This bundle comprises outsider modules which additionally makes Python more capable.
Within budget
With regards to making an item, inclination and ability may come down to your choice for any language. Python development can be the best choice for your company on the off chance that you are on a budget. Truth be told, Python can be a decent choice when you have greater ventures. It is a perfect choice for startups and bootstrappers in light of its brisk development and less coding alongside C, PHP, Java, and others.
IoT well disposed
In the period of IoT(Internet of things), it has brought countless open doors for Python programmers. Platforms like Raspberry Pi enable developers to build their exciting gadgets like radios, telephones, cameras, even games through Python easily. By utilising propelled concepts in Python development, developers can associate with true markets freely and can homebrew their special contraptions at little to no cost.
Blue chipsets and services
Given Python’s proclivity for scale, it ought not to be astounding why it is the primary language in many Blue chip sites and services. The report incorporates eBay, Yelp, Reddit, Disqus, Dropbox, YouTube, Instagram, PayPal, and games, for example, EVE Online and Second Life, among others. For the Python web community, this indicates acing Python and its popular moved structures like Django would guarantee that you can look for some kind of work or even make your item or service as a startup.
Viitorcloud is an IT company based in Ahmedabad from where you can hire a python developer for your work. A company other than python development offers services like IoT development, blockchain development, AR/VR, AI development, React Js development, and a lot more.
0 notes
Photo

What is the best source to learn JavaScript on the internet for free for web development?
1. Codecademy:
Codecademy is a popular website to learn JavaScript online. Codecademy currently offers Java, Git, UNIX command line and many other courses. It has built itself a reputation for learning at its own pace online thanks to the interactive JavaScript course.
2. Udemy:
One of the best places to study online. You can get online courses using the latest technology and programming language.
Here are some helpful free courses to help you learn cool technology:
a. The Complete JavaScript Course 2019: Building Real Projects b. Bootcamp for web developers c. JavaScript Bootcamp - Creating Real Applications d. Javascript Essentials
3. Coursera
This is another amazing website to learn online. It was founded by Stanford professors Andrew Ng and Daphne Koller and offers online courses, majors and degrees. The best thing about Coursera is that it offers online courses from universities like Stanford, University of London, University of Michigan, Colorado, Imperial College of London and more.
Some of free programming courses from Coursera:
a. Basics of programming with JavaScript, HTML and CSS (Link) b. HTML, CSS and Javascript for web developers (Link) c. Interactivity with JavaScript (link)
Coursera also provides you with a certificate that you can view on your Linkedin profile, but you have to pay for it.
4. Pluralsight:
One of the best websites for learning technologies like web development, mobile development and programming languages like Java, web development framework like Angular, React, Spring Framework, Hibernate, etc.
The website also offers a number of useful courses for learning JavaScript from a beginner's perspective, such as:
a. JavaScript Fundamentals By Liam McLennan b. JavaScript From Scratch By Jesse Liberty c. Basics of Programming with JavaScript By Kyle Simpson
5. freecodecamp.org
This is one of the most amazing social networking sites where you can learn to code, create real projects and find a developer job for free. You will find a collection of tutorials and exercises on JavaScript, data structure and algorithms, frontend development and more. Founded by Quincy Larson, this is a really wonderful place to start your coding journey. They even have a great Facebook group to ask questions and share your learning experiences.
6. Code Combat
CodeCombat is a platform where students can learn computer science while playing real games. The courses are specifically tested to stand out in the classroom, even for teachers with little or no programming experience. They have some web development courses to learn the basics of JavaScript.
7. Code School
This is another website that I really like. Their jQuery tutorial is very cool, but the JavaScript course is not to be missed. It gives you the real opportunity to learn JavaScript online at your own pace for FREE.
8. Learning the Web? - ?Mozilla Developer Network
Mozilla is for the beginner and also the official source for JavaScript information.
9. Learn JavaScript Online? - ?CodeMentor
This website offers a 4 week course on learning JavaScript. In addition to learning the core of JavaScript, you can learn other famous JavaScript libraries and related concepts like Ajax, Json, jQuery, Angular js and so on.
These are the best websites to learn JavaScript online. If you really want to master JavaScript, don't just sit back and relax. Start working on a real project.
0 notes