#installing iptables
Explore tagged Tumblr posts
Text
#TheeWaterCompany
#CyberSecurity #Risk #Reward
!/bin/bash
BACKUP_DIR="/backup" DATA_DIR="/important_data/" ENCRYPTED_BACKUP="$BACKUP_DIR/encrypted_backup_$(date +%F).gpg"
tar -czf $BACKUP_DIR/backup_$(date +%F).tar.gz $DATA_DIR gpg --symmetric --cipher-algo AES256 --output $ENCRYPTED_BACKUP $BACKUP_DIR/backup_$(date +%F).tar.gz rm -f $BACKUP_DIR/backup_$(date +%F).tar.gz echo "Encrypted backup completed."
To refine encryption-related code, consider the following improvements:
Use Stronger Algorithms: Implement AES256 instead of AES128 for better encryption strength.
Add Error Handling: Ensure that the encryption process handles errors, such as failed encryption or permission issues.
Secure Storage of Keys: Use a secure method to store encryption keys (e.g., environment variables or hardware security modules).
Refined Script Example:
!/bin/bash
Encrypt sensitive data with AES256 and store encrypted backup securely
BACKUP_DIR="/backup" ENCRYPTED_BACKUP="/backup/encrypted_backup_$(date +%F).gpg" DATA_DIR="/important_data/"
Perform backup of important files
tar -czf $BACKUP_DIR/backup_$(date +%F).tar.gz $DATA_DIR
Encrypt the backup with AES256
gpg --batch --yes --symmetric --cipher-algo AES256 --output $ENCRYPTED_BACKUP $BACKUP_DIR/backup_$(date +%F).tar.gz
Remove the unencrypted backup file
rm -f $BACKUP_DIR/backup_$(date +%F).tar.gz echo "Backup and encryption completed securely."
This script enhances security by using AES256 and ensures encrypted files are properly handled.
To proceed with creating scripts for securing water companies' networks, we would outline some basic examples and operational strategies that could be implemented. Here’s a breakdown of each element:
Monitoring and Intrusion Detection
These scripts would monitor traffic and detect any suspicious activity on the network.
Example Script: Network Traffic Monitoring
!/bin/bash
Monitor network traffic and detect anomalies
LOGFILE="/var/log/network_traffic.log" ALERT_FILE="/var/log/alerts.log"
Use 'netstat' to monitor active network connections
netstat -an > $LOGFILE
Check for unusual activity, such as unexpected IP addresses
grep "192.168." $LOGFILE | grep -v "127.0.0.1" > $ALERT_FILE if [ -s $ALERT_FILE ]; then echo "Unusual activity detected!" | mail -s "Security Alert: Network Anomaly Detected" [email protected] fi
This script monitors network traffic using netstat, checks for connections from suspicious IP addresses, and sends an alert if any are found.
Intrusion Prevention (Automated Response)
This script would automatically take action to block malicious activity upon detection.
Example Script: IP Blocking on Intrusion Detection
!/bin/bash
Block suspicious IP addresses detected during intrusion attempts
SUSPICIOUS_IPS=$(grep "FAILED LOGIN" /var/log/auth.log | awk '{print $NF}' | sort | uniq)
for ip in $SUSPICIOUS_IPS; do iptables -A INPUT -s $ip -j DROP echo "$ip has been blocked due to multiple failed login attempts" >> /var/log/security_block.log done
This script automatically blocks IP addresses with failed login attempts, adding a layer of protection by preventing brute-force attacks.
Security Updates and Patch Management
Automated patch management ensures that all security vulnerabilities are addressed as soon as updates are available.
Example Script: Automatic Updates
!/bin/bash
Update system packages and apply security patches
echo "Updating system packages…" apt-get update -y apt-get upgrade -y apt-get dist-upgrade -y
Apply only security updates
apt-get install unattended-upgrades dpkg-reconfigure -plow unattended-upgrades
This script ensures that the system receives the latest security patches automatically, which is essential for keeping critical infrastructure secure.
Data Encryption and Backup
Regular backups and ensuring sensitive data is encrypted are vital.
Example Script: Data Encryption and Backup
!/bin/bash
Encrypt sensitive data and create backups
BACKUP_DIR="/backup" ENCRYPTED_BACKUP="/backup/encrypted_backup.gpg"
Perform backup of important files
tar -czf $BACKUP_DIR/backup_$(date +%F).tar.gz /important_data/
Encrypt the backup
gpg --symmetric --cipher-algo AES256 $BACKUP_DIR/backup_$(date +%F).tar.gz
Remove the unencrypted backup file after encryption
rm -f $BACKUP_DIR/backup_$(date +%F).tar.gz echo "Backup and encryption completed."
This script automates backups of sensitive data and encrypts it using gpg with AES256 encryption, ensuring that even if data is accessed illegally, it cannot be read without the encryption key.
Access Control
Strong access control is necessary to ensure that only authorized personnel can access critical systems.
Example Script: Access Control with Multi-Factor Authentication (MFA)
!/bin/bash
Ensure all users have MFA enabled for critical systems
Check if MFA is enabled on SSH login
if ! grep -q "auth required pam_google_authenticator.so" /etc/pam.d/sshd; then echo "MFA is not enabled on SSH. Enabling MFA…" echo "auth required pam_google_authenticator.so" >> /etc/pam.d/sshd service sshd restart else echo "MFA is already enabled on SSH." fi
This script checks if multi-factor authentication (MFA) is enabled on SSH logins, and if not, it enables it, ensuring an additional layer of security.
Security Audits
Regular audits help identify vulnerabilities and ensure the system is secure.
Example Script: Automated Security Audit
!/bin/bash
Run a security audit to check for common vulnerabilities
Check for open ports
echo "Checking for open ports…" nmap -p 1-65535 localhost > /var/log/open_ports.log
Check for outdated software
echo "Checking for outdated software…" apt list --upgradable > /var/log/outdated_software.log
Check file permissions for sensitive files
echo "Checking file permissions…" find /etc /var /usr -type f -name "*.conf" -exec ls -l {} \; > /var/log/file_permissions.log
Send the audit report to the administrator
mail -s "Security Audit Report" [email protected] < /var/log/security_audit_report.log
This script performs a security audit, checking for open ports, outdated software, and sensitive file permission issues, then sends a report to the administrator.
Conclusion
These scripts are designed to help secure the water companies' networks by automating essential security functions like monitoring, response to threats, patching, encryption, and access control. It’s important that these scripts be customized to the specific needs of each company, taking into account their existing systems, infrastructure, and any unique security concerns they may face. Additionally, regular updates to these scripts will be necessary as new vulnerabilities and threats emerge.
For a basic firewall script that blocks unauthorized access and monitors network traffic, here's an example:
!/bin/bash
Define allowed IPs (replace with actual allowed IP addresses)
ALLOWED_IPS=("192.168.1.1" "192.168.1.2")
Block all incoming connections by default
iptables -P INPUT DROP iptables -P FORWARD DROP iptables -P OUTPUT ACCEPT
Allow traffic from specified IPs
for ip in "${ALLOWED_IPS[@]}"; do iptables -A INPUT -s $ip -j ACCEPT done
Log and monitor incoming traffic
iptables -A INPUT -j LOG --log-prefix "Firewall Log: " --log-level 4
This script sets a default block on incoming connections, allows traffic from specific IP addresses, and logs all traffic for monitoring.
4 notes
·
View notes
Text
(this is a small story of how I came to write my own intrusion detection/prevention framework and why I'm really happy with that decision, don't mind me rambling)
Preface
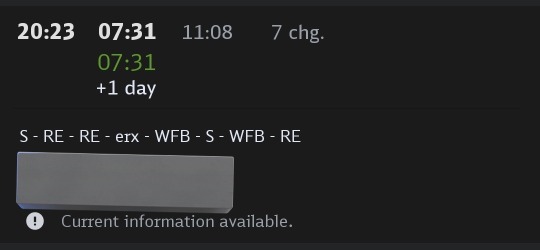
About two weeks ago I was faced with a pretty annoying problem. Whilst I was going home by train I have noticed that my server at home had been running hot and slowed down a lot. This prompted me to check my nginx logs, the only service that is indirectly available to the public (more on that later), which made me realize that - due to poor access control - someone had been sending me hundreds of thousands of huge DNS requests to my server, most likely testing for vulnerabilities. I added an iptables rule to drop all traffic from the aforementioned source and redirected remaining traffic to a backup NextDNS instance that I set up previously with the same overrides and custom records that my DNS had to not get any downtime for the service but also allow my server to cool down. I stopped the DNS service on my server at home and then used the remaining train ride to think. How would I stop this from happening in the future? I pondered multiple possible solutions for this problem, whether to use fail2ban, whether to just add better access control, or to just stick with the NextDNS instance.
I ended up going with a completely different option: making a solution, that's perfectly fit for my server, myself.
My Server Structure
So, I should probably explain how I host and why only nginx is public despite me hosting a bunch of services under the hood.
I have a public facing VPS that only allows traffic to nginx. That traffic then gets forwarded through a VPN connection to my home server so that I don't have to have any public facing ports on said home server. The VPS only really acts like the public interface for the home server with access control and logging sprinkled in throughout my configs to get more layers of security. Some Services can only be interacted with through the VPN or a local connection, such that not everything is actually forwarded - only what I need/want to be.
I actually do have fail2ban installed on both my VPS and home server, so why make another piece of software?
Tabarnak - Succeeding at Banning
I had a few requirements for what I wanted to do:
Only allow HTTP(S) traffic through Cloudflare
Only allow DNS traffic from given sources; (location filtering, explicit white-/blacklisting);
Webhook support for logging
Should be interactive (e.g. POST /api/ban/{IP})
Detect automated vulnerability scanning
Integration with the AbuseIPDB (for checking and reporting)
As I started working on this, I realized that this would soon become more complex than I had thought at first.
Webhooks for logging This was probably the easiest requirement to check off my list, I just wrote my own log() function that would call a webhook. Sadly, the rest wouldn't be as easy.
Allowing only Cloudflare traffic This was still doable, I only needed to add a filter in my nginx config for my domain to only allow Cloudflare IP ranges and disallow the rest. I ended up doing something slightly different. I added a new default nginx config that would just return a 404 on every route and log access to a different file so that I could detect connection attempts that would be made without Cloudflare and handle them in Tabarnak myself.
Integration with AbuseIPDB Also not yet the hard part, just call AbuseIPDB with the parsed IP and if the abuse confidence score is within a configured threshold, flag the IP, when that happens I receive a notification that asks me whether to whitelist or to ban the IP - I can also do nothing and let everything proceed as it normally would. If the IP gets flagged a configured amount of times, ban the IP unless it has been whitelisted by then.
Location filtering + Whitelist + Blacklist This is where it starts to get interesting. I had to know where the request comes from due to similarities of location of all the real people that would actually connect to the DNS. I didn't want to outright ban everyone else, as there could be valid requests from other sources. So for every new IP that triggers a callback (this would only be triggered after a certain amount of either flags or requests), I now need to get the location. I do this by just calling the ipinfo api and checking the supplied location. To not send too many requests I cache results (even though ipinfo should never be called twice for the same IP - same) and save results to a database. I made my own class that bases from collections.UserDict which when accessed tries to find the entry in memory, if it can't it searches through the DB and returns results. This works for setting, deleting, adding and checking for records. Flags, AbuseIPDB results, whitelist entries and blacklist entries also get stored in the DB to achieve persistent state even when I restart.
Detection of automated vulnerability scanning For this, I went through my old nginx logs, looking to find the least amount of paths I need to block to catch the biggest amount of automated vulnerability scan requests. So I did some data science magic and wrote a route blacklist. It doesn't just end there. Since I know the routes of valid requests that I would be receiving (which are all mentioned in my nginx configs), I could just parse that and match the requested route against that. To achieve this I wrote some really simple regular expressions to extract all location blocks from an nginx config alongside whether that location is absolute (preceded by an =) or relative. After I get the locations I can test the requested route against the valid routes and get back whether the request was made to a valid URL (I can't just look for 404 return codes here, because there are some pages that actually do return a 404 and can return a 404 on purpose). I also parse the request method from the logs and match the received method against the HTTP standard request methods (which are all methods that services on my server use). That way I can easily catch requests like:
XX.YYY.ZZZ.AA - - [25/Sep/2023:14:52:43 +0200] "145.ll|'|'|SGFjS2VkX0Q0OTkwNjI3|'|'|WIN-JNAPIER0859|'|'|JNapier|'|'|19-02-01|'|'||'|'|Win 7 Professional SP1 x64|'|'|No|'|'|0.7d|'|'|..|'|'|AA==|'|'|112.inf|'|'|SGFjS2VkDQoxOTIuMTY4LjkyLjIyMjo1NTUyDQpEZXNrdG9wDQpjbGllbnRhLmV4ZQ0KRmFsc2UNCkZhbHNlDQpUcnVlDQpGYWxzZQ==12.act|'|'|AA==" 400 150 "-" "-"
I probably over complicated this - by a lot - but I can't go back in time to change what I did.
Interactivity As I showed and mentioned earlier, I can manually white-/blacklist an IP. This forced me to add threads to my previously single-threaded program. Since I was too stubborn to use websockets (I have a distaste for websockets), I opted for probably the worst option I could've taken. It works like this: I have a main thread, which does all the log parsing, processing and handling and a side thread which watches a FIFO-file that is created on startup. I can append commands to the FIFO-file which are mapped to the functions they are supposed to call. When the FIFO reader detects a new line, it looks through the map, gets the function and executes it on the supplied IP. Doing all of this manually would be way too tedious, so I made an API endpoint on my home server that would append the commands to the file on the VPS. That also means, that I had to secure that API endpoint so that I couldn't just be spammed with random requests. Now that I could interact with Tabarnak through an API, I needed to make this user friendly - even I don't like to curl and sign my requests manually. So I integrated logging to my self-hosted instance of https://ntfy.sh and added action buttons that would send the request for me. All of this just because I refused to use sockets.
First successes and why I'm happy about this After not too long, the bans were starting to happen. The traffic to my server decreased and I can finally breathe again. I may have over complicated this, but I don't mind. This was a really fun experience to write something new and learn more about log parsing and processing. Tabarnak probably won't last forever and I could replace it with solutions that are way easier to deploy and way more general. But what matters is, that I liked doing it. It was a really fun project - which is why I'm writing this - and I'm glad that I ended up doing this. Of course I could have just used fail2ban but I never would've been able to write all of the extras that I ended up making (I don't want to take the explanation ad absurdum so just imagine that I added cool stuff) and I never would've learned what I actually did.
So whenever you are faced with a dumb problem and could write something yourself, I think you should at least try. This was a really fun experience and it might be for you as well.
Post Scriptum
First of all, apologies for the English - I'm not a native speaker so I'm sorry if some parts were incorrect or anything like that. Secondly, I'm sure that there are simpler ways to accomplish what I did here, however this was more about the experience of creating something myself rather than using some pre-made tool that does everything I want to (maybe even better?). Third, if you actually read until here, thanks for reading - hope it wasn't too boring - have a nice day :)
10 notes
·
View notes
Text
Stepping into the Portal: Building a Captive Wi-Fi Experience on our Raspberry Pi
Today's adventure in our Raspberry Pi networking project took a fascinating turn! Having successfully established our Pi as an internet-sharing router via Ethernet, we set our sights on a more sophisticated user experience: implementing a captive portal.
For those unfamiliar, a captive portal is the web page that pops up when you connect to a public Wi-Fi network, often requiring you to agree to terms of service or log in before you can access the internet. This is exactly what we aimed to create for our little network managed by the Raspberry Pi.
Laying the Foundation: Installing a Web Server
Our first step was to install a web server on the Raspberry Pi. We opted for Nginx, a lightweight and powerful choice that's perfect for this task. With a few simple commands in the terminal (sudo apt update and sudo apt install nginx), our web server was up and running. We even verified its status to ensure everything was as it should be.
To make sure our captive portal page would be accessible, we also opened up the necessary ports (HTTP on port 80 and HTTPS on port 443) in the Raspberry Pi's firewall using iptables. We made sure to save these new rules so they persist across reboots.
Crafting the Welcome Mat: Creating the Portal Web Page
Next, we rolled up our sleeves and created a basic HTML page to serve as our captive portal. We replaced the default Nginx welcome page with our own index.html. This simple page includes a welcome message, a (very basic for now!) terms of service agreement, and a button that users will eventually click to gain internet access.
We even added a little bit of basic CSS to make it look presentable. The idea is to have a clear and concise page that informs users and gets their agreement before they hop online.
What's Next? The Magic of Redirection
With the web server in place and our initial captive portal page ready, the next stages will involve the more intricate parts of making the portal truly functional. We'll be diving into:
* DNS Interception: Configuring dnsmasq to redirect all DNS requests from unconnected clients to the Raspberry Pi's IP address.
* HTTP/HTTPS Redirection with iptables: Setting up rules to intercept all web traffic from unauthorized users and force it to our captive portal page.
* The "Agreement" Mechanism: Developing the backend logic (likely a simple script for now) that gets triggered when a user clicks "I Agree," and then modifies the firewall to grant that user internet access.
Today was all about laying the groundwork – installing the necessary tools and creating the content for our captive portal. It's exciting to see the project evolving from basic internet sharing to a more controlled and user-aware network. Stay tuned for the next steps as we delve into the redirection magic!
0 notes
Text
💥 Hướng dẫn cài đặt và chặn IP bằng iptables trên server Linux (CentOS/Ubuntu) 💻🔥
💥 Hướng dẫn cài đặt và chặn IP bằng iptables trên server Linux (CentOS/Ubuntu) 💻🔥 👉 Áp dụng để chặn các IP tấn công, bot xấu hoặc tiết kiệm tài nguyên server 💖 Queen Mobile – Liên hệ hỗ trợ server 0906849968 🌿 1. Cài đặt iptables (nếu chưa có) 🔸 Trên CentOS/RHEL/AlmaLinux: yum install iptables-services -y systemctl start iptables systemctl enable iptables 🔸 Trên Ubuntu/Debian: apt update apt…
0 notes
Text
This tutorial aims to show the steps of installing and configuring iptables on Debian 12 (Bookworm), starting from the installation all the way to executing the first set of rules.
https://greenwebpage.com/community/how-to-install-and-configure-iptables-on-debian-12/
0 notes
Link
#configuration#encryption#firewall#IPmasking#Linux#networking#OpenVPN#Performance#PiVPN#Privacy#RaspberryPi#remoteaccess#Security#self-hosted#Server#Setup#simplest#systemadministration#tunneling#VPN#WireGuard
0 notes
Text
How to Install UFW on Ubuntu 24.04
This post will explain how to install the UFW on Ubuntu 24.04 OS. UFW (Uncomplicated Firewall) is an interface for iptables for configuring a firewall. The UFW firewall is way easier than the iptables for securing the server. It is used daily by system administrators, developers, and other familiar Linux users. The most important thing about the UFW firewall is that it protects the server from…
0 notes
Text
Linux Zero to Hero: Mastering the Open-Source Operating System
Linux, an open-source operating system, is the backbone of countless systems, from personal computers to enterprise servers and supercomputers. It has earned its reputation as a robust, versatile, and secure platform for developers, administrators, and tech enthusiasts. In this comprehensive guide, we explore the journey from being a Linux beginner to mastering its vast ecosystem.
Why Learn Linux?
1. Open-Source Freedom
Linux provides unparalleled flexibility, allowing users to customize and modify the system according to their needs. With its open-source nature, you have access to thousands of applications and tools free of charge.
2. Industry Relevance
Major companies, including Google, Amazon, and Facebook, rely on Linux for their servers and infrastructure. Learning Linux opens doors to lucrative career opportunities in IT and software development.
3. Secure and Reliable
Linux boasts a strong security model and is known for its stability. Its resistance to malware and viruses makes it the operating system of choice for critical applications.
Getting Started with Linux
Step 1: Understanding Linux Distributions
Linux comes in various distributions, each catering to specific needs. Popular distributions include:
Ubuntu: User-friendly, ideal for beginners.
Fedora: Known for cutting-edge technology and innovation.
Debian: Stable and versatile, preferred for servers.
CentOS: Enterprise-grade, often used in businesses.
Choosing the right distribution depends on your goals, whether it’s desktop use, development, or server management.
Step 2: Setting Up Your Linux Environment
You can use Linux in several ways:
Dual Boot: Install Linux alongside Windows or macOS.
Virtual Machines: Run Linux within your current OS using tools like VirtualBox.
Live USB: Try Linux without installation by booting from a USB drive.
Mastering Linux Basics
1. The Linux File System
Linux organizes data using a hierarchical file system. Key directories include:
/root: Home directory for the root user.
/etc: Configuration files for the system.
/home: User-specific data.
/var: Variable files, such as logs and databases.
2. Essential Linux Commands
Understanding basic commands is crucial for navigating and managing the Linux system. Examples include:
ls: Lists files and directories.
cd: Changes directories.
mkdir: Creates new directories.
rm: Deletes files or directories.
chmod: Changes file permissions.
3. User and Permission Management
Linux enforces strict user permissions to enhance security. The system categorizes users into three groups:
Owner
Group
Others
Permissions are represented as read (r), write (w), and execute (x). Adjusting permissions ensures secure access to files and directories.
Advanced Linux Skills
1. Shell Scripting
Shell scripting automates repetitive tasks and enhances efficiency. Using bash scripts, users can create programs to execute commands in sequence.
Example: A Simple Bash Script
bash
Copy code
#!/bin/bash
echo "Hello, World!"
2. System Administration
System administrators use Linux for tasks like:
Managing users and groups.
Monitoring system performance.
Configuring firewalls using tools like iptables.
Scheduling tasks with cron jobs.
3. Package Management
Each Linux distribution uses a package manager to install, update, and remove software:
APT (Ubuntu/Debian): sudo apt install package_name
YUM (CentOS/Fedora): sudo yum install package_name
Zypper (openSUSE): sudo zypper install package_name
Linux for Developers
Linux provides a robust environment for coding and development. Key features include:
Integrated Development Environments (IDEs): Tools like Eclipse, IntelliJ IDEA, and Visual Studio Code are supported.
Version Control Systems: Git integration makes Linux ideal for collaborative software development.
Containerization and Virtualization: Tools like Docker and Kubernetes thrive in Linux environments.
Troubleshooting and Debugging
Learning to troubleshoot is vital for any Linux user. Common methods include:
Viewing Logs: Logs in /var/log offer insights into system errors.
Using Debugging Tools: Commands like strace and gdb help debug applications.
Network Diagnostics: Tools like ping, traceroute, and netstat diagnose connectivity issues.
Linux Certifications
Earning a Linux certification validates your skills and enhances your career prospects. Notable certifications include:
CompTIA Linux+
Red Hat Certified Engineer (RHCE)
Linux Professional Institute Certification (LPIC)
Certified Kubernetes Administrator (CKA)
These certifications demonstrate proficiency in Linux administration, security, and deployment.
Tips for Success in Linux Mastery
Practice Regularly: Familiarity with commands and tools comes through consistent practice.
Join Communities: Engage with Linux forums, such as Stack Overflow and Reddit, to learn from experienced users.
Contribute to Open-Source Projects: Hands-on involvement in projects deepens your understanding of Linux and enhances your resume.
Stay Updated: Follow Linux news and updates to stay informed about advancements and changes.
Conclusion
Mastering Linux is a transformative journey that equips individuals and organizations with the tools to thrive in a technology-driven world. By following the steps outlined in this guide, you can progress from a Linux novice to a seasoned expert, ready to tackle real-world challenges and opportunities.
0 notes
Text
Build and Secure Your Linux Server: A Quick Guide
Want to create a powerful and secure Linux server? Here's your step-by-step guide to get started!
Why Linux? Linux is the go-to for flexibility, security, and stability. Whether you’re hosting websites or managing data, it's the perfect choice for tech enthusiasts.
1. Choose Your Distribution Pick the right distro based on your needs:
Ubuntu for beginners.
CentOS for stable enterprise use.
Debian for secure, critical systems.
2. Install the OS Keep it lean by installing only what you need. Whether on a physical machine or virtual, the installation is simple.
3. Secure SSH Access Lock down SSH by:
Disabling root login.
Using SSH keys instead of passwords.
Changing the default port for added security.
4. Set Up the Firewall Configure UFW or iptables to control traffic. Block unnecessary ports and only allow trusted sources.
5. Regular Updates Always keep your system updated. Run updates often to patch vulnerabilities and keep your server running smoothly.
6. Backup Your Data Use tools like rsync to back up regularly. Don’t wait for disaster to strike.
7. Monitor and Maintain Regular check logs and monitor server health to catch any issues early. Stay ahead with security patches.
0 notes
Text
how to open vpn port on ufw
🔒🌍✨ Erhalten Sie 3 Monate GRATIS VPN - Sicherer und privater Internetzugang weltweit! Hier klicken ✨🌍🔒
how to open vpn port on ufw
VPN-Anschluss öffnen
Ein VPN-Anschluss, auch Virtual Private Network genannt, ermöglicht es Benutzern, eine sichere und verschlüsselte Verbindung zum Internet herzustellen. Durch die Verwendung eines VPN können Sie Ihre Online-Aktivitäten schützen und Ihre Privatsphäre wahren.
Um einen VPN-Anschluss zu öffnen, benötigen Sie zunächst ein VPN-Konto bei einem Anbieter Ihrer Wahl. Sobald Sie über ein Konto verfügen, können Sie die erforderliche Software entweder auf Ihrem Computer, Smartphone oder Tablet installieren. Starten Sie die Anwendung und melden Sie sich mit Ihren Anmeldedaten an.
Sobald Sie angemeldet sind, wählen Sie den gewünschten Serverstandort aus, mit dem Sie eine Verbindung herstellen möchten. Dies kann beispielsweise ein Server in einem anderen Land sein, um auf geo-blockierte Inhalte zuzugreifen oder Ihre IP-Adresse zu verschleiern. Klicken Sie auf "Verbinden" und warten Sie, bis die Verbindung hergestellt ist.
Sobald die Verbindung erfolgreich hergestellt wurde, können Sie sicher im Internet surfen, ohne dass Ihre Aktivitäten von Dritten verfolgt werden können. Ein VPN kann auch nützlich sein, um öffentliche WLAN-Netzwerke abzusichern und Ihre Daten vor Hackern zu schützen.
Insgesamt ist das Öffnen eines VPN-Anschlusses ein einfacher und effektiver Weg, um Ihre Online-Privatsphäre und Sicherheit zu gewährleisten. Es lohnt sich, in ein hochwertiges VPN-Abonnement zu investieren, um von den zahlreichen Vorteilen dieser Technologie zu profitieren.
UFW-Port für VPN freigeben
Um eine UFW-Port für VPN freizugeben, ist es wichtig, die notwendigen Schritte sorgfältig zu befolgen. UFW steht für Uncomplicated Firewall und ist ein benutzerfreundliches Frontend für das iptables-System, das auf vielen Linux-Systemen verwendet wird. VPN, oder Virtual Private Network, ermöglicht es Benutzern, sicher auf das Internet zuzugreifen, insbesondere wenn sie sich in öffentlichen Netzwerken befinden.
Um einen Port für VPN im UFW freizugeben, müssen Sie zunächst sicherstellen, dass UFW auf Ihrem System installiert ist. Dies kann mit dem Befehl "sudo apt-get install ufw" erfolgen. Sobald UFW installiert ist, können Sie die erforderlichen Ports für Ihren VPN-Anbieter freigeben. Dies kann je nach Anbieter variieren, aber häufig werden Ports wie UDP 500 oder UDP 4500 für den Betrieb von VPN benötigt.
Um einen Port freizugeben, können Sie den Befehl "sudo ufw allow /udp" verwenden. Stellen Sie sicher, dass Sie die richtige Portnummer für Ihren VPN-Anbieter angeben. Nachdem der Port freigegeben wurde, können Sie den Status von UFW überprüfen, um sicherzustellen, dass die Regeln korrekt angewendet wurden.
Es ist wichtig zu beachten, dass das Öffnen von Ports im Firewall die Sicherheit Ihres Systems beeinträchtigen kann, daher sollten Sie nur die Ports freigeben, die für den reibungslosen Betrieb Ihres VPN-Dienstes erforderlich sind. Durch das sorgfältige Freigeben von Ports im UFW können Sie eine sichere Verbindung zu Ihrem VPN herstellen und die Vorteile einer verschlüsselten Internetverbindung genießen.
Anleitung VPN-Port UFW öffnen
Eine VPN-Verbindung bietet Sicherheit und Anonymität beim Surfen im Internet. Um eine reibungslose Nutzung von VPN-Diensten zu gewährleisten, müssen manchmal bestimmte Ports in der Firewall geöffnet werden. In diesem Artikel geht es darum, wie man den VPN-Port in der Uncomplicated Firewall (UFW) öffnet.
Zunächst müssen Sie sicherstellen, dass die UFW auf Ihrem System installiert ist. Wenn nicht, können Sie sie einfach über die Befehlszeile installieren. Sobald die UFW eingerichtet ist, können Sie den benötigten Port für Ihren VPN-Dienst öffnen. Dies geschieht durch Eingabe eines einfachen Befehls in der Konsole.
Öffnen Sie dazu die Terminalanwendung und geben Sie den Befehl "sudo ufw allow /tcp" ein, wobei durch die Portnummer Ihres VPN-Dienstes ersetzt werden muss. Zum Beispiel, wenn Ihr VPN-Port 1194 ist, lautet der Befehl "sudo ufw allow 1194/tcp". Nachdem Sie den Befehl eingegeben haben, bestätigen Sie die Aktion und starten Sie die UFW neu, damit die Änderungen wirksam werden.
Es ist wichtig zu beachten, dass das Öffnen von Ports in der Firewall potenzielle Sicherheitsrisiken birgt. Stellen Sie sicher, dass Sie nur die Ports öffnen, die für Ihren VPN-Dienst erforderlich sind, und überprüfen Sie regelmäßig Ihre Firewall-Einstellungen.
Mit diesen einfachen Schritten können Sie den VPN-Port in der UFW öffnen und eine sichere Verbindung zu Ihrem VPN-Dienst herstellen. So können Sie das Internet anonym und geschützt nutzen.
VPN-Verbindung über UFW herstellen
Eine VPN-Verbindung über UFW (Uncomplicated Firewall) herzustellen, ist eine effektive Möglichkeit, Ihre Online-Privatsphäre und -Sicherheit zu gewährleisten. UFW ist ein einfach zu verwendendes Front-End für die iptables-Firewall, das eine sichere Kommunikation zwischen Ihrem Gerät und dem VPN-Server ermöglicht.
Um eine VPN-Verbindung über UFW herzustellen, müssen Sie zunächst sicherstellen, dass UFW auf Ihrem System installiert und konfiguriert ist. Öffnen Sie dazu das Terminal und geben Sie die Befehle ein, um UFW zu installieren und zu aktivieren. Stellen Sie sicher, dass die erforderlichen VPN-Ports (z. B. UDP 1194 für OpenVPN) in der UFW-Konfiguration geöffnet sind.
Sobald UFW konfiguriert ist, können Sie die VPN-Verbindung einrichten, indem Sie die erforderlichen VPN-Einstellungen in Ihrem Netzwerkmanager oder in der VPN-Client-Software eingeben. Stellen Sie sicher, dass Sie die richtigen Anmeldedaten und Serverinformationen bereit haben.
Sobald die VPN-Verbindung eingerichtet ist, können Sie überprüfen, ob die Verbindung ordnungsgemäß funktioniert, indem Sie Ihre IP-Adresse überprüfen oder eine DNS-Leak-Test durchführen. Dadurch können Sie sicherstellen, dass Ihr gesamter Internetverkehr über die VPN-Verbindung geschützt ist und nicht durchsickert.
Insgesamt bietet die Einrichtung einer VPN-Verbindung über UFW eine einfache und effektive Möglichkeit, Ihre Online-Aktivitäten zu schützen und Ihre Privatsphäre zu wahren. Es ist wichtig, sich bewusst zu sein, wie man solche Verbindungen sicher konfiguriert, um maximale Sicherheit und Anonymität zu gewährleisten.
Konfiguration VPN-Port und UFW
Eine wichtige Maßnahme zur Sicherung von Daten und Netzwerken ist die Konfiguration eines VPN-Ports in Verbindung mit einer Firewall wie der Uncomplicated Firewall (UFW). Ein Virtual Private Network (VPN) ermöglicht es, eine verschlüsselte Verbindung zwischen zwei Geräten oder Netzwerken herzustellen, was besonders wichtig ist, wenn sensible Daten über öffentliche Netzwerke übertragen werden.
Die Konfiguration eines VPN-Ports beinhaltet in der Regel die Auswahl eines Protokolls wie OpenVPN oder IPsec, die Festlegung von Zugriffsrechten und die Konfiguration von Verschlüsselungseinstellungen. Durch die Einrichtung eines VPN-Ports können Unternehmen ihren Mitarbeitern sicheren Remote-Zugriff auf das Unternehmensnetzwerk ermöglichen und gleichzeitig die Datenübertragung vor externen Bedrohungen schützen.
Die UFW ist eine Firewall-Software für Linux-Systeme, die es ermöglicht, eingehenden und ausgehenden Netzwerkverkehr zu steuern und zu überwachen. Durch die Konfiguration der UFW können Administratoren den Datenverkehr anhand von Regeln filtern und unerwünschte Verbindungen blockieren. Die Kombination eines VPN-Ports mit der UFW bietet eine umfassende Sicherheitslösung für Unternehmen, die ihre Netzwerke vor Cyberangriffen schützen möchten.
Insgesamt ist die Konfiguration eines VPN-Ports in Verbindung mit der UFW eine effektive Maßnahme, um die Sicherheit von Daten und Netzwerken zu gewährleisten. Durch die Implementierung dieser Sicherheitsmaßnahmen können Unternehmen vertrauliche Informationen schützen und die Integrität ihres Netzwerks sicherstellen.
0 notes
Text
Building a self-functioning Wi-Fi network requires both hardware and software components. The software part includes a script that configures the network settings (such as the SSID, security protocols, IP allocation, etc.) and raw code that manages the functioning of the network. Here’s a basic outline for a self-functioning Wi-Fi network setup using a Raspberry Pi, Linux server, or similar device.
Key Components:
• Router: Acts as the hardware for the network.
• Access Point (AP): Software component that makes a device act as a wireless access point.
• DHCP Server: Automatically assigns IP addresses to devices on the network.
• Firewall and Security: Ensure that only authorized users can connect.
Scripting a Wi-Fi Access Point
1. Set Up the Host Access Point (hostapd):
• hostapd turns a Linux device into a wireless AP.
2. Install Necessary Packages:
sudo apt-get update
sudo apt-get install hostapd dnsmasq
sudo systemctl stop hostapd
sudo systemctl stop dnsmasq
3. Configure the DHCP server (dnsmasq):
• Create a backup of the original configuration file and configure your own.
sudo mv /etc/dnsmasq.conf /etc/dnsmasq.conf.orig
sudo nano /etc/dnsmasq.conf
Add the following configuration:
interface=wlan0 # Use the wireless interface
dhcp-range=192.168.4.2,192.168.4.20,255.255.255.0,24h
This tells the server to use the wlan0 interface and provide IP addresses from 192.168.4.2 to 192.168.4.20.
4. Configure the Wi-Fi Access Point (hostapd):
Create a new configuration file for hostapd.
sudo nano /etc/hostapd/hostapd.conf
Add the following:
interface=wlan0
driver=nl80211
ssid=YourNetworkName
hw_mode=g
channel=7
wmm_enabled=0
macaddr_acl=0
auth_algs=1
ignore_broadcast_ssid=0
wpa=2
wpa_passphrase=YourSecurePassphrase
wpa_key_mgmt=WPA-PSK
wpa_pairwise=TKIP
rsn_pairwise=CCMP
Set up hostapd to use this configuration file:
sudo nano /etc/default/hostapd
Add:
DAEMON_CONF="/etc/hostapd/hostapd.conf"
5. Enable IP Forwarding:
Edit sysctl.conf to enable packet forwarding so traffic can flow between your devices:
sudo nano /etc/sysctl.conf
Uncomment the following line:
net.ipv4.ip_forward=1
6. Configure NAT (Network Address Translation):
sudo iptables -t nat -A POSTROUTING -o eth0 -j MASQUERADE
sudo sh -c "iptables-save > /etc/iptables.ipv4.nat"
Edit /etc/rc.local to restore the NAT rule on reboot:
sudo nano /etc/rc.local
Add the following before the exit 0 line:
iptables-restore < /etc/iptables.ipv4.nat
7. Start the Services:
sudo systemctl start hostapd
sudo systemctl start dnsmasq
8. Auto-Start on Boot:
Enable the services to start on boot:
sudo systemctl enable hostapd
sudo systemctl enable dnsmasq
Raw Code for Wi-Fi Network Management
You may want a custom script to manage the network, auto-configure settings, or monitor status.
Here’s a basic Python script that can be used to start/stop the network, check connected clients, and monitor activity.
import subprocess
def start_network():
"""Start the hostapd and dnsmasq services."""
subprocess.run(['sudo', 'systemctl', 'start', 'hostapd'])
subprocess.run(['sudo', 'systemctl', 'start', 'dnsmasq'])
print("Wi-Fi network started.")
def stop_network():
"""Stop the hostapd and dnsmasq services."""
subprocess.run(['sudo', 'systemctl', 'stop', 'hostapd'])
subprocess.run(['sudo', 'systemctl', 'stop', 'dnsmasq'])
print("Wi-Fi network stopped.")
def check_clients():
"""Check the connected clients using arp-scan."""
clients = subprocess.run(['sudo', 'arp-scan', '-l'], capture_output=True, text=True)
print("Connected Clients:\n", clients.stdout)
def restart_network():
"""Restart the network services."""
stop_network()
start_network()
if __name__ == "__main__":
while True:
print("1. Start Wi-Fi")
print("2. Stop Wi-Fi")
print("3. Check Clients")
print("4. Restart Network")
print("5. Exit")
choice = input("Enter your choice: ")
if choice == '1':
start_network()
elif choice == '2':
stop_network()
elif choice == '3':
check_clients()
elif choice == '4':
restart_network()
elif choice == '5':
break
else:
print("Invalid choice. Try again.")
Optional Security Features
To add further security features like firewalls, you could set up UFW (Uncomplicated Firewall) or use iptables rules to block/allow specific ports and traffic types.
sudo ufw allow 22/tcp # Allow SSH
sudo ufw allow 80/tcp # Allow HTTP
sudo ufw allow 443/tcp # Allow HTTPS
sudo ufw enable # Enable the firewall
Final Notes:
This setup is intended for a small, controlled environment. In a production setup, you’d want to configure more robust security measures, load balancing, and possibly use a more sophisticated router OS like OpenWRT or DD-WRT.
Would you like to explore the hardware setup too?
1 note
·
View note
Text
ORACLE APEX LINUX
Oracle APEX on Linux: A Powerful Combination for Rapid Web Development
Oracle Application Express (APEX) is a low-code development framework that delivers a fast and efficient way to build scalable, secure, and visually appealing web applications. Its seamless integration with Linux operating systems makes it a fantastic choice for developers who favor the stability, flexibility, and cost-effectiveness of Linux as a deployment platform.
Critical Advantages of Running Oracle APEX on Linux
Open-Source Affinity: APEX and Linux have a natural synergy within the open-source ecosystem. There are typically no licensing costs involved, making it a budget-friendly solution.
Robustness: Linux distributions are renowned for their reliability, making them the bedrock of many mission-critical systems. APEX inherits this stability.
Security: Linux employs rigorous security mechanisms and frequent updates – hardening your APEX installation against potential threats.
Customization and Scalability: Linux gives you unparalleled control for tailoring the system to your project’s exact needs. APEX applications can fluidly scale alongside your Linux environment.
Essential Considerations
Firewall: Properly configure your Linux firewall (e.g., iptables or firewalls) to permit traffic to your APEX applications.
Web Server: While APEX has a built-in web listener, production environments often use a robust web server like Apache or Nginx for added performance and security.
Backup Strategy: Implement a meticulous backup plan for your Oracle Database and APEX applications to safeguard your data.
Maximizing Your Oracle APEX on Linux Experience
Embrace the Community: Linux and APEX boast vibrant online communities for troubleshooting and finding innovative solutions.
Consider Performance Tuning: As your applications grow, delve into Linux performance optimization and APEX best practices for a consistently smooth user experience.
Leverage Linux Tools: Linux offers a wealth of command-line utilities and monitoring tools to streamline APEX development and management.
The Power of Choice
Using Oracle APEX on Linux unlocks the power of low-code development on a dependable and customizable platform. Whether deploying internal enterprise applications or building web solutions for clients, the APEX-Linux combination gives you the flexibility and tools you need to deliver outstanding results.
youtube
You can find more information about Oracle Apex in this Oracle Apex Link
Conclusion:
Unogeeks is the No.1 IT Training Institute for Oracle Apex Training. Anyone Disagree? Please drop in a comment
You can check out our other latest blogs on Oracle Apex here – Oarcle Apex Blogs
You can check out our Best In Class Oracle Apex Details here – Oracle Apex Training
Follow & Connect with us:
———————————-
For Training inquiries:
Call/Whatsapp: +91 73960 33555
Mail us at: [email protected]
Our Website ➜ https://unogeeks.com
Follow us:
Instagram: https://www.instagram.com/unogeeks
Facebook: https://www.facebook.com/UnogeeksSoftwareTrainingInstitute
Twitter: https://twitter.com/unogeeks
0 notes
Text
How to Allow IP Addresses through Firewall Linux?
Configuring IP Access with iptables
Verifying iptables Installation
Listing Current Firewall Rules
Allowing Specific IP Addresses
Saving iptables Rules
Creating a Secure IP Whitelist
Defining Your IP Whitelist
Configuring iptables Rules
Testing Connectivity
Streamlining Firewall Management with BeStarHost
Introducing BeStarHost
Using BeStarHost for IP Whitelisting
Best Practices for Linux Firewall Management
Regularly Review and Update Whitelist
Implement Fail2Ban for Additional Security
Conclusion:
Effectively managing your Linux firewall is an integral part of maintaining a secure server environment. By mastering iptables and understanding how to allow specific IP addresses, you fortify your defenses against potential threats. Whether you opt for manual configuration or utilize tools like BeStarHost, the key is to stay vigilant, update your rules regularly, and adapt your security measures to evolving threats. Implementing these practices will empower you to keep your Linux server secure and resilient.
To Learn More, Click this Link:
#Linux Firewall Configuration#IP Address Whitelisting#Linux Firewall Rules#Network Security on Linux#Firewall Tutorial
0 notes
Text
Linux Network Services and Configuration
Linux Learning, Linux network services and configuration are essential aspects of managing a Linux system, whether it's a server or a desktop. Here's an overview of key concepts and tasks:
Network Configuration:
Configure network interfaces, typically found in files like /etc/network/interfaces (Debian-based) or /etc/sysconfig/network-scripts/ifcfg-ethX (Red Hat-based).
Use tools like ifconfig and ip to view and manage network interfaces.
IP Addressing:
Assign static IP addresses or configure DHCP to obtain dynamic ones.
Manage IP routes using route or ip route.
DNS Configuration:
Set up DNS servers in /etc/resolv.conf.
Configure custom DNS resolutions in /etc/hosts.
Firewall Configuration:
Use firewall management tools like iptables (legacy) or firewalld (modern) to control incoming and outgoing network traffic.
Define rules for allowing or denying specific ports and services.
SSH Configuration:
Securely access remote Linux systems via SSH (Secure Shell).
Configure SSH server settings in /etc/ssh/sshd_config.
Network Time Protocol (NTP):
Synchronize system time with NTP servers using the ntpdate or chronyd service.
Network File Sharing:
Set up file sharing using protocols like NFS (Network File System) or Samba for Windows file sharing.
Control access to shared resources through permissions and user authentication.
Network Services:
Install and configure network services like DNS (BIND), web servers (Apache or Nginx), email servers (Postfix or Exim), and more.
Manage these services using tools like systemctl or service-specific configuration files.
Proxy Servers and VPNs:
Configure proxy servers (e.g., Squid) and VPNs (e.g., OpenVPN) to control internet access and establish secure connections.
Monitoring and Troubleshooting:
Monitor network activity with tools like netstat, iftop, and network analyzers like Wireshark.
Troubleshoot connectivity issues using ping, traceroute, and examining system logs in /var/log.
Security Considerations:
Implement security best practices, such as disabling unnecessary services, using strong authentication, and regularly updating the system.
A solid understanding of Linux network services and configuration is crucial for maintaining reliable and secure network connectivity, whether it's for personal use, corporate IT, or hosting web services.
0 notes
Text
Linux Course in Chandigarh: A Comprehensive Guide for Aspiring IT Professionals
In today’s digital world, Linux has become an essential component of IT infrastructure, powering everything from web servers and cloud systems to embedded devices. As demand for Linux professionals continues to rise, Chandigarh has emerged as a growing hub for quality IT training. This article delves into the importance of Linux, the structure of a typical Linux course in Chandigarh, and how such training can open up a world of opportunities for IT aspirants.
Why Learn Linux?
Linux is a Unix-like operating system that is free, open-source, and widely used in servers, data centers, and cloud computing environments. Here's why learning Linux is a smart career move:
Widely Used: Over 90% of cloud infrastructure and web servers run on Linux.
Career Demand: System administrators, DevOps engineers, cloud professionals, and cybersecurity experts require strong Linux skills.
Open Source Flexibility: Linux allows customization and is at the core of many enterprise technologies including Red Hat, Ubuntu, and CentOS.
Industry Certifications: Linux knowledge is a stepping stone to certifications like RHCSA, RHCE, CompTIA Linux+, and LFCS.
Chandigarh: A Growing IT Education Hub
Chandigarh, with its growing IT infrastructure and educational institutions, is an ideal place for pursuing technical training. The city offers:
Reputed Training Institutes: Several well-established institutes offer Linux and other IT courses.
Affordable Living: Compared to metro cities, Chandigarh is cost-effective for students.
Safe and Student-Friendly: The city has a strong student population and a clean, organized environment.
Who Should Take a Linux Course?
A Linux course is suitable for:
Students pursuing Computer Science or IT.
Working professionals looking to enhance their system administration or DevOps skills.
Fresher graduates who want to enter the IT industry with a specialized skill.
Network administrators aiming to deepen their understanding of Linux-based servers.
Structure of a Linux Course in Chandigarh
Most Linux training programs in Chandigarh are structured to suit both beginners and intermediate learners. Here's what a standard curriculum includes:
1. Introduction to Linux
History and philosophy of Linux
Types of Linux distributions
Installation and configuration
2. Linux Command Line
Basic shell commands
Directory and file manipulation
Text editing using vi or nano
3. File System Management
File types and permissions
Disk partitions and mounting
Logical Volume Manager (LVM)
4. User and Group Management
Creating and managing users/groups
Setting permissions and access control
Understanding /etc/passwd and /etc/shadow
5. Package Management
Using RPM, YUM, DNF (Red Hat-based)
Using APT (Debian/Ubuntu-based)
Installing, updating, and removing software
6. System Services and Daemons
Managing services with systemctl
Configuring startup processes
Working with cron jobs and background tasks
7. Networking Basics
IP addressing and configuration
Configuring DNS, DHCP, SSH
Troubleshooting tools: ping, netstat, traceroute
8. Shell Scripting
Writing and executing bash scripts
Variables, loops, and conditionals
Automating repetitive tasks
9. Security and Firewall Configuration
Setting up a firewall with iptables or firewalld
User authentication and sudo privileges
Securing services and open ports
10. Advanced Topics
Kernel management
Virtualization basics using KVM
Introduction to containers (Docker)
Modes of Training
Chandigarh-based institutes offer various formats of training to suit different needs:
Classroom Training: Instructor-led sessions with hands-on practice in a lab environment.
Online Classes: Live interactive classes or self-paced video modules.
Weekend Batches: Ideal for working professionals.
Fast-Track Courses: Intensive programs for those on a tight schedule.
Certification Support
Many Linux courses in Chandigarh also prepare students for globally recognized certifications, such as:
RHCSA (Red Hat Certified System Administrator)
RHCE (Red Hat Certified Engineer)
LFCS (Linux Foundation Certified System Administrator)
CompTIA Linux+
Certification-oriented training includes mock tests, practical labs, and real-time scenario-based exercises.
Top Institutes Offering Linux Training in Chandigarh
Several reputed training centers offer Linux courses in Chandigarh. While names may vary over time, some consistent features to look for include:
Experienced Trainers with industry background.
Updated Curriculum aligned with Red Hat and Linux Foundation.
Lab Infrastructure to practice real-world scenarios.
Placement Support with interview preparation and resume building.
Some institutes also have partnerships with Red Hat or offer authorized training, giving students access to official courseware and exams.
Career Opportunities After a Linux Course
After completing a Linux course, students can pursue various career roles depending on their skill level and interest:
1. Linux System Administrator
Responsible for installation, configuration, and maintenance of Linux servers.
2. DevOps Engineer
Combines Linux skills with tools like Docker, Jenkins, and Kubernetes to streamline software delivery.
3. Cloud Engineer
Works with cloud platforms like AWS, Azure, and Google Cloud, where Linux is often the default OS.
4. Network Administrator
Manages routers, firewalls, and switches with Linux as the control system.
5. Cybersecurity Analyst
Uses Linux tools for penetration testing, log analysis, and threat detection.
Salary Expectations
Starting salaries for Linux professionals in India typically range from ₹3–6 LPA for freshers, and go up to ₹12–20 LPA for experienced professionals in DevOps or cloud roles.
Benefits of Taking a Linux Course in Chandigarh
Affordable Quality Training: Courses in Chandigarh offer a good balance of cost and content.
Industry-Relevant Curriculum: Most institutes design their syllabus with inputs from industry professionals.
Community and Networking: Access to tech meetups, local developer groups, and hackathons.
Strong Placement Records: Many institutes have tie-ups with IT companies and conduct placement drives.
Tips for Choosing the Right Institute
When selecting a Linux course in Chandigarh, consider the following:
Check the trainer’s credentials and experience.
Ask for a demo class to evaluate teaching style.
Look for hands-on labs and real-world projects.
Ensure they offer certification guidance.
Verify placement support and success stories.
Final Thoughts
With its increasing demand in the job market and wide-ranging applications, Linux has become an indispensable skill for IT professionals. Chandigarh, with its vibrant tech training ecosystem, offers an excellent environment to learn Linux from the ground up.
Whether you are a student planning your career path, a professional looking to upgrade your skills, or an entrepreneur wanting to manage your servers more efficiently, a Linux course in Chandigarh can be your gateway to a successful IT journey.
0 notes
Text
In this article, you learned how to install IPtables on Debian
0 notes