#Extracting Numbers From a String in javascript
Explore tagged Tumblr posts
Text
I don’t even use it to write JavaScript.
I may be one of like five people in this 70-person department who can actually write simple code.
Like, I’m not saying I’m necessarily good at it; but at least I can google “JavaScript substring” and learn to write the one line of code required to extract the first three characters of a string, rather than relying on some sort of fucking circuitous nonsense from chatGPT that ultimately doesn’t actually work.
It’s variablename.substring(0,3) btw.
var variablename = “fuck chatGPT”
var first3characters = variablename.substring(0,3)
…will leave you with the value fuc in the variable first3characters. 🌈✨
…or, if you’re getting e164-format phone numbers (+18677371111) and you need the 3rd to 5th characters, to isolate the area code (867), that would be variablename.substring(2,5).
(This is the part of my job I actually like. )
a writing competition i was going to participate in again this year has announced that they now allow AI generated content to be submitted
their reasoning being that "we couldn't ban it even if we wanted to, every writer already uses it anyway"
"Every writer"?
come on
64K notes
·
View notes
Text
7 Essential JavaScript Features Every Developer Should Know Early.
JavaScript is the backbone of modern web development. Whether you're just starting out or already have some coding experience, mastering the core features of JavaScript early on can make a big difference in your growth as a developer. These essential features form the building blocks for writing cleaner, faster, and more efficient code.

Here are 7 JavaScript features every developer should get familiar with early in their journey:
Let & Const Before ES6, var was the only way to declare variables. Now, let and const offer better ways to manage variable scope and immutability.
let allows you to declare block-scoped variables.
const is for variables that should not be reassigned.
javascript Copy Edit let count = 10; const name = "JavaScript"; // name = "Python"; // This will throw an error Knowing when to use let vs. const helps prevent bugs and makes code easier to understand.
Arrow Functions Arrow functions offer a concise syntax and automatically bind this, which is useful in callbacks and object methods.
javascript Copy Edit // Traditional function function add(a, b) { return a + b; }
// Arrow function const add = (a, b) => a + b; They’re not just syntactic sugar—they simplify your code and avoid common scope issues.
Template Literals Template literals (${}) make string interpolation more readable and powerful, especially when dealing with dynamic content.
javascript Copy Edit const user = "Alex"; console.log(Hello, ${user}! Welcome back.); No more awkward string concatenation—just cleaner, more intuitive strings.
Destructuring Assignment Destructuring allows you to extract values from objects or arrays and assign them to variables in a single line.
javascript Copy Edit const user = { name: "Sara", age: 25 }; const { name, age } = user; console.log(name); // "Sara" This feature reduces boilerplate and improves clarity when accessing object properties.
Spread and Rest Operators The spread (…) and rest (…) operators may look the same, but they serve different purposes:
Spread: Expands an array or object.
Rest: Collects arguments into an array.
javascript Copy Edit // Spread const arr1 = [1, 2]; const arr2 = […arr1, 3, 4];
// Rest function sum(…numbers) { return numbers.reduce((a, b) => a + b); } Understanding these makes working with arrays and objects more flexible and expressive.
Promises & Async/Await JavaScript is asynchronous by nature. Promises and async/await are the key to writing asynchronous code that reads like synchronous code.
javascript Copy Edit // Promise fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data));
// Async/Await async function getData() { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); } Mastering these will help you handle APIs, databases, and other async operations smoothly.
Array Methods (map, filter, reduce) High-order array methods are essential for transforming and managing data.
javascript Copy Edit const numbers = [1, 2, 3, 4, 5];
// map const doubled = numbers.map(n => n * 2);
// filter const even = numbers.filter(n => n % 2 === 0);
// reduce const sum = numbers.reduce((total, n) => total + n, 0); These methods are clean, efficient, and favored in modern JavaScript codebases.
Final Thoughts Learning these JavaScript features early gives you a solid foundation to write better, more modern code. They’re widely used in frameworks like React, Vue, and Node.js, and understanding them will help you grow faster as a developer.
Start with these, build projects to apply them, and your JavaScript skills will take off.
1 note
·
View note
Text
Phone Number Library: The Backbone of Modern Telecommunication Software
Introduction
In today’s digitally driven world, phone numbers serve as critical identifiers for individuals and businesses alike. Whether it’s authenticating a user, sending a message, or dialing a call, the phone number is central to modern communication systems. However, handling phone numbers across different countries, formats, and use cases is no trivial task. This is where a Phone Number Library becomes invaluable.
A phone number library is a software component that enables developers to validate, parse, format, and manage phone numbers across global telecommunications standards. These libraries are crucial in mobile apps, CRM systems, phone number library messaging services, and any software that interacts with phone numbers. This article explores the concept of a phone number library, its components, popular implementations, challenges, privacy concerns, and future relevance.
What is a Phone Number Library?
A Phone Number Library is a collection of tools, functions, or APIs that provide developers with robust capabilities to handle phone numbers programmatically. Its key responsibilities typically include:
Parsing: Extracting meaningful data from a raw phone number string
Validation: Checking whether a phone number is valid for a given region or format
Formatting: Converting numbers into local, international, or E.164 formats
Carrier Lookup: Identifying the telecom provider associated with a number
Region Detection: Identifying the country or location based on number prefix
Normalization: Cleaning up and unifying numbers for consistent processing
By using a phone number library, developers can avoid pitfalls associated with regional formatting, country codes, and inconsistent user inputs.
Why Do We Need Phone Number Libraries?
Phone numbers are deceptively complex. Consider this:
Different countries have varying lengths for numbers (e.g., 10 digits in the US, up to 15 in others).
Local formats vary: a UK number might be +44 20 7946 0958, while a US number is +1 (202) 555-0190.
Some numbers are mobile, others landlines, toll-free, or VoIP.
Inputs from users can be inconsistent: 001-202-555-0190, 202.555.0190, (202)5550190.
A robust phone number library abstracts these challenges and ensures accurate handling across global systems, enabling:
Internationalization
Reliable communication
Accurate billing
Fraud prevention
Enhanced user experience
Core Features of a Phone Number Library
Parsing and Formatting Parses unstructured input and converts it to a standardized form:
Local Format: (415) 555-2671
International Format: +1 415-555-2671
E.164 Format (standardized): +14155552671
Validation Verifies if a number is:
The correct length for the region
Using a valid country or area code
Associated with a mobile, landline, or VoIP line
Carrier and Region Identification Determines:
Mobile carrier (e.g., Verizon, Vodafone)
Country and locality
Line type (mobile, landline, toll-free)
Number Formatting for Display or Storage Adjusts the number based on:
Country-specific formatting rules
User locale or UI requirements
Storage standards like E.164
Localization Support Allows developers to handle phone numbers according to cultural and national standards for input, display, and processing.
Popular Phone Number Libraries
1. Libphone number by Google
The most widely used phone number library, originally created for Android. It supports:
Parsing, validation, formatting, and geocoding
Over 200 countries and territories
Carrier lookup and timezone info
Multiple language support
Languages Available:
Java (original)
JavaScript, Python, C#, Go (ports)
2. PhoneNumberKit (Swift)
An iOS-compatible version inspired by libphone number:
Easy to use in iOS applications
Fully localized and supports real-time formatting
Lightweight and well-maintained
3. Phony (Ruby)
Popular in the Ruby ecosystem for working with international phone numbers:
E.164 formatting
National and international formats
Country-specific rules
4. libphonenumber-js (JavaScript)
A lightweight version of libphone number for web applications:
Smaller bundle size
Useful for form validations
Ideal for front-end validation on browsers
How Phone Number Libraries are Used in Applications
1. User Registration and Authentication
Apps often use phone numbers for sign-up and verification via OTP. A phone number library ensures:
Correct formatting
No duplication (e.g., +1 202-555-0190 = 0012025550190)
Region-based validation
2. CRM and Contact Management
In enterprise software:
Ensures uniformity in contact databases
Supports global customer outreach
Enables filtering and reporting based on country/region
3. Messaging and Calling Apps
Used to:
Format numbers before dialing or sending a message
Detect line type (mobile vs landline)
Prevent spam or invalid call attempts
4. E-commerce and Delivery Apps
Valid phone numbers are critical for order confirmations, delivery updates, and support communication.
5. Fraud Prevention
Detects anomalies such as:
Invalid or spoofed numbers
SIM farms or mass registration attempts
Geolocation mismatches
Implementing a Phone Number Library: Code Example
Here’s a quick example using libphonenumber-js in JavaScript:
import { parsePhoneNumberFromString } from 'libphonenumber-js'
const phone = parsePhoneNumberFromString('+14155552671')
if (phone.isValid()) {
console.log('Region:', phone.country)
console.log('E.164 Format:', phone.format('E.164'))
console.log('National Format:', phone.formatNational())
}
This simple code:
Validates the number
Extracts country
Outputs the number in multiple formats
Challenges in Using Phone Number Libraries
Despite their utility, developers face certain challenges:
1. Constant Updates
Numbering plans change frequently. Libraries need to update regularly to stay current with telecom regulations.
2. Data Size
Some versions of libphone number are large, making them unsuitable for client-side apps without tree-shaking or slimming.
3. Privacy Implications
Using carrier lookup and region detection can raise privacy issues, especially in regulated markets.
4. False Positives
A number may be valid in structure but still be unreachable or dormant.
Privacy and Compliance Considerations
Handling phone number data must comply with data protection regulations like:
GDPR (EU)
Phone numbers are considered personal data.
Must obtain consent for processing.
Users have the right to request deletion and access to their data.
CCPA (California)
Requires transparency in phone data collection.
Users must be able to opt out of data selling or sharing.
Industry Best Practices
Never store raw user inputs without normalization.
Encrypt stored numbers, especially if combined with other identifiers.
Provide clear terms about how numbers are used.
Future of Phone Number Libraries
As global connectivity evolves, phone number libraries are adapting to meet new demands:
1. Support for eSIM and Virtual Numbers
With the rise of digital SIMs and temporary phone numbers, libraries are being updated to recognize and manage these new identifiers.
2. AI Integration
Phone data is being used to feed machine learning models that detect fraud, personalize communication, or predict churn.
3. Real-time Validation APIs
Developers now have access to API-based services that not only validate format but also check if the number is active or reachable in real time.
4. Voice Assistant and IoT Expansion
As devices like smart speakers and IoT gadgets adopt voice calling, phone libraries will need to be embedded in non-traditional environments.
Thanks for reading..
SEO Expate Bangladesh Ltd.
0 notes
Video
youtube
Tech talk with Arcoiris Logics #javascript #mobileappdevelopment #codin... Title: Unlocking JavaScript Secrets: Hidden Tech Tips Every Developer Should KnowJavaScript continues to reign as one of the most powerful and widely used programming languages. From creating interactive websites to building complex applications, JavaScript powers the web in ways many developers are yet to fully understand. In this post, we’re diving into some hidden tech tips that can help you improve your JavaScript coding skills and efficiency.Here are some JavaScript tips for developers that will take your development game to the next level:1. Use Destructuring for Cleaner CodeJavaScript’s destructuring assignment allows you to extract values from arrays or objects into variables in a cleaner and more readable way. It’s perfect for dealing with complex data structures and improves the clarity of your code.javascriptCopy codeconst user = { name: "John", age: 30, location: "New York" }; const { name, age } = user; console.log(name, age); // John 30 2. Master Arrow FunctionsArrow functions provide a shorter syntax for writing functions and fix some common issues with the this keyword. They are especially useful in callback functions and higher-order functions.javascriptCopy codeconst greet = (name) => `Hello, ${name}!`; console.log(greet("Alice")); // Hello, Alice! 3. Leverage Default ParametersDefault parameters allow you to set default values for function parameters when no value is passed. This feature can help prevent errors and make your code more reliable.javascriptCopy codefunction greet(name = "Guest") { return `Hello, ${name}!`; } console.log(greet()); // Hello, Guest! 4. Mastering Promises and Async/AwaitPromises and async/await are essential for handling asynchronous operations in JavaScript. While callbacks were once the go-to solution, promises provide a more manageable way to handle complex asynchronous code.javascriptCopy codeconst fetchData = async () => { try { const response = await fetch("https://api.example.com"); const data = await response.json(); console.log(data); } catch (error) { console.error(error); } }; fetchData(); 5. Use Template Literals for Dynamic StringsTemplate literals make string interpolation easy and more readable. They also support multi-line strings and expression evaluation directly within the string.javascriptCopy codeconst user = "Alice"; const message = `Hello, ${user}! Welcome to JavaScript tips.`; console.log(message); 6. Avoid Global Variables with IIFE (Immediately Invoked Function Expressions)Global variables can be a source of bugs, especially in large applications. An IIFE helps by creating a local scope for your variables, preventing them from polluting the global scope.javascriptCopy code(function() { const temp = "I am local!"; console.log(temp); })(); 7. Array Methods You Should KnowJavaScript offers powerful array methods such as map(), filter(), reduce(), and forEach(). These methods allow for more functional programming techniques, making code concise and easier to maintain.javascriptCopy codeconst numbers = [1, 2, 3, 4]; const doubled = numbers.map(num => num * 2); console.log(doubled); // [2, 4, 6, 8] 8. Take Advantage of Spread OperatorThe spread operator (...) can be used to copy elements from arrays or properties from objects. It’s a game changer when it comes to cloning data or merging multiple arrays and objects.javascriptCopy codeconst arr1 = [1, 2, 3]; const arr2 = [...arr1, 4, 5]; console.log(arr2); // [1, 2, 3, 4, 5] 9. Debugging with console.table()When working with complex data structures, console.table() allows you to output an object or array in a neat table format, making debugging much easier.javascriptCopy codeconst users = [{ name: "John", age: 25 }, { name: "Alice", age: 30 }]; console.table(users); 10. Use Strict Mode for Cleaner CodeActivating strict mode in JavaScript helps eliminate some silent errors by making them throw exceptions. It’s especially useful when debugging code or working in larger teams.javascriptCopy code"use strict"; let x = 3.14; ConclusionJavaScript offers a wealth of features and hidden gems that, when mastered, can drastically improve your coding skills. By incorporating these tips into your everyday coding practices, you'll write cleaner, more efficient code and stay ahead of the curve in JavaScript development.As technology continues to evolve, staying updated with JavaScript tips and best practices will ensure you're always on top of your development game. Happy coding!#JavaScript #WebDevelopment #TechTips #Coding #DeveloperLife #WebDevTips #JSDevelopment #JavaScriptTips #FrontendDevelopment #ProgrammerLife #JavaScriptForDevelopers #TechCommunity #WebDev
0 notes
Text
10 JavaScript Tricks You Didn't Know: Unleash Your Inner JavaScript Ninja

JavaScript is a powerful and versatile language, but even seasoned developers can discover hidden gems lurking beneath the surface. Brush up your skills and impress your colleagues with these 10 JavaScript tricks you might not have known existed:
Destructuring with Aliases: Unpack objects and arrays in a single step while assigning cleaner variable names.
JavaScript
const person = { firstName: 'John', lastName: 'Doe' };
const { firstName: fName, lastName: lName } = person;
console.log(fName); // Output: John
console.log(lName); // Output: Doe
Optional Chaining: Safely access nested object properties without worrying about errors if intermediate objects are null or undefined.
JavaScript
const user = { info: { address: { street: '123 Main St' } } };
const street = user?.info?.address?.street; // Outputs: '123 Main St' (or undefined if 'address' is missing)
Nullish Coalescing Operator (??): Provide a default value when dealing with null or undefined values, ignoring falsy values (0, "", false).
JavaScript
const defaultValue = 'Default';
const name = user?.name ?? defaultValue; // Provides default value if user.name is null or undefined
Spread Operator (...): Unpack the contents of an array or object into individual elements or properties.
JavaScript
const numbers = [1, 2, 3];
const newArray = [...numbers, 4, 5]; // Creates a new array with numbers and 4, 5
Template Literals (Backticks): Create dynamic strings with embedded expressions and multi-line capabilities.
JavaScript
const name = 'John';
const greeting = `Hello, ${name}! How are you today?`;
console.log(greeting); // Outputs: Hello, John! How are you today?
Default Function Parameters: Set default values for function parameters if no arguments are provided.
JavaScript
function greet(name = 'World') {
console.log(`Hello, ${name}!`);
}
greet(); // Outputs: Hello, World!
greet('Alice'); // Outputs: Hello, Alice!
Arrow Functions: Concise function syntax for cleaner and more readable code.
JavaScript
const numbers = [1, 2, 3].map(number => number * 2); // Doubles each number in the array
Rest and Spread Operator with Functions: Use ... to handle an indefinite number of arguments or unpack function arguments into an array.
JavaScript
function sum(...numbers) {
let total = 0;
for (const num of numbers) {
total += num;
}
return total;
}
const result = sum(1, 2, 3, 4); // Calculates the sum of all arguments
Symbol Data Type: Create unique and immutable identifiers for properties or objects.
JavaScript
const uniqueSymbol = Symbol('My Unique Symbol');
console.log(typeof uniqueSymbol); // Outputs: symbol
Destructuring Assignment with Arrays: Extract elements from arrays into individual variables.
JavaScript
const numbers = [10, 20, 30];
const [first, second] = numbers;
console.log(first); // Outputs: 10
console.log(second); // Outputs: 20
By incorporating these tricks into your JavaScript code, you can write cleaner, more maintainable, and more impressive applications. Now go forth and code like a ninja!
0 notes
Text
Extracting Numbers From a String in JavaScript
In this article, we are going to see the Extracting Numbers from a String in JavaScript. As we know, extracting a number from the given string will be easier when we apply some methods like parseInt() and parseFloat() methods, right? But it also has some limitation which we are going to see in here, and also we will see some different ways to extract numbers from a string. Extracting Numbers…
View On WordPress
0 notes
Photo
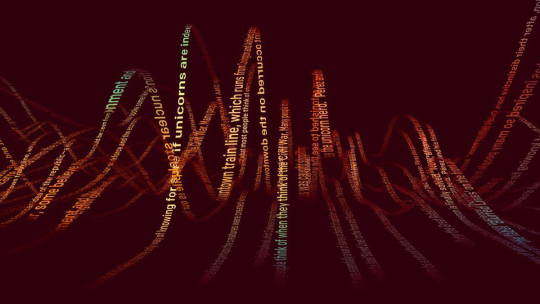
Language supermodel: How GPT-3 is quietly ushering in the A.I. revolution https://ift.tt/3mAgOO1
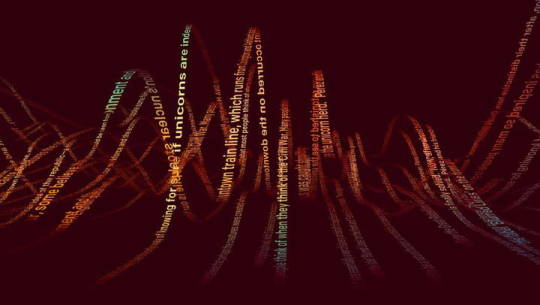
OpenAI
OpenAI’s GPT-2 text-generating algorithm was once considered too dangerous to release. Then it got released — and the world kept on turning.
In retrospect, the comparatively small GPT-2 language model (a puny 1.5 billion parameters) looks paltry next to its sequel, GPT-3, which boasts a massive 175 billion parameters, was trained on 45 TB of text data, and cost a reported $12 million (at least) to build.
“Our perspective, and our take back then, was to have a staged release, which was like, initially, you release the smaller model and you wait and see what happens,” Sandhini Agarwal, an A.I. policy researcher for OpenAI told Digital Trends. “If things look good, then you release the next size of model. The reason we took that approach is because this is, honestly, [not just uncharted waters for us, but it’s also] uncharted waters for the entire world.”
Jump forward to the present day, nine months after GPT-3’s release last summer, and it’s powering upward of 300 applications while generating a massive 4.5 billion words per day. Seeded with only the first few sentences of a document, it’s able to generate seemingly endless more text in the same style — even including fictitious quotes.
Is it going to destroy the world? Based on past history, almost certainly not. But it is making some game-changing applications of A.I. possible, all while posing some very profound questions along the way.
What is it good for? Absolutely everything
Recently, Francis Jervis, the founder of a startup called Augrented, used GPT-3 to help people struggling with their rent to write letters negotiating rent discounts. “I’d describe the use case here as ‘style transfer,'” Jervis told Digital Trends. “[It takes in] bullet points, which don’t even have to be in perfect English, and [outputs] two to three sentences in formal language.”
Powered by this ultra-powerful language model, Jervis’s tool allows renters to describe their situation and the reason they need a discounted settlement. “Just enter a couple of words about why you lost income, and in a few seconds you’ll get a suggested persuasive, formal paragraph to add to your letter,” the company claims.
This is just the tip of the iceberg. When Aditya Joshi, a machine learning scientist and former Amazon Web Services engineer, first came across GPT-3, he was so blown away by what he saw that he set up a website, www.gpt3examples.com, to keep track of the best ones.
“Shortly after OpenAI announced their API, developers started tweeting impressive demos of applications built using GPT-3,” he told Digital Trends. “They were astonishingly good. I built [my website] to make it easy for the community to find these examples and discover creative ways of using GPT-3 to solve problems in their own domain.”
Fully interactive synthetic personas with GPT-3 and https://t.co/ZPdnEqR0Hn ????
They know who they are, where they worked, who their boss is, and so much more. This is not your father's bot… pic.twitter.com/kt4AtgYHZL
— Tyler Lastovich (@tylerlastovich) August 18, 2020
Joshi points to several demos that really made an impact on him. One, a layout generator, renders a functional layout by generating JavaScript code from a simple text description. Want a button that says “subscribe” in the shape of a watermelon? Fancy some banner text with a series of buttons the colors of the rainbow? Just explain them in basic text, and Sharif Shameem’s layout generator will write the code for you. Another, a GPT-3 based search engine created by Paras Chopra, can turn any written query into an answer and a URL link for providing more information. Another, the inverse of Francis Jervis’ by Michael Tefula, translates legal documents into plain English. Yet another, by Raphaël Millière, writes philosophical essays. And one other, by Gwern Branwen, can generate creative fiction.
“I did not expect a single language model to perform so well on such a diverse range of tasks, from language translation and generation to text summarization and entity extraction,” Joshi said. “In one of my own experiments, I used GPT-3 to predict chemical combustion reactions, and it did so surprisingly well.”
More where that came from
The transformative uses of GPT-3 don’t end there, either. Computer scientist Tyler Lastovich has used GPT-3 to create fake people, including backstory, who can then be interacted with via text. Meanwhile, Andrew Mayne has shown that GPT-3 can be used to turn movie titles into emojis. Nick Walton, chief technology officer of Latitude, the studio behind GPT-generated text adventure game AI Dungeon recently did the same to see if it could turn longer strings of text description into emoji. And Copy.ai, a startup that builds copywriting tools with GPT-3, is tapping the model for all it’s worth, with a monthly recurring revenue of $67,000 as of March — and a recent $2.9 million funding round.
“Definitely, there was surprise and a lot of awe in terms of the creativity people have used GPT-3 for,” Sandhini Agarwal, an A.I. policy researcher for OpenAI told Digital Trends. “So many use cases are just so creative, and in domains that even I had not foreseen, it would have much knowledge about. That’s interesting to see. But that being said, GPT-3 — and this whole direction of research that OpenAI pursued — was very much with the hope that this would give us an A.I. model that was more general-purpose. The whole point of a general-purpose A.I. model is [that it would be] one model that could like do all these different A.I. tasks.”
Many of the projects highlight one of the big value-adds of GPT-3: The lack of training it requires. Machine learning has been transformative in all sorts of ways over the past couple of decades. But machine learning requires a large number of training examples to be able to output correct answers. GPT-3, on the other hand, has a “few shot ability” that allows it to be taught to do something with only a small handful of examples.
Plausible bull***t
GPT-3 is highly impressive. But it poses challenges too. Some of these relate to cost: For high-volume services like chatbots, which could benefit from GPT-3’s magic, the tool might be too pricey to use. (A single message could cost 6 cents which, while not exactly bank-breaking, certainly adds up.)
Others relate to its widespread availability, meaning that it’s likely going to be tough to build a startup exclusively around since fierce competition will likely drive down margins.
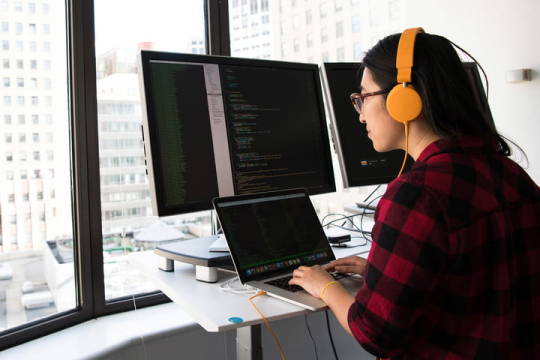
Christina Morillo/Pexels
Another is the lack of memory; its context window runs a little under 2,000 words at a time before, like Guy Pierce’s character in the movie Memento, its memory is reset. “This significantly limits the length of text it can generate, roughly to a short paragraph per request,” Lastovich said. “Practically speaking, this means that it is unable to generate long documents while still remembering what happened at the beginning.”
Perhaps the most notable challenge, however, also relates to its biggest strength: Its confabulation abilities. Confabulation is a term frequently used by doctors to describe the way in which some people with memory issues are able to fabricate information that appears initially convincing, but which doesn’t necessarily stand up to scrutiny upon closer inspection. GPT-3’s ability to confabulate is, depending upon the context, a strength and a weakness. For creative projects, it can be great, allowing it to riff on themes without concern for anything as mundane as truth. For other projects, it can be trickier.
Francis Jervis of Augrented refers to GPT-3’s ability to “generate plausible bullshit.” Nick Walton of AI Dungeon said: “GPT-3 is very good at writing creative text that seems like it could have been written by a human … One of its weaknesses, though, is that it can often write like it’s very confident — even if it has no idea what the answer to a question is.”
Back in the Chinese Room
In this regard, GPT-3 returns us to the familiar ground of John Searle’s Chinese Room. In 1980, Searle, a philosopher, published one of the best-known A.I. thought experiments, focused on the topic of “understanding.” The Chinese Room asks us to imagine a person locked in a room with a mass of writing in a language that they do not understand. All they recognize are abstract symbols. The room also contains a set of rules that show how one set of symbols corresponds with another. Given a series of questions to answer, the room’s occupant must match question symbols with answer symbols. After repeating this task many times, they become adept at performing it — even though they have no clue what either set of symbols means, merely that one corresponds to the other.
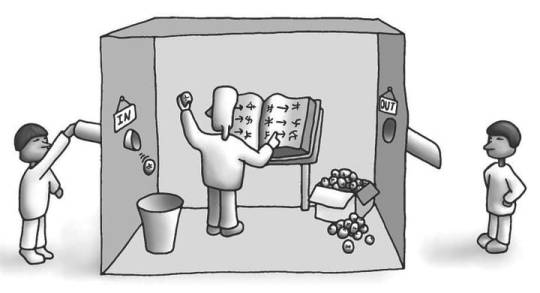
GPT-3 is a world away from the kinds of linguistic A.I. that existed at the time Searle was writing. However, the question of understanding is as thorny as ever.
“This is a very controversial domain of questioning, as I’m sure you’re aware, because there’s so many differing opinions on whether, in general, language models … would ever have [true] understanding,” said OpenAI’s Sandhini Agarwal. “If you ask me about GPT-3 right now, it performs very well sometimes, but not very well at other times. There is this randomness in a way about how meaningful the output might seem to you. Sometimes you might be wowed by the output, and sometimes the output will just be nonsensical. Given that, right now in my opinion … GPT-3 doesn’t appear to have understanding.”
An added twist on the Chinese Room experiment today is that GPT-3 is not programmed at every step by a small team of researchers. It’s a massive model that’s been trained on an enormous dataset consisting of, well, the internet. This means that it can pick up inferences and biases that might be encoded into text found online. You’ve heard the expression that you’re an average of the five people you surround yourself with? Well, GPT-3 was trained on almost unfathomable amounts of text data from multiple sources, including books, Wikipedia, and other articles. From this, it learns to predict the next word in any sequence by scouring its training data to see word combinations used before. This can have unintended consequences.
Feeding the stochastic parrots
This challenge with large language models was first highlighted in a groundbreaking paper on the subject of so-called stochastic parrots. A stochastic parrot — a term coined by the authors, who included among their ranks the former co-lead of Google’s ethical A.I. team, Timnit Gebru — refers to a large language model that “haphazardly [stitches] together sequences of linguistic forms it has observed in its vast training data, according to probabilistic information about how they combine, but without any reference to meaning.”
“Having been trained on a big portion of the internet, it’s important to acknowledge that it will carry some of its biases,” Albert Gozzi, another GPT-3 user, told Digital Trends. “I know the OpenAI team is working hard on mitigating this in a few different ways, but I’d expect this to be an issue for [some] time to come.”
OpenAI’s countermeasures to defend against bias include a toxicity filter, which filters out certain language or topics. OpenAI is also working on ways to integrate human feedback in order to be able to specify which areas not to stray into. In addition, the team controls access to the tool so that certain negative uses of the tool will not be granted access.
“One of the reasons perhaps you haven’t seen like too many of these malicious users is because we do have an intensive review process internally,” Agarwal said. “The way we work is that every time you want to use GPT-3 in a product that would actually be deployed, you have to go through a process where a team — like, a team of humans — actually reviews how you want to use it. … Then, based on making sure that it is not something malicious, you will be granted access.”
Some of this is challenging, however — not least because bias isn’t always a clear-cut case of using certain words. Jervis notes that, at times, his GPT-3 rent messages can “tend towards stereotypical gender [or] class assumptions.” Left unattended, it might assume the subject’s gender identity on a rent letter, based on their family role or job. This may not be the most grievous example of A.I. bias, but it highlights what happens when large amounts of data are ingested and then probabilistically reassembled in a language model.
“Bias and the potential for explicit returns absolutely exist and require effort from developers to avoid,” Tyler Lastovich said. “OpenAI does flag potentially toxic results, but ultimately it does add a liability customers have to think hard about before putting the model into production. A specifically difficult edge case to develop around is the model’s propensity to lie — as it has no concept of true or false information.”
Language models and the future of A.I.
Nine months after its debut, GPT-3 is certainly living up to its billing as a game changer. What once was purely potential has shown itself to be potential realized. The number of intriguing use cases for GPT-3 highlights how a text-generating A.I. is a whole lot more versatile than that description might suggest.
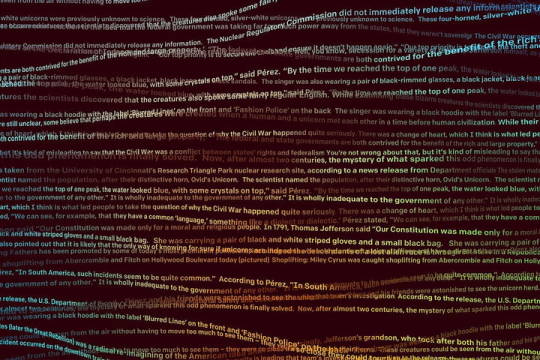
Not that it’s the new kid on the block these days. Earlier this year, GPT-3 was overtaken as the biggest language model. Google Brain debuted a new language model with some 1.6 trillion parameters, making it nine times the size of OpenAI’s offering. Nor is this likely to be the end of the road for language models. These are extremely powerful tools — with the potential to be transformative to society, potentially for better and for worse.
Challenges certainly exist with these technologies, and they’re ones that companies like OpenAI, independent researchers, and others, must continue to address. But taken as a whole, it’s hard to argue that language models are not turning to be one of the most interesting and important frontiers of artificial intelligence research.
Who would’ve thought text generators could be so profoundly important? Welcome to the future of artificial intelligence.
1 note
·
View note
Text
Fandom Userscript Cookbook: Five Projects to Get Your Feet Wet
Target audience: This post is dedicated, with love, to all novice, aspiring, occasional, or thwarted coders in fandom. If you did a code bootcamp once and don’t know where to start applying your new skillz, this is for you. If you're pretty good with HTML and CSS but the W3Schools Javascript tutorials have you feeling out of your depth, this is for you. If you can do neat things in Python but don’t know a good entry point for web programming, this is for you. Seasoned programmers looking for small, fun, low-investment hobby projects with useful end results are also welcome to raid this post for ideas.
You will need:
The Tampermonkey browser extension to run and edit userscripts
A handful of example userscripts from greasyfork.org. Just pick a few that look nifty and install them. AO3 Savior is a solid starting point for fandom tinkering.
Your browser dev tools. Hit F12 or right click > Inspect Element to find the stuff on the page you want to tweak and experiment with it. Move over to the Console tab once you’ve got code to test out and debug.
Javascript references and tutorials. W3Schools has loads of both. Mozilla’s JS documentation is top-notch, and I often just keep their reference lists of built-in String and Array functions open in tabs as I code. StackOverflow is useful for questions, but don’t assume the code snippets you find there are always reliable or copypastable.
That’s it. No development environment. No installing node.js or Ruby or Java or two different versions of Python. No build tools, no dependency management, no fucking Docker containers. No command line, even. Just a browser extension, the browser’s built-in dev tools, and reference material. Let’s go.
You might also want:
jQuery and its documentation. If you’re wrestling with a mess of generic spans and divs and sparse, unhelpful use of classes, jQuery selectors are your best bet for finding the element you want before you snap and go on a murderous rampage. jQuery also happens to be the most ubiquitous JS library out there, the essential Swiss army knife for working with Javascript’s... quirks, so experience with it is useful. It gets a bad rap because trying to build a whole house with a Swiss army knife is a fool’s errand, but it’s excellent for the stuff we're about to do.
Git or other source control, if you’ve already got it set up. By all means share your work on Github. Greasy Fork can publish a userscript from a Github repo. It can also publish a userscript from an uploaded text file or some code you pasted into the upload form, so don’t stress about it if you’re using a more informal process.
A text editor. Yes, seriously, this is optional. It’s a question of whether you’d rather code everything right there in Tampermonkey’s live editor, or keep a separate copy to paste into Tampermonkey’s live editor for testing. Are you feeling lucky, punk?
Project #1: Hack on an existing userscript
Install some nifty-looking scripts for websites you visit regularly. Use them. Ponder small additions that would make them even niftier. Take a look at their code in the Tampermonkey editor. (Dashboard > click on the script name.) Try to figure out what each bit is doing.
Then change something, hit save, and refresh the page.
Break it. Make it select the wrong element on the page to modify. Make it blow up with a huge pile of console errors. Add a console.log("I’m a teapot"); in the middle of a loop so it prints fifty times. Savor your power to make the background wizardry of the internet do incredibly dumb shit.
Then try a small improvement. It will probably break again. That's why you've got the live editor and the console, baby--poke it, prod it, and make it log everything it's doing until you've made it work.
Suggested bells and whistles to make the already-excellent AO3 Savior script even fancier:
Enable wildcards on a field that currently requires an exact match. Surely there’s at least one song lyric or Richard Siken quote you never want to see in any part of a fic title ever again, right?
Add some text to the placeholder message. Give it a pretty background color. Change the amount of space it takes up on the page.
Blacklist any work with more than 10 fandoms listed. Then add a line to the AO3 Savior Config script to make the number customizable.
Add a global blacklist of terms that will get a work hidden no matter what field they're in.
Add a list of blacklisted tag combinations. Like "I'm okay with some coffee shop AUs, but the ones that are also tagged as fluff don't interest me, please hide them." Or "Character A/Character B is cute but I don't want to read PWP about them."
Anything else you think of!
Project #2: Good Artists Borrow, Great Artists Fork (DIY blacklisting)
Looking at existing scripts as a model for the boilerplate you'll need, create a script that runs on a site you use regularly that doesn't already have a blacklisting/filtering feature. If you can't think of one, Dreamwidth comments make a good guinea pig. (There's a blacklist script for them out there, but reinventing wheels for fun is how you learn, right? ...right?) Create a simple blacklisting script of your own for that site.
Start small for the site-specific HTML wrangling. Take an array of blacklisted keywords and log any chunk of post/comment text that contains one of them.
Then try to make the post/comment it belongs to disappear.
Then add a placeholder.
Then get fancy with whitelists and matching metadata like usernames/titles/tags as well.
Crib from existing blacklist scripts like AO3 Savior as shamelessly as you feel the need to. If you publish the resulting userscript for others to install (which you should, if it fills an unmet need!), please comment up any substantial chunks of copypasted or closely-reproduced code with credit/a link to the original. If your script basically is the original with some key changes, like our extra-fancy AO3 Savior above, see if there’s a public Git repo you can fork.
Project #3: Make the dread Tumblr beast do a thing
Create a small script that runs on the Tumblr dashboard. Make it find all the posts on the page and log their IDs. Then log whether they're originals or reblogs. Then add a fancy border to the originals. Then add a different fancy border to your own posts. All of this data should be right there in the post HTML, so no need to derive it by looking for "x reblogged y" or source links or whatever--just make liberal use of Inspect Element and the post's data- attributes.
Extra credit: Explore the wildly variable messes that Tumblr's API spews out, and try to recreate XKit's timestamps feature with jQuery AJAX calls. (Post timestamps are one of the few reliable API data points.) Get a zillion bright ideas about what else you could do with the API data. Go through more actual post data to catalogue all the inconsistencies you’d have to catch. Cry as Tumblr kills the dream you dreamed.
Project #4: Make the dread Tumblr beast FIX a thing
Create a script that runs on individual Tumblr blogs (subdomains of tumblr.com). Browse some blogs with various themes until you've found a post with the upside-down reblog-chain bug and a post with reblogs displaying normally. Note the HTML differences between them. Make the script detect and highlight upside-down stacks of blockquotes. Then see if you can make it extract the blockquotes and reassemble them in the correct order. At this point you may be mobbed by friends and acquaintainces who want a fix for this fucking bug, which you can take as an opportunity to bury any lingering doubts about the usefulness of your scripting adventures.
(Note: Upside-down reblogs are the bug du jour as of September 2019. If you stumble upon this post later, please substitute whatever the latest Tumblr fuckery is that you'd like to fix.)
Project #5: Regular expressions are a hard limit
I mentioned up above that Dreamwidth comments are good guinea pigs for user scripting? You know what that means. Kinkmemes. Anon memes too, but kinkmemes (appropriately enough) offer so many opportunities for coding masochism. So here's a little exercise in sadism on my part, for anyone who wants to have fun (or "fun") with regular expressions:
Write a userscript that highlights all the prompts on any given page of a kinkmeme that have been filled.
Specifically, scan all the comment subject lines on the page for anything that looks like the title of a kinkmeme fill, and if you find one, highlight the prompt at the top of its thread. The nice ones will start with "FILL:" or end with "part 1/?" or "3/3 COMPLETE." The less nice ones will be more like "(former) minifill [37a / 50(?)] still haven't thought of a name for this thing" or "title that's just the subject line of the original prompt, Chapter 3." Your job is to catch as many of the weird ones as you can using regular expressions, while keeping false positives to a minimum.
Test it out on a real live kinkmeme, especially one without strict subject-line-formatting policies. I guarantee you, you will be delighted at some of the arcane shit your script manages to catch. And probably astonished at some of the arcane shit you never thought to look for because who the hell would even format a kinkmeme fill like that? Truly, freeform user input is a wonderful and terrible thing.
If that's not enough masochism for you, you could always try to make the script work on LiveJournal kinkmemes too!
64 notes
·
View notes
Text
Javascript splice array

#Javascript splice array how to
#Javascript splice array code
#Javascript splice array plus
#Javascript splice array free
If deleteCount is omitted or its value is equal to or greater than array.length - start, all elements from the beginning to the end of the array are deleted.Ġ or negative, no elements are deleted. This parameter specifies the number of elements of the array to get rid of from the start. The second parameter is deleteCount, an optional parameter. If negative, start with that many elements at the end of the array. In this case, no elements are removed, but the method behaves like an addition function, adding as many elements as elements are provided. If greater than the length of an array, the start is set to the array’s length. The required parameter specifies an array’s starting position/index to modify the array. The JavaScript splice method takes three input parameters, out of which the first one is start. Splice(start, deleteCount, item1, item2, itemN) This is done by removing, replacing existing items, and adding new items in their place. The splice() method affects or modifies the contents of an array.
#Javascript splice array code
Once you run the above code in any browser, it’ll print something like this.Įxtract the Subset of Array Elements From an Array With splice() in JavaScript Interestingly, the input parameter is considered an offset from the end of the sequence if you specify a negative index. If the starting index is larger than the length of an array, it will appear empty and also, the empty array will be returned as output. When we call slice(0, 1) the element 1 is copied from the initial array, inputArray to outputArray1. const inputArray = Ĭonst outputArray1 = inputArray.slice(0, 1) Ĭonst outputArray2 = inputArray.slice(1, 3) Ĭonst outputArra圓 = inputArray.slice(-2) See the slice() method documentation for more information. The end index is completely an optional parameter. The advantage of using slice over splice is that the original array is not mutated as it is in a splice.Įach element present in the start and end indexes (including the starting element and stopping an element before the end) is inserted into the new array. If only the starting index is specified, it returns to the last element. And based on that, the part of the array between the indices will be returned. This cut occurs by taking two inputs, the start index and the end index. This method cuts the array in two places. The slice() method is a built-in method provided by JavaScript. Extract the Subset of Array Elements From an Array Using slice() in JavaScript
#Javascript splice array how to
We will learn how to extract some of the elements or subsets of an array in JavaScript. Unlike other languages, JS arrays can hold different data types at different indices of the same array. The JavaScript array can store anything like direct values or store JavaScript objects. In JavaScript, arrays are regular objects containing the value at the specified key, a numeric key.Īrrays are JavaScript objects with a fixed numeric key and dynamic values containing any amount of data in a single variable.Īn array can be one-dimensional or multi-dimensional.
#Javascript splice array free
The XSP library contains classes that access the browser context.Examination App in JavaScript with Source Code 2021 | Examination App in JavaScript freeloadĪll of these items are accessed through an index. Represents common mathematical values and functions. Gets the string representation of an Array object. Gets the string representation of an Array object taking into account the host locale. Gets elements from an array to form another array.ĭeletes elements from an array, optionally replacing them, to form another array. Reverses the order of the elements of an array. Joins the elements of an array to form one string.Įxtends the object with additional properties and methods.
#Javascript splice array plus
The Standard library contains classes for manipulating data of different types and performing common operations.Ĭonstructs a new array from an array plus appended elements. The Runtime library contains classes that provide useful methods for globalization. This library provides access to the HCL Domino® back-end. Represents a document in XML Document Object Model format. Entering the name of a global object instantiates it. Global objects provide entry points to server-side scripts.
Global objects and functions (JavaScript™).
Client-side scripts are interpreted by the browser.Ī simple action performs a pre-programmed activity that can be modified by arguments. The JavaScript described here applies to the server-side interpreter. The JavaScript™ language elements are based on the ECMAScript Language Specification Standard ECMA-262 (see ).
JavaScript™ language elements (JavaScript™).
This reference describes the JavaScript™ language elements, Application Programming Interfaces (APIs), and other artifacts that you need to create scripts, plus the XPages simple actions.

0 notes
Text
Pdfkit italic

#Pdfkit italic pdf#
#Pdfkit italic update#
#Pdfkit italic code#
ttf file for each font that you downloaded. For example, when you download a Google Font, you’ll get the. Most fonts that you purchase or download will have this format available. The API embraces chainability, and includes both low level functions as well as abstractions for higher level functionality.
#Pdfkit italic pdf#
Description PDFKit is a PDF document generation library for Node and the browser that makes creating complex, multi-page, printable documents easy. To use a custom font in your PDF file, you need a. PDFKit A JavaScript PDF generation library for Node and the browser. Let's get started! Adding Custom Fonts to jsPDF Otherwise, follow the steps in this tutorial. If you want use one of the above fonts, then you can set it using the setFont() method without taking any additional actions. By default, pdfmake will look for 'Roboto' in style 'normal'. The thing is I dont know the size of the PDF's content, it comes from the client side. Before getting started, we will need fonts. I am using pdfkit to create a new PDF, and node-typescript to code, and I want to each new page in a separate PDF file (with 1 page only).
#Pdfkit italic update#
The 14 standard PDF fonts are as follows: Let's create an index.js file, and update the package.json by adding the start script. If you want to include custom fonts to match your brand or style guide, then you're going to need to take a few extra steps. If you are using a standard PDF font, just pass the name to the font method. Of course, the fonts in question are fairly expensive, and acquiring a copy just for this purpose would be poor form.The standard jsPDF 1.5.3 implementation only supports the 14 standard PDF fonts. doc.font ('Helvetica-Bold') // as an example If you want to use ur own font: PDFKit supports embedding font files in the TrueType (.ttf), TrueType Collection (.ttc), and Datafork TrueType (.dfont) formats.To change the font used to render text, just call the font method. PDFSelection objects have an attributedString property, but for fonts that are not installed on my computer, the NSFont object is Helvetica. While PDFKit makes it easy to retrieve color, retrieving the original font name seems to be non-obvious. I now find that I have the opposite problem.
#Pdfkit italic code#
Pdfminer provides scripts with the font name, but not the color, and it has a number of other issues so I'm working on a Swift version of that program, using Apple's PDFKit, to extract the same features. doc PDFDocument the PDF document created with PDFKit svg SVGElement or string the SVG object or XML code x, y number the position where the SVG will be added options Object > - width, height number initial viewport, by default it's the page dimensions - preserveAspectRatio string override alignment of the SVG content inside its viewport - useCSS boolean use the CSS. For instance: bold, italic and color should trigger different behavior. The PDFKit API is designed to be simple, so generating complex documents. On some text segments, the font style can have semantic significance. PDFKit is a PDF document generation library for Node and the browser that makes creating complex, multi-page, printable documents easy. Besides Symbol and Zapf Dingbats this includes 4 styles (regular, bold, italic/oblique, bold+italic) of Helvetica. I wrote a script which parses information from PDF files and outputs it to HTML. PDFKit supports each of them out of the box.

0 notes
Text
Javascript splice array
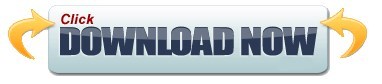
Javascript splice array how to#
Javascript splice array free#
It takes 2 parameters, and both are optional. It divides a string into substrings and returns them as an array. Slice( ) and splice( ) methods are for arrays. log ( months ) // adding by index, 5 elements removed log ( months ) // adding by index, no element removedĬonsole. splice ( 1, 0, 'Feb', 'March' ) Ĭonsole. Var months = // adding by index, no element removed log ( months ) // removing by index and number of element , itemX) Parameters Return Value An array containing the removed items (if any). The splice () method overwrites the original array. Var months = // removing by indexĬonsole. Definition and Usage The splice () method adds and/or removes array elements. Removing an element using splice method : Number of elements: number of element to remove
Javascript splice array how to#
Lets see how to add and remove elements with splice( ):Īrray. The splice( ) method changes an array, by adding or removing elements from it. The name of this function is very similar to slice( ). slice ( 2, 4 )) // Extract array element from index 1 and n-1 indexĬonsole. slice ( 2 )) // Extract array element from index 2 and n-1 indexĬonsole. Var employeeName = // Extract array element from index-2Ĭonsole. Removes deleteCount elements starting from startIdx (in-place) // 2. Let's take a closer look: // Splice does the following two things: // 1. Even though the splice() method may seem similar to the slice() method, its use and side-effects are very different. Lets see the below example for slice method : Splitting the Array Into Even Chunks Using splice() Method. Until: Slice the array until another element index It doesn’t change the original array.įrom: Slice the array starting from an element index The javascript splice() method is used to add or remove elements/items from an array.
Javascript splice array free#
I hope it helps, feel free to ask if you have any queries about this blog or our JavaScript and front-end engineering services.The slice( ) method copies a given part of an array and returns that copied part as a new array. If either argument is greater than the Array’s length, either argument will use the Array’s length If either argument is NaN, it is treated as if it were 0. shows, original array remains intact. Unlike slice() and concat(), splice() modifies the array on which it is. Use negative numbers to select from the end of an array. splice() is a general-purpose method for inserting or removing elements from an array. If omitted, all elements from the start position and to the end of the array will be selected. An integer that specifies where to end the selection. No problem Use the method Array.isArray to check⦠No problem Use the method Array. Use negative numbers to select from the end of an array.Īrgument 2: Optional. Better Array check with Array.isArray Because arrays are not true array in JavaScript, there is no simple typeof check. An integer that specifies where to start the selection (The first element has an index of 0). The slice() method can take 2 arguments:Īrgument 1: Required. If Argument(1) or Argument(2) is greater than Array’s length, either argument will use the Array’s length.Ĭonsole.log(array7.splice(23,3,"Add Me")) Ĭonsole.log(array7.splice(2,34,"Add Me Too")) Ĥ. It is a general purpose method to either replace or remove elements. If Argument(2) is less than 0 or equal to NaN, it is treated as if it were 0.Ĭonsole.log(array6.splice(2,-5,"Hello")) Ĭonsole.log(array6.splice(3,NaN,"World")) Array.splice() method in JavaScript provides a more refined way to manipulate an array. If Argument(1) is NaN, it is treated as if it were 0.Ĭonsole.log(array5.splice(NaN,4,"NaN is Treated as 0")) shows, returned removed item(s) as a new array object.Ĭonsole.log(arra圓.splice(2,1,"Hello","World")) howMany An integer indicating the number of old array elements to remove. syntax array.splice(index, howMany, element1,, elementN) index Index param specifies where a new item should be inserted. The new item(s) to be added to the array. The javascript splice() method is used to add and remove items from an array. And if not passed, all item(s) from provided index will be removed.Īrgument 3…n: Optional. If set to 0(zero), no items will be removed. An integer that specifies at what position to add /remove items, Use negative values to specify the position from the end of the array.Īrgument 2: Optional. The splice() method can take n number of arguments:Īrgument 1: Index, Required. However, in this case we can reimplement it to transform it into immutable. The splice() method changes the original array and slice() method doesn’t change the original array.ģ. The splice method allows us to add elements from a position inside an array (or replace them if we indicate how many we want to eliminate), but unfortunately it is mutable. The splice() method returns the removed item(s) in an array and slice() method returns the selected element(s) in an array, as a new array object.Ģ.
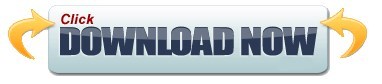
0 notes
Text
Javascript password generator

Javascript password generator how to#
Javascript password generator generator#
Javascript password generator code#
Javascript password generator license#
Javascript password generator license#
For more license details you can check the readme file in the downloaded folder. Remember to use this project just for educational purposes. If you face any issues running it then firstly, We suggest you use the latest versions of browsers like Google Chrome and Mozilla Firefox, etc then check for other solutions. So, load the Font Awesome 5 CSS into the head tag of your HTML page.
Javascript password generator generator#
This password generator tool uses Font Awesome for the copy icon for the generated password copy to the clipboard button.
Javascript password generator how to#
Requires Font Awesome Iconic Font in this instance. How to Create JavaScript Random Password Generator 1. Create Copy buttons contained in the password subject. Create a button to generate a powerful password. How To Run the Password Generator Project Without Any Problem?Īfter downloading this project, extract the zip file, open the folder and click on the index file which will open in your default browser. Create an input file to place the generated password strings. This created password will not be saved at any place. You can copy the generated password to the clipboard and use it easily. Now a password will appear on the screen which consists of different characters as per your given instructions. To get the character of the string of a particular index we can use.
Javascript password generator code#
the we will use Math.random () and Math.floor () method to generate a number in between 0 and l-1 (where l is length of string). Copy the below and paste it into the source code of your web page. You will have to choose a minimum of one option to create a password. Approach 1: Make a string consist of Alphabets (lowercase and uppercase), Numbers and Special Characters. Other boxes will be for numeric, lower, and upper characters respectively. html file or opening the file using Google Chrome or any other browser.Then the next section for the special characters clicks on confirm if you want to add them to your password. You can also open the project by double clicking on. After you are done, open your browser and type the URL for example. After extracting, copy the project folder to your destination folder. zip file using any zip programs such as Winrar or 7Zip. After you download the program, unzip the. To run the project, you will need to download WINrar or 7Zip to extract the. This application uses a different input field like numbers, texts, Uppercases and symbols to generate a perfect password for you.
You can select whether to inlclude Symbols like $%# or not(checkbox)Ī simple web application designed to run on browser and to generate strong password using input from the users. How to create a password generator - JavaScript - For this, use Math.random() to create random passwords.ExampleFollowing is the code function makePassword(.
You can select whether to inlclude Numbers or not(checkbox).
You can select whether to inlclude Uppercase letter or not(checkbox).
You can select the number of characters you want on your password.
If you fall within this category, you should consider using this Password Generator web application which quickly generate a perfect strong password along with the input you feed to the application. About Easy Password Generator Project In JavaScript Other. So, many people often struggle with creating a strong password including symbols and numbers. Learn to create utility applications using JavaScript Programming Language. There you have it we successfully created a Simple Random Password Generator using javascript. To do this just copy write the code as shown below inside the text editor and save it as script.js. This code will generate a password characters where the length is base on the input type value. Because I can't use any HTML element directly in JavaScript. Here the constant of the display and the button is determined. Create an input file to place the generated password strings. First, the constant of some HTML functions is determined. Now you need to use some JavaScript to activate this Random Password Generator. In the internet, we often use various passwords in various websites, using similar passwords might be risky. This code contains the script of the application. Activate Password Generator with JavaScript.

0 notes
Text
Json to xml converter
JSON to XML is an online tool that converts JSON to XML files. If you want to convert the file, just put the website URL, upload the file and click the "Convert to XML" button to get the result. JSON, as you know, is text written using JS object notation, a human-readable form of data exchange. It uses only the standards of the JavaScript language, but since it's a textual structure, it's language agnostic, so you don't have to read or write any JavaScript code.
JSON runs smoothly everywhere and contains few JavaScript-like elements. Since the data requested by servers and browsers must be in text format, and JSON is text, JavaScript can easily be converted to JSON and sent to the server for data exchange.
JSON can be converted back to JavaScript when received from the server. So there is no way to get into the complexity of translation. Data stored in a JavaScript object can be easily converted to JSON using the JSON.stringify() function and available for reception using the JSON.parse() function. Below are the JSON data types and elements, which are the same as in JavaScript.
These are enclosed in curly braces, also called curly braces, and are described as key-value pairs. The key must be a string and the value must be a valid JSON data type. These keys and values are separated by columns and each key-value pair is separated by a comma. Objects can be easily accessed with dot or bracket notation. You can also iterate over the properties using a for-in loop. We know that the values are stored in a JSON object. The value can also be another JSON object that can be accessed in the same way. You can also use dot notation to change JSON values if you want.
If you haven't already noticed that these arrays are similar to those used in JavaScript, the values in the array can be strings, another object, another array, null, or booleans. The only difference is that JavaScript can contain more functions and valid JS expressions. A JSON array can contain multiple values. These values are comma separated and can also contain objects. Values can be accessed and deleted/modified by index number. Values in the array start at zero. JSON is now widely used and considered a better format than XML, but sometimes you need to use a JSON converter tool. The most common reason is, if your program only supports XML, how can you convert JSON to XML on the fly? Is the best way to use an online converter to convert the JSON to XML, or develop an entirely new version of the file and deploy it to the program?
They share similar characteristics, although there are some significant differences. Almost half of the world uses XML as a data exchange format, as data exchange has not yet fully switched to JSON.
By comparison, JSON and XML are the same because both are human-readable or self-describing, and contain hierarchical values. That means value within value and is used in different programming languages for parsing.
A question arises here. What's the difference? The answer is very simple. If you already know how to write JSON, you'll see little difference because it doesn't require closing tags and is shorter than XML. Reading a file is more convenient because it's less verbose, you can quickly check if a value is spelled correctly, and you can use arrays in JSON. This is a big advantage.
The only reason you need a transform is if your program has an XML parser, as JSON can only be parsed using JS functions. Also, JSON is faster in AJAX applications because you don't have to traverse the document to extract the value and store it in a variable. I only need the Json.parse function and nothing else. The reason why XML is still so widely used is that it is a markup language. This means that you can add additional information to plain text. Also, the object notation does not support it. This is also good, but XML is not. We can solve your problem quickly.
XML uses a tag structure and supports namespaces, but JSON does not. XML doesn't support arrays, so its documents are a little less readable than JSON. XML is more secure than JSON. It supports comments and various encodings, but JSON does not support comments, only UTF-8 encoding.
visit : https://jsontoxmlconverter.com
1 note
·
View note
Text
February 7, API Lab - Arduino as API I
Introduction of the Arduino sketch to connect the LED to the Arduino.
In this class we had to get the ArduinoJson library v.6.19.1, compile the Arduino sketch Love prepared for us and upload it to the Arduino. He explained the code, beginning with the creation of the serial port for the browser to connect with the Arduino.
1. Request Access to a Point
The readJsonFromSerial function in the sketch is where the Arduino gets the Json document from the JavaScript frontend over the serial connection and displays the value of the LED.
It is important that the StaticJsonDocument value is <32>, otherwise we won’t get correct responses. Then, if the Json document we know has a serial we then deserialize, this to translate it, so the Arduino makes sense over the Json and the input.
The input is to extract the brightness property and give it to the LED based on the value. Then we set the pins correctly.
In the JavaScript we start with an ‘if’ to set an ‘alert’ if the browser does not support the web serial. We need a Chromium based browser. Then, we create a button with a variable to request a port with a document.querySelector and add an event.Listener with an asynchronous event. Inside of this function we are going to await for the navigator to give us a port for the serial request:
navigate.serial.requestPort
The console.dir(port); to give permission to the user to communicate with the port.
2.Make sure the Arduino is connected
First we make a state object to call on our application data, now we can reference the serial anywhere in the program like this:
state.serial = port;
document.querySelector("#connection-status").innerHTML = "Arduino is connected!";
It will be set to be our serial device, so it can change the connection status. The Baud rate is set to specify the way we want to communicate, it should be the same in the Arduino as in the JavaScript.
3.Send Data
In order to send the data we need to access the brightness slider first, and to do that we create a function (almost the same way we did with the serial port button). We select a document with a querySelector to the brightness slider and we add an eventListener for it to listen to the input event. A function for the brightness with an event to target the value, to finally get the brightness state. We also need to add a value of 0 to a brightness state. To avoid getting our values in ‘string’ we add parseInt to get number values. Now we need to send these brightness values to our Arduino, to do that we need to create a function:
write.JSONToArduino()
We call it then to be an asynchronous function that is going to take a property name. In this part we need to make sure we have a serial device connected, if not, we throw an error.
We now want to get the data so, we first need to have the data stored in an object but we can instead have it stored in the state as dataToWrite, so the code looks like this:
const data = state.dataToWrite;
To finish, we want to listen to JSON so we write a variable that stringyfies the data. The writer function was said not to be of our interest but what we need to know is that it is something in which we send the data over the serial. The writer will write whatever is in our payload and our payload is going to be a new text encoder that encodes the JSON data.
youtube
0 notes
Text
Find Usb Serial Number Registry

Please suggest a solution to configure virtual com port over USB 2 type A so that the software may communicate with the device through USB port. Note that device manager does not show any USB-to-serial devices even with variable 'devmgrshownonpresentdevices=1'. For USB device, the device instance ID consists of VID/PID and serial number, like USB VIDvvvv&PIDpppp serialnumber where, vvvv, pppp: 4-digit HEX number for VID/PID 'Device Identification Strings' on MSDN Then, the serial number is retrieved from the registry as the device instance ID. SetupDiGetDeviceInstanceId is the function to do it. The volumes that have been mounted and the assigned drive letters are stored in the following registry entry: System MountedDevices Locating the USB drive’s manufacturer name and serial number in this registry key may provide examiners with the drive letter of the volume. Figure 4: Locating USB drive in /System/MountedDevices.

Export HKEYLOCALMACHINESOFTWAREMicrosoftWindows NTCurrentVersion registry key to text file on USB thumbdrive. Boot into Windows, open registry key dump in Notepad, copy DigitalProductId value into the clipboard. Paste clipboard into Javascript.
Your installation of Windows no longer boots, and unfortunately you don't have a copy of your Windows product key in order to reinstall the operating system? Don't worry, it's possible to recover Windows Product Key from registry in offline mode, even if Windows is no longer bootable.
This article explains how to find and recover Windows Product Key from the registry, extract Windows Serial Number from registry in offline mode, and get Windows CD Key from unbootable computer.
Contents
1. Intended audience of this article
This article is for people who want to reinstall Windows, but lost or forgotten Windows Product Key.
Using Registry of your old Windows installation, you can retrieve Product Key once upon a time used to install Windows. This method works even if Windows is broken and unbootable, or if your computer crashes during startup.
All you need to recover your Windows Product Key is readable (non-broken) HKEY_LOCAL_MACHINESOFTWARE Registry hive.
2. Solution in a nutshell
Boot into Emergency Boot Kit
Run Emergency Boot Kit Registry Editor, choose Windows installation and open HKEY_LOCAL_MACHINESOFTWARE Registry hive
Export HKEY_LOCAL_MACHINESOFTWAREMicrosoftWindows NTCurrentVersion registry key to text file on USB thumbdrive.
Boot into Windows, open registry key dump in Notepad, copy DigitalProductId value into the clipboard.
Paste clipboard into Javascript form on this website to decode your Product Key.
3. Extracting DigitalProductId from registry
1) Download Emergency Boot Kit and deploy it USB thumbdrive according to the instructions, then set up your BIOS to boot from USB thumbdrive. Demo version of Emergency Boot Kit will suffice for the purpose of this article.
2) Boot Emergency Boot Kit and run Registry Editor from the main menu:
3) Wait for Emergency Boot Kit Registry Editor to start:
4) Choose Windows Installation to extract Product Key (this screen will not appear if you have one copy of Windows installed on your PC):
5) Choose HKEY_LOCAL_MACHINESOFTWARE on this screen:
6) Emergency Boot Kit Registry Editor is loading Registry Hive with Windows Product Key:
Usb Serial Number
7) Using arrow UP and DOWN keys on keyboard, go to Microsoft key and press ENTER:
8) Using arrow UP and DOWN keys on keyboard (and optionally PAGE UP and PAGE DOWN), go to Windows NT subkey and press ENTER:
9) Using arrow UP and DOWN keys on keyboard, go to CurrentVersion subkey and press ENTER:
10) Press TAB to switch input focus to the right panel. Scroll down with arrow keys and PAGE DOWN / PAGE UP keys:
11) Make sure DigitalProductId value of type REG_BINARY exists:
Usb Serial Driver
12) Press F3 on the keyboard to open Registry Key Export dialog window, then click (...) button with a mouse:
13) In this Save File common dialog, click on the USB thumbdrive with Emergency Boot Kit, then click on the text area next to 'File Name:' label and type some file name from keyboard. File name must have .txt suffix.
14) Click OK to confirm export of Registry Key to the file:
15) Message like this should appear on the screen:
16) Choose 'Reboot' in the main menu of Emergency Boot Kit:
Unplug Emergency Boot Kit from USB port and boot into Windows (maybe on the different PC, where you are browsing this page). Plug USB thumbdrive with registry key dump and open My Computer:
Open USB thumbdrive with registry key dump in Exporer:
Double-click on registry key dump file to open it in the Notepad (won't open if it wasn't saved with .txt extension):
Find 'DigitalProductId'=... registry value, select it using your mouse or SHIFT + arrow keys and the copy it to the clipboard:
4. Decoding DigitalProductId
5. References
Inside Windows Product Activation, a paper from Fully Licensed GmbH: http://www.licenturion.com/xp/fully-licensed-wpa.txt
Getting Windows Product Key from Registry, an answer from SuperUser.com: https://superuser.com/questions/617886/recovery-disk-install-to-a-specific-partition
Interactive JavaScript-based Microsoft Digital Product ID Decoder, by Ed Scherer: http://www.ed.scherer.name/Tools/MicrosoftDigitalProductIDDecoder.html
Find Usb Serial Number Registry Tool

0 notes