#design patterns python
Explore tagged Tumblr posts
Text
Normally I just post about movies but I'm a software engineer by trade so I've got opinions on programming too.
Apparently it's a month of code or something because my dash is filled with people trying to learn Python. And that's great, because Python is a good language with a lot of support and job opportunities. I've just got some scattered thoughts that I thought I'd write down.
Python abstracts a number of useful concepts. It makes it easier to use, but it also means that if you don't understand the concepts then things might go wrong in ways you didn't expect. Memory management and pointer logic is so damn annoying, but you need to understand them. I learned these concepts by learning C++, hopefully there's an easier way these days.
Data structures and algorithms are the bread and butter of any real work (and they're pretty much all that come up in interviews) and they're language agnostic. If you don't know how to traverse a linked list, how to use recursion, what a hash map is for, etc. then you don't really know how to program. You'll pretty much never need to implement any of them from scratch, but you should know when to use them; think of them like building blocks in a Lego set.
Learning a new language is a hell of a lot easier after your first one. Going from Python to Java is mostly just syntax differences. Even "harder" languages like C++ mostly just mean more boilerplate while doing the same things. Learning a new spoken language in is hard, but learning a new programming language is generally closer to learning some new slang or a new accent. Lists in Python are called Vectors in C++, just like how french fries are called chips in London. If you know all the underlying concepts that are common to most programming languages then it's not a huge jump to a new one, at least if you're only doing all the most common stuff. (You will get tripped up by some of the minor differences though. Popping an item off of a stack in Python returns the element, but in Java it returns nothing. You have to read it with Top first. Definitely had a program fail due to that issue).
The above is not true for new paradigms. Python, C++ and Java are all iterative languages. You move to something functional like Haskell and you need a completely different way of thinking. Javascript (not in any way related to Java) has callbacks and I still don't quite have a good handle on them. Hardware languages like VHDL are all synchronous; every line of code in a program runs at the same time! That's a new way of thinking.
Python is stereotyped as a scripting language good only for glue programming or prototypes. It's excellent at those, but I've worked at a number of (successful) startups that all were Python on the backend. Python is robust enough and fast enough to be used for basically anything at this point, except maybe for embedded programming. If you do need the fastest speed possible then you can still drop in some raw C++ for the places you need it (one place I worked at had one very important piece of code in C++ because even milliseconds mattered there, but everything else was Python). The speed differences between Python and C++ are so much smaller these days that you only need them at the scale of the really big companies. It makes sense for Google to use C++ (and they use their own version of it to boot), but any company with less than 100 engineers is probably better off with Python in almost all cases. Honestly thought the best programming language is the one you like, and the one that you're good at.
Design patterns mostly don't matter. They really were only created to make up for language failures of C++; in the original design patterns book 17 of the 23 patterns were just core features of other contemporary languages like LISP. C++ was just really popular while also being kinda bad, so they were necessary. I don't think I've ever once thought about consciously using a design pattern since even before I graduated. Object oriented design is mostly in the same place. You'll use classes because it's a useful way to structure things but multiple inheritance and polymorphism and all the other terms you've learned really don't come into play too often and when they do you use the simplest possible form of them. Code should be simple and easy to understand so make it as simple as possible. As far as inheritance the most I'm willing to do is to have a class with abstract functions (i.e. classes where some functions are empty but are expected to be filled out by the child class) but even then there are usually good alternatives to this.
Related to the above: simple is best. Simple is elegant. If you solve a problem with 4000 lines of code using a bunch of esoteric data structures and language quirks, but someone else did it in 10 then I'll pick the 10. On the other hand a one liner function that requires a lot of unpacking, like a Python function with a bunch of nested lambdas, might be easier to read if you split it up a bit more. Time to read and understand the code is the most important metric, more important than runtime or memory use. You can optimize for the other two later if you have to, but simple has to prevail for the first pass otherwise it's going to be hard for other people to understand. In fact, it'll be hard for you to understand too when you come back to it 3 months later without any context.
Note that I've cut a few things for simplicity. For example: VHDL doesn't quite require every line to run at the same time, but it's still a major paradigm of the language that isn't present in most other languages.
Ok that was a lot to read. I guess I have more to say about programming than I thought. But the core ideas are: Python is pretty good, other languages don't need to be scary, learn your data structures and algorithms and above all keep your code simple and clean.
#programming#python#software engineering#java#java programming#c++#javascript#haskell#VHDL#hardware programming#embedded programming#month of code#design patterns#common lisp#google#data structures#algorithms#hash table#recursion#array#lists#vectors#vector#list#arrays#object oriented programming#functional programming#iterative programming#callbacks
19 notes
·
View notes
Text
Do you know the Facade Pattern?
youtube
#java#100daysofcode#coding is fun#design patterns#python#python for beginners#coding#programming#software#technology#internet#javaprogramming#developer#software development#java for beginners#python programming#pythonprojects#facade#architecture#pattern#Youtube
0 notes
Text
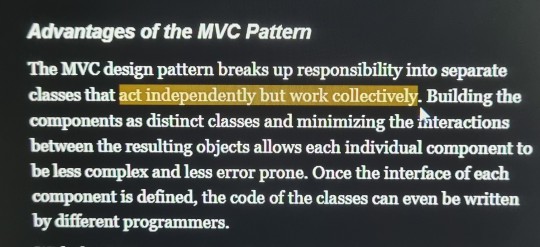
So true for life as well!
"Act independently but work collectively."
_ Irv Kalb
0 notes
Text
Understanding Design Patterns in Python: A Beginner's Guide
Design patterns in Python offer structured solutions to common programming problems. They assist developers in creating scalable, efficient, and maintainable code. Let's delve into some fundamental design patterns and their significance.
Singleton Pattern: Ensures a class has only one instance and provides a global point of access to it.
Factory Pattern: Creates objects without specifying the exact class to be instantiated.
Decorator Pattern: Adds new functionalities to objects dynamically, without altering their structure.
Observer Pattern: Establishes a one-to-many dependency between objects, allowing changes in one object to reflect in others.
Strategy Pattern: Defines a family of algorithms, encapsulates each one and makes them interchangeable.
Implementing these patterns in Python enhances code readability and flexibility. They provide standard solutions, aiding in better software architecture and improved problem-solving capabilities.
Learning and implementing design patterns in Python is pivotal for aspiring developers. They simplify complex coding scenarios and foster efficient software development practices.
For an in-depth understanding of Design Patterns in Python, explore Tutorial and Example's comprehensive guide. Dive deeper into these patterns and elevate your Python programming skills!
0 notes
Text
Explore comprehensive design patterns tutorial, unraveling concepts for software development efficiency and mastery. Learn essential strategies and implementations for optimal coding structures.
1 note
·
View note
Text
Special Method and Singleton Class in Python
Every Python class has some special method start with __ and end with __. Let’s start with a empty class and see what is special methods are defined in it. class EmptyClass: pass if __name__ == "__main__": print(dir(EmptyClass)) Here is the output of it. [‘__class__’, ‘__delattr__’, ‘__dict__’, ‘__dir__’, ‘__doc__’, ‘__eq__’, ‘__format__’, ‘__ge__’, ‘__getattribute__’, ‘__getstate__’,…
View On WordPress
0 notes
Text
Backend for Frontend Design Pattern for Microservices Explained with Examples
Full Video Link https://youtu.be/CRtVz_kw9qA Hello friends, new #video on #backendforfrontend #bff #designpattern for #microservices #tutorial for #api #developer #programmers with #examples are published on #codeonedigest #youtube channel
In this video we will learn about Backend for Frontend design pattern for microservices. Backends for Frontends (BFF) is a microservice design pattern to handle the complexity of client-server communication where we have multiple user interfaces. This pattern suggests having a separate back-end service for each frontend to handle the specific needs of that interface. This pattern allows…
View On WordPress
#backend for frontend#backend for frontend (bff) pattern#backend for frontend design pattern#backend for frontend developers#backend for frontend microservices#backend for frontend pattern#backend for frontend pattern example#backend for frontend pattern vs api gateway#backend for frontend python#backend for frontend tutorial#bff pattern#microservices#microservices architecture#microservices tutorial#system design#what are microservices
0 notes
Text
i 100% absolutely cannot i repeat CANNOT allow myself 2 fail this course bc this is my last chance at taking it otherwise im removed from the program but i
cannot make myself do the work. i can't start. we're halfway thru the term ive lost a HUGE percentage of the grade already and i sit down 2 start googling how tf to do what i need 2 do and i fucking c a n t and now the whole course has become this hot-stove-item in my brain and im lying in bed practically vibrating with anxiety abt to let another (re-negotiated!!!!!!!) deadline pass and like!!! why am ilike this!!!!!!
ANYWAYS if any of yall know literally fuckall abt python...... pls........ 🙏 help........ 🙏
#if i could just figure out how 2 get started but like..... what does a python project even LOOk LIKE???#whats the file structure??? is it like java with classes?? how many files should i even end up with???#i hate college#literally just thinking abt this project in order 2 write this post has me on the verge of crying#how hard can it possibly be. and yet. 💀#nothing worse than asking 4 an extension then fucking that up too ohhh my godddd#in theory i agree w/ the principle of a self directed course where u gotta teach urself bc obvs thats important in coding but.....#i am very small. and very stupid. and need some1 2 hold my hand thru the dreaded Getting Started process#except i shouldve been Getting Started 7 weeks ago not uhhh 4 or 5 deliverables tho#technically. TECHNICALLY. i can still pass. maybe. theres 60 marks remaining. so far i have................................................5#:(#gotta uhhhh use a record object and file i/o and a given dataset and smth smth CRUD functions smth smth MVC design pattern & idek what else#if ever there was a time 4 a random unexplained jet engine 2 take me out donnie darko style...... jus saying....
0 notes
Text
EDIT: the viper example picture got changed to a proper viper species, thank you @/the-white-eye for the correction!
everytime i see the winged serpents drawn/modelled as vipers, my heart breaks a little bit
guys, they are specifically pythons!! :3 mouth shape and all!! the sole viper head we see is Messmer/abyssal serpent!!


pythons kill prey by strangling them with their bodies. vipers hunt through venom bite pounces. pythons are also more notably languid and slow compared to vipers or cobras. this isn't just a thematic distinction, but also a clear visual design cue, too!
in Volcano Manor and the Temple of Eiglay, you can see that the winged snake statues are Also python-headed. it's also notable that the snakes in the statues have fangs, and so do the winged serpents Messmer has. i doubt that's a coincidence.
and most importantly, the Great Serpent is also closer to a python than a viper or even a cobra. it's admittedly a more distinct head shape than any real-life snakes i'm aware of, but the longer snout, the scale patterns (particularly on the temples and side of the head), and the teeth type are not unlike an anaconda, actually.

#minor vent but GUYS THIS IS VISUALLY IMPORTANT#elden ring#messmer the impaler#elden ring meta#elden ring theory#sote dlc#sote spoilers#elden ring dlc#shadow of the erdtree#pardal rambles
844 notes
·
View notes
Text

Welcome back to Overcomplicating the Pyrrhian Tribes! This week: the beloved RainWings!!
You know what's up. Joy Ang and Tui are so cool and I am just me.
Details and explanation below!
Otherwise, next week are the chilly IceWings! See you then!!!
More overcomplicated dragons.
I knew the RainWings would be really important, and I think they turned out the best of all the ones I've done. I think they're my favourite because they are basically the perfect mix of extra realism spice without altering Joy's design too much. The SkyWing design is awesome and I love it to bits, but it is one of the two that are the farthest from canon.
As for the RainWing.... I had. So. Much. FUN. I heavily used chameleons and snakes - they're basically the two main species on my research board - but there is a dash of cuttlefish and frilled lizard in there. Where, you ask? Well if you look closely, all over the RainWing are little tiny flecks of darker colour. I found a beautiful reference of a close-up on a cuttlefish eye. Its skin is dotted in thousands of little marks and I thought that would be perfect for the RainWing, who can camouflage just as well as them. I don't know if it's been discussed in canon but I bet they could animate their scales more than just colour shifting - cuttlefish are known for using their rapidly shifting patterns to hypnotize prey. RainWings could do it too, sort of like Ka from Disney's 2D animated Jungle Book.
Speaking of Ka - snakes. I love snakes. The head structure of the RainWing here is very smooth and rounded with muscles based on snakes like the python. I was even going to originally draw them in a venom striking pose and got as far as completing the lineart, but ultimately decided it wouldn't fit the calm portraits of the other tribes.
Will you see it in the future? Hell yeah! Pure, unhinged, magical death spit. Looking at it now I might try to alter it to be a full piece of Glory attacking Scarlet or Crocodile.
In the striking pose you can see the frills much better, but I still took my time on this serene pose (this is where the frilled lizard influence comes in). If you notice that I've drawn every scale (every single scale) then, yes, I am insane. If you didn't know that yet, you know it now. You have to draw guide lines and follow them meticulously while you wonder why you don't make a scale brush, and then cry because you know the randomness and imperfections that come from drawing a thousand circles is how it looks natural. The eye area is actually my favourite part, since drawing dragon eyelids was the original inspiration for doing this. Did I mention that? I wanted to draw eyelids.
EYELIDS.
I digress. Besides the eyelids, I like the frills on the action pose, but this pose is where I like the body scales more. When zooming in on my chameleon colour refs, I noticed the very rhythmical distribution of their scales and figured I would give it a try. They actually do have extra large circular scales along their bodies, which is where I guess the canon RainWing design gets it from. Very clever, Joy!
Anyway, on this version, those small circular scales appear on the face. Not only that, but I added a bit of influence from the snouts of my ref chameleons by extending the nose bridges to wrap around the nose horn. They blend in so seamlessly and that's the reason why I love this design - it's subtle, barely there, mostly Joy but a little extra.
Wow, I talk too much. If you're here, thank you! It's not mandatory to read, but very appreciated. I heard once that visitors at an art gallery look at each piece an average of 2-3 seconds. Or was it 3-6? Idk, but it was shockingly short, and ever since then I've tried to encourage myself to pay more respect to other artists and glean their work for little details I skip after that quick glance. I could talk so much more about these designs but that would be like an hour long video, each, lol. If you have questions about anything, ask away!
#wof#wings of fire#wof art#my art#digital art#art#rainwing#wof rainwing#wof fanart#Overcomplicating the WOF Tribes
483 notes
·
View notes
Text
The Assistant Book Seller
Edit 1 Dec 2023 - added missing information on the "ribbon pattern."
Edit: 3 Dec 2023 - correct information about middle pattern from creator
GABRIEL: Greetings! I'm Jim! It's short for James, but I don't need to keep telling everyone that. I'm an assistant book seller.
I'm sorry. Before I do anything else, I need to apologize for something I need to write further in. I didn't plan to write it, I just kind of bumped into it and, well, I can't ignore it. So...sorry. It's said. Forgive me for what needs to be done.
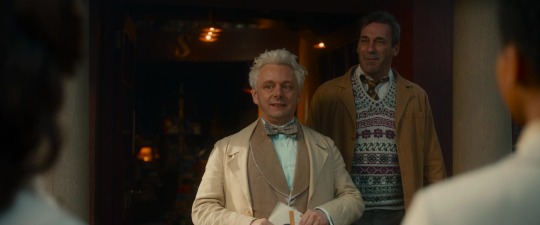
Although he arrived with nothing but a cardboard box and Rodney the Stunt Fly, Aziraphale made sure Jim was clothed in appropriate raiment while under his protection. We'll forgive him that he took a step back about, oh, fifty years or so to the 1970's, as Jim's overall look is a nod to the famous old sitcom "Open All Hours." So if he looks a little bit out of place, or, a little bit familiar, even, that's why.
While we are used to seeing angels in overcoats, it's Jim's vest that is the particular feature here. But I will take a moment to comment on the overcoat - not just the colour but its lapels. Aziraphale has obviously given him a colour with an earthly connection and one that indicate that he has bought Jim under his protection, but the lapels look quite neutral, with one up and one down. (Muriel is the same in their Inspector uniform, btw) This is the first indication they are between two things at the moment.
Onto the vest.
There is so, so much work and thought put into this vest! It was a one-off commission for the show, and the creator, Sandy Higgins, has said she is not allowed to give away the final design pattern. I have tried to contact her, and I'm waiting for a reply, so in the mean time I thought I would ask my keen knitter of a sister-in-law about one of the patterns I'm not sure about. "Well, that's Fair Isle knitting," she said, but she knew nothing about the individual line pattern I was interested in. Hmm, I kind of know that already, its in the notes that are guiding me for this meta, but hey, why not do a broader search and see what comes up?
So once I got back home I did. "Fair Isle knitting patterns" hmm...Wikipedia page for starters...what on *earth* is that at the bottom of the page...? YOU ARE. FRIKKING. KIDDING ME!!!!!!!
"See also: Gumbys"
oh ffs
I am so sorry that needs must make me mention Monty Python yet again, but here we are. And we must mention them, because this link is just too...unbelievably, deliciously good.
If you aren't familiar with the Monty Python catalogue, and don't recognize the mention of Gumbys, they were a set of characters that dressed and spoke in a certain way but the main points to take away were they wore woolen vests in the Fair Isle knitted style and their catch-phrase was - wait for it - "My brain hurts!"
I think we've heard that somewhere before?
CROWLEY: When you first arrived, you said you were here because they were planning to do 'Something Terrible' to you. So you remembered it then. Remember it now. GABRIEL: It hurts to remember. My head isn't built for that.
Right. Now we've got that out of the way...back to the serious stuff.
The colours used in the vest are not your typical angel colours. There is a base of angelic off-white and there are some bits of purple for his royalty around the shoulder area - sometimes you need to look carefully for it. Otherwise it is dominated by vintage shades of red and green. Well. Who's an agent of change driven by love, then?
The horizontal stripe pattern is partly to remind us of the classic biblical robes with stripes that ran along them, much like the style of Crowley's black and red robe in the Job minisode, but is also part of the traditional Fair Isles pattern work. And each row only has two colours, but up around the shoulder area we do see purple start to sneak in as a third colour.
On to the incorporated symbols! I'm going to go from bottom to top.
On the lowest two we feature Crowley and Aziraphale. We have Crowley's demon satyr tail from the Good Omens logo on the lowest stripe - the double-headed arrow.
The next stripe is Aziraphale, with a variation of the classic OXO pattern ("hugs and kisses.") The X is meant to represent his angel wings, and the O is modified to mimic the "o" with a halo in the Good Omens logo. I've highlighted all three in the image on the right.
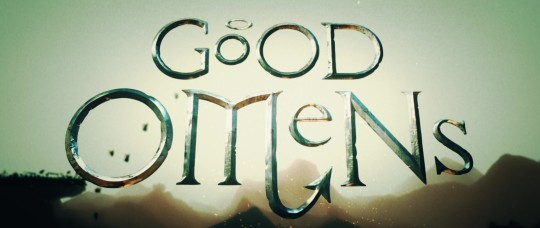
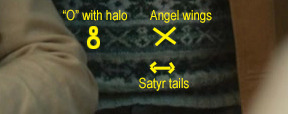
The third row up is a Sumerian Star pattern that represents one of the flowers associated with Gabriel, the lily. They are supposed to represent the purity of Mary, mother of Jesus, as he had one in his hand when he visited her during the Annunciation.
The row above that is what I believe to be a Byzantium pattern, and is included to show "an Angel's ability to be timeless."

The next three rows are still under a bit of a question mark as I write this. I plan to come back and edit it in if I find the answer.
The bottom of the three is the Duke of Buccleuch pattern, "to celebrate the long and necessary contribution that the cottage industry of hand knitted items."
The middle one - ? (perhaps you, the reader, know? It looks like a spiralling ribbon if I stand back, but that isn't sparking any connections, either.)
Edit: @noneorother tells me in a reblog (below) that this pattern represents the shoelace from the magic incantation Aziraphale uses "Banana Fish Gorilla Shoelace." So it is ribbon-like! This then points to the Second Coming, as it the shoelace references the end of the book, and the last paragraph of the book references Yeats poem "The Second Coming" as well as the novel 1984. To me it is then also telling us there is a cycle occurring, or a cycle that needs to be renewed. This fits in with some other clues other meta-writers have been picking up.
Edit 2: Turns out none of that was correct - I heard back from the creator herself and it's actually the double-ended satyr tail pattern again! It just seems to make a bit of an illusion of a ribbon or shoelace.

The pattern below is a modified OXO pattern.
The top one looks like two rams horns facing each other. A hollowed out rams horn can be used as a trumpet, and is known as a shofar in Jewish religion. Gabriel was traditionally known to carry a trumpet.


The ancient meander pattern would be recognized by most people, included as another classic timeless pattern found all over the world. For some it symbolizes eternity and endless flow.

The wheels here appear to be Michael's ophanim wheels, that would have eyes around the rims.
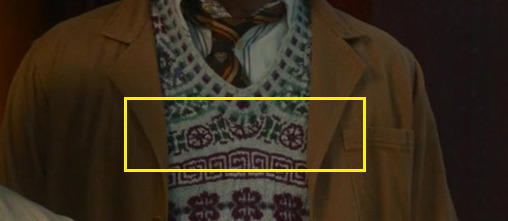
The hourglass is to remind us that time is running out. Memento mori - "Remember that you die." It is a major theme in both series.

Right up high, just before we lose the rest of the vest inside the overcoat, we get a glimpse of a large diamond-shaped icon. I wonder if this is another stylized set of angel wings, like we saw in the Job minisode on Aziraphale's golden collar.
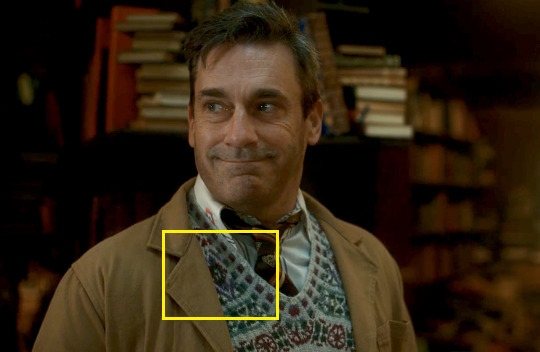
To finish off the outfit, he is wearing dark gray trousers with sneakers! I'm sure that's so he could keep sneaking up on Aziraphale in the shop, haha. His shirt seems a little too large for him and the tie is knotted too high and is not settled along his centerline. It's all at odds with his previous neat and sharp appearance as Supreme Archangel Gabriel.
I'd like to say a big thank you to @aduckwithears for helping me with information on the vest and finding the creator's other social media sites. You can see their two posts about it here and here.
#good omens#good omens 2#good omens meta#good omens analysis#aziraphale#gabriel#jimbriel#jim's vest#satyr tails#angel wings#halos#lily#meander pattern#rams horn pattern#hourglass#memento mori#open all hours#good omens costume meta#monty python#gumbys#my brain hurts!#fair isle
333 notes
·
View notes
Text
LETS STOP GATEKEEPING
I didn't use all those, it's just all the info i could gather
My patreon (free)|Discord
Apps to use to make CC's:
sims 4 studio (must)
canva (to make thumbnails and stuff, can be also any other program but it works best for me)
Krita/ibispaint or other drawing/photo editing software (that's where most of the work happens in making tattoo's/recoloring and stuff)
sims 4 manager (to make loading screens, here again canva comes handy, and pinterest of course)
Pinterest (to look for inspo/patterns)
Blender (to make 3d objects)
Marvelous Designer (to make clothes, not free unfortunately>:( )
Gshade/ReShade (to make reshades for the game)
Apps to use to make MODS:
Mod Constructor V5 (AN ABSOLUTE MUST IF YOU DON'T KNOW PROGRAMMING LIKE ME)
Sims 4 Studio (again, a must especially if you want to translate it or smt)
PyCharm (To my knowledge python is a really handy language that can enable you to make mods!)
Good to have:
mods that show you corrupted mods and CC's in the game(and if yours is not one of them)
CurseForge (for the same reason, it shows you which files are corrupted)
reddit (handy to ask for help there)
discords for ts4 creators, where u can ask 4 advice!
If i'll remember/see something else I'll let you know!!!
#the sims 4#ts4#sims 4#the sims custom content#ts4 custom content#ts4 simblr#ts4cc#ts4 cc#ts4 cc download
75 notes
·
View notes
Text
Hey people! As announced in my last post, my new post about the Abstract Factory Pattern is now happily available on my channel!😀
youtube
#java #javaprogramming #javatutorial #javaforbeginners #javaprojects #python #python3 #pythonprogramming #pythontutorial #pythonforbeginners #pythonprojects #programming #factory #abstractfactory #abstractfactorypattern #method #pattern #designpatterns #oop #objectorientedprogramming
#java#javaprogramming#java for beginners#100daysofcode#coding is fun#design patterns#python#python for beginners#coding#programming#software#technology#code#developer#python3#java online training#python programming#pythonprojects#abstract#factory#pattern#Youtube
0 notes
Text
Hmmm okay I have my shitty python script most of the way there. Basically, you know those stereographic projection sculptures Henry Segerman did? The 3d printed ones?
These:
youtube
https://youtu.be/lbUOScpu0ws?si=L0CtMawBZNWuzfdm
youtube
I want to do that with a lace sphere. Problem 1: map the intended shadow to a sphere.
Problem 2: project the spherical coordinates such that I can make the needlelace flat, with minimal distortion of the spherical image.
I got both of those figured out. So I can convert from the left photo below to the right photo below. Or. I can convert from a given photo to a maybe-not-too-distorted approximation of a flattened globe (I’m using an interrupted sinusoidal projection; if anyone even sees this and has suggestions for a better projection to use for this purpose, I would very much appreciate hearing about it. I got sick of reading about map projections very quickly).
The right image below would (hopefully, if I did it right) be the pattern that creates a sphere that will project the target image. It’s supposed to a tiling of abstract lotuses based on Greek pottery but. I don’t think they read very clearly. And they ended up upside down because I was checking that the coordinates were right by plotting them instead of converting them to an image. So. That’s a thing. I’ll fix it when I’m not tired of staring at my computer.
It will take forever to actually make a design I’m happy with and then execute it with thread, but I at least have the ability to make my computer do the projection for me now. Still have some things to work out regarding what maximum resolution i can use for the final image, but if need be I can fuss with the arguments until it’s a doable pattern.
#personal project#lacemaking#lace sphere#math#art idea#needle lace#mathematical art#sort of#Youtube#my post
31 notes
·
View notes
Text
NAGA BULLY AU!!!!! this is so niche oh my god
if u don't know nagas are basically snake people from religious mythology. the top half is a human, while the lower is a snake. there's all different kinds of interpretations of how they look, with some people perferring them to have a humanoid top half BUT keeping the snake head and patterns. like the serpentines from ninjago 💀
in my opinion, I see them with their snake patterns starting from the top of their head to the tail but keeping a human face and front torso of their human half if that makes sense?? I'm really bad at explaining things if u couldn't tell
anatomy designs!!


now that Ive given a breif description- I'm gonna be focusing on derby and bif because I'm unfortunately very biased and autistic (also tumblr is limiting photos anyways). feel free to give species hcs for other characters!!!
derby is an albino gaboon viper. they're my favorite snake ever and I could yap about them for hours and this choice is very much out of bias

look at this diva
facts about gaboon vipers!!!
-they do this thing called rectilinear locomotion (heavy bodied species of snakes do this) which makes they teeter in a straight line rather than slither. it makes them look like inch worms
-despite being the heaviest of any venomous snake, they have the fastest strike of any snake species
-gaboons have 2 inch fangs which is the longest of any snake. very impressive cheese wedge head ass
-they are impossible to spot in stray leaf litter, only moving their pupils and slowly puffing out when seeing a potentional threat bigger than them. they stay in the same spot for days on end until an unfortunate critter steps on their stupid faces
BIF!!!!! he's a giant albino burmese python with orange rings because.... ginger. he looks like the first picture but much bigger

burmese pythons don't need venom -- they use their entire body basically made of pure muscle to constrict prey and suffocate them. they are just big babies idc
that's all I need sleep
#bully canis canem edit#bully#canis canem edit#derby harrington#bif taylor#naga#serpent#snake#gaboon viper#burmese python#snake people
30 notes
·
View notes
Text
Introduction
Heya it’s mith! This is a nickname from my original name. I am Tamil and I’m from the state of Tamilnadu from south India :)
This is a study or/blog about my life as a CBSE student where I’ll post about motivation, any interesting topics and just tips that I found useful. I am planning on posting my 11th chemistry notes by the end of this year, once I’ve finished typing them up as I’ve hand written them! So you will see resources being posted!!
I am a high-school student, 17 years old ( year 12/ 12th grade )studying in the science stream. My subjects include —
Physics.
Chemistry.
Mathematics.
Computer Science (python)
English
Why a studyblr?
I opened this studyblr in order to stay productive and help others to do so too if I can. Also through this account I would like to share bits of my student life and motivate other students to do what a student should do like - study and enjoy life. I will post a lot about how to get motivation and how I study certain subjects and these posts will update as I go along!
Any competitive exams?
I am doing four exams ( sobs) which are for design and architecture respectively. These are NATA, JEE paper 2 ( this includes, maths, drawing and aptitude , not physics chemistry and maths ) , UCEED ( design) and NIFT ( design)
Future career?
I am hoping to study design or architecture and maybe get a literature or business degree as a side goal.
Any goals for 2025?
I wanna learn foreign languages such as Italian since it’s my current obsession. I’ve already learnt a bit of French so I’m planning on learning all of the Romance languages, so you will see me post about language learning too!!
More about me:
I was born in England and I’ve lived in India and England on and off, every few years I’d shift to England and then come back to India. I did my boards (10th) in the uk in the form of GCSEs. GCSEs are usually done in 10th and 11th so I’m repeating a year again in India so it’s easier for me for my 12th boards!
For Quick Navigation:
study blogging!
Tagged as #studydaily- it's where I post about my daily study logs.
study_plans!
Tagged as #study plans - it's in the name lol. It's where I post about what I'm gonna do to keep myself disciplined.
motivation!
Tagged as #motivation - to keep myself and you motivated! :)
litblr!
Tagged as #litblr - for literature and any other interesting topics
questions!
Tagged as #questions - just some questions lol!
know me!
Tagged as #know me — it's in the name :p
NATA and JEE2 help
Tagged as #NATAandJEE2 - where I post about the architecture exams and general tips and resources
UCEED and NIFT help
Tagged as #UCEEDandNIFT - where I post about the design exams and general tips and resources
Language learning!
Tagged as #languageblr - I post about my progress in learning languages, this will be separate to my daily logs so i won’t post whatever I learnt in languages in my daily logs. I might create a separate account for langauges alone!!
Tips
Tagged as #chemtips, #mathtips, #phytips, #Cstips and #engtips - these tags are specifically for tips that I’d found useful sharing in that particular subject! You can also use the tag #tips to find all the tips and tricks in one go!
Notes!
Tagged as #notetaking- as I have said earlier, I am planning on posting my chemistry notes by the end of December once I’ve finished typing them up and making them colourful to read. This will be free Ofc, and it does follow the ncert pattern.
Well nice meeting you, maybe drop a comment so that I can know you too?
( note: the template for this introduction was heavily inspired by another blogger! pxasee , do check her account out too!!)
I'm hoping for the best to happen and also working for it!! <3 Show some support please for this account and have a great day/ night!!
#cbse#cbse education#cbse board#cbse students#cbse school#ncert#cbse syllabus#study aesthetic#physics#study blog#study#studying#daily study#studyblr#langauges#gettoknowme#introductions
13 notes
·
View notes